-Logo.png)
What is R?
R is a programming language and software environment designed specifically for statistical computing, data analysis, and graphical representation. Developed by Ross Ihaka and Robert Gentleman in the mid-1990s, R has become a cornerstone in the field of data science due to its flexibility, open-source nature, and the vast ecosystem of packages available for diverse analytical tasks.
History of R
The R language is an implementation of the S programming language, which was developed at Bell Laboratories. R was created as an open-source alternative to S, providing a free and extensible platform for statisticians, data analysts, and researchers to perform data manipulation, statistical modeling, and visualization. Over the years, R has grown into one of the most popular languages in data science, supported by an active community and a rich collection of libraries.
R Features
Below are the key features that make R a powerful choice for data analysis and statistical computing:
Feature | Description |
---|---|
Statistical Computing | R offers a wide range of statistical techniques, including linear and nonlinear modeling, time-series analysis, classification, clustering, and more. |
Data Visualization | R excels in creating high-quality, customizable graphs and plots using libraries like ggplot2 and lattice. |
Extensibility | R is highly extensible, allowing users to create their own functions and packages, or install those created by the community. |
Open Source | R is free to use, modify, and distribute, making it accessible to individuals and organizations worldwide. |
Platform Independent | R runs on a variety of platforms, including Windows, macOS, and Linux, ensuring compatibility and flexibility. |
Setting Up R
Before using R, you need to install it on your system. Follow these steps to set up R:
- Download the R installer from the official R website.
- Run the installer and follow the on-screen instructions to complete the installation.
- Optionally, install RStudio, a popular IDE for R, from the RStudio website.
- After installation, open the R console or RStudio to start working with R.
Code Example: Basic Arithmetic in R
Here’s a simple example of performing arithmetic operations in R:
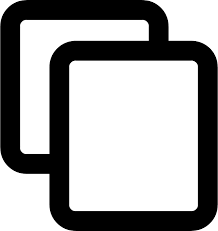
# Basic Arithmetic in R
# Addition
result_add <- 5 + 3
print(result_add) # Output: 8
# Multiplication
result_multiply <- 6 * 7
print(result_multiply) # Output: 42
Diagram: R Workflow
The following diagram provides an overview of a typical workflow in R, from data import to analysis and visualization:

In this workflow, data is imported, cleaned, and analyzed using R’s rich suite of functions and visualized using its powerful plotting capabilities.
Features and Benefits of R Programming
R programming offers a robust set of features and numerous benefits that make it an ideal choice for statistical computing, data analysis, and data visualization. Below, we outline the core features and the advantages of using R for various applications:
Core Features of R Programming
The following features highlight what makes R programming unique:
Feature | Description |
---|---|
Comprehensive Statistical Analysis | R provides a wide variety of statistical techniques, such as regression models, hypothesis testing, and time-series analysis. |
Rich Visualization Tools | R excels in creating high-quality visualizations, including custom plots, graphs, and charts, through libraries like ggplot2 and lattice. |
Extensibility with Packages | R's functionality can be extended using thousands of packages available on CRAN (Comprehensive R Archive Network) and other repositories. |
Interactive Data Analysis | R's interactive environment allows users to analyze data step-by-step and make changes on the fly. |
Cross-Platform Compatibility | R is platform-independent and runs seamlessly on Windows, macOS, and Linux. |
Integration with Other Tools | R integrates well with other programming languages like Python, C++, and Java, as well as with databases and web applications. |
Key Benefits of R Programming
R provides several advantages that make it a preferred choice for data scientists, statisticians, and researchers:
- Open Source: R is free to use, modify, and distribute, which makes it accessible to individuals and organizations with limited budgets.
- Strong Community Support: R has a large, active community that contributes packages, tutorials, and documentation, ensuring continuous development and support.
- Ideal for Data Science: R is tailored for statistical computing and data visualization, making it a go-to tool for data scientists and analysts.
- Customizable: Users can create custom functions and libraries to address specific analysis needs.
- Advanced Visualization Capabilities: R can produce publication-quality visualizations that are customizable and aesthetically pleasing.
- Wide Range of Applications: R is used in industries ranging from finance and healthcare to academia and government for tasks such as predictive modeling, bioinformatics, and market analysis.
Code Example: Installing and Using Packages in R
One of R's strengths is its extensibility through packages. Here’s an example of installing and using a package:
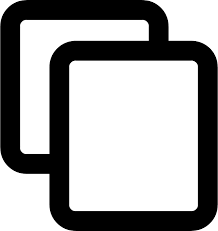
# Installing and Loading the ggplot2 Package
# Install the ggplot2 package
install.packages("ggplot2")
# Load the ggplot2 library
library(ggplot2)
# Create a simple scatter plot
data(mtcars)
ggplot(mtcars, aes(x = wt, y = mpg)) +
geom_point() +
labs(title = "Scatter Plot of Weight vs. MPG",
x = "Weight (1000 lbs)",
y = "Miles per Gallon")
Diagram: R Ecosystem
The diagram below illustrates the R ecosystem, showcasing its integration with various tools and its wide range of applications:

This ecosystem demonstrates R's ability to handle diverse tasks such as data import, analysis, visualization, and reporting.
Installing R and RStudio (Windows, macOS, Linux)
R and RStudio are essential tools for statistical computing and data visualization. While R is the programming language, RStudio provides a user-friendly Integrated Development Environment (IDE) for working with R. Follow the steps below to install R and RStudio on your system:
Step 1: Install R
To begin, you need to install R, which is the core programming language. Here’s how:
Operating System | Steps to Install |
---|---|
Windows |
|
macOS |
|
Linux |
|
Step 2: Install RStudio
Once R is installed, you can install RStudio, a powerful IDE for R:
- Visit the RStudio website.
- Download the free version of RStudio Desktop suitable for your operating system.
- Run the installer and follow the on-screen instructions.
- Launch RStudio after installation to start coding in R.
Verifying Installation
After installing R and RStudio, verify that everything is set up correctly:
- Open RStudio.
- In the R console, type the following command to check the installed R version:
version
- The output will display the R version and other details, confirming a successful installation.
Code Example: Running Your First R Script
Once everything is installed, you can run your first R script in RStudio:
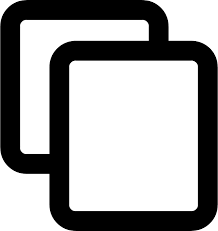
# Print a greeting message
print("Hello, R and RStudio are successfully installed!")
Diagram: Installation Workflow
The following diagram illustrates the workflow for installing R and RStudio:

With R and RStudio installed, you are now ready to explore the power of R programming for data analysis and visualization.
Setting Up Your First R Script
After installing R and RStudio, you can write and run your first R script. RStudio provides an intuitive interface for creating, saving, and executing scripts. Follow the steps below to set up and execute your first R script:
Step 1: Open RStudio
Launch RStudio from your desktop or applications menu. The RStudio interface has several key panels:
- Console: Where commands are executed immediately.
- Source Editor: Where you write and edit scripts.
- Environment/History: Displays variables and command history.
- Plots/Files/Packages: Displays plots, file directories, and installed packages.
Step 2: Create a New Script
To create a new R script:
- Click on File in the menu bar.
- Select New File > R Script or press
Ctrl + Shift + N
(Cmd + Shift + N
on macOS). - A new script editor tab will open where you can write your R code.
Step 3: Write Your First R Code
Write the following code in the script editor to calculate the sum of two numbers and print a message:
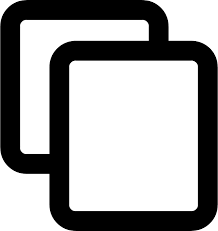
# This is your first R script
# Calculate the sum of two numbers
a <- 5
b <- 10
sum <- a + b
# Print the result
print(paste("The sum of", a, "and", b, "is:", sum))
Step 4: Save Your Script
Before running your script, save it to your desired location:
- Click on File > Save As.
- Choose a directory and give your script a name, such as
first_script.R
. - Click Save.
Step 5: Run Your Script
To execute your script, use one of the following methods:
- Select the line(s) of code and press
Ctrl + Enter
(Cmd + Enter
on macOS). - Click the Run button in the editor toolbar.
- Use Source to run the entire script at once.
The output of your script will appear in the Console panel.
Code Output Example
After running the above script, the console will display:
[1] "The sum of 5 and 10 is: 15"
Diagram: RStudio Interface
The following diagram highlights the key components of the RStudio interface:

Now you’re ready to create, save, and execute more complex R scripts as you explore the language further.
Understanding RStudio Interface
RStudio is a powerful integrated development environment (IDE) for R programming that provides a user-friendly interface for writing, running, and debugging R scripts. Here’s a breakdown of the main panels and features you’ll encounter in RStudio:
Key Components of the RStudio Interface
The RStudio interface is divided into four main panes, each serving a specific purpose:
Pane | Description |
---|---|
Source Editor | The area where you write and edit your R scripts (.R files). It supports syntax highlighting, code completion, and commenting features. You can also open multiple tabs for different scripts. |
Console | Displays the output of your R code. You can also execute individual commands directly in the console for quick tests or calculations. |
Environment/History | The Environment tab shows the objects (variables, data frames, functions) currently in memory. The History tab records all previously executed commands, which you can reuse. |
Files/Plots/Packages/Help | This pane provides access to file directories, visualization of plots, management of installed packages, and R’s extensive help system. |
Navigation Tips
Below are some quick tips for navigating and using the RStudio interface effectively:
- Run Code: Use
Ctrl + Enter
(Windows/Linux) orCmd + Enter
(macOS) to execute selected lines or the current line in the Source Editor. - Clear Console: Press
Ctrl + L
to clear the Console output. - View Variables: Click on any variable in the Environment tab to open it in a tabular viewer.
- Search Help: Use the Help pane or type
?function_name
in the Console to get documentation for any R function.
Diagram: RStudio Interface
The following diagram provides a visual layout of the RStudio interface, highlighting its key components:

Customizing RStudio
You can customize RStudio’s appearance and functionality to suit your preferences:
- Appearance: Go to Tools > Global Options > Appearance to change the theme and font size.
- Pane Layout: Rearrange the panes by going to Tools > Global Options > Panes.
Conclusion
The RStudio interface is designed to make R programming efficient and intuitive. By understanding its layout and features, you can streamline your workflow and focus on analyzing data and writing code effectively.
R Syntax and Basic Commands
R has a simple and intuitive syntax, making it accessible for beginners while being powerful for advanced users. This section introduces the fundamental elements of R syntax and some basic commands to get you started.
Basic Syntax Rules
- Case Sensitivity: R is case-sensitive, so
Variable
andvariable
are treated as different identifiers. - Assignment Operator: Use
<-
or=
to assign values to variables. The traditional assignment operator in R is<-
. - Comments: Use the
#
symbol to add comments to your code. Comments are ignored during execution.
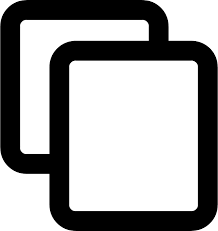
# Example of assignment
x <- 10 # Assigns the value 10 to x
y = 20 # Also assigns 20 to y
z <- x + y # Assigns the sum of x and y to z
# Example of a comment
# This is a single-line comment
Basic Commands
Below are some commonly used R commands to perform basic operations:
Command | Description |
---|---|
print() |
Displays the value of an object or result of an expression. |
c() |
Combines values into a vector. |
class() |
Returns the class (data type) of an object. |
length() |
Returns the length of a vector or list. |
str() |
Displays the internal structure of an object. |
summary() |
Provides a statistical summary of an object. |
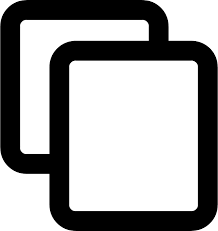
# Examples of basic commands
print("Hello, R!") # Prints a message
vec <- c(1, 2, 3, 4, 5) # Creates a vector
class(vec) # Displays the data type of vec
length(vec) # Returns the length of vec
str(vec) # Shows the structure of vec
summary(vec) # Provides a summary of vec
Mathematical Operations
R supports basic arithmetic operations directly:
+
Addition-
Subtraction*
Multiplication/
Division^
or**
Exponentiation%%
Modulus (remainder)%/%
Integer Division
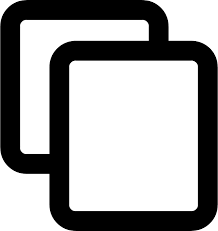
# Examples of mathematical operations
a <- 15
b <- 4
sum <- a + b # Addition
difference <- a - b # Subtraction
product <- a * b # Multiplication
quotient <- a / b # Division
exponentiation <- a^2 # Exponentiation
modulus <- a %% b # Modulus
integer_division <- a %/% b # Integer Division
Conclusion
Understanding R’s basic syntax and commands is the first step toward becoming proficient in R programming. Practice these commands and explore their variations to build a strong foundation for more advanced topics.
Variables and Data Types in R
In R, variables are used to store data values. Data types define the kind of data a variable can hold. R supports several common data types, including numeric, character, logical, and more. Understanding these data types is essential for effective programming in R.
Variables in R
Variables in R are created by simply assigning a value to a name. The assignment operator in R is <-
, but =
can also be used. The variable name should start with a letter and can include numbers, dots, and underscores.
Example of variable assignment:
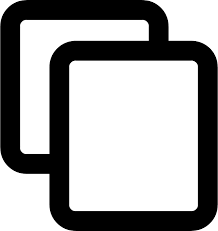
# Variable assignment in R
x <- 10 # Numeric variable
name <- "John" # Character variable
is_active <- TRUE # Logical variable
Data Types in R
R supports several types of data, each used for different kinds of information. The primary data types in R are:
Data Type | Description |
---|---|
Numeric | Used for numbers, either integers or decimal values. The default data type for numbers in R is numeric. |
Character | Used for text or string data. Character data is enclosed in either single or double quotes. |
Logical | Used for boolean values. A logical variable can hold values TRUE or FALSE . |
Complex | Used for complex numbers with real and imaginary parts. For example, 3 + 2i . |
Integer | Used to represent integer values. You can specify an integer by appending L to the number (e.g., 5L ). |
Examples of Data Types in R
Below are examples of how different data types are used in R:
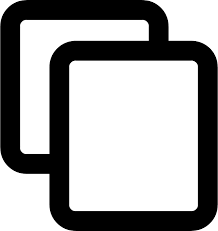
# Numeric example
num_var <- 42 # Numeric variable
# Character example
char_var <- "Hello, R!" # Character variable
# Logical example
logical_var <- TRUE # Logical variable
# Complex example
complex_var <- 3 + 4i # Complex number
# Integer example
int_var <- 10L # Integer variable
Type Checking in R
R provides functions to check the data type of a variable:
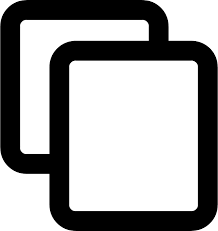
# Check the type of a variable
typeof(num_var) # Output: "double" (numeric)
typeof(char_var) # Output: "character"
typeof(logical_var) # Output: "logical"
typeof(complex_var) # Output: "complex"
typeof(int_var) # Output: "integer"
Conclusion
Variables and data types are foundational concepts in R programming. Understanding how to define and manipulate different data types enables you to write efficient and effective R code for statistical analysis, data visualization, and more.
Input and Output Functions in R (readline(), print())
In R, input and output functions are used to interact with users, display results, and collect data. The two most commonly used functions for input and output in R are readline()
for input and print()
for output.
Input in R: readline()
The readline()
function is used to capture user input from the console. It allows the user to enter a value, which can then be stored in a variable for further use. The input is always returned as a character string, so you may need to convert it to other data types (e.g., numeric or logical) as needed.
Example of using readline()
to get user input:
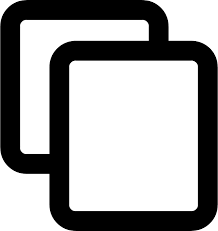
# Using readline() to get user input
name <- readline(prompt = "Enter your name: ")
age <- readline(prompt = "Enter your age: ")
# Displaying the input values
cat("Hello,", name, "you are", age, "years old.\n")
In the example above, readline()
is used to capture the user's name and age. The cat()
function is used to display the output, as it allows for more flexible formatting than print()
.
Output in R: print()
The print()
function is used to display values or expressions on the console. It is one of the most commonly used output functions in R and is useful for debugging, displaying results, or simply showing information to the user.
Example of using print()
to display a message:
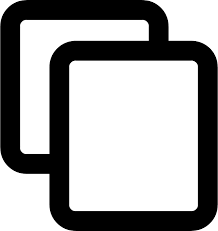
# Using print() to display output
message <- "Welcome to R programming!"
print(message)
In the example above, print()
outputs the value of the message
variable to the console.
Converting Input Data Types
Since readline()
always returns a character string, you may need to convert the input to a different data type such as numeric or logical. You can use functions like as.numeric()
, as.integer()
, or as.logical()
to perform these conversions.
Example of converting user input to numeric:
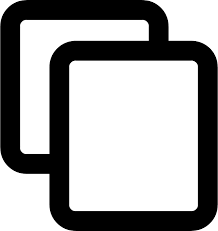
# Getting numeric input from the user
num1 <- as.numeric(readline(prompt = "Enter a number: "))
num2 <- as.numeric(readline(prompt = "Enter another number: "))
# Performing arithmetic operation
sum <- num1 + num2
print(paste("The sum is:", sum))
Best Practices for Input and Output Functions
- Use
readline()
for text-based input and provide clear prompts for users. - Convert inputs to the appropriate data type (e.g., numeric, integer) to avoid errors in calculations.
- Use
print()
to display simple outputs andcat()
for more complex or formatted outputs. - Ensure that the output is informative and user-friendly, especially when debugging or displaying results to users.
Conclusion
Input and output functions are essential for interacting with users and displaying results in R. By effectively using readline()
and print()
, you can create dynamic programs that respond to user input and provide useful feedback.
Vectors: Creation, Indexing, and Operations in R
In R, vectors are one of the most basic and essential data structures. A vector is an ordered collection of elements, all of the same type (numeric, character, logical, etc.). Vectors are used extensively for data manipulation, mathematical computations, and more in R programming.
Creating Vectors in R
Vectors can be created using the c()
function, which combines elements into a vector. You can create numeric, character, and logical vectors using this function. Other functions like seq()
and rep()
can also be used to generate vectors.
Examples of creating vectors:
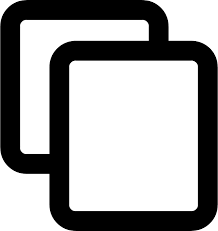
# Creating a numeric vector
num_vector <- c(1, 2, 3, 4, 5)
# Creating a character vector
char_vector <- c("apple", "banana", "cherry")
# Creating a logical vector
logical_vector <- c(TRUE, FALSE, TRUE)
# Creating a sequence of numbers using seq()
seq_vector <- seq(1, 10, by = 2)
# Creating a vector with repeated values using rep()
rep_vector <- rep(3, times = 5) # Repeats the number 3, 5 times
Indexing Vectors in R
Vector elements can be accessed using indices. In R, indexing starts at 1, meaning the first element of a vector has an index of 1. You can use square brackets []
to access elements by their index.
Examples of indexing vectors:
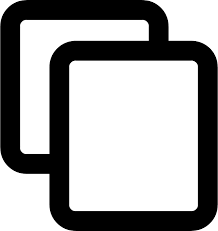
# Accessing the first element of num_vector
first_element <- num_vector[1]
# Accessing a range of elements (index 2 to 4)
sub_vector <- num_vector[2:4]
# Accessing elements using logical indexing
logical_index <- num_vector[c(TRUE, FALSE, TRUE, FALSE, TRUE)] # First, third, and fifth elements
Vector Operations in R
R supports vectorized operations, meaning you can perform mathematical operations on vectors element-wise without using loops. You can apply arithmetic, comparison, and logical operations directly to vectors.
Examples of vector operations:
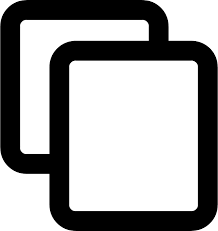
# Arithmetic operations on numeric vectors
sum_vector <- num_vector + 5 # Adds 5 to each element of num_vector
product_vector <- num_vector * 2 # Multiplies each element of num_vector by 2
# Performing element-wise comparison
comparison_vector <- num_vector > 3 # Returns TRUE for elements greater than 3
# Logical operations
logical_and <- logical_vector & c(TRUE, TRUE, FALSE) # Element-wise logical AND
logical_or <- logical_vector | c(FALSE, FALSE, TRUE) # Element-wise logical OR
Combining Vectors
You can combine vectors using functions like c()
or by appending elements at the end of an existing vector. You can also concatenate vectors to form a larger vector.
Example of combining vectors:
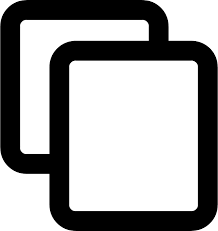
# Combining two vectors
combined_vector <- c(num_vector, seq_vector)
# Appending elements to an existing vector
extended_vector <- c(num_vector, 6, 7, 8)
Best Practices for Working with Vectors
- Ensure that all elements in a vector are of the same type (e.g., all numeric, all character). If elements of different types are combined, R will automatically coerce them to a common type.
- Use vectorized operations to perform calculations efficiently instead of writing explicit loops.
- Use appropriate indexing to access and manipulate elements. Avoid using loops for simple indexing tasks.
- Consider using functions like
length()
,sum()
, andmean()
for common operations on vectors.
Conclusion
Vectors are a fundamental data structure in R and play a critical role in data analysis and manipulation. Understanding how to create, index, and perform operations on vectors is essential for effective programming in R.
Matrices: Creation, Manipulation, and Matrix Algebra in R
In R, matrices are two-dimensional arrays with elements of the same data type. Matrices are useful for mathematical computations, data analysis, and storing structured data. R provides a variety of functions for creating, manipulating, and performing algebraic operations on matrices.
Creating Matrices in R
Matrices in R can be created using the matrix()
function. You need to specify the data to fill the matrix, the number of rows and columns, and optionally, whether to fill the matrix by row or by column.
Examples of creating matrices:
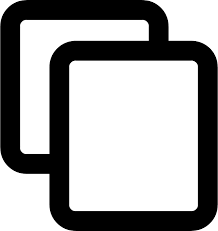
# Creating a matrix with 3 rows and 3 columns, filled by column
matrix1 <- matrix(1:9, nrow = 3, ncol = 3, byrow = FALSE)
# Creating a matrix with 2 rows and 4 columns, filled by row
matrix2 <- matrix(1:8, nrow = 2, ncol = 4, byrow = TRUE)
# Creating a matrix with named rows and columns
matrix3 <- matrix(1:6, nrow = 2, ncol = 3, dimnames = list(c("Row1", "Row2"), c("Col1", "Col2", "Col3")))
Manipulating Matrices in R
Once a matrix is created, you can manipulate it by accessing specific elements, rows, or columns. You can also modify matrix elements and perform operations on entire rows and columns.
Examples of matrix manipulation:
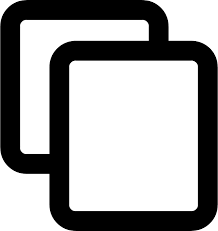
# Accessing a specific element (row 2, column 3)
element <- matrix1[2, 3]
# Accessing an entire row (row 1)
row1 <- matrix1[1, ]
# Accessing an entire column (column 2)
col2 <- matrix1[, 2]
# Modifying an element (changing element in row 2, column 3)
matrix1[2, 3] <- 10
# Adding a new row to the matrix
new_row <- c(10, 11, 12)
matrix1 <- rbind(matrix1, new_row)
# Adding a new column to the matrix
new_col <- c(13, 14, 15)
matrix1 <- cbind(matrix1, new_col)
Matrix Algebra in R
R supports matrix algebra, including matrix addition, subtraction, multiplication, and inversion. These operations are typically element-wise or matrix-specific, depending on the function used.
Examples of matrix algebra:
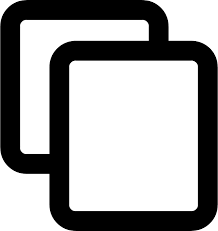
# Matrix addition (element-wise addition)
matrix_sum <- matrix1 + matrix2
# Matrix subtraction (element-wise subtraction)
matrix_diff <- matrix1 - matrix2
# Matrix multiplication (using the %*% operator)
matrix_prod <- matrix1 %*% matrix2
# Element-wise multiplication (using the * operator)
matrix_elem_prod <- matrix1 * matrix2
# Matrix transpose
matrix_transpose <- t(matrix1)
# Matrix inversion (only for square matrices)
matrix_inv <- solve(matrix1)
Best Practices for Working with Matrices
- Ensure that the matrix dimensions are consistent when performing operations like addition, subtraction, and multiplication.
- Always check for matrix compatibility before performing matrix multiplication (
%*%
operator). - Use
dim()
andnrow()
/ncol()
functions to check matrix dimensions. - For non-square matrices, functions like
solve()
should be used with caution as they may not always work.
Conclusion
Matrices are a powerful tool for performing complex mathematical operations and structuring data in R. Understanding how to create, manipulate, and perform matrix algebra will help you solve a wide range of mathematical and data analysis problems in R.
Lists: Combining Different Types of Data in R
In R, lists are versatile data structures that can store elements of different types, including numeric, character, logical, and even other lists. Unlike vectors, where elements must be of the same data type, lists allow you to combine various data types in a single object, making them highly useful for complex data manipulation.
Creating Lists in R
Lists in R can be created using the list()
function. You can include any type of object in a list, such as vectors, matrices, data frames, and other lists.
Examples of creating lists:
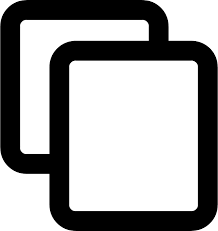
# Creating a simple list with different data types
my_list <- list(name = "John", age = 25, scores = c(85, 90, 88), is_active = TRUE)
# Creating a list with a matrix, a vector, and a character string
matrix1 <- matrix(1:9, nrow = 3)
my_complex_list <- list(matrix = matrix1, vector = c(1, 2, 3), message = "Hello, R!")
# Creating a nested list
nested_list <- list(name = "Alice", details = list(age = 30, city = "New York", languages = c("English", "Spanish")))
Accessing Elements of a List
To access elements in a list, you can use either the $
operator or double square brackets [[]]
. The $
operator is typically used for named elements, while [[]]
can be used for both named and unnamed elements.
Examples of accessing list elements:
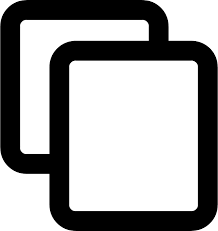
# Accessing an element by name using the $ operator
name_value <- my_list$name
# Accessing an element by position using [[ ]] operator
age_value <- my_list[[2]]
# Accessing a nested element from a nested list
city_name <- nested_list$details$city
Modifying Lists
Lists can be modified by assigning new values to their elements. You can also add new elements to a list or remove elements.
Examples of modifying lists:
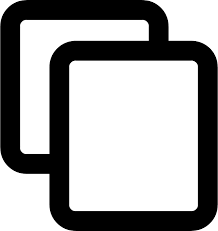
# Modifying an element in the list
my_list$age <- 26
# Adding a new element to the list
my_list$country <- "USA"
# Removing an element from the list
my_list$country <- NULL
List Operations
Although lists do not support the same operations as vectors or matrices, you can still perform some useful operations such as appending, combining, and applying functions to list elements.
Examples of list operations:
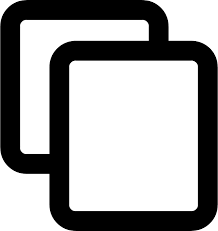
# Combining two lists
list1 <- list(a = 1, b = 2)
list2 <- list(c = 3, d = 4)
combined_list <- c(list1, list2)
# Applying a function to all elements in a list
squared_list <- lapply(my_list$scores, function(x) x^2)
# Checking the length of a list
list_length <- length(my_list)
Best Practices for Working with Lists
- Use the
$
operator for named elements when you know the element's name. For unnamed elements, use[[]]
to access them by index. - Keep in mind that lists are more flexible than vectors, but they can be slower for simple operations due to their heterogeneous nature.
- Consider using
sapply()
orlapply()
functions to apply operations across list elements, especially when working with nested lists. - Ensure proper naming of list elements for better readability and access. Using descriptive names can help you avoid confusion when working with complex lists.
Conclusion
Lists in R are a powerful data structure that allows you to store and manipulate data of different types in a single object. They are especially useful when working with more complex data structures and hierarchical data, such as nested lists. Mastering list creation, access, modification, and operations will enhance your ability to work with R efficiently.
Data Frames: Working with Tabular Data in R
Data frames are one of the most commonly used data structures in R for handling tabular data, such as spreadsheets or relational databases. A data frame is a list of vectors of equal length, where each vector represents a column, and all columns have the same number of rows. Data frames allow you to store and manipulate data efficiently and are suitable for a wide range of data analysis tasks.
Creating Data Frames in R
Data frames can be created using the data.frame()
function. You can include vectors of different data types (numeric, character, logical) as columns in the data frame.
Examples of creating data frames:
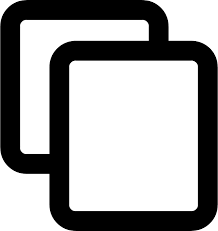
# Creating a simple data frame
my_data <- data.frame(
name = c("John", "Alice", "Bob"),
age = c(25, 30, 22),
scores = c(85, 92, 78),
is_active = c(TRUE, FALSE, TRUE)
)
# Creating a data frame with different column types
employee_data <- data.frame(
ID = c(101, 102, 103),
name = c("John", "Alice", "Bob"),
salary = c(50000, 60000, 45000),
start_date = as.Date(c("2020-01-15", "2019-11-25", "2021-06-10"))
)
Accessing Elements of a Data Frame
You can access columns, rows, and individual elements in a data frame using various indexing methods. The $
operator is commonly used to access columns by name, and you can also use index-based access for rows and columns.
Examples of accessing data frame elements:
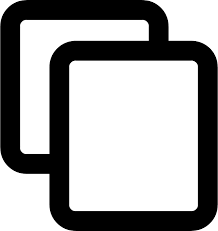
# Accessing a column by name
ages <- my_data$age
# Accessing a specific row by index (row 2)
row2 <- my_data[2, ]
# Accessing a specific element (row 2, column "scores")
score_bob <- my_data[2, "scores"]
# Accessing multiple columns by index (columns 1 and 3)
subset_data <- my_data[, c(1, 3)]
Modifying Data Frames
Data frames can be modified by assigning new values to individual elements, rows, or columns. You can also add new rows and columns to an existing data frame.
Examples of modifying data frames:
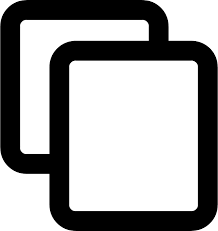
# Modifying an element (changing "age" of Bob)
my_data[2, "age"] <- 23
# Adding a new column to the data frame
my_data$gender <- c("Male", "Female", "Male")
# Adding a new row to the data frame
new_row <- data.frame(name = "Charlie", age = 28, scores = 91, is_active = TRUE, gender = "Male")
my_data <- rbind(my_data, new_row)
Subsetting Data Frames
You can subset data frames based on certain conditions using logical operators. This is useful for filtering rows that meet specific criteria.
Examples of subsetting data frames:
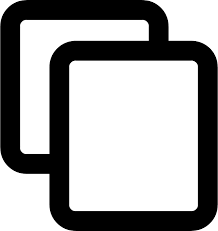
# Subsetting rows where "age" is greater than 25
older_than_25 <- my_data[my_data$age > 25, ]
# Subsetting rows where "is_active" is TRUE
active_users <- my_data[my_data$is_active == TRUE, ]
# Selecting specific columns using column names
selected_columns <- my_data[, c("name", "scores")]
Handling Missing Data in Data Frames
R provides several methods for handling missing data in data frames. You can identify missing values using the is.na()
function and remove or replace them as needed.
Examples of handling missing data:
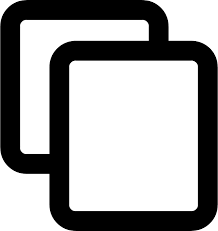
# Identifying missing values in a column
missing_values <- is.na(my_data$scores)
# Removing rows with missing values
clean_data <- na.omit(my_data)
# Replacing missing values with a specific value (e.g., replacing NA with 0)
my_data$scores[is.na(my_data$scores)] <- 0
Best Practices for Working with Data Frames
- Use descriptive column names to make your data frame more readable.
- Always check for missing values and handle them appropriately before performing analysis.
- When subsetting data frames, ensure that you understand how logical indexing works to avoid unexpected results.
- For large datasets, consider using the
dplyr
package for more efficient data manipulation, especially for filtering, summarizing, and transforming data.
Conclusion
Data frames are a fundamental data structure in R, and understanding how to work with them is essential for data manipulation and analysis. Whether you are dealing with small datasets or large, complex data, mastering data frames will help you perform a wide range of operations and analyses in R.
Factors: Handling Categorical Data in R
In R, factors are used to represent categorical data. Unlike regular character vectors, factors store both the unique categories (levels) and the actual data. Factors are essential when working with categorical variables, as they allow R to efficiently store and manipulate data while maintaining the integrity of the categories.
Creating Factors in R
Factors can be created using the factor()
function. By default, R will treat character vectors as factors when dealing with categorical data, but you can specify the levels (categories) explicitly if needed.
Examples of creating factors:
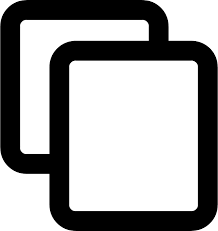
# Creating a factor from a character vector
gender <- factor(c("Male", "Female", "Male", "Female", "Male"))
# Creating a factor with specified levels
education <- factor(c("High School", "Bachelor", "Master", "PhD", "Bachelor"),
levels = c("High School", "Bachelor", "Master", "PhD"))
# Creating an ordered factor (ordinal data)
rating <- factor(c("Good", "Excellent", "Average", "Good", "Poor"),
levels = c("Poor", "Average", "Good", "Excellent"), ordered = TRUE)
Accessing Factors and Their Levels
You can access the levels of a factor, as well as the underlying numeric codes assigned to the factor levels, using the levels()
and as.numeric()
functions.
Examples of accessing factors and their levels:
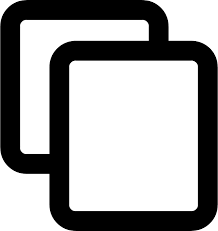
# Accessing the levels of a factor
levels(gender)
# Accessing the underlying numeric codes of a factor
num_codes <- as.numeric(gender)
# Accessing the levels of an ordered factor
levels(rating)
# Accessing the numeric codes of an ordered factor
rating_codes <- as.numeric(rating)
Modifying Factors
Factors can be modified by changing their levels or by reordering them. You can also add new levels to a factor or remove existing ones if necessary.
Examples of modifying factors:
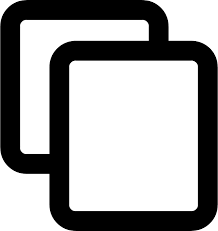
# Modifying the levels of a factor
education <- factor(education, levels = c("Bachelor", "Master", "PhD", "High School"))
# Adding new levels to a factor
gender <- factor(gender, levels = c("Male", "Female", "Non-binary"))
# Changing the level of a factor
gender[gender == "Non-binary"] <- "Other"
Using Factors in Data Frames
Factors are frequently used in data frames to represent categorical variables, particularly when working with survey data, experimental results, or any dataset with a finite number of categories.
Examples of using factors in data frames:
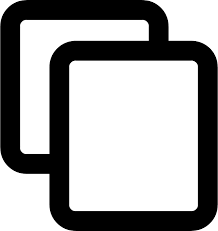
# Creating a data frame with a factor column
survey_data <- data.frame(
respondent_id = 1:5,
gender = factor(c("Male", "Female", "Male", "Female", "Male")),
education = factor(c("High School", "Bachelor", "Master", "PhD", "Bachelor"),
levels = c("High School", "Bachelor", "Master", "PhD"))
)
# Subsetting data by factor levels
male_respondents <- survey_data[survey_data$gender == "Male", ]
Factor Levels and Ordering
When factors represent ordered data (e.g., ratings, rankings), it is important to specify the order of the levels. This allows R to recognize the inherent order of the categories and perform appropriate comparisons or calculations.
Examples of working with ordered factors:
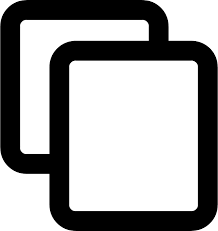
# Creating an ordered factor
rating <- factor(c("Good", "Excellent", "Average", "Good", "Poor"),
levels = c("Poor", "Average", "Good", "Excellent"), ordered = TRUE)
# Sorting data based on ordered factors
sorted_rating <- sort(rating)
Best Practices for Working with Factors
- Always explicitly define the levels of factors when working with categorical data to avoid unexpected results, especially when working with data from different sources.
- Use ordered factors when the data has a meaningful order (e.g., "Low", "Medium", "High" or "Poor", "Good", "Excellent").
- Factors are more memory-efficient than character vectors, so use them when dealing with large datasets with repeated categories.
- Be careful when reordering or modifying factor levels, as it can change the interpretation of the data.
Conclusion
Factors are a powerful tool for handling categorical data in R. They help you efficiently store and manipulate categorical variables while preserving the integrity of the data. Mastering the use of factors will enhance your ability to work with survey data, experimental results, and other forms of categorical data in R.
Conditional Statements (if, else, ifelse) in R
Conditional statements are used to perform different actions based on different conditions. In R, conditional statements like if
, else
, and ifelse
are essential for controlling the flow of execution. They allow you to execute code only when certain conditions are met, making your programs more dynamic and adaptable.
The if
Statement
The if
statement evaluates a condition, and if the condition is TRUE, the code block inside the if
block is executed.
Example of an if
statement:
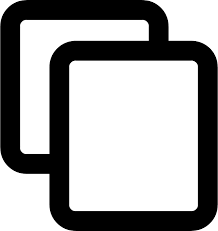
# Checking if a number is positive
x <- 10
if (x > 0) {
print("x is positive")
}
The ifelse
Function
The ifelse
function is a vectorized conditional function in R, meaning it can apply conditions to entire vectors or arrays. It takes three arguments: the condition, the value to return if the condition is TRUE, and the value to return if the condition is FALSE.
