Introduction to Laravel
Laravel is a powerful, open-source PHP framework designed to make web development easier and more efficient. Created by Taylor Otwell, Laravel is known for its elegant syntax, robust features, and developer-friendly tools. It is primarily used for building web applications and APIs, providing a clean and structured way to develop complex applications.
History of Laravel
Laravel was first introduced in 2011 as a more refined alternative to other PHP frameworks like CodeIgniter. Over the years, it gained popularity due to its simplicity, scalability, and comprehensive documentation. Laravel introduced features such as Artisan commands, Eloquent ORM, and Blade templating that revolutionized the way PHP developers build applications.
Laravel Features
Below are the key features that make Laravel a preferred choice for developers:
Feature | Description |
---|---|
Routing | Laravel provides an easy and flexible routing system, allowing developers to define clean URLs and organize the application’s routes efficiently. |
Artisan CLI | The Artisan command-line interface allows developers to automate common tasks, such as database migrations, testing, and managing the application’s environment. |
Blade Templating Engine | Laravel includes Blade, a simple and powerful templating engine for clean and efficient front-end development. It provides control structures like loops and conditionals. |
Eloquent ORM | Laravel’s Eloquent ORM simplifies working with databases. It allows developers to interact with databases using PHP syntax, making it easier to manage and query data. |
Middleware | Laravel's middleware provides a convenient mechanism for filtering HTTP requests. Middleware can be used for tasks like authentication and logging. |
Security | Laravel has built-in features for securing applications, such as password hashing, CSRF protection, and SQL injection prevention, making it easier to build secure applications. |
Setting Up Laravel
To get started with Laravel, follow these steps to install it on your system:
- Ensure that you have PHP and Composer installed on your machine. You can download PHP from the official PHP website and Composer from the Composer website.
- Once PHP and Composer are installed, open your terminal and run the following command to create a new Laravel project:
- Change to the directory of your project and run the Laravel development server:
- Visit
http://localhost:8000
in your browser to see your new Laravel application in action.
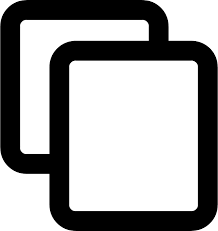
composer create-project --prefer-dist laravel/laravel your-project-name
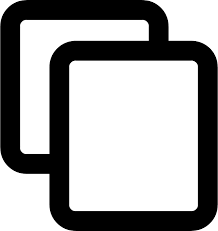
php artisan serve
Code Example: Basic Laravel Route
Here’s an example of how to define a basic route in Laravel:
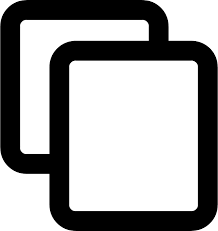
// routes/web.php
Route::get('/', function () {
return 'Hello, Laravel!';
});
Diagram: Laravel Request Lifecycle
The following diagram explains the flow of a request in a Laravel application, from the incoming HTTP request to the final response:

This diagram illustrates how Laravel handles requests, including the role of middleware, routing, controllers, and views in the request lifecycle.
Benefits of Using Laravel for Web Development
Laravel is a comprehensive PHP framework that offers a wealth of benefits for developers. Whether you're building a small application or a complex enterprise-level platform, Laravel's features can significantly speed up development and reduce the complexity of building web applications.
1. Elegant Syntax
Laravel provides an elegant and readable syntax that allows developers to write clean and maintainable code. Its syntax is designed to be simple and expressive, making it easier to understand and work with. This results in faster development cycles and reduced learning curves for new developers.
2. Powerful Eloquent ORM
Laravel’s Eloquent ORM (Object-Relational Mapping) makes database interactions simple and intuitive. It allows developers to interact with the database using PHP syntax instead of writing complex SQL queries. This reduces the need for boilerplate code and allows for more readable and efficient database management.
3. Built-in Authentication and Authorization
Laravel comes with built-in tools for authentication and authorization, making it easier to handle user registration, login, and access control. With just a few lines of code, developers can implement role-based access control, protecting sensitive data and resources from unauthorized users.
4. Artisan Command-Line Tool
Laravel includes Artisan, a powerful command-line interface that helps automate repetitive tasks like database migrations, testing, and clearing cache. Artisan provides a set of helpful commands for developers to streamline the development workflow, saving time and effort.
5. MVC Architecture
Laravel follows the Model-View-Controller (MVC) architecture, which is a widely adopted design pattern in web development. MVC helps organize code into separate sections, making it easier to maintain, test, and scale web applications. Laravel’s implementation of MVC encourages the use of best practices and simplifies the development process.
6. Blade Templating Engine
Laravel’s Blade templating engine allows developers to create dynamic and reusable views with minimal effort. Blade provides features like template inheritance, conditional statements, and loops. It also integrates seamlessly with Laravel’s routing system, making it an essential tool for front-end development.
7. Security Features
Laravel includes several built-in security features that help protect web applications from common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Laravel automatically escapes output to prevent XSS attacks, and it also offers protection against SQL injection through prepared statements in Eloquent ORM.
8. Task Scheduling and Queues
Laravel makes it easy to schedule tasks and manage background jobs. You can define scheduled tasks using Laravel’s built-in scheduler, and Laravel’s queue system allows you to defer the execution of time-consuming tasks such as sending emails or processing payments, improving the performance and user experience of your application.
9. Ecosystem and Community Support
Laravel has a rich ecosystem that includes tools and libraries for common tasks such as authentication, payments, and email services. The Laravel community is active and growing, offering a wealth of tutorials, guides, forums, and packages that can help developers solve problems quickly and efficiently.
10. Testing and Debugging
Laravel provides built-in support for testing with PHPUnit, making it easy to write unit and feature tests for your application. It also includes tools for debugging, such as the Laravel Debugbar, which helps developers monitor and troubleshoot their applications during development.
11. Scalability
Laravel is highly scalable, which means it can be used to build both small and large applications. Whether you're building a simple blog or a complex e-commerce site, Laravel’s flexible architecture allows you to scale your application as your business grows, ensuring high performance even under heavy traffic loads.
12. RESTful API Support
Laravel makes it easy to develop RESTful APIs for mobile apps or other web applications. It provides built-in support for API authentication with Passport or Sanctum, allowing for secure and efficient API development with minimal configuration.
Diagram: Laravel Benefits
The following diagram visually summarizes the benefits of using Laravel for web development:

This diagram highlights the key features and advantages that Laravel offers for developers building web applications.
Installing and Setting Up Laravel (Local and Cloud Environments)
Laravel is a powerful PHP framework that can be easily set up both locally and in cloud environments. Below are the steps to install and configure Laravel in different environments.
1. Prerequisites
Before installing Laravel, ensure that your development environment meets the following requirements:
- PHP 8.1 or higher (Laravel 9.x and above require PHP 8.1+)
- Composer (PHP dependency manager)
- Database (MySQL, PostgreSQL, SQLite, or SQL Server)
- Node.js and npm (for front-end development and asset management)
2. Installing Laravel Locally
Follow these steps to install Laravel on your local machine:
- Ensure that PHP, Composer, and other prerequisites are installed on your system. You can check this by running the following commands:
php -v
(to check PHP version)composer -v
(to check Composer version)
- Open your terminal or command prompt and run the following command to install Laravel globally using Composer:
composer global require laravel/installer
- Once the Laravel installer is set up, you can create a new Laravel project by running the following command:
laravel new project-name
- Alternatively, you can create a Laravel project by running:
composer create-project --prefer-dist laravel/laravel project-name
- Once the project is created, navigate to your project directory:
cd project-name
- Run the built-in Laravel development server:
php artisan serve
http://localhost:8000
.
3. Installing Laravel in Cloud Environments
Setting up Laravel in cloud environments like AWS, DigitalOcean, or Heroku involves similar steps but requires additional configurations for deployment. Below is an overview of how to set up Laravel on a cloud platform.
Setting Up Laravel on AWS EC2
- Create an EC2 instance with your preferred Linux distribution (Ubuntu is a common choice).
- SSH into the EC2 instance:
ssh -i your-key.pem ubuntu@your-ec2-public-ip
- Update the instance:
sudo apt update && sudo apt upgrade
- Install required software packages (PHP, Composer, Nginx, etc.):
sudo apt install php php-cli php-fpm php-mbstring php-xml php-bcmath nginx
sudo apt install composer
- Clone your Laravel project repository from GitHub or upload your project files using SFTP or FTP.
- Set up the necessary environment variables in the
.env
file (database credentials, APP_KEY, etc.). - Run migrations and seed the database:
php artisan migrate --seed
- Configure Nginx to serve your Laravel application. Add a new site configuration under
/etc/nginx/sites-available/
, then create a symlink to/etc/nginx/sites-enabled/
to enable the site. - Finally, restart Nginx and PHP-FPM services:
sudo systemctl restart nginx
sudo systemctl restart php7.x-fpm
Setting Up Laravel on Heroku
Heroku offers a simple platform for deploying Laravel applications.
- Create a Heroku account if you don’t have one and install the Heroku CLI tool on your local machine.
- Log in to Heroku:
heroku login
- Create a new Heroku app:
heroku create your-app-name
- Push your Laravel project to Heroku using Git:
git push heroku master
- Heroku automatically detects that your project is a PHP application and installs the necessary dependencies using Composer. It also provides a PostgreSQL database by default, but you can configure other databases through Heroku add-ons.
- Configure the
.env
file with the correct database and environment settings for Heroku. - To access your Laravel app, open the URL provided by Heroku:
heroku open
4. Final Steps
After setting up Laravel in your local or cloud environment, you need to configure your application for production:
- Set the APP_ENV to production in the
.env
file. - Run the Artisan commands to optimize your application:
php artisan config:cache
php artisan route:cache
php artisan view:cache
- Ensure that your storage and cache directories are writable:
chmod -R 775 storage/ bootstrap/cache/
Diagram: Laravel Installation Workflow
The following diagram shows the steps involved in installing and setting up Laravel in both local and cloud environments:

Understanding MVC Architecture in Laravel
The Model-View-Controller (MVC) architecture is a software design pattern widely used in web development to separate application logic into three interconnected components. Laravel follows the MVC architecture, which helps in organizing code and separating concerns. Below is an overview of MVC in Laravel.
1. What is MVC?
MVC stands for:
- Model: Represents the data and the business logic of the application. Models interact with the database and define the data structure.
- View: Represents the user interface. It displays the data to the user and provides an interface for user interaction.
- Controller: Acts as an intermediary between the Model and View. It receives user input, processes it (using Models if necessary), and returns a response (usually using Views).
2. MVC Architecture in Laravel
In Laravel, MVC is implemented as follows:
Model
The Model represents the data layer of the application. Laravel provides an elegant ORM called Eloquent to interact with the database. Eloquent models are responsible for retrieving and manipulating data in the database. For example, a User
model might be responsible for retrieving user-related data from the users
table.
Example of a simple Eloquent Model:
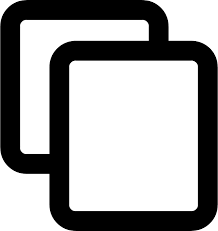
// User.php (Model)
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $table = 'users';
protected $fillable = ['name', 'email', 'password'];
}
View
The View is responsible for displaying the data to the user. Laravel's Blade templating engine is used to build dynamic views. Blade allows you to embed PHP code in your HTML while keeping the syntax simple and clean.
Example of a Blade View (resources/views/user.blade.php):
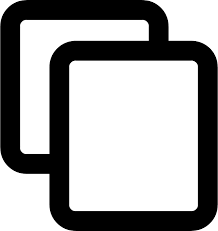
{{ $user->name }}
Email: {{ $user->email }}
Controller
The Controller in Laravel acts as the middleman between the Model and View. It handles user input, retrieves data from Models, and passes it to the Views. Controllers are stored in the app/Http/Controllers
directory.
Example of a simple Controller that fetches data from the User
model and passes it to the View:
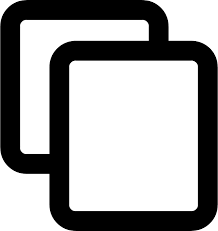
// UserController.php (Controller)
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function show($id)
{
$user = User::find($id);
return view('user', compact('user'));
}
}
3. How MVC Works in Laravel
In Laravel, the flow of an MVC-based application is as follows:
- A user sends a request (e.g., visiting
/user/{id}
). - The route is matched to a controller method in the
routes/web.php
file. - The controller method interacts with the Model to fetch or manipulate data.
- The Controller then returns a View, passing the data to be displayed in the View.
- The View renders the data and is sent back to the user's browser to be displayed.
4. Example Flow
Consider an example where a user wants to view their profile:
- The user visits
/user/1
on your Laravel app. - This request is routed to the
UserController@show
method. - The controller fetches the user data from the database using the
User::find(1)
method. - The controller then passes the data to the
user.blade.php
view. - The view displays the user's name and email on the page.
5. MVC Diagram
The following diagram demonstrates how the Model, View, and Controller work together in Laravel:

Directory Structure and Key Files in Laravel
Laravel follows an organized directory structure that helps developers to navigate and maintain the application efficiently. Below is an overview of the key directories and files in a default Laravel installation:
1. Key Directories
- app/ - Contains the core code for your application such as controllers, models, and middleware.
- bootstrap/ - Contains the files responsible for bootstrapping the Laravel framework and initializing settings.
- config/ - Holds configuration files for the Laravel application, such as database, mail, and cache configurations.
- database/ - Contains database migrations, factories, and seeders.
- public/ - The public-facing directory where the main entry point
index.php
is located. Also contains assets like images, CSS, and JavaScript files. - resources/ - Contains views (Blade templates) and raw assets like Sass, Less, or JavaScript files that will be compiled into production assets.
- routes/ - Houses the route definitions for the application. The routes are typically defined in files like
web.php
andapi.php
. - storage/ - Contains logs, file uploads, and cached files.
