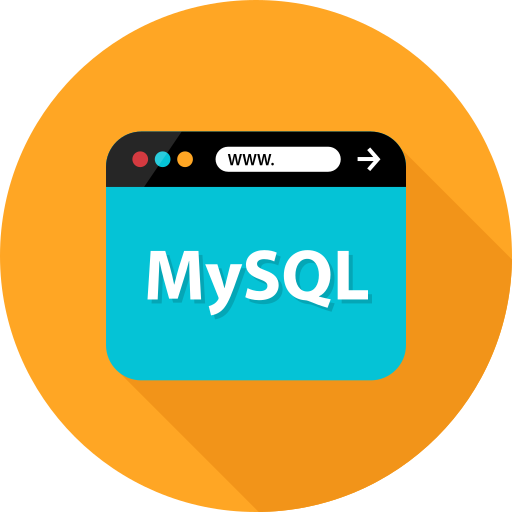
What is MySQL?
MySQL is an open-source relational database management system (RDBMS) that uses Structured Query Language (SQL) to store, retrieve, manage, and manipulate data. It is widely used for web applications, data warehousing, and embedded database systems due to its reliability, ease of use, and performance.
History of MySQL
MySQL was originally developed by a Swedish company called MySQL AB in 1995. It was later acquired by Sun Microsystems in 2008, which was subsequently acquired by Oracle Corporation in 2010. MySQL has grown to become one of the most popular database systems used worldwide.
MySQL Features
Below are the key features that make MySQL a popular choice for developers:
Feature | Description |
---|---|
Open Source | MySQL is free to use and open-source, providing flexibility and community-driven development. |
High Performance | MySQL is optimized for high-speed query processing, making it suitable for large-scale applications. |
Cross-Platform | MySQL can run on a variety of operating systems, including Windows, Linux, and macOS. |
Scalability | MySQL supports large databases and can handle millions of records efficiently. |
Replication | MySQL provides replication features for high availability and disaster recovery. |
Setting Up MySQL
To use MySQL, you need to install it on your system. Follow these steps to install MySQL:
- Download the MySQL installer from the official website.
- Run the installer and follow the setup instructions.
- Configure the server by setting up a root password and other preferences.
- Verify the installation by running the following command in your terminal:
mysql --version
Code Example: Connecting to MySQL
Here’s a simple example of connecting to a MySQL database using Python:
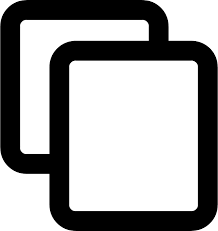
import mysql.connector
# Establishing the connection
connection = mysql.connector.connect(
host="localhost",
user="your_username",
password="your_password",
database="your_database"
)
# Checking connection
if connection.is_connected():
print("Connected to MySQL database")
# Closing the connection
connection.close()
Diagram: MySQL Database Architecture
The following diagram illustrates the architecture of a MySQL database:

In this diagram, you can see the main components of MySQL, including the SQL interface, query optimizer, storage engine, and more.
Relational Database Management Systems (RDBMS) Overview
A Relational Database Management System (RDBMS) is a type of database management system that stores data in a structured format using rows and columns. This tabular structure allows for easy organization, retrieval, and manipulation of data, making RDBMS one of the most widely used database systems in the world.
Key Features of RDBMS
RDBMS offers several features that make it a preferred choice for managing data in various applications:
Feature | Description |
---|---|
Structured Data Storage | Data is stored in tables with predefined columns and types, ensuring consistency and organization. |
Data Relationships | RDBMS allows for defining relationships between tables (e.g., one-to-one, one-to-many, many-to-many). |
SQL Support | RDBMS uses Structured Query Language (SQL) for querying and manipulating data. |
Transaction Management | RDBMS supports ACID (Atomicity, Consistency, Isolation, Durability) properties to ensure reliable transactions. |
Scalability | RDBMS can handle large datasets efficiently and supports scaling as data grows. |
Advantages of RDBMS
RDBMS provides numerous benefits for managing data:
- Ensures data integrity and consistency through constraints such as primary keys and foreign keys.
- Facilitates complex queries and data analysis with SQL.
- Supports concurrent access by multiple users with robust locking and transaction mechanisms.
- Offers data security with user access controls and authentication.
- Provides backup and recovery features to safeguard data.
Popular RDBMS Software
Some of the most commonly used RDBMS software include:
- MySQL
- PostgreSQL
- Oracle Database
- Microsoft SQL Server
- SQLite
Code Example: Simple SQL Query
Below is an example of a simple SQL query to retrieve data from an RDBMS table:
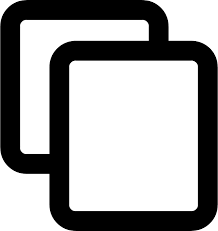
-- Retrieve all rows from the 'employees' table
SELECT * FROM employees;
-- Retrieve specific columns from the 'employees' table
SELECT first_name, last_name, department FROM employees WHERE department = 'Sales';
Diagram: RDBMS Table Structure
The following diagram shows an example of how data is structured in an RDBMS table:

In the diagram, you can see that data is organized into rows (records) and columns (fields), forming a table structure.
MySQL Architecture and Components
MySQL is a popular Relational Database Management System (RDBMS) that follows a client-server architecture. Its architecture is designed to ensure high performance, scalability, and reliability while managing complex data efficiently.
Overview of MySQL Architecture
The MySQL architecture consists of three main layers:
- Client Layer: Handles user interactions, including query execution and results display.
- Server Layer: Manages query parsing, optimization, and execution.
- Storage Layer: Handles physical storage and retrieval of data.
Key Components of MySQL
The following are the primary components of MySQL architecture:
Component | Description |
---|---|
Connection Manager | Manages client connections, handles authentication, and maintains session-level settings. |
Query Parser | Analyzes and validates SQL queries to ensure syntax correctness. |
Query Optimizer | Determines the most efficient execution plan for SQL queries. |
Storage Engine | Handles data storage and retrieval. MySQL supports multiple storage engines like InnoDB and MyISAM. |
Buffer Pool | Caches frequently accessed data and query results to enhance performance. |
Transaction Manager | Ensures ACID compliance by managing transactions and locks. |
MySQL Storage Engines
MySQL supports a variety of storage engines to cater to different use cases:
- InnoDB: The default storage engine, supporting transactions, foreign keys, and row-level locking.
- MyISAM: Suitable for read-intensive applications, offering fast read speeds but lacking transaction support.
- Memory: Stores data in memory for ultra-fast access but is not persistent.
- CSV: Stores data in plain-text CSV files for portability.
Code Example: Switching Storage Engines
Here is an example of how to specify a storage engine for a table in MySQL:
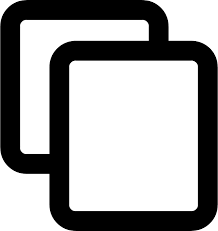
-- Create a table using the InnoDB storage engine
CREATE TABLE employees (
id INT AUTO_INCREMENT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50)
) ENGINE=InnoDB;
-- Alter a table to use the MyISAM storage engine
ALTER TABLE employees ENGINE=MyISAM;
Diagram: MySQL Architecture
The following diagram illustrates the MySQL architecture and its key components:

The diagram depicts how the client, server, and storage layers interact to process queries and manage data efficiently.
Why Choose MySQL Over Other Databases?
MySQL is one of the most widely used relational database management systems in the world. It offers a compelling combination of performance, reliability, and ease of use, making it a popular choice for developers and organizations. Here are the key reasons to choose MySQL over other databases:
1. Open Source and Cost-Effective
MySQL is open-source, meaning it is free to use and has no licensing costs, making it an ideal choice for startups and small to medium-sized businesses. It also has enterprise editions for advanced features and support.
2. High Performance
MySQL delivers fast query processing and supports high-traffic applications. Its optimized storage engines like InnoDB ensure efficient handling of large datasets and complex queries.
3. Scalability
MySQL is highly scalable and can handle applications ranging from small-scale websites to enterprise-level systems with millions of queries per second.
4. Cross-Platform Compatibility
MySQL is platform-independent and runs seamlessly on a variety of operating systems, including Windows, Linux, macOS, and Unix.
5. Wide Adoption and Community Support
MySQL has a large and active user community. This ensures access to extensive documentation, tutorials, forums, and third-party tools, making it easier to find solutions to common problems.
6. Rich Ecosystem of Tools
MySQL offers a variety of tools for database management, development, and optimization, such as MySQL Workbench, MySQL Router, and MySQL Shell. These tools simplify database administration and integration.
7. Security and Reliability
MySQL provides robust security features, including user authentication, encryption, SSL support, and granular privilege control. It is also known for its reliability and stability in production environments.
8. Flexible Licensing
MySQL offers dual licensing models—GPL for open-source users and commercial licenses for enterprises needing advanced features and official support.
9. Integration with Programming Languages
MySQL integrates seamlessly with popular programming languages like PHP, Python, Java, and Node.js, making it easy to use in web and software development projects.
10. Support for Modern Features
MySQL includes support for modern database features like JSON data types, spatial data, full-text search, and replication, ensuring it remains relevant for contemporary applications.
Comparison Table: MySQL vs. Other Databases
Below is a comparison of MySQL with other popular database systems:
Feature | MySQL | PostgreSQL | SQLite | MongoDB |
---|---|---|---|---|
Cost | Free (Open Source) | Free (Open Source) | Free (Open Source) | Free (Open Source) |
Scalability | High | High | Low | High |
ACID Compliance | Yes (InnoDB) | Yes | Yes | Partial |
Ease of Use | Beginner-Friendly | Intermediate | Beginner-Friendly | Beginner-Friendly |
Best Use Case | Web Applications | Complex Queries | Embedded Systems | NoSQL Applications |
Code Example: Connecting to MySQL
Here is an example of connecting to MySQL using Python:
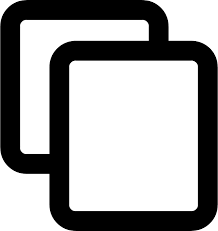
import mysql.connector
# Establish connection
connection = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="example_db"
)
# Check if the connection is successful
if connection.is_connected():
print("Connected to MySQL Database")
# Close the connection
connection.close()
Installing MySQL on Windows, macOS, and Linux
MySQL is compatible with multiple operating systems and can be easily installed on Windows, macOS, and Linux. Below is a step-by-step guide for installing MySQL on each platform.
Installing MySQL on Windows
- Download the MySQL Installer from the official MySQL website.
- Run the downloaded installer and choose the setup type (e.g., Developer Default or Server Only).
- Follow the installation wizard to set up MySQL Server and MySQL Workbench.
- During configuration, set the root password and choose the authentication method.
- Complete the installation and verify by opening the MySQL Command Line Client or MySQL Workbench.
Tip: Ensure that the MySQL service is running by checking the "Services" panel in Windows.
Installing MySQL on macOS
- Download the MySQL DMG archive from the official MySQL website.
- Open the DMG file and follow the installation instructions.
- During setup, configure the root password and optionally enable MySQL to start automatically at system boot.
- After installation, open the terminal and run
mysql -u root -p
to verify the installation.
Note: On macOS, you can also install MySQL using Homebrew
:
brew install mysql
brew services start mysql
Installing MySQL on Linux
For Ubuntu/Debian:
- Update the package repository:
sudo apt update
- Install MySQL Server:
sudo apt install mysql-server
- Secure the installation:
sudo mysql_secure_installation
- Verify the installation by logging into MySQL:
mysql -u root -p
For CentOS/RHEL:
- Add the MySQL Yum repository:
sudo yum install https://dev.mysql.com/get/mysql80-community-release-el7-1.noarch.rpm
- Install MySQL Server:
sudo yum install mysql-server
- Start the MySQL service:
sudo systemctl start mysqld
- Secure the installation and verify:
sudo mysql_secure_installation mysql -u root -p
Verifying MySQL Installation
After installation, you can verify the MySQL version using the following command:
mysql --version
Code Example: Testing the MySQL Connection
Below is a Python script to verify your MySQL connection:
import mysql.connector
try:
connection = mysql.connector.connect(
host="localhost",
user="root",
password="your_password"
)
if connection.is_connected():
print("Connected to MySQL successfully!")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("MySQL connection closed.")
Introduction to MySQL Workbench (GUI for MySQL)
MySQL Workbench is a unified visual tool designed for database architects, developers, and administrators. It provides an intuitive GUI to interact with MySQL databases, making it easier to design, query, and manage databases.
Key Features of MySQL Workbench
MySQL Workbench offers a wide range of features that enhance database management:
Feature | Description |
---|---|
Database Design | Provides a visual interface to create, edit, and manage database schemas. |
Query Editor | Enables writing and executing SQL queries with syntax highlighting and result visualization. |
Data Modeling | Supports forward and reverse engineering, allowing easy schema management. |
Server Administration | Offers tools for managing users, monitoring server performance, and configuring settings. |
Data Export/Import | Facilitates importing and exporting data in various formats like CSV and JSON. |
Installing MySQL Workbench
- Download the MySQL Workbench installer from the official MySQL website.
- Run the installer and follow the setup wizard to complete the installation.
- Launch MySQL Workbench and configure your first database connection.
Setting Up a Database Connection
Follow these steps to connect MySQL Workbench to a MySQL database:
- Open MySQL Workbench.
- Click on the + icon next to "MySQL Connections" to create a new connection.
- Fill in the connection details:
- Connection Name: A descriptive name for the connection.
- Hostname: The database server's hostname (e.g., localhost).
- Port: Typically 3306 for MySQL.
- Username: Your MySQL username (e.g., root).
- Password: (Optional) Save your password for easier access.
- Click Test Connection to verify the connection settings.
- If successful, click OK to save the connection.
Using MySQL Workbench
Querying a Database
Write and execute SQL queries in the query editor:
-- Example: Retrieve all rows from the 'employees' table
SELECT * FROM employees;
Designing a Schema
Create and modify schemas using the visual schema designer:
- Navigate to the Database menu and select Model.
- Add tables, define relationships, and forward-engineer the model to the database.
Code Example: Creating a Table in MySQL Workbench
The following SQL script creates a simple "users" table in the database:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Diagram: MySQL Workbench Overview
The diagram below illustrates the main components of the MySQL Workbench interface:

Advantages of MySQL Workbench
- Streamlined database design and management.
- Integrated tools for administration and querying.
- Support for multiple platforms and MySQL versions.
- User-friendly interface for beginners and experts alike.
Connecting to MySQL Through Command-Line and GUI Tools
To interact with a MySQL database, you can use both command-line tools and graphical user interfaces (GUIs). Each method has its advantages, and the choice depends on your preference and use case.
Connecting to MySQL via Command-Line
The command-line interface (CLI) offers a powerful way to manage MySQL databases directly. Follow these steps to connect:
- Open your terminal or command prompt.
- Run the following command to connect to the MySQL server:
mysql -u username -p
- Replace
username
with your MySQL username (e.g.,root
). - Enter your password when prompted. The terminal will not display the password for security reasons.
- Once connected, you will see the MySQL prompt:
mysql>
Example Commands
Here are some common MySQL commands to use in the CLI:
Command | Description |
---|---|
SHOW DATABASES; |
Lists all available databases. |
USE database_name; |
Selects a database to work with. |
SHOW TABLES; |
Displays all tables in the selected database. |
EXIT; |
Exits the MySQL prompt. |
Connecting to MySQL via GUI Tools
Graphical User Interface (GUI) tools like MySQL Workbench provide an intuitive way to interact with MySQL databases. Follow these steps to connect:
- Open the GUI tool (e.g., MySQL Workbench).
- Create a new connection:
- Click the + icon next to "MySQL Connections."
- Fill in the connection details:
- Connection Name: Give your connection a name.
- Hostname: Enter the server’s hostname (e.g.,
localhost
). - Port: Use the default MySQL port
3306
. - Username: Your MySQL username.
- Password: Enter your password or click Store in Vault to save it.
- Click Test Connection to verify the connection.
- If the test is successful, click OK to save the connection.
- Select the connection from the home screen to connect to the database.
Advantages of GUI Tools
- Easy-to-use interface for database management.
- Visual tools for designing schemas and running queries.
- Built-in options for data import/export and backups.
Code Example: Querying a Database
Here’s an example of querying a database using both methods:
Using Command-Line:
-- Select all rows from the 'employees' table
SELECT * FROM employees;
Using GUI Tool:
Open the query editor, paste the same SQL query, and execute it to see the results in a tabular format.
Diagram: Command-Line vs GUI Tools
The following diagram compares the workflows of connecting to MySQL through CLI and GUI:

Conclusion
Both command-line and GUI tools have their strengths. The CLI is ideal for quick tasks and scripting, while GUI tools are better suited for complex database design and management tasks.
Databases, Tables, and Records
In MySQL, data is organized into databases, which are collections of tables that store records. A database acts as a container for all of the related data, and tables are used to structure that data in rows and columns. Records, also known as rows, are individual entries within a table that contain the actual data.
Databases
A database is a logical container for tables. It allows you to organize data in a way that makes it easier to manage and query. A database can contain multiple tables, and each table can store different types of data. You can create, delete, and manage databases using SQL commands.
Tables
Tables are the primary structure where data is stored in a database. A table consists of rows and columns, where each column represents a different attribute or field of the data, and each row represents a record. Tables should be designed with appropriate columns to store data in an organized way, ensuring it can be easily queried and updated.
Records
Records, also known as rows, represent individual entries in a table. Each record contains a set of data values corresponding to the columns of the table. A record is created when new data is inserted into a table, and it can be updated or deleted later as needed.
Creating a Database and Table
Here’s how you can create a database and a table in MySQL:
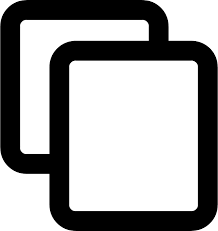
-- Create a new database
CREATE DATABASE my_database;
-- Use the created database
USE my_database;
-- Create a new table
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
email VARCHAR(100)
);
Inserting Records
Once you have a table, you can insert records (rows) into it using the INSERT INTO
statement. Here’s an example of adding a new record to the "users" table:
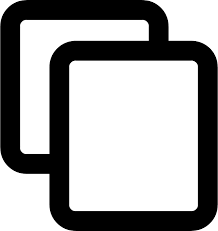
-- Insert a new record into the users table
INSERT INTO users (first_name, last_name, email)
VALUES ('John', 'Doe', 'john.doe@example.com');
Retrieving Records
You can retrieve records from a table using the SELECT
statement. Here’s how you can select all records from the "users" table:
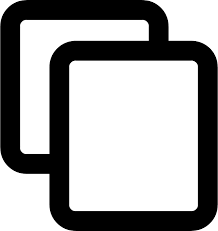
-- Retrieve all records from the users table
SELECT * FROM users;
Diagram: Database Structure
The following diagram illustrates the structure of a MySQL database with tables and records:

The diagram shows a database containing multiple tables, with each table holding rows (records) of data that are categorized by columns (fields).
Data Types in MySQL (INT, VARCHAR, DATE, etc.)
In MySQL, data types define the type of data that can be stored in a column. Each column in a table is assigned a specific data type, which determines what kind of values can be inserted into that column. MySQL supports various data types, including numeric, string, and date types, among others.
Numeric Data Types
Numeric data types are used to store numbers. These can be integers, floating-point numbers, or fixed-point numbers. Below are some of the most commonly used numeric data types:
Data Type | Description |
---|---|
INT | Stores a whole number, both positive and negative, within a specified range. It is often used for counting or storing quantities. |
FLOAT | Stores a floating-point number, which is a number with a decimal point. It is used for more precise calculations. |
DECIMAL | Stores fixed-point numbers with exact precision. It is often used for currency or financial calculations where rounding errors are not acceptable. |
String Data Types
String data types are used to store text or alphanumeric data. The most commonly used string data types include:
Data Type | Description |
---|---|
VARCHAR | Stores variable-length strings. The length of the string can be specified when creating the column (e.g., VARCHAR(255)). This is ideal for text fields that can vary in length. |
CHAR | Stores fixed-length strings. The length is defined when creating the column (e.g., CHAR(50)). If the string is shorter than the defined length, it will be padded with spaces. |
TEXT | Stores long text strings, often used for descriptions, comments, or other large chunks of text. The maximum length is larger than that of VARCHAR. |
Date and Time Data Types
MySQL also supports various data types for handling date and time values. These data types allow you to store information related to dates, times, and timestamps:
Data Type | Description |
---|---|
DATE | Stores a date value in the format YYYY-MM-DD. It is used to store calendar dates. |
TIME | Stores a time value in the format HH:MM:SS. It is used to store time of day values. |
DATETIME | Stores both date and time values in the format YYYY-MM-DD HH:MM:SS. It is commonly used for timestamps. |
TIMESTAMP | Stores a timestamp value, which is a combination of a date and time, but it is stored as the number of seconds since the Unix epoch (1970-01-01 00:00:00). It is often used for automatically recording the date and time of record creation or updates. |
Other Data Types
In addition to the common data types mentioned above, MySQL also supports other data types for various use cases:
Data Type | Description |
---|---|
BOOLEAN | Stores boolean values, either TRUE or FALSE. Internally, this is stored as a TINYINT (0 for FALSE, 1 for TRUE). |
BLOB | Stores binary large objects (BLOBs), such as images, audio, or other multimedia files. It is used to store non-text data in a table. |
ENUM | Stores one of a predefined set of values. It is useful for columns that have a limited set of possible values, such as "Male" or "Female" for a gender column. |
Example: Creating a Table with Different Data Types
Here’s an example of how you can create a table that uses different data types for its columns:
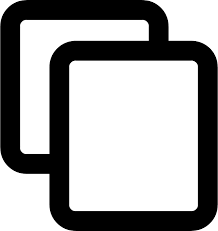
-- Create a new table with various data types
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
email VARCHAR(100),
birthdate DATE,
registration_time DATETIME,
is_active BOOLEAN
);
Diagram: MySQL Data Types
The following diagram illustrates the various MySQL data types and their use cases:

The diagram shows the different categories of data types in MySQL and how they are used to store different kinds of data.
Basic SQL Syntax
SQL (Structured Query Language) is the standard language used for interacting with relational databases. It allows you to perform various operations such as querying data, inserting records, updating data, and deleting records. SQL syntax follows a set of rules for writing queries, and understanding its basic structure is essential for working with databases.
SQL Statements
SQL queries consist of statements, which are used to perform specific tasks. Some of the most common SQL statements include:
Statement | Description |
---|---|
SELECT | Used to retrieve data from one or more tables in a database. |
INSERT | Used to add new records (rows) to a table. |
UPDATE | Used to modify existing records in a table. |
DELETE | Used to remove records from a table. |
Basic SQL Query Structure
Most SQL queries follow a basic structure: the SELECT
statement to retrieve data, and the FROM
clause to specify the table from which to retrieve data. Other clauses can be added to filter, group, or order the results.
Example: SELECT Statement
The following example retrieves all columns from the "users" table:
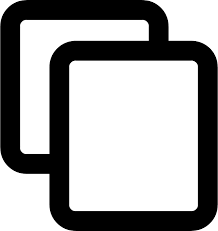
-- Retrieve all columns from the users table
SELECT * FROM users;
Clauses in SQL
SQL queries can include additional clauses to refine the data you want to retrieve or modify. Below are some of the most commonly used clauses:
Clause | Description |
---|---|
WHERE | Filters records based on a condition. Used with SELECT , UPDATE , and DELETE to specify which rows to affect. |
ORDER BY | Sorts the result set by one or more columns, either in ascending (ASC ) or descending (DESC ) order. |
GROUP BY | Groups rows that have the same values into summary rows, often used with aggregate functions like COUNT, AVG, SUM, etc. |
LIMIT | Limits the number of rows returned by a query. |
Example: Using WHERE, ORDER BY, and LIMIT
This query retrieves the first 5 users whose age is greater than 30, ordered by their last name in ascending order:
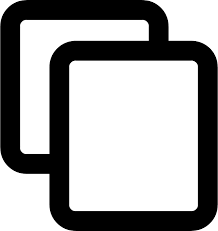
-- Retrieve the first 5 users with age > 30, ordered by last name
SELECT * FROM users
WHERE age > 30
ORDER BY last_name ASC
LIMIT 5;
SQL Data Modification
SQL is also used to modify data in a database. Below are examples for inserting, updating, and deleting records:
Example: INSERT Statement
This query adds a new record to the "users" table:
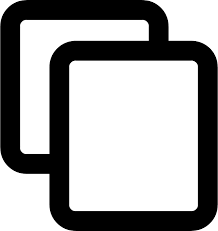
-- Insert a new record into the users table
INSERT INTO users (first_name, last_name, email)
VALUES ('Jane', 'Doe', 'jane.doe@example.com');
Example: UPDATE Statement
This query updates the email address of a user with a specific ID:
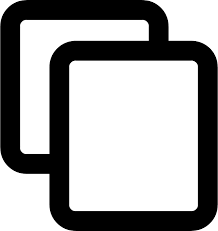
-- Update the email address of the user with ID 1
UPDATE users
SET email = 'new.email@example.com'
WHERE id = 1;
Example: DELETE Statement
This query deletes a record from the "users" table based on a specific condition:
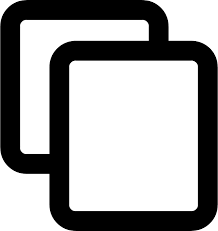
-- Delete a user with ID 1 from the users table
DELETE FROM users
WHERE id = 1;
Diagram: SQL Query Flow
The following diagram illustrates how an SQL query flows from writing the query to getting the result:

This diagram shows the steps involved in executing an SQL query, from writing the statement to the database returning the result.
Creating and Selecting Databases (CREATE DATABASE, USE)
In MySQL, a database is a container for storing tables, views, stored procedures, and other objects. To interact with a database, you need to create it and select it as the active database. The two primary SQL statements used for this purpose are CREATE DATABASE
and USE
.
CREATE DATABASE
The CREATE DATABASE
statement is used to create a new database in MySQL. You must specify a unique name for the database. After creating a database, you can begin creating tables and other database objects within it.
Syntax:
-- Create a new database named 'company_db'
CREATE DATABASE company_db;
USE Statement
After creating a database, you need to select it for use. The USE
statement sets the specified database as the active database for the current session. Once a database is selected, all subsequent operations (such as creating tables, inserting data, etc.) will be performed within that database unless another database is selected.
Syntax:
-- Select the 'company_db' database for use
USE company_db;
Example: Creating and Selecting a Database
The following example demonstrates how to create a database and then select it for use:
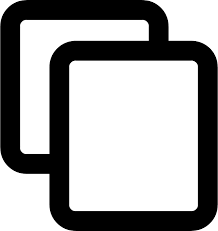
-- Create a database named 'company_db'
CREATE DATABASE company_db;
-- Select 'company_db' to use it
USE company_db;
-- Now you can perform operations like creating tables, inserting data, etc.
Listing All Databases
If you want to see a list of all databases available in your MySQL server, you can use the SHOW DATABASES
statement:
Syntax:
-- Show all databases
SHOW DATABASES;
Example: Listing Databases
The following example demonstrates how to view all databases in MySQL:
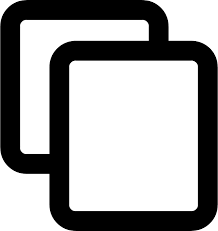
-- Show all databases in MySQL
SHOW DATABASES;
Example: Dropping a Database
If you no longer need a database, you can delete it using the DROP DATABASE
statement. Be cautious when using this command, as it permanently deletes the database and all its contents.
Syntax:
-- Drop (delete) the 'company_db' database
DROP DATABASE company_db;
Diagram: Creating and Selecting Databases
The following diagram illustrates the flow of creating and selecting a database in MySQL:

This diagram shows the steps involved in creating a new database, selecting it for use, and performing operations within it.
Creating Tables (CREATE TABLE)
In MySQL, a table is a collection of data organized in rows and columns. The CREATE TABLE
statement is used to define a new table and its structure within a database. You specify the table name and define each column's name, data type, and constraints.
CREATE TABLE Syntax
The syntax for creating a table involves specifying the table name followed by the definition of each column, including the column name, data type, and optional constraints (such as NOT NULL
, PRIMARY KEY
, etc.).
Syntax:
-- Create a table named 'employees' with columns: id, first_name, last_name, and hire_date
CREATE TABLE employees (
id INT AUTO_INCREMENT,
first_name VARCHAR(100) NOT NULL,
last_name VARCHAR(100) NOT NULL,
hire_date DATE,
PRIMARY KEY (id)
);
Explanation
In the example above, the table 'employees' is created with the following columns:
- id: An integer column that automatically increments for each new row. It is the primary key of the table.
- first_name: A string column (up to 100 characters) that cannot be null.
- last_name: A string column (up to 100 characters) that cannot be null.
- hire_date: A date column that stores the hiring date of an employee.
Common Data Types in CREATE TABLE
When creating a table, you need to specify the appropriate data type for each column. Some common data types include:
Data Type | Description |
---|---|
INT | Used for integer values. Often used for columns like IDs, ages, etc. |
VARCHAR | Used for variable-length strings. You specify the maximum length (e.g., VARCHAR(100) ). |
DATE | Used for storing date values (in the format YYYY-MM-DD ). |
FLOAT | Used for storing floating-point numbers (decimal values). |
Example: Creating a Table with Multiple Columns
The following example creates a 'departments' table with columns for the department's name, location, and the number of employees:
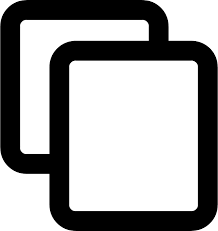
-- Create a table named 'departments' with columns: name, location, and number of employees
CREATE TABLE departments (
id INT AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
location VARCHAR(100),
num_employees INT,
PRIMARY KEY (id)
);
Adding Constraints to Columns
While creating tables, you can also specify constraints to enforce rules on the data. Some commonly used constraints include:
- NOT NULL: Ensures a column cannot have a
NULL
value. - PRIMARY KEY: Uniquely identifies each row in the table. A primary key must be unique for each record and cannot be
NULL
. - UNIQUE: Ensures all values in a column are unique.
- FOREIGN KEY: Establishes a relationship between two tables by linking a column in one table to the primary key of another table.
Example: Using Constraints in CREATE TABLE
This example demonstrates the use of constraints while creating a table:
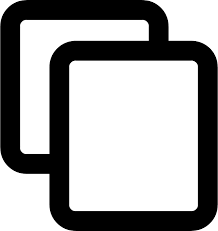
-- Create a table 'employees' with constraints: id as PRIMARY KEY, and first_name as NOT NULL
CREATE TABLE employees (
id INT AUTO_INCREMENT,
first_name VARCHAR(100) NOT NULL,
last_name VARCHAR(100) NOT NULL,
email VARCHAR(100) UNIQUE,
hire_date DATE,
PRIMARY KEY (id)
);
Example: Creating a Table with a Foreign Key
The following example creates a 'projects' table with a foreign key that links each project to an employee:
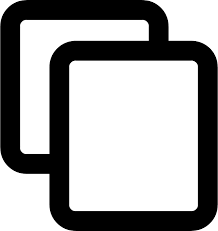
-- Create a table 'projects' with a foreign key linking to the 'employees' table
CREATE TABLE projects (
project_id INT AUTO_INCREMENT,
project_name VARCHAR(100) NOT NULL,
employee_id INT,
PRIMARY KEY (project_id),
FOREIGN KEY (employee_id) REFERENCES employees(id)
);
Diagram: Table Creation Process
The following diagram illustrates how the process of creating a table works, from defining the table structure to adding constraints and relationships:

This diagram shows the steps involved in creating a table, including defining columns, setting data types, and adding constraints such as primary and foreign keys.
Inserting Data into Tables (INSERT INTO)
In MySQL, the INSERT INTO
statement is used to add new records (rows) to a table. You can insert data into a table by specifying the column names and the values to be inserted. The data types of the values must match the column data types defined when the table was created.
INSERT INTO Syntax
The syntax for inserting data into a table involves specifying the table name, followed by the column names and corresponding values to be inserted. The values must be provided in the same order as the columns.
Syntax:
-- Insert data into the 'employees' table
INSERT INTO employees (first_name, last_name, hire_date)
VALUES ('John', 'Doe', '2025-01-21');
Explanation
In the example above, the INSERT INTO
statement is used to insert a new employee record into the employees
table. The columns first_name
, last_name
, and hire_date
are specified, followed by the corresponding values.
Inserting Data into All Columns
If you want to insert data into all columns of a table, you can omit the column names and provide values for all columns in the correct order as they appear in the table definition.
Syntax:
-- Insert data into all columns of the 'employees' table
INSERT INTO employees
VALUES (NULL, 'Jane', 'Smith', '2025-01-22');
Example: Inserting Multiple Rows
You can insert multiple rows at once by separating each set of values with commas. This is more efficient than inserting each row individually.
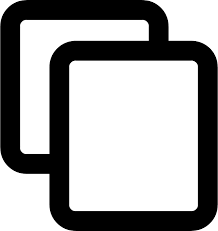
-- Insert multiple rows into the 'employees' table
INSERT INTO employees (first_name, last_name, hire_date)
VALUES
('Alice', 'Johnson', '2025-01-23'),
('Bob', 'Williams', '2025-01-24'),
('Charlie', 'Brown', '2025-01-25');
Inserting Data with Default Values
If a column has a default value (such as an auto-incrementing primary key), you can omit that column in the INSERT INTO
statement. The database will automatically assign the default value for that column.
Syntax:
-- Insert data into the 'employees' table, omitting the 'id' column (auto-increment)
INSERT INTO employees (first_name, last_name, hire_date)
VALUES ('David', 'Miller', '2025-01-26');
Example: Inserting Data with Default Value
The following example inserts a new employee record without specifying the id
column, allowing the database to automatically assign a value:
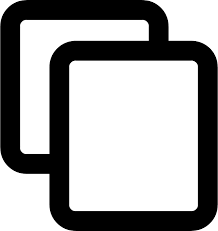
-- Insert data into the 'employees' table without specifying the 'id' column
INSERT INTO employees (first_name, last_name, hire_date)
VALUES ('Emma', 'Davis', '2025-01-27');
Inserting Data into a Table with Foreign Key Constraints
If a table contains a foreign key constraint, you must ensure that the inserted value in the foreign key column corresponds to an existing value in the referenced table. Otherwise, the insertion will fail.
Syntax:
-- Insert data into the 'projects' table, ensuring the 'employee_id' corresponds to an existing employee
INSERT INTO projects (project_name, employee_id)
VALUES ('Project A', 1);
Example: Inserting Data with Foreign Key
Below is an example of inserting a project into the projects
table, where the employee_id
must match an existing employee ID from the employees
table:
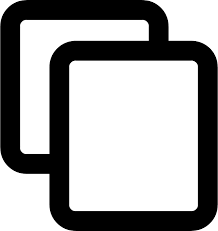
-- Insert a project for employee with id 1
INSERT INTO projects (project_name, employee_id)
VALUES ('Website Development', 1);
Diagram: Inserting Data into a Table
The following diagram illustrates the process of inserting data into a table, from specifying the table and columns to entering the values and executing the statement:

This diagram shows the steps involved in inserting data into a table, including handling default values and foreign key constraints.
Retrieving Data from Tables (SELECT)
The SELECT
statement in MySQL is used to retrieve data from one or more tables. It allows you to specify which columns to retrieve, as well as apply conditions, sorting, and aggregation to the results. The SELECT
statement is one of the most commonly used SQL commands to interact with a database.
SELECT Syntax
The basic syntax for retrieving data from a table includes the SELECT
keyword followed by the columns you want to retrieve. You can also use *
to select all columns from the table.
Syntax:
-- Select all columns from the 'employees' table
SELECT * FROM employees;
Explanation
In the example above, the SELECT *
statement retrieves all columns from the employees
table. The *
wildcard means "all columns." You can specify specific columns if you only want to retrieve certain ones.
Selecting Specific Columns
If you only need to retrieve specific columns, you can list them after the SELECT
keyword, separated by commas.
Syntax:
-- Select the 'first_name' and 'last_name' columns from the 'employees' table
SELECT first_name, last_name FROM employees;
Example: Selecting Specific Columns
The following example retrieves only the first_name
and hire_date
columns from the employees
table:
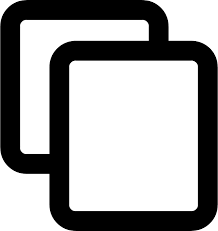
-- Select 'first_name' and 'hire_date' from the 'employees' table
SELECT first_name, hire_date FROM employees;
Filtering Data with WHERE Clause
To filter the results based on specific conditions, you can use the WHERE
clause. This allows you to retrieve only the rows that meet the specified condition.
Syntax:
-- Select employees who were hired after '2025-01-01'
SELECT first_name, last_name, hire_date
FROM employees
WHERE hire_date > '2025-01-01';
Example: Using WHERE Clause
The following example retrieves employees whose hire date is after '2025-01-01':
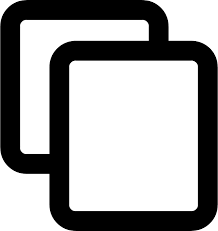
-- Select employees hired after '2025-01-01'
SELECT first_name, last_name, hire_date
FROM employees
WHERE hire_date > '2025-01-01';
Sorting Data with ORDER BY
The ORDER BY
clause is used to sort the result set by one or more columns. By default, ORDER BY
sorts in ascending order, but you can specify DESC
for descending order.
Syntax:
-- Select employees and sort by 'hire_date' in descending order
SELECT first_name, last_name, hire_date
FROM employees
ORDER BY hire_date DESC;
Example: Sorting Data
This example retrieves a list of employees sorted by hire date, with the most recently hired employees at the top:
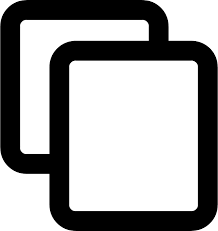
-- Select employees and sort by 'hire_date' in descending order
SELECT first_name, last_name, hire_date
FROM employees
ORDER BY hire_date DESC;
Limiting the Number of Results
To limit the number of rows returned by the SELECT
statement, you can use the LIMIT
clause.
Syntax:
-- Select the first 5 employees from the 'employees' table
SELECT first_name, last_name, hire_date
FROM employees
LIMIT 5;
Example: Using LIMIT
This example selects the first 3 employees from the employees
table:
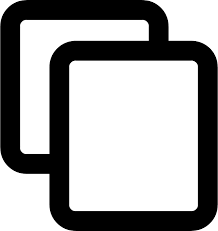
-- Select the first 3 employees
SELECT first_name, last_name, hire_date
FROM employees
LIMIT 3;
Combining Filtering, Sorting, and Limiting
You can combine the WHERE
, ORDER BY
, and LIMIT
clauses to filter, sort, and limit the rows returned in a single query.
Syntax:
-- Select the first 3 employees hired after '2025-01-01' and sort by 'hire_date'
SELECT first_name, last_name, hire_date
FROM employees
WHERE hire_date > '2025-01-01'
ORDER BY hire_date DESC
LIMIT 3;
Example: Combining Clauses
This example retrieves the first 3 employees hired after '2025-01-01', sorted by the most recent hire date:
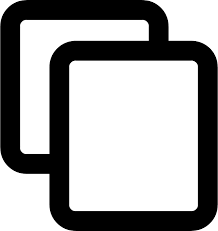
-- Select the first 3 employees hired after '2025-01-01' and sort by 'hire_date'
SELECT first_name, last_name, hire_date
FROM employees
WHERE hire_date > '2025-01-01'
ORDER BY hire_date DESC
LIMIT 3;
Diagram: Retrieving Data from a Table
The following diagram illustrates how data retrieval works in MySQL, from the SELECT
statement to filtering, sorting, and limiting the results:

This diagram shows the flow of the SELECT
query, including how you can filter, sort, and limit the rows returned by the database.
Updating Data in Tables (UPDATE)
The UPDATE
statement in MySQL is used to modify existing records in a table. It allows you to update one or more columns with new values, based on a condition. The UPDATE
statement can be used with the WHERE
clause to specify which rows should be updated, otherwise, all rows in the table will be modified.
UPDATE Syntax
The basic syntax for updating data in a table involves the UPDATE
keyword, followed by the table name, and then the SET
clause, which specifies which column(s) to update and their new values. The WHERE
clause is optional but often necessary to limit the update to specific rows.
Syntax:
-- Update the 'salary' column for the employee with 'employee_id' = 5
UPDATE employees
SET salary = 60000
WHERE employee_id = 5;
Explanation
In the example above, the UPDATE
statement updates the salary
column for the employee with employee_id
equal to 5. The WHERE
clause ensures that only the specific employee's salary is updated, and not all employees in the table.
Updating Multiple Columns
You can update multiple columns in a single UPDATE
statement by separating each column assignment with a comma.
Syntax:
-- Update both 'salary' and 'hire_date' columns for the employee with 'employee_id' = 5
UPDATE employees
SET salary = 65000, hire_date = '2025-01-01'
WHERE employee_id = 5;
Example: Updating Multiple Columns
The following example updates both the salary
and hire_date
for the employee with employee_id
equal to 5:
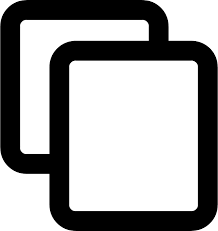
-- Update 'salary' and 'hire_date' for employee with 'employee_id' = 5
UPDATE employees
SET salary = 65000, hire_date = '2025-01-01'
WHERE employee_id = 5;
Updating Data Without a WHERE Clause
If you omit the WHERE
clause, all rows in the table will be updated. This can be dangerous if you intend to update only specific records, so always double-check your conditions when using the UPDATE
statement.
Syntax:
-- Update the 'salary' column for all employees in the 'employees' table
UPDATE employees
SET salary = 70000;
Example: Updating All Rows
The following example updates the salary
for all employees in the employees
table:
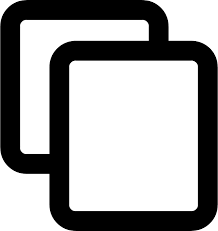
-- Update 'salary' for all employees to 70000
UPDATE employees
SET salary = 70000;
Using Expressions in the UPDATE Statement
You can also use expressions, calculations, or subqueries in the SET
clause to update column values dynamically.
Syntax:
-- Increase the 'salary' by 10% for all employees
UPDATE employees
SET salary = salary * 1.10;
Example: Using Expressions
The following example increases the salary
by 10% for all employees:
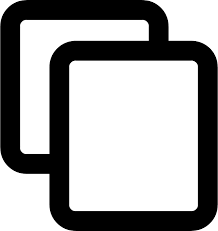
-- Increase 'salary' by 10% for all employees
UPDATE employees
SET salary = salary * 1.10;
Combining UPDATE with JOIN (Advanced)
You can also use the UPDATE
statement in combination with a JOIN
to update records in one table based on data from another table.
Syntax:
-- Update the 'salary' column in 'employees' table based on matching 'department_id' in 'departments' table
UPDATE employees e
JOIN departments d ON e.department_id = d.department_id
SET e.salary = 75000
WHERE d.department_name = 'Sales';
Example: UPDATE with JOIN
This example updates the salary
for employees in the "Sales" department to 75000:
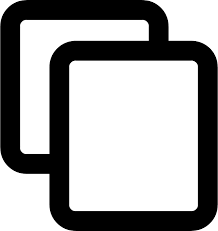
-- Update 'salary' for employees in the 'Sales' department
UPDATE employees e
JOIN departments d ON e.department_id = d.department_id
SET e.salary = 75000
WHERE d.department_name = 'Sales';
Diagram: Updating Data in a Table
The following diagram illustrates the flow of the UPDATE
statement, from the selection of the rows to be updated to the final modification of the data:

This diagram shows the flow of the UPDATE
command, including the process of selecting rows, updating column values, and committing the changes to the table.
Deleting Data from Tables (DELETE)
The DELETE
statement in MySQL is used to remove records from a table. It allows you to delete one or more rows based on a condition. The DELETE
statement can be used with the WHERE
clause to specify which rows should be deleted; otherwise, all rows in the table will be removed.
DELETE Syntax
The basic syntax for deleting data from a table involves the DELETE
keyword, followed by the FROM
clause and the table name. The WHERE
clause is optional but highly recommended to avoid deleting all rows in the table.
Syntax:
-- Delete the record for the employee with 'employee_id' = 5
DELETE FROM employees
WHERE employee_id = 5;
Explanation
In the example above, the DELETE
statement removes the record for the employee with employee_id
equal to 5. The WHERE
clause ensures that only the specific employee's record is deleted, not all employees in the table.
Deleting Multiple Rows
You can delete multiple rows by specifying a condition that matches more than one row. For example, you can delete all employees from a specific department.
Syntax:
-- Delete all employees in the 'Sales' department
DELETE FROM employees
WHERE department = 'Sales';
Example: Deleting Multiple Rows
The following example deletes all employees in the "Sales" department:
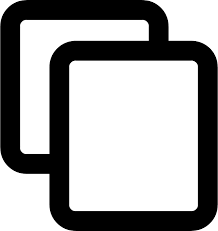
-- Delete all employees from the 'Sales' department
DELETE FROM employees
WHERE department = 'Sales';
Deleting All Rows in a Table
If you omit the WHERE
clause, all rows in the table will be deleted. This action is irreversible, so use it with caution.
Syntax:
-- Delete all records from the 'employees' table
DELETE FROM employees;
Example: Deleting All Rows
The following example deletes all records from the employees
table:
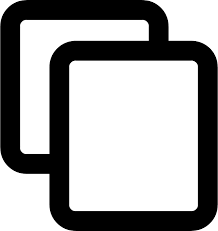
-- Delete all rows from the 'employees' table
DELETE FROM employees;
Using DELETE with JOIN (Advanced)
You can also use the DELETE
statement with a JOIN
to delete records from one table based on matching records in another table. This is useful when you need to delete rows from a table that have related records in another table.
Syntax:
-- Delete employees who belong to a specific department in the 'departments' table
DELETE e FROM employees e
JOIN departments d ON e.department_id = d.department_id
WHERE d.department_name = 'Sales';
Example: DELETE with JOIN
This example deletes all employees in the "Sales" department:
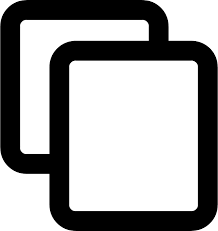
-- Delete employees in the 'Sales' department
DELETE e FROM employees e
JOIN departments d ON e.department_id = d.department_id
WHERE d.department_name = 'Sales';
Using DELETE with Subqueries (Advanced)
You can also use a subquery in a DELETE
statement to delete records based on results from another query.
Syntax:
-- Delete employees who have a salary lower than the average salary
DELETE FROM employees
WHERE salary < (SELECT AVG(salary) FROM employees);
Example: DELETE with Subquery
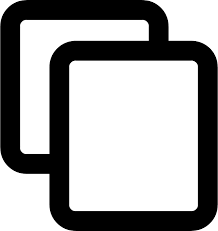
-- Delete employees with salary lower than the average salary
DELETE FROM employees
WHERE salary < (SELECT AVG(salary) FROM employees);
Diagram: Deleting Data from a Table
The following diagram illustrates the flow of the DELETE
statement, from the selection of the rows to be deleted to the final removal of the data:

This diagram shows the flow of the DELETE
command, including the process of selecting rows and removing them from the table.
SELECT Statement with Different Clauses (WHERE, ORDER BY, LIMIT)
The SELECT
statement in MySQL is used to query data from one or more tables. It allows you to retrieve specific columns, filter data, sort results, and limit the number of rows returned. You can apply various clauses, such as WHERE
, ORDER BY
, and LIMIT
, to refine your query.
Filtering Data with WHERE Clause
The WHERE
clause is used to filter records based on a specified condition. It allows you to retrieve only the rows that meet the condition.
Syntax:
-- Select all employees where the department is 'Sales'
SELECT * FROM employees
WHERE department = 'Sales';
Using ORDER BY Clause
The ORDER BY
clause is used to sort the result set. You can sort the results in ascending (ASC
) or descending (DESC
) order.
Syntax:
-- Select all employees and order them by salary in descending order
SELECT * FROM employees
ORDER BY salary DESC;
Using LIMIT Clause
The LIMIT
clause is used to specify the number of rows to return. It is useful for pagination or limiting the size of your result set.
Syntax:
-- Select the first 5 employees from the 'employees' table
SELECT * FROM employees
LIMIT 5;
Combining WHERE, ORDER BY, and LIMIT
You can combine the WHERE
, ORDER BY
, and LIMIT
clauses in a single query to filter, sort, and limit your results.
Syntax:
-- Select the first 5 highest paid employees from the 'Sales' department
SELECT * FROM employees
WHERE department = 'Sales'
ORDER BY salary DESC
LIMIT 5;
Filtering with LIKE
The LIKE
operator is used to filter records based on a pattern. The pattern can include wildcards such as %
for multiple characters and _
for a single character.
Syntax:
-- Select employees whose name starts with 'J'
SELECT * FROM employees
WHERE name LIKE 'J%';
Filtering with IN
The IN
operator is used to filter records that match any value in a list. It is useful when you need to check against multiple values.
Syntax:
-- Select employees who work in either 'Sales' or 'Marketing'
SELECT * FROM employees
WHERE department IN ('Sales', 'Marketing');
Filtering with BETWEEN
The BETWEEN
operator is used to filter records within a specific range. It is typically used with numeric, date, or time values.
Syntax:
-- Select employees with a salary between 30000 and 50000
SELECT * FROM employees
WHERE salary BETWEEN 30000 AND 50000;
Sorting and Pagination
Sorting and pagination are essential for managing large datasets. You can use the ORDER BY
clause for sorting and the LIMIT
clause for pagination. Combining both allows you to retrieve a specific subset of records, sorted in a particular order.
Example: Sorting and Pagination
-- Select employees 6 to 10 from the 'employees' table, ordered by salary in ascending order
SELECT * FROM employees
ORDER BY salary ASC
LIMIT 5 OFFSET 5;
Diagram: SELECT Statement Execution Flow
The following diagram explains how the SELECT
statement is processed with different clauses, from filtering to sorting and limiting the result set:

This diagram illustrates the flow of a SELECT
query, showing how clauses like WHERE
, ORDER BY
, and LIMIT
interact during query execution.
Aggregate Functions: COUNT(), SUM(), AVG(), MIN(), MAX()
Aggregate functions in MySQL are used to perform calculations on multiple rows of data and return a single value. These functions are useful for summarizing data and are often used in conjunction with the SELECT
statement. The most commonly used aggregate functions are COUNT()
, SUM()
, AVG()
, MIN()
, and MAX()
.
COUNT() Function
The COUNT()
function is used to count the number of rows that match a specified condition. It can also count non-NULL values in a particular column.
Syntax:
-- Count the total number of employees in the 'employees' table
SELECT COUNT(*) FROM employees;
The example above counts all rows in the employees
table. If you want to count specific rows based on a condition, you can add a WHERE
clause.
Syntax with WHERE:
-- Count the number of employees in the 'Sales' department
SELECT COUNT(*) FROM employees
WHERE department = 'Sales';
SUM() Function
The SUM()
function is used to calculate the total sum of a numeric column. It is often used to sum values such as salaries, prices, or quantities.
Syntax:
-- Calculate the total salary of all employees
SELECT SUM(salary) FROM employees;
The example above calculates the total salary for all employees in the employees
table. You can also sum values based on a specific condition.
Syntax with WHERE:
-- Calculate the total salary of employees in the 'Sales' department
SELECT SUM(salary) FROM employees
WHERE department = 'Sales';
AVG() Function
The AVG()
function is used to calculate the average value of a numeric column. It is useful for finding the mean of values such as salaries, prices, or scores.
Syntax:
-- Calculate the average salary of all employees
SELECT AVG(salary) FROM employees;
The example above calculates the average salary for all employees in the employees
table. You can also calculate the average based on a specific condition.
Syntax with WHERE:
-- Calculate the average salary of employees in the 'Sales' department
SELECT AVG(salary) FROM employees
WHERE department = 'Sales';
MIN() Function
The MIN()
function is used to retrieve the smallest value from a numeric, date, or string column. It is useful for finding the lowest salary, earliest date, or smallest value in a dataset.
Syntax:
-- Get the minimum salary from the 'employees' table
SELECT MIN(salary) FROM employees;
The example above retrieves the minimum salary from the employees
table. You can also use MIN()
with a WHERE
clause to find the lowest value in a specific group.
Syntax with WHERE:
-- Get the minimum salary of employees in the 'Sales' department
SELECT MIN(salary) FROM employees
WHERE department = 'Sales';
MAX() Function
The MAX()
function is used to retrieve the largest value from a numeric, date, or string column. It is useful for finding the highest salary, latest date, or largest value in a dataset.
Syntax:
-- Get the maximum salary from the 'employees' table
SELECT MAX(salary) FROM employees;
The example above retrieves the maximum salary from the employees
table. You can also use MAX()
with a WHERE
clause to find the highest value in a specific group.
Syntax with WHERE:
-- Get the maximum salary of employees in the 'Sales' department
SELECT MAX(salary) FROM employees
WHERE department = 'Sales';
Combining Aggregate Functions
You can combine multiple aggregate functions in a single query to get a variety of calculations at once.
Syntax:
-- Get the total, average, minimum, and maximum salary from the 'employees' table
SELECT
SUM(salary) AS total_salary,
AVG(salary) AS average_salary,
MIN(salary) AS minimum_salary,
MAX(salary) AS maximum_salary
FROM employees;
Example: Using Multiple Aggregate Functions
This example calculates the total, average, minimum, and maximum salaries for all employees:
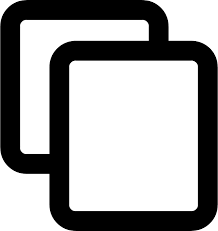
-- Get the total, average, minimum, and maximum salary
SELECT
SUM(salary) AS total_salary,
AVG(salary) AS average_salary,
MIN(salary) AS minimum_salary,
MAX(salary) AS maximum_salary
FROM employees;
Diagram: Aggregate Function Usage
The following diagram illustrates how aggregate functions such as COUNT()
, SUM()
, AVG()
, MIN()
, and MAX()
are applied to a dataset:

This diagram shows the flow of data through the aggregate functions to produce the final result.
Grouping Data: GROUP BY and HAVING
In MySQL, GROUP BY
is used to group rows that share a property into summary rows, such as finding the total sum of salaries per department. HAVING
is used to filter records after the grouping operation, which is not possible with the WHERE
clause.
GROUP BY Clause
The GROUP BY
clause groups rows that have the same values in specified columns into summary rows. It is often used with aggregate functions to perform calculations on each group.
Syntax:
-- Group employees by department and calculate the total salary for each department
SELECT department, SUM(salary) AS total_salary
FROM employees
GROUP BY department;
The example above groups employees by department and calculates the total salary for each department. You can group by one or more columns depending on your needs.
HAVING Clause
The HAVING
clause is used to filter records after the GROUP BY
operation. It allows you to apply conditions to grouped data, something that cannot be done with the WHERE
clause.
Syntax:
-- Group employees by department and calculate the total salary, only including departments with total salary greater than 100000
SELECT department, SUM(salary) AS total_salary
FROM employees
GROUP BY department
HAVING SUM(salary) > 100000;
The example above groups employees by department, calculates the total salary for each department, and filters the result to include only those departments whose total salary exceeds 100,000.
GROUP BY with Multiple Columns
You can also group by multiple columns to create more specific groupings. For example, grouping by both department and job title.
Syntax:
-- Group employees by department and job title, and calculate the total salary for each group
SELECT department, job_title, SUM(salary) AS total_salary
FROM employees
GROUP BY department, job_title;
This example groups employees first by department and then by job title, calculating the total salary for each combination of department and job title.
Combining GROUP BY and Aggregate Functions
Aggregate functions such as COUNT()
, SUM()
, AVG()
, MIN()
, and MAX()
are commonly used with the GROUP BY
clause to summarize data.
Example with COUNT() and GROUP BY:
-- Count the number of employees in each department
SELECT department, COUNT(*) AS num_employees
FROM employees
GROUP BY department;
This example counts the number of employees in each department and groups the data by department.
Example with HAVING and Multiple Conditions
You can also use HAVING
with multiple conditions to filter grouped data. For example, finding departments where the total salary exceeds 100,000 and the number of employees is greater than 5:
Syntax:
-- Find departments with total salary greater than 100000 and more than 5 employees
SELECT department, SUM(salary) AS total_salary, COUNT(*) AS num_employees
FROM employees
GROUP BY department
HAVING SUM(salary) > 100000 AND COUNT(*) > 5;
GROUP BY with ORDER BY
You can combine the GROUP BY
clause with the ORDER BY
clause to sort the results based on the aggregated values.
Syntax:
-- Group employees by department and order the results by total salary in descending order
SELECT department, SUM(salary) AS total_salary
FROM employees
GROUP BY department
ORDER BY total_salary DESC;
This example groups employees by department, calculates the total salary, and orders the results by total salary in descending order.
Example: Grouping with Multiple Conditions
This example demonstrates how to use GROUP BY
with multiple aggregate functions and the HAVING
clause:
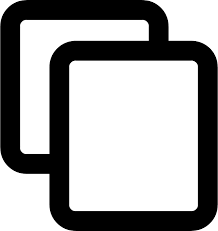
-- Group employees by department, calculate the total salary, count employees, and include only departments with more than 5 employees
SELECT department, SUM(salary) AS total_salary, COUNT(*) AS num_employees
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
Diagram: Grouping Data
The following diagram illustrates how data is grouped using the GROUP BY
clause and then filtered with the HAVING
clause:

This diagram demonstrates how the grouping of data works and how the HAVING
clause filters the results after the grouping.
JOIN Operations: INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL JOIN
In MySQL, JOIN
operations are used to combine data from two or more tables based on a related column. The four most common types of joins are INNER JOIN
, LEFT JOIN
, RIGHT JOIN
, and FULL JOIN
. Each join type defines how the rows from the tables will be combined.
INNER JOIN
The INNER JOIN
returns only the rows that have matching values in both tables. If there is no match, those rows are excluded from the result set.
Syntax:
-- Retrieve data from two tables, employees and departments, where there is a match on department_id
SELECT employees.name, employees.salary, departments.department_name
FROM employees
INNER JOIN departments
ON employees.department_id = departments.department_id;
The example above retrieves the employee name, salary, and department name for employees who belong to a department. Only employees with a matching department will be included in the result set.
LEFT JOIN
The LEFT JOIN
(or LEFT OUTER JOIN
) returns all rows from the left table (the first table in the join), and the matching rows from the right table. If there is no match, the result will contain NULL
for columns from the right table.
Syntax:
-- Retrieve all employees, along with their department name if available, including employees without a department
SELECT employees.name, employees.salary, departments.department_name
FROM employees
LEFT JOIN departments
ON employees.department_id = departments.department_id;
This query returns all employees, including those who do not belong to any department. For employees without a department, the department_name
field will be NULL
.
RIGHT JOIN
The RIGHT JOIN
(or RIGHT OUTER JOIN
) returns all rows from the right table (the second table in the join), and the matching rows from the left table. If there is no match, the result will contain NULL
for columns from the left table.
Syntax:
-- Retrieve all departments, along with employee names if available, including departments with no employees
SELECT employees.name, employees.salary, departments.department_name
FROM employees
RIGHT JOIN departments
ON employees.department_id = departments.department_id;
This query returns all departments, including those with no employees. For departments with no employees, the name
and salary
fields will be NULL
.
FULL JOIN
The FULL JOIN
(or FULL OUTER JOIN
) returns all rows when there is a match in either the left or right table. If there is no match, the result will contain NULL
for columns from the table with no match. Note that MySQL does not support FULL JOIN
directly, but you can simulate it using a combination of LEFT JOIN
and RIGHT JOIN
with a UNION
.
Syntax (Simulated FULL JOIN):
-- Simulating a FULL JOIN by combining LEFT JOIN and RIGHT JOIN
SELECT employees.name, employees.salary, departments.department_name
FROM employees
LEFT JOIN departments
ON employees.department_id = departments.department_id
UNION
SELECT employees.name, employees.salary, departments.department_name
FROM employees
RIGHT JOIN departments
ON employees.department_id = departments.department_id;
This query combines the results of a LEFT JOIN
and a RIGHT JOIN
to simulate a FULL JOIN
. It returns all employees and all departments, including those without matches in the other table. Where there are no matches, the corresponding fields will be NULL
.
JOIN with Multiple Tables
You can perform joins with multiple tables by chaining multiple JOIN
operations. For example, retrieving data from three tables: employees, departments, and projects.
Syntax:
-- Retrieve employees, their department, and the project they are working on
SELECT employees.name, departments.department_name, projects.project_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id
INNER JOIN projects ON employees.project_id = projects.project_id;
This query joins the employees
, departments
, and projects
tables to retrieve the employee name, department name, and project name for each employee.
JOIN with Aliases
Using table aliases can make your SQL queries more readable, especially when working with multiple joins. Here’s an example using aliases for the tables:
Syntax:
-- Using aliases for tables
SELECT e.name, d.department_name, p.project_name
FROM employees AS e
INNER JOIN departments AS d ON e.department_id = d.department_id
INNER JOIN projects AS p ON e.project_id = p.project_id;
In this example, we use e
for employees, d
for departments, and p
for projects, making the query more concise and easier to understand.
Diagram: JOIN Operations
The following diagram illustrates how different types of joins combine data from two tables:

This diagram demonstrates the results of an INNER JOIN
, LEFT JOIN
, and RIGHT JOIN
on two example tables.
Subqueries: SELECT inside SELECT
A subquery is a SQL query that is nested inside another query. Subqueries are used to perform operations that would require multiple steps in a single query. You can use subqueries in the SELECT
, FROM
, WHERE
, and HAVING
clauses, among others. Subqueries can either return a single value (scalar) or multiple rows.
Types of Subqueries
There are two main types of subqueries:
- Single-row Subquery: Returns a single value, used when the outer query expects a single value.
- Multi-row Subquery: Returns multiple rows, used when the outer query expects multiple values.
Subquery in the SELECT Clause
You can use a subquery in the SELECT
clause to retrieve a value from a related table for each row in the outer query.
Syntax:
-- Retrieve employee names and their department's average salary using a subquery in the SELECT clause
SELECT employees.name,
(SELECT AVG(salary) FROM employees WHERE department_id = employees.department_id) AS avg_salary
FROM employees;
This query retrieves the name of each employee along with the average salary of their department. The subquery calculates the average salary for each department, and the outer query retrieves the data for each employee.
Subquery in the WHERE Clause
Subqueries in the WHERE
clause allow you to filter records based on the result of another query. This is useful when you need to filter data based on a condition that involves another table.
Syntax:
-- Retrieve employees whose salary is above the average salary of their department
SELECT name, salary
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = employees.department_id);
This query uses a subquery to find employees who earn more than the average salary in their department. The subquery calculates the average salary for each department and compares it to the employee’s salary.
Subquery in the FROM Clause
Subqueries in the FROM
clause are used when the result of a subquery is treated as a temporary table. This allows you to manipulate the result of the subquery as if it were a regular table.
Syntax:
-- Retrieve department names along with the average salary of employees in each department
SELECT department_name, avg_salary
FROM (SELECT department_id, AVG(salary) AS avg_salary
FROM employees
GROUP BY department_id) AS dept_avg_salaries
JOIN departments
ON dept_avg_salaries.department_id = departments.department_id;
This query calculates the average salary for each department in a subquery and then uses that result as a temporary table to join with the departments
table and retrieve the department name along with the average salary.
Subquery with EXISTS
The EXISTS
operator is used in subqueries to check for the existence of rows returned by the subquery. It returns TRUE
if the subquery returns one or more rows, and FALSE
otherwise.
Syntax:
-- Retrieve employees who are assigned to at least one project
SELECT name
FROM employees
WHERE EXISTS (SELECT 1 FROM projects WHERE projects.employee_id = employees.employee_id);
This query checks if an employee is assigned to any project by using the EXISTS
operator. If the subquery returns any rows (i.e., if the employee is assigned to a project), the employee's name is included in the results.
Subquery with IN
The IN
operator is used to compare a value against a list of values returned by a subquery. It is commonly used to filter rows based on a list of values from another table.
Syntax:
-- Retrieve employees who work in departments with more than 10 employees
SELECT name
FROM employees
WHERE department_id IN (SELECT department_id FROM employees GROUP BY department_id HAVING COUNT(*) > 10);
This query retrieves employees who belong to departments with more than 10 employees. The subquery finds the departments that have more than 10 employees, and the outer query retrieves the employees working in those departments.
Correlated Subqueries
A correlated subquery is a subquery that references columns from the outer query. Unlike a regular subquery, which is executed once for the entire outer query, a correlated subquery is executed once for each row processed by the outer query.
Syntax:
-- Retrieve employees whose salary is higher than the average salary in their department
SELECT name, salary
FROM employees e1
WHERE salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
This query finds employees who earn more than the average salary in their department. The subquery is correlated because it references the department_id
from the outer query (alias e1
) in order to compute the average salary for each department.
Diagram: Subqueries in SQL
The following diagram illustrates how a subquery works inside another query:

This diagram shows how a subquery is nested within a larger query and how the outer query uses the result of the subquery to perform its operations.
Primary Key, Foreign Key, and Unique Key Constraints
In MySQL, constraints are used to define rules for the data in a table. They help maintain data integrity and ensure that the data entered into the database is valid. The three common types of key constraints are Primary Key, Foreign Key, and Unique Key.
Primary Key
A Primary Key uniquely identifies each record in a table. It must contain unique values, and it cannot contain NULL
values. Every table can have only one primary key.
Syntax:
-- Create a table with a primary key constraint
CREATE TABLE employees (
employee_id INT NOT NULL,
name VARCHAR(100),
department_id INT,
PRIMARY KEY (employee_id)
);
In this example, employee_id
is the primary key, which ensures that each employee has a unique identifier. The employee_id
cannot have NULL
values.
Foreign Key
A Foreign Key is a column or a set of columns in one table that references the primary key of another table. Foreign keys are used to create relationships between tables and ensure data consistency. A foreign key constraint ensures that the value in the foreign key column matches a value in the referenced primary key column.
Syntax:
-- Create a table with a foreign key constraint
CREATE TABLE departments (
department_id INT NOT NULL,
department_name VARCHAR(100),
PRIMARY KEY (department_id)
);
CREATE TABLE employees (
employee_id INT NOT NULL,
name VARCHAR(100),
department_id INT,
PRIMARY KEY (employee_id),
FOREIGN KEY (department_id) REFERENCES departments(department_id)
);
In this example, the employees
table has a foreign key department_id
that references the department_id
column in the departments
table. This ensures that every employee is assigned to a valid department.
Unique Key
A Unique Key constraint ensures that all values in a column or a group of columns are unique across all rows in the table. Unlike a primary key, a table can have multiple unique keys, and unique keys can accept NULL
values, but only one NULL
value per column.
Syntax:
-- Create a table with a unique key constraint
CREATE TABLE users (
user_id INT NOT NULL,
username VARCHAR(100) UNIQUE,
email VARCHAR(100) UNIQUE,
PRIMARY KEY (user_id)
);
In this example, the username
and email
columns have unique constraints, ensuring that no two users can have the same username or email address. The user_id
is the primary key.
Differences Between Primary Key, Foreign Key, and Unique Key
Constraint | Explanation |
---|---|
Primary Key | Used to uniquely identify each record in a table. Cannot contain NULL values. Only one primary key per table. |
Foreign Key | Used to create a relationship between two tables. A foreign key in one table points to the primary key in another table. |
Unique Key | Ensures that all values in a column are unique. Can contain NULL values, but only one NULL value per column. |
Modifying Constraints
You can also add or drop constraints after a table has been created:
Adding a Foreign Key Constraint:
-- Add a foreign key constraint to an existing table
ALTER TABLE employees
ADD CONSTRAINT fk_department
FOREIGN KEY (department_id) REFERENCES departments(department_id);
Dropping a Foreign Key Constraint:
-- Drop a foreign key constraint from a table
ALTER TABLE employees
DROP FOREIGN KEY fk_department;
Diagram: Key Constraints
The following diagram shows how primary keys, foreign keys, and unique keys work in a relational database:

This diagram visualizes how the primary key uniquely identifies records in a table, how foreign keys link tables together, and how unique keys ensure the uniqueness of data in a column.
NOT NULL and Default Values
In MySQL, constraints like NOT NULL and DEFAULT are used to ensure data integrity by controlling how data is inserted into a table. These constraints are used to define rules for data entries in a column.
NOT NULL Constraint
The NOT NULL constraint ensures that a column cannot have a NULL
value. This is useful when a column is mandatory and must always have a value. If a column is defined as NOT NULL
, then attempting to insert a row without a value for that column will result in an error.
Syntax:
-- Create a table with a NOT NULL constraint
CREATE TABLE employees (
employee_id INT NOT NULL,
name VARCHAR(100) NOT NULL,
department_id INT NOT NULL,
PRIMARY KEY (employee_id)
);
In this example, the name
and department_id
columns are both defined with the NOT NULL
constraint, meaning that every employee must have a name and be assigned to a department.
Default Values
The DEFAULT constraint is used to assign a default value to a column when no value is provided during an insert operation. If a value is not supplied for the column, the default value is used instead. The default value can be a constant, such as a string, number, or date.
Syntax:
-- Create a table with default values
CREATE TABLE orders (
order_id INT NOT NULL AUTO_INCREMENT,
product_name VARCHAR(100) NOT NULL,
order_date DATE DEFAULT CURRENT_DATE,
status VARCHAR(50) DEFAULT 'Pending',
PRIMARY KEY (order_id)
);
In this example, the order_date
column has a default value of CURRENT_DATE
, which inserts the current date if no date is specified. The status
column has a default value of 'Pending'
, which is used if no status is provided when the order is created.
Combining NOT NULL and DEFAULT Constraints
You can use both NOT NULL and DEFAULT constraints in the same column. This is useful when you want to ensure that a column cannot be NULL
, but also provide a default value to avoid errors when a value is not supplied.
Example:
-- Create a table with both NOT NULL and DEFAULT constraints
CREATE TABLE students (
student_id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
enrollment_status VARCHAR(20) NOT NULL DEFAULT 'Enrolled',
PRIMARY KEY (student_id)
);
In this example, the name
column is required (NOT NULL), and the enrollment_status
column has a default value of 'Enrolled'
. If no enrollment status is specified, the default value will be used, ensuring that the column cannot be left empty.
Modifying the NOT NULL and Default Values
You can modify existing columns to add or remove the NOT NULL
constraint or change the default value. This is useful when you need to update your table schema.
Syntax for Modifying the NOT NULL Constraint:
-- Modify a column to set it as NOT NULL
ALTER TABLE employees
MODIFY COLUMN name VARCHAR(100) NOT NULL;
Syntax for Changing the Default Value:
-- Modify a column to change its default value
ALTER TABLE orders
ALTER COLUMN status SET DEFAULT 'Shipped';
Diagram: NOT NULL and Default Values
The following diagram shows how NOT NULL and default values work in MySQL:

This diagram visualizes how NOT NULL ensures that a column must contain a value, and how default values are automatically used when no value is provided during an insert operation.
Indexing and Performance Optimization
Indexing is a technique used in databases to improve the speed of data retrieval operations. It is especially useful when dealing with large datasets. MySQL provides various types of indexes to optimize query performance. In this section, we will discuss indexing, how it works, and best practices for optimizing performance.
What is Indexing?
An index is a data structure that improves the speed of data retrieval operations on a database table at the cost of additional space and maintenance overhead. Indexes allow MySQL to find rows more efficiently, particularly during SELECT
queries with WHERE
, JOIN
, and ORDER BY
clauses.
Types of Indexes
MySQL supports several types of indexes that can be used to optimize performance:
- Primary Index: Automatically created when a primary key is defined. It ensures that rows are unique and can be accessed quickly.
- Unique Index: Ensures that all values in the indexed column are unique. It is created when a
UNIQUE
constraint is applied to a column. - Full-text Index: Used for text searching and is typically applied to
TEXT
columns. - Composite Index: An index on multiple columns. It is useful when queries frequently filter on multiple columns.
Creating Indexes
To create an index in MySQL, you can use the CREATE INDEX
statement or define the index while creating the table. Here is the syntax:
Syntax:
-- Create a simple index on a single column
CREATE INDEX idx_name ON employees (name);
-- Create a composite index on multiple columns
CREATE INDEX idx_department_name ON employees (department_id, name);
In the above example, the idx_name
index is created on the name
column of the employees
table, and the idx_department_name
index is created on both department_id
and name
columns for more optimized searches on both fields.
When to Use Indexes?
Indexes are beneficial when used in the following scenarios:
- When you frequently query a table by a column (e.g., using
WHERE
,JOIN
, orORDER BY
clauses). - When you need to enforce uniqueness on a column.
- For optimizing read-heavy workloads, where data retrieval speed is critical.
However, indexes come with trade-offs. While they speed up data retrieval, they also slow down data modification operations (such as INSERT
, UPDATE
, and DELETE
) due to the need to maintain the index.
Drop Indexes
If an index is no longer needed, you can drop it using the DROP INDEX
statement:
Syntax:
-- Drop an index
DROP INDEX idx_name ON employees;
Performance Optimization Tips
Here are some best practices for optimizing the performance of your MySQL database:
- Use Indexes Wisely: Indexes should be used on columns that are frequently used in
WHERE
,JOIN
, orORDER BY
clauses. Avoid over-indexing, as it can slow down write operations. - Optimize Queries: Analyze and optimize your queries to ensure that they use indexes effectively. Use
EXPLAIN
to check how MySQL is executing a query. - Use Proper Data Types: Choose the most efficient data type for your columns. For example, use
INT
for integer values rather thanBIGINT
if the range of values doesn’t require it. - Use Caching: Enable query caching to store the results of frequently executed queries. This reduces the load on the database and speeds up subsequent requests.
- Partitioning: For very large tables, consider partitioning them based on certain criteria (e.g., by date or region) to improve query performance.
Using EXPLAIN to Analyze Queries
The EXPLAIN
statement helps you understand how MySQL executes your queries and whether it’s using indexes effectively. Here’s an example:
Syntax:
-- Use EXPLAIN to analyze a query
EXPLAIN SELECT * FROM employees WHERE department_id = 1;
The result of the EXPLAIN
query will show whether the query uses an index and how MySQL plans to execute the query. Look for the key
column in the output to see which index is being used.
Diagram: Indexing and Query Optimization
The following diagram shows how indexes improve query performance by allowing MySQL to search for rows more efficiently:

This diagram visualizes the impact of indexing on query performance, illustrating the difference between searching with and without indexes.
Foreign Key Relationships and Referential Integrity
In relational databases, foreign keys are used to establish a link between two tables. A foreign key in one table points to the primary key of another table, creating a relationship between the two tables. This ensures that data remains consistent and that relationships between tables are maintained. In this section, we will discuss foreign key relationships, referential integrity, and how to define and manage foreign keys in MySQL.
What is a Foreign Key?
A foreign key is a column (or a set of columns) in a table that is used to establish a link between the data in two tables. It is a reference to the primary key or a unique key in another table. Foreign keys help maintain referential integrity, ensuring that the data in the database remains consistent and accurate.
Referential Integrity
Referential integrity is a concept in relational database design that ensures the consistency and accuracy of data by enforcing relationships between tables. When referential integrity is maintained, it ensures that one table’s foreign key values match valid primary key values in another table, or that the foreign key values are null if allowed.
Referential integrity is important because it prevents actions that would leave orphaned records in the database, ensuring that relationships between tables are meaningful.
Creating Foreign Key Relationships
You can define foreign keys when creating a table or by altering an existing table. Below is the syntax for creating a foreign key relationship in MySQL:
Syntax:
-- Creating a table with a foreign key relationship
CREATE TABLE orders (
order_id INT AUTO_INCREMENT PRIMARY KEY,
customer_id INT,
order_date DATE,
FOREIGN KEY (customer_id) REFERENCES customers(customer_id)
);
-- Adding a foreign key to an existing table
ALTER TABLE orders
ADD CONSTRAINT fk_customer_id
FOREIGN KEY (customer_id) REFERENCES customers(customer_id);
In the example above, the orders
table has a customer_id
column that is a foreign key referencing the customer_id
column of the customers
table. This ensures that each order is associated with a valid customer.
Foreign Key Constraints
When defining a foreign key, you can specify various actions that should be taken when data in the referenced table is modified. The actions are defined using the ON DELETE
and ON UPDATE
clauses:
- CASCADE: If a record in the parent table is deleted or updated, the corresponding records in the child table are also deleted or updated.
- SET NULL: If a record in the parent table is deleted or updated, the foreign key in the child table is set to
NULL>.
- NO ACTION: The default behavior. If a record in the parent table is deleted or updated, and it would violate the foreign key constraint, the operation is not allowed.
- RESTRICT: Similar to
NO ACTION
, it prevents the deletion or update of a record in the parent table if any child records exist.
Syntax:
-- Creating a foreign key with actions on update and delete
CREATE TABLE orders (
order_id INT AUTO_INCREMENT PRIMARY KEY,
customer_id INT,
order_date DATE,
FOREIGN KEY (customer_id)
REFERENCES customers(customer_id)
ON DELETE CASCADE
ON UPDATE CASCADE
);
In the above example, if a customer is deleted from the customers
table, all orders associated with that customer will also be deleted due to the ON DELETE CASCADE
clause. Similarly, if a customer's ID is updated, the customer ID in the orders
table will also be updated.
Diagram: Foreign Key Relationship
The following diagram illustrates the relationship between the customers
and orders
tables:

This diagram shows that the customer_id
column in the orders
table is a foreign key referencing the customer_id
column in the customers
table, creating a one-to-many relationship between customers and their orders.
Foreign Key Violations
If referential integrity is violated (e.g., by attempting to delete a customer that still has associated orders), MySQL will prevent the action from being performed. Foreign key violations are errors that occur when a foreign key constraint is violated, such as:
- Inserting a record in the child table with a foreign key value that does not exist in the parent table.
- Deleting or updating a record in the parent table that is referenced by a record in the child table, and no proper action is defined (e.g., CASCADE or SET NULL).
To avoid foreign key violations, always ensure that the data in the child table correctly references a valid primary key in the parent table, and choose the correct actions for ON DELETE
and ON UPDATE
.
Checking Constraints and Field Validation
In relational databases, constraints are rules that ensure the integrity of the data stored in tables. Constraints can be used to enforce validation on the data being inserted or updated in a table, ensuring that the data adheres to specific rules. MySQL provides several types of constraints, including CHECK, NOT NULL, DEFAULT, and others. In this section, we will focus on the CHECK constraint and how to perform field validation in MySQL.
What are Constraints?
Constraints are rules that limit the type of data that can be inserted or updated in a table. These rules help maintain data accuracy, integrity, and reliability. Constraints can be applied on columns or tables and can restrict various actions, such as disallowing null values, enforcing unique values, and setting ranges for numerical data.
CHECK Constraint
The CHECK constraint is used to limit the range of values that can be inserted into a column. It ensures that the values in a column satisfy a given condition. If a value does not meet the specified condition, the database will reject the operation.
In MySQL, the CHECK
constraint was introduced in MySQL 8.0.16, and it allows you to specify conditions for column values.
Syntax for CHECK Constraint:
-- Creating a table with a CHECK constraint
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
age INT,
salary DECIMAL(10, 2),
CHECK (age >= 18), -- Ensures that the age is at least 18
CHECK (salary > 0) -- Ensures that the salary is greater than zero
);
In the example above, the CHECK
constraint ensures that the age
column contains only values greater than or equal to 18, and the salary
column only contains positive values.
Field Validation with NOT NULL
One of the most common field validation constraints is NOT NULL. This constraint ensures that a column cannot contain NULL
values, forcing users to provide a value for that column when inserting or updating a record.
Syntax for NOT NULL Constraint:
-- Creating a table with NOT NULL constraint
CREATE TABLE products (
product_id INT PRIMARY KEY,
product_name VARCHAR(100) NOT NULL, -- Product name cannot be NULL
price DECIMAL(10, 2) NOT NULL -- Price cannot be NULL
);
In this example, the product_name
and price
columns are defined with the NOT NULL
constraint, meaning that every product record must have values for both fields.
Default Values for Fields
The DEFAULT constraint is used to provide a default value for a column when no value is specified during an insert operation. This ensures that fields are always populated with a valid value, even if the user does not explicitly provide one.
Syntax for DEFAULT Constraint:
-- Creating a table with a DEFAULT constraint
CREATE TABLE orders (
order_id INT PRIMARY KEY,
order_date DATE DEFAULT CURRENT_DATE, -- Default value is the current date
status VARCHAR(20) DEFAULT 'Pending' -- Default value is 'Pending'
);
In the orders
table example, the order_date
column will default to the current date if no date is provided, and the status
column will default to 'Pending'.
Field Validation with UNIQUE
The UNIQUE constraint ensures that the values in a column (or a combination of columns) are unique across all rows in a table. This is useful for fields that should not have duplicate values, such as email addresses or usernames.
Syntax for UNIQUE Constraint:
-- Creating a table with UNIQUE constraint
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50) UNIQUE, -- Ensures that each username is unique
email VARCHAR(100) UNIQUE -- Ensures that each email is unique
);
In this example, the username
and email
columns are defined with the UNIQUE
constraint to prevent duplicate usernames or emails from being inserted into the users
table.
Validating Data with CHECK Constraints
You can perform more complex data validation with CHECK constraints. For example, if you need to ensure that a column value falls within a specific range, you can use the CHECK
constraint to enforce that:
Example: Validating Age Range
-- Creating a table with a CHECK constraint for age validation
CREATE TABLE students (
student_id INT PRIMARY KEY,
name VARCHAR(50),
age INT,
CHECK (age >= 18 AND age <= 30) -- Ensures age is between 18 and 30
);
In the example above, the CHECK
constraint ensures that the age
column only accepts values between 18 and 30, inclusive.
Diagram: Field Validation and Constraints
The following diagram illustrates the relationship between different constraints applied on columns in a table:

This diagram shows how different constraints like NOT NULL
, DEFAULT
, CHECK
, and UNIQUE
are applied to ensure data integrity and validation during data insertion and updating.
Complex JOINs (JOINs with Multiple Tables)
In MySQL, complex JOIN operations allow you to combine data from multiple tables based on related columns. These operations are essential for retrieving and analyzing data from normalized databases. Complex JOINs typically involve joining more than two tables in a single query to extract data that is spread across multiple related tables.
Types of Complex JOINs
Complex JOINs generally refer to queries that involve more than one INNER JOIN, LEFT JOIN, RIGHT JOIN, or even a mix of different types of JOINs to retrieve data from several tables. By combining multiple JOINs, you can create more sophisticated queries that provide deeper insights into your data.
Example of Complex JOIN with Multiple Tables
Consider the following example, where we have three tables: employees
, departments
, and projects
. Each table is related to the others through foreign keys:
Table Structure:
-- Table: employees
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(100),
department_id INT,
FOREIGN KEY (department_id) REFERENCES departments(department_id)
);
-- Table: departments
CREATE TABLE departments (
department_id INT PRIMARY KEY,
department_name VARCHAR(100)
);
-- Table: projects
CREATE TABLE projects (
project_id INT PRIMARY KEY,
project_name VARCHAR(100),
employee_id INT,
FOREIGN KEY (employee_id) REFERENCES employees(employee_id)
);
In this example, employees
is linked to departments
through the department_id
column, and projects
is linked to employees
through the employee_id
column.
Complex JOIN Query
To retrieve the list of employees, their department names, and the names of the projects they are working on, we can perform a complex JOIN across all three tables:
Query:
-- Complex JOIN with multiple tables
SELECT
e.employee_name,
d.department_name,
p.project_name
FROM
employees e
JOIN
departments d ON e.department_id = d.department_id
JOIN
projects p ON e.employee_id = p.employee_id;
This query uses two INNER JOINs to combine the employees
, departments
, and projects
tables. The first JOIN
links employees
to departments
based on the department_id
, and the second JOIN
links employees
to projects
based on the employee_id
.
Using Different Types of JOINs Together
Sometimes, you may need to use different types of JOINs in a single query to get the desired results. Here is an example using both LEFT JOIN and INNER JOIN:
Query:
-- Complex JOIN with LEFT JOIN and INNER JOIN
SELECT
e.employee_name,
d.department_name,
p.project_name
FROM
employees e
LEFT JOIN
departments d ON e.department_id = d.department_id
INNER JOIN
projects p ON e.employee_id = p.employee_id;
In this example, we use a LEFT JOIN between employees
and departments
to include all employees even if they do not belong to a department. We also use an INNER JOIN between employees
and projects
, ensuring that only employees who are working on a project will be included in the result set.
Example with More Than Three Tables
You can also join more than three tables in a single query. Consider a fourth table, clients
, that holds information about the clients associated with each project. The projects
table has a foreign key reference to the clients
table.
Table Structure:
-- Table: clients
CREATE TABLE clients (
client_id INT PRIMARY KEY,
client_name VARCHAR(100)
);
Now we can write a complex query to get the employee names, project names, department names, and client names:
Query:
-- Complex JOIN with more than three tables
SELECT
e.employee_name,
d.department_name,
p.project_name,
c.client_name
FROM
employees e
JOIN
departments d ON e.department_id = d.department_id
JOIN
projects p ON e.employee_id = p.employee_id
JOIN
clients c ON p.client_id = c.client_id;
In this query, we use four tables: employees
, departments
, projects
, and clients
. The JOIN operations link these tables together to retrieve data from all of them in one query.
Diagram: Complex JOINs with Multiple Tables
The following diagram illustrates how different tables are joined together to create a complex query:

The diagram visually represents the relationships between the employees
, departments
, projects
, and clients
tables, showing how data is combined through JOINs.
Self-joins and Recursive Queries
A self-join is a query that joins a table to itself, which can be useful when you need to find relationships within the same table. Recursive queries, on the other hand, allow you to retrieve data that is hierarchically structured, such as organizational charts or folder structures. In MySQL, recursive queries are typically implemented using Common Table Expressions (CTEs).
Self-Join
A self-join is a type of join where a table is joined with itself. This is usually done when a table contains hierarchical data, such as an employee structure where an employee has a manager who is also an employee.
Example of Self-Join
Consider a table called employees
that contains information about employees and their managers. Each employee record has a manager_id
that references another employee in the same table. To find the names of employees along with their manager names, we can perform a self-join:
Table Structure:
-- Table: employees
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(100),
manager_id INT,
FOREIGN KEY (manager_id) REFERENCES employees(employee_id)
);
Now, let's assume that the manager_id
column refers to the employee_id
of the employee's manager. To list employees along with their managers, we perform a self-join:
Query:
-- Self-join to get employees and their managers
SELECT
e.employee_name AS Employee,
m.employee_name AS Manager
FROM
employees e
LEFT JOIN
employees m ON e.manager_id = m.employee_id;
In this query, we are joining the employees
table to itself. The alias e
represents the employees, while m
represents the managers. The LEFT JOIN
ensures that even employees without managers (i.e., top-level employees) are included in the result.
Recursive Queries
Recursive queries are useful for querying hierarchical data, where a record references itself or another record in the same table. In MySQL, recursive queries are achieved using Common Table Expressions (CTEs) and the WITH RECURSIVE
clause.
Example of Recursive Query
Imagine we have an organization
table that stores an employee's ID and their direct reports. We want to retrieve all employees who report to a specific manager, including indirect reports (i.e., employees who report to employees who report to the manager).
Table Structure:
-- Table: organization
CREATE TABLE organization (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(100),
manager_id INT,
FOREIGN KEY (manager_id) REFERENCES organization(employee_id)
);
The organization
table has a manager_id
column that refers to an employee's manager. To find all employees who report to a specific manager (directly or indirectly), we use a recursive query.
Recursive Query:
-- Recursive query to find all employees reporting to a specific manager
WITH RECURSIVE employee_hierarchy AS (
-- Base case: Select employees directly reporting to the manager
SELECT employee_id, employee_name, manager_id
FROM organization
WHERE manager_id = 1 -- Replace with the manager's ID
UNION ALL
-- Recursive case: Select employees reporting to those in the previous result
SELECT o.employee_id, o.employee_name, o.manager_id
FROM organization o
INNER JOIN employee_hierarchy eh ON o.manager_id = eh.employee_id
)
SELECT * FROM employee_hierarchy;
In this query:
- The
WITH RECURSIVE
clause defines a Common Table Expression (CTE) calledemployee_hierarchy
. - The base case (the first
SELECT
statement) retrieves employees who report directly to the manager (withmanager_id = 1
in this case). - The recursive case (the second
SELECT
statement) selects employees who report to the employees found in the previous step, thus building the hierarchy recursively. - The result will include all employees who report to the specified manager, either directly or indirectly.
Diagram: Recursive Query
The following diagram illustrates how the recursive query builds a hierarchical structure:

The diagram shows the relationships between employees and their managers, and how a recursive query can retrieve all employees in the hierarchy starting from a specific manager.
Using UNION and INTERSECT
The UNION and INTERSECT operators are used in SQL to combine the results of two or more queries. These operators allow you to perform set operations on the result sets of different SELECT queries.
UNION
The UNION operator is used to combine the result sets of two or more SELECT
queries. It returns all distinct records from the result sets. If you want to include duplicates, you can use the UNION ALL
operator.
Example of UNION
Consider two tables, employees
and contractors
, both containing a list of people working for the company. You want to combine the results of both tables to get a list of all people working for the company.
Table Structure:
-- Table: employees
CREATE TABLE employees (
id INT PRIMARY KEY,
name VARCHAR(100)
);
-- Table: contractors
CREATE TABLE contractors (
id INT PRIMARY KEY,
name VARCHAR(100)
);
To get a combined list of employees and contractors, we can use the UNION
operator:
Query:
-- Using UNION to combine employees and contractors
SELECT name FROM employees
UNION
SELECT name FROM contractors;
This query retrieves distinct names from both the employees
and contractors
tables. The UNION
operator ensures that there are no duplicate names in the final result.
Using UNION ALL
If you want to include duplicates in the result, use UNION ALL
instead:
Query:
-- Using UNION ALL to include duplicates
SELECT name FROM employees
UNION ALL
SELECT name FROM contractors;
INTERSECT
The INTERSECT operator returns only the common records between two SELECT
queries. It is used to find the intersection of two result sets, i.e., rows that appear in both queries.
Example of INTERSECT
Consider the same employees
and contractors
tables, but now you want to find the people who appear in both tables—those who are both employees and contractors.
Query:
-- Using INTERSECT to find common names in both tables
SELECT name FROM employees
INTERSECT
SELECT name FROM contractors;
The result will include only the names that are present in both the employees
and contractors
tables. If a name appears in one table but not the other, it will not be included in the result.
Diagram: UNION and INTERSECT
The following Venn diagram illustrates the difference between UNION
and INTERSECT
:

In this diagram:
- The
UNION
operator combines all distinct records from both result sets (the entire area of both circles). - The
INTERSECT
operator only returns the records that are common to both result sets (the overlapping region of both circles).
Full-text Search in MySQL
MySQL provides full-text search capabilities that allow you to perform more advanced and efficient text searches on string columns. Full-text search in MySQL is typically used for searching large amounts of text data, such as articles, blog posts, and product descriptions, and it can be much faster than using the LIKE
operator.
Full-Text Index
To enable full-text search, you need to create a FULLTEXT index on the columns you want to search. MySQL supports full-text indexing for CHAR
, VARCHAR
, and TEXT
columns. Full-text indexes allow MySQL to perform text searches much more efficiently.
Creating a Full-text Index
Here is an example of how to create a FULLTEXT
index on a description
column of a products
table:
Table Structure:
-- Table: products
CREATE TABLE products (
id INT PRIMARY KEY,
name VARCHAR(100),
description TEXT,
FULLTEXT(description)
);
The FULLTEXT(description)
statement creates a full-text index on the description
column, which will allow you to search the text data in this column more efficiently.
Searching with MATCH...AGAINST
Once a full-text index has been created, you can use the MATCH
and AGAINST
operators to perform full-text searches. The MATCH
operator is used to specify the columns you want to search, and the AGAINST
operator is used to define the search query.
Example Query: Searching for Products
If you want to search for products that match a specific keyword in their description, you can use the following query:
-- Searching for products with the word "wireless" in the description
SELECT name, description
FROM products
WHERE MATCH(description) AGAINST('wireless');
This query will return all products whose description
contains the word "wireless". The MATCH...AGAINST
operator performs a full-text search on the description
column and returns the rows where the search term is found.
Boolean Mode Search
In addition to the natural language search, you can perform more advanced searches using Boolean mode. Boolean mode allows you to use operators like +
(must include), -
(must exclude), and *
(wildcard) in your search query.
Example: Boolean Mode Search
If you want to search for products that must include the word "wireless" but exclude the word "headphones", you can use the following query:
-- Searching for products with "wireless" but not "headphones"
SELECT name, description
FROM products
WHERE MATCH(description) AGAINST('+wireless -headphones' IN BOOLEAN MODE);
This query will return products whose description
contains the word "wireless" but does not contain the word "headphones". The +wireless
indicates that the word "wireless" must be included, and the -headphones
indicates that the word "headphones" must be excluded.
Full-Text Search with Relevance
MySQL also allows you to rank the search results by relevance. The relevance score indicates how well the search term matches the content. You can retrieve the relevance score using the MATCH...AGAINST
query.
Example: Relevance Score
To retrieve the products along with their relevance score, you can use the following query:
-- Searching for products and displaying relevance score
SELECT name, description, MATCH(description) AGAINST('wireless') AS relevance
FROM products
WHERE MATCH(description) AGAINST('wireless');
The relevance
column will display the relevance score for each product, with higher scores indicating better matches to the search term.
Diagram: Full-Text Search Process
The following diagram explains the process of performing a full-text search in MySQL:

In this diagram, you can see how MySQL performs the full-text search by first creating a full-text index on the column, then executing a query with the MATCH...AGAINST
operator to retrieve relevant results.
Handling NULL Values in MySQL
NULL values in MySQL represent missing or unknown data. Handling NULL values is an essential part of working with relational databases, as they can appear in any column and affect queries and calculations. MySQL provides several functions to handle NULL values effectively.
Understanding NULL
In MySQL, NULL is used to signify that a value is absent, unknown, or undefined. It is not the same as zero or an empty string. NULL can appear in any column of a table, and its behavior must be handled carefully in SQL queries to avoid errors or unexpected results.
IS NULL Operator
The IS NULL
operator is used to check whether a column contains a NULL value. It is commonly used in WHERE
clauses to filter rows where a column is NULL.
Example: Using IS NULL
This query retrieves rows where the email
column is NULL, indicating that the email address is missing:
-- Retrieving rows with NULL email addresses
SELECT id, name, email
FROM users
WHERE email IS NULL;
IS NOT NULL Operator
The IS NOT NULL
operator is used to filter rows where a column does not contain NULL values.
Example: Using IS NOT NULL
This query retrieves rows where the email
column is not NULL, meaning that the email address is provided:
-- Retrieving rows with non-NULL email addresses
SELECT id, name, email
FROM users
WHERE email IS NOT NULL;
COALESCE Function
The COALESCE
function is used to return the first non-NULL value in a list of arguments. It is useful when you want to replace NULL values with a default value.
Example: Using COALESCE
If you want to retrieve the user's email address or a default value (e.g., "No email provided") if the email is NULL, you can use the COALESCE
function:
-- Retrieving email or default value if NULL
SELECT name, COALESCE(email, 'No email provided') AS email
FROM users;
In this example, if the email
column contains NULL, the query will return "No email provided" instead of NULL.
IFNULL Function
The IFNULL
function is similar to COALESCE
, but it only accepts two arguments. It returns the first argument if it is not NULL, and the second argument if the first argument is NULL.
Example: Using IFNULL
This query uses IFNULL
to replace NULL values in the email
column with the default string "Unknown email":
-- Retrieving email or default value using IFNULL
SELECT name, IFNULL(email, 'Unknown email') AS email
FROM users;
In this case, if the email
column is NULL, "Unknown email" will be returned.
Diagram: Handling NULL Values
The following diagram demonstrates how NULL values are handled using the IS NULL
, COALESCE
, and IFNULL
functions:

The diagram explains how MySQL processes NULL values and how these functions can be used to handle and replace NULL values in queries.
String Functions in MySQL
MySQL provides several string functions that allow you to manipulate and transform string data in various ways. These functions are essential for working with textual data and can help in tasks such as concatenation, extraction, and replacement of substrings.
CONCAT() Function
The CONCAT()
function is used to concatenate two or more strings together. If any of the arguments is NULL, the function returns NULL.
Example: Using CONCAT()
This query combines the first name and last name of a user into a single string:
-- Concatenating first name and last name
SELECT CONCAT(first_name, ' ', last_name) AS full_name
FROM users;
In this example, the first name and last name are combined with a space in between.
SUBSTRING() Function
The SUBSTRING()
function is used to extract a substring from a string. You can specify the starting position and the length of the substring.
Example: Using SUBSTRING()
This query extracts the first three characters from the email
column:
-- Extracting the first three characters of the email
SELECT SUBSTRING(email, 1, 3) AS email_prefix
FROM users;
Here, the substring starting from position 1 and consisting of 3 characters is extracted from the email address.
REPLACE() Function
The REPLACE()
function is used to replace all occurrences of a substring within a string with another substring.
Example: Using REPLACE()
This query replaces all occurrences of the word "old" with "new" in the description
column:
-- Replacing occurrences of 'old' with 'new' in description
SELECT REPLACE(description, 'old', 'new') AS updated_description
FROM products;
In this example, every instance of the word "old" in the description
column will be replaced with the word "new".
UPPER() and LOWER() Functions
The UPPER()
and LOWER()
functions are used to convert a string to uppercase and lowercase, respectively.
Example: Using UPPER() and LOWER()
This query converts the name
column to uppercase and lowercase:
-- Converting name to uppercase and lowercase
SELECT UPPER(name) AS upper_name, LOWER(name) AS lower_name
FROM users;
In this case, the UPPER()
function converts the name
column values to uppercase, while the LOWER()
function converts them to lowercase.
LENGTH() Function
The LENGTH()
function returns the length of a string in characters.
Example: Using LENGTH()
This query retrieves the length of the description
column:
-- Retrieving the length of the description
SELECT LENGTH(description) AS description_length
FROM products;
This query will return the number of characters in the description
column for each row.
TRIM() Function
The TRIM()
function is used to remove leading and trailing spaces from a string.
Example: Using TRIM()
This query removes any leading and trailing spaces from the username
column:
-- Removing leading and trailing spaces from username
SELECT TRIM(username) AS trimmed_username
FROM users;
In this case, any extra spaces before or after the username
are removed.
Diagram: String Functions in MySQL
The following diagram demonstrates how various string functions, such as CONCAT()
, SUBSTRING()
, and REPLACE()
, work to manipulate string data:

This diagram visually explains how the string functions transform the input data.
Date and Time Functions in MySQL
MySQL provides several date and time functions that allow you to manipulate and work with date and time values. These functions are essential for performing operations such as getting the current date and time, adding or subtracting time intervals, and calculating the difference between dates.
NOW() Function
The NOW()
function returns the current date and time in the format YYYY-MM-DD HH:MM:SS
.
Example: Using NOW()
This query retrieves the current date and time:
-- Retrieving the current date and time
SELECT NOW() AS current_datetime;
The NOW()
function returns the current date and time when the query is executed.
DATE_ADD() Function
The DATE_ADD()
function is used to add a time interval to a date or datetime value. You can specify the interval to be added in terms of days, months, years, etc.
Example: Using DATE_ADD()
This query adds 10 days to the current date:
-- Adding 10 days to the current date
SELECT DATE_ADD(NOW(), INTERVAL 10 DAY) AS new_date;
The DATE_ADD()
function adds 10 days to the current date and time returned by NOW()
.
DATE_SUB() Function
The DATE_SUB()
function is used to subtract a time interval from a date or datetime value. Similar to DATE_ADD()
, you can specify the interval to be subtracted.
Example: Using DATE_SUB()
This query subtracts 5 days from the current date:
-- Subtracting 5 days from the current date
SELECT DATE_SUB(NOW(), INTERVAL 5 DAY) AS new_date;
In this example, 5 days are subtracted from the current date and time.
DATEDIFF() Function
The DATEDIFF()
function returns the number of days between two dates. It calculates the difference between two date values, and the result is returned as an integer.
Example: Using DATEDIFF()
This query calculates the number of days between two dates:
-- Calculating the number of days between two dates
SELECT DATEDIFF('2025-01-31', '2025-01-01') AS days_difference;
In this example, the difference between January 31, 2025, and January 1, 2025, is calculated as 30 days.
Diagram: Date and Time Functions in MySQL
The following diagram illustrates how date and time functions such as NOW()
, DATE_ADD()
, and DATE_SUB()
work to manipulate date and time values:

This diagram visually explains how date and time functions transform the input data.
Numeric Functions in MySQL
MySQL provides a variety of numeric functions that are used to perform mathematical calculations and operations on numbers. These functions are crucial for tasks such as rounding, truncating, and performing basic arithmetic operations.
ROUND() Function
The ROUND()
function is used to round a number to a specified number of decimal places. If no decimal places are specified, the number will be rounded to the nearest integer.
Example: Using ROUND()
This query rounds the number 123.4567 to two decimal places:
-- Rounding a number to two decimal places
SELECT ROUND(123.4567, 2) AS rounded_value;
The ROUND()
function rounds the number 123.4567 to 123.46 when rounded to two decimal places.
FLOOR() Function
The FLOOR()
function returns the largest integer less than or equal to a specified number, effectively rounding down to the nearest whole number.
Example: Using FLOOR()
This query rounds the number 123.4567 down to the nearest integer:
-- Rounding down a number to the nearest integer
SELECT FLOOR(123.4567) AS floored_value;
The FLOOR()
function rounds 123.4567 down to 123.
CEIL() Function
The CEIL()
function returns the smallest integer greater than or equal to a specified number, effectively rounding up to the nearest whole number.
Example: Using CEIL()
This query rounds the number 123.4567 up to the nearest integer:
-- Rounding up a number to the nearest integer
SELECT CEIL(123.4567) AS ceiled_value;
The CEIL()
function rounds 123.4567 up to 124.
ABS() Function
The ABS()
function returns the absolute value of a number, which means it returns the number without its sign (i.e., turning negative values into positive ones).
Example: Using ABS()
This query returns the absolute value of the number -123.4567:
-- Getting the absolute value of a number
SELECT ABS(-123.4567) AS absolute_value;
The ABS()
function returns 123.4567, removing the negative sign.
MOD() Function
The MOD()
function returns the remainder of a division operation. It is essentially the modulus operator, which can be used to calculate remainders.
Example: Using MOD()
This query calculates the remainder when 10 is divided by 3:
-- Calculating the remainder of a division
SELECT MOD(10, 3) AS remainder;
The MOD()
function returns 1, as the remainder when dividing 10 by 3 is 1.
PI() Function
The PI()
function returns the value of Pi (π), which is approximately 3.14159.
Example: Using PI()
This query returns the value of Pi:
-- Getting the value of Pi
SELECT PI() AS pi_value;
The PI()
function returns the value 3.14159265358979.
Diagram: Numeric Functions in MySQL
The following diagram demonstrates how numeric functions such as ROUND()
, FLOOR()
, and CEIL()
work to manipulate numeric data:

This diagram visually explains how the numeric functions transform the input data.
Conditional Functions in MySQL
MySQL provides conditional functions such as CASE
and IF()
that allow you to perform conditional logic directly in your SQL queries. These functions are useful for making decisions and returning different results based on certain conditions.
CASE Statement
The CASE
statement in MySQL allows you to perform conditional logic similar to an IF
statement in programming. It can be used to return a value based on multiple conditions. The CASE
statement supports both simple and searched cases.
Example: Using CASE for Simple Conditions
This query uses the CASE
statement to check the value of a column and return a result based on that value:
-- Using CASE for a simple condition
SELECT
employee_name,
salary,
CASE
WHEN salary > 50000 THEN 'High Salary'
WHEN salary > 30000 THEN 'Medium Salary'
ELSE 'Low Salary'
END AS salary_grade
FROM employees;
The CASE
statement checks the salary column and returns 'High Salary' for salaries greater than 50,000, 'Medium Salary' for salaries between 30,000 and 50,000, and 'Low Salary' for salaries below 30,000.
Example: Using CASE for Searched Conditions
This query uses the CASE
statement with multiple conditions to determine the discount rate:
-- Using CASE for multiple conditions
SELECT
order_id,
order_amount,
CASE
WHEN order_amount > 1000 THEN '20% Discount'
WHEN order_amount BETWEEN 500 AND 1000 THEN '10% Discount'
ELSE 'No Discount'
END AS discount
FROM orders;
The CASE
statement checks the order_amount
and applies a discount based on the value of the amount.
IF() Function
The IF()
function is a simpler conditional function that evaluates a condition and returns one value if the condition is true, and another value if the condition is false. It is equivalent to the IF
function in many programming languages.
Example: Using IF() for a Simple Condition
This query uses the IF()
function to check if the employee's salary is above a certain threshold:
-- Using IF() for a simple condition
SELECT
employee_name,
salary,
IF(salary > 50000, 'High Salary', 'Low Salary') AS salary_grade
FROM employees;
The IF()
function checks if the salary
is greater than 50,000 and returns 'High Salary' if true, or 'Low Salary' if false.
Example: Using IF() with a NULL Check
This query uses IF()
to check for NULL
values in the bonus
column:
-- Using IF() to check for NULL values
SELECT
employee_name,
bonus,
IF(bonus IS NULL, 'No Bonus', 'Bonus Available') AS bonus_status
FROM employees;
The IF()
function checks if the bonus
column is NULL
and returns 'No Bonus' if true, or 'Bonus Available' if false.
Diagram: Conditional Functions in MySQL
The following diagram explains how conditional functions like CASE
and IF()
evaluate conditions and return results:

This diagram visually illustrates the flow of conditional logic in SQL queries using CASE
and IF()
functions.
Regular Expressions and Pattern Matching in MySQL
MySQL provides support for regular expressions (regex) through the REGEXP
and RLIKE
operators, which allow pattern matching in queries. Regular expressions are sequences of characters that form search patterns, enabling powerful text searching and manipulation operations.
Basic Regular Expression Syntax
Regular expressions in MySQL follow the syntax of the POSIX standard, which includes a variety of special characters used to define patterns. Here are some of the most common regex elements:
- Dot (.): Matches any single character except a newline.
- Caret (^): Matches the beginning of the string.
- Dollar sign ($): Matches the end of the string.
- Asterisk (*): Matches zero or more occurrences of the preceding element.
- Plus (+): Matches one or more occurrences of the preceding element.
- Question mark (?): Matches zero or one occurrence of the preceding element.
- Square brackets ([]): Matches any single character within the brackets.
- Pipe (|): Acts as an OR operator to match one of several patterns.
Using REGEXP in MySQL
The REGEXP
operator in MySQL is used to search for a pattern in a column. It returns TRUE
if the pattern matches, and FALSE
if it does not.
Example: Basic Pattern Matching
This query searches for rows where the email
column contains the domain gmail.com
:
-- Using REGEXP to match email pattern
SELECT email
FROM users
WHERE email REGEXP 'gmail\\.com';
In this example, the pattern gmail\\.com
matches any email addresses ending with gmail.com
. The backslash (\\
) is used to escape the dot (.
) because it is a special character in regular expressions.
Example: Matching Multiple Patterns
This query uses the REGEXP
operator to match either gmail.com
or yahoo.com
email domains:
-- Using REGEXP to match either gmail.com or yahoo.com
SELECT email
FROM users
WHERE email REGEXP 'gmail\\.com|yahoo\\.com';
The |
operator acts as an OR, so the query matches both email domains.
Using RLIKE in MySQL
The RLIKE
operator is similar to REGEXP
and can be used interchangeably. It also allows pattern matching in string columns.
Example: Using RLIKE to Match a Pattern
This query finds rows where the phone_number
column matches a pattern of 10 digits:
-- Using RLIKE to match a 10-digit phone number
SELECT phone_number
FROM contacts
WHERE phone_number RLIKE '^[0-9]{10}$';
The pattern ^[0-9]{10}$
matches exactly 10 digits. The ^
denotes the beginning of the string, [0-9]
matches a digit, and {10}
ensures that exactly 10 digits are present.
Using Regular Expressions with LIKE
While LIKE
supports basic pattern matching, it is not as powerful as REGEXP
or RLIKE
. However, LIKE
can still be used for simpler patterns with the %
(wildcard for any number of characters) and _
(wildcard for a single character).
Example: Using LIKE for Simple Pattern Matching
This query uses LIKE
to find rows where the product_name
starts with 'Apple':
-- Using LIKE for pattern matching
SELECT product_name
FROM products
WHERE product_name LIKE 'Apple%';
The %
wildcard matches any sequence of characters following 'Apple'.
Diagram: Regular Expression Flow
The following diagram explains how regular expressions are used in MySQL to match patterns:

This diagram illustrates how different patterns are matched using the REGEXP
and RLIKE
operators in MySQL queries.
Database Normalization (1NF, 2NF, 3NF, BCNF)
Database normalization is the process of organizing data within a database to reduce redundancy and improve data integrity. The goal of normalization is to eliminate undesirable characteristics like insertion, update, and deletion anomalies by dividing large tables into smaller, more manageable ones.
What is Normalization?
Normalization involves breaking down a database into multiple tables and defining relationships between them. By doing so, you reduce data redundancy and ensure that data is logically stored. The process is typically carried out in stages, known as normal forms (NF), each with specific rules and requirements.
First Normal Form (1NF)
A table is in First Normal Form (1NF) if it meets the following conditions:
- All columns contain atomic (indivisible) values.
- Each column contains values of a single type.
- Each column contains unique values for each row (no repeating groups or arrays).
In other words, there should be no multi-valued attributes, and each record in the table should be uniquely identifiable.
Example: Unnormalized Table
-- Example of an unnormalized table
+----+------------+---------------------+
| ID | Name | Phone Numbers |
+----+------------+---------------------+
| 1 | John Smith | 123-4567, 234-5678 |
| 2 | Jane Doe | 345-6789 |
+----+------------+---------------------+
The above table has a column with multiple phone numbers, which violates 1NF.
Normalized to 1NF
-- Normalized to 1NF
+----+------------+-----------------+
| ID | Name | Phone Number |
+----+------------+-----------------+
| 1 | John Smith | 123-4567 |
| 1 | John Smith | 234-5678 |
| 2 | Jane Doe | 345-6789 |
+----+------------+-----------------+
In 1NF, each row has a unique combination of values, and there are no multi-valued attributes.
Second Normal Form (2NF)
A table is in Second Normal Form (2NF) if it is in 1NF and meets the following condition:
- All non-key attributes are fully functionally dependent on the primary key (no partial dependency).
In 2NF, every non-key column must depend on the whole primary key, not just part of it (this is particularly relevant for composite primary keys).
Example: Table in 1NF but not in 2NF
-- Table in 1NF but not in 2NF
+----+------------+------------+-----------+
| ID | Course | Instructor | Instructor Phone |
+----+------------+------------+-----------+
| 1 | Math | Dr. Smith | 123-4567 |
| 1 | Science | Dr. Johnson| 234-5678 |
| 2 | History | Dr. Brown | 345-6789 |
+----+------------+------------+-----------+
In this example, the instructor's phone number is dependent only on the instructor (not the course), which violates 2NF.
Normalized to 2NF
-- Normalized to 2NF
-- Table 1: Courses
+----+------------+
| ID | Course |
+----+------------+
| 1 | Math |
| 1 | Science |
| 2 | History |
+----+------------+
-- Table 2: Instructors
+----+------------+---------------------+
| ID | Instructor | Instructor Phone |
+----+------------+---------------------+
| 1 | Dr. Smith | 123-4567 |
| 2 | Dr. Johnson| 234-5678 |
| 3 | Dr. Brown | 345-6789 |
+----+------------+---------------------+
In 2NF, the instructor's phone number is now stored in a separate table, removing the partial dependency.
Third Normal Form (3NF)
A table is in Third Normal Form (3NF) if it is in 2NF and meets the following condition:
- There are no transitive dependencies (non-key attributes should not depend on other non-key attributes).
In 3NF, all attributes must depend directly on the primary key, and indirect dependencies are eliminated.
Example: Table in 2NF but not in 3NF
-- Table in 2NF but not in 3NF
+----+------------+------------+----------+-----------+
| ID | Name | Department | Location | Manager |
+----+------------+------------+----------+-----------+
| 1 | John Smith | Sales | New York | Robert |
| 2 | Jane Doe | Marketing | London | Susan |
+----+------------+------------+----------+-----------+
The manager's name depends on the department, not just the employee ID, which violates 3NF.
Normalized to 3NF
-- Normalized to 3NF
-- Table 1: Employees
+----+------------+------------+----------+
| ID | Name | Department | Location |
+----+------------+------------+----------+
| 1 | John Smith | Sales | New York |
| 2 | Jane Doe | Marketing | London |
+----+------------+------------+----------+
-- Table 2: Departments
+------------+----------+-----------+
| Department | Manager | Location |
+------------+----------+-----------+
| Sales | Robert | New York |
| Marketing | Susan | London |
+------------+----------+-----------+
In 3NF, the manager's information is moved to a separate table that contains data about departments, eliminating the transitive dependency.
Boyce-Codd Normal Form (BCNF)
A table is in Boyce-Codd Normal Form (BCNF) if it is in 3NF and satisfies the following condition:
- For every functional dependency, the left-hand side must be a superkey.
BCNF is a stricter version of 3NF, ensuring that every determinant is a candidate key.
Example: Table in 3NF but not in BCNF
-- Table in 3NF but not in BCNF
+----+------------+------------+-----------+
| ID | Name | Course | Instructor|
+----+------------+------------+-----------+
| 1 | John Smith | Math | Dr. Smith |
| 2 | Jane Doe | Science | Dr. Johnson|
+----+------------+------------+-----------+
The instructor is functionally dependent on the course, and the course is not a superkey, violating BCNF.
Normalized to BCNF
-- Normalized to BCNF
-- Table 1: Students
+----+------------+
| ID | Name |
+----+------------+
| 1 | John Smith |
| 2 | Jane Doe |
+----+------------+
-- Table 2: Courses
+----+------------+-----------+
| ID | Course | Instructor|
+----+------------+-----------+
| 1 | Math | Dr. Smith |
| 2 | Science | Dr. Johnson|
+----+------------+-----------+
In BCNF, each table has a superkey that determines the other attributes, ensuring no violations of functional dependencies.
Summary of Normal Forms
Normal Form | Conditions |
---|---|
1NF | Atomic values, no repeating groups, no arrays. |
2NF | 1NF + no partial dependency (all non-key attributes depend on the whole primary key). |
3NF | 2NF + no transitive dependency (non-key attributes depend only on the primary key). |
BCNF | 3NF + every determinant is a candidate key. |
Relationships between Tables (One-to-One, One-to-Many, Many-to-Many)
In relational databases, tables are related to each other through foreign keys. The relationships between tables are established based on how the data in one table corresponds to data in another table. There are three main types of relationships: One-to-One, One-to-Many, and Many-to-Many.
One-to-One Relationship
A one-to-one relationship means that for each record in Table A, there is at most one related record in Table B, and vice versa. In this type of relationship, each row in one table is linked to exactly one row in another table.
Example
Consider two tables: Employees
and EmployeeDetails
. Each employee has exactly one set of details, and each set of details belongs to only one employee.
-- Table 1: Employees
+----+------------+-----------+
| ID | Name | Position |
+----+------------+-----------+
| 1 | John Smith | Manager |
| 2 | Jane Doe | Developer|
+----+------------+-----------+
-- Table 2: EmployeeDetails
+----+------------+------------------------+
| ID | EmployeeID | Address |
+----+------------+------------------------+
| 1 | 1 | 123 Elm St, NY |
| 2 | 2 | 456 Oak St, LA |
+----+------------+------------------------+
-- Create Foreign Key in EmployeeDetails
ALTER TABLE EmployeeDetails
ADD CONSTRAINT fk_employee_id
FOREIGN KEY (EmployeeID) REFERENCES Employees(ID);
In this example, each employee has a unique set of details, and the relationship between the Employees
and EmployeeDetails
tables is one-to-one. The EmployeeDetails
table includes a foreign key linking it to the Employees
table.
One-to-Many Relationship
A one-to-many relationship means that one record in Table A can be associated with many records in Table B, but each record in Table B is associated with only one record in Table A. This is the most common type of relationship between tables.
Example
Consider two tables: Departments
and Employees
. A department can have multiple employees, but each employee belongs to only one department.
-- Table 1: Departments
+----+--------------+------------+
| ID | Department | Manager |
+----+--------------+------------+
| 1 | HR | Mr. A |
| 2 | IT | Mr. B |
+----+--------------+------------+
-- Table 2: Employees
+----+------------+------------+--------------+
| ID | Name | Position | DepartmentID |
+----+------------+------------+--------------+
| 1 | John Smith | Developer | 1 |
| 2 | Jane Doe | HR Manager | 1 |
| 3 | Emily Lee | Developer | 2 |
+----+------------+------------+--------------+
-- Create Foreign Key in Employees
ALTER TABLE Employees
ADD CONSTRAINT fk_department_id
FOREIGN KEY (DepartmentID) REFERENCES Departments(ID);
In this example, a department can have many employees, but each employee is associated with only one department. The foreign key DepartmentID
in the Employees
table establishes the one-to-many relationship.
Many-to-Many Relationship
A many-to-many relationship means that records in Table A can be associated with multiple records in Table B, and records in Table B can be associated with multiple records in Table A. This relationship requires an additional table, often called a join table, to manage the associations.
Example
Consider two tables: Students
and Courses
. A student can enroll in many courses, and a course can have many students. To represent this relationship, we need a third table, StudentCourses
, to store the associations between students and courses.
-- Table 1: Students
+----+------------+
| ID | Name |
+----+------------+
| 1 | John Smith |
| 2 | Jane Doe |
+----+------------+
-- Table 2: Courses
+----+------------+
| ID | Course |
+----+------------+
| 1 | Math |
| 2 | Science |
+----+------------+
-- Join Table: StudentCourses
+------------+------------+
| StudentID | CourseID |
+------------+------------+
| 1 | 1 |
| 1 | 2 |
| 2 | 1 |
+------------+------------+
-- Create Foreign Keys in StudentCourses
ALTER TABLE StudentCourses
ADD CONSTRAINT fk_student_id
FOREIGN KEY (StudentID) REFERENCES Students(ID);
ALTER TABLE StudentCourses
ADD CONSTRAINT fk_course_id
FOREIGN KEY (CourseID) REFERENCES Courses(ID);
In this example, the StudentCourses
join table manages the many-to-many relationship between students and courses. The foreign keys StudentID
and CourseID
in the StudentCourses
table establish the connections between the students and courses tables.
Summary of Table Relationships
Relationship Type | Description | Example Tables |
---|---|---|
One-to-One | Each row in Table A is related to one row in Table B. | Employees & EmployeeDetails |
One-to-Many | One row in Table A is related to many rows in Table B. | Departments & Employees |
Many-to-Many | Rows in Table A are related to multiple rows in Table B, and vice versa. Requires a join table. | Students & Courses (via StudentCourses) |
Schema Design Best Practices
Designing a database schema involves organizing data into tables and establishing relationships between them. A well-designed schema improves data integrity, reduces redundancy, and ensures efficient queries. Here are some best practices for designing an effective MySQL database schema:
1. Use Descriptive Table and Column Names
Choose clear, descriptive names for tables and columns that reflect the data they hold. Avoid using abbreviations or non-standard terms that could confuse future developers.
-- Example: Descriptive Table and Column Names
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(100),
LastName VARCHAR(100),
Department VARCHAR(50)
);
In this example, the table and column names clearly indicate their purpose, making it easier to understand the schema.
2. Use Consistent Naming Conventions
Establish a consistent naming convention for tables and columns. This includes using singular or plural forms consistently and choosing either camelCase or snake_case for column names.
-- Example: Consistent Naming Convention
CREATE TABLE Orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
total_amount DECIMAL(10, 2)
);
The consistent naming of the table Orders
and columns like order_id
ensures clarity and avoids confusion.
3. Normalize Data to Avoid Redundancy
Database normalization is the process of organizing data to minimize redundancy and dependency. Normalize your schema to at least the third normal form (3NF). This involves breaking down large tables into smaller, more manageable ones and removing unnecessary data duplication.
-- Example: Normalization (1NF, 2NF, 3NF)
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
FirstName VARCHAR(100),
LastName VARCHAR(100),
Address VARCHAR(200)
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATE,
TotalAmount DECIMAL(10, 2),
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
In this example, the Customers
and Orders
tables are normalized to reduce redundancy. The CustomerID
in the Orders
table avoids storing customer information with every order.
4. Use Foreign Keys to Enforce Relationships
Enforce relationships between tables using foreign keys. This ensures referential integrity, meaning that the data in related tables stays consistent. Foreign keys prevent orphaned records and ensure that updates and deletions are propagated across related tables.
-- Example: Foreign Key Constraint
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATE,
TotalAmount DECIMAL(10, 2),
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
In this example, the foreign key constraint ensures that every order is associated with a valid customer.
5. Use Indexes for Faster Queries
Indexes improve query performance by allowing MySQL to quickly locate rows based on the values of indexed columns. You should index columns that are frequently used in WHERE
, JOIN
, and ORDER BY
clauses. However, avoid over-indexing, as it can slow down write operations.
-- Example: Adding Index
CREATE INDEX idx_customer_id ON Orders (CustomerID);
In this example, an index is added to the CustomerID
column in the Orders
table to optimize queries that filter by customer.
6. Choose the Right Data Types
Select appropriate data types for your columns to ensure data integrity and optimize storage. For example, use INT
for whole numbers, VARCHAR
for variable-length strings, and DATE
for date values. Avoid using large data types like TEXT
or LONGTEXT
unless necessary.
-- Example: Choosing Appropriate Data Types
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255),
Price DECIMAL(10, 2),
StockQuantity INT
);
In this example, Price
is stored as DECIMAL
to store exact values, and StockQuantity
is stored as INT
for whole numbers.
7. Avoid Storing Redundant Data
Storing redundant data can lead to data anomalies and increased storage requirements. Instead, use foreign keys to reference data in other tables. This ensures that your schema remains efficient and scalable.
-- Example: Avoiding Redundant Data
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255),
Price DECIMAL(10, 2)
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
ProductID INT,
Quantity INT,
FOREIGN KEY (ProductID) REFERENCES Products(ProductID)
);
In this example, the Orders
table does not store product names or prices directly; instead, it uses the ProductID
foreign key to reference data from the Products
table.
8. Consider Using Views for Complex Queries
If you have complex queries that need to be reused frequently, consider creating views. A view is a virtual table that contains the result of a query. Views can simplify complex queries by allowing users to interact with them as if they were regular tables.
-- Example: Creating a View
CREATE VIEW OrderDetails AS
SELECT o.OrderID, o.OrderDate, o.TotalAmount, c.CustomerID, c.FirstName, c.LastName
FROM Orders o
JOIN Customers c ON o.CustomerID = c.CustomerID;
In this example, the OrderDetails
view simplifies the query by combining data from the Orders
and Customers
tables, allowing users to access it directly.
9. Use Proper Data Constraints
Use data constraints like NOT NULL
, UNIQUE
, CHECK
, and DEFAULT
to enforce business rules and prevent invalid data from being inserted into the database.
-- Example: Adding Constraints
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(100) NOT NULL,
LastName VARCHAR(100) NOT NULL,
Salary DECIMAL(10, 2) CHECK (Salary > 0)
);
In this example, the NOT NULL
constraint ensures that employees have a first and last name, and the CHECK
constraint ensures that salaries are positive numbers.
10. Document Your Schema
Document your schema design, including table structures, relationships, and constraints. This documentation will help developers understand the design and maintain the database over time.
Summary
Best Practice | Description |
---|---|
Descriptive Names | Use clear, descriptive names for tables and columns to improve readability. |
Consistent Naming | Maintain consistent naming conventions for easier management. |
Data Normalization | Normalize tables to reduce redundancy and improve data integrity. |
Foreign Keys | Use foreign keys to enforce relationships and referential integrity. |
Indexing | Use indexes to speed up queries but avoid over-indexing. |
Right Data Types | Choose appropriate data types for efficient storage and accuracy. |
Avoid Redundancy | Use foreign keys to avoid redundant data storage. |
Views | Use views for complex or reusable queries. |
Data Constraints | Use constraints like NOT NULL , UNIQUE , and CHECK to enforce data integrity. |
Documentation | Document the schema design to ensure clarity and maintainability. |
De-normalization and Its Use Cases
De-normalization is the process of intentionally introducing redundancy into a database schema by merging tables or copying data from one table to another. While normalization eliminates redundancy and improves data integrity, de-normalization can improve performance for specific use cases, especially in read-heavy applications. However, de-normalization can increase storage requirements and make data updates more complex.
1. What is De-normalization?
De-normalization involves combining tables or adding redundant data to simplify queries, improve performance, or reduce the number of joins required in frequent queries. It can be useful for applications where query performance is more important than minimizing storage space or maintaining strict data consistency.
-- Example: De-normalization by Merging Tables
CREATE TABLE OrderSummary (
OrderID INT PRIMARY KEY,
CustomerName VARCHAR(100),
OrderDate DATE,
TotalAmount DECIMAL(10, 2)
);
In this example, the OrderSummary
table is a de-normalized version that combines data from the Orders
and Customers
tables to avoid the need for joins when querying order summaries.
2. When to Use De-normalization
De-normalization is typically used when:
- Read performance is critical: If your application performs frequent, complex queries that require multiple joins, de-normalization can reduce query time by eliminating the need to perform those joins.
- Data is mostly read-only: If your data is rarely updated, de-normalization might be a good option because it reduces the overhead of joining tables.
- Reporting and analytics: In cases where large-scale reporting and analytics require fast access to aggregated or summarized data, de-normalization can optimize query times.
- Query simplicity: De-normalization can simplify queries by reducing the need to join multiple tables.
3. Use Cases for De-normalization
Here are common scenarios where de-normalization can be beneficial:
3.1. Reporting and Data Warehousing
In data warehouses or reporting systems, where queries often involve aggregating large amounts of data, de-normalization helps by reducing the complexity of the queries. For example, instead of joining multiple tables, the data can be pre-aggregated and stored in a single table.
-- Example: Reporting Table with Pre-aggregated Data
CREATE TABLE SalesReport (
ReportID INT PRIMARY KEY,
ProductID INT,
TotalSales DECIMAL(10, 2),
TotalQuantity INT,
ReportDate DATE
);
In this example, sales data is pre-aggregated into a SalesReport
table to improve query performance during reporting without having to perform expensive joins and aggregations on transactional data.
3.2. Caching Frequently Accessed Data
De-normalization can be used to cache the results of frequently accessed queries. For example, storing the result of an expensive join or aggregation in a separate table can speed up subsequent queries.
-- Example: Caching Joined Data
CREATE TABLE CachedOrderDetails (
OrderID INT PRIMARY KEY,
CustomerName VARCHAR(100),
TotalAmount DECIMAL(10, 2),
OrderDate DATE
);
In this example, the CachedOrderDetails
table is a de-normalized version of the data that is frequently queried in a joined format, eliminating the need to join multiple tables each time.
3.3. Read-heavy Applications
For applications where the majority of database operations are read-heavy, de-normalization can help speed up read queries by reducing the number of tables involved and thus the time it takes to fetch the data.
-- Example: De-normalized Table for Fast Retrieval
CREATE TABLE ProductCatalog (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255),
Category VARCHAR(100),
Price DECIMAL(10, 2),
StockQuantity INT
);
In this example, the ProductCatalog
table stores product details in a single table, including product name, category, price, and stock quantity, instead of splitting this information across multiple tables.
4. Risks of De-normalization
While de-normalization can improve performance, it comes with trade-offs:
- Data Redundancy: De-normalizing leads to redundant data, which increases storage requirements and can cause data anomalies if not carefully managed.
- Data Integrity Issues: With redundant data, it becomes more difficult to maintain consistency across tables. Updates must be made in multiple places, which increases the risk of errors.
- Complex Updates: When data changes, it must be propagated across all redundant copies, which can be time-consuming and error-prone.
- Increased Maintenance: De-normalized databases may require more effort to maintain and update, as developers must ensure consistency between redundant copies of data.
5. Best Practices for De-normalization
- Use only when necessary: Don't de-normalize unless performance improvements are required for specific, high-traffic queries.
- Monitor performance: Regularly monitor the performance of your database to ensure that de-normalization is achieving the desired improvements.
- Keep updates in mind: If you decide to de-normalize, ensure that you have processes in place to handle data updates and maintain consistency across redundant copies.
- Consider caching strategies: In some cases, caching mechanisms outside the database (such as in-memory caches) may be a better solution than de-normalization.
Summary
Use Case | Description |
---|---|
Reporting and Data Warehousing | Pre-aggregate and store data for fast reporting without expensive joins. |
Caching Frequently Accessed Data | Store the result of expensive queries to reduce the need for repeated complex operations. |
Read-heavy Applications | Improve query performance by reducing the need for joins and combining data into fewer tables. |
Data Redundancy | Redundant data increases storage and the risk of inconsistencies. |
Data Integrity Issues | Ensure consistency between redundant data copies to prevent errors. |
Increased Maintenance | De-normalized structures may require more effort to maintain due to redundant data. |
Entity-Relationship (ER) Diagrams
Entity-Relationship (ER) diagrams are a visual representation of the entities within a database and the relationships between them. They are used to model the logical structure of a database, making it easier to design and understand how different data entities interact with each other.
1. What is an ER Diagram?
An Entity-Relationship (ER) diagram is a graphical tool used in database design. It visually depicts the entities (tables) in a system and how they are related. ER diagrams use symbols like rectangles for entities, diamonds for relationships, and ovals for attributes to represent the structure of the database.
2. Components of an ER Diagram
ER diagrams consist of the following key components:
- Entities: Represented by rectangles, entities are objects or things within the system that have data stored about them (e.g., Users, Orders, Products).
- Attributes: Represented by ovals, attributes provide more details about an entity (e.g., a
Customer
entity might have attributes likeFirstName
,LastName
,Email
). - Relationships: Represented by diamonds, relationships show how two or more entities are related to each other (e.g., an
Order
is related to aCustomer
and aProduct
). - Primary Key: An attribute (or combination of attributes) that uniquely identifies an entity within the database. This is often denoted with a key symbol.
- Foreign Key: An attribute in one entity that links to the primary key of another entity. This is used to establish relationships between entities.
3. Types of Relationships
In an ER diagram, relationships between entities can be classified into the following types:
- One-to-One (1:1): A relationship where one entity is related to exactly one instance of another entity. For example, a
Person
may be related to exactly onePassport
. - One-to-Many (1:N): A relationship where one entity is related to multiple instances of another entity. For example, one
Customer
can have multipleOrders
. - Many-to-Many (M:N): A relationship where multiple instances of one entity are related to multiple instances of another entity. For example, a
Student
can enroll in multipleCourses
, and eachCourse
can have multipleStudents
.
4. Example of an ER Diagram
Here is an example of an ER diagram that represents the relationship between Customers
, Orders
, and Products
:

The diagram shows that:
- A
Customer
can place multipleOrders
(one-to-many relationship). - An
Order
can contain multipleProducts
(many-to-many relationship), which is represented by a junction tableOrderDetails
linking theOrders
andProducts
entities.
5. Creating an ER Diagram
To create an ER diagram, follow these general steps:
- Identify entities: Determine the key objects in the system (e.g.,
Customer
,Product
,Order
). - Define attributes: For each entity, determine the attributes that describe it (e.g.,
Customer
may haveFirstName
,LastName
,Email
). - Establish relationships: Determine how entities are related (e.g., a
Customer
places anOrder
, anOrder
containsProducts
). - Define keys: Identify primary keys for each entity and foreign keys to represent relationships.
6. Tools for Creating ER Diagrams
Various tools can be used to create ER diagrams, including:
- MySQL Workbench: A powerful tool for database design that allows you to create ER diagrams visually and generate SQL scripts.
- Lucidchart: An online diagramming tool that offers templates for creating ER diagrams.
- dbdiagram.io: A free online tool for creating and sharing database diagrams.
- Microsoft Visio: A diagramming tool that can be used to create ER diagrams and flowcharts.
7. Best Practices for ER Diagram Design
- Keep it simple: Avoid adding unnecessary details. Focus on the key entities, attributes, and relationships.
- Follow naming conventions: Use clear and consistent naming for entities, attributes, and relationships.
- Normalize your design: Ensure that your design follows the principles of normalization to avoid redundancy and data anomalies.
- Use proper cardinality: Clearly define the relationships (1:1, 1:N, M:N) between entities to avoid confusion.
8. Summary
Entity | Description |
---|---|
Entities | Represent real-world objects or things that store data, such as Customer , Order , and Product . |
Attributes | Describe the details of an entity, such as FirstName , LastName , or Price . |
Relationships | Show how entities are related, such as one-to-many or many-to-many relationships. |
Primary Key | A unique identifier for each entity instance, such as CustomerID . |
Foreign Key | Links one entity to another, such as CustomerID in the Orders table. |
What are Indexes and Why Use Them?
In MySQL, an index is a data structure that improves the speed of data retrieval operations on a database table. Indexes are used to quickly locate rows based on the values of one or more columns, significantly speeding up query performance, especially for large datasets.
1. What is an Index?
An index is a database object that stores a small portion of the table's data in a way that allows for faster searches. It is similar to an index in a book, which helps you quickly find specific information without having to read the entire content.
When a query is executed, MySQL can use indexes to quickly locate rows that match the query conditions, instead of scanning the entire table. This results in faster data retrieval and improved overall query performance.
2. How Does an Index Work?
MySQL uses a variety of index types, but the most common type is the B-tree index. This index structure is balanced, meaning that the database can quickly navigate from the root of the tree to the leaf nodes where the actual data is stored. This allows for logarithmic-time complexity in searches, making the process much faster than a full table scan.
3. Types of Indexes in MySQL
MySQL supports several types of indexes, including:
- Primary Index: The primary index is automatically created on the
PRIMARY KEY
column of a table. It ensures that each row in the table has a unique identifier and stores the data in a sorted order based on the primary key. - Unique Index: A unique index ensures that the values in the indexed column(s) are unique across the entire table, preventing duplicate values.
- Normal Index: A regular index that does not enforce any uniqueness constraint. It speeds up query performance but does not prevent duplicate values.
- Full-text Index: A special type of index used for full-text searching within text-based columns (e.g.,
TEXT
,VARCHAR
). It allows for efficient searching of words or phrases within large text fields. - Spatial Index: An index type used for spatial data types, such as geographic coordinates, to optimize spatial queries.
4. Why Use Indexes?
Indexes are crucial for the performance of database queries, especially when working with large datasets. Below are some reasons why you should use indexes:
- Faster Query Performance: Indexes allow the database to quickly locate the rows that match the query conditions, reducing the need to scan the entire table. This can drastically improve the performance of SELECT, JOIN, and WHERE queries.
- Efficient Sorting and Filtering: Indexes help optimize sorting (ORDER BY) and filtering (WHERE) operations by maintaining an ordered structure that can be used to find matching rows quickly.
- Improved Join Performance: When joining multiple tables, indexes on the columns used in the join conditions can significantly speed up the operation by reducing the number of rows that need to be examined.
- Enforcing Uniqueness: Unique indexes ensure that duplicate values do not exist in a column (e.g., primary keys), helping to maintain data integrity.
- Optimizing Full-text Search: Full-text indexes make it possible to perform fast searches in large text-based columns, improving the performance of text search queries.
5. Example of Creating an Index
To create an index on a column, you can use the CREATE INDEX
statement. Here is an example:
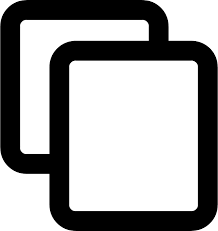
-- Creating an index on the 'email' column of the 'customers' table
CREATE INDEX idx_email ON customers (email);
6. When Not to Use Indexes
While indexes offer significant performance benefits, they should be used carefully. Below are some situations where indexes may not be beneficial:
- Small Tables: If a table is small (i.e., contains only a few rows), using indexes might not provide any performance improvement and can actually slow down operations due to the overhead of maintaining the index.
- Frequent Updates: Indexes need to be updated every time a row is inserted, updated, or deleted. If a table has frequent data modifications, the overhead of maintaining the indexes can outweigh the benefits.
- Multiple Indexes on the Same Table: Using too many indexes on a single table can negatively impact performance. Each index takes up disk space and requires maintenance during data changes. It's important to carefully choose which columns should be indexed.
7. Best Practices for Using Indexes
- Index Columns Used in WHERE Clauses: Index the columns that are commonly used in
WHERE
clauses to optimize filtering queries. - Index Join Columns: Index columns that are frequently used in join conditions to improve join performance.
- Use Composite Indexes: If multiple columns are often used together in queries, consider creating a composite index (an index on multiple columns) to improve performance.
- Monitor Index Usage: Regularly monitor index usage to ensure that they are being used effectively. Use tools like
EXPLAIN
to analyze query execution plans. - Remove Unused Indexes: Drop indexes that are not being used to save storage space and improve database performance.
8. Summary
Benefit | Description |
---|---|
Faster Query Performance | Indexes help to quickly locate rows, improving query speed for large datasets. |
Efficient Sorting and Filtering | Indexes optimize sorting (ORDER BY) and filtering (WHERE) operations by maintaining an ordered structure. |
Improved Join Performance | Indexes speed up join operations by reducing the number of rows to be processed. |
Enforcing Uniqueness | Unique indexes ensure that duplicate values do not exist in a column, ensuring data integrity. |
Optimizing Full-text Search | Full-text indexes improve the performance of text search queries in large text-based columns. |
Types of Indexes in MySQL
Indexes in MySQL are used to optimize the performance of queries. Different types of indexes are available, each suited for specific use cases. These include single-column indexes, composite indexes, and full-text indexes. Below, we will explore each type and when to use them.
1. Single-column Index
A single-column index is the simplest form of an index. It is created on a single column of a table. This index is helpful when a query often filters or sorts by a single column. The index speeds up the retrieval of rows based on the indexed column.
For example, if you have a `users` table and frequently filter by the `email` column, you can create a single-column index on the `email` column to improve performance:
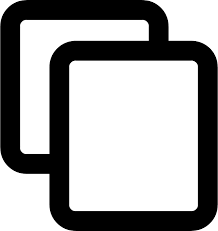
-- Creating a single-column index on the 'email' column of the 'users' table
CREATE INDEX idx_email ON users (email);
2. Composite Index
A composite index is an index created on multiple columns of a table. This type of index is useful when queries involve conditions on multiple columns. The composite index helps to speed up the query execution by combining multiple columns into a single index structure.
For example, if you frequently filter by both `first_name` and `last_name` columns, you can create a composite index on both columns:
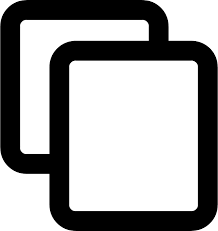
-- Creating a composite index on the 'first_name' and 'last_name' columns of the 'employees' table
CREATE INDEX idx_name ON employees (first_name, last_name);
Note: The order of columns in a composite index matters. In the example above, the index will be used effectively for queries filtering by both `first_name` and `last_name`. However, it may not be as effective for queries filtering only by `last_name`.
3. Full-text Index
A full-text index is used for full-text searches in columns that contain large amounts of text. It allows you to perform complex text searches, such as finding rows that match a specific word or phrase, with high efficiency. Full-text indexes are most commonly used on TEXT
or VARCHAR
columns.
Full-text indexing can be useful for applications that require searching through content, such as article or product descriptions. To create a full-text index on a text-based column, you can use the following syntax:
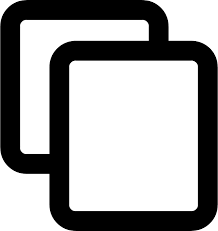
-- Creating a full-text index on the 'content' column of the 'articles' table
CREATE FULLTEXT INDEX idx_content ON articles (content);
Once the full-text index is created, you can use the MATCH()
and AGAINST()
functions to perform full-text searches:
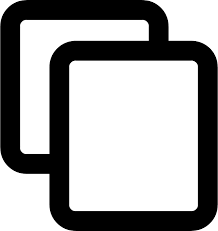
-- Searching for articles containing the word 'MySQL'
SELECT * FROM articles
WHERE MATCH(content) AGAINST('MySQL');
4. Comparison of Index Types
Here is a comparison of the three index types:
Index Type | Columns Involved | Use Case |
---|---|---|
Single-column Index | One column | Used when queries filter or sort by a single column. |
Composite Index | Multiple columns | Used when queries filter or sort by multiple columns together. |
Full-text Index | Text-based columns (e.g., VARCHAR, TEXT) | Used for efficient full-text searches within large text columns. |
5. Performance Considerations
While indexes can significantly improve query performance, they also come with some trade-offs:
- Storage Overhead: Indexes take up additional storage space, especially for large tables with multiple columns indexed.
- Insert/Update Performance: Every time data is inserted, updated, or deleted in a table with indexes, the indexes need to be updated as well. This can slow down data modification operations.
- Choosing the Right Index: It's important to carefully choose which columns to index based on query patterns. Indexing the wrong columns or using too many indexes can degrade performance.
6. Summary
- Single-column Index: A simple index on a single column, ideal for queries filtering or sorting by that column.
- Composite Index: An index on multiple columns, useful when queries filter or sort based on multiple columns.
- Full-text Index: A specialized index for performing full-text searches in large text fields.
Creating Indexes in MySQL
Indexes are critical for improving query performance in MySQL by allowing the database to locate and retrieve data more efficiently. This section explains how to create and drop indexes using the CREATE INDEX
and DROP INDEX
statements.
1. Creating an Index (CREATE INDEX)
The CREATE INDEX
statement is used to create an index on one or more columns of a table. Indexes can improve the performance of SELECT queries that filter, join, or sort by the indexed columns. Here’s the basic syntax:
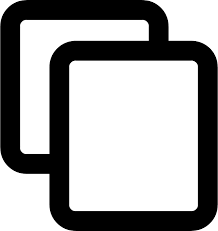
-- Basic syntax for creating an index
CREATE INDEX index_name ON table_name (column_name);
For example, to create an index on the email
column of a table called users
, you would use the following query:
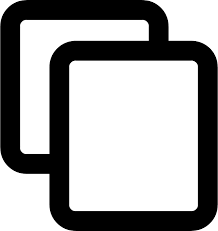
-- Creating an index on the 'email' column of the 'users' table
CREATE INDEX idx_email ON users (email);
If you want to create a composite index (an index on multiple columns), you can specify multiple columns within the parentheses:
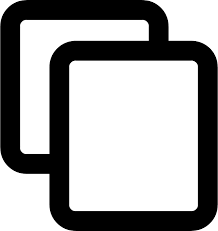
-- Creating a composite index on 'first_name' and 'last_name' columns of the 'employees' table
CREATE INDEX idx_name ON employees (first_name, last_name);
Indexes can also be created on unique, full-text, or spatial columns depending on the use case. For example, for a full-text search, you can create a full-text index as follows:
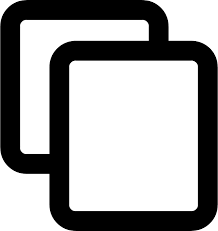
-- Creating a full-text index on the 'content' column of the 'articles' table
CREATE FULLTEXT INDEX idx_content ON articles (content);
2. Dropping an Index (DROP INDEX)
If an index is no longer needed or if it is affecting performance negatively (e.g., due to excessive storage or slowing down inserts), you can remove it using the DROP INDEX
statement. Here’s the basic syntax:
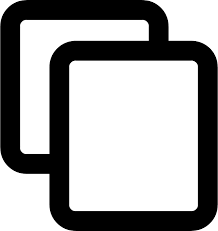
-- Basic syntax for dropping an index
DROP INDEX index_name ON table_name;
For example, to drop the idx_email
index on the users
table, you would use the following query:
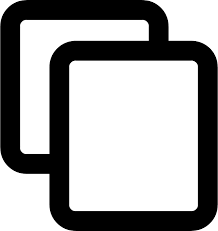
-- Dropping the 'idx_email' index from the 'users' table
DROP INDEX idx_email ON users;
3. Considerations when Creating Indexes
While indexes improve query performance, they come with some trade-offs that should be considered when creating them:
- Storage Overhead: Indexes consume additional disk space. Creating too many indexes on large tables can significantly increase storage requirements.
- Insert/Update Performance: Indexes need to be updated whenever data is inserted, updated, or deleted in the table. This can slow down data modification operations.
- Choosing the Right Columns: Indexes should be created on columns that are frequently used in
WHERE
,ORDER BY
, orJOIN
clauses. Creating indexes on columns that are rarely used in queries can negatively impact performance.
4. Summary
- Create Index: Use
CREATE INDEX
to improve query performance by creating indexes on one or more columns. - Drop Index: Use
DROP INDEX
to remove an index when it is no longer needed or causing performance issues. - Considerations: Be mindful of storage and performance trade-offs when creating indexes. Select the right columns to index to maximize query optimization.
Using EXPLAIN for Query Performance Analysis
The EXPLAIN
statement in MySQL provides valuable insights into how the database engine executes a query. It helps you understand the query execution plan, which can be used to optimize performance, identify slow queries, and improve indexing strategies.
1. What is EXPLAIN?
The EXPLAIN
statement is used to display information about how MySQL executes a query. It shows details about table scans, joins, and the order in which the tables are accessed. This information can help identify inefficiencies like unnecessary full table scans or missing indexes.
2. Basic Syntax
To use EXPLAIN
, simply prefix your query with the EXPLAIN
keyword. Here’s the basic syntax:
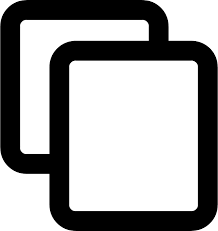
-- Basic syntax of EXPLAIN
EXPLAIN SELECT * FROM table_name WHERE column_name = value;
For example, to analyze a SELECT
query on the users
table, you can run:
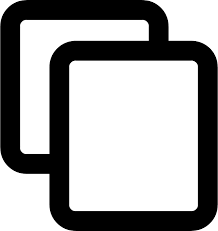
-- Using EXPLAIN with a SELECT query
EXPLAIN SELECT * FROM users WHERE email = 'example@example.com';
3. Understanding the EXPLAIN Output
When you run a query with EXPLAIN
, you’ll receive a table of information. The columns in the result provide details on how the query is executed:
Column | Description |
---|---|
id |
Query identifier. If there are subqueries, each part will have a different id . |
select_type |
Type of query, such as SIMPLE , PRIMARY , or SUBQUERY . |
table |
The name of the table being accessed in the query. |
type |
Join type used, such as ALL , index , range , etc. This is important to assess whether the query is optimized. |
possible_keys |
Indexes that MySQL could use to optimize the query. |
key |
The actual index that MySQL uses for the query. If NULL , no index is used. |
key_len |
The length of the index used. A smaller value is better as it indicates fewer bytes are being scanned. |
ref |
Columns or constants that are used with the key. |
rows |
The number of rows MySQL estimates it needs to examine. |
Extra |
Additional information such as whether a temporary table is used, or if filesort is required. |
4. Example of EXPLAIN Output
Here is an example of the output for a simple SELECT
query:
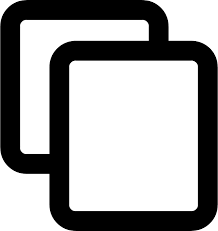
-- Example of EXPLAIN output
+----+-------------+--------+-------+---------------+---------+---------+-------+------+-------------+
| id | select_type | table | type | possible_keys | key | key_len | ref | rows | Extra |
+----+-------------+--------+-------+---------------+---------+---------+-------+------+-------------+
| 1 | SIMPLE | users | ref | email_index | email | 102 | const | 5 | Using where |
+----+-------------+--------+-------+---------------+---------+---------+-------+------+-------------+
In this example, MySQL is using the email_index
index to fetch data from the users
table. It estimates 5 rows will be scanned, and it uses the WHERE
clause to filter the results.
5. Improving Query Performance with EXPLAIN
By interpreting the output of EXPLAIN
, you can make improvements to your queries:
- Indexing: Ensure that indexes are used for columns in
WHERE
,JOIN
, andORDER BY
clauses. Consider adding indexes if none are used (check thekey
andpossible_keys
columns). - Avoiding Full Table Scans: If the
type
column showsALL
, it indicates a full table scan, which is usually slow. Creating appropriate indexes can help. - Reduce Row Scans: If the
rows
value is high, this indicates that many rows are being examined. Try to refine your query or add indexes to narrow the results.
6. Advanced EXPLAIN: EXPLAIN ANALYZE
For even more detailed analysis, you can use EXPLAIN ANALYZE
(available in MySQL 8.0 and above). This provides the actual execution time and more precise performance data:
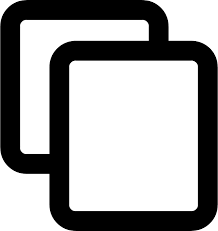
-- Using EXPLAIN ANALYZE for detailed query performance
EXPLAIN ANALYZE SELECT * FROM users WHERE email = 'example@example.com';
7. Summary
- EXPLAIN helps you analyze the query execution plan, identify inefficiencies, and optimize queries.
- Key columns like
type
,key
, androws
provide insights into how MySQL executes the query. - Use
EXPLAIN ANALYZE
for more detailed performance data in MySQL 8.0 and above.
Query Optimization Techniques (Joins, Subqueries, etc.)
Query optimization is the process of improving the performance of SQL queries. By implementing effective query optimization techniques, you can reduce query execution time, minimize resource consumption, and improve the overall performance of your database. Common techniques include optimizing joins, subqueries, indexing, and query restructuring.
1. Optimizing Joins
Joins are a vital part of SQL queries, but they can also be a source of inefficiency. Optimizing joins can significantly improve query performance.
- Use Proper Join Types: Choose the most appropriate join type for your query. For example, use
INNER JOIN
when you only need matching rows from both tables, and useLEFT JOIN
when you need all records from the left table. - Limit the Number of Joins: Avoid excessive joins in a query. Each join adds processing time, especially when joining large tables. If possible, break up complex queries into smaller, more manageable parts.
- Use Indexes on Join Columns: Ensure that the columns used for joins have indexes to speed up the matching process. This can significantly reduce the query execution time.
2. Optimizing Subqueries
Subqueries are queries nested inside other queries. While they are powerful, they can sometimes lead to inefficient query execution. Optimizing subqueries can improve performance significantly.
- Use Joins Instead of Subqueries: In many cases, replacing subqueries with
JOIN
operations can improve performance. Joins are typically more efficient because they allow the database engine to optimize the execution plan more effectively. - Use EXISTS Instead of IN: When checking for the existence of values in a subquery, use
EXISTS
instead ofIN
. TheEXISTS
operator stops as soon as it finds a match, whileIN
may evaluate all records. - Avoid Correlated Subqueries: Correlated subqueries can be slow because they are executed once for each row of the outer query. Whenever possible, try to avoid correlated subqueries, or use joins instead.
3. Using Indexes
Indexes are one of the most powerful tools for optimizing query performance. An index is a data structure that improves the speed of data retrieval operations. Use the following strategies:
- Indexing Frequently Queried Columns: Index columns that are frequently used in
WHERE
,JOIN
, orORDER BY
clauses. This can significantly speed up queries that filter or sort by those columns. - Composite Indexes: Create composite indexes for queries that filter on multiple columns. This can make the query execution faster by reducing the number of index lookups.
- Covering Indexes: Use covering indexes, which include all the columns needed for a query. This allows the query to be answered entirely from the index, without needing to access the table data.
4. Query Restructuring
Restructuring a query can often lead to significant performance improvements. Consider the following approaches:
- Use LIMIT to Restrict Results: Use the
LIMIT
clause to restrict the number of rows returned by a query. This is especially useful when you don’t need to fetch all rows but only a subset. - Filter Early: Apply filters as early as possible in the query. For example, put
WHERE
clauses before anyJOIN
operations to reduce the amount of data being processed in subsequent steps. - Remove Unnecessary Columns: Select only the columns you actually need. Avoid using
SELECT *
as it retrieves all columns, even those you do not need, leading to unnecessary I/O operations.
5. Query Caching
Query caching stores the result of queries in memory so that subsequent executions of the same query do not need to be processed again, thus speeding up performance.
- Leverage Query Cache (if supported): Enable query caching in MySQL to store frequently executed queries and their results. This can improve performance for read-heavy workloads.
- Avoid Stale Data: Be cautious when using query caching, as it can return stale data. Ensure that caches are invalidated when the underlying data changes.
6. Optimizing Aggregations
Aggregation queries, such as those using COUNT()
, SUM()
, and AVG()
, can be slow, especially on large datasets. Here are some tips to optimize them:
- Use Indexes for Grouping: If you are using the
GROUP BY
clause, ensure that the grouped columns have indexes to speed up the process. - Filter Before Aggregating: Apply filters using
WHERE
before grouping to reduce the number of rows that need to be aggregated. - Consider Materialized Views: For very large datasets, consider using materialized views to precompute aggregated results and store them for fast retrieval.
7. Analyzing Query Performance with EXPLAIN
As mentioned in a previous section, using EXPLAIN
helps you understand how MySQL executes your queries. By analyzing the output of EXPLAIN
, you can identify slow parts of your query and optimize them.
- Look for Full Table Scans: If the query uses a full table scan, consider adding indexes on the relevant columns.
- Check Join Order: EXPLAIN will show the order of joins. Make sure the most selective tables are joined first to reduce the size of intermediate result sets.
8. Optimizing Large Data Set Queries
When dealing with large datasets, it’s crucial to optimize queries to handle the volume efficiently. Consider the following techniques:
- Partitioning Tables: Break large tables into smaller, more manageable partitions. This can improve query performance by limiting the number of rows scanned.
- Batch Processing: For large inserts or updates, use batch processing to process data in smaller chunks rather than all at once.
- Use Pagination: Use the
LIMIT
andOFFSET
clauses to paginate queries that return large datasets, reducing the load on the database.
9. Summary
Effective query optimization techniques can dramatically improve your database performance. Here are the key takeaways:
- Optimize joins by using appropriate join types and indexes on join columns.
- Consider using joins instead of subqueries for better performance.
- Use indexes wisely and create composite and covering indexes for frequent queries.
- Restructure queries to limit results, filter early, and remove unnecessary columns.
- Leverage query caching to speed up frequently executed queries.
- Optimize aggregation queries with indexes and filtering before aggregation.
- Always analyze query performance using
EXPLAIN
and adjust your approach accordingly.
Full-text Indexing and Search Optimization
Full-text indexing is a powerful feature in MySQL that allows you to perform fast and efficient searches on large text-based data. It enables more advanced search capabilities than basic pattern matching, such as natural language searches, boolean searches, and relevance ranking. Full-text search optimization helps in improving query performance and ensuring that search results are accurate and fast.
1. What is Full-text Indexing?
Full-text indexing is a type of index designed to allow for fast searching of large amounts of text. It indexes words in a text column and uses them to perform quick text searches. Unlike normal indexes, full-text indexes can index words within a text and rank results by their relevance.
- Supported Data Types: Full-text indexes work with
CHAR
,VARCHAR
, andTEXT
data types. - Searchable Words: MySQL’s full-text indexing skips common words (stopwords) like "the", "and", "of", etc., as well as words that are too short (default minimum length is 3 characters).
2. Creating Full-text Indexes
To enable full-text searching, you must first create a full-text index on the column you want to search. This index can be created during table creation or added later to existing tables.
-- Creating a full-text index during table creation
CREATE TABLE articles (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255),
content TEXT,
FULLTEXT(title, content) -- Full-text index on title and content columns
);
-- Adding a full-text index to an existing table
CREATE INDEX ft_articles ON articles(title, content);
3. Full-text Search Queries
After creating the full-text index, you can perform full-text searches using the MATCH
and AGAINST
keywords.
- Basic Full-text Search: Use
MATCH
andAGAINST
to search for words or phrases in the indexed columns.
-- Basic full-text search
SELECT * FROM articles
WHERE MATCH(title, content) AGAINST('MySQL optimization');
4. Types of Full-text Searches
MySQL supports two types of full-text searches:
- Natural Language Search: This type of search uses the natural language processing algorithm to rank search results by relevance. The query is processed as if the user is typing a search query in a search engine.
SELECT * FROM articles
WHERE MATCH(title, content) AGAINST('MySQL optimization');
+
(required), -
(excluded), and " "
(phrase) to refine your search.
-- Boolean search example: find articles containing 'MySQL' but not 'optimization'
SELECT * FROM articles
WHERE MATCH(title, content) AGAINST('+MySQL -optimization' IN BOOLEAN MODE);
5. Search Result Ranking
MySQL ranks the results of a full-text search based on relevance. The higher the relevance, the higher the rank. You can retrieve the relevance score by using the WITH QUERY EXPANSION
option to improve result ranking.
-- Retrieving search results with relevance scores
SELECT id, title, content,
MATCH(title, content) AGAINST('MySQL optimization') AS score
FROM articles
WHERE MATCH(title, content) AGAINST('MySQL optimization')
ORDER BY score DESC; -- Order results by relevance score
6. Optimizing Full-text Search Performance
To optimize the performance of full-text searches, consider the following techniques:
- Limit the Number of Indexed Columns: Index only the columns that are necessary for your full-text search. Indexing too many columns can slow down write operations.
- Optimize Query Structure: Use the boolean search mode for more control over search results and to avoid unnecessary matches.
- Use
AGAINST
with Optimized Queries: When performing search queries, be mindful of the structure of yourAGAINST
clause. Complex queries can cause performance degradation if not optimized. - Increase the Full-text Index Size: In MySQL, you can adjust the size of the full-text index to improve performance for large datasets by modifying the
ft_min_word_len
variable (for words length) andft_max_word_len
(for word length limit).
7. Full-text Search Limitations
While full-text search is powerful, it has some limitations to be aware of:
- Stop Words: Common words such as "a", "the", "is", etc., are ignored in a full-text search by default. You can configure the stopword list, but it’s not always possible to disable it completely.
- Minimum Word Length: MySQL ignores words shorter than a specified length (default is 3 characters). You can change this limit by adjusting the
ft_min_word_len
variable. - Inconsistent with Non-Text Columns: Full-text indexing is not as effective for non-text columns, like numeric or date columns.
8. Advanced Search Techniques
For more advanced full-text search capabilities, you can use these techniques:
- Use
WITH QUERY EXPANSION
: This feature automatically expands the query by adding synonyms to improve the chances of finding relevant results.
SELECT * FROM articles
WHERE MATCH(title, content) AGAINST('optimization' WITH QUERY EXPANSION);
9. Summary
Full-text indexing is a powerful tool that enhances the search capabilities of MySQL databases. By creating full-text indexes on text-based columns, using full-text search queries, and optimizing performance, you can create fast and efficient search systems. However, it’s important to be aware of the limitations and implement best practices to ensure good performance.
Query Caching in MySQL
Query caching in MySQL is a feature that stores the result of SELECT queries in memory, allowing subsequent identical queries to be retrieved quickly without having to execute the query again. This can significantly speed up query execution and improve the overall performance of the database, especially for read-heavy workloads.
1. What is Query Caching?
Query caching is a mechanism where MySQL stores the result set of SELECT queries in memory. When the same query is executed again, MySQL retrieves the result directly from the cache instead of re-executing the query. This is particularly useful for queries that are frequently executed with the same parameters and do not modify data.
- Cache Hit: When a query result is found in the cache, it’s called a cache hit.
- Cache Miss: When a query result is not found in the cache, it's a cache miss, and MySQL will execute the query and store the result in the cache for future use.
2. Enabling Query Cache
Query caching is not enabled by default in newer versions of MySQL, but it can be enabled by adjusting configuration settings.
- MySQL Configuration: To enable query caching, you need to set the
query_cache_type
andquery_cache_size
variables in your MySQL configuration file.
-- Edit my.cnf or my.ini file
[mysqld]
query_cache_type = 1 -- Enables query cache
query_cache_size = 1048576 -- Sets cache size to 1MB
After updating the configuration, restart MySQL to apply the changes.
3. Query Cache SizeThe size of the query cache can be adjusted to improve performance. The query_cache_size
variable determines the amount of memory allocated for caching query results. If the cache size is too small, you may experience cache evictions, while a too-large cache can consume excessive memory.
-- Check current query cache size
SHOW VARIABLES LIKE 'query_cache_size';
-- Set a new cache size (e.g., 16MB)
SET GLOBAL query_cache_size = 16777216;
4. Query Cache Behavior
MySQL determines which queries to cache based on the query_cache_type
setting. Below are the types of query cache behavior:
- Query Cache Type 0: Disables query caching.
- Query Cache Type 1: Enables query caching for all SELECT queries that do not modify data (such as INSERT, UPDATE, DELETE, etc.).
- Query Cache Type 2: Enables query caching only for SELECT queries that explicitly request caching using the
SQL_CACHE
hint.
5. Query Cache and Data Modifications
When data in a table is modified (via INSERT
, UPDATE
, or DELETE
), MySQL automatically invalidates the cached results for queries that access the modified data. This prevents outdated data from being returned in subsequent queries.
- Cache Invalidation: Modifications to a table invalidate the entire query cache for SELECT queries that refer to the modified table, ensuring the cache is updated with new results.
- Effect on Performance: While query caching can improve read performance, frequent data changes may lead to increased cache invalidations, reducing the effectiveness of caching.
6. Using SQL_CACHE and SQL_NO_CACHE
MySQL allows you to control query caching behavior on a per-query basis using the SQL_CACHE
and SQL_NO_CACHE
hints:
- SQL_CACHE: Forces MySQL to use the query cache, even if caching is disabled globally.
- SQL_NO_CACHE: Prevents MySQL from using the query cache, even if it is enabled globally.
-- Force query cache usage
SELECT SQL_CACHE * FROM articles WHERE title LIKE 'MySQL%';
-- Prevent query cache usage
SELECT SQL_NO_CACHE * FROM articles WHERE title LIKE 'MySQL%';
7. Checking Cache Status
You can monitor the performance and status of the query cache using the following commands:
-- Check query cache status
SHOW STATUS LIKE 'Qcache%';
-- Check cache hits and misses
SHOW STATUS LIKE 'Qcache_hits';
SHOW STATUS LIKE 'Qcache_inserts';
SHOW STATUS LIKE 'Qcache_not_cached';
These commands provide information about the number of cache hits, inserts, and the number of queries not cached.
8. Query Cache Limitations
While query caching can improve performance, it has several limitations:
- Not suited for write-heavy workloads: In environments with frequent data modifications, query cache effectiveness can decrease due to frequent invalidations.
- Only SELECT queries are cached: Data modification operations (INSERT, UPDATE, DELETE) do not benefit from query caching.
- Large data sets: Caching large result sets can consume a lot of memory, which may lead to memory pressure and performance degradation.
9. Disabling Query Cache
If query caching is not needed or causes performance issues, you can disable it by setting the query_cache_type
to 0 in the MySQL configuration file, followed by a MySQL restart:
-- Disable query cache
SET GLOBAL query_cache_type = 0;
10. Summary
Query caching in MySQL is a useful feature for speeding up SELECT queries by storing result sets in memory. It can be especially useful in read-heavy applications, but it requires careful management, especially in write-heavy environments. Understanding how query caching works, monitoring its effectiveness, and configuring the cache size appropriately can significantly improve your database performance.
Introduction to Transactions in MySQL
A transaction in MySQL is a sequence of one or more SQL operations that are executed as a single unit. The transaction ensures that all the operations are completed successfully. If any operation in the transaction fails, the entire transaction is rolled back, maintaining the integrity of the database.
1. What is a Transaction?
In the context of databases, a transaction is a logical unit of work that contains one or more SQL operations. A transaction is designed to be atomic, consistent, isolated, and durable (ACID properties). A transaction ensures that either all changes made by the transaction are committed to the database, or none of them are if an error occurs.
2. ACID Properties
Transactions in MySQL follow the ACID properties to ensure data integrity:
- Atomicity: Ensures that all operations in a transaction are either fully completed or fully rolled back, meaning no partial changes are saved.
- Consistency: Ensures that the database transitions from one valid state to another, maintaining all constraints, rules, and relationships.
- Isolation: Ensures that transactions are executed independently, without interference from other transactions. It prevents issues such as dirty reads, non-repeatable reads, and phantom reads.
- Durability: Ensures that once a transaction is committed, its changes are permanent, even in the event of a system failure.
3. Transaction Lifecycle
The lifecycle of a transaction consists of the following steps:
- Start Transaction: Begin the transaction using the
START TRANSACTION
statement. - Execute SQL Operations: Perform SQL operations such as
INSERT
,UPDATE
, orDELETE
within the transaction. - Commit or Rollback: If all operations are successful, use
COMMIT
to save the changes. If any operation fails, useROLLBACK
to undo all changes made during the transaction.
4. Starting a Transaction
To begin a transaction in MySQL, use the START TRANSACTION
statement. This marks the beginning of a new transaction.
START TRANSACTION;
5. Committing a Transaction
If all operations within a transaction are successful, you can commit the transaction to make the changes permanent using the COMMIT
statement.
COMMIT;
6. Rolling Back a Transaction
If an error occurs or if you want to undo the changes made during the transaction, you can roll back the transaction using the ROLLBACK
statement. This will undo all changes made since the transaction started.
ROLLBACK;
7. Example: Simple Transaction
Here’s a basic example of a transaction in MySQL:
-- Start the transaction
START TRANSACTION;
-- Perform some SQL operations
INSERT INTO accounts (account_id, balance) VALUES (1, 500);
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- Commit the transaction to save changes
COMMIT;
8. Transaction Isolation Levels
Transaction isolation levels define the degree to which a transaction is isolated from the effects of other concurrent transactions. MySQL supports several isolation levels, including:
- READ UNCOMMITTED: Allows transactions to read uncommitted changes made by other transactions (lowest level of isolation).
- READ COMMITTED: Only allows transactions to read committed changes made by other transactions.
- REPEATABLE READ: Ensures that the results of a query are consistent throughout the transaction, even if other transactions modify the data in between.
- SERIALIZABLE: Ensures complete isolation, forcing transactions to execute one at a time (highest level of isolation).
To set the isolation level for a transaction, use the SET TRANSACTION ISOLATION LEVEL
statement:
SET TRANSACTION ISOLATION LEVEL SERIALIZABLE;
9. Transaction Rollback on Error
In MySQL, if a transaction is interrupted by an error, the changes made by the transaction are automatically rolled back. You can also manually trigger a rollback when an error occurs in the application logic.
-- Start a transaction
START TRANSACTION;
-- Insert data
INSERT INTO orders (order_id, customer_id) VALUES (1, 123);
-- Simulate an error
UPDATE customers SET balance = balance - 500 WHERE customer_id = 123;
-- If error occurs, rollback the transaction
ROLLBACK;
10. Saving Points in Transactions
In complex transactions, you may want to set savepoints, which are markers within a transaction. You can roll back to a specific savepoint, rather than rolling back the entire transaction.
-- Set a savepoint
SAVEPOINT my_savepoint;
-- Perform some operations
INSERT INTO orders VALUES (1, 'item');
-- Roll back to the savepoint
ROLLBACK TO SAVEPOINT my_savepoint;
11. Conclusion
Transactions in MySQL ensure that database operations are completed in a reliable and consistent manner, maintaining the integrity of the database even in the event of errors. Understanding transactions, their isolation levels, and how to commit or roll back changes is crucial for building robust and error-free database applications.
ACID Properties in MySQL
ACID properties are a set of four key principles that ensure reliable processing of database transactions. These properties—Atomicity, Consistency, Isolation, and Durability—guarantee that a database remains accurate and stable even in the event of system failures, power outages, or other unexpected disruptions.
1. Atomicity
Atomicity refers to the "all-or-nothing" property of a transaction. This means that a transaction is indivisible and irreducible. If a transaction is successful, all of its operations (such as inserts, updates, or deletes) are committed to the database. If any operation within the transaction fails, the entire transaction is rolled back, and no changes are made to the database.
-- Start a transaction
START TRANSACTION;
-- Execute multiple operations
INSERT INTO accounts (account_id, balance) VALUES (1, 500);
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- If there's an error, all changes are rolled back
ROLLBACK;
2. Consistency
Consistency ensures that a transaction brings the database from one valid state to another. After a transaction is completed, all data in the database must satisfy integrity constraints, such as foreign key constraints, unique constraints, and check constraints. If a transaction violates any constraints, it will not be committed to the database, ensuring that the database always remains in a valid state.
-- Example: Consistency is maintained by enforcing constraints
CREATE TABLE accounts (
account_id INT PRIMARY KEY,
balance DECIMAL(10,2) CHECK (balance >= 0) -- balance cannot be negative
);
3. Isolation
Isolation ensures that transactions are executed independently of each other, even if they are running concurrently. Each transaction should behave as if it is the only operation being performed on the database. This prevents issues such as dirty reads (where one transaction reads uncommitted data from another), non-repeatable reads (where data read by one transaction is modified by another before the first transaction is complete), and phantom reads (where new rows are added by another transaction during the execution of a query).
MySQL allows you to set different isolation levels to control how transactions are isolated from each other:
- READ UNCOMMITTED: Transactions can read uncommitted changes from other transactions (least isolated).
- READ COMMITTED: Transactions can only read committed data from other transactions.
- REPEATABLE READ: Guarantees that once a transaction reads data, it will see the same data throughout the transaction (most commonly used in MySQL).
- SERIALIZABLE: Ensures the highest level of isolation, where transactions are executed one after another.
-- Set the isolation level for a transaction
SET TRANSACTION ISOLATION LEVEL REPEATABLE READ;
4. Durability
Durability ensures that once a transaction is committed, its effects are permanent, even in the event of a system crash or power failure. The changes made by a committed transaction are written to disk and will not be lost. This guarantees that the database will always be in a consistent state, even after unexpected failures.
-- After committing a transaction, the data is permanent
START TRANSACTION;
INSERT INTO orders (order_id, total_amount) VALUES (1001, 250);
COMMIT;
-- Even if the system crashes after commit, the order will be saved
ACID in Action: A Complete Example
Let’s look at an example demonstrating all four ACID properties:
-- Start a transaction
START TRANSACTION;
-- Atomicity: All operations are treated as a single unit of work
INSERT INTO accounts (account_id, balance) VALUES (1, 1000);
UPDATE accounts SET balance = balance - 200 WHERE account_id = 1;
-- Consistency: Ensure data integrity by checking constraints
-- If the balance would go negative, the transaction would fail
UPDATE accounts SET balance = balance - 200 WHERE account_id = 1 AND balance >= 200;
-- Isolation: The transaction is isolated from other transactions
-- No other transaction will see these changes until the transaction is committed
-- Durability: Once the transaction is committed, the changes are permanent
COMMIT;
Why ACID Properties Are Important
ACID properties are essential for ensuring the reliability, consistency, and integrity of data in MySQL databases. By adhering to these properties, MySQL ensures that even in the face of errors or system failures, the database can recover to a consistent state, and all transactions are processed correctly.
Conclusion
In summary, ACID properties are crucial for maintaining the integrity of a database and ensuring that transactions are processed reliably. Understanding how to work with ACID principles in MySQL can help developers build robust applications that handle data safely and efficiently.
Managing Transactions: BEGIN, COMMIT, ROLLBACK
In MySQL, transactions allow you to group a series of operations into a single unit of work. This ensures that either all the operations are successfully completed (committed) or none of them are (rolled back), maintaining the integrity of the database. In MySQL, three main commands are used to manage transactions:
- BEGIN: Starts a new transaction.
- COMMIT: Commits the current transaction, making all changes permanent.
- ROLLBACK: Rolls back the current transaction, undoing all changes made since the transaction started.
1. BEGIN Transaction
The BEGIN
statement is used to start a new transaction. Once a transaction has begun, any changes made to the database will not be permanent until the COMMIT
command is issued. If an error occurs during the transaction, the changes can be undone using ROLLBACK
.
-- Start a new transaction
BEGIN;
2. COMMIT Transaction
The COMMIT
statement is used to save all changes made during the current transaction to the database. Once committed, the changes are permanent and cannot be rolled back. This is typically done after all operations in the transaction have been successfully executed.
-- Commit the transaction, making changes permanent
COMMIT;
3. ROLLBACK Transaction
The ROLLBACK
statement is used to undo all changes made during the current transaction. This is useful when an error occurs, and you want to revert the database to its previous state. After a ROLLBACK
, the database will no longer reflect any changes made since the BEGIN
statement.
-- Rollback the transaction, undoing all changes
ROLLBACK;
Transaction Example
Let’s take an example of a simple banking transaction where we transfer money from one account to another. If any step fails, we want to rollback the transaction.
-- Start a transaction
BEGIN;
-- Deduct money from account 1
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- Add money to account 2
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
-- If both updates are successful, commit the transaction
COMMIT;
Error Handling Example
If an error occurs in the middle of the transaction (for example, if one of the updates fails), we can roll back the entire transaction to ensure that no inconsistent data remains in the database.
-- Start a transaction
BEGIN;
-- Deduct money from account 1
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- Simulate an error (e.g., insufficient balance)
-- If this fails, we will rollback the transaction
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
-- If everything is fine, commit the transaction
COMMIT;
-- If there was an error, rollback the transaction
ROLLBACK;
Transaction Management in Practice
In practice, you can use BEGIN
to start a transaction, perform multiple operations, and then use either COMMIT
or ROLLBACK
depending on the outcome of the operations. This helps ensure the consistency and integrity of your data.
Conclusion
Managing transactions in MySQL allows developers to ensure that changes to the database are executed atomically, consistently, and reliably. By using the BEGIN
, COMMIT
, and ROLLBACK
statements, you can control how data is modified, ensuring that partial or failed changes do not corrupt the database.
Transaction Isolation Levels: READ UNCOMMITTED, READ COMMITTED, REPEATABLE READ, SERIALIZABLE
Transaction isolation levels define the degree to which the operations in one transaction are isolated from other concurrent transactions. These levels control the visibility of uncommitted data and how transactions interact with each other. MySQL supports four standard isolation levels:
- READ UNCOMMITTED: Allows transactions to read uncommitted changes made by other transactions.
- READ COMMITTED: Ensures that transactions only read committed data, but allows non-repeatable reads.
- REPEATABLE READ: Guarantees that if a value is read within a transaction, it will not change throughout the transaction.
- SERIALIZABLE: Ensures the highest level of isolation, where transactions are executed serially, with no concurrent execution.
1. READ UNCOMMITTED
The READ UNCOMMITTED
isolation level allows a transaction to read data that has been modified by other transactions but not yet committed. This level allows for the highest concurrency but can lead to dirty reads, where a transaction might read data that is later rolled back.
-- Set the isolation level to READ UNCOMMITTED
SET TRANSACTION ISOLATION LEVEL READ UNCOMMITTED;
2. READ COMMITTED
The READ COMMITTED
isolation level ensures that a transaction only reads data that has been committed by other transactions. This prevents dirty reads, but it still allows non-repeatable reads, meaning that the value of a field might change if it is read multiple times in a transaction.
-- Set the isolation level to READ COMMITTED
SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
3. REPEATABLE READ
The REPEATABLE READ
isolation level ensures that if a transaction reads a value, the value will not change during the transaction. It prevents non-repeatable reads by locking the data read by the transaction. However, phantom reads (where new records can appear in the result set of a query during the transaction) might still occur.
-- Set the isolation level to REPEATABLE READ
SET TRANSACTION ISOLATION LEVEL REPEATABLE READ;
4. SERIALIZABLE
The SERIALIZABLE
isolation level ensures the highest level of isolation. Transactions are executed serially, meaning that no other transactions can access the data being modified by the current transaction until it is complete. This prevents dirty reads, non-repeatable reads, and phantom reads, but it significantly reduces concurrency and can lead to performance bottlenecks.
-- Set the isolation level to SERIALIZABLE
SET TRANSACTION ISOLATION LEVEL SERIALIZABLE;
Transaction Isolation Example
Let’s consider two transactions running simultaneously. With different isolation levels, each transaction will behave differently:
-- Transaction 1
BEGIN;
-- Transaction 2 (running concurrently)
BEGIN;
-- Transaction 1 updates a row
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- Transaction 2 reads the uncommitted data (only possible with READ UNCOMMITTED)
SELECT balance FROM accounts WHERE account_id = 1;
-- Transaction 1 commits the changes
COMMIT;
-- Transaction 2 can now see the committed value (depending on isolation level)
COMMIT;
Isolation Level Comparison
Here’s a comparison of the behavior of each isolation level:
Isolation Level | Dirty Reads | Non-Repeatable Reads | Phantom Reads |
---|---|---|---|
READ UNCOMMITTED | Allowed | Allowed | Allowed |
READ COMMITTED | Not Allowed | Allowed | Allowed |
REPEATABLE READ | Not Allowed | Not Allowed | Allowed |
SERIALIZABLE | Not Allowed | Not Allowed | Not Allowed |
Conclusion
Choosing the appropriate isolation level is crucial for balancing data consistency with performance. While higher isolation levels offer more consistency and prevent anomalies like dirty reads and non-repeatable reads, they can lead to reduced performance due to increased locking and blocking. Lower isolation levels offer better performance but at the cost of potential anomalies, such as dirty or non-repeatable reads. It’s important to select the right isolation level based on the specific needs of your application.
Locking Mechanisms and Deadlock Prevention
Locking mechanisms in MySQL are essential for controlling access to data in a multi-user environment, ensuring that transactions are executed in a safe and consistent manner. Locks prevent multiple transactions from modifying the same data concurrently, which could lead to inconsistent results. However, improper use of locks can lead to deadlocks, where two or more transactions are stuck waiting for each other to release locks.
Types of Locks in MySQL
MySQL supports several locking mechanisms to control access to data:
- Table Locks: Locks the entire table, preventing other transactions from accessing the table until the lock is released. Table locks are simpler but can reduce concurrency.
- Row Locks: Locks a specific row within a table, allowing other rows in the table to be accessed by other transactions concurrently. Row-level locking provides better concurrency than table locking.
- Intent Locks: Indicate that a transaction intends to acquire a lock on a specific row or table. These locks prevent conflicts between different types of locks at the table or row level.
- Gap Locks: Used to prevent other transactions from inserting rows into a gap in the result set of a query. This prevents phantom reads and ensures that no new rows can be inserted into the result set during the transaction.
Locking Modes in MySQL
There are two primary types of locking modes in MySQL:
- Shared Locks (S): Allow a transaction to read a row but prevent other transactions from modifying it. Multiple transactions can acquire shared locks on the same row simultaneously.
- Exclusive Locks (X): Allow a transaction to modify a row, preventing other transactions from reading or modifying the locked row. Only one transaction can acquire an exclusive lock on a row at a time.
Deadlock in MySQL
A deadlock occurs when two or more transactions are each waiting for the other to release a lock, resulting in a situation where none of the transactions can proceed. Deadlocks can significantly affect database performance and should be avoided at all costs.
Deadlock Prevention and Resolution
MySQL automatically detects deadlocks and resolves them by rolling back one of the transactions involved in the deadlock. However, there are several strategies that can help prevent deadlocks:
1. Proper Lock Ordering
Ensure that transactions acquire locks in a consistent order. For example, if two transactions need to lock two tables, always lock them in the same order (e.g., always lock table1
before table2
). This reduces the chances of deadlocks occurring.
2. Lock Timeouts
Set a timeout for lock acquisitions. If a transaction is unable to acquire a lock within a specified period, it should be rolled back or retried. This prevents transactions from waiting indefinitely for a lock to be released.
-- Set a lock wait timeout of 10 seconds
SET innodb_lock_wait_timeout = 10;
3. Keeping Transactions Short
Shorter transactions are less likely to cause deadlocks because they hold locks for a shorter period of time. Try to minimize the number of operations within a transaction, and avoid user input or delays that could extend the duration of a transaction.
4. Use of ROW-Level Locking
Whenever possible, use row-level locking to reduce contention between transactions. This allows transactions to work with different rows of the same table concurrently, rather than locking entire tables.
5. Detecting Deadlocks in MySQL
MySQL provides a SHOW ENGINE INNODB STATUS
command that can be used to detect and analyze deadlocks. This command provides details about the most recent deadlock, including the transactions involved and the resources they were waiting for:
-- Show InnoDB status to check for deadlocks
SHOW ENGINE INNODB STATUS;
Example of Deadlock
Here is an example of how a deadlock might occur:
-- Transaction 1
BEGIN;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- Transaction 2 (running concurrently)
BEGIN;
UPDATE accounts SET balance = balance - 50 WHERE account_id = 2;
-- Transaction 1 tries to update account 2, but it is already locked by Transaction 2
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
-- Transaction 2 tries to update account 1, but it is already locked by Transaction 1
UPDATE accounts SET balance = balance + 50 WHERE account_id = 1;
COMMIT; -- Both transactions are stuck waiting for each other to release locks
Deadlock Detection and Resolution
In the case of a deadlock, MySQL will automatically roll back one of the transactions to resolve the deadlock. The transaction that is rolled back will receive an error indicating that it was chosen as the victim of the deadlock. The transaction can be retried or handled accordingly.
-- Example deadlock error message
ERROR 1213 (40001): Deadlock found when trying to get lock; try restarting transaction
Conclusion
Locking mechanisms play a crucial role in maintaining data integrity and consistency in a multi-user database environment. However, improper use of locks can lead to deadlocks, which can severely impact performance and result in the need for transaction rollbacks. By using proper lock ordering, timeouts, and row-level locking, and by keeping transactions short, it is possible to reduce the likelihood of deadlocks and improve database performance.
Introduction to Views: CREATE VIEW, DROP VIEW
A view in MySQL is a virtual table that provides a way to simplify complex queries. It is essentially a stored query that you can treat like a table, allowing you to encapsulate complex logic and present it in a simplified manner. Views can be used to display data from one or more tables, and they can be queried just like regular tables.
What is a View?
A view is a stored query that can be used to retrieve and display data from one or more tables. Views do not store data themselves; they only store the SQL query. When a view is queried, the SQL query defined in the view is executed, and the result is returned. Views can help improve the readability and reusability of complex queries, and they can also improve security by restricting access to specific columns or rows of a table.
Creating a View: CREATE VIEW
The CREATE VIEW
statement is used to create a new view in MySQL. A view can include data from one or more tables, and it can be based on a complex SQL query. Here is the syntax for creating a view:
CREATE VIEW view_name AS
SELECT column1, column2, ...
FROM table_name
WHERE condition;
For example, to create a view that displays employee names and their departments:
CREATE VIEW employee_departments AS
SELECT employees.name, departments.department_name
FROM employees
JOIN departments ON employees.department_id = departments.id;
After this view is created, you can query the view just like a regular table:
SELECT * FROM employee_departments;
Modifying a View
If you need to modify the definition of an existing view, you can use the CREATE OR REPLACE VIEW
statement. This will update the view with a new definition:
CREATE OR REPLACE VIEW employee_departments AS
SELECT employees.name, departments.department_name, employees.salary
FROM employees
JOIN departments ON employees.department_id = departments.id;
This command will replace the existing view with the new query, and the view will now include the employee salary along with the name and department.
Dropping a View: DROP VIEW
The DROP VIEW
statement is used to delete a view from the database. Once a view is dropped, it can no longer be used. Here is the syntax for dropping a view:
DROP VIEW view_name;
For example, to drop the employee_departments
view:
DROP VIEW employee_departments;
Advantages of Using Views
- Simplification: Views can simplify complex queries by encapsulating the complexity into a reusable object.
- Security: Views can be used to restrict access to sensitive data by showing only specific columns or rows from the base tables.
- Reusability: Once a view is created, you can use it repeatedly in multiple queries without having to rewrite the SQL logic.
- Consistency: Views help ensure that the same query is executed consistently across multiple applications or reports.
Limitations of Views
- Views do not store data; they only store the SQL query.
- Some views, especially those based on multiple tables or complex joins, may not be updatable (i.e., you cannot directly insert, update, or delete data through them).
- Views can have performance overhead, especially if they are based on complex queries or large datasets.
Example: Creating and Using a View
Let's say we have two tables: employees
and departments
, and we want to create a view that shows each employee’s name along with their department:
CREATE VIEW employee_details AS
SELECT employees.name, departments.department_name
FROM employees
JOIN departments ON employees.department_id = departments.id;
You can then query the view like this:
SELECT * FROM employee_details;
This will return a list of employee names and their corresponding department names, without needing to manually write the join each time.
Conclusion
Views in MySQL are powerful tools that help simplify complex queries, improve security, and enhance reusability. They allow you to encapsulate complex logic into a virtual table, making data retrieval easier. However, it is important to understand that views do not store data and can have performance implications, especially if based on complex queries. Use views wisely to improve your database design and data access efficiency.
Using Views in SELECT Statements
Once a view is created in MySQL, it can be used in SELECT
statements just like a regular table. Views simplify complex queries by providing a stored query that you can reference in multiple parts of your application or analysis. This section explains how to use views in SELECT
statements, including joining views with other tables, filtering data, and optimizing queries.
Querying a View
After creating a view, you can query it in the same way as a regular table. Below is an example of querying a view:
SELECT * FROM view_name;
For example, if you created a view called employee_departments
that shows employees and their departments, you can retrieve all the data from the view:
SELECT * FROM employee_departments;
Using WHERE Clause with Views
You can also filter the data returned by a view using the WHERE
clause, just like with regular tables. For instance, if you want to retrieve employees from a specific department:
SELECT * FROM employee_departments
WHERE department_name = 'Sales';
This will return only the employees who work in the 'Sales' department, based on the data in the employee_departments
view.
Using JOIN with Views
Just like tables, views can be joined with other tables or views. If you need to combine data from multiple views or tables, you can use JOIN
in your SELECT
statement:
SELECT employees.name, departments.department_name
FROM employees
JOIN employee_departments ON employees.id = employee_departments.employee_id;
In this example, you are joining the employees
table with the employee_departments
view to retrieve the names of employees and their department names.
Using GROUP BY with Views
Views can also be used with aggregate functions by applying the GROUP BY
clause to group the results. For instance, if you want to count the number of employees in each department, you can group the data from a view:
SELECT department_name, COUNT(*) AS employee_count
FROM employee_departments
GROUP BY department_name;
This query will return the number of employees in each department, based on the information stored in the employee_departments
view.
Using ORDER BY with Views
You can sort the results of a view using the ORDER BY
clause. For example, to retrieve employees ordered by their names:
SELECT * FROM employee_departments
ORDER BY name ASC;
This will return all employees in the employee_departments
view, sorted by their names in ascending order.
Performance Considerations
While using views is a great way to simplify queries, it's important to keep in mind that views do not store data and are re-executed each time they are queried. For complex views, especially those involving multiple joins or large datasets, performance can be impacted. Here are a few tips to optimize performance when using views:
- Keep Views Simple: Avoid overly complex views with multiple joins or subqueries that could slow down performance.
- Use Indexes: Ensure that the underlying tables of the view are properly indexed to speed up query execution.
- Limit the Number of Views: Using too many views in a query can cause performance issues, especially if they depend on complex operations. Consider consolidating views where possible.
Example: Combining Views with Other Queries
In this example, we will use a view and a table together to retrieve a list of employees working in the 'Sales' department, ordered by their hire date:
SELECT employees.name, employee_departments.department_name, employees.hire_date
FROM employees
JOIN employee_departments ON employees.id = employee_departments.employee_id
WHERE employee_departments.department_name = 'Sales'
ORDER BY employees.hire_date DESC;
This query uses a combination of the employees
table and the employee_departments
view to display the employee name, department, and hire date, with results filtered and sorted accordingly.
Conclusion
Using views in SELECT
statements is an efficient way to simplify complex queries, improve code reusability, and enhance data security. Views can be queried just like tables, and you can perform operations such as filtering, joining, grouping, and sorting on them. However, it's essential to consider performance implications and optimize queries when working with complex views. By following best practices, you can leverage views to improve both the clarity and performance of your SQL queries.
Benefits and Limitations of Views
Views in MySQL offer a convenient way to simplify complex queries and manage data efficiently. However, they also come with certain limitations that should be considered when designing your database. This section highlights the key benefits and limitations of using views in MySQL.
Benefits of Views
Views provide several advantages that make them a powerful tool for database design and querying:
- Simplification of Complex Queries: Views allow you to encapsulate complex logic, such as multiple joins or subqueries, into a single query. Once the view is created, you can reference it just like a table, making queries easier to write and maintain.
- Improved Code Reusability: Instead of writing the same complex query multiple times, you can create a view and reuse it in various parts of your application. This reduces duplication and promotes code consistency.
- Data Security: Views can be used to limit access to sensitive data. For example, you can create a view that only exposes a subset of columns or rows from a table, protecting sensitive information from unauthorized users.
- Logical Data Independence: Views provide a layer of abstraction between the user and the underlying data. This means that the structure of the underlying tables can change without affecting the queries that rely on the views.
- Improved Query Readability: By using views to encapsulate complex logic, you can make your queries more readable and easier to understand. This simplifies maintenance and collaboration between developers.
Limitations of Views
While views offer many benefits, they also have some limitations that can impact performance and flexibility:
- Performance Overhead: Views do not store data; they are re-executed every time they are queried. For complex views with multiple joins or subqueries, this can lead to significant performance overhead, especially when working with large datasets.
- Limited Modifiability: Views are read-only by default, which means you cannot directly update, insert, or delete data in a view. Although some simple views can be updatable, many complex views (especially those with joins or aggregations) cannot be modified directly.
- Lack of Indexing: Unlike tables, views do not have indexes. This means that queries on views cannot benefit from the performance optimizations provided by indexes. If the underlying tables are not properly indexed, query performance may suffer.
- Complexity of Nested Views: While views can simplify queries, using views within other views (nested views) can lead to performance issues and make debugging more difficult. Nested views may cause MySQL to re-execute queries multiple times, further impacting performance.
- Dependency on Base Tables: Views depend on the underlying tables they are based on. If the structure of the base table changes (e.g., columns are added or removed), the view may become invalid, requiring maintenance and adjustments to the view.
- Limited Support for DDL Operations: Views cannot be used for certain types of database design operations, such as creating or altering tables. They are strictly used for querying and cannot support direct schema modifications.
When to Use Views
Despite the limitations, views can be an essential tool in certain scenarios. Here are some cases where using views is recommended:
- Simplifying Repetitive Queries: If you find yourself writing the same complex query repeatedly, creating a view can help simplify the process and make your code more efficient.
- Providing a Layer of Abstraction: Views are ideal for abstracting the underlying database schema. For example, you can use views to expose only the necessary data to end-users, hiding the complexity of the underlying tables.
- Security and Access Control: If you need to limit access to certain data, a view can help you expose only the relevant portions of data to specific users or applications.
- Working with Aggregated Data: Views are useful when working with aggregated data, such as sum, average, or count, and can help simplify complex aggregation queries.
Conclusion
Views are a powerful tool for simplifying complex queries, improving code reusability, enhancing data security, and providing a layer of abstraction. However, they come with some performance overhead and limitations, such as the inability to modify data directly and the lack of indexing. It's important to weigh the benefits and limitations of views to determine when they are appropriate for your specific use case. When used appropriately, views can significantly improve the maintainability and readability of your database queries.
Stored Procedures: Creating, Calling, and Managing Stored Procedures
Stored procedures are precompiled SQL statements that are stored in the database and can be executed on demand. They encapsulate complex SQL logic and make it easier to reuse and manage queries. In this section, we will explore how to create, call, and manage stored procedures in MySQL.
What is a Stored Procedure?
A stored procedure is a set of SQL statements that are executed together as a single unit. Unlike regular SQL queries, stored procedures are stored in the database and can be invoked by name. They can accept parameters and return values, making them highly versatile for repetitive database operations and business logic.
Creating a Stored Procedure
To create a stored procedure in MySQL, you use the CREATE PROCEDURE
statement. The syntax includes specifying the procedure name, any input parameters, and the SQL statements that make up the procedure.
CREATE PROCEDURE procedure_name ([parameters])
BEGIN
-- SQL statements
END;
Example: Creating a Simple Stored Procedure