Example of using ifelse
:
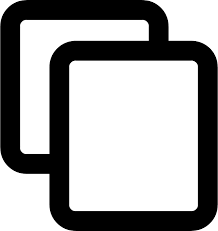
# Using ifelse to check if a number is positive or negative
y <- -5
result <- ifelse(y > 0, "Positive", "Negative")
print(result)
The else
Statement
The else
statement is used in conjunction with an if
statement to specify the action to take if the condition is FALSE. The else
block is optional, but when used, it provides an alternative action if the condition in the if
statement is not satisfied.
Example of using if-else
:
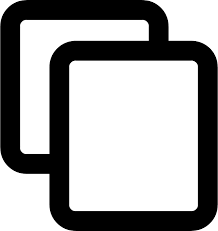
# Check if a number is even or odd
z <- 7
if (z %% 2 == 0) {
print("z is even")
} else {
print("z is odd")
}
Using Multiple if
Statements: if-else if-else
If you have multiple conditions to check, you can chain multiple if
and else if
statements together. This allows for more complex decision-making logic.
Example of using if-else if-else
:
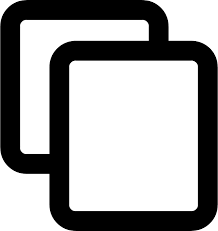
# Check the range of a number
a <- 15
if (a < 10) {
print("a is less than 10")
} else if (a >= 10 & a <= 20) {
print("a is between 10 and 20")
} else {
print("a is greater than 20")
}
Best Practices for Conditional Statements
- Use
ifelse
when you need to apply a condition to entire vectors or data frames for efficiency. - Ensure that conditions are logically clear and cover all possible cases to avoid unexpected results.
- When dealing with multiple conditions, use
else if
to avoid checking the same condition multiple times. - When possible, try to simplify the logic to make the code more readable and maintainable.
Conclusion
Conditional statements like if
, else
, and ifelse
are integral to decision-making in R. Whether you're working with individual values or entire datasets, understanding how to use these statements effectively will make your R programming more dynamic and powerful.
Loops (for, while) in R
Loops are used to repeat a block of code multiple times, making it easier to perform repetitive tasks without writing the same code repeatedly. In R, two common types of loops are for
loops and while
loops. Each of these allows you to iterate over data structures or run code while a condition is true.
The for
Loop
The for
loop is used to iterate over a sequence (such as a vector, list, or range of numbers) and execute a block of code for each element in the sequence.
Basic syntax of a for
loop:
# Syntax of a for loop
for (variable in sequence) {
# Code to be executed
}
Example of using a for
loop to print each element in a vector:
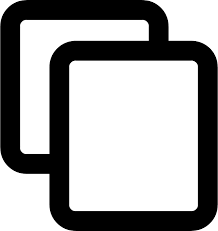
# Creating a vector
numbers <- c(1, 2, 3, 4, 5)
# Using a for loop to print each number
for (num in numbers) {
print(num)
}
Using for
Loops with Indices
You can also use a for
loop to iterate over the indices of a vector or list. This is useful when you want to perform operations that depend on the position of the elements.
Example of using indices in a for
loop:
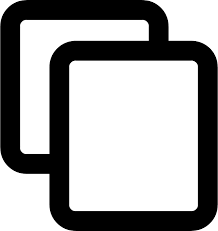
# Iterating over indices of a vector
for (i in 1:length(numbers)) {
print(paste("Element at index", i, "is", numbers[i]))
}
The while
Loop
The while
loop continues to execute a block of code as long as a specified condition is TRUE. It is particularly useful when you do not know the exact number of iterations in advance, but you want to repeat an action until a condition is met.
Basic syntax of a while
loop:
# Syntax of a while loop
while (condition) {
# Code to be executed
}
Example of using a while
loop to print numbers until a condition is met:
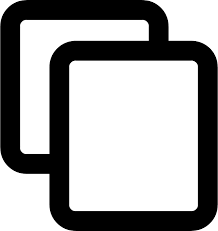
# Using a while loop to print numbers until a condition is met
counter <- 1
while (counter <= 5) {
print(counter)
counter <- counter + 1
}
Breaking and Continuing in Loops
You can control the flow of loops using break
and next
statements. The break
statement exits the loop, while the next
statement skips to the next iteration of the loop.
Example of using break
and next
:
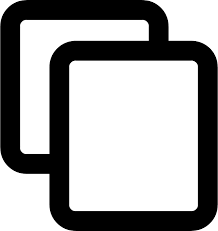
# Using break to exit the loop when a condition is met
for (i in 1:10) {
if (i == 5) {
break # Exit the loop when i equals 5
}
print(i)
}
# Using next to skip an iteration
for (i in 1:5) {
if (i == 3) {
next # Skip the iteration when i equals 3
}
print(i)
}
Nested Loops
In some cases, you might need to use a loop inside another loop, referred to as a nested loop. This allows you to perform more complex operations, such as iterating over a two-dimensional structure (e.g., a matrix or data frame).
Example of using a nested for
loop:
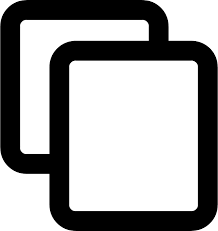
# Nested for loop to print a multiplication table
for (i in 1:3) {
for (j in 1:3) {
print(paste(i, "x", j, "=", i * j))
}
}
Best Practices for Using Loops in R
- Avoid using
for
loops when vectorized operations (such as those provided byapply()
,lapply()
, and other functions) can achieve the same result, as they are usually faster and more efficient. - Always ensure that the condition in a
while
loop will eventually become FALSE, otherwise, the loop will run indefinitely. - Be mindful of the number of iterations, especially in large datasets, as loops can be computationally expensive.
- Use
next
andbreak
judiciously to control the flow of loops and avoid unnecessary iterations.
Conclusion
Loops are essential for automating repetitive tasks in R. Whether you are iterating over elements of a vector, handling complex conditions in a while
loop, or working with nested loops for multidimensional data, mastering loops will help you write more efficient and flexible R programs.
Using break
and next
in Loops in R
In R, the break
and next
statements allow you to control the flow of a loop. These statements are useful for modifying the behavior of a loop, such as stopping it early or skipping certain iterations. Here's how you can use them:
The break
Statement
The break
statement is used to exit a loop prematurely when a specific condition is met. Once break
is encountered, the loop terminates, and the program continues with the code that follows the loop.
Example of using break
to exit a for
loop early:
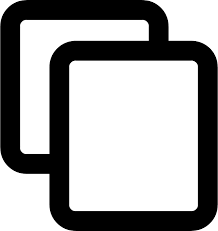
# Loop through numbers and stop when the number is 5
for (i in 1:10) {
if (i == 5) {
break # Exit the loop when i equals 5
}
print(i)
}
In the example above, the loop will print numbers from 1 to 4, but when it reaches 5, the break
statement will stop the loop and prevent printing the numbers after 5.
The next
Statement
The next
statement is used to skip the current iteration of the loop and move on to the next iteration. This can be useful when you want to avoid certain iterations based on a condition, but you don't want to stop the entire loop.
Example of using next
to skip an iteration:
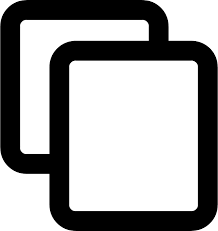
# Loop through numbers and skip the iteration when the number is 3
for (i in 1:5) {
if (i == 3) {
next # Skip the iteration when i equals 3
}
print(i)
}
In the example above, the loop prints numbers from 1 to 5, but when it reaches 3, the next
statement skips the printing of 3 and moves to the next iteration (4).
Using break
and next
in while
Loops
You can also use break
and next
in while
loops to control when to exit the loop or skip an iteration based on conditions. These statements function similarly to how they work in for
loops.
Example of using break
in a while
loop:
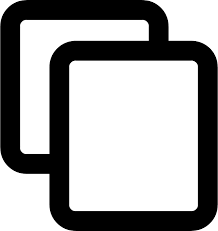
# Using a while loop with break
counter <- 1
while (counter <= 10) {
if (counter == 6) {
break # Exit the loop when counter equals 6
}
print(counter)
counter <- counter + 1
}
Example of using next
in a while
loop:
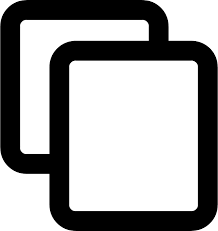
# Using a while loop with next
counter <- 1
while (counter <= 5) {
if (counter == 3) {
counter <- counter + 1
next # Skip the iteration when counter equals 3
}
print(counter)
counter <- counter + 1
}
Best Practices for Using break
and next
- Use
break
when you need to exit a loop early based on a specific condition, especially if continuing the loop would be inefficient or unnecessary. - Use
next
when you want to skip certain iterations of a loop but continue with the rest of the iterations. - Make sure that the conditions in the loop are properly defined to avoid infinite loops, especially when using
while
loops withbreak
. - Keep the loop logic simple and maintainable by using
next
andbreak
sparingly to avoid creating complex or hard-to-understand code.
Conclusion
The break
and next
statements are powerful tools for controlling the flow of loops in R. By using break
, you can exit a loop early when a condition is met, while next
allows you to skip specific iterations of the loop. Mastering these statements will help you write more efficient and flexible loops in your R programs.
The apply
Family of Functions in R
R provides a set of powerful functions for applying operations over arrays, lists, and data frames. These functions fall under the "apply family," which includes apply
, lapply
, and sapply
. These functions allow you to apply a function to data structures without needing to write explicit loops. Let's explore how each of these functions works.
apply()
: Apply a Function to Rows or Columns of a Matrix
The apply()
function is used to apply a function to the rows or columns of a matrix or 2D array. It simplifies operations that would normally require nested loops.
Syntax:
apply(X, MARGIN, FUN, ...)
X
: The matrix or data frame.MARGIN
: The margin to apply the function over. Use1
for rows,2
for columns.FUN
: The function to apply.
Example of using apply()
to calculate the sum of each row in a matrix:
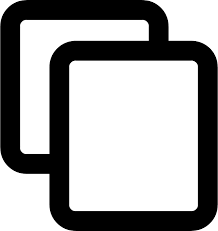
# Create a matrix
matrix_data <- matrix(1:9, nrow = 3, byrow = TRUE)
# Apply the sum function to each row
row_sums <- apply(matrix_data, 1, sum)
print(row_sums)
In this example, apply()
calculates the sum of each row (since MARGIN = 1
) in the matrix.
lapply()
: Apply a Function to Each Element of a List
The lapply()
function applies a function to each element of a list or vector and returns a list. It is useful when you need to perform operations on each element of a list.
Syntax:
lapply(X, FUN, ...)
X
: The list or vector.FUN
: The function to apply.
Example of using lapply()
to calculate the square of each element in a list:
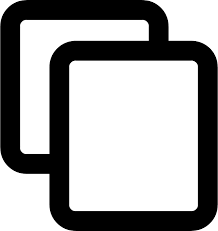
# Create a list
my_list <- list(a = 1, b = 2, c = 3)
# Apply the square function to each element
squared_list <- lapply(my_list, function(x) x^2)
print(squared_list)
In this example, lapply()
calculates the square of each element in the list and returns a list with the results.
sapply()
: Apply a Function to Each Element and Simplify the Output
The sapply()
function is similar to lapply()
, but it tries to simplify the result. If the result is a list of length 1, sapply()
will return a vector or matrix instead of a list.
Syntax:
sapply(X, FUN, ...)
X
: The list or vector.FUN
: The function to apply.
Example of using sapply()
to calculate the square of each element and return a vector:
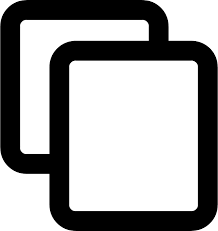
# Create a list
my_list <- list(a = 1, b = 2, c = 3)
# Apply the square function and simplify the result
squared_vector <- sapply(my_list, function(x) x^2)
print(squared_vector)
In this example, sapply()
applies the square function and simplifies the result into a vector rather than a list.
Comparing apply()
, lapply()
, and sapply()
Function | Input | Output | Common Use Case |
---|---|---|---|
apply() |
Matrix or 2D array | Vector, matrix, or array | Apply a function to rows or columns of a matrix |
lapply() |
List or vector | List | Apply a function to each element of a list |
sapply() |
List or vector | Vector or matrix (simplified from list) | Apply a function to each element of a list and simplify output |
Best Practices for Using Apply Functions
- Use
apply()
when you need to perform operations on the rows or columns of a matrix. - Use
lapply()
when working with lists or vectors and you want the result to be a list. - Use
sapply()
when you want to simplify the result into a vector or matrix, especially when the function results in a scalar. - Remember to check the output type of
sapply()
, as it may simplify the output in ways that might not be desirable in all cases.
Conclusion
The apply
family of functions in R is a powerful tool for performing operations on data structures such as matrices, lists, and vectors. By using apply()
, lapply()
, and sapply()
, you can perform complex data manipulations efficiently and concisely, without the need for explicit loops.
Defining and Calling Functions in R
Functions in R allow you to encapsulate reusable pieces of code. By defining a function, you can create custom operations that can be called multiple times throughout your script, improving readability and reducing code repetition.
Defining a Function in R
To define a function in R, you use the function()
keyword followed by the function body. A function can have inputs (parameters) and an output (return value). Below is the syntax for defining a function:
function_name <- function(arg1, arg2, ...) {
# Function body
# Perform operations
return(result) # Optional: return a value
}
In this structure:
function_name
is the name of the function.arg1, arg2, ...
are the parameters (inputs) to the function.return(result)
is used to specify the function's output (optional).
Example: Defining a Function
Let’s define a simple function that calculates the square of a number:
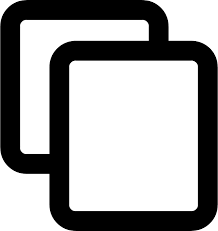
# Define a function to calculate the square of a number
square <- function(x) {
return(x^2)
}
# Call the function with an argument
result <- square(4)
print(result) # Output: 16
In this example, the function square()
takes one argument x
and returns its square. We then call the function with the argument 4
, and the result is printed.
Calling a Function
Once a function is defined, you can call it by using its name followed by parentheses. You pass values (arguments) to the function within these parentheses.
For example, to call the square()
function defined earlier:
result <- square(5)
print(result) # Output: 25
The function is called with the argument 5
, and the result is printed.
Functions with Multiple Arguments
Functions can accept multiple arguments. You can define and call a function with more than one parameter by specifying them in both the function definition and the function call.
Example: Define a function that adds two numbers:
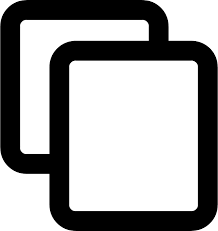
# Define a function to add two numbers
add_numbers <- function(a, b) {
return(a + b)
}
# Call the function with two arguments
sum_result <- add_numbers(3, 7)
print(sum_result) # Output: 10
In this example, the function add_numbers()
accepts two arguments a
and b
and returns their sum. We then call the function with the values 3
and 7
.
Functions with Default Arguments
You can also specify default values for function arguments. If the caller does not provide a value for an argument, the default value will be used.
Example: Define a function with a default argument for b
:
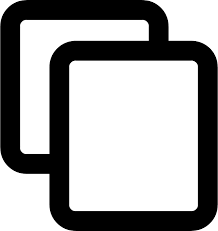
# Define a function with a default argument
multiply_numbers <- function(a, b = 2) {
return(a * b)
}
# Call the function with one argument
result1 <- multiply_numbers(3)
print(result1) # Output: 6
# Call the function with both arguments
result2 <- multiply_numbers(3, 4)
print(result2) # Output: 12
In this example, the function multiply_numbers()
has a default value of 2
for the second argument b
. If no value is provided for b
, it defaults to 2
.
Returning Multiple Values from a Function
In R, you can return multiple values from a function by returning a list, vector, or data frame containing the desired values.
Example: Define a function that returns both the sum and the product of two numbers:
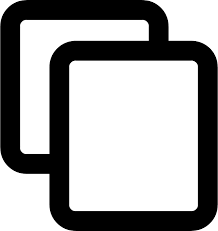
# Define a function to return both sum and product
sum_and_product <- function(a, b) {
sum_val <- a + b
product_val <- a * b
return(list(sum = sum_val, product = product_val))
}
# Call the function and store the result
result <- sum_and_product(3, 5)
# Access the returned values
print(result$sum) # Output: 8
print(result$product) # Output: 15
In this example, the function sum_and_product()
returns a list containing both the sum and the product of the two input numbers. We then access the individual elements of the returned list using $
.
Conclusion
Defining and calling functions in R is a fundamental practice for writing clean, reusable, and modular code. Functions allow you to encapsulate logic and apply it multiple times in your scripts, making code more readable and maintainable. Functions can accept parameters, return values, and even have default arguments to handle different use cases.
Function Arguments and Defaults in R
In R, functions can accept arguments (also known as parameters) that provide input values to the function. These arguments allow the function to perform operations on different inputs each time it is called. You can also define default values for function arguments to handle cases when no argument is provided.
Function Arguments
When you define a function in R, you can specify the parameters that the function will take. These parameters are placeholders for the values that will be passed to the function when it is called.
# Define a function that takes two arguments
add_numbers <- function(a, b) {
return(a + b)
}
# Call the function with two arguments
result <- add_numbers(5, 3)
print(result) # Output: 8
In this example, the function add_numbers()
takes two arguments a
and b
and returns their sum. We call the function with the values 5
and 3
, and the result is printed.
Default Arguments
In R, you can assign default values to function arguments. If the caller does not provide a value for the argument, the default value is used instead. Default arguments are especially useful when you want to provide flexibility in function usage.
# Define a function with default arguments
greet <- function(name = "Guest", greeting = "Hello") {
return(paste(greeting, name))
}
# Call the function without any arguments
result1 <- greet()
print(result1) # Output: Hello Guest
# Call the function with one argument
result2 <- greet("Alice")
print(result2) # Output: Hello Alice
# Call the function with both arguments
result3 <- greet("Bob", "Hi")
print(result3) # Output: Hi Bob
In this example, the function greet()
has two arguments, name
and greeting
, both of which have default values. If no values are passed to the function, the defaults are used. You can also provide values for one or both arguments when calling the function.
Order of Arguments
The order of arguments matters when calling a function. If you do not use named arguments, the values are assigned to the parameters in the order in which they are defined in the function.
# Define a function with two arguments
multiply <- function(x, y) {
return(x * y)
}
# Call the function with arguments in order
result <- multiply(4, 5)
print(result) # Output: 20
Here, we call the multiply()
function by passing 4
as x
and 5
as y
. The order of the arguments is important since x
will receive the first argument and y
the second.
Named Arguments
You can also call a function by explicitly naming the arguments. This allows you to pass arguments in any order, as long as you specify the names of the parameters.
# Define a function with two arguments
divide <- function(numerator, denominator) {
return(numerator / denominator)
}
# Call the function using named arguments
result <- divide(denominator = 2, numerator = 10)
print(result) # Output: 5
In this example, we call the divide()
function using named arguments, so the order of the arguments does not matter. We specify denominator = 2
and numerator = 10
, and the result is computed correctly.
Variable Number of Arguments (Ellipsis)
R allows you to pass a variable number of arguments to a function using the ellipsis (...
) syntax. This is useful when you want a function to handle different numbers of input arguments.
# Define a function with variable arguments
sum_values <- function(...) {
return(sum(...))
}
# Call the function with different numbers of arguments
result1 <- sum_values(1, 2, 3)
print(result1) # Output: 6
result2 <- sum_values(5, 10, 15, 20)
print(result2) # Output: 50
In this example, the function sum_values()
accepts a variable number of arguments and returns their sum. We call the function with different numbers of arguments, and the function computes the correct sum each time.
Argument Matching
When calling a function with named arguments, R will match the arguments based on their names. If you provide an argument without a name, it will be matched by position.
# Define a function with named arguments
calculate_area <- function(length, width) {
return(length * width)
}
# Call the function with positional arguments
area1 <- calculate_area(5, 7)
print(area1) # Output: 35
# Call the function with named arguments
area2 <- calculate_area(width = 7, length = 5)
print(area2) # Output: 35
In this example, both calls to calculate_area()
produce the same result, but the second call uses named arguments, which allows the order of the arguments to be reversed.
Conclusion
Function arguments and default values in R offer flexibility and enhance the power of functions. By using default arguments, named arguments, and variable numbers of arguments, you can write functions that are more general and can handle a variety of inputs. Understanding how to define and call functions with different argument types is essential for writing reusable and efficient code in R.
Anonymous Functions in R
In R, an anonymous function is a function that is defined without a name. These functions are often used for short, one-off operations where you do not need to reuse the function. Anonymous functions are commonly used in conjunction with functions like apply()
, lapply()
, and sapply()
for performing operations on data structures.
Defining Anonymous Functions
Anonymous functions are created using the function()
keyword, but without giving the function a name. Instead of assigning the function to a variable or name, you use it directly as an argument to other functions.
# Define an anonymous function that adds two numbers
result <- (function(x, y) {
return(x + y)
})(5, 3)
print(result) # Output: 8
In the example above, we define an anonymous function that takes two arguments, x
and y
, adds them together, and returns the result. We immediately invoke the function by passing the values 5
and 3
, and the result is stored in result
.
Using Anonymous Functions with apply()
and Other Functions
Anonymous functions are often used as arguments to functions that apply operations to elements of data structures like vectors, lists, and matrices. The apply()
, lapply()
, and sapply()
functions are commonly used with anonymous functions to perform operations on each element of a data structure.
# Use an anonymous function with apply() to calculate the sum of each row in a matrix
matrix_data <- matrix(1:9, nrow = 3, byrow = TRUE)
result <- apply(matrix_data, 1, function(row) {
return(sum(row))
})
print(result) # Output: 6 15 24
In this example, we use an anonymous function with apply()
to calculate the sum of each row in a matrix. The function is passed directly as an argument to apply()
and is applied to each row of the matrix.
Using Anonymous Functions with lapply()
and sapply()
Anonymous functions can also be used with lapply()
and sapply()
to iterate over lists or vectors. lapply()
returns a list, while sapply()
tries to simplify the result into a vector or array.
# Use an anonymous function with lapply() to square each number in a list
numbers <- list(1, 2, 3, 4)
squared_numbers <- lapply(numbers, function(x) {
return(x^2)
})
print(squared_numbers) # Output: List of squared numbers: 1, 4, 9, 16
# Use an anonymous function with sapply() to square each number and return a vector
squared_numbers_vector <- sapply(numbers, function(x) {
return(x^2)
})
print(squared_numbers_vector) # Output: 1 4 9 16
In the first example, we use an anonymous function with lapply()
to square each number in the list numbers
. In the second example, we use sapply()
to square each number and return the result as a vector.
Advantages of Using Anonymous Functions
Anonymous functions are useful for simple operations where defining a named function would be overkill. Some of the key advantages include:
- Simplicity: Anonymous functions allow you to define quick, one-off operations without needing to create a separate function definition.
- Efficiency: They help reduce the need for writing extra lines of code or creating unnecessary named functions.
- Readability: Using anonymous functions can make code more concise and readable, especially when used in functions like
apply()
orlapply()
.
When to Use Anonymous Functions
Anonymous functions are ideal for situations where you need to perform a short operation once or in a specific context. They are especially useful in functional programming paradigms, where functions are passed as arguments to other functions. However, if the operation is complex or needs to be reused multiple times, it's often better to define a named function for clarity and reusability.
Conclusion
Anonymous functions in R provide a concise and flexible way to define functions for one-time use. They are often used in conjunction with functions like apply()
, lapply()
, and sapply()
to perform operations on data structures. Understanding how to use anonymous functions effectively can help you write cleaner and more efficient code in R.
Returning Values from Functions in R
In R, functions are used to perform specific tasks, and they can return values to the caller. The return()
statement is used to specify what value a function should return. If no return()
statement is used, the last evaluated expression in the function is automatically returned.
Returning a Single Value
When defining a function, you can use the return()
function to return a single value. The returned value can be of any data type, such as numeric, character, or logical.
# Define a function that returns the sum of two numbers
add_numbers <- function(a, b) {
return(a + b)
}
# Call the function and store the returned value
result <- add_numbers(5, 3)
# Print the returned value
print(result) # Output: 8
In this example, the function add_numbers()
returns the sum of two numbers. The return()
statement ensures that the result is sent back to the caller, where it is stored in the result
variable and printed.
Returning Multiple Values
R functions can return multiple values by returning a list or another data structure that holds multiple elements. You can return vectors, lists, or other composite objects to return more than one value from a function.
# Define a function that returns multiple values using a list
get_stats <- function(numbers) {
mean_value <- mean(numbers)
sum_value <- sum(numbers)
return(list(mean = mean_value, sum = sum_value))
}
# Call the function and store the returned values
stats <- get_stats(c(1, 2, 3, 4, 5))
# Print the returned values
print(stats) # Output: List with mean and sum values
In this example, the function get_stats()
returns a list containing the mean and sum of the input vector numbers
. The returned list is stored in the stats
variable and printed. You can access individual values in the list using the $
operator.
# Access the mean and sum from the returned list
mean_value <- stats$mean
sum_value <- stats$sum
# Print the accessed values
print(mean_value) # Output: 3
print(sum_value) # Output: 15
Implicit Return (No return() Statement)
If you don't explicitly use the return()
statement, R will automatically return the result of the last evaluated expression in the function. This is useful for simple functions where you want to return the result without writing an explicit return()
statement.
# Define a function that implicitly returns the sum of two numbers
add_numbers_implicit <- function(a, b) {
a + b # The result of this expression is returned implicitly
}
# Call the function and store the returned value
result <- add_numbers_implicit(5, 3)
# Print the returned value
print(result) # Output: 8
In this example, the add_numbers_implicit()
function does not use the return()
statement. However, the last evaluated expression, a + b
, is automatically returned, and the result is printed.
Returning Values from a Function Early
You can also use the return()
statement to exit a function early and return a value before executing the rest of the code in the function. This is often useful for conditional logic.
# Define a function that returns early if a number is negative
check_positive <- function(x) {
if (x < 0) {
return("Negative number")
}
return("Positive number")
}
# Call the function with a positive number
result1 <- check_positive(5)
print(result1) # Output: Positive number
# Call the function with a negative number
result2 <- check_positive(-3)
print(result2) # Output: Negative number
In this example, the function check_positive()
uses the return()
statement to return a value early if the input number is negative. If the number is positive, it proceeds to return a different value.
Returning NULL
A function can return NULL
explicitly when there is no meaningful result or when you want to signal that no value is available. NULL
is often used when you want to indicate that a function has failed or that there is no data to return.
# Define a function that returns NULL if the input is empty
check_empty <- function(x) {
if (length(x) == 0) {
return(NULL)
}
return(x)
}
# Call the function with a non-empty vector
result1 <- check_empty(c(1, 2, 3))
print(result1) # Output: 1 2 3
# Call the function with an empty vector
result2 <- check_empty(c())
print(result2) # Output: NULL
In this example, the check_empty()
function returns NULL
if the input vector is empty. Otherwise, it returns the input vector itself.
Conclusion
Returning values from functions in R is a fundamental concept that allows you to pass results back to the caller. You can return a single value, multiple values (via lists or other structures), or use implicit return for simple functions. The return()
statement provides flexibility in controlling the flow of a function and managing its output. Understanding how to return values efficiently is key to writing effective and reusable functions in R.
Importing Data from CSV, Excel, and Databases in R
In R, importing data from various formats such as CSV, Excel, and databases is a common task. R provides various built-in functions and packages to work with different data formats. In this section, we will explore how to import data from CSV files, Excel files, and databases into R for analysis.
Importing Data from CSV Files
CSV (Comma-Separated Values) files are one of the most commonly used formats for storing data. R provides the read.csv()
function to read CSV files into R.
# Import data from a CSV file
data_csv <- read.csv("data.csv")
# Print the imported data
print(data_csv)
In this example, the read.csv()
function is used to import data from a CSV file named data.csv
. The resulting data is stored in the variable data_csv
, and the contents are printed to the console.
Customizing CSV Import
The read.csv()
function also allows customization of how the data is imported, such as specifying a different delimiter, setting column types, or handling missing values.
# Import data with custom delimiter (semicolon)
data_semicolon <- read.csv("data_semicolon.csv", sep = ";")
# Import data and handle missing values by setting NA values
data_na <- read.csv("data_with_na.csv", na.strings = c("NA", ""))
In this example, we specify a different delimiter (semicolon) using the sep
argument and handle missing values by using the na.strings
argument to define custom missing value markers.
Importing Data from Excel Files
Excel files are another popular format for storing data. To import data from Excel files, you can use the readxl
package, which provides the read_excel()
function.
First, you need to install the readxl
package if it's not already installed:
# Install the readxl package (if not installed already)
install.packages("readxl")
After installing the package, you can use read_excel()
to read data from Excel files.
# Load the readxl package
library(readxl)
# Import data from an Excel file
data_excel <- read_excel("data.xlsx")
# Print the imported data
print(data_excel)
In this example, the read_excel()
function is used to import data from an Excel file named data.xlsx
. The resulting data is stored in the variable data_excel
, and the contents are printed to the console.
Importing Specific Sheets from Excel
If the Excel file contains multiple sheets, you can specify which sheet to import using the sheet
argument.
# Import data from a specific sheet
data_sheet <- read_excel("data.xlsx", sheet = "Sheet2")
# Print the imported data from Sheet2
print(data_sheet)
In this example, we specify the sheet name "Sheet2" to import data from that specific sheet in the Excel file.
Importing Data from Databases
R can also connect to databases and import data using SQL queries. The DBI
package is commonly used to interface with databases, and you can use the dbConnect()
function to establish a connection to a database.
Before using the DBI
package, you may need to install it along with a driver for the specific database (e.g., RMySQL
, RSQLite
, or RPostgres
):
# Install the DBI package (if not installed already)
install.packages("DBI")
# Install a database-specific driver (e.g., RSQLite)
install.packages("RSQLite")
Once the packages are installed, you can connect to a database and import data using a SQL query.
# Load DBI and RSQLite packages
library(DBI)
library(RSQLite)
# Connect to a SQLite database
conn <- dbConnect(RSQLite::SQLite(), "database.sqlite")
# Query data from a table in the database
data_db <- dbGetQuery(conn, "SELECT * FROM employees")
# Print the imported data
print(data_db)
# Close the database connection
dbDisconnect(conn)
In this example, we use the dbConnect()
function from the DBI
package to connect to an SQLite database. We then use the dbGetQuery()
function to execute a SQL query and retrieve data from the employees
table. Finally, the connection is closed with dbDisconnect()
.
Conclusion
R provides various methods for importing data from different sources such as CSV files, Excel files, and databases. The read.csv()
function is ideal for CSV files, while the readxl
package is used for Excel files. For databases, the DBI
package can be used to connect to different types of databases and execute SQL queries. Understanding how to import data into R is crucial for data analysis, as it allows you to work with diverse data sources efficiently.
Exporting Data to Files in R
In R, after performing data analysis or manipulation, you might want to export your results to files for further use or sharing. R provides various functions to export data to different formats such as CSV, Excel, and other file types. In this section, we will explore how to export data from R to these formats.
Exporting Data to CSV Files
CSV (Comma-Separated Values) files are one of the most common formats for storing and sharing tabular data. R provides the write.csv()
function to export data frames to CSV files.
# Example data
data <- data.frame(Name = c("John", "Alice", "Bob"),
Age = c(30, 25, 35),
Occupation = c("Engineer", "Doctor", "Artist"))
# Export data to a CSV file
write.csv(data, "data_export.csv", row.names = FALSE)
In this example, the write.csv()
function is used to export a data frame called data
to a CSV file named data_export.csv
. The row.names = FALSE
argument is used to avoid writing row numbers to the file.
Customizing CSV Export
You can customize the export by specifying different arguments such as the separator (e.g., semicolon) or including row names.
# Export data with a different separator (semicolon)
write.csv(data, "data_export_semicolon.csv", sep = ";", row.names = FALSE)
In this example, we use the sep
argument to specify a semicolon as the separator in the CSV file.
Exporting Data to Excel Files
R also allows you to export data to Excel files using the writexl
package, which provides the write_xlsx()
function. This is particularly useful when you need to share data with users who prefer Excel files.
First, you need to install the writexl
package if it's not already installed:
# Install the writexl package (if not installed already)
install.packages("writexl")
After installing the package, you can use the write_xlsx()
function to export data to an Excel file.
# Load the writexl package
library(writexl)
# Export data to an Excel file
write_xlsx(data, "data_export.xlsx")
In this example, we use the write_xlsx()
function to export the data frame data
to an Excel file named data_export.xlsx
.
Exporting Data to Other File Formats
R also supports exporting data to other formats, such as text files or JSON files. Below are examples of how to export data to these formats:
Exporting Data to a Text File
# Export data to a text file with tab-separated values
write.table(data, "data_export.txt", sep = "\t", row.names = FALSE)
The write.table()
function can be used to export data to a text file, where you can specify the separator, such as a tab character (e.g., \t
) or any other character you choose.
Exporting Data to a JSON File
To export data to a JSON file, you can use the jsonlite
package, which provides the toJSON()
function to convert R objects to JSON format.
# Install the jsonlite package (if not installed already)
install.packages("jsonlite")
# Load the jsonlite package
library(jsonlite)
# Convert data to JSON format and save to a file
write_json(data, "data_export.json")
In this example, the write_json()
function is used to export the data frame data
to a JSON file named data_export.json
.
Conclusion
Exporting data from R is a simple process with various built-in functions and packages to suit your needs. You can export data to CSV files using write.csv()
, to Excel files using the writexl
package, and to other formats like text files or JSON files using write.table()
or jsonlite
, respectively. Understanding how to export data allows you to share your results and collaborate with others efficiently.
Filtering, Sorting, and Selecting Data in R
In R, filtering, sorting, and selecting data are essential tasks when working with datasets. These operations allow you to extract specific information, arrange data in meaningful ways, and select particular variables or rows for analysis. In this section, we will explore how to filter, sort, and select data using different techniques in R.
Filtering Data
Filtering data involves selecting rows based on certain conditions. You can filter data in R using the subset()
function or by using logical indexing.
Using subset()
Function
The subset()
function allows you to filter rows based on specific conditions. It is particularly useful when you need to filter data based on column values.
# Example data
data <- data.frame(Name = c("John", "Alice", "Bob"),
Age = c(30, 25, 35),
Occupation = c("Engineer", "Doctor", "Artist"))
# Filter data where Age is greater than 30
filtered_data <- subset(data, Age > 30)
# Print the filtered data
print(filtered_data)
In this example, the subset()
function filters rows where the Age
column is greater than 30. The resulting filtered data is stored in the filtered_data
variable.
Using Logical Indexing
You can also filter data using logical conditions directly inside square brackets.
# Filter data using logical indexing
filtered_data <- data[data$Age > 30, ]
# Print the filtered data
print(filtered_data)
Here, we use logical indexing to select rows where the Age
column is greater than 30. The condition data$Age > 30
returns a logical vector, which is used to filter the rows.
Sorting Data
Sorting data involves arranging rows in a specific order, either ascending or descending. You can use the order()
function to sort data based on one or more columns.
# Sort data by Age in ascending order
sorted_data_asc <- data[order(data$Age), ]
# Sort data by Age in descending order
sorted_data_desc <- data[order(-data$Age), ]
# Print the sorted data
print(sorted_data_asc)
print(sorted_data_desc)
In this example, the order()
function is used to sort the data. To sort in ascending order, we simply pass the column name to order()
. To sort in descending order, we use the negative sign before the column name -data$Age
.
Selecting Specific Columns
Sometimes, you may only want to select specific columns from a dataset. You can do this using the column names or column indices.
Using Column Names
# Select specific columns by name
selected_columns <- data[, c("Name", "Age")]
# Print the selected columns
print(selected_columns)
Here, we select the Name
and Age
columns from the data frame using their column names inside the c()
function.
Using Column Indices
# Select specific columns by index
selected_columns <- data[, c(1, 2)]
# Print the selected columns
print(selected_columns)
In this example, we select the first and second columns by their indices (1 and 2). Column indexing allows you to select columns without referring to their names.
Combining Filters and Selections
You can combine filtering and column selection to extract specific parts of your data. Below is an example that filters data based on a condition and then selects specific columns.
# Filter data where Age is greater than 30 and select the Name and Occupation columns
result <- subset(data, Age > 30)[, c("Name", "Occupation")]
# Print the result
print(result)
In this example, we first filter the data for rows where the Age
is greater than 30, and then select the Name
and Occupation
columns from the filtered data.
Conclusion
Filtering, sorting, and selecting data are key operations when working with datasets in R. The subset()
function and logical indexing are useful for filtering data, while the order()
function allows you to sort data. You can select specific columns using either column names or indices. By combining these techniques, you can efficiently manipulate and extract the data you need for analysis.
Handling Missing Data (NA) in R
Missing data is a common issue when working with real-world datasets. In R, missing values are represented by NA
(Not Available), and handling them properly is essential for accurate analysis. R provides various functions to detect, remove, or replace missing values. In this section, we will explore how to handle missing data in R.
Identifying Missing Data
You can identify missing data in a dataset by using the is.na()
function, which returns a logical vector indicating whether each element is NA
or not.
# Example data with missing values
data <- data.frame(Name = c("John", "Alice", "Bob"),
Age = c(30, NA, 35),
Occupation = c("Engineer", NA, "Artist"))
# Check for missing values
missing_data <- is.na(data)
# Print missing data indicator
print(missing_data)
The is.na()
function returns a logical matrix where TRUE
indicates the presence of missing values and FALSE
indicates non-missing values.
Counting Missing Values
To count the number of missing values in a dataset, you can use the sum()
function along with is.na()
.
# Count the number of missing values in the dataset
missing_count <- sum(is.na(data))
# Print the count of missing values
print(missing_count)
In this example, the sum(is.na(data))
expression counts the total number of missing values in the dataset data
.
Removing Missing Data
Sometimes, you may want to remove rows or columns with missing values. R provides several ways to remove missing data using functions like na.omit()
or complete.cases()
.
Removing Rows with Missing Data
The na.omit()
function removes any rows that contain missing values.
# Remove rows with missing values
clean_data <- na.omit(data)
# Print the cleaned data
print(clean_data)
In this example, na.omit(data)
removes any row in the data frame data
that contains at least one NA
value.
