Understanding Recursion in Data Structures: Types & Examples
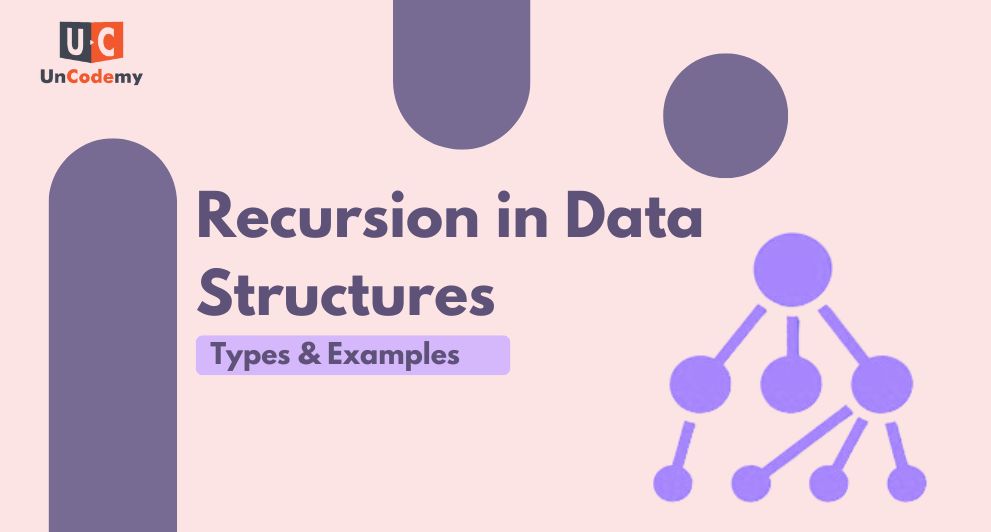
What is Recursion in Data Structure?
Recursion is a programming technique where a function calls itself to solve a problem. In data structures, recursion is often used to handle complex problems by breaking them down into smaller, easier-to-solve parts.
The idea behind recursion is based on the “divide and conquer” approach. It works by dividing a problem into smaller versions of itself and solving each one step by step. Eventually, the process reaches a base case—a point where the solution is simple and doesn’t need further breaking down. Each recursive call tackles a smaller piece of the problem until the base case is reached, and then the results are combined to solve the original problem.
How Does Recursion Work?
Recursion works using two key parts: the base case and the recursive case.
The base case is the stopping point for the recursion. It’s a simple condition that tells the function when to stop calling itself and provides a solution to the smallest version of the problem.
The recursive case is where the problem is broken into smaller parts, and the function calls itself with a smaller or simpler input.
When the function hits the recursive case, it calls itself with a smaller version of the original problem. Each of these calls creates a new instance of the function, which continues to solve smaller pieces of the problem. This process repeats until the base case is reached. At that point, the function stops calling itself and starts returning values or performing actions. These results are then combined as the function “unwinds” to solve the original problem.
To create effective recursive algorithms, it’s crucial to:
Clearly define the base case to prevent infinite loops.
Ensure that each recursive call gets closer to the base case to avoid errors like stack overflow or excessive memory use.
Recursion is especially useful in data structures and algorithms, such as:
Tree traversals (e.g., in-order, pre-order, post-order).
Sorting algorithms (like quicksort and merge sort).
Graph traversal (e.g., depth-first search).
Classic problems like the Towers of Hanoi, the Fibonacci sequence, and many others.
Five Main Recursion Methods in Data Structure
Recursion comes in different forms, each with its unique characteristics and use cases. Let’s explore five main types of recursions in an easy-to-understand way.
- Tail Recursion
In tail recursion, the recursive call happens as the last step in the function. This means there’s no extra work left after the call, which allows some programming languages to optimize the function and use less memory.
Example (Python):
def factorial(n, result=1): if n == 0: return result return factorial(n - 1, result * n)
Advantages:
- Saves memory by avoiding extra stack frames.
- Prevents stack overflow for large inputs.
