Floyd’s Algorithm: Learning Path, Key Steps, and Applications in Machine Learning
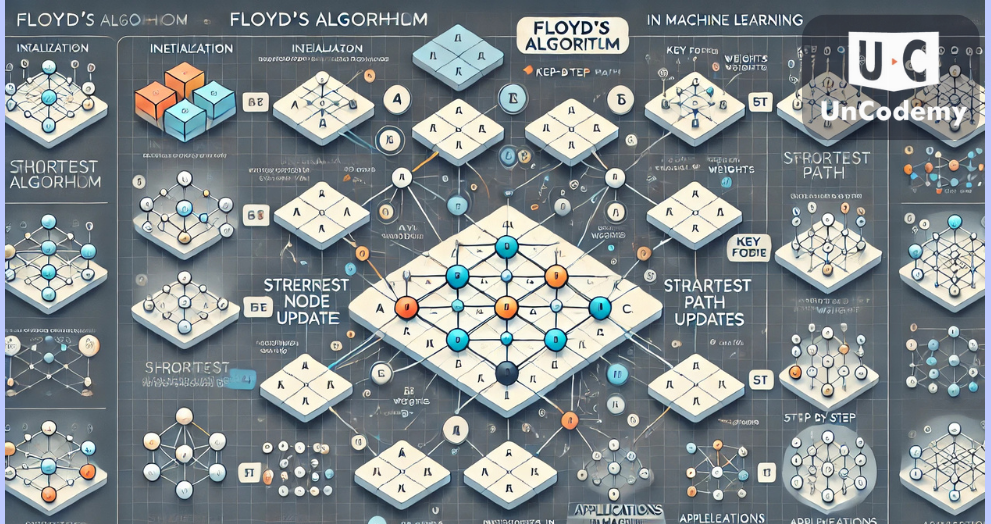
Ever wondered how algorithms find the shortest paths in complex networks? That’s where Floyd’s Algorithm comes in. It’s a key tool for data science and machine learning, driving projects like internet routing, social network analysis, and product recommendations.
Floyd’s Algorithm tackles the all-pairs shortest path problem, efficiently finding the shortest routes between every pair of nodes in a weighted graph. It works by iteratively updating path lengths, making it ideal for optimizing routing, analyzing connectivity, and solving logistics challenges.
This guide will simplify Floyd’s Algorithm, explain its principles, compare it to Dijkstra’s Algorithm, and highlight real-world examples of its use.
What is Floyd’s Algorithm?
Floyd’s Algorithm, also known as the Floyd-Warshall Algorithm, is a graph analysis method for finding the shortest paths between all pairs of nodes in a weighted graph. It works by iteratively updating path lengths to calculate the shortest possible distances, making it a reliable solution for the all-pairs shortest path problem.
Key Difference from Dijkstra’s Algorithm
While Dijkstra’s Algorithm finds the shortest path from one node to all others, Floyd’s Algorithm calculates the shortest paths between every pair of nodes. It is particularly effective for dense graphs or when multiple shortest paths are needed.
Core Representations in Floyd’s Algorithm
- Graph (G): A set of nodes (V) connected by edges (E), with each edge having a weight.
- Distance Matrix (dist[][]): A table where each cell holds the shortest distance between two nodes. Initially, it contains direct edge weights or infinity (∞) if no edge exists.
- Intermediate Node (k): A variable used to iteratively update path lengths, ranging from 1 to n (number of nodes).
Initialization Example in Floyd’s Algorithm
Before starting, initialize the distance matrix as follows:
for each node i in V: for each node j in V: if i == j: dist[i][j] = 0 elif (i, j) is an edge in E: dist[i][j] = weight(i, j) else: dist[i][j] = ∞
Floyd’s Algorithm: Step-by-Step Implementation and Optimization
Floyd’s Algorithm uses dynamic programming to break down the problem into smaller subproblems and solve them iteratively. The key is to update a 2D array, dist[][], which tracks the shortest path between all pairs of nodes while considering each node as an intermediate point.
1) Pseudo-code for Floyd’s Algorithm
for each intermediate node k in V: for each node i in V: for each node j in V: if dist[i][j] > dist[i][k] + dist[k][j]: dist[i][j] = dist[i][k] + dist[k][j]
- Step-by-Step Breakdown:
Floyd’s Algorithm works by iterating through each node and updating the shortest distance between every pair of nodes. The main formula used for updating the distance matrix is:
Distance[i][j]=min(Distance[i][j],Distance[i][k]+Distance[k][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][k] + \text{Distance}[k][j])Distance[i][j]=min(Distance[i][j],Distance[i][k]+Distance[k][j])
This formula checks if a shorter path between nodes i and j can be found through an intermediate node k.
