Logical Operators in Python (With Examples)
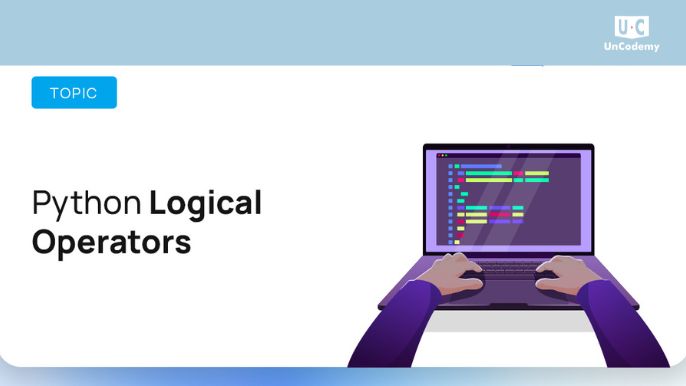
Learn how to effectively use logical operators in Python, including and, or, and not. Explore their functions, operator precedence, and practical, Pythonic applications through detailed examples.
Python logical operators are key for making decisions in your code. These operators check conditions and return either True or False based on the logic you set. The three main logical operators in Python are and, or, and not. They help you build more complex conditions to control how your program runs. For example, and checks if both conditions are true, or checks if at least one condition is true, and not reverses the result of a condition. By combining these operators, you can create flexible and powerful decision-making structures in your code.
What are Logical Operators in Python?
In Python, logical operators are used to work with True or False values (Boolean values) to make decisions in your code. These operators help evaluate conditions and control the flow of your program. The main places where you’ll use logical operators include:
- Conditional statements (like if, elif, else) to check whether certain conditions are true or false.
- Loops (while and for) to control how long the loop runs based on conditions.
- Boolean algebra to combine or reverse conditions.
- Complex conditions to create more detailed rules for decision-making.
Logical operators in Python include and, or, and not, and they follow specific rules to avoid unnecessary calculations. For example, in an and operation, if the first condition is False, Python won’t check the second condition (this is called short-circuiting). This makes the program more efficient.
Types of Logical Operators in Python
Python provides three main logical operators: and, or, and not. These operators are used to combine or reverse conditions and control the flow of your program.
- The and Operator
The and operator checks if both conditions are True. It returns True only if both conditions are true, otherwise, it returns False. Here’s how it works:
- True and True → True
- True and False → False
- False and True → False
- False and False → False
Example:
age = 25
income = 50000
if age > 18 and income > 30000:
print(“Eligible for loan”)
In this example, the program checks if age is greater than 18 and income is greater than 30,000. Since both conditions are true, it prints “Eligible for loan.”
- The or Operator
The or operator checks if at least one of the conditions is true. It returns True if any of the conditions are true, and False only if both are false. Here’s how it works:
- True or True → True
- True or False → True
- False or True → True
- False or False → False
Example:
age = 17
income = 35000
if age > 18 or income > 30000:
print(“Eligible for partial loan”)
In this case, even though age is not greater than 18, the income is more than 30,000. So, the program prints “Eligible for partial loan.”
- The not Operator
The not operator reverses the Boolean value of a condition. It changes True to False and False to True. Here’s how it works:
- not True → False
- not False → True
Example:
is_student = False
if not is_student:
print(“Eligible for membership discount”)
Here, is_student is False, so not is_student becomes True. The program then prints “Eligible for membership discount.”
Difference between the Logical Operators in Python:
Operator | Description | Example Expression | Result | Explanation |
and | Logical AND | True and True | True | Returns True only if both expressions are True. |
and | Logical AND | True and False | False | If any expression is False, the result is False. |
or | Logical OR | True or False | True | Returns True if at least one expression is True. |
or | Logical OR | False or False | False | Returns False only if both expressions are False. |
not | Logical NOT (negation) | not True | False | Inverts the Boolean value; True becomes False, and False becomes True. |
not | Logical NOT (negation) | not False | True | Reverses the truth value; False becomes True. |
Logical Operators in Conditional Statements
Logical operators are often used in conditional statements to make decisions and control the flow of the program.
Example of Combining Logical Operators
You can combine logical operators to check multiple conditions at once:
age = 20
is_employed = True
has_bad_credit = False
if age > 18 and is_employed and not has_bad_credit:
print(“Loan approved”)
Explanation: In this example, the program checks three conditions:
- The person must be over 18 years old.
- The person must be employed.
- The person must not have bad credit.
If all three conditions are true, it will print “Loan approved.” If the person has bad credit (has_bad_credit is True), the condition not has_bad_credit will become false, and the program won’t print “Loan approved.”