- tests/ - Contains automated tests for the application, using PHPUnit.
2. Key Files
- .env - The environment configuration file where sensitive information like database credentials and application keys are stored.
- composer.json - Defines the PHP dependencies of your project.
- artisan - The command-line interface for Laravel, used to run various tasks like migrations, tests, and generating boilerplate code.
- webpack.mix.js - Used to define asset compilation settings when using Laravel Mix.
Creating a New Laravel Project
To create a new Laravel project, you can use Composer, which is the recommended package manager for PHP. Follow these steps:
1. Install Composer
If you don't have Composer installed, you can download it from the official website.
2. Create a New Laravel Project
Once Composer is installed, you can create a new Laravel project by running the following command in your terminal:
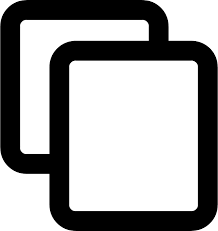
composer create-project --prefer-dist laravel/laravel project-name
This will download and install the latest version of Laravel into a directory called project-name
.
3. Serve the Application
To serve your Laravel application locally, navigate to the project directory and run:
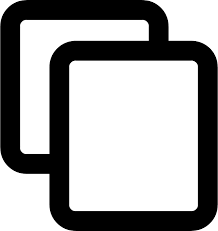
php artisan serve
This will start the built-in development server, and your application will be accessible at http://localhost:8000
.
Laravel Artisan Console: Common Commands
Laravel Artisan is a command-line interface (CLI) that provides several helpful commands for tasks like database migrations, routing, and running tests. Below are some common Artisan commands:
1. Starting the Development Server
To start the local development server, run:
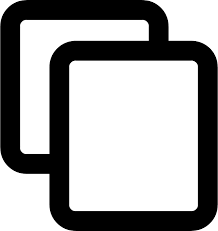
php artisan serve
2. Running Migrations
To run database migrations, use the following command:
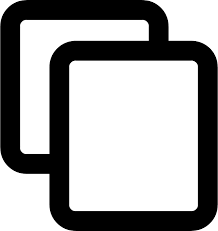
php artisan migrate
3. Rolling Back Migrations
If you need to roll back the last database migration, you can run:
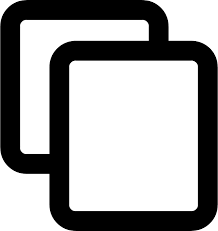
php artisan migrate:rollback
4. Generating Artisan Commands
You can generate various classes such as controllers, models, and migrations using Artisan:
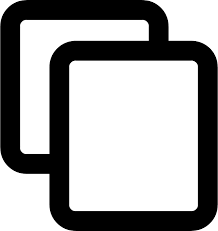
php artisan make:controller ControllerName
php artisan make:model ModelName
php artisan make:migration migration_name
5. Viewing All Artisan Commands
To see a list of all available Artisan commands, run:
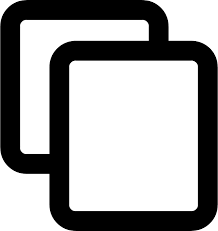
php artisan list
Environment Configuration (.env file)
The .env
file in Laravel is used for environment-specific configurations. This file contains key-value pairs for various settings like database credentials, application keys, and other environment-dependent settings.
1. Structure of the .env File
The .env
file contains the following common configuration settings:
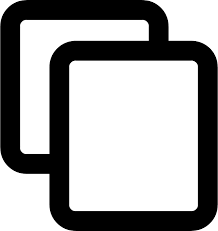
APP_NAME=Laravel
APP_ENV=local
APP_KEY=base64:your-app-key
APP_DEBUG=true
APP_URL=http://localhost
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your-database
DB_USERNAME=root
DB_PASSWORD=
2. Common .env Settings
- APP_NAME - The name of your application.
- APP_ENV - Defines the environment (local, production, staging).
- APP_KEY - The application encryption key. You can generate it using
php artisan key:generate
. - APP_DEBUG - Whether debugging is enabled for the application.
- DB_CONNECTION - The database connection type (e.g., MySQL, SQLite).
- DB_HOST - The database host.
- DB_DATABASE - The name of the database.
- DB_USERNAME - The username for the database.
- DB_PASSWORD - The password for the database.
3. Environment-Specific Configurations
Laravel allows you to use different .env files for different environments. By default, the .env
file is used for local development. For production, you can create a .env.production
file with different settings such as production database credentials, caching configurations, etc.
To ensure your application runs smoothly in different environments, make sure to configure the .env file accordingly.
Defining Routes in web.php and api.php
In Laravel, routes define the actions that should be taken when a user accesses a specific URL. The routes are typically defined in two main files: web.php
for web routes and api.php
for API routes.
1. Defining Web Routes (web.php)
Web routes are defined in the routes/web.php
file. These routes are typically used for handling requests that return HTML views or interact with the user interface.
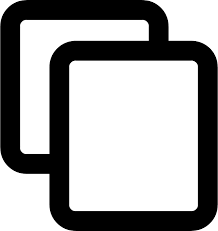
Route::get('/', function () {
return view('welcome');
});
Route::get('/about', function () {
return view('about');
});
2. Defining API Routes (api.php)
API routes are defined in the routes/api.php
file. These routes are typically used for handling requests that return JSON responses.
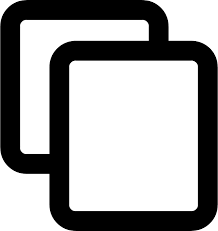
Route::get('/users', function () {
return User::all();
});
Route::get('/posts/{id}', 'PostController@show');
3. Route Prefixing and Grouping
You can also group routes using route groups and apply prefixes, middleware, etc. Example:
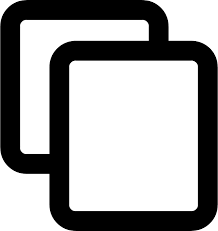
Route::prefix('admin')->group(function () {
Route::get('/dashboard', function () {
return view('admin.dashboard');
});
});
Route Parameters and Named Routes
Laravel allows you to pass parameters through routes and also gives you the ability to name your routes for easier reference.
1. Route Parameters
Route parameters allow you to capture values from the URL and pass them to your controller or closure function.
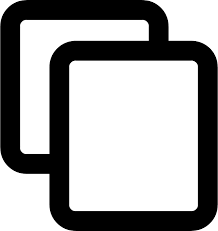
Route::get('/user/{id}', function ($id) {
return 'User ID: ' . $id;
});
2. Optional Parameters
You can define optional parameters by appending a question mark to the parameter name:
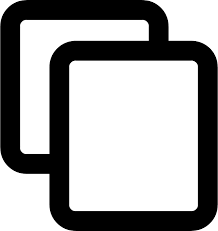
Route::get('/user/{name?}', function ($name = null) {
return $name ? 'User: ' . $name : 'No user specified.';
});
3. Named Routes
Named routes allow you to refer to routes by name rather than URL. This is useful when generating URLs or redirects.
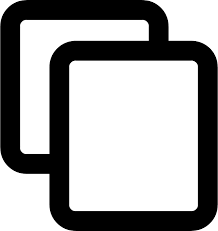
Route::get('/profile/{id}', 'UserController@profile')->name('profile');
// Generate URL to named route
$url = route('profile', ['id' => 1]);
Using Controllers for Logic Separation
In Laravel, controllers help separate the application's logic from the routes. Controllers organize and handle the business logic and can be used in conjunction with routes to manage HTTP requests.
1. Creating a Controller
You can create a new controller using Artisan:
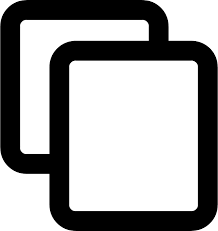
php artisan make:controller UserController
2. Defining Methods in a Controller
You can define methods in the controller to handle different actions. These methods can then be linked to specific routes.
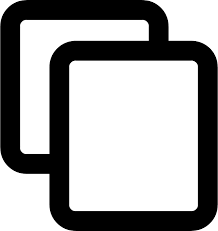
class UserController extends Controller
{
public function show($id)
{
return view('user.profile', ['user' => User::find($id)]);
}
}
3. Registering a Controller in Routes
You can link a controller method to a route like this:
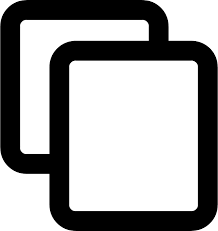
Route::get('/user/{id}', 'UserController@show');
Resource Controllers and Route Model Binding
Laravel provides a simple way to handle CRUD (Create, Read, Update, Delete) operations using resource controllers and route model binding.
1. Resource Controllers
A resource controller is a special type of controller that is designed to handle common CRUD operations. Laravel can automatically generate routes to handle actions like storing, updating, and deleting records.
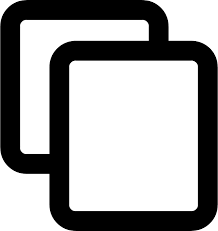
php artisan make:controller PostController --resource
This will generate a controller with methods for each CRUD operation. You can register the resource controller in your web.php
routes file:
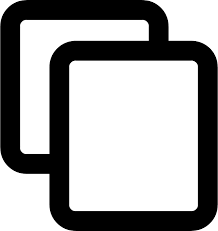
Route::resource('posts', 'PostController');
2. Route Model Binding
Route Model Binding allows you to automatically inject model instances into your controller methods based on the route parameters. This eliminates the need for manual queries to find a model instance.
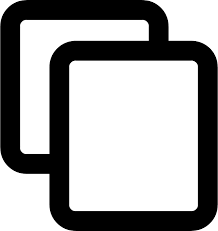
Route::get('/post/{post}', 'PostController@show');
// In PostController, Laravel will automatically inject the Post model
public function show(Post $post)
{
return view('post.show', compact('post'));
}
Introduction to Blade Template Syntax
Blade is Laravel's powerful templating engine that allows you to build dynamic web pages with ease. Blade templates are compiled into plain PHP code and cached for better performance. Blade provides a clean and simple syntax for writing PHP code in your views.
Basic Syntax
Blade uses curly braces to echo data: {{ $variable }}
You can also escape HTML characters in Blade using {{{ $variable }}}
if you need to output raw HTML content.
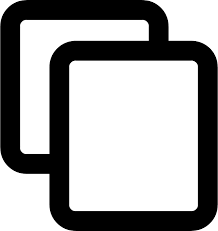
<!-- Blade Syntax -->
<h1>Hello, {{ $name }}</h1>
Displaying Data
To display data, you can use the following Blade syntax:
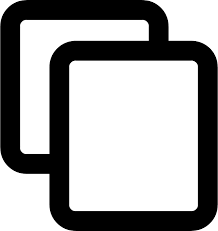
<div>
{{ $user->name }} // Displaying dynamic data from a variable
</div>
Blade Directives (@if, @foreach, @include)
Blade provides a wide range of control structures and directives to make templates more readable and maintainable. Below are some commonly used Blade directives.
1. @if Directive
The @if directive is used to define conditionals in Blade templates.
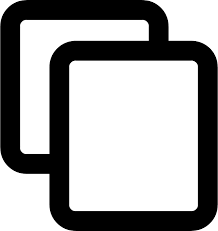
@if ($user->isAdmin())
<p>Welcome, Admin!</p>
@else
<p>Welcome, User!</p>
@endif
2. @foreach Directive
The @foreach directive is used to iterate over arrays or collections.
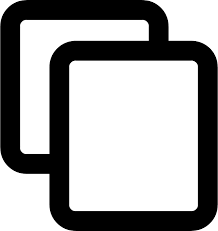
@foreach ($users as $user)
<div>{{ $user->name }}</div>
@endforeach
3. @include Directive
The @include directive is used to include a Blade view inside another view.
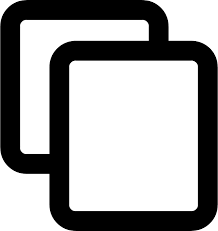
@include('partials.header') // Including header Blade view
Layouts and Sections (@yield, @extends)
Layouts in Blade are used to define the skeleton of your views. You can use @extends
to define a layout and @yield
to specify where content should be injected into the layout.
1. @extends Directive
The @extends directive is used in a view to specify which layout to use.
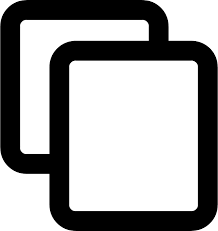
@extends('layouts.app') // Extending the app layout
2. @yield Directive
The @yield directive is used in the layout to define a section where content should be injected.
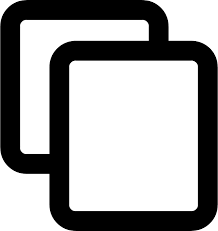
<body>
<div>
@yield('content') // Content from child view will be injected here
</div>
</body>
3. @section Directive
The @section directive is used to define the content for a section in a child view.
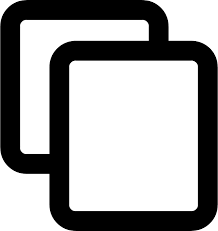
@section('content')
<p>This is the content section!</p>
@endsection
Working with Components and Slots
Laravel Blade components allow you to create reusable pieces of code. Components can accept data and display it dynamically. Slots are a way to pass content into components.
1. Creating a Component
To create a component, you can use the Artisan command:
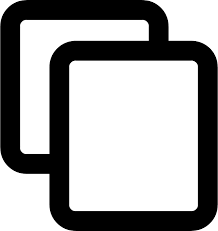
php artisan make:component Alert
This will create a new component class and a corresponding Blade view.