Example:
Let’s walk through the example with nodes A, B, C, D, and E.
Using Node A as Intermediate:
-
- Update the distance matrix with the formula:
Distance[i][j]=min(Distance[i][j],Distance[i][A]+Distance[A][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][A] + \text{Distance}[A][j])Distance[i][j]=min(Distance[i][j],Distance[i][A]+Distance[A][j])
Using Node B as Intermediate:
-
- Similarly, update with:
Distance[i][j]=min(Distance[i][j],Distance[i][B]+Distance[B][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][B] + \text{Distance}[B][j])Distance[i][j]=min(Distance[i][j],Distance[i][B]+Distance[B][j])
Using Node C as Intermediate:
-
- Again, apply the formula:
Distance[i][j]=min(Distance[i][j],Distance[i][C]+Distance[C][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][C] + \text{Distance}[C][j])Distance[i][j]=min(Distance[i][j],Distance[i][C]+Distance[C][j])
Using Node D as an Intermediate:
-
- Update for all node pairs:
Distance[i][j]=min(Distance[i][j],Distance[i][D]+Distance[D][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][D] + \text{Distance}[D][j])Distance[i][j]=min(Distance[i][j],Distance[i][D]+Distance[D][j])
Using Node E as an Intermediate:
-
- Finally, use node E to complete the distance matrix updates:
Distance[i][j]=min(Distance[i][j],Distance[i][E]+Distance[E][j])\text{Distance}[i][j] = \min(\text{Distance}[i][j], \text{Distance}[i][E] + \text{Distance}[E][j])Distance[i][j]=min(Distance[i][j],Distance[i][E]+Distance[E][j])
Result Extraction:
After completing all iterations, the dist[][] matrix will contain the shortest distance between every pair of nodes. Each entry dist[i][j] represents the shortest path from node i to node j.
2) Time and Space Complexity of Floyd’s Algorithm
Floyd’s Algorithm, as discussed, operates with n nodes, and its complexity is:
- Time complexity: O(n³)
- Space complexity: O(n²)
Although the time complexity is cubic, it can be improved using more efficient data structures like heaps, reducing the time complexity to O(n² log n). While this is still less efficient than Dijkstra’s algorithm for sparse graphs, it performs well for dense graphs or scenarios needing multiple shortest-path calculations.
3) Optimizations for Floyd’s Algorithm
Several optimizations can enhance Floyd’s Algorithm’s efficiency and reduce both time and space complexity:
Path Reconstruction
Path reconstruction allows us to not only find the shortest distances but also trace the actual paths. This is done using an auxiliary matrix path[][], which tracks intermediate nodes on the shortest paths.
To implement path reconstruction:
- Initialize path[i][j]:
- Set path[i][j] = i if there’s a direct edge from i to j.
- Set path[i][j] = -1 if no direct edge exists.
- Update path[i][j] whenever the dist[i][j] matrix changes.
for each intermediate node k in V: for each node i in V: for each node j in V: if dist[i][j] > dist[i][k] + dist[k][j]: dist[i][j] = dist[i][k] + dist[k][j] path[i][j] = path[k][j]
After completing the algorithm, reconstruct the shortest path using the path matrix.
Space Optimizations
We can reuse the input matrix for storing distances to reduce memory usage instead of using an extra matrix. This in-place update reduces memory overhead:
for each intermediate node k in V: for each node i in V: for each node j in V: dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
Parallelization
Floyd-Warshall’s nested loops make it a good candidate for parallel processing. By using multi-threading or GPU computing, we can parallelize the inner loops to take advantage of modern processors and speed up the algorithm.
for each intermediate node k in V: # Parallelize the following loops for each node i in V in parallel: for each node j in V in parallel: if dist[i][j] > dist[i][k] + dist[k][j]: dist[i][j] = dist[i][k] + dist[k][j]
These optimizations significantly improve the algorithm’s performance, especially for large datasets.
4) Alternative Algorithms for Specific Graph Types
For sparse graphs, alternative algorithms might be more efficient:
- Dijkstra’s Algorithm: Better for single-source shortest paths. It has a time complexity of O((V + E) log V) with a binary heap and works faster on sparse graphs.
- Bellman-Ford Algorithm: Can handle negative edge weights but has a higher time complexity of O(VE), making it slower for large graphs.
Floyd-Warshall is best for dense graphs or when multiple shortest-path calculations are needed. On the other hand, Dijkstra and Bellman-Ford are more efficient for sparse graphs or single-source shortest-path problems. Choosing the right algorithm depends on the graph type and the specific requirements of your problem.