The following example creates a stored procedure to calculate the total salary of an employee based on their department:
CREATE PROCEDURE GetEmployeeSalary (IN department_id INT)
BEGIN
SELECT SUM(salary) AS total_salary
FROM employees
WHERE department_id = department_id;
END;
In this example:
GetEmployeeSalary
is the name of the stored procedure.department_id
is an input parameter of typeINT
.- The SQL statement inside the procedure calculates the sum of salaries for employees in the specified department.
Calling a Stored Procedure
After creating a stored procedure, you can call it using the CALL
statement. You need to provide any required parameters when calling the procedure.
CALL GetEmployeeSalary(3);
In this example, the stored procedure GetEmployeeSalary
is called with the department ID of 3. This will return the total salary for employees in that department.
Managing Stored Procedures
Once a stored procedure is created, you can manage it with various statements. Below are some common operations for managing stored procedures:
Show Existing Stored Procedures
To view a list of stored procedures in the current database, you can query the information_schema
:
SELECT ROUTINE_NAME
FROM information_schema.ROUTINES
WHERE ROUTINE_TYPE = 'PROCEDURE' AND ROUTINE_SCHEMA = 'your_database_name';
Modifying a Stored Procedure
MySQL does not allow direct modification of stored procedures. If you need to modify a stored procedure, you must first drop it and then recreate it with the new definition.
DROP PROCEDURE IF EXISTS procedure_name;
CREATE PROCEDURE procedure_name ([parameters])
BEGIN
-- Updated SQL statements
END;
Dropping a Stored Procedure
To remove a stored procedure from the database, use the DROP PROCEDURE
statement:
DROP PROCEDURE IF EXISTS procedure_name;
Example: Managing a Stored Procedure
Here is an example of dropping and creating a new version of the GetEmployeeSalary
procedure:
-- Drop the existing procedure
DROP PROCEDURE IF EXISTS GetEmployeeSalary;
-- Create a new version with updated logic
CREATE PROCEDURE GetEmployeeSalary (IN department_id INT)
BEGIN
SELECT department_id, SUM(salary) AS total_salary
FROM employees
WHERE department_id = department_id
GROUP BY department_id;
END;
Benefits of Using Stored Procedures
Stored procedures offer several benefits in database management:
- Code Reusability: Stored procedures allow you to encapsulate complex logic that can be reused across multiple applications or queries.
- Improved Performance: Since stored procedures are precompiled, they can execute faster than executing multiple individual SQL queries. This reduces the amount of work the database server must do on each request.
- Increased Security: By using stored procedures, you can limit direct access to tables and control how users interact with the database. You can grant access to specific stored procedures rather than tables directly.
- Better Maintainability: Stored procedures allow you to centralize logic in the database. If you need to make changes to the logic, you only have to do it in one place rather than in every query.
Limitations of Stored Procedures
While stored procedures are powerful, they also have some limitations:
- Limited Flexibility: Stored procedures are static and cannot adapt dynamically to different conditions like ad-hoc SQL queries.
- Complexity: As stored procedures grow in size and complexity, they can become difficult to manage and maintain.
- Not Easily Portable: Stored procedures are often specific to a particular database system, which can make it harder to migrate between different database platforms.
Conclusion
Stored procedures are a powerful tool for encapsulating and optimizing SQL logic. They offer benefits such as code reusability, improved performance, enhanced security, and better maintainability. However, they also have limitations like reduced flexibility and complexity. Understanding when and how to use stored procedures effectively can significantly improve the performance and manageability of your database operations.
Parameters and Return Values in Stored Procedures
Stored procedures in MySQL can accept parameters and return values to make them more dynamic and reusable. This section explains how to pass input and output parameters to stored procedures, as well as how to return values from a procedure.
What are Parameters in Stored Procedures?
Parameters are values passed to a stored procedure when it is called. They allow stored procedures to handle dynamic input and provide more flexibility. There are two types of parameters:
- IN Parameters: These parameters are used to pass values into the stored procedure. They are read-only within the procedure.
- OUT Parameters: These parameters allow the stored procedure to return a value to the caller. They are used for output from the procedure.
- INOUT Parameters: These parameters can be used for both input and output. They allow values to be passed in and also modified within the procedure.
Creating Stored Procedures with Parameters
When creating a stored procedure, you define the parameters after the procedure name. Below is the syntax for creating a stored procedure with different types of parameters:
CREATE PROCEDURE procedure_name (IN param1 datatype, OUT param2 datatype, INOUT param3 datatype)
BEGIN
-- SQL statements
END;
Example: Creating a Stored Procedure with IN, OUT, and INOUT Parameters
This example demonstrates how to create a stored procedure that accepts an IN
parameter, returns an OUT
parameter, and modifies an INOUT
parameter:
CREATE PROCEDURE CalculateEmployeeBonus (IN employee_id INT, OUT bonus_amount DECIMAL(10, 2), INOUT performance_rating INT)
BEGIN
-- Calculate the bonus based on performance rating
IF performance_rating >= 8 THEN
SET bonus_amount = 1000;
ELSE
SET bonus_amount = 500;
END IF;
-- Modify the performance rating (INOUT parameter)
SET performance_rating = performance_rating + 1;
END;
In this example:
employee_id
is anIN
parameter that accepts the employee's ID.bonus_amount
is anOUT
parameter that returns the calculated bonus.performance_rating
is anINOUT
parameter that is both input and output (it is modified within the procedure).
Calling Stored Procedures with Parameters
To call a stored procedure with parameters, you need to provide the necessary values when calling the procedure. Here is an example of calling the stored procedure CalculateEmployeeBonus
:
-- Declare a variable to hold the bonus amount
SET @bonus DECIMAL(10, 2);
-- Declare a variable for the performance rating
SET @rating INT = 7;
-- Call the stored procedure with parameters
CALL CalculateEmployeeBonus(101, @bonus, @rating);
-- Display the results
SELECT @bonus AS BonusAmount, @rating AS UpdatedPerformanceRating;
In this example:
@bonus
is a variable used to store the output value of the bonus.@rating
is a variable that holds the performance rating. It will be updated by the procedure.- The procedure is called with the employee ID (101), and the bonus and performance rating values are returned in the
@bonus
and@rating
variables.
Returning Values from a Stored Procedure
Stored procedures do not directly return values like functions do. However, they can pass values back through OUT
or INOUT
parameters, or by using a SELECT
statement to return data. If you need a return value from a stored procedure, you should use OUT
parameters or SELECT
to return values to the caller.
Example: Returning Data Using SELECT
In this example, we’ll create a stored procedure that returns the total salary of an employee using a SELECT
statement:
CREATE PROCEDURE GetEmployeeSalary (IN employee_id INT)
BEGIN
SELECT salary FROM employees WHERE id = employee_id;
END;
When the procedure is called, it will return the salary of the employee with the specified employee_id
.
CALL GetEmployeeSalary(101);
Advantages of Using Parameters in Stored Procedures
Using parameters in stored procedures offers several advantages:
- Modularity: Parameters allow you to create reusable procedures that can operate on different data sets.
- Security: Parameters help prevent SQL injection attacks by ensuring that input is treated as data and not executable code.
- Flexibility: With parameters, stored procedures can be customized to perform different operations based on the input values.
Conclusion
Parameters are a powerful feature of stored procedures, allowing them to be more dynamic and reusable. By using IN
, OUT
, and INOUT
parameters, you can pass data into and out of stored procedures and modify them as needed. Understanding how to create and call stored procedures with parameters is essential for making the most of stored procedures in MySQL.
Stored Functions and Triggers
Stored functions and triggers are important tools in MySQL that allow you to encapsulate logic and automate database tasks. They can be used to perform operations when data is modified or to calculate values based on input parameters. This section covers the basics of creating and using stored functions and triggers in MySQL.
What are Stored Functions?
A stored function is similar to a stored procedure but is designed to return a value. It is a user-defined function that is stored in the database and can be invoked in SQL queries. Stored functions are used to encapsulate logic that can be reused across multiple queries.
Creating Stored Functions
To create a stored function, you use the CREATE FUNCTION
statement. A function can take input parameters and return a value. Below is the syntax for creating a stored function:
CREATE FUNCTION function_name (parameter_list)
RETURNS return_type
DETERMINISTIC
BEGIN
-- Function logic
RETURN value;
END;
Example: Creating a Stored Function
This example shows how to create a stored function that calculates the total price after applying a discount:
CREATE FUNCTION CalculateDiscountPrice(original_price DECIMAL(10, 2), discount_rate DECIMAL(5, 2))
RETURNS DECIMAL(10, 2)
DETERMINISTIC
BEGIN
DECLARE discounted_price DECIMAL(10, 2);
SET discounted_price = original_price - (original_price * discount_rate);
RETURN discounted_price;
END;
In this example:
original_price
anddiscount_rate
are input parameters.- The function returns the
discounted_price
after applying the discount.
Calling Stored Functions
Once a stored function is created, it can be called in a SQL query just like a built-in MySQL function:
SELECT CalculateDiscountPrice(100, 0.15) AS discounted_price;
This will return the discounted price of 100 after applying a 15% discount.
What are Triggers?
A trigger in MySQL is a set of SQL statements that are automatically executed or "triggered" when a specific event occurs on a table or view. Triggers can be used to enforce business rules, maintain audit logs, or update related data automatically.
Creating Triggers
Triggers are created using the CREATE TRIGGER
statement. They are associated with a specific event (INSERT, UPDATE, or DELETE) and occur before or after the event is executed. Below is the basic syntax for creating a trigger:
CREATE TRIGGER trigger_name
{BEFORE | AFTER} {INSERT | UPDATE | DELETE}
ON table_name
FOR EACH ROW
BEGIN
-- Trigger logic
END;
Example: Creating a Trigger
This example shows how to create a trigger that automatically updates the last_updated
column whenever a row in the employees
table is updated:
CREATE TRIGGER update_employee_timestamp
AFTER UPDATE
ON employees
FOR EACH ROW
BEGIN
UPDATE employees
SET last_updated = NOW()
WHERE id = OLD.id;
END;
In this example:
- The trigger
update_employee_timestamp
runs AFTER UPDATE on theemployees
table. - It automatically updates the
last_updated
column with the current timestamp whenever a row is updated. OLD
is used to refer to the value of the column before the update.
Trigger Events and Timing
Triggers can be defined for the following events:
- INSERT: Triggered when a new row is inserted into the table.
- UPDATE: Triggered when an existing row is updated.
- DELETE: Triggered when a row is deleted from the table.
Triggers can also be defined to execute BEFORE or AFTER the event:
- BEFORE: The trigger is executed before the operation (INSERT/UPDATE/DELETE) is performed.
- AFTER: The trigger is executed after the operation is performed.
Managing Stored Functions and Triggers
You can manage stored functions and triggers using the following commands:
DROP FUNCTION
— Deletes a stored function from the database.DROP TRIGGER
— Deletes a trigger from the database.
Example:
-- Dropping a function
DROP FUNCTION CalculateDiscountPrice;
-- Dropping a trigger
DROP TRIGGER update_employee_timestamp;
Advantages of Using Stored Functions and Triggers
Stored functions and triggers provide several benefits:
- Automation: Triggers automate tasks like updating related tables or enforcing constraints, reducing the need for manual intervention.
- Reusability: Stored functions can be reused in multiple queries, making them efficient for repetitive tasks.
- Encapsulation: Logic is encapsulated within the database, ensuring it is executed consistently across different applications.
- Data Integrity: Triggers can enforce business rules and maintain data integrity by preventing invalid operations.
Limitations of Stored Functions and Triggers
While stored functions and triggers are powerful tools, they come with some limitations:
- Performance: Overuse of triggers can lead to performance issues, especially if they are triggered frequently or on large data sets.
- Debugging: Stored functions and triggers can be difficult to debug, especially when errors occur silently or affect large portions of data.
- Complexity: Complex logic within stored functions or triggers can make the database schema harder to maintain and understand.
Conclusion
Stored functions and triggers are powerful features in MySQL that allow you to automate tasks, enforce data integrity, and encapsulate logic directly in the database. Understanding how to create, manage, and use stored functions and triggers is essential for developing efficient and reliable database applications.
Creating and Managing MySQL Users
In MySQL, user management is an essential part of database security. MySQL allows you to create users, grant them specific privileges, and revoke those privileges when necessary. This section covers how to create and manage users in MySQL using the CREATE USER
, GRANT
, and REVOKE
commands.
Creating MySQL Users
The CREATE USER
statement is used to create a new MySQL user. When creating a user, you can specify the username, the host from which the user can connect, and optionally, a password. The general syntax for creating a user is:
CREATE USER 'username'@'host' IDENTIFIED BY 'password';
Example: Creating a New User
The following example creates a user named 'john'
who can connect from any host ('%'
) and has the password 'password123'
:
CREATE USER 'john'@'%' IDENTIFIED BY 'password123';
In this example:
'john'
is the username.'%'
means the user can connect from any host. You can replace it with a specific IP address or hostname for more restricted access.'password123'
is the password for the user.
Granting Privileges to MySQL Users
Once a user is created, you can grant them specific privileges to access databases and perform various operations. The GRANT
statement is used to assign privileges to a user. The general syntax is:
GRANT privileges ON database_name.* TO 'username'@'host';
Example: Granting Privileges
This example grants SELECT
and INSERT
privileges on the employees
database to the user 'john'
:
GRANT SELECT, INSERT ON employees.* TO 'john'@'%';
This means:
- The user
'john'
can performSELECT
andINSERT
operations on all tables within theemployees
database. '%'
allows'john'
to connect from any host.
Granting All Privileges
If you want to grant all privileges to a user on a specific database, you can use the ALL PRIVILEGES
keyword:
GRANT ALL PRIVILEGES ON employees.* TO 'john'@'%';
This allows 'john'
to perform any operation (SELECT, INSERT, UPDATE, DELETE, etc.) on the employees
database.
Revoking Privileges from MySQL Users
The REVOKE
statement is used to remove privileges from a user. The general syntax for revoking privileges is:
REVOKE privileges ON database_name.* FROM 'username'@'host';
Example: Revoking Privileges
This example revokes the INSERT
privilege from the user 'john'
on the employees
database:
REVOKE INSERT ON employees.* FROM 'john'@'%';
After running this command, 'john'
will no longer be able to perform INSERT
operations on the employees
database, but they will still have their SELECT
privilege.
Flushing Privileges
After granting or revoking privileges, you should run the FLUSH PRIVILEGES
command to ensure that MySQL reloads the privilege tables and applies the changes:
FLUSH PRIVILEGES;
Viewing User Privileges
To view the privileges granted to a specific user, you can use the SHOW GRANTS
command:
SHOW GRANTS FOR 'john'@'%';
This will display a list of all the privileges granted to 'john'
.
Dropping MySQL Users
To delete a MySQL user, use the DROP USER
statement:
DROP USER 'john'@'%';
This will permanently remove the user 'john'
and revoke all their privileges.
Managing Passwords for MySQL Users
You can change the password for a user using the SET PASSWORD
statement:
SET PASSWORD FOR 'john'@'%' = PASSWORD('newpassword123');
Alternatively, you can use the ALTER USER
statement in newer versions of MySQL:
ALTER USER 'john'@'%' IDENTIFIED BY 'newpassword123';
Best Practices for Managing MySQL Users
- Limit Privileges: Always follow the principle of least privilege. Grant only the necessary privileges to users.
- Use Strong Passwords: Ensure that MySQL users have strong, secure passwords to prevent unauthorized access.
- Regularly Review Privileges: Periodically check the privileges granted to users and revoke any unnecessary ones.
- Use Specific Hosts: Instead of using
'%'
(wildcard for any host), specify the exact host or IP address whenever possible to limit access to the database.
Conclusion
Creating and managing MySQL users is an essential part of database security. By using the CREATE USER
, GRANT
, and REVOKE
statements, you can control access to your MySQL databases and ensure that only authorized users can perform certain actions. Always follow best practices to minimize security risks and protect your data.
Privileges and Access Control in MySQL
In MySQL, privileges are assigned to users in order to control what operations they can perform on databases and tables. Access control ensures that users can only perform actions for which they have permission. This section explains the concept of MySQL privileges, access control mechanisms, and how to manage them using various commands.
What are MySQL Privileges?
Privileges in MySQL define the actions a user can perform. These actions can be granted on databases, tables, views, or even individual columns. Privileges can be granted at different levels, such as global, database, table, or column level. Some common MySQL privileges include:
- SELECT: Allows reading data from tables.
- INSERT: Allows inserting new rows into a table.
- UPDATE: Allows modifying existing data in a table.
- DELETE: Allows deleting data from a table.
- ALL PRIVILEGES: Grants all available privileges to the user on a specific database or table.
- CREATE: Allows creating new databases or tables.
- DROP: Allows deleting databases or tables.
- ALTER: Allows modifying the structure of tables (e.g., adding or dropping columns).
- GRANT OPTION: Allows a user to grant privileges to other users.
Privilege Types and Levels
Privileges can be granted at various levels:
- Global Level: Privileges granted at the global level apply to all databases and objects in the MySQL server. These privileges are granted using the
'*.*'
syntax. For example,GRANT ALL PRIVILEGES ON *.* TO 'user'@'%'
grants all privileges on all databases to the user. - Database Level: Privileges granted at the database level apply to all objects within a specific database. The syntax for this is
GRANT privileges ON database_name.* TO 'user'@'host'
. - Table Level: Privileges granted at the table level apply to specific tables within a database. Syntax:
GRANT privileges ON database_name.table_name TO 'user'@'host'
. - Column Level: Privileges granted at the column level apply to specific columns of a table. Syntax:
GRANT privileges ON database_name.table_name(column_name) TO 'user'@'host'
.
Granting Privileges
The GRANT
statement is used to assign privileges to a user. Here is the general syntax:
GRANT privileges ON object TO 'username'@'host';
Example: Granting Privileges
The following example grants SELECT
and INSERT
privileges on the employees
database to the user 'john'
:
GRANT SELECT, INSERT ON employees.* TO 'john'@'%';
This grants 'john'
the ability to read and insert data into any table within the employees
database.
Revoking Privileges
To remove privileges from a user, you can use the REVOKE
statement. This is similar to the GRANT
statement, but it removes the specified privileges.
REVOKE privileges ON object FROM 'username'@'host';
Example: Revoking Privileges
This example revokes the INSERT
privilege on the employees
database from the user 'john'
:
REVOKE INSERT ON employees.* FROM 'john'@'%';
Viewing Privileges
To view the privileges granted to a specific user, the SHOW GRANTS
statement can be used:
SHOW GRANTS FOR 'john'@'%';
This will display the privileges assigned to the user 'john'
on the MySQL server. It will show the exact privileges granted for each database, table, or column.
Privilege Inheritance and the GRANT OPTION
If a user has the GRANT OPTION
privilege, they can grant the same privileges to other users. This allows for hierarchical privilege management. However, granting privileges with the GRANT OPTION
can be risky if not handled carefully.
Example: Granting Privileges with GRANT OPTION
The following example grants ALL PRIVILEGES
on the employees
database to the user 'john'
, with the ability to grant those privileges to others:
GRANT ALL PRIVILEGES ON employees.* TO 'john'@'%' WITH GRANT OPTION;
This gives 'john'
full control over the employees
database and allows them to grant those privileges to other users.
Access Control Best Practices
- Principle of Least Privilege: Always grant only the necessary privileges to users. Avoid granting unnecessary or broad privileges like
ALL PRIVILEGES
. - Use Strong Passwords: Ensure that MySQL users have strong, secure passwords to prevent unauthorized access.
- Use Specific Hosts: Restrict access by using specific hosts or IP addresses rather than the wildcard
'%'
to limit potential attackers. - Review Privileges Regularly: Periodically review the privileges granted to users to ensure they are still appropriate and remove any unnecessary privileges.
- Use Roles: MySQL 8.0 and above supports roles, which allow you to group sets of privileges and assign them to users. Use roles for better privilege management.
Conclusion
Privilege and access control are fundamental to MySQL security. By carefully managing privileges, you can ensure that only authorized users can perform certain actions on your databases. Always follow best practices to minimize security risks and protect your data from unauthorized access.
Managing User Authentication in MySQL
In MySQL, user authentication is a critical component of database security. It ensures that only authorized users can access the database. This section explains how to manage user authentication in MySQL, including password policies and authentication plugins.
What is User Authentication?
User authentication in MySQL involves verifying the identity of users who attempt to connect to the MySQL server. Authentication ensures that only users with valid credentials (such as a username and password) are allowed to access the database. MySQL provides several methods for managing authentication, including password policies and the use of authentication plugins.
Managing Password Policies
MySQL allows administrators to enforce password policies to ensure that passwords are strong and secure. A password policy is a set of rules that governs the complexity and strength of passwords used by MySQL users.
Enabling Password Validation Plugin
MySQL provides the validate_password
plugin, which helps enforce password strength rules. The plugin can be configured to check passwords against various criteria, such as length, character complexity, and whether they are commonly used passwords.
INSTALL PLUGIN validate_password SONAME 'validate_password.so';
This command installs the validate_password
plugin on the MySQL server.
Configuring Password Validation Rules
The password validation plugin offers the following options to control password strength:
- validate_password_length: Sets the minimum length for passwords (default is 8 characters).
- validate_password_mixed_case_count: Specifies the number of uppercase and lowercase characters required.
- validate_password_number_count: Specifies the number of numeric characters required.
- validate_password_special_char_count: Specifies the number of special characters required.
- validate_password_policy: Defines the overall policy strength. It can have the following values:
- LOW: Requires only a minimum password length.
- MEDIUM: Enforces mixed case, numeric, and special character requirements.
- STRONG: Requires a complex password that meets the policies for length and character diversity.
Example: Configuring a Strong Password Policy
This example sets a strong password policy that requires a minimum password length of 12 characters and at least one uppercase letter, one number, and one special character:
SET GLOBAL validate_password_policy = 'STRONG';
SET GLOBAL validate_password_length = 12;
SET GLOBAL validate_password_mixed_case_count = 1;
SET GLOBAL validate_password_number_count = 1;
SET GLOBAL validate_password_special_char_count = 1;
Authentication Plugins
MySQL provides multiple authentication plugins that control how users authenticate to the server. These plugins extend MySQL’s authentication features and allow for integration with external authentication systems like LDAP, PAM, and more.
Common MySQL Authentication Plugins
- mysql_native_password: The default authentication plugin in MySQL. It uses a hashed password stored in the
mysql.user
table. - caching_sha2_password: A more secure authentication plugin that uses SHA-256 hashing and provides better security than
mysql_native_password
. - sha256_password: Uses SHA-256 for authentication and can work with SSL/TLS for secure password transmission.
- auth_socket: Provides authentication based on the operating system’s user credentials, allowing users to log in automatically if they are logged into the operating system.
- pam: Allows MySQL to authenticate users using the Pluggable Authentication Modules (PAM) system.
- ldap: Provides authentication through an LDAP directory service.
Changing Authentication Plugin
To change the authentication plugin for a user, you can use the ALTER USER
statement. For example, to change a user’s authentication plugin to caching_sha2_password
, use the following command:
ALTER USER 'username'@'host' IDENTIFIED WITH caching_sha2_password;
Example: Changing Authentication Plugin
This example changes the authentication plugin for the user 'john'
to caching_sha2_password
:
ALTER USER 'john'@'localhost' IDENTIFIED WITH caching_sha2_password;
Managing User Accounts and Password Expiry
MySQL allows administrators to manage user passwords, including setting expiration dates for passwords and enforcing password changes after a certain period.
Setting Password Expiry
You can set a password expiration period by using the DEFAULT PASSWORD EXPIRE
option when creating a user, or you can alter an existing user’s password expiration settings:
ALTER USER 'username'@'host' PASSWORD EXPIRE INTERVAL 90 DAY;
This command forces the user to change their password every 90 days.
Best Practices for User Authentication
- Use Strong Passwords: Always enforce strong password policies that require a mix of uppercase, lowercase, numeric, and special characters.
- Use Secure Authentication Plugins: Consider using
caching_sha2_password
orsha256_password
for enhanced security. - Limit User Access: Limit user access to the database by granting the minimum required privileges and using secure authentication methods.
- Enforce Password Expiry: Set password expiry policies to ensure that users change their passwords periodically to reduce the risk of unauthorized access.
- Use SSL/TLS: Enable SSL/TLS encryption for user authentication to protect passwords during transmission over the network.
Conclusion
Managing user authentication in MySQL is essential for securing your database. By enforcing strong password policies, using secure authentication plugins, and implementing password expiry policies, you can ensure that your MySQL server is protected from unauthorized access. Always follow best practices to maintain high security and minimize the risk of data breaches.
Encryption Techniques in MySQL
Encryption is a vital aspect of database security, used to protect sensitive data by converting it into a format that is unreadable without the appropriate decryption key. MySQL provides several methods for encrypting data, both at rest and in transit. In this section, we'll explore the different encryption techniques available in MySQL and how they can help safeguard your data.
What is Encryption?
Encryption in MySQL refers to the process of encoding data in such a way that only authorized users or systems can decode it back to its original form. This can be applied to data at rest (stored data) or data in transit (data being transferred over the network).
Types of Encryption in MySQL
MySQL offers two primary types of encryption: Data-at-Rest Encryption and Data-in-Transit Encryption.
1. Data-at-Rest Encryption
Data-at-rest refers to data that is stored in a database, such as user credentials, credit card numbers, and other sensitive information. Encryption of this data ensures it remains secure even if an unauthorized person gains access to the physical storage system.
Transparent Data Encryption (TDE)
MySQL Enterprise Edition supports Transparent Data Encryption (TDE), which encrypts the entire database (including logs and backups) without modifying the applications that access the database. TDE ensures that data is encrypted on disk but remains transparent to the applications querying it.
Encrypting Individual Columns
You can encrypt individual columns in MySQL using the AES_ENCRYPT()
and AES_DECRYPT()
functions. These functions use the Advanced Encryption Standard (AES) algorithm to encrypt and decrypt data.
Example: Encrypting Data in a Column
The following example shows how to encrypt and decrypt data in MySQL using the AES_ENCRYPT()
and AES_DECRYPT()
functions:
-- Encrypting a user's credit card number
UPDATE users
SET credit_card = AES_ENCRYPT('1234-5678-9876-5432', 'encryption_key')
WHERE user_id = 1;
-- Decrypting the user's credit card number
SELECT AES_DECRYPT(credit_card, 'encryption_key') AS decrypted_credit_card
FROM users
WHERE user_id = 1;
In this example, the credit card number is encrypted using the AES algorithm with the key 'encryption_key'
.
2. Data-in-Transit Encryption
Data-in-transit refers to data being transferred between the MySQL server and clients. Encrypting data in transit ensures that sensitive data is protected from eavesdropping and tampering during transmission.
SSL/TLS Encryption
MySQL supports SSL (Secure Sockets Layer) and TLS (Transport Layer Security) encryption for data in transit. By using SSL/TLS, MySQL clients and servers can encrypt all communication, including queries and responses, ensuring the security of the transmitted data.
Setting Up SSL/TLS Encryption in MySQL
To enable SSL/TLS encryption, you need to generate SSL certificates and configure the MySQL server and client accordingly.
Steps to Enable SSL in MySQL:
- Generate SSL certificates using OpenSSL:
- Configure the MySQL server to use SSL by updating the
my.cnf
file with the paths to the generated certificate and key files: - Restart the MySQL server to apply the changes.
- On the client side, configure the MySQL client to connect using SSL by providing the necessary certificates:
openssl genpkey -algorithm RSA -out server-key.pem
openssl req -new -key server-key.pem -out server-req.pem
openssl x509 -req -in server-req.pem -signkey server-key.pem -out server-cert.pem
[mysqld]
ssl-ca = /path/to/ca-cert.pem
ssl-cert = /path/to/server-cert.pem
ssl-key = /path/to/server-key.pem
mysql -u username -p --ssl-ca=/path/to/ca-cert.pem --ssl-cert=/path/to/client-cert.pem --ssl-key=/path/to/client-key.pem
Encryption Best Practices
- Use Strong Encryption Keys: Always use long, randomly generated keys for encryption to ensure the security of your data.
- Encrypt Sensitive Data: Encrypt sensitive data such as passwords, credit card numbers, and personal information both at rest and in transit.
- Enable SSL/TLS for Data-in-Transit: Always enable SSL/TLS encryption for client-server communication to protect data from eavesdropping.
- Secure Your Keys: Store encryption keys securely, and limit access to them. Avoid hardcoding keys directly in the application code.
- Regularly Rotate Keys: Periodically rotate encryption keys to reduce the risk of key compromise.
Limitations of MySQL Encryption
- Performance Impact: Encryption adds overhead to both disk I/O and network traffic, which can affect performance. It is important to balance security and performance when using encryption.
- Key Management: Proper key management is crucial. If an encryption key is lost or compromised, it can lead to data loss or unauthorized access.
- Limited Features in MySQL Community Edition: Some advanced encryption features, such as Transparent Data Encryption (TDE), are available only in the MySQL Enterprise Edition.
Conclusion
Encryption is an essential security measure for protecting sensitive data in MySQL. Whether you're encrypting data at rest with AES, using SSL/TLS for data in transit, or leveraging MySQL’s built-in encryption functions, it is crucial to ensure that your data remains secure from unauthorized access. By following best practices and using the appropriate encryption techniques, you can enhance the security of your MySQL database and safeguard your data.
MySQL Roles and Best Practices for User Management
Managing users and their privileges is a critical part of database security. In MySQL, user management involves creating users, assigning roles, and controlling access to various database resources. In this section, we will discuss how to manage MySQL users effectively, the use of roles, and best practices to ensure secure and efficient user management.
What are MySQL Roles?
A role in MySQL is a collection of privileges that can be granted to users. Roles allow for easier user management by grouping together common sets of privileges. Once a role is created, it can be assigned to multiple users, simplifying the process of managing permissions across users with similar responsibilities.
Creating and Managing Users in MySQL
In MySQL, users are created using the CREATE USER
statement, and their privileges are granted using the GRANT
statement. It is important to assign only the necessary privileges to each user based on their role to minimize the risk of unauthorized access.
Creating a New User
To create a new user in MySQL, the CREATE USER
statement is used. You can specify the user's username, password, and the host from which the user can connect.
Example: Creating a User
-- Creating a new user with a password
CREATE USER 'new_user'@'localhost' IDENTIFIED BY 'password123';
Granting Privileges to a User
Once a user is created, you can grant specific privileges to the user based on the tasks they will be performing. The GRANT
statement is used to assign privileges such as SELECT, INSERT, UPDATE, DELETE, and more.
Example: Granting Privileges
-- Granting SELECT and INSERT privileges on a specific database
GRANT SELECT, INSERT ON database_name.* TO 'new_user'@'localhost';
Revoking Privileges from a User
If you need to revoke privileges from a user, the REVOKE
statement is used. This is helpful for removing access when a user's responsibilities change or they no longer require access to certain resources.
Example: Revoking Privileges
-- Revoking SELECT privilege from a user
REVOKE SELECT ON database_name.* FROM 'new_user'@'localhost';
MySQL Roles
MySQL allows you to create and manage roles for better user privilege management. A role is a set of privileges that can be assigned to users. Roles are especially helpful when multiple users require the same set of permissions.
Creating a Role
To create a role in MySQL, you use the CREATE ROLE
statement. After creating a role, you can grant the necessary privileges to it. Once the role is created and assigned privileges, it can be granted to individual users.
Example: Creating a Role
-- Creating a new role with no privileges
CREATE ROLE 'read_only';
Granting Privileges to a Role
After creating a role, you can assign privileges to it using the GRANT
statement. This allows you to manage the permissions for a group of users efficiently by assigning the role to them.
Example: Granting Privileges to a Role
-- Granting SELECT privilege to the read_only role
GRANT SELECT ON database_name.* TO 'read_only';
Assigning a Role to a User
Once a role is created and privileges are assigned, it can be granted to a user using the GRANT
statement. This is useful when you want to assign the same set of privileges to multiple users without granting them individually.
Example: Assigning a Role to a User
-- Assigning the read_only role to a user
GRANT 'read_only' TO 'new_user'@'localhost';
Setting Default Roles
You can set a default role for a user, meaning that when the user logs in, the role is automatically activated. This ensures that the user always has the required privileges without having to manually assign the role each time.
Example: Setting a Default Role
-- Setting the default role for a user
SET DEFAULT ROLE 'read_only' TO 'new_user'@'localhost';
Best Practices for User Management
Following best practices for user management is essential for ensuring security and efficiency in a MySQL environment. Here are some key best practices for managing MySQL users:
- Principle of Least Privilege: Always grant users the minimum privileges necessary to perform their tasks. Avoid granting unnecessary permissions like
ALL PRIVILEGES
unless absolutely required. - Use Roles for Easier Management: Use roles to group privileges that are commonly used together. This makes it easier to manage users, especially in large organizations.
- Regularly Review User Privileges: Periodically review user privileges to ensure they align with the user’s job responsibilities. Revoke any unnecessary privileges.
- Enable Password Policies: Enforce strong password policies to ensure user accounts are protected. This can include password complexity, expiration, and history policies.
- Limit Host Access: Restrict user access to specific hosts or IP addresses. This reduces the risk of unauthorized access from external sources.
- Use SSL/TLS for Secure Connections: Always use SSL/TLS encryption for connections to ensure that sensitive data like passwords and queries are encrypted during transmission.
- Audit User Activity: Enable auditing to track user activity, especially for privileged accounts. This can help detect suspicious actions or potential security breaches.
Conclusion
Managing MySQL users and roles is essential for maintaining database security and operational efficiency. By using roles to group privileges, assigning the appropriate permissions to users, and following best practices for user management, you can ensure that your MySQL database is both secure and well-organized. Regularly reviewing user access and privileges, enforcing strong passwords, and using SSL/TLS encryption are key components of a secure MySQL environment.
Securing MySQL Server with Firewalls and Access Controls
Securing your MySQL server is essential to protect your data and ensure that unauthorized users cannot access or manipulate the database. Firewalls and access controls are key components in securing MySQL and ensuring that only authorized users and services can interact with the server.
Why Securing MySQL is Important
MySQL is a critical part of many applications, and securing it ensures that sensitive data remains protected from unauthorized access, loss, or damage. Without proper security measures, MySQL databases are vulnerable to attacks, including SQL injection, brute-force login attempts, and unauthorized access from the network.
Securing MySQL with Firewalls
Firewalls are a fundamental security measure that protect your MySQL server from unwanted traffic. By configuring a firewall, you can restrict access to the MySQL server to only trusted IP addresses or networks, reducing the attack surface.
Setting Up a Firewall for MySQL
To secure your MySQL server, you can configure a firewall to allow access only from trusted sources. Below are examples for configuring firewalls with iptables and ufw (Uncomplicated Firewall).
Example 1: Using iptables
If you are using iptables
on your server, you can allow MySQL access only from specific IP addresses by modifying the firewall rules. Below is an example to allow connections only from a trusted IP address (e.g., 192.168.1.100
) and block others:
Allowing MySQL Access from a Specific IP
# Allow MySQL access from trusted IP address
sudo iptables -A INPUT -p tcp -s 192.168.1.100 --dport 3306 -j ACCEPT
# Block access from all other IP addresses
sudo iptables -A INPUT -p tcp --dport 3306 -j DROP
Example 2: Using UFW
If you are using ufw
, you can allow MySQL access from a specific IP address by running the following command:
Allowing MySQL Access with UFW
# Allow MySQL access from a specific IP
sudo ufw allow from 192.168.1.100 to any port 3306
# Deny access from all other sources
sudo ufw deny 3306
MySQL Access Control: User Authentication and Permissions
Access control in MySQL involves managing user authentication and privileges. You should follow the principle of least privilege to ensure that users can only access the necessary parts of the database to perform their tasks. Properly managing MySQL user accounts and their access rights is a crucial step in securing your server.
Managing User Privileges
MySQL uses a privilege system that controls access to databases, tables, and specific operations (e.g., SELECT, INSERT, UPDATE). You can assign privileges to users using the GRANT
statement and revoke them using the REVOKE
statement.
Example: Granting and Revoking Privileges
-- Granting SELECT privilege to a user
GRANT SELECT ON database_name.* TO 'username'@'hostname';
-- Revoking SELECT privilege from a user
REVOKE SELECT ON database_name.* FROM 'username'@'hostname';
Using Strong Passwords
Ensuring that MySQL users have strong passwords is another important aspect of securing the server. You can implement strong password policies to enforce complex passwords that are difficult to guess. MySQL provides the validate_password
plugin, which can help you enforce password strength policies.
Example: Enabling the validate_password Plugin
-- Enabling the validate_password plugin
INSTALL PLUGIN validate_password SONAME 'validate_password.so';
-- Setting password policy level (LOW, MEDIUM, or STRONG)
SET GLOBAL validate_password.policy = MEDIUM;
Securing MySQL with SSL/TLS
Encrypting MySQL connections using SSL/TLS helps protect sensitive data during transmission, especially when accessing the server remotely. SSL/TLS encryption ensures that data is encrypted between the client and the server, preventing eavesdropping and man-in-the-middle attacks.
Enabling SSL for MySQL
To enable SSL for MySQL, you need to generate SSL certificates, configure MySQL to use these certificates, and then enforce SSL connections for specific users.
Example: Enabling SSL in MySQL
-- Enabling SSL support in the MySQL configuration
[mysqld]
ssl-ca=/path/to/ca-cert.pem
ssl-cert=/path/to/server-cert.pem
ssl-key=/path/to/server-key.pem
-- Restart MySQL to apply changes
sudo service mysql restart
Once SSL is enabled, you can enforce SSL connections for specific users by using the REQUIRE SSL
clause:
Example: Enforcing SSL for a User
-- Enforcing SSL for user connections
GRANT ALL PRIVILEGES ON database_name.* TO 'username'@'hostname' REQUIRE SSL;
Securing MySQL with Hosts and IP Restrictions
Restricting access based on host or IP address can significantly reduce the surface area for attacks. By configuring MySQL to allow access only from certain trusted hosts or IP addresses, you can effectively limit unauthorized access attempts.
Limiting Host Access
When creating MySQL users, you can specify the host or IP address from which they can connect. This limits the access to only trusted sources, and prevents users from connecting from unapproved locations.
Example: Limiting Host Access
-- Creating a user with access limited to a specific IP
CREATE USER 'username'@'192.168.1.100' IDENTIFIED BY 'password';
Best Practices for Securing MySQL Server
- Use Firewalls and IP Restrictions: Use firewalls like iptables or ufw to limit access to MySQL to trusted IP addresses and networks only.
- Enforce Strong Password Policies: Use the
validate_password
plugin to enforce strong password policies for MySQL users. - Use SSL/TLS Encryption: Enable SSL/TLS for MySQL connections to encrypt data during transmission and prevent eavesdropping.
- Limit User Privileges: Use the principle of least privilege and grant users only the permissions they need to perform their tasks.
- Regularly Review User Access: Periodically review user privileges and revoke any unnecessary or outdated privileges to minimize security risks.
- Enable Secure Authentication: Use secure authentication methods, including strong passwords and SSL/TLS encryption, to secure user connections.
- Audit MySQL Activity: Enable MySQL auditing to track user activity and detect suspicious behavior or unauthorized access attempts.
Conclusion
Securing a MySQL server is a multi-layered process that involves configuring firewalls, managing user privileges, enforcing strong passwords, enabling SSL encryption, and restricting access from trusted hosts. By following best practices and properly configuring access controls, you can ensure that your MySQL server is secure from unauthorized access and potential attacks.
Backup Strategies in MySQL (mysqldump, mysqlpump, etc.)
Backing up your MySQL databases is a critical part of database administration. It ensures that in the event of data loss, corruption, or hardware failure, you can restore your data to a previous state. In this section, we will explore different backup strategies, including methods like mysqldump
, mysqlpump
, and others, to help you create a reliable backup strategy for your MySQL database.
Why Backup Strategies are Important
Database backups are essential for protecting your data. In case of a system failure, accidental deletion, or data corruption, backups allow you to restore your database to a consistent state. A well-planned backup strategy can minimize downtime and data loss, ensuring the continuity of your business operations.
Types of MySQL Backups
- Logical Backups: This type of backup creates a dump of the database contents. These backups are typically created using tools like
mysqldump
andmysqlpump
. They are easy to create and restore but can be slower for large databases. - Physical Backups: These backups involve copying the actual data files that MySQL uses to store data. Physical backups are usually faster than logical backups but require more complex procedures to ensure consistency (e.g., using file-system snapshots).
- Incremental Backups: These backups only save the changes made since the last backup, reducing the backup size and time required to perform backups.
Backup Tools in MySQL
MySQL offers several tools for creating backups. Each tool has its advantages depending on the specific requirements of your environment.
1. mysqldump
mysqldump
is a command-line utility for creating logical backups of MySQL databases. It creates a text file containing SQL statements that can be used to recreate the database schema and insert the data.
Basic Usage of mysqldump
# Backup a single database
mysqldump -u username -p database_name > backup.sql
# Backup multiple databases
mysqldump -u username -p --databases db1 db2 > backup.sql
# Backup all databases
mysqldump -u username -p --all-databases > backup.sql
Exporting with Compression
You can also use compression to reduce the backup file size. For example, by piping the output of mysqldump
through gzip
:
# Backup a database with compression
mysqldump -u username -p database_name | gzip > backup.sql.gz
2. mysqlpump
mysqlpump
is a newer, faster alternative to mysqldump
that was introduced in MySQL 5.7. It supports parallel processing, which allows for faster backups, especially for large databases or multiple databases.
Basic Usage of mysqlpump
# Backup a single database
mysqlpump -u username -p database_name > backup.sql
# Backup all databases with parallel processing
mysqlpump -u username -p --all-databases --parallel=4 > backup.sql
3. MySQL Enterprise Backup (MEB)
MySQL Enterprise Backup (MEB) is a commercial product provided by Oracle for MySQL backups. It offers hot backups (backing up databases while they are running) and incremental backups, making it suitable for high-availability environments. It also supports compression and encryption of backup files.
Basic Usage of MySQL Enterprise Backup
# Full backup using MySQL Enterprise Backup
mysqlbackup --user=username --password=password --backup-dir=/path/to/backup_dir --with-timestamp backup
# Incremental backup
mysqlbackup --user=username --password=password --backup-dir=/path/to/backup_dir --incremental backup
Backup Strategies for MySQL
Choosing the right backup strategy depends on your specific use case, database size, and uptime requirements. Below are some common strategies:
1. Full Backups
Full backups involve backing up the entire database, including all tables, data, and schema. These backups can be taken using mysqldump
, mysqlpump
, or physical backup methods.
Best Practices for Full Backups
- Perform full backups regularly (e.g., once a day or week) depending on your data change rate.
- Store full backups in a secure location with redundant copies (e.g., cloud storage, external drives).
- Automate the backup process using scripts or backup software.
2. Incremental Backups
Incremental backups capture changes made to the database since the last backup. They are faster and consume less storage space compared to full backups.
Best Practices for Incremental Backups
- Combine incremental backups with periodic full backups to ensure data consistency and minimize restoration time.
- Schedule incremental backups more frequently (e.g., hourly or every few minutes) depending on your data change volume.
3. Point-in-Time Recovery
Point-in-time recovery allows you to restore a backup and then apply transaction logs to bring the database to a specific point in time. This is often used for disaster recovery scenarios where you need to recover the database to the exact moment before a failure or mistake occurred.
Implementing Point-in-Time Recovery
- Enable binary logging in MySQL (e.g.,
log-bin
in the MySQL configuration). - Regularly back up the binary log files along with full and incremental backups.
- Restore the full backup, and then apply the binary logs to reach the desired point in time.
Best Practices for MySQL Backups
- Automate Backups: Set up cron jobs or task scheduler jobs to automate the backup process, ensuring that backups are created regularly without manual intervention.
- Store Backups Securely: Store backup files in a secure, off-site location, such as cloud storage or external drives, to protect against data loss due to hardware failure or disasters.
- Test Restore Procedures: Regularly test your restore procedures to ensure that you can successfully recover from backups in the event of a failure.
- Monitor Backup Performance: Monitor backup processes and check for errors or issues in the backup logs. Ensure that backups are completing successfully and within acceptable time limits.
- Use Encryption: If the backup files contain sensitive data, ensure that backups are encrypted to prevent unauthorized access.
Conclusion
Having a robust backup strategy is essential for any MySQL database. By understanding the different backup tools and methods such as mysqldump
, mysqlpump
, and MySQL Enterprise Backup, you can implement a reliable backup strategy that meets the needs of your business. Regularly backing up your MySQL databases, combining full and incremental backups, and securely storing backups will help ensure data integrity and availability in the event of an emergency.
Restoring from Backups (mysql, mysqlimport)
Restoring from backups is a critical part of database management, ensuring that in the event of data loss, corruption, or system failure, you can recover your MySQL database to a previous state. In this section, we will discuss the different methods for restoring MySQL databases from backups using tools like mysql
and mysqlimport
.
Why Restoration is Important
Restoring from backups allows you to recover data after a failure, whether due to a hardware issue, human error, or corruption. A proper restoration procedure ensures minimal downtime and helps ensure data integrity after a disaster. It's essential to know how to restore databases from backups to prevent permanent data loss.
Methods of Restoring MySQL Databases
MySQL provides multiple ways to restore databases, depending on the type of backup you have. Below are the most commonly used methods for restoring MySQL databases.
1. Restoring from mysqldump Backups (SQL Dump Files)
If you created a backup using mysqldump
, the backup file is typically an SQL dump file that contains the SQL statements required to recreate the database structure and insert the data.
Basic Restoring from mysqldump Backup
To restore a database from a mysqldump
backup, you can use the mysql
command-line tool. This tool takes the SQL dump file and executes the SQL statements within it to restore the database.
# Restore a single database from a mysqldump file
mysql -u username -p database_name < backup.sql
In this command:
username
: Your MySQL user.database_name
: The name of the database you want to restore.backup.sql
: The path to themysqldump
backup file.
Restoring to a New Database
If you want to restore the backup to a new database, you need to first create the database and then restore it:
# Create the new database
mysql -u username -p -e "CREATE DATABASE new_database_name;"
# Restore the backup into the new database
mysql -u username -p new_database_name < backup.sql
2. Restoring from mysqlpump Backups
If you used mysqlpump
to back up your database, the process to restore it is similar to mysqldump
. You can use mysqlpump
to restore the backup file, but typically, mysql
is used for restoring the backup in SQL format.
If your backup was created with parallel processing, you can restore it using the mysqlpump
tool to take advantage of its parallel restoration capabilities, although mysql
is generally more widely used for restoration.
3. Restoring from MySQL Enterprise Backup (MEB)
If you are using MySQL Enterprise Backup (MEB), the restoration procedure is different from using dump files. MySQL Enterprise Backup supports hot backups and incremental backups, and it provides command-line tools for restoring from these backups.
Basic Restoration Using MySQL Enterprise Backup
# Restore a full backup using MySQL Enterprise Backup
mysqlbackup --user=username --password=password --backup-dir=/path/to/backup_dir --copy-back
In this command, the --copy-back
option restores the backup to the MySQL data directory.
4. Restoring from Physical Backups (File System Level)
If you have a physical backup (e.g., a copy of MySQL’s data directory), restoring involves copying the backup files back into the MySQL data directory. This method is typically faster but requires you to ensure that MySQL is stopped during the restoration to avoid data corruption.
To restore from a physical backup, simply copy the files back to the appropriate directory:
# Stop MySQL server
systemctl stop mysql
# Restore backup files to MySQL data directory
cp -r /path/to/backup/* /var/lib/mysql/
# Start MySQL server
systemctl start mysql
Using mysqlimport for Data Import
Another method of restoring data is by importing data files using the mysqlimport
utility. This tool is typically used for importing large datasets from flat files (e.g., CSV files) into MySQL tables.
Basic Usage of mysqlimport
To restore data from a CSV file using mysqlimport
, you can run the following command:
# Import data into a specific table
mysqlimport -u username -p --local database_name /path/to/data.csv
In this command:
--local
: Indicates that the file is located on the local machine.database_name
: The name of the database to import the data into.data.csv
: The path to the CSV file containing the data.
Restoring with Point-in-Time Recovery
If you are performing point-in-time recovery, you will need to restore a full backup followed by applying the binary log files to bring the database to a specific point in time.
Steps for Point-in-Time Recovery
- Restore the full backup using
mysql
ormysqlpump
. - Apply the binary logs to recover the changes made after the backup.
# Apply binary logs for point-in-time recovery
mysql -u username -p < binary_log_file
Best Practices for Restoring Databases
- Test your backups regularly: Ensure that you can restore from your backups by testing the restoration process periodically.
- Restore to a separate environment: Before applying backups to your production environment, restore them to a test environment to ensure that the data is correct and the restore process works as expected.
- Monitor the restoration process: Ensure that you monitor the restoration process to detect any errors or failures early.
- Use version control: Keep track of all backup versions and ensure that you can identify the correct version to restore.
- Document the restoration procedure: Ensure that there is a clear, documented procedure for restoring databases, including steps for both full and incremental recovery.
Conclusion
Restoring from backups is an essential task in MySQL database management, and there are several methods and tools available depending on the type of backup and your specific needs. By understanding the various ways to restore data, including using mysql
, mysqlimport
, and MySQL Enterprise Backup, you can ensure that your MySQL databases can be quickly and reliably restored in case of data loss or corruption. Implementing best practices for restoring backups can help minimize downtime and ensure business continuity during a disaster recovery scenario.
Point-in-Time Recovery and Binary Logs
Point-in-time recovery (PITR) is a technique used to restore a MySQL database to a specific moment in time, typically after a failure or corruption. It allows for recovering data from a known good backup and then applying any changes made since that backup using binary logs. This method minimizes data loss and downtime by enabling recovery to a precise point just before the failure occurred.
Why Point-in-Time Recovery is Important
Point-in-time recovery is crucial for situations where data loss needs to be minimized, and a backup alone cannot restore the database to an acceptable state. For example, if a corruption or human error occurs after a backup, PITR allows you to restore the database to the state it was in just before the issue, ensuring that no recent data is lost.
How Point-in-Time Recovery Works
Point-in-time recovery involves two main components:
- Full Backup: A backup that contains the entire database, typically taken periodically (e.g., daily).
- Binary Logs: MySQL’s binary log files contain a record of all changes made to the database since the last backup (including INSERT, UPDATE, DELETE operations).
The process is as follows:
- Restore the most recent full backup.
- Apply the binary logs from the time of the backup until the desired point in time.
Enabling Binary Logging
To perform point-in-time recovery, MySQL must be configured to log changes to the binary log. This is done by enabling binary logging in the MySQL configuration file (my.cnf
or my.ini
), if it's not already enabled.
Enable Binary Logging in MySQL Configuration
# Edit the MySQL configuration file (my.cnf or my.ini)
[mysqld]
log-bin=mysql-bin
binlog-format=row
In this configuration:
log-bin
: Enables binary logging and specifies the log file prefix (e.g.,mysql-bin
).binlog-format
: Specifies the binary log format (e.g.,row
,statement
, ormixed
).row
is the most accurate for PITR.
After updating the configuration, restart MySQL for the changes to take effect.
Backing Up Binary Logs
In addition to full backups, it's essential to regularly back up the binary logs. This ensures that you have the logs necessary for point-in-time recovery.
To Back Up Binary Logs
# List the available binary log files
mysql -u username -p -e "SHOW BINARY LOGS;"
# Copy the binary log file to a backup location
cp /var/lib/mysql/mysql-bin.000001 /path/to/backup/
Performing Point-in-Time Recovery
To restore the database to a specific point in time, follow these general steps:
- Restore the full backup of the database.
- Apply the binary log entries from the time of the backup until the target point in time.
Step 1: Restoring the Full Backup
# Restore the full backup
mysql -u username -p database_name < /path/to/backup/full_backup.sql
Step 2: Applying the Binary Logs
Once the full backup is restored, apply the binary logs to bring the database to the specific time. You can use the mysqlbinlog
command to read and apply the binary log entries.
Restore the Binary Logs Up to a Specific Point in Time
# Apply binary logs to restore to a specific point in time
mysqlbinlog --stop-datetime="2025-01-21 15:00:00" /path/to/backup/mysql-bin.000001 | mysql -u username -p
In this command:
--stop-datetime
: Specifies the point in time to stop applying the binary logs. The format isYYYY-MM-DD HH:MM:SS
.mysql-bin.000001
: The binary log file that contains the changes since the last full backup.
The mysqlbinlog
command reads the log entries, and the changes are then applied to the database using the mysql
command-line tool.
Using Binary Logs for Point-in-Time Recovery Across Multiple Servers
If you have multiple MySQL servers, you can use binary logs for PITR to synchronize the databases across different instances. For example, when setting up replication, the master server’s binary logs can be used to keep the slave servers in sync, and point-in-time recovery can be applied if needed to catch up with the master or recover from a failure.
Best Practices for Point-in-Time Recovery
- Enable binary logging from the start: To ensure that you can perform point-in-time recovery, binary logging must be enabled before any data loss occurs.
- Back up binary logs regularly: Ensure that binary logs are included in your backup strategy to support point-in-time recovery.
- Test recovery procedures: Regularly test your point-in-time recovery process to ensure it works as expected and you can recover your database in a disaster scenario.
- Use a separate server for backups: Storing backups and binary logs on a separate server from the production database can help prevent data corruption and ensure faster recovery.
- Monitor binary log space usage: Binary logs can consume significant disk space over time, so regularly purge old logs to prevent storage issues.
Conclusion
Point-in-time recovery is a powerful tool for recovering MySQL databases to a specific moment in time, using full backups and binary logs. By enabling binary logging, backing up logs regularly, and testing your recovery process, you can ensure that your database can be restored with minimal data loss in the event of an issue. Point-in-time recovery is essential for maintaining data integrity and business continuity during disasters and outages.
Automated Backup Solutions
Automated backup solutions are essential for ensuring that MySQL databases are regularly backed up without manual intervention. These solutions provide a systematic approach to backing up databases at scheduled intervals, reducing the risk of data loss due to failures, human error, or other disasters.
Why Use Automated Backup Solutions?
Manual backups can be error-prone and inconsistent. Automated backups ensure that backups are taken regularly, reducing the risk of data loss. Automated solutions also allow for backups to be taken during off-peak hours, minimizing the impact on database performance. By automating the backup process, you ensure that your MySQL database can be restored quickly and reliably in the event of failure or data corruption.
Types of Automated Backup Solutions
There are several types of automated backup solutions you can implement for MySQL databases:
- Scheduled Backup Scripts: Using shell scripts or cron jobs to automate the process of backing up MySQL databases at regular intervals.
- Backup Software: Third-party software solutions that offer more advanced backup features such as encryption, compression, and cloud backups.
- Cloud Backup Solutions: Cloud services like AWS RDS, Google Cloud SQL, and Azure Database for MySQL provide automated backup functionality, including point-in-time recovery and backup retention policies.
- Replication-Based Backup: Set up replication to create backups by periodically taking snapshots of the slave server or using the slave for backup operations.
Setting Up Automated Backups Using Cron Jobs
One of the most common methods for automating MySQL backups is using cron
jobs in Linux. A cron job allows you to schedule regular backups of your MySQL database at specific intervals (e.g., daily, weekly, etc.).
Step 1: Create a Backup Script
Start by creating a script that will back up your MySQL database. This script can use mysqldump
or mysqlpump
to take the backup.
Example Backup Script:
#!/bin/bash
# MySQL backup script
# Set MySQL credentials
USER="root"
PASSWORD="your_password"
DB_NAME="your_database"
BACKUP_DIR="/path/to/backup/directory"
DATE=$(date +\%Y-\%m-\%d_\%H-\%M-\%S)
# Create a backup
mysqldump -u $USER -p$PASSWORD $DB_NAME > $BACKUP_DIR/$DB_NAME_$DATE.sql
# Optionally, compress the backup file
gzip $BACKUP_DIR/$DB_NAME_$DATE.sql
This script creates a backup of the specified database and saves it in a backup directory, compressing the file using gzip.
Step 2: Schedule the Backup Using Cron
Once the backup script is ready, schedule it using a cron job. You can set it to run at a specific time each day, week, or month.
Example Cron Job Entry (Run Daily at 2:00 AM):
# Edit the crontab file
crontab -e
# Add a cron job entry to run the backup script every day at 2:00 AM
0 2 * * * /path/to/backup_script.sh
This cron job ensures that the backup script runs every day at 2:00 AM, creating regular backups of the MySQL database.
Using MySQL Enterprise Backup
MySQL Enterprise Backup is an advanced, commercial backup solution that offers features like hot backups, incremental backups, compression, and encryption. It provides high-performance, consistent backups for MySQL databases, including backup options for large-scale enterprise environments.
Features of MySQL Enterprise Backup:
- Hot Backups: Allows backups without locking the database, ensuring minimal downtime during the backup process.
- Incremental Backups: Only changes to the database are backed up, reducing the size and time required for backups.
- Backup Encryption: Provides encryption of backup files for secure storage and transfer.
- Compression: Reduces the storage space required for backups by compressing the backup files.
Cloud-Based Automated Backups
Many cloud providers offer managed MySQL services with built-in automated backup solutions. These services typically allow you to schedule backups, retain multiple backup versions, and perform point-in-time recovery. Cloud solutions also provide the advantage of off-site backup storage for added redundancy and security.
Example: AWS RDS Automated Backups
AWS RDS provides an automated backup feature that enables daily backups of your MySQL instances. It allows you to retain backups for a specified period, and you can restore your database to any point within the retention window.
Configuring Automated Backups in AWS RDS:
- Go to the RDS console in AWS Management Console.
- Select your MySQL database instance.
- Choose the Backup tab and configure the backup retention period (up to 35 days).
- Enable Automated Backups and set the preferred backup window.
Backup Verification and Testing
Regularly verify and test your automated backups to ensure they are working as expected. Automated backups are only useful if they can be restored effectively. It's essential to periodically restore your backups to a test environment and verify that the data can be recovered successfully.
Backup Verification Tips:
- Test Restores: Periodically perform test restores to confirm that backup files are not corrupted and that the data can be restored correctly.
- Check Backup Logs: Review backup logs to ensure no errors occurred during the backup process.
- Validate Backup Completeness: Ensure that all critical data is included in the backup and no essential tables are missed.
Best Practices for Automated Backups
- Store Backups Off-Site: Keep backups in a separate location, preferably in the cloud or on a different physical server, to protect against site-wide failures.
- Use Multiple Backup Types: Combine full backups with incremental or differential backups for better efficiency and recovery options.
- Automate Backup Cleanup: Set up automatic deletion of old backups to avoid running out of storage space.
- Monitor Backup Status: Implement monitoring to alert you if a backup fails or is incomplete.
- Encrypt Backups: Encrypt backup files to ensure they are secure, especially when storing them off-site or in the cloud.
Conclusion
Automated backup solutions provide a reliable way to ensure that your MySQL databases are consistently backed up, minimizing the risk of data loss. Whether you use cron jobs, third-party backup software, or cloud-based solutions, automating your backups saves time and ensures your backups are taken regularly and securely. Proper verification, testing, and monitoring are essential to ensure that your backup strategy is robust and effective in case of an emergency.
Replication for Backup and Failover
Replication is a technique in MySQL where data from one database (the master) is copied to another database (the slave). This process can be used for backup, failover, or load balancing purposes. Replication helps to ensure high availability and fault tolerance in MySQL databases by creating redundant copies of the data in real-time.
Why Use Replication for Backup and Failover?
Replication provides a solution for both backup and failover scenarios. It ensures that even if the primary database (master) goes down, the secondary database (slave) can take over, minimizing downtime. Replication also allows for offloading read queries to slaves, improving the overall performance of read-heavy applications. Additionally, replication can be used to create real-time backups of your data, ensuring that you always have a live copy available for restoration in case of failure.
Types of Replication
There are several types of replication that can be used for backup and failover purposes:
- Asynchronous Replication: In asynchronous replication, the master sends updates to the slave, but the master does not wait for the slave to confirm receipt. This method is most commonly used for backup and failover scenarios.
- Synchronous Replication: In synchronous replication, the master waits for the slave to acknowledge receipt of the data before committing the transaction. This ensures data consistency but can introduce latency.
- Semi-Synchronous Replication: A hybrid approach that ensures the master waits for at least one slave to acknowledge receipt of the data before committing the transaction. This provides a balance between data consistency and performance.
Setting Up Master-Slave Replication
In a typical master-slave replication setup, changes made on the master database are replicated in real-time to the slave(s). This setup ensures that the slave is always synchronized with the master, providing a live copy of the data in case of failure.
Step 1: Configure the Master Server
First, you need to configure the master server to allow replication. This involves enabling binary logging, setting a unique server ID, and specifying the replication user.
