HTML Forms
1. Introduction to Forms
The <form>
tag is used to collect user input. Forms are a core component of interactive web applications, allowing users to submit data to a server.
Attributes like action
and method
define where and how the data will be submitted.
Example of a Simple Form:
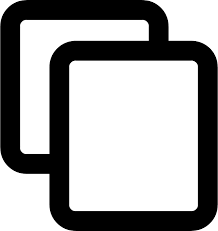
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<br>
<input type="submit" value="Submit">
</form>
In this example, the action
attribute specifies the form submission URL, and method
determines the HTTP method (GET or POST).
2. Input Fields
The <input>
element is used to create interactive controls for forms. Common types include text
, password
, checkbox
, and radio
.
Example of Input Fields:
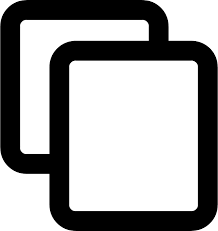
<form>
<label>Username:</label>
<input type="text" name="username" required>
<br>
<label>Password:</label>
<input type="password" name="password" required>
<br>
<label>Gender:</label>
<input type="radio" name="gender" value="male"> Male
<input type="radio" name="gender" value="female"> Female
<br>
<input type="submit" value="Login">
</form>
In this example, radio
buttons allow users to select a single option.
3. Dropdowns and Text Areas
The <select>
element creates a dropdown menu, and the <textarea>
element allows multi-line text input.
Example of Dropdown and Text Area:
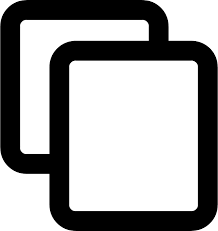
<form>
<label for="country">Select your country:</label>
<select id="country" name="country">
<option value="india">India</option>
<option value="usa">USA</option>
<option value="uk">UK</option>
</select>
<br>
<label for="feedback">Feedback:</label>
<textarea id="feedback" name="feedback" rows="4" cols="50"></textarea>
<br>
<input type="submit" value="Submit Feedback">
</form>
This example demonstrates how to create a dropdown menu and a text area for user feedback.
HTML Basics
1. Tags, Elements, and Attributes
In HTML, everything is structured using tags. Tags are the building blocks of HTML and they define different elements on the page. An element is the combination of a tag, its content, and any attributes it may have. For example:
In the example above, <a>
is the tag, the element is the
<a>
tag and its content ("Click here"), and
href="https://example.com"
is an attribute of the <a>
tag.
2. Text Formatting Tags
HTML provides various tags to format text. Common formatting tags include:
<p>
- Paragraph element.<b>
- Bold text.<i>
- Italic text.<h1> to <h6>
- Headings from largest (<h1>
) to smallest (<h6>
).
Example:
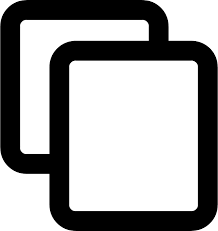
This is a Heading 1
This is a bold and italic paragraph.
3. Lists
HTML supports different types of lists:
- Ordered Lists: Created using the
<ol>
tag, with each item enclosed in<li>
. - Unordered Lists: Created using the
<ul>
tag, with each item enclosed in<li>
. - Definition Lists: Created using the
<dl>
,<dt>
, and<dd>
tags for defining terms and their descriptions.
Example of an ordered list:
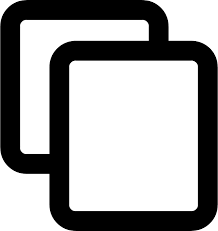
- First item
- Second item
- Third item
4. Links
Links are created using the <a>
tag. The href
attribute specifies
the URL of the page the link goes to:
5. Images
To display images in HTML, you use the <img>
tag. The src
attribute specifies the image source URL, and the alt
attribute provides a
description of the image for accessibility.
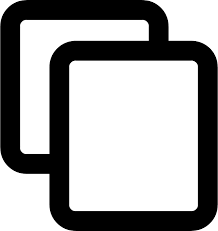
6. Tables
Tables are created using the <table>
tag. Rows are defined with
<tr>
, and table headers and data are enclosed in <th>
and
<td>
, respectively:
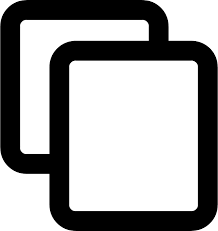
Name
Age
John
30
Jane
25
HTML Tags and Attributes
1. What are HTML Tags?
HTML tags are the fundamental building blocks of an HTML document. They are used to define and structure content on a webpage. Tags are enclosed in angle brackets < >
. Most tags have an opening and a closing tag, with the content placed between them.
Example of a tag:
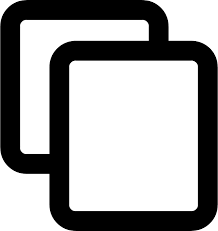
This is a paragraph.
In this example, <p>
is the opening tag, </p>
is the closing tag, and "This is a paragraph." is the content.
2. What are HTML Attributes?
Attributes provide additional information about an element. They are written inside the opening tag and usually come in name-value pairs, such as name="value"
.
Commonly used attributes include:
href
- Specifies the URL for a link.src
- Specifies the source file for an image, video, or audio.alt
- Provides alternative text for an image.class
- Assigns one or more class names to an element for CSS styling.id
- Specifies a unique identifier for an element.
Example of an attribute:
In this example, href
specifies the link destination, and target="_blank"
makes the link open in a new tab.
3. Self-Closing Tags
Some tags do not require a closing tag and are called self-closing tags. Examples include <img>
, <br>
, and <hr>
.