2. Using Components in Views
You can then use the component in your Blade views like this:
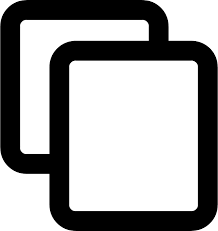
@component('components.alert')
<p>This is an alert message!</p>
@endcomponent
3. Passing Data to Components
You can pass data to Blade components using an array:
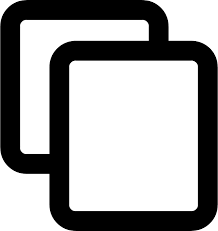
@component('components.alert', ['type' => 'success'])
<p>This is a success message!</p>
@endcomponent
4. Working with Slots
Components can accept slots, which are pieces of content passed into the component from the parent view.
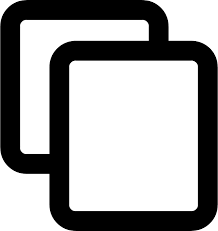
<x-alert>
<p>This is a custom alert message!</p>
</x-alert>
Handling Form Requests
Laravel provides an easy way to handle form submissions with its request handling system. You can use controllers to handle form data and then perform validation or other logic before storing or processing it.
Form Handling Process
The basic steps for handling form requests are:
- Create a form in your Blade view.
- Define a route that handles the form submission.
- Create a controller method to process the form data.
Laravel will automatically validate and handle the incoming request based on the rules you define.
Example: Handling a Simple Form
Here's an example of how to handle a form submission:
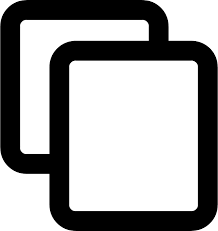
// web.php
Route::post('/submit', [FormController::class, 'handleForm']);
In the FormController:
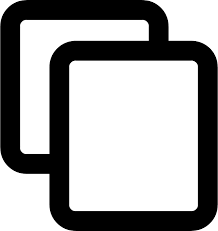
public function handleForm(Request $request)
{
// Process the form data
$validated = $request->validate([
'name' => 'required|max:255',
'email' => 'required|email',
]);
// Store or process data
}
Validating Input Data
Laravel provides a simple and powerful way to validate form inputs using various validation rules. The validation happens automatically when you call the validate()
method on the incoming request.
Validation Rules
Some of the common validation rules include:
required
: Ensures that the field is not empty.email
: Validates that the field is a valid email address.max
: Ensures that the field's value is not greater than a given length.min
: Ensures that the field's value is not less than a given length.
Example: Simple Validation
This example shows how to validate input data inside a controller:
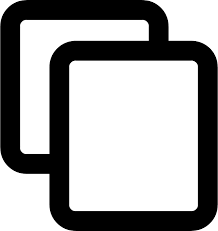
public function store(Request $request)
{
$validated = $request->validate([
'name' => 'required|max:255',
'email' => 'required|email',
]);
// Process the validated data
}
Custom Validation Rules and Messages
In Laravel, you can define custom validation rules and error messages to meet specific requirements.
Custom Validation Rules
To create a custom validation rule, you can use the Validator::extend()
method to define the rule.
Example: Custom Validation Rule
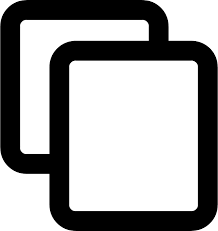
// In a ServiceProvider
Validator::extend('uppercase', function ($attribute, $value, $parameters, $validator) {
return strtoupper($value) === $value;
});
Once you've defined the custom rule, you can use it like any other validation rule:
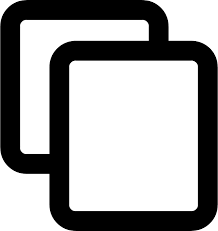
$request->validate([
'username' => 'uppercase',
]);
Custom Error Messages
If you want to customize the error messages for validation rules, you can pass an array of custom messages to the validate()
method:
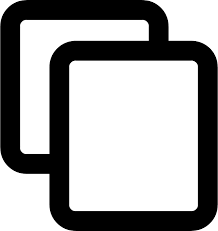
$request->validate([
'email' => 'required|email',
], [
'email.required' => 'We need to know your email address!',
]);
Using Request Classes for Form Validation
Laravel provides a more organized approach to handle form validation using custom request classes. These classes encapsulate validation logic, making your controllers cleaner and more maintainable.
Generating a Request Class
To generate a custom form request class, use the Artisan command:
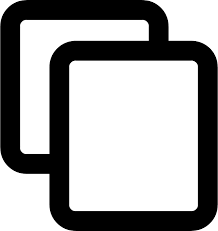
php artisan make:request StoreUserRequest
This will create a new request class in the app/Http/Requests
directory.
Defining Validation Logic
Within the request class, you define the validation rules and authorization logic.
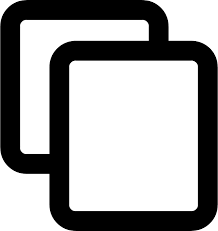
class StoreUserRequest extends FormRequest
{
public function authorize()
{
return true; // Authorize the request
}
public function rules()
{
return [
'name' => 'required|max:255',
'email' => 'required|email',
];
}
}
Using the Request Class in Controllers
Now, you can type-hint the custom request class in your controller methods to automatically validate the form data.
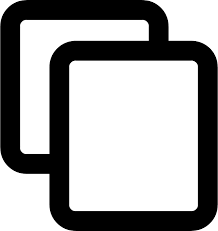
public function store(StoreUserRequest $request)
{
// Data is automatically validated
$validated = $request->validated();
// Process the data
}
Connecting Laravel to Databases (MySQL, SQLite, PostgreSQL)
Laravel supports various database systems, including MySQL, SQLite, and PostgreSQL. You can easily configure your Laravel application to connect to a database through the .env
configuration file.
Configuring Database Connection
To connect Laravel to a database, you need to set up the database connection in the .env
file and config/database.php
file.
MySQL Database Configuration
For MySQL, set the database connection details in the .env
file like this:
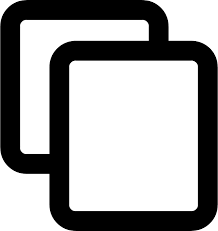
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
SQLite Database Configuration
To use SQLite, you just need to set the DB_CONNECTION
to sqlite
and specify the SQLite file path:
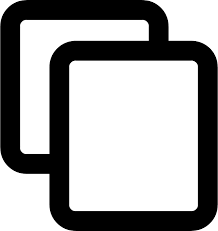
DB_CONNECTION=sqlite
DB_DATABASE=/path_to_your_database/database.sqlite
PostgreSQL Database Configuration
For PostgreSQL, configure it similarly to MySQL by setting the connection details:
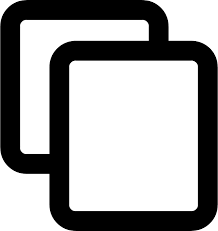
DB_CONNECTION=pgsql
DB_HOST=127.0.0.1
DB_PORT=5432
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
Introduction to Eloquent ORM
Eloquent is Laravel's Object-Relational Mapper (ORM), which provides an easy and expressive way to interact with your database. It eliminates the need to write complex SQL queries by providing a fluent, object-oriented interface for database operations.
Basic Eloquent Usage
With Eloquent, you can perform all database operations using models. Each model corresponds to a database table, and its instances represent rows in that table.
Example: Retrieving Data Using Eloquent
Here's an example of how to retrieve all records from a Post
model:
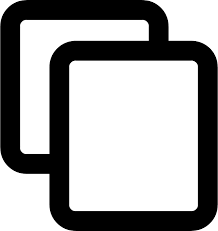
$posts = App\Models\Post::all();
For more complex queries, you can chain Eloquent methods:
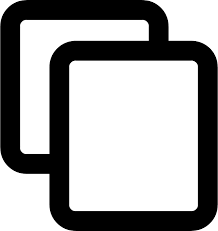
$posts = App\Models\Post::where('status', 'published')
->orderBy('created_at', 'desc')
->get();
Defining Models and Migrations
In Laravel, models represent database tables, and migrations allow you to define and manipulate the database schema. Migrations help keep your database structure consistent across different environments.
Creating a Model and Migration
To create a model along with a migration file, run the following Artisan command:
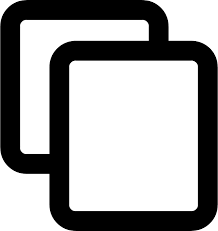
php artisan make:model Post -m
This command will generate a Post
model in the app/Models
directory and a migration file in the database/migrations
directory.
Defining the Migration
In the migration file, you can define the table's columns and their properties:
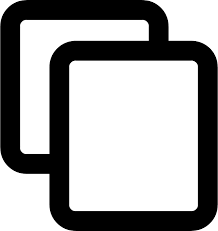
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Running the Migration
To apply the migration and create the table in the database, run the following Artisan command:
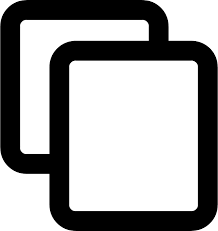
php artisan migrate
Creating, Reading, Updating, and Deleting Records (CRUD)
Laravel provides a simple and intuitive syntax for performing CRUD (Create, Read, Update, Delete) operations with Eloquent ORM. Below is an overview of how to use Eloquent for CRUD operations.
Creating a Record
To create a new record, you can use the create()
method or instantiate the model and save it:
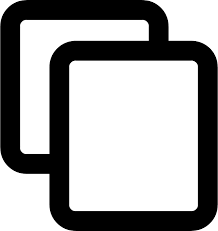
$post = App\Models\Post::create([
'title' => 'New Post',
'content' => 'This is the content of the new post.',
]);
Reading Records
To read records from the database, use methods like all()
, find()
, or where()
:
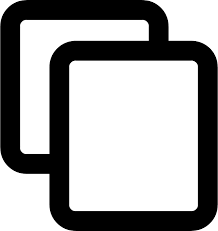
// Get all posts
$posts = App\Models\Post::all();
// Find a single post by ID
$post = App\Models\Post::find(1);
Updating Records
To update an existing record, retrieve it first and then use the update()
method:
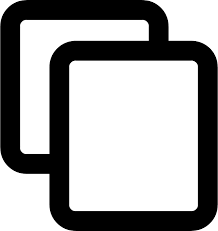
$post = App\Models\Post::find(1);
$post->update([
'title' => 'Updated Post Title',
]);
Deleting Records
To delete a record, retrieve it and use the delete()
method:
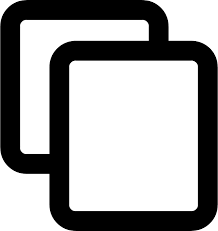
$post = App\Models\Post::find(1);
$post->delete();
Creating and Running Migrations
Migrations are a way to version control your database schema, allowing you to modify and share the database structure across different environments. Laravel provides a simple way to create and run migrations.
Creating a Migration
To create a new migration, use the Artisan command:
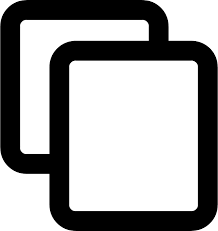
php artisan make:migration create_posts_table
This command generates a migration file in the database/migrations
directory. You can then define the schema for your table inside the migration file.
Running Migrations
Once you have defined your migration, run the following Artisan command to apply the migration and update the database:
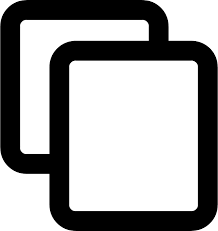
php artisan migrate
This will execute all pending migrations and apply the changes to your database.
Adding Columns and Modifying Tables
Laravel makes it easy to modify your database schema using migrations, whether you want to add new columns, modify existing ones, or even remove columns.
Adding a Column
To add a new column to an existing table, create a new migration with the following command:
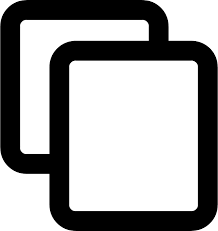
php artisan make:migration add_status_to_posts_table --table=posts
Then, inside the migration file, you can add a new column like this:
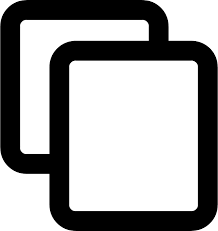
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->string('status')->default('draft');
});
}
Modifying a Column
To modify an existing column, you can use the change()
method:
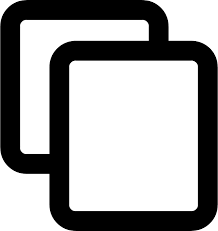
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->string('status')->nullable()->change();
});
}
Running the Migration
Run the migration to apply the changes to the database:
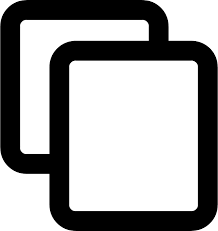
php artisan migrate
Using Seeders and Factories for Test Data
Seeders and factories allow you to generate test data for your application, making it easier to populate the database with fake data for development or testing purposes.
Creating a Seeder
Seeders are used to populate your database with data. You can create a seeder using the Artisan command:
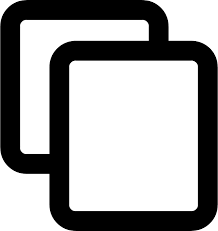
php artisan make:seeder PostsTableSeeder
Inside the generated seeder file, you can define how to populate the database with data:
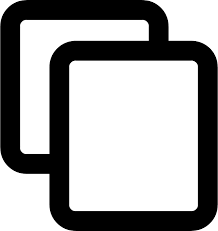
public function run()
{
\App\Models\Post::factory(10)->create();
}
Using Factories for Data Generation
Factories allow you to define how fake data should look. You can create a factory using the following Artisan command:
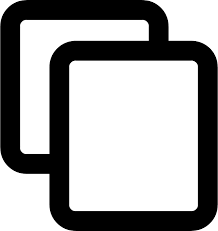
php artisan make:factory PostFactory --model=Post
Define the fake data in the factory file:
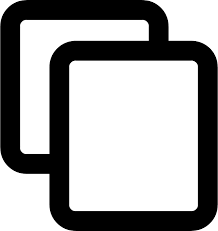
public function definition()
{
return [
'title' => $this->faker->sentence,
'content' => $this->faker->paragraph,
'status' => 'published',
];
}
Running Seeders
To run the seeder and populate the database, execute the following command:
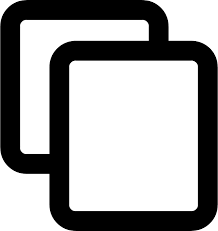
php artisan db:seed --class=PostsTableSeeder
Database Transactions in Laravel
In Laravel, database transactions allow you to perform multiple database operations as a single unit of work. If any operation fails, all changes made during the transaction will be rolled back, ensuring data consistency.
