Applications of Stack in Data Structure
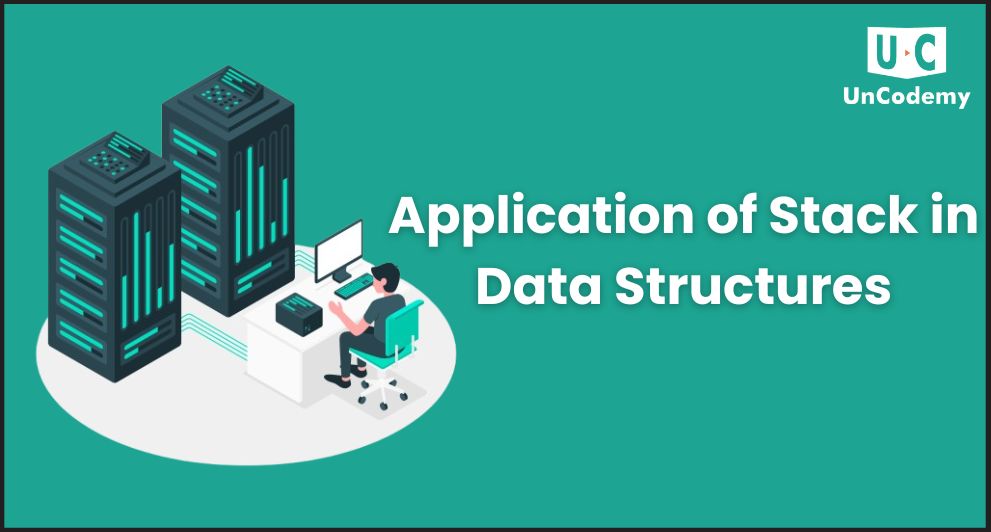
In the vast world of computer science, data structures serve as the foundation for creating efficient and scalable software applications. One such crucial data structure is the stack, which has wide-ranging applications due to its simplicity and versatility. Stacks are primarily used for storing data in a manner that follows the Last In, First Out (LIFO) principle, meaning the last element added to the stack is the first one to be removed.
This blog explores the fundamental concept of stacks, their various applications in real-world scenarios, and their relevance in problem-solving in computer science.
What is a Stack?
A stack is a linear data structure that allows operations mainly at one end, known as the top of the stack.
Below are the key functions of a stack:
1. Push
- Description: The push function adds an element to the top of the stack.
- Syntax: push(element)
Purpose: To insert an element into the stack. This operation is always performed at the top of the stack.
Example:
stack.push(10) # Adds 10 to the stack stack.push(20) # Adds 20 to the stack
- Time Complexity: The time complexity of push is O(1), meaning it takes constant time to add an element.
2. Pop
- Description: The pop function removes and returns the element from the top of the stack.
- Syntax: pop()
Purpose: This operation removes the most recently added element from the stack and returns it. After this operation, the next element in line becomes the top of the stack.
Example:
top = stack.pop() # Removes the top element and assigns it to 'top' print(top) # Output: 20, since 20 was the most recent element added
- Time Complexity: The time complexity of pop is O(1) because removing an element from the top of the stack happens in constant time.
3. Peek (Top)
- Description: The peek function returns the top element of the stack without removing it.
- Syntax: peek()
Purpose: To view the element at the top of the stack, without modifying the stack. This is useful when you want to examine the current top element without popping it.
Example:
top = stack.peek() # Returns the top element without removing it print(top) # Output: 10, assuming 10 is the top element
- Time Complexity: The time complexity of peek is O(1), as no elements are modified and the top element is simply returned.
4. IsEmpty
- Description: The isEmpty function checks whether the stack is empty or not.
- Syntax: isEmpty()
Purpose: This function helps determine if there are any elements left in the stack. It returns a boolean value:
-
- True if the stack is empty.
- False if the stack contains one or more elements.
Example:
if stack.isEmpty(): print("Stack is empty.") else: print("Stack is not empty.")
- Time Complexity: The time complexity of isEmpty is O(1), as it only checks whether the stack has any elements.
5. Size
- Description: The size function returns the number of elements currently present in the stack.
- Syntax: size()
Purpose: To get the total number of elements in the stack. This is useful when you need to know the stack’s current size.
Example:
if stack.isEmpty(): print("Stack is empty.") else: print("Stack is not empty.")
- Time Complexity: The time complexity of size is O(1), as it only requires the stack to keep track of its size.
6. Clear (Optional)
- Description: Some stack implementations may offer a clear function, which removes all elements from the stack.
- Syntax: clear()
Purpose: To reset the stack to its empty state. This function is not always available in every stack implementation, but it can be useful in cases where you need to completely remove all elements at once.
