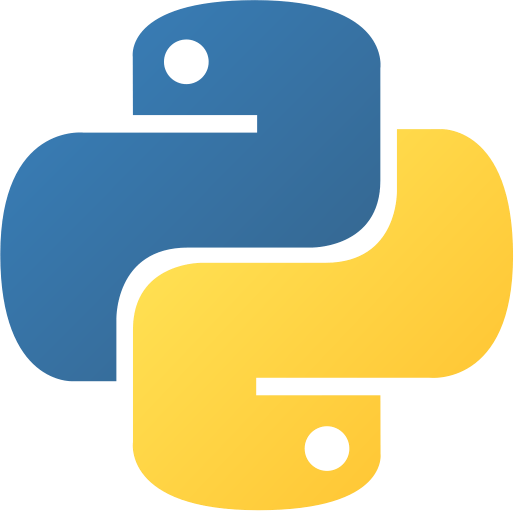
Introduction to Python
Python is a versatile, high-level programming language that prioritizes readability and ease of use. Created by Guido van Rossum in the late 1980s and released in 1991, Python’s simple syntax allows developers to focus on solving problems rather than the complexities of the language itself.
History of Python
Python was designed as an easy-to-understand alternative to other programming languages like C and Java. Over time, it became widely popular due to its broad applications, ease of use, and large community support. Python is now one of the most popular languages used for web development, data science, machine learning, automation, and more.
Python Features
Below are the key features that make Python a preferred choice for developers:
Feature | Description |
---|---|
Simple Syntax | Python has a clean and easy-to-understand syntax, which makes it beginner-friendly. The syntax of Python is designed to closely resemble the English language. |
Interpreted Language | Python code is executed line-by-line by an interpreter, which simplifies debugging and enhances flexibility in development. |
Cross-Platform | Python is platform-independent, meaning that the same Python code can run on different operating systems like Windows, macOS, and Linux without modification. |
Extensive Libraries | Python provides a rich ecosystem of libraries and frameworks, such as NumPy, Pandas, Django, Flask, and more, making it suitable for diverse applications like data analysis, machine learning, web development, etc. |
Setting Up Python
Before writing Python code, you need to set up Python on your system. Follow the steps below to install Python:
- Download the Python installer from the official website.
- Run the installer and make sure to check the box that says "Add Python to PATH".
- Once installed, open your command line or terminal and type
python --version
to verify that Python is installed correctly.
Code Example: Hello World
Let’s write a simple Python program that outputs the text "Hello, World!" to the console:
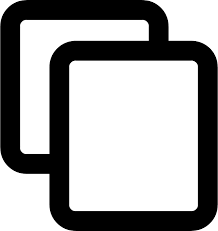
# Python program to print Hello World
print("Hello, World!")
Diagram: Python Program Execution
The following diagram explains the execution flow of a Python program:

In this diagram, you can see the flow of Python code starting from the script creation to the execution in the Python interpreter.
Features and Benefits of Python
Python is a widely used programming language known for its simplicity and versatility. It offers a variety of features that make it an excellent choice for beginners and experienced developers alike. Below are some of the key features and benefits of Python:
1. Easy to Learn and Use
Python has a clear and readable syntax that closely resembles the English language, making it easy to learn. It is known for its simplicity and readability, reducing the learning curve for new programmers.
2. High-Level Language
As a high-level language, Python abstracts away most of the complex details of the computer's operation. This allows developers to focus more on solving problems and implementing solutions rather than worrying about memory management or low-level programming details.
3. Interpreted Language
Python is an interpreted language, meaning that the code is executed line-by-line by an interpreter rather than being compiled into machine code. This makes Python code easier to debug and test, as well as faster for development cycles.
4. Cross-Platform Compatibility
Python is platform-independent, meaning that Python programs can run on various operating systems like Windows, macOS, and Linux without requiring any changes to the code. This ensures portability and flexibility for developers.
5. Extensive Standard Library
Python comes with a large standard library that provides built-in modules and packages for tasks like file handling, networking, web scraping, and more. This allows developers to quickly implement functionality without the need for third-party packages.
6. Object-Oriented and Modular
Python supports object-oriented programming (OOP), allowing developers to design programs using classes and objects. Python’s modularity also allows code to be organized into reusable modules, improving maintainability and scalability.
7. Large Community Support
Python has an active and thriving community of developers worldwide. This extensive support system includes online forums, tutorials, and libraries, making it easy for newcomers to find help, resources, and solutions to common problems.
8. Versatile and Powerful
Python is used in a wide range of applications, from web development and data analysis to machine learning, artificial intelligence, scientific computing, and automation. Its versatility makes it suitable for many domains and industries.
9. Integration Capabilities
Python can be easily integrated with other languages like C, C++, and Java, and it supports integration with technologies like REST APIs, databases, and cloud services. This makes Python a powerful tool for building complex systems and applications.
10. High Demand in Job Market
Python is one of the most in-demand programming languages in the job market, especially in fields like data science, machine learning, and web development. Its popularity ensures that there are many job opportunities for developers skilled in Python.
Summary of Python’s Benefits
- Easy to learn and use
- High-level and interpreted language
- Cross-platform compatibility
- Extensive standard library
- Object-oriented and modular programming
- Large and supportive community
- Versatile and can be used for various applications
- Strong integration capabilities
- High demand in the job market
Installing Python on Windows, macOS, and Linux
Python is easy to install on all major operating systems. Below are the steps for installing Python on Windows, macOS, and Linux systems:
Installing Python on Windows
Follow these steps to install Python on a Windows machine:
- Visit the official Python website: Download Python.
- Click on the "Download Python" button for the latest version (make sure to select the version compatible with your system).
- Run the installer after downloading it.
- Ensure that the option “Add Python to PATH” is checked during installation.
- Click "Install Now" and wait for the installation to complete.
- Once installed, open Command Prompt and type
python --version
to confirm the installation.
Installing Python on macOS
Follow these steps to install Python on macOS:
- Visit the official Python website: Download Python.
- Click on the "Download Python" button for macOS (choose the latest version available).
- Open the downloaded .pkg file to begin the installation process.
- Follow the on-screen instructions to complete the installation.
- Once the installation is complete, open the Terminal and type
python3 --version
to confirm the installation.
Note: macOS comes with a version of Python 2.x pre-installed, but you will need to install Python 3.x for the latest features and libraries. Use python3
instead of python
to use the newer version.
Installing Python on Linux
Python is typically pre-installed on most Linux distributions. However, if it's not installed or if you want to install a different version, follow these steps:
- Open the terminal on your Linux machine.
- To check if Python is already installed, type
python --version
(for Python 2.x) orpython3 --version
(for Python 3.x). - If Python is not installed, use the following commands to install it:
- For Ubuntu/Debian-based systems:
sudo apt update && sudo apt install python3
- For Fedora:
sudo dnf install python3
- For CentOS/RHEL:
sudo yum install python3
- For Ubuntu/Debian-based systems:
- Once installed, verify the installation by typing
python3 --version
in the terminal.
Setting Up pip (Python's Package Installer)
After installing Python, you may want to install additional Python libraries and packages. This is done using pip, Python's package installer. To install pip:
- On Windows and macOS, pip is automatically installed with Python 3.x versions. To check if pip is installed, type
pip --version
in the command prompt or terminal. - On Linux, if pip is not installed, you can install it using the following commands:
- For Ubuntu/Debian:
sudo apt install python3-pip
- For Fedora:
sudo dnf install python3-pip
- For CentOS/RHEL:
sudo yum install python3-pip
- For Ubuntu/Debian:
- Once pip is installed, you can use it to install libraries. For example, to install the requests library, use:
pip install requests
.
Verifying the Installation
After installing Python and pip, you can verify the installation by running the following commands in your terminal or command prompt:
python --version
(orpython3 --version
on macOS/Linux) to check Python version.pip --version
to verify that pip is installed.
Conclusion
Now that Python is installed on your system, you’re ready to start writing and running Python programs. You can use a text editor or Integrated Development Environment (IDE) like PyCharm or Visual Studio Code to write your Python code.
Setting Up Your First Python Program
Now that you have Python installed, let's set up your first Python program. This section will guide you through creating and running a simple Python script.
Step 1: Choosing a Text Editor or IDE
To write Python code, you need a text editor or an Integrated Development Environment (IDE). You can choose from several options:
- VS Code: A popular code editor with Python support and extensions.
- PyCharm: A full-featured IDE specifically designed for Python development.
- Sublime Text: A lightweight text editor with Python syntax highlighting.
- Jupyter Notebooks: An interactive environment ideal for data science and machine learning projects.
If you are just starting, we recommend using VS Code as it is lightweight, highly customizable, and has a great set of Python extensions.
Step 2: Writing Your First Python Program
Let’s create a simple program that prints “Hello, World!” to the console. Follow these steps:
- Open your text editor or IDE.
- Create a new file and save it with the name
hello_world.py
. - Write the following Python code in the file:
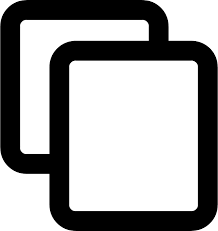
# Python program to print Hello World
print("Hello, World!")
Step 3: Running Your Python Program
Now that you have written your Python program, let’s run it to see the output:
- Open the command prompt (Windows) or terminal (macOS/Linux).
- Navigate to the directory where you saved the
hello_world.py
file. For example, if you saved it in the "Documents" folder, you would type: - Run the Python program by typing:
- You should see the output:
cd Documents
python hello_world.py
Hello, World!
Step 4: Modifying Your Program
Now that you’ve seen your first Python program, let’s try modifying it to do something different. Edit the code to print a personalized message:
# Python program to print a personalized message
name = "Alice"
print("Hello, " + name + "!")
Save the file and run the program again. You should now see:
Hello, Alice!
Step 5: Debugging Your Program
Sometimes, you may encounter errors in your code. These errors are part of the learning process, and understanding how to debug them is an important skill. Here are a few tips:
- Check for syntax errors: Python will tell you if there’s a mistake in the code (e.g., missing parentheses or quotation marks).
- Use print statements: If your program isn't doing what you expect, add
print()
statements to check the values of variables. - Read error messages: Python's error messages are usually helpful, and they tell you where the error occurred and what type of error it is.
Conclusion
Congratulations! You’ve just written and run your first Python program. From here, you can start experimenting with more complex programs and explore the many features Python has to offer.
Python IDEs: VS Code, PyCharm, Jupyter Notebook, etc.
When developing Python applications, choosing the right Integrated Development Environment (IDE) can greatly enhance your productivity. In this section, we’ll explore some of the most popular Python IDEs and code editors: VS Code, PyCharm, and Jupyter Notebook.
1. Visual Studio Code (VS Code)
VS Code is a lightweight, open-source code editor from Microsoft. It has powerful features and extensions, making it a popular choice for Python developers. Below are some key features of VS Code for Python development:
- Extensions: VS Code has a wide range of extensions, including the Python extension that provides features like IntelliSense, debugging, and linting.
- Debugging: You can set breakpoints, inspect variables, and control the program flow to easily debug your Python code.
- Integrated Terminal: The integrated terminal allows you to run Python scripts directly from the editor.
- Git Integration: VS Code has built-in Git support, which makes version control easy within the editor.
- Cross-Platform: VS Code works on Windows, macOS, and Linux, making it accessible for developers on all platforms.
To install VS Code, visit the official website and download the installer for your operating system.
2. PyCharm
PyCharm is a full-featured IDE specifically designed for Python development. Developed by JetBrains, PyCharm provides a comprehensive set of tools for coding, testing, and deploying Python applications. It comes in two editions: Community (free) and Professional (paid).
- Code Assistance: PyCharm offers intelligent code completion, syntax highlighting, and error checking to improve code quality and productivity.
- Integrated Debugger: PyCharm’s debugger supports both local and remote debugging, making it easy to troubleshoot your Python code.
- Built-in Testing: It provides tools for running and debugging unit tests and integrates with popular testing frameworks like pytest, unittest, and nose.
- Database Tools: PyCharm Professional comes with built-in support for working with databases like MySQL, PostgreSQL, and SQLite.
- Virtual Environment Support: PyCharm makes it easy to create and manage Python virtual environments, isolating dependencies for each project.
To install PyCharm, visit the official website and download the appropriate edition for your needs.
3. Jupyter Notebook
Jupyter Notebook is an open-source web application that allows you to create and share documents that contain live code, equations, visualizations, and narrative text. It’s widely used in data science, machine learning, and academic research. Here are some key features of Jupyter Notebook:
- Interactive Coding: Jupyter allows you to write and execute Python code in cells, making it easy to test and visualize code snippets instantly.
- Visualizations: You can create and display plots using libraries like Matplotlib, Seaborn, and Plotly directly in the notebook.
- Markdown Support: Jupyter supports markdown, so you can add formatted text, equations, and images to document your code and analysis.
- Data Science Friendly: It integrates seamlessly with Python libraries like Pandas, NumPy, and SciPy, making it an ideal choice for data analysis and visualization.
To install Jupyter Notebook, you can use the pip
package manager:
pip install notebook
Then, start the notebook server by running jupyter notebook
in your terminal or command prompt.
4. Other Python IDEs and Code Editors
In addition to VS Code, PyCharm, and Jupyter Notebook, there are other popular IDEs and code editors for Python development:
- Spyder: An IDE specifically designed for data science and scientific computing, with integrated IPython support and advanced debugging features.
- Sublime Text: A lightweight and fast text editor that supports Python syntax highlighting and plugins for additional functionality.
- Atom: An open-source, customizable text editor with support for Python development through community plugins.
- Eclipse with PyDev: Eclipse is a widely used Java IDE, and with the PyDev plugin, it can be used for Python development as well.
Conclusion
Choosing the right IDE depends on your development needs and preferences. For beginners, VS Code and PyCharm are great options due to their user-friendly interfaces and robust features. Jupyter Notebook is perfect for data scientists and those who want to create interactive coding sessions. No matter which IDE you choose, make sure it enhances your workflow and helps you write efficient, error-free code.
Python Syntax and Indentation
Python's syntax is known for its simplicity and readability. Unlike many programming languages that use braces or parentheses to delimit code blocks, Python relies on indentation to define the structure of the code. This section will explain the basic syntax rules of Python and emphasize the importance of indentation.
1. Python Syntax Basics
Python's syntax is designed to be clean and easy to read. Here are some basic rules of Python syntax:
- Statements and Expressions: A statement in Python is an instruction that the Python interpreter can execute. An expression is a combination of values, variables, and operators that the interpreter evaluates to produce a result.
- Comments: Comments are used to explain the code. In Python, comments begin with a
#
symbol. Everything after the#
on that line is ignored by the interpreter.
# This is a comment in Python
x = 5 # integer
y = 3.14 # float
name = "John" # string
print()
function. This function outputs text or variables to the console.print("Hello, Python!")
2. Python Indentation
In Python, indentation is critical for defining the scope of loops, functions, classes, and conditionals. Unlike many languages, Python does not use braces ({}
) or other symbols to indicate a block of code. Instead, it relies on consistent indentation (usually 4 spaces per indentation level) to group code.
Why is Indentation Important?
Indentation is used to define the grouping of statements. For example, all the statements inside a loop or function must be indented at the same level. If the indentation is incorrect, Python will raise an IndentationError
.
Example of Indentation in Python
Here’s an example of how indentation works in Python:
if x > 0:
print("x is positive")
else:
print("x is non-positive")
In this example, both print
statements are indented to indicate they belong to the corresponding if
or else
block. The Python interpreter uses this indentation to determine which code belongs inside the conditional blocks.
Common Indentation Errors
- Mixing Tabs and Spaces: Python recommends using spaces for indentation (typically 4 spaces per level). Mixing tabs and spaces can cause errors. Use your IDE or text editor to set the indentation style to spaces.
# Incorrect indentation
if x > 0:
print("x is positive")
else:
print("x is non-positive") # Error due to mixing tabs and spaces
IndentationError
.# Incorrect indentation level
if x > 0:
print("x is positive")
print("This line is incorrectly indented") # Error due to wrong indentation
3. Indentation in Loops and Functions
Indentation is used to define blocks of code inside loops, functions, and classes. Below is an example of using indentation in a for
loop and a function:
def greet(name):
print("Hello, " + name)
greet("John") # Calling the function
for i in range(3):
print("Iteration:", i) # Indented code inside the loop
Function Definition
In the example above, the code inside the function greet()
is indented to indicate that it belongs to the function. Similarly, the loop block is indented inside the for
loop to define the scope of that loop.
4. Best Practices for Indentation
To avoid errors and maintain readability in your code, follow these best practices:
- Always use 4 spaces for each indentation level.
- Do not mix tabs and spaces. Use spaces exclusively for indentation.
- Ensure that all code inside loops, functions, and conditionals is consistently indented.
- Use an IDE or text editor that automatically handles indentation and highlights errors.
Conclusion
Python's syntax and indentation rules are designed to make code more readable and maintainable. By following the indentation guidelines, you will avoid syntax errors and improve the clarity of your code. Remember, Python relies heavily on indentation to define code structure, so always be consistent and use spaces for indentation.
Variables and Data Types
In Python, variables are used to store data that can be referenced and manipulated in a program. Python is a dynamically typed language, meaning that you do not need to declare the type of a variable when you create it. Python will automatically determine the data type based on the assigned value.
1. Variables
A variable in Python is created by simply assigning a value to a name. The name of the variable should be meaningful and follow these rules:
- Variable names must begin with a letter (a-z, A-Z) or an underscore (_).
- Subsequent characters can be letters, numbers (0-9), or underscores.
- Variable names are case-sensitive (e.g.,
Age
andage
are different variables).
Here’s an example of how to create and assign a value to a variable:
age = 25 # Creating a variable and assigning an integer value
In this example, the variable age
is assigned the value 25
.
2. Data Types in Python
Python supports several built-in data types to represent different kinds of data. The most commonly used data types include:
Numeric Types
Python provides three main numeric types:
- int: Represents integer values (whole numbers).
x = 5 # Integer
y = 3.14 # Float
z = 2 + 3j # Complex number
String Type
Strings are used to represent text in Python. A string is a sequence of characters enclosed in either single quotes ('
) or double quotes ("
).
name = "John" # String enclosed in double quotes
String values can be manipulated using various string methods such as upper()
, lower()
, and split()
.
Boolean Type
The Boolean type represents truth values: True
or False
. It is often used in conditional statements and comparisons.
is_active = True # Boolean value
List Type
A list is a collection of ordered items. Lists can store different types of elements, and they are defined using square brackets ([]
).
fruits = ["apple", "banana", "cherry"] # List of strings
Lists are mutable, meaning you can change, add, or remove items after the list is created.
Tuple Type
A tuple is similar to a list, but it is immutable, meaning its values cannot be changed once assigned. Tuples are defined using parentheses (()
).
coordinates = (4, 5) # Tuple with two integers
Set Type
A set is an unordered collection of unique items. Sets are defined using curly braces ({}
) or the set()
function.
unique_numbers = {1, 2, 3} # Set with unique integers
Dictionary Type
A dictionary is a collection of key-value pairs. It is defined using curly braces and colons (key: value
).
person = {"name": "John", "age": 25} # Dictionary with keys and values
In a dictionary, each key must be unique, and values can be of any data type.
3. Type Conversion
Python allows you to convert between different data types using type conversion functions. Here are some common type conversion functions:
int(x)
: Convertsx
to an integer.
age = int("30") # Converts string to integer
float(x)
: Converts x
to a float.price = float("19.99") # Converts string to float
str(x)
: Converts x
to a string.name = str(25) # Converts integer to string
4. Dynamic Typing
Python is dynamically typed, meaning you do not need to declare the type of a variable when you create it. The type is assigned automatically when a value is assigned to the variable, and the type can change during program execution.
x = 10 # x is an integer
x = "Hello" # Now x is a string
5. Type Checking
You can check the type of a variable using the type()
function:
x = 5
print(type(x)) # Output:
Conclusion
Understanding variables and data types is fundamental in Python programming. Python's dynamic typing makes it easy to work with different data types without explicitly declaring them. By using the appropriate data types for different scenarios, you can write cleaner, more efficient Python code.