Using Transactions
You can use the DB::transaction()
method to wrap database operations in a transaction:
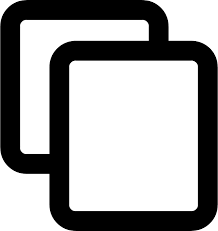
use Illuminate\Support\Facades\DB;
DB::transaction(function () {
$post = new \App\Models\Post;
$post->title = 'Transaction Post';
$post->content = 'This post was created using a transaction.';
$post->save();
// Any other database operations can go here
});
Handling Failures
If an exception is thrown inside the transaction, Laravel will automatically roll back the transaction and no changes will be made to the database.
Manually Committing and Rolling Back Transactions
If you prefer to manually control transactions, you can do so using the DB::beginTransaction()
, DB::commit()
, and DB::rollBack()
methods:
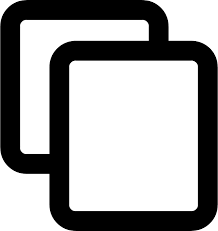
DB::beginTransaction();
try {
// Perform database operations
$post = new \App\Models\Post;
$post->title = 'Manual Transaction Post';
$post->save();
// Commit the transaction
DB::commit();
} catch (\Exception $e) {
// Rollback the transaction if something goes wrong
DB::rollBack();
throw $e;
}
One-to-One, One-to-Many, and Many-to-Many Relationships
Laravel's Eloquent ORM makes it easy to define and work with relationships between models. You can define a variety of relationships, such as one-to-one, one-to-many, and many-to-many, to model complex database structures.
One-to-One Relationship
A one-to-one relationship is used when one model is associated with exactly one other model. For example, a user might have one profile.
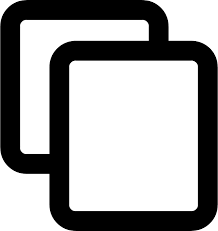
// In User model
public function profile()
{
return $this->hasOne(Profile::class);
}
// In Profile model
public function user()
{
return $this->belongsTo(User::class);
}
One-to-Many Relationship
A one-to-many relationship is used when one model is associated with many other models. For instance, a post might have many comments.
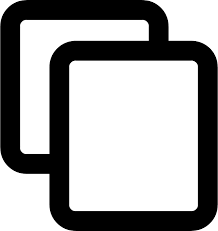
// In Post model
public function comments()
{
return $this->hasMany(Comment::class);
}
// In Comment model
public function post()
{
return $this->belongsTo(Post::class);
}
Many-to-Many Relationship
A many-to-many relationship is used when both models are related to each other in a many-to-many way. For example, a user can belong to many roles, and a role can belong to many users.
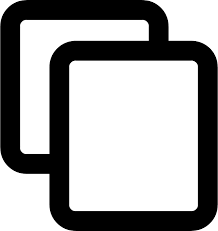
// In User model
public function roles()
{
return $this->belongsToMany(Role::class);
}
// In Role model
public function users()
{
return $this->belongsToMany(User::class);
}
HasOneThrough and HasManyThrough
Laravel also supports advanced relationships like HasOneThrough
and HasManyThrough
, which allow you to define relationships that span multiple models.
HasOneThrough
A HasOneThrough
relationship is used when you need to retrieve a related model through an intermediate model. For example, a country might have one related post through a user.
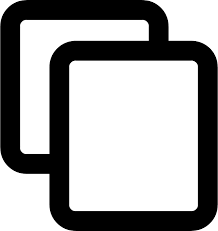
// In Country model
public function post()
{
return $this->hasOneThrough(Post::class, User::class);
}
HasManyThrough
A HasManyThrough
relationship is used when you need to retrieve multiple related models through an intermediate model. For instance, a country might have many posts through users.
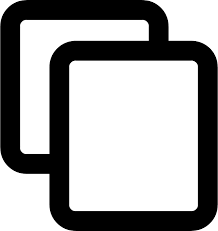
// In Country model
public function posts()
{
return $this->hasManyThrough(Post::class, User::class);
}
Eager Loading and Lazy Loading
Eloquent provides two ways to load relationships: eager loading and lazy loading. Eager loading helps reduce the number of queries executed by loading related models in advance, while lazy loading loads related models only when needed.
Eager Loading
Eager loading loads the related models when the parent model is retrieved. This avoids the N+1 query problem where multiple queries are executed for each related model.
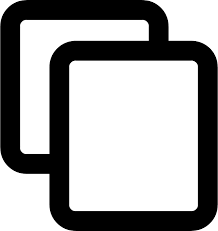
$posts = Post::with('comments')->get();
Lazy Loading
Lazy loading loads the related models only when they are accessed. This can result in additional queries being executed as you access the relationships.
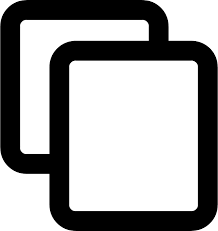
$post = Post::find(1);
$comments = $post->comments;
Using Pivot Tables for Many-to-Many Relationships
In many-to-many relationships, you often need an intermediary table, known as a pivot table, to manage the relationship between the two models. Laravel makes it simple to work with pivot tables and even add additional data to the pivot table.
Defining a Many-to-Many Relationship with a Pivot Table
When defining many-to-many relationships, you can use the belongsToMany
method. If your pivot table has additional columns, you can access them with the pivot
attribute.
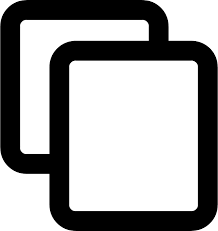
// In User model
public function roles()
{
return $this->belongsToMany(Role::class)->withPivot('created_at');
}
// In Role model
public function users()
{
return $this->belongsToMany(User::class);
}
Accessing Pivot Data
You can access the pivot data like this:
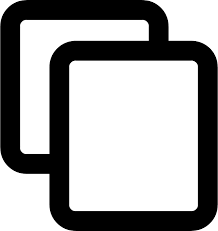
$user = User::find(1);
foreach ($user->roles as $role) {
echo $role->pivot->created_at;
}
Attaching and Detaching Records
You can attach or detach records from the pivot table like this:
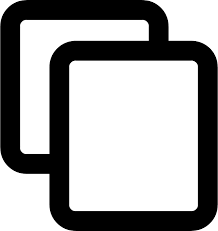
// Attach a role to a user
$user->roles()->attach($roleId);
// Detach a role from a user
$user->roles()->detach($roleId);
Setting Up Authentication with php artisan make:auth
Laravel provides an easy way to set up authentication using the php artisan make:auth
command. This command automatically generates authentication views, routes, and controllers for login, registration, and password resets. It simplifies the process of adding user authentication to your application.
Running the Command
To set up authentication in your Laravel app, run the following command:
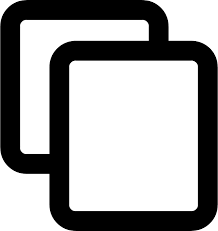
php artisan make:auth
What the Command Does
- Generates authentication views (login, register, password reset).
- Creates routes for authentication in
routes/web.php
. - Creates a controller for handling authentication logic.
After running this command, you can access the authentication routes and views in your application.
Manual Authentication (Login, Registration)
Manual authentication allows you to implement custom login and registration functionality without relying on Laravel's built-in authentication system. You can create the necessary routes, controllers, and views to handle user authentication manually.
Creating Login and Registration Routes
Start by defining the routes for login and registration in routes/web.php
:
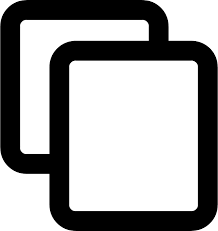
Route::get('login', 'AuthController@showLoginForm');
Route::post('login', 'AuthController@login');
Route::get('register', 'AuthController@showRegistrationForm');
Route::post('register', 'AuthController@register');
Handling User Authentication in Controller
In the AuthController
, you can define the logic for displaying the forms and handling user login and registration:
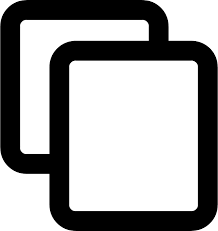
public function showLoginForm()
{
return view('auth.login');
}
public function login(Request $request)
{
// Validate and authenticate user
}
public function showRegistrationForm()
{
return view('auth.register');
}
public function register(Request $request)
{
// Validate and create new user
}
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a method of restricting access to certain parts of your application based on the user's role. Laravel provides a simple way to implement RBAC by using middleware and policies to check user roles before allowing access to specific routes or resources.
Defining User Roles
First, create a roles
table in your database to store roles. You can then assign roles to users in your users
table or using a separate pivot table.
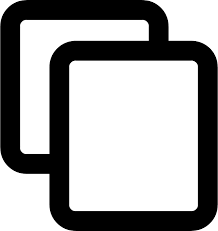
// Migration for roles table
Schema::create('roles', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamps();
});
Assigning Roles to Users
You can create a many-to-many relationship between users and roles. Each user can have one or more roles:
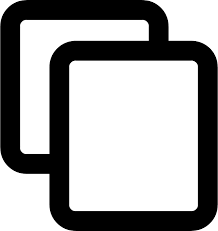
// In User model
public function roles()
{
return $this->belongsToMany(Role::class);
}
Protecting Routes with Role Middleware
Once roles are defined and assigned, you can restrict access to routes based on the user's role:
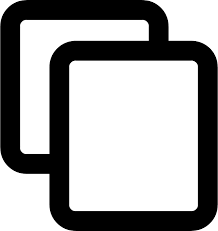
Route::middleware('role:admin')->get('/admin', function() {
return view('admin.dashboard');
});
Policies and Gates for Authorization
Laravel provides Policies and Gates as powerful ways to manage authorization logic in your application. Policies are used for model-based authorization, while gates are used for more general authorization logic.
Creating Policies
To create a policy, you can use the php artisan make:policy
command. Policies are usually tied to specific models and are stored in the app/Policies
directory.
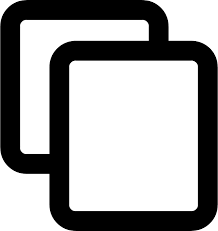
php artisan make:policy PostPolicy
Registering Policies
Once you’ve created a policy, you need to register it in the AuthServiceProvider
:
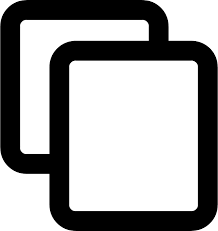
protected $policies = [
Post::class => PostPolicy::class,
];
Using Policies
Now, you can use policies in your controllers or views to check if a user is authorized to perform a given action:
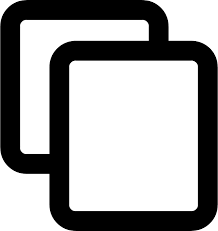
// In controller
public function update(Post $post)
{
$this->authorize('update', $post);
}
Using Gates for General Authorization
Gates are used when you need to define simple, general authorization logic. You can define gates in the AuthServiceProvider
:
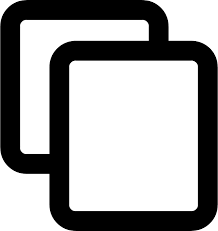
Gate::define('update-post', function ($user, $post) {
return $user->id === $post->user_id;
});
Checking Gates
You can check gates using the Gate
facade:
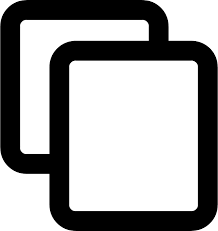
if (Gate::allows('update-post', $post)) {
// The user can update the post
}
Creating and Using Middleware
Middleware in Laravel provides a convenient mechanism for filtering HTTP requests entering your application. For example, middleware can be used to check if the user is authenticated, log requests, or handle CORS. Laravel includes several built-in middleware and also allows you to create custom middleware for specific tasks.
Creating Middleware
To create custom middleware, you can use the php artisan make:middleware
command:
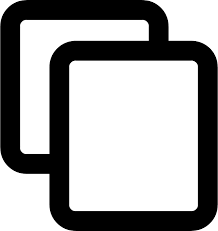
php artisan make:middleware CheckAge
Middleware Logic
The generated middleware will be placed in the app/Http/Middleware
directory. You can define the logic inside the handle
method:
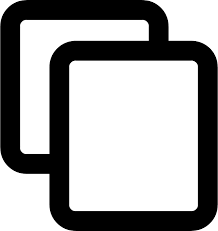
public function handle($request, Closure $next)
{
if ($request->age < 18) {
return redirect('home');
}
return $next($request);
}
In this example, the middleware checks if the user is under 18 and redirects them to the home page.
Applying Middleware to Routes
You can apply middleware to routes in your application to ensure that a certain condition is met before accessing the route's logic. Middleware can be applied globally, to specific routes, or even to route groups.
Applying Middleware to a Single Route
To apply middleware to a specific route, use the middleware
method in the route definition:
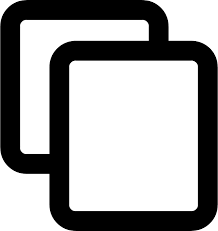
Route::get('/profile', function () {
// Only accessible if middleware passes
})->middleware('checkAge');
Applying Middleware to Route Groups
You can also apply middleware to a group of routes:
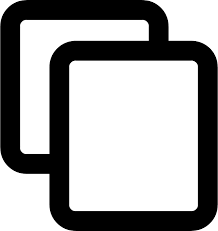
Route::middleware(['checkAge'])->group(function () {
Route::get('/profile', function () { /* ... */ });
Route::get('/settings', function () { /* ... */ });
});
In this example, the checkAge
middleware will be applied to both the /profile
and /settings
routes.