Example:
print(stack.size()) # Output: 2, assuming there are two elements in the stack
- Time Complexity: The time complexity of clear is O(n), where n is the number of elements in the stack, as all elements must be removed.
Applications of Stacks in Data Structures
1. Expression Evaluation and Conversion
Stacks are extensively used in evaluating mathematical expressions and converting expressions from one notation to another. In particular, they play a significant role in:
- Infix to Postfix Conversion: Infix notation (e.g., A + B) is common in mathematics, but computers cannot directly evaluate such expressions. To evaluate infix expressions, we first convert them into postfix (or Reverse Polish) notation, where the operator follows the operands. A stack can be used to help with this conversion by maintaining operators and parentheses in proper order.
- Postfix Evaluation: Once an expression is in postfix form (e.g., AB+), it can be easily evaluated using a stack. Each operand is pushed onto the stack, and when an operator is encountered, the operands are popped from the stack, the operation is performed, and the result is pushed back onto the stack. This process continues until the entire expression is evaluated.
Example: Infix to Postfix Conversion
Consider the infix expression: A + B * C
- Convert to postfix: A B C * +
- Using the stack to evaluate the postfix expression:
- Push A
- Push B
- Push C
- Encounter *: Pop C and B, compute B * C, and push the result.
- Encounter +: Pop the results of A and B * C, compute A + (B * C), and push the result.
2. Undo Mechanism in Software Applications
Stacks are the backbone of implementing the undo and redo features in many software applications like word processors, graphic design tools, and code editors. When a user makes changes to a document, those changes can be stored on a stack:
- Undo: Each change is pushed onto a stack as an operation. When the user presses “undo,” the application pops the most recent operation from the stack and reverses it.
- Redo: After an undo operation, the user might want to redo the action. This can be implemented by maintaining a second stack for redo operations. When an action is undone, it is pushed onto the redo stack, and when a redo is invoked, the action is popped from the redo stack and reapplied.
This stack-based model allows users to traverse through a history of operations, efficiently managing the complexity of undoing and redoing multiple operations.
3. Depth-First Search (DFS) in Graphs
The stack plays a central role in performing Depth-First Search (DFS) in graph traversal. In DFS, the algorithm starts at a root node and explores as far as possible along each branch before backtracking.
- Recursive DFS: Although recursion uses the system’s call stack internally, DFS can also be implemented explicitly with a stack. Nodes are pushed onto the stack as they are discovered, and when the algorithm reaches a node with no further unexplored neighbors, it pops the stack to backtrack.
This use of a stack ensures that the algorithm efficiently explores all paths in a graph, making DFS suitable for applications such as solving puzzles (like mazes), analyzing networks, and checking the connectivity of components in a graph.
4. Balanced Parentheses Checking
Stacks are invaluable in validating whether a given sequence of parentheses (or brackets) is balanced. This is a common problem in compilers and interpreters, which require the validation of syntactic correctness in mathematical expressions, source code, or markup languages.
In this case, each opening parenthesis is pushed onto the stack. When a closing parenthesis is encountered, the algorithm checks if the top of the stack contains the corresponding opening parenthesis. If so, the parenthesis is balanced, and the algorithm continues. If the stack is empty or there’s a mismatch between the parentheses, the expression is deemed unbalanced.
Example: Checking Balanced Parentheses
Expression: { [ ( ) ] }
- Start with an empty stack.
- Push {, then [, then ( onto the stack.
- Encounter ): Pop the top of the stack ((), matching parentheses.
- Encounter ]: Pop the top of the stack ([), matching parentheses.
- Encounter }: Pop the top of the stack ({), matching parentheses.
- The stack is empty, indicating the expression is balanced.
5. Recursion Implementation
Many recursive algorithms can be transformed into an iterative form using a stack. Recursion, by nature, uses the call stack to store function calls, and this can be emulated explicitly using a stack to manage the function calls and their respective states.
For example, a simple recursive algorithm to calculate the factorial of a number:
def factorial(n): if n == 1: return 1 else: return n * factorial(n-1)
This can be rewritten using a stack:
def factorial(n): stack = [] result = 1 while n > 0: stack.append(n) n -= 1 while stack: result *= stack.pop() return result
By managing the recursive function calls with an explicit stack, we can avoid issues like stack overflow in languages that have limited recursion depth.
Also Read: Understanding Recursion in Data Structures: Types & Examples
6. Memory Management in Function Calls
In the context of low-level programming languages (like C or C++), stacks are used for managing function calls and local variables. The call stack keeps track of function calls, arguments, and local variables, ensuring that after a function finishes, the control returns to the correct location in the program. Each time a function is called, a new “stack frame” is created and pushed onto the stack, and when the function finishes executing, the stack frame is popped.
This memory management technique is essential for maintaining the correct program state and handling nested or recursive function calls efficiently.