- Some languages optimize tail-recursive functions for better performance.
Considerations:
- Only works if the recursive call is the last step.
- Not all programming languages support tail call optimization.
- Binary Recursion
Binary recursion splits the problem into two smaller subproblems, solves them separately, and combines their results. It’s often used in tasks involving binary trees or problems with a natural two-part structure.
Example (Java): public int sumBinaryTree(Node node) { if (node == null) { return 0; } return node.value + sumBinaryTree(node.left) + sumBinaryTree(node.right); }
Use Cases:
- Traversing or modifying binary trees.
- Solving problems like finding tree height or calculating the sum of all nodes.
- Sorting algorithms like quicksort and binary search.
Considerations:
- The problem must naturally divide into two subproblems.
- Combining results should lead to the solution of the original problem.
- Linear Recursion
Linear recursion solves problems by reducing them to a single smaller subproblem. Each step simplifies the problem until it hits the base case.
Example (C++):
int fibonacci(int n) { if (n <= 1) { return n; } return fibonacci(n - 1) + fibonacci(n - 2); }
Key Points:
- Simple and easy to understand.
- Commonly used for tasks like computing Fibonacci numbers or factorials.
- May lead to higher time complexity if not optimized.
- Mutual Recursion
Mutual recursion happens when two or more functions call each other in a cycle to solve a problem.
Example (Python):
def isPalindrome(s): if len(s) <= 1: return True if s[0] == s[-1]: return isPalindrome(s[1:-1]) return False def checkPalindrome(s): return isPalindrome(s)
Benefits:
- Breaks problems into modular pieces handled by different functions.
- Encourages code reuse and separation of responsibilities.
Challenges:
- Requires careful design to avoid infinite recursion.
- Debugging can be tricky due to the interdependence of functions.
- Nested Recursion
In nested recursion, a function calls itself with another recursive call as an argument.
Example (Python):
def ackermann(m, n): if m == 0: return n + 1 if m > 0 and n == 0: return ackermann(m - 1, 1) return ackermann(m - 1, ackermann(m, n - 1))
Advantages:
- Useful for solving problems with complex dependencies.
- Can handle intricate patterns that other recursion methods can’t.
Considerations:
- Often has high time complexity, especially for large inputs.
- Needs careful planning to avoid infinite loops and ensure termination.
Types of Recursions:
Recursion in data structures can be categorized based on how a function calls itself. The two main types are direct recursion and indirect recursion: –
Direct Recursion
Definition and Explanation:
Direct recursion happens when a function calls itself directly during its execution. It breaks the problem into smaller subproblems, solves one of them, and then calls itself with the reduced version of the problem. The process continues until it reaches a base case that stops the recursion. In simple terms, the function refers to itself within its body to solve the task step by step.
Example (Python):
Here’s an example of calculating the factorial of a number using direct recursion: def factorial(n): if n == 0: # Base case return 1 return n * factorial(n - 1) # Recursive call In this code, the factorial() function keeps calling itself with n - 1 until it reaches n = 0. It then works backward, multiplying all the values to compute the factorial.
Pros of Direct Recursion:
Simple to Write: Direct recursion provides an easy and natural way to solve problems with repetitive subproblems.
Readable Code: Recursive functions are often more concise and easier to understand for problems like factorials or Fibonacci numbers.
Cons of Direct Recursion:
High Memory Usage: Each recursive call uses memory to store a stack frame. With too many calls, this can lead to a stack overflow.
Slower Performance: Recursive calls add overhead due to function calls, which can make them slower compared to loops.
No Tail Optimization: If the recursive call is not the last step in the function, it can’t benefit from tail recursion optimization. This optimization reduces memory usage by removing the need for multiple stack frames.
Indirect Recursion
Definition and Explanation:
Indirect recursion occurs when a function doesn’t call itself directly but instead calls another function, which eventually calls the original function, forming a cycle. This type of recursion involves a chain of function calls where the dependency loops back to the starting function. The recursion continues until a base case is reached, which stops the cycle.
