All About Python 3.13.0
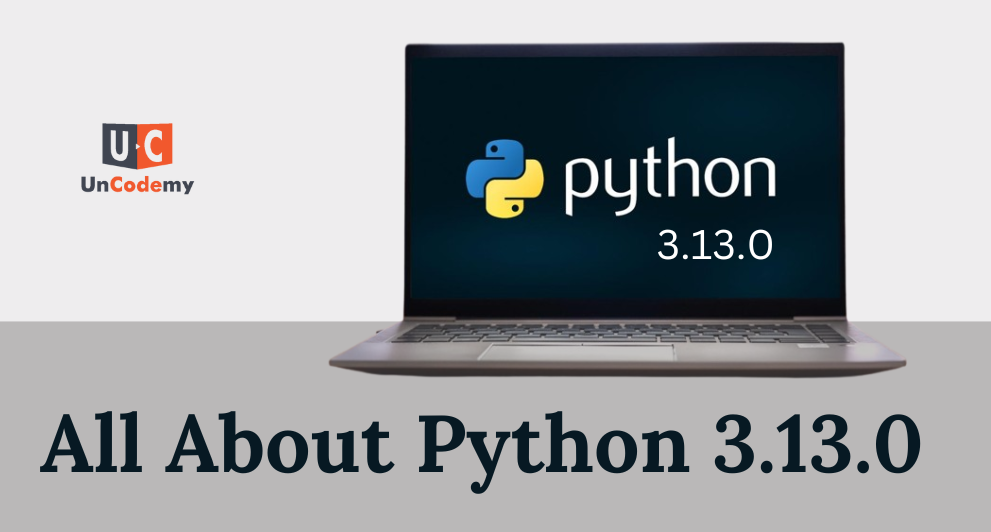
Python 3.13.0, released on October 7, 2024, brings a host of new features and improvements that make coding more efficient and enjoyable. Let’s dive into the key enhancements that this version offers.
Enhanced Interactive Interpreter
The interactive interpreter, also known as the Read-Eval-Print Loop (REPL), has received a significant upgrade. Inspired by PyPy’s features, it now supports multi-line editing and colorized output, making coding more intuitive and visually appealing.
Example:
python CopyEdit def greet(name): return f"Hello, {name}!" print(greet("World")) Output: CopyEdit Hello, World!
With the new REPL, writing, and testing functions like greet becomes a walk in the park.
Experimental Free-Threaded Build Mode
One of the most anticipated features is the experimental support for running Python in a free-threaded mode, achieved by disabling the Global Interpreter Lock (GIL). This allows multiple threads to execute simultaneously, enhancing performance in multi-threaded applications.
Example:
python CopyEdit import threading def print_numbers(): for i in range(5): print(i) threads = []for _ in range(5): thread = threading.Thread(target=print_numbers) threads.append(thread) thread.start() for thread in threads: thread.join()
Output:
python CopyEdit 0 1 2 3 4 0 1 2 3 4...
In this example, multiple threads print numbers concurrently, showcasing the potential of the free-threaded mode.
Preliminary Just-In-Time (JIT) Compiler
Python 3.13.0 introduces an experimental Just-In-Time (JIT) compiler, aiming to boost execution speed by compiling parts of the code during runtime. While still in its early stages, this feature lays the groundwork for significant performance enhancements in future releases.
Illustration:
Imagine your Python code as a recipe. Traditionally, Python interprets the recipe step by step each time you run it. With JIT compilation, it’s like having a chef memorize the recipe, allowing them to prepare the dish more quickly in subsequent attempts.
Improved locals() Function
The behavior of the locals() function has been refined to provide well-defined semantics when modifying the returned mapping. This ensures more predictable behavior, especially useful for debugging purposes.
Example:
python CopyEdit def example_function(): x = 10 y = 20 local_vars = locals() local_vars['x'] += 5Â # Modify local variable return x, y print(example_function())
Output:
scss CopyEdit (10, 20)
In this case, modifying local_vars[‘x’] does not change the actual local variable x, highlighting the importance of understanding locals() behavior.
New Memory Management Features
Python 3.13.0 includes an updated version of the mimalloc memory allocator, which is now optional but enabled by default if supported by the platform. This allocator helps reduce memory usage, particularly for applications that utilize extensive docstrings.
Example:
python CopyEdit def large_docstring_function(): """ This is a function with a large docstring that is intended to demonstrate how leading indentation is stripped to save memory. """ pass
Efficient memory handling contributes to better performance and lower memory consumption in applications.
Updated dbm Module
The dbm module now defaults to using the dbm.sqlite3 backend when creating new database files, enhancing its functionality and reliability.
Example:
python CopyEdit import dbm with dbm.open('example.db', 'c') as db: db['key'] = 'value' print(db['key'])Â # Output: b'value'
This change simplifies the use of the dbm module by leveraging SQLiteâs robust features.
Changes to macOS Support
The minimum supported version of macOS has been updated from 10.9 to 10.13 (High Sierra), meaning that older macOS versions will no longer be supported.
This change allows developers to focus on modern macOS features and optimizations.
Typing Enhancements
Python 3.13.0 introduces several typing enhancements, including improved type inference and new syntax options, making it easier to write and maintain type-safe code.
Example:
python CopyEdit def greet(name: str) -> str: return f"Hello, {name}!"
These enhancements help identify potential errors early in the development process, which is particularly useful when learning Python programming and writing clean, error-free code.
Deprecations and Removals
As with any major release, certain outdated features have been deprecated or removed to streamline the language and encourage best practices. It’s advisable to review the official documentation to identify any changes that might affect your existing code.
Illustration:
Think of this as cleaning out your closetâremoving old, unused items to make space for new, more useful ones.
Conclusion
Python 3.13.0 is a significant step forward, introducing features that enhance performance, usability, and code clarity. As the saying goes, “The proof of the pudding is in the eating,” so don’t hesitate to explore these new features and see how they can benefit your coding projects.