Example Configuration for Master Server:
[mysqld]
server-id = 1
log-bin = mysql-bin
binlog-do-db = your_database
In this configuration, you enable binary logging (log-bin
), set a unique server ID (server-id
), and specify the database to replicate (binlog-do-db
).
Step 2: Create a Replication User on the Master Server
Create a user with replication privileges that will be used by the slave to connect to the master and fetch updates.
Example SQL to Create Replication User:
CREATE USER 'replicator'@'%' IDENTIFIED BY 'password';
GRANT REPLICATION SLAVE ON *.* TO 'replicator'@'%';
FLUSH PRIVILEGES;
Step 3: Record the Master’s Binary Log Position
To set up replication properly, you need the current binary log position from the master server. Run the following command on the master to get this information:
SHOW MASTER STATUS;
Note the File
and Position
values, which are required to configure the slave server.
Step 4: Configure the Slave Server
On the slave server, configure the replication settings by specifying the master’s IP address, replication user, and binary log position.
Example Configuration for Slave Server:
[mysqld]
server-id = 2
Then, run the following SQL commands to set up the slave server to connect to the master:
CHANGE MASTER TO
MASTER_HOST='master_ip',
MASTER_USER='replicator',
MASTER_PASSWORD='password',
MASTER_LOG_FILE='mysql-bin.000001',
MASTER_LOG_POS= 106;
START SLAVE;
Step 5: Verify Replication
Finally, verify that the replication is working by checking the slave status:
SHOW SLAVE STATUS\G
Ensure that both Slave_IO_Running
and Slave_SQL_Running
are set to Yes
, indicating that replication is active.
Using Replication for Failover
Replication can be used for automatic failover in case the master server fails. This involves promoting a slave server to become the master and redirecting application traffic to the new master. This process can be automated using tools like Orchestrator or MySQL Router to monitor the health of the master and perform automatic failover when necessary.
Steps for Failover Using Replication:
- If the master server fails, a monitoring tool detects the failure.
- The slave server is promoted to become the new master.
- Application traffic is redirected to the new master.
- The old master is either restored or added as a slave to the new master.
Using Replication for Backup
Replication provides a real-time backup of your data. In the event of a failure or data corruption, you can use the slave server as a backup source for recovery. You can also take periodic backups from the slave server without affecting the performance of the master server.
Backup from Slave Server:
To back up the slave database, you can use the same mysqldump
or mysqlpump
utility as you would on the master server. Since the slave is a read-only copy, backing it up will not impact the master database’s performance.
Monitoring and Maintenance
It’s essential to monitor the replication process to ensure that data is being copied correctly and there are no replication errors. Use tools like Percona Toolkit or MySQL Enterprise Monitor to track replication health and troubleshoot issues.
Best Practices for Replication for Backup and Failover
- Set up multiple slaves: To improve availability, set up multiple slaves that can act as failover candidates in case of master failure.
- Use dedicated backup servers: Replication can be used to offload backup operations to a dedicated slave, ensuring that backups do not impact the master’s performance.
- Regularly monitor replication health: Use monitoring tools to check the status of replication, identify issues, and resolve them promptly.
- Test failover scenarios: Periodically test failover scenarios to ensure that the failover process works smoothly and quickly in case of a disaster.
Conclusion
Replication is a powerful tool for MySQL backup and failover scenarios. By setting up master-slave replication, you can ensure high availability, minimize downtime, and create real-time backups for disaster recovery. Combining replication with automated failover tools ensures that your system can quickly recover from failures and continue serving application traffic without disruption.
Introduction to MySQL Replication
MySQL replication is a powerful feature that enables data from one MySQL database server (the master) to be copied or replicated to one or more other MySQL database servers (the slaves). This replication process allows for the distribution of data across multiple servers, making it an essential tool for high availability, backup, load balancing, and fault tolerance in MySQL-based applications.
What is MySQL Replication?
Replication in MySQL refers to the process of copying data from one database (the master) to another (the slave) to ensure that multiple copies of the data are synchronized. The primary purpose of replication is to maintain data redundancy and availability by creating real-time or near-real-time copies of the master database on slave servers.
Benefits of MySQL Replication
- High Availability: Replication helps ensure the availability of your data even if the master server fails. A slave can be promoted to become the master in case of failure.
- Load Balancing: Replication allows read-heavy applications to offload read queries to slave servers, reducing the load on the master server.
- Backup Strategy: Replication can be used to create real-time backups of your data. By using a slave server for backup purposes, you can avoid affecting the performance of the master server.
- Disaster Recovery: In case of failure, replicated data on the slave can be used for recovery, ensuring minimal downtime.
Types of MySQL Replication
MySQL replication can be configured in different ways depending on the requirements of your infrastructure. The primary types of replication are:
- Asynchronous Replication: In asynchronous replication, the master writes changes to a binary log and sends them to the slave. However, the master does not wait for the slave to acknowledge the changes before committing them. This is the most commonly used replication method due to its simplicity and performance.
- Synchronous Replication: In synchronous replication, the master waits for the slave to acknowledge receipt of the changes before committing the transaction. This ensures data consistency but can introduce latency in high-traffic environments.
- Semi-Synchronous Replication: A combination of both asynchronous and synchronous replication, where the master waits for at least one slave to acknowledge receipt of the change before committing it. This provides a balance between consistency and performance.
Key Concepts in MySQL Replication
Understanding key concepts is essential when setting up and managing MySQL replication:
- Master Server: The server that holds the original data and sends updates to the slave servers.
- Slave Server: The server that receives the updates from the master and maintains an exact copy of the master's data.
- Binary Log: A log file on the master server that records all changes to the database (inserts, updates, deletes). The slave reads this log to replicate the changes.
- IO Thread: The thread on the slave server that fetches binary log events from the master and applies them to the slave.
- SQL Thread: The thread on the slave that executes the SQL statements received from the master.
- Replication User: A user with specific privileges that are used by the slave to connect to the master and retrieve changes.
How MySQL Replication Works
Here’s a basic overview of how MySQL replication works:
- The master server writes all changes to its binary log.
- The slave server’s I/O thread connects to the master and reads the binary log events from the master.
- The slave’s SQL thread executes these changes, keeping the slave database synchronized with the master.
Setting Up MySQL Replication
Setting up MySQL replication involves configuring both the master and slave servers. Here’s a simplified overview of the steps:
- Configure the Master Server: Set up the master server to enable binary logging, configure a unique server ID, and specify the databases to replicate. You also need to create a replication user.
- Configure the Slave Server: Set up the slave server to connect to the master, specifying the master's IP address, replication user, and binary log file and position.
- Start Replication: Start the replication process on the slave server, and ensure that the slave is receiving and applying changes from the master.
Common Replication Setups
There are various replication configurations depending on the needs of your system. Some common setups include:
- Master-Slave Replication: One master server with one or more slave servers.
- Master-Master Replication: Two servers that act as both master and slave, providing redundancy and load balancing.
- Multi-Master Replication: Multiple master servers with each server acting as both a master and a slave to other servers. This setup is typically used for high availability and fault tolerance.
Challenges with MySQL Replication
While MySQL replication is a great tool for high availability and backup, there are a few challenges that you may encounter:
- Replication Lag: In asynchronous replication, there may be some delay between the master and slave, especially in write-heavy systems. This could lead to outdated data on the slave.
- Data Consistency: In multi-master or asynchronous replication, it is possible for data inconsistencies to arise if both the master and slaves make conflicting changes to the same data.
- Network Failures: Replication heavily depends on network connectivity. If a network failure occurs, the replication process may stop, causing lag or data loss.
Monitoring and Troubleshooting Replication
It’s important to monitor the replication process to ensure that it is functioning correctly. Here are some common commands and tools to help:
- SHOW SLAVE STATUS: This command shows the status of the slave server, including whether it is connected to the master and whether replication is running smoothly.
- SHOW MASTER STATUS: Displays information about the master’s binary log file and position.
- MySQL Enterprise Monitor: A tool to monitor the health of your MySQL replication setup and ensure everything is running efficiently.
Conclusion
MySQL replication is a powerful and essential tool for ensuring high availability, fault tolerance, and scalability in MySQL-based applications. By understanding the types of replication, how it works, and how to set it up, you can ensure that your database remains synchronized and resilient to failures. However, it’s important to monitor and maintain your replication environment to avoid common issues like replication lag and data inconsistencies.
Master-Slave Replication Setup
Master-slave replication is one of the most common replication setups in MySQL. In this configuration, one server acts as the master, and one or more servers act as slaves, where the data from the master is replicated to the slaves. This setup can be used for load balancing, high availability, and backup purposes.
What is Master-Slave Replication?
In a master-slave replication setup, the master server writes all changes to its binary log, and the slave servers continuously fetch these changes and apply them. The master server is the authoritative source of data, while the slave servers maintain an identical copy of the master’s database. The slave servers can handle read queries, reducing the load on the master server.
Benefits of Master-Slave Replication
- Improved Read Performance: Slaves can handle read queries, thus offloading the master server and improving performance.
- High Availability: If the master fails, one of the slaves can be promoted to become the new master, minimizing downtime.
- Backup Solution: Slaves can be used for taking backups without affecting the performance of the master server.
- Data Redundancy: The data on the slave is an exact replica of the master, providing redundancy in case of failure.
Setting Up Master-Slave Replication
Here’s a step-by-step guide to set up master-slave replication in MySQL:
1. Configure the Master Server
On the master server, you need to enable binary logging, set a unique server ID, and create a replication user. Follow these steps:
- Open the MySQL configuration file (
my.cnf
ormy.ini
depending on your OS). - Add or modify the following lines:
[mysqld] log-bin=mysql-bin server-id=1 binlog-do-db=your_database_name
- log-bin: Enables binary logging. - server-id: Sets a unique ID for the master server (must be different for each server). - binlog-do-db: Specifies the database to replicate (optional, if you want to replicate only specific databases). - Restart the MySQL server for the changes to take effect.
- Create a replication user by running the following SQL command:
CREATE USER 'replica_user'@'%' IDENTIFIED BY 'password'; GRANT REPLICATION SLAVE ON *.* TO 'replica_user'@'%'; FLUSH PRIVILEGES;
2. Configure the Slave Server
On the slave server, you need to specify the master server’s details and start the replication process. Follow these steps:
- Open the MySQL configuration file on the slave server (
my.cnf
ormy.ini
). - Add or modify the following lines:
[mysqld] server-id=2
- server-id: Set a unique ID for the slave server. Ensure this is different from the master’s server ID. - Restart the MySQL server on the slave for the changes to take effect.
- Log in to the slave MySQL instance and configure replication by running the following command:
CHANGE MASTER TO MASTER_HOST='master_ip_address', MASTER_USER='replica_user', MASTER_PASSWORD='password', MASTER_LOG_FILE='master_log_file', MASTER_LOG_POS=master_log_position;
- MASTER_HOST: The IP address or hostname of the master server. - MASTER_USER: The replication user you created on the master server. - MASTER_PASSWORD: The password for the replication user. - MASTER_LOG_FILE: The binary log file from which the slave will start replicating (can be found by runningSHOW MASTER STATUS
on the master server). - MASTER_LOG_POS: The position in the binary log file to start replication from (again, found viaSHOW MASTER STATUS
). - Start the slave replication process:
START SLAVE;
- Check the status of replication by running:
SHOW SLAVE STATUS\G
Ensure that Slave_IO_Running and Slave_SQL_Running are both set toYes
.
Monitoring and Troubleshooting Master-Slave Replication
Once replication is set up, it’s important to monitor the system to ensure everything is working smoothly. Here are some helpful commands:
- SHOW MASTER STATUS: Displays the current binary log file and position on the master server.
- SHOW SLAVE STATUS: Displays the status of the slave server, including the replication process and any errors that may occur.
- CHECK SLAVE: Checks whether the slave is running and synchronized with the master.
Common Issues and Solutions
- Replication Lag: Replication may lag if the slave server is overwhelmed with queries. To fix this, ensure the slave has enough resources, such as CPU and memory, or offload some read operations to other slaves.
- Errors in the Slave: If the slave encounters an error, you can skip the problematic event by running:
SET GLOBAL SQL_SLAVE_SKIP_COUNTER = 1; START SLAVE;
- Data Inconsistency: If the master and slave become out of sync, you may need to re-synchronize the slave by dumping the master’s data and importing it to the slave.
Conclusion
Master-slave replication in MySQL is a simple yet powerful way to improve the performance and availability of your database. With a properly configured master-slave setup, you can offload read queries to slaves, ensure high availability, and provide a backup solution without affecting your production environment. Regular monitoring and maintenance are essential to ensure smooth operation and avoid replication issues.
Master-Master Replication
Master-master replication in MySQL involves setting up two MySQL servers to replicate each other, making both of them masters. This means that both servers can handle both read and write queries, providing a more flexible, fault-tolerant, and highly available database setup.
What is Master-Master Replication?
In master-master replication, two MySQL servers are configured to act as both master and slave to each other. Each server replicates the changes to the other, ensuring that both servers are in sync. This configuration provides high availability and redundancy as both servers are capable of accepting read and write queries.
Benefits of Master-Master Replication
- High Availability: If one master server goes down, the other can continue to serve requests, ensuring minimal downtime.
- Load Balancing: Both servers can handle both read and write queries, distributing the load more evenly across both systems.
- Fault Tolerance: Both servers contain the same data, reducing the risk of data loss if one server fails.
- Data Redundancy: Both servers hold identical data, ensuring that replication failures do not result in data inconsistency.
Setting Up Master-Master Replication
Setting up master-master replication involves configuring both servers to replicate each other. Here’s a step-by-step guide:
1. Configure the First Server (Master 1)
On the first server (Master 1), you will need to enable binary logging and set the server ID. Follow these steps:
- Open the MySQL configuration file (
my.cnf
ormy.ini
depending on your OS). - Add the following lines to configure binary logging:
[mysqld] log-bin=mysql-bin server-id=1 auto-increment-increment=2 auto-increment-offset=1
- log-bin: Enables binary logging. - server-id: Sets a unique ID for this master server. - auto-increment-increment: Makes auto-increment values increase by 2 to avoid conflicts. - auto-increment-offset: Starts auto-increment values at 1. - Restart the MySQL server for the changes to take effect.
- Create a replication user by running the following SQL command:
CREATE USER 'replica_user'@'%' IDENTIFIED BY 'password'; GRANT REPLICATION SLAVE ON *.* TO 'replica_user'@'%'; FLUSH PRIVILEGES;
2. Configure the Second Server (Master 2)
On the second server (Master 2), you will need to configure it similarly as Master 1, but with a different server ID. Follow these steps:
- Open the MySQL configuration file on the second server (
my.cnf
ormy.ini
). - Add the following lines:
[mysqld] log-bin=mysql-bin server-id=2 auto-increment-increment=2 auto-increment-offset=2
- server-id: Set a unique ID for Master 2 (must be different from Master 1). - auto-increment-increment: Ensures auto-increment values increase by 2, avoiding conflicts. - auto-increment-offset: Starts auto-increment values at 2. - Restart the MySQL server for the changes to take effect.
- Create a replication user by running the following SQL command:
CREATE USER 'replica_user'@'%' IDENTIFIED BY 'password'; GRANT REPLICATION SLAVE ON *.* TO 'replica_user'@'%'; FLUSH PRIVILEGES;
3. Configure Replication on Both Servers
Now, configure replication on both servers so that they replicate to each other. Follow these steps:
- On Master 1, run the following command to get the current binary log file and position:
SHOW MASTER STATUS;
Note the File and Position values. - On Master 2, configure replication to Master 1 by running the following command:
CHANGE MASTER TO MASTER_HOST='master1_ip_address', MASTER_USER='replica_user', MASTER_PASSWORD='password', MASTER_LOG_FILE='master1_log_file', MASTER_LOG_POS=master1_log_position;
Replace master1_ip_address, master1_log_file, and master1_log_position with the actual values from Master 1. - Start replication on Master 2:
START SLAVE;
- On Master 2, run SHOW SLAVE STATUS to ensure that replication is running without errors.
- On Master 2, run the following command to get the current binary log file and position:
SHOW MASTER STATUS;
Note the File and Position values. - On Master 1, configure replication to Master 2 by running the following command:
CHANGE MASTER TO MASTER_HOST='master2_ip_address', MASTER_USER='replica_user', MASTER_PASSWORD='password', MASTER_LOG_FILE='master2_log_file', MASTER_LOG_POS=master2_log_position;
Replace master2_ip_address, master2_log_file, and master2_log_position with the actual values from Master 2. - Start replication on Master 1:
START SLAVE;
- On Master 1, run SHOW SLAVE STATUS to ensure that replication is running without errors.
Monitoring and Troubleshooting Master-Master Replication
Once master-master replication is set up, you should monitor both servers to ensure that replication is working correctly. Use the following commands:
- SHOW MASTER STATUS: Displays the current binary log file and position on the master server.
- SHOW SLAVE STATUS: Displays the status of the slave server, including the replication process and any errors that may occur.
- CHECK SLAVE: Checks whether the slave is running and synchronized with the master.
Common Issues and Solutions
- Replication Conflict: This occurs when both servers try to modify the same data. You can handle this by using conflict resolution strategies like using different auto-increment values or writing custom conflict detection and resolution logic in your application.
- Replication Lag: Replication may lag if the slave server is overwhelmed with queries. To fix this, ensure the slave has enough resources such as CPU and memory.
- Data Inconsistency: If the masters become out of sync, you may need to re-synchronize them by dumping the data from one master and importing it to the other.
Conclusion
Master-master replication provides high availability, fault tolerance, and load balancing by enabling both MySQL servers to handle both read and write queries. It requires careful management of conflict resolution and monitoring to avoid potential issues. By setting up master-master replication, you can ensure your database is both highly available and scalable, with minimized risk of downtime and data loss.
Replication Filters and Configurations
Replication filters in MySQL allow you to control which data is replicated from the master server to the slave server. These filters can help you exclude certain databases, tables, or even specific queries from being replicated. This section discusses the different replication filters and configurations available in MySQL.
What are Replication Filters?
Replication filters are used to control the flow of data between the master and slave servers during replication. By default, all changes made to the master server are replicated to the slave server. However, sometimes you may want to exclude certain databases, tables, or events from being replicated. Replication filters allow you to define which data should be replicated and which should not.
Types of Replication Filters
- Database Filter: This filter allows you to specify which databases should be included or excluded from replication. You can use the
--replicate-do-db
and--replicate-ignore-db
options to include or exclude specific databases. - Table Filter: This filter allows you to specify which tables within a database should be included or excluded. You can use the
--replicate-do-table
and--replicate-ignore-table
options to include or exclude specific tables. - Column Filter: This filter allows you to specify which columns within a table should be included or excluded from replication. This is a more advanced feature and is rarely used in practice.
- Replication-Ignore-Server-Id: This filter allows you to exclude specific servers from replicating changes to the slave server. This is useful when setting up replication filters in a multi-master replication setup.
Configuring Replication Filters
Replication filters can be configured in MySQL by modifying the my.cnf
(or my.ini
) configuration file on both the master and slave servers. Below are examples of how to configure each type of filter.
1. Database Filters
To include or exclude specific databases from replication, you can use the --replicate-do-db
and --replicate-ignore-db
options in the my.cnf
file.
[mysqld] replicate-do-db=database_name # Include a specific database replicate-ignore-db=unwanted_database # Exclude a specific database
For example, to replicate only the sales
database and exclude the logs
database, you would configure:
[mysqld] replicate-do-db=sales replicate-ignore-db=logs
2. Table Filters
To include or exclude specific tables from replication, you can use the --replicate-do-table
and --replicate-ignore-table
options in the my.cnf
file.
[mysqld] replicate-do-table=database_name.table_name # Include a specific table replicate-ignore-table=database_name.table_name # Exclude a specific table
For example, to replicate only the customers
table from the sales
database, you would configure:
[mysqld] replicate-do-table=sales.customers
3. Column Filters
Column filtering is an advanced feature that is generally not used in MySQL replication. This feature allows for filtering based on specific columns in a table, but it is not natively supported by MySQL and would require custom replication logic.
4. Excluding Servers in Multi-Master Replication
If you are using multi-master replication, you may want to exclude changes from specific servers using the --replication-ignore-server-id
option. This prevents certain servers from replicating events to the slave.
[mysqld] replication-ignore-server-id=server_id_to_ignore
For example, if you want to exclude the server with ID 3 from replicating events, you would configure:
[mysqld] replication-ignore-server-id=3
Other Replication Configurations
In addition to filters, MySQL replication can be customized with various configuration settings. Below are some of the most commonly used replication configurations:
- server-id: A unique identifier for each MySQL server in a replication setup. Each server must have a different
server-id
. - log-bin: Enables binary logging on the master server, which is required for replication.
- replicate-wild-do-table: This option allows for the replication of all tables matching a wildcard pattern. It is useful for replicating tables that have dynamic names or when you want to replicate multiple tables.
- replicate-ignore-db: Excludes specific databases from replication.
- replicate-do-db: Includes specific databases in replication.
- read-only: This option is used on the slave server to prevent write operations on the slave while allowing replication.
- skip-slave-start: Prevents the slave from automatically starting replication when the server is restarted. This is useful for situations where you want to manually start replication.
Example Configuration for Master-Slave Replication with Filters
Here’s an example of how to configure a master server to replicate only specific databases and tables to a slave:
[mysqld] log-bin=mysql-bin server-id=1 replicate-do-db=sales replicate-ignore-db=logs replicate-do-table=sales.customers replicate-ignore-table=sales.orders
Monitoring Replication Filters
To monitor replication filters, you can check the replication status on the slave server using the following command:
SHOW SLAVE STATUS\G
This will display the current replication status, including whether the filters are being applied correctly and if there are any replication errors.
Troubleshooting Replication Filters
When using replication filters, you may encounter issues such as:
- Replication Lag: Filters may cause replication to lag if a large amount of data is excluded or included. Monitor replication performance and adjust filters as needed.
- Data Inconsistency: If filters are not configured correctly, data may become inconsistent across the master and slave servers. Ensure that filters are applied correctly and avoid filtering necessary data.
- Configuration Errors: Ensure that the filter options are set correctly in the
my.cnf
file, and restart the MySQL server after making changes.
Conclusion
Replication filters allow for fine-grained control over the data that gets replicated between MySQL servers. By using filters such as --replicate-do-db
, --replicate-ignore-db
, and --replicate-do-table
, you can exclude unnecessary data from replication, optimizing the performance of your replication setup. It is important to monitor the replication process and ensure that filters are applied correctly to prevent data inconsistencies.
Monitoring Replication Status and Health
Monitoring the replication status and health of your MySQL servers is crucial to ensuring that data is being replicated correctly and that there are no issues with the replication process. In this section, we will explore how to monitor the replication status, check for errors, and ensure that replication is functioning as expected.
Viewing Replication Status
To monitor the replication status on a slave server, you can use the SHOW SLAVE STATUS
command. This command provides important information about the replication process, such as the current position of the replication, whether the slave is up-to-date, and if there are any errors in the replication process.
Run the following command on the slave server to view the replication status:
SHOW SLAVE STATUS\G
The output will include the following key fields:
- Slave_IO_State: The current state of the I/O thread. If this is
Waiting for master to send event
, it indicates that the slave is waiting for the master to send data. - Master_Host: The hostname or IP address of the master server.
- Master_Log_File: The name of the binary log file on the master server that the slave is reading.
- Read_Master_Log_Pos: The position of the slave in the master’s binary log. This indicates the last event read by the slave.
- Relay_Master_Log_File: The name of the binary log file on the master from which the slave is reading.
- Exec_Master_Log_Pos: The position of the slave in the relay log. This indicates the last event executed by the slave.
- Seconds_Behind_Master: The number of seconds the slave is behind the master. A value of
0
indicates no lag, while a higher number indicates that the slave is lagging behind the master. - Last_Error: The last error encountered by the slave during replication. If there is no error, this field will be empty.
Checking for Replication Errors
Replication errors can occur for various reasons, such as network issues, data inconsistencies, or incorrect configurations. To check for replication errors, you can look at the Last_Error
field in the SHOW SLAVE STATUS\G
output. If there is an error, this field will contain details about the issue.
If an error occurs, you can attempt to resolve it by re-syncing the slave, fixing the underlying issue, and then restarting the replication process:
STOP SLAVE; START SLAVE;
Monitoring Replication Lag
The Seconds_Behind_Master
field in the SHOW SLAVE STATUS\G
output shows how far behind the slave is from the master in terms of replication. If this value is consistently high, it could indicate that the slave is lagging behind the master, and you may need to troubleshoot the replication process.
To monitor replication lag more closely, you can set up periodic checks using a script that runs the SHOW SLAVE STATUS
command and alerts you when replication lag exceeds a threshold.
Using Performance Schema for Replication Monitoring
The Performance Schema in MySQL provides additional tools for monitoring replication performance. You can use the performance_schema.replication_applier_status
table to get detailed information about the replication process on the slave.
To view the replication applier status, run the following query:
SELECT * FROM performance_schema.replication_applier_status\G
This table provides detailed information about the replication threads, including whether they are running and the positions they are currently reading from the master.
Using MySQL Enterprise Monitor
If you are using MySQL Enterprise Edition, you can take advantage of MySQL Enterprise Monitor, which provides a graphical interface for monitoring replication health and status. It provides real-time monitoring of the replication process, replication lag, and any errors that occur during replication.
MySQL Enterprise Monitor can alert you to replication issues and performance bottlenecks, allowing you to resolve problems before they affect your systems.
Using Third-Party Tools for Monitoring
Several third-party tools can help monitor MySQL replication and provide insights into replication performance and health. These tools typically offer enhanced features such as graphical dashboards, email alerts, and in-depth analysis of replication metrics. Some popular tools include:
- Percona Monitoring and Management (PMM): A free and open-source tool for monitoring MySQL replication and performance.
- ClusterControl: A comprehensive database management platform that provides MySQL replication monitoring and health checks.
- Datadog: A cloud-based monitoring service that supports MySQL replication health checks and provides alerts and dashboards.
Replication Monitoring Best Practices
- Regularly Check Replication Status: Periodically check the replication status on the slave server using the
SHOW SLAVE STATUS
command to ensure that replication is running smoothly. - Monitor Replication Lag: Keep an eye on replication lag using the
Seconds_Behind_Master
field. Set up alerts if the lag exceeds a certain threshold. - Automate Monitoring: Use scripts or third-party tools to automate the monitoring of replication status and health.
- Set Up Alerts: Configure alerts to notify you when replication errors occur, or if there is significant lag in replication.
- Perform Regular Backups: Always ensure that you have up-to-date backups of your master and slave servers in case of replication failure.
- Monitor Slave Performance: Use the Performance Schema or third-party tools to monitor the performance of the replication applier threads on the slave server.
Conclusion
Monitoring the replication status and health of your MySQL servers is essential for ensuring that your replication setup is functioning as expected. By using tools like SHOW SLAVE STATUS
, Performance Schema, and third-party monitoring solutions, you can keep track of replication status, monitor performance, and troubleshoot issues. Regular monitoring helps to identify problems early and ensures that your MySQL replication setup is always running smoothly and efficiently.
Horizontal and Vertical Scaling in MySQL
Scaling a MySQL database is essential for handling increased traffic and ensuring high availability. There are two primary methods of scaling a MySQL database: horizontal scaling and vertical scaling. Both have their advantages and are used based on the specific needs of your application and infrastructure. In this section, we will explore both types of scaling and their use cases.
Vertical Scaling (Scaling Up)
Vertical scaling, also known as "scaling up," involves adding more resources (CPU, RAM, storage, etc.) to a single MySQL server. This method increases the performance and capacity of the server by upgrading its hardware.
How Vertical Scaling Works
In vertical scaling, you typically add more resources to your server, such as:
- CPU: Adding more CPU cores or upgrading to a faster processor.
- RAM: Increasing the amount of memory available to the MySQL server.
- Storage: Adding more disk space to accommodate growing databases.
Vertical scaling can be a quick solution when the database is not yet at a level where horizontal scaling is necessary. However, it has its limitations:
- Hardware Limits: There are practical limits to how much hardware you can add to a single machine. Eventually, you will hit the maximum capacity of the hardware.
- Single Point of Failure: Since all resources are contained in one server, if the server goes down, your entire database will be unavailable.
- Cost: While adding more resources to a server can be cost-effective in the short term, it can become expensive as your needs grow.
Use Cases for Vertical Scaling
Vertical scaling is suitable for applications with moderate database workloads, where the database does not require complex clustering or high availability. Some use cases include:
- Small to medium-sized businesses with steady database growth.
- Applications that do not anticipate significant traffic spikes or rapidly growing data.
- Prototyping or development environments where high availability is not a priority.
Horizontal Scaling (Scaling Out)
Horizontal scaling, also known as "scaling out," involves adding more MySQL servers to distribute the load. In this method, you add additional servers to your infrastructure and partition your data across them.
How Horizontal Scaling Works
In horizontal scaling, you distribute the database load across multiple servers. There are different approaches to achieve this, including:
- Sharding: Sharding involves splitting the database into smaller, more manageable pieces called shards. Each shard is stored on a different server. This allows you to distribute the load and handle more traffic by adding more servers as needed.
- Replication: MySQL replication can be used to replicate data from a master server to one or more slave servers. This improves read scalability by allowing read queries to be distributed across multiple servers, reducing the load on the master.
- Clustering: MySQL Cluster is a high-availability, high-performance solution that provides distributed storage and automatic failover. It uses multiple nodes to store data and automatically replicates data across all nodes.
Benefits of Horizontal Scaling
Horizontal scaling provides several advantages over vertical scaling:
- No Hardware Limits: You can continue adding servers as needed to handle increased traffic and data volume, avoiding hardware limits.
- High Availability: In a well-designed horizontal scaling system, you can achieve high availability by distributing data across multiple servers. If one server fails, others can take over its workload.
- Cost-Effective: Horizontal scaling can be more cost-effective than vertical scaling in the long term because you can add smaller, less expensive servers instead of upgrading to larger, more expensive machines.
Challenges of Horizontal Scaling
While horizontal scaling offers many benefits, it also comes with its own set of challenges:
- Complexity: Managing a horizontally scaled database is more complex than a single-server solution. You need to handle data distribution, replication, and consistency across multiple servers.
- Consistency: Ensuring data consistency across multiple servers can be challenging, especially when using sharding or replication. You need to handle scenarios like network partitions and conflicting updates.
- Latency: Since data is distributed across multiple servers, there may be higher latency when accessing data from different nodes, especially if the servers are geographically distributed.
Use Cases for Horizontal Scaling
Horizontal scaling is ideal for large, high-traffic applications that require high availability and performance. Some use cases include:
- Large e-commerce websites with millions of customers and transactions.
- Social media platforms with large amounts of user-generated content.
- Applications that need to handle sudden spikes in traffic or data growth, such as online gaming or financial services.
When to Choose Horizontal vs. Vertical Scaling
Deciding between horizontal and vertical scaling depends on your application’s needs, growth projections, and budget:
- Choose Vertical Scaling: When you have a relatively small database workload or when you are just starting and need a simple solution that can be upgraded as needed.
- Choose Horizontal Scaling: When your application needs to handle large amounts of traffic, data, or both, and when you need high availability and fault tolerance.
Conclusion
Both horizontal and vertical scaling have their place in MySQL database management, and the choice between them depends on your application's specific requirements. Vertical scaling is a good solution for smaller workloads or when you need a quick, cost-effective way to increase resources. Horizontal scaling, on the other hand, is the preferred solution for large-scale applications that need high availability, fault tolerance, and the ability to scale out as traffic and data grow.
Clustering with MySQL Cluster
MySQL Cluster is a high-availability, high-performance solution for deploying MySQL databases. It is designed to provide scalability and fault tolerance through distributed data storage and automatic failover. In this section, we will explore MySQL Cluster, its architecture, setup, and benefits.
What is MySQL Cluster?
MySQL Cluster is an integrated package of MySQL Database and the NDB (Network DataBase) storage engine. It provides a distributed, high-availability database solution that supports both transactional and analytical workloads. MySQL Cluster is often used in applications that require high uptime, linear scalability, and real-time performance.
Key Features of MySQL Cluster
- High Availability: MySQL Cluster is designed to provide high availability with automatic failover and data replication across multiple nodes.
- Scalability: It can scale horizontally by adding new nodes to distribute the data, ensuring better performance as the workload grows.
- Real-Time Performance: It is optimized for low-latency reads and writes, making it suitable for real-time applications like telecommunications, financial services, and gaming.
- Automatic Failover: In case of a node failure, MySQL Cluster automatically handles failover, ensuring that the system remains operational without manual intervention.
- In-Memory Storage: MySQL Cluster uses in-memory storage for fast data access, but also allows for disk-based storage for large datasets.
Architecture of MySQL Cluster
The architecture of MySQL Cluster is based on a distributed model, with multiple components working together to provide high availability and performance. The main components are:
- Data Nodes: Data nodes store the actual database data. These nodes provide both storage and processing capabilities. Data is distributed across multiple nodes for scalability and redundancy.
- Management Nodes: Management nodes are responsible for managing the cluster’s configuration, monitoring health, and performing administrative tasks. These nodes do not store any user data.
- SQL Nodes: SQL nodes are MySQL servers that handle client connections and process queries. They act as access points to the data stored in the data nodes. SQL nodes communicate with data nodes to retrieve and manipulate data.
Setting Up MySQL Cluster
Setting up MySQL Cluster involves configuring the different nodes (data nodes, management nodes, and SQL nodes) and ensuring they can communicate with each other. Here are the basic steps to set up MySQL Cluster:
- Install MySQL Cluster Software: Install MySQL Cluster on all nodes (management, data, and SQL nodes) using the MySQL Cluster distribution package.
- Configure Management Node: Set up a management node that will manage the cluster’s configuration and monitor the nodes. This node does not store any data.
- Configure Data Nodes: Configure data nodes to handle data storage and provide high availability. Data nodes should be distributed across different physical machines or virtual machines for fault tolerance.
- Configure SQL Nodes: Configure SQL nodes to interact with data nodes and provide query processing. SQL nodes can be placed on separate servers or alongside data nodes.
- Start the Cluster: Start all the nodes (management, data, and SQL nodes). The management node will ensure the nodes can communicate and are properly configured.
Cluster Configuration Files
MySQL Cluster requires configuration files to define the roles and settings for each node in the cluster. These files are typically located in the /etc/mysql/cluster
directory. The main configuration file for data nodes is my.cnf
or ndb.cnf
, which includes information such as:
- Data Node Settings: Configuration of the data nodes, including memory allocation, disk storage, and replication settings.
- Management Node Settings: Configuration of the management node, including the list of data nodes and SQL nodes.
- SQL Node Settings: Configuration of the SQL nodes to connect to data nodes and handle incoming client queries.
Benefits of MySQL Cluster
MySQL Cluster provides several benefits that make it a suitable solution for high-demand applications:
- High Availability: MySQL Cluster’s automatic failover and replication ensure that the system remains operational even during node failures.
- Scalability: The ability to scale horizontally by adding more nodes allows MySQL Cluster to handle increasing workloads as your application grows.
- Real-Time Performance: MySQL Cluster is optimized for low-latency operations, making it ideal for real-time applications that require fast data access and processing.
- Fault Tolerance: Data replication and automatic failover mechanisms ensure that your application remains available even in the event of hardware failures.
Limitations of MySQL Cluster
While MySQL Cluster offers many benefits, it also comes with some limitations:
- Complex Configuration: Setting up and managing a MySQL Cluster can be complex, especially for larger clusters with many nodes.
- Memory Usage: MySQL Cluster is memory-intensive, as it stores all data in memory for fast access. This can be limiting for applications with large datasets that do not fit into memory.
- Limited SQL Features: Some SQL features, such as foreign key constraints and certain JOIN operations, are not fully supported in MySQL Cluster.
Use Cases for MySQL Cluster
MySQL Cluster is particularly suited for applications that require:
- High availability and fault tolerance, such as telecommunications, e-commerce, and financial services.
- Real-time data processing with low-latency access, such as gaming, social media, and online analytics.
- Applications with high data throughput and scalability requirements that need to handle millions of transactions per second.
Conclusion
MySQL Cluster is a powerful solution for high-availability and high-performance MySQL databases. By distributing data across multiple nodes, it offers scalability and fault tolerance, making it ideal for applications that require low-latency access to large datasets. While it comes with some limitations and configuration complexity, its benefits for large-scale, real-time applications make it a valuable tool for organizations with demanding database needs.
Load Balancing and Read-Write Splitting
Load balancing and read-write splitting are strategies used to enhance the performance, availability, and scalability of MySQL databases. These techniques distribute database traffic efficiently across multiple servers, improving response times and reducing the load on a single server.
What is Load Balancing?
Load balancing is the process of distributing incoming database requests across multiple MySQL servers to ensure that no single server is overwhelmed with requests. This improves the performance, availability, and redundancy of the database system, providing fault tolerance in the event of server failures.
Types of Load Balancing
- DNS Load Balancing: A DNS server redirects traffic to different MySQL servers based on the DNS settings.
- Hardware Load Balancers: Dedicated hardware devices distribute traffic across multiple MySQL servers based on predefined algorithms.
- Software Load Balancers: These are software solutions that distribute traffic across multiple MySQL instances. Examples include ProxySQL, HAProxy, and MySQL Router.
What is Read-Write Splitting?
Read-write splitting is a technique where database traffic is separated into two types: read operations (such as SELECT) and write operations (such as INSERT, UPDATE, DELETE). Read operations are directed to read-only replicas (secondary servers), while write operations are directed to the primary server (master). This allows read-heavy applications to scale by distributing read traffic across multiple servers, while write traffic is handled by a single server.
How Read-Write Splitting Works
In a typical read-write splitting setup, the application or a proxy server performs the following tasks:
- For read requests, the application sends queries to one or more read-only replica servers.
- For write requests, the application directs queries to the primary server that can handle write operations.
- The primary server replicates the changes to the read-only replicas, ensuring that the data is synchronized across all servers.
Load Balancing with Read-Write Splitting
Combining load balancing with read-write splitting allows for efficient distribution of both read and write operations. By using a load balancer and read-write splitting together, you can:
- Distribute read operations among multiple replicas, improving query performance.
- Ensure that write operations are handled by the primary server while maintaining data consistency.
- Improve scalability and fault tolerance by adding more read replicas and balancing the load between them.
- Ensure high availability by redirecting traffic to healthy nodes in case of server failure.
Benefits of Load Balancing and Read-Write Splitting
- Improved Performance: Distributing read queries across multiple replicas reduces the load on the primary server and enhances the overall performance of the system.
- Scalability: By adding more read replicas, you can scale the read operations horizontally, which is especially beneficial for read-heavy applications.
- High Availability: Load balancing and read-write splitting ensure that requests can still be served even if one server becomes unavailable, providing fault tolerance.
- Optimized Resource Utilization: By separating read and write traffic, you can optimize the resources of each server according to its role (e.g., primary for writes, replicas for reads).
Setting Up Load Balancing and Read-Write Splitting
To set up load balancing and read-write splitting, follow these general steps:
- Deploy MySQL Replication: Set up master-slave or master-master replication, where the primary server handles writes and the replicas handle reads.
- Set Up a Load Balancer: Use a load balancer (e.g., ProxySQL, HAProxy, MySQL Router) to distribute traffic between the primary server and the read-only replicas. The load balancer should be configured to send read queries to replicas and write queries to the primary server.
- Configure Read-Write Splitting: Configure your application or the load balancer to detect the type of query (read or write) and direct it to the appropriate server. For example, SELECT queries should go to replicas, while INSERT, UPDATE, DELETE queries should go to the primary server.
- Monitor and Maintain the Cluster: Continuously monitor the health of the servers and ensure data consistency across all nodes. In case of failure, the load balancer should reroute traffic to healthy servers.
Tools for Load Balancing and Read-Write Splitting
Several tools and technologies can help implement load balancing and read-write splitting for MySQL:
- ProxySQL: A high-performance SQL proxy for MySQL that provides advanced read-write splitting, query routing, and load balancing capabilities.
- HAProxy: An open-source load balancer that can be configured to distribute traffic based on various algorithms, including round-robin, least connections, and more.
- MySQL Router: A lightweight middleware solution from MySQL that facilitates traffic routing between application clients and MySQL servers, supporting read-write splitting and load balancing.
Limitations and Considerations
While load balancing and read-write splitting offer significant benefits, there are some considerations to keep in mind:
- Replication Lag: There may be a slight delay between the primary server and its replicas, meaning that recent write operations may not immediately appear on replicas for read queries.
- Consistency vs. Availability: In some cases, there may be a trade-off between maintaining strict consistency and ensuring high availability. Applications need to handle potential data inconsistencies that may arise from replication lag.
- Complexity: Implementing load balancing and read-write splitting adds complexity to your database architecture. Proper configuration and monitoring are essential to ensure the system is reliable and efficient.
Conclusion
Load balancing and read-write splitting are powerful techniques for enhancing MySQL database performance, scalability, and availability. By distributing traffic across multiple servers, these methods enable your system to handle more queries while maintaining optimal resource utilization and fault tolerance. However, it's important to carefully configure the system and monitor replication health to ensure the system operates smoothly and efficiently.
MySQL High Availability with MHA, Orchestrator, and ProxySQL
High availability (HA) is crucial for MySQL databases to ensure that they remain operational and accessible even during server failures. Tools like MHA (MySQL High Availability), Orchestrator, and ProxySQL help manage and automate MySQL failover, replication, and load balancing, providing improved database availability, scalability, and fault tolerance.
What is MySQL High Availability?
MySQL High Availability refers to ensuring continuous database service by minimizing downtime and preventing single points of failure. By using replication, failover mechanisms, and load balancing, MySQL systems can be kept available and reliable even during hardware or software failures.
Key Concepts of MySQL High Availability
- Replication: Replicating data between a primary (master) server and secondary (slave) servers for redundancy and load distribution.
- Failover: The automatic or manual process of switching to a standby server in case of primary server failure.
- Load Balancing: Distributing database queries among multiple MySQL servers to optimize performance and prevent overload on a single server.
Tools for MySQL High Availability
Several tools are available for achieving high availability in MySQL. These tools provide functionalities like automatic failover, centralized management, and query routing to ensure that MySQL databases are resilient and performant.
1. MHA (MySQL High Availability)
MySQL High Availability (MHA) is a solution for MySQL replication with automated failover and recovery. It enables the automatic promotion of a replica to the primary role when the master server fails, ensuring high availability of the MySQL database.
Key Features of MHA
- Automatic Failover: MHA automatically promotes a slave to be the new master when the current master fails.
- Automatic Recovery: Once the failed master is recovered, MHA automatically reconfigures the cluster and synchronizes data.
- Non-Blocking: MHA ensures that failover occurs without blocking ongoing database operations.
How MHA Works
In a typical MHA setup, the system consists of a master server and several replica servers. MHA watches the master server for failures. When a failure is detected, it promotes one of the replica servers to master and reconfigures the cluster to ensure minimal downtime. The data on the newly promoted master is synchronized with the other replicas.
2. Orchestrator
Orchestrator is a MySQL high availability and management tool that provides automated failover, replication management, and cluster topology management for MySQL. It is designed to ensure that MySQL replication is properly maintained while providing monitoring and failover capabilities.
Key Features of Orchestrator
- Cluster Management: Orchestrator supports the management of MySQL replication topologies and can visualize the cluster topology.
- Automated Failover: In case of a failure, Orchestrator can automatically promote a replica to be the master.
- Replication Health Monitoring: It continuously monitors replication health and alerts administrators if there are issues with replication.
- Topology Reconfiguration: Orchestrator can reconfigure replication topologies and apply changes to MySQL clusters.
How Orchestrator Works
Orchestrator continuously monitors the MySQL replication setup and keeps track of the topology. It provides features for failover, failback, and topology reconfiguration, ensuring the MySQL cluster remains healthy and available. Orchestrator can also be used to promote slaves to master and reconfigure the cluster without manual intervention.
3. ProxySQL
ProxySQL is an advanced proxy for MySQL that is used for query routing, load balancing, and high availability in MySQL environments. It allows for dynamic query routing based on various conditions, such as read-write splitting, and supports load balancing across multiple MySQL servers.
Key Features of ProxySQL
- Read-Write Splitting: ProxySQL can route read queries to replicas and write queries to the master server, improving the scalability and availability of MySQL databases.
- Load Balancing: ProxySQL supports load balancing for both read and write queries, distributing traffic to multiple MySQL servers to optimize performance.
- Query Caching: ProxySQL can cache frequent queries to reduce load on the MySQL servers and improve response times.
- Connection Pooling: It improves connection efficiency by pooling database connections and reusing them for multiple queries.
How ProxySQL Works
ProxySQL sits between the application and MySQL servers. It intercepts queries and decides where to route them based on predefined rules. For example, read queries can be routed to replicas, while write queries can be routed to the master. ProxySQL also provides features like query caching and connection pooling to optimize MySQL performance.
Combining MHA, Orchestrator, and ProxySQL for High Availability
By combining MHA, Orchestrator, and ProxySQL, you can achieve a highly available MySQL setup that includes automated failover, efficient query routing, and replication management.
- Automated Failover: MHA or Orchestrator can handle automatic failover in case of server failure, promoting a replica to be the new master.
- Query Routing and Load Balancing: ProxySQL can route queries to the appropriate server (read queries to replicas, write queries to the master), and load balance traffic to ensure even distribution across servers.
- Replication Health Monitoring: Orchestrator provides continuous monitoring of replication health, ensuring that any issues are detected early.
- Scalability and Fault Tolerance: The combined setup enables you to scale your MySQL environment horizontally, with multiple replicas for read traffic and automatic recovery mechanisms in place for failures.
Setting Up MySQL High Availability with MHA, Orchestrator, and ProxySQL
- Deploy MySQL Servers: Set up your MySQL primary and replica servers with the appropriate replication configuration.
- Install MHA: Install MHA and configure it to automatically detect and handle master failures.
- Set Up Orchestrator: Install Orchestrator and configure it to monitor your MySQL replication cluster and handle failover and topology management.
- Install ProxySQL: Set up ProxySQL as a proxy between your application and MySQL servers to handle query routing, load balancing, and read-write splitting.
- Configure Failover and Load Balancing: Configure ProxySQL to route read queries to replicas and write queries to the master server. Set up MHA or Orchestrator for automated failover.
- Monitor the Setup: Continuously monitor the health of your MySQL environment using MHA, Orchestrator, and ProxySQL to ensure everything is running smoothly.
Limitations and Considerations
- Replication Lag: There may be a slight delay in replication, which can affect the consistency of data between the master and replicas.
- Complexity: Setting up high availability with these tools requires careful configuration and ongoing monitoring to ensure optimal performance and reliability.
- Cost: Implementing high availability can increase infrastructure costs, as multiple servers and tools are required for proper functioning.
Conclusion
Achieving high availability for MySQL databases is critical for ensuring business continuity and performance. Tools like MHA, Orchestrator, and ProxySQL provide comprehensive solutions for automatic failover, load balancing, replication management, and query routing. By combining these tools, you can create a robust, scalable, and highly available MySQL environment that can withstand failures and scale to meet growing demand.
Using MySQL on AWS (RDS, EC2)
Amazon Web Services (AWS) offers robust solutions for hosting MySQL databases, primarily through Amazon RDS (Relational Database Service) and EC2 (Elastic Compute Cloud). These services allow you to leverage the scalability, flexibility, and reliability of AWS while managing your MySQL databases. This section covers both approaches and their benefits.
What is Amazon RDS?
Amazon RDS is a fully managed database service that supports multiple database engines, including MySQL. It abstracts much of the administrative overhead, such as patching, backups, and scaling, allowing you to focus on application development and data management.
Key Features of Amazon RDS for MySQL
- Fully Managed Service: AWS takes care of database administration tasks like backups, patching, and monitoring.
- Scalability: Easily scale your MySQL instance vertically or horizontally by modifying instance sizes or adding read replicas.
- High Availability: Multi-AZ deployments offer automatic failover to a standby instance in case of a primary instance failure.
- Automated Backups: RDS provides automated backups with point-in-time recovery, ensuring data durability and reliability.
- Security: Encryption at rest and in transit, with integration with AWS Identity and Access Management (IAM) and Virtual Private Cloud (VPC).
Creating a MySQL Database on Amazon RDS
Follow these steps to create a MySQL database instance on Amazon RDS:
- Log in to the AWS Management Console and navigate to RDS.
- Click on "Create database" and select MySQL as the database engine.
- Choose the desired DB instance type (e.g., db.t3.micro for small instances or db.m5.large for larger workloads).
- Configure settings like DB instance identifier, master username, and password.
- Set up storage and backup options based on your needs (e.g., general-purpose SSD storage and automated backups).
- Choose a VPC and configure security settings (e.g., enabling encryption and connecting to a private subnet).
- Review the configuration and click "Create database" to launch your MySQL instance.
What is EC2?
Amazon EC2 is a scalable compute service that allows you to launch virtual servers (instances) in the cloud. With EC2, you can install and configure MySQL on a virtual machine (VM) of your choice, giving you more control over the database environment compared to RDS.
Key Features of EC2 for MySQL Hosting
- Full Control: EC2 provides complete control over the server, allowing you to install custom configurations, extensions, and software.
- Customizability: You can choose the underlying operating system (e.g., Linux, Windows) and instance types tailored to your workload.
- Scalability: Scale vertically by changing instance types or horizontally by adding more EC2 instances with load balancing.
- Cost Flexibility: Pay only for the compute resources you use, with options for reserved, on-demand, or spot instances.
Setting Up MySQL on Amazon EC2
Follow these steps to set up MySQL on an EC2 instance:
- Log in to the AWS Management Console and navigate to EC2.
- Launch a new EC2 instance, selecting your desired instance type (e.g., t2.micro for small workloads) and operating system (e.g., Ubuntu, Amazon Linux).
- Configure instance details, including network settings, security groups, and key pairs.
- Once the instance is running, connect to it using SSH (Linux) or RDP (Windows).
- Install MySQL by running the appropriate installation command:
- On Ubuntu: `sudo apt-get update && sudo apt-get install mysql-server`
- On Amazon Linux: `sudo yum install mysql`
- Start the MySQL service: `sudo systemctl start mysql`.
- Configure MySQL settings as needed (e.g., adjusting buffer sizes, setting up users, etc.).
- Secure the installation by running `sudo mysql_secure_installation` to set root passwords, remove test databases, and configure security settings.
- Ensure that the EC2 instance’s security group allows inbound traffic on the MySQL port (default 3306) from trusted IP addresses.
Comparing RDS and EC2 for MySQL
Below is a comparison between Amazon RDS and Amazon EC2 for hosting MySQL databases:
Feature | RDS | EC2 |
---|---|---|
Management Overhead | Low - Fully managed by AWS | High - Requires manual setup and maintenance |
Control | Limited - Managed service with predefined configurations | High - Full control over the server and database configurations |
Scalability | Easy - Vertical and horizontal scaling with minimal effort | Manual - Requires additional configuration for scaling |
High Availability | Built-in - Multi-AZ deployments for automatic failover | Manual - Requires setting up replication and failover mechanisms |
Backup and Recovery | Automated - Automated backups and point-in-time recovery | Manual - Requires manual backup solutions |
Cost | Higher - Managed service with additional features | Variable - Depending on instance types, storage, and network usage |
When to Use RDS for MySQL
RDS is ideal for users who want a fully managed, easy-to-use MySQL database solution without managing the underlying infrastructure. It is recommended for:
- Applications requiring quick deployment and minimal administration.
- Users who prefer automated backups, patching, and failover mechanisms.
- Scalable applications that need high availability and disaster recovery options.
When to Use EC2 for MySQL
EC2 is ideal for users who require full control over their MySQL environment and need to customize the server configuration. It is recommended for:
- Advanced users who need the flexibility to install custom software and configurations.
- Organizations with specific performance or security requirements that RDS cannot meet.
- Users who wish to manage their backups, replication, and failover manually.
Conclusion
Both Amazon RDS and EC2 provide powerful options for running MySQL databases on AWS. RDS offers a hassle-free, fully managed MySQL service that is ideal for most users, while EC2 provides flexibility and full control over the MySQL environment for advanced use cases. Choosing the right option depends on the level of control, customizability, and administrative overhead you are willing to handle for your MySQL deployment.
Using MySQL on Google Cloud SQL
Google Cloud SQL is a fully managed relational database service that supports MySQL, PostgreSQL, and SQL Server. It allows you to run MySQL databases in the cloud with ease, offering features such as automatic backups, scaling, and security. In this section, we'll explore how to use MySQL on Google Cloud SQL and its key benefits.
What is Google Cloud SQL?
Google Cloud SQL is a fully managed database service that provides scalable MySQL database instances on Google Cloud. It takes care of database management tasks such as patching, backups, and failover, allowing developers to focus on building applications instead of managing infrastructure.
Key Features of Google Cloud SQL for MySQL
- Fully Managed Service: Cloud SQL handles database administration tasks such as patch management, backups, and automatic failover.
- Scalability: Cloud SQL instances can be scaled vertically (by adjusting CPU and memory) or horizontally (by adding read replicas).
- High Availability: Cloud SQL supports high availability configurations with automatic failover between primary and standby instances.
- Security: Cloud SQL provides built-in encryption at rest and in transit, and integrates with Google Cloud IAM for access control and authentication.
- Automated Backups: Automated daily backups ensure data durability, with the ability to restore your database to any point in time within the backup retention period.
Creating a MySQL Database on Google Cloud SQL
Follow these steps to create a MySQL database instance on Google Cloud SQL:
- Log in to the Google Cloud Console and navigate to the SQL section.
- Click "Create instance" and select MySQL as the database engine.
- Choose the instance configuration, including database version, machine type, and storage options (e.g., SSD or HDD).
- Set the instance ID, root password, and configure the database settings.
- Choose the region and zone for the instance to ensure it is close to your application for low-latency access.
- Configure additional options such as backups, high availability, and security settings (e.g., SSL, IAM, and firewall rules).
- Click "Create" to launch your MySQL instance.
Connecting to MySQL on Google Cloud SQL
Once the MySQL instance is created, you can connect to it using several methods, including the MySQL command-line client, Google Cloud SDK, or through application code:
- Using MySQL Client: You can connect from your local machine or an instance within Google Cloud using the following command:
gcloud sql connect [INSTANCE_ID] --user=root
- From an Application: You can connect to Cloud SQL from your application by using the Cloud SQL Auth proxy or by configuring the Cloud SQL instance connection string in your application's database configuration.
Managing MySQL on Google Cloud SQL
Google Cloud SQL provides several features for managing MySQL instances:
- Backups: You can configure automated daily backups and manually initiate on-demand backups as needed. Backups can be used to restore the database to a specific point in time.
- Monitoring: Cloud SQL integrates with Google Cloud Monitoring to provide real-time insights into database performance, including CPU utilization, disk I/O, and database queries.
- Scaling: You can resize your Cloud SQL instances on-demand to adjust resources based on load. Additionally, read replicas can be created to offload read traffic and improve performance.
- Security: You can configure SSL/TLS certificates for encrypted connections, enable IAM database authentication, and set up firewalls to restrict IP access to the database.
Cloud SQL vs. Google Compute Engine (GCE) for MySQL
Google Cloud provides multiple ways to run MySQL, with Cloud SQL and Google Compute Engine (GCE) as two popular options. Here’s a comparison:
Feature | Cloud SQL | Compute Engine |
---|---|---|
Management Overhead | Low - Fully managed by Google | High - Requires manual setup and ongoing maintenance |
Control | Limited - Google manages most aspects of the database | High - Full control over the server, OS, and database |
Scaling | Easy - Automated vertical scaling and horizontal read replicas | Manual - Requires manual configuration of scaling and replication |
Availability | Built-in - High availability with automatic failover | Manual - Requires custom configuration for failover and high availability |
Backups | Automated - Built-in automated backups with point-in-time recovery | Manual - Requires custom scripts for backup and recovery |
Cost | Higher - Fully managed service with additional features | Variable - Pay for compute resources and storage separately |
When to Use Google Cloud SQL for MySQL
Google Cloud SQL is ideal for most users who want a fully managed, low-maintenance MySQL database that is easy to scale and secure. It is recommended for:
- Applications that require quick deployment and minimal database management.
- Users who need high availability and automated backups without the complexity of managing infrastructure.
- Projects that need seamless scaling and integration with other Google Cloud services.
When to Use Google Compute Engine for MySQL
Google Compute Engine is best for advanced users who need full control over their MySQL environment. It is ideal for:
- Users who require custom configurations, extensions, or specific performance tuning for MySQL.
- Users who need a high degree of flexibility in managing both the database and the underlying server.
- Organizations with complex or legacy database environments that need to be migrated or integrated with Google Cloud.
Conclusion
Google Cloud SQL offers a fully managed, scalable, and secure MySQL database solution that is well-suited for most cloud-based applications. If you prefer a hassle-free, managed database service, Cloud SQL is the best option. However, if you need more control or custom configurations, running MySQL on Google Compute Engine might be a better fit for your needs.
Using MySQL on Microsoft Azure