Example of a self-closing tag:
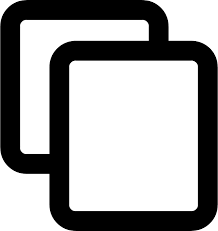
In this example, the <img>
tag is self-closing and includes attributes for the image source and alternative text.
4. Nesting Tags
Tags can be nested inside each other to create a hierarchical structure. It is important to close inner tags before closing outer tags.
Example of nested tags:
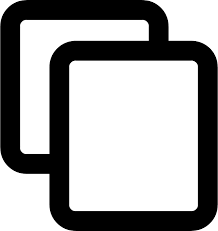
Welcome
This is a nested structure.
In this example, the <h1>
and <p>
tags are nested inside a <div>
container.
HTML Elements
HTML elements are the building blocks of web pages, each serving a specific purpose in defining content and structure. Understanding the difference between block-level and inline elements is crucial for creating well-structured web pages.
Block-Level vs Inline Elements
HTML elements can be broadly categorized into two types:
Type | Description |
---|---|
Block-Level Elements |
|
Inline Elements |
|
Common HTML Elements
Below are some of the most commonly used HTML elements and their purposes:
Element | Description |
---|---|
<p> | Defines a paragraph. It is a block-level element used to group sentences or blocks of text. |
<div> | Defines a container for grouping other HTML elements. Often used with CSS for layout purposes. |
<span> | Defines an inline container, typically used for styling small parts of text or grouping inline elements. |
<h1> to <h6> | Defines headings, with <h1> being the highest (most important) level and <h6> being the lowest. |
Code Example: Block-Level and Inline Elements
Here’s an example showcasing block-level and inline elements:
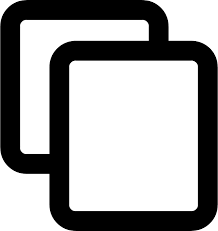
<html>
<head>
<title>HTML Elements Example</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a paragraph inside a block-level element.</p>
<div>
<p>This is a paragraph inside a div container.</p>
</div>
<p>This text contains an <span style="color:blue;">inline span</span> element.</p>
<p>Visit <a href="https://www.example.com">Example</a> for more information.</p>
</body>
</html>
Diagram: Block vs Inline Elements
The following diagram illustrates the behavior of block-level and inline elements:

In this diagram, you can see how block-level elements stack vertically, while inline elements flow within the same line.
HTML Multimedia
1. Adding Audio
The <audio>
tag in HTML is used to embed audio content in a webpage. It
supports various audio formats like MP3, OGG, and WAV.
To ensure compatibility across different browsers, you should provide multiple source formats for
the same audio file. The <audio>
tag comes with attributes like
controls
, autoplay
, loop
, and muted
.
Example of Adding Audio:
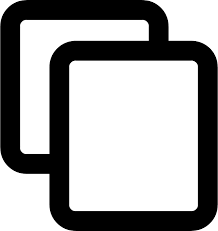
<audio controls>
<source src="audio-file.mp3" type="audio/mpeg">
<source src="audio-file.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
In this example, the controls
attribute adds playback controls like play, pause, and
volume adjustment.
2. Adding Video
The <video>
tag is used to embed video content in HTML. Similar to the
<audio>
tag, it supports multiple formats, such as MP4, WebM, and OGG.
The <video>
tag also supports attributes like controls
,
autoplay
, loop
, muted
, and poster
(a
placeholder image before the video plays).
Example of Adding Video:
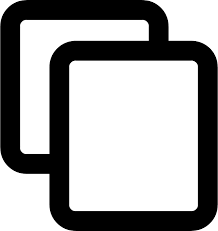
<video controls width="640" height="360">
<source src="video-file.mp4" type="video/mp4">
<source src="video-file.webm" type="video/webm">
Your browser does not support the video element.
</video>
The width and height attributes define the dimensions of the video player. You can add fallback
text inside the <video>
tag for unsupported browsers.
3. Embedding Content
The <iframe>
tag is used to embed external content, such as videos, maps, or
other webpages, within a webpage. It's commonly used for embedding content from platforms like
YouTube or Google Maps.
Attributes like src
, width
, height
, and
frameborder
control the appearance and behavior of the embedded content.
Example of Embedding a YouTube Video:
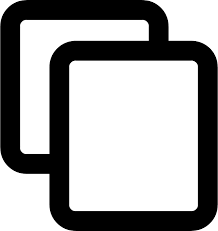
<iframe
src="https://www.youtube.com/embed/example-video-id"
width="560"
height="315"
frameborder="0"
allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture"
allowfullscreen>
</iframe>
The allowfullscreen
attribute enables full-screen playback. You can customize the
dimensions of the <iframe>
to fit your layout.
Example of Embedding a Google Map:
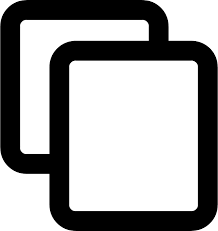
<iframe
src="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!..."
width="600"
height="450"
style="border:0;"
allowfullscreen=""
loading="lazy">
</iframe>
This example demonstrates how to embed a Google Map into your webpage. The
loading="lazy"
attribute defers loading the iframe content for better performance.
HTML Semantics
1. Importance of Semantic HTML
Semantic HTML refers to using HTML tags that have a clear and meaningful purpose in defining the
structure of web content. Unlike non-semantic tags like <div>
or
<span>
, semantic tags such as <header>
,
<footer>
, and <article>
provide additional meaning to the
browser, developers, and assistive technologies.
The benefits of semantic HTML include:
- Improved Accessibility: Assistive technologies like screen readers can better interpret semantic elements, making the web more accessible for users with disabilities.