Using complete.cases()
to Remove Rows
You can also use the complete.cases()
function, which returns a logical vector indicating whether each row contains no missing values. You can use this to filter out rows with missing data.
# Remove rows with missing values using complete.cases()
clean_data <- data[complete.cases(data), ]
# Print the cleaned data
print(clean_data)
In this example, complete.cases(data)
returns a logical vector that is used to filter out any rows containing missing values.
Replacing Missing Data
In some cases, instead of removing missing data, you may want to replace it with a specific value, such as the mean, median, or a custom value.
Replacing Missing Values with a Specific Value
You can replace missing values in a dataset with a specific value by using logical indexing. For example, to replace NA
values with zero:
# Replace NA values with zero
data[is.na(data)] <- 0
# Print the modified data
print(data)
This example replaces all NA
values in the dataset with zero. You can also replace NA
values with any other value, such as the mean or median.
Replacing Missing Values with the Mean or Median
To replace missing values in numerical columns with the mean or median of the respective column, you can use the mean()
or median()
functions along with na.rm = TRUE
to ignore NA
values when calculating the mean or median.
# Replace missing values in Age column with the mean
data$Age[is.na(data$Age)] <- mean(data$Age, na.rm = TRUE)
# Replace missing values in Occupation column with "Unknown"
data$Occupation[is.na(data$Occupation)] <- "Unknown"
# Print the modified data
print(data)
In this example, missing values in the Age
column are replaced with the mean of the column, and missing values in the Occupation
column are replaced with "Unknown".
Conclusion
Handling missing data is a crucial step in data preprocessing. In R, you can identify missing data using the is.na()
function, count the number of missing values, and remove or replace missing data using functions like na.omit()
, complete.cases()
, and logical indexing. Depending on the context of your analysis, you can choose to remove or replace missing values to ensure the quality and accuracy of your analysis.
Data Manipulation with dplyr
and tidyverse
The dplyr
package, part of the tidyverse
, provides a set of functions that make data manipulation easier and more intuitive. It allows you to perform operations like filtering, selecting, mutating, and summarizing data in a simple, readable way. In this section, we will explore how to manipulate data using dplyr
and other tidyverse
packages.
Loading the tidyverse
Package
The tidyverse
is a collection of R packages for data science, including dplyr
, ggplot2
, tidyr
, and others. To use dplyr
, you need to install and load the tidyverse
package:
# Install tidyverse (if not already installed)
install.packages("tidyverse")
# Load the tidyverse package
library(tidyverse)
Common dplyr
Functions
dplyr
provides a variety of functions to manipulate data. Below are some of the most commonly used functions:
filter()
: Filtering Rows
The filter()
function is used to filter rows based on certain conditions. For example, you can filter data to include only rows where a certain column meets a condition.
# Example data
data <- data.frame(Name = c("John", "Alice", "Bob"),
Age = c(30, 25, 35),
Occupation = c("Engineer", "Doctor", "Artist"))
# Filter rows where Age is greater than 30
filtered_data <- data %>% filter(Age > 30)
# Print filtered data
print(filtered_data)
In this example, the filter()
function filters the rows where the Age
column is greater than 30. The %>%
operator is used to pass the data to the filter()
function.
select()
: Selecting Columns
The select()
function allows you to select specific columns from a data frame.
# Select specific columns (Name and Age)
selected_data <- data %>% select(Name, Age)
# Print selected data
print(selected_data)
This example selects only the Name
and Age
columns from the dataset.
mutate()
: Adding or Modifying Columns
The mutate()
function allows you to add new columns or modify existing ones. For example, you can create a new column based on calculations from existing columns.
# Add a new column with a 10% increase in Age
data_with_increase <- data %>% mutate(New_Age = Age * 1.1)
# Print the modified data
print(data_with_increase)
In this example, the mutate()
function creates a new column called New_Age
, which is 10% greater than the original Age
column.
arrange()
: Sorting Data
The arrange()
function is used to sort data by one or more columns. You can sort in ascending or descending order.
# Sort data by Age in ascending order
sorted_data <- data %>% arrange(Age)
# Sort data by Age in descending order
sorted_data_desc <- data %>% arrange(desc(Age))
# Print sorted data
print(sorted_data)
print(sorted_data_desc)
The arrange()
function sorts the data by the Age
column in ascending order. To sort in descending order, the desc()
function is used.
summarize()
: Summarizing Data
The summarize()
function is used to create summary statistics like mean, sum, and count for one or more columns.
# Summarize the data by calculating the mean Age
summary_data <- data %>% summarize(mean_age = mean(Age))
# Print summary data
print(summary_data)
In this example, the summarize()
function calculates the mean of the Age
column.
Chaining Multiple Operations with %>%
The %>%
(pipe) operator is a key feature of dplyr
, allowing you to chain multiple operations together. This makes the code more readable and concise. Here is an example of chaining multiple functions:
# Chain multiple operations: filter, select, and arrange
result <- data %>%
filter(Age > 25) %>%
select(Name, Age) %>%
arrange(desc(Age))
# Print the result
print(result)
This example chains the filter()
, select()
, and arrange()
functions to filter data, select specific columns, and sort the results—all in one pipeline.
Other Useful dplyr
Functions
dplyr
also provides many other useful functions for data manipulation, such as:
rename()
: Renaming columnsdistinct()
: Removing duplicate rowsgroup_by()
: Grouping data for summary operationsleft_join(), right_join(), inner_join(), full_join()
: Joining data frames
Conclusion
Data manipulation with dplyr
and the tidyverse
is efficient and intuitive. The dplyr
package provides a powerful set of functions for filtering, selecting, mutating, sorting, and summarizing data. By using the %>%
operator, you can chain operations together to create readable and concise code. The tidyverse
makes data manipulation in R easier and more accessible for data scientists and analysts.
Introduction to Visualization in R
Data visualization is an essential aspect of data analysis, allowing you to present data in a graphical form that is easier to understand and interpret. In R, there are several packages available to create visualizations, but the most commonly used package is ggplot2
, which is part of the tidyverse
suite. In this section, we will introduce you to the basics of data visualization in R, focusing on creating simple plots using ggplot2
.
Installing and Loading ggplot2
Before creating visualizations, you need to install and load the ggplot2
package, which can be done with the following commands:
# Install ggplot2 (if not already installed)
install.packages("ggplot2")
# Load the ggplot2 package
library(ggplot2)
Basic Structure of a Plot in ggplot2
The basic structure of a plot in ggplot2
is built using the following components:
ggplot()
: Initializes the plot.aesthetic mappings (aes)
: Defines the relationship between variables and visual properties (e.g., x and y axes, color, size).geoms
: Defines the type of plot (e.g., points, lines, bars).
Basic Plot Example: Scatter Plot
Let's start by creating a simple scatter plot with ggplot2
. In this example, we will plot a dataset with two variables: x
and y
.
# Example data
data <- data.frame(x = c(1, 2, 3, 4, 5),
y = c(2, 4, 6, 8, 10))
# Create a scatter plot
ggplot(data, aes(x = x, y = y)) +
geom_point() # Scatter plot (points)
In this example, we first define the dataset data
, then use the ggplot()
function to initialize the plot, specifying x
and y
as the variables to be plotted. The geom_point()
function adds points to the plot, creating a scatter plot.
Customizing Plots
ggplot2
allows you to customize your plots by adding additional elements such as titles, labels, and themes. Below is an example of customizing the scatter plot:
# Create a customized scatter plot
ggplot(data, aes(x = x, y = y)) +
geom_point(color = "blue", size = 3) + # Blue points with size 3
labs(title = "Scatter Plot of x and y",
x = "X Axis",
y = "Y Axis") + # Add title and axis labels
theme_minimal() # Apply minimal theme
In this example, we customize the plot by changing the point color to blue, adjusting the point size, adding a title and axis labels using the labs()
function, and applying a minimal theme using the theme_minimal()
function.
Creating Bar Plots
Bar plots are another common visualization type. Below is an example of creating a bar plot to visualize categorical data:
# Example data
data_bar <- data.frame(category = c("A", "B", "C", "D"),
value = c(10, 15, 7, 12))
# Create a bar plot
ggplot(data_bar, aes(x = category, y = value)) +
geom_bar(stat = "identity", fill = "steelblue") + # Bar plot with blue color
labs(title = "Bar Plot of Categories",
x = "Category",
y = "Value") +
theme_minimal()
In this example, we use the geom_bar()
function to create a bar plot. The stat = "identity"
argument indicates that the heights of the bars represent actual values, not counts. The fill
argument specifies the color of the bars.
Creating Histograms
Histograms are used to visualize the distribution of a numeric variable. Below is an example of creating a histogram:
# Example data
data_hist <- data.frame(values = c(1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5))
# Create a histogram
ggplot(data_hist, aes(x = values)) +
geom_histogram(binwidth = 1, fill = "lightgreen", color = "black") +
labs(title = "Histogram of Values",
x = "Values",
y = "Frequency") +
theme_minimal()
In this example, the geom_histogram()
function is used to create a histogram with a bin width of 1. The fill
and color
arguments customize the appearance of the bars.
Saving Plots to Files
You can save your plots to various file formats (e.g., PNG, PDF) using the ggsave()
function. Below is an example of saving a plot as a PNG image:
# Save plot as PNG
ggsave("scatter_plot.png", plot = last_plot(), width = 8, height = 6)
In this example, the ggsave()
function saves the last created plot as a PNG file named "scatter_plot.png" with a width of 8 inches and a height of 6 inches.
Conclusion
Visualization is an important part of data analysis, and R provides powerful tools like ggplot2
to create insightful and aesthetically pleasing plots. In this section, we covered the basics of creating scatter plots, bar plots, and histograms, as well as customizing and saving plots. With the ggplot2
package, you can easily create a wide variety of visualizations to better understand and communicate your data.
Basic Plots in R
In R, creating visualizations is an essential part of exploratory data analysis. R provides several basic plotting functions that help you quickly visualize and understand your data. In this section, we will cover three commonly used basic plots: scatter plots using plot()
, histograms using hist()
, and boxplots using boxplot()
.
Scatter Plot with plot()
The plot()
function is one of the most versatile and commonly used functions in R for creating scatter plots. It can be used to visualize the relationship between two numeric variables. Here's an example:
# Example data
x <- c(1, 2, 3, 4, 5)
y <- c(2, 4, 6, 8, 10)
# Create a scatter plot
plot(x, y, main = "Scatter Plot of x and y", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "blue")
In this example, x
and y
are the numeric vectors representing the data points. The plot()
function plots these points as a scatter plot, with the title and axis labels specified using main
, xlab
, and ylab
. The pch = 19
argument sets the point type (solid circle), and col = "blue"
changes the color of the points to blue.
Histogram with hist()
The hist()
function is used to create histograms, which are useful for visualizing the distribution of a numeric variable. Here’s an example of creating a histogram:
# Example data
data <- c(1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5)
# Create a histogram
hist(data, main = "Histogram of Data", xlab = "Values", col = "lightblue", border = "black", breaks = 5)
In this example, the hist()
function creates a histogram of the values in the data
vector. The breaks = 5
argument specifies the number of bins. You can customize the color of the bars with col
, add borders with border
, and set the title and axis labels similarly to plot()
.
Boxplot with boxplot()
Boxplots are useful for visualizing the distribution of a numeric variable, especially the median, quartiles, and potential outliers. The boxplot()
function creates boxplots in R. Here’s an example:
# Example data
data_box <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
# Create a boxplot
boxplot(data_box, main = "Boxplot of Data", ylab = "Values", col = "lightgreen", border = "black")
In this example, the boxplot()
function creates a boxplot of the data_box
vector. The col
argument changes the color of the box, and the border
argument sets the color of the border. The ylab
argument sets the label for the y-axis.
Customizing Plots
All three basic plots (plot()
, hist()
, and boxplot()
) can be customized using various arguments. You can modify things like axis labels, colors, titles, and the appearance of points or bars. For example:
# Customized scatter plot
plot(x, y, main = "Customized Scatter Plot", xlab = "X Axis", ylab = "Y Axis", pch = 16, col = "red", cex = 1.5)
# Customized histogram
hist(data, main = "Customized Histogram", xlab = "Values", col = "orange", border = "darkgreen", breaks = 4)
# Customized boxplot
boxplot(data_box, main = "Customized Boxplot", ylab = "Values", col = "lightpink", border = "blue")
In this example, cex
is used to adjust the size of the points in the scatter plot, breaks
is used to adjust the number of bins in the histogram, and the color options are customized for each plot type.
Conclusion
In this section, we covered the basics of three essential plot types in R: scatter plots with plot()
, histograms with hist()
, and boxplots with boxplot()
. These plots are fundamental tools for understanding and visualizing data distributions, relationships, and outliers. Customizing plots with titles, labels, colors, and other parameters helps in creating clear, informative visualizations for data analysis.
Customizing Plots in R
Customizing plots is a powerful way to make your visualizations more informative and visually appealing. In R, you can customize various aspects of your plots, such as titles, axis labels, colors, point types, and more. In this section, we’ll explore how to customize your plots to make them more readable and visually attractive.
Adding Titles and Labels
Titles and labels are essential for making your plots understandable. You can add a main title, axis titles, and more using the main
, xlab
, and ylab
arguments. Here’s an example of a scatter plot with titles and labels:
# Example data
x <- c(1, 2, 3, 4, 5)
y <- c(2, 4, 6, 8, 10)
# Scatter plot with titles and axis labels
plot(x, y, main = "Scatter Plot of x vs y", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "blue")
In this example:
main
adds a main title to the plot.xlab
adds a label to the x-axis.ylab
adds a label to the y-axis.
Customizing Colors
Colors play an important role in visualizing data. You can customize the color of points, lines, bars, and other elements of your plot using the col
argument. Here’s an example of customizing the color of points in a scatter plot:
# Scatter plot with customized color
plot(x, y, main = "Colored Scatter Plot", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "red")
In this example, the col = "red"
argument changes the color of the points to red. You can also use other color names or RGB values to customize colors.
Customizing Point Types
In scatter plots, you can change the type of points using the pch
argument. The pch
argument specifies the symbol used for the points. Here are some common values for pch
:
pch = 16
for solid circles (default).pch = 17
for triangles.pch = 18
for solid diamonds.pch = 19
for filled circles.
Here’s an example of changing the point type:
# Scatter plot with customized point type
plot(x, y, main = "Scatter Plot with Different Point Types", xlab = "X Axis", ylab = "Y Axis", pch = 17, col = "green")
In this example, pch = 17
changes the points to triangles, and col = "green"
changes their color to green.
Customizing Axis Limits
You can adjust the limits of the x and y axes using the xlim
and ylim
arguments. These arguments allow you to specify the range of values displayed on the axes. Here’s an example:
# Scatter plot with customized axis limits
plot(x, y, main = "Scatter Plot with Custom Axis Limits", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "blue", xlim = c(0, 6), ylim = c(0, 12))
In this example, xlim = c(0, 6)
sets the x-axis range from 0 to 6, and ylim = c(0, 12)
sets the y-axis range from 0 to 12.
Adding Grid Lines
Grid lines can make plots easier to read. You can add grid lines to a plot using the grid()
function. Here’s how to add grid lines to a scatter plot:
# Scatter plot with grid lines
plot(x, y, main = "Scatter Plot with Grid Lines", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "purple")
grid()
After creating the scatter plot, the grid()
function adds grid lines to both the x and y axes.
Adding Legends
Legends help explain the meaning of different plot elements. You can add a legend using the legend()
function. Here’s an example:
# Scatter plot with legend
plot(x, y, main = "Scatter Plot with Legend", xlab = "X Axis", ylab = "Y Axis", pch = 19, col = "blue")
legend("topleft", legend = "Data Points", col = "blue", pch = 19)
In this example, legend("topleft")
adds a legend in the top-left corner, and legend = "Data Points"
specifies the legend text. The col
and pch
arguments match the color and point type used in the plot.
Conclusion
In this section, we explored how to customize basic plots in R. By adjusting titles, labels, colors, point types, axis limits, and adding grid lines and legends, you can create more informative and visually appealing plots. Customizing your plots helps in conveying your data’s story more effectively, making it easier for others to understand and analyze your findings.
Advanced Visualization with ggplot2
ggplot2
is a powerful visualization package in R that allows you to create a wide range of plots with ease. It uses a layered approach to building plots, where different components (data, aesthetics, geoms, etc.) can be added on top of each other. In this section, we will explore how to create scatter plots, line plots, and bar plots using ggplot2
, along with advanced features like faceting and themes to enhance your visualizations.
Installing and Loading ggplot2
If you don’t have ggplot2
installed yet, you can install it using the following command:
# Install ggplot2
install.packages("ggplot2")
# Load ggplot2
library(ggplot2)
Creating a Basic Scatter Plot
Scatter plots are a great way to show the relationship between two continuous variables. Here's how you can create a basic scatter plot using ggplot2
:
# Example data
data <- data.frame(x = c(1, 2, 3, 4, 5),
y = c(2, 4, 6, 8, 10))
# Create scatter plot
ggplot(data, aes(x = x, y = y)) +
geom_point() +
labs(title = "Scatter Plot", x = "X Axis", y = "Y Axis")
In this example:
ggplot(data, aes(x = x, y = y))
specifies the data and aesthetics (mapping variables to axes).geom_point()
creates the scatter plot by adding points.labs()
adds the title and axis labels.
Creating a Line Plot
Line plots are useful for visualizing trends over time or ordered data. Here's an example of creating a line plot:
# Example data
data_line <- data.frame(x = c(1, 2, 3, 4, 5),
y = c(2, 4, 6, 8, 10))
# Create line plot
ggplot(data_line, aes(x = x, y = y)) +
geom_line() +
labs(title = "Line Plot", x = "X Axis", y = "Y Axis")
In this case, geom_line()
adds a line to the plot, connecting the points.
Creating a Bar Plot
Bar plots are typically used to visualize categorical data. Here’s how you can create a bar plot:
# Example data
data_bar <- data.frame(x = c("A", "B", "C", "D", "E"),
y = c(3, 5, 2, 8, 7))
# Create bar plot
ggplot(data_bar, aes(x = x, y = y)) +
geom_bar(stat = "identity", fill = "skyblue") +
labs(title = "Bar Plot", x = "Category", y = "Values")
In this case:
geom_bar(stat = "identity")
specifies that the heights of the bars should correspond to the values in the dataset (not the count of occurrences).fill = "skyblue"
changes the color of the bars.
Faceting: Creating Subplots
Faceting allows you to split your data into multiple plots based on a categorical variable. This is useful when you want to compare multiple groups side by side. Here’s how to use faceting:
# Example data with category
data_facet <- data.frame(x = c(1, 2, 3, 4, 5, 1, 2, 3, 4, 5),
y = c(2, 4, 6, 8, 10, 1, 3, 5, 7, 9),
category = c("A", "A", "A", "A", "A", "B", "B", "B", "B", "B"))
# Create scatter plot with faceting
ggplot(data_facet, aes(x = x, y = y)) +
geom_point() +
facet_wrap(~ category) +
labs(title = "Scatter Plot with Faceting", x = "X Axis", y = "Y Axis")
In this example:
facet_wrap(~ category)
creates separate plots for each category.
Customizing Themes
ggplot2 allows you to customize the overall appearance of your plots using themes. For example, you can use the theme_minimal()
or theme_bw()
to apply different themes to your plot:
# Create a scatter plot with a minimal theme
ggplot(data, aes(x = x, y = y)) +
geom_point() +
theme_minimal() +
labs(title = "Scatter Plot with Minimal Theme", x = "X Axis", y = "Y Axis")
Here, theme_minimal()
applies a clean, minimalistic theme to the plot. You can explore other themes like theme_classic()
, theme_light()
, etc.
Conclusion
In this section, we explored advanced plotting techniques with ggplot2
. By creating scatter plots, line plots, and bar plots, and utilizing faceting and themes, you can produce highly customizable and visually appealing visualizations. ggplot2
provides a wide range of customization options, making it one of the most powerful tools for data visualization in R.
Descriptive Statistics
Descriptive statistics are used to summarize, describe, and present data in a meaningful way. In this section, we will cover the calculation of common descriptive statistics such as the mean, median, variance, and standard deviation using R.
Mean
The mean is the average of all data points in a dataset. It is calculated by summing all values and dividing by the total number of values.
The formula for the mean is:
Mean = (Σx) / n
In R, you can calculate the mean of a numeric vector using the mean()
function:
# Example data
data <- c(5, 10, 15, 20, 25)
# Calculate mean
mean_value <- mean(data)
mean_value
The result will be the mean of the values in the data
vector.
Median
The median is the middle value in a dataset when the values are ordered from lowest to highest. If there is an even number of values, the median is the average of the two middle values.
The formula for the median is:
Median = middle value (or average of two middle values)
In R, you can calculate the median using the median()
function:
# Calculate median
median_value <- median(data)
median_value
The result will be the median of the data
vector.
Variance
Variance measures how far the values in a dataset are spread out from the mean. It is calculated by averaging the squared differences from the mean.
The formula for variance is:
Variance = (Σ(x - Mean)²) / n
In R, you can calculate variance using the var()
function:
# Calculate variance
variance_value <- var(data)
variance_value
The result will be the variance of the data
vector. Note that by default, var()
calculates the sample variance (dividing by n - 1
).
Standard Deviation
The standard deviation is the square root of the variance and provides a measure of the spread or dispersion of the dataset in the same units as the original data.
The formula for standard deviation is:
Standard Deviation = √Variance
In R, you can calculate standard deviation using the sd()
function:
# Calculate standard deviation
sd_value <- sd(data)
sd_value
The result will be the standard deviation of the data
vector.
Example: Calculating All Descriptive Statistics
Here’s an example that calculates the mean, median, variance, and standard deviation of a dataset:
# Example data
data <- c(5, 10, 15, 20, 25)
# Calculate mean
mean_value <- mean(data)
# Calculate median
median_value <- median(data)
# Calculate variance
variance_value <- var(data)
# Calculate standard deviation
sd_value <- sd(data)
# Print results
cat("Mean:", mean_value, "\n")
cat("Median:", median_value, "\n")
cat("Variance:", variance_value, "\n")
cat("Standard Deviation:", sd_value, "\n")
This code will print the mean, median, variance, and standard deviation of the data
vector.
Conclusion
Descriptive statistics provide a simple and effective way to summarize and understand the characteristics of a dataset. In R, the mean()
, median()
, var()
, and sd()
functions are easy to use and provide quick insights into the central tendency and variability of your data.
Inferential Statistics: Hypothesis Testing
Inferential statistics allow us to make conclusions about a population based on a sample of data. Hypothesis testing is a core concept in inferential statistics, which helps us determine whether there is enough evidence to support a specific hypothesis or claim about a population. In this section, we will cover common hypothesis tests such as the t-test, ANOVA, and Chi-Square test in R.
t-tests
A t-test is used to compare the means of two groups to determine if there is a statistically significant difference between them. It can be a one-sample t-test, independent two-sample t-test, or paired t-test.
One-Sample t-test
A one-sample t-test is used to compare the mean of a sample to a known value or population mean.
In R, you can perform a one-sample t-test using the t.test()
function:
# Sample data
data <- c(5, 10, 15, 20, 25)
# Perform one-sample t-test (compare sample mean to population mean 15)
t_test_result <- t.test(data, mu = 15)
t_test_result
The result will provide the t-statistic, p-value, confidence interval, and other details. You can interpret the p-value to determine whether the difference is statistically significant.
Two-Sample t-test
An independent two-sample t-test compares the means of two independent groups to determine if there is a significant difference between them.
# Sample data for two groups
group1 <- c(5, 10, 15, 20, 25)
group2 <- c(30, 35, 40, 45, 50)
# Perform independent two-sample t-test
t_test_result <- t.test(group1, group2)
t_test_result
The result will show if the means of the two groups are significantly different.
ANOVA (Analysis of Variance)
ANOVA is used to compare the means of three or more groups to determine if at least one group mean is different from the others. It tests the null hypothesis that all group means are equal.
In R, you can perform a one-way ANOVA using the aov()
function:
# Example data: three groups
group1 <- c(5, 10, 15, 20)
group2 <- c(25, 30, 35, 40)
group3 <- c(45, 50, 55, 60)
# Combine data into a single vector
data <- c(group1, group2, group3)
# Group labels
group_labels <- factor(c(rep("Group 1", length(group1)), rep("Group 2", length(group2)), rep("Group 3", length(group3))))
# Perform one-way ANOVA
anova_result <- aov(data ~ group_labels)
summary(anova_result)
The result will provide the F-statistic and the p-value. A significant p-value (typically < 0.05) indicates that there is a difference between at least one of the group means.
Chi-Square Test
The Chi-Square test is used to determine if there is a significant association between two categorical variables. It compares the observed frequencies with the expected frequencies under the null hypothesis that there is no association.
In R, you can perform a Chi-Square test using the chisq.test()
function:
# Example data: Contingency table of two categorical variables
observed <- matrix(c(10, 20, 30, 40), nrow = 2, byrow = TRUE)
# Perform Chi-Square test
chi_square_result <- chisq.test(observed)
chi_square_result
The result will provide the Chi-Square statistic, degrees of freedom, and p-value. A significant p-value indicates that the variables are not independent and there is an association between them.
Conclusion
Hypothesis testing is a powerful tool for making inferences about populations based on sample data. In R, you can perform common tests like the t-test, ANOVA, and Chi-Square test using simple functions like t.test()
, aov()
, and chisq.test()
. By interpreting the p-values and test statistics, you can determine whether the evidence supports or rejects your hypothesis about the data.
Correlation and Regression Analysis
Correlation and regression analysis are statistical methods used to understand relationships between variables. In this section, we will cover how to calculate correlations and perform regression analysis using R.
Correlation Analysis
Correlation measures the strength and direction of a relationship between two variables. The correlation coefficient, denoted by r
, ranges from -1 to 1. A positive value indicates a positive relationship, while a negative value indicates a negative relationship. A value of 0 means no linear relationship.
The formula for the correlation coefficient is:
r = Σ((X - X̄) * (Y - Ȳ)) / √(Σ(X - X̄)² * Σ(Y - Ȳ)²)
In R, you can calculate the correlation between two variables using the cor()
function:
# Example data
x <- c(1, 2, 3, 4, 5)
y <- c(2, 4, 6, 8, 10)
# Calculate correlation
correlation_result <- cor(x, y)
correlation_result
The result will provide the correlation coefficient r
. In this case, the correlation should be 1, indicating a perfect positive linear relationship.
Regression Analysis
Regression analysis is used to model the relationship between a dependent variable and one or more independent variables. The most basic form is simple linear regression, where one independent variable is used to predict the dependent variable.
The simple linear regression equation is:
Y = β₀ + β₁ * X + ε
Where:
Y
is the dependent variableβ₀
is the interceptβ₁
is the slopeX
is the independent variableε
is the error term
In R, you can perform simple linear regression using the lm()
function:
# Example data
x <- c(1, 2, 3, 4, 5)
y <- c(2, 4, 6, 8, 10)
# Perform linear regression
model <- lm(y ~ x)
# View regression summary
summary(model)
The lm()
function fits a linear model, and the summary()
function provides detailed information about the regression, including the intercept, slope, and p-values. In this case, the model will show a perfect fit with a slope of 2 and an intercept of 0.
Multiple Linear Regression
In multiple linear regression, you can model the relationship between a dependent variable and multiple independent variables.
The multiple linear regression equation is:
Y = β₀ + β₁ * X₁ + β₂ * X₂ + ... + βn * Xn + ε
In R, you can perform multiple linear regression in the same way as simple linear regression, but by adding more predictors:
# Example data
x1 <- c(1, 2, 3, 4, 5)
x2 <- c(10, 9, 8, 7, 6)
y <- c(2, 4, 6, 8, 10)
# Perform multiple linear regression
model_multiple <- lm(y ~ x1 + x2)
# View regression summary
summary(model_multiple)
The result will show the coefficients for both x1
and x2
and how they contribute to predicting y
.
Model Diagnostics
After performing regression analysis, it is essential to check the assumptions of the model, such as linearity, independence, homoscedasticity, and normality of residuals. You can use diagnostic plots to check these assumptions:
# Diagnostic plots
plot(model)
This will generate a set of plots that help assess the validity of the regression model.
Conclusion
Correlation and regression analysis are powerful techniques for understanding relationships between variables. In R, the cor()
function calculates correlation, while the lm()
function is used for performing simple and multiple linear regression. By interpreting the results, you can make predictions and assess the strength of relationships between variables in your data.
Probability Distributions
Probability distributions describe the likelihood of different outcomes in an experiment or process. In statistics, they are used to model real-world phenomena and assess uncertainty. R provides functions for working with both discrete and continuous probability distributions. In this section, we will cover key probability distributions, including the normal distribution, binomial distribution, and Poisson distribution, and how to work with them in R.
Continuous Probability Distributions
Continuous probability distributions represent outcomes that can take any value within a specified range. The most commonly used continuous distribution is the normal distribution.
Normal Distribution
The normal distribution is a symmetric, bell-shaped distribution that is widely used in statistics. It is characterized by its mean (μ) and standard deviation (σ). The probability density function (PDF) of the normal distribution is:
f(x) = (1 / (σ * √(2π))) * exp(-0.5 * ((x - μ) / σ)^2)
In R, you can work with the normal distribution using the following functions:
dnorm(x, mean, sd)
: Probability density function (PDF)pnorm(q, mean, sd)
: Cumulative distribution function (CDF)qnorm(p, mean, sd)
: Quantile functionrnorm(n, mean, sd)
: Random sampling
Example of generating random numbers from a normal distribution:
# Generate 1000 random numbers from a normal distribution with mean=0 and sd=1
random_numbers <- rnorm(1000, mean = 0, sd = 1)
# Plot histogram of random numbers
hist(random_numbers, main = "Histogram of Normally Distributed Data", xlab = "Value", col = "blue", breaks = 30)
Exponential Distribution
The exponential distribution models the time between events in a Poisson process. It is often used to model waiting times or lifetimes of objects. The probability density function (PDF) of the exponential distribution is:
f(x) = λ * exp(-λx), x ≥ 0
In R, you can work with the exponential distribution using these functions:
dexp(x, rate)
: PDFpexp(q, rate)
: CDFqexp(p, rate)
: Quantile functionrexp(n, rate)
: Random sampling
Example of generating random numbers from an exponential distribution:
# Generate 1000 random numbers from an exponential distribution with rate=1
random_numbers_exp <- rexp(1000, rate = 1)
# Plot histogram of random numbers
hist(random_numbers_exp, main = "Histogram of Exponentially Distributed Data", xlab = "Value", col = "green", breaks = 30)
Discrete Probability Distributions
Discrete probability distributions represent outcomes that can only take a finite number of values. Common discrete distributions include the binomial distribution and the Poisson distribution.
Binomial Distribution
The binomial distribution is used to model the number of successes in a fixed number of independent Bernoulli trials (e.g., coin flips). The probability mass function (PMF) is:
P(X = k) = (n choose k) * p^k * (1 - p)^(n - k)
Where n
is the number of trials, p
is the probability of success, and k
is the number of successes.
In R, you can work with the binomial distribution using these functions:
dbinom(x, size, prob)
: Probability mass function (PMF)pbinom(q, size, prob)
: Cumulative distribution function (CDF)qbinom(p, size, prob)
: Quantile functionrbinom(n, size, prob)
: Random sampling
Example of generating random numbers from a binomial distribution:
# Generate 1000 random numbers from a binomial distribution with size=10 and probability=0.5
random_numbers_binom <- rbinom(1000, size = 10, prob = 0.5)
# Plot histogram of random numbers
hist(random_numbers_binom, main = "Histogram of Binomially Distributed Data", xlab = "Number of Successes", col = "red", breaks = 30)
Poisson Distribution
The Poisson distribution models the number of events occurring within a fixed interval of time or space. It is often used in scenarios such as modeling the number of phone calls received by a call center within an hour. The probability mass function (PMF) is:
P(X = k) = (λ^k * exp(-λ)) / k!
Where λ
is the rate of occurrence, and k
is the number of events.
In R, you can work with the Poisson distribution using these functions:
dpois(x, lambda)
: PMFppois(q, lambda)
: CDFqpois(p, lambda)
: Quantile functionrpois(n, lambda)
: Random sampling
Example of generating random numbers from a Poisson distribution:
# Generate 1000 random numbers from a Poisson distribution with lambda=3
random_numbers_pois <- rpois(1000, lambda = 3)
# Plot histogram of random numbers
hist(random_numbers_pois, main = "Histogram of Poisson Distributed Data", xlab = "Number of Events", col = "purple", breaks = 30)
Conclusion
Probability distributions are essential for modeling uncertainty and making inferences based on data. In R, you can easily work with continuous and discrete distributions such as the normal, exponential, binomial, and Poisson distributions. By using functions like dnorm()
, dbinom()
, and rpois()
, you can calculate probabilities, generate random samples, and visualize data from different distributions.
String Operations
String operations in R allow you to manipulate and work with text data. These functions help you perform common tasks such as concatenating strings, extracting substrings, searching for patterns, and more. In this section, we will cover some essential string operations in R using functions like paste()
, substr()
, and grep()
.
Concatenating Strings: paste()
The paste()
function is used to concatenate multiple strings into one. You can specify a separator between the strings by using the sep
argument. By default, paste()
separates the strings with a space.
Syntax:
paste(..., sep = " ", collapse = NULL)
Example:
# Concatenating two strings
greeting <- paste("Hello", "World")
print(greeting) # Output: "Hello World"
# Concatenating with a custom separator
greeting_custom <- paste("Hello", "World", sep = "-")
print(greeting_custom) # Output: "Hello-World"
Extracting Substrings: substr()
The substr()
function allows you to extract a portion of a string based on the specified start and end positions.
Syntax:
substr(x, start, stop)
Example:
# Extract a substring from a string
text <- "Hello World"
substring <- substr(text, start = 1, stop = 5)
print(substring) # Output: "Hello"
Searching for Patterns: grep()
The grep()
function searches for patterns within a character vector and returns the indices of the elements that match the pattern. You can use regular expressions (regex) to define the pattern you want to search for.
Syntax:
grep(pattern, x, ignore.case = FALSE, value = FALSE, fixed = FALSE)
Example:
# Search for a pattern in a character vector
text_vector <- c("apple", "banana", "cherry", "apricot")
matches <- grep("ap", text_vector)
print(matches) # Output: 1 4 (indices of "apple" and "apricot")
# Search for a pattern and return the matching values
matches_values <- grep("ap", text_vector, value = TRUE)
print(matches_values) # Output: "apple" "apricot"
Case Insensitive Search: grep() with ignore.case
By setting the ignore.case
argument to TRUE
, you can perform a case-insensitive search.
Example:
# Case-insensitive search
matches_case_insensitive <- grep("AP", text_vector, ignore.case = TRUE)
print(matches_case_insensitive) # Output: 1 4 (indices of "apple" and "apricot")
Counting Matches: gregexpr()
If you want to count the number of matches of a particular pattern within a string, you can use the gregexpr()
function. It returns the positions of all matches, and you can count the number of matches using length()
.
Syntax:
gregexpr(pattern, text)
Example:
# Count occurrences of a pattern
count_matches <- gregexpr("ap", "apple apricot apple")
match_count <- length(unlist(regmatches("apple apricot apple", count_matches)))
print(match_count) # Output: 3
String Operations in Summary
paste()
: Concatenates multiple strings into one, with optional separators.substr()
: Extracts a substring from a string based on given positions.grep()
: Searches for a pattern in a character vector and returns the indices or the values that match.gregexpr()
: Finds the positions of all matches of a pattern in a string and counts the matches.
These functions make string manipulation in R easy and efficient, allowing you to customize, search, and extract information from text data.
String Formatting and Manipulation
String formatting and manipulation in R allows you to work with text data more efficiently. You can format strings, manipulate the case, change whitespace, substitute parts of a string, and more. This section covers key functions for string formatting and manipulation in R, including sprintf()
, toupper()
, tolower()
, sub()
, and gsub()
.
Formatting Strings: sprintf()
The sprintf()
function is used for string formatting, allowing you to create formatted strings with placeholders. It works similarly to the printf()
function in other programming languages, where you can specify the format for different types of data.
Syntax:
sprintf(format, ...)
Example:
# Using sprintf() for string formatting
name <- "John"
age <- 25
formatted_string <- sprintf("My name is %s and I am %d years old", name, age)
print(formatted_string) # Output: "My name is John and I am 25 years old"
Changing Case: toupper() and tolower()
R provides two functions, toupper()
and tolower()
, to change the case of a string. toupper()
converts a string to uppercase, while tolower()
converts a string to lowercase.
Syntax:
toupper(x)
tolower(x)
Example:
# Converting to uppercase and lowercase
text <- "Hello World"
upper_text <- toupper(text)
lower_text <- tolower(text)
print(upper_text) # Output: "HELLO WORLD"
print(lower_text) # Output: "hello world"
Substituting Parts of a String: sub() and gsub()
The sub()
and gsub()
functions are used for replacing parts of a string that match a specified pattern. The difference is that sub()
replaces only the first occurrence, while gsub()
replaces all occurrences of the pattern.
Syntax:
sub(pattern, replacement, x)
gsub(pattern, replacement, x)
Example:
# Replace the first occurrence of "apple" with "orange"
text <- "apple banana apple"
new_text_sub <- sub("apple", "orange", text)
print(new_text_sub) # Output: "orange banana apple"
# Replace all occurrences of "apple" with "orange"
new_text_gsub <- gsub("apple", "orange", text)
print(new_text_gsub) # Output: "orange banana orange"
Trimming Whitespace: trimws()
The trimws()
function is used to remove leading and trailing whitespace from a string.
Syntax:
trimws(x, which = c("both", "left", "right"))
Example:
# Removing leading and trailing whitespace
text_with_spaces <- " Hello World! "
trimmed_text <- trimws(text_with_spaces)
print(trimmed_text) # Output: "Hello World!"
String Padding: str_pad()
The str_pad()
function from the stringr
package is used to pad a string to a specified length with a character, such as spaces or zeros.
Syntax:
str_pad(string, width, side = c("left", "right", "both"), pad = " ")
Example:
# Using str_pad to pad a string with spaces
library(stringr)
padded_text <- str_pad("123", width = 5, side = "left", pad = "0")
print(padded_text) # Output: "00123"
String Manipulation in Summary
sprintf()
: Formats strings with placeholders, similar toprintf()
.toupper()
andtolower()
: Convert a string to uppercase or lowercase.sub()
andgsub()
: Replace parts of a string based on a pattern, withgsub()
replacing all occurrences.trimws()
: Removes leading and trailing whitespace from a string.str_pad()
: Pads a string to a specified length with a character.