Input and Output in Python
Python provides a simple and intuitive way to handle input and output (I/O) operations. You can take input from the user and display output using built-in functions like input()
and print()
.
1. Output in Python
In Python, the print()
function is used to display output to the console. It can accept multiple arguments and automatically converts them to strings, separating them with spaces.
print("Hello, World!") # Outputs: Hello, World!
You can also use formatted strings to display variables within text. There are several ways to format strings:
String Concatenation
You can concatenate strings and variables using the +
operator:
name = "John"
print("Hello, " + name) # Outputs: Hello, John
String Formatting with f-strings (Python 3.6+)
f-strings allow you to insert variables directly into a string by prefixing the string with f
and enclosing variables in curly braces:
name = "John"
age = 25
print(f"Hello, {name}. You are {age} years old.") # Outputs: Hello, John. You are 25 years old.
String Formatting with format()
Method
You can also use the format()
method to format strings:
name = "John"
age = 25
print("Hello, {}. You are {} years old.".format(name, age)) # Outputs: Hello, John. You are 25 years old.
2. Input in Python
The input()
function in Python allows you to take input from the user. The function reads the input as a string, and you can convert it to other data types if necessary.
name = input("Enter your name: ") # Prompts the user for input
print(f"Hello, {name}!") # Outputs the inputted name
If you want to get numeric input, you need to convert the input to the desired data type, such as int()
or float()
:
age = int(input("Enter your age: ")) # Converts input to an integer
print(f"You are {age} years old.") # Outputs the inputted age
3. Reading from Files
In addition to taking input from the user, you can also read input from files. To read from a file, use the open()
function:
file = open("example.txt", "r") # Opens a file in read mode
content = file.read() # Reads the entire content of the file
print(content) # Prints the file content
file.close() # Closes the file
For larger files, you can read the file line by line using a loop:
with open("example.txt", "r") as file:
for line in file:
print(line.strip()) # Strips newline characters and prints each line
4. Writing to Files
You can also write output to a file. To do this, open the file in write mode ("w") and use the write()
method:
with open("output.txt", "w") as file:
file.write("This is a test.") # Writes text to the file
If you want to append data to an existing file, open the file in append mode ("a"):
with open("output.txt", "a") as file:
file.write("\nAdding another line.") # Appends a new line to the file
5. Error Handling in I/O
When working with I/O operations, it’s important to handle errors like file not found or permission errors. You can use a try-except
block to catch and handle such errors:
try:
with open("example.txt", "r") as file:
content = file.read()
print(content)
except FileNotFoundError:
print("The file does not exist.")
except PermissionError:
print("You do not have permission to read this file.") # Handles specific errors
6. Conclusion
Python’s input()
and print()
functions allow you to interact with users effectively. Additionally, Python provides simple and intuitive methods for reading from and writing to files. Handling file I/O with proper error checking is essential for building robust programs.
Python Operators (Arithmetic, Logical, Relational, etc.)
In Python, operators are used to perform operations on variables and values. There are several types of operators, including arithmetic, relational, logical, and others. Understanding these operators is essential for performing calculations, comparisons, and logical operations in Python.
1. Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, etc.
Operator | Example | Description |
---|---|---|
+ | 5 + 3 |
Addition |
- | 5 - 3 |
Subtraction |
* | 5 * 3 |
Multiplication |
/ | 5 / 3 |
Division (returns float) |
// | 5 // 3 |
Floor Division (returns integer) |
% | 5 % 3 |
Modulus (returns remainder) |
** | 5 ** 3 |
Exponentiation (power) |
Example: Using Arithmetic Operators
# Using Arithmetic Operators
a = 5
b = 3
print(a + b) # Addition: 8
print(a - b) # Subtraction: 2
print(a * b) # Multiplication: 15
print(a / b) # Division: 1.666...
print(a // b) # Floor Division: 1
print(a % b) # Modulus: 2
print(a ** b) # Exponentiation: 125
2. Relational (Comparison) Operators
Relational operators are used to compare two values. They return either True or False depending on whether the comparison is true or false.
Operator | Example | Description |
---|---|---|
== | 5 == 3 |
Equal to |
!= | 5 != 3 |
Not equal to |
> | 5 > 3 |
Greater than |
< | 5 < 3 |
Less than |
>= | 5 >= 3 |
Greater than or equal to |
<= | 5 <= 3 |
Less than or equal to |
Example: Using Relational Operators
# Using Relational Operators
a = 5
b = 3
print(a == b) # False
print(a != b) # True
print(a > b) # True
print(a < b) # False
print(a >= b) # True
print(a <= b) # False
3. Logical Operators
Logical operators are used to combine conditional statements and return True or False based on the conditions.
Operator | Example | Description |
---|---|---|
and | True and False |
Returns True if both statements are true |
or | True or False |
Returns True if at least one statement is true |
not | not True |
Returns the opposite of the statement |
Example: Using Logical Operators
# Using Logical Operators
a = True
b = False
print(a and b) # False
print(a or b) # True
print(not a) # False
4. Assignment Operators
Assignment operators are used to assign values to variables. They combine an operation with assignment.
Operator | Example | Description |
---|---|---|
= | a = 5 |
Assigns a value to a variable |
+= | a += 3 |
Adds a value to a variable and assigns the result |
-= | a -= 3 |
Subtracts a value from a variable and assigns the result |
*= | a *= 3 |
Multiplies a variable by a value and assigns the result |
/= | a /= 3 |
Divides a variable by a value and assigns the result |
//= | a //= 3 |
Floor division of a variable by a value and assigns the result |
%= | a %= 3 |
Modulus of a variable by a value and assigns the result |
**= | a **= 3 |
Exponentiation of a variable by a value and assigns the result |
Example: Using Assignment Operators
# Using Assignment Operators
a = 5
a += 3 # a = a + 3
print(a) # 8
a -= 2 # a = a - 2
print(a) # 6
a *= 2 # a = a * 2
print(a) # 12
5. Membership Operators
Membership operators are used to test if a value is a member of a sequence (e.g., a list, tuple, or string).
Operator | Example | Description |
---|---|---|
in | 5 in [1, 2, 3, 4, 5] |
Returns True if the value is found in the sequence |
not in | 5 not in [1, 2, 3, 4] |
Returns True if the value is not found in the sequence |
Example: Using Membership Operators
# Using Membership Operators
numbers = [1, 2, 3, 4, 5]
print(5 in numbers) # True
print(6 not in numbers) # True
6. Identity Operators
Identity operators are used to compare the memory location of two objects.
Operator | Example | Description |
---|---|---|
is | a is b |
Returns True if both variables refer to the same object |
is not | a is not b |
Returns True if both variables do not refer to the same object |
Example: Using Identity Operators
# Using Identity Operators
a = [1, 2, 3]
b = [1, 2, 3]
c = a
print(a is b) # False
print(a is c) # True
Conclusion
Python operators are essential for performing various operations on variables and values. By understanding the different types of operators such as arithmetic, relational, logical, and assignment operators, you can create effective Python programs for a wide range of tasks.
Conditional Statements: if, elif, else
Conditional statements are used to make decisions in Python based on certain conditions. These statements allow you to execute different code blocks depending on whether a condition is true or false. Python provides three main types of conditional statements: if
, elif
, and else
.
1. The if
Statement
The if
statement is used to check if a condition is true. If the condition is true, the block of code inside the if
statement will be executed.
# Example: if statement
age = 18
if age >= 18:
print("You are an adult.")
In this example, the condition age >= 18
is true, so the message "You are an adult." will be printed.
2. The elif
Statement
The elif
(short for "else if") statement is used when you have multiple conditions to check. It allows you to test additional conditions if the initial if
condition is false.
# Example: if-elif statement
age = 16
if age >= 18:
print("You are an adult.")
elif age >= 13:
print("You are a teenager.")
In this example, the condition age >= 18
is false, so Python checks the next condition age >= 13
. Since age = 16
, the message "You are a teenager." will be printed.
3. The else
Statement
The else
statement is used to execute a block of code when all preceding conditions in the if
and elif
statements are false.
# Example: if-elif-else statement
age = 10
if age >= 18:
print("You are an adult.")
elif age >= 13:
print("You are a teenager.")
else:
print("You are a child.")
In this case, since age = 10
, neither of the first two conditions is true, so the code inside the else
block will be executed, and the message "You are a child." will be printed.
4. Nested Conditional Statements
You can also use conditional statements inside other conditional statements. This is called nesting. This is useful when you have more complex conditions to check.
# Example: Nested if-else statement
age = 20
is_student = True
if age >= 18:
if is_student:
print("You are an adult and a student.")
else:
print("You are an adult.")
else:
print("You are a minor.")
In this example, the outer if
checks if the person is an adult, and if so, the inner if
checks if the person is a student. The message "You are an adult and a student." will be printed because age = 20
and is_student = True
.
5. Logical Operators in Conditional Statements
You can combine multiple conditions using logical operators such as and
, or
, and not
in your conditional statements.
# Example: Logical operators in if statement
age = 25
has_id = True
if age >= 18 and has_id:
print("You are eligible to vote.")
In this example, both conditions age >= 18
and has_id
need to be true for the message "You are eligible to vote." to be printed.
Conclusion
Conditional statements are fundamental in Python programming as they allow you to control the flow of your program based on dynamic conditions. By using if
, elif
, and else
, you can create programs that make decisions and respond accordingly to different scenarios.
Loops in Python: for and while
Loops are used in Python to execute a block of code repeatedly as long as a certain condition is true. Python provides two main types of loops: the for
loop and the while
loop.
1. The for
Loop
The for
loop is used to iterate over a sequence (such as a list, tuple, string, or range) and execute a block of code for each item in the sequence.
# Example: for loop to iterate through a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
In this example, the loop iterates over each fruit in the fruits
list and prints each one. The output will be:
apple
banana
cherry
2. The range()
Function
The range()
function is commonly used in a for
loop to generate a sequence of numbers. It can take one, two, or three arguments: range(start, stop, step)
.
# Example: for loop with range function
for i in range(1, 6):
print(i)
This loop will print the numbers 1 to 5 (the stop
value is not inclusive).
1
2
3
4
5
3. The while
Loop
The while
loop is used to execute a block of code as long as a condition is true. The condition is evaluated before each iteration, so if the condition is false initially, the code inside the loop won't execute.
# Example: while loop
count = 1
while count <= 5:
print(count)
count += 1
In this example, the loop will print the numbers 1 to 5. The loop continues as long as the condition count <= 5
is true, and after each iteration, the value of count
is incremented by 1.
1
2
3
4
5
4. Infinite Loops
Both for
and while
loops can run indefinitely if the condition is always true. These are called infinite loops and are usually avoided unless explicitly needed (for example, in server programs).
# Example: infinite while loop (Not recommended)
while True:
print("This will run forever!")
The above code will print "This will run forever!" indefinitely. To stop it, you'll need to interrupt the program manually.
5. Breaking Out of Loops
You can use the break
statement to exit a loop prematurely when a certain condition is met. This is useful when you want to stop the loop before it completes all iterations.
# Example: break statement
for num in range(1, 10):
if num == 5:
break
print(num)
In this example, the loop will break when num == 5
, so the output will be:
1
2
3
4
6. Continuing to the Next Iteration
The continue
statement is used to skip the current iteration of a loop and move to the next one. This can be useful when you want to skip over certain conditions.
# Example: continue statement
for num in range(1, 6):
if num == 3:
continue
print(num)
In this example, when num == 3
, the continue
statement skips printing 3, so the output will be:
1
2
4
5
7. Nested Loops
It is possible to have loops inside other loops. These are called nested loops. The inner loop will execute once for each iteration of the outer loop.
# Example: nested loops
for i in range(1, 4):
for j in range(1, 4):
print(f"i = {i}, j = {j}")
This will produce the following output:
i = 1, j = 1
i = 1, j = 2
i = 1, j = 3
i = 2, j = 1
i = 2, j = 2
i = 2, j = 3
i = 3, j = 1
i = 3, j = 2
i = 3, j = 3
Conclusion
Loops are essential for automating repetitive tasks and iterating over collections of data. By using for
and while
loops, you can efficiently process data and handle complex tasks in Python. Mastering loops is a crucial step in becoming proficient with Python.
Using break
, continue
, and pass
In Python, the break
, continue
, and pass
statements are control flow tools that help you manage how loops and conditionals behave. These statements can alter the flow of the program by terminating loops, skipping iterations, or providing empty blocks of code.
1. The break
Statement
The break
statement is used to exit a loop prematurely. When the break
statement is encountered, the loop terminates immediately, and the program continues with the next statement after the loop.
# Example: break statement
for i in range(1, 6):
if i == 3:
break
print(i)
In this example, when i == 3
, the break
statement will stop the loop, and the output will be:
1
2
2. The continue
Statement
The continue
statement is used to skip the current iteration of a loop and move to the next iteration. The code that follows the continue
statement within the loop is skipped for the current iteration.
# Example: continue statement
for i in range(1, 6):
if i == 3:
continue
print(i)
In this example, the continue
statement will skip the iteration when i == 3
, so the output will be:
1
2
4
5
3. The pass
Statement
The pass
statement is a placeholder used when a statement is required syntactically but you do not want to execute any code. It is commonly used in situations where you need to define an empty function or class, or when you want to write a block of code that has not yet been implemented.
# Example: pass statement
for i in range(1, 6):
if i == 3:
pass # Do nothing for 3
print(i)
In this example, the pass
statement does nothing when i == 3
, and the output will be:
1
2
3
4
5
4. Using break
, continue
, and pass
Together
These statements can also be used together in more complex situations to control the flow of loops and conditionals effectively.
# Example: using break, continue, and pass together
for i in range(1, 6):
if i == 2:
continue # Skip 2
elif i == 4:
break # Break the loop when i is 4
elif i == 3:
pass # Do nothing for 3
print(i)
In this example, the output will be:
1
3
The continue
statement skips 2, the pass
statement does nothing for 3, and the break
statement stops the loop when i == 4
.
Conclusion
The break
, continue
, and pass
statements are essential control flow tools that help you manage loops and conditionals. They provide fine-grained control over how your program executes, allowing you to skip iterations, terminate loops early, or define placeholder code for future implementation.
Lists: Creation, Manipulation, and Comprehensions
Lists are one of the most versatile and commonly used data structures in Python. They are ordered collections of items that can store elements of any data type, including other lists. This section will guide you through creating and manipulating lists, as well as using list comprehensions for concise and efficient code.
1. Creating Lists
In Python, a list is created by placing elements inside square brackets [ ]
and separating them with commas. Lists can contain items of different data types, including strings, numbers, and even other lists.
# Example: Creating a list
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
mixed_list = [1, "apple", 3.14, True]
nested_list = [[1, 2, 3], ["a", "b", "c"]]
In the above example, fruits
is a list of strings, numbers
is a list of integers, mixed_list
contains different data types, and nested_list
contains lists within lists.
2. Manipulating Lists
Lists in Python are mutable, meaning you can modify, add, and remove items after the list has been created.
Accessing List Elements
List elements can be accessed by their index, starting from 0 for the first element.
# Example: Accessing elements by index
first_fruit = fruits[0] # "apple"
last_fruit = fruits[-1] # "cherry"
Modifying List Elements
You can change the value of a specific element by accessing its index and assigning a new value.
# Example: Modifying elements
fruits[1] = "blueberry" # Changes "banana" to "blueberry"
Adding Elements to a List
Use append()
to add an element to the end of the list, or insert()
to insert an element at a specific index.
# Example: Adding elements
fruits.append("grape") # Adds "grape" to the end of the list
fruits.insert(1, "orange") # Inserts "orange" at index 1
Removing Elements from a List
Use remove()
to remove a specific element by value, or pop()
to remove an element by index.
# Example: Removing elements
fruits.remove("orange") # Removes "orange" from the list
removed_fruit = fruits.pop(2) # Removes and returns the element at index 2
3. List Comprehensions
List comprehensions provide a concise way to create lists. They allow you to apply an expression to each item in a sequence, and optionally filter items using an if
statement.
# Example: List comprehension
squares = [x**2 for x in range(1, 6)] # [1, 4, 9, 16, 25]
This example creates a list of squares for the numbers 1 through 5. List comprehensions are often more efficient and readable compared to using traditional loops.
With Conditional Statements
You can add a conditional statement to filter elements based on a condition.
# Example: List comprehension with condition
even_squares = [x**2 for x in range(1, 6) if x % 2 == 0] # [4, 16]
In this case, only even numbers are squared and included in the list.
4. Nested List Comprehensions
List comprehensions can also be nested to work with multi-dimensional lists (lists within lists).
# Example: Nested list comprehension
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [elem for row in matrix for elem in row] # [1, 2, 3, 4, 5, 6, 7, 8, 9]
This example flattens a 2D matrix into a 1D list.
Conclusion
Lists in Python are an essential tool for managing collections of data. By mastering list creation, manipulation, and list comprehensions, you can write more efficient and readable Python code. List comprehensions, in particular, allow you to create and filter lists in a single, concise line of code.
Tuples: Immutable Data Structures
In Python, tuples are similar to lists but with one major difference: they are immutable. Once a tuple is created, its content cannot be changed. Tuples are used when you want to store a collection of items that should not be modified.
1. Creating Tuples
Tuples are created using parentheses ()
and separating elements with commas. Here's how to create a tuple:
# Creating a simple tuple
my_tuple = (1, 2, 3, 4, 5)
Tuples can contain different types of data:
# Tuple with different data types
mixed_tuple = (1, "Hello", 3.14, True)
Empty tuples can be created like this:
# Creating an empty tuple
empty_tuple = ()
For a tuple with a single element, you must include a trailing comma:
# Creating a tuple with one element
single_element_tuple = (5,)
2. Accessing Elements in a Tuple
You can access individual elements in a tuple using indices, just like with lists. Python tuples are also zero-indexed:
# Accessing elements by index
my_tuple = (1, 2, 3, 4, 5)
print(my_tuple[0]) # Output: 1
print(my_tuple[3]) # Output: 4
Negative indexing can be used to access elements from the end of the tuple:
# Negative indexing
print(my_tuple[-1]) # Output: 5 (last element)
print(my_tuple[-2]) # Output: 4 (second last element)
3. Tuple Operations
Although tuples are immutable, you can still perform some operations on them like concatenation and repetition:
# Concatenation
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
combined_tuple = tuple1 + tuple2
print(combined_tuple) # Output: (1, 2, 3, 4, 5, 6)
# Repetition
repeated_tuple = tuple1 * 2
print(repeated_tuple) # Output: (1, 2, 3, 1, 2, 3)
4. Tuple Slicing
Just like lists, tuples can be sliced. The syntax is tuple[start:end]
, where start
is the starting index (inclusive), and end
is the ending index (exclusive).
# Tuple slicing
my_tuple = (1, 2, 3, 4, 5, 6)
print(my_tuple[1:4]) # Output: (2, 3, 4)
# Slicing with step
print(my_tuple[::2]) # Output: (1, 3, 5) (every second element)
5. Tuple Packing and Unpacking
Tuples can be packed into a single variable and unpacked into multiple variables:
# Tuple packing
packed_tuple = 1, 2, 3 # Packing values into a tuple
# Tuple unpacking
a, b, c = packed_tuple # Unpacking tuple into variables
print(a, b, c) # Output: 1 2 3
6. Nested Tuples
Tuples can contain other tuples, making them "nested". You can access elements from nested tuples using multiple indices:
# Example of a nested tuple
nested_tuple = ((1, 2), (3, 4), (5, 6))
print(nested_tuple[1][1]) # Output: 4 (second tuple, second element)
7. Why Use Tuples?
Tuples are used when you want to ensure that the data cannot be altered. Since they are immutable, they are faster than lists and can be used as keys in dictionaries, while lists cannot be.
Additionally, tuples provide some safety in your code as you are guaranteed that the stored data will not be inadvertently modified.
Conclusion
Tuples are a great choice when you need an immutable collection of items. They are lightweight, faster than lists, and offer a safe way to store data that should remain unchanged throughout the program.
Sets: Union, Intersection, and Differences
In Python, sets are unordered collections of unique elements. They are useful when you want to store a collection of items without duplicates and perform operations like union, intersection, and differences efficiently.
