Virtual Function In Java | Run-Time Polymorphism In Java
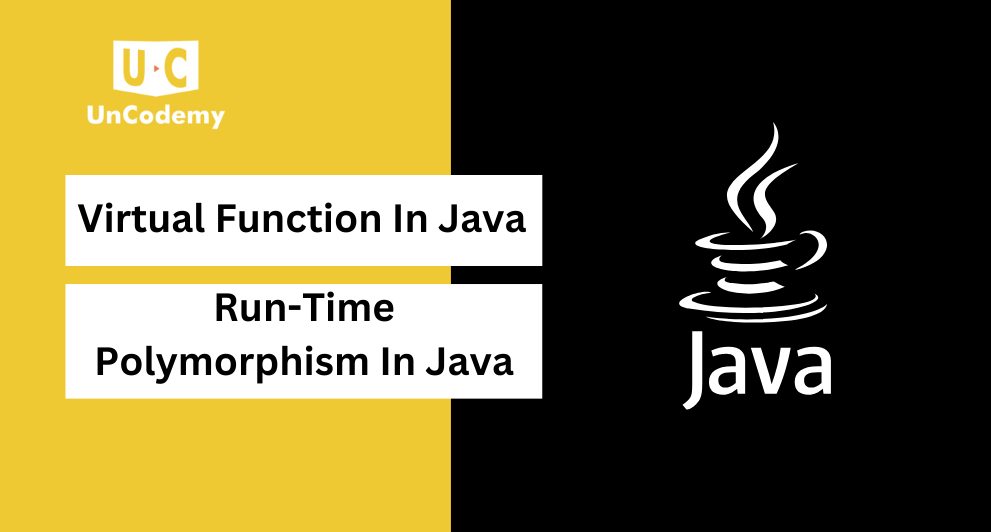
Virtual functions, also known as run-time polymorphism, are a key feature of Java that allow for code reuse and extensibility. In essence, a virtual function is a method that can be overridden in a derived class, allowing different implementations of the same method in different classes. This enables code reuse and makes it easier to extend and modify existing code. Additionally, it allows for dynamic dispatching, where the appropriate implementation of a method is chosen at run-time based on the type of object that the method is being called on. Virtual functions are a powerful tool for creating flexible and extensible code in Java.
HERE ARE SOME POTENTIAL USES OF VIRTUAL FUNCTION:
- Virtual functions are marked with the “virtual” keyword in the base class.
- A virtual function can be overridden in a derived class by simply defining a new method with the same name and argument list.
- When a virtual function is called on an object, the actual function that is executed is determined by the object’s type at run-time.
- Virtual functions are particularly useful for inheritance hierarchies, where a base class defines a set of virtual functions that can be overridden by derived classes to provide different behavior.
- Virtual functions can also be abstract, meaning that they don’t have an implementation in the base class and must be implemented in the derived classes.
- Virtual functions are not thread-safe by default. If multiple threads are accessing the same object and calling a virtual function, it’s important to ensure that the function is thread-safe to prevent race conditions.
- Virtual functions in Java, can be declared as final, which prevents it from being overridden in a derived class.
- Virtual functions play an important role in the Object-Oriented Programming (OOP) paradigm, which emphasizes the concept of abstraction, encapsulation, inheritance, and polymorphism.
SOME ADVANCED USES OF VIRTUAL FUNCTION:
- Virtual functions in Java, are a key feature of interfaces. An interface is a contract that defines a set of methods that a class must implement. When a class implements an interface, it is agreeing to provide an implementation for all of the methods defined in the interface. These methods are implicitly virtual, allowing them to be overridden by derived classes.
- Virtual functions can have different access modifiers, such as public, private, and protected. The access modifier determines the visibility of the function to other classes.
- Java uses a “dynamic dispatch” mechanism to determine which implementation of a virtual function to call at run-time. This involves examining the object’s type at run-time and selecting the appropriate implementation based on the object’s class hierarchy.
- Overriding a virtual function in a derived class allows you to customize the behavior of the function without modifying the base class. This makes code more flexible and extensible.
- Virtual functions can be used to implement design patterns such as the Strategy pattern, where different algorithms can be implemented as different classes and selected at run-time based on the requirements.
- Virtual functions can be overloaded, meaning that multiple functions with the same name can exist in the same class, as long as they have different parameter lists.
- In Java, virtual functions are automatically inherited by derived classes, unless they are explicitly marked as “final” or “private”.
RUNTIME POLYMORPHISM IN JAVA
Runtime polymorphism, also known as dynamic polymorphism, is a key concept in Java that allows different implementations of a behavior to be selected at runtime based on the object’s type. It is achieved through the use of virtual functions and interfaces. When a virtual function is called on an object, the Java Virtual Machine (JVM) dynamically determines which implementation of the function to call based on the type of the object. This allows for greater flexibility and extensibility in Java code.