Microsoft Azure offers fully managed MySQL databases through the Azure Database for MySQL service, which provides high availability, scalability, security, and automated backups for MySQL databases. This service allows users to focus on application development instead of managing infrastructure. In this section, we will explore the features and benefits of using MySQL on Microsoft Azure and how to set it up.
What is Azure Database for MySQL?
Azure Database for MySQL is a fully managed database service provided by Microsoft Azure for running MySQL databases in the cloud. It offers features such as automated backups, scaling, high availability, and security, all managed by Azure, reducing the administrative overhead.
Key Features of Azure Database for MySQL
- Fully Managed Service: Azure handles database management tasks such as patching, backups, and automatic failover, allowing users to focus on application development.
- Scalability: Azure Database for MySQL supports vertical scaling (changing the CPU, memory, and storage) and horizontal scaling using read replicas.
- High Availability: Built-in high availability with automatic failover and data replication across availability zones ensures minimal downtime.
- Security: The service provides encryption in transit and at rest, integrated authentication using Azure Active Directory, and firewalls for secure access control.
- Automated Backups: Azure provides automatic daily backups with the ability to restore to any point in time within the retention period (up to 35 days).
Creating MySQL Database on Azure
Follow these steps to create a MySQL instance on Azure Database for MySQL:
- Log in to the Azure portal (portal.azure.com).
- Navigate to the "Create a resource" section and search for "Azure Database for MySQL".
- Click "Create" to start the process of creating a new MySQL database instance.
- Configure the basic settings, including the subscription, resource group, server name, and region.
- Choose the pricing tier based on your performance and storage needs (e.g., Basic, General Purpose, or Memory Optimized).
- Configure the administrator username and password for your MySQL instance.
- Set up the networking options (e.g., virtual network, public access, and firewall rules).
- Click "Review + Create" and then "Create" to deploy the MySQL instance.
Connecting to MySQL on Azure
Once your MySQL instance is created, you can connect to it using the MySQL command-line client or through your application:
- Using MySQL Client: You can connect using the following command from a local machine or VM within Azure:
mysql -h [hostname] -u [username] -p
Replace [hostname] with the server name and [username] with your MySQL admin username. - From an Application: You can use your application's database configuration to connect to Azure Database for MySQL by specifying the server name, username, and password in the connection string.
Managing MySQL on Azure
Azure Database for MySQL offers several management capabilities:
- Backups: Automatic daily backups are enabled by default, and you can restore your database to any point in time within the retention period.
- Monitoring: Azure integrates with Azure Monitor to provide real-time performance metrics such as CPU usage, memory usage, and query performance.
- Scaling: You can resize your server instance to adjust resources based on performance needs. You can also create read replicas to offload read traffic and improve scalability.
- Security: Azure Database for MySQL supports SSL encryption for connections, Virtual Network (VNet) service endpoints, and advanced firewall rules to control access to the database.
Azure Database for MySQL vs. Azure Virtual Machines
Microsoft Azure offers two main ways to run MySQL databases: Azure Database for MySQL and Azure Virtual Machines (VMs). Here's a comparison:
Feature | Azure Database for MySQL | Azure Virtual Machines |
---|---|---|
Management Overhead | Low - Fully managed service with automated backups, patching, and scaling | High - Requires manual setup, patching, and maintenance of the MySQL instance |
Control | Limited - Managed by Azure | Full - You manage the server and MySQL installation |
Scaling | Easy - Vertical scaling and read replicas available | Manual - Requires manual configuration for scaling and replication |
High Availability | Built-in - Automatic failover and replication across availability zones | Manual - Requires custom configuration for HA and failover |
Backups | Automated - Built-in daily backups with point-in-time restore | Manual - Requires custom backup solutions |
Cost | Higher - Fully managed service with features like backups and scaling | Variable - Pay for VM resources, storage, and MySQL management |
When to Use Azure Database for MySQL
Azure Database for MySQL is ideal for most users who want a fully managed MySQL service with minimal administrative overhead. It is recommended for:
- Applications that require a hassle-free, highly available, and scalable MySQL database in the cloud.
- Users who want automatic backups, point-in-time recovery, and built-in security features without managing the database infrastructure.
- Projects that benefit from deep integration with Azure’s other services, such as Azure Functions, Azure App Service, and more.
When to Use Azure Virtual Machines for MySQL
Azure Virtual Machines is best suited for users who need full control over their MySQL environment. It is ideal for:
- Advanced users who require custom configurations, performance tuning, or specific MySQL extensions.
- Organizations that need more control over the operating system and database software.
- Projects with specific requirements for MySQL that are not supported by the managed service.
Conclusion
Azure Database for MySQL is a fully managed, scalable, and secure database service that provides the benefits of high availability, automatic backups, and integrated monitoring without the complexity of managing the underlying infrastructure. It is well-suited for most cloud-based applications, offering a simple and efficient way to run MySQL in the Azure cloud. For more advanced users who need full control over the MySQL instance or require custom configurations, running MySQL on Azure Virtual Machines may be a better fit.
Best Practices for Cloud Deployments
Cloud deployments offer flexibility, scalability, and cost-efficiency, but to fully leverage these benefits, it's important to follow best practices. Whether you are deploying on platforms like AWS, Azure, or Google Cloud, the following best practices will help ensure your cloud infrastructure is secure, performant, and reliable.
1. Design for Scalability
One of the key advantages of cloud deployments is the ability to scale resources up or down as needed. To take full advantage of this, you should:
- Use auto-scaling: Configure auto-scaling to automatically adjust resources based on demand, ensuring optimal performance and cost-efficiency.
- Design microservices architecture: Break down applications into smaller, independently deployable services that can be scaled individually.
- Consider elasticity: Build applications that can scale horizontally by adding more instances or vertically by increasing resource capacity.
2. Implement Strong Security Practices
Security should be a top priority in cloud deployments to protect sensitive data and maintain user trust. Key security practices include:
- Use encryption: Encrypt data both in transit and at rest to protect against unauthorized access.
- Leverage identity and access management (IAM): Implement role-based access control (RBAC) and the principle of least privilege to limit access to resources.
- Enable multi-factor authentication (MFA): Use MFA to add an extra layer of security to user accounts.
- Monitor security events: Regularly monitor and audit cloud resources for unusual activity or security threats using cloud-native tools or third-party services.
3. Optimize for Cost Efficiency
Cloud cost management is essential to avoid overpaying for unused resources. Follow these practices to optimize cloud costs:
- Right-size resources: Continuously monitor resource usage and adjust the size of your compute instances, storage, and networking to match actual demand.
- Use reserved instances: For long-term workloads, use reserved instances or committed usage plans for discounts over on-demand pricing.
- Leverage spot instances: For non-critical workloads, use spot instances or preemptible VMs to take advantage of lower costs.
- Monitor usage: Use cloud cost management tools (e.g., AWS Cost Explorer, Azure Cost Management) to track and manage usage and spending.
4. Automate and Streamline Deployment
Automation is a cornerstone of cloud deployment best practices. It improves efficiency, reduces human error, and enhances consistency. Key automation strategies include:
- Implement Infrastructure as Code (IaC): Use tools like Terraform, AWS CloudFormation, or Azure Resource Manager to define infrastructure in code for easy deployment and versioning.
- Automate CI/CD pipelines: Implement continuous integration and continuous delivery (CI/CD) pipelines to automate the process of deploying code updates to production environments.
- Use configuration management tools: Use tools like Ansible, Chef, or Puppet to automate the configuration and management of cloud resources.
5. Ensure High Availability and Fault Tolerance
Cloud applications must be resilient to failures to ensure high availability and reliability. Implement the following practices:
- Use multi-region and multi-zone deployments: Distribute your application across multiple data centers (availability zones or regions) to prevent downtime in case of failure.
- Implement load balancing: Use load balancing to evenly distribute traffic across multiple servers or services to avoid overloading any single resource.
- Backup and disaster recovery: Set up automated backups and disaster recovery plans to quickly recover from failures or data loss.
6. Monitor and Log Cloud Resources
Effective monitoring and logging are essential for identifying performance issues, security threats, and opportunities for optimization. Follow these practices:
- Centralized logging: Collect and centralize logs from all cloud resources, applications, and services to make it easier to identify issues.
- Real-time monitoring: Set up real-time monitoring to track key metrics such as system performance, resource utilization, and error rates.
- Use cloud-native monitoring tools: Use tools like AWS CloudWatch, Azure Monitor, and Google Cloud Operations suite to gain insights into the health and performance of your cloud infrastructure.
7. Regularly Review and Update Cloud Architecture
Cloud technologies evolve rapidly, and your architecture should evolve with them. Periodically review your architecture and make adjustments to incorporate new features, improve performance, and reduce costs. Best practices include:
- Perform architecture reviews: Regularly assess your cloud architecture to identify potential improvements or new cloud services that could enhance your deployment.
- Stay up to date with cloud updates: Keep track of new features, services, and best practices provided by your cloud provider to ensure your deployment is using the most efficient and secure options available.
- Refactor when necessary: As your application grows and evolves, refactor parts of the application or architecture to improve scalability, security, and maintainability.
8. Manage Compliance and Legal Requirements
Cloud deployments must adhere to various legal, regulatory, and compliance requirements, especially when dealing with sensitive data. Consider the following:
- Understand compliance needs: Ensure that your cloud deployment complies with relevant laws and regulations such as GDPR, HIPAA, PCI-DSS, etc.
- Use cloud provider certifications: Choose cloud providers who offer certifications for compliance with industry standards and regulations.
- Implement audit and access controls: Enable logging and auditing of access to sensitive data and resources to support compliance efforts.
9. Use Managed Services Where Possible
Managed services provided by cloud providers can help reduce the operational complexity of managing infrastructure and resources. Consider using the following managed services:
- Database as a Service (DBaaS): Use managed databases such as Amazon RDS, Azure SQL Database, or Google Cloud SQL to offload database management tasks like patching, backups, and scaling.
- Managed Kubernetes: Use managed container orchestration services like Amazon EKS, Azure Kubernetes Service (AKS), or Google Kubernetes Engine (GKE) to simplify container management.
- Serverless computing: Use serverless computing services such as AWS Lambda, Azure Functions, or Google Cloud Functions to run code without managing servers.
Conclusion
By following these best practices, you can ensure that your cloud deployment is secure, scalable, cost-efficient, and reliable. Continuously monitor and optimize your cloud resources to make the most of the cloud's flexibility while avoiding common pitfalls. Implementing these best practices will help you deliver high-performance applications and services in the cloud with minimal operational overhead.
MySQL Monitoring Tools
Monitoring is critical to ensuring the health, performance, and stability of MySQL databases. There are several tools available to help monitor MySQL instances, databases, and queries. The two most commonly used tools for MySQL monitoring are MySQL Enterprise Monitor and Percona Monitoring and Management (PMM). Below is an overview of these tools and their features.
1. MySQL Enterprise Monitor
MySQL Enterprise Monitor is a commercial solution from Oracle designed to provide real-time monitoring, diagnostics, and performance tuning for MySQL databases. It offers a user-friendly interface and a comprehensive set of features to help database administrators (DBAs) manage their MySQL infrastructure effectively.
Key Features of MySQL Enterprise Monitor:
- Real-Time Monitoring: Continuously tracks MySQL performance metrics such as server load, query response time, and database health.
- Query Analyzer: Identifies slow or problematic queries and suggests optimizations for improving database performance.
- Alerting and Notifications: Configurable alerts for various performance issues such as high disk usage, slow queries, or replication lag.
- Performance Tuning: Provides recommendations for optimizing server configuration, query execution, and index usage.
- Replication Monitoring: Monitors replication health to ensure that replication between master and slave servers is functioning correctly.
- Dashboard and Reporting: Provides a customizable dashboard that gives an at-a-glance overview of the health and performance of MySQL instances and the ability to generate reports for analysis.
Advantages of MySQL Enterprise Monitor:
- Comprehensive Features: Offers complete monitoring, diagnostics, and tuning for MySQL databases.
- Vendor Support: Comes with support from Oracle, ensuring reliable assistance for any issues.
- Seamless Integration: Integrates easily with other Oracle products and tools.
Disadvantages of MySQL Enterprise Monitor:
- Cost: MySQL Enterprise Monitor is a paid service, making it less accessible for smaller businesses or those with limited budgets.
- Complexity: It may be overkill for smaller MySQL environments with simple monitoring needs.
2. Percona Monitoring and Management (PMM)
Percona Monitoring and Management (PMM) is an open-source monitoring tool designed to provide deep insights into MySQL performance. PMM offers a wide range of features for monitoring MySQL, MariaDB, and PostgreSQL databases, and it's a popular choice for those looking for a free and community-supported alternative to MySQL Enterprise Monitor.
Key Features of Percona Monitoring and Management (PMM):
- Comprehensive Database Metrics: Provides metrics for MySQL, MariaDB, and PostgreSQL, such as server health, query performance, and replication status.
- Query Analytics: Identifies slow queries and provides detailed analysis for tuning them, including query execution time and index usage.
- Real-Time Monitoring: Tracks server and database performance in real-time, including CPU, memory, disk usage, and network traffic.
- Customizable Dashboards: Offers customizable dashboards for visualizing database metrics and performance data in a user-friendly format.
- Alerting System: Configurable alerts for various database health parameters like replication issues, slow queries, and high resource utilization.
- Free and Open-Source: PMM is completely free and open-source, making it an attractive choice for small to medium-sized businesses.
Advantages of Percona Monitoring and Management (PMM):
- Cost-Effective: Free and open-source, making it an excellent choice for businesses with budget constraints.
- Comprehensive Support: Offers support for multiple database engines, including MySQL, MariaDB, and PostgreSQL.
- Customizable Dashboards: Dashboards can be tailored to meet specific monitoring needs, providing more flexibility than other monitoring tools.
Disadvantages of Percona Monitoring and Management (PMM):
- Limited Enterprise Support: While PMM is community-supported, there is no official enterprise-level support unless you purchase Percona's commercial support options.
- Requires Setup: Setting up PMM can be more complex compared to other monitoring tools, especially for users unfamiliar with Percona's ecosystem.
Comparison of MySQL Enterprise Monitor and Percona Monitoring and Management
Feature | MySQL Enterprise Monitor | Percona Monitoring and Management (PMM) |
---|---|---|
Cost | Paid | Free (open-source) |
Vendor Support | Provided by Oracle | Community-supported (Commercial support available) |
Supported Databases | MySQL | MySQL, MariaDB, PostgreSQL |
Real-Time Monitoring | Yes | Yes |
Alerting System | Yes | Yes |
Query Analytics | Yes | Yes |
Dashboards | Customizable | Highly customizable |
Conclusion
Both MySQL Enterprise Monitor and Percona Monitoring and Management (PMM) offer strong monitoring capabilities for MySQL databases. However, the choice between the two depends on factors such as budget, support needs, and the complexity of the MySQL environment. MySQL Enterprise Monitor is ideal for businesses seeking comprehensive support and advanced features, while PMM is an excellent choice for those looking for a free, open-source, and customizable monitoring solution.
Key Performance Metrics in MySQL
To effectively monitor and optimize MySQL databases, it's important to track several key performance metrics that provide insights into the health, speed, and efficiency of the system. These metrics help database administrators (DBAs) identify issues and optimize performance. Below are some of the most important performance metrics to watch in MySQL.
1. Queries Per Second (QPS)
Queries Per Second (QPS) is a metric that tracks the number of queries processed by MySQL in one second. It helps to evaluate how much load the server is handling and can indicate if there are performance bottlenecks. A low QPS can be a sign of underutilization, while an unusually high QPS may point to a server under stress.
Why QPS is Important:
- Load Analysis: Helps determine if the MySQL server is processing enough queries and can handle the expected workload.
- Performance Bottlenecks: A sudden drop in QPS may indicate issues such as server overload, inefficient queries, or hardware limitations.
- Capacity Planning: QPS can help estimate future server needs and optimize resources for growing databases.
2. Latency
Latency refers to the time taken for a query to travel from the client to the server and back. It’s a critical metric for understanding the speed and responsiveness of MySQL. High latency can lead to slow response times and poor user experiences.
Why Latency is Important:
- Response Time: Latency directly affects how quickly users receive results from their queries.
- Network Delays: High latency can indicate network issues between MySQL clients and the server, as well as possible issues with hardware or configurations.
- Optimization Opportunities: Identifying latency bottlenecks can help in tuning MySQL settings, optimizing queries, or upgrading hardware.
3. Buffer Pool Metrics
The InnoDB buffer pool is a key component in MySQL that holds data and index pages in memory to speed up read and write operations. Monitoring buffer pool metrics is essential for understanding how well MySQL is handling cache and memory.
Key Buffer Pool Metrics:
- Buffer Pool Hit Rate: This metric shows how often MySQL can retrieve data from the buffer pool rather than from disk. A high hit rate indicates effective memory usage, while a low hit rate suggests that MySQL is relying too heavily on disk I/O.
- Buffer Pool Size: The total amount of memory allocated for the buffer pool. Ensuring it is large enough to hold frequently accessed data can significantly improve performance.
- Buffer Pool Efficiency: Measures how efficiently memory is being used. Low efficiency can indicate wasted memory, which could be reallocated for better performance.
Why Buffer Pool Metrics are Important:
- Memory Efficiency: Helps assess if MySQL is using memory optimally to reduce disk I/O.
- Performance Tuning: Identifies when the buffer pool may need to be resized or optimized for better performance.
- Disk I/O Reduction: A well-sized buffer pool helps reduce the need for disk access, improving query speed and overall database performance.
4. CPU and Memory Usage
CPU and memory usage metrics give insights into the overall resource utilization of the MySQL server. Excessive CPU or memory usage can lead to resource contention and slow performance.
Why CPU and Memory Usage are Important:
- Resource Allocation: Helps ensure that MySQL has sufficient CPU and memory resources for optimal performance.
- Bottleneck Detection: High CPU usage or excessive memory consumption may indicate performance bottlenecks caused by inefficient queries, improper server configuration, or hardware limitations.
- Server Health: Continuous monitoring of CPU and memory usage can help prevent system crashes and performance degradation.
5. Disk I/O Metrics
Disk I/O metrics track the rate at which MySQL reads and writes to the disk. High disk I/O can significantly slow down MySQL performance, especially for data-intensive applications.
Why Disk I/O Metrics are Important:
- Query Performance: Excessive disk reads or writes can slow down query execution and increase response times.
- Data Storage Efficiency: Helps identify when disk performance is becoming a limiting factor and whether SSDs or faster storage solutions are needed.
- Optimization: Identifying heavy disk usage can guide you towards optimizing queries, indexing strategies, or the buffer pool size to reduce disk access.
6. Replication Metrics
Replication metrics are used to track the health and performance of MySQL replication. These metrics help ensure that data is replicated from the master to the slave without issues, which is crucial for high availability and failover scenarios.
Why Replication Metrics are Important:
- Replication Lag: Measures the delay between the master and slave servers. High replication lag can cause inconsistent data between servers and affect the performance of read queries on the slave.
- Replication Health: Ensures that replication is functioning properly, with no errors or interruptions.
- Failover Readiness: Helps ensure that, in case of a master failure, the slave can take over with minimal data loss and downtime.
7. Connections and Threads
Connections and threads metrics track the number of active client connections and threads executing queries. Monitoring these metrics can help understand the load on the server and detect issues related to connection handling.
Why Connections and Threads Metrics are Important:
- Connection Limits: Helps track whether the server is hitting connection limits, which may require adjusting configuration settings.
- Thread Utilization: Identifies whether threads are being used efficiently, and if there are any long-running or blocked queries.
- Concurrency: Helps determine how many concurrent queries the server is handling and whether it’s approaching its limits.
Conclusion
Monitoring these key performance metrics in MySQL is essential for maintaining a healthy, efficient, and responsive database system. By keeping track of QPS, latency, buffer pool metrics, CPU and memory usage, disk I/O, replication health, and connection metrics, DBAs can ensure that their MySQL databases are performing optimally, troubleshoot issues quickly, and plan for future scaling needs.
Slow Query Log and Identifying Performance Bottlenecks
MySQL's slow query log is a useful tool for monitoring the performance of database queries. It helps in identifying slow-running queries that may be causing performance bottlenecks. By analyzing the slow query log, database administrators (DBAs) can optimize queries, improve database performance, and ensure the system is running efficiently.
What is the Slow Query Log?
The slow query log is a feature in MySQL that logs all queries that take longer than a specified threshold to execute. This log helps identify queries that may be inefficient, lack proper indexing, or require optimization. By examining slow queries, DBAs can pinpoint performance bottlenecks and take corrective actions to improve overall database performance.
Key Features of the Slow Query Log:
- Threshold Time: MySQL allows you to set a threshold time for queries that exceed a certain execution time. Any query that runs longer than this time is logged in the slow query log.
- Query Details: Each logged query includes details such as the query execution time, lock time, number of rows examined, and the actual SQL query.
- Query Tuning: The slow query log provides a foundation for query optimization by highlighting inefficient queries that require indexing, rewriting, or other performance improvements.
Enabling and Configuring the Slow Query Log
To enable the slow query log in MySQL, the following steps can be followed. You can configure the log file location, threshold execution time, and whether to log queries without indexes.
Steps to Enable the Slow Query Log:
- Edit MySQL Configuration File: Open your MySQL configuration file (my.cnf or my.ini) and add the following configurations to enable slow query logging:
- Restart MySQL Server: After modifying the configuration file, restart the MySQL server to apply the changes.
- Verify Log is Enabled: You can verify that the slow query log is enabled by running the following command in the MySQL client:
SHOW VARIABLES LIKE 'slow_query_log';
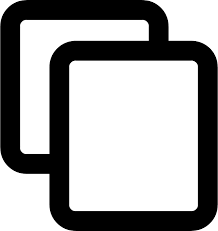
slow_query_log = 1
slow_query_log_file = /path/to/slow-query.log
long_query_time = 2 # Log queries taking longer than 2 seconds
log_queries_not_using_indexes = 1 # Optionally log queries without indexes
Analyzing the Slow Query Log
Once the slow query log is enabled, MySQL will start logging slow queries. The log entries provide valuable information about query performance, which can be analyzed to identify and resolve performance issues.
Key Fields in the Slow Query Log:
- Query Time: The time it took to execute the query (in seconds). This is the most important field for identifying slow queries.
- Lock Time: The time spent waiting for locks to be released. A high lock time may indicate issues with concurrency and locking.
- Rows Examined: The number of rows MySQL needed to examine to execute the query. A high number of rows examined often indicates that the query is not optimized and might need indexing.
- SQL Query: The actual query that was executed. This allows you to inspect the query and determine if optimizations are needed.
Tools for Analyzing the Slow Query Log:
- mysqldumpslow: A command-line tool that summarizes slow query logs and groups similar queries to provide a high-level overview of performance issues. Example:
mysqldumpslow -s t /path/to/slow-query.log
- Percona Toolkit (pt-query-digest): A more advanced tool for analyzing slow query logs. It provides detailed insights and can help identify query patterns, optimize indexes, and suggest changes.
- MySQL Enterprise Monitor: A monitoring tool that provides real-time insights into MySQL performance, including slow query log analysis.
Identifying Performance Bottlenecks
Analyzing the slow query log is an essential step for identifying performance bottlenecks in MySQL databases. Here are some common bottlenecks and optimization strategies:
Common Performance Bottlenecks:
- Missing Indexes: Queries that examine many rows often lack appropriate indexes. Adding indexes to frequently queried columns can drastically improve performance.
- Inefficient Queries: Complex or suboptimal queries may take a long time to execute. Optimizing queries by rewriting them or breaking them into smaller queries can improve performance.
- Large Datasets: Queries that need to process large amounts of data, such as full table scans, can be slow. Consider partitioning tables or optimizing query conditions to reduce the dataset size.
- Lock Contention: Queries that take a long time to acquire locks can cause bottlenecks in a multi-threaded environment. Reducing lock contention by optimizing transactions and ensuring indexes are used can improve overall performance.
Optimization Strategies:
- Indexing: Ensure queries use appropriate indexes to speed up data retrieval. Use the
EXPLAIN
command to analyze query execution plans and identify missing or ineffective indexes. - Query Rewrite: Refactor inefficient queries to reduce execution time. This might involve breaking down complex queries or using more efficient JOINs, WHERE clauses, or aggregate functions.
- Optimizing Schema: Optimize table structure and relationships. Consider de-normalization for performance in certain use cases or partitioning large tables for faster query performance.
- Reducing Query Load: Use caching mechanisms, query optimization, and materialized views to reduce the number of expensive queries executed by the database.
- Monitoring and Alerting: Implement automated monitoring systems to track slow queries, replication lag, and other performance issues in real-time and take proactive measures.
Conclusion
Using the slow query log in MySQL is a powerful technique for identifying and addressing performance bottlenecks. By enabling the slow query log and analyzing its contents, DBAs can optimize query performance, reduce database load, and ensure that MySQL runs efficiently. Regularly monitoring and optimizing slow queries is key to maintaining a high-performing database, especially in production environments where performance is critical.
Optimizing Hardware Resources for Better Performance
Optimizing hardware resources is essential for improving the overall performance of MySQL databases, especially under heavy workloads or high-traffic scenarios. Properly tuned hardware can significantly enhance query execution times, reduce latency, and improve system stability. In this section, we will discuss how to optimize different hardware components for better MySQL performance.
Key Hardware Components for MySQL Performance
Several hardware components play a crucial role in MySQL database performance. Optimizing these components can lead to a more efficient and high-performing database system:
- CPU: The processor is responsible for executing queries and database operations. A faster CPU with more cores can handle concurrent requests more efficiently.
- Memory (RAM): Adequate RAM is essential for caching data and reducing disk I/O. Insufficient memory can lead to excessive paging, slowing down query execution.
- Disk Storage: Disk speed affects data retrieval times, especially for I/O-bound operations. SSDs (Solid-State Drives) are preferred over HDDs (Hard Disk Drives) for faster read/write operations.
- Network: A high-speed network connection is necessary for MySQL replication, data transfer, and client-server communication. Network latency can impact query performance, especially in distributed systems.
Optimizing CPU for MySQL Performance
The CPU plays a significant role in query execution, especially for complex queries with many joins, aggregations, or calculations. Optimizing CPU resources can greatly improve MySQL performance.
Strategies for CPU Optimization:
- Multi-Core Processors: MySQL can take advantage of multi-core processors for parallel query execution. Ensure that your MySQL server is running on a machine with a high-performance multi-core CPU to handle concurrent queries.
- Query Optimization: Poorly optimized queries can put unnecessary strain on the CPU. Use `EXPLAIN` to analyze and optimize your queries, reducing CPU load by eliminating inefficient joins and subqueries.
- Thread Pooling: Thread pooling is a technique that manages and limits the number of concurrent threads handled by the CPU. This helps prevent CPU overload during periods of high database traffic.
Optimizing Memory (RAM) for MySQL Performance
Memory is a crucial resource for MySQL, as it is used for caching query results, indexes, and data. Insufficient RAM can lead to heavy disk I/O, which severely impacts performance. Optimizing memory ensures faster access to data and reduces the need for expensive disk reads.
Strategies for Memory Optimization:
- InnoDB Buffer Pool Size: The InnoDB buffer pool stores data and index pages in memory. Increasing the `innodb_buffer_pool_size` variable allows MySQL to cache more data, reducing disk reads and improving performance. A good rule of thumb is to allocate up to 80% of your server’s total RAM to the buffer pool.
- Query Cache: MySQL's query cache stores the result of SELECT queries for faster retrieval in subsequent requests. Tuning the `query_cache_size` and enabling the query cache can reduce CPU and disk load by serving cached results. However, this feature is deprecated in newer MySQL versions, and its use is limited to certain workloads.
- Memory Allocation for Sorting and Join Operations: Increase the `sort_buffer_size`, `join_buffer_size`, and `read_buffer_size` parameters to optimize memory usage for complex operations such as sorting and joining. This helps reduce the need for temporary tables on disk.
Optimizing Disk Storage for MySQL Performance
Disk I/O is often a bottleneck in MySQL performance, especially when dealing with large datasets or complex queries. Optimizing disk storage can improve query response times and reduce latency in I/O-bound operations.
Strategies for Disk Storage Optimization:
- Solid-State Drives (SSDs): SSDs provide faster read and write speeds compared to traditional HDDs, which results in reduced I/O latency. If you're running a high-performance MySQL database, consider upgrading to SSDs for faster data retrieval and storage.
- RAID Configurations: RAID (Redundant Array of Independent Disks) configurations can improve disk performance and reliability. RAID 0 offers better read/write speeds by striping data across multiple disks, while RAID 1 ensures redundancy with mirrored disks. RAID 10 provides both speed and redundancy.
- Separate Data and Log Files: Separating MySQL data and log files onto different physical disks can help improve performance. This reduces contention between read/write operations on the data and log files, ensuring faster transactions and lower latency.
- Disk I/O Scheduling: Use the `noatime` mount option for disk partitions where MySQL data is stored. This prevents MySQL from writing access times to disk, reducing unnecessary disk I/O operations.
Optimizing Network Resources for MySQL Performance
Network latency and bandwidth can impact MySQL performance, especially in distributed environments where replication, clustering, and client-server communication are involved. Optimizing network resources ensures smooth communication and faster query execution.
Strategies for Network Optimization:
- High-Speed Network Connections: Ensure that the network connection between your MySQL server and clients or replication nodes is fast and reliable. Use gigabit or 10-gigabit Ethernet for high-throughput environments.
- Network Latency Reduction: Minimize network latency by optimizing network configurations, using low-latency switches, and ensuring that MySQL servers are geographically located close to their clients or replication nodes.
- Use Compression for Data Transfer: When replicating data or performing backups, enable compression to reduce the amount of data transmitted over the network. This can improve the performance of replication and reduce bandwidth consumption.
Monitoring and Scaling Hardware Resources
Constant monitoring of hardware resources ensures that you can identify resource limitations and address them before they impact performance. Use monitoring tools to keep track of CPU, memory, disk, and network usage in real-time.
Tools for Monitoring Hardware Resources:
- MySQL Enterprise Monitor: A comprehensive monitoring tool for tracking MySQL performance, including CPU, memory, disk I/O, and query performance.
- Percona Monitoring and Management (PMM): An open-source tool for monitoring MySQL server performance, resource usage, and query analysis.
- System Monitoring Tools: Tools like
top
,htop
, andiostat
provide real-time monitoring of system resources like CPU, memory, disk I/O, and network usage.
Conclusion
Optimizing hardware resources is a crucial aspect of improving MySQL database performance. By fine-tuning CPU, memory, disk storage, and network resources, you can ensure that your MySQL server operates at its peak performance. Regular monitoring and proactive adjustments to hardware configurations can help reduce bottlenecks, minimize latency, and support high-throughput database operations.
Adjusting Configuration Settings for MySQL Optimization
Optimizing MySQL performance requires tuning several configuration settings to match the specific needs and workload of your database. Properly adjusting these settings can lead to faster query execution, improved resource utilization, and better overall system performance. In this section, we'll discuss key MySQL configuration settings and how to adjust them for optimization.
Key MySQL Configuration Parameters for Optimization
MySQL provides a wide range of configuration parameters that influence how the database manages memory, handles connections, performs queries, and more. Here are some of the most important parameters to adjust for performance optimization:
- innodb_buffer_pool_size: This setting controls the size of the InnoDB buffer pool, which is where InnoDB caches data and indexes. A larger buffer pool improves performance by reducing disk I/O operations, as more data can be stored in memory. Set it to 60-80% of available system memory for optimal performance.
- max_connections: This parameter defines the maximum number of simultaneous client connections allowed to the MySQL server. If your application experiences a large number of concurrent connections, increase this setting. However, setting it too high can lead to excessive memory usage. It should be balanced with available system resources.
- query_cache_size: The query cache stores the result of SELECT queries and returns cached results for identical queries, reducing CPU and disk I/O load. However, query caching is deprecated in newer MySQL versions. If you're using MySQL 5.7 or later, consider disabling the query cache and relying on other caching mechanisms.
- innodb_log_file_size: This parameter sets the size of the InnoDB redo log files. Larger log files can improve performance for write-heavy workloads, as they reduce disk I/O for logging operations. However, increasing this value also increases recovery time in case of crashes, so balance this with your system's tolerance for downtime.
- innodb_flush_log_at_trx_commit: This setting controls the frequency at which InnoDB flushes the redo log to disk. The default value of 1 ensures ACID compliance, but setting it to 2 or 0 can improve performance at the cost of durability in case of a crash.
- tmp_table_size: This parameter determines the maximum size of temporary tables in memory. If a temporary table exceeds this size, it is written to disk, which can reduce performance. Increasing the value of `tmp_table_size` can reduce disk writes and improve performance for complex queries and joins.
- sort_buffer_size: This buffer is used for sorting operations. Increasing this value can improve performance for queries that require sorting, such as ORDER BY or GROUP BY operations. However, setting it too high can lead to excessive memory usage, especially with many concurrent connections.
- join_buffer_size: This buffer is used for joins that cannot use indexes efficiently. Increasing this value can improve the performance of certain joins, especially for large tables, but it should be adjusted carefully to avoid excessive memory usage for each connection.
- read_rnd_buffer_size: This buffer is used for reading rows in a random order. If your queries perform many random reads, increasing this value can improve performance. However, setting it too high can negatively affect performance and memory usage.
Optimizing MySQL Configuration for Different Workloads
The optimal configuration settings for MySQL depend on the type of workload your database handles. Here are some recommendations for different use cases:
For Transactional Workloads (OLTP):
- innodb_buffer_pool_size: Set this to a high value to cache more data and reduce disk I/O. A common recommendation is 60-80% of available system memory.
- innodb_flush_log_at_trx_commit: The default value (1) ensures full ACID compliance, but for write-heavy workloads, you can set it to 2 or 0 to improve performance at the cost of durability.
- innodb_log_file_size: Increase this for write-heavy workloads to reduce disk I/O and improve write performance.
- max_connections: Set an appropriate value based on the expected number of concurrent connections. A larger value may be needed for high-traffic transactional systems.
For Analytical Workloads (OLAP):
- innodb_buffer_pool_size: Allocate a large portion of system memory to the buffer pool for caching large datasets.
- sort_buffer_size: Increase this value to improve the performance of sorting and aggregation operations, which are common in analytical queries.
- read_rnd_buffer_size: Increase this setting if your queries perform many random reads, such as in data analysis or reporting scenarios.
- tmp_table_size: Increase the size of temporary tables to avoid disk writes when performing complex queries or aggregations.
For Mixed Workloads:
- innodb_buffer_pool_size: Set this value based on the balance between transactional and analytical workload requirements.
- max_connections: Set this value based on the expected number of simultaneous connections, but avoid setting it too high to prevent memory overuse.
- join_buffer_size: Fine-tune this value based on the performance of joins in your queries, especially for large tables.
MySQL Tuning for High-Availability Environments
In high-availability environments, such as those using MySQL replication or clustering, additional tuning is necessary to ensure minimal downtime, fast failover, and optimal performance during replication.
Recommended Settings for High-Availability MySQL:
- sync_binlog: Set this parameter to 1 to ensure that binary logs are synchronized to disk after each transaction. This ensures data durability in the event of a crash but may impact performance in write-heavy environments.
- innodb_flush_log_at_trx_commit: Set this to 2 or 0 for improved performance during replication, but be aware that this may compromise durability in certain cases.
- read_only: Set this parameter on replication slave servers to prevent writes to the slave, ensuring that it only serves read requests.
- gtid_mode: Enable GTID (Global Transaction Identifiers) mode for easier replication management and failover in clustered environments.
Other Configuration Settings to Consider
- table_open_cache: Increase this value to allow MySQL to open and cache more table descriptors, improving performance for databases with many tables.
- max_allowed_packet: Increase this setting if you are working with large BLOBs or long queries to prevent errors related to packet size.
- innodb_thread_concurrency: Adjust this setting based on the number of available CPU cores to control the number of threads InnoDB can use simultaneously.
- innodb_read_io_threads and innodb_write_io_threads: Increase these values to allow InnoDB to use multiple threads for I/O operations, improving performance on systems with multiple CPU cores.
Monitoring and Adjusting Configuration Settings
After making changes to MySQL configuration settings, it is important to monitor the database’s performance to ensure that the adjustments are effective. Use MySQL’s built-in performance schema, as well as tools like Percona Monitoring and Management (PMM), to track key metrics such as query response time, buffer pool usage, and disk I/O performance.
Tools for Monitoring MySQL Performance:
- MySQL Enterprise Monitor: Provides in-depth insights into MySQL performance, including query analysis, memory usage, and configuration settings.
- Percona Monitoring and Management (PMM): An open-source tool that offers real-time monitoring, historical reporting, and query performance optimization.
- MySQL Performance Schema: A built-in feature that allows you to track internal MySQL performance metrics, including query execution times and resource usage.
Conclusion
Adjusting MySQL configuration settings is a critical step in optimizing database performance. By carefully tuning key parameters like InnoDB buffer pool size, query cache, memory allocation, and disk settings, you can significantly improve MySQL’s efficiency and responsiveness. Regular monitoring and adjustments based on workload requirements will help maintain optimal performance as your database grows.
Using MySQL Workbench for Database Design and Management
MySQL Workbench is a powerful visual tool used for database design, administration, and management. It allows users to design, model, manage, and maintain MySQL databases in a user-friendly graphical interface. In this section, we will cover how to use MySQL Workbench for effective database management, including database design, query development, server administration, and performance monitoring.
Overview of MySQL Workbench
MySQL Workbench is an integrated environment that provides a set of tools for managing MySQL databases. It includes features such as:
- Database Design: Tools for creating and managing database schemas, tables, and relationships using a visual interface.
- SQL Development: A powerful SQL editor for writing and executing queries, with features such as syntax highlighting, code completion, and query execution plans.
- Server Administration: Tools for managing MySQL server instances, users, permissions, and backups.
- Performance Monitoring: Tools for monitoring server health, query performance, and resource usage.
Database Design with MySQL Workbench
MySQL Workbench allows you to design your database visually using an Entity-Relationship (ER) diagram. This tool simplifies the database creation process by enabling you to create tables, define relationships, and set constraints without writing SQL code manually.
Creating a New Database Model
- Open MySQL Workbench and go to the File menu. Select New Model to create a new database design.
- In the new model, you can start adding tables, views, and relationships by dragging and dropping elements from the sidebar.
- To add a new table, click on the Place a New Table icon from the toolbar, then click on the model canvas to create the table.
- Define the table’s name, columns, data types, and constraints (primary keys, foreign keys, etc.).
- To define relationships between tables, use the Place a New Relationship tool. Select the tables you want to relate and define the relationship type (e.g., one-to-many, many-to-many).
- Once the design is complete, you can generate the SQL script to create the database using the Forward Engineer option, which will generate the corresponding SQL statements to create the schema in MySQL.
Modifying a Database Model
If you need to modify an existing database model, simply open the model in MySQL Workbench, and make changes to the tables, relationships, or other schema elements. Once you’ve made changes, use the Forward Engineer feature to apply those modifications to the live database.
SQL Development in MySQL Workbench
MySQL Workbench provides a robust SQL editor that allows you to write, execute, and analyze SQL queries. It offers features like syntax highlighting, code completion, and easy access to query execution plans, which make query development more efficient and user-friendly.
Running Queries
- Open MySQL Workbench and connect to your MySQL server instance.
- Navigate to the SQL Editor tab and start writing your SQL queries in the editor window.
- To execute a query, click on the Execute button or press Ctrl + Enter.
- Query results will be displayed in the output panel below the editor, where you can review the results, export them, or analyze execution plans.
Using Query Execution Plans
MySQL Workbench can generate query execution plans that help optimize SQL queries. An execution plan outlines how MySQL will execute a given query, including which indexes will be used, the order of operations, and the amount of data that will be processed.
- To view the execution plan for a query, click on the Explain button next to the Execute button.
- The execution plan will appear in a tab below the query editor, providing insights into the efficiency of the query and any potential bottlenecks.
- Use the execution plan information to optimize slow queries by adding indexes or revising the query structure.
Server Administration in MySQL Workbench
MySQL Workbench provides a suite of tools for server management, including user and privilege management, server configuration, and server logs.
Managing Users and Privileges
- In the Server Administration tab, go to the Users and Privileges section.
- You can add new users, assign privileges, and manage roles from the Users and Privileges interface.
- Click on the Add Account button to create a new user and assign specific privileges (e.g., SELECT, INSERT, UPDATE).
- To modify an existing user’s privileges, select the user from the list, and edit the privileges as needed.
Configuring Server Settings
MySQL Workbench allows you to configure the MySQL server settings directly from the GUI.
- In the Server Administration tab, go to the Configuration section.
- You can modify variables related to memory, connections, and other parameters. Click Apply to save changes to the server configuration.
Performance Monitoring in MySQL Workbench
MySQL Workbench includes performance monitoring tools that allow you to monitor server health, query performance, and resource usage in real-time.
Monitoring Server Health
- Navigate to the Performance tab in MySQL Workbench.
- You can view real-time statistics on server health, including CPU usage, memory usage, and disk I/O.
- Use the Server Status tab to view the current status of the server, including active connections, query cache usage, and other important metrics.
Analyzing Queries
MySQL Workbench provides the Query Statistics panel, which displays information about long-running queries, query execution times, and resource utilization.
- Go to the Server Status section and click on Threads to view a list of running threads and their query performance.
- Use this information to identify slow queries and optimize them accordingly.
Best Practices for Using MySQL Workbench
- Regular Backups: Use MySQL Workbench to create and schedule regular backups of your databases to prevent data loss.
- Use Version Control: For complex database designs, consider using version control systems (e.g., Git) to manage changes to your database schema and SQL scripts.
- Optimize Queries: Regularly analyze and optimize slow queries using the query execution plan and performance monitoring tools.
- Use ER Diagrams: Leverage ER diagrams to visualize relationships between tables and ensure your database schema is properly designed.
- Monitor Server Health: Continuously monitor server health and performance to identify and resolve any issues before they affect your application.
Conclusion
MySQL Workbench is a comprehensive tool for database design, management, and optimization. By using its visual design features, SQL development tools, server administration capabilities, and performance monitoring tools, you can manage your MySQL databases efficiently and ensure optimal performance. Regularly using MySQL Workbench for database management tasks like creating, modifying, and optimizing your databases will help streamline your workflows and improve overall productivity.
Query Building and Execution
Building and executing SQL queries is a core aspect of working with databases. Whether you are retrieving data, inserting new records, updating existing data, or performing complex analysis, understanding how to build and execute SQL queries is essential. This section will guide you through the process of building SQL queries, executing them, and analyzing the results using MySQL Workbench and other tools.
Types of SQL Queries
SQL queries can be categorized into various types depending on the operation you want to perform on the database. Some of the most common types of SQL queries include:
- SELECT: Retrieves data from one or more tables.
- INSERT: Adds new records to a table.
- UPDATE: Modifies existing records in a table.
- DELETE: Removes records from a table.
- CREATE: Defines a new table, database, or other database object.
- ALTER: Modifies an existing database object, like a table or column.
- DROP: Deletes a table, database, or other database object.
Building SQL Queries
When building SQL queries, it is important to understand the structure of SQL syntax and how to write efficient queries. Below are the basic components of SQL queries:
1. SELECT Query
The SELECT statement is used to retrieve data from one or more tables. The basic syntax of a SELECT query is:
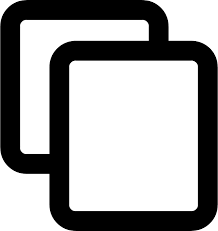
SELECT column1, column2, ... FROM table_name WHERE condition;
Example:
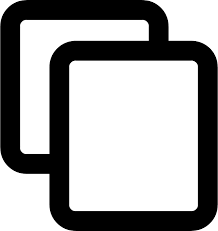
SELECT first_name, last_name FROM employees WHERE department = 'Sales';
This query retrieves the first name and last name of employees working in the 'Sales' department.
2. INSERT Query
The INSERT statement is used to insert new records into a table. The syntax is:
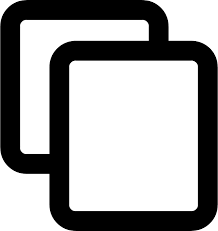
INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...);
Example:
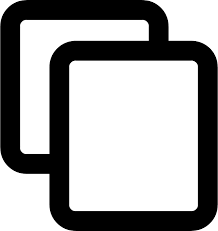
INSERT INTO employees (first_name, last_name, department) VALUES ('John', 'Doe', 'HR');
This query inserts a new employee named John Doe into the 'employees' table with the department set to 'HR'.
3. UPDATE Query
The UPDATE statement is used to modify existing records in a table. The syntax is:
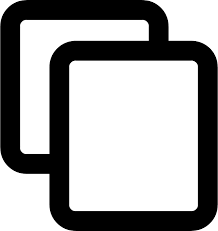
UPDATE table_name SET column1 = value1, column2 = value2 WHERE condition;
Example:
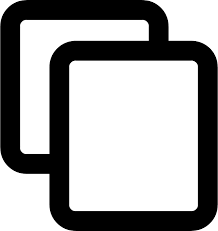
UPDATE employees SET department = 'Marketing' WHERE first_name = 'John' AND last_name = 'Doe';
This query updates the department of the employee named John Doe to 'Marketing'.
4. DELETE Query
The DELETE statement is used to remove records from a table. The syntax is:
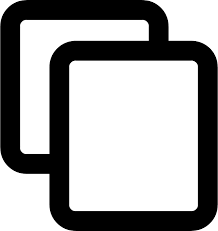
DELETE FROM table_name WHERE condition;
Example:
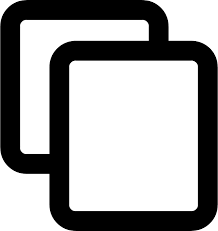
DELETE FROM employees WHERE department = 'HR';
This query deletes all employees in the 'HR' department.
Executing SQL Queries
Once you have built your SQL query, the next step is to execute it. The process of executing a query will depend on the tool you are using. In MySQL Workbench, here's how you can execute a query:
1. Connecting to the Database
Before executing any queries, you must connect to your MySQL server and select the appropriate database. Follow these steps:
- Open MySQL Workbench and connect to your MySQL server instance by providing the necessary credentials (host, username, password).
- Once connected, select the database you want to query from the left sidebar under SCHEMAS.
2. Executing a Query in MySQL Workbench
- Open the SQL Editor by navigating to the SQL tab.
- Write your SQL query in the editor window.
- To execute the query, click the Execute (lightning bolt) button or press Ctrl + Enter.
- Results will appear in the lower part of the screen. If the query modifies data, such as an INSERT, UPDATE, or DELETE query, you will see the affected rows count in the output panel.
3. Viewing Query Results
After executing a SELECT query, the results will be displayed in a tab below the SQL editor. You can:
- View the data in a tabular format.
- Export the results to formats like CSV, JSON, or Excel by clicking the Export button in the result set panel.
- Analyze the results to verify whether the data retrieved matches your expectations.
4. Handling Errors
If there is an error in the query, it will be displayed in the output panel. Common errors include:
- Syntax errors: These are caused by incorrect SQL syntax, such as missing commas or incorrect keywords.
- Logical errors: These occur when the query executes but doesn't return the expected results, such as a missing WHERE condition in a DELETE query.
- Connection errors: These happen when MySQL Workbench cannot connect to the MySQL server, possibly due to incorrect credentials or network issues.
Best Practices for Query Building and Execution
- Use clear and descriptive aliases: When working with multiple tables, use table aliases to make your queries more readable.
- Test your queries: Always test your queries on a small dataset or in a development environment before running them on production data.
- Use parameterized queries: When developing applications, always use parameterized queries to prevent SQL injection attacks.
- Optimize queries: Use indexes, avoid SELECT *, and analyze execution plans to improve query performance.
- Keep backup copies: Before executing UPDATE or DELETE queries that modify data, ensure you have a backup of the data in case you need to revert changes.
Conclusion
Building and executing SQL queries is a fundamental skill for database management and application development. By understanding the various types of SQL queries, learning how to build them correctly, and practicing effective query execution, you can efficiently interact with your database and retrieve or modify data as needed. Following best practices will help ensure that your queries are both effective and secure, providing optimal performance and avoiding common pitfalls.
Database Administration through GUI
Database administration through a Graphical User Interface (GUI) simplifies many administrative tasks, making it easier for users to manage, monitor, and optimize databases without needing to write complex SQL queries. In this section, we will explore the tools and techniques for administering MySQL databases using a GUI, focusing on MySQL Workbench, phpMyAdmin, and other popular tools.
Why Use a GUI for Database Administration?
Using a GUI for database administration can offer several benefits:
- Ease of Use: GUIs provide a user-friendly interface for managing databases, making it easier to interact with database objects and perform administrative tasks.
- Visual Representation: Databases, tables, and relationships are often represented visually, which helps users understand the database structure quickly.
- Minimized Error Risk: With GUIs, many common tasks (like creating tables or executing queries) are simplified, reducing the chances of making errors in SQL syntax.
- Faster Setup and Configuration: Many common configuration tasks, such as user management or backup creation, can be done quickly through a GUI, making it ideal for those who are not comfortable with command-line tools.
Popular Database Administration GUI Tools
There are several tools available to manage MySQL databases through a GUI. Some of the most popular options include:
1. MySQL Workbench
MySQL Workbench is a powerful and comprehensive GUI tool developed by Oracle for MySQL database design, administration, and management. It provides features like:
- Database Design: Create and manage databases visually using an easy-to-use interface.
- SQL Development: Write, execute, and debug SQL queries in a visual editor.
- Server Administration: Manage server settings, user accounts, backups, and more.
- Data Modeling: Create Entity-Relationship (ER) diagrams for visual representation of tables and their relationships.
- Performance Monitoring: Monitor server health and performance with built-in tools and dashboards.
2. phpMyAdmin
phpMyAdmin is a popular web-based GUI tool for managing MySQL databases. It is especially popular in shared hosting environments, as it is easy to install and use. Some of its features include:
- Database Management: Create, delete, and modify databases, tables, and columns.
- SQL Query Execution: Execute custom SQL queries directly from the web interface.
- Backup and Restore: Easily back up and restore databases through the web interface.
- User Management: Manage MySQL user accounts and assign privileges to control access.
- Import/Export Data: Import and export databases and tables in various formats, including CSV, SQL, and Excel.
3. DBeaver
DBeaver is a universal database administration tool that supports multiple database types, including MySQL. It provides features like:
- Multi-Database Support: Connect to and manage multiple database types, including MySQL, PostgreSQL, Oracle, and more.
- SQL Editor: Write and execute SQL queries with syntax highlighting and auto-completion.
- Data Visualization: Visualize database structure and relationships in ER diagrams.
- Database Management: Manage tables, indexes, views, and other database objects.
- Cross-Platform: Available on Windows, macOS, and Linux.
Administering MySQL Databases Using a GUI
Database administration through a GUI typically involves performing common tasks such as managing users, backing up data, optimizing queries, and monitoring server health. Below are some of the key tasks that can be managed through GUIs like MySQL Workbench and phpMyAdmin:
1. Creating and Managing Databases
Using a GUI, you can easily create new databases, modify existing ones, and delete databases that are no longer needed. Here’s how it’s done in MySQL Workbench:
- Open MySQL Workbench and connect to the MySQL server.
- In the left sidebar, right-click under the SCHEMAS section and select Create Schema to create a new database.
- Give the database a name, choose a character set and collation, then click Apply.
- To modify an existing database, right-click on the database name and select Alter Schema to change properties.
2. Managing Users and Privileges
GUIs make it easy to create new users, assign privileges, and manage user accounts. In MySQL Workbench:
- Go to the Server menu and select Users and Privileges.
- Click on the + sign to add a new user or select an existing user to modify their privileges.
- Assign global, database, or table-level privileges to the user based on their role.
- Click Apply to save the changes.
3. Backing Up and Restoring Databases
Backing up and restoring databases is another essential task for database administrators. In MySQL Workbench, you can perform a backup by:
- Selecting the Data Export option under the Management tab.
- Choose the databases and tables you want to back up and select the export format (e.g., SQL or CSV).
- Click Start Export to back up the selected data.
To restore a database, use the Data Import feature and select a backup file to import.
4. Query Optimization and Performance Monitoring
GUIs like MySQL Workbench provide tools to analyze and optimize queries. You can use the Query Profiler to identify slow or inefficient queries, and the Performance Dashboard to monitor key metrics like query execution time, cache hit rate, and server load.
Additionally, indexes and query execution plans can be visualized and optimized directly from the GUI to improve performance.
5. Importing and Exporting Data
GUIs also allow you to import and export data between databases and external files. In phpMyAdmin:
- To import data, go to the database, click the Import tab, and select the file to import.
- To export, go to the Export tab, choose the format (e.g., SQL, CSV), and click Go to download the file.
Best Practices for Using a GUI for Database Administration
- Stay Organized: Keep your database objects (tables, views, indexes) well-organized to make it easier to find and manage them.
- Use Database Modeling: Use ER diagrams and visual tools to design and understand your database structure before making changes.
- Backup Regularly: Always create backups before performing risky operations like deleting or altering tables.
- Test Queries Before Executing: Test any complex queries or modifications on a small or test database to prevent errors on production data.
- Manage User Access: Use the GUI to manage user accounts and set appropriate privileges to ensure security.
Conclusion
Using a GUI for database administration simplifies many of the tasks that would otherwise require complex SQL commands. Whether you're managing users, performing backups, or optimizing queries, tools like MySQL Workbench, phpMyAdmin, and DBeaver provide powerful features that make database management more accessible and efficient. By using these tools wisely, you can manage your databases more effectively and ensure their optimal performance and security.
Data Import/Export Using MySQL Workbench
MySQL Workbench is a powerful GUI tool that allows you to manage MySQL databases and perform various tasks such as data import and export. Importing and exporting data is essential for database migration, backup, or integration with external systems. This section provides a comprehensive guide on how to import and export data using MySQL Workbench.
Why Use MySQL Workbench for Data Import/Export?
MySQL Workbench provides an easy-to-use interface for importing and exporting databases and tables. Some of the key benefits include:
- User-Friendly Interface: MySQL Workbench simplifies complex tasks by providing a GUI, making it easier to handle large datasets.
- Support for Multiple Formats: You can export and import data in various formats like SQL, CSV, JSON, and XML.
- Data Transformation: During export and import, you can transform data formats, adjust field mappings, and set specific options to suit your needs.
- Backup and Restore: MySQL Workbench allows you to create backups of your databases and restore them efficiently.
Data Import Using MySQL Workbench
Importing data into MySQL Workbench can be done from various sources, such as CSV files, SQL scripts, or other database formats. Follow the steps below to import data into your MySQL database:
1. Importing from a CSV File
If you have a CSV file that contains data you want to import into a table, follow these steps:
- Open MySQL Workbench and connect to the MySQL server.
- Go to the Server menu and select Data Import.
- In the Import Options section, select Import from Self-Contained File and choose the CSV file from your local system.
- Select the target database and table where you want to import the data. If the table doesn't exist, you can create a new one.
- In the Options section, you can specify how the CSV data should be imported, such as delimiter settings, quotes, and encoding.
- Click Start Import to begin the import process. You can track the progress in the Action Output section.
2. Importing from an SQL Script
If you have an SQL file with INSERT statements or schema definitions, you can import it using MySQL Workbench:
- Open MySQL Workbench and connect to the MySQL server.
- Go to the File menu and select Open SQL Script to open the desired SQL file.
- Click the Execute button (lightning bolt icon) to run the SQL script and import the data into the target database.
Data Export Using MySQL Workbench
Exporting data from MySQL Workbench is just as simple as importing. You can export databases, tables, or specific data to different file formats like SQL, CSV, or JSON. Here's how to export data:
1. Exporting to an SQL File
Exporting data to an SQL file is useful for creating backups or migrating data. To export a database to an SQL file:
- Open MySQL Workbench and connect to the MySQL server.
- Go to the Server menu and select Data Export.
- In the Export Options section, select the database(s) and table(s) you want to export.
- Choose the export format as SQL (this will generate a file with SQL statements to recreate the database schema and data).
- Specify the output location for the exported file.
- Click Start Export to begin the export process. The SQL file will be saved at the specified location.
2. Exporting to a CSV File
Exporting data to CSV files is useful for reporting or integrating with other systems. To export a table to a CSV file:
- Open MySQL Workbench and connect to the MySQL server.
- Right-click on the table you want to export from the schema navigator and select Table Data Export Wizard.
- In the wizard, select CSV as the export format.
- Choose the fields you want to export, adjust delimiter settings, and specify the location to save the CSV file.
- Click Next and then Finish to complete the export process.
3. Exporting to JSON or Other Formats
To export data to JSON or other formats, MySQL Workbench provides the ability to write custom queries and export the result set in the desired format. Here's how to do it:
- Write a SELECT query in the SQL editor to fetch the desired data.
- Click the Execute button to run the query.
- Right-click the result set and select Export Resultset.
- Choose the file format (e.g., JSON, CSV) and specify the file location.
- Click Save to export the data in the selected format.
Handling Large Datasets
When importing or exporting large datasets, MySQL Workbench may take longer to process, depending on the size of the data. Here are some tips for handling large datasets:
- Use Batch Processing: If possible, split large imports or exports into smaller batches to avoid timeouts and improve performance.
- Increase Timeout Settings: Modify the timeout settings in MySQL Workbench to allow longer import/export durations.
- Use Command-Line Tools for Large Data: For very large datasets, consider using command-line tools like `mysqldump` or `mysqlimport`, which are optimized for handling large-scale data operations.
Best Practices for Data Import/Export
- Backup Before Importing: Always back up your database before performing data imports or exports to avoid data loss.
- Check Data Integrity: Ensure that the imported data maintains its integrity by validating the results after import.
- Use Proper Data Formats: Select the appropriate data format (e.g., CSV, SQL) based on your use case, whether it’s for data migration or integration.
- Limit Data Size: When exporting, avoid exporting too much data at once. Break large datasets into smaller parts if necessary.
Conclusion
MySQL Workbench provides a straightforward and efficient way to import and export data in various formats, making it an essential tool for database administrators and developers. Whether you're migrating data, creating backups, or integrating with other systems, MySQL Workbench simplifies these tasks with its user-friendly interface and powerful features.
Connecting MySQL with PHP (PDO, MySQLi)
Connecting MySQL with PHP is essential for developing dynamic websites and applications that interact with databases. PHP provides two primary methods for connecting to MySQL: PDO (PHP Data Objects) and MySQLi (MySQL Improved). Each method has its own features and advantages. In this guide, we will explore both PDO and MySQLi and help you choose the best approach for your project.
Why Connect MySQL with PHP?
PHP and MySQL are commonly used together for building database-driven applications. By connecting PHP to MySQL, you can perform operations such as:
- Inserting, updating, and deleting records in MySQL databases.
- Retrieving data from MySQL databases and displaying it on web pages.
- Executing complex queries and transactions to manipulate data.
PDO (PHP Data Objects)
PDO is a database access layer providing a uniform interface for interacting with different databases, including MySQL. It supports prepared statements, which offer better security and performance.