7. Backtracking Algorithms
Backtracking is a problem-solving technique used to find all possible solutions to a problem by exploring all possibilities one by one. When a potential solution path is found to be unviable, the algorithm backtracks by undoing the most recent choices, typically by popping elements from a stack.
Common problems where backtracking and stacks are used include:
- Solving mazes: The stack stores the current path, and when a dead-end is encountered, the algorithm backtracks by popping the stack and exploring alternate paths.
- N-Queens problem: The stack can be used to keep track of the positions of queens on the chessboard while the algorithm attempts to place queens in a way that no two queens threaten each other.
Stacks provide an efficient and organized way to explore and backtrack through potential solutions in problems involving constraint satisfaction or combinatorics.
Conclusion
The stack is a simple yet powerful data structure with a wide variety of applications in computer science. From evaluating mathematical expressions to managing function calls, enabling undo features, or even performing complex graph algorithms, the stack proves its importance in solving numerous computational problems. Its Last In, First Out (LIFO) nature ensures that elements are processed in a controlled order, which is crucial for a variety of tasks.
Understanding the applications of stacks not only strengthens your problem-solving skills but also provides insight into how data can be managed and manipulated efficiently in software systems. Whether you’re a beginner or an experienced developer, mastering the stack and recognizing its applications will help you write more efficient, reliable, and scalable code.
FREQUENTLY ASKED QUESTION [FAQs]
1. What is a stack in data structures?
- A stack is a linear data structure that follows the Last In, First Out (LIFO) principle, meaning the last element added to the stack is the first one to be removed. The main operations are push (to add an element), pop (to remove the top element), and peek (to view the top element without removing it).
2. What are the common applications of stacks?
- Stacks have a wide range of applications in various domains. Common uses include:
- Expression evaluation (infix, postfix, and prefix expressions)
- Undo operations in software applications (word processors, graphic design tools)
- Depth-first search (DFS) in graph traversal
3. How is a stack used in expression evaluation?
- In expression evaluation, stacks help convert and evaluate expressions. For example:
- Infix to Postfix Conversion: Stacks help rearrange an infix expression (A + B) to postfix (AB+), which can be more easily evaluated by a computer.
- Postfix Evaluation: After converting an expression to postfix notation (e.g., AB+), stacks are used to evaluate it by pushing operands and applying operators in the correct order.
4. What is the role of a stack in the undo functionality of applications?
- The stack is used to keep track of previous states or actions in applications that support undo and redo functionality. Every action (e.g., typing a letter or moving an object) is pushed onto the stack. When the user presses “undo,” the most recent action is popped from the stack, and the system reverts to the previous state.
5. How does a stack help with depth-first search (DFS) in graphs?
- In Depth-First Search (DFS), a stack is used to explore nodes in a graph. Starting from a source node, the algorithm pushes nodes onto the stack and explores as far as possible along each branch before backtracking by popping nodes off the stack. This LIFO approach ensures the algorithm explores deep paths before backtracking to explore others.
6. Can you explain how stacks are used in recursive function calls?
- In recursion, the system’s call stack automatically stores the state of each function call, including local variables and the function’s execution context. Each recursive call pushes a new stack frame, and when the base case is reached, the stack frames are popped off, returning control to the previous call. Stacks allow recursion to function efficiently without overwriting previous calls.
7. How does a stack check for balanced parentheses in an expression?
- A stack can be used to check if parentheses (or other symbols like curly braces or square brackets) in an expression are balanced. For every opening parenthesis, it is pushed onto the stack. For each closing parenthesis, the stack is popped, and the corresponding opening parenthesis is checked. If the stack is empty at the end, the expression is balanced.
8. What is the time complexity of the basic stack operations?
- The time complexity of basic stack operations is as follows:
- Push: O(1), adding an element to the stack happens in constant time.
- Pop: O(1), removing an element from the stack also takes constant time.
- Peek: O(1), viewing the top element takes constant time.
- IsEmpty: O(1), checking whether the stack is empty is a constant time operation.
- Size: O(1), returning the number of elements in the stack takes constant time.
9. How do stacks help in solving backtracking problems?
- In backtracking algorithms (e.g., solving a maze or the N-Queens problem), a stack is used to store the current state of the solution. If a path or solution does not work (e.g., reaching a dead-end in a maze), the algorithm backtracks by popping the stack to explore alternative paths. This LIFO order allows the algorithm to efficiently explore all possible solutions.
10. What are the advantages of using a stack in algorithm design?
- Some advantages of using a stack in algorithms include:
- Simplicity: The LIFO principle of the stack makes it straightforward to implement and reason about.
- Efficiency: Many algorithms, such as DFS, expression evaluation, and backtracking, can be efficiently implemented using stacks.
- Memory management: Stacks are useful for managing function calls and handling recursion, ensuring proper execution flow and memory usage.