Global vs. Route-Specific Middleware
Laravel allows you to define middleware that can either be applied globally to all routes or only to specific routes. Understanding the difference will help you manage the flow of requests effectively.
Global Middleware
Global middleware is applied to every HTTP request entering your application. It is registered in the app/Http/Kernel.php
file under the $middleware
property:
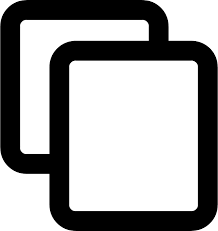
protected $middleware = [
\App\Http\Middleware\CheckAge::class,
\App\Http\Middleware\EncryptCookies::class,
// Other global middleware
];
Route-Specific Middleware
Route-specific middleware, on the other hand, is applied only to specific routes or route groups. You can register these middleware in the same Kernel.php
file under the $routeMiddleware
property:
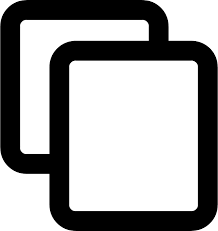
protected $routeMiddleware = [
'checkAge' => \App\Http\Middleware\CheckAge::class,
// Other route-specific middleware
];
Route-specific middleware allows you to apply filtering conditions only when needed, rather than globally.
Using Middleware for Authentication
Middleware is commonly used for authentication purposes in Laravel. You can use the built-in auth
middleware to ensure that only authenticated users can access certain routes.
Using the Built-In Authentication Middleware
To apply authentication middleware to a route, simply use the auth
middleware:
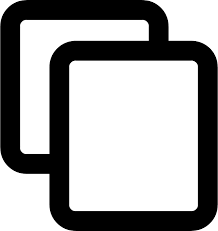
Route::get('/profile', function () {
return view('profile');
})->middleware('auth');
Customizing Authentication Middleware
If you need to customize the authentication middleware, you can create your own middleware or modify the existing one. For example, you can create a middleware that checks the user’s role in addition to checking if they are authenticated.
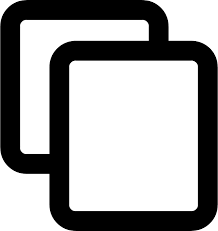
public function handle($request, Closure $next)
{
if (!auth()->check() || auth()->user()->role != 'admin') {
return redirect('home');
}
return $next($request);
}
In this example, the middleware checks if the user is authenticated and if their role is 'admin'. If either of these conditions is not met, the user will be redirected.
Creating and Using Service Classes
In Laravel, service classes are typically used to organize business logic or handle complex tasks that don’t belong in controllers. By encapsulating logic inside service classes, you can keep your controllers clean and maintainable.
Creating a Service Class
You can create a service class using the php artisan make:service
command (you may need to create the service directory manually):
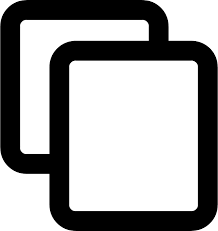
php artisan make:service ExampleService
In the generated service class, you can define methods to handle specific logic. For example:
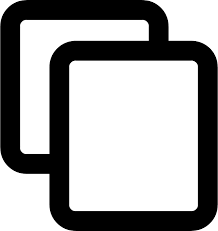
namespace App\Services;
class ExampleService {
public function processData($data)
{
// Business logic here
return $data * 2;
}
}
Using the Service Class in a Controller
To use the service class in your controllers, you can inject it via dependency injection:
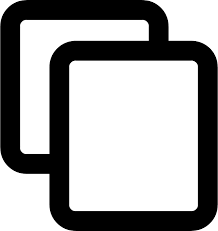
use App\Services\ExampleService;
class ExampleController extends Controller {
protected $exampleService;
public function __construct(ExampleService $exampleService)
{
$this->exampleService = $exampleService;
}
public function show()
{
$result = $this->exampleService->processData(5);
return view('example.show', compact('result'));
}
}
Service Providers in Laravel
Service providers are the central place where you bind your application's services into the service container. Laravel uses service providers to perform any initialization or bootstrapping of services when the application starts.
Creating a Service Provider
You can create a service provider using the php artisan make:provider
command:
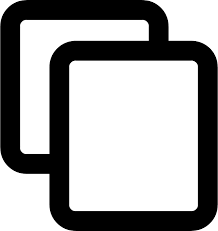
php artisan make:provider ExampleServiceProvider
Registering a Service Provider
After creating a service provider, you must register it in the config/app.php
file under the providers
array:
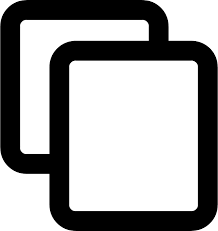
'providers' => [
// Other providers...
App\Providers\ExampleServiceProvider::class,
]
Defining Logic in Service Providers
Inside the service provider, you typically define two methods: register
and boot
. The register
method binds services to the container, while the boot
method is used for initialization logic:
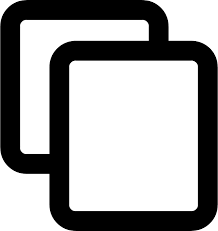
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
class ExampleServiceProvider extends ServiceProvider {
public function register()
{
$this->app->bind('example', function ($app) {
return new \App\Services\ExampleService();
});
}
public function boot()
{
//
}
}
Binding Interfaces to Implementations
In Laravel, the service container is used to bind interfaces to concrete implementations. This allows you to swap out implementations without changing the code that depends on the interface, adhering to the Dependency Inversion Principle (DIP).
Binding Interface to Implementation
You can bind an interface to an implementation in the register
method of a service provider:
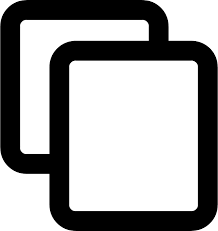
namespace App\Providers;
use App\Contracts\ExampleInterface;
use App\Services\ExampleService;
class ExampleServiceProvider extends ServiceProvider {
public function register()
{
$this->app->bind(ExampleInterface::class, ExampleService::class);
}
public function boot()
{
//
}
}
Using the Bound Interface
Once the binding is in place, you can type-hint the interface in your classes, and Laravel will automatically inject the appropriate implementation:
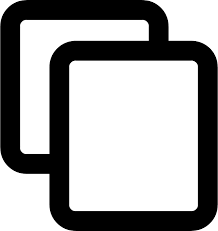
use App\Contracts\ExampleInterface;
class ExampleController extends Controller {
protected $exampleService;
public function __construct(ExampleInterface $exampleService)
{
$this->exampleService = $exampleService;
}
public function show()
{
$result = $this->exampleService->processData(5);
return view('example.show', compact('result'));
}
}
Dependency Injection and Inversion of Control
Dependency Injection (DI) is a design pattern where a class's dependencies are provided (injected) rather than being created within the class. This decouples the class from its dependencies, making it easier to test, maintain, and swap out implementations.
Inversion of Control (IoC)
Inversion of Control is a principle that helps achieve Dependency Injection. In Laravel, the service container manages the IoC, handling the instantiation of objects and injecting their dependencies.
Using Dependency Injection in Laravel
Laravel automatically handles dependency injection for controllers. For example, if you type-hint a class in a controller's constructor, Laravel will resolve and inject its dependencies:
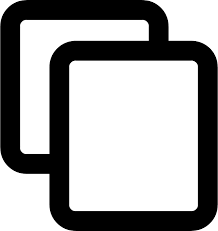
use App\Services\ExampleService;
class ExampleController extends Controller {
protected $exampleService;
public function __construct(ExampleService $exampleService)
{
$this->exampleService = $exampleService;
}
public function show()
{
$result = $this->exampleService->processData(5);
return view('example.show', compact('result'));
}
}
Benefits of Dependency Injection
- Improves testability by allowing you to mock dependencies.
- Decouples components, making your code more flexible and easier to maintain.
- Improves the structure and readability of code.
Uploading Files and Validating Uploads
Laravel provides a simple way to handle file uploads. You can easily upload files and validate them to ensure they meet your application's requirements.
Uploading Files
To upload files, you can use the file
method on the Request
object. Here's an example of how to handle file uploads:
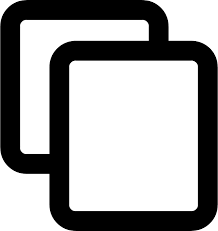
public function store(Request $request) {
$request->validate([
'file' => 'required|file|mimes:jpg,png,pdf|max:10240',
]);
$file = $request->file('file');
$path = $file->store('uploads');
return response()->json(['path' => $path]);
}
In this example, the file is validated to be of type JPG, PNG, or PDF and not larger than 10MB. The file is then stored in the uploads
directory.
Validating Files
You can validate file uploads easily using Laravel's validation rules. Some common rules for file validation include:
mimes
: Specifies the allowed file types (e.g., jpg, png, pdf).max
: Limits the file size (in kilobytes).required
: Ensures a file is uploaded.
Storing Files in the Public and Storage Folders
Files uploaded in Laravel can be stored in different locations, such as the public
or storage
directories. You can manage where files are stored based on your needs.
Storing Files in the Public Folder
Files stored in the public
directory are publicly accessible. You can store files using the public_path
method:
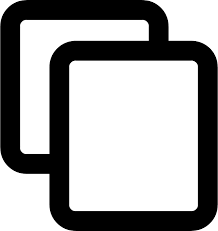
$path = $request->file('file')->move(public_path('uploads'), $filename);
Storing Files in the Storage Folder
To store files securely, you can use the storage
folder. Files stored here are not publicly accessible unless explicitly made so. Use the store
method on the file instance:
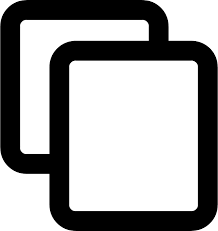
$path = $request->file('file')->store('documents');
To ensure the files are publicly accessible, use php artisan storage:link
to create a symbolic link from the public
folder to the storage/app/public
folder.
Using the Storage Facade
The Storage
facade provides a convenient way to interact with your storage disks. Laravel supports multiple storage options, such as local, S3, and FTP. You can easily store, retrieve, and delete files using the Storage
facade.
Storing Files Using the Storage Facade
To store a file using the Storage
facade, simply use the put
method for files:
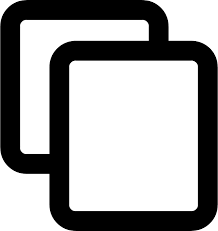
use Illuminate\Support\Facades\Storage;
Storage::put('uploads/filename.txt', 'File content');
Retrieving Files Using the Storage Facade
To retrieve a file, use the get
method:
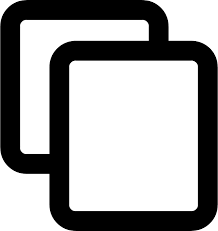
$content = Storage::get('uploads/filename.txt');
Deleting Files Using the Storage Facade
To delete a file, use the delete
method:
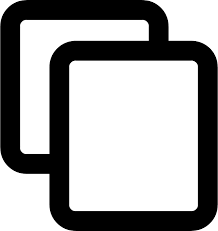
Storage::delete('uploads/filename.txt');
The Storage
facade makes it easy to work with different file storage backends and abstract file handling in your application.
Managing File Downloads
Laravel makes it easy to handle file downloads by providing helper methods to serve files to users. You can use the response()->download()
method to send a file to the browser for download.
Basic File Download
To trigger a download of a file, use the download
method:
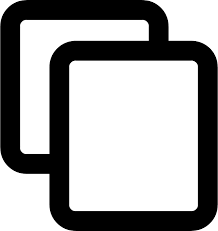
public function downloadFile($filename)
{
$path = storage_path('app/uploads/' . $filename);
return response()->download($path);
}
Setting Custom Headers
You can also set custom headers for the download, such as content type:
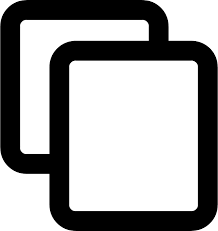
return response()->download($path, 'new-name.txt', [
'Content-Type' => 'application/octet-stream',
]);
This allows you to specify a custom filename and content type for the file being downloaded. Laravel makes it easy to manage file downloads with a simple API.
Building RESTful APIs
Laravel makes it easy to build RESTful APIs, which are essential for building modern web and mobile applications. You can create routes, controllers, and responses that follow REST conventions.
Defining API Routes
API routes in Laravel are defined in the routes/api.php
file. Here’s an example of defining a simple API route:
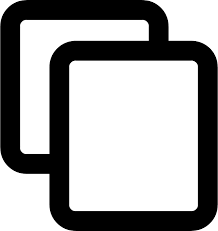
Route::get('/posts', [PostController::class, 'index']);
This route will respond to GET
requests to /posts
and invoke the index
method in the PostController
.
Creating Controllers for API Logic
Controllers in Laravel help separate the logic for handling API requests. You can create API controllers using the following Artisan command:
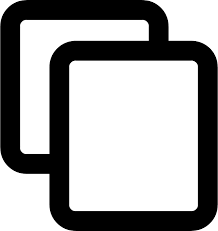
php artisan make:controller Api/PostController
Responding with JSON
For RESTful APIs, responses are typically in JSON format. Laravel makes it easy to return JSON responses using the response()->json()
method:
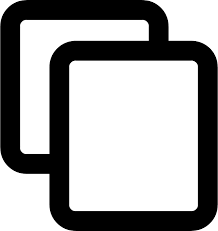
public function index() {
$posts = Post::all();
return response()->json($posts);
}
Using API Resources and Resource Collections
API Resources in Laravel provide a way to transform models and collections of models into JSON responses. This helps keep the API responses consistent and structured.