These functions enable efficient and flexible string manipulation, making it easier to format, clean, and modify text data in R.
Regular Expressions in R
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. In R, regular expressions are often used with functions like grep()
, grepl()
, sub()
, and gsub()
to search, extract, replace, or test for patterns in strings.
Basic Syntax of Regular Expressions
Regular expressions use special characters to define search patterns. Some of the most common symbols include:
^
: Matches the beginning of a string.$
: Matches the end of a string..
: Matches any character except a newline.*
: Matches zero or more of the preceding element.+
: Matches one or more of the preceding element.?
: Matches zero or one of the preceding element.[ ]
: Matches any one of the characters inside the brackets.[^ ]
: Matches any character not inside the brackets.: Alternation (logical OR) between two patterns.
Using grep() and grepl()
The grep()
function is used to search for patterns in text and return the indices of the matches, while grepl()
returns a logical vector indicating whether the pattern is found in each element of a vector.
Syntax:
grep(pattern, x)
grepl(pattern, x)
Example:
# Using grep() to find the index of matching elements
text <- c("apple", "banana", "cherry", "apple pie")
indices <- grep("apple", text)
print(indices) # Output: 1 4
# Using grepl() to check if the pattern is present
match_logical <- grepl("apple", text)
print(match_logical) # Output: TRUE FALSE FALSE TRUE
Using sub() and gsub() for Substitution
The sub()
and gsub()
functions are used to replace patterns in a string. The difference between them is that sub()
replaces only the first occurrence of the pattern, while gsub()
replaces all occurrences.
Syntax:
sub(pattern, replacement, x)
gsub(pattern, replacement, x)
Example:
# Replacing the first occurrence of the pattern
text <- "apple banana apple"
result_sub <- sub("apple", "orange", text)
print(result_sub) # Output: "orange banana apple"
# Replacing all occurrences of the pattern
result_gsub <- gsub("apple", "orange", text)
print(result_gsub) # Output: "orange banana orange"
Extracting Matches with regexpr() and gregexpr()
The regexpr()
and gregexpr()
functions are used to extract matching substrings from a string. regexpr()
returns the first match, while gregexpr()
returns all matches.
Syntax:
regexpr(pattern, x)
gregexpr(pattern, x)
Example:
# Using regexpr() to find the first match
text <- "apple banana apple"
first_match <- regexpr("apple", text)
print(first_match) # Output: 1
# Using gregexpr() to find all matches
all_matches <- gregexpr("apple", text)
print(all_matches) # Output: list of positions
Using regmatches() to Extract Substrings
The regmatches()
function is used in combination with regexpr()
or gregexpr()
to extract substrings that match a given regular expression pattern.
Syntax:
regmatches(x, m)
Example:
# Extracting matched substrings
matches <- regmatches(text, gregexpr("apple", text))
print(matches) # Output: list with the matched substrings
Using Regular Expressions in Data Manipulation
Regular expressions are commonly used in data manipulation tasks like cleaning data, filtering, and transforming text fields. The stringr
and tidyverse
packages provide additional functions for working with regular expressions, such as str_detect()
, str_replace()
, and str_extract()
.
Summary of Key Functions
grep()
: Finds the indices of matches for a pattern.grepl()
: Returns a logical vector indicating whether a pattern is found.sub()
: Replaces the first occurrence of a pattern.gsub()
: Replaces all occurrences of a pattern.regexpr()
: Finds the position of the first match.gregexpr()
: Finds the positions of all matches.regmatches()
: Extracts matched substrings.
Regular expressions are an essential tool for text processing and data cleaning in R. Mastering regular expressions allows you to perform complex pattern matching and text manipulation tasks with ease.
Working with Dates (Date Class)
In R, dates are handled using the Date
class, which represents calendar dates without time. The Date
class is part of R's base package, and it allows you to perform various operations with date objects, such as comparing, adding, and formatting dates. Dates in R are typically stored as the number of days since January 1, 1970 (the Unix epoch).
Creating Date Objects
You can create date objects in R using the as.Date()
function. The default date format is "YYYY-MM-DD"
, but you can specify other formats using the format
argument.
Syntax:
as.Date(x, format = "%Y-%m-%d")
Example:
# Creating a date object from a string
date1 <- as.Date("2025-01-23")
print(date1) # Output: "2025-01-23"
If the date is in a different format, specify the format argument:
# Creating a date object with a custom date format
date2 <- as.Date("23/01/2025", format = "%d/%m/%Y")
print(date2) # Output: "2025-01-23"
Extracting Components of Dates
You can extract individual components like the year, month, and day from a date object using functions like format()
.
Syntax:
format(x, format = "%Y") # Extracts the year
format(x, format = "%m") # Extracts the month
format(x, format = "%d") # Extracts the day
Example:
# Extracting the year, month, and day
year <- format(date1, "%Y")
month <- format(date1, "%m")
day <- format(date1, "%d")
print(year) # Output: "2025"
print(month) # Output: "01"
print(day) # Output: "23"
Performing Date Calculations
R allows you to perform calculations on dates, such as adding or subtracting days, comparing dates, or finding the difference between two dates.
Adding and Subtracting Days
You can add or subtract days from a date object by using the +
and -
operators, respectively.
# Adding 10 days to a date
new_date <- date1 + 10
print(new_date) # Output: "2025-02-02"
# Subtracting 5 days from a date
earlier_date <- date1 - 5
print(earlier_date) # Output: "2025-01-18"
Finding the Difference Between Dates
The difference between two date objects can be calculated using the -
operator, which returns an object of class difftime
representing the time difference in days.
# Finding the difference between two dates
date3 <- as.Date("2025-02-01")
date_diff <- date3 - date1
print(date_diff) # Output: Time difference of 9 days
Handling Time Zones
R's Date
class does not include time information, so time zones do not apply. If you need to handle time zones, you can use the POSIXct
or POSIXlt
classes, which handle both date and time information, including time zone adjustments.
Formatting Dates
You can format dates in R using the format()
function. This allows you to display dates in a custom format.
Common date format codes include:
%Y
: Year with century (e.g., 2025)%m
: Month (01–12)%d
: Day of the month (01–31)%a
: Abbreviated weekday name (e.g., Mon)%A
: Full weekday name (e.g., Monday)
Example:
# Formatting a date in different formats
formatted_date <- format(date1, "%A, %d %B %Y")
print(formatted_date) # Output: "Thursday, 23 January 2025"
Summary of Key Functions
as.Date()
: Converts a string or number to a date object.format()
: Extracts or formats specific components of a date.+ / -
: Adds or subtracts days from a date.difftime()
: Calculates the difference between two dates.format()
: Formats a date in a custom string format.
Working with dates in R is essential for time-based analysis, and understanding the Date
class allows you to manipulate and analyze date information efficiently.
Handling Time (POSIXct and POSIXlt Classes)
In R, the POSIXct
and POSIXlt
classes are used to represent date-time objects, which store both the date and the time. These classes are essential for working with time-based data, such as timestamps, and allow you to perform operations that involve both the date and the time components.
POSIXct Class
The POSIXct
class represents the number of seconds since the Unix epoch (January 1, 1970). It is a simple and efficient class for representing date-time objects, particularly when working with large datasets.
To create a POSIXct
object, use the as.POSIXct()
function:
as.POSIXct(x, format = "%Y-%m-%d %H:%M:%S", tz = "UTC")
Example:
# Creating a POSIXct object
datetime1 <- as.POSIXct("2025-01-23 14:30:00", format = "%Y-%m-%d %H:%M:%S")
print(datetime1) # Output: "2025-01-23 14:30:00 UTC"
POSIXlt Class
The POSIXlt
class is a list-like structure that stores individual components of a date-time object, such as the year, month, day, hour, minute, second, and time zone. It allows for easier extraction and manipulation of date-time components.
To create a POSIXlt
object, use the as.POSIXlt()
function:
as.POSIXlt(x, format = "%Y-%m-%d %H:%M:%S", tz = "UTC")
Example:
# Creating a POSIXlt object
datetime2 <- as.POSIXlt("2025-01-23 14:30:00", format = "%Y-%m-%d %H:%M:%S")
print(datetime2) # Output: "2025-01-23 14:30:00 UTC"
Differences Between POSIXct and POSIXlt
While both POSIXct
and POSIXlt
represent date-time information, they differ in how they store the data:
POSIXct
: Stores the number of seconds since the Unix epoch. It is a compact and efficient format, suitable for large datasets and operations that require fast processing.POSIXlt
: Stores the components of a date-time object (year, month, day, hour, minute, second) in a list format. It is more flexible when you need to extract or manipulate individual components of the date-time.
Extracting Components of Date-Time Objects
Both POSIXct
and POSIXlt
objects allow you to extract individual components, but they do so differently:
For POSIXlt
objects, you can directly access components like the year, month, day, etc., using the $
operator:
# Extracting components from POSIXlt
year <- datetime2$year + 1900 # Adding 1900 to get the correct year
month <- datetime2$mon + 1 # Adding 1 to get the correct month
day <- datetime2$mday
hour <- datetime2$hour
minute <- datetime2$min
second <- datetime2$sec
print(year) # Output: 2025
print(month) # Output: 1
print(day) # Output: 23
print(hour) # Output: 14
print(minute) # Output: 30
print(second) # Output: 0
For POSIXct
objects, you can use the format()
function to extract specific components:
# Extracting components from POSIXct using format()
year_ct <- format(datetime1, "%Y")
month_ct <- format(datetime1, "%m")
day_ct <- format(datetime1, "%d")
hour_ct <- format(datetime1, "%H")
minute_ct <- format(datetime1, "%M")
second_ct <- format(datetime1, "%S")
print(year_ct) # Output: "2025"
print(month_ct) # Output: "01"
print(day_ct) # Output: "23"
print(hour_ct) # Output: "14"
print(minute_ct) # Output: "30"
print(second_ct) # Output: "00"
Formatting Date-Time Objects
You can format date-time objects in R using the format()
function. This allows you to display date-time objects in a custom format, such as displaying only the time or formatting the date in a specific way.
Common time format codes include:
%Y
: Year with century (e.g., 2025)%m
: Month (01–12)%d
: Day of the month (01–31)%H
: Hour (00–23)%M
: Minute (00–59)%S
: Second (00–59)
Example:
# Formatting a POSIXct object
formatted_time <- format(datetime1, "%Y-%m-%d %H:%M:%S")
print(formatted_time) # Output: "2025-01-23 14:30:00"
Time Zones
Both POSIXct
and POSIXlt
objects can handle time zones. You can specify the time zone when creating a date-time object using the tz
argument:
# Creating a POSIXct object with a time zone
datetime3 <- as.POSIXct("2025-01-23 14:30:00", tz = "America/New_York")
print(datetime3) # Output: "2025-01-23 14:30:00 EST"
Summary of Key Functions
as.POSIXct()
: Converts a string or number to aPOSIXct
object.as.POSIXlt()
: Converts a string or number to aPOSIXlt
object.format()
: Extracts or formats specific components of a date-time object.tz
: Specifies or modifies the time zone of a date-time object.
The POSIXct
and POSIXlt
classes are powerful tools for working with both date and time in R, allowing you to perform a wide range of operations, such as extracting individual components, formatting date-time objects, and handling time zones.
Date and Time Formatting (strptime(), format())
In R, you can format date and time objects using the strptime()
and format()
functions. These functions allow you to control how date and time values are parsed and displayed, making it easy to work with different formats for input or output.
strptime(): Parsing Date-Time Strings
The strptime()
function is used to convert date-time strings into R's date-time objects. You need to specify the format of the date-time string using format codes so that R can correctly interpret the string.
The basic syntax for strptime()
is as follows:
strptime(x, format, tz = "")
Where:
x
: The character string representing the date-time.format
: A string specifying the format of the date-time inx
.tz
: An optional argument to specify the time zone.
Example:
# Converting a date-time string to POSIXlt using strptime
datetime_str <- "2025-01-23 14:30:00"
datetime_obj <- strptime(datetime_str, format = "%Y-%m-%d %H:%M:%S")
print(datetime_obj) # Output: "2025-01-23 14:30:00"
In the above example, the strptime()
function converts the date-time string "2025-01-23 14:30:00"
into a POSIXlt
object, specifying the format %Y-%m-%d %H:%M:%S
which represents the year, month, day, hour, minute, and second.
format(): Formatting Date-Time Objects
The format()
function is used to convert date-time objects into a string with a specified format. You can use format codes to display the date and time in different styles based on your requirements.
The basic syntax for format()
is as follows:
format(x, format, tz = "")
Where:
x
: The date-time object to be formatted.format
: A string specifying the desired output format.tz
: An optional argument to specify the time zone.
Example:
# Formatting a POSIXlt object using format
formatted_datetime <- format(datetime_obj, "%Y-%m-%d %H:%M:%S")
print(formatted_datetime) # Output: "2025-01-23 14:30:00"
In this example, the format()
function is used to format the POSIXlt
object datetime_obj
into the string "2025-01-23 14:30:00"
using the format %Y-%m-%d %H:%M:%S
.
Common Format Codes
Both strptime()
and format()
use format codes to specify how date and time values should be parsed or displayed. Here are some common format codes:
%Y
: Year with century (e.g., 2025)%m
: Month (01–12)%d
: Day of the month (01–31)%H
: Hour (00–23)%M
: Minute (00–59)%S
: Second (00–59)%a
: Abbreviated weekday name (e.g., Mon)%A
: Full weekday name (e.g., Monday)%b
: Abbreviated month name (e.g., Jan)%B
: Full month name (e.g., January)
Examples of format codes:
# Formatting date-time with different format codes
formatted_date <- format(datetime_obj, "%A, %B %d, %Y")
print(formatted_date) # Output: "Thursday, January 23, 2025"
formatted_time <- format(datetime_obj, "%H:%M:%S")
print(formatted_time) # Output: "14:30:00"
Parsing and Formatting Example
Here’s an example that demonstrates both parsing a date-time string using strptime()
and formatting it using format()
:
# Parsing a date-time string with strptime
parsed_datetime <- strptime("2025-01-23 14:30:00", format = "%Y-%m-%d %H:%M:%S")
print(parsed_datetime) # Output: "2025-01-23 14:30:00"
# Formatting the parsed date-time object
formatted_datetime <- format(parsed_datetime, "%A, %B %d, %Y")
print(formatted_datetime) # Output: "Thursday, January 23, 2025"
Time Zones
Both strptime()
and format()
can handle time zones. When formatting or parsing date-time objects, you can specify the time zone using the tz
argument:
# Parsing with a time zone
datetime_with_tz <- strptime("2025-01-23 14:30:00", format = "%Y-%m-%d %H:%M:%S", tz = "America/New_York")
print(datetime_with_tz) # Output: "2025-01-23 14:30:00 EST"
# Formatting with a time zone
formatted_with_tz <- format(datetime_with_tz, "%Y-%m-%d %H:%M:%S %Z")
print(formatted_with_tz) # Output: "2025-01-23 14:30:00 EST"
Summary of Key Functions
strptime()
: Converts a date-time string to a date-time object based on the specified format.format()
: Converts a date-time object to a string based on the specified format.
In summary, the strptime()
and format()
functions are powerful tools in R for parsing and formatting date-time values. These functions make it easy to handle and display date-time data in a variety of formats, giving you flexibility when working with time-based information.
Working with Large Datasets
In R, working with large datasets can be challenging due to memory limitations and processing time. However, R provides several methods and packages designed to handle large datasets efficiently. This section covers strategies, tools, and best practices for working with large datasets in R.
Challenges with Large Datasets
When working with large datasets, you may face the following challenges:
- Memory Limitations: R stores data in memory, which can cause issues if the dataset exceeds your computer's available memory.
- Slow Processing: Operations on large datasets can be slow, especially when using base R functions on large objects.
- Data Size Limits: Large datasets can be difficult to visualize or summarize, making analysis more complex.
Strategies for Handling Large Datasets
Here are some strategies that can help you handle large datasets in R:
1. Use Data Table Package
The data.table
package is a fast and memory-efficient alternative to data frames. It is designed for large datasets and allows efficient indexing, joining, and manipulation of data.
To install and load the data.table
package:
# Installing and loading the data.table package
install.packages("data.table")
library(data.table)
Example of creating a data table:
# Creating a data table
dt <- data.table(a = 1:1000000, b = rnorm(1000000))
head(dt) # Display the first few rows of the data table
2. Use fread() for Faster Data Import
The fread()
function from the data.table
package is much faster than the base R read.csv()
function for reading large CSV files.
Example of using fread()
to import a large CSV file:
# Importing large CSV files using fread
large_data <- fread("large_data.csv")
head(large_data)
fread()
automatically detects the column types, making it more efficient than read.csv()
.
3. Use Chunking for Large Files
When dealing with extremely large datasets that can't be loaded into memory at once, you can process the data in smaller chunks. This technique is called "chunking" and is useful when performing read or write operations on large files.
Example of reading a large file in chunks:
# Reading a large file in chunks
library(readr)
chunk_size <- 100000 # Define the chunk size
con <- file("large_data.csv", "r") # Open a connection to the file
while(length(chunk <- read.csv(con, nrows = chunk_size)) > 0) {
# Process each chunk
print(head(chunk)) # Example of processing the chunk
}
close(con) # Close the file connection
4. Use Disk-Based Storage with ff Package
The ff
package allows you to store data on disk instead of in memory. This is particularly useful for datasets that are larger than your available RAM.
To install and load the ff
package:
# Installing and loading the ff package
install.packages("ff")
library(ff)
Example of creating a large ff object:
# Creating an ff object
large_ff_data <- ff(1:1000000)
print(large_ff_data)
5. Use HDF5 Format for Large Datasets
The HDF5 format allows you to store large datasets efficiently, and the rhdf5
package in R can be used to read and write data in this format.
To install and load the rhdf5
package:
# Installing and loading the rhdf5 package
install.packages("rhdf5", repos = "https://bioconductor.org/packages/release/bioc")
library(rhdf5)
Example of creating and reading HDF5 data:
# Writing data to HDF5 format
h5createFile("large_data.h5")
h5write(large_data, "large_data.h5", "dataset")
# Reading data from HDF5 format
data_from_hdf5 <- h5read("large_data.h5", "dataset")
head(data_from_hdf5)
Best Practices for Working with Large Datasets
Here are some best practices to help you work efficiently with large datasets in R:
- Use efficient data structures: Use
data.table
instead of data frames, and consider using packages likeff
andbigmemory
for memory-efficient operations. - Clean your data: Ensure that your dataset is properly cleaned and formatted to avoid unnecessary processing overhead.
- Use parallel processing: For computationally expensive operations, consider using parallel computing techniques, such as the
parallel
package, to speed up processing. - Optimize R code: Profile your R code using tools like
Rprof
to identify bottlenecks and optimize code performance.
Summary
Working with large datasets in R requires efficient methods and tools to avoid memory issues and optimize processing time. By using packages like data.table
, ff
, and rhdf5
, along with chunking and parallel processing, you can handle large datasets effectively and perform analysis without running into performance issues.
Data Reshaping (spread(), gather())
Data reshaping is a crucial part of data cleaning and transformation. In R, the tidyr
package provides functions like spread()
and gather()
to reshape your data efficiently. These functions allow you to manipulate the structure of your data to make it easier to analyze and visualize.
Understanding Data Reshaping
Reshaping data involves changing the format of your data from wide to long or vice versa. This is often necessary when data is not in the format that is suitable for analysis or visualization. The spread()
function turns long data into wide format, while gather()
does the opposite, converting wide data into long format.
Reshaping Data from Long to Wide: spread()
The spread()
function is used to convert long-format data into wide-format data by spreading one or more key-value pairs across multiple columns.
To install and load the tidyr
package:
# Installing and loading the tidyr package
install.packages("tidyr")
library(tidyr)
Example of reshaping data from long to wide using spread()
:
# Example data in long format
long_data <- data.frame(
Name = c("Alice", "Bob", "Alice", "Bob"),
Subject = c("Math", "Math", "Science", "Science"),
Score = c(85, 90, 88, 92)
)
# Using spread() to reshape data from long to wide
wide_data <- spread(long_data, key = Subject, value = Score)
print(wide_data)
Output:
# Name Math Science
# 1 Alice 85 88
# 2 Bob 90 92
Reshaping Data from Wide to Long: gather()
The gather()
function is used to reshape wide-format data into long-format data by gathering columns into key-value pairs.
Example of reshaping data from wide to long using gather()
:
# Example data in wide format
wide_data <- data.frame(
Name = c("Alice", "Bob"),
Math = c(85, 90),
Science = c(88, 92)
)
# Using gather() to reshape data from wide to long
long_data <- gather(wide_data, key = "Subject", value = "Score", Math, Science)
print(long_data)
Output:
# Name Subject Score
# 1 Alice Math 85
# 2 Bob Math 90
# 3 Alice Science 88
# 4 Bob Science 92
Key Parameters in spread()
and gather()
Both spread()
and gather()
have key parameters:
- key: The column that will hold the new variable names (in the case of
spread()
) or the column that will hold the variable names to be gathered (in the case ofgather()
). - value: The column that will hold the values (in the case of
spread()
) or the values corresponding to the gathered columns (in the case ofgather()
).
Additional Example: Using spread()
and gather()
with Real Data
Here is an example of using spread()
and gather()
with a dataset containing survey data on product ratings:
# Example of survey data
survey_data <- data.frame(
Product = c("A", "B", "C", "A", "B", "C"),
Rating = c(5, 3, 4, 4, 5, 3),
Respondent = c("John", "John", "John", "Jane", "Jane", "Jane")
)
# Reshaping survey data from long to wide (spread)
wide_survey <- spread(survey_data, key = Product, value = Rating)
print(wide_survey)
# Reshaping survey data from wide to long (gather)
long_survey <- gather(wide_survey, key = "Product", value = "Rating", A, B, C)
print(long_survey)
Summary
Reshaping data is an essential task in data analysis, and the spread()
and gather()
functions in the tidyr
package allow you to easily convert between long and wide data formats. These functions are particularly useful when preparing data for analysis or visualization, as they can help you organize your data in a way that is easier to work with.
Pivot Tables in R
A pivot table is a data summarization tool used in data analysis. It allows you to summarize and aggregate data by transforming it into a more readable format. In R, you can easily create pivot tables using the tidyverse
package, particularly the dplyr
and tidyr
functions, along with the pivot_wider()
and pivot_longer()
functions from the tidyr
package.
Understanding Pivot Tables
A pivot table allows you to summarize data in a table format by applying aggregation functions such as sum, mean, or count to the data. You can reshape the data by specifying which variables will be rows, columns, and values, allowing you to better understand trends, patterns, and distributions in your data.
Creating Pivot Tables using pivot_wider()
and pivot_longer()
In R, the tidyr
package provides the pivot_wider()
and pivot_longer()
functions for creating pivot tables. The pivot_wider()
function reshapes the data from long to wide format, and the pivot_longer()
function does the opposite, converting wide-format data into long format.
Example 1: Pivoting Data from Long to Wide using pivot_wider()
The pivot_wider()
function converts long-format data into wide-format data by spreading the values of a column into multiple columns.
# Loading the necessary package
library(tidyr)
# Example data in long format
long_data <- data.frame(
Name = c("Alice", "Bob", "Alice", "Bob"),
Subject = c("Math", "Math", "Science", "Science"),
Score = c(85, 90, 88, 92)
)
# Using pivot_wider() to reshape data from long to wide
wide_data <- pivot_wider(long_data, names_from = Subject, values_from = Score)
print(wide_data)
Output:
# Name Math Science
# 1 Alice 85 88
# 2 Bob 90 92
Example 2: Pivoting Data from Wide to Long using pivot_longer()
The pivot_longer()
function is used to convert wide-format data into long-format data by gathering multiple columns into a single column.
# Example data in wide format
wide_data <- data.frame(
Name = c("Alice", "Bob"),
Math = c(85, 90),
Science = c(88, 92)
)
# Using pivot_longer() to reshape data from wide to long
long_data <- pivot_longer(wide_data, cols = c(Math, Science), names_to = "Subject", values_to = "Score")
print(long_data)
Output:
# Name Subject Score
# 1 Alice Math 85
# 2 Bob Math 90
# 3 Alice Science 88
# 4 Bob Science 92
Using Aggregation Functions with Pivot Tables
When creating pivot tables, you may want to apply aggregation functions like sum, mean, or count to the data. You can use the summarise()
function from dplyr
in combination with the pivot_wider()
function to create pivot tables with aggregated values.
Example: Aggregating data with a pivot table:
# Example data
data <- data.frame(
Name = c("Alice", "Bob", "Alice", "Bob", "Alice"),
Subject = c("Math", "Math", "Science", "Science", "Math"),
Score = c(85, 90, 88, 92, 87)
)
# Aggregating data and creating a pivot table
pivot_table <- data %>%
group_by(Name) %>%
summarise(
Math_Avg = mean(Score[Subject == "Math"]),
Science_Avg = mean(Score[Subject == "Science"])
)
print(pivot_table)
Output:
# Name Math_Avg Science_Avg
# 1 Alice 86.0 88.0
# 2 Bob 90.0 92.0
Summary
Creating pivot tables in R is an efficient way to summarize and aggregate data. The pivot_wider()
and pivot_longer()
functions in the tidyr
package allow you to reshape data between long and wide formats. Additionally, you can use aggregation functions such as mean()
, sum()
, and count()
to summarize data within pivot tables, enabling more insightful analyses of your dataset.
Data Aggregation in R
Data aggregation is the process of summarizing or grouping data to provide insights into certain aspects of the data. In R, you can perform data aggregation using various functions such as aggregate()
, group_by()
from dplyr
, and summarise()
.
Using aggregate()
Function
The aggregate()
function is a built-in function in R that allows you to perform aggregation operations on a dataset. It enables grouping of data by one or more variables and then applies a function like mean
, sum
, or count
to each group.
Syntax:
aggregate(x, by, FUN, ...)
x
: The data to be aggregated.by
: A list of grouping variables.FUN
: The function to apply (e.g.,mean
,sum
, etc.).
Example 1: Aggregating Data using aggregate()
In this example, we will aggregate a dataset by the Category
column and calculate the mean
of the Value
column for each category.
# Example data
data <- data.frame(
Category = c("A", "B", "A", "B", "A", "B"),
Value = c(10, 20, 30, 40, 50, 60)
)
# Aggregating data by Category and calculating the mean of Value
aggregated_data <- aggregate(Value ~ Category, data = data, FUN = mean)
print(aggregated_data)
Output:
# Category Value
# 1 A 30
# 2 B 40
Using dplyr
for Data Aggregation
The dplyr
package provides more intuitive and flexible functions for data aggregation. The group_by()
function is used to group data by one or more variables, and the summarise()
function applies aggregation functions like mean
or sum
to each group.
Syntax:
data %>% group_by(variable) %>% summarise(aggregation_function)
Example 2: Aggregating Data using dplyr
In this example, we will use dplyr
to group the dataset by Category
and calculate the sum of Value
for each category.
# Loading the dplyr package
library(dplyr)
# Aggregating data by Category and calculating the sum of Value
aggregated_data_dplyr <- data %>%
group_by(Category) %>%
summarise(Sum_Value = sum(Value))
print(aggregated_data_dplyr)
Output:
# # A tibble: 2 x 2
# Category Sum_Value
#
# 1 A 90
# 2 B 120
Using Multiple Aggregations in dplyr
With dplyr
, you can also perform multiple aggregation operations at once. Here’s an example where we calculate both the mean
and sum
of the Value
column for each Category
.
# Aggregating data by Category and calculating both mean and sum of Value
aggregated_data_multiple <- data %>%
group_by(Category) %>%
summarise(
Mean_Value = mean(Value),
Sum_Value = sum(Value)
)
print(aggregated_data_multiple)
Output:
# # A tibble: 2 x 3
# Category Mean_Value Sum_Value
#
# 1 A 30 90
# 2 B 40 120
Using data.table
for Fast Aggregation
The data.table
package is another popular option for data aggregation, especially when working with large datasets. It allows for very efficient grouping and aggregation.
Example 3: Aggregating Data using data.table
In this example, we will use data.table
to calculate the mean of the Value
column by Category
.
# Loading the data.table package
library(data.table)
# Converting data to data.table
data_dt <- as.data.table(data)
# Aggregating data by Category and calculating the mean of Value
aggregated_data_dt <- data_dt[, .(Mean_Value = mean(Value)), by = Category]
print(aggregated_data_dt)
Output:
# Category Mean_Value
# 1: A 30
# 2: B 40
Summary
Data aggregation in R allows you to summarize and group data effectively. You can use the aggregate()
function, dplyr
functions like group_by()
and summarise()
, or the data.table
package for fast aggregation. These methods help you calculate statistics like sum, mean, count, and more for different subsets of your data, making it easier to analyze and extract insights.
Introduction to Machine Learning in R
Machine learning (ML) in R involves using algorithms and statistical models to analyze data, identify patterns, and make predictions. R is widely used for data analysis and has a variety of libraries and packages for machine learning, making it a great tool for both beginners and advanced practitioners.
Overview of Machine Learning
Machine learning can be divided into three primary types:
- Supervised Learning: The model is trained on labeled data, where both the input and the correct output are provided. Examples include regression and classification tasks.
- Unsupervised Learning: The model is given data without labels, and it tries to find patterns, structures, or relationships within the data. Examples include clustering and dimensionality reduction.
- Reinforcement Learning: The model learns through trial and error by interacting with an environment and receiving feedback in the form of rewards or penalties.
Machine Learning Packages in R
R offers a variety of packages for implementing machine learning algorithms. Some of the most popular ones include:
- caret: A comprehensive package for building predictive models and includes tools for data pre-processing, feature selection, and model training.
- randomForest: A package for constructing random forest models, which are a popular ensemble learning method.
- e1071: A package for support vector machines (SVM), which can be used for classification and regression tasks.
- xgboost: A package for gradient boosting, a powerful technique for supervised learning tasks, particularly for structured/tabular data.
- keras: A deep learning library that allows you to build neural networks and deep learning models in R.
Steps in Building a Machine Learning Model
Building a machine learning model typically follows these steps:
- Data Preparation: Collect and clean the data. This involves removing missing values, handling categorical variables, and splitting the data into training and testing sets.
- Feature Selection/Engineering: Identify the most important features (variables) that will be used by the model. Sometimes, new features are created through domain knowledge.
- Model Selection: Choose the appropriate machine learning algorithm based on the problem at hand (e.g., regression, classification, clustering).
- Model Training: Train the model using the training data and optimize its parameters to minimize prediction error.
- Model Evaluation: Evaluate the model’s performance using metrics like accuracy, precision, recall, F1-score (classification), or RMSE (regression).
- Model Deployment: Once the model is trained and evaluated, deploy it into a production environment where it can be used to make predictions on new data.
Example: Building a Simple Classification Model
Here is an example of building a simple classification model using the famous iris
dataset in R. We will use the caret
package to train a decision tree model.
# Loading necessary libraries
library(caret)
library(rpart)
# Loading the iris dataset
data(iris)
# Splitting the data into training and testing sets
set.seed(123)
trainIndex <- createDataPartition(iris$Species, p = 0.7, list = FALSE)
trainData <- iris[trainIndex, ]
testData <- iris[-trainIndex, ]
# Building a decision tree model
model <- rpart(Species ~ ., data = trainData, method = "class")
# Making predictions on the test data
predictions <- predict(model, testData, type = "class")
# Evaluating the model's accuracy
confusionMatrix(predictions, testData$Species)
Output:
# Confusion Matrix and Statistics
#
# Reference
# Prediction setosa versicolor virginica
# setosa 14 0 0
# versicolor 0 15 1
# virginica 0 0 15
#
# Overall Statistics
# Accuracy : 0.98
# 95% CI : (0.92, 1)
# No Information Rate : 0.33
# P-Value [Acc > NIR] : < 2e-16
Types of Machine Learning Algorithms
1. Supervised Learning Algorithms
Supervised learning algorithms are used when we have labeled data. Some examples include:
- Linear Regression: Used for predicting a continuous value based on input features.
- Logistic Regression: Used for binary classification tasks.
- Decision Trees: Tree-based models used for both classification and regression tasks.
- Random Forest: An ensemble method that uses multiple decision trees to improve accuracy.
- Support Vector Machines (SVM): Used for classification and regression tasks by finding the optimal hyperplane that separates classes in the data.
2. Unsupervised Learning Algorithms
Unsupervised learning algorithms are used when the data is unlabeled. Some examples include:
- K-means Clustering: A method used to group similar data points into clusters.
- Principal Component Analysis (PCA): A technique for reducing the dimensionality of data while retaining as much variance as possible.
3. Reinforcement Learning
Reinforcement learning involves training an agent to make decisions by interacting with an environment and receiving rewards or penalties. It is used in applications like game playing, robotics, and autonomous driving.
Summary
Machine learning in R allows you to build predictive models and gain insights from data. R provides powerful packages and functions that make it easier to implement algorithms for both supervised and unsupervised learning tasks. By following the steps of data preparation, model selection, training, and evaluation, you can create robust machine learning models in R.
Data Preprocessing in R
Data preprocessing is a crucial step in the machine learning pipeline. It involves cleaning and transforming raw data into a usable format that can be fed into machine learning models. In R, data preprocessing involves handling missing values, encoding categorical variables, normalizing numerical data, and scaling the data to ensure that the model performs optimally.
Steps in Data Preprocessing
The following are common steps involved in data preprocessing:
- Handling Missing Data: Missing values must be identified and treated before building any model. Methods include removing rows with missing values or imputing missing values using mean, median, or other techniques.
- Encoding Categorical Variables: Categorical variables (e.g., gender, country) need to be converted into numeric values using encoding techniques like one-hot encoding or label encoding.
- Feature Scaling: Features (variables) should be scaled to ensure that models like k-Nearest Neighbors (k-NN) and Support Vector Machines (SVM) perform optimally. Common techniques include normalization and standardization.
- Data Transformation: This involves transforming data into a more suitable form for modeling, such as log transformations for skewed data.
Handling Missing Data
Missing data is a common issue in real-world datasets. In R, there are several ways to deal with missing data:
- Removing Missing Data: If the dataset is large and the missing data is small, you can remove rows with missing values using the
na.omit()
function. - Imputing Missing Data: Imputation involves replacing missing values with estimated values. Common techniques include replacing missing values with the mean, median, or using the
mice
package for multiple imputation.
Example: Handling Missing Data
# Load the dataset
data(iris)
# Introduce missing values
iris_with_na <- iris
iris_with_na[1:10, 1] <- NA
# Remove rows with missing values
cleaned_data <- na.omit(iris_with_na)
# Impute missing values with the mean
iris_with_na[is.na(iris_with_na)] <- mean(iris_with_na$Sepal.Length, na.rm = TRUE)
Encoding Categorical Variables
Categorical variables need to be encoded into numeric values for machine learning algorithms to process them. In R, encoding can be done using the following methods:
- Label Encoding: Assigning a unique number to each category.
- One-Hot Encoding: Creating binary columns for each category (1 for the presence of the category, 0 for absence).
Example: One-Hot Encoding
# Using the caret package for one-hot encoding
library(caret)
data(iris)
# Convert categorical variable into dummy variables
dummy_vars <- dummyVars(Species ~ ., data = iris)
encoded_data <- predict(dummy_vars, newdata = iris)
Feature Scaling
Feature scaling is essential to ensure that all features contribute equally to the model. Two common methods for feature scaling are:
- Normalization: Scaling the feature values between 0 and 1 using the formula:
scaled_value = (value - min) / (max - min)
. - Standardization: Scaling the feature values to have a mean of 0 and a standard deviation of 1 using the formula:
scaled_value = (value - mean) / standard_deviation
.
Example: Standardizing Features
# Standardizing the Sepal.Length feature
scaled_data <- scale(iris$Sepal.Length)
Data Transformation
Sometimes data may need to be transformed to meet the assumptions of certain algorithms. For example, if the data is highly skewed, a log transformation can help in normalizing the data distribution. In R, you can use the log()
function for this transformation.
Example: Log Transformation
# Log-transforming the Sepal.Length feature
log_transformed_data <- log(iris$Sepal.Length + 1) # Adding 1 to avoid log(0)
Splitting Data into Training and Test Sets
After preprocessing the data, you should split the data into training and testing sets. This allows you to evaluate the performance of the model on unseen data. In R, the caret
package provides a function to split the data.
Example: Splitting the Data
# Load the caret package
library(caret)
# Split the data into 70% training and 30% testing
set.seed(123)
split <- createDataPartition(iris$Species, p = 0.7, list = FALSE)
train_data <- iris[split, ]
test_data <- iris[-split, ]
Summary
Data preprocessing is an essential step in preparing data for machine learning models. It involves handling missing values, encoding categorical variables, scaling features, and transforming data to ensure optimal model performance. By following these preprocessing steps, you can ensure that your data is ready for training machine learning models in R.
Supervised Learning in R
Supervised learning is a type of machine learning where the model is trained on labeled data. In this approach, the algorithm learns from the input-output pairs and makes predictions based on the relationship between the features and the target variable. Below are some common supervised learning algorithms implemented in R:
Linear Regression
Linear regression is used to predict a continuous target variable based on one or more predictors (independent variables). It assumes a linear relationship between the dependent and independent variables.
Example: Linear Regression
# Load the dataset
data(mtcars)
# Fit a linear regression model to predict 'mpg' (miles per gallon) based on other features
linear_model <- lm(mpg ~ wt + hp + disp, data = mtcars)
# View model summary
summary(linear_model)
In this example, the linear regression model predicts the 'mpg' column based on the 'wt', 'hp', and 'disp' columns from the `mtcars` dataset. The lm()
function is used to create a linear regression model in R.
Logistic Regression
Logistic regression is used for binary classification problems. It predicts the probability that an observation belongs to one of the two classes. The target variable is categorical and can take values 0 or 1, indicating the class of the data point.
Example: Logistic Regression
# Load the dataset
data(iris)
# Convert the Species column into a binary factor (setosa vs. non-setosa)
iris$Species_binary <- ifelse(iris$Species == "setosa", 1, 0)
# Fit a logistic regression model
logistic_model <- glm(Species_binary ~ Sepal.Length + Sepal.Width + Petal.Length,
family = binomial(link = "logit"),
data = iris)
# View model summary
summary(logistic_model)
In this example, the logistic regression model is used to predict whether the species is "setosa" or not, based on the features 'Sepal.Length', 'Sepal.Width', and 'Petal.Length'. The glm()
function with the binomial
family is used to create a logistic regression model in R.
