Lifecycle and States of a Thread in Java
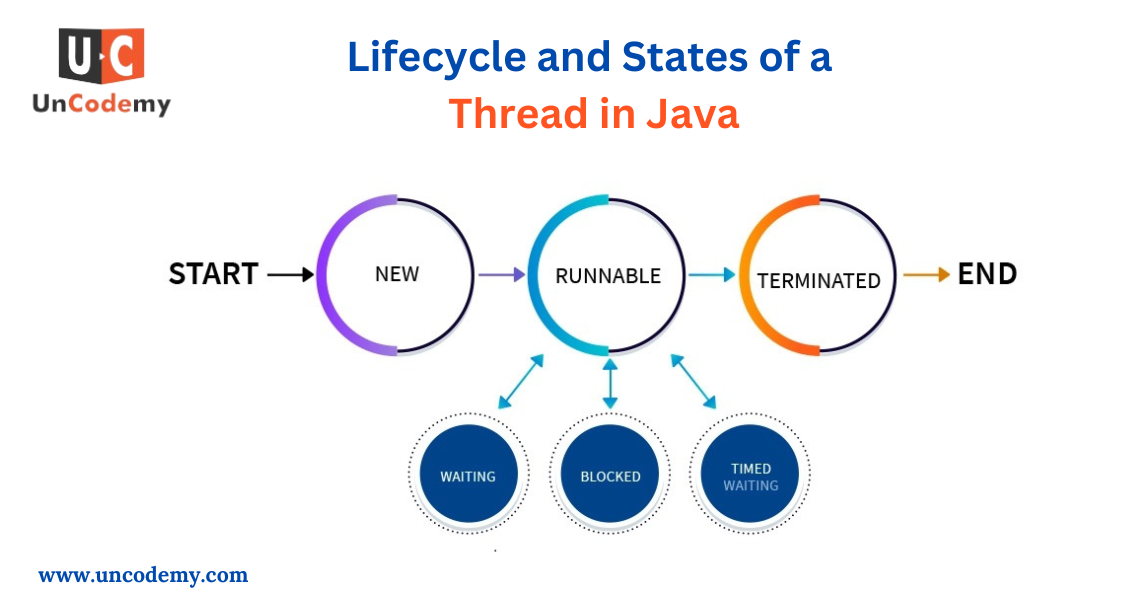
Thread is a lightweight process that can execute in parallel with other threads within a program. Threads allow a program to perform multiple tasks concurrently, improving performance and reducing latency. Understanding the lifecycle and states of a thread in Java is essential for developers to write efficient and robust multithreaded programs. This article will explain the thread lifecycle in Java and the various states a thread can be in.
Lifecycle of Thread in Java
The life cycle of a thread in Java refers to the various states that a thread can be in throughout its execution. The Java Thread class provides a set of methods that allow developers to manage the lifecycle of a thread. The lifecycle of a thread in Java is as follows:
- New State
- Runnable State
- Running State
- Blocked State
- Waiting State
- Timed Waiting State
- Terminated State
Let’s look at each state in detail.
New State
When a new instance of the Thread class is created, the thread is in the New state. In this state, the thread has not yet started to execute. The thread remains in this state until the start() method of the thread is called.
Runnable State
When the start() method is called, the thread transitions to the Runnable state. The thread is ready to run in this state but may not be executed immediately. Thread scheduler decides when to schedule the thread for execution. The thread remains in the Runnable state until it is selected by the thread scheduler to execute.
Want to become a Java Developer? Sign up for this Java Course in Noida
Running State
When the thread scheduler selects the thread to execute, the thread transitions to the Running state. In this state, the thread is executing its code. The thread remains Running until one of the following happens:
- The thread completes its execution.
- The thread is blocked for some reason, such as waiting for I/O or a synchronization lock.
- The thread yields the CPU voluntarily by calling the yield() method.
Blocked State
When a thread is blocked, it transitions to the Blocked state. A thread can be blocked for various reasons, such as waiting for I/O, a lock, or sleeping. In this state, the thread is not executing and is not eligible for execution until the condition that caused it to block is resolved. The thread transitions back to the Runnable state when the condition is resolved.
Waiting State
When a thread is waiting, it transitions to the Waiting state. A thread can enter the Waiting state by calling the wait() method on an object. In this state, the thread is not executing and is not eligible for execution until another thread notifies it by calling the notify() or notifyAll() method on the same object. When the thread is notified, it transitions back to the Runnable state.