Creating an API Resource
You can create an API resource using the Artisan command:
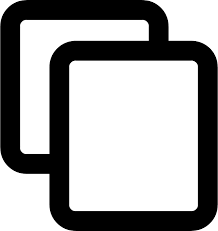
php artisan make:resource PostResource
Then, you can define how the data should be returned by modifying the toArray
method in the resource:
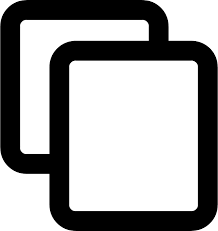
public function toArray($request) {
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
];
}
Using Resource Collections
To return multiple resources, you can use a resource collection. Laravel automatically handles collections of resources when you return them from your controller:
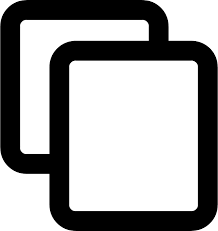
public function index() {
return PostResource::collection(Post::all());
}
Handling API Authentication with Passport or Sanctum
Laravel provides two solutions for API authentication: Passport and Sanctum. Both tools allow you to authenticate API requests but have different use cases.
Using Passport for API Authentication
Passport is a full OAuth2 server implementation for API authentication. To get started, install Passport and run its migrations:
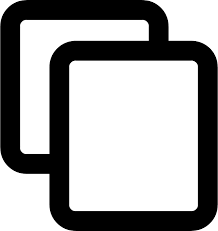
composer require laravel/passport
php artisan migrate
php artisan passport:install
After setting up Passport, you can protect your API routes by using the auth:api
middleware:
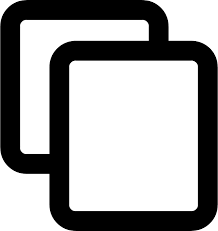
Route::middleware('auth:api')->get('/user', function (Request $request) {
return $request->user();
});
Using Sanctum for API Authentication
Sanctum is a simpler alternative to Passport for SPAs (Single Page Applications) or mobile apps that need simple token-based authentication. To get started with Sanctum, install it and publish its configuration:
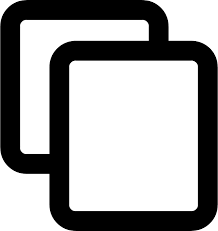
composer require laravel/sanctum
php artisan sanctum:install
Sanctum uses tokens for authentication, and you can issue tokens like this:
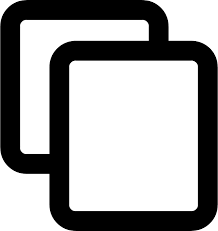
$user = User::find(1);
$token = $user->createToken('API Token')->plainTextToken;
Rate Limiting for API Requests
To ensure that your API can handle the traffic and prevent abuse, Laravel provides an easy way to define rate limits for API requests.
Defining Rate Limits
You can define rate limits for your API routes using the throttle
middleware in the routes/api.php
file:
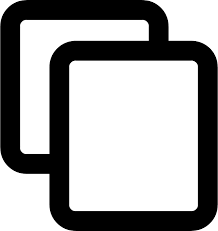
Route::middleware('throttle:60,1')->get('/user', function () {
return response()->json(auth()->user());
});
In this example, the throttle:60,1
middleware limits the number of requests to 60 per minute. The parameters are the maximum number of requests and the time window in minutes.
Customizing Rate Limits
You can also define custom rate limits in the RouteServiceProvider
by overriding the configureRateLimiting
method:
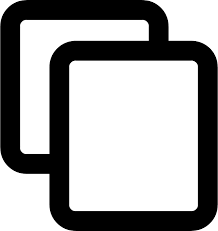
public function configureRateLimiting()
{
RateLimiter::for('api', function (Request $request) {
return Limit::perMinute(60);
});
}
This allows you to configure different rate limits for various types of requests or routes in your application.
Working with Events and Listeners
In Laravel, events and listeners provide a simple observer pattern implementation. You can dispatch events when specific actions occur in your application, and listeners can handle those events asynchronously or synchronously.
Creating an Event
To create an event, use the Artisan command:
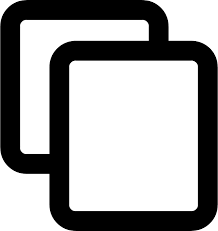
php artisan make:event PostCreated
This will generate an event class in the app/Events
directory. You can add the event's data to the constructor:
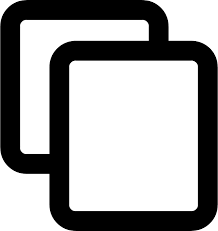
class PostCreated
{
public $post;
public function __construct(Post $post)
{
$this->post = $post;
}
}
Creating a Listener
To handle the event, create a listener class using the Artisan command:
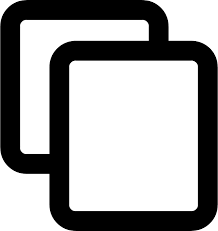
php artisan make:listener SendPostCreatedNotification --event=PostCreated
In the listener, you can define the logic to handle the event:
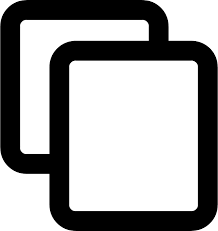
class SendPostCreatedNotification
{
public function handle(PostCreated $event)
{
// Send notification, email, etc.
}
}
Dispatching Events
Events are dispatched using the event()
helper. Here's an example of dispatching the PostCreated
event after a new post is created:
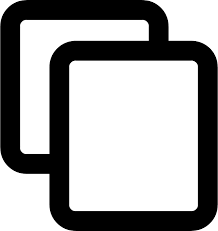
event(new PostCreated($post));
Creating and Dispatching Events
Laravel's event system allows you to create and dispatch events easily. Events can be dispatched either synchronously or asynchronously, depending on your needs.
Creating an Event Class
Use the Artisan command to create an event class. The event class should contain the data you want to pass to the listeners:
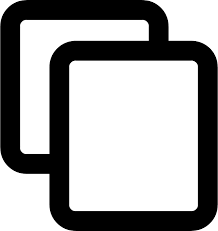
php artisan make:event OrderShipped
Here’s an example of an event class that passes an order object:
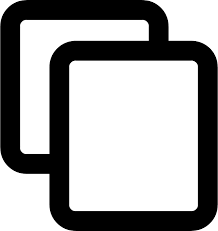
class OrderShipped
{
public $order;
public function __construct(Order $order)
{
$this->order = $order;
}
}
Dispatching the Event
To dispatch the event, you can use the event()
function:
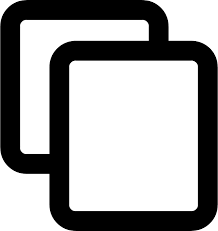
event(new OrderShipped($order));
Queueing Jobs and Managing Queues
Laravel queues provide a unified API across a variety of queue backends, such as database, Redis, and more. Jobs are pushed to a queue and processed in the background.
Creating a Job Class
To create a job, use the Artisan command:
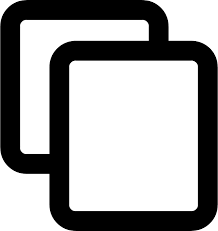
php artisan make:job SendEmailNotification
The job class will contain the logic for the task you want to perform. Here’s an example of a job that sends an email:
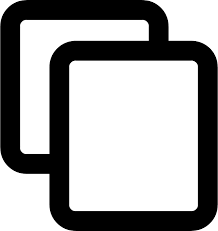
class SendEmailNotification implements ShouldQueue
{
public function handle()
{
// Send email logic
}
}
Dispatching the Job
Jobs are dispatched using the dispatch()
function:
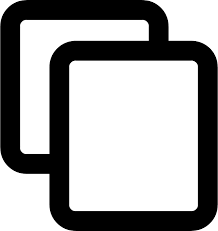
SendEmailNotification::dispatch($user);
Running the Queue Worker
To process jobs in the queue, run the queue worker using the following Artisan command:
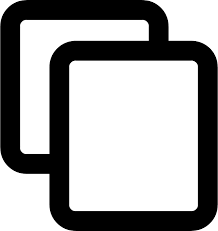
php artisan queue:work
Using Redis for Queue Management
Redis is a popular in-memory data store that can be used as a backend for Laravel queues. It offers high-performance queue processing capabilities.
Installing Redis
To use Redis in Laravel, you need to install the predis/predis
package:
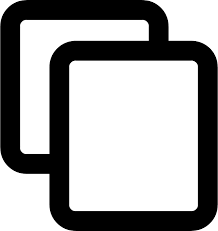
composer require predis/predis
Configuring Redis
In the config/queue.php
file, set the default queue connection to Redis:
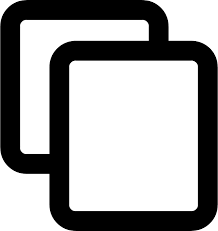
'default' => env('QUEUE_CONNECTION', 'redis'),
Using Redis for Queueing Jobs
Once Redis is configured, you can use it to queue jobs just like any other queue backend. Laravel will automatically handle job processing using Redis:
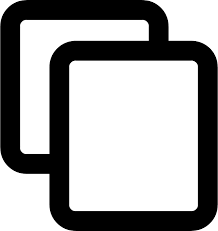
php artisan queue:work redis
Setting Up Task Scheduling with schedule:run
Laravel offers an elegant way to schedule tasks through its built-in task scheduler, which allows you to fluently define your scheduled commands within your application.
Setting Up the Scheduler
To set up task scheduling, you need to add a cron entry to your server. Run the following command to open your crontab file:
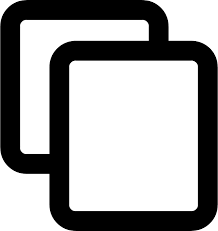
crontab -e
Then, add the following cron entry to run Laravel's task scheduler every minute:
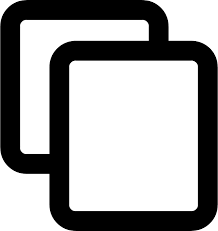
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
This will call Laravel's schedule:run
command every minute, which will check your scheduled tasks and run them if needed.
Defining and Running Cron Jobs
In Laravel, you can define tasks to be run at specific intervals using the app/Console/Kernel.php
file, where you can schedule various Artisan commands or closures.
Defining Scheduled Jobs
To define a scheduled task, open the app/Console/Kernel.php
file and use the schedule()
method in the schedule
method. Here’s an example of scheduling a task to run daily:
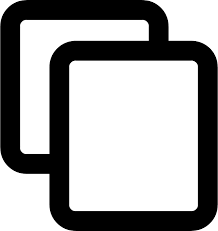
protected function schedule(Schedule $schedule)
{
$schedule->command('inspire')->daily();
}
In this example, the inspire
command will run once every day. You can use any Artisan command or closures here.
Running Cron Jobs
The cron job will automatically be triggered when the schedule:run
command is executed every minute, as configured earlier. Laravel will evaluate the schedule and run the jobs if the time has come.
Using Closures and Artisan Commands for Scheduled Tasks
Laravel's task scheduler allows you to run custom closures (functions) or Artisan commands at specified intervals. This feature makes it easy to manage scheduled tasks within your Laravel application.
Using Closures for Scheduled Tasks
In addition to scheduling Artisan commands, you can also define custom closures. For example, here’s how you can run a closure every minute:
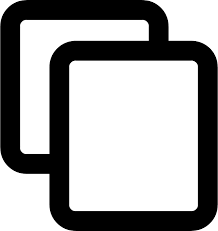
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
// Your task logic here
})->everyMinute();
}
In this example, the closure will be executed every minute. You can replace the closure with any logic you need to execute periodically.
Using Artisan Commands for Scheduled Tasks
Laravel allows you to schedule any Artisan command. For instance, if you want to run a custom command every hour, you can do so like this:
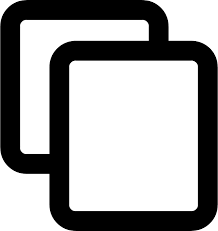
protected function schedule(Schedule $schedule)
{
$schedule->command('custom:command')->hourly();
}
In this example, the custom:command
will be executed every hour. You can define your own commands and schedule them as needed.
Unit Testing with PHPUnit
Unit testing is a fundamental practice for ensuring the integrity of your application. Laravel uses PHPUnit, a widely used testing framework for PHP. You can write unit tests to ensure that your application’s logic behaves as expected.
Setting Up PHPUnit in Laravel
Laravel comes pre-configured with PHPUnit, and the configuration is stored in the phpunit.xml
file at the root of the project. To run tests, use the following Artisan command:
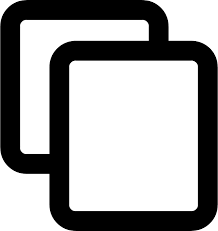
php artisan test
Writing Unit Tests
Unit tests can be created in the tests/Unit
directory. For instance, you can create a test for a service class:
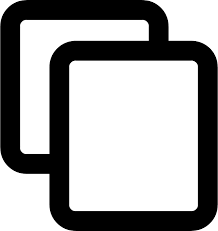
// Example of a unit test
public function testAddition()
{
$result = $this->add(2, 3);
$this->assertEquals(5, $result);
}
In this example, the test checks if the add
function correctly adds two numbers.
Feature Testing with HTTP Requests
Feature tests allow you to test the functionality of your application as a whole, including HTTP requests. Laravel provides methods to simulate HTTP requests and test your application's responses.
Writing Feature Tests
Feature tests are stored in the tests/Feature
directory. You can test routes, controllers, and more. To test an HTTP request, use the get()
, post()
, and other HTTP methods:
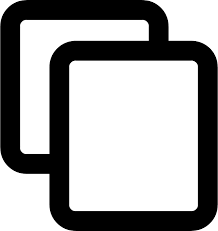
// Example of a feature test for a GET request
public function testHomePage()
{
$response = $this->get('/');
$response->assertStatus(200);
}
In this example, the test sends a GET request to the home page and checks if the status code is 200 (OK).