Decision Trees
Decision trees are a supervised learning algorithm used for both classification and regression tasks. The model splits the data into subsets based on the most significant feature at each level, and it creates a tree-like structure of decisions.
Example: Decision Tree
# Load the necessary library
library(rpart)
# Fit a decision tree model for classification
tree_model <- rpart(Species ~ Sepal.Length + Sepal.Width + Petal.Length + Petal.Width,
data = iris, method = "class")
# Plot the decision tree
plot(tree_model)
text(tree_model, use.n = TRUE)
In this example, the decision tree model is used to classify the iris species based on the features of the flower. The rpart()
function is used to create the decision tree, and the plot()
function visualizes the tree.
Random Forests
Random forests are an ensemble learning method that creates multiple decision trees and combines their predictions to improve accuracy and robustness. Random forests are less prone to overfitting compared to a single decision tree.
Example: Random Forest
# Load the necessary library
library(randomForest)
# Fit a random forest model for classification
rf_model <- randomForest(Species ~ Sepal.Length + Sepal.Width + Petal.Length + Petal.Width,
data = iris, ntree = 100)
# View the random forest model details
print(rf_model)
# Plot the importance of each feature
importance(rf_model)
plot(rf_model)
In this example, the random forest model is used to classify the iris species based on the flower's features. The randomForest()
function is used to create the random forest model, and the importance()
function shows the importance of each feature in the classification task.
Model Evaluation
Once the models are trained, it is essential to evaluate their performance. Common evaluation metrics include accuracy, precision, recall, and F1-score for classification tasks, and mean squared error (MSE) for regression tasks.
Example: Model Evaluation (Accuracy)
# Predict using the logistic regression model
logistic_predictions <- predict(logistic_model, newdata = iris, type = "response")
logistic_predictions_class <- ifelse(logistic_predictions > 0.5, 1, 0)
# Calculate accuracy
accuracy <- mean(logistic_predictions_class == iris$Species_binary)
accuracy
Summary
Supervised learning algorithms like linear regression, logistic regression, decision trees, and random forests are powerful tools for both classification and regression tasks. By understanding the structure of these models and applying them to real-world data, you can build predictive models capable of making accurate forecasts and classifications.
Unsupervised Learning in R
Unsupervised learning is a type of machine learning where the model is trained on data without labeled outcomes. The goal is to identify hidden patterns or structures in the data. Common unsupervised learning techniques include clustering and dimensionality reduction methods like Principal Component Analysis (PCA). Below are some of these techniques implemented in R:
Clustering
Clustering is an unsupervised learning technique used to group similar data points together. The goal is to segment the data into clusters such that data points within each cluster are more similar to each other than to those in other clusters. Two common clustering algorithms are K-Means and Hierarchical Clustering.
K-Means Clustering
K-Means clustering is a partitioning method that divides the data into a predefined number of clusters. The algorithm iterates to minimize the variance within each cluster.
Example: K-Means Clustering
# Load the dataset
data(iris)
# Select numeric columns for clustering
iris_data <- iris[, 1:4]
# Apply K-Means clustering (k = 3 clusters)
set.seed(123) # Set seed for reproducibility
kmeans_model <- kmeans(iris_data, centers = 3)
# View the cluster centers
kmeans_model$centers
# Assign clusters to the data
iris$cluster <- as.factor(kmeans_model$cluster)
# Plot the clusters
library(ggplot2)
ggplot(iris, aes(x = Sepal.Length, y = Sepal.Width, color = cluster)) +
geom_point() +
labs(title = "K-Means Clustering (k = 3)", x = "Sepal Length", y = "Sepal Width")
In this example, we apply K-Means clustering to the `iris` dataset, using the first four columns as numeric features. The kmeans()
function is used to perform clustering, and the resulting clusters are visualized using ggplot2
.
Hierarchical Clustering
Hierarchical clustering is another clustering technique that builds a tree-like structure called a dendrogram. It does not require the number of clusters to be specified beforehand and can be agglomerative (bottom-up) or divisive (top-down).
Example: Hierarchical Clustering
# Compute the distance matrix
distance_matrix <- dist(iris_data)
# Apply hierarchical clustering
hclust_model <- hclust(distance_matrix)
# Plot the dendrogram
plot(hclust_model, main = "Hierarchical Clustering Dendrogram", xlab = "Data Points", ylab = "Height")
In this example, we perform hierarchical clustering on the `iris` dataset by first calculating the distance matrix using the dist()
function. Then, we apply hierarchical clustering using the hclust()
function and visualize the dendrogram.
Principal Component Analysis (PCA)
Principal Component Analysis (PCA) is a dimensionality reduction technique that transforms the data into a set of orthogonal components, or "principal components," that capture the most variance in the data. PCA is useful for reducing the number of features while retaining the most important information in the dataset.
Example: PCA
# Standardize the data (important for PCA)
iris_scaled <- scale(iris[, 1:4])
# Apply PCA
pca_model <- prcomp(iris_scaled, center = TRUE, scale. = TRUE)
# View the summary of PCA
summary(pca_model)
# Plot the first two principal components
biplot(pca_model, main = "PCA Biplot")
In this example, we perform PCA on the `iris` dataset by first standardizing the numeric features using the scale()
function. Then, we apply PCA using the prcomp()
function and visualize the result using a biplot.
Choosing the Number of Clusters (K) in K-Means
One important aspect of K-Means clustering is determining the optimal number of clusters. The elbow method is a common technique to find the ideal value of K, by plotting the within-cluster sum of squares (WSS) for different values of K and looking for an "elbow" in the plot.
Example: Elbow Method
# Elbow method to determine the optimal number of clusters
wss <- numeric(10)
for (k in 1:10) {
wss[k] <- sum(kmeans(iris_data, centers = k)$tot.withinss)
}
# Plot the WSS for different values of k
plot(1:10, wss, type = "b", main = "Elbow Method", xlab = "Number of Clusters", ylab = "Within-Cluster Sum of Squares")
In this example, we calculate and plot the within-cluster sum of squares (WSS) for K values from 1 to 10. The "elbow" in the plot helps us choose the optimal number of clusters.
Summary
Unsupervised learning techniques like clustering and PCA are powerful tools for discovering patterns in data. By grouping similar data points (clustering) or reducing the dimensionality of the data (PCA), unsupervised learning enables you to uncover hidden structures and simplify complex datasets for further analysis.
Exploratory Data Analysis (EDA) in R
Exploratory Data Analysis (EDA) is an essential step in data analysis, where we explore and summarize the main characteristics of a dataset. EDA helps us understand the underlying structure of the data, detect outliers, check for missing values, and gain insights before applying more complex statistical methods. In R, we can perform EDA using a variety of functions and visualization tools.
Key Steps in EDA
- Data Summarization: Summary statistics (mean, median, etc.) help us understand the central tendency and spread of the data.
- Data Visualization: Visualizing the data through plots helps us identify patterns, trends, and outliers.
- Handling Missing Data: Checking for and handling missing values in the dataset is a crucial part of the EDA process.
- Correlation Analysis: Identifying relationships between variables can help reveal patterns and associations.
Data Summarization
Summary statistics provide a quick overview of the data. Common methods include measures of central tendency (mean, median) and measures of dispersion (standard deviation, range).
Example: Summary Statistics
# Load the dataset
data(iris)
# Get a summary of the dataset
summary(iris)
# Get the mean and standard deviation of a column
mean(iris$Sepal.Length)
sd(iris$Sepal.Length)
The summary()
function provides basic statistics, such as the minimum, maximum, mean, median, and quartiles for each column. We can also calculate individual statistics like the mean and standard deviation using the mean()
and sd()
functions.
Data Visualization
Visualizing the data is essential for understanding the relationships between variables and identifying patterns. Common plots include histograms, boxplots, scatter plots, and bar charts.
Example: Visualizing Data
# Load necessary library
library(ggplot2)
# Histogram of Sepal Length
ggplot(iris, aes(x = Sepal.Length)) +
geom_histogram(binwidth = 0.2, fill = "blue", color = "black") +
labs(title = "Histogram of Sepal Length", x = "Sepal Length", y = "Frequency")
# Boxplot to visualize distribution by species
ggplot(iris, aes(x = Species, y = Sepal.Length, color = Species)) +
geom_boxplot() +
labs(title = "Boxplot of Sepal Length by Species")
In this example, we use ggplot2
to create a histogram of the Sepal Length and a boxplot to visualize the distribution of Sepal Length by species. These plots help identify the spread and outliers in the data.
Checking for Missing Data
It's important to check for missing data as it can affect the quality and validity of the analysis. In R, we can check for missing values using the is.na()
function.
Example: Handling Missing Data
# Check for missing values
sum(is.na(iris))
# Remove rows with missing values
iris_clean <- na.omit(iris)
# Impute missing values (example: replace with mean)
iris$Sepal.Length[is.na(iris$Sepal.Length)] <- mean(iris$Sepal.Length, na.rm = TRUE)
In this example, we first check for missing values using is.na()
and sum()
. Then, we handle missing data by either removing rows with missing values using na.omit()
or imputing missing values by replacing them with the mean of the column.
Correlation Analysis
Correlation analysis helps identify relationships between two or more variables. The cor()
function computes the correlation coefficient, which indicates the strength and direction of the relationship.
Example: Correlation Matrix
# Compute the correlation matrix
correlation_matrix <- cor(iris[, 1:4])
# Print the correlation matrix
correlation_matrix
# Visualize the correlation matrix using a heatmap
library(corrplot)
corrplot(correlation_matrix, method = "circle", type = "upper",
title = "Correlation Matrix", mar = c(0, 0, 1, 0))
In this example, we calculate the correlation matrix for the numeric columns of the `iris` dataset using the cor()
function. We also visualize the correlation matrix using a heatmap with the corrplot
library.
Outlier Detection
Outliers can significantly affect the results of statistical analysis. Identifying outliers is an important part of EDA. One common method is to use boxplots to visualize the presence of outliers in the data.
Example: Outlier Detection
# Boxplot to detect outliers in Sepal Length
boxplot(iris$Sepal.Length, main = "Outlier Detection in Sepal Length")
# Identify outliers
outliers <- boxplot(iris$Sepal.Length, plot = FALSE)$out
outliers
In this example, we use a boxplot to detect outliers in the `Sepal.Length` column. The outliers are identified and stored in the outliers
object.
EDA Summary
Exploratory Data Analysis (EDA) is a critical part of the data analysis process. It helps us understand the structure, patterns, and anomalies in the data, which lays the foundation for more advanced analysis and modeling. By performing EDA, we gain insights into the dataset, which guide decisions on data cleaning, transformation, and model selection.
Handling Big Data with data.table
The data.table package in R provides an efficient and fast way to handle large datasets. It is an enhanced version of the data.frame, designed to offer speed and memory efficiency for large-scale data manipulation. The package provides an intuitive syntax and powerful functions for data manipulation, aggregation, and transformation. It is particularly useful when working with big data that needs to be processed quickly.
Key Features of data.table
- Speed:
data.table
is optimized for fast data manipulation, outperformingdata.frame
in many scenarios. - Memory Efficiency: It minimizes memory usage while performing operations on large datasets, which is crucial when handling big data.
- Flexible Syntax: The syntax of
data.table
allows you to perform complex operations with fewer lines of code. - In-Place Modifications: Data can be modified in place, without the need for creating copies, which is efficient in terms of both time and space.
Installing and Loading data.table
To get started with data.table
, you first need to install the package and load it into your R environment.
Example: Installing and Loading data.table
# Install data.table package
install.packages("data.table")
# Load data.table package
library(data.table)
Creating a data.table
Similar to data.frame
, a data.table
can be created from a variety of sources, such as vectors, lists, or data frames. You can create a data.table
using the data.table()
function.
Example: Creating a data.table
# Create a simple data.table
dt <- data.table(
ID = c(1, 2, 3, 4),
Name = c("Alice", "Bob", "Charlie", "David"),
Age = c(25, 30, 35, 40)
)
# Print the data.table
print(dt)
Basic Data Manipulation with data.table
data.table
provides an efficient way to manipulate and transform your data. The primary syntax for data manipulation is: DT[ , .(expression), by = .(grouping_columns)]
, where DT
is the data.table, and you can perform operations like filtering, selecting, and aggregating.
Example: Selecting and Filtering Data
# Filter rows where Age is greater than 30
dt[Age > 30]
# Select specific columns
dt[, .(Name, Age)]
Example: Aggregating Data
# Calculate the mean Age by Name
dt[, .(Mean_Age = mean(Age)), by = Name]
Efficient Grouping and Aggregation
One of the key strengths of data.table
is its ability to efficiently group and aggregate data. You can use the by
argument to group data by one or more columns and apply aggregation functions to each group.
Example: Grouping and Aggregating Data
# Group by Name and calculate the sum of Age
dt[, .(Total_Age = sum(Age)), by = Name]
In-Place Modifications
One of the powerful features of data.table
is its ability to modify data in place. This allows you to update or transform data without creating copies, resulting in better performance when working with large datasets.
Example: In-Place Modifications
# Add a new column to the data.table
dt[, Salary := c(50000, 55000, 60000, 65000)]
# Update an existing column (e.g., Age)
dt[Age > 30, Age := Age + 1]
Joining Data.tables
data.table
allows you to perform fast joins on large datasets using the on
argument. You can join multiple data.tables using various types of joins (inner, left, right, and outer).
Example: Joining Two data.tables
# Create another data.table
dt2 <- data.table(
ID = c(1, 2, 3, 5),
Department = c("HR", "IT", "Finance", "Sales")
)
# Perform an inner join on ID
result <- merge(dt, dt2, by = "ID", all = FALSE)
print(result)
Efficient Sorting and Ordering
data.table
provides efficient ways to sort and order data. The setorder()
function allows you to reorder your data.table based on one or more columns.
Example: Sorting Data
# Sort the data.table by Age in ascending order
setorder(dt, Age)
# Sort by multiple columns (e.g., Name and Age)
setorder(dt, Name, Age)
Handling Big Data with data.table
When working with big data, it's important to keep memory usage and performance in mind. data.table
optimizes memory usage and supports parallel processing, which can help speed up computations. Additionally, data.table
supports the use of SQL-like operations, making it a powerful tool for large-scale data analysis in R.
Summary
data.table
is an essential tool in R for efficiently handling large datasets. It offers powerful features like fast data manipulation, in-place modifications, and efficient aggregation, which make it an ideal choice for big data analysis. By leveraging its syntax and performance optimizations, you can work with large-scale data more effectively and reduce memory consumption.
Time Series Analysis in R
Time Series Analysis is a statistical technique used to analyze and forecast data that is collected over time. Time series data is often collected at regular intervals (e.g., daily, monthly, or yearly). In R, there are several tools and packages available for performing time series analysis. The most commonly used are the ts
class and the forecast
package, which provide functions for modeling and forecasting time series data.
Components of Time Series Data
Time series data typically consists of the following components:
- Trend: The long-term movement in the data, which can be upwards, downwards, or remain constant.
- Seasonality: The repeating short-term patterns or cycles in the data, such as weekly, monthly, or yearly patterns.
- Cyclic Patterns: Long-term fluctuations that are not of a fixed period, often influenced by economic or other factors.
- Irregularity (Noise): Random variations or anomalies in the data that cannot be explained by trend, seasonality, or cyclic patterns.
Creating a Time Series Object in R
The ts()
function is used to create a time series object in R. This function allows you to specify the frequency and start date for the time series data.
Example: Creating a Time Series
# Creating a simple time series object
data <- c(100, 120, 130, 140, 150, 160, 170, 180)
time_series <- ts(data, start = c(2020, 1), frequency = 12)
# Print the time series object
print(time_series)
Plotting Time Series Data
Once you have a time series object, you can use the plot()
function to visualize the data over time.
Example: Plotting a Time Series
# Plot the time series data
plot(time_series, main = "Time Series Plot", ylab = "Values", xlab = "Time")
Decomposition of Time Series
Decomposition refers to the process of breaking down a time series into its individual components: trend, seasonality, and noise. In R, the decompose()
function can be used for seasonal decomposition of time series data.
Example: Decomposing a Time Series
# Decompose the time series
decomposed_ts <- decompose(time_series)
# Plot the decomposition
plot(decomposed_ts)
Time Series Forecasting
Time series forecasting aims to predict future values based on historical data. In R, the forecast
package provides functions like auto.arima()
and forecast()
for building and evaluating forecasting models.
Example: Forecasting with ARIMA
# Install and load the forecast package
install.packages("forecast")
library(forecast)
# Fit an ARIMA model to the time series data
arima_model <- auto.arima(time_series)
# Forecast the next 12 periods
forecasted_values <- forecast(arima_model, h = 12)
# Plot the forecast
plot(forecasted_values)
Exponential Smoothing
Exponential smoothing is another popular method for time series forecasting. It assigns exponentially decreasing weights to past observations. The ets()
function from the forecast
package can be used to apply exponential smoothing models.
Example: Exponential Smoothing Forecasting
# Fit an exponential smoothing model
ets_model <- ets(time_series)
# Forecast the next 12 periods
ets_forecast <- forecast(ets_model, h = 12)
# Plot the forecast
plot(ets_forecast)
ARIMA Model Diagnostics
After fitting an ARIMA model, it's important to check the residuals to ensure that the model fits the data well. You can use diagnostic plots and statistical tests to evaluate the model's performance.
Example: ARIMA Model Diagnostics
# Check residuals of the ARIMA model
checkresiduals(arima_model)
Time Series Cross-Validation
Cross-validation for time series data involves splitting the data into training and testing sets in a way that respects the temporal order of the observations. R provides various techniques for time series cross-validation, such as rolling forecasting origin and walk-forward validation.
Example: Time Series Cross-Validation with caret
# Install and load the caret package
install.packages("caret")
library(caret)
# Define the time series cross-validation method
train_control <- trainControl(method = "timeslice", initialWindow = 12, horizon = 6)
# Fit a time series model using caret
model <- train(time_series ~ 1, data = data.frame(time_series), method = "arima", trControl = train_control)
# Print the model summary
print(model)
Summary
Time series analysis is a powerful tool for analyzing and forecasting data that is collected over time. R provides a variety of functions and packages, such as ts()
, forecast
, and caret
, to handle time series data efficiently. By leveraging methods like ARIMA, exponential smoothing, and decomposition, you can derive insights and make predictions about future trends in your data.
Text Analysis and Natural Language Processing (NLP) in R
Text Analysis and Natural Language Processing (NLP) are fields of artificial intelligence that focus on the interaction between computers and human language. In R, a variety of packages are available for text mining, text analysis, and natural language processing. These tools can be used for tasks such as text classification, sentiment analysis, topic modeling, and more.
Popular R Packages for NLP
Some popular R packages used for NLP and text analysis include:
tm:
Text Mining package for text cleaning and preprocessing.textclean:
Provides functions for cleaning text data.tidytext:
Allows for tidy text mining using the tidyverse principles.text:
A package for NLP and text embedding tasks.sentimentr:
Package for sentiment analysis of text.quanteda:
A framework for managing and analyzing textual data.
Text Preprocessing
Before performing any analysis on text data, it is crucial to preprocess the text. Text preprocessing typically involves the following steps:
- Converting text to lowercase to ensure uniformity.
- Removing punctuation and special characters that do not contribute to the analysis.
- Removing stop words (common words like 'the', 'and', 'is') that do not add meaningful information.
- Stemming and Lemmatization to reduce words to their root forms.
- Tokenization to break the text into words, sentences, or other units.
Example: Preprocessing Text in R
# Install the required packages
install.packages("tm")
library(tm)
# Sample text
text <- "Text analysis and Natural Language Processing are important fields in AI!"
# Create a corpus (collection of text)
corpus <- Corpus(VectorSource(text))
# Convert text to lowercase
corpus <- tm_map(corpus, content_transformer(tolower))
# Remove punctuation
corpus <- tm_map(corpus, removePunctuation)
# Remove stopwords
corpus <- tm_map(corpus, removeWords, stopwords("en"))
# Remove numbers
corpus <- tm_map(corpus, removeNumbers)
# View cleaned text
inspect(corpus)
Tokenization
Tokenization is the process of splitting a text into smaller units, such as words or sentences. In R, you can use the tidytext
package to tokenize text data.
Example: Tokenizing Text
# Install tidytext package
install.packages("tidytext")
library(tidytext)
# Tokenizing the cleaned text
tokenized_text <- data_frame(text = text) %>%
unnest_tokens(word, text)
# View tokenized words
print(tokenized_text)
Sentiment Analysis
Sentiment analysis is the process of determining the sentiment or emotion expressed in a piece of text (positive, negative, or neutral). In R, you can use the sentimentr
package to perform sentiment analysis.
Example: Sentiment Analysis
# Install the sentimentr package
install.packages("sentimentr")
library(sentimentr)
# Sample text for sentiment analysis
text <- "I love this product. It's amazing!"
# Perform sentiment analysis
sentiment_score <- sentiment(text)
# View sentiment analysis result
print(sentiment_score)
Text Classification
Text classification involves categorizing text into predefined categories or classes. This can be done using machine learning algorithms such as Naive Bayes, SVM, or Random Forest. In R, you can use the tm
and text2vec
packages for text classification tasks.
Example: Text Classification
# Install text2vec for text classification
install.packages("text2vec")
library(text2vec)
# Example of text data and labels
texts <- c("Positive text", "Negative text", "Positive text", "Negative text")
labels <- factor(c("Positive", "Negative", "Positive", "Negative"))
# Tokenizing and vectorizing the text
tokenizer <- word_tokenizer(texts)
vectorizer <- vocab_vectorizer(vocabulary = tokenizer)
dtm <- create_dtm(tokenizer, vectorizer)
# Train a simple model (e.g., Naive Bayes)
model <- naiveBayes(dtm, labels)
# Predict class for new text
new_text <- c("Positive text")
new_dtm <- create_dtm(word_tokenizer(new_text), vectorizer)
prediction <- predict(model, new_dtm)
# Display prediction
print(prediction)
Topic Modeling
Topic modeling is a technique to identify the topics that are present in a collection of texts. The most common method for topic modeling is Latent Dirichlet Allocation (LDA). In R, you can use the topicmodels
package to perform topic modeling.
Example: Topic Modeling with LDA
# Install the topicmodels package
install.packages("topicmodels")
library(topicmodels)
# Create a document-term matrix (DTM)
dtm <- DocumentTermMatrix(corpus)
# Fit an LDA model with 2 topics
lda_model <- LDA(dtm, k = 2)
# View the top terms for each topic
terms(lda_model, 10)
Word Clouds
Word clouds are a visual representation of the most frequent words in a text. R provides the wordcloud
package for creating word clouds based on word frequency.
Example: Creating a Word Cloud
# Install the wordcloud package
install.packages("wordcloud")
library(wordcloud)
# Create a word cloud from the tokenized text
wordcloud(tokenized_text$word)
Summary
Text Analysis and Natural Language Processing (NLP) in R offer powerful methods for extracting insights from text data. With packages like tm
, tidytext
, sentimentr
, and topicmodels
, you can perform tasks such as sentiment analysis, text classification, topic modeling, and creating word clouds. By preprocessing text, tokenizing it, and applying machine learning algorithms, you can analyze and interpret large volumes of text data effectively in R.
Generalized Linear Models (GLMs) in R
Generalized Linear Models (GLMs) are a broad class of models used to analyze data where the dependent variable (response variable) does not follow a normal distribution. GLMs extend linear models by allowing for non-normal distributions and linking the mean of the distribution to the predictors via a link function. In R, GLMs are typically fitted using the glm()
function.
Components of GLMs
A GLM consists of three main components:
- Random Component: The distribution of the dependent variable (e.g., Normal, Binomial, Poisson).
- Systematic Component: The linear predictor, which is a linear combination of the explanatory variables (e.g.,
y = β0 + β1 * x1 + β2 * x2 + ...
). - Link Function: A function that connects the linear predictor to the mean of the distribution (e.g., identity link, logit link, log link).
Choosing a Distribution and Link Function
Depending on the nature of the dependent variable, the distribution and link function are chosen:
- Binomial distribution: Often used for binary or proportion data (e.g., logistic regression, with the logit link function).
- Poisson distribution: Used for count data (e.g., Poisson regression, with the log link function).
- Gaussian distribution: Used for continuous data (e.g., linear regression, with the identity link function).
Fitting a GLM in R
The glm()
function in R is used to fit GLMs. The syntax is as follows:
# General syntax for glm()
glm(formula, family = , data = , weights = NULL, subset = NULL, na.action = na.omit)
Where:
formula:
The model formula (e.g.,y ~ x1 + x2
).family:
Specifies the distribution and link function (e.g.,binomial(link = "logit")
for logistic regression).data:
The dataset to be used.
Example: Logistic Regression (Binomial GLM)
Consider a logistic regression model where we predict the probability of success based on a predictor variable x
. The model follows a binomial distribution with a logit link function.
# Load necessary libraries
data("mtcars")
# Fit a logistic regression model (binary outcome)
# Here, we're predicting whether a car has more than 20 miles per gallon (mpg)
mtcars$mpg_binary <- ifelse(mtcars$mpg > 20, 1, 0)
# Fit the GLM
model <- glm(mpg_binary ~ wt + hp + qsec, data = mtcars, family = binomial(link = "logit"))
# View the model summary
summary(model)
This code fits a logistic regression model predicting whether a car has more than 20 mpg based on weight, horsepower, and quarter-mile time.
Example: Poisson Regression (Count Data)
For count data, we use the Poisson distribution with a log link function. This example fits a Poisson regression model to predict the number of accidents based on a predictor variable.
# Sample data: Number of accidents based on traffic volume
traffic_volume <- c(100, 200, 300, 400, 500)
accidents <- c(2, 3, 5, 7, 9)
# Fit the GLM with Poisson distribution
poisson_model <- glm(accidents ~ traffic_volume, family = poisson(link = "log"))
# View the model summary
summary(poisson_model)
This code fits a Poisson regression model predicting the number of accidents based on traffic volume.
Model Diagnostics
After fitting a GLM, it is important to check the model’s fit and diagnostics:
- Residuals: Check residuals to assess model fit. Use
residuals()
andplot()
functions. - Deviance: The deviance is a measure of model fit. Use
deviance()
to view the model’s deviance. - AIC (Akaike Information Criterion): AIC helps compare models. Use
AIC()
to obtain the AIC value.
Example: Checking Residuals
# Plot residuals for the logistic regression model
plot(residuals(model))
Interpreting GLM Coefficients
In GLMs, the coefficients represent the effect of a predictor on the response variable. The interpretation depends on the link function used:
- For logistic regression (logit link): The coefficients represent the log-odds of the outcome. Exponentiating the coefficients (using
exp()
) gives the odds ratio. - For Poisson regression (log link): The coefficients represent the log of the expected count. Exponentiating the coefficients gives the rate ratio.
- For Gaussian regression (identity link): The coefficients represent the change in the response variable for a one-unit change in the predictor.
Example: Interpreting Coefficients
# Get coefficients of the logistic regression model
coefficients(model)
# Exponentiate to get odds ratios
exp(coefficients(model))
Summary
Generalized Linear Models (GLMs) are a powerful and flexible tool for modeling data with non-normal distributions. In R, GLMs can be fitted using the glm()
function with various families and link functions, including logistic regression, Poisson regression, and linear regression. It is crucial to assess model fit through diagnostics like residuals, deviance, and AIC. Understanding the interpretation of model coefficients is also key to drawing meaningful conclusions from a GLM.
Survival Analysis in R
Survival analysis is a statistical approach used to model the time until an event occurs, such as the time until a patient experiences a relapse, a machine fails, or a customer churns. It involves analyzing data in which the outcome is time-to-event data, often referred to as "survival times." In R, survival analysis can be performed using the survival
package, which provides tools for analyzing survival data and fitting various survival models.
Components of Survival Analysis
Survival analysis typically involves two main components:
- Survival Time: The time from the start of observation to the event of interest, such as death, failure, or relapse.
- Censoring: Data may be censored if the event has not occurred before the end of the study, such as if a patient leaves the study or the study ends before the event happens.
Key Concepts
- Survival Function (S(t)): The probability that an individual survives beyond a certain time
t
. - Hazard Function (λ(t)): The rate at which events occur over time, conditional on survival up to that time.
- Cox Proportional Hazards Model: A popular model used to assess the effect of several variables on survival time while assuming that the hazard ratio between different groups is constant over time.
Installing and Loading the Survival Package
To perform survival analysis in R, you need to install and load the survival
package:
# Install the survival package
install.packages("survival")
# Load the survival package
library(survival)
Example: Kaplan-Meier Survival Curve
The Kaplan-Meier estimator is a non-parametric statistic used to estimate the survival function from lifetime data. It is particularly useful when dealing with censored data.
We can use the survfit()
function in the survival
package to fit a Kaplan-Meier survival curve.
# Example dataset: lung cancer dataset
data(lung)
# Create a survival object
surv_obj <- Surv(time = lung$time, event = lung$status)
# Fit the Kaplan-Meier survival curve
km_fit <- survfit(surv_obj ~ 1, data = lung)
# Plot the survival curve
plot(km_fit, main = "Kaplan-Meier Survival Curve", xlab = "Time", ylab = "Survival Probability")
This code fits a Kaplan-Meier survival curve to the lung cancer dataset and plots the survival probability over time.
Cox Proportional Hazards Model
The Cox Proportional Hazards model is used to examine the effect of several variables on survival time. The model assumes that the effect of the predictor variables on the hazard function is constant over time.
# Fit Cox Proportional Hazards model
cox_model <- coxph(surv_obj ~ age + sex + ph.ecog, data = lung)
# View model summary
summary(cox_model)
In this code, the coxph()
function fits a Cox model using age, sex, and ECOG performance status as predictor variables. The summary of the model provides estimates of the hazard ratios for each predictor.
Checking Proportional Hazards Assumption
The proportional hazards assumption is crucial in the Cox model. It assumes that the effect of the covariates on the hazard rate is constant over time. We can check this assumption using the cox.zph()
function:
# Check proportional hazards assumption
ph_assumption <- cox.zph(cox_model)
# Plot the results
plot(ph_assumption)
If the proportional hazards assumption holds, the plots should show no significant trends over time.
Survival Analysis with Time-Dependent Covariates
In some cases, covariates may change over time. The Cox model can be extended to handle time-dependent covariates by using the tt()
function in the formula.
# Example with time-dependent covariates
cox_model_td <- coxph(surv_obj ~ age + sex + tt(ph.ecog), data = lung, tt = function(x, t, ...) x * log(t))
# View the model summary
summary(cox_model_td)
In this example, the ph.ecog
covariate is modeled as time-dependent by multiplying it by the log of time.
Summary
Survival analysis is an essential tool for analyzing time-to-event data and dealing with censored observations. In R, the survival
package provides powerful functions for fitting survival models, such as Kaplan-Meier curves and Cox Proportional Hazards models. These models can help uncover important relationships between survival time and predictor variables. It is also important to check the proportional hazards assumption when using Cox models and to extend the models for time-dependent covariates when necessary.
Bayesian Analysis in R
Bayesian analysis is a statistical method that applies Bayes' theorem to update the probability for a hypothesis as more evidence or information becomes available. Unlike classical frequentist statistics, which interprets probability as the long-run frequency of events, Bayesian statistics treats probability as a measure of belief or certainty about an event. In R, Bayesian analysis can be performed using packages such as rjags
, Stan
, and bayesm
.
Bayes' Theorem
Bayes' theorem describes the relationship between prior knowledge, likelihood of data, and the posterior probability of a hypothesis. It is given by:
P(H|D) = (P(D|H) * P(H)) / P(D)
Where:
- P(H|D): Posterior probability (updated belief after seeing the data)
- P(D|H): Likelihood (probability of observing the data given the hypothesis)
- P(H): Prior probability (initial belief before seeing the data)
- P(D): Marginal likelihood (probability of observing the data)
Installing Required Packages
To perform Bayesian analysis in R, you'll need to install packages like rjags
and rstan
. These packages allow you to fit Bayesian models using Markov Chain Monte Carlo (MCMC) methods.
# Install the rjags package (for JAGS)
install.packages("rjags")
# Install the rstan package (for Stan)
install.packages("rstan")
Bayesian Analysis with JAGS
JAGS (Just Another Gibbs Sampler) is a popular software for performing Bayesian analysis using MCMC methods. In R, the rjags
package provides an interface to JAGS. Below is an example where we fit a simple Bayesian model:
# Load the rjags package
library(rjags)
# Define the model in JAGS syntax
model_string <- "model {
for (i in 1:N) {
y[i] ~ dnorm(mu, tau)
}
mu ~ dnorm(0, 0.001)
tau ~ dgamma(0.001, 0.001)
}"
# Data
data_list <- list(y = c(2.3, 2.9, 3.1, 2.5, 3.0), N = 5)
# Create the JAGS model
model <- jags.model(textConnection(model_string), data = data_list)
# Run MCMC to get posterior samples
update(model, 1000) # Burn-in
samples <- coda.samples(model, variable.names = c("mu", "tau"), n.iter = 5000)
# View the results
summary(samples)
In this example, we define a simple Bayesian model where the data y
is assumed to follow a normal distribution with unknown mean mu
and precision tau
. We specify prior distributions for mu
and tau
and then perform MCMC sampling to obtain posterior samples of these parameters.
Bayesian Analysis with Stan
Stan is a powerful tool for Bayesian statistical modeling, and the rstan
package provides an interface to Stan from R. Below is an example of a simple linear regression model using rstan
:
# Load the rstan package
library(rstan)
# Define the Stan model
stan_model <- "
data {
int N;
real y[N];
real x[N];
}
parameters {
real alpha;
real beta;
real sigma;
}
model {
y ~ normal(alpha + beta * x, sigma);
}
"
# Data
data_list <- list(N = 10, y = c(1.1, 1.3, 2.0, 2.1, 2.3, 2.9, 3.2, 3.5, 4.0, 4.2),
x = c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10))
# Fit the model using Stan
fit <- stan(model_code = stan_model, data = data_list, iter = 2000, chains = 4)
# View the results
print(fit)
This code fits a Bayesian linear regression model to data where y
is the dependent variable and x
is the independent variable. The model estimates the coefficients alpha
(intercept), beta
(slope), and sigma
(error standard deviation) using MCMC sampling.
Diagnostic Checks for MCMC
After running MCMC sampling, it's essential to check the convergence and mixing of the chains. You can use diagnostic plots like trace plots and autocorrelation plots to assess the quality of the MCMC sampling.
# Trace plot for the 'mu' parameter
traceplot(samples, pars = "mu")
# Autocorrelation plot
acf(as.matrix(samples)[, "mu"])
The trace plot shows how the parameter mu
evolves over iterations, and the autocorrelation plot shows how much the samples are correlated with each other. Well-mixed chains should have a "random walk" appearance in the trace plot and minimal autocorrelation.
Summary
Bayesian analysis provides a powerful framework for statistical modeling and inference, allowing for the incorporation of prior knowledge and updating beliefs as new data becomes available. In R, packages like rjags
and rstan
provide efficient tools for fitting Bayesian models using MCMC methods. It is important to ensure proper convergence and mixing of the MCMC chains to obtain reliable estimates. Bayesian analysis is widely used in various fields, including medicine, economics, engineering, and more.
Interactive Visualization with Plotly
Plotly is a powerful library for creating interactive visualizations in R. It allows users to create highly customizable plots such as line charts, scatter plots, bar charts, and more, while providing interactivity features such as zooming, panning, and tooltips. Plotly can be easily integrated with other R visualization tools like ggplot2, and it is a great choice for developing dashboards and web applications.
Installing Plotly
To get started with Plotly in R, you need to install the Plotly package. You can install it from CRAN using the following command:
# Install the Plotly package
install.packages("plotly")
Once installed, you can load the package and start creating interactive plots.
Basic Interactive Plot
Here’s an example of creating a basic interactive scatter plot using Plotly:
# Load the Plotly package
library(plotly)
# Create a basic scatter plot
plot_ly(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'markers',
marker = list(size = 12, color = 'rgba(255, 182, 193, .9)', line = list(width = 2))) %>%
layout(title = "Interactive Scatter Plot",
xaxis = list(title = "Miles per Gallon"),
yaxis = list(title = "Horsepower"))
This code creates an interactive scatter plot where the x-axis represents miles per gallon and the y-axis represents horsepower from the built-in mtcars
dataset. The plot is interactive, meaning you can zoom, hover over points to see values, and pan the chart.
Customizing Plot Appearance
Plotly allows you to customize various aspects of the plot such as titles, axis labels, colors, and marker styles. Below is an example with customized markers and axis labels:
# Customize the plot appearance
plot_ly(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'markers',
marker = list(size = 14, color = 'rgba(50, 171, 96, .6)', line = list(width = 2))) %>%
layout(title = "Customized Interactive Scatter Plot",
xaxis = list(title = "Miles per Gallon", tickangle = 45),
yaxis = list(title = "Horsepower", range = c(50, 350)))
In this example, we customized the marker size, color, and added a title and axis labels with rotated x-axis ticks. The y-axis range is also adjusted.
Adding Tooltips
Tooltips provide additional information when you hover over data points. You can customize the tooltip to display more details. Below is an example that shows the car model names in the tooltip:
# Add tooltips to display car names
plot_ly(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'markers',
text = rownames(mtcars), hoverinfo = 'text',
marker = list(size = 12, color = 'rgba(255, 165, 0, .7)', line = list(width = 2))) %>%
layout(title = "Interactive Scatter Plot with Tooltips",
xaxis = list(title = "Miles per Gallon"),
yaxis = list(title = "Horsepower"))
In this plot, the tooltip will display the car's name when you hover over a point. The text
argument is used to specify the information shown in the tooltip.
Line Plot Example
Plotly can also be used to create interactive line plots. Here’s an example of a simple line plot with customized axes:
# Create a line plot
plot_ly(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'lines',
line = list(color = 'rgba(255, 99, 132, .8)', width = 2)) %>%
layout(title = "Interactive Line Plot",
xaxis = list(title = "Miles per Gallon"),
yaxis = list(title = "Horsepower"))
This code creates a line plot with customized line color and width. The interactive functionality allows users to zoom, pan, and inspect the data.