Example (C++):
Here’s an example to show how indirect recursion works: #include <iostream> using namespace std; void function1(int n); void function2(int n) { if (n > 0) { cout << n << " "; function1(n - 1); // Calls function1 } } void function1(int n) { if (n > 1) { cout << n << " "; function2(n / 2); // Calls function2 } } int main() { function1(20); return 0; } In this example, function1() calls function2(), and function2() calls function1(). Together, they create a cycle of indirect recursion. The base case ensures the recursion eventually stops.
Pros of Indirect Recursion:
- Breaks Down Complex Problems: Indirect recursion is helpful for solving problems that can be divided into smaller, interdependent tasks.
- Improved Modularity: By spreading the logic across multiple functions, the code becomes more organized and easier to manage.
Cons of Indirect Recursion:
- Added Complexity: The dependency between multiple functions can make the logic harder to follow and debug.
- Execution Challenges: Ensuring the correct order of function calls and proper base cases is crucial to avoid infinite loops or errors.
- Performance Issues: Like direct recursion, indirect recursion can use a lot of memory and processing time due to repeated function calls.
While direct recursion involves a function directly calling itself, indirect recursion relies on multiple functions calling each other in a loop. Both approaches are useful for specific scenarios, but indirect recursion requires careful planning to maintain clarity and efficiency. By understanding the differences and trade-offs, you can choose the right type of recursion for solving a given problem.
How to Use Recursion?
Recursion is a technique where a function calls itself to solve smaller parts of a problem. It can be implemented in various programming languages like C++, C, JavaScript, and Scala. Here’s a simple and easy explanation for using recursion in each language.
Using Recursion in C++
Recursion in C++ is all about defining a function that calls itself. The syntax is straightforward. Here’s how you can write a recursive function:
Syntax and Basic Implementation:
return_type function_name(parameters) { // Base case: defines when the recursion stops if (base_case_condition) { return base_case_value; } // Recursive case: the function calls itself return recursive_function_call(arguments); }
- Base Case: Stops the recursion when a certain condition is met.
- Recursive Case: Breaks the problem into smaller parts and solves it by calling the same function.
Best Practices:
- Define a Clear Base Case: Always ensure the function stops recursion at some point.
- Progress Toward the Base Case: Each recursive call should bring you closer to the base case.
- Be Mindful of Memory: Deep recursion can use a lot of memory and may cause stack overflow.
Common Pitfalls:
- Stack Overflow: Happens when recursion goes too deep. Always ensure your recursion stops within a reasonable depth.
- Incorrect Recursive Calls: Passing wrong arguments may cause infinite loops or wrong results.
Using Recursion in C
The concept is similar to C++, but C requires manual memory management. Here’s how to use recursion in C:
Syntax:
return_type function_name(parameters) { // Base case if (base_case_condition) { return base_case_value; } // Recursive case return recursive_function_call(arguments); }
Limitations:
- Manual Memory Management: C doesn’t automatically handle memory like C++ does, so you must manage it yourself.
- Limited Recursion Depth: C compilers often have a smaller stack size, which can lead to stack overflow for deep recursion.
Using Recursion in JavaScript
JavaScript also supports recursion and uses it for various tasks like working with trees, graphs, or sorting algorithms.
Syntax and Basic Implementation:
function function_name(parameters) { // Base case if (base_case_condition) { return base_case_value; } // Recursive case return recursive_function_call(arguments); }
Use Cases:
- Tree Traversal: Useful for navigating tree-like structures.
- Sorting and Searching: Recursive algorithms like merge sort or binary search work well here.
Considerations:
- Event Loop Blocking: Too many recursive calls can block JavaScript’s single-threaded event loop. Optimize your recursion to avoid this.
Using Recursion in Scala
Scala, being a functional programming language, has strong support for recursion.
Syntax and Basic Implementation:
def function_name(parameters): return_type = { // Base case if (base_case_condition) { base_case_value } else { // Recursive case recursive_function_call(arguments) } }
Benefits of Recursion in Scala:
- Immutable Data: Works well with recursion because it avoids side effects.