Essential Skills for Effective Use of Floyd’s Algorithm
To use the Floyd-Warshall algorithm effectively, a clear understanding of key graph theory concepts is essential:
- Paths: A path is a sequence of vertices connected by edges. Key types of paths to know include:
- Simple Paths: Paths that do not repeat vertices.
- Shortest Paths: Paths that minimize the total edge weight.
- Directed Paths: Paths that follow the direction of edges.
- Undirected Paths: Paths where edges can be traversed in both directions.
- Connectedness: A graph is connected if there is a path between any two nodes.
- Cycles: A cycle is a path that starts and ends at the same vertex. Recognizing cycles, especially negative-weight cycles, is critical in shortest-path calculations to avoid infinite loops.
- Weighted Edges: Weighted edges assign costs to traversing between vertices. Understanding how edge weights impact pathfinding is crucial.
Key Concepts in Dynamic Programming (DP)
Floyd-Warshall relies on Dynamic Programming (DP) principles to solve graph problems efficiently. These include:
- Optimal Substructure: The optimal solution of a problem can be broken down into optimal solutions of subproblems.
- Overlapping Subproblems: Repeatedly solving the same subproblems can be avoided by storing and reusing previous results.
- Memoization and Tabulation: Techniques for storing results to improve efficiency.
DP Techniques for Efficiency
- Memoization (Top-Down Approach):
- The problem is broken into smaller subproblems, and their results are stored to avoid redundant calculations.
- Space Complexity: Requires additional memory for storing results.
- Efficiency: Reduces time complexity by reusing stored results.
Example: Calculating Fibonacci numbers using memoization prevents redundant calculations, reducing time complexity.
- Tabulation (Bottom-Up Approach):
- Subproblems are solved iteratively and stored in a table.
- Space Complexity: Like memoization, it requires memory to store solutions.
- Efficiency: Tabulation can be more space-efficient than memoization in some cases.
Example: Computing Fibonacci numbers using tabulation builds up the solution step-by-step and stores each result.
How to Master a Programming Language for Implementing Algorithms
To effectively implement the Floyd-Warshall algorithm or any graph theory solution, mastering a programming language is essential. Here’s how you can achieve proficiency:
- Understand Syntax and Semantics: Start by learning the basic structure of the language, such as variables, loops, conditionals, functions, and classes. This will allow you to write clean, functional code.
- Master Core Data Structures: Get comfortable with fundamental data structures like arrays, lists, dictionaries, and sets. These will help you represent graphs, store intermediate values, and perform quick lookups.
- Learn Relevant Libraries: Familiarize yourself with libraries and frameworks that simplify algorithm implementation. For example, Python’s NetworkX and C++’s Boost Graph Library are great tools for graph manipulation.
- Optimize Performance: Understand how time and space complexities affect your code. Learn how to optimize loops, use efficient data structures, and leverage built-in functions to improve performance.
- Manage Memory: If you’re using languages like C or C++, learn to manage memory allocation and deallocation to avoid memory issues. Even in languages with automatic garbage collection, understanding memory usage can still be helpful.
- Improve Debugging and Testing Skills: Learn debugging tools and techniques to ensure your code works efficiently. Write tests and use debugging tools like IDE debuggers or logging to verify your implementation.
- Develop Algorithmic Thinking: Sharpen your ability to break down problems and choose the best algorithm. Understanding the complexity of different approaches is key to solving problems efficiently.
- Write Clear and Maintainable Code: Focus on writing readable code by using meaningful names, writing comments, and following coding conventions. This makes it easier to maintain and collaborate on projects.
To truly master a language, continuous practice is key. Engage with coding challenges, online communities, and open-source projects to gain hands-on experience and expand your problem-solving skills.
Learning Path and Resources for Mastering Floyd’s Algorithm
To learn and implement Floyd’s Algorithm effectively, follow this structured roadmap:
Prerequisites
- Graph Theory Basics: Understand the fundamental concepts of graphs, such as vertices, edges, and the difference between directed and undirected graphs. Know how to represent graphs using adjacency matrices, as Floyd’s Algorithm uses them for graph representation.
- Algorithms and Data Structures: Familiarize yourself with key concepts like recursion, iteration, dynamic programming, and matrix operations. Understanding basic data structures such as arrays and matrices will be crucial for storing graph representations and analyzing algorithm performance.
- Programming Skills: Be proficient in a programming language like Python, focusing on loops, conditionals, functions, and classes. Knowing libraries such as NumPy for matrix operations or NetworkX for graph manipulation can simplify the implementation process. Debugging and testing skills are also essential to handle edge cases.