Multiple Traces (Subplots)
Plotly also allows you to combine multiple plots into one using multiple traces. Below is an example where we create a scatter plot and a line plot in the same graph:
# Create multiple traces (scatter and line plot)
plot_ly() %>%
add_trace(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'markers',
marker = list(size = 12, color = 'rgba(0, 128, 255, .7)')) %>%
add_trace(data = mtcars, x = ~mpg, y = ~hp, type = 'scatter', mode = 'lines',
line = list(color = 'rgba(255, 99, 132, .8)', width = 2)) %>%
layout(title = "Scatter and Line Plot Combined",
xaxis = list(title = "Miles per Gallon"),
yaxis = list(title = "Horsepower"))
This code creates a plot with both scatter and line traces in the same visualization. The add_trace()
function is used to add multiple types of plots to the same graph.
Summary
Plotly in R allows for the creation of highly interactive and customizable visualizations. It supports a wide range of plot types, including scatter plots, line plots, bar charts, and more. Plotly also provides features like zooming, panning, tooltips, and multiple traces, making it a powerful tool for data exploration and presentation. By combining Plotly with other R packages, you can create sophisticated dashboards and web applications for data visualization.
Creating Dashboards with Shiny
Shiny is an R package that makes it easy to build interactive web applications and dashboards directly from R. It is particularly useful for displaying real-time data, creating dynamic visualizations, and building interactive reports. With Shiny, you can create highly interactive dashboards by combining UI components, server functions, and reactive programming.
Installing Shiny
To create dashboards using Shiny, you first need to install the Shiny package. You can install it from CRAN using the following command:
# Install the Shiny package
install.packages("shiny")
Once installed, you can load the package and start building your dashboard.
Basic Structure of a Shiny App
A Shiny app consists of two main components:
- UI (User Interface): Defines the layout and appearance of the dashboard.
- Server: Contains the logic that defines the app’s behavior and how inputs are processed.
Below is the basic structure of a Shiny app:
# Load the Shiny package
library(shiny)
# Define the UI
ui <- fluidPage(
titlePanel("Shiny Dashboard Example"),
sidebarLayout(
sidebarPanel(
sliderInput("slider", "Choose a number:", min = 1, max = 100, value = 50)
),
mainPanel(
textOutput("result")
)
)
)
# Define the server logic
server <- function(input, output) {
output$result <- renderText({
paste("You selected:", input$slider)
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
This example creates a simple Shiny app with a slider input and a text output. The UI is defined using fluidPage()
, and the server logic uses renderText()
to output the selected slider value. The app is run with shinyApp()
.
Interactive Components in Shiny
Shiny provides several interactive UI components, such as sliders, text inputs, buttons, plots, and tables. Here are some of the common components:
- sliderInput(): Creates a slider for selecting a range of values.
- textInput(): Creates a text box for user input.
- actionButton(): Creates a button that triggers an action when clicked.
- plotOutput(): Displays a plot in the UI.
- tableOutput(): Displays a table in the UI.
For example, you can add a plot to your dashboard using plotOutput()
:
# Define the UI with a plot
ui <- fluidPage(
titlePanel("Interactive Plot Example"),
sidebarLayout(
sidebarPanel(
sliderInput("slider", "Choose a value for x:", min = 1, max = 100, value = 50)
),
mainPanel(
plotOutput("plot")
)
)
)
# Define the server logic for the plot
server <- function(input, output) {
output$plot <- renderPlot({
x <- input$slider
plot(x, x^2, main = paste("Plot of x and x^2 (x =", x, ")"))
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
This example creates a Shiny app with a slider and a plot. The plot dynamically updates as the user moves the slider, displaying the relationship between x
and x^2
.
Reactive Programming in Shiny
Shiny uses a reactive programming model, meaning that outputs automatically update when inputs change. This is achieved through reactive expressions and observers. Reactive expressions are functions that depend on inputs and automatically re-run when those inputs change. Here’s an example of a simple reactive expression:
# Define the server logic with a reactive expression
server <- function(input, output) {
# Reactive expression that calculates the square of the input
square <- reactive({
input$slider^2
})
# Display the square in the output
output$result <- renderText({
paste("Square of the number:", square())
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
In this example, the square of the number selected by the slider is automatically calculated and displayed in the output.
Advanced Layout and Customization
Shiny allows you to create more advanced layouts using panels, tabs, and grids. You can also customize the appearance of your dashboard with themes and CSS. Below is an example of a dashboard with a tab layout:
# Load the shinydashboard package
library(shinydashboard)
# Define the UI with a dashboard layout
ui <- dashboardPage(
dashboardHeader(title = "Shiny Dashboard"),
dashboardSidebar(
sidebarMenu(
menuItem("Tab 1", tabName = "tab1", icon = icon("dashboard")),
menuItem("Tab 2", tabName = "tab2", icon = icon("th"))
)
),
dashboardBody(
tabItems(
tabItem(tabName = "tab1", h2("Welcome to Tab 1")),
tabItem(tabName = "tab2", h2("Welcome to Tab 2"))
)
)
)
# Define the server logic (empty for this example)
server <- function(input, output) {}
# Run the Shiny app
shinyApp(ui = ui, server = server)
This example uses the shinydashboard
package to create a dashboard layout with two tabs. The user can switch between the tabs to view different content.
Summary
Shiny is a powerful tool for building interactive dashboards and web applications in R. It provides an intuitive way to create user interfaces and define server logic using reactive programming. With Shiny, you can create sophisticated, dynamic dashboards that automatically update based on user input, making it an ideal choice for data visualization, reporting, and real-time data monitoring.
Embedding Plots and Tables in Shiny Apps
Shiny applications allow you to embed interactive plots and tables directly into the app’s user interface, making it easy for users to visualize data and explore results dynamically. In this section, we will explore how to embed both static and interactive plots, as well as tables, into a Shiny app.
Embedding Static Plots in Shiny
You can embed static plots, such as those created using the base R plot()
function or the ggplot2
package, into a Shiny app using plotOutput()
in the UI and renderPlot()
in the server function. Below is an example of embedding a static plot using base R's plot()
function:
# Load the Shiny package
library(shiny)
# Define the UI
ui <- fluidPage(
titlePanel("Embedding Static Plot Example"),
sidebarLayout(
sidebarPanel(
sliderInput("slider", "Choose a value for x:", min = 1, max = 100, value = 50)
),
mainPanel(
plotOutput("plot")
)
)
)
# Define the server logic
server <- function(input, output) {
output$plot <- renderPlot({
x <- input$slider
plot(x, x^2, main = paste("Plot of x and x^2 (x =", x, ")"))
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
In this example, a simple scatter plot of x
and x^2
is generated based on the value selected by the user through a slider input.
Embedding Interactive Plots in Shiny
For more dynamic and interactive plots, you can use the plotly
package, which enables you to create interactive plots that users can zoom, pan, and hover over. To embed an interactive plot from plotly
into your Shiny app, use plotlyOutput()
in the UI and renderPlotly()
in the server function.
# Load the necessary packages
library(shiny)
library(plotly)
# Define the UI
ui <- fluidPage(
titlePanel("Embedding Interactive Plot Example"),
sidebarLayout(
sidebarPanel(
sliderInput("slider", "Choose a value for x:", min = 1, max = 100, value = 50)
),
mainPanel(
plotlyOutput("plot")
)
)
)
# Define the server logic
server <- function(input, output) {
output$plot <- renderPlotly({
x <- input$slider
plot_ly(x = ~c(x, x^2), type = 'scatter', mode = 'lines+markers', name = 'x and x^2')
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
In this example, we use the plot_ly()
function from the plotly
package to create an interactive scatter plot. The plot allows users to interact with the data, such as zooming and hovering to view specific values.
Embedding Tables in Shiny
Shiny also allows you to embed tables into the app using renderTable()
and tableOutput()
. These functions enable you to display static or reactive tables in your app. Below is an example of embedding a simple table into a Shiny app:
# Load the Shiny package
library(shiny)
# Define the UI
ui <- fluidPage(
titlePanel("Embedding Table Example"),
sidebarLayout(
sidebarPanel(
selectInput("column", "Select a column:", choices = c("mpg", "hp", "wt"))
),
mainPanel(
tableOutput("table")
)
)
)
# Load a sample dataset
data(mtcars)
# Define the server logic
server <- function(input, output) {
output$table <- renderTable({
# Select a column from the dataset based on user input
selected_column <- mtcars[[input$column]]
data.frame(Value = selected_column)
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
This example displays a table of values from the selected column of the mtcars
dataset. The column is selected dynamically by the user through a dropdown menu.
Embedding Interactive Tables with DT
For more interactive tables with features like sorting and filtering, you can use the DT
package, which provides a convenient interface for working with DataTables
in Shiny. To embed an interactive table, use DTOutput()
in the UI and renderDT()
in the server function.
# Load the necessary packages
library(shiny)
library(DT)
# Define the UI
ui <- fluidPage(
titlePanel("Embedding Interactive Table Example"),
sidebarLayout(
sidebarPanel(
selectInput("column", "Select a column:", choices = c("mpg", "hp", "wt"))
),
mainPanel(
DTOutput("table")
)
)
)
# Load a sample dataset
data(mtcars)
# Define the server logic
server <- function(input, output) {
output$table <- renderDT({
# Select a column from the dataset based on user input
selected_column <- mtcars[[input$column]]
datatable(data.frame(Value = selected_column))
})
}
# Run the Shiny app
shinyApp(ui = ui, server = server)
This example uses the DT
package to create an interactive table where the user can select a column from the mtcars
dataset and interact with the table (e.g., sorting, searching).
Summary
Embedding plots and tables into Shiny applications is a powerful way to display and explore data interactively. Whether you are using static plots, interactive plots from plotly
, or tables from the DT
package, Shiny provides a flexible and dynamic environment to present data and allow users to interact with it. You can combine these elements to create dashboards and reports that are both informative and engaging.
Connecting to Databases with DBI and RSQLite
In R, databases can be accessed and manipulated using the DBI
package, which provides a consistent interface for working with various database management systems. For SQLite, a lightweight, serverless database, the RSQLite
package is commonly used. This section will guide you through the process of connecting to an SQLite database in R using these packages, performing queries, and retrieving results.
Installing DBI and RSQLite
Before you can connect to a database in R, you need to install the required packages. You can install DBI
and RSQLite
using the following commands:
# Install DBI and RSQLite packages
install.packages("DBI")
install.packages("RSQLite")
Once these packages are installed, you can load them into your R session:
# Load the necessary packages
library(DBI)
library(RSQLite)
Connecting to an SQLite Database
To connect to an SQLite database, use the dbConnect()
function from the DBI
package, specifying the driver (e.g., RSQLite::SQLite()
) and the database file path. If the database file does not exist, it will be created automatically.
# Connect to an SQLite database
con <- dbConnect(RSQLite::SQLite(), "my_database.db")
This command creates a connection to a database named my_database.db
. If the database does not already exist, it will be created in your working directory.
Creating Tables and Inserting Data
After establishing a connection, you can create tables and insert data into the database using SQL commands. The dbExecute()
function allows you to run SQL queries that modify the database, such as creating tables and inserting data.
# Create a table in the SQLite database
dbExecute(con, "
CREATE TABLE users (
id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER
)
")
# Insert data into the table
dbExecute(con, "INSERT INTO users (name, age) VALUES ('Alice', 30)")
dbExecute(con, "INSERT INTO users (name, age) VALUES ('Bob', 25)")
In this example, we create a table called users
with three columns: id
, name
, and age
. Then, we insert two rows of data into the table.
Querying Data from the Database
To retrieve data from the database, you can use the dbGetQuery()
function. This function allows you to execute a SELECT query and return the results as a data frame.
# Retrieve data from the users table
result <- dbGetQuery(con, "SELECT * FROM users")
print(result)
The result of this query is a data frame containing all rows from the users
table. You can then manipulate and analyze the data as needed in R.
Updating and Deleting Data
To modify or delete data in the database, you can use dbExecute()
to run UPDATE or DELETE SQL queries. For example, you can update a user's age or delete a row from the table:
# Update data in the users table
dbExecute(con, "UPDATE users SET age = 35 WHERE name = 'Alice'")
# Delete data from the users table
dbExecute(con, "DELETE FROM users WHERE name = 'Bob'")
In this example, we update Alice's age to 35 and then delete Bob from the table.
Disconnecting from the Database
After performing your database operations, it's important to disconnect from the database using the dbDisconnect()
function. This ensures that resources are properly released.
# Disconnect from the SQLite database
dbDisconnect(con)
Summary
Using the DBI
and RSQLite
packages, you can easily connect to SQLite databases, execute SQL queries, and retrieve or manipulate data in R. This allows you to work with databases directly within your R environment, making it easier to integrate R with other systems and manage large datasets. The DBI
package provides a unified interface for working with various database backends, making it a powerful tool for data analysis and management.
Querying Databases with R
R provides several tools to connect to and query databases directly from your R environment. The DBI
package, together with specific database drivers like RSQLite
for SQLite or RMySQL
for MySQL, allows you to execute SQL queries and retrieve results in R. This section will cover how to query databases efficiently using SQL commands in R and work with the results.
Setting Up the Database Connection
Before you can query a database, you need to establish a connection using the dbConnect()
function. You'll need to load the necessary library, such as DBI
and a specific driver like RSQLite
or RMySQL
, depending on the type of database you are working with.
# Install and load DBI and database-specific package (RSQLite in this case)
install.packages("DBI")
install.packages("RSQLite")
library(DBI)
library(RSQLite)
# Establish a connection to the database
con <- dbConnect(RSQLite::SQLite(), "my_database.db")
In this example, we have connected to an SQLite database named my_database.db
. Replace RSQLite::SQLite()
with the appropriate driver if you are working with other types of databases (e.g., RMySQL::MySQL()
for MySQL).
Executing Queries
You can execute SQL queries in R using the dbGetQuery()
function. This function allows you to run any valid SQL query and returns the results as a data frame. Here’s an example of how to query the database to retrieve specific data:
# Query the database to retrieve all rows from the users table
result <- dbGetQuery(con, "SELECT * FROM users")
print(result)
The query returns all records from the users
table. The result is stored as a data frame, which you can manipulate and analyze within R.
Using SQL Queries with Filtering
To filter data, you can add SQL conditions to your queries using WHERE
. For example, if you want to retrieve users over the age of 30, you can use a query like this:
# Query to select users older than 30
result <- dbGetQuery(con, "SELECT * FROM users WHERE age > 30")
print(result)
This query will return only the users whose age
column is greater than 30.
Joining Tables
If you need to retrieve data from multiple tables, you can use SQL JOIN
statements. Here’s an example that joins two tables, users
and orders
, based on a common column:
# Query to join users and orders tables based on user_id
result <- dbGetQuery(con, "
SELECT users.name, users.age, orders.order_id
FROM users
INNER JOIN orders ON users.id = orders.user_id
")
print(result)
This query retrieves the user's name and age along with the associated order ID by joining the users
and orders
tables.
Aggregating Data
SQL provides powerful aggregation functions such as COUNT()
, SUM()
, AVG()
, and more. You can use these functions to summarize data. For example, to calculate the average age of users in the database:
# Query to calculate the average age of users
result <- dbGetQuery(con, "SELECT AVG(age) AS avg_age FROM users")
print(result)
This query calculates and returns the average age of all users in the users
table.
Working with Date and Time in Queries
If your database contains date or time values, you can use SQL functions to filter and manipulate these types of data. For instance, to select users who were added after a certain date:
# Query to select users added after a specific date
result <- dbGetQuery(con, "
SELECT * FROM users
WHERE created_at > '2022-01-01'
")
print(result)
In this case, the query selects users whose created_at
field is later than January 1, 2022.
Closing the Database Connection
After you finish querying the database, it’s good practice to close the connection. You can use the dbDisconnect()
function to safely disconnect from the database:
# Disconnect from the database
dbDisconnect(con)
Summary
Querying databases with R is straightforward using the DBI
package, combined with specific database drivers such as RSQLite
or RMySQL
. You can execute SQL queries to retrieve, filter, aggregate, and join data directly within R. The results are returned as data frames, which can be manipulated and analyzed further. Always ensure to disconnect from the database once you're done to release resources properly.
Working with SQL in R
R provides powerful capabilities for integrating with SQL databases. You can use R to run SQL queries directly against relational databases, execute complex queries, and store the results for further analysis. The DBI
package and database-specific drivers like RSQLite
or RMySQL
allow seamless interaction with SQL databases. This section will cover the essentials of working with SQL in R, including executing queries, retrieving results, and manipulating data.
Setting Up the Database Connection
To interact with a SQL database, first, you need to establish a connection using the dbConnect()
function from the DBI
package. You will also need to install and load a database driver depending on the type of database you are working with. For example, RSQLite
for SQLite databases or RMySQL
for MySQL databases.
# Install and load DBI and specific database driver (RSQLite for SQLite database)
install.packages("DBI")
install.packages("RSQLite")
library(DBI)
library(RSQLite)
# Connect to the database (SQLite example)
con <- dbConnect(RSQLite::SQLite(), "my_database.db")
Replace RSQLite::SQLite()
with the appropriate driver (e.g., RMySQL::MySQL()
) if you are working with MySQL or another database system.
Executing SQL Queries
Once connected to the database, you can use the dbGetQuery()
function to execute SQL queries and retrieve the results. The result will be returned as a data frame. You can run SELECT queries to retrieve specific data from the database.
# Execute a SQL query to get all records from the users table
result <- dbGetQuery(con, "SELECT * FROM users")
print(result)
The query will retrieve all data from the users
table and return it as a data frame that you can manipulate further in R.
Filtering Data with SQL
You can filter the results of a query using the WHERE
clause in SQL. For example, if you want to retrieve records where the age is greater than 30, you can use the following SQL query:
# Query to get users older than 30
result <- dbGetQuery(con, "SELECT * FROM users WHERE age > 30")
print(result)
This query returns only the users where the age
column is greater than 30.
Using SQL Aggregation Functions
SQL provides several aggregation functions, such as COUNT()
, SUM()
, AVG()
, and MAX()
, that allow you to perform calculations on your data. Here's an example of how to calculate the average age of users in the database:
# Query to calculate the average age
result <- dbGetQuery(con, "SELECT AVG(age) AS avg_age FROM users")
print(result)
This query calculates and returns the average age of all users from the users
table.
Joining Tables in SQL
To retrieve data from multiple tables, you can use SQL JOIN
statements. For example, you might want to join the users
table with an orders
table to get information about users and their orders:
# Query to join users and orders tables based on user_id
result <- dbGetQuery(con, "
SELECT users.name, users.age, orders.order_id
FROM users
INNER JOIN orders ON users.id = orders.user_id
")
print(result)
This query joins the users
table with the orders
table based on the user_id
column and retrieves users' names, ages, and their corresponding order IDs.
Inserting Data into a Table
In addition to querying data, you can also insert data into your database using SQL INSERT INTO
statements. Here's how to insert a new user into the users
table:
# Insert a new user into the users table
dbExecute(con, "INSERT INTO users (name, age) VALUES ('John Doe', 28)")
The dbExecute()
function is used for SQL commands that do not return data, like INSERT
, UPDATE
, or DELETE
.
Updating Data in the Database
To update existing data in the database, you can use an SQL UPDATE
statement. For example, to update the age of a specific user:
# Update the age of a user based on name
dbExecute(con, "UPDATE users SET age = 29 WHERE name = 'John Doe'")
This query updates the age of the user named "John Doe" to 29.
Deleting Data from the Database
If you need to delete data from the database, you can use the DELETE
statement. For example, to delete a user from the users
table:
# Delete a user from the users table
dbExecute(con, "DELETE FROM users WHERE name = 'John Doe'")
This query deletes the user named "John Doe" from the users
table.
Closing the Database Connection
It’s important to close the database connection once you have finished working with it. Use the dbDisconnect()
function to close the connection:
# Close the database connection
dbDisconnect(con)
Summary
Working with SQL in R is straightforward using the DBI
package and database-specific drivers. You can execute SQL queries to retrieve, filter, aggregate, update, and delete data directly from relational databases. The results of queries are returned as data frames, which you can work with in R. Always remember to close the database connection after completing your tasks to release resources properly.
Fetching Data from APIs with httr
R provides the httr
package to interact with RESTful APIs and fetch data over HTTP. This package allows you to send requests to APIs, handle responses, and process the data returned from the API in a convenient way. Common operations like sending GET, POST, PUT, and DELETE requests, along with handling authentication, headers, and query parameters, are simple with httr
.
Installing and Loading the httr Package
Before you can use the httr
package, you need to install it and load it into your R session:
# Install and load the httr package
install.packages("httr")
library(httr)
Sending a GET Request
The most common API request is a GET request, which retrieves data from an API. You can use GET()
function to send a GET request and retrieve the response. The response can then be parsed into a usable format, such as JSON or XML.
# Sending a GET request to an API
response <- GET("https://api.example.com/data")
# Check the status code of the response
status_code(response)
# Parse the response content into a JSON object
data <- content(response, "parsed")
print(data)
The status_code()
function checks the response status code (e.g., 200 for success), and the content()
function extracts the content of the response. We use the "parsed"
argument to parse the content into a structured format like JSON.
Handling Query Parameters
Often, APIs require query parameters to filter or customize the response. You can easily add query parameters to your GET request using the query
argument:
# Sending a GET request with query parameters
response <- GET("https://api.example.com/data", query = list(limit = 10, page = 2))
# Parse the response
data <- content(response, "parsed")
print(data)
In this example, we are requesting the first 10 items from page 2 of the API's data using the query
argument to specify limit
and page
parameters.
Sending a POST Request
In addition to GET requests, you can send POST requests to submit data to an API. The POST()
function allows you to send data in the body of the request, which is useful for creating new records or performing actions.
# Sending a POST request with JSON data
response <- POST("https://api.example.com/data",
body = list(name = "John", age = 30),
encode = "json")
# Check the response status code
status_code(response)
# Parse the response content
data <- content(response, "parsed")
print(data)
In this example, we send a POST request to create a new record with a name and age. The data is encoded as JSON using encode = "json"
.
Handling Authentication
Some APIs require authentication using an API key, OAuth tokens, or other methods. The httr
package provides functions for handling various types of authentication, including API keys in headers or OAuth tokens.
# Sending a GET request with an API key for authentication
response <- GET("https://api.example.com/data",
add_headers(Authorization = "Bearer YOUR_API_KEY"))
# Check the response status code
status_code(response)
# Parse the response
data <- content(response, "parsed")
print(data)
In this example, we add an API key in the Authorization header using the add_headers()
function. Replace YOUR_API_KEY
with your actual API key.
Handling Response Formats
APIs often return data in formats such as JSON, XML, or plain text. The httr
package can automatically parse common formats like JSON. You can specify the format you expect in the content()
function.
# Handling JSON response
response <- GET("https://api.example.com/data")
data <- content(response, "parsed") # Automatically parses JSON
print(data)
# Handling raw text response
response <- GET("https://api.example.com/textdata")
text_data <- content(response, "text")
print(text_data)
If the API returns plain text, use "text"
to retrieve and print it as text. The content()
function also supports other formats like XML and raw binary data.
Handling Errors
APIs may return errors if the request is not successful. It's essential to handle errors gracefully, check the status code of the response, and manage different failure cases.
# Check if the request was successful
if (status_code(response) == 200) {
data <- content(response, "parsed")
print(data)
} else {
print("Error: API request failed")
}
In this example, we check if the status code is 200 (OK). If not, we print an error message. You can handle other status codes accordingly (e.g., 404 for "Not Found", 500 for "Server Error").
Summary
The httr
package in R makes it easy to interact with APIs by providing functions for sending GET, POST, PUT, and DELETE requests. You can pass query parameters, handle authentication, and work with different response formats like JSON and text. With proper error handling and API interactions, R can be a powerful tool for retrieving and processing data from APIs for various applications.
Parsing JSON and XML Data in R
R provides packages like jsonlite
and xml2
to parse and work with JSON and XML data, respectively. These formats are commonly used for data exchange in APIs, and R makes it easy to read, process, and analyze this data.
Parsing JSON Data
JSON (JavaScript Object Notation) is a lightweight data interchange format. The jsonlite
package provides functions to convert JSON data into R objects and vice versa. You can use the fromJSON()
function to parse JSON data into R objects.
# Install and load the jsonlite package
install.packages("jsonlite")
library(jsonlite)
# Sample JSON data
json_data <- '{"name": "John", "age": 30, "city": "New York"}'
# Parse the JSON data into an R object
parsed_data <- fromJSON(json_data)
# Print the parsed data
print(parsed_data)
In this example, we use the fromJSON()
function to convert a JSON string into a list. JSON objects are typically converted into R lists, where each key-value pair becomes an element of the list.
Handling JSON from API Responses
When you fetch data from an API that returns JSON, you can directly parse it using fromJSON()
after retrieving the response. Here’s how you can do it:
# Fetching JSON data from an API and parsing it
library(httr)
response <- GET("https://api.example.com/data")
json_data <- content(response, "text")
# Parse the JSON data
parsed_data <- fromJSON(json_data)
# Print the parsed data
print(parsed_data)
Parsing XML Data
XML (Extensible Markup Language) is another popular data format used in data exchange. The xml2
package allows you to parse XML data and extract information from it. The read_xml()
function from the xml2
package reads XML data into an R object.
# Install and load the xml2 package
install.packages("xml2")
library(xml2)
# Sample XML data
xml_data <- 'John 30 New York '
# Parse the XML data
parsed_xml <- read_xml(xml_data)
# Print the parsed XML data
print(parsed_xml)
The read_xml()
function parses the XML string and converts it into an XML document object. You can then extract specific elements using XPath queries or simple extraction functions.
Extracting Data from XML
Once the XML data is parsed, you can extract individual elements such as text values, attributes, and nodes using various functions from the xml2
package.
# Extracting data from the parsed XML
name <- xml_text(xml_find_first(parsed_xml, ".//name"))
age <- xml_text(xml_find_first(parsed_xml, ".//age"))
city <- xml_text(xml_find_first(parsed_xml, ".//city"))
# Print extracted values
print(paste("Name:", name))
print(paste("Age:", age))
print(paste("City:", city))
In this example, the xml_find_first()
function is used to find the first occurrence of an element (e.g., name
), and xml_text()
extracts the text content of the element.
Parsing XML from an API
Just like with JSON, you can fetch XML data from an API and parse it using xml2
. Here's how you can handle XML data from an API response:
# Fetching XML data from an API and parsing it
response <- GET("https://api.example.com/xml-data")
xml_data <- content(response, "text")
# Parse the XML data
parsed_xml <- read_xml(xml_data)
# Extract specific elements from the XML
name <- xml_text(xml_find_first(parsed_xml, ".//name"))
print(paste("Name:", name))
Converting Between JSON and XML
Sometimes, you might need to convert JSON data to XML or vice versa. While there is no direct function to convert between these formats in R, you can manually parse the data and then convert it.
# Convert a JSON object to an XML-like structure
json_data <- fromJSON('{"name": "John", "age": 30}')
xml_data <- as_xml_document(list(person = json_data))
# Print the XML-like structure
print(xml_data)
Summary
R makes it easy to parse JSON and XML data using the jsonlite
and xml2
packages. Whether you're interacting with APIs or working with local data files, these packages provide simple and efficient methods for converting these formats into R objects for analysis. The fromJSON()
function helps parse JSON data, while the read_xml()
function allows you to work with XML documents. You can also extract specific elements from these formats and manipulate the data as needed.
Automating API Requests in R
Automating API requests is a common task in data collection, especially when you need to fetch data from an API at regular intervals or process multiple API endpoints programmatically. In R, you can automate API requests using the httr
package for sending requests and handling responses, and cronR
or base R functions like Sys.sleep()
for scheduling and repeating the requests.
Making API Requests with httr
The httr
package is a powerful tool for interacting with APIs in R. It allows you to send GET, POST, PUT, and DELETE requests, handle responses, and work with different types of data formats (JSON, XML, etc.).
# Install and load the httr package
install.packages("httr")
library(httr)
# Example of a GET request to an API endpoint
url <- "https://api.example.com/data"
response <- GET(url)
# Check the response status
status_code(response)
# Extract and print the content (JSON, XML, etc.)
data <- content(response, "text")
print(data)
In this example, a GET request is made to an API endpoint, and the response status code is checked. The response content is then extracted as text and printed.
Automating Requests with a Loop
To automate API requests for multiple endpoints or repeated requests, you can use a loop to iterate over a list of API endpoints or make periodic requests. Here's an example of automating requests with a loop:
# Define a list of API endpoints
endpoints <- c("https://api.example.com/data1", "https://api.example.com/data2", "https://api.example.com/data3")
# Loop over the endpoints and make GET requests
for (url in endpoints) {
response <- GET(url)
data <- content(response, "text")
print(paste("Data from", url, ":", data))
Sys.sleep(2) # Sleep for 2 seconds to avoid overloading the server
}
This loop iterates over a list of API endpoints, sends a GET request to each endpoint, retrieves the data, and prints it. The Sys.sleep(2)
function is used to pause for 2 seconds between requests to avoid overwhelming the server with too many requests in a short period.
Scheduling Repeated Requests
For more advanced automation, such as making requests at fixed intervals, you can use a scheduling package like cronR
, which allows you to schedule tasks (e.g., API requests) to run at specified times. Here's an example of scheduling a function to run every hour:
# Install and load the cronR package
install.packages("cronR")
library(cronR)
# Define the function that will make the API request
api_request_function <- function() {
url <- "https://api.example.com/data"
response <- GET(url)
data <- content(response, "text")
print(data)
}
# Schedule the function to run every hour
cron_add(api_request_function, frequency = "hourly")
In this example, the cron_add()
function schedules the api_request_function
to run every hour. The function fetches data from the API and prints the response.
Handling API Rate Limits
Many APIs impose rate limits to avoid overloading their servers. It’s important to respect these limits when automating requests. You can handle rate limits by checking the response headers for the X-RateLimit-Remaining
field and adjusting the request frequency accordingly. Here's how you can handle rate limits:
# Example of handling rate limits
rate_limit <- function() {
url <- "https://api.example.com/data"
response <- GET(url)
# Check the rate limit status
remaining <- headers(response)$`X-RateLimit-Remaining`
if (remaining == 0) {
reset_time <- headers(response)$`X-RateLimit-Reset`
wait_time <- as.numeric(reset_time) - Sys.time()
message("Rate limit exceeded. Waiting for ", wait_time, " seconds.")
Sys.sleep(wait_time) # Wait for the rate limit reset
}
data <- content(response, "text")
print(data)
}
# Make a request with rate limit handling
rate_limit()
This function checks the remaining API calls by looking at the X-RateLimit-Remaining
header. If the limit is reached, it waits until the rate limit resets using the X-RateLimit-Reset
header.
Logging and Error Handling
When automating API requests, it’s important to include error handling to manage any issues that arise (e.g., network failures, invalid responses, etc.). You can log the status of each request and handle errors gracefully:
# Function with error handling and logging
make_api_request <- function(url) {
tryCatch({
response <- GET(url)
stop_for_status(response) # Check if the request was successful
data <- content(response, "text")
print(paste("Data from", url, ":", data))
}, error = function(e) {
message("Error occurred while making request to ", url, ": ", e$message)
})
}
# Example of calling the function
make_api_request("https://api.example.com/data")
This function uses tryCatch()
to handle errors that may occur during the API request. If an error occurs, a message is logged, and the function continues without crashing.
Summary
Automating API requests in R is easy using the httr
package for sending requests and receiving responses. You can schedule requests using loops or scheduling packages like cronR
, and handle rate limits and errors using appropriate logic. Whether you're collecting data from multiple endpoints or making requests at regular intervals, R provides a robust set of tools to automate your workflow efficiently.
Debugging R Code with browser() and traceback()
Debugging is an essential part of programming, and R provides several tools to help identify and fix issues in your code. Two commonly used functions for debugging in R are browser()
and traceback()
. These functions allow you to inspect your code's execution flow, examine variables, and identify errors or unexpected behavior.
Using browser() for Interactive Debugging
The browser()
function is used to pause code execution at a specific point and open an interactive debugging environment. This allows you to inspect variables, step through the code line by line, and evaluate expressions during runtime. It is useful when you want to debug a specific part of your code in more detail.
# Example of using browser() for debugging
my_function <- function(x, y) {
z <- x + y
browser() # Execution will pause here
result <- z / (x - y)
return(result)
}
# Call the function
my_function(5, 0)
In this example, the execution of my_function()
will pause when it reaches the browser()
function. At this point, you can interact with the R environment, check the values of variables, and step through the code to understand what is happening. For instance, you can type z
in the console to check its value, or use commands like n
to step to the next line of code.
Using traceback() to View Call Stack
The traceback()
function is used to view the call stack after an error occurs. It provides a list of function calls that led to the error, making it easier to trace the origin of the problem and identify where the error occurred.
# Example of using traceback() after an error
error_function <- function(x) {
result <- 10 / x
return(result)
}
# Call the function with 0 to generate an error
error_function(0)
traceback() # View the call stack after the error
In this example, calling error_function(0)
will cause a division by zero error. After the error, traceback()
will show the sequence of function calls that led to the error, helping you identify the specific line in the code where the issue occurred.
Combining browser() and traceback()
You can combine browser()
and traceback()
to make your debugging process more efficient. For example, you can use browser()
to pause execution at a specific point in your code, inspect variables, and then use traceback()
to check the call stack if an error occurs.
# Example combining browser() and traceback()
debugging_function <- function(x) {
y <- x + 2
browser() # Pause here for debugging
result <- 10 / (x - y) # This line will cause an error
return(result)
}
# Call the function
debugging_function(5)
traceback() # Use traceback() after the error
In this example, the function will pause at the browser()
line, allowing you to inspect the variables x
and y
. After stepping through the code, you will reach the point where the error occurs, and you can use traceback()
to view the call stack.
Additional Debugging Tips
- Using print statements: In addition to
browser()
, you can useprint()
to print the values of variables at different points in your code to track the flow of execution. - Using debug() and undebug(): The
debug()
function allows you to step through a function line by line, similar tobrowser()
, but without pausing the entire code. You can useundebug()
to remove the debugging functionality after you're done. - Using options(error = recover): This option allows you to enter a browser-like environment when an error occurs, similar to using
browser()
at the point of the error.
Summary
Debugging R code with browser()
and traceback()
is a powerful way to identify and fix errors in your code. browser()
allows you to pause execution and inspect variables interactively, while traceback()
helps you view the call stack after an error to understand the sequence of function calls that led to the issue. By combining these tools with other debugging techniques, you can streamline the process of identifying and resolving bugs in your R code.
Handling Errors and Warnings with tryCatch()
In R, errors and warnings are common during the execution of code, especially when working with external data or complex calculations. The tryCatch()
function is used to handle errors and warnings gracefully, allowing your code to continue running even when an error occurs. This function is particularly useful when you want to handle specific errors or warnings and take appropriate actions without terminating the entire program.
Understanding tryCatch()
The tryCatch()
function allows you to catch errors and warnings, and execute different actions based on the type of issue. The basic syntax of tryCatch()
looks like this:
tryCatch({
# Code that might produce an error or warning
expr
}, error = function(e) {
# Code to handle the error
message("An error occurred: ", e$message)
}, warning = function(w) {
# Code to handle the warning
message("A warning occurred: ", w$message)
}, finally = {
# Code to execute after the tryCatch block, regardless of error or warning
message("Execution completed.")
})
In this syntax:
expr
: The expression or code that might cause an error or warning.error
: A function that defines how to handle errors. It takes an error object as an argument (e
), which contains details about the error.warning
: A function that defines how to handle warnings. It takes a warning object (w
) as an argument.finally
: A block of code that is always executed, whether or not an error or warning occurred.
Example: Handling Errors with tryCatch()
In the following example, we will attempt to divide by zero, which would normally result in an error. Using tryCatch()
, we can catch the error and handle it gracefully:
# Example of error handling
safe_divide <- function(x, y) {
tryCatch({
result <- x / y
return(result)
}, error = function(e) {
message("Error: Division by zero is not allowed.")
return(NA) # Return NA if an error occurs
})
}
# Call the function with a divisor of 0
safe_divide(10, 0)
In this example, if the user attempts to divide by zero, the tryCatch()
function will catch the error and print a custom error message. The function will return NA
instead of causing the program to stop.
Example: Handling Warnings with tryCatch()
Warnings are less severe than errors but still require attention. Here’s an example of handling warnings when performing an operation that might trigger a warning, such as coercing a value to a different type:
# Example of warning handling
convert_to_numeric <- function(x) {
tryCatch({
result <- as.numeric(x)
return(result)
}, warning = function(w) {
message("Warning: Could not convert to numeric.")
return(NA) # Return NA if a warning occurs
})
}
# Call the function with a non-numeric string
convert_to_numeric("abc")
In this case, the function will attempt to convert a non-numeric string to a number, which will trigger a warning. The tryCatch()
function will catch the warning and print a custom warning message, returning NA
instead of causing an issue.
Using finally in tryCatch()
The finally
block is always executed, regardless of whether an error or warning occurs. It can be used to perform cleanup tasks or execute code that should always run after the main expression is evaluated:
# Example of using finally
cleanup_example <- function(x, y) {
tryCatch({
result <- x / y
return(result)
}, error = function(e) {
message("Error: ", e$message)
return(NA)
}, finally = {
message("Cleanup done. Execution finished.")
})
}
# Call the function with a divisor of 0
cleanup_example(10, 0)
Even if an error occurs, the finally
block will be executed, printing the message "Cleanup done. Execution finished." This ensures that important cleanup code is always run.
Summary
The tryCatch()
function in R is a powerful tool for handling errors and warnings. By using error
and warning
handlers, you can catch issues and respond to them without stopping the execution of your code. The finally
block ensures that certain actions are taken regardless of whether an error or warning occurred. This makes tryCatch()
an essential tool for writing robust and reliable R code.
Creating Reports with R Markdown
R Markdown is a powerful tool that allows you to combine R code and narrative text in a single document. It enables you to create dynamic reports, presentations, and dashboards that can be rendered into various formats such as HTML, PDF, and Word. R Markdown is widely used for data analysis, reproducible research, and generating reports that integrate code, output, and analysis.
What is R Markdown?
R Markdown documents consist of three main components:
- Markdown Text: A lightweight markup language used for formatting the text. Markdown allows you to write formatted text using simple symbols (e.g., for headings, bullet points, links, etc.).
- R Code Chunks: Blocks of R code embedded within the document. These chunks are executed when the report is rendered, and their output is included in the document.
