Mastering Python: A Journey to Become a Pro
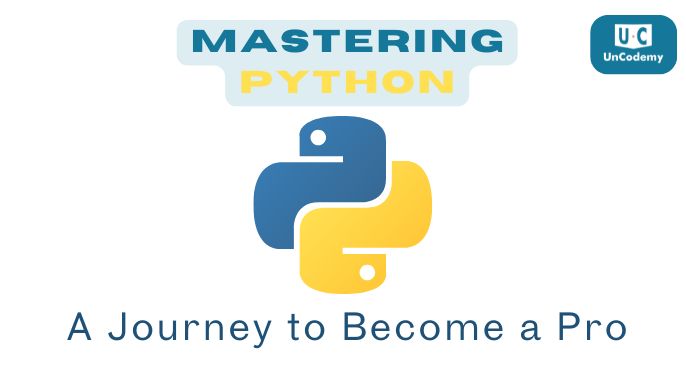
Build a Strong Foundation with Python Basics
Before diving into advanced Python concepts, it’s crucial to have a strong foundation in the basics. Here are a few key areas to focus on:
- Syntax and Structure: Understanding Python’s simple and readable syntax is the first step. Learn how to work with variables, data types (strings, integers, lists, tuples, and dictionaries), operators, loops (for, while), and conditionals (if-else). This gives you the building blocks for writing functional programs.
- Functions: Functions are the heart of any programming language. Learn how to define functions, pass parameters, and return values. Mastering functions will help you write more modular and reusable code.
- Error Handling: Python is known for its user-friendly error messages, which makes it easier to troubleshoot and debug. Learn how to use try, except, and finally to handle exceptions gracefully.
- Data Structures: Mastering Python’s built-in data structures like lists, sets, dictionaries, and tuples will significantly improve your ability to organize and manipulate data.
Dive Into Object-Oriented Programming (OOP) in Python
Once you’re comfortable with the basics, it’s time to dive into Object-Oriented Programming (OOP), which is a powerful paradigm used extensively in Python. Here’s what you need to focus on:
- Classes and Objects: Understand the core concepts of classes, objects, and methods. A class is a blueprint for creating objects (instances), and methods define the behaviors of these objects.
- Inheritance: Learn how inheritance allows a new class to acquire the attributes and methods of an existing class. This is a great way to reuse and extend code.
- Polymorphism: This concept allows objects to be treated as instances of their parent class, enabling you to use a single interface for different data types.
- Encapsulation: Encapsulation involves bundling the data (attributes) and methods that operate on the data into a single unit or class, and restricting direct access to some of the class’s components.
Work with Libraries and Frameworks
One of Python’s greatest strengths is its vast ecosystem of libraries and frameworks. Mastering these tools will help you accomplish complex tasks with ease.
- NumPy & Pandas: These libraries are essential for data manipulation and analysis. NumPy is great for numerical computations, while Pandas is widely used for handling structured data, like CSV files, Excel sheets, and databases.
- Matplotlib & Seaborn: If you’re working with data visualization, these libraries will help you create insightful and compelling charts, graphs, and plots.
- Flask & Django: If you’re looking to build web applications, Flask and Django are two of the most popular Python frameworks. Flask is lightweight and flexible, while Django is a full-stack framework designed for rapid development.
- TensorFlow & PyTorch: For machine learning and deep learning projects, TensorFlow and PyTorch are widely used libraries. They provide powerful tools for building neural networks, working with data, and training models.
- Requests & BeautifulSoup: For web scraping and interacting with web APIs, the Requests library simplifies HTTP requests, while BeautifulSoup is ideal for parsing and extracting data from HTML documents.
The Importance of Practice: Sharpen Your Python Skills
No matter how much you read or watch tutorials, there’s no substitute for hands-on practice. Apply what you’ve learned by working on real-world projects or participating in coding challenges.
- Projects: Choose projects that align with your interests. For example, build a web scraper, a to-do list application, or a machine learning model for sentiment analysis. Working on projects gives you the chance to apply concepts in a practical, problem-solving environment.
- Coding Challenges: Platforms like LeetCode, HackerRank, and Codewars offer coding problems that can help you refine your problem-solving skills. These challenges will teach you how to approach complex algorithms and optimize your code.
- Contribute to Open Source: Once you’re comfortable, try contributing to open-source Python projects. This gives you a chance to collaborate with others, learn best practices, and build your portfolio.
Build Real-World Projects
The best way to master Python is by applying what you’ve learned to real-world projects. Projects give you the opportunity to solve problems, experiment with different Python features, and improve your skills in a practical context.
- Web Scraper: Build a tool that extracts data from websites and stores it in a structured format.
- To-Do List Application: Create a simple to-do list app with features like adding, editing, and deleting tasks.
- Personal Blog: Use Flask or Django to build a basic personal blog with user authentication and content management.
- Weather Application: Build an app that fetches weather data from an API and displays it to the user.
- Data Dashboard: Create an interactive dashboard that visualizes real-time data with charts and graphs.
Participate in Coding Challenges
Participating in coding challenges is an excellent way to refine your problem-solving skills and improve your Python abilities. Platforms like:
- LeetCode
- HackerRank
- Codewars
offer coding problems that range from beginner to advanced levels. These platforms will help you think algorithmically and get accustomed to solving problems under time constraints.
Learn Advanced Concepts
As you become more comfortable with Python, you can start diving into more advanced topics:
- Decorators: Decorators are a powerful way to modify the behavior of functions or methods without changing their source code. Learning how to use and write decorators is a crucial skill for Python developers.
- Generators and Iterators: Generators allow you to create iterators in a more efficient way. Understanding how to create and use generators will help you write more memory-efficient code.
- Concurrency and Parallelism: As Python is widely used for web development, data analysis, and machine learning, it’s important to understand how to handle multiple tasks simultaneously using threading, multiprocessing, and asynchronous programming.
- Design Patterns: Learn common design patterns (e.g., Singleton, Factory, Observer) to write more structured, efficient, and maintainable code.
Stay Up-to-Date with Python’s Ecosystem
Python is an evolving language, with regular updates and new features being added. To stay at the forefront, it’s important to:
- Follow the Python Enhancement Proposals (PEPs): PEPs are formal proposals for changes and improvements to Python. Keeping an eye on new PEPs can help you stay informed about upcoming features and best practices.
- Engage with the Community: The Python community is vast and active. Participate in Python meetups, forums (like Stack Overflow), or contribute to discussions on GitHub. Engaging with others will expose you to different perspectives and coding styles.
- Read Blogs and Tutorials: There are many great Python resources online. Subscribe to Python blogs, follow developers on social media, and keep reading tutorials to stay up-to-date with the latest trends and tools.
Harness the Power of Python with the Right Training
To truly master Python, it’s essential to have access to comprehensive learning resources that guide you from basics to advanced concepts. Whether you’re starting from scratch or refining your skills, a structured Python training course can provide
âś… Conclusion
Mastering Python is a continuous journey. By laying a strong foundation, embracing advanced concepts, practicing consistently, and staying connected with the Python community, you’ll gain the skills necessary to become a Python expert. Whether you’re building a career in data science, web development, automation, or artificial intelligence, Python will be a key tool in your arsenal.