Order of Evaluation (Precedence) in Logical Operators
Python follows a specific order when evaluating logical operators:
- not has the highest precedence.
- and comes next.
- or has the lowest precedence.
This order ensures that Python evaluates expressions in a predictable way. For example:
result = True or False and not False
print(result) # Outputs: True
Explanation:
- First, not False is evaluated, which becomes True.
- Next, False and True is evaluated, which becomes False.
- Finally, True or False is evaluated, which results in True.
You can change the order of evaluation by using parentheses:
result = (True or False) and not False
print(result) # Outputs: True
Short-Circuit Evaluation in Logical Operators
Python uses short-circuit evaluation to make programs run more efficiently. This means that Python stops checking further conditions as soon as the result is determined.
- For and: If the first condition is False, Python won’t check the rest because the result will always be False.
- For or: If the first condition is True, Python doesn’t need to check the rest because the result will always be True.
Example of Short-Circuiting:
def func1():
print(“Function 1 executed”)
return True
def func2():
print(“Function 2 executed”)
return False
print(func1() or func2()) # Only “Function 1 executed” is printed
Explanation:
- The first function func1() returns True.
- Because of short-circuiting in the or operator, Python doesn’t need to check func2()—it skips it and only prints “Function 1 executed.”
Logical Operators in Python with Examples
Example 1: User Login Validation
username = “admin”
password = “12345”
if username == “admin” and password == “12345”:
print(“Login successful”)
else:
print(“Invalid credentials”)
In this example, the and operator checks if both the username is “admin” and the password is “12345”. If both conditions are true, the login is successful. Otherwise, it shows an “Invalid credentials” message.
Example 2: Eligibility Check for Discount
age = 16
is_student = True
if age < 18 or is_student:
print(“Eligible for discount”)
Here, the or operator checks if the user is either under 18 or a student. If either condition is true, the user is eligible for a discount.
Example 3: Negating a Condition
is_registered = False
if not is_registered:
print(“User needs to register”)
In this example, the not operator is used to check if the user is not registered. If is_registered is False, it prompts the user to register.
Common Pitfalls in Using Logical Operators in Python
- Ignoring Operator Precedence: Not understanding the order in which logical operators are evaluated can lead to unexpected results. To avoid this, use parentheses to clearly define the order of operations.
- Misusing Short-Circuiting: Relying on all conditions to be evaluated when using and or or can sometimes lead to code segments not being executed. Remember that Python stops evaluating once the result is determined due to short-circuiting.
- Overcomplicating Conditions: Having too many logical conditions in one statement can make your code harder to read and understand. It’s better to break complex conditions into smaller parts or use functions to improve clarity and readability.
Logical Operators in Pythonic Code
Logical operators are essential for writing efficient, concise, and Pythonic code. By using them effectively, Python developers can create clean, expressive code for tasks like filtering data, validating inputs, and managing exceptions.
Example of Pythonic Use of Logical Operators
# Checking if both variables have values
value1, value2 = 10, 20
if value1 and value2:
print(“Both values are non-zero”)
This code takes advantage of Python’s “truthy” values, where non-zero numbers are treated as True. This simplifies the condition, making it more concise and readable compared to checking explicitly for value1 != 0 and value2 != 0.
Conclusion
Logical operators in Python—and, or, and not—are crucial for controlling the flow of execution in programs. These operators enable developers to build effective conditional logic, manage loop behavior, and create more expressive, readable code. By mastering logical operators, Python programmers can write clean, efficient, and optimized code that can handle various decision-making and logic-based scenarios. Understanding their precedence, proper use, and Pythonic applications ensures better program performance and clarity.
FAQs: –
- What are the main logical operators in Python?
The main logical operators in Python are and, or, and not. These operators are used to combine or negate conditions in order to control the flow of execution in your program. - How does the and operator work in Python?
The and operator returns True only if both conditions it connects are True. If any condition is False, the result will be False. For example, True and False evaluates to False. - What is short-circuiting in Python’s logical operators?
Short-circuiting occurs when Python stops evaluating further conditions once the result is already determined. For example, with the and operator, if the first condition is False, Python doesn’t evaluate the second condition, as the result will definitely be False. Similarly, with or, if the first condition is True, Python skips evaluating the rest. - How can parentheses help with logical operators in Python?
Parentheses can be used to clarify the order of operations in logical expressions. This is especially important when dealing with multiple logical operators, as Python has a specific precedence order (with not evaluated first, followed by and, and then or). - What are some common pitfalls when using logical operators in Python?
Common pitfalls include neglecting operator precedence, which can lead to unexpected results, and misusing short-circuiting, which might cause some conditions to go unexecuted. Additionally, writing complex logical conditions can reduce code readability, so it’s often better to break them into smaller parts or use functions.