- Enhanced SEO: Search engines prioritize semantic content because it helps them understand the structure and importance of your content.
- Maintainable Code: Semantic tags make the code more readable and easier to maintain by providing a clear structure.
Example of Semantic vs Non-Semantic HTML:
Non-Semantic HTML:
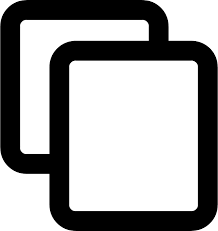
<div class="header">
<h1>Welcome</h1>
</div>
Semantic HTML:
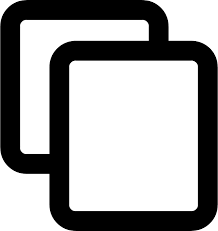
<header>
<h1>Welcome</h1>
</header>
The semantic version is more meaningful and easier to interpret.
2. Common Semantic Tags
HTML5 introduced several semantic tags to define different parts of a webpage. These tags enhance readability and provide structure to the content.
1. <header>
The <header>
element represents the introductory content or navigational links
for its parent section.
Example:
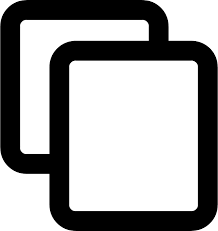
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
</ul>
</nav>
</header>
2. <footer>
The <footer>
element represents the footer of a document or section, often
containing metadata, links, or copyright information.
Example:
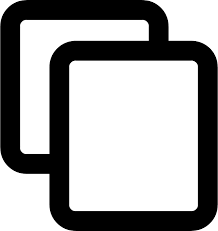
<footer>
<p>© 2024 My Website. All rights reserved.</p>
</footer>
3. <main>
The <main>
element represents the central content of a webpage, excluding
headers, footers, and sidebars.
Example:
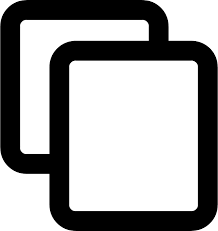
<main>
<h2>Welcome to My Website</h2>
<p>Here is some important content.</p>
</main>
4. <section>
The <section>
element defines a thematic grouping of content, typically with a
heading.
Example:
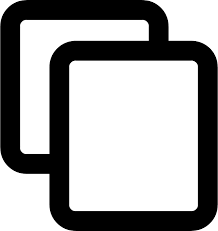
<section>
<h2>About Us</h2>
<p>We are a leading company in the industry.</p>
</section>
5. <article>
The <article>
element represents self-contained content that can be
independently distributed or reused.
Example:
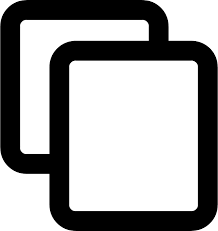
<article>
<h3>News Title</h3>
<p>This is the content of the news article.</p>
</article>
6. <aside>
The <aside>
element contains content that is indirectly related to the main
content, such as sidebars or pull quotes.
Example:
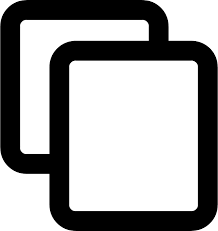
<aside>
<p>Did you know? HTML5 introduced over 20 new tags!</p>
</aside>
7. <nav>
The <nav>
element is used for navigational links.
Example:
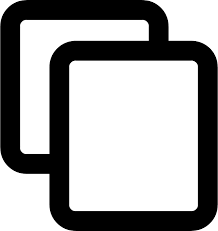
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
HTML Graphics
1. SVG (Scalable Vector Graphics)
SVG (Scalable Vector Graphics) is a vector-based graphics format for creating and displaying images directly in HTML. Unlike raster images, SVG graphics can be scaled without losing quality, making them ideal for responsive design and high-resolution displays.
SVG is XML-based, which means you can use it to define images with elements and attributes. This makes it highly customizable and animatable with CSS and JavaScript.
Advantages of SVG:
- Scalability: SVG images retain quality at any size.
- Interactive: SVG elements can be styled, animated, and interacted with using JavaScript and CSS.
- Lightweight: SVG files are often smaller than equivalent raster images for complex graphics.
Basic SVG Example:
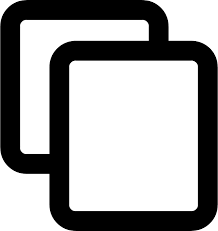
<svg width="200" height="200">
<circle cx="100" cy="100" r="80" fill="blue" stroke="black" stroke-width="2" />
<text x="50" y="110" font-size="20" fill="white">Hello SVG</text>
</svg>
SVG File as an <img>
Element:
You can also use an external SVG file like a regular image:
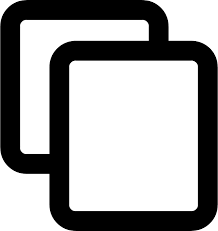
<img src="graphic.svg" alt="Sample SVG Graphic">
2. Canvas API (
Canvas API provides a way to draw graphics programmatically using JavaScript.
The <canvas>
element creates a drawing area where you can render shapes,
text, images, and animations.
Unlike SVG, which is vector-based, <canvas>
is pixel-based and does not retain
scalability or interactivity natively. It is suitable for dynamic graphics like charts, games,
and animations.
Basic Canvas Example:
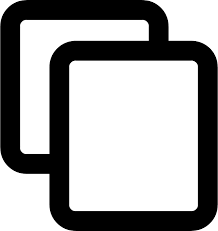
<canvas id="myCanvas" width="200" height="200" style="border:1px solid #000;">
Your browser does not support the HTML canvas element.
</canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Draw a rectangle
ctx.fillStyle = 'blue';
ctx.fillRect(50, 50, 100, 100);
// Draw a circle
ctx.beginPath();
ctx.arc(100, 100, 40, 0, 2 * Math.PI);
ctx.fillStyle = 'red';
ctx.fill();
</script>
Comparing SVG and Canvas:
Feature | SVG | Canvas |
---|---|---|
Graphics Type | Vector-based | Pixel-based |
Interactivity | Directly interactive | Requires JavaScript for interactivity |
Performance | Better for complex static images | Better for dynamic graphics |
Scalability | Scales without quality loss | Fixed resolution, scaling causes quality loss |
When to Use:
- Use SVG for static images, logos, icons, and scalable graphics that need interactivity.
- Use Canvas for dynamic content like animations, games, or real-time data visualizations.
HTML Advanced
1. Data Attributes (data-* attributes)
Data attributes are custom attributes that allow developers to store extra
information directly in HTML elements. They are prefixed with data-
and can be
accessed using JavaScript. Data attributes are commonly used to store metadata that can be
dynamically retrieved or manipulated.
Example of Data Attributes:
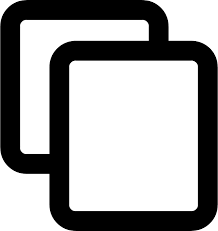
<button data-id="123" data-role="admin">Click Me</button>
<script>
const button = document.querySelector('button');
console.log(button.dataset.id); // Outputs: 123
console.log(button.dataset.role); // Outputs: admin
</script>
Advantages:
- Enhances HTML elements with additional metadata.
- Easy to access and manipulate using JavaScript (
dataset
). - Does not interfere with standard HTML attributes.
2. Microdata and Structured Data
Microdata is a way to embed structured data within HTML, making it easier for search engines and other tools to parse and understand the content of your web pages. Structured data enhances the visibility of your content in search results, enabling rich snippets.
Example of Microdata:
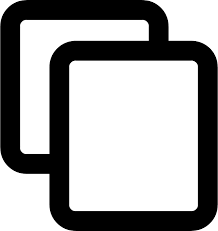
<article itemscope itemtype="http://schema.org/Book">
<h2 itemprop="name">HTML for Beginners</h2>
<p>Author: <span itemprop="author">John Doe</span></p>
<p>Price: <span itemprop="price">$19.99</span></p>
</article>
Advantages of Structured Data:
- Improves SEO with rich snippets.
- Makes your content more discoverable by search engines.
- Provides additional context for your content.
3. Custom Elements
Custom elements allow developers to create their own HTML tags with custom behavior. These elements enhance the functionality of web applications by extending the standard HTML vocabulary.
Example of a Custom Element:
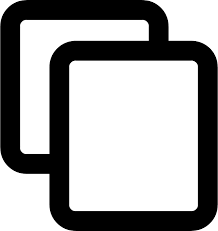
class MyElement extends HTMLElement {
connectedCallback() {
this.innerHTML = '<h3>Hello from MyElement!</h3>';
}
}
customElements.define('my-element', MyElement);
// Usage:
<my-element></my-element>
Custom elements are often used in conjunction with web components to create reusable and modular code.
4. Web Components
Web components are a set of technologies that allow developers to create reusable and encapsulated components for web applications. They include:
- Custom Elements: Define new HTML elements.
- Shadow DOM: Encapsulate the internal structure of components.
- HTML Templates: Define reusable templates for components.
Example of a Web Component:
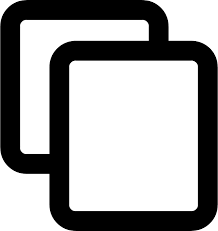
class GreetingComponent extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `
<style>
p { color: blue; font-size: 18px; }
</style>
<p>Hello, World!</p>
`;
}
}
customElements.define('greeting-component', GreetingComponent);
// Usage:
<greeting-component></greeting-component>
5. Shadow DOM
The Shadow DOM is a core technology of web components that provides encapsulation for the internal structure of elements. It allows developers to isolate styles and markup, ensuring that the component's implementation does not affect the rest of the page.
Example of Shadow DOM:
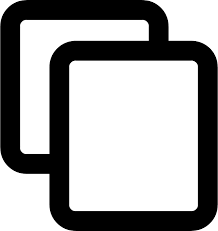
const host = document.querySelector('#host');
const shadowRoot = host.attachShadow({ mode: 'open' });
shadowRoot.innerHTML = `
<style>
p { color: red; }
</style>
<p>This is inside the Shadow DOM.</p>
`;
When using Shadow DOM, styles and scripts defined inside are scoped to the shadow root and do not interfere with global styles.
6. HTML Templates
HTML Templates allow you to define reusable blocks of HTML that are not rendered
until explicitly invoked. These templates are defined using the <template>
tag.
Example of an HTML Template:
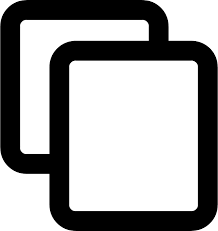
<template id="card-template">
<div class="card">
<h3>Card Title</h3>
<p>Card content goes here.</p>
</div>
</template>
<script>
const template = document.querySelector('#card-template');
const content = template.content.cloneNode(true);
document.body.appendChild(content);
</script>
HTML templates are not rendered by default and can be reused multiple times in a document, making them ideal for dynamic content generation.
HTML APIs
1. Geolocation API
The Geolocation API allows web applications to access the geographic location of a user’s device. This is useful for location-based services such as maps, weather updates, and local search.
With the Geolocation API, you can retrieve the user’s current position using either GPS or other available location services, such as Wi-Fi or IP address.