Advantages of PDO
- Database Agnostic: PDO supports multiple databases, including MySQL, PostgreSQL, SQLite, and more. This makes it easier to switch databases without major changes to your code.
- Prepared Statements: PDO supports prepared statements, which can help prevent SQL injection attacks.
- Better Error Handling: PDO offers better error handling and debugging features than MySQLi.
Connecting to MySQL Using PDO
Here’s how to connect to a MySQL database using PDO:
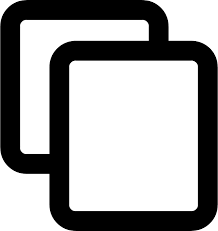
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully!";
}
catch(PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
In this example, replace `localhost` with your MySQL server address, `my_database` with your database name, and provide the correct `username` and `password` for the connection.
MySQLi (MySQL Improved)
MySQLi is an extension specifically designed for MySQL. It provides both a procedural and object-oriented interface for connecting and interacting with MySQL databases. Like PDO, MySQLi also supports prepared statements for increased security.
Advantages of MySQLi
- MySQL-Specific Features: MySQLi is tailored to work with MySQL, providing additional features like multi-queries and support for stored procedures.
- Performance: MySQLi may offer slightly better performance than PDO when working exclusively with MySQL databases.
- Support for Transactions: MySQLi supports transactions, which are useful for handling critical database operations.
Connecting to MySQL Using MySQLi (Object-Oriented)
Here’s how to connect to a MySQL database using MySQLi in an object-oriented style:
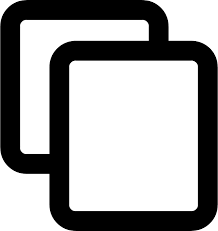
connect_error) {
die("Connection failed: " . $mysqli->connect_error);
}
echo "Connected successfully!";
?>
Connecting to MySQL Using MySQLi (Procedural)
You can also use the procedural style to connect to MySQL using MySQLi:
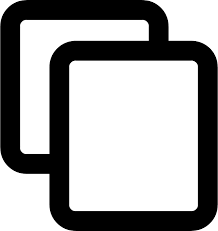
Prepared Statements with PDO and MySQLi
Both PDO and MySQLi support prepared statements, which are used to execute SQL queries securely. Prepared statements help prevent SQL injection attacks by binding variables to SQL statements before execution.
Prepared Statements in PDO
Here’s an example of using prepared statements in PDO:
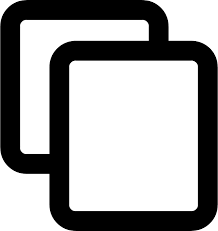
prepare("SELECT * FROM users WHERE email = :email");
// Bind the email parameter to the prepared statement
$stmt->bindParam(':email', $email);
// Set the email value and execute the statement
$email = 'user@example.com';
$stmt->execute();
// Fetch results
$results = $stmt->fetchAll(PDO::FETCH_ASSOC);
?>
Prepared Statements in MySQLi
Here’s an example of using prepared statements in MySQLi:
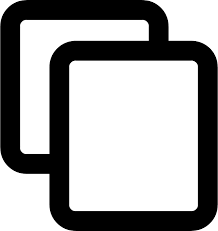
prepare("SELECT * FROM users WHERE email = ?");
// Bind the email parameter
$stmt->bind_param('s', $email);
// Set the email value and execute the statement
$email = 'user@example.com';
$stmt->execute();
// Bind result variables and fetch the results
$stmt->bind_result($id, $name, $email);
while ($stmt->fetch()) {
echo "ID: $id, Name: $name, Email: $email
";
}
?>
Choosing Between PDO and MySQLi
Both PDO and MySQLi are capable of handling MySQL database connections, but here are some key points to consider when choosing between them:
- Use PDO: If you want a more flexible solution that works with multiple database systems and provides better error handling.
- Use MySQLi: If you are working exclusively with MySQL and need features like multi-queries or stored procedures.
Conclusion
Connecting MySQL with PHP using PDO or MySQLi allows you to perform secure and efficient database operations. Both methods offer unique features, and the choice between them depends on your project requirements. For flexibility and database-agnostic solutions, PDO is the way to go. For MySQL-specific features, MySQLi is a great choice. Whichever method you choose, it’s important to use prepared statements to ensure the security of your application.
Using MySQL with Node.js (using mysql2 or sequelize)
Node.js is a powerful JavaScript runtime for building scalable network applications. When developing applications that require database interaction, MySQL is a popular relational database management system (RDBMS). In Node.js, you can connect to MySQL using libraries like mysql2
and sequelize
. This guide will walk you through how to use MySQL with Node.js using these two libraries.
Why Use MySQL with Node.js?
Node.js is widely used for developing web applications, and MySQL is a popular choice for managing relational data. By connecting MySQL with Node.js, you can:
- Perform CRUD (Create, Read, Update, Delete) operations on your MySQL database from your Node.js application.
- Execute complex queries and transactions to interact with data.
- Leverage asynchronous programming and promises in Node.js to handle database operations efficiently.
Using mysql2 with Node.js
mysql2
is a MySQL client for Node.js that provides a simple and efficient way to interact with MySQL databases. It supports both promises and callbacks.
Installing mysql2
To get started, you need to install the mysql2
package. You can install it using npm:
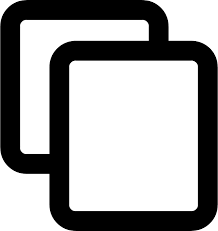
npm install mysql2
Connecting to MySQL with mysql2
Here’s how you can connect to a MySQL database using mysql2
:
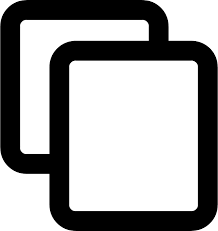
const mysql = require('mysql2');
// Create a connection to the database
const connection = mysql.createConnection({
host: 'localhost',
user: 'username',
password: 'password',
database: 'my_database'
});
// Connect to the database
connection.connect(err => {
if (err) {
console.error('error connecting: ' + err.stack);
return;
}
console.log('connected as id ' + connection.threadId);
});
// Query the database
connection.query('SELECT * FROM users', (err, results) => {
if (err) {
console.error(err);
return;
}
console.log(results);
});
// Close the connection
connection.end();
Using Promises with mysql2
mysql2
also supports promises, which allows you to use async/await syntax for database queries:
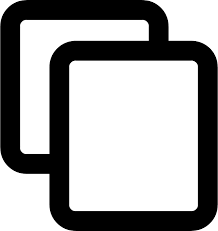
const mysql = require('mysql2/promise');
async function main() {
const connection = await mysql.createConnection({
host: 'localhost',
user: 'username',
password: 'password',
database: 'my_database'
});
const [rows, fields] = await connection.execute('SELECT * FROM users');
console.log(rows);
await connection.end();
}
main().catch(err => console.error(err));
Using Sequelize with Node.js
Sequelize
is an Object-Relational Mapper (ORM) for Node.js that provides a higher-level API for interacting with databases. It allows you to work with MySQL using JavaScript objects, making database operations more intuitive.
Installing Sequelize and mysql2
To use Sequelize with MySQL, you need to install both sequelize
and mysql2
:
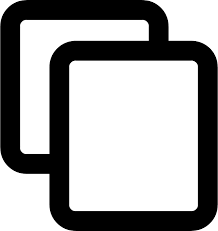
npm install sequelize mysql2
Setting Up Sequelize
Here’s how to set up Sequelize with MySQL in your Node.js project:
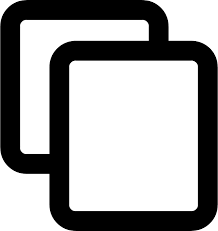
const { Sequelize, DataTypes } = require('sequelize');
// Create a Sequelize instance and connect to the database
const sequelize = new Sequelize('mysql://username:password@localhost/my_database');
// Define a model
const User = sequelize.define('User', {
name: {
type: DataTypes.STRING,
allowNull: false
},
email: {
type: DataTypes.STRING,
unique: true,
allowNull: false
}
});
// Sync the model with the database
sequelize.sync()
.then(() => console.log('Database synced'))
.catch(err => console.error('Error syncing database:', err));
Performing CRUD Operations with Sequelize
Here’s how to perform basic CRUD operations using Sequelize:
Create a new record:
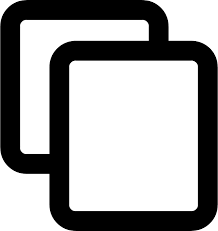
User.create({
name: 'John Doe',
email: 'john.doe@example.com'
})
.then(user => console.log('User created:', user))
.catch(err => console.error('Error creating user:', err));
Read records:
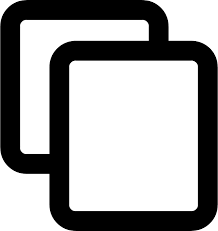
User.findAll()
.then(users => console.log('Users:', users))
.catch(err => console.error('Error fetching users:', err));
Update a record:
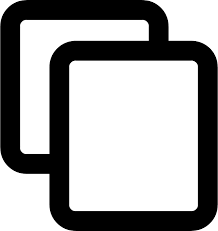
User.update({ name: 'Jane Doe' }, {
where: { email: 'john.doe@example.com' }
})
.then(() => console.log('User updated'))
.catch(err => console.error('Error updating user:', err));
Delete a record:
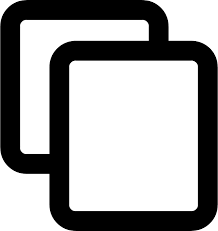
User.destroy({
where: { email: 'john.doe@example.com' }
})
.then(() => console.log('User deleted'))
.catch(err => console.error('Error deleting user:', err));
Choosing Between mysql2 and Sequelize
The choice between mysql2
and sequelize
depends on your needs:
- Use mysql2: If you prefer writing raw SQL queries and need a simple, lightweight solution for interacting with your MySQL database.
- Use Sequelize: If you prefer working with an ORM and want to interact with MySQL using JavaScript objects, which makes it easier to manage relationships and perform CRUD operations.
Conclusion
Both mysql2
and sequelize
are excellent choices for integrating MySQL with Node.js. If you need to execute raw SQL queries with promises or callbacks, mysql2
is a great option. On the other hand, if you prefer working with an ORM and want to simplify database operations, sequelize
is a powerful tool. Choose the one that best fits your project requirements and coding style.
Integrating MySQL with Python (using MySQL Connector or SQLAlchemy)
Python is a versatile programming language that is widely used for web development, data analysis, automation, and more. When working with MySQL databases, Python provides two popular libraries for database interaction: mysql-connector
and SQLAlchemy
. This guide will show you how to integrate MySQL with Python using these libraries.
Why Use MySQL with Python?
Integrating MySQL with Python allows you to easily interact with MySQL databases for performing tasks such as:
- Executing CRUD (Create, Read, Update, Delete) operations on MySQL data.
- Running complex queries and transactions to manipulate data.
- Building database-driven applications like web apps, data analysis tools, and more.
Using MySQL Connector with Python
mysql-connector-python
is an official MySQL library for Python, which provides easy-to-use methods for connecting to and interacting with MySQL databases.
Installing MySQL Connector
To install mysql-connector-python
, you can use the following pip command:
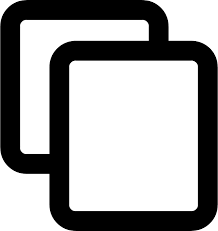
pip install mysql-connector-python
Connecting to MySQL with MySQL Connector
Here’s how you can connect to a MySQL database using mysql-connector-python
:
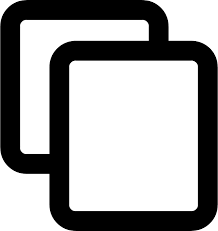
import mysql.connector
# Create a connection to the database
connection = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="my_database"
)
# Check if the connection was successful
if connection.is_connected():
print("Connected to MySQL database")
# Creating a cursor object to interact with the database
cursor = connection.cursor()
# Running a query
cursor.execute("SELECT * FROM users")
# Fetching the results
results = cursor.fetchall()
for row in results:
print(row)
# Closing the connection
connection.close()
Performing CRUD Operations with MySQL Connector
Below are examples of CRUD operations using MySQL Connector:
Create (Insert a new record):
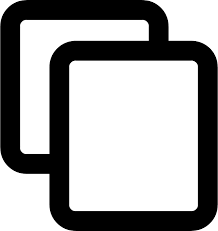
cursor.execute("INSERT INTO users (name, email) VALUES (%s, %s)", ('John Doe', 'john.doe@example.com'))
connection.commit() # Commit the transaction
Read (Select records):
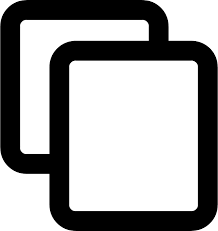
cursor.execute("SELECT * FROM users")
results = cursor.fetchall()
for row in results:
print(row)
Update (Update a record):
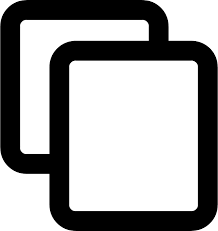
cursor.execute("UPDATE users SET name = %s WHERE email = %s", ('Jane Doe', 'john.doe@example.com'))
connection.commit()
Delete (Delete a record):
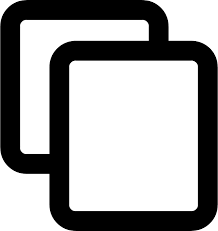
cursor.execute("DELETE FROM users WHERE email = %s", ('john.doe@example.com',))
connection.commit()
Using SQLAlchemy with Python
SQLAlchemy
is a popular Object-Relational Mapping (ORM) library for Python, which provides a higher-level API for interacting with relational databases like MySQL. It allows you to work with SQL databases using Python objects and provides more flexibility than raw SQL queries.
Installing SQLAlchemy and MySQL Driver
To use SQLAlchemy with MySQL, you need to install SQLAlchemy
and a MySQL driver such as mysqlclient
or PyMySQL
. You can install them using pip:
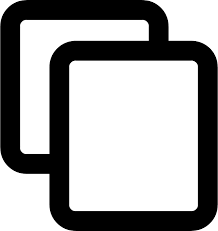
pip install sqlalchemy mysqlclient
Setting Up SQLAlchemy
Here’s how to set up SQLAlchemy to connect to a MySQL database:
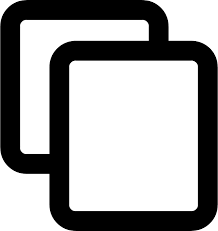
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
from sqlalchemy.orm import sessionmaker
# Create a connection to MySQL
engine = create_engine('mysql://username:password@localhost/my_database')
# Base class for class definitions
Base = declarative_base()
# Define a model for a table
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
# Create all tables defined by the Base class
Base.metadata.create_all(engine)
# Create a session
Session = sessionmaker(bind=engine)
session = Session()
Performing CRUD Operations with SQLAlchemy
Here’s how to perform basic CRUD operations using SQLAlchemy:
Create (Insert a new record):
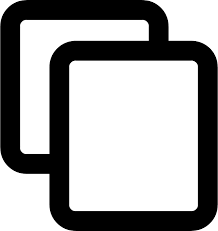
new_user = User(name='John Doe', email='john.doe@example.com')
session.add(new_user)
session.commit()
Read (Query records):
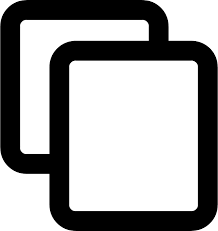
users = session.query(User).all()
for user in users:
print(user.name, user.email)
Update (Update a record):
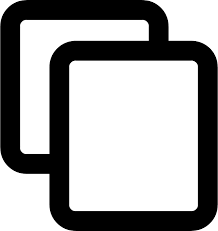
user = session.query(User).filter_by(email='john.doe@example.com').first()
user.name = 'Jane Doe'
session.commit()
Delete (Delete a record):
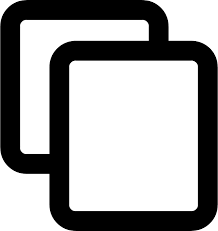
user = session.query(User).filter_by(email='john.doe@example.com').first()
session.delete(user)
session.commit()
Choosing Between MySQL Connector and SQLAlchemy
The choice between mysql-connector-python
and SQLAlchemy
depends on your project needs:
- Use MySQL Connector: If you prefer working with raw SQL queries and need a simple, straightforward solution for interacting with MySQL databases.
- Use SQLAlchemy: If you prefer an ORM approach and want to work with databases using Python objects, which can simplify complex database queries and relationships.
Conclusion
Both mysql-connector-python
and SQLAlchemy
are excellent choices for integrating MySQL with Python. If you need to write raw SQL queries and execute them efficiently, mysql-connector-python
is a great option. If you prefer working with Python objects and want an ORM-based approach, SQLAlchemy
provides a powerful and flexible solution. Choose the one that best fits your project’s requirements and your preferred development style.
ORM (Object-Relational Mapping) in MySQL
Object-Relational Mapping (ORM) is a programming technique that allows developers to interact with relational databases using object-oriented programming languages. In the context of MySQL, ORM provides a way to work with MySQL databases using objects rather than writing raw SQL queries. This improves productivity, reduces boilerplate code, and allows for a more abstracted and intuitive way of managing the database.
What is ORM?
ORM is a technique that maps database tables to programming language objects. This means that each row in a database table corresponds to an instance of a class, and each column corresponds to an attribute or property of that class. ORM frameworks allow developers to manipulate database records as objects, without having to deal with SQL queries directly.
Benefits of Using ORM
- Abstraction: ORM abstracts away the details of SQL, allowing developers to work with objects instead of writing complex SQL queries.
- Code Consistency: ORM provides a consistent way to interact with the database across different programming languages and platforms.
- Improved Productivity: ORM tools save time by automating repetitive tasks like query generation and result mapping.
- Database Independence: ORM makes it easier to switch databases since queries are abstracted away from the underlying database system.
Popular ORM Frameworks for MySQL
Several ORM frameworks are available for MySQL, providing developers with powerful tools for database interaction. Some of the most popular ORM libraries include:
- SQLAlchemy: A widely-used ORM for Python that supports multiple relational databases, including MySQL. SQLAlchemy allows developers to work with SQLAlchemy ORM objects instead of SQL queries.
- Sequelize: A promise-based ORM for Node.js that supports MySQL, PostgreSQL, and other databases. Sequelize provides an easy-to-use API for interacting with MySQL databases in a node.js environment.
- Doctrine: A powerful ORM framework for PHP that provides an abstraction layer for working with databases, including MySQL. Doctrine integrates with various PHP frameworks such as Symfony and Laravel.
- Hibernate: A Java-based ORM framework that provides a robust solution for database interaction, offering support for multiple databases, including MySQL.
How ORM Works with MySQL
Here’s how ORM typically works with MySQL:
- Define Entity Classes: For each table in the MySQL database, you define a corresponding class (entity). Each class attribute corresponds to a column in the table.
- Establish Relationships: Define relationships between entity classes, such as one-to-many, many-to-one, and many-to-many, to represent foreign key relationships between database tables.
- Perform CRUD Operations: ORM frameworks allow you to perform Create, Read, Update, and Delete operations on database records by interacting with entity objects, rather than writing raw SQL queries.
- Mapping SQL Queries: When you perform operations on objects, the ORM framework automatically generates the corresponding SQL queries in the background and executes them on the MySQL database.
Example: Using ORM in Python with SQLAlchemy
In this example, we will use SQLAlchemy (a Python ORM) to interact with a MySQL database. We will create a simple `User` class that corresponds to a `users` table in the MySQL database.
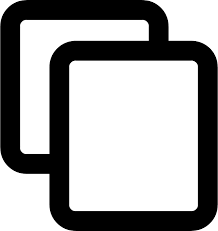
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Create an engine that connects to the MySQL database
engine = create_engine('mysql://username:password@localhost/my_database')
# Base class for our ORM classes
Base = declarative_base()
# Define a User class that represents the 'users' table
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
# Create the 'users' table in MySQL
Base.metadata.create_all(engine)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
# Adding a new user to the database
new_user = User(name='Alice', email='alice@example.com')
session.add(new_user)
session.commit()
# Querying the user from the database
user = session.query(User).filter_by(name='Alice').first()
print(f'User: {user.name}, Email: {user.email}')
# Closing the session
session.close()
In this example:
- We defined a `User` class that maps to the `users` table in MySQL.
- We created a new user and added it to the MySQL database using the ORM.
- We queried the `users` table to retrieve the user we just added.
Example: Using ORM in Node.js with Sequelize
Here’s an example of how you can use Sequelize (a Node.js ORM) to interact with a MySQL database.
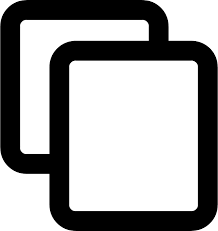
const { Sequelize, DataTypes } = require('sequelize');
// Create a Sequelize instance to connect to MySQL
const sequelize = new Sequelize('mysql://username:password@localhost/my_database');
// Define a User model that maps to the 'users' table
const User = sequelize.define('User', {
name: {
type: DataTypes.STRING,
allowNull: false
},
email: {
type: DataTypes.STRING,
allowNull: false
}
});
// Synchronize the model with the MySQL database
sequelize.sync();
// Create a new user
User.create({ name: 'Bob', email: 'bob@example.com' })
.then(() => {
console.log('User created successfully');
})
.catch(error => {
console.error('Error creating user:', error);
});
In this example:
- We defined a `User` model that corresponds to the `users` table in the MySQL database.
- We created a new user and saved it to the MySQL database using Sequelize.
Choosing an ORM Framework for MySQL
When selecting an ORM framework for MySQL, consider the following factors:
- Language Support: Choose an ORM that works with the programming language you're using (e.g., Python, Node.js, PHP, Java).
- Features: Look for features like relationship mapping (one-to-many, many-to-many), automatic query generation, and migrations support.
- Community and Documentation: Choose a framework that has a strong community and comprehensive documentation to help you troubleshoot issues.
- Performance: ORM frameworks can introduce overhead, so ensure the chosen ORM performs well for your use case, especially in high-traffic applications.
Conclusion
ORM provides an efficient way to interact with MySQL databases by mapping database tables to programming language objects. Using ORM frameworks like SQLAlchemy (Python), Sequelize (Node.js), and Doctrine (PHP) simplifies database operations and increases productivity. However, it’s important to choose an ORM that best fits your language, needs, and performance requirements.
Common MySQL Errors and How to Resolve Them
Working with MySQL can sometimes lead to errors during development, configuration, or execution. Below are some of the most common MySQL errors and how to resolve them.
1. Error 1045: Access Denied for User
Error Message: ERROR 1045 (28000): Access denied for user 'username'@'host' (using password: YES)
This error occurs when MySQL denies access to the specified user because of incorrect credentials or insufficient permissions.
Resolution:
- Check the username and password you are using to ensure they are correct.
- Verify that the user has the necessary privileges to connect from the host you're accessing MySQL from.
- You can reset the password by running the following command in MySQL:
- If you are running MySQL locally, you may want to check the MySQL user privileges with:
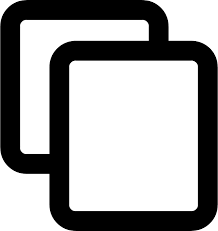
SET PASSWORD FOR 'username'@'host' = PASSWORD('new_password');
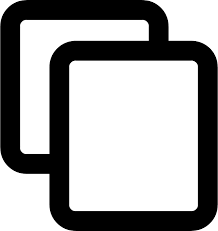
SHOW GRANTS FOR 'username'@'localhost';
2. Error 1049: Unknown Database
Error Message: ERROR 1049 (42000): Unknown database 'database_name'
This error occurs when MySQL cannot find the specified database. The database may not exist, or you may have misspelled its name.
Resolution:
- Ensure that the database name is correct.
- If the database does not exist, create it by running:
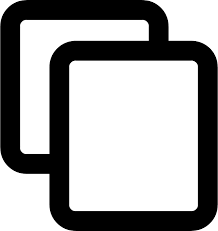
CREATE DATABASE database_name;
3. Error 2002: Can't Connect to MySQL Server
Error Message: ERROR 2002 (HY000): Can't connect to local MySQL server through socket '/tmp/mysql.sock' (2)
This error occurs when MySQL cannot connect to the server. It can happen if the MySQL service is not running or if there are socket file issues.
Resolution:
- Check if the MySQL server is running using:
- If MySQL isn't running, start the service with:
- If it's a socket issue, check the MySQL configuration file (typically
/etc/my.cnf
) for the correct socket path. - Ensure that you're using the correct hostname or IP address in case the server isn't running locally.
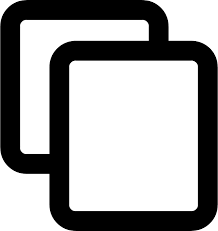
sudo systemctl status mysql
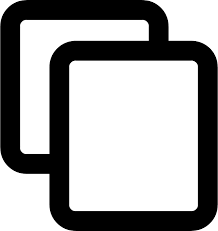
sudo systemctl start mysql
4. Error 1130: Host 'host' is not allowed to connect to this MySQL server
Error Message: ERROR 1130 (00000): Host 'host' is not allowed to connect to this MySQL server
This error occurs when MySQL does not allow the specified host to connect to the server. It could be due to host-based authentication or the user's privileges.
Resolution:
- Check MySQL user privileges using:
- Grant the necessary privileges to the user for the specific host with:
- Ensure that the MySQL server is configured to listen on all IP addresses if you are connecting remotely. Check the
bind-address
in the MySQL config file.
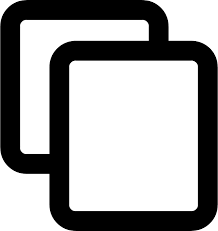
SHOW GRANTS FOR 'username'@'host';
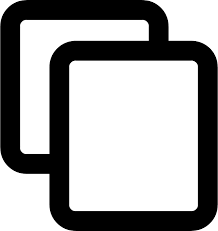
GRANT ALL PRIVILEGES ON database_name.* TO 'username'@'host';
5. Error 1064: Syntax Error
Error Message: ERROR 1064 (42000): You have an error in your SQL syntax
This error occurs when MySQL cannot understand your SQL query due to incorrect syntax.
Resolution:
- Check the SQL query for common mistakes such as missing or misplaced punctuation, incorrect keywords, or invalid table/column names.
- Ensure that any string literals are enclosed in quotes, and numerical values are appropriately formatted.
- Use MySQL's
EXPLAIN
command to analyze the query structure:
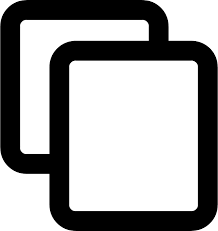
EXPLAIN SELECT * FROM table_name;
6. Error 1213: Deadlock Found When Trying to Get Lock
Error Message: ERROR 1213 (40001): Deadlock found when trying to get lock
This error occurs when two or more transactions are waiting for each other to release locks, creating a deadlock situation.
Resolution:
- Identify the queries involved in the deadlock using the
SHOW ENGINE INNODB STATUS
command. - Try to optimize the queries by reducing lock contention or making sure that transactions acquire locks in a consistent order.
- In some cases, you may need to adjust the transaction isolation level or use explicit locking mechanisms such as
SELECT ... FOR UPDATE
.
7. Error 1451: Cannot Delete or Update a Parent Row
Error Message: ERROR 1451 (23000): Cannot delete or update a parent row: a foreign key constraint fails
This error occurs when trying to delete or update a row that is referenced by a foreign key constraint in another table.
Resolution:
- Ensure that you are not trying to delete or update rows that are referenced by foreign keys in other tables.
- To resolve this, you can either delete the dependent rows first or modify the foreign key constraint to allow cascading updates or deletes. Example:
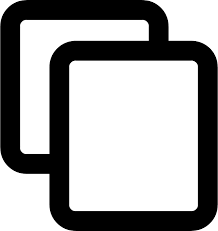
ALTER TABLE child_table ADD CONSTRAINT fk_name FOREIGN KEY (parent_id) REFERENCES parent_table(id) ON DELETE CASCADE;
8. Error 2006: MySQL Server Has Gone Away
Error Message: ERROR 2006 (HY000): MySQL server has gone away
This error occurs when the client loses connection to the MySQL server during an operation, usually due to a timeout or server restart.
Resolution:
- Check the server's
wait_timeout
andinteractive_timeout
settings. Increase these values if necessary. - Ensure that the server is not restarting during long queries or operations.
- If using a persistent connection, try reestablishing the connection before retrying the query.
9. Error 1062: Duplicate Entry for a Primary Key
Error Message: ERROR 1062 (23000): Duplicate entry 'value' for key 'PRIMARY'
This error occurs when attempting to insert a row with a primary key that already exists in the table.
Resolution:
- Check for duplicate data in the primary key column before attempting to insert.
- Use the
INSERT IGNORE
statement if you want to silently ignore duplicate entries: - Alternatively, use
ON DUPLICATE KEY UPDATE
to update the existing row if a duplicate key is found.
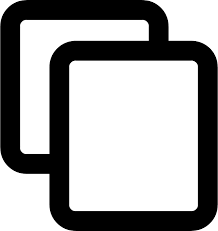
INSERT IGNORE INTO table_name (column1, column2) VALUES (value1, value2);
Conclusion
MySQL errors are common and can often be resolved by checking the error code and message, understanding the cause, and applying the appropriate solution. Regularly reviewing MySQL logs, ensuring correct syntax, and configuring your server properly can help you avoid many of these errors and improve the stability and performance of your database.
Analyzing and Interpreting MySQL Error Logs
MySQL error logs are an essential tool for identifying issues in your MySQL server. These logs provide detailed information about server events, including startup, shutdown, and errors. Understanding and interpreting the error logs can help you diagnose problems, optimize performance, and ensure the server's stability.
1. Locating MySQL Error Logs
MySQL logs are typically stored in the directory specified in the MySQL configuration file (my.cnf or my.ini). The error log file is usually named mysql_error.log
or similar.
You can find the location of the error log by running the following command:
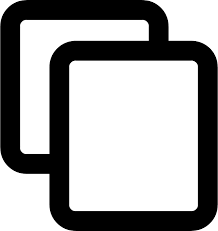
SHOW VARIABLES LIKE 'log_error';
This will return the path to the error log file. Common locations include:
/var/log/mysql/error.log
(Linux systems)C:\ProgramData\MySQL\MySQL Server X.Y\data\mysql_error.log
(Windows systems)
2. Interpreting MySQL Error Log Entries
Each entry in the MySQL error log typically contains a timestamp, the log level, and a message describing the event. Here are some common types of entries you'll find:
Startup and Shutdown Messages
When MySQL starts or stops, it logs important information about the process:
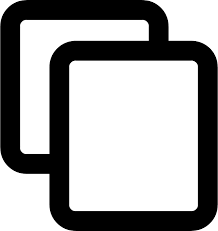
[Note] /usr/sbin/mysqld: ready for connections. Version: '5.7.25' socket: '/var/run/mysqld/mysqld.sock' port: 3306 MySQL Community Server (GPL)
These logs indicate the version of MySQL, the socket file used for connections, and the port number. A successful startup message usually includes "ready for connections."
Error Messages
If MySQL encounters an issue during startup, it logs an error message. For example:
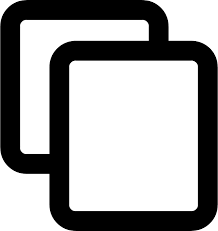
[ERROR] /usr/sbin/mysqld: Can't create/write to file '/tmp/ib3ABCDEF' (Errcode: 13 - Permission denied)
This error indicates a permissions issue where MySQL is trying to write to a file but does not have the necessary permissions. You can resolve this by adjusting the file permissions or changing the directory location in the configuration file.
Connection Errors
MySQL logs errors related to failed connection attempts:
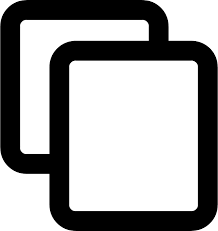
[ERROR] Host 'localhost' is not allowed to connect to this MySQL server
This error occurs when a client tries to connect to MySQL, but the host is not permitted to do so. You can fix this by granting the necessary privileges to the user or host:
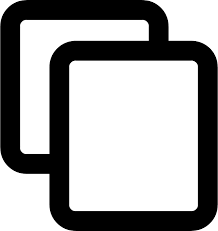
GRANT ALL PRIVILEGES ON *.* TO 'username'@'localhost';
Deadlock Errors
When MySQL detects a deadlock situation, it logs the error:
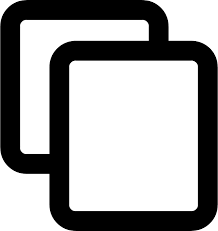
[ERROR] InnoDB: Deadlock found when trying to get lock; try restarting transaction
Deadlock errors occur when two or more transactions are waiting on each other to release locks. You can analyze the deadlock information in the log to determine which queries are involved and optimize them to reduce lock contention.
3. Using MySQL Error Logs for Troubleshooting
Analyzing MySQL error logs is essential for identifying and resolving issues. Here’s how to use the logs for troubleshooting:
Identifying Performance Issues
If MySQL is running slowly, check the error log for any unusual messages or warnings. Common performance-related issues include:
- Running out of disk space (e.g.,
[ERROR] InnoDB: Unable to write to file
) - Insufficient memory or buffer pool size
- Long-running queries or deadlocks
These messages can point to the root cause of performance issues, such as inadequate hardware resources, configuration settings, or inefficient queries.
Diagnosing Database Crashes
If MySQL crashes unexpectedly, the error log will provide valuable information about the crash. Look for the last few entries in the log before the crash, which may include:
- Stack traces indicating where the crash occurred
- Memory allocation errors
- InnoDB errors related to corrupt tables or indexes
In many cases, crash logs provide enough information to identify the cause of the crash, whether it's a corrupted table, a configuration issue, or resource exhaustion.
Monitoring for Security Issues
MySQL error logs also record security-related events, such as failed login attempts. Look for entries like:
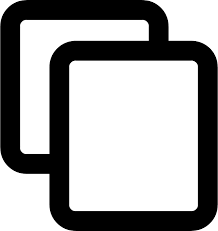
[Warning] Access denied for user 'root'@'localhost' (using password: YES)
These messages can help you detect unauthorized access attempts. If you notice repeated failed login attempts, investigate the source IP and take appropriate action, such as blocking the IP or strengthening your authentication policies.
4. Enabling and Configuring MySQL Error Logs
If MySQL error logging is not enabled, you can enable it by modifying the my.cnf
configuration file:
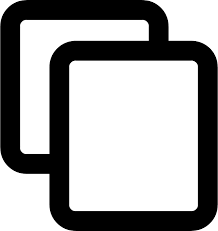
[mysqld]
log_error = /path/to/mysql_error.log
After making changes to the configuration file, restart MySQL for the changes to take effect:
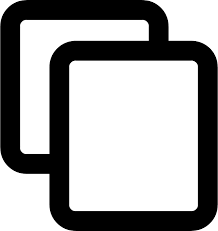
sudo systemctl restart mysql
You can also configure the log level to capture different types of events:
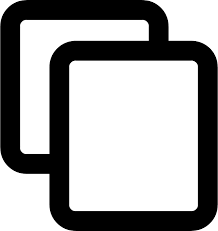
log_error_verbosity = 3
Levels range from 1 (minimal information) to 3 (maximum detail).
5. Best Practices for Managing MySQL Error Logs
To ensure efficient error log management and troubleshooting, follow these best practices:
- Regularly monitor error logs for signs of issues.
- Rotate error logs to prevent disk space exhaustion, especially on high-traffic servers.
- Use log management tools to centralize and analyze logs from multiple MySQL instances.
- Set up alerts for critical errors (e.g., server crashes, deadlocks, or connection issues).
Conclusion
MySQL error logs are an invaluable resource for diagnosing and troubleshooting issues with your MySQL server. By regularly reviewing and interpreting these logs, you can catch issues early, improve performance, and ensure the stability and security of your database server.
Optimizing Queries and Avoiding Locks
Query optimization is a critical task in MySQL to ensure fast response times, efficient resource usage, and overall system performance. One of the key challenges in query optimization is minimizing or avoiding database locks, which can lead to performance bottlenecks. This section outlines strategies to optimize queries and reduce locking issues in MySQL.
1. Understanding Database Locks
A database lock is a mechanism used to control access to a resource in a multi-user environment, ensuring data integrity. Locks can be classified into two main types:
- Shared Locks (Read Locks): Allow multiple transactions to read the same data but prevent data modification.
- Exclusive Locks (Write Locks): Prevent other transactions from reading or modifying the data until the transaction is complete.
While locks are necessary for maintaining data integrity, excessive or improper locking can significantly degrade performance by blocking other queries from executing.
2. Optimizing Queries to Reduce Lock Contention
Optimizing queries can help reduce locking and improve overall performance. Here are several strategies for query optimization:
2.1. Use Indexes Effectively
Indexes allow MySQL to quickly locate the data needed for a query, reducing the time spent on scanning entire tables and reducing the likelihood of table-level locks. Ensure the following:
- Create indexes on columns that are frequently used in
WHERE
,JOIN
,ORDER BY
, orGROUP BY
clauses. - Use
EXPLAIN
to analyze query execution plans and identify potential index issues.
2.2. Avoid Using SELECT *
Using SELECT *
retrieves all columns from a table, which may result in unnecessary data being fetched. Instead, specify only the necessary columns in your SELECT
statement:
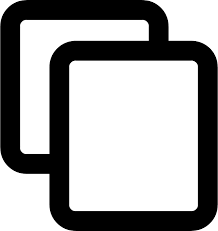
SELECT column1, column2 FROM table WHERE condition;
This reduces the amount of data processed and can help reduce locking, especially in large tables.
2.3. Optimize Join Queries
When performing JOIN
operations, ensure that:
- Indexes are used on the columns being joined to minimize scanning.
- Use
INNER JOIN
instead ofLEFT JOIN
when possible, as it typically leads to faster performance. - Be cautious with
CROSS JOIN
as it can result in a large number of rows being processed unnecessarily.
2.4. Break Long Transactions into Smaller Batches
Long transactions increase the chance of locking conflicts as they hold locks for a longer period. Break long-running transactions into smaller, manageable batches:
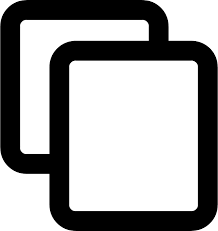
START TRANSACTION;
UPDATE table SET column=value WHERE condition LIMIT 1000;
COMMIT;
This helps reduce the lock duration, allowing other queries to execute in parallel.
2.5. Use LIMIT
to Restrict Data
When performing updates or deletes on large datasets, use the LIMIT
clause to restrict the number of rows affected in each operation:
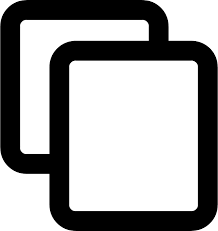
UPDATE table SET column=value WHERE condition LIMIT 1000;
This reduces the time locks are held and minimizes the impact on other transactions.
3. Avoiding Lock Contention
Lock contention occurs when multiple transactions try to access the same data simultaneously, leading to delays and potential deadlocks. To avoid lock contention:
3.1. Use READ COMMITTED
Isolation Level
The READ COMMITTED
isolation level allows a transaction to read only committed data, reducing the likelihood of locking conflicts. This is often a good balance between consistency and performance.
3.2. Optimize Transaction Timing
Keep transactions as short as possible to minimize lock contention. Start the transaction as late as possible and commit it as soon as the necessary operations are complete.
3.3. Implement NOWAIT
or SKIP LOCKED
MySQL 8.0+ supports NOWAIT
and SKIP LOCKED
options to avoid blocking when a row is already locked by another transaction:
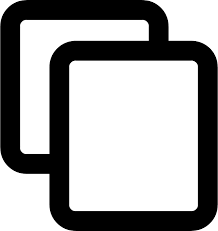
SELECT * FROM table WHERE condition FOR UPDATE NOWAIT;
These options can help prevent a query from waiting indefinitely for a lock to be released.
3.4. Use ROW-LEVEL
Locks Instead of TABLE-LEVEL
Locks
Whenever possible, use ROW-LEVEL
locking (enabled by default with InnoDB) instead of TABLE-LEVEL
locking. Row-level locks allow multiple transactions to access different rows in the same table simultaneously, reducing contention.
4. Deadlock Detection and Prevention
Deadlocks occur when two or more transactions hold locks that the other transactions need, resulting in an infinite wait. MySQL automatically detects and resolves deadlocks, but you can take steps to prevent them:
4.1. Analyze Deadlock Information
When a deadlock occurs, MySQL logs detailed information about it in the error log. Use this information to identify the transactions and queries involved:
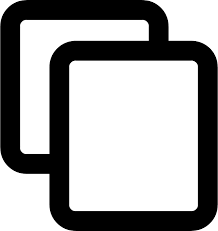
SHOW ENGINE INNODB STATUS;
Examine the TRANSACTIONS
section in the output for deadlock details.
4.2. Design Transactions to Avoid Deadlocks
To prevent deadlocks, ensure that:
- Transactions acquire locks in a consistent order to avoid circular dependencies.
- Keep transactions short and use
LOCK IN SHARE MODE
where appropriate. - Use
SELECT ... FOR UPDATE
judiciously to lock rows only when necessary.
5. Using EXPLAIN for Query Optimization
The EXPLAIN
statement provides detailed information about how MySQL executes a query. Use it to identify inefficiencies in your queries and optimize them:
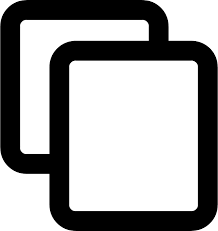
EXPLAIN SELECT * FROM table WHERE condition;
The EXPLAIN
output can show whether indexes are being used, which join algorithms are used, and whether table scans are occurring. Based on this information, you can make adjustments to improve query performance.
6. Conclusion
Optimizing queries and avoiding locks are essential for maintaining high performance and reducing contention in MySQL. By following best practices for query optimization, using proper indexing, and managing transactions effectively, you can minimize locking issues and improve the overall efficiency of your MySQL server.
Handling Crashes and Data Corruption
Crashes and data corruption are serious concerns for MySQL databases, as they can lead to data loss and degraded system performance. Understanding how to handle crashes and recover from data corruption is vital to maintaining the integrity and reliability of your database. This section covers strategies for handling crashes and preventing or mitigating data corruption in MySQL.
1. Causes of Crashes and Data Corruption
Crashes and data corruption can result from a variety of factors, including hardware failures, software bugs, improper shutdowns, or disk space issues. Common causes include:
- Hardware Failures: Issues with the server’s disk, memory, or CPU can lead to MySQL crashes or corruption.
- Improper Shutdown: Abrupt shutdowns or power failures can leave MySQL in an inconsistent state, potentially causing corruption.
- Disk Space Issues: Running out of disk space can cause MySQL to crash or corrupt data files.
- Software Bugs: Bugs in MySQL or third-party software interacting with MySQL can lead to crashes or corruption.
- Table Corruption: Corruption in InnoDB or MyISAM storage engines, often due to hardware or disk issues, can lead to data corruption.
2. Preventing Crashes and Data Corruption
While crashes and data corruption cannot always be fully prevented, there are several best practices to reduce the risk of occurrence:
2.1. Regular Backups
The most effective way to recover from data corruption or crashes is by having regular, reliable backups. Use tools like mysqldump
or mysqlpump
to create backups on a regular basis. Store backups off-site or in the cloud to protect against hardware failures.
2.2. Use InnoDB Storage Engine
InnoDB is a transactional storage engine with ACID properties that helps maintain data consistency even in the event of crashes. It has built-in crash recovery mechanisms that can automatically roll back incomplete transactions and repair minor corruption.
2.3. Enable Binary Logging
Enable binary logging in MySQL to track all changes to the database. Binary logs can help you recover from crashes by replaying changes made to the database after the last backup:
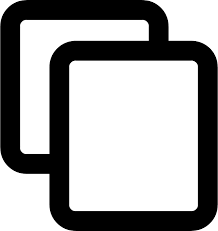
--log-bin=mysql-bin
Ensure that binary logs are archived regularly to prevent them from consuming too much disk space.
2.4. Use RAID for Disk Redundancy
Implement RAID (Redundant Array of Independent Disks) configurations to ensure redundancy in your storage subsystem. RAID 1 (mirroring) or RAID 5 (striping with parity) are popular choices for MySQL databases, as they help prevent data loss in the event of a disk failure.
2.5. Monitor Disk Space and Hardware Health
Regularly monitor disk space, hardware health, and system logs. Disk space issues or failing hardware can cause MySQL to crash or corrupt data. Set up alerts to notify administrators when disk space is running low, or hardware issues arise.
3. Detecting and Diagnosing Crashes
When MySQL crashes, it is important to detect the issue quickly and diagnose the underlying cause. MySQL provides logs and diagnostic tools that can help troubleshoot the problem:
3.1. MySQL Error Log
The MySQL error log provides information about the server startup, shutdown, and errors that occur during operation. It is the first place to check when diagnosing a crash:
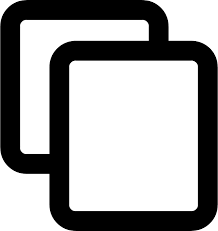
tail -f /var/log/mysql/error.log
The error log may provide information about the crash, such as the specific query or transaction that caused the issue.
3.2. InnoDB Crash Recovery
InnoDB has a built-in crash recovery mechanism that attempts to recover from crashes by rolling back incomplete transactions. In some cases, InnoDB may not be able to recover automatically, and manual intervention is required.
To check for corruption in InnoDB tables, you can use the following command:
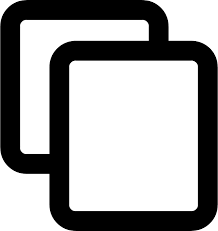
CHECK TABLE table_name;
3.3. Core Dumps
In the event of a crash, MySQL may generate a core dump file that contains detailed information about the crash. You can use tools like gdb
to analyze the core dump and identify the root cause of the issue.
4. Recovering from Crashes and Data Corruption
There are several methods to recover from crashes and data corruption, depending on the severity of the issue:
4.1. Crash Recovery with InnoDB
InnoDB automatically performs crash recovery when the server is restarted after a crash. It checks the logs and undoes any incomplete transactions. If the recovery is successful, the database will be restored to a consistent state.
4.2. Using Binary Logs for Point-in-Time Recovery
If you have binary logs enabled, you can perform point-in-time recovery by restoring the latest backup and applying the binary logs from the point of the crash:
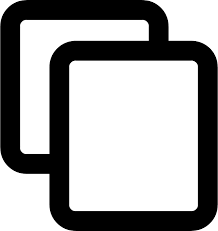
mysqlbinlog /path/to/binlog | mysql -u root -p
This will replay all changes made after the last backup, restoring the database to the point of failure.
4.3. Repairing Corrupted Tables
If a table is corrupted, you can attempt to repair it using the REPAIR TABLE
command for MyISAM tables. For InnoDB tables, you may need to restore from a backup if the corruption is severe:
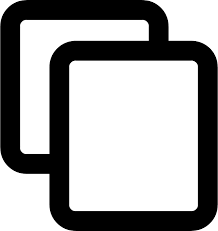
REPAIR TABLE table_name;
For InnoDB, you can try using the innodb_force_recovery
option to start the server and recover as much data as possible:
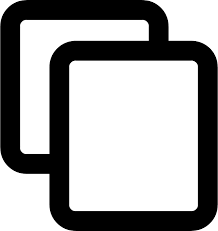
innodb_force_recovery = 4
However, be cautious with this option, as it can cause data loss if not handled correctly.
4.4. Using MySQL Utilities for Recovery
MySQL provides utilities like mysqlcheck
and myisamchk
for repairing and checking tables:
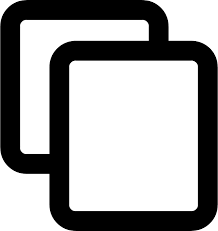
mysqlcheck -u root -p --auto-repair --all-databases
These utilities can help identify and repair corruption issues in MySQL databases.
5. Conclusion
While crashes and data corruption are unavoidable in some cases, implementing preventive measures such as regular backups, proper hardware management, and using InnoDB for transactional consistency can minimize their impact. Being able to diagnose and recover from crashes effectively is vital for maintaining the integrity and availability of your MySQL database.
Building a Blog Application with MySQL
Building a blog application is a great way to get hands-on experience with MySQL and web development. In this section, we will walk through the process of creating a blog application that allows users to create, read, update, and delete blog posts (CRUD operations) using MySQL as the backend database.
1. Planning the Blog Application
Before diving into coding, it’s important to plan the structure of your blog application and the database schema. Here are some essential features of a typical blog application:
- User Authentication: Allow users to sign up, log in, and manage their accounts.
- CRUD Operations: Users should be able to create, read, update, and delete blog posts.
- Categories: Blog posts can be categorized for easier navigation.
- Comments: Users can comment on blog posts.
- Admin Panel: Admin users should be able to manage posts, users, and comments.
2. Designing the Database Schema
We will need a few key tables to manage users, posts, categories, and comments. Here’s a simple database schema:
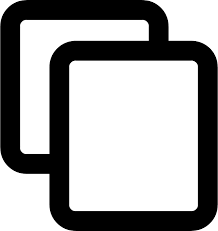
CREATE DATABASE blog_app;
USE blog_app;
-- Users Table
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(100) NOT NULL UNIQUE,
email VARCHAR(100) NOT NULL UNIQUE,
password VARCHAR(255) NOT NULL,
role ENUM('user', 'admin') DEFAULT 'user',
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Categories Table
CREATE TABLE categories (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL UNIQUE
);
-- Posts Table
CREATE TABLE posts (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
content TEXT NOT NULL,
category_id INT,
user_id INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (category_id) REFERENCES categories(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
-- Comments Table
CREATE TABLE comments (
id INT AUTO_INCREMENT PRIMARY KEY,
content TEXT NOT NULL,
post_id INT,
user_id INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (post_id) REFERENCES posts(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
The schema consists of the following tables:
- Users: Stores user details such as username, email, password, and role (user or admin).
- Categories: Stores the different categories for the blog posts.
- Posts: Stores the actual blog posts with title, content, category, and user who created the post.
- Comments: Stores comments on blog posts, linked to both the post and the user who commented.
3. Setting Up the Backend
The backend will handle requests to create, read, update, and delete blog posts, comments, and other features. We'll use a server-side language like Node.js with an ORM like Sequelize or a query builder like mysql2.
3.1. Installing Dependencies
First, initialize your Node.js project and install the necessary dependencies:
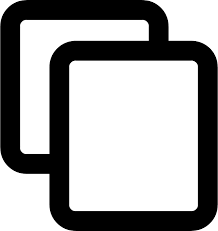
npm init -y
npm install express mysql2 sequelize body-parser
3.2. Connecting to MySQL Database
Set up the connection to MySQL using the mysql2 or Sequelize library:
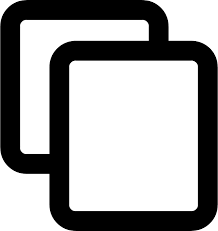
const mysql = require('mysql2');
// MySQL connection configuration
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'your_password',
database: 'blog_app'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL database!');
});
3.3. Implementing CRUD Operations for Posts
Here’s an example of how you can implement CRUD operations for blog posts:
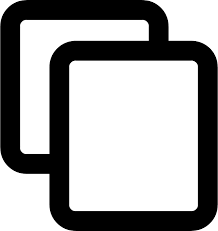
// Create a new post
app.post('/posts', (req, res) => {
const { title, content, category_id, user_id } = req.body;
const query = 'INSERT INTO posts (title, content, category_id, user_id) VALUES (?, ?, ?, ?)';
connection.query(query, [title, content, category_id, user_id], (err, result) => {
if (err) throw err;
res.send('Post created successfully!');
});
});
// Read all posts
app.get('/posts', (req, res) => {
const query = 'SELECT * FROM posts';
connection.query(query, (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Update a post
app.put('/posts/:id', (req, res) => {
const { title, content, category_id } = req.body;
const query = 'UPDATE posts SET title = ?, content = ?, category_id = ? WHERE id = ?';
connection.query(query, [title, content, category_id, req.params.id], (err, result) => {
if (err) throw err;
res.send('Post updated successfully!');
});
});
// Delete a post
app.delete('/posts/:id', (req, res) => {
const query = 'DELETE FROM posts WHERE id = ?';
connection.query(query, [req.params.id], (err, result) => {
if (err) throw err;
res.send('Post deleted successfully!');
});
});
4. Frontend for the Blog Application
The frontend can be built using any JavaScript framework like React, Vue, or Angular, or even a simple HTML/CSS setup. The frontend will interact with the backend via RESTful APIs to fetch, display, and manipulate the blog data.
4.1. Displaying Posts on the Frontend
In your frontend, you can fetch the blog posts from the backend API and display them to users:
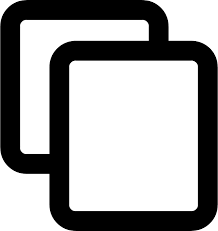
fetch('/posts')
.then((response) => response.json())
.then((data) => {
console.log(data); // Display posts on the page
});
4.2. Creating a New Post
To allow users to create posts, you can create a form that sends a POST request to the backend:
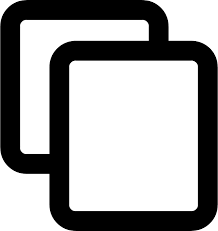
const createPost = (title, content, category_id, user_id) => {
fetch('/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ title, content, category_id, user_id })
})
.then(response => response.json())
.then(data => console.log(data));
};
5. Conclusion
By following the steps outlined in this section, you can create a full-fledged blog application powered by MySQL. The combination of MySQL for data storage and a backend framework like Node.js allows you to build a robust and scalable blog platform. You can enhance this basic application with more features like user authentication, commenting, and tagging to meet specific requirements.
Creating an Inventory Management System
An inventory management system helps businesses track stock levels, orders, sales, and deliveries. In this section, we’ll walk through the process of building a simple inventory management system with MySQL as the backend database.
1. Planning the Inventory Management System
Before developing the system, it’s essential to define the core features. Here are some key functionalities for an inventory management system:
- Product Management: Add, update, delete, and view products in the inventory.
- Stock Tracking: Track product quantities and manage stock levels.
- Sales Transactions: Record sales and update stock accordingly.
- Suppliers Management: Manage supplier details and link them to products.
- Reports: Generate reports for stock levels, sales, and transactions.
2. Designing the Database Schema
We’ll need a few key tables to store information about products, suppliers, sales transactions, and inventory adjustments. Here’s a simple database schema:
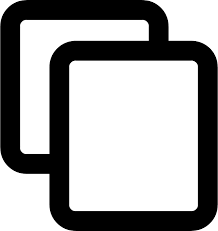
CREATE DATABASE inventory_system;
USE inventory_system;
-- Products Table
CREATE TABLE products (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
description TEXT,
price DECIMAL(10, 2) NOT NULL,
quantity INT NOT NULL DEFAULT 0,
supplier_id INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (supplier_id) REFERENCES suppliers(id)
);
-- Suppliers Table
CREATE TABLE suppliers (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
contact_name VARCHAR(255),
contact_email VARCHAR(255),
contact_phone VARCHAR(50)
);
-- Sales Table
CREATE TABLE sales (
id INT AUTO_INCREMENT PRIMARY KEY,
product_id INT,
quantity INT NOT NULL,
sale_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
total DECIMAL(10, 2) NOT NULL,
FOREIGN KEY (product_id) REFERENCES products(id)
);
-- Inventory Adjustments Table
CREATE TABLE inventory_adjustments (
id INT AUTO_INCREMENT PRIMARY KEY,
product_id INT,
quantity_adjusted INT NOT NULL,
adjustment_type ENUM('increase', 'decrease') NOT NULL,
adjustment_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (product_id) REFERENCES products(id)
);
The schema includes four essential tables:
- Products: Stores information about the products, including name, description, price, quantity, and the supplier.
- Suppliers: Stores supplier details like name, contact information, and supplier relationships with products.
- Sales: Records the sales transactions, linking them to the products sold and tracking quantities and total sale amounts.
- Inventory Adjustments: Tracks any changes in inventory, such as stock increases or decreases.
3. Setting Up the Backend
The backend will handle requests to add, update, view, and delete products, track sales transactions, and adjust inventory. We’ll use Node.js with mysql2 to interact with the MySQL database.
3.1. Installing Dependencies
Start by initializing your Node.js project and installing the necessary dependencies:
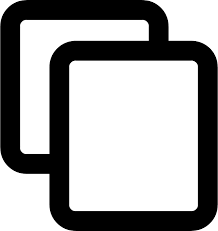
npm init -y
npm install express mysql2 body-parser
3.2. Connecting to MySQL Database
Set up the MySQL connection in your application using the mysql2 library:
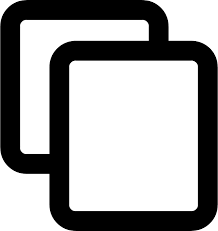
const mysql = require('mysql2');
// MySQL connection configuration
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'your_password',
database: 'inventory_system'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL database!');
});
3.3. Implementing CRUD Operations for Products
Here’s how you can implement CRUD operations for managing products:
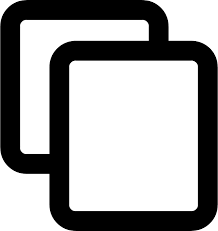
// Create a new product
app.post('/products', (req, res) => {
const { name, description, price, quantity, supplier_id } = req.body;
const query = 'INSERT INTO products (name, description, price, quantity, supplier_id) VALUES (?, ?, ?, ?, ?)';
connection.query(query, [name, description, price, quantity, supplier_id], (err, result) => {
if (err) throw err;
res.send('Product created successfully!');
});
});
// Read all products
app.get('/products', (req, res) => {
const query = 'SELECT * FROM products';
connection.query(query, (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Update a product
app.put('/products/:id', (req, res) => {
const { name, description, price, quantity, supplier_id } = req.body;
const query = 'UPDATE products SET name = ?, description = ?, price = ?, quantity = ?, supplier_id = ? WHERE id = ?';
connection.query(query, [name, description, price, quantity, supplier_id, req.params.id], (err, result) => {
if (err) throw err;
res.send('Product updated successfully!');
});
});
// Delete a product
app.delete('/products/:id', (req, res) => {
const query = 'DELETE FROM products WHERE id = ?';
connection.query(query, [req.params.id], (err, result) => {
if (err) throw err;
res.send('Product deleted successfully!');
});
});
4. Managing Sales Transactions
When a product is sold, you need to update the stock and record the sale. Here’s an example of how to implement sales transactions:
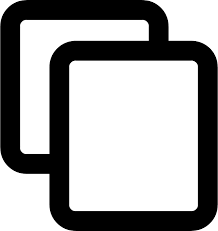
// Record a sale
app.post('/sales', (req, res) => {
const { product_id, quantity } = req.body;
// Fetch product price
connection.query('SELECT price, quantity FROM products WHERE id = ?', [product_id], (err, result) => {
if (err) throw err;
const product = result[0];
const total = product.price * quantity;
// Update product quantity
connection.query('UPDATE products SET quantity = ? WHERE id = ?', [product.quantity - quantity, product_id], (err, result) => {
if (err) throw err;
// Record sale transaction
const query = 'INSERT INTO sales (product_id, quantity, total) VALUES (?, ?, ?)';
connection.query(query, [product_id, quantity, total], (err, result) => {
if (err) throw err;
res.send('Sale recorded successfully!');
});
});
});
});
5. Frontend for the Inventory Management System
The frontend will allow users to interact with the system, such as adding products, viewing inventory, and recording sales. You can use any frontend framework or plain HTML/CSS with JavaScript for handling API requests.
5.1. Displaying Products
To display products on the frontend, you can fetch the product list from the backend:
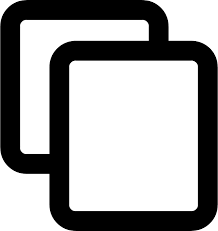
fetch('/products')
.then(response => response.json())
.then(data => {
console.log(data); // Display products on the page
});
5.2. Adding a New Product
To allow users to add products, create a form that sends a POST request to the backend:
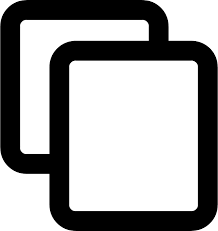
const createProduct = (name, description, price, quantity, supplier_id) => {
fetch('/products', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name, description, price, quantity, supplier_id })
})
.then(response => response.json())
.then(data => console.log(data));
};
6. Conclusion
By following these steps, you can create a basic inventory management system that tracks products, sales, and inventory adjustments. This system can be extended to include more complex features like automated stock alerts, supplier management, and detailed reporting. You can also integrate user roles (admin, staff) to control access and security in your system.
Building a Simple E-Commerce Website with MySQL Backend
In this section, we'll walk through the process of building a simple e-commerce website with a MySQL database as the backend. The website will include features such as product management, user authentication, shopping cart functionality, and order processing.
1. Planning the E-Commerce Website
Before we start developing, we need to define the core features for the e-commerce site. Here are the key components:
- User Authentication: Users can register, log in, and manage their account.
- Product Management: Admin can add, update, and delete products.
- Shopping Cart: Users can add products to the cart and proceed to checkout.
- Order Processing: Admin can view orders and update their status.
- Payment Integration (optional): Integrate payment gateways like PayPal or Stripe for processing payments.
2. Designing the Database Schema
We need to design a database to store user data, products, shopping cart details, and orders. Here's an example of how the database schema might look:
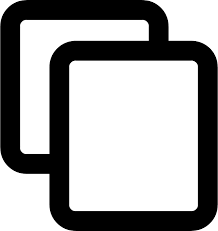
CREATE DATABASE ecommerce;
USE ecommerce;
-- Users Table
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) UNIQUE NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Products Table
CREATE TABLE products (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
description TEXT,
price DECIMAL(10, 2) NOT NULL,
quantity INT NOT NULL DEFAULT 0,
image_url VARCHAR(255),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Cart Items Table
CREATE TABLE cart_items (
id INT AUTO_INCREMENT PRIMARY KEY,
user_id INT,
product_id INT,
quantity INT NOT NULL,
added_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (product_id) REFERENCES products(id)
);
-- Orders Table
CREATE TABLE orders (
id INT AUTO_INCREMENT PRIMARY KEY,
user_id INT,
total_amount DECIMAL(10, 2) NOT NULL,
order_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
status ENUM('pending', 'shipped', 'delivered', 'cancelled') DEFAULT 'pending',
FOREIGN KEY (user_id) REFERENCES users(id)
);
-- Order Items Table
CREATE TABLE order_items (
id INT AUTO_INCREMENT PRIMARY KEY,
order_id INT,
product_id INT,
quantity INT NOT NULL,
price DECIMAL(10, 2) NOT NULL,
FOREIGN KEY (order_id) REFERENCES orders(id),
FOREIGN KEY (product_id) REFERENCES products(id)
);
The schema includes five main tables:
- Users: Stores user information like username, email, and password.
- Products: Stores information about products such as name, description, price, and quantity.
- Cart Items: Tracks the items in a user's shopping cart.
- Orders: Stores order details, including the user ID, total amount, and order status.
- Order Items: Stores the details of the products associated with an order.
3. Setting Up the Backend
We will use Node.js as the backend to serve API endpoints for managing users, products, shopping carts, and orders. We'll use the mysql2 package for MySQL database interaction.
3.1. Installing Dependencies
Start by setting up a Node.js project and installing the required dependencies:
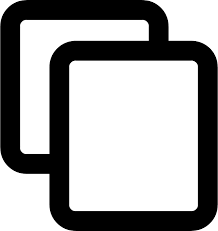
npm init -y
npm install express mysql2 body-parser bcryptjs jsonwebtoken
3.2. Connecting to MySQL Database
Next, set up the MySQL connection in your Node.js application:
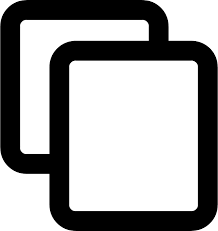
const mysql = require('mysql2');
// MySQL connection configuration
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'your_password',
database: 'ecommerce'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL database!');
});
3.3. User Authentication
To allow users to register and log in, we will create API endpoints for user registration and authentication using JWT (JSON Web Token) for session management:
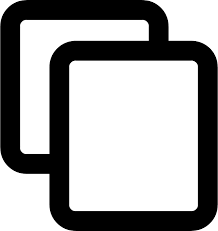
// User registration
app.post('/register', (req, res) => {
const { username, email, password } = req.body;
const hashedPassword = bcrypt.hashSync(password, 10);
const query = 'INSERT INTO users (username, email, password) VALUES (?, ?, ?)';
connection.query(query, [username, email, hashedPassword], (err, result) => {
if (err) throw err;
res.send('User registered successfully!');
});
});
// User login
app.post('/login', (req, res) => {
const { email, password } = req.body;
const query = 'SELECT * FROM users WHERE email = ?';
connection.query(query, [email], (err, results) => {
if (err) throw err;
const user = results[0];
if (user && bcrypt.compareSync(password, user.password)) {
const token = jwt.sign({ id: user.id }, 'secretkey', { expiresIn: '1h' });
res.json({ token });
} else {
res.status(400).send('Invalid credentials');
}
});
});
4. Implementing Product Management
Admins should be able to add, update, and delete products. Here's an example of how to implement product management:
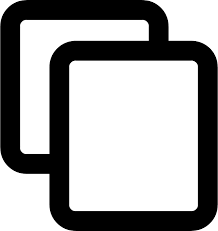
// Add a product
app.post('/products', (req, res) => {
const { name, description, price, quantity, image_url } = req.body;
const query = 'INSERT INTO products (name, description, price, quantity, image_url) VALUES (?, ?, ?, ?, ?)';
connection.query(query, [name, description, price, quantity, image_url], (err, result) => {
if (err) throw err;
res.send('Product added successfully!');
});
});
// Get all products
app.get('/products', (req, res) => {
const query = 'SELECT * FROM products';
connection.query(query, (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Update a product
app.put('/products/:id', (req, res) => {
const { name, description, price, quantity, image_url } = req.body;
const query = 'UPDATE products SET name = ?, description = ?, price = ?, quantity = ?, image_url = ? WHERE id = ?';
connection.query(query, [name, description, price, quantity, image_url, req.params.id], (err, result) => {
if (err) throw err;
res.send('Product updated successfully!');
});
});
// Delete a product
app.delete('/products/:id', (req, res) => {
const query = 'DELETE FROM products WHERE id = ?';
connection.query(query, [req.params.id], (err, result) => {
if (err) throw err;
res.send('Product deleted successfully!');
});
});
5. Implementing Shopping Cart and Order Processing
We will need endpoints to add items to the cart, view the cart, and process orders:
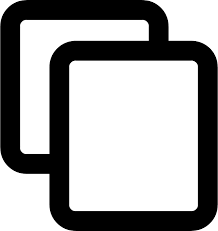
// Add to cart
app.post('/cart', (req, res) => {
const { user_id, product_id, quantity } = req.body;
const query = 'INSERT INTO cart_items (user_id, product_id, quantity) VALUES (?, ?, ?)';
connection.query(query, [user_id, product_id, quantity], (err, result) => {
if (err) throw err;
res.send('Product added to cart!');
});
});
// View cart
app.get('/cart/:user_id', (req, res) => {
const query = 'SELECT * FROM cart_items WHERE user_id = ?';
connection.query(query, [req.params.user_id], (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Place order
app.post('/order', (req, res) => {
const { user_id, total_amount } = req.body;
const query = 'INSERT INTO orders (user_id, total_amount) VALUES (?, ?)';
connection.query(query, [user_id, total_amount], (err, result) => {
if (err) throw err;
res.send('Order placed successfully!');
});
});
6. Frontend Implementation
The frontend can be built using HTML, CSS, and JavaScript. You can use frameworks like React, Angular, or Vue to build a dynamic user interface that interacts with your backend API. Use the RESTful API endpoints to handle user registration, login, product display, shopping cart management, and order processing.
7. Conclusion
With the above steps, we have built the foundation for a simple e-commerce website with a MySQL backend. You can extend this website by adding payment gateways, improving security, adding a recommendation engine, and more.
Implementing a Task Management System Using MySQL
In this section, we will build a simple task management system that allows users to create, update, delete, and manage tasks. The system will have features for task categorization, user assignment, task statuses, and deadlines.
1. Planning the Task Management System
Before we begin, we need to identify the key features and functionality of the system:
- User Authentication: Users can sign up, log in, and manage their tasks.
- Task Management: Users can create, update, delete, and view tasks.
- Task Categories: Tasks can be categorized into different types (e.g., Work, Personal, etc.).
- Task Status: Tasks can have different statuses (e.g., Pending, In Progress, Completed).
- Deadlines: Tasks can have deadlines and due dates.
2. Designing the Database Schema
We need to design a database to store users, tasks, task categories, and task statuses. Below is an example of how the schema might look:
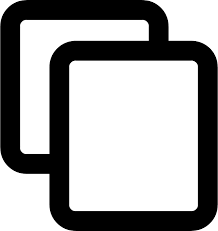
CREATE DATABASE task_manager;
USE task_manager;
-- Users Table
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) UNIQUE NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Task Categories Table
CREATE TABLE task_categories (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL
);
-- Task Status Table
CREATE TABLE task_statuses (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL
);
-- Tasks Table
CREATE TABLE tasks (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
description TEXT,
user_id INT,
category_id INT,
status_id INT,
deadline DATE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (category_id) REFERENCES task_categories(id),
FOREIGN KEY (status_id) REFERENCES task_statuses(id)
);
The schema includes the following tables:
- Users: Stores user information such as username, email, and password.
- Task Categories: Stores different categories for tasks (e.g., Work, Personal).
- Task Statuses: Stores various task statuses (e.g., Pending, In Progress, Completed).
- Tasks: Stores the task details, including the title, description, assigned user, category, status, and deadline.
3. Setting Up the Backend
We will use Node.js and Express for the backend to handle API requests for task management, including user authentication and task CRUD operations. We will use the mysql2 package to interact with the MySQL database.
3.1. Installing Dependencies
Start by setting up a Node.js project and installing the required dependencies:
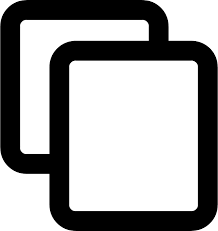
npm init -y
npm install express mysql2 bcryptjs jsonwebtoken body-parser
3.2. Connecting to MySQL
Next, set up the MySQL connection in your Node.js application:
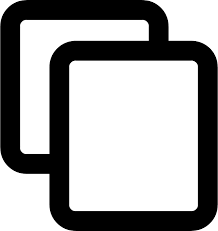
const mysql = require('mysql2');
// MySQL connection configuration
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'your_password',
database: 'task_manager'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL database!');
});
3.3. User Authentication
To allow users to register and log in, we will create API endpoints for user authentication using JWT (JSON Web Token) for session management:
// User registration
app.post('/register', (req, res) => {
const { username, email, password } = req.body;
const hashedPassword = bcrypt.hashSync(password, 10);
const query = 'INSERT INTO users (username, email, password) VALUES (?, ?, ?)';
connection.query(query, [username, email, hashedPassword], (err, result) => {
if (err) throw err;
res.send('User registered successfully!');
});
});
// User login
app.post('/login', (req, res) => {
const { email, password } = req.body;
const query = 'SELECT * FROM users WHERE email = ?';
connection.query(query, [email], (err, results) => {
if (err) throw err;
const user = results[0];
if (user && bcrypt.compareSync(password, user.password)) {
const token = jwt.sign({ id: user.id }, 'secretkey', { expiresIn: '1h' });
res.json({ token });
} else {
res.status(400).send('Invalid credentials');
}
});
});
4. Task Management API
We will now create the endpoints for managing tasks, including creating, updating, deleting, and viewing tasks:
// Create a new task
app.post('/tasks', (req, res) => {
const { title, description, user_id, category_id, status_id, deadline } = req.body;
const query = 'INSERT INTO tasks (title, description, user_id, category_id, status_id, deadline) VALUES (?, ?, ?, ?, ?, ?)';
connection.query(query, [title, description, user_id, category_id, status_id, deadline], (err, result) => {
if (err) throw err;
res.send('Task created successfully!');
});
});
// Get all tasks
app.get('/tasks', (req, res) => {
const query = 'SELECT * FROM tasks';
connection.query(query, (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Get tasks by user
app.get('/tasks/user/:user_id', (req, res) => {
const query = 'SELECT * FROM tasks WHERE user_id = ?';
connection.query(query, [req.params.user_id], (err, results) => {
if (err) throw err;
res.json(results);
});
});
// Update a task
app.put('/tasks/:id', (req, res) => {
const { title, description, category_id, status_id, deadline } = req.body;
const query = 'UPDATE tasks SET title = ?, description = ?, category_id = ?, status_id = ?, deadline = ? WHERE id = ?';
connection.query(query, [title, description, category_id, status_id, deadline, req.params.id], (err, result) => {
if (err) throw err;
res.send('Task updated successfully!');
});
});
// Delete a task
app.delete('/tasks/:id', (req, res) => {
const query = 'DELETE FROM tasks WHERE id = ?';
connection.query(query, [req.params.id], (err, result) => {
if (err) throw err;
res.send('Task deleted successfully!');
});
});
5. Frontend Implementation
The frontend can be built using HTML, CSS, and JavaScript. You can use a modern frontend framework such as React, Angular, or Vue to interact with the backend API. The frontend should allow users to:
- Create and edit tasks.
- View tasks by user, category, and status.
- Delete tasks.
- Filter tasks by deadline and status.
6. Conclusion
By following the steps outlined above, you will have built a task management system using MySQL as the backend database. This system will allow users to manage their tasks, organize them by category, and track progress using different task statuses. You can extend this system by adding features like notifications, task prioritization, and more!