- Output Format: The desired format for the final report, such as HTML, PDF, or Word.
Basic Structure of an R Markdown File
An R Markdown document typically starts with a YAML header that defines metadata like the title, author, and output format. The body of the document contains markdown-formatted text and R code chunks. Here's a basic example of an R Markdown file:
---
title: "Sample Report"
author: "John Doe"
output: html_document
---
## Introduction
This is a report created using R Markdown. Below is an R code chunk that calculates the mean of a dataset.
```{r}
data <- c(10, 20, 30, 40, 50)
mean_value <- mean(data)
mean_value
```
## Conclusion
The mean of the dataset is displayed above.
The document starts with the YAML header, followed by sections of markdown text. The R code chunk is enclosed by triple backticks and preceded by `{r}` to indicate that the code should be evaluated. The output of the code chunk (the mean value) will be displayed in the final report.
Rendering the Report
Once you've written your R Markdown document, you can render it to your desired output format (e.g., HTML, PDF, Word) by using the knitr
package in R. You can do this either by clicking the "Knit" button in RStudio or using the following R command:
rmarkdown::render("your_report.Rmd")
RStudio will execute the R code chunks in the document and create the output file (e.g., your_report.html
) with the results embedded within the narrative text.
Customizing the Output
R Markdown allows you to customize the output in various ways. Some common options include:
- Output Format: You can specify the output format in the YAML header (e.g.,
html_document
,pdf_document
,word_document
). - Code Chunk Options: You can control the behavior of individual code chunks using options such as
echo
(whether to display the code),results
(how to display the results), andmessage
(whether to show messages or warnings).
Example of a customized R code chunk:
```{r, echo=FALSE, results='hide'}
data <- c(10, 20, 30, 40, 50)
mean(data)
```
In this example, the echo=FALSE
option hides the code from the output, and results='hide'
hides the output of the code chunk.
Including Plots in R Markdown
R Markdown makes it easy to include plots generated in R directly into the report. For example, you can create a plot using ggplot2
and embed it in the document:
library(ggplot2)
ggplot(mtcars, aes(x=mpg, y=hp)) + geom_point()
The plot will automatically be rendered and included in the output report when you knit the document.
Including Tables in R Markdown
You can also include tables in your R Markdown reports. You can create tables using the knitr::kable()
function or other libraries like DT
for interactive tables. For example:
library(knitr)
kable(head(mtcars))
This will generate a table of the first few rows of the mtcars
dataset in the report.
Conclusion
R Markdown is an excellent tool for creating dynamic and reproducible reports in R. By combining R code with markdown text, you can produce clear, well-documented analysis results. Whether you're preparing a simple report or a complex analysis, R Markdown provides the flexibility to customize the output to suit your needs.
Exporting Reports to PDF, HTML, and Word
One of the powerful features of R Markdown is its ability to export reports to multiple formats, including PDF, HTML, and Word. This allows you to share your analysis in the format most suitable for your audience or requirements. Below, we will explore how to export R Markdown reports to these formats.
Basic Structure of an R Markdown File
To export a report, start by creating an R Markdown file with the desired content. The output format is defined in the YAML header of the document:
---
title: "Sample Report"
author: "Jane Doe"
output: html_document
---
Change the output
value to specify the desired format. The available options are:
html_document
: Exports the report as an HTML file, viewable in any web browser.pdf_document
: Exports the report as a PDF file. Requires a LaTeX distribution to be installed.word_document
: Exports the report as a Microsoft Word file.
Exporting to HTML
HTML is the default output format for R Markdown. It is ideal for sharing reports online or viewing in a web browser. To export the report to HTML:
- Set
output: html_document
in the YAML header. - Click the "Knit" button in RStudio or use the following R code:
rmarkdown::render("your_report.Rmd")
The generated HTML file can be opened in any browser and shared easily.
Exporting to PDF
Exporting to PDF requires a LaTeX distribution (e.g., TeX Live, MiKTeX, or TinyTeX) to format the report. To export to PDF:
- Install a LaTeX distribution if it is not already installed. TinyTeX is a lightweight and easy-to-install option:
install.packages("tinytex") tinytex::install_tinytex()
- Set
output: pdf_document
in the YAML header. - Click the "Knit" button or use the
rmarkdown::render()
function:
rmarkdown::render("your_report.Rmd")
The output will be a PDF file, which can be printed or shared.
Exporting to Word
Exporting to Microsoft Word is useful for creating editable documents. To export to Word:
- Set
output: word_document
in the YAML header. - Click the "Knit" button or use the
rmarkdown::render()
function:
rmarkdown::render("your_report.Rmd")
The output will be a Word document (.docx), which can be opened in Microsoft Word or similar software for further editing.
Generating Multiple Formats
You can generate multiple formats simultaneously by specifying them in the YAML header:
---
title: "Sample Report"
author: "Jane Doe"
output:
html_document: default
pdf_document: default
word_document: default
---
When you knit the document, R Markdown will create all specified output formats.
Customizing the Output
Each output format supports additional customization. For example, you can specify themes for HTML, templates for PDF, and styles for Word:
- HTML Customization: Add themes or self-contained HTML files:
output: html_document: theme: cerulean self_contained: true
- PDF Customization: Specify LaTeX templates or margins:
output: pdf_document: number_sections: true latex_engine: xelatex
- Word Customization: Use custom styles by providing a Word template:
output: word_document: reference_docx: custom_template.docx
Conclusion
Exporting reports to PDF, HTML, and Word in R Markdown provides flexibility to present your findings in the most appropriate format. With options for customization, you can tailor the output to meet specific requirements and share your analysis effectively with your audience.
Automating Reports with Parameters
R Markdown allows you to create parameterized reports, enabling dynamic customization of the output by passing different values at runtime. This is especially useful when generating reports for multiple datasets, scenarios, or users without manually altering the code.
Defining Parameters in R Markdown
To use parameters in your R Markdown file, define them in the YAML header. Here’s an example:
---
title: "Parameterized Report"
author: "Your Name"
output: html_document
params:
dataset: "default_data.csv"
report_title: "Analysis Report"
---
In this example, we define two parameters: dataset
and report_title
. These parameters can be referenced in the R Markdown document.
Using Parameters in the Report
You can access the parameters using the params
object. For instance:
# Load the dataset specified in the parameters
data <- read.csv(params$dataset)
# Use the parameterized report title
cat("##", params$report_title, "\n")
Rendering Reports with Parameters
To generate a report with specific parameter values, use the rmarkdown::render()
function in R. For example:
rmarkdown::render(
"report.Rmd",
params = list(
dataset = "sales_data.csv",
report_title = "Sales Analysis Report"
),
output_file = "sales_report.html"
)
This command renders the R Markdown file report.Rmd
with the specified parameter values and saves the output as sales_report.html
.
Interactive Parameter Input
You can enable interactive parameter input by setting params
to ask
in the YAML header:
---
title: "Interactive Report"
author: "Your Name"
output: html_document
params:
dataset:
label: "Select a dataset"
value: "default_data.csv"
input: file
report_title:
label: "Report Title"
value: "Analysis Report"
input: text
---
When the file is knitted, a dialog box will appear to collect parameter values interactively.
Generating Reports for Multiple Scenarios
Parameterized reports make it easy to automate the generation of multiple reports for different datasets or conditions. For instance:
datasets <- c("data1.csv", "data2.csv", "data3.csv")
for (dataset in datasets) {
rmarkdown::render(
"report.Rmd",
params = list(
dataset = dataset,
report_title = paste("Analysis of", dataset)
),
output_file = paste0("report_", dataset, ".html")
)
}
This loop generates separate reports for each dataset, customizing the title and file name dynamically.
Customizing Output Formats
You can also generate parameterized reports in different formats by specifying the output in the rmarkdown::render()
function:
rmarkdown::render(
"report.Rmd",
params = list(dataset = "data.csv"),
output_format = "pdf_document",
output_file = "report.pdf"
)
Conclusion
Using parameters in R Markdown adds flexibility and automation to your reporting workflow. By defining parameters and dynamically rendering reports, you can efficiently generate customized outputs for various use cases, saving time and effort.
Object-Oriented Programming in R (S3, S4, R6)
R supports object-oriented programming (OOP), enabling developers to create and manage objects with specific properties and methods. R provides three main OOP systems: S3, S4, and R6. Each system has its unique features and use cases.
S3: Simplest OOP System
S3 is a lightweight and flexible OOP system in R. It uses generic functions and method dispatch based on the object class.
Creating an S3 Object
# Define an S3 object as a list and assign a class
person <- list(name = "Alice", age = 30)
class(person) <- "Person"
Defining Methods for S3 Objects
Methods are defined for generic functions based on the object's class:
# Define a print method for the Person class
print.Person <- function(obj) {
cat("Name:", obj$name, "\nAge:", obj$age, "\n")
}
# Call the print method
print(person)
S4: Formal OOP System
S4 is a more rigorous OOP system with formal class and method definitions. It is suitable for complex object hierarchies and stricter validation.
Defining an S4 Class
# Define an S4 class
setClass(
"Person",
slots = list(name = "character", age = "numeric")
)
# Create an S4 object
person <- new("Person", name = "Bob", age = 25)
Defining Methods for S4 Objects
Methods are defined using setMethod()
:
# Define a method for the show function
setMethod(
"show",
"Person",
function(object) {
cat("Name:", object@name, "\nAge:", object@age, "\n")
}
)
# Call the show method
person
R6: Modern OOP System
R6 provides encapsulated objects with fields and methods, similar to OOP in languages like Python and Java. It is commonly used for mutable objects and advanced applications.
Defining an R6 Class
library(R6)
# Define an R6 class
Person <- R6Class(
"Person",
public = list(
name = NULL,
age = NULL,
initialize = function(name, age) {
self$name <- name
self$age <- age
},
introduce = function() {
cat("Hi, I'm", self$name, "and I'm", self$age, "years old.\n")
}
)
)
# Create an R6 object
person <- Person$new(name = "Charlie", age = 35)
person$introduce()
Comparison of S3, S4, and R6
System | Features | Use Cases |
---|---|---|
S3 | Simple, flexible, uses generic functions and dispatch. | Quick and lightweight applications, prototyping. |
S4 | Formal class and method definitions, strict validation. | Complex object hierarchies, when validation is crucial. |
R6 | Encapsulation, fields, and methods, mutable objects. | Advanced applications, reusable and modular design. |
Conclusion
R’s OOP systems provide flexibility for various needs. S3 is simple and informal, S4 is more structured, and R6 offers modern object-oriented features. Choosing the right system depends on the complexity and requirements of your application.
Writing Custom R Packages
R packages are a convenient way to bundle reusable code, datasets, and documentation. Creating a custom R package allows you to distribute your functions and tools to others or use them across multiple projects seamlessly.
Steps to Create an R Package
- Set Up the Package Directory: Use the
usethis
package or manually create the package structure. - Add Functions: Place your R functions in the
R/
directory. Each file typically contains one or more related functions. - Document Functions: Use
roxygen2
to generate documentation from comments above your functions. - Add a Description File: The
DESCRIPTION
file contains metadata about your package, such as its name, version, author, and dependencies. - Include a Namespace File: The
NAMESPACE
file defines which functions are exported for users. - Add Tests: Use the
testthat
package to write tests for your functions. - Build and Check the Package: Use
devtools
to build and test your package. - Share the Package: Distribute your package by sharing the source code or publishing it on CRAN or GitHub.
# Install the usethis package
install.packages("usethis")
# Create a new package
usethis::create_package("path/to/your/packageName")
# Example function in R/hello.R
hello <- function() {
print("Hello, world!")
}
#' Say Hello
#'
#' This function prints a simple greeting.
#' @return NULL
#' @examples
#' hello()
hello <- function() {
print("Hello, world!")
}
Run the following command to create documentation:
# Generate documentation
devtools::document()
Package: packageName
Type: Package
Title: A Brief Title for Your Package
Version: 0.1.0
Author: Your Name
Description: A short description of your package.
License: MIT
Depends: R (>= 4.0.0)
Imports: dplyr, ggplot2
# Generated by roxygen2
export(hello)
importFrom(dplyr, select)
# Install testthat
install.packages("testthat")
# Create a test file
usethis::use_test("hello")
# Example test in tests/testthat/test-hello.R
test_that("hello works", {
expect_output(hello(), "Hello, world!")
})
# Build the package
devtools::build()
# Check for issues
devtools::check()
# Publish on GitHub
usethis::use_git()
usethis::use_github()
# Install from GitHub
devtools::install_github("yourusername/packageName")
Directory Structure of an R Package
packageName/
├── R/ # R scripts with functions
├── man/ # Documentation files
├── tests/ # Test cases
├── DESCRIPTION # Package metadata
├── NAMESPACE # Exported functions and imports
├── data/ # Datasets (if any)
└── vignettes/ # Long-form documentation or tutorials
Conclusion
Creating an R package involves organizing your code, writing documentation, and testing functionality. Tools like usethis
, roxygen2
, and devtools
simplify the process, making it easier to share your work with others.
Profiling and Optimizing R Code
Profiling and optimizing R code is crucial for improving the performance of scripts and applications, especially when working with large datasets or computationally expensive tasks. R provides built-in tools and packages to identify bottlenecks and optimize code execution.
Profiling R Code
Profiling involves measuring the time and memory taken by different parts of your code to identify inefficiencies. R provides the Rprof()
function and the profvis
package for this purpose.
Using Rprof()
- Start profiling using
Rprof()
: - Analyze the profiling output with
summaryRprof()
:
# Start profiling
Rprof("profile.out")
# Code to profile
result <- sapply(1:1000, function(x) sum(rnorm(1000)))
# Stop profiling
Rprof(NULL)
# Summarize the profiling results
summaryRprof("profile.out")
Using profvis
The profvis
package provides an interactive visualization of profiling results:
# Install the profvis package
install.packages("profvis")
# Profile code interactively
library(profvis)
profvis({
result <- sapply(1:1000, function(x) sum(rnorm(1000)))
})
The output includes a graphical view of function calls and the time spent in each function.
Optimizing R Code
Once bottlenecks are identified, you can optimize your code using the following techniques:
1. Use Vectorized Operations
Replace loops with vectorized operations wherever possible, as they are faster and more efficient.
# Inefficient loop
result <- numeric(1000)
for (i in 1:1000) {
result[i] <- i^2
}
# Vectorized operation
result <- (1:1000)^2
2. Avoid Growing Objects in Loops
Pre-allocate memory for objects to avoid repeated memory allocation during loops.
# Inefficient
result <- NULL
for (i in 1:1000) {
result <- c(result, i^2)
}
# Efficient
result <- numeric(1000)
for (i in 1:1000) {
result[i] <- i^2
}
3. Use Efficient Functions
Leverage efficient functions from packages like data.table
and dplyr
for data manipulation tasks.
# Using dplyr for efficient filtering
library(dplyr)
filtered_data <- iris %>% filter(Species == "setosa")
4. Parallelize Code
Use parallel computing to distribute tasks across multiple cores for computationally intensive operations.
# Install parallel package
library(parallel)
# Parallelize using mclapply
result <- mclapply(1:1000, function(x) sum(rnorm(1000)), mc.cores = 4)
5. Profile Regularly
Regularly profile your code as you optimize it to ensure that improvements are effective and do not introduce new bottlenecks.
Conclusion
Profiling and optimizing R code is an iterative process that helps improve performance and efficiency. Tools like Rprof()
, profvis
, and efficient coding practices such as vectorization, pre-allocation, and parallelization are essential for handling large datasets and computationally intensive tasks in R.
Parallel Computing in R
Parallel computing in R allows you to execute tasks simultaneously across multiple CPU cores, reducing computation time for resource-intensive operations. R provides several packages for parallel computing, including the built-in parallel
package and the foreach
package for more flexibility.
Using the parallel
Package
The parallel
package provides core functionalities for parallel computation. It includes functions like mclapply()
and parLapply()
.
1. Multi-core Processing with mclapply()
mclapply()
applies a function to each element of a list or vector in parallel on multiple cores (Linux and macOS only).
# Example: Using mclapply()
library(parallel)
# Function to compute the sum of random numbers
compute_sum <- function(x) sum(rnorm(1000))
# Parallel execution
result <- mclapply(1:4, compute_sum, mc.cores = 4)
# Print result
print(result)
Note: On Windows, use parLapply()
instead.
2. Cluster-Based Parallelism with parLapply()
parLapply()
creates a cluster and applies a function in parallel across the cluster nodes. It works on all platforms, including Windows.
# Example: Using parLapply()
library(parallel)
# Create a cluster with 4 cores
cl <- makeCluster(4)
# Export variables and functions to the cluster
clusterExport(cl, varlist = c("rnorm"))
# Parallel execution
result <- parLapply(cl, 1:4, function(x) sum(rnorm(1000)))
# Stop the cluster
stopCluster(cl)
# Print result
print(result)
Using the foreach
Package
The foreach
package provides a flexible way to execute loops in parallel using various backends such as doParallel
or doSNOW
.
1. Setting Up doParallel
The doParallel
package enables the use of multiple cores with the foreach
package.
# Install and load required packages
install.packages("foreach")
install.packages("doParallel")
library(foreach)
library(doParallel)
# Register parallel backend
cl <- makeCluster(4)
registerDoParallel(cl)
# Parallel loop using foreach
result <- foreach(i = 1:4, .combine = c) %dopar% {
sum(rnorm(1000))
}
# Stop the cluster
stopCluster(cl)
# Print result
print(result)
2. Customizing Parallel Tasks
You can customize the behavior of foreach
loops by specifying options like the combination function, error handling, and progress monitoring.
# Example: Combining results into a list
result <- foreach(i = 1:4, .combine = list) %dopar% {
list(mean = mean(rnorm(1000)), sd = sd(rnorm(1000)))
}
# Print result
print(result)
Best Practices for Parallel Computing
- Ensure your tasks are independent and can be executed in parallel without dependencies.
- Use the appropriate number of cores to prevent system overload (e.g.,
detectCores() - 1
). - Export all necessary variables and functions to the cluster environment.
- Monitor memory usage, especially when working with large datasets.
Conclusion
Parallel computing in R significantly speeds up computational tasks by utilizing multiple CPU cores. The parallel
and foreach
packages provide robust tools for implementing parallelism in R scripts, making them valuable for handling complex and time-consuming operations.
Working with Maps in R
R provides powerful tools for creating and analyzing maps. Two popular packages for working with spatial data and maps are leaflet
for interactive maps and sf
for handling spatial data. These tools are widely used for geospatial analysis and visualization.
Creating Interactive Maps with leaflet
The leaflet
package allows you to create interactive web maps directly in R. It supports adding layers, markers, popups, and more.
1. Installing and Loading leaflet
# Install and load leaflet
install.packages("leaflet")
library(leaflet)
2. Creating a Simple Map
This example demonstrates how to create a basic map with a marker and popup.
# Create a basic leaflet map
leaflet() %>%
addTiles() %>% # Add default OpenStreetMap tiles
addMarkers(lng = -0.1276, lat = 51.5072, popup = "London, UK") # Add a marker
3. Adding Layers and Customizations
You can add multiple layers like polygons, circles, and customized tile layers to enhance the map.
# Adding layers to a leaflet map
leaflet() %>%
addProviderTiles(providers$CartoDB.Positron) %>% # Use a different tile layer
addCircles(lng = -0.1276, lat = 51.5072, radius = 500, color = "blue", fillOpacity = 0.5, popup = "Circle in London") %>%
addPolygons(lng = c(-0.15, -0.1, -0.1, -0.15), lat = c(51.5, 51.5, 51.55, 51.55), color = "green", popup = "Polygon")
Handling Spatial Data with sf
The sf
package (simple features) is a modern approach to working with spatial data. It supports reading, writing, and analyzing geospatial data in various formats like shapefiles, GeoJSON, etc.
1. Installing and Loading sf
# Install and load sf
install.packages("sf")
library(sf)
2. Reading Spatial Data
You can load spatial data from files like shapefiles or GeoJSON using st_read()
.
# Read spatial data (shapefile)
shapefile_path <- "path/to/shapefile.shp"
spatial_data <- st_read(shapefile_path)
# View the structure of the spatial data
print(spatial_data)
3. Plotting Spatial Data
Spatial data loaded with sf
can be plotted using base R or integrated with packages like ggplot2
.
# Plot spatial data
plot(spatial_data)
# Integrate with ggplot2 for customized visualization
library(ggplot2)
ggplot(data = spatial_data) +
geom_sf(fill = "lightblue", color = "darkblue") +
theme_minimal() +
labs(title = "Spatial Data Visualization")
4. Combining sf
and leaflet
You can convert sf
objects into leaflet
layers for interactive mapping.
# Convert sf object to leaflet map
leaflet(data = spatial_data) %>%
addTiles() %>%
addPolygons(fillColor = "lightgreen", color = "darkgreen", popup = ~NAME_COLUMN)
Replace NAME_COLUMN
with the appropriate column name in your spatial dataset.
Best Practices
- Choose
leaflet
for interactive web maps andsf
for spatial data analysis. - Use high-quality geospatial data from reliable sources.
- Combine multiple tools for advanced geospatial workflows, such as linking
leaflet
withsf
.
Conclusion
By leveraging leaflet
and sf
, R provides a comprehensive platform for creating, analyzing, and visualizing geospatial data. These tools empower users to perform everything from basic map creation to advanced geospatial analysis.
Geocoding and Spatial Analysis in R
Geocoding is the process of converting addresses or place names into geographic coordinates (latitude and longitude). Spatial analysis involves analyzing and visualizing geospatial data to extract meaningful insights. R provides several packages like ggmap
, tmap
, and sf
for these tasks.
Geocoding in R
To perform geocoding in R, you can use the ggmap
or tidygeocoder
package. These tools rely on APIs like Google Maps, OpenStreetMap, or others for geocoding.
1. Installing and Loading Required Packages
# Install and load ggmap and tidygeocoder
install.packages("ggmap")
install.packages("tidygeocoder")
library(ggmap)
library(tidygeocoder)
2. Geocoding with ggmap
To use ggmap
, you need a Google Maps API key for geocoding.
# Register your Google API key
register_google(key = "your_api_key")
# Geocode a single address
address <- "1600 Amphitheatre Parkway, Mountain View, CA"
geocode_result <- geocode(address, output = "latlon")
print(geocode_result)
3. Geocoding with tidygeocoder
tidygeocoder
supports batch geocoding and does not require a Google Maps API key if using free providers like OpenStreetMap's Nominatim.
# Geocode multiple addresses
addresses <- data.frame(place = c("New York, NY", "Los Angeles, CA"))
geocoded_data <- addresses %>%
geocode(place, method = "osm", lat = latitude, long = longitude)
print(geocoded_data)
Spatial Analysis in R
Spatial analysis involves operations like calculating distances, finding neighbors, and analyzing spatial patterns. The sf
package is commonly used for these tasks.
1. Calculating Distances
Use sf::st_distance()
to calculate distances between spatial objects.
library(sf)
# Create two points
point1 <- st_point(c(-73.935242, 40.730610)) # New York
point2 <- st_point(c(-118.243683, 34.052235)) # Los Angeles
# Convert to spatial objects
point1_sf <- st_sfc(point1, crs = 4326)
point2_sf <- st_sfc(point2, crs = 4326)
# Calculate the distance
distance <- st_distance(point1_sf, point2_sf)
print(distance)
2. Buffer Analysis
Create a buffer around a spatial object to analyze areas within a certain distance.
# Create a buffer of 1 km around a point
buffer <- st_buffer(point1_sf, dist = 1000)
print(buffer)
3. Spatial Joins
Perform spatial joins to combine data from different spatial datasets based on their geometry.
# Example: Join two spatial datasets
joined_data <- st_join(dataset1, dataset2, join = st_intersects)
4. Visualizing Spatial Data
Plot spatial data using ggplot2
or tmap
.
# Visualize using ggplot2
library(ggplot2)
ggplot() +
geom_sf(data = spatial_data, fill = "lightblue", color = "darkblue") +
labs(title = "Spatial Data Visualization")
Best Practices for Geocoding and Spatial Analysis
- Choose a geocoding API provider based on the scale and cost requirements of your project.
- Ensure your spatial data uses consistent coordinate reference systems (CRS).
- Use appropriate spatial joins and buffers for meaningful analysis.
Conclusion
R provides a rich ecosystem of tools for geocoding and spatial analysis. By combining packages like ggmap
, tidygeocoder
, and sf
, you can perform complex geospatial workflows, from geocoding addresses to analyzing spatial relationships.
Creating Choropleth Maps in R
Choropleth maps are a type of thematic map in which areas are shaded or patterned in proportion to a statistical variable. In R, packages like ggplot2
, tmap
, and leaflet
are commonly used to create choropleth maps.
1. Using ggplot2
to Create a Choropleth Map
ggplot2
is a powerful package for creating static choropleth maps. It works well with spatial data from the sf
package.
Example: Mapping Population Density
# Load required libraries
library(ggplot2)
library(sf)
# Load a sample shapefile (replace with your shapefile)
shapefile <- st_read(system.file("shape/nc.shp", package = "sf"))
# Add a dummy variable for population density
shapefile$population_density <- shapefile$AREA * 1000
# Create the choropleth map
ggplot(data = shapefile) +
geom_sf(aes(fill = population_density)) +
scale_fill_viridis_c(option = "plasma", name = "Population Density") +
labs(title = "Choropleth Map of Population Density") +
theme_minimal()
2. Using tmap
for Thematic Mapping
tmap
is specifically designed for creating thematic maps. It supports both static and interactive maps.
Example: Mapping Median Income
# Load tmap library
library(tmap)
# Create a choropleth map with tmap
tm_shape(shapefile) +
tm_polygons("population_density",
title = "Population Density",
palette = "Blues") +
tm_layout(title = "Population Density Map")
3. Using leaflet
for Interactive Choropleth Maps
leaflet
is ideal for creating interactive web-based maps.
Example: Interactive Map of Population Density
# Load leaflet library
library(leaflet)
# Create an interactive map
leaflet(data = shapefile) %>%
addTiles() %>%
addPolygons(fillColor = ~colorNumeric("viridis", population_density)(population_density),
weight = 1,
color = "white",
fillOpacity = 0.7,
popup = ~paste("Density:", population_density)) %>%
addLegend(pal = colorNumeric("viridis", shapefile$population_density),
values = shapefile$population_density,
title = "Population Density",
position = "bottomright")
4. Best Practices for Creating Choropleth Maps
- Ensure your data is properly preprocessed and has a meaningful variable for visualization.
- Choose an appropriate color scale that highlights differences clearly but avoids misinterpretation.
- Include legends, titles, and annotations to make the map informative.
- Use interactive maps for detailed exploration and static maps for presentations or publications.
5. Conclusion
Choropleth maps are an effective way to visualize spatial data. With packages like ggplot2
, tmap
, and leaflet
, you can create both static and interactive maps for a variety of applications. Experiment with different tools to find the best fit for your project.
Working with Biological Data in R
R is widely used in the field of bioinformatics and computational biology for analyzing and visualizing biological data. It provides a rich ecosystem of packages and tools for handling genomic, proteomic, and other types of biological datasets.
1. Key Packages for Biological Data Analysis
Bioconductor
: A comprehensive suite of tools for bioinformatics, including packages for genomic data analysis, sequencing, and annotation.seqinr
: For reading and analyzing nucleotide and protein sequences.ape
: For phylogenetic analysis and evolutionary studies.edgeR
: For differential expression analysis of RNA-Seq data.ggbio
: For genomic data visualization integrated withggplot2
.
2. Importing Biological Data
Biological data often comes in specialized formats such as FASTA, GFF, or BAM. R provides packages to handle these formats efficiently.
Example: Reading a FASTA File
# Load seqinr package
library(seqinr)
# Read a FASTA file
fasta_data <- read.fasta(file = "example.fasta")
# Display the sequences
print(fasta_data)
3. Sequence Analysis
R can be used to analyze DNA, RNA, and protein sequences, including tasks like base composition analysis, sequence alignment, and motif finding.
Example: Calculating GC Content
# Calculate GC content of a DNA sequence
gc_content <- GC(fasta_data[[1]])
print(paste("GC Content:", gc_content, "%"))
4. Genomic Data Analysis
With Bioconductor packages, you can analyze high-throughput genomic data such as RNA-Seq, ChIP-Seq, or SNP data.
Example: Differential Expression Analysis with edgeR
# Load edgeR library
library(edgeR)
# Example count matrix
counts <- matrix(c(100, 200, 150, 300, 400, 500), ncol = 2)
group <- factor(c("Control", "Treated"))
# Create DGEList object
dge <- DGEList(counts = counts, group = group)
# Estimate dispersions
dge <- estimateDisp(dge)
# Perform differential expression analysis
fit <- exactTest(dge)
topTags(fit)
5. Visualizing Biological Data
Visualization is essential for exploring and presenting biological data. R provides various plotting tools for this purpose.
Example: Visualizing Gene Expression
# Visualize gene expression using a boxplot
library(ggplot2)
gene_expression <- data.frame(
Sample = c("Control", "Control", "Treated", "Treated"),
Expression = c(5.2, 4.8, 6.3, 6.7)
)
ggplot(gene_expression, aes(x = Sample, y = Expression)) +
geom_boxplot() +
labs(title = "Gene Expression Levels", x = "Sample", y = "Expression") +
theme_minimal()
6. Phylogenetic Analysis
Phylogenetic trees represent evolutionary relationships. The ape
package allows for building and visualizing these trees.
Example: Plotting a Phylogenetic Tree
# Load ape package
library(ape)
# Create a random phylogenetic tree
tree <- rtree(5)
# Plot the tree
plot(tree, main = "Phylogenetic Tree")
7. Best Practices for Biological Data Analysis
- Ensure the integrity and preprocessing of biological data before analysis.
- Use domain-specific databases and annotations for enrichment analysis.
- Document the analysis workflow for reproducibility.
- Combine R with other tools like Python or specialized software for complex pipelines.
8. Conclusion
R is a powerful tool for biological data analysis, offering robust packages and tools for handling, analyzing, and visualizing various types of biological data. Whether you're studying sequences, genomes, or evolutionary relationships, R provides the flexibility and functionality needed for cutting-edge research.
Sequence Analysis and Genomics in R
Sequence analysis and genomics are key areas of bioinformatics where R excels. With a wide array of packages, R offers powerful tools for analyzing DNA, RNA, and protein sequences, as well as for performing various genomics-related tasks, such as alignment, variant calling, and genome-wide association studies.
1. Key Packages for Sequence Analysis and Genomics
Bioconductor
: This repository provides numerous packages for genomic data analysis, such asGenomicRanges
,edgeR
, andDESeq2
.seqinr
: A package for reading and analyzing biological sequences, including DNA, RNA, and protein sequences in formats like FASTA and GenBank.biomaRt
: Provides access to bioinformatics databases for querying genomic data, such as gene annotations, SNPs, and more.GenomicRanges
: A key package for handling and manipulating genomic ranges (e.g., genes, exons) and performing overlap analysis.vcfR
: A tool for working with VCF (Variant Call Format) files, which are commonly used for storing genetic variation data.
2. Importing Sequence Data
R can handle various sequence data formats, such as FASTA, GenBank, and GFF. The seqinr
package is frequently used for importing sequence data in these formats.
Example: Reading a FASTA File
# Load seqinr package
library(seqinr)
# Read a FASTA file
fasta_data <- read.fasta(file = "example.fasta")
# Display sequence data
print(fasta_data)
3. Sequence Alignment
Sequence alignment is a critical step in sequence analysis. It involves arranging sequences to identify regions of similarity. R provides tools for both local and global sequence alignment.
Example: Pairwise Sequence Alignment
# Load seqinr package
library(seqinr)
# Define two sequences
seq1 <- "AGCTGAC"
seq2 <- "AGCTGCC"
# Perform pairwise sequence alignment
alignment <- pairwiseAlignment(pattern = seq1, subject = seq2)
print(alignment)
4. Sequence Motif Discovery
Motif discovery is the process of identifying recurring patterns (motifs) in biological sequences. The Bioconductor
package Biostrings
helps in motif discovery and analysis.
Example: Finding Motifs in a DNA Sequence
# Load Biostrings package
library(Biostrings)
# Define a DNA sequence
dna_seq <- DNAString("AGCTAGCTGACAGT")
# Find a motif (e.g., "AGC") in the sequence
motif <- vmatchPattern("AGC", dna_seq)
print(motif)
5. Genomic Data Analysis
Genomic data analysis involves tasks like differential expression analysis, variant calling, and visualizing genomic data. R, with packages like edgeR
and DESeq2
, allows for analyzing RNA-Seq and other genomics datasets.
Example: Differential Expression Analysis with DESeq2
# Load DESeq2 package
library(DESeq2)
# Example count matrix
counts <- matrix(c(100, 200, 150, 300, 400, 500), ncol = 2)
colnames(counts) <- c("Sample1", "Sample2")
condition <- factor(c("Control", "Treated"))
# Create DESeqDataSet object
dds <- DESeqDataSetFromMatrix(countData = counts, colData = data.frame(condition), design = ~ condition)
# Perform differential expression analysis
dds <- DESeq(dds)
res <- results(dds)
print(res)
6. Variant Calling and Analysis
Variant calling is the process of identifying genetic variants such as SNPs (Single Nucleotide Polymorphisms) from sequence data. The vcfR
package is widely used for working with VCF files.
Example: Reading VCF Files
# Load vcfR package
library(vcfR)
# Read a VCF file
vcf_data <- read.vcfR("example.vcf")
# Display the VCF data
print(vcf_data)
7. Visualizing Genomic Data
Visualization is essential in genomics for understanding complex data. R offers several packages like ggbio
and plotly
for creating publication-quality plots of genomic data.
Example: Genomic Plot with ggbio
# Load ggbio package
library(ggbio)
# Example genomic range data
gr <- GRanges(seqnames = "chr1", ranges = IRanges(1, 1000))
# Visualize the genomic data
autoplot(gr)
8. Genome-Wide Association Studies (GWAS)
GWAS is a research method used to identify genetic variants associated with diseases or traits. R provides tools for performing GWAS, such as the GenomicRanges
package for handling genomic ranges.
Example: Visualizing GWAS Results
# Load ggplot2 for visualization
library(ggplot2)
# Example GWAS results (p-values)
gwas_results <- data.frame(
SNP = c("rs1", "rs2", "rs3", "rs4"),
P_value = c(0.01, 0.05, 0.0001, 0.2)
)
# Plot GWAS results
ggplot(gwas_results, aes(x = SNP, y = -log10(P_value))) +
geom_bar(stat = "identity") +
labs(title = "GWAS Results", x = "SNP", y = "-log10(P-value)") +
theme_minimal()
9. Conclusion
R provides an extensive suite of tools for sequence analysis and genomics. From DNA sequence alignment to variant calling and genomic data visualization, R is a powerful language for bioinformatics and computational biology. By leveraging packages like Bioconductor
, edgeR
, vcfR
, and more, researchers can perform comprehensive genomic analyses to gain insights into biological data.
Visualization with Bioinformatics Libraries in R
Visualization is a critical step in bioinformatics for understanding complex biological datasets. R offers several bioinformatics libraries that are tailored to visualize genomic and biological data. These libraries allow researchers to create publication-quality plots, heatmaps, genome tracks, and more, helping to interpret and communicate results effectively.
1. Key Bioinformatics Libraries for Visualization
ggplot2
: A versatile plotting system in R that can be used for a wide range of biological data visualizations, including gene expression, variant data, and more.ggbio
: An extension ofggplot2
designed specifically for genomic data visualization. It helps visualize genomic ranges, tracks, and sequence data.plotly
: A library for creating interactive plots. It's used for visualizing data dynamically and is particularly useful for exploring large-scale bioinformatics data.ComplexHeatmap
: A powerful package for creating complex heatmaps, which are particularly useful for gene expression analysis and clustering.circlize
: A package for circular visualizations, useful for creating circular heatmaps, genome-wide visualizations, and other circular plots.
2. Visualizing Genomic Data with ggbio
ggbio
is designed to integrate genomic data with ggplot2
to create plots of genomic ranges, sequence alignment, and other bioinformatics visualizations.
Example: Genomic Range Plot
# Load ggbio package
library(ggbio)
# Create a genomic range
gr <- GRanges(seqnames = "chr1", ranges = IRanges(1, 1000))
# Visualize the genomic range with autoplot
autoplot(gr)
3. Gene Expression Visualization with ggplot2
Gene expression data is typically visualized using bar plots, box plots, and scatter plots. ggplot2
is an excellent tool for these visualizations.
Example: Gene Expression Boxplot
# Load ggplot2 package
library(ggplot2)
# Example gene expression data
gene_expression <- data.frame(
Gene = c("GeneA", "GeneB", "GeneC", "GeneA", "GeneB", "GeneC"),
Expression = c(3.5, 2.8, 4.1, 3.9, 2.5, 4.0),
Condition = c("Control", "Control", "Control", "Treated", "Treated", "Treated")
)
# Create a boxplot of gene expression
ggplot(gene_expression, aes(x = Gene, y = Expression, fill = Condition)) +
geom_boxplot() +
labs(title = "Gene Expression", x = "Gene", y = "Expression Level") +
theme_minimal()
4. Visualizing Heatmaps with ComplexHeatmap
ComplexHeatmap
is a powerful package for creating complex heatmaps that are commonly used to visualize gene expression data across different samples or conditions.
Example: Creating a Heatmap
# Load ComplexHeatmap package
library(ComplexHeatmap)
# Example gene expression matrix
gene_matrix <- matrix(c(1, 3, 2, 5, 4, 3), nrow = 3, ncol = 2)
colnames(gene_matrix) <- c("Control", "Treated")
rownames(gene_matrix) <- c("GeneA", "GeneB", "GeneC")
# Create a heatmap
Heatmap(gene_matrix, name = "Gene Expression")
5. Interactive Visualization with plotly
plotly
is an interactive visualization library that makes it easy to create dynamic plots for exploring data. It is useful for visualizing large datasets, such as gene expression or variant data, where interactivity can help with data exploration.
Example: Scatter Plot with plotly
# Load plotly package
library(plotly)
# Example data
data <- data.frame(
Gene = c("GeneA", "GeneB", "GeneC"),
Expression = c(3.5, 2.8, 4.1)
)
# Create an interactive scatter plot
plot_ly(data, x = ~Gene, y = ~Expression, type = 'scatter', mode = 'markers')
6. Circular Visualizations with circlize
circlize
is a package for creating circular visualizations, which can be used for visualizing genomic data, such as chromosome-wide variant data, interactions, and more.