Mocking and Stubbing in Tests
Mocking and stubbing are techniques used in testing to isolate parts of your code. They allow you to simulate the behavior of objects and methods, without having to rely on actual dependencies like databases or external services.
Mocking Methods
To mock methods in Laravel, use the mock()
method to create mock objects:
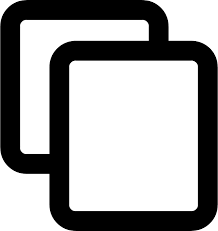
public function testMocking()
{
$mock = Mockery::mock('ClassName');
$mock->shouldReceive('methodName')->andReturn('value');
$this->assertEquals('value', $mock->methodName());
}
Stubbing Methods
Stubbing allows you to replace a method's behavior with a predefined response:
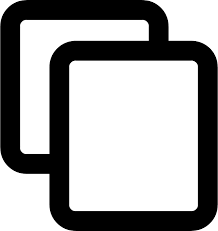
public function testStub()
{
$stub = $this->createMock(ClassName::class);
$stub->method('methodName')->willReturn('stubbed value');
$this->assertEquals('stubbed value', $stub->methodName());
}
Using Factories for Test Data
Laravel provides a powerful factory feature to generate test data. Factories allow you to easily create fake data for your tests, making it easier to test various scenarios in your application.
Creating and Using Factories
To create a factory, use the following Artisan command:
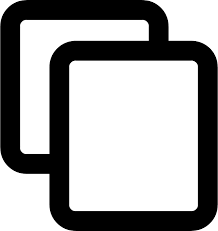
php artisan make:factory UserFactory --model=User
This will create a factory for the User
model. You can define default attributes for the model inside the factory:
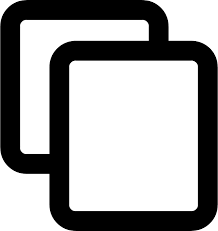
public function definition()
{
return [
'name' => $this->faker->name,
'email' => $this->faker->unique()->safeEmail,
'password' => bcrypt('password'),
];
}
Using Factories in Tests
You can now use the factory to create test data in your tests:
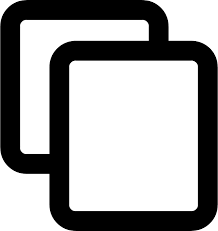
public function testUserCreation()
{
$user = \App\Models\User::factory()->create();
$this->assertDatabaseHas('users', [
'email' => $user->email,
]);
}
This test creates a user using the factory and then checks if the user exists in the database.
Installing and Managing Composer Packages
Composer is a dependency manager for PHP that allows you to manage libraries and packages in your Laravel project. It simplifies the process of adding, updating, and removing packages for your application.
Installing Packages with Composer
To install a new package, run the following command:
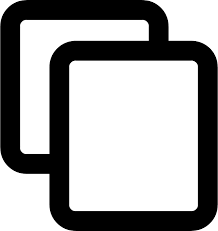
composer require vendor/package
For example, to install the guzzlehttp/guzzle
package, use:
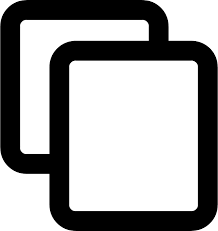
composer require guzzlehttp/guzzle
Updating Composer Packages
To update all your installed packages to their latest versions, use:
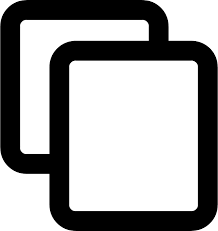
composer update
Removing Packages
If you no longer need a package, you can remove it using the following command:
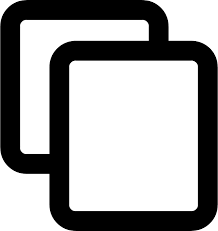
composer remove vendor/package
Popular Laravel Packages (Spatie, Laravel Debugbar, etc.)
Laravel has a rich ecosystem of third-party packages that can help you extend the functionality of your application. Below are some of the most popular Laravel packages:
Spatie Packages
Spatie is a well-known company that offers a variety of highly useful Laravel packages. Some of their most popular packages include:
- Spatie Laravel Permission: A package for handling role-based permissions in Laravel.
- Spatie Media Library: A package for associating files and images with your Eloquent models.
- Spatie Backup: A package for backing up your application, including database and files.
To install Spatie's packages, use composer require spatie/package-name
. For example:
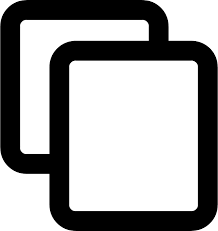
composer require spatie/laravel-permission
Laravel Debugbar
Laravel Debugbar is a package that provides a developer toolbar for debugging Laravel applications. It displays detailed information about your application, including routes, queries, and more.
To install Laravel Debugbar, run:
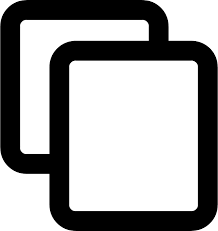
composer require barryvdh/laravel-debugbar --dev
Once installed, you can enable or disable it by setting the DEBUGBAR_ENABLED
option in your .env
file.
Creating Custom Laravel Packages
Laravel makes it easy to create your own packages for reusable functionality. A custom Laravel package can contain controllers, routes, views, and other components that you can easily share across different projects.
Creating a New Package
To create a custom package, follow these steps:
- Create a new directory for your package inside the
packages
folder in your Laravel project. - Inside the package directory, create a service provider class that will register your package's functionality:
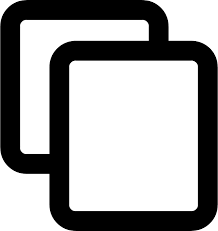
namespace Vendor\PackageName;
use Illuminate\Support\ServiceProvider;
class PackageServiceProvider extends ServiceProvider
{
public function register()
{
// Register your package services here
}
public function boot()
{
// Bootstrapping code for your package
}
}
Next, register the service provider in the config/app.php
file, inside the providers
array:
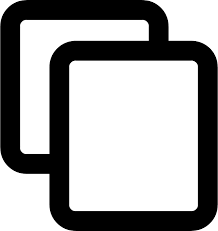
'providers' => [
// Other Service Providers
Vendor\PackageName\PackageServiceProvider::class,
],
Publishing Package Assets
If your package includes assets like configuration files, views, or migrations, you can publish them to the Laravel application using Artisan:
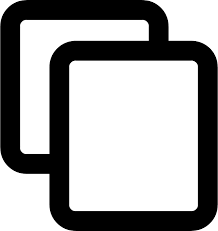
php artisan vendor:publish --provider="Vendor\PackageName\PackageServiceProvider"
Package Autoloading
To ensure that your package’s classes are autoloaded, you need to configure the composer.json
file of your package:
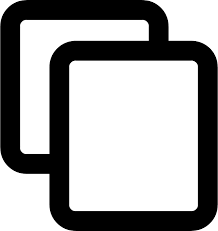
{
"autoload": {
"psr-4": {
"Vendor\\PackageName\\": "src/"
}
}
}
After updating composer.json
, run the following command to regenerate the autoload files:
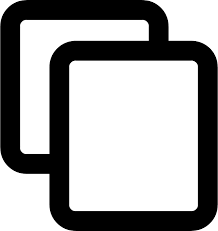
composer dump-autoload
Protecting Against CSRF Attacks
Cross-Site Request Forgery (CSRF) is an attack where a malicious user can trick a victim into making unwanted requests on a site where they are authenticated. Laravel includes CSRF protection by default in all of its routes that use the web
middleware group.
CSRF Token
Laravel automatically generates a CSRF token for each active user session. This token is used to verify that the requests made to the server are legitimate. To include the CSRF token in your forms, use the @csrf
directive:
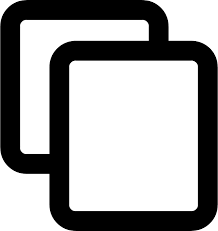
Excluding Routes from CSRF Protection
If you need to exclude certain routes from CSRF protection (e.g., for APIs), you can add them to the VerifyCsrfToken
middleware in the app/Http/Middleware/VerifyCsrfToken.php
file:
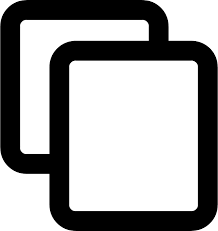
protected $except = [
'api/*',
];
Hashing Passwords with bcrypt or argon2
Laravel provides an easy way to securely hash user passwords using either bcrypt or argon2. Password hashing ensures that sensitive information is stored securely.
Hashing with bcrypt
To hash a password using bcrypt
, use Laravel's built-in Hash
facade:
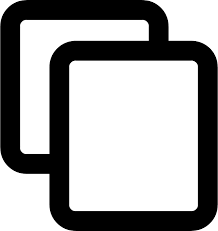
use Illuminate\Support\Facades\Hash;
$hashedPassword = Hash::make('secret');
Hashing with argon2
Laravel also supports argon2
hashing for added security. To use argon2
, you can configure it in the config/hashing.php
file:
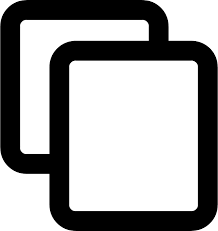
'driver' => 'argon',
Verifying a Password
To verify a password against a hash, use:
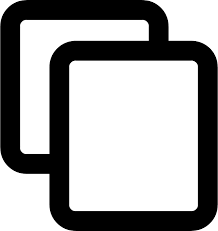
if (Hash::check('secret', $hashedPassword)) {
// The passwords match
}
Preventing XSS and SQL Injection
Laravel offers built-in mechanisms to prevent common security vulnerabilities like Cross-Site Scripting (XSS) and SQL Injection.
Preventing XSS
Laravel automatically escapes data in views to prevent XSS attacks. To display raw HTML, use the {!! !!} syntax:
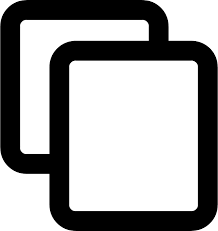
{{-- Safe --}}
{{ $user->bio }}
{{-- Unsafe --}}
{!! $user->bio !!}
Preventing SQL Injection
Laravel’s Eloquent ORM and query builder use prepared statements, which protect against SQL injection attacks. For example:
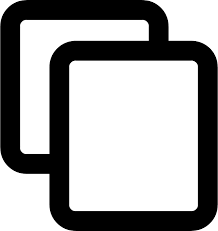
// Safe
$users = DB::table('users')->where('name', '=', $name)->get();
Never concatenate user inputs directly into SQL queries. Always use parameterized queries or Eloquent's query builder to avoid SQL injection vulnerabilities.
Using Rate Limiting for Brute Force Protection
Laravel includes built-in support for rate limiting, which can help prevent brute-force attacks on routes like login or password reset. You can limit the number of requests a user can make within a given time period.
Rate Limiting with Throttles
Laravel uses the ThrottleRequests
middleware to limit the number of requests. To apply throttling to a route, use the throttle
middleware:
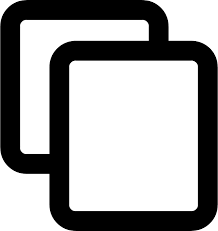
Route::post('login', 'AuthController@login')->middleware('throttle:5,1');
This will limit users to 5 requests per minute. If they exceed this limit, they will receive a 429 Too Many Requests
response.
Customizing Rate Limits
You can customize rate limits in the RouteServiceProvider
using the RateLimiter
facade:
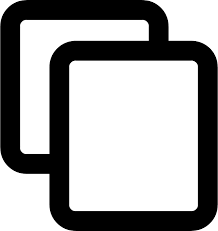
use Illuminate\Cache\RateLimiter;
public function boot(RateLimiter $rateLimiter)
{
$rateLimiter->for('login', function (Request $request) {
return Limit::perMinute(5);
});
}
This allows you to define custom rate-limiting strategies for different routes or actions.
Adding Localization and Translations
Localization allows you to build applications that can be translated into different languages. Laravel provides an easy way to handle localization, enabling you to create multilingual applications.
Setting Up Localization
To enable localization in Laravel, you need to configure language files in the resources/lang
directory. Laravel comes with a default language (English) located in resources/lang/en
.
To add additional languages, simply create a new folder under resources/lang
(e.g., fr
for French). Inside this folder, you can create translation files for different components of your application.
Example
For French localization, create a file resources/lang/fr/messages.php
with the following content:
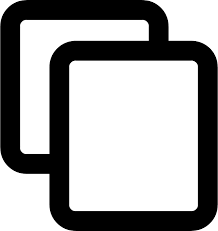
return [
'welcome' => 'Bienvenue sur notre site',
];
Now, you can switch the language in the application by setting the locale using the App::setLocale
method:
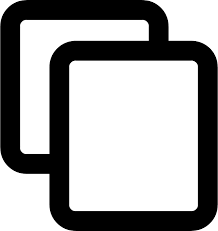
use Illuminate\Support\Facades\App;
App::setLocale('fr');
Using the trans() and Lang::get() Methods
Laravel provides two methods for retrieving translations: trans()
and Lang::get()
. Both methods work similarly, but trans()
is the preferred method in recent versions of Laravel.
Using trans() Method
The trans()
method retrieves a translated string from the language files:
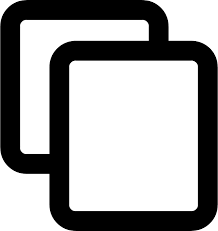
echo trans('messages.welcome');
This will print "Bienvenue sur notre site" if the locale is set to French, as defined in the previous section.
Using Lang::get() Method
The Lang::get()
method works similarly to trans()
:
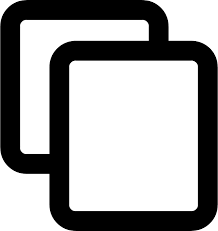
use Illuminate\Support\Facades\Lang;
echo Lang::get('messages.welcome');
Both methods are interchangeable, but trans()
is the recommended approach in modern Laravel applications.