Example of Geolocation API:
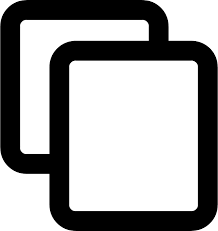
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(function(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
console.log('Latitude: ' + latitude + ', Longitude: ' + longitude);
});
} else {
console.log("Geolocation is not supported by this browser.");
}
Important Considerations:
- The user must grant permission for their location to be accessed.
- It’s important to handle errors gracefully, for example, when location services are unavailable or the user denies permission.
2. Drag-and-Drop API
The Drag-and-Drop API enables users to drag elements within a web page and drop them onto other elements. This feature is commonly used in file uploaders, image galleries, and interactive UIs.
Basic Concept:
The drag-and-drop interaction involves three main steps:
- Drag: Initiating the drag operation on an element.
- Dragover: Handling the element while it's being dragged.
- Drop: Defining the target area where the dragged element can be dropped.
Example of Drag-and-Drop API:
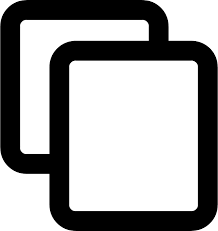
<div id="drag-item" draggable="true">Drag Me</div>
<div id="drop-target">Drop Here</div>
<script>
const dragItem = document.getElementById('drag-item');
const dropTarget = document.getElementById('drop-target');
dragItem.addEventListener('dragstart', function(e) {
e.dataTransfer.setData('text', e.target.id);
});
dropTarget.addEventListener('dragover', function(e) {
e.preventDefault();
});
dropTarget.addEventListener('drop', function(e) {
e.preventDefault();
const data = e.dataTransfer.getData('text');
const draggedElement = document.getElementById(data);
dropTarget.appendChild(draggedElement);
});
</script>
Considerations:
- Use
dragstart>,
dragover>, and
drop
events to implement drag-and-drop functionality. - Ensure the
dragover
event hase.preventDefault()
to allow a drop.
3. Web Storage (Local and Session Storage)
Web Storage provides a way for web applications to store data on the client
side. This data can be saved persistently or temporarily, and can be accessed across page
reloads and browser sessions. It consists of two main types: localStorage
and
sessionStorage
.
- localStorage: Stores data with no expiration time. The data persists even after the browser is closed and reopened.
- sessionStorage: Stores data for the duration of the page session. The data is lost when the page is closed.
Example of Using Web Storage:
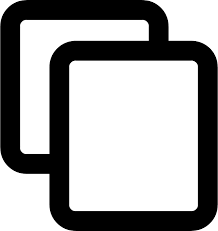
// Storing data
localStorage.setItem('username', 'JohnDoe');
sessionStorage.setItem('sessionData', '12345');
// Retrieving data
const username = localStorage.getItem('username');
const sessionData = sessionStorage.getItem('sessionData');
console.log(username); // Output: JohnDoe
console.log(sessionData); // Output: 12345
// Removing data
localStorage.removeItem('username');
sessionStorage.removeItem('sessionData');
Considerations:
- Both
localStorage
andsessionStorage
store data as key-value pairs. - Data stored in
localStorage
is available across browser sessions, whilesessionStorage
is specific to the page session. - Web storage is synchronous, meaning it can impact performance if used improperly for large amounts of data.
4. HTML5 Offline Capabilities (file)
The HTML5 Offline Web Application features allow web applications to work
offline, even when the device is not connected to the internet. This is achieved using the
manifest
file, which contains a list of resources that should be cached for offline
use.
Creating a Manifest File:
A manifest file is a simple text file with the CACHE MANIFEST
header, followed by a
list of resources. These resources are cached when the web application is first loaded and can
be accessed offline afterward.
Example of a Manifest File:
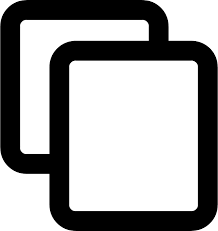
CACHE MANIFEST
# Version 1.0
/index.html
/styles.css
script.js
/images/logo.png
NETWORK:
*
Using the Manifest File in HTML:
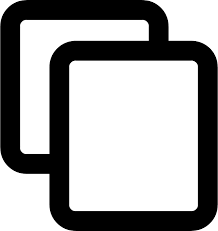
<html manifest="app.manifest">
<head>
<title>Offline App</title>
</head>
<body>
<h1>Welcome to the Offline App</h1>
</body>
</html>
Considerations:
- The manifest file is referenced in the HTML
<html>
tag with themanifest
attribute. - Resources listed in the
CACHE
section will be cached for offline use. TheNETWORK
section allows resources to be fetched from the network if they are not cached. - Modern alternatives like Service Workers provide more advanced offline capabilities and
caching strategies, as the
manifest
file has been deprecated.
Responsive Design with HTML
1. Meta Tags (<meta viewport>
for Responsiveness)
To make web pages responsive and adapt to different screen sizes, you need to use the
<meta>
tag with the viewport
attribute. This tag ensures that
your web content is displayed correctly on mobile devices and that the layout adapts to the
device’s width.
Example of a Viewport Meta Tag:
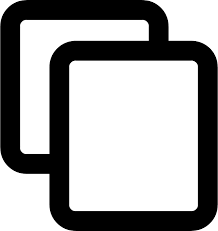
<meta name="viewport" content="width=device-width, initial-scale=1">
Attributes:
width=device-width
: Sets the width of the viewport to the width of the device.initial-scale=1
: Ensures that the page is scaled at a 1:1 ratio when first loaded.
This tag is critical for mobile-first design, helping the page to scale properly across various devices like smartphones, tablets, and desktops.
2. Mobile-First Approach
The Mobile-First Approach is a design philosophy where you design for the smallest screen sizes first (mobile), and then use responsive techniques like CSS media queries to progressively enhance the layout for larger screen sizes such as tablets and desktops.