1. Creating Sets
Sets are created using curly braces {}
or the set()
constructor:
# Creating a set using curly braces
my_set = {1, 2, 3, 4, 5}
# Creating a set using the set() constructor
another_set = set([4, 5, 6, 7])
Note that sets do not allow duplicate elements:
# Duplicate elements are ignored
duplicate_set = {1, 2, 2, 3, 4}
print(duplicate_set) # Output: {1, 2, 3, 4}
2. Union of Sets
The union of two sets combines all the unique elements from both sets. You can use the |
operator or the union()
method to perform a union:
# Union using the | operator
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1 | set2
print(union_set) # Output: {1, 2, 3, 4, 5}
# Union using the union() method
union_set_method = set1.union(set2)
print(union_set_method) # Output: {1, 2, 3, 4, 5}
The union operation ensures that only unique elements are kept, even if there are duplicates in the original sets.
3. Intersection of Sets
The intersection of two sets returns only the elements that are common to both sets. You can use the &
operator or the intersection()
method to perform an intersection:
# Intersection using the & operator
set1 = {1, 2, 3}
set2 = {3, 4, 5}
intersection_set = set1 & set2
print(intersection_set) # Output: {3}
# Intersection using the intersection() method
intersection_set_method = set1.intersection(set2)
print(intersection_set_method) # Output: {3}
4. Difference of Sets
The difference of two sets returns the elements that are present in the first set but not in the second set. You can use the -
operator or the difference()
method to perform a difference:
# Difference using the - operator
set1 = {1, 2, 3}
set2 = {3, 4, 5}
difference_set = set1 - set2
print(difference_set) # Output: {1, 2}
# Difference using the difference() method
difference_set_method = set1.difference(set2)
print(difference_set_method) # Output: {1, 2}
5. Symmetric Difference of Sets
The symmetric difference of two sets returns the elements that are in either set but not in both. You can use the ^
operator or the symmetric_difference()
method to perform a symmetric difference:
# Symmetric Difference using the ^ operator
set1 = {1, 2, 3}
set2 = {3, 4, 5}
symmetric_difference_set = set1 ^ set2
print(symmetric_difference_set) # Output: {1, 2, 4, 5}
# Symmetric Difference using the symmetric_difference() method
symmetric_difference_set_method = set1.symmetric_difference(set2)
print(symmetric_difference_set_method) # Output: {1, 2, 4, 5}
6. Set Methods for Other Operations
Python provides several other useful methods for sets:
add()
: Adds a single element to a set.remove()
: Removes a specific element from a set. Throws aKeyError
if the element is not found.discard()
: Removes a specific element from a set without throwing an error if the element is not found.clear()
: Removes all elements from the set.copy()
: Returns a shallow copy of the set.
Code Examples:
# Adding an element to a set
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
# Removing an element from a set (with remove)
my_set.remove(3)
print(my_set) # Output: {1, 2, 4}
# Removing an element from a set (with discard)
my_set.discard(5) # Does not raise an error even if the element is not found
print(my_set) # Output: {1, 2, 4}
# Clearing a set
my_set.clear()
print(my_set) # Output: set()
7. Why Use Sets?
Sets are useful when you need to perform operations like union, intersection, and differences on collections of data efficiently. They are also beneficial when you need to guarantee that the data is unique, as they automatically eliminate duplicates.
Conclusion
Sets in Python are powerful data structures that allow for fast membership tests and mathematical set operations. They're an essential tool for working with collections of unique elements and performing operations like union, intersection, and differences.
Dictionaries: Key-Value Pairs
In Python, dictionaries are mutable, unordered collections that store data in key-value pairs. Each key is unique, and values can be of any data type. Dictionaries are used to store data that is associated with a unique identifier (key).
1. Creating Dictionaries
Dictionaries are created using curly braces {}
, with key-value pairs separated by colons. Keys must be immutable types (e.g., strings, numbers, tuples), and values can be any data type:
# Creating a dictionary with key-value pairs
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
2. Accessing Dictionary Values
You can access the values in a dictionary by using the corresponding key inside square brackets or with the get()
method:
# Accessing values using square brackets
print(my_dict["name"]) # Output: Alice
# Accessing values using the get() method
print(my_dict.get("age")) # Output: 25
The get()
method is preferred as it prevents a KeyError
if the key doesn't exist, returning None
instead.
3. Modifying Dictionary Values
To modify a value in a dictionary, simply assign a new value to the existing key:
# Modifying a value
my_dict["age"] = 30
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
4. Adding New Key-Value Pairs
To add new key-value pairs to a dictionary, assign a value to a new key:
# Adding a new key-value pair
my_dict["email"] = "alice@example.com"
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York', 'email': 'alice@example.com'}
5. Removing Key-Value Pairs
You can remove key-value pairs from a dictionary using the del
keyword, the pop()
method, or the popitem()
method:
# Removing a key-value pair using del
del my_dict["email"]
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
# Removing a key-value pair using pop()
email = my_dict.pop("email", "Key not found")
print(email) # Output: Key not found
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
# Removing and returning the last key-value pair using popitem()
last_item = my_dict.popitem()
print(last_item) # Output: ('city', 'New York')
print(my_dict) # Output: {'name': 'Alice', 'age': 30}
6. Looping Through Dictionaries
You can loop through a dictionary to access its keys, values, or key-value pairs:
# Looping through keys
for key in my_dict:
print(key)
# Output: name, age
# Looping through values
for value in my_dict.values():
print(value)
# Output: Alice, 30
# Looping through key-value pairs
for key, value in my_dict.items():
print(key, value)
# Output: name Alice, age 30
7. Dictionary Methods
Python dictionaries come with several useful methods:
keys()
: Returns a view of all the keys in the dictionary.values()
: Returns a view of all the values in the dictionary.items()
: Returns a view of all the key-value pairs in the dictionary.clear()
: Removes all key-value pairs from the dictionary.copy()
: Returns a shallow copy of the dictionary.update()
: Updates the dictionary with key-value pairs from another dictionary or iterable of key-value pairs.
Example of using these methods:
# Using keys(), values(), and items()
print(my_dict.keys()) # Output: dict_keys(['name', 'age'])
print(my_dict.values()) # Output: dict_values(['Alice', 30])
print(my_dict.items()) # Output: dict_items([('name', 'Alice'), ('age', 30)])
# Using the update() method to merge dictionaries
new_data = {"city": "New York", "email": "alice@example.com"}
my_dict.update(new_data)
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York', 'email': 'alice@example.com'}
8. Nested Dictionaries
Python allows you to create dictionaries inside dictionaries, which is known as a nested dictionary. You can access the values in a nested dictionary by chaining key lookups:
# Creating a nested dictionary
nested_dict = {
"person1": {"name": "Alice", "age": 25},
"person2": {"name": "Bob", "age": 30}
}
# Accessing values in a nested dictionary
print(nested_dict["person1"]["name"]) # Output: Alice
9. Dictionary Comprehensions
Just like list comprehensions, Python supports dictionary comprehensions, which provide a concise way to create dictionaries:
# Creating a dictionary using dictionary comprehension
squares = {x: x**2 for x in range(5)}
print(squares) # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
10. Why Use Dictionaries?
Dictionaries are useful when you want to store data that is associated with a specific key (e.g., user profiles, product details, etc.). They allow you to quickly look up values based on keys, making them ideal for fast access to data.
Conclusion
Dictionaries are a powerful data structure in Python, providing an efficient way to store and retrieve data based on unique keys. With their versatile methods and support for various operations, dictionaries are widely used in real-world Python applications.
Strings: Manipulation, Slicing, and Formatting
Strings in Python are sequences of characters enclosed in either single quotes '
or double quotes "
. Python provides a variety of methods to manipulate and format strings, which are essential in handling text-based data.
1. Creating Strings
Strings can be created using either single or double quotes:
# Creating strings
string1 = "Hello, World!"
string2 = 'Python Programming'
2. String Indexing and Slicing
Strings are indexed, and indexing starts at 0. You can also use negative indices to start counting from the end of the string.
# Indexing
my_string = "Hello"
print(my_string[0]) # Output: H
print(my_string[-1]) # Output: o
# Slicing
print(my_string[1:4]) # Output: ell
print(my_string[:3]) # Output: Hel
print(my_string[2:]) # Output: llo
The slicing
syntax is my_string[start:end]
, where start
is the starting index, and end
is the ending index (exclusive).
3. String Concatenation
To concatenate strings, use the +
operator:
# Concatenating strings
greeting = "Hello" + " " + "World!"
print(greeting) # Output: Hello World!
4. String Repetition
You can repeat a string using the *
operator:
# Repeating a string
repeat_string = "Python! " * 3
print(repeat_string) # Output: Python! Python! Python!
5. String Length
To find the length of a string, use the len()
function:
# Finding the length of a string
my_string = "Hello"
print(len(my_string)) # Output: 5
6. String Methods
Python provides many built-in methods to manipulate strings. Some commonly used ones include:
lower()
: Converts all characters to lowercase.upper()
: Converts all characters to uppercase.strip()
: Removes any leading and trailing spaces.replace()
: Replaces a substring with another substring.split()
: Splits a string into a list based on a delimiter.find()
: Finds the first occurrence of a substring and returns its index.
Example of using these methods:
# Using string methods
my_string = " Hello, Python! "
print(my_string.lower()) # Output: hello, python!
print(my_string.upper()) # Output: HELLO, PYTHON!
print(my_string.strip()) # Output: Hello, Python!
print(my_string.replace("Python", "World")) # Output: Hello, World!
print(my_string.split(", ")) # Output: ['Hello', 'Python!']
print(my_string.find("Python")) # Output: 8
7. String Formatting
Python offers multiple ways to format strings:
Using f-strings (Python 3.6+)
f-strings provide a concise way to embed expressions inside string literals:
# Using f-strings
name = "Alice"
age = 25
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string) # Output: My name is Alice and I am 25 years old.
Using format()
method
The format()
method allows you to insert placeholders in the string and then fill them with values:
# Using the format() method
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # Output: My name is Alice and I am 25 years old.
Using % formatting (older method)
The %
operator can also be used for string formatting, though it is less common in modern Python code:
# Using % formatting
formatted_string = "My name is %s and I am %d years old." % (name, age)
print(formatted_string) # Output: My name is Alice and I am 25 years old.
8. Multiline Strings
Multiline strings can be created using triple quotes '''
or """
:
# Creating a multiline string
multiline_string = """This is a string
that spans multiple lines."""
print(multiline_string)
9. Escape Characters
Escape characters allow you to include special characters in a string, such as newlines, tabs, or quotation marks:
# Using escape characters
escaped_string = "This is a string with a newline character\nand a tab character\t."
print(escaped_string)
10. String Encoding and Decoding
Strings in Python are Unicode by default. You can encode a string into bytes using encode()
and decode bytes back to a string using decode()
:
# Encoding a string
byte_string = my_string.encode("utf-8")
print(byte_string) # Output: b'Hello'
# Decoding bytes back to a string
decoded_string = byte_string.decode("utf-8")
print(decoded_string) # Output: Hello
11. Why Use Strings?
Strings are one of the most commonly used data types in Python. They are essential for handling text data, displaying messages, and processing user input. The flexibility provided by Python’s string manipulation methods makes it a powerful tool for working with text in various applications.
Conclusion
Python strings offer a wide range of functionalities for text processing and manipulation. Understanding string operations is fundamental to mastering Python, whether you are working with user input, file handling, or web development.
Defining and Calling Functions
Functions in Python are blocks of reusable code that perform a specific task. By defining functions, you can avoid repetition and improve code organization. Functions can take inputs (parameters), perform operations, and return outputs.
1. Defining a Function
To define a function in Python, use the def
keyword followed by the function name and parentheses. The function's code block is indented under the function definition:
# Defining a function
def greet(name):
print(f"Hello, {name}!")
In the example above, the function greet
is defined with one parameter, name
. This function prints a greeting message when called.
2. Calling a Function
To call a function, simply use its name followed by parentheses. If the function requires parameters, pass the arguments inside the parentheses:
# Calling the function
greet("Alice") # Output: Hello, Alice!
In this case, the function greet
is called with the argument "Alice"
, and the output will be Hello, Alice!
.
3. Function Parameters and Arguments
Functions can accept multiple parameters. These parameters act as placeholders for values passed during the function call (arguments). You can define default values for parameters, making them optional when calling the function:
# Function with multiple parameters and default values
def greet(name, greeting="Hello"):
print(f"{greeting}, {name}!")
# Calling the function
greet("Alice") # Output: Hello, Alice!
greet("Bob", "Good morning") # Output: Good morning, Bob!
The second parameter greeting
has a default value of "Hello"
. If no argument is passed for this parameter, it will default to "Hello"
.
4. Return Statement
Functions can return values using the return
keyword. When a function returns a value, it can be captured in a variable or used directly in expressions:
# Function with a return value
def add(a, b):
return a + b
# Calling the function and capturing the return value
result = add(3, 5)
print(result) # Output: 8
In this example, the function add
returns the sum of the two parameters a
and b
.
5. Function Scope
The scope of a variable refers to where the variable is accessible in the code. Variables defined inside a function are local to that function and cannot be accessed outside:
# Example of function scope
def example():
x = 10 # x is local to the function
example()
# print(x) # This would cause an error because x is not defined outside the function
In the example above, the variable x
is local to the example
function and cannot be accessed outside it.
6. Variable Scope: Global vs. Local
Variables can be either global (accessible everywhere) or local (accessible only within the function where they are defined). You can modify a global variable inside a function using the global
keyword:
# Example of global and local variables
x = 5 # Global variable
def modify_global():
global x
x = 10 # Modifying the global variable
modify_global()
print(x) # Output: 10
In this case, the function modify_global
changes the global variable x
to 10
by using the global
keyword.
7. Lambda Functions
Lambda functions, also known as anonymous functions, are small one-line functions that can be defined using the lambda
keyword. They are typically used for short-term tasks:
# Defining a lambda function
multiply = lambda a, b: a * b
# Calling the lambda function
result = multiply(4, 5)
print(result) # Output: 20
In this example, the lambda
function multiply
takes two parameters and returns their product.
8. Function Documentation
It’s a good practice to document your functions using docstrings
. A docstring is a string literal that appears as the first statement in a function, and it describes what the function does:
# Example of a function with a docstring
def greet(name):
"""
This function takes a name and prints a greeting message.
"""
print(f"Hello, {name}!")
# Accessing the function's docstring
print(greet.__doc__) # Output: This function takes a name and prints a greeting message.
By using greet.__doc__
, you can access the documentation string of the function.
9. Recursion in Functions
Recursion is a programming technique where a function calls itself. It’s often used in problems that can be broken down into smaller subproblems. Here’s an example of a recursive function to calculate the factorial of a number:
# Recursive function to calculate factorial
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Calling the recursive function
result = factorial(5)
print(result) # Output: 120
The function factorial
calls itself with a smaller value of n
until it reaches the base case, n == 0
.
Conclusion
Functions are one of the core building blocks of Python. They allow you to break down complex problems into smaller, manageable pieces. By defining functions, you can make your code more modular, reusable, and maintainable. Additionally, understanding function scope, recursion, and lambda functions helps you write more efficient and organized code.
Function Arguments: Positional, Keyword, and Default
In Python, functions can take different types of arguments. Understanding the types of arguments is crucial for writing flexible and reusable functions. The three main types of arguments are positional arguments, keyword arguments, and default arguments.
1. Positional Arguments
Positional arguments are the most common type of function arguments. When calling a function, you pass values in the order that they are defined in the function signature. The values you pass are assigned to the corresponding parameters in the function definition based on their positions:
# Example of positional arguments
def greet(name, age):
print(f"Hello, {name}! You are {age} years old.")
# Calling the function with positional arguments
greet("Alice", 30) # Output: Hello, Alice! You are 30 years old.
In this example, name
and age
are positional arguments. The value "Alice"
is assigned to name
, and 30
is assigned to age
, based on their positions.
2. Keyword Arguments
Keyword arguments allow you to specify arguments by name when calling a function, rather than relying on their position. This makes the function call more readable and allows you to pass arguments in any order:
# Example of keyword arguments
def greet(name, age):
print(f"Hello, {name}! You are {age} years old.")
# Calling the function with keyword arguments
greet(age=30, name="Alice") # Output: Hello, Alice! You are 30 years old.
In this case, the arguments age=30
and name="Alice"
are passed as keyword arguments. You can pass them in any order, and the function will correctly map the values to the parameters based on their names.
3. Default Arguments
Default arguments are parameters that have a predefined value. If a value is not passed for a parameter with a default, the function will use the default value instead. Default arguments must be placed after non-default arguments in the function signature:
# Example of default arguments
def greet(name, age=25):
print(f"Hello, {name}! You are {age} years old.")
# Calling the function with one argument
greet("Alice") # Output: Hello, Alice! You are 25 years old.
# Calling the function with both arguments
greet("Bob", 30) # Output: Hello, Bob! You are 30 years old.
In this example, the parameter age
has a default value of 25
. When calling greet("Alice")
, the default value is used because no age is provided. When calling greet("Bob", 30)
, the passed argument 30
overrides the default value.
4. Combining Positional, Keyword, and Default Arguments
You can combine positional, keyword, and default arguments in a function call. However, positional arguments must come before keyword arguments, and keyword arguments must come after positional arguments:
# Example of combining all types of arguments
def greet(name, age=25, city="Unknown"):
print(f"Hello, {name}! You are {age} years old and live in {city}.")
# Calling the function with mixed arguments
greet("Alice", 30, city="New York") # Output: Hello, Alice! You are 30 years old and live in New York.
In this case, name
is a positional argument, age
is a default argument (using the default value if not provided), and city
is a keyword argument (passed by name).
5. Variable-Length Arguments
Python also allows you to pass a variable number of arguments to a function using *args
(for positional arguments) and **kwargs
(for keyword arguments). This provides flexibility in handling a dynamic number of arguments:
# Example of *args (variable-length positional arguments)
def greet(*names):
for name in names:
print(f"Hello, {name}!")
greet("Alice", "Bob", "Charlie") # Output: Hello, Alice! Hello, Bob! Hello, Charlie!
# Example of **kwargs (variable-length keyword arguments)
def greet(**people):
for name, age in people.items():
print(f"Hello, {name}! You are {age} years old.")
greet(Alice=30, Bob=25) # Output: Hello, Alice! You are 30 years old. Hello, Bob! You are 25 years old.
*args
collects additional positional arguments as a tuple, and **kwargs
collects additional keyword arguments as a dictionary.
6. Function Call Order
When calling a function, follow this order of arguments:
- Positional arguments
- Keyword arguments
- Default arguments
Remember that keyword arguments can be passed in any order, but positional arguments must appear first.
7. Conclusion
Understanding the different types of function arguments is essential for writing flexible and efficient Python code. By using positional, keyword, and default arguments appropriately, you can make your functions more readable and reusable. Additionally, variable-length arguments allow you to handle dynamic input in your functions.
Lambda Functions
In Python, a lambda function is a small, anonymous function defined using the lambda
keyword. Unlike regular functions that are defined with the def
keyword, lambda functions can have any number of input parameters but can only have a single expression. They are often used for short-term, simple operations and can be passed as arguments to higher-order functions like map()
, filter()
, and reduce()
.
Syntax of Lambda Functions
The syntax for a lambda function is:
lambda arguments: expression
Where:
- arguments are the parameters you pass into the function.
- expression is a single expression that is evaluated and returned by the function.
Example: Basic Lambda Function
Let’s look at a simple example of a lambda function that adds two numbers:
# Regular function
def add(x, y):
return x + y
# Lambda function equivalent
add_lambda = lambda x, y: x + y
# Using both functions
print(add(5, 3)) # Output: 8
print(add_lambda(5, 3)) # Output: 8
In this example, the lambda function add_lambda
behaves the same as the regular function add
, but it is defined in a single line.
Using Lambda Functions with Higher-Order Functions
Lambda functions are commonly used with functions like map()
, filter()
, and reduce()
for applying an operation to a sequence of elements.
1. Using map()
with Lambda
map()
applies a function to all items in an input list (or any iterable). It returns a map object, which is an iterator, so you need to convert it to a list or another collection type to view the results.