Managing Language Files in Laravel
Laravel stores language translations in files located in the resources/lang
directory. Each language has its own folder, and within these folders, translation files are stored in a key-value format.
Creating Language Files
You can create any number of language files in the resources/lang/{locale}
directory. For example, for French, you can create resources/lang/fr/messages.php
and add key-value pairs for translations.
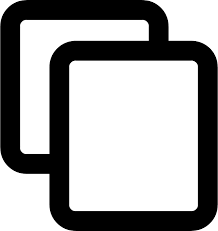
return [
'login' => 'Se connecter',
'register' => 'S\'inscrire',
];
Accessing Translations in Views
In your views, you can access the translation strings like this:
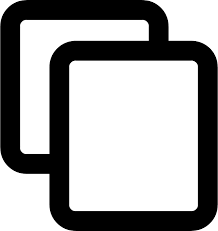
{{ __('messages.login') }}
Handling Missing Translations
If a translation string is missing, Laravel will fall back to the default language (usually English). You can handle missing translations by customizing the resources/lang/{locale}/validation.php
file.
Additionally, you can add custom fallback logic in config/app.php
:
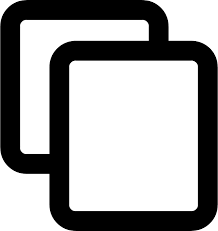
'fallback_locale' => 'en',
Using Laravel Mix for Asset Compilation
Laravel Mix is a powerful tool for compiling and optimizing assets such as JavaScript, CSS, and images. It provides a clean, fluent API for defining Webpack build steps.
Installing Laravel Mix
Laravel Mix is included by default in Laravel, but you need to install the required dependencies to get started:
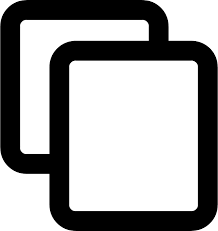
npm install
Configuring Laravel Mix
Laravel Mix is configured via the webpack.mix.js
file in the root of your project. For example, to compile your main app.js
and app.scss
, you can use the following:
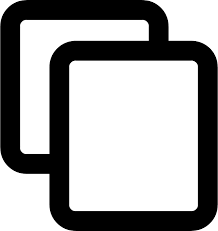
const mix = require('laravel-mix');
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css');
Running Laravel Mix
Once your webpack.mix.js
file is set up, you can run the following command to compile your assets:
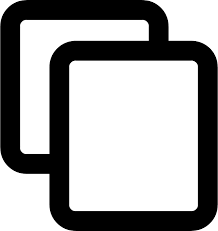
npm run dev
For production, you can optimize your assets with:
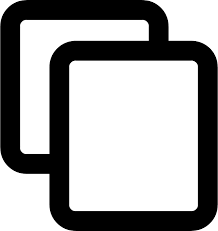
npm run prod
Event Broadcasting with Pusher and Laravel Echo
Event broadcasting allows you to broadcast events from your Laravel application to your frontend in real-time. Laravel Echo provides an easy-to-use API for listening to events.
Setting Up Pusher
First, create an account on Pusher and create a new app. Afterward, install the Pusher package in Laravel:
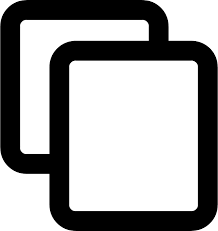
composer require pusher/pusher-php-server
Configuring Broadcasting
Update your .env
file with your Pusher credentials:
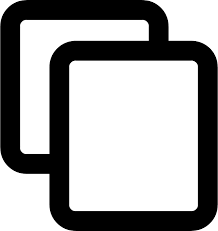
BROADCAST_DRIVER=pusher
PUSHER_APP_ID=your_app_id
PUSHER_APP_KEY=your_app_key
PUSHER_APP_SECRET=your_app_secret
PUSHER_APP_CLUSTER=your_app_cluster
Creating Events
Create an event class that will be broadcast:
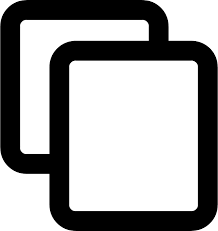
php artisan make:event MessageSent
Listening for Events
On the frontend, use Laravel Echo to listen for events broadcasted by Pusher. Install Laravel Echo:
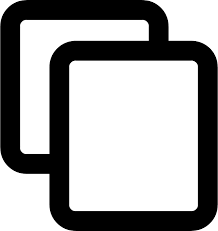
npm install --save laravel-echo pusher-js
Then, listen for the event in your JavaScript:
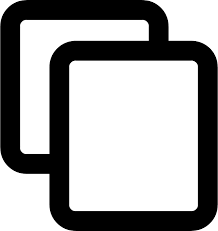
import Echo from 'laravel-echo';
window.Pusher = require('pusher-js');
const echo = new Echo({
broadcaster: 'pusher',
key: 'your-app-key',
cluster: 'your-app-cluster'
});
echo.channel('chat')
.listen('MessageSent', (event) => {
console.log(event.message);
});
Using Traits for Reusable Code
In Laravel, traits allow you to group reusable methods that can be included in multiple classes. Traits are a great way to avoid code duplication.
Creating a Trait
To create a trait, use the php artisan make:trait
command:
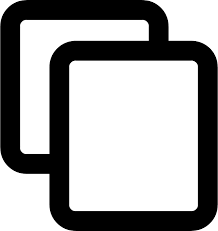
php artisan make:trait Logger
Inside the trait file app/Traits/Logger.php
, define methods you want to reuse:
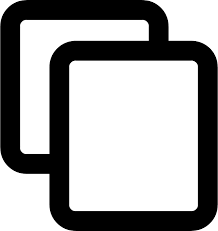
namespace App\Traits;
trait Logger
{
public function log($message)
{
\Log::info($message);
}
}
Using the Trait in Classes
To use the trait in your classes, simply include it at the top of your class:
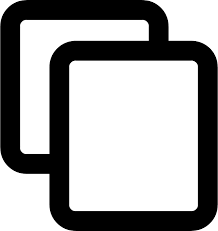
use App\Traits\Logger;
class User
{
use Logger;
public function createUser($data)
{
$this->log('User created: ' . $data['name']);
}
}
Repository Pattern and Service Layer Architecture
The Repository Pattern and Service Layer Architecture are design patterns that help to organize the application structure, reduce duplication, and improve maintainability.
Repository Pattern
The Repository Pattern abstracts the data layer, making it easier to switch between data sources (e.g., MySQL, MongoDB, etc.) without changing business logic. You can create a repository to handle data queries and operations.
Create a repository interface:
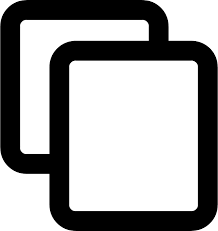
namespace App\Repositories;
interface UserRepositoryInterface
{
public function getAllUsers();
}
Then, implement the interface in a concrete repository class:
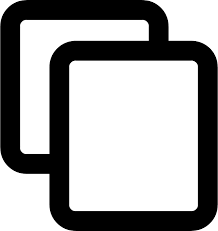
namespace App\Repositories;
use App\Models\User;
class UserRepository implements UserRepositoryInterface
{
public function getAllUsers()
{
return User::all();
}
}
Service Layer Architecture
The Service Layer pattern is used to define business logic in service classes. This helps to keep controllers clean and focused on handling HTTP requests.
Create a service class:
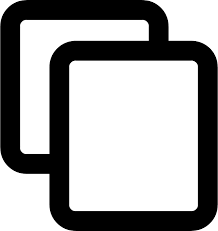
namespace App\Services;
class UserService
{
protected $userRepository;
public function __construct(UserRepositoryInterface $userRepository)
{
$this->userRepository = $userRepository;
}
public function getAllUsers()
{
return $this->userRepository->getAllUsers();
}
}
In your controller, you can inject the service to handle business logic:
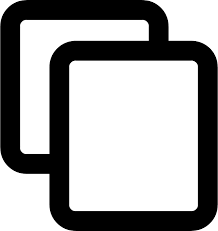
namespace App\Http\Controllers;
use App\Services\UserService;
class UserController extends Controller
{
protected $userService;
public function __construct(UserService $userService)
{
$this->userService = $userService;
}
public function index()
{
$users = $this->userService->getAllUsers();
return view('users.index', compact('users'));
}
}
Preparing a Laravel Project for Production
Before deploying your Laravel application to production, it is important to perform some key steps to ensure the application runs efficiently and securely.
Setting Up Environment Variables
Ensure your .env
file is properly configured for the production environment. Set the APP_ENV
and APP_DEBUG
values accordingly:
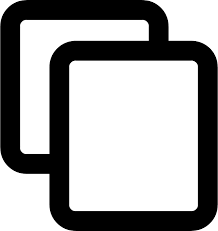
APP_ENV=production
APP_DEBUG=false
Running Artisan Commands
Run the following Artisan commands to optimize your application for production:
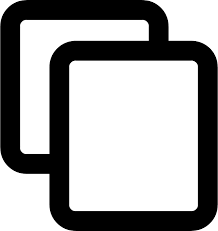
php artisan config:cache
php artisan route:cache
php artisan view:cache
php artisan optimize
Setting Proper Permissions
Ensure the storage
and bootstrap/cache
directories are writable by the web server:
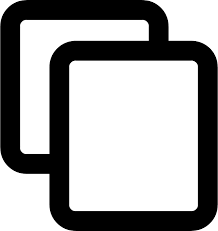
sudo chmod -R 775 storage bootstrap/cache
Using .env for Environment Configuration
The .env
file in Laravel is used to set environment variables for different environments (local, staging, production). It helps separate sensitive information like API keys and database credentials from the codebase.
Setting Up the .env File
In the .env
file, you can define various configuration values. For example:
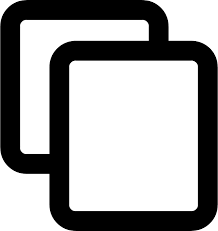
APP_NAME=Laravel
APP_ENV=local
APP_KEY=base64:yourkeyhere
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=yourdatabase
DB_USERNAME=root
DB_PASSWORD=rootpassword
Accessing Environment Variables
You can access these variables anywhere in your application using the env()
helper function:
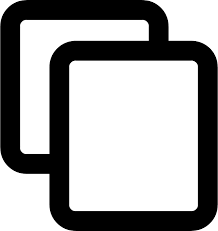
$dbHost = env('DB_HOST');
Environment-Specific Configuration
You can create different .env
files for local, staging, and production environments. Make sure to securely store sensitive production credentials.
Caching Routes, Config, and Views
Laravel provides caching mechanisms to improve application performance by storing routes, configuration, and views. These cached files are loaded faster than recalculating them every time.
Caching Routes
To cache your routes, run the following command:
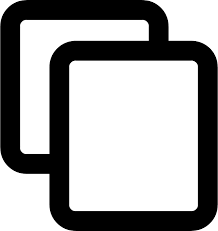
php artisan route:cache
This command will store a cached version of your routes, improving the application's performance. If you add new routes, you will need to clear and recache them:
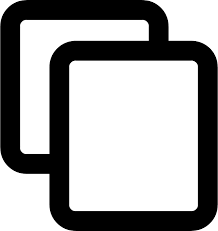
php artisan route:clear
php artisan route:cache
Caching Configuration
Laravel allows you to cache your configuration files with the following command:
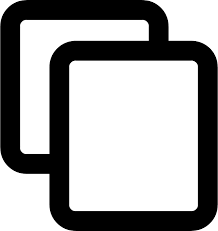
php artisan config:cache
Caching Views
To cache your views, use the following command:
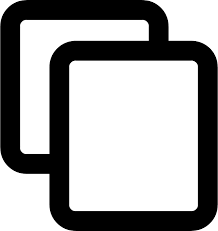
php artisan view:cache
Optimizing Laravel for Speed and Performance
Optimizing Laravel applications for speed and performance is essential for handling large traffic loads and ensuring a smooth user experience.
Use Eager Loading
When dealing with relationships in Eloquent, eager loading helps load related models upfront, avoiding the N+1 query problem:
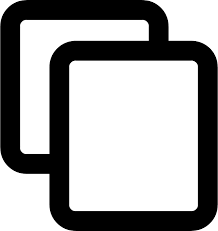
$posts = Post::with('comments')->get();
Use Database Indexes
Ensure that your database tables are properly indexed to improve query performance. For example, index foreign key columns:
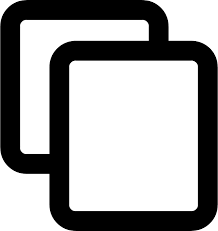
Schema::table('posts', function (Blueprint $table) {
$table->index('user_id');
});
Minimize Database Queries
Avoid unnecessary database queries by optimizing your logic and using the select()
method to retrieve only the necessary columns:
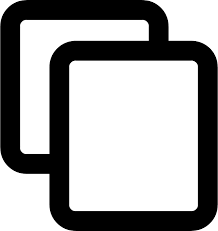
$users = User::select('id', 'name')->get();
Enable Query Logging
Enable query logging to debug slow queries, and analyze them for optimization:
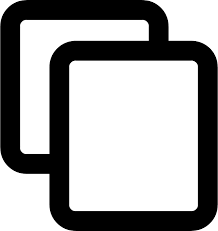
DB::enableQueryLog();
$users = User::all();
dd(DB::getQueryLog());
Use Redis for Caching
Laravel supports caching with Redis, which is faster for data storage compared to file-based caching:
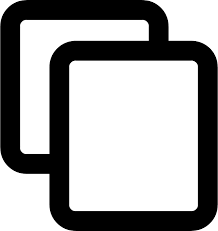
Cache::store('redis')->put('key', 'value', 10);