Why Mobile-First?
- Mobile devices are the most commonly used for web browsing, so designing with mobile in mind ensures better usability for a larger audience.
- It results in faster page load times because smaller screens require fewer resources.
- Responsive design ensures that your website provides an optimal user experience across all devices.
Example of Mobile-First Design Concept:
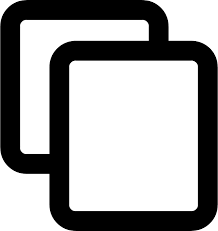
/* Default styles for mobile devices */
body {
font-size: 16px;
}
/* Media Query for tablets and larger devices */
@media (min-width: 768px) {
body {
font-size: 18px;
}
}
/* Media Query for large screens */
@media (min-width: 1024px) {
body {
font-size: 20px;
}
}
In this example, the default font size is designed for mobile, and as the screen size increases, the font size increases as well.
3. Integration with CSS and Media Queries
CSS media queries play a key role in responsive web design. They allow you to apply different styles depending on the screen size, resolution, and other device characteristics.
What Are Media Queries?
Media queries are a part of CSS that allows you to define different styles based on certain conditions like the width of the viewport, device type, or orientation.
Example of Media Queries:
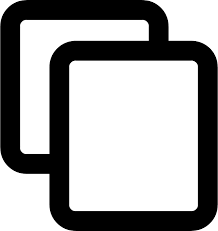
/* Base styles for small screens */
body {
font-size: 16px;
}
/* Apply styles for tablets and larger screens */
@media (min-width: 600px) {
body {
font-size: 18px;
}
}
/* Apply styles for desktop screens */
@media (min-width: 1024px) {
body {
font-size: 20px;
}
}
In this example, the font size increases as the viewport width grows. The @media
rule is used to define the styles for each screen size range.
Common Media Query Conditions:
min-width
: Used to apply styles for viewports larger than a certain width.max-width
: Used to apply styles for viewports smaller than a certain width.orientation
: Can be used to target devices in portrait or landscape mode.
Example of Using Orientation:
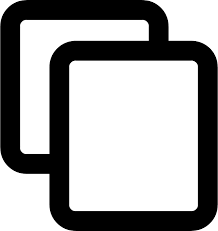
/* Landscape orientation */
@media (orientation: landscape) {
body {
background-color: lightblue;
}
}
/* Portrait orientation */
@media (orientation: portrait) {
body {
background-color: lightgreen;
}
}
Considerations:
- Mobile-first design encourages the use of
@media
queries for larger screens, ensuring efficient loading and performance on mobile devices. - Responsive design should not only consider screen size but also other factors like resolution (e.g., Retina displays) and orientation (landscape or portrait).
HTML Accessibility
1. ARIA (Accessible Rich Internet Applications) Attributes
ARIA (Accessible Rich Internet Applications) is a set of attributes added to HTML elements to enhance accessibility for users with disabilities. These attributes provide additional information to assistive technologies (like screen readers) about the behavior and structure of elements that might not be adequately described by default HTML elements.
Common ARIA Attributes:
aria-label
: Provides a label for an element, especially when the element's content is not descriptive enough on its own. For example, a button with an icon might require a label for screen readers to convey its purpose.aria-hidden
: Used to hide elements from screen readers. This can be useful for decorative elements that don't add any functional content.aria-live
: Defines how assertive the screen reader should be when notifying the user about dynamic content updates.aria-describedby
: Provides a reference to another element that describes the current element, like providing extra information about a form field.aria-expanded
: Indicates the expanded or collapsed state of an element, often used with accordions or dropdowns.
Example of Using ARIA Attributes:
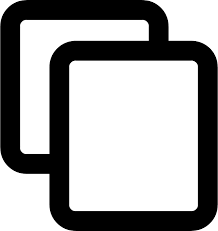
<button aria-label="Close" onclick="closeWindow()">X</button>
This button uses the aria-label
attribute to provide a description for screen
readers, allowing users to understand the purpose of the button even if it's just a simple icon.
2. Best Practices for Accessible HTML
Creating accessible web content ensures that users with disabilities can interact with your website. Here are some best practices to follow:
1. Use Semantic HTML:
Always use semantic HTML elements (such as <header>
,
<footer>
, <main>
, <article>
,
<nav>
, etc.) to structure your content. These elements help screen readers
understand the document’s structure and provide a better navigation experience for users.
2. Provide Text Alternatives:
Ensure that every non-text element (such as images, videos, and icons) has a text alternative.
Use the alt
attribute for images and aria-label
for elements like
buttons with icons.
3. Ensure Keyboard Accessibility:
Make sure all interactive elements (like forms, links, buttons, etc.) are keyboard accessible. Users should be able to navigate the website without relying on a mouse.
4. Use <label>
Elements for Forms:
Ensure that all form fields have associated <label>
elements. This provides a
clear association between the form control and its description, which helps users with screen
readers.
5. Avoid Using Color Alone:
Don’t rely solely on color to convey important information. For example, using red to highlight errors in a form may not be accessible to users who are colorblind. Instead, use text labels or icons alongside color changes.
6. Ensure Proper Contrast:
Make sure there’s enough contrast between text and its background. This ensures readability for users with low vision.
7. Use Accessible Forms:
Forms should have clearly defined labels and instructions. Make sure that users can navigate the form fields via keyboard and screen readers.