# Using map() with a lambda function
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(lambda x: x ** 2, numbers)
# Converting map object to list
print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25]
2. Using filter()
with Lambda
filter()
filters elements from an iterable based on a function’s return value (True or False). The lambda function is applied to each item in the iterable, and only the items where the lambda returns True
are kept.
# Using filter() with a lambda function
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
# Converting filter object to list
print(list(even_numbers)) # Output: [2, 4, 6]
3. Using reduce()
with Lambda
reduce()
(from the functools
module) applies a binary function cumulatively to the items in an iterable, from left to right, to reduce the iterable to a single value.
# Using reduce() with a lambda function
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
print(product) # Output: 120 (1 * 2 * 3 * 4 * 5)
Advantages of Lambda Functions
- Concise Code: Lambda functions are useful for writing small, one-off functions in a single line, which reduces the lines of code.
- Functional Programming: They are a key feature of functional programming in Python, allowing you to pass functions as arguments and return them from other functions.
- Readability: When used in the right context, lambda functions can make code more readable and expressive by eliminating the need for a full function definition for simple operations.
Disadvantages of Lambda Functions
- Limited Functionality: Since lambda functions can only contain a single expression, they are not suitable for complex operations. For more intricate logic, regular functions defined with
def
should be used. - Hard to Debug: Lambda functions can be harder to debug, especially when they are used inside other functions or expressions.
Conclusion
Lambda functions in Python are a convenient and concise way to define simple functions. They are often used in functional programming paradigms and are especially useful when working with higher-order functions like map()
, filter()
, and reduce()
. While lambda functions offer many advantages, it's important to use them in appropriate situations and avoid overly complex lambda expressions.
Python Modules and Import Statement
In Python, modules are files that contain Python code. They can include functions, classes, and variables that allow you to organize your code and reuse it across multiple programs. The import statement is used to include these modules in your current program, making their functionality available for use.
What is a Python Module?
A Python module is simply a file containing Python code. The file must have a .py
extension. For example, if you have a file named math_operations.py
containing functions for addition, subtraction, and multiplication, it is considered a Python module.
Creating a Python Module
To create a Python module, simply write your Python code and save it in a file with a .py
extension. Here’s an example of a simple module:
# math_operations.py
def add(x, y):
return x + y
def subtract(x, y):
return x - y
Importing a Module
To use the functions or variables defined in a module, you need to import the module into your script. You can do this using the import
statement:
# Importing the entire module
import math_operations
# Using functions from the module
result1 = math_operations.add(5, 3)
result2 = math_operations.subtract(5, 3)
print(result1) # Output: 8
print(result2) # Output: 2
You can also import specific functions from a module using the from
keyword:
# Importing specific functions
from math_operations import add
# Using the imported function directly
result = add(5, 3)
print(result) # Output: 8
Importing with Aliases
If a module or function name is long, you can assign an alias to it using the as
keyword, making it easier to reference in your code:
# Importing a module with an alias
import math_operations as mo
result1 = mo.add(5, 3)
result2 = mo.subtract(5, 3)
print(result1) # Output: 8
print(result2) # Output: 2
Importing All Functions from a Module
If you want to import all functions and variables from a module directly into your script’s namespace, you can use the *
symbol:
# Importing all functions from the module
from math_operations import *
result1 = add(5, 3)
result2 = subtract(5, 3)
print(result1) # Output: 8
print(result2) # Output: 2
Built-in Python Modules
Python comes with a wide range of built-in modules that you can use in your programs. Some of the most commonly used built-in modules include:
- math: Provides mathematical functions like
sqrt()
,sin()
,log()
, etc. - datetime: Used for working with dates and times.
- random: Generates random numbers and selections.
- os: Provides functions for interacting with the operating system, such as file manipulation.
- sys: Provides access to system-specific parameters and functions.
Example: Using Built-in Modules
Here’s an example of using the math
module to calculate the square root of a number:
# Using the math module
import math
number = 16
sqrt_value = math.sqrt(number)
print(sqrt_value) # Output: 4.0
Creating Your Own Package
A Python package is a collection of Python modules that are organized in directories. To create a package, you need to create a directory with an __init__.py
file inside it. Here’s an example:
# Directory structure:
# mypackage/
# ├── __init__.py
# ├── module1.py
# └── module2.py
Inside the __init__.py
file, you can import specific modules from the package:
# mypackage/__init__.py
from .module1 import function1
from .module2 import function2
Now, you can import the package and use its functions:
# Importing from the package
import mypackage
mypackage.function1()
mypackage.function2()
Relative Imports in Packages
Inside a package, you can use relative imports to import modules from other modules within the same package. Here’s an example:
# Inside mypackage/module1.py
from .module2 import function2
Conclusion
Python modules and the import
statement allow you to organize your code and make use of external libraries and built-in functionalities. By creating reusable modules and packages, you can structure your projects more efficiently, making your code cleaner and easier to maintain.
Writing Your Own Python Modules
Python modules allow you to organize your code into manageable pieces, which can be reused across multiple projects. Writing your own Python modules is a great way to structure your code and promote reusability. A module is simply a file containing Python code, and it can define functions, classes, and variables that you want to use in other Python programs.
What is a Python Module?
A Python module is a Python file with a .py
extension. For example, a file named math_operations.py
is a Python module. You can define functions and variables in a module, and then use them in other Python files by importing that module.
Steps to Write Your Own Python Module
- Create a Python file: Write your functions, classes, or variables in a Python file with a
.py
extension. For example,math_operations.py
. - Save the Python file: Store the Python file in a directory where you can easily access it or place it in a folder that you want to use as a module package.
- Import the module: Once you have created the module, you can import it into another Python file and use its functions, classes, or variables.
Example: Writing a Simple Module
Let’s create a simple module that contains two functions: one for adding numbers and another for subtracting them. Save this code in a file called math_operations.py
:
# math_operations.py
def add(x, y):
return x + y
def subtract(x, y):
return x - y
Now, this module contains two functions, add
and subtract
. You can import this module into another Python file and use these functions.
Importing Your Custom Module
Once the module is created, you can import and use it in other Python files. Here’s how you can import and use the functions from the math_operations.py
module:
# importing the entire module
import math_operations
result1 = math_operations.add(5, 3)
result2 = math_operations.subtract(5, 3)
print(result1) # Output: 8
print(result2) # Output: 2
Alternatively, you can import specific functions from the module:
# importing specific functions
from math_operations import add
result = add(5, 3)
print(result) # Output: 8
Directory Structure for Modules
If you plan to create multiple Python files for a module, it’s a good practice to organize them in a directory. For example, you can create a directory called mymathmodule
to store your related files:
# Directory structure:
# mymathmodule/
# ├── __init__.py
# ├── math_operations.py
# └── advanced_math.py
Using the __init__.py
File
The __init__.py
file is used to mark a directory as a Python package. If you have multiple modules in a directory, you can include this file to allow Python to recognize the directory as a module package. The __init__.py
file can also include import statements that make the modules in the directory easier to use.
# mymathmodule/__init__.py
from .math_operations import add, subtract
from .advanced_math import multiply, divide
Now, you can import the package and access the functions directly:
# importing from the package
import mymathmodule
result1 = mymathmodule.add(5, 3)
result2 = mymathmodule.subtract(5, 3)
print(result1) # Output: 8
print(result2) # Output: 2
Using Your Custom Module in Other Projects
Once you have written your custom module, you can use it in any other project by importing it. If you are working on multiple projects, you can install your custom module using pip
or simply copy the module file to the project directory where it’s required.
Conclusion
Writing your own Python modules is a great way to organize your code and make it reusable. By creating Python modules, you can break your code into smaller, more manageable pieces, improve code readability, and make your code easier to maintain. Whether you're writing a small utility or a larger package, Python modules help you structure your code effectively and efficiently.
Introduction to Classes and Objects
In Python, object-oriented programming (OOP) is a programming paradigm that is based on the concept of objects. These objects represent data and the methods that operate on them. A class is a blueprint for creating objects, and an object is an instance of a class.
What is a Class?
A class is a template or blueprint for creating objects. It defines a set of attributes (variables) and behaviors (methods) that its objects can have. Classes allow for code organization and reuse, as you can define attributes and methods once and create multiple objects (instances) from it.
What is an Object?
An object is an instance of a class. It contains the attributes and methods defined in the class. Objects are created by calling the class as if it were a function.
Defining a Class
To define a class in Python, you use the class
keyword followed by the class name. The class body is indented, and you can define attributes and methods inside it. Here's an example:
# Defining a simple class named 'Car'
class Car:
# Constructor (initializer) method
def __init__(self, make, model, year):
self.make = make # Instance variable
self.model = model
self.year = year
# Method to display car details
def display_info(self):
print(f"{self.year} {self.make} {self.model}")
# Creating an object (instance) of the Car class
my_car = Car("Toyota", "Corolla", 2020)
# Calling the display_info method on the object
my_car.display_info() # Output: 2020 Toyota Corolla
Class Constructor: __init__
The __init__
method is a special method called a constructor. It is automatically called when a new object is created from a class. The constructor is used to initialize the object's attributes. In the example above, the __init__
method takes three arguments: make
, model
, and year
, and assigns them to the instance variables using the self
keyword.
Creating Objects from a Class
Once a class is defined, you can create objects (instances) of that class. You create an object by calling the class like a function, passing any required arguments to the __init__
method:
# Creating objects of the Car class
car1 = Car("Honda", "Civic", 2019)
car2 = Car("Ford", "Mustang", 2021)
# Calling methods on the objects
car1.display_info() # Output: 2019 Honda Civic
car2.display_info() # Output: 2021 Ford Mustang
Instance Variables and Methods
Each object can have its own set of instance variables. These variables are specific to each instance of the class. You can access and modify these variables using the self
keyword within the class methods. For example:
# Modifying an instance variable
car1.year = 2020
# Displaying updated car details
car1.display_info() # Output: 2020 Honda Civic
Accessing Class Variables
In addition to instance variables, a class can also have class variables. These are shared across all instances of the class. You can define class variables directly inside the class but outside any methods:
# Defining a class variable
class Car:
wheels = 4 # Class variable
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f"{self.year} {self.make} {self.model}, Wheels: {Car.wheels}")
# Creating objects
car1 = Car("Honda", "Civic", 2019)
car2 = Car("Ford", "Mustang", 2021)
# Accessing class variable
car1.display_info() # Output: 2019 Honda Civic, Wheels: 4
car2.display_info() # Output: 2021 Ford Mustang, Wheels: 4
Inheritance in OOP
One of the key concepts in OOP is inheritance. Inheritance allows a new class to inherit the attributes and methods of an existing class. This promotes reusability and allows you to extend existing classes with new functionality.
# Inheriting from the Car class to create an ElectricCar class
class ElectricCar(Car):
def __init__(self, make, model, year, battery_size):
super().__init__(make, model, year) # Calling the constructor of the parent class
self.battery_size = battery_size
def display_info(self):
super().display_info() # Calling the method from the parent class
print(f"Battery size: {self.battery_size} kWh")
# Creating an object of the ElectricCar class
my_electric_car = ElectricCar("Tesla", "Model S", 2022, 75)
# Calling the display_info method of the ElectricCar object
my_electric_car.display_info()
# Output:
# 2022 Tesla Model S
# Battery size: 75 kWh
Conclusion
Classes and objects are foundational concepts in Python and many other programming languages that support object-oriented programming (OOP). Classes provide a way to model real-world entities, while objects represent instances of those entities. By using classes and objects, you can create well-structured, reusable, and maintainable code. As you get more comfortable with Python, you’ll find yourself using OOP concepts like inheritance, polymorphism, and encapsulation to solve more complex problems.
Attributes and Methods
In Python, classes and objects have two key components: attributes and methods. Understanding how to define and use both is essential for object-oriented programming (OOP). In this section, we'll explore attributes and methods in detail.
What are Attributes?
Attributes are variables that are associated with a class or an object. They represent the data or properties of the object. There are two types of attributes in Python:
- Instance Attributes: These are attributes that are specific to an instance (object) of a class. They are defined within methods, typically in the
__init__
constructor, using theself
keyword. - Class Attributes: These are attributes that are shared across all instances of the class. They are defined directly within the class and are not tied to any particular object.
Defining Instance Attributes
Instance attributes are created inside the __init__
method using the self
keyword. Each object can have its own set of instance attributes. Here's an example:
# Defining a class with instance attributes
class Dog:
def __init__(self, name, breed, age):
self.name = name # Instance attribute
self.breed = breed # Instance attribute
self.age = age # Instance attribute
# Creating an object of the Dog class
dog1 = Dog("Buddy", "Golden Retriever", 3)
dog2 = Dog("Charlie", "Beagle", 2)
# Accessing the instance attributes
print(dog1.name) # Output: Buddy
print(dog2.age) # Output: 2
Defining Class Attributes
Class attributes are defined within the class but outside the methods. They are shared by all instances of the class. Here's an example:
# Defining a class with class attributes
class Dog:
species = "Canis lupus familiaris" # Class attribute
def __init__(self, name, breed, age):
self.name = name
self.breed = breed
self.age = age
# Creating objects of the Dog class
dog1 = Dog("Buddy", "Golden Retriever", 3)
dog2 = Dog("Charlie", "Beagle", 2)
# Accessing the class attribute
print(dog1.species) # Output: Canis lupus familiaris
print(dog2.species) # Output: Canis lupus familiaris
What are Methods?
Methods are functions that belong to a class and are used to perform operations on objects or modify their state. Methods are defined within a class and can access the class's attributes. There are two types of methods in Python:
- Instance Methods: These methods operate on instance attributes and are typically used to modify or retrieve the data of a specific object.
- Class Methods: These methods operate on class attributes and are used to perform operations that affect the entire class, not just individual objects.
Defining Instance Methods
Instance methods are defined like regular functions, but they include the self
parameter as the first argument. This allows the method to access and modify the instance attributes. Here's an example:
# Defining a class with instance methods
class Dog:
def __init__(self, name, breed, age):
self.name = name
self.breed = breed
self.age = age
def bark(self):
print(f"{self.name} says Woof!")
def birthday(self):
self.age += 1
print(f"Happy Birthday, {self.name}! You are now {self.age} years old.")
# Creating an object of the Dog class
dog1 = Dog("Buddy", "Golden Retriever", 3)
# Calling instance methods
dog1.bark() # Output: Buddy says Woof!
dog1.birthday() # Output: Happy Birthday, Buddy! You are now 4 years old.
Defining Class Methods
Class methods are defined using the @classmethod
decorator and take cls
as the first parameter instead of self
. This allows class methods to access and modify class-level attributes. Here's an example:
# Defining a class with a class method
class Dog:
species = "Canis lupus familiaris" # Class attribute
def __init__(self, name, breed, age):
self.name = name
self.breed = breed
self.age = age
@classmethod
def get_species(cls):
print(f"The species of all dogs is: {cls.species}")
# Calling a class method
Dog.get_species() # Output: The species of all dogs is: Canis lupus familiaris
Modifying Attributes Using Methods
Methods can be used to modify both instance and class attributes. Here's an example of modifying instance attributes using methods:
# Modifying instance attributes through a method
class Dog:
def __init__(self, name, breed, age):
self.name = name
self.breed = breed
self.age = age
def change_name(self, new_name):
self.name = new_name
# Creating an object of the Dog class
dog1 = Dog("Buddy", "Golden Retriever", 3)
# Modifying the name of the dog using the method
dog1.change_name("Max")
print(dog1.name) # Output: Max
Conclusion
Attributes and methods are fundamental to object-oriented programming in Python. Attributes hold the data associated with an object, and methods define the behavior or operations that can be performed on that data. By using attributes and methods, you can organize your code in a way that makes it more modular, reusable, and easier to maintain. As you continue learning Python, you'll encounter more advanced techniques for working with classes, attributes, and methods that will help you create more complex and powerful programs.
Constructor and Destructor in Python
In Python, constructors and destructors are special methods that are automatically invoked when an object is created or destroyed. These methods are used to initialize object attributes and perform cleanup tasks when an object is no longer in use. Let's explore both constructors and destructors in detail.
What is a Constructor?
A constructor is a special method that is automatically called when a new object of a class is created. It is used to initialize the object's attributes and perform any setup required for the object. In Python, the constructor method is called __init__
.
Syntax of Constructor
The __init__
method takes at least one argument: self
. Other arguments can be passed when creating the object. The __init__
method is executed when the object is instantiated, and it allows you to set the initial state of the object.
# Defining a class with a constructor
class Car:
def __init__(self, make, model, year):
self.make = make # Instance attribute
self.model = model # Instance attribute
self.year = year # Instance attribute
# Creating an object of the Car class
car1 = Car("Toyota", "Corolla", 2020)
# Accessing object attributes
print(car1.make) # Output: Toyota
print(car1.model) # Output: Corolla
print(car1.year) # Output: 2020
In this example, when the Car
object is created, the constructor __init__
is called, and the attributes make
, model
, and year
are initialized with the given values.
What is a Destructor?
A destructor is a special method that is automatically called when an object is about to be destroyed, i.e., when it is no longer in use or when the program ends. The destructor method in Python is called __del__
.
Syntax of Destructor
The __del__
method is usually used to perform cleanup activities, like closing files or releasing resources, before the object is destroyed. However, Python handles memory management automatically using garbage collection, so you rarely need to manually define a destructor.
# Defining a class with a destructor
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def __del__(self):
print(f"The car {self.make} {self.model} is being deleted.")
# Creating an object of the Car class
car1 = Car("Honda", "Civic", 2021)
# Deleting the object explicitly
del car1 # Output: The car Honda Civic is being deleted.
In this example, the __del__
method is called when the car1
object is deleted using the del
statement. The destructor prints a message before the object is destroyed.
Constructor vs Destructor
Here’s a quick comparison between constructors and destructors:
Aspect | Constructor | Destructor |
---|---|---|
Purpose | Initialize the object and set its attributes | Clean up the object and free resources |
Method Name | __init__ |
__del__ |
Invocation | Automatically called when an object is created | Automatically called when an object is about to be destroyed |
Usage | Used to initialize attributes and allocate resources | Used to release resources and perform cleanup tasks |
When to Use Destructor?
In Python, you generally don’t need to use destructors unless you're working with resources that need to be explicitly released, such as file handles or network connections. Python’s garbage collector handles memory management, so destructors are rarely required. However, they can be helpful when managing external resources or cleaning up before an object is deleted.
Constructor and Destructor in Action
Let’s see an example where both the constructor and destructor are used for managing resources such as file handling:
# Defining a class with both constructor and destructor
class FileHandler:
def __init__(self, filename):
self.filename = filename
self.file = open(filename, "w") # Opening a file for writing
def __del__(self):
self.file.close() # Closing the file when the object is destroyed
print(f"File {self.filename} has been closed.")
# Creating an object of the FileHandler class
file1 = FileHandler("sample.txt")
file1.file.write("Hello, World!") # Writing to the file
# When the object is deleted, the destructor is called
del file1 # Output: File sample.txt has been closed.
In this example, the constructor __init__
opens a file, and the destructor __del__
ensures the file is closed when the object is destroyed, freeing up the resource.
Conclusion
Constructors and destructors are fundamental in object-oriented programming. They allow you to set up and clean up objects as they are created and destroyed. While constructors are typically used to initialize object attributes, destructors handle resource cleanup. In Python, constructors are essential for setting up objects, while destructors are less commonly used because of Python's automatic garbage collection system.
Inheritance and Polymorphism
Inheritance and polymorphism are two key concepts in object-oriented programming (OOP). These concepts allow objects to inherit properties and methods from other objects and enable a single interface to be used for different types of data. Let's dive into both concepts.
What is Inheritance?
Inheritance is a mechanism in OOP that allows a new class (called the child class) to inherit the properties and behaviors (methods) from an existing class (called the parent class). This allows for code reuse and the creation of more specialized classes based on general ones.
Syntax of Inheritance
In Python, inheritance is implemented by passing the parent class as an argument to the child class during class definition. The child class can then access the methods and attributes of the parent class.
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} makes a sound.")