- Higher-Order Functions: Functions like map, filter, and fold make recursion simpler and more powerful.
- Tail Recursion Optimization: The Scala compiler converts tail-recursive functions into loops, preventing stack overflow.
Difference Between Recursion and Iteration:
Recursion and iteration are two different ways of solving problems, each with its own approach and set of advantages. Here’s a simple breakdown of the key differences between the two:
Recursion | Iteration |
---|---|
A function calls itself during execution. | Repeats a set of instructions in a loop. |
Breaks a problem into smaller, similar problems. | Repeats a block of code until a condition is met. |
Ideal for problems like tree traversal or factorial. | Best for repetitive tasks like searching or sorting. |
Requires a base case to stop the recursion. | Uses a loop condition to control repetition. |
Can be more concise and intuitive for some problems. | Usually simpler and easier to follow. |
May use more memory because of function calls. | Uses less memory as it doesn’t require additional calls. |
Can be less time-efficient for some tasks. | Often more efficient in terms of time. |
Can lead to deeply nested code. | Keeps the code structure simpler and flatter. |
When deciding between recursion and iteration, several factors should be considered:
Problem Complexity: Recursion works well for problems that naturally split into smaller, similar parts, like traversing a tree or solving a divide-and-conquer problem. Iteration is better for repetitive tasks, such as searching through lists or calculating numbers like factorials.
Readability and Maintainability: Recursion can be more elegant and concise, but too many recursive calls can make the code hard to read. Iteration is often easier to understand because it follows a clear sequence of steps.
Memory Use: Recursion can consume a lot of memory because each function call adds to the call stack. Too many recursive calls can even cause a stack overflow. Iteration uses less memory because it doesn’t rely on additional function calls, making it better for handling large datasets.
Use Cases
Recursion is commonly used for:
- Tree and Graph Traversal (e.g., depth-first search)
- Divide-and-Conquer Algorithms (e.g., merge sort)
- Finding Permutations and Combinations
- Expression Parsing and Evaluation
Iteration is better suited for:
- Searching Algorithms (e.g., binary search)
- Sorting Algorithms (e.g., bubble sort, insertion sort)
- Numerical Computations (e.g., factorial, Fibonacci sequence)
- Repetitive Tasks (e.g., input validation, looping through lists)
Drawbacks of Recursion in Data Structures
While recursion can be a powerful tool in data structures, it comes with some drawbacks that need to be considered:
Stack Overflow and Memory Usage: Recursion uses the function call stack, meaning each time a function calls itself, a new “frame” is added to the stack. If the recursion goes too deep, it can overwhelm the stack, leading to a stack overflow error. This is especially problematic when dealing with large input sizes or deep recursion, as it can cause the program to crash due to running out of memory.
Performance Issues: Recursion isn’t always the most time-efficient method. Each function call introduces overhead, and if the recursion involves repeated calculations, it can lead to slower execution compared to iterative solutions. In certain cases, recursion can be less efficient in terms of both time and space complexity, so it’s important to carefully analyze the problem to decide if recursion is the best choice.
Complexity Analysis and Optimization: Understanding and analyzing the time and space complexity of recursive algorithms can be tricky. Many recursive solutions have exponential time complexity, which can make them inefficient. Optimizing these algorithms often requires techniques such as memoization or tail recursion to avoid redundant calculations and reduce the number of recursive calls, thus improving performance.
Conclusion
Recursion is a powerful concept in data structures that allows programmers to solve complex problems in a simple and intuitive way. By understanding how recursion works, including its different types and how it can be implemented in various programming languages, you can improve your problem-solving and algorithm design skills. However, it’s important to keep in mind the potential drawbacks of recursion, like memory usage and performance issues, so you can make smart choices when using it in real-world applications. With the right knowledge, you can use recursion effectively to write clear, efficient, and elegant code in your data structure projects.