Example of Accessible Form:
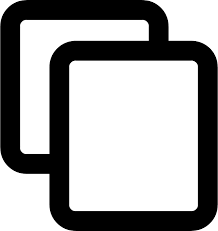
<form>
<label for="email">Email Address</label>
<input type="email" id="email" name="email" required />
<label for="password">Password</label>
<input type="password" id="password" name="password" required />
<button type="submit">Submit</button>
</form>
This form uses <label>
elements, which are crucial for screen readers and
keyboard navigation.
3. Screen Reader Testing
Screen readers are assistive technologies that read the content of the webpage aloud, allowing users with visual impairments to interact with the web. Testing your website with a screen reader is crucial for ensuring that your content is accessible.
Popular Screen Readers:
- NVDA (NonVisual Desktop Access): A free, open-source screen reader for Windows.
- VoiceOver: Built into macOS and iOS devices.
- JAWS (Job Access With Speech): A popular screen reader for Windows.
- TalkBack: A screen reader for Android devices.
How to Test with Screen Readers:
- Install a screen reader tool (like NVDA or VoiceOver).
- Navigate through your website using only the keyboard (no mouse).
- Listen for announcements from the screen reader and ensure that all interactive elements (such as buttons, links, and form fields) are described appropriately.
- Ensure that content is read in the correct order, and that ARIA attributes and labels are working as expected.
- Test for focus management, making sure that focus remains within interactive elements and that the user can easily move between sections.
Example of Screen Reader Friendly Content:
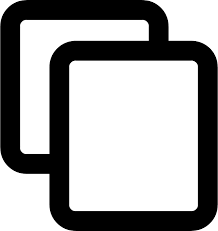
<button aria-label="Close the menu">X</button>
In this example, the screen reader will read out "Close the menu" when the user focuses on the button, helping them understand its purpose.
HTML Performance Optimization
1. Lazy Loading Images and Iframes (loading="lazy")
Lazy loading is a technique used to improve web page load times by deferring the loading of images and other resources until they are needed. This is particularly useful for images or iframes below the fold (i.e., off-screen initially), reducing the number of requests made when the page is first loaded and improving overall performance.
The loading="lazy"
attribute can be added to <img>
and
<iframe>
elements to enable native lazy loading. With this attribute, the
browser will wait to load these resources until they are about to be displayed on the user's
screen.
Example of Lazy Loading an Image:
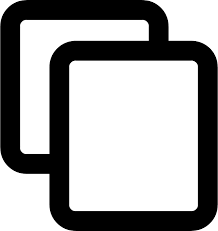
<img src="large-image.jpg" alt="A beautiful landscape" loading="lazy">
This image will not be loaded until the user scrolls near its position in the viewport, saving bandwidth and speeding up the initial load of the page.
Example of Lazy Loading an Iframe:
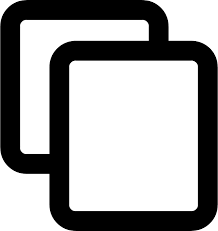
<iframe src="https://www.example.com" loading="lazy" width="600" height="400"></iframe>
Similarly, an <iframe>
can be lazy-loaded, which is beneficial when embedding
external content like videos or maps.
2. Minification of HTML
Minification is the process of removing unnecessary characters from HTML files, such as white spaces, newlines, and comments, without affecting the functionality of the code. This reduces the size of HTML files, leading to faster load times and improved performance.
Minification of HTML can significantly reduce the file size, especially for large web pages or complex applications, by eliminating redundancies in the code. It is essential to perform minification as part of the build process before deploying to production.
Example of Minified HTML:
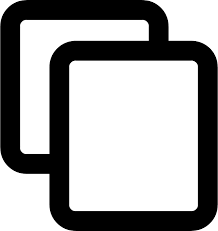
<!DOCTYPE html><html><head><title>My Page</title></head><body><h1>Hello World</h1></body></html>
Here, the minified HTML version is just a single line of code, without unnecessary spaces, making it more efficient to load and process.
Tools for Minifying HTML:
- HTMLMinifier: A popular tool for minifying HTML code. It can be used as a command-line tool or integrated into build systems like Gulp or Webpack.
- Minify Code: An online tool that allows you to minify HTML, CSS, and JavaScript directly from your browser.
Many build tools and task runners, such as Webpack or Gulp, offer plugins or tasks that can automate the minification process during development.
3. Preloading and Prefetching Resources
Preloading and prefetching are strategies used to optimize the loading of critical resources, ensuring that the most important resources are available as soon as they are needed.
Preloading Resources:
Preloading allows you to instruct the browser to fetch a resource (such as a stylesheet, script, or image) as soon as possible, even before it's needed in the document. This is especially useful for resources that are critical to the page's initial rendering and interaction.
The <link rel="preload">
tag is used to preload resources. It's important to
specify the as
attribute to define the type of content being loaded.
Example of Preloading a CSS File:
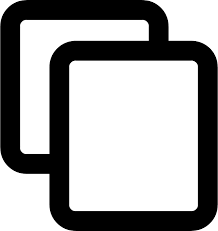
<link rel="preload" href="styles.css" as="style">
This tells the browser to start fetching the styles.css
file early, so it is ready
when it's referenced later in the HTML document.
Prefetching Resources:
Prefetching is used to tell the browser to fetch resources that may be needed in the future, but not immediately. This can be useful for resources on pages that the user might navigate to next, reducing load times when they do.
The <link rel="prefetch">
tag is used for prefetching, and it works similarly
to preloading, but with a lower priority.
Example of Prefetching a Resource:
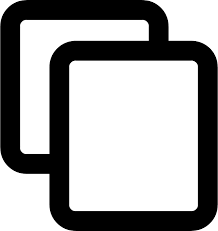
<link rel="prefetch" href="next-page">
This tells the browser to fetch the next-page.html
file ahead of time, so that when
the user navigates to that page, it loads faster.