Recommended Books & Tutorials
- Graph Theory:
- Introduction to Graph Theory by Douglas West
- Graph Theory by Reinhard Diestel
- Shortest Path Algorithms:
- Algorithms by Robert Sedgewick and Kevin Wayne
- The Shortest-Path Problem – Analysis and Comparison of Methods by Hector Ortega-Arranz
- Floyd-Warshall Algorithm & Dynamic Programming:
- Introduction to Algorithms by Cormen, Leiserson, Rivest, and Stein (Chapter on Shortest Paths)
Learning Platforms
Online Learning Platforms
- Uncodemy: Uncodemy’s machine learning course covers everything from foundational algorithms to advanced techniques.
- LeetCode and HackerRank: Practice implementing Floyd’s Algorithm with coding challenges.
- VisuAlgo and Graph Online: Use interactive tools to visualize graph algorithms in action.
Use Cases of Floyd’s Algorithm in Machine Learning
Floyd’s Algorithm is widely used in various ML tasks. Here are some key examples:
- Network Analysis In large-scale networks, nodes represent routers, and edges represent communication links, with weights indicating metrics like latency or bandwidth.
- Network Optimization: Floyd’s algorithm helps compute the shortest paths between routers, optimizing routing tables to reduce latency and improve data throughput.
- Traffic Management: It allows network administrators to adjust routes dynamically in response to network conditions like congestion or outages.
- Recommendation Systems In recommendation systems, both users and items (like products or movies) are represented as nodes, with edges indicating interactions (e.g., purchases or ratings).
- User Similarity: Floyd’s algorithm helps identify how closely related users are by computing the shortest paths between them based on their interactions with items.
- Item Recommendations: By analyzing user similarity, the algorithm helps recommend items that are popular among similar users.
- Social Network Analysis In social networks, nodes represent users, and edges represent interactions or friendships, with edge weights showing the strength or frequency of interactions.
- Influence and Reach: Floyd’s algorithm helps identify influential users by finding the shortest paths between users and calculating centrality measures.
- Information Spread: It helps determine the best paths for spreading information, aiding strategies for viral marketing or awareness campaigns.
- Bioinformatics In bioinformatics, protein-protein interaction (PPI) networks are represented as graphs, with nodes as proteins and edges as interactions.
- Pathway Discovery: Floyd’s algorithm helps identify efficient communication pathways between proteins, which is useful for understanding biological processes like signal transduction.
- Disease Mechanisms: It can uncover disrupted pathways in diseased cells by analyzing changes in shortest paths in PPI networks.
Conclusion
Floyd’s Algorithm is a dynamic programming technique that efficiently computes the shortest paths between all pairs of vertices in a weighted graph, handling both positive and negative edge weights. Its versatility makes it ideal for applications in transportation logistics, social media analytics, and circuit design.
With a time, complexity of O(n^3), Floyd’s Algorithm excels in network routing and resource allocation. However, it may not be the best choice for very large or sparse graphs, where algorithms like Dijkstra’s or Bellman-Ford might offer better performance.
Ongoing research is exploring hybrid methods to improve its efficiency for various graph structures. As machine learning continues to evolve, the focus will shift toward developing more scalable and robust algorithms for handling large and complex graphs.
Mastering Floyd’s Algorithm equips you with valuable problem-solving skills for complex graph-related tasks, enhancing your ability to select the right algorithm for the problem at hand.
FAQs
1. What is Floyd’s Algorithm and what problem does it solve?
Floyd’s Algorithm is a dynamic programming technique that calculates the shortest paths between all pairs of vertices in a weighted graph. It works with both positive and negative edge weights, making it useful in various applications like network routing, transportation logistics, and social media analytics.
2. What is the time complexity of Floyd’s Algorithm?
The time complexity of Floyd’s Algorithm is O(n^3), where n is the number of vertices in the graph. This makes it suitable for smaller or denser graphs, but less efficient for very large or sparse graphs.
3. How does Floyd’s Algorithm compare to other shortest-path algorithms like Dijkstra’s and Bellman-Ford?
Floyd’s Algorithm is ideal for computing shortest paths between all pairs of vertices, whereas Dijkstra’s and Bellman-Ford’s algorithms are more efficient for single-source shortest-path problems. For large or sparse graphs, Dijkstra’s or Bellman-Ford might offer better performance due to their lower time complexities.
4. In which real-world applications is Floyd’s Algorithm used?
Floyd’s Algorithm is widely used in network routing, recommendation systems, social network analysis, and bioinformatics. It helps optimize routes in networks, recommend related items, analyze social influence, and discover efficient pathways in biological networks.