# Child class inheriting from the Animal class
class Dog(Animal):
def speak(self):
print(f"{self.name} barks.")
# Creating objects
animal = Animal("Generic Animal")
dog = Dog("Buddy")
# Calling methods
animal.speak() # Output: Generic Animal makes a sound.
dog.speak() # Output: Buddy barks.
In this example, the class Dog
inherits from the class Animal
. The Dog
class overrides the speak
method to provide a specific implementation, while still inheriting the name
attribute from the Animal
class.
What is Polymorphism?
Polymorphism is the ability of different classes to respond to the same method or function call in different ways. In Python, this is achieved through method overriding, where a child class provides a specific implementation of a method defined in the parent class.
Types of Polymorphism
There are two types of polymorphism in Python:
- Method Overriding: A child class provides its own implementation of a method that is already defined in the parent class.
- Method Overloading: Not directly supported in Python, but can be simulated by using default arguments or variable-length arguments in methods.
Method Overriding Example
In the example above, method overriding is demonstrated where the speak
method is overridden in the Dog
class. Let’s extend this example to show polymorphism in action with multiple animal types:
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} makes a sound.")
# Child classes
class Dog(Animal):
def speak(self):
print(f"{self.name} barks.")
class Cat(Animal):
def speak(self):
print(f"{self.name} meows.")
# Function to demonstrate polymorphism
def animal_sound(animal):
animal.speak()
# Creating objects
dog = Dog("Buddy")
cat = Cat("Whiskers")
# Calling polymorphic function
animal_sound(dog) # Output: Buddy barks.
animal_sound(cat) # Output: Whiskers meows.
In this example, the function animal_sound
demonstrates polymorphism by calling the speak
method on both Dog
and Cat
objects. Each object responds differently, based on its own implementation of the speak
method.
Advantages of Inheritance and Polymorphism
Here are some key advantages of using inheritance and polymorphism:
- Code Reusability: Inheritance allows code from the parent class to be reused in the child class, reducing duplication.
- Extensibility: New classes can be created by extending existing ones, making it easy to add new features and maintain code.
- Flexibility: Polymorphism provides flexibility in handling different object types using the same interface.
- Maintainability: By using inheritance and polymorphism, you can organize and structure your code in a way that is easier to maintain and extend.
Constructor in Inheritance
In inheritance, the constructor of the parent class is not automatically called in the child class. If the child class needs to initialize attributes from the parent class, you must explicitly call the parent class’s constructor using super()
.
# Parent class
class Animal:
def __init__(self, name):
self.name = name
# Child class
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name) # Calling the parent class constructor
self.breed = breed
# Creating an object of the Dog class
dog = Dog("Buddy", "Golden Retriever")
print(dog.name) # Output: Buddy
print(dog.breed) # Output: Golden Retriever
In this example, the child class Dog
calls the parent class's constructor using super().__init__(name)
to initialize the name
attribute.
Conclusion
Inheritance and polymorphism are powerful features of object-oriented programming that make it easier to reuse and extend code. Inheritance allows classes to inherit attributes and methods from other classes, while polymorphism enables different classes to respond to the same method call in their own way. Together, these concepts improve code organization, flexibility, and maintainability in Python programs.
Encapsulation and Abstraction
Encapsulation and abstraction are fundamental principles of object-oriented programming (OOP) that help in designing systems that are modular, easy to maintain, and more secure. These principles hide unnecessary details from the user and provide a clean interface for interaction. Let’s dive into both concepts.
What is Encapsulation?
Encapsulation is the concept of bundling data and the methods that operate on that data within a single unit, typically a class. It restricts direct access to some of an object's components and provides controlled access through methods. This is done to protect the internal state of the object and ensure that the data can only be accessed or modified in a predefined way.
Encapsulation in Python
In Python, encapsulation is implemented by defining attributes as private or protected using naming conventions and providing public methods (getters and setters) to access and modify these private attributes. By using this approach, the internal state of the object is hidden from the outside world, and the object’s behavior is controlled.
Private and Protected Attributes
In Python, attributes that are intended to be private are prefixed with a double underscore (e.g., __private_attr
). Protected attributes are prefixed with a single underscore (e.g., _protected_attr
), which is a convention rather than a restriction.
# Encapsulation Example
class Employee:
def __init__(self, name, salary):
self.name = name
self.__salary = salary # private attribute
# Getter method for salary
def get_salary(self):
return self.__salary
# Setter method for salary
def set_salary(self, salary):
if salary > 0:
self.__salary = salary
else:
print("Salary must be positive.")
# Creating an object of Employee
employee = Employee("John", 5000)
# Accessing private attribute using getter method
print(employee.get_salary()) # Output: 5000
# Modifying private attribute using setter method
employee.set_salary(5500)
print(employee.get_salary()) # Output: 5500
In this example, the salary attribute is private and can only be accessed or modified through the getter and setter methods. This ensures that the salary cannot be set to a negative value, thereby protecting the integrity of the data.
What is Abstraction?
Abstraction is the concept of hiding the complex implementation details of a system and exposing only the essential features to the user. It allows the user to interact with an object at a higher level, without needing to understand the underlying complexities.
Abstraction in Python
In Python, abstraction can be achieved using abstract classes and methods. An abstract class is a class that cannot be instantiated directly, and it contains one or more abstract methods. These abstract methods are defined without implementation and must be implemented by any subclass that inherits from the abstract class.
Using the abc Module
Python provides the abc
module to create abstract classes. The ABC
class and abstractmethod
decorator are used to define an abstract class and its abstract methods.
# Abstraction Example
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
class Square(Shape):
def __init__(self, side):
self.side = side
def area(self):
return self.side * self.side
# Creating objects
circle = Circle(5)
square = Square(4)
# Calling the abstract method implemented in subclasses
print(circle.area()) # Output: 78.5
print(square.area()) # Output: 16
In this example, the Shape
class is abstract and contains an abstract method area
. Both the Circle
and Square
classes inherit from Shape
and provide their own implementation of the area
method. This allows the user to interact with different shapes without worrying about the specific implementation of each one.
Advantages of Encapsulation and Abstraction
- Data Security: Encapsulation allows data to be protected from unauthorized access and modification.
- Code Reusability: Abstraction allows for reusable code by defining common behaviors in abstract classes, which can be implemented by different subclasses.
- Maintenance: Both encapsulation and abstraction make the code easier to maintain by separating implementation details from the user interface.
- Modularity: Encapsulation and abstraction promote modular design by defining clear interfaces and hiding complex logic.
Encapsulation vs Abstraction
While both encapsulation and abstraction aim to hide details and simplify interaction with objects, they differ in the following ways:
- Encapsulation: Focuses on bundling data and methods that operate on that data into a single unit (class) and restricting access to the internal state. It’s about hiding the internal details of how data is stored and modified.
- Abstraction: Focuses on hiding the complex implementation details and exposing only the necessary features to the user. It’s about hiding the complexities of the system and providing a simple interface.
Conclusion
Encapsulation and abstraction are essential principles in object-oriented programming that promote code security, reusability, and maintainability. Encapsulation ensures that data is safely accessed and modified only through specific methods, while abstraction hides implementation details and exposes only the essential features of a class. Together, these concepts enable the creation of well-structured and modular Python programs.
Reading and Writing Files (.txt, .csv, etc.)
Working with files is a crucial part of many Python programs. Python provides built-in functions for reading from and writing to various types of files such as text files (.txt) and CSV files (.csv). This section covers how to read and write data to files using Python.
Reading Text Files
To read a text file in Python, you can use the open()
function and specify the mode as 'r'
(read mode). After opening the file, you can read its contents using methods such as read()
, readline()
, or readlines()
.
# Reading a text file
with open('example.txt', 'r') as file:
content = file.read() # Reads the entire file
print(content)
The with
statement ensures the file is properly closed after reading, even if an error occurs. The read()
method reads the entire content of the file into a string.
Writing to Text Files
To write to a text file, you can use the open()
function with the 'w'
mode for writing. If the file doesn’t exist, it will be created. If it does exist, its content will be overwritten.
# Writing to a text file
with open('example.txt', 'w') as file:
file.write('Hello, World!\nThis is a new line.')
In this example, the content is written to the file, and if the file already exists, it will be overwritten with the new content. If you want to append to an existing file, you can use the 'a'
mode.
Reading CSV Files
CSV (Comma Separated Values) files are commonly used for storing tabular data. Python’s csv
module provides functionality to read from and write to CSV files.
Reading a CSV File
To read a CSV file, you can use the csv.reader()
method, which allows you to iterate over each row of the file.
# Reading a CSV file
import csv
with open('data.csv', 'r') as file:
csv_reader = csv.reader(file)
for row in csv_reader:
print(row) # Prints each row of the CSV file
This will print each row in the CSV file as a list of values. You can customize the behavior by passing additional parameters to the csv.reader()
function, such as specifying a delimiter if it's not a comma.
Writing to a CSV File
To write data to a CSV file, you can use the csv.writer()
method to create a writer object and use the writerow()
or writerows()
methods to write data to the file.
# Writing to a CSV file
import csv
data = [['Name', 'Age'], ['Alice', 25], ['Bob', 30]]
with open('data.csv', 'w', newline='') as file:
csv_writer = csv.writer(file)
csv_writer.writerows(data) # Writes multiple rows
In this example, we write a list of lists to a CSV file. The newline=''
argument is passed to prevent extra blank lines from being inserted between rows on Windows.
Handling File Paths
When working with files, it’s essential to provide the correct file path. If the file is in the current working directory, you can just specify the filename. However, if the file is in a different location, provide the full path.
# Example of full file path
file_path = 'C:/Users/YourName/Documents/example.txt'
with open(file_path, 'r') as file:
content = file.read()
print(content)
Alternatively, you can use the os.path
module to handle file paths in a more platform-independent manner.
Working with Binary Files
Python can also handle binary files, such as images or audio files, by opening them in binary mode ('b
'). You can use the read()
and write()
methods to read and write binary data.
# Reading a binary file (e.g., an image)
with open('image.png', 'rb') as file:
data = file.read()
# Writing to a binary file
with open('copy_image.png', 'wb') as file:
file.write(data)
File Handling Best Practices
- Always close files: Using the
with
statement automatically handles file closing. - Handle exceptions: Always handle exceptions, such as file not found or permission errors, when working with files.
- Use appropriate file modes: Use 'r' for reading, 'w' for writing (overwrite), 'a' for appending, and 'rb'/'wb' for binary files.
Conclusion
Reading and writing files is a fundamental task in Python. By using Python’s built-in functions and modules like open()
and csv
, you can easily work with various file formats such as text and CSV files. Whether you’re processing data, logging information, or handling binary data, Python provides the tools you need to efficiently manage file input and output.
File Modes: Read, Write, Append
When you open a file in Python, you need to specify the mode in which the file is opened. The mode determines whether you are reading from, writing to, or appending to the file. Python provides several modes that can be used with the open()
function to perform different file operations.
Read Mode ('r')
The 'r'
mode is used to open a file for reading. If the file doesn't exist, Python will raise a FileNotFoundError
.
# Opening a file in read mode
with open('example.txt', 'r') as file:
content = file.read() # Reads the entire file
print(content)
In this example, the file is opened for reading, and its content is printed to the console. You can also use readline()
or readlines()
for reading line by line.
Write Mode ('w')
The 'w'
mode is used to open a file for writing. If the file already exists, its contents will be overwritten. If the file doesn't exist, it will be created.
# Opening a file in write mode
with open('example.txt', 'w') as file:
file.write('Hello, World!') # Writes to the file, overwriting existing content
In this example, the file is opened in write mode, and the content "Hello, World!" is written to the file. If the file already contains data, it will be replaced with the new content.
Append Mode ('a')
The 'a'
mode is used to open a file for appending. If the file doesn't exist, it will be created. If the file exists, new content will be added to the end of the file without overwriting the existing data.
# Opening a file in append mode
with open('example.txt', 'a') as file:
file.write('\nAppended text!') # Adds new content at the end of the file
In this example, the text "Appended text!" is added to the end of the file, leaving the existing content intact. The \n
ensures that the new content is added on a new line.
Other File Modes
In addition to the basic file modes, Python also supports some other useful modes:
'r+'
: Read and write (file must exist)'w+'
: Write and read (creates file or overwrites existing content)'a+'
: Append and read (creates file if it doesn't exist)'b'
: Binary mode (used in combination with other modes for handling binary files)
Working with Binary Files
In addition to text files, you can also work with binary files (such as images or audio files). To do so, you need to open the file in binary mode by adding the 'b'
character to the mode. For example, to read and write binary files, you can use the 'rb'
and 'wb'
modes respectively.
# Reading a binary file
with open('image.png', 'rb') as file:
data = file.read()
# Writing to a binary file
with open('copy_image.png', 'wb') as file:
file.write(data)
In this case, the file is opened in binary read mode ('rb') to read the image and in binary write mode ('wb') to write the image data to another file.
Best Practices for File Modes
- Always choose the right mode: Use
'r'
for reading,'w'
for writing, and'a'
for appending. - Handle file not found errors: When using
'r'
mode, ensure the file exists to avoid errors. - Ensure proper closing: Always use the
with
statement to automatically close the file after the operation is complete. - Use binary mode for non-text files: When dealing with binary files, make sure to use the
'b'
mode (e.g.,'rb'
,'wb'
).
Conclusion
Understanding file modes is essential when working with files in Python. The read, write, and append modes provide flexibility in how data is managed in files. Always choose the appropriate mode based on your requirements to ensure smooth file operations and prevent data loss.
Working with Binary Files
Binary files contain data that is not human-readable, such as images, audio files, video files, and other non-text data. In Python, you can work with binary files by using the 'b'
mode when opening a file. This mode tells Python to treat the file's contents as raw binary data, ensuring the correct handling of non-text data.
Opening a Binary File
To open a binary file, you must include the 'b'
character in the file mode. For example, use 'rb'
for reading binary files and 'wb'
for writing binary files.
Reading Binary Files
When reading a binary file, you can use the read()
method to read the file's contents. This will return the binary data of the file as a byte object, which you can then manipulate as needed.
# Reading a binary file (e.g., an image)
with open('example_image.png', 'rb') as file:
binary_data = file.read() # Read the entire file's binary data
# You can process the binary_data as needed, for example, save it to another file
Writing to Binary Files
To write binary data to a file, open the file in 'wb'
mode (write binary mode). This mode is used to write raw bytes to the file. If the file doesn't exist, Python will create it; if it exists, it will be overwritten.
# Writing binary data to a new file (e.g., saving an image)
with open('copy_image.png', 'wb') as file:
file.write(binary_data) # Write the binary data to the file
Appending to Binary Files
If you want to append binary data to an existing binary file, use the 'ab'
mode. This will add new data to the end of the file without overwriting its existing content.
# Appending binary data to an existing file
with open('existing_image.png', 'ab') as file:
file.write(additional_binary_data) # Append new binary data
Working with Binary Data in Memory
In Python, you can manipulate binary data in memory using the bytearray
type. This allows you to modify binary data before writing it back to a file or sending it over the network.
# Creating a bytearray from binary data
binary_data = bytearray(binary_data)
# Modifying the binary data
binary_data[0] = 255 # Change the first byte
# Writing the modified binary data to a new file
with open('modified_image.png', 'wb') as file:
file.write(binary_data)
Working with Binary Files for Images
Binary files are commonly used for images. When working with images in Python, you can use libraries like PIL
(Python Imaging Library) or OpenCV
to load, manipulate, and save images in binary format.
# Using PIL to open, manipulate, and save an image
from PIL import Image
# Open an image
image = Image.open('example_image.png')
# Perform operations on the image (e.g., resize)
resized_image = image.resize((100, 100))
# Save the modified image to a new file in binary format
resized_image.save('resized_image.png')
Working with Binary Files for Audio and Video
For audio and video files, you can read or write raw binary data directly using the 'rb'
and 'wb'
modes. Additionally, specialized libraries such as pydub
(for audio) or opencv
(for video) allow for easier manipulation of these types of binary files.
# Example: Reading a binary audio file
from pydub import AudioSegment
audio = AudioSegment.from_file('example_audio.mp3', format='mp3')
# Perform audio manipulation (e.g., change volume)
audio = audio + 10 # Increase volume by 10 dB
# Export the manipulated audio to a new file
audio.export('modified_audio.mp3', format='mp3')
Best Practices for Working with Binary Files
- Always use the
'b'
mode: To handle binary files correctly, always include the'b'
in the mode (e.g.,'rb'
,'wb'
,'ab'
). - Be mindful of file size: Binary files can be large, so ensure you have enough memory if you're working with sizable files.
- Use suitable libraries: For complex operations on images, audio, or video, consider using specialized libraries like
PIL
,pydub
, oropencv
. - Binary data manipulation: Use
bytearray
to modify binary data in memory before saving it to a file.
Conclusion
Working with binary files in Python is straightforward with the correct file modes and methods. By using 'rb'
, 'wb'
, and 'ab'
modes, you can efficiently read, write, and append binary data. Specialized libraries make it easy to work with multimedia files like images, audio, and video. Always ensure you're using the appropriate tools for the task and managing binary data efficiently.
Using with Statement for File Handling
In Python, file handling is an essential skill. The with
statement is a powerful tool for managing files and ensures that files are properly closed after their use, even if an error occurs during their handling. This approach is known as a context manager and simplifies the process of dealing with external resources like files.
Why Use the with
Statement?
Using the with
statement is the recommended approach for file handling in Python because it automatically takes care of closing the file when the block of code is finished executing. This eliminates the need to explicitly call file.close()
.
Advantages of Using with
Statement
- Automatic Resource Management: Ensures that the file is closed properly, preventing potential memory leaks or file locks.
- Cleaner Code: Reduces the need for manually closing files and avoids errors caused by forgetting to call
close()
. - Error Handling: Even if an error occurs while working with the file, the
with
statement ensures that the file is closed correctly, avoiding resource wastage.
Basic Example of Using with
for File Reading
Here's a simple example demonstrating how to read a file using the with
statement:
# Using the with statement for reading a file
with open('example.txt', 'r') as file:
content = file.read() # Read the entire file's contents
print(content) # Print the file's contents
In this example:
open('example.txt', 'r')
opens the file in read mode.as file
assigns the opened file to the variablefile
.file.read()
reads the entire content of the file.- Once the block inside the
with
statement finishes executing, the file is automatically closed, even if there’s an exception.
Writing to a File with with
The with
statement can also be used for writing data to a file. The following example demonstrates how to write to a file:
# Using the with statement for writing to a file
with open('output.txt', 'w') as file:
file.write("Hello, this is a test!") # Write text to the file
In this example:
open('output.txt', 'w')
opens the file in write mode.file.write()
writes the specified text to the file.- If the file does not exist, it will be created automatically. If it exists, its content will be overwritten.
Appending to a File with with
If you want to append data to an existing file without overwriting it, you can use 'a'
mode. Here's an example:
# Using the with statement to append to a file
with open('output.txt', 'a') as file:
file.write("\nAppended text.") # Append text to the file
In this example:
open('output.txt', 'a')
opens the file in append mode, which allows new content to be added to the end of the file.file.write("\nAppended text.")
appends a new line with the specified text.
Working with Multiple Files Using with
You can also use the with
statement to work with multiple files. The syntax allows you to open several files simultaneously in a safe way:
# Using the with statement to handle multiple files
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2:
content1 = file1.read() # Read content from the first file
content2 = file2.read() # Read content from the second file
print(content1)
print(content2)
In this case:
- Two files are opened in read mode using a single
with
statement. - Both files are closed automatically when the block finishes executing.
Best Practices for Using with
Statement
- Always use
with
for file handling: This ensures that files are always closed properly, even in case of errors. - Use appropriate file modes: Choose the correct mode (read, write, append) based on the operations you want to perform on the file.
- Work with multiple files: If you're handling multiple files, use a single
with
statement for better resource management. - Handle exceptions if necessary: Although the
with
statement automatically handles file closure, you may still need to handle exceptions for specific use cases within the block.
Conclusion
The with
statement is an essential feature in Python for working with files, ensuring proper resource management and simplifying file handling. It eliminates the need for manually closing files and guarantees that files are always closed, even if an error occurs. By using the with
statement, you can write cleaner, more reliable code when dealing with file I/O operations.