When to Use Preloading and Prefetching:
- Preload: Use for resources critical to the initial rendering of the page, such as stylesheets, JavaScript files, or fonts.
- Prefetch: Use for resources that will likely be used in the future, such as images, scripts, or pages that users might visit next.
Example of Using Preload and Prefetch Together:
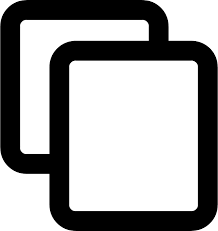
<link rel="preload" href="critical-style.css" as="style">
<link rel="prefetch" href="future-resource.js">
This combination ensures that the most critical resources are fetched immediately, while less important resources are prefetched for future use.
Performance Considerations:
Using preload
and prefetch
can significantly speed up your website's
performance, but be careful not to preload too many resources, as this can overwhelm the network
and lead to unnecessary delays in other parts of the page loading.
HTML and SEO
1. Meta Tags ( , )
Meta tags are HTML elements used to provide structured metadata about a web
page, which is essential for search engines and social media platforms. Two of the most
important meta tags for SEO are the <meta name="description">
and
<meta name="keywords">
.
Meta Description
The <meta name="description">
tag defines a brief description of the page
content. It is often displayed in search engine results and can influence click-through rates.
It's important to make the description clear, concise, and relevant to the content of the page.
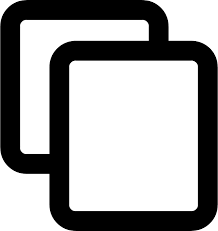
<meta name="description" content="Learn about the best practices in SEO and how to optimize your website for search engines">
Meta Keywords
The <meta name="keywords">
tag was used in the past to specify a list of
keywords relevant to the page. While it is no longer as influential in modern SEO, some search
engines may still use it to understand the page content.
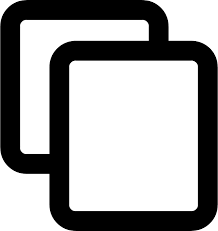
<meta name="keywords" content="SEO, search engine optimization, HTML, website optimization">
Best Practices for Meta Tags:
- Keep the description under 160 characters for optimal display in search results.
- Use relevant, specific keywords for the
keywords
tag, though its impact is minimal today. - Ensure both tags accurately reflect the content of the page to avoid misleading users and search engines.
2. Headings and Hierarchy
Headings (<h1>
to <h6>
) are important for SEO because they
help search engines understand the structure of your content. Proper use of headings improves
accessibility, readability, and helps with SEO rankings by providing search engines with clear
indications of the page's topic.
Importance of Headings for SEO
<h1>
: Represents the main topic or heading of the page, ideally used once per page.<h2>
: Used for subheadings, breaking down the main topic into sections.<h3>
to<h6>
: Used for further breakdowns or subcategories.
Example of Proper Heading Hierarchy:
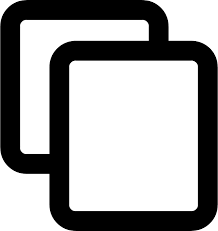
<h1>SEO Optimization for Websites</h1>
<h2>Meta Tags and Their Importance</h2>
<h3>Meta Description</h3>
<h3>Meta Keywords</h3>
<h2>Headings and Structure</h2>
<h3>Using <h1>
for Page Titles</h3>
Using a logical heading hierarchy helps both users and search engines understand the content and flow of the page. This also makes the page more accessible, particularly for screen readers.
3. Structured Data for SEO
Structured data uses a specific format (often JSON-LD or Microdata) to help search engines understand the context and content of the page more effectively. It is a way to provide additional details about the content, such as products, events, or reviews, which can improve visibility in search results through rich snippets.
Structured Data Example (JSON-LD):
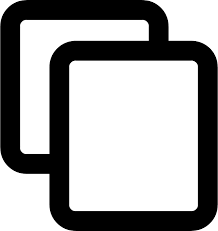
<script type="application/ld+json">
{
"@context": "http://schema.org",
"@type": "Article",
"headline": "SEO Optimization for Websites",
"author": {
"@type": "Person",
"name": "John Doe"
},
"datePublished": "2024-11-25"
}
</script>
This structured data markup adds context to the article, making it easier for search engines to understand and display the information in search results.
Benefits of Structured Data for SEO:
- Increases the likelihood of rich snippets in search results.
- Enhances the visibility and engagement of your content.
- Improves understanding by search engines, leading to better rankings.
4. Sitemap Creation
A sitemap is a file that lists all the pages on your website, helping search engines discover and index your content more efficiently. It can also guide search engine bots in crawling dynamic or complex websites.
Types of Sitemaps:
- XML Sitemap: Most commonly used by search engines for better indexing of pages. This is a machine-readable format.
- HTML Sitemap: A user-friendly list of links that allows visitors to navigate the website easily.
Example of an XML Sitemap:
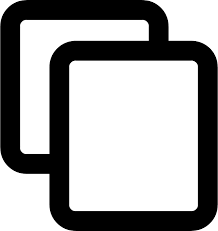
<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>https://www.example.com/page1</loc>
<lastmod>2024-11-25</lastmod>
<priority>0.8</priority>
</url>
<url>
<loc>https://www.example.com/page2</loc>
<lastmod>2024-11-24</lastmod>
<priority>0.7</priority>
</url>
</urlset>
How to Submit a Sitemap:
- Use Google Search Console to submit your XML sitemap.
- Ensure the sitemap is up-to-date and includes all important pages of your website.
Benefits of Creating a Sitemap:
- Helps search engines find all the important pages on your site.
- Ensures that newly added pages or updates are indexed quickly.
- Improves the efficiency of website crawling, especially for large websites.