Example: Creating a Circular Heatmap
# Load circlize package
library(circlize)
# Example data
data <- matrix(runif(100), ncol = 10)
# Create a circular heatmap
circlize::circos.initialize(factors = rep(1:10, each = 10), xlim = c(0, 1))
circlize::circos.trackPlotRegion(factors = rep(1:10, each = 10), ylim = c(0, 1), panel.fun = function(x, y) {
circos.rect(0, 0, 1, 1, col = colorRampPalette(c("white", "blue"))(10)[1])
})
7. Conclusion
R offers a wide array of powerful libraries for visualizing biological and genomic data. Whether you’re working with sequence alignment, gene expression, or genomic ranges, packages like ggbio
, ggplot2
, ComplexHeatmap
, plotly
, and circlize
can help you create informative and effective visualizations. These visualizations can aid in the exploration of complex biological data and help communicate research findings effectively.
Sales Dashboard with Shiny
A Sales Dashboard in R using Shiny provides an interactive, dynamic interface for displaying key sales metrics, such as revenue, units sold, profit margins, and more. Shiny allows you to build web applications in R and create real-time interactive visualizations to analyze sales data and track performance over time.
1. Creating a Simple Sales Dashboard
To create a Sales Dashboard, you need to use Shiny's UI and server components. The UI will contain elements like tables, charts, and inputs, and the server will handle the logic, such as processing inputs and updating the dashboard.
Example: Basic Sales Dashboard
# Load required libraries
library(shiny)
library(ggplot2)
# Sample sales data
sales_data <- data.frame(
Product = c("Product A", "Product B", "Product C", "Product D"),
Sales = c(5000, 7000, 8000, 6000),
Profit = c(1000, 1500, 2000, 1200)
)
# Define the UI
ui <- fluidPage(
titlePanel("Sales Dashboard"),
sidebarLayout(
sidebarPanel(
h3("Sales Overview"),
selectInput("product", "Choose a Product:", choices = sales_data$Product)
),
mainPanel(
h4("Sales and Profit Overview"),
textOutput("sales_text"),
textOutput("profit_text"),
plotOutput("sales_plot")
)
)
)
# Define the server logic
server <- function(input, output) {
output$sales_text <- renderText({
product_sales <- sales_data[sales_data$Product == input$product, "Sales"]
paste("Sales for", input$product, ":", product_sales)
})
output$profit_text <- renderText({
product_profit <- sales_data[sales_data$Product == input$product, "Profit"]
paste("Profit for", input$product, ":", product_profit)
})
output$sales_plot <- renderPlot({
ggplot(sales_data, aes(x = Product, y = Sales)) +
geom_bar(stat = "identity", fill = "skyblue") +
labs(title = "Sales by Product", x = "Product", y = "Sales")
})
}
# Run the application
shinyApp(ui = ui, server = server)
This basic dashboard allows users to select a product from a dropdown menu and displays its corresponding sales and profit information. It also generates a bar chart showing sales by product.
2. Adding More Features: Filtering and Date Range
To enhance the dashboard, you can add features like filtering sales data by date range or viewing sales trends over time. A date range input allows users to select a specific period and filter the data accordingly.
Example: Sales Dashboard with Date Range
# Sample sales data with dates
sales_data <- data.frame(
Product = rep(c("Product A", "Product B", "Product C", "Product D"), each = 5),
Sales = c(5000, 6000, 7000, 8000, 9000, 5500, 6500, 7200, 7800, 8200, 6000, 6800, 7300, 7900, 8500, 6400, 7100, 7800, 8200, 8800),
Date = rep(seq.Date(from = as.Date("2023-01-01"), by = "month", length.out = 5), 4)
)
# Define the UI with date range input
ui <- fluidPage(
titlePanel("Sales Dashboard with Date Range"),
sidebarLayout(
sidebarPanel(
dateRangeInput("date_range", "Select Date Range:",
start = min(sales_data$Date), end = max(sales_data$Date)),
selectInput("product", "Choose a Product:", choices = sales_data$Product)
),
mainPanel(
plotOutput("sales_trend_plot")
)
)
)
# Define the server logic with date filtering
server <- function(input, output) {
filtered_data <- reactive({
subset(sales_data, Product == input$product & Date >= input$date_range[1] & Date <= input$date_range[2])
})
output$sales_trend_plot <- renderPlot({
ggplot(filtered_data(), aes(x = Date, y = Sales)) +
geom_line(color = "blue") +
labs(title = paste("Sales Trend for", input$product), x = "Date", y = "Sales")
})
}
# Run the application
shinyApp(ui = ui, server = server)
This version of the dashboard allows users to filter the sales data by date range and plot the sales trend for a specific product over time. The date range input ensures that users can interactively explore different time periods.
3. Conclusion
Shiny makes it easy to build interactive dashboards in R. By incorporating elements such as product selection, date filtering, and visualization, you can create a dynamic and user-friendly sales dashboard. This can help in tracking key performance indicators, analyzing sales trends, and making data-driven business decisions.
Sentiment Analysis with tidytext
Sentiment analysis is the process of determining the emotional tone behind a series of words. It is commonly used to analyze customer feedback, social media posts, reviews, and other text data to understand opinions, emotions, and attitudes. In R, the tidytext
package offers a simple and efficient way to perform sentiment analysis, leveraging the tidyverse principles for text data manipulation.
1. Introduction to tidytext
The tidytext
package provides a set of tools to work with text data in a tidy format, allowing you to easily break down text into words or n-grams, and perform various text mining tasks such as sentiment analysis, word frequency analysis, and more.
2. Installing and Loading tidytext
To get started, you need to install the tidytext
package and load it into your R session:
# Install the tidytext package if not already installed
install.packages("tidytext")
# Load the tidytext library
library(tidytext)
3. Performing Sentiment Analysis
Let's perform sentiment analysis on a sample dataset. For this purpose, we will use the get_sentiments()
function from tidytext
to access sentiment lexicons like bing
, afinn
, or nrc
. These lexicons assign sentiment scores to words, which can then be used to analyze the sentiment of a text.
Example: Sentiment Analysis on Movie Reviews
# Load additional libraries
library(dplyr)
library(tidyr)
# Sample movie reviews data
movie_reviews <- tibble(
review = c("I love this movie!", "This was a terrible film.", "An outstanding performance by the cast.", "I really hated the plot.")
)
# Tokenizing the reviews into words
movie_reviews_tidy <- movie_reviews %>%
unnest_tokens(word, review)
# Get the sentiment lexicon (using Bing lexicon)
sentiment_lexicon <- get_sentiments("bing")
# Join the reviews with the sentiment lexicon to determine sentiment
sentiment_analysis <- movie_reviews_tidy %>%
inner_join(sentiment_lexicon, by = "word") %>%
count(review, sentiment)
# View the sentiment analysis results
sentiment_analysis
In this example, the text from movie reviews is tokenized into individual words, and then we join the words with the bing
sentiment lexicon to classify each word as either positive or negative. The result is a count of positive and negative sentiments for each review.
4. Visualizing Sentiment Scores
You can visualize the sentiment analysis results using a simple bar plot to see the distribution of sentiments across the reviews.
# Visualizing sentiment distribution
library(ggplot2)
ggplot(sentiment_analysis, aes(x = review, fill = sentiment)) +
geom_bar(stat = "count") +
labs(title = "Sentiment Distribution in Movie Reviews", x = "Review", y = "Count") +
theme_minimal()
This plot will display how many words in each review are classified as positive or negative, providing a clear view of the overall sentiment in the text.
5. Sentiment Analysis with Different Lexicons
The tidytext
package offers several sentiment lexicons that can be used to analyze text. Some of the popular ones include:
bing
: Classifies words as positive or negative.afinn
: Provides a numeric sentiment score, where negative scores represent negative sentiment and positive scores indicate positive sentiment.nrc
: Assigns emotions (e.g., joy, sadness, anger, etc.) to words.
Here’s how to perform sentiment analysis using the afinn
lexicon:
# Get the afinn lexicon
sentiment_lexicon_afinn <- get_sentiments("afinn")
# Join the reviews with the afinn lexicon
sentiment_analysis_afinn <- movie_reviews_tidy %>%
inner_join(sentiment_lexicon_afinn, by = "word") %>%
summarise(sentiment_score = sum(value))
# View the sentiment score for the reviews
sentiment_analysis_afinn
In this example, the afinn
lexicon provides a sentiment score for each word. The final sentiment score for each review is the sum of the individual word scores, which gives an overall sentiment value for the text.
6. Conclusion
Sentiment analysis with the tidytext
package in R is a powerful method for analyzing text data. By leveraging different sentiment lexicons and visualizing the results, you can gain valuable insights into the emotional tone of textual data, which is useful in various applications such as customer feedback analysis, social media monitoring, and market research.
Predictive Analytics on Time Series Data
Predictive analytics on time series data involves using historical data to make forecasts and predictions about future values. Time series analysis is crucial in various fields such as finance, economics, weather forecasting, and sales. By applying statistical models and machine learning techniques, we can uncover patterns, trends, and seasonality in data, and use them to predict future behavior.
1. Introduction to Time Series Data
Time series data refers to data points collected or recorded at specific time intervals. It can include data such as stock prices, temperature readings, or sales figures. The main components of time series data include:
- Trend: A long-term movement in the data (e.g., increasing sales over time).
- Seasonality: Regular fluctuations that occur at specific intervals (e.g., monthly or yearly patterns).
- Cyclic Patterns: Fluctuations that are not fixed in time but occur over irregular periods (e.g., economic cycles).
- Noise: Random variations that do not follow any trend or pattern.
2. Preparing Time Series Data
Before performing predictive analytics, it is essential to prepare time series data. You need to ensure that the data is in a format suitable for modeling and analysis. R provides several functions for working with time series data, including the ts()
function for creating time series objects.
Example: Creating a Time Series Object
# Example of creating a time series object from monthly data
sales_data <- c(200, 220, 250, 280, 300, 350, 400, 450, 500, 550, 600, 650)
sales_ts <- ts(sales_data, start = c(2021, 1), frequency = 12)
# View the time series object
sales_ts
In this example, we create a time series object sales_ts
from monthly sales data starting in January 2021 with a frequency of 12, indicating monthly data.
3. Time Series Decomposition
Before applying predictive models, it is helpful to decompose the time series to understand its underlying components (trend, seasonality, and noise). R provides the decompose()
function for classical decomposition and the stl()
function for seasonal decomposition of time series.
Example: Decomposing the Time Series
# Decomposing the time series
decomposed_sales <- decompose(sales_ts)
# Plot the decomposition
plot(decomposed_sales)
The decomposition will split the time series into its trend, seasonal, and residual components, allowing you to better understand the data structure.
4. Forecasting Time Series Data
Once the data is prepared and decomposed, you can use statistical and machine learning models to forecast future values. In R, the forecast
package provides functions for time series forecasting, including models such as ARIMA (AutoRegressive Integrated Moving Average), Exponential Smoothing, and more.
Example: Forecasting with ARIMA Model
# Install and load the forecast package
install.packages("forecast")
library(forecast)
# Fit an ARIMA model
arima_model <- auto.arima(sales_ts)
# Forecast the next 12 months
sales_forecast <- forecast(arima_model, h = 12)
# Plot the forecast
plot(sales_forecast)
In this example, we use the auto.arima()
function to fit an ARIMA model to the sales time series data and forecast the next 12 months. The forecast()
function generates the predicted values, and we visualize the forecast using a plot.
5. Evaluating Model Performance
To assess the quality of your predictive model, you should evaluate its performance using various metrics. Common evaluation metrics for time series forecasting include Mean Absolute Error (MAE), Mean Squared Error (MSE), and Root Mean Squared Error (RMSE).
Example: Evaluating Model Accuracy
# Actual vs Predicted values (using first 12 months as test data)
actual_values <- tail(sales_ts, 12)
predicted_values <- sales_forecast$mean
# Calculate accuracy metrics
mae <- mean(abs(actual_values - predicted_values))
mse <- mean((actual_values - predicted_values)^2)
rmse <- sqrt(mse)
# Display the accuracy metrics
mae
mse
rmse
The evaluation metrics will help you understand the accuracy of the forecast and guide you in improving the model if necessary.
6. Advanced Forecasting Techniques
In addition to ARIMA models, there are other advanced techniques for forecasting time series data, such as:
- Exponential Smoothing State Space Model (ETS): Suitable for data with trend and seasonality.
- Prophet: A robust forecasting tool developed by Facebook for time series data with seasonal effects.
- Long Short-Term Memory (LSTM): A type of Recurrent Neural Network (RNN) used for time series forecasting with deep learning methods.
Each of these techniques has its own advantages and is suitable for different types of time series data.
7. Conclusion
Predictive analytics on time series data allows you to make informed decisions by forecasting future trends and behaviors. With the right techniques and tools in R, such as ARIMA, Exponential Smoothing, and machine learning models, you can effectively predict future values, identify patterns, and improve decision-making across various applications like sales, finance, and more.
Social Media Data Analysis
Social media data analysis involves examining data from platforms like Twitter, Facebook, Instagram, LinkedIn, and others to gain insights about user behavior, trends, sentiment, engagement, and more. By analyzing social media data, businesses and researchers can better understand their audience, improve marketing strategies, and make informed decisions. In this section, we will explore how to collect, analyze, and visualize social media data using R.
1. Collecting Social Media Data
Collecting social media data requires using APIs provided by the platforms or third-party tools. Popular methods for collecting data include:
- Twitter API: Allows you to collect tweets, hashtags, and user information.
- Facebook Graph API: Provides access to public posts, likes, comments, and more.
- Instagram API: Used for collecting data from Instagram posts, likes, and comments.
- LinkedIn API: Provides data about posts, connections, and other professional interactions.
R provides packages such as rtweet
for collecting Twitter data, Rfacebook
for Facebook data, and httr
for interacting with APIs in general.
Example: Collecting Tweets with rtweet
# Install and load the rtweet package
install.packages("rtweet")
library(rtweet)
# Collect tweets containing a specific hashtag
tweets <- search_tweets("#DataScience", n = 1000, include_rts = FALSE)
# View the first few tweets
head(tweets)
In this example, we use the search_tweets()
function from the rtweet
package to collect tweets containing the hashtag "#DataScience". The parameter n = 1000
specifies that we want to collect 1000 tweets.
2. Data Cleaning and Preprocessing
Once you collect social media data, it is important to clean and preprocess it before analysis. This may involve removing stopwords, handling missing values, and formatting the data for further analysis. Common preprocessing steps include:
- Removing stopwords: Words like "the", "is", and "and" that don't contribute to the analysis.
- Tokenizing: Breaking text into smaller units (e.g., words or sentences).
- Removing special characters: Stripping out URLs, hashtags, mentions, or punctuation marks.
Example: Text Preprocessing in R
# Install and load the tidyverse and tm packages for text preprocessing
install.packages(c("tidyverse", "tm"))
library(tidyverse)
library(tm)
# Clean the text data (remove punctuation, stopwords, etc.)
clean_tweets <- tweets %>%
mutate(text = tolower(text)) %>%
mutate(text = removePunctuation(text)) %>%
mutate(text = removeWords(text, stopwords("en"))) %>%
mutate(text = stripWhitespace(text))
# View the cleaned tweets
head(clean_tweets$text)
In this example, we preprocess tweet text by converting it to lowercase, removing punctuation, removing stopwords, and stripping excess whitespace using functions from the tm
package.
3. Sentiment Analysis
Sentiment analysis is a common application in social media data analysis. It involves determining whether the sentiment of a piece of text is positive, negative, or neutral. In R, you can perform sentiment analysis using the tidytext
package, which provides tools for text mining and sentiment analysis.
Example: Performing Sentiment Analysis with tidytext
# Install and load the tidytext package
install.packages("tidytext")
library(tidytext)
# Perform sentiment analysis using the Bing lexicon
sentiment <- clean_tweets %>%
unnest_tokens(word, text) %>%
inner_join(get_sentiments("bing")) %>%
count(sentiment) %>%
spread(sentiment, n, fill = 0)
# View the sentiment counts
sentiment
In this example, we use the get_sentiments("bing")
function from the tidytext
package to analyze the sentiment of the words in the tweets. The output shows the count of positive and negative words in the tweets.
4. Visualizing Social Media Data
Visualization is a powerful tool for understanding social media data. You can use R packages such as ggplot2
and wordcloud
to create informative visualizations.
Example: Creating a Word Cloud
# Install and load the wordcloud package
install.packages("wordcloud")
library(wordcloud)
# Create a word cloud of the most common words in the tweets
wordcloud(clean_tweets$text, max.words = 100, random.order = FALSE, colors = "darkblue")
In this example, we use the wordcloud
function to create a visualization of the most frequent words in the tweets. The result is a word cloud where the size of each word represents its frequency in the dataset.
5. Analyzing Engagement Metrics
Besides sentiment analysis, social media data analysis often includes analyzing engagement metrics, such as likes, shares, comments, and retweets. These metrics can provide valuable insights into the popularity and reach of a particular post or hashtag. R can be used to calculate and visualize these metrics to gauge the success of social media campaigns.
Example: Analyzing Engagement on Twitter
# Calculate the average number of retweets and favorites per tweet
engagement_metrics <- tweets %>%
summarise(avg_retweets = mean(retweet_count, na.rm = TRUE),
avg_favorites = mean(favorite_count, na.rm = TRUE))
# View the engagement metrics
engagement_metrics
In this example, we calculate the average number of retweets and favorites for the collected tweets. This can help assess how well the content is engaging the audience.
6. Advanced Social Media Analysis
Advanced social media analysis techniques involve network analysis, trend analysis, and geographic analysis. For example, you can analyze how users interact with each other (network analysis), track the popularity of certain topics over time (trend analysis), or analyze the geographic distribution of posts (geospatial analysis).
R provides additional packages like igraph
for network analysis and sf
for spatial data analysis, allowing for more in-depth social media insights.
7. Conclusion
Social media data analysis in R allows businesses, researchers, and analysts to gain valuable insights into user behavior, sentiment, engagement, and trends. By leveraging various R packages and techniques such as sentiment analysis, text mining, and data visualization, you can unlock the potential of social media data and make informed decisions that drive success.
Climate Data Visualization
Climate data visualization is an essential tool for understanding complex climate patterns, trends, and anomalies. By leveraging visual representations, we can effectively communicate the impacts of climate change, the variability of weather conditions, and other important climate-related data. In this section, we will explore how to visualize climate data using R, from basic plots to advanced mapping techniques.
1. Understanding Climate Data
Climate data typically includes information on temperature, precipitation, humidity, wind speed, and other weather-related parameters. This data can span from daily to annual records and is often provided by meteorological agencies, research institutions, and climate models. Common sources of climate data include:
- NASA's Earth Observatory: Provides global climate and satellite data.
- NOAA (National Oceanic and Atmospheric Administration): Offers climate data on temperature, precipitation, and ocean conditions.
- World Bank Climate Data: Offers climate data for development and research purposes.
R provides several packages for handling and visualizing climate data, including ggplot2
, leaflet
, and sf
for spatial data analysis.
2. Loading and Preparing Climate Data
Before visualizing climate data, you need to load and clean it. Climate data often comes in formats such as CSV, Excel, or NetCDF files. Common preprocessing steps include handling missing values, converting units, and filtering data for specific time periods or locations.
Example: Loading Climate Data from CSV
# Install and load necessary packages
install.packages(c("tidyverse"))
library(tidyverse)
# Load climate data from a CSV file
climate_data <- read.csv("climate_data.csv")
# View the first few rows
head(climate_data)
In this example, we load climate data from a CSV file using the read.csv()
function. You can use head()
to preview the first few rows of the dataset.
3. Basic Climate Data Visualization
Once the data is loaded and cleaned, the next step is to visualize the climate trends. You can use basic plots like line charts, histograms, and boxplots to show temperature trends, precipitation patterns, or variability over time.
Example: Visualizing Temperature Trends Over Time
# Install and load ggplot2 for plotting
install.packages("ggplot2")
library(ggplot2)
# Create a line plot to visualize temperature trends over time
ggplot(climate_data, aes(x = Year, y = Temperature)) +
geom_line(color = "blue") +
labs(title = "Annual Temperature Trends", x = "Year", y = "Temperature (°C)") +
theme_minimal()
In this example, we use ggplot2
to create a line plot showing the temperature trends over time. The geom_line()
function is used to plot the data points connected by a line.
4. Visualizing Climate Data Distribution
Understanding the distribution of climate data is essential for identifying patterns and anomalies. Histograms and boxplots can help visualize the distribution of variables like temperature or precipitation.
Example: Visualizing Temperature Distribution with a Histogram
# Create a histogram to visualize the distribution of temperature
ggplot(climate_data, aes(x = Temperature)) +
geom_histogram(binwidth = 1, fill = "blue", color = "black", alpha = 0.7) +
labs(title = "Temperature Distribution", x = "Temperature (°C)", y = "Frequency") +
theme_minimal()
In this example, we create a histogram using the geom_histogram()
function to visualize the distribution of temperatures in the dataset. The binwidth
parameter controls the width of each bin in the histogram.
5. Mapping Climate Data
Geospatial analysis is an important aspect of climate data visualization. By mapping climate data, we can visualize patterns and trends across different geographic locations. R packages like leaflet
and sf
allow for interactive maps and spatial analysis.
Example: Mapping Temperature Across Regions with Leaflet
# Install and load the leaflet package
install.packages("leaflet")
library(leaflet)
# Create a simple map to display temperature data by region
leaflet(climate_data) %>%
addTiles() %>%
addCircleMarkers(lng = ~Longitude, lat = ~Latitude, color = ~Temperature, radius = 5, popup = ~paste("Temp: ", Temperature, "°C"))
In this example, we use the leaflet
package to create an interactive map that shows temperature data for different geographic locations. The addCircleMarkers()
function is used to display temperature data as colored circle markers on the map.
6. Climate Change Visualization
Visualizing climate change is a powerful way to communicate the impacts of global warming. You can visualize temperature anomalies, sea-level rise, or the frequency of extreme weather events over time. Animated plots and interactive visualizations can be especially effective for illustrating climate change trends.
Example: Visualizing Temperature Anomalies
# Create an animated plot to visualize temperature anomalies over time
install.packages("gganimate")
library(gganimate)
ggplot(climate_data, aes(x = Year, y = Temperature)) +
geom_line(color = "red") +
labs(title = "Temperature Anomalies Over Time", x = "Year", y = "Temperature (°C)") +
transition_time(Year) +
ease_aes('linear')
In this example, we use the gganimate
package to create an animated plot showing temperature anomalies over time. The animation helps illustrate the gradual increase in temperature due to climate change.
7. Advanced Climate Data Visualization
Advanced techniques for visualizing climate data include heatmaps, contour plots, and 3D surface plots. These techniques can help visualize complex climate models, such as temperature variations across different altitudes or latitudes.
Example: Visualizing Temperature Variations with a Heatmap
# Install and load the ggplot2 package
install.packages("ggplot2")
library(ggplot2)
# Create a heatmap to visualize temperature variations
ggplot(climate_data, aes(x = Longitude, y = Latitude, fill = Temperature)) +
geom_tile() +
scale_fill_viridis_c() +
labs(title = "Temperature Variations by Region", x = "Longitude", y = "Latitude") +
theme_minimal()
In this example, we create a heatmap using geom_tile()
to visualize the temperature variations across different regions. The scale_fill_viridis_c()
function is used to apply a color scale to the temperature values.
8. Conclusion
Climate data visualization is a powerful tool for understanding and communicating the impacts of climate change. By leveraging R's powerful visualization packages such as ggplot2
, leaflet
, and gganimate
, you can create informative and engaging visualizations that help make sense of complex climate data and highlight important trends and anomalies.
Writing Clean and Efficient R Code
Writing clean and efficient R code is essential for maintaining readability, improving performance, and ensuring that your code is reusable and scalable. Whether you're working on small scripts or large data analysis projects, following best practices can save time and effort in the long run. In this section, we will explore strategies for writing clean and efficient R code, from code organization to optimizing performance.
1. Use Descriptive Variable and Function Names
One of the simplest and most effective ways to write clean code is by choosing meaningful and descriptive names for variables and functions. Avoid using short and ambiguous names like x
or temp
, and instead opt for names that clearly describe the purpose of the variable or function.
Example: Descriptive Variable Names
# Bad practice
x <- 10
temp <- 20
# Good practice
temperature_in_celsius <- 10
temperature_in_fahrenheit <- 20
In this example, the variable names are more descriptive, making it easier to understand what each variable represents. This is especially helpful when working on larger projects or collaborating with others.
2. Keep Code DRY (Don't Repeat Yourself)
Repetition of code should be avoided. Instead of duplicating code, you can create functions to handle repetitive tasks. This improves maintainability and readability, as you only need to make changes in one place.
Example: Avoiding Repetitive Code
# Bad practice
area_square <- 4 * 4
area_rectangle <- 5 * 3
# Good practice
calculate_area <- function(length, width) {
return(length * width)
}
area_square <- calculate_area(4, 4)
area_rectangle <- calculate_area(5, 3)
In this example, we've created a function calculate_area()
that can be reused for different shapes, reducing code repetition and making it more modular.
3. Comment Your Code
Comments are essential for explaining the purpose of your code, especially when the logic is complex or non-intuitive. Write comments that describe what the code is doing and why certain decisions were made, rather than just explaining what the code is doing.
Example: Writing Useful Comments
# Calculate the area of a rectangle (length * width)
calculate_area <- function(length, width) {
return(length * width)
}
In this example, the comment explains the purpose of the function, making it easier for others (or yourself) to understand the code later.
4. Format Your Code Properly
Consistent formatting makes your code more readable and easier to follow. Use indentation, spaces, and line breaks to separate different logical parts of your code. Many R style guides recommend using 2 or 4 spaces for indentation, and a consistent style should be followed throughout your code.
Example: Proper Code Formatting
# Bad practice
x=2;y=3;sum=x+y;print(sum)
# Good practice
x <- 2
y <- 3
sum <- x + y
print(sum)
In this example, the properly formatted code is easier to read and follow. Each statement is on a new line, and the use of spaces around operators improves clarity.
5. Optimize for Performance
Performance optimization is particularly important when working with large datasets or running time-consuming tasks. There are several ways to optimize performance in R:
- Use vectorized operations instead of loops whenever possible. R is optimized for vectorized operations and will execute them faster than loops.
- Use efficient data structures like
data.table
instead ofdata.frame
for large datasets. - Profile your code using the
profvis
package to identify performance bottlenecks.
Example: Vectorized Operation vs Loop
# Bad practice (using a loop)
result <- numeric(1000)
for (i in 1:1000) {
result[i] <- i * 2
}
# Good practice (using a vectorized operation)
result <- 1:1000 * 2
In this example, the vectorized operation is faster and more concise than the loop. Avoiding loops when possible will make your code more efficient and easier to read.
6. Avoid Hard-Coding Values
Hard-coding values in your code makes it less flexible and harder to maintain. Instead of directly using numbers or strings in your code, define them as variables or constants. This makes it easier to update the values later and improves the reusability of your code.
Example: Avoiding Hard-Coded Values
# Bad practice
area_square <- 4 * 4
area_rectangle <- 5 * 3
# Good practice
length_square <- 4
width_square <- 4
area_square <- length_square * width_square
length_rectangle <- 5
width_rectangle <- 3
area_rectangle <- length_rectangle * width_rectangle
In this example, the hard-coded values have been replaced by variables that are defined at the beginning. This makes the code more flexible and easier to modify later on.
7. Test Your Code
Testing is an important aspect of writing reliable code. Always test your functions and scripts to ensure that they work as expected. Use unit testing frameworks like testthat
to automate the testing process and catch potential errors early.
Example: Writing Unit Tests with testthat
# Install and load the testthat package
install.packages("testthat")
library(testthat)
# Define a simple function
add <- function(a, b) {
return(a + b)
}
# Write a unit test
test_that("addition works correctly", {
expect_equal(add(2, 3), 5)
expect_equal(add(-1, 1), 0)
})
In this example, we use the testthat
package to write unit tests that ensure the add()
function works correctly. Unit tests help ensure that your code behaves as expected and can help identify bugs early in the development process.
8. Conclusion
Writing clean and efficient R code is essential for maintaining high-quality, reliable, and scalable projects. By following best practices like using descriptive names, avoiding repetition, formatting your code consistently, and optimizing for performance, you can ensure that your code is easy to maintain and understand. Additionally, testing and profiling your code will help you identify potential issues early and improve the overall quality of your work.
Organizing R Projects
Organizing an R project efficiently is critical for maintainability, scalability, and collaboration. Good project structure helps keep code modular, readable, and easier to debug. In this section, we will discuss best practices for organizing R projects, from directory structure to version control, and managing dependencies.
1. Use a Standard Directory Structure
Having a clear and consistent directory structure is essential for organizing your R project. A well-structured project makes it easier to locate files, keep code modular, and maintain a clean workflow. A typical R project structure might look like this:
project/
├── R/ # R scripts (functions, analysis)
├── data/ # Raw and processed data files
├── output/ # Plots, tables, and other output files
├── docs/ # Documentation (e.g., README, project report)
├── tests/ # Unit tests or test scripts
├── scripts/ # Analysis scripts
└── README.md # Project overview and instructions
This structure separates scripts, data, outputs, and documentation, making it easier to navigate through the project.
2. Use RStudio Projects
RStudio Projects are a great way to manage R projects. They allow you to set up a working directory that includes all project-specific files and settings. When you create an RStudio Project, the IDE automatically sets the working directory to the project folder, which helps avoid path issues.
To create an RStudio Project, simply go to File > New Project
and follow the prompts. This will create a .Rproj
file in your project directory, which can be used to open the project later.
3. Keep Code Modular
In larger projects, it's important to break your code into smaller, reusable pieces. This can be achieved by creating functions that perform specific tasks and organizing them into scripts or files based on functionality. Avoid writing long scripts with hundreds of lines of code.
For example, you could have separate files for:
- Data loading and cleaning functions
- Data visualization functions
- Modeling functions
- Utility functions (e.g., helpers for data manipulation)
4. Use Version Control with Git
Version control is a vital tool for tracking changes to your code, collaborating with others, and rolling back to previous versions when needed. Git is the most commonly used version control system and integrates well with RStudio.
To set up Git in your project, follow these steps:
- Initialize a Git repository in your project folder using the command
git init
in the terminal. - Create a
.gitignore
file to exclude files that should not be tracked (e.g., temporary files, large datasets, etc.). - Commit changes regularly with
git commit
. - Push changes to a remote repository (e.g., GitHub, GitLab) to collaborate with others.
Using Git will help you maintain a history of changes, track bugs, and collaborate with team members effectively.
5. Manage Dependencies with renv
Managing R package dependencies is essential for project reproducibility. The renv
package helps you manage the libraries your project depends on. It creates a virtual environment for your project, ensuring that the exact versions of packages are used, regardless of what packages are installed globally on your system.
To use renv
, follow these steps:
- Install and initialize the project environment:
renv::init()
- Install packages as usual with
install.packages()
. - Once packages are installed, use
renv::snapshot()
to record the project's state (e.g., versions of packages). - To recreate the environment, use
renv::restore()
on another machine or after cloning the repository.
With renv
, you can ensure that other collaborators or users can install the exact same packages and versions you used, making your analysis reproducible.
6. Document Your Code
Proper documentation is essential for maintaining and sharing your R project. Documenting your code, functions, and analysis will help others (and future you) understand the logic behind your work. Some best practices for documentation include:
- Write clear comments explaining the purpose of functions and key sections of your code.
- Use
roxygen2
to add documentation to your functions, making it easier to generate help files. - Create a README file to provide an overview of the project, including instructions on how to run the code and details about the data.
7. Use Consistent Naming Conventions
Consistent naming conventions help keep code readable and organized. Use meaningful names for variables, functions, and files. There are several popular naming conventions in R, such as:
- Snake case:
my_function_name()
- Camel case:
myFunctionName()
- Dot notation:
my.function.name()
Choose a convention and stick with it throughout the project to ensure consistency.
8. Keep Outputs Separate from Code
It's important to separate your outputs (e.g., plots, tables, reports) from your code. This helps keep the project structure clean and prevents clutter. Store outputs in separate folders, such as output/
, and avoid mixing data and code with output files.
9. Use R Markdown for Reproducible Reports
R Markdown is a powerful tool for creating dynamic and reproducible reports. You can combine R code, text, and plots in a single document, which can be rendered to HTML, PDF, or Word format. This is useful for generating reports that include code, results, and explanations in one place.
Use R Markdown files to document your analysis and share the results in an accessible format. This ensures your work is reproducible and transparent.
10. Conclusion
Organizing your R project effectively is key to maintaining a clean, reproducible, and scalable workflow. By following best practices such as using a standard directory structure, managing dependencies with renv
, version control with Git, and documenting your work, you'll ensure that your project is maintainable and easy to collaborate on. With these practices, you'll be able to manage your R projects more efficiently and build high-quality, reproducible analyses.
Version Control with Git and RStudio
Version control is an essential tool for managing changes in code, collaborating with others, and tracking the history of a project. Git is the most popular version control system, and RStudio integrates seamlessly with Git to streamline the version control process. In this section, we will learn how to use Git with RStudio for efficient version control in R projects.
1. Introduction to Git
Git is a distributed version control system that allows developers to track changes in code, collaborate with multiple people, and manage different versions of a project. It enables you to:
- Track changes to files over time.
- Roll back to previous versions of your code.
- Work collaboratively with others without overwriting each other's changes.
- Merge contributions from multiple people into a single project.
Git creates a local repository in your project directory that tracks all changes. You can also push changes to a remote repository (e.g., GitHub, GitLab) for collaboration and backup.
2. Setting Up Git in RStudio
To use Git in RStudio, follow these steps:
- Install Git: Before using Git with RStudio, you need to install Git on your system. Download and install Git from https://git-scm.com/.
- Configure Git: After installation, open a terminal or Git Bash and configure your user name and email address, which will be associated with your commits:
- Enable Git in RStudio: Open RStudio, go to
Tools > Global Options > Git/SVN
, and make sure Git is enabled. You will also need to specify the path to the Git executable (RStudio will often detect it automatically).
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Once Git is set up, you can create and manage repositories directly from RStudio.
3. Creating a Git Repository
To create a new Git repository for your R project, follow these steps:
- Create a New Project: In RStudio, go to
File > New Project
. Choose "New Directory" and select "New Project". - Initialize Git: In the "Create Project" dialog, check the box labeled "Create a git repository". This will initialize a new Git repository in your project folder.
If you already have a project, you can initialize Git by going to Tools > Project Options > Git/SVN
and enabling Git for the project. You can also initialize a Git repository manually by running git init
in the terminal.
4. Basic Git Commands in RStudio
Once Git is initialized, RStudio provides an integrated Git interface to perform common Git operations. Here's a quick overview of the basic commands you'll use:
- Commit: After making changes to your project, you can commit them to Git by clicking on the "Git" tab in RStudio. Select the changes to commit, write a commit message, and click "Commit".
- Push: To upload your changes to a remote repository (e.g., on GitHub), click "Push" in the Git tab. You’ll need to set up a remote repository first (more on that later).
- Pull: To download changes from a remote repository, click "Pull". This will synchronize your local repository with the remote repository.
- View Changes: The Git tab shows the files that have been modified. You can see which files have been added, modified, or deleted.
5. Working with Remote Repositories (e.g., GitHub)
To collaborate on a project, you’ll need to push and pull changes from a remote repository. Here’s how to set up a remote repository on GitHub:
- Create a GitHub Account: If you don’t already have one, create an account on GitHub.
- Create a Repository: After logging in, click on "New" to create a new repository. Give it a name and description, and click "Create repository".
- Link Your Local Repository to GitHub: In RStudio, open the terminal and run the following command to add the remote GitHub repository:
- Push Changes to GitHub: Once the remote is set up, you can push changes to GitHub by clicking "Push" in the Git tab.
git remote add origin https://github.com/username/repository.git
This will synchronize your local Git repository with GitHub, making it easy to share code and collaborate with others.
6. Branching and Merging
Branching allows you to work on new features or bug fixes without affecting the main codebase. You can create a branch, make changes, and later merge those changes into the main branch. Here's how to use branches in RStudio:
- Create a Branch: In the Git tab, click on "Branch" and select "New Branch". Give your branch a name.
- Switch Branches: You can switch between branches by clicking on "Branch" and selecting an existing branch.
- Merge Branches: Once you’ve finished working on your branch, you can merge it into the main branch (usually called "master" or "main") by clicking "Merge" in the Git tab.
Using branches helps you manage different versions of the code and avoid conflicts when working with collaborators.
7. Resolving Conflicts
Sometimes, when two people edit the same part of a file, Git may not be able to automatically merge the changes. This is called a merge conflict. To resolve a merge conflict:
- Git will mark the conflicting file with special conflict markers.
- Open the file and manually resolve the conflict by choosing which changes to keep or combining the changes.
- After resolving the conflict, stage the file and commit the changes as usual.
RStudio will show you the conflicting file, and you can resolve the conflict directly in the editor.
8. Conclusion
Git is a powerful tool for managing code and collaborating on projects. By using Git within RStudio, you can keep track of changes, work with others, and ensure that your analysis is reproducible. Whether you're working on a solo project or collaborating with a team, version control with Git is an essential skill that will improve your R workflow and project management.
Comments in R
In R, comments are used to explain and annotate code. They help make the code more understandable to others (or yourself) and are ignored by the R interpreter during execution. Comments are essential for documenting your code, especially when working on complex projects or collaborating with others.
Single-Line Comments
In R, a comment begins with the
#
symbol. Everything following the#
on that line is treated as a comment, and it will not be executed. Single-line comments are commonly used to explain parts of the code or provide additional context.Example of a single-line comment:
Multi-Line Comments
R does not have a specific syntax for multi-line comments like some other languages. However, you can create multi-line comments by placing a
#
at the beginning of each line. It is common to use multi-line comments to describe a block of code or provide detailed explanations.Example of multi-line comments:
Commenting Out Code
Another common use of comments is to temporarily disable parts of the code. This is helpful for debugging or testing different sections of a script. You can comment out a line or a block of code by adding a
#
in front of it.Example of commenting out code:
Best Practices for Commenting
Conclusion
Comments are a critical aspect of writing clean, maintainable code in R. By using comments effectively, you can make your code more readable and easier to understand for others (and yourself) in the future.