Standard Libraries (e.g., math, datetime, os, random)
Python comes with a rich set of built-in libraries that provide essential functionality for many common tasks. These libraries are bundled with Python, so you don't need to install them separately. Some of the most commonly used standard libraries include math
, datetime
, os
, and random
. Here’s an overview of these libraries and examples of how to use them.
1. math Library
The math
library provides mathematical functions and constants. It includes operations such as trigonometric functions, logarithms, and constants like pi
and e
.
Common Functions in the math
Library
math.sqrt(x)
: Returns the square root ofx
.math.factorial(x)
: Returns the factorial ofx
.math.pow(x, y)
: Returnsx
raised to the power ofy
.math.pi
: The mathematical constant π.
Example Usage
# Using the math library
import math
# Calculate the square root of 16
sqrt_value = math.sqrt(16)
print(f"Square root of 16: {sqrt_value}")
# Calculate the factorial of 5
factorial_value = math.factorial(5)
print(f"Factorial of 5: {factorial_value}")
# Value of pi
print(f"Value of pi: {math.pi}")
2. datetime Library
The datetime
library is used for working with dates and times. It provides classes for manipulating dates and times, and for performing arithmetic on them.
Common Functions in the datetime
Library
datetime.datetime.now()
: Returns the current date and time.datetime.date.today()
: Returns the current date (year, month, day).datetime.timedelta(days=, hours=, minutes=, seconds=)
: Represents a duration of time.
Example Usage
# Using the datetime library
import datetime
# Get the current date and time
current_datetime = datetime.datetime.now()
print(f"Current date and time: {current_datetime}")
# Get today's date
today = datetime.date.today()
print(f"Today's date: {today}")
# Calculate a future date
future_date = today + datetime.timedelta(days=10)
print(f"Date after 10 days: {future_date}")
3. os Library
The os
library provides functions for interacting with the operating system. It allows you to perform tasks like file manipulation, directory operations, and interacting with environment variables.
Common Functions in the os
Library
os.getcwd()
: Returns the current working directory.os.listdir(path)
: Returns a list of files and directories in the specifiedpath
.os.mkdir(path)
: Creates a directory at the specifiedpath
.os.remove(path)
: Removes the file at the specifiedpath
.
Example Usage
# Using the os library
import os
# Get the current working directory
current_directory = os.getcwd()
print(f"Current working directory: {current_directory}")
# List files in the current directory
files = os.listdir()
print(f"Files in the current directory: {files}")
# Create a new directory
os.mkdir('new_directory')
print("New directory 'new_directory' created.")
4. random Library
The random
library provides functions for generating random numbers and selecting random items from sequences such as lists or tuples.
Common Functions in the random
Library
random.randint(a, b)
: Returns a random integer betweena
andb
, inclusive.random.choice(sequence)
: Returns a random element from the given sequence (list, tuple, etc.).random.shuffle(sequence)
: Shuffles the elements of the given sequence in place.random.uniform(a, b)
: Returns a random floating-point number betweena
andb
.
Example Usage
# Using the random library
import random
# Generate a random integer between 1 and 10
random_integer = random.randint(1, 10)
print(f"Random integer: {random_integer}")
# Select a random item from a list
colors = ['red', 'green', 'blue', 'yellow']
random_color = random.choice(colors)
print(f"Random color: {random_color}")
# Shuffle the list of colors
random.shuffle(colors)
print(f"Shuffled colors: {colors}")
Conclusion
Python's standard libraries provide a wealth of functionality that makes it easier to work with data, files, operating systems, and other essential tasks. The math
, datetime
, os
, and random
libraries are just the beginning. By learning how to use these libraries effectively, you can save time and effort when building Python applications.
Installing Libraries with pip
Python’s package manager pip
is a powerful tool for installing and managing third-party libraries and packages that are not part of Python's standard library. It simplifies the process of adding functionality to your Python projects, allowing you to install packages from the Python Package Index (PyPI).
What is pip?
pip
stands for "Pip Installs Packages" and is the default package manager for Python. It allows you to easily install, upgrade, and remove Python libraries. It is included automatically when you install Python, starting from Python 3.4 and later.
Installing a Library Using pip
To install a library using pip
, you can use the following command:
pip install library_name
For example, to install the popular web framework Flask
, you would run:
pip install flask
Installing Specific Versions
If you want to install a specific version of a library, you can specify the version number like this:
pip install library_name==version_number
For example, to install version 2.0.1 of the Flask
library:
pip install flask==2.0.1
Upgrading a Library
To upgrade an already installed library to the latest version, you can use the --upgrade
flag:
pip install --upgrade library_name
For example, to upgrade the Flask
library to the latest version:
pip install --upgrade flask
Installing Libraries from a Requirements File
If you are working on a project that has a requirements.txt
file (a list of dependencies), you can install all the libraries listed in the file using the following command:
pip install -r requirements.txt
This is useful when setting up a project on a new machine or sharing your project with others, ensuring that they have the same set of libraries installed.
Uninstalling Libraries
If you no longer need a library, you can uninstall it using the following command:
pip uninstall library_name
For example, to uninstall Flask
:
pip uninstall flask
Checking Installed Libraries
To view a list of all the libraries installed in your environment, you can run:
pip list
This will display all installed libraries along with their version numbers.
Checking for Outdated Libraries
You can check if any of your installed libraries are outdated by running:
pip list --outdated
This will show you which libraries have newer versions available for installation.
Conclusion
Using pip
is the most common way to install third-party libraries in Python. It allows you to easily manage dependencies in your projects, install specific versions, and ensure that your environment is up to date. By mastering pip
, you can efficiently add functionality and work with Python packages as you develop more complex projects.
Overview of Popular Libraries
Python boasts an extensive ecosystem of libraries that serve a wide variety of purposes. Here, we'll explore some of the most popular libraries that have become essential tools for Python developers:
1. NumPy (Numerical Computing)
NumPy is the fundamental package for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is fast and efficient, making it the go-to library for numerical computing in Python.
Key Features of NumPy:
- Efficient array operations.
- Support for multi-dimensional arrays and matrices.
- Mathematical and statistical functions for numerical data.
- Integration with other scientific libraries like SciPy, Matplotlib, and Pandas.
Example of using NumPy to create an array and perform a mathematical operation:
import numpy as np
# Create an array
arr = np.array([1, 2, 3, 4, 5])
# Perform a mathematical operation
arr_squared = np.square(arr)
print(arr_squared)
2. Pandas (Data Manipulation)
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series to handle structured data, making it easy to clean, manipulate, and analyze data in Python.
Key Features of Pandas:
- Data structures for handling structured data (DataFrame, Series).
- Tools for handling missing data.
- Data manipulation (filtering, merging, grouping, etc.).
- Integration with data sources like CSV, Excel, SQL, and more.
Example of using Pandas to load a CSV file and filter data:
import pandas as pd
# Load a CSV file into a DataFrame
df = pd.read_csv('data.csv')
# Filter data based on a condition
filtered_df = df[df['age'] > 30]
print(filtered_df)
3. Matplotlib and Seaborn (Data Visualization)
Matplotlib is a popular library for creating static, animated, and interactive visualizations in Python. It provides a wide range of plotting functions to create charts, histograms, and other data visualizations.
Seaborn is built on top of Matplotlib and provides a high-level interface for drawing attractive and informative statistical graphics. It simplifies the creation of complex visualizations and supports advanced features like heatmaps and regression plots.
Key Features of Matplotlib and Seaborn:
- Matplotlib: Basic plotting, line plots, bar plots, scatter plots, histograms, etc.
- Seaborn: Advanced plots, heatmaps, violin plots, categorical plots, etc.
- Customization options for visualizations.
- Support for interactive and publication-quality plots.
Example of using Matplotlib and Seaborn to create a plot:
import matplotlib.pyplot as plt
import seaborn as sns
# Create a simple dataset
data = [1, 2, 3, 4, 5]
# Create a line plot using Matplotlib
plt.plot(data)
plt.title('Line Plot with Matplotlib')
plt.show()
# Create a bar plot using Seaborn
sns.barplot(x=['A', 'B', 'C'], y=[3, 5, 7])
plt.title('Bar Plot with Seaborn')
plt.show()
4. Requests (HTTP Requests)
Requests is a simple and elegant HTTP library for Python. It allows you to send HTTP requests to web servers and handle responses easily. This library abstracts many complexities of working with HTTP, making it incredibly easy to interact with web APIs, download files, or submit forms.
Key Features of Requests:
- Sending HTTP GET, POST, PUT, DELETE, and other types of requests.
- Handling response data (JSON, HTML, XML, etc.).
- Automatic handling of cookies and sessions.
- Support for authentication, timeouts, and headers.
Example of using Requests to make an HTTP GET request:
import requests
# Send an HTTP GET request
response = requests.get('https://api.github.com')
# Print the response JSON data
print(response.json())
5. BeautifulSoup (Web Scraping)
BeautifulSoup is a library for parsing HTML and XML documents. It is commonly used for web scraping, allowing you to extract data from web pages efficiently. BeautifulSoup helps you navigate the structure of HTML documents and extract relevant data from tags, attributes, and elements.
Key Features of BeautifulSoup:
- Parsing and navigating HTML and XML documents.
- Extracting data from tags, attributes, and elements.
- Compatible with HTML5 and handles malformed HTML.
- Easy integration with other libraries like Requests for web scraping.
Example of using BeautifulSoup to scrape a webpage:
from bs4 import BeautifulSoup
import requests
# Send an HTTP GET request to fetch a webpage
response = requests.get('https://example.com')
# Parse the HTML content of the page
soup = BeautifulSoup(response.content, 'html.parser')
# Extract and print the title of the webpage
print(soup.title.string)
Conclusion
These libraries—NumPy, Pandas, Matplotlib/Seaborn, Requests, and BeautifulSoup—are just a few examples of Python's vast ecosystem. They serve as essential tools for developers working with numerical data, data analysis, visualization, web scraping, and interacting with web APIs. Learning to use these libraries will help you build powerful Python applications in various domains.
Introduction to Web Development with Python
Python is not only a powerful tool for scientific computing and data analysis but also a popular language for web development. With its simple syntax, robust libraries, and frameworks, Python has become one of the top choices for building web applications, ranging from simple websites to complex data-driven platforms.
Why Use Python for Web Development?
Python offers several advantages when it comes to web development:
- Easy to Learn and Use: Python has a clean and readable syntax that is beginner-friendly, making it easier to learn for new developers.
- Powerful Frameworks: Python has a variety of web frameworks (like Django, Flask, and FastAPI) that simplify web application development.
- Large Community and Ecosystem: Python’s large community means there is plenty of documentation, tutorials, and third-party libraries to choose from.
- Versatility: Python can be used for full-stack web development, from backend development to data processing, web scraping, and even frontend integration.
- Integration with Databases: Python can easily interact with databases such as MySQL, PostgreSQL, and MongoDB, making it an ideal choice for building data-driven applications.
Popular Python Web Frameworks
Python has several powerful web frameworks that help developers build web applications quickly and efficiently. Here are some of the most popular ones:
1. Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It follows the "batteries-included" philosophy, providing many built-in tools and libraries for everything from routing, templates, authentication, and more, making it ideal for building large-scale web applications.
Features of Django:
- Built-in ORM (Object-Relational Mapping) for database handling.
- Automatic admin interface for easy site management.
- Robust security features like protection against cross-site scripting (XSS), SQL injection, and cross-site request forgery (CSRF).
- Highly extensible with a large number of third-party packages available.
2. Flask
Flask is a lightweight, micro-framework for building web applications. Unlike Django, Flask gives developers more flexibility by providing the essentials for building web applications and leaving the choice of additional libraries and tools up to the developer. This makes Flask a great choice for small to medium-sized applications or for developers who want more control over their project.
Features of Flask:
- Minimalistic and flexible, allowing you to choose your tools and libraries.
- Simple to get started with, making it ideal for small projects or prototyping.
- Extensible with plug-ins for ORM, forms, authentication, and more.
3. FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It is designed for building APIs quickly and with minimal code, while maintaining high performance and security.
Features of FastAPI:
- Built on top of Starlette and Pydantic, providing automatic validation and interactive documentation.
- Fast and efficient, with performance comparable to Node.js and Go.
- Ideal for building RESTful APIs and web services.
Setting Up a Simple Web Application with Flask
Now that we’ve covered some popular frameworks, let’s set up a simple web application using Flask. Follow these steps:
1. Install Flask
First, install Flask using pip, Python’s package manager:
pip install flask
2. Write the Flask Application
Create a new Python file (e.g., app.py
) and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
3. Run the Application
Now, run the Flask application using the following command:
python app.py
This will start a development server, and you can access the application in your browser at http://127.0.0.1:5000/
.
Working with Templates and Static Files
Flask supports Jinja2 templating for rendering dynamic HTML pages. You can organize your application’s files into folders like templates/
and static/
for better project structure.
- Templates: Store your HTML files in the
templates/
folder. These files can contain placeholders for dynamic content. - Static Files: Store CSS, JavaScript, and image files in the
static/
folder. These files will be served as-is to the client.
Example of rendering a template in Flask:
from flask import render_template
@app.route('/hello/')
def hello_name(name):
return render_template('hello.html', name=name)
Conclusion
Python offers a variety of frameworks that make web development easy and efficient. Whether you're building a simple website with Flask or a large web application with Django, Python’s flexibility and powerful libraries will make the process smoother and faster. With Python, developers can focus on writing clean and maintainable code, allowing them to bring their web projects to life quickly and effectively.
Flask: Creating Your First Web App
Flask is a lightweight and flexible web framework for Python, which allows developers to build web applications quickly and with minimal setup. In this section, we will walk through the steps to create your first Flask web app, covering everything from installation to running the app locally.
Prerequisites
Before you begin, ensure that you have Python installed on your system. You can check this by running python --version
in your terminal or command prompt. If you don't have Python installed, refer to the Python installation guide.
Step 1: Install Flask
Start by installing Flask using the pip
package manager. Open your terminal or command prompt and run the following command:
pip install flask
Step 2: Create a Basic Flask Application
Now that Flask is installed, let's create a simple web application. Create a new Python file (e.g., app.py
) and add the following code:
from flask import Flask
# Create a Flask application instance
app = Flask(__name__)
# Define a route for the homepage
@app.route('/')
def home():
return "Hello, Flask!"
# Run the application
if __name__ == "__main__":
app.run(debug=True)
This basic Flask app does the following:
- Creates a Flask app instance: The
Flask(__name__)
creates an app object that you can use to define routes. - Defines a route: The
@app.route('/')
decorator defines the homepage route, which returns the message "Hello, Flask!" when accessed. - Runs the app: The
app.run(debug=True)
runs the app locally on your machine and enables debugging features for better development.
Step 3: Run the Flask Application
Once the code is ready, go to your terminal or command prompt, navigate to the directory where app.py
is saved, and run the following command:
python app.py
Flask will start a development server, and you can view the web app by opening a web browser and going to http://127.0.0.1:5000/
. You should see the message "Hello, Flask!" displayed on the page.
Step 4: Adding More Routes
Flask allows you to define multiple routes for different pages. Here’s how you can add another route to your app:
@app.route('/about')
def about():
return "Welcome to the About Page!"
Now, if you visit http://127.0.0.1:5000/about
in your browser, you will see the message "Welcome to the About Page!" displayed on the page.
Step 5: Using HTML Templates
Flask allows you to render dynamic HTML pages using templates. Let's use the Jinja2 templating engine to create a simple HTML page. First, create a new folder called templates
in the same directory as your app.py
file. Inside the templates
folder, create a new HTML file called home.html
with the following content:
Flask Web App
{{ greeting }}
Next, modify the home()
route in your app.py
file to render the HTML template:
from flask import render_template
@app.route('/')
def home():
return render_template('home.html', greeting="Hello, Flask with Templates!")
This will pass the value "Hello, Flask with Templates!" to the greeting
variable in the template, and the rendered HTML page will display this message.
Step 6: Adding Static Files (CSS, JS)
You can also add static files like CSS and JavaScript to your Flask application. Create a folder called static
in your project directory, and place your CSS or JavaScript files there. Flask automatically serves static files from this folder.
To link a CSS file, you would use the following code in your HTML template:
Here, the url_for('static', filename='style.css')
function generates the correct URL for the static file.
Step 7: Conclusion
Congratulations! You’ve just created your first Flask web app. You learned how to:
- Install Flask and create a basic application.
- Define routes and create dynamic responses.
- Render HTML templates using Jinja2.
- Work with static files like CSS and JavaScript.
This is just the beginning. Flask offers many advanced features that allow you to build more complex web applications, such as form handling, database integration, and authentication. Keep exploring and building!
Django: Building Scalable Web Applications
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It's built to handle large-scale applications and provides a lot of built-in features, such as authentication, database management, and more. In this section, we will explore how to build a scalable web application using Django.
Prerequisites
Before you begin, ensure that you have Python and pip installed on your system. You can check this by running python --version
and pip --version
in your terminal or command prompt. If you haven't installed Django yet, you can do so by running:
pip install django
Step 1: Create a New Django Project
Start by creating a new Django project. Open your terminal or command prompt and run the following command to create a new Django project called myproject
:
django-admin startproject myproject
This will create a new directory named myproject
with the following structure:
myproject/
manage.py
myproject/
__init__.py
settings.py
urls.py
wsgi.py
Now, navigate into the myproject
directory:
cd myproject
Step 2: Run the Development Server
Once your project is created, you can start the Django development server by running the following command:
python manage.py runserver
This will start the server, and you can visit http://127.0.0.1:8000/
in your browser to see the default Django welcome page.
Step 3: Create a Django App
In Django, a project can contain multiple "apps," each serving a specific function. To create an app, run the following command:
python manage.py startapp myapp
This will create a new directory called myapp
with the following structure:
myapp/
__init__.py
admin.py
apps.py
models.py
views.py
migrations/
tests.py
Now, add myapp
to the INSTALLED_APPS
list in your project's settings.py
file:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'myapp',
]
Step 4: Create a View and URL Mapping
In Django, views are functions that handle requests and return responses. To create a basic view, open the views.py
file in your app directory and add the following code:
from django.http import HttpResponse
def home(request):
return HttpResponse("Hello, Django!")
Next, map the view to a URL by editing the urls.py
file in your project directory:
from django.urls import path
from myapp import views
urlpatterns = [
path('', views.home, name='home'),
]
Now, when you visit http://127.0.0.1:8000/
in your browser, you should see the message "Hello, Django!"
Step 5: Working with Templates
Django uses the Jinja2 templating engine to dynamically generate HTML pages. To use templates, create a new folder called templates
inside the myapp
directory. Inside the templates
folder, create an HTML file called home.html
with the following content:
Welcome to Django
Welcome to Django!
This is your first Django web app.
Next, update the home
view in views.py
to render the template:
from django.shortcuts import render
def home(request):
return render(request, 'home.html')
Now, when you visit the homepage, Django will render the home.html
template instead of returning a plain text response.
Step 6: Using Django's Admin Panel
Django provides an admin panel that allows you to manage your app's data through a web interface. To enable the admin panel, first, create a superuser account by running the following command:
python manage.py createsuperuser
Follow the prompts to create a superuser account. Then, run the server again and go to http://127.0.0.1:8000/admin/
in your browser. You can log in with the superuser account and start managing your app's models.
Step 7: Conclusion
Congratulations! You’ve just built a simple Django application. In this section, you learned how to:
- Create a Django project and app.
- Run the Django development server.
- Define views and map them to URLs.
- Use Django templates to render dynamic HTML content.
- Access the Django admin panel to manage data.
Django is a powerful framework that scales well for large applications. It includes many advanced features like authentication, database management, form handling, and more. Keep exploring Django to build more complex applications!
Using Templates and Static Files
In Django, templates are used to generate dynamic HTML content, while static files (like CSS, JavaScript, and images) are used to style and enhance the user interface of your web application. This section will guide you through using templates and static files in Django.
What Are Templates?
Templates in Django are HTML files that are dynamically generated by the server. They allow you to insert dynamic content into static HTML pages. Django uses the Jinja2 templating engine, which enables you to include logic, variables, and loops in your HTML files.