Timed Waiting State
When a thread is timed waiting, it transitions to the Timed Waiting state. A thread can enter the Timed Waiting state by calling the sleep() method or the wait() method with a timeout value. In this state, the thread is not executing and is not eligible for execution until the timeout period has elapsed or another thread notifies it by calling the notify() or notifyAll() method on the same object. When the timeout period elapses or the thread is notified, it transitions back to the Runnable state.
Terminated State
When a thread completes its execution or is terminated, it transitions to the Terminated state. The thread is no longer executing in this state, and the system can reclaim its resources.
Become a master of Java Full Stack by signing up for this Full Stack Training Course in Noida
Explaining the States of Thread in Java
Now that we have seen the various states of a thread in Java let’s understand each state in detail.
New State
New state. In this state, the thread has not yet started to execute. The thread is created using the Thread constructor, which can take a Runnable object or a ThreadGroup object as a parameter. When the thread is created, it is assigned a name and a priority. The name and priority can be set using the setName() and setPriority() methods of the Thread class.
Declaration: public static final Thread.State NEW
Runnable State
When the start() method is called, the thread transitions to the Runnable state. The thread is ready to run in this state but may not be executed immediately. Thread scheduler decides when to schedule the thread for execution. The thread remains in the Runnable state until it is selected by the thread scheduler to execute. In a multithreaded program, multiple threads in the Runnable state can occur at any given time.
Declaration: public static final Thread.State RUNNABLE
Running State
When the thread scheduler selects the thread to execute, the thread transitions to the Running state. In this state, the thread is executing its code. The thread remains Running until one of the following happens:
- The thread completes its execution.
- The thread is blocked for some reason, such as waiting for I/O or a synchronization lock.
- The thread yields the CPU voluntarily by calling the yield() method.
Blocked State
When a thread is blocked, it transitions to the Blocked state. A thread can be blocked for various reasons, such as waiting for I/O, a lock, or sleeping. In this state, the thread is not executing and is not eligible for execution until the condition that caused it to block is resolved. The thread transitions back to the Runnable state when the condition is resolved. In a multithreaded program, multiple threads can be in the Blocked state at any time.
Declaration: public static final Thread.State BLOCKED
Waiting State
When a thread is waiting, it transitions to the Waiting state. A thread can enter the Waiting state by calling the wait() method on an object. In this state, the thread is not executing and is not eligible for execution until another thread notifies it by calling the notify() or notifyAll() method on the same object. When the thread is notified, it transitions back to the Runnable state. In a multithreaded program, multiple threads can be in the Waiting state at any time.
Declaration: public static final Thread.State WAITING
Timed Waiting State
When a thread is timed waiting, it transitions to the Timed Waiting state. A thread can enter the Timed Waiting state by calling the sleep() method or the wait() method with a timeout value. In this state, the thread is not executing and is not eligible for execution until the timeout period has elapsed or another thread notifies it by calling the notify() or notifyAll() method on the same object. When the timeout period elapses, or the thread is notified, it transitions back to the Runnable state. In a multithreaded program, multiple threads can be in the Timed Waiting state at any time.
Declaration: public static final Thread.State TIMED_WAITING
Terminated State
When a thread completes its execution or is terminated, it transitions to the Terminated state. In this state, the thread is no longer executing, and its resources can be reclaimed by the system. Once a thread is in the Terminated state, it cannot be restarted. In a multithreaded program, multiple threads can be in the Terminated state at any time.
Declaration: public static final Thread.State TERMINATED
Conclusion
In this article, we have explained the lifecycle and states of a thread in Java. Understanding the thread lifecycle and states is important for writing efficient and robust multithreaded programs. By knowing the various states that a thread can be in, developers can write code that takes advantage of the concurrency features provided by Java. It is important to note that writing multithreaded programs can be challenging and error-prone. Developers should be careful when writing multithreaded code and use synchronization mechanisms, such as locks and semaphores, to ensure that threads do not interfere.
In summary, the thread lifecycle in Java consists of six states: New, Runnable, Running, Blocked, Waiting, Timed Waiting, and Terminated. Each state represents a different stage in the life of a thread, and a thread can transition between states based on its execution and synchronization needs. Understanding the thread lifecycle and states is crucial for developing efficient and reliable multithreaded programs in Java.