Runtime polymorphism also facilitates code reuse, making it easier to maintain and update programs over time. It is a fundamental concept in object-oriented programming and an essential tool for developing robust and scalable Java applications.
- Runtime polymorphism is achieved through method overriding, which allows a subclass to provide a specific implementation of a method that is already defined in the superclass.
- Runtime polymorphism enables late binding, which means that the actual function that is called is determined at runtime based on the type of the object.
- Runtime polymorphism is an essential feature of inheritance, allowing subclasses to extend and modify the behavior of their superclass.
- Runtime polymorphism is also known as “dynamic method dispatch” or “virtual method invocation” in Java.
SOME ADVANCED CONCEPTS AND USES
- In Java, runtime polymorphism can also be achieved using interfaces, which define a set of behaviors that a class must implement. When an interface is implemented by a class, the class provides its own implementation of the interface’s methods. This allows multiple classes to share a common interface, even if they have different implementations.
- Runtime polymorphism can improve the efficiency of a Java program by reducing the amount of code that needs to be written and executed. By using polymorphism, common functionality can be shared among different classes, reducing the need for duplicated code.
- Runtime polymorphism can also be used to implement design patterns such as the Decorator pattern, which allows additional functionality to be added to an object without modifying the original class.
- Runtime polymorphism can also be used to implement dynamic class loading, which allows classes to be loaded at runtime, rather than during compilation. This allows for greater flexibility and adaptability in Java applications.
- Runtime polymorphism can be used to implement pluggable architectures, which allow new functionality to be added to an application without requiring a complete re-compilation of the code. This can be particularly useful in situations where the application needs to be easily extended or updated.
- Runtime polymorphism can also be used to implement runtime type checking, which allows the type of an object to be checked at runtime, rather than at compile time. This can be useful for ensuring type safety in Java applications.
- Runtime polymorphism can also be used to implement dynamic proxy objects, which allow for the transparent implementation of interfaces. This can be useful for implementing features like debugging or logging, without affecting the core functionality of an application.
- Runtime polymorphism can also be used in combination with reflection, which allows Java code to inspect and modify itself at runtime. This can be useful for creating highly dynamic and adaptable applications.
- Runtime polymorphism can also be used in combination with aspect-oriented programming, which allows for the modularization of cross-cutting concerns in an application, such as logging, security, and transaction management.
- Runtime polymorphism can impact performance, as the dynamic dispatching of method calls can introduce some overhead. In some cases, it may be more efficient to use compile-time polymorphism, which can be achieved through the use of generic types and generics in Java.
- Runtime polymorphism can also be abused, leading to fragile and hard-to-maintain code. To avoid this, it’s important to use runtime polymorphism judiciously, and to carefully design the interfaces and hierarchies used in an application.
- Finally, runtime polymorphism can make code harder to understand and debug, particularly if the interfaces and hierarchies used are complex or poorly designed.
VIRTUAL KEYWORD IN JAVA
The virtual keyword in Java is a modifier that can be applied to a method or class, and it indicates that the method or class can be overridden by a subclass. Essentially, it means that a subclass can provide its own implementation of a method that was originally defined in the superclass. This allows for subclasses to specialize or extend the behavior of the superclass, while still maintaining compatibility with the original implementation.
For example, consider a superclass Animal, with a virtual method called speak(). A subclass Dog might override this method to provide a bark() implementation, while a subclass Cat might provide a meow() implementation. Both subclasses would still be considered Animals, and would be compatible with code that was written for the superclass, but would provide more specialized behavior.
- A Java virtual method can only be overridden in a subclass if it is declared as virtual in the superclass. If it is declared as final, it cannot be overridden.
- Java virtual method can be polymorphic, which means that the actual method that is executed depends on the type of the object that is being referenced. This is known as “dynamic method dispatch” or “late binding.”
- Virtual methods are often used in conjunction with inheritance, as they allow subclasses to extend or override the behavior of their superclasses in a controlled and structured way.
- Overriding a virtual method can be useful for implementing the Decorator pattern, where a class can add functionality to another class without modifying the original class.
- Java virtual method can be used for implementing the Strategy pattern, where different algorithms can be implemented as classes, and the specific algorithm to use is chosen at runtime.
- Java virtual method can also be used to implement the Observer pattern, where objects can subscribe to other objects and be notified when they change state. This is a common pattern used in event-driven programming.
Conclusion:
In conclusion, virtual functions are a powerful feature of Java, and play an important role in Object-Oriented Programming (OOP). They allow for code reusability, modularity, extensibility, adaptability, and flexibility. By using virtual functions, developers can create more maintainable, reusable, and scalable code. In addition, virtual functions enable several important design patterns, such as the Template Method pattern, the Builder pattern, the Command pattern, the Chain of Responsibility pattern, the Visitor pattern, the Mediator pattern, and the Composite pattern. Overall, virtual functions are a key tool in the Java developer’s toolkit, and should be considered when designing complex and flexible systems.