Setting Up Templates in Django
To use templates in Django, you need to create a templates
directory within your app and tell Django where to look for templates.
Follow these steps:
- Create a
templates
directory inside your app directory (e.g.,myapp/templates/
). - Inside the
templates
directory, create an HTML file (e.g.,home.html
) for your template. - Tell Django where to find these templates by updating the
DIRS
setting in thesettings.py
file:
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [BASE_DIR / 'templates'], # Add this line
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
Rendering Templates in Views
Once you have set up your templates, you can use them in your views. In your views.py
file, use the render()
function to render the template and pass any context (data) you want to display.
from django.shortcuts import render
def home(request):
return render(request, 'home.html', {'message': 'Welcome to Django Templates!'})
In the above example, the context dictionary contains the variable message
, which will be passed to the template.
Using Template Variables
To use variables passed from views in your templates, you can include them within double curly braces {{ variable_name }}
:
{{ message }}
This will render the message
variable value in the HTML when the page is loaded.
Template Tags and Filters
Django templates allow you to use tags and filters to add logic and process data in your templates. For example:
{% if %}
tag for conditional statements.{% for %}
tag for loops.{{ variable|filter }}
for applying filters to variables.
{% if message %}
{{ message }}
{% else %}
No message available.
{% endif %}
What Are Static Files?
Static files include CSS, JavaScript, images, fonts, etc., that are not dynamically generated but serve as part of the frontend to style and enrich your web application. In Django, static files are handled separately from templates.
Setting Up Static Files in Django
To use static files in your Django project, follow these steps:
- Create a
static
directory inside your app (e.g.,myapp/static/
) for your CSS, JavaScript, and image files. - Tell Django where to find static files by updating the
STATICFILES_DIRS
setting in yoursettings.py
file:
STATICFILES_DIRS = [
BASE_DIR / 'static',
]
Linking Static Files in Templates
Once your static files are set up, you can link them to your templates using the {% load static %}
tag. For example, to include a CSS file:
{% load static %}
Similarly, you can link JavaScript files and images using the static
tag:
Serving Static Files in Development
In development, Django automatically serves static files, but in production, you’ll need to configure a web server (like Nginx or Apache) to serve static files. To enable static file serving in production, you’ll need to run the collectstatic
command:
python manage.py collectstatic
This command collects all static files from your apps and moves them to the STATIC_ROOT
directory, where they can be served by the web server.
Step 1: Create Template and Static Files
Now, let’s create a simple template and static files for a more dynamic web page.
- Create the
home.html
template inmyapp/templates/
with dynamic content. - Place your CSS file in
myapp/static/css/styles.css
. - Link the CSS file to your HTML template as demonstrated above.
Step 2: Update Views
In the views.py
file of your app, update the home view to render the home.html
template:
def home(request):
return render(request, 'home.html', {'message': 'Welcome to Django with Static Files!'})
Step 3: Run the Server
Now, run the Django development server again:
python manage.py runserver
Visit http://127.0.0.1:8000/
to view the dynamically generated page with the static CSS styling applied.
Conclusion
In this section, you learned how to:
- Use Django templates to dynamically generate HTML.
- Pass context data from views to templates.
- Utilize template tags and filters for logic and data manipulation.
- Set up static files (CSS, JS, images) in Django.
- Link static files to templates using the
{% static %}
tag. - Use the
collectstatic
command to collect static files for production.
Templates and static files are fundamental to creating dynamic and interactive web applications in Django. By combining templates for content and static files for styling and functionality, you can build sophisticated web pages with ease.
Data Analysis with Pandas
Pandas is one of the most popular Python libraries for data manipulation and analysis. It provides powerful data structures like Series and DataFrames that make working with structured data easier and more efficient. This section will guide you through using Pandas for data analysis tasks.
What is Pandas?
Pandas is an open-source Python library that provides fast, flexible, and expressive data structures designed to work with structured data. It is built on top of NumPy and is used extensively in data analysis, data science, and machine learning workflows.
Installing Pandas
To get started with Pandas, you need to install it first. If you don't have Pandas installed, you can install it using pip
:
pip install pandas
Importing Pandas
Once installed, you can import Pandas into your Python script or Jupyter notebook:
import pandas as pd
Creating DataFrames
A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. You can create DataFrames from various data sources, such as lists, dictionaries, and CSV files. Here's how to create a simple DataFrame from a dictionary:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston']
}
df = pd.DataFrame(data)
print(df)
This will output the following DataFrame:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
3 David 40 Houston
Reading Data from CSV Files
Pandas makes it easy to read data from CSV files using the read_csv()
function. Here's how to load data from a CSV file:
df = pd.read_csv('data.csv')
print(df.head()) # Display the first 5 rows of the DataFrame
You can replace 'data.csv' with the path to your CSV file. The head()
function displays the first 5 rows of the DataFrame.
Basic DataFrame Operations
Pandas offers a variety of operations to manipulate and analyze data in DataFrames. Here are some common operations:
- View the first few rows:
df.head()
- View the last few rows:
df.tail()
- Display column names:
df.columns
- Describe the data (summary statistics):
df.describe()
- Get the shape of the DataFrame (number of rows and columns):
df.shape
Data Selection and Filtering
To select specific rows or columns from a DataFrame, you can use various indexing and selection techniques:
- Select a column:
df['Age']
- Select multiple columns:
df[['Name', 'Age']]
- Select rows by index:
df.iloc[0]
(selects the first row) - Select rows by condition:
df[df['Age'] > 30]
(selects rows where Age is greater than 30)
Data Cleaning
Data cleaning is a crucial step in data analysis. Pandas provides various functions to clean and prepare data:
- Handle missing values:
df.isnull()
to check for missing values anddf.fillna()
ordf.dropna()
to handle them. - Remove duplicates:
df.drop_duplicates()
- Convert data types:
df['Age'] = df['Age'].astype(int)
Data Aggregation and Grouping
Pandas allows you to group data based on certain criteria and perform aggregate operations:
# Group by 'City' and calculate the average age for each city
grouped = df.groupby('City').agg({'Age': 'mean'})
print(grouped)
This will group the data by the 'City' column and calculate the mean of the 'Age' column for each group.
Data Visualization with Pandas
Pandas integrates well with Matplotlib to create basic visualizations. You can easily plot data using the plot()
function:
import matplotlib.pyplot as plt
df['Age'].plot(kind='bar') # Creates a bar plot of the 'Age' column
plt.show()
Saving Data to CSV
After analyzing and manipulating your data, you may want to save it back to a CSV file. You can use the to_csv()
function to save a DataFrame:
df.to_csv('output.csv', index=False)
This will save the DataFrame to a CSV file named 'output.csv' in the current directory.
Conclusion
Pandas is an incredibly powerful tool for data analysis. In this section, you have learned how to:
- Create and manipulate DataFrames.
- Load and save data from/to CSV files.
- Filter, clean, and aggregate data.
- Visualize data using Pandas and Matplotlib.
Mastering Pandas will greatly enhance your ability to work with structured data and perform data analysis tasks efficiently.
Visualization with Matplotlib and Seaborn
Data visualization is a crucial part of data analysis as it helps to convey insights and patterns in the data. Python provides two powerful libraries for creating visualizations: Matplotlib and Seaborn. In this section, we will explore how to use these libraries to create various types of plots.
What is Matplotlib?
Matplotlib is a low-level plotting library in Python. It provides an object-oriented API for embedding plots into applications. Matplotlib is highly customizable and allows you to create a wide variety of visualizations, such as line plots, bar charts, histograms, and more.
What is Seaborn?
Seaborn is built on top of Matplotlib and provides a high-level interface for drawing attractive and informative statistical graphics. It simplifies creating complex visualizations and comes with a built-in set of themes and color palettes that make it easier to create beautiful plots.
Installing Matplotlib and Seaborn
If you haven't already installed Matplotlib and Seaborn, you can install them using pip
:
pip install matplotlib seaborn
Importing Matplotlib and Seaborn
Once installed, you can import the libraries into your Python script:
import matplotlib.pyplot as plt
import seaborn as sns
Creating Basic Plots with Matplotlib
Matplotlib allows you to create a variety of plots. Here's how to create some basic visualizations:
Line Plot
Line plots are commonly used to visualize trends over time. Here's how to create a simple line plot:
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
# Create a line plot
plt.plot(x, y)
# Adding labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
# Show plot
plt.show()
Bar Chart
Bar charts are useful for comparing quantities across different categories. Here's how to create a simple bar chart:
# Data
categories = ['A', 'B', 'C', 'D']
values = [3, 7, 5, 10]
# Create a bar chart
plt.bar(categories, values)
# Adding labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Simple Bar Chart')
# Show plot
plt.show()
Creating Plots with Seaborn
Seaborn simplifies the process of creating complex visualizations with a high-level interface. Here are a few examples:
Histogram
Histograms show the distribution of a dataset. Here's how to create a histogram using Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
# Load a sample dataset
tips = sns.load_dataset('tips')
# Create a histogram of the total bill
sns.histplot(tips['total_bill'], kde=True)
# Adding title
plt.title('Histogram of Total Bill')
# Show plot
plt.show()
Scatter Plot
Scatter plots are used to visualize the relationship between two variables. Here's how to create a scatter plot using Seaborn:
# Create a scatter plot
sns.scatterplot(x='total_bill', y='tip', data=tips)
# Adding title
plt.title('Scatter Plot of Total Bill vs Tip')
# Show plot
plt.show()
Customizing Plots with Seaborn
Seaborn comes with several built-in themes and color palettes that make it easy to customize your plots:
Setting Themes
Seaborn provides several themes for customizing the look of your plots. You can set the theme using the set_theme()
function:
# Set the theme
sns.set_theme(style='darkgrid')
# Create a simple plot
sns.lineplot(x='total_bill', y='tip', data=tips)
# Show plot
plt.show()
Using Color Palettes
Seaborn also allows you to customize color palettes for your plots:
# Set a color palette
sns.set_palette('husl')
# Create a boxplot
sns.boxplot(x='day', y='total_bill', data=tips)
# Show plot
plt.show()
Seaborn Plot Types
Seaborn provides a variety of plot types to visualize different kinds of data:
- lineplot(): Line plot for showing trends over time.
- barplot(): Bar plot for comparing categories.
- boxplot(): Box plot for visualizing the distribution of data.
- heatmap(): Heat map for visualizing correlation matrices or 2D data.
- pairplot(): Pair plot for visualizing relationships between multiple variables.
Conclusion
Matplotlib and Seaborn are powerful tools for data visualization in Python. By combining these libraries, you can create a wide range of plots and charts to analyze and present data effectively. In this section, you have learned how to:
- Create basic plots with Matplotlib (line plots, bar charts).
- Create more advanced plots with Seaborn (histograms, scatter plots, etc.).
- Customize plots with themes and color palettes.
By mastering data visualization with these libraries, you will be able to generate insightful visual representations of your data that can help you make better decisions and communicate your findings effectively.
Basics of Machine Learning with Scikit-learn
Machine Learning (ML) is a subset of Artificial Intelligence that enables computers to learn patterns from data and make predictions or decisions. Scikit-learn is one of the most popular and user-friendly libraries for implementing machine learning algorithms in Python. It provides a wide range of tools for data mining, data analysis, and machine learning tasks such as classification, regression, clustering, and more.
What is Scikit-learn?
Scikit-learn is an open-source machine learning library for Python. It is built on top of other popular libraries such as NumPy, SciPy, and matplotlib. Scikit-learn provides simple and efficient tools for data analysis and machine learning, making it accessible to both beginners and experts.
Installing Scikit-learn
To begin using Scikit-learn, you need to install it. You can install it using pip:
pip install scikit-learn
Importing Scikit-learn
Once installed, you can import Scikit-learn and start using its features for machine learning tasks. Here’s how to import it:
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
Basic Steps in a Machine Learning Workflow
To implement a machine learning model with Scikit-learn, we follow a basic workflow:
- Load and Prepare Data - Import datasets and prepare them for training.
- Split the Data - Divide the data into training and testing sets.
- Choose a Model - Select an appropriate machine learning algorithm for the task (e.g., regression, classification).
- Train the Model - Train the model using the training data.
- Evaluate the Model - Test the model’s accuracy on the testing data.
Example: Classification with Scikit-learn
Let’s walk through a simple example of classification using Scikit-learn. We will use the famous Iris dataset and implement a Random Forest Classifier to classify flowers based on their features.
Step 1: Load the Dataset
Scikit-learn provides several built-in datasets, such as the Iris dataset. Let’s load this dataset:
# Load the Iris dataset
from sklearn.datasets import load_iris
iris = load_iris()
# Display the features and target
print("Features:\n", iris.data)
print("Target:\n", iris.target)
Step 2: Split the Data
Next, we split the data into training and testing sets. This allows us to train our model on one subset of the data and evaluate it on another:
from sklearn.model_selection import train_test_split
# Split the data into training (80%) and testing (20%)
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
Step 3: Choose a Model
In this example, we will use a Random Forest Classifier, which is an ensemble method that combines multiple decision trees to improve classification accuracy:
from sklearn.ensemble import RandomForestClassifier
# Initialize the Random Forest Classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
Step 4: Train the Model
Now that we have chosen our model, we can train it using the training data:
# Train the model using the training data
clf.fit(X_train, y_train)
Step 5: Evaluate the Model
Finally, we evaluate the model's performance using the testing data. We will calculate the accuracy of the model:
# Predict the labels for the test data
y_pred = clf.predict(X_test)
# Calculate the accuracy of the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy * 100:.2f}%")
Common Machine Learning Models in Scikit-learn
Scikit-learn supports a wide variety of machine learning algorithms. Some of the most commonly used models include:
- Linear Regression: Used for predicting continuous values.
- Logistic Regression: Used for binary classification tasks.
- Decision Trees: A tree-like structure for making decisions based on input features.
- Random Forest: An ensemble method that combines multiple decision trees.
- Support Vector Machines (SVM): A powerful algorithm for classification tasks.
- K-Means Clustering: Unsupervised learning algorithm used for clustering data.
Model Evaluation and Tuning
Once you have trained a model, it is important to evaluate its performance and fine-tune it. Scikit-learn provides several evaluation metrics, including:
- Accuracy: The percentage of correct predictions.
- Precision, Recall, and F1-Score: Metrics used in classification tasks to evaluate the balance between true positives and false positives/negatives.
- Cross-validation: A technique to assess the model's performance by splitting the data into multiple subsets.
You can also fine-tune hyperparameters using GridSearchCV
or RandomizedSearchCV
to find the optimal parameters for your model.
Conclusion
Scikit-learn is a powerful library that makes it easy to implement machine learning algorithms in Python. In this section, we covered the basics of machine learning with Scikit-learn, including how to:
- Load and prepare datasets.
- Split the data into training and testing sets.
- Train and evaluate a machine learning model.
By mastering Scikit-learn, you can apply machine learning to a wide range of problems, from classification and regression to clustering and more.
Working with Jupyter Notebooks
Jupyter Notebooks are an essential tool for data science and machine learning, offering an interactive environment where you can write and execute code in a step-by-step manner. They are widely used for exploratory data analysis, creating reports, and sharing projects in a reproducible format. Jupyter Notebooks support Python and many other languages, making them a versatile tool for developers and researchers alike.
What is Jupyter Notebook?
Jupyter Notebook is an open-source, web-based interactive computing environment that allows you to create and share documents that contain live code, equations, visualizations, and narrative text. The name "Jupyter" comes from the combination of Julia, Python, and R, though it now supports many other programming languages.
Installing Jupyter Notebook
To start using Jupyter Notebooks, you first need to install them. The easiest way to install Jupyter is by using pip
or by installing Anaconda, which includes Jupyter and other data science tools.
Installing Jupyter with pip
pip install notebook
Installing Jupyter via Anaconda
If you use Anaconda, Jupyter is already included in the distribution. You can install Anaconda from the official website.
Starting Jupyter Notebook
After installation, you can start Jupyter Notebook from the command line or terminal by running:
jupyter notebook
This command will open a new tab in your default web browser, where you can create, edit, and run Jupyter Notebooks.
Basic Features of Jupyter Notebook
Jupyter Notebooks are highly interactive and allow you to combine code, visualizations, and text in a single document. Key features include:
- Code Execution: You can run code blocks (cells) one at a time, allowing for an interactive and iterative approach to programming.
- Markdown Support: Use Markdown to format text, create headings, lists, and include hyperlinks or images in your notebook.
- Interactive Visualizations: You can use libraries such as Matplotlib, Seaborn, and Plotly to display visualizations directly in your notebook.
- Exporting Notebooks: Notebooks can be exported to various formats, including HTML, PDF, and slides, making it easy to share your work.
Creating and Running Cells
A Jupyter notebook consists of cells. There are two main types of cells:
- Code Cells: These cells contain code that can be executed. You can run the code by pressing
Shift + Enter
or clicking the "Run" button in the toolbar. - Markdown Cells: These cells contain text formatted using Markdown syntax. They can be used to add documentation, instructions, or explanations to your code.
Example: Simple Python Code in Jupyter Notebook
Let’s write a simple Python program in a Jupyter notebook that prints "Hello, Jupyter!" and calculate the square of a number:
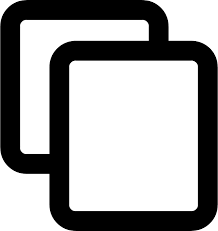
# Python code in Jupyter Notebook
print("Hello, Jupyter!")
# Calculate the square of a number
number = 4
square = number ** 2
print(f"The square of {number} is {square}")
Interactive Visualizations in Jupyter
Jupyter Notebooks are particularly useful for visualizing data. Let’s create a simple plot using Matplotlib:
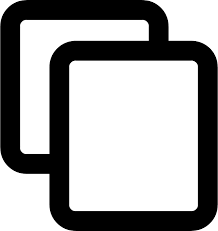
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
# Create a plot
plt.plot(x, y)
plt.title("Simple Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Using Magic Commands
Jupyter Notebooks provide special commands known as "magic commands" that enhance productivity. These commands are prefixed with the %
symbol. Here are a few commonly used magic commands:
- %time: Used to measure the execution time of a single statement or block of code.
- %matplotlib inline: Ensures that Matplotlib plots are displayed inline in the notebook.
- %run: Runs a Python script as a separate process within the notebook environment.
Sharing and Exporting Notebooks
Once your work is complete, you can share your Jupyter Notebook with others. You can save your notebook in the following formats:
- Notebook Format (.ipynb): The native format for Jupyter Notebooks.
- HTML: Export your notebook as an HTML file that can be viewed in a web browser.
- PDF: Generate a PDF version of your notebook for printing or sharing.
To export your notebook, go to the "File" menu and select "Download as" followed by the desired format.
Conclusion
Jupyter Notebooks are an invaluable tool for data analysis, machine learning, and research. They provide an interactive environment that allows for quick experimentation, visualization, and sharing of results. Whether you are working on a small project or a complex data analysis task, Jupyter Notebooks help streamline the process and enhance your productivity.
Automating Tasks with Python Scripts
Python is widely used for automating repetitive tasks, making it an essential tool for developers and sysadmins. From file handling to web scraping, Python scripts can save time and effort by automating routine processes. This section will guide you through the basics of automating tasks with Python scripts, allowing you to work more efficiently and focus on complex problems.
Why Automate with Python?
Python’s simple syntax and powerful libraries make it an ideal language for automation. Whether you need to automate file management, data processing, web scraping, or system monitoring, Python provides built-in functionality and third-party libraries to help you automate almost anything.
Getting Started with Automation
Before starting automation with Python, you need to have a basic understanding of Python programming. Once you're comfortable with Python's syntax, you can begin writing scripts to automate simple tasks. Below are the common steps to start automating with Python:
- Identify the Task: Determine the repetitive tasks you want to automate, such as file management, system maintenance, or data processing.
- Write the Script: Create a Python script that performs the task. This might include using built-in libraries like
os
,shutil
, orsubprocess
for system operations. - Schedule the Script: Use task schedulers like
cron
(Linux/macOS) or Task Scheduler (Windows) to run your Python script at specific intervals.
Example: Automating File Backup
Let’s automate the process of backing up files from one directory to another using Python. Below is a simple Python script that copies files from a source directory to a backup directory:
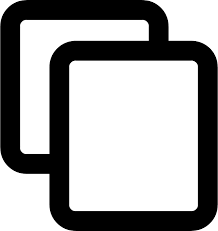
import os
import shutil
import time
# Source and backup directories
source_dir = "/path/to/source"
backup_dir = "/path/to/backup"
# Function to back up files
def backup_files():
# Get the current time for backup folder naming
current_time = time.strftime("%Y%m%d-%H%M%S")
backup_folder = os.path.join(backup_dir, f"backup_{current_time}")
# Create backup folder
os.makedirs(backup_folder)
# Copy files from source to backup
for file_name in os.listdir(source_dir):
source_file = os.path.join(source_dir, file_name)
if os.path.isfile(source_file):
shutil.copy(source_file, backup_folder)
print(f"Backup completed at {backup_folder}")
# Call the backup function
backup_files()
This script copies all files from the source_dir
to a backup folder with a timestamp, ensuring that each backup is unique and easy to identify.
Scheduling the Script
Once the script is ready, you can schedule it to run automatically at certain intervals. Below are instructions for scheduling the script on different operating systems:
Linux/macOS: Using Cron
On Linux and macOS, you can use cron
to schedule the script. To schedule the script to run every day at 2:00 AM, follow these steps:
- Open your terminal and type
crontab -e
to edit the cron jobs. - Add the following line to schedule the backup script:
0 2 * * * /usr/bin/python3 /path/to/your_script.py
This cron job runs the script every day at 2:00 AM.
Windows: Using Task Scheduler
On Windows, you can use Task Scheduler to run Python scripts automatically. Follow these steps to create a task:
- Open Task Scheduler and click "Create Basic Task".
- Set the trigger to daily and specify the time.
- In the "Action" section, select "Start a Program" and browse for the Python executable and your script.
- Finish the setup and your script will run automatically at the specified time.
Example: Automating Web Scraping
Python can also be used for automating web scraping tasks. Here’s an example of using the requests
and BeautifulSoup
libraries to scrape headlines from a news website and save them to a text file:
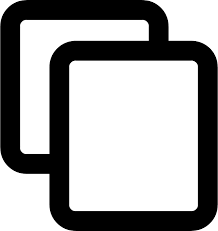
import requests
from bs4 import BeautifulSoup
# URL of the website to scrape
url = "https://www.example.com/news"
# Send an HTTP request to get the page content
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# Find all headlines (adjust based on the website's structure)
headlines = soup.find_all("h2", class_="headline")
# Save headlines to a text file
with open("headlines.txt", "w") as file:
for headline in headlines:
file.write(headline.get_text() + "\n")
print("Web scraping completed. Headlines saved in headlines.txt.")
This script fetches the latest news headlines from a website and saves them into a text file. You can schedule it to run periodically to fetch the latest data automatically.
Benefits of Automating with Python
Automating tasks with Python offers many advantages:
- Save Time: Automate repetitive tasks to save hours of manual work.
- Reduce Errors: Automation minimizes the risk of human error in repetitive tasks.
- Increase Efficiency: Automating tasks ensures they are done consistently and promptly, without requiring constant monitoring.
- Focus on High-Level Tasks: By automating mundane tasks, you can focus on more complex and rewarding aspects of your work.
Conclusion
Python is an excellent tool for automating tasks. By writing simple scripts, you can automate everything from file management and system maintenance to data scraping and reporting. With Python’s rich ecosystem of libraries and easy-to-understand syntax, you can significantly improve your workflow and focus on more creative tasks. Whether you are a developer, sysadmin, or data analyst, automating tasks with Python will increase your productivity and save you valuable time.
Web Scraping with BeautifulSoup and Selenium
Web scraping is a technique used to extract data from websites. Python provides powerful libraries like BeautifulSoup
and Selenium
for scraping and automating web interactions. BeautifulSoup is great for parsing HTML and extracting information, while Selenium is useful for web automation and dealing with dynamic content. This section covers both libraries and demonstrates how to use them for web scraping tasks.
Why Web Scraping?
Web scraping allows you to gather information from the web in an automated and structured manner. Whether it's for data analysis, gathering news, monitoring competitors, or collecting product prices, web scraping provides a reliable way to collect large volumes of data from websites that don't offer an API.
BeautifulSoup: Parsing HTML for Web Scraping
BeautifulSoup
is a Python library used to parse HTML and XML documents and extract data in a structured format. It works well with static websites where the content is readily available in the page source.
Installation
To use BeautifulSoup, you need to install the beautifulsoup4
and requests
libraries:
pip install beautifulsoup4 requests
Basic Example: Scraping Data from a Website
Let’s scrape some data from a static webpage. In this example, we’ll extract all the headings (h2) from a webpage:
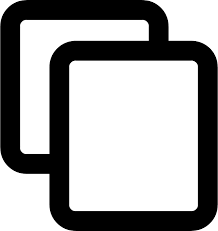
import requests
from bs4 import BeautifulSoup
# URL of the website to scrape
url = "https://example.com"
# Send a request to the website
response = requests.get(url)
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.text, "html.parser")
# Find all h2 headings and print them
headings = soup.find_all("h2")
for heading in headings:
print(heading.get_text())
This script sends an HTTP request to the webpage, parses the HTML content, and extracts all the h2
headings from the page.
Selenium: Automating Web Interactions
While BeautifulSoup works well for static websites, Selenium
is designed for web automation and scraping dynamic content (like content rendered by JavaScript). Selenium can simulate user interactions like clicking buttons, filling forms, and navigating through multiple pages.
Installation
To use Selenium, you need to install the selenium
library and a WebDriver (e.g., ChromeDriver for Google Chrome):
pip install selenium
You also need to download the appropriate WebDriver for your browser (e.g., ChromeDriver for Chrome).
Basic Example: Using Selenium to Automate a Browser
Let’s use Selenium to navigate to a page and scrape dynamic content. In this example, we’ll automate Chrome to open a webpage and extract all the headings:
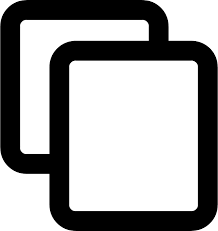
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the WebDriver (make sure ChromeDriver is in your PATH)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
# Open the webpage
driver.get("https://example.com")
# Find all h2 headings on the page
headings = driver.find_elements(By.TAG_NAME, "h2")
# Print the headings
for heading in headings:
print(heading.text)
# Close the browser
driver.quit()
This script uses Selenium to open the webpage, search for all h2
tags, and print the text of each heading. After scraping the data, it closes the browser.
When to Use BeautifulSoup vs. Selenium
Both libraries have their strengths, and the choice between BeautifulSoup and Selenium depends on the type of website you're scraping:
- Use BeautifulSoup: When the website is static, and the data is available in the page source (HTML).
- Use Selenium: When the website is dynamic (content is generated with JavaScript), or you need to simulate user interactions, such as clicking buttons or filling forms.
Challenges in Web Scraping
While web scraping is a powerful tool, it comes with several challenges:
- Legal Issues: Some websites prohibit web scraping in their terms of service. Always check a website’s
robots.txt
file and terms to make sure you are not violating any rules. - IP Blocking: Websites may block or throttle your IP if they detect excessive scraping activity. Use techniques like rotating IPs, user agents, and headers to mitigate this.
- Dynamic Content: Some websites load content dynamically using JavaScript, making it harder to scrape. In these cases, Selenium or using an API (if available) is preferred.
Conclusion
Web scraping with Python is a powerful way to automate data collection from websites. BeautifulSoup is great for parsing static HTML, while Selenium is useful for automating interactions with dynamic websites. By leveraging these tools, you can collect valuable data from the web for analysis, reporting, or any other purpose. Be mindful of legal and technical challenges, and always respect the rules set by the website you’re scraping.
Sending Emails with Python
Sending emails programmatically is a common task in Python, whether it’s for sending notifications, automated reports, or bulk emails. Python offers several ways to send emails, with the most common being the smtplib
library, which allows you to send emails via an SMTP server. In this section, we’ll cover how to set up email sending with Python, handle attachments, and customize email content.
Why Send Emails with Python?
There are several reasons why you might want to send emails through a Python script:
- Automated Notifications: Send alerts or notifications automatically based on certain events or conditions.
- Reports and Updates: Automatically send reports, summaries, or updates to users or stakeholders.
- Bulk Emails: Send marketing or promotional emails to a list of recipients in an efficient manner.
Using smtplib
for Sending Emails
Python provides the smtplib
module for sending emails using the Simple Mail Transfer Protocol (SMTP). SMTP is the protocol used by most email services to send messages between mail servers. Below is an example of how to use smtplib
to send an email.
Basic Example: Sending a Simple Email
First, let’s look at how to send a basic email using Python’s smtplib
library:
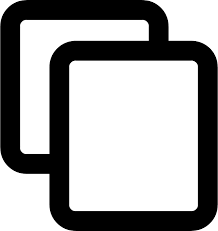
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# Email server settings (Gmail in this case)
smtp_server = "smtp.gmail.com"
smtp_port = 587
sender_email = "your_email@gmail.com"
receiver_email = "recipient_email@example.com"
password = "your_email_password"
# Create the email message
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = "Test Email from Python"
# Email body content
body = "This is a test email sent from Python using smtplib."
msg.attach(MIMEText(body, 'plain'))
# Connect to the mail server and send the email
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # Secure the connection
server.login(sender_email, password) # Login to the server
text = msg.as_string()
server.sendmail(sender_email, receiver_email, text) # Send the email
print("Email sent successfully!")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit() # Close the server connection
This script sends a basic email using Gmail’s SMTP server. It first sets up the email message, then connects to the Gmail SMTP server, logs in, and sends the email. The MIMEText
and MIMEMultipart
classes allow us to structure the email message properly.
Sending HTML Emails
You can also send HTML emails with Python. Here’s an example of how to send an HTML email instead of plain text:
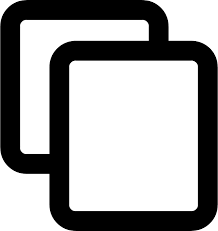
html_content = """
This is an HTML email
Hello, this email contains HTML content.
"""
msg.attach(MIMEText(html_content, 'html'))
In this case, we attach an HTML string to the email using the MIMEText
class, but specify the type as 'html' instead of 'plain'. This allows the email to be rendered as HTML in the recipient's inbox.
Sending Emails with Attachments
In addition to sending plain text or HTML emails, you can also attach files (such as images, PDFs, or documents) to your emails. Here’s how you can send an email with an attachment:
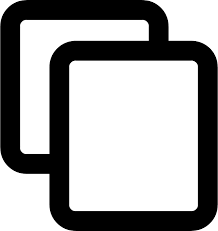
from email.mime.base import MIMEBase
from email import encoders
# Attachment file path
attachment_path = "path/to/your/file.pdf"
# Open the file in binary mode
with open(attachment_path, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
encoders.encode_base64(part) # Encode the attachment
# Add header to the attachment
part.add_header(
"Content-Disposition",
f"attachment; filename={attachment_path.split('/')[-1]}"
)
# Attach the file to the email
msg.attach(part)
This code opens a file in binary mode, encodes it as Base64, and attaches it to the email message. The Content-Disposition
header specifies that the file should be treated as an attachment, and the filename is extracted from the file path.
Security Considerations
When sending emails programmatically, it’s important to consider security:
- Use App-Specific Passwords: When using Gmail or other email providers, consider using app-specific passwords rather than your main account password for better security.
- Use Environment Variables: Avoid hardcoding sensitive information like email passwords directly in your script. Store them in environment variables instead.
- Enable Two-Factor Authentication: For added security, enable two-factor authentication (2FA) on your email account and use OAuth2 for authentication instead of your password.
Alternative: Using Email APIs
While smtplib
is a great choice for sending emails, there are also email APIs like SendGrid, Mailgun, and Amazon SES that provide more advanced features like email tracking, templates, and analytics. These services typically offer free tiers and are easier to set up for bulk email sending.
Conclusion
Sending emails with Python is a simple and powerful tool for automating communications. The smtplib
library makes it easy to send plain text, HTML emails, and even attachments. Always remember to handle sensitive information securely and consider using email APIs for more complex email tasks like bulk emailing and analytics.
File and Folder Automation with Python
Automating file and folder operations is a common task in Python, allowing for seamless file management, backups, and organization. Python’s built-in libraries make it easy to automate tasks like creating, renaming, moving, and deleting files and directories.
Why Automate File and Folder Operations?
There are many scenarios where automating file and folder operations can save time and effort:
- File Organization: Automatically organize files into folders based on specific criteria, such as file type or date.
- Backup and Archiving: Create backups of important files by copying or compressing them into archives.
- File Renaming: Rename multiple files in a folder based on patterns or sequences, such as for batch processing.
- Log File Management: Automatically move or delete old log files to keep your system clean and organized.
Working with Files in Python
Python has several modules for handling files, such as os
, shutil
, and pathlib
. Below are some common file and folder operations you can automate.
Creating and Writing to Files
To create a new file and write data to it, you can use the built-in open()
function:
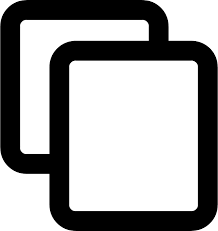
# Creating and writing to a file
with open("example.txt", "w") as file:
file.write("This is a sample file created by Python.")
This script creates a file called example.txt
and writes a string to it. The with
statement ensures the file is properly closed after writing.
Reading from Files
You can also read the contents of a file using the open()
function with the "r" mode:
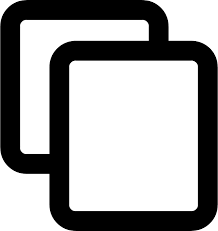
# Reading from a file
with open("example.txt", "r") as file:
content = file.read()
print(content)
This script reads the contents of example.txt
and prints it to the console. The read()
method retrieves the entire file content.
File Operations with os
Module
The os
module provides a wide range of functions for interacting with the operating system, including file and directory management.
Renaming Files
To rename a file or folder, use os.rename()
:
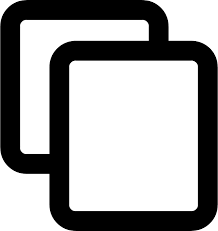
import os
# Renaming a file
os.rename("example.txt", "new_example.txt")
This code renames the file example.txt
to new_example.txt
.
Deleting Files
To delete a file, you can use os.remove()
:
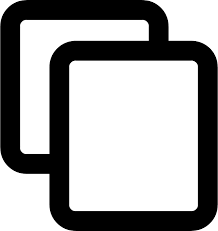
# Deleting a file
os.remove("new_example.txt")
Use os.remove()
to delete the file new_example.txt
. Be cautious with this operation, as it permanently deletes the file.
Working with Directories
The os
module also provides functions for managing directories.
Creating Directories
To create a new directory, use os.mkdir()
:
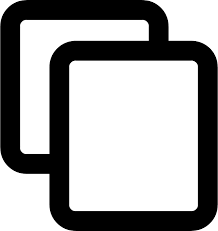
# Creating a directory
os.mkdir("new_folder")
This code creates a new folder called new_folder
in the current directory.
Listing Files in a Directory
To list the contents of a directory, use os.listdir()
:
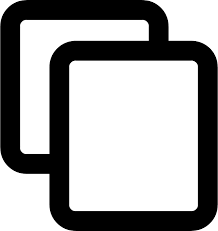
# Listing files in a directory
files = os.listdir("new_folder")
print(files)
This script lists all files and subdirectories in the new_folder
directory and prints the list to the console.
Shutil: Advanced File and Folder Operations
The shutil
module extends the functionality of os
by providing higher-level file operations like copying and moving files.
Copying Files
To copy a file, use shutil.copy()
:
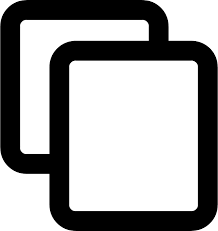
import shutil
# Copying a file
shutil.copy("example.txt", "new_folder/example_copy.txt")
This code copies the file example.txt
to the new_folder
directory and renames it to example_copy.txt
.
Moving Files
To move a file, use shutil.move()
:
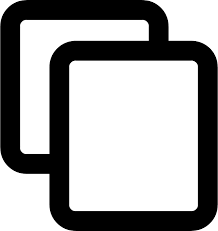
# Moving a file
shutil.move("example.txt", "new_folder/example_moved.txt")
This code moves example.txt
to the new_folder
directory and renames it to example_moved.txt
.
Deleting Folders
To delete an empty folder, use os.rmdir()
. To delete a folder with content, use shutil.rmtree()
:
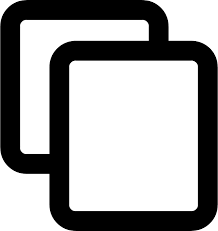
# Deleting a folder with content
shutil.rmtree("new_folder")
The shutil.rmtree()
function deletes the entire folder, including any files inside it.
Pathlib: Object-Oriented File and Directory Management
pathlib
is a modern alternative to the os
and shutil
modules, offering an object-oriented approach to file and directory operations.
Creating and Checking Files with Pathlib
To create a new file or check if a file exists, use pathlib.Path
:
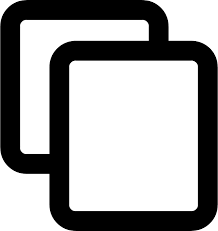
from pathlib import Path
# Creating a new file
file = Path("example_pathlib.txt")
file.write_text("This is a file created using pathlib.")
# Checking if the file exists
if file.exists():
print("File exists!")
This code demonstrates how to create a file using pathlib.Path
and check if the file exists.
Conclusion
Automating file and folder operations with Python is a powerful way to manage your file system efficiently. Whether you are creating, moving, renaming, or deleting files, Python provides a variety of tools and libraries like os
, shutil
, and pathlib
to help streamline these tasks. By automating these processes, you can save time, reduce errors, and improve productivity.
Consuming REST APIs with Python
Consuming REST APIs is a common task in Python when interacting with web services. The requests
module is a simple and elegant way to make HTTP requests to REST APIs and handle responses. This section will introduce you to making GET, POST, PUT, and DELETE requests using the requests
library, as well as handling responses.
What is a REST API?
REST (Representational State Transfer) is an architectural style for designing networked applications. A REST API is an interface that allows you to interact with data over HTTP. REST APIs expose endpoints that can be called using different HTTP methods, such as GET, POST, PUT, and DELETE.
Installing the Requests Library
To use the requests
library, you first need to install it. You can install it using pip
:
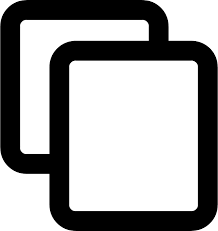
pip install requests
Once installed, you can begin using it to make HTTP requests to REST APIs.
Making HTTP GET Requests
The most common operation when consuming a REST API is making a GET request to retrieve data. Here's how you can do it using the requests
library:
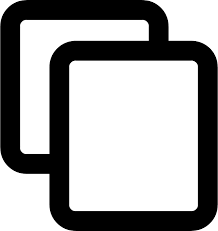
import requests
# Making a GET request to a REST API
response = requests.get("https://api.example.com/data")
# Checking the status code
if response.status_code == 200:
print("Data retrieved successfully!")
print(response.json()) # Print the response data as JSON
else:
print(f"Failed to retrieve data. Status code: {response.status_code}")
In this example, we make a GET request to the API at https://api.example.com/data
. We then check the HTTP response status code. If it's 200, the request was successful, and we print the returned data in JSON format.
Making HTTP POST Requests
To send data to a REST API, you typically use a POST request. Here's an example of how to make a POST request using the requests
library:
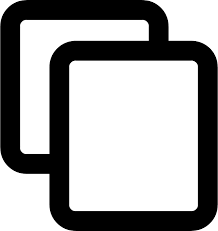
# Data to send in the POST request
data = {
"name": "John",
"email": "john@example.com"
}
# Making a POST request
response = requests.post("https://api.example.com/users", json=data)
# Checking the status code
if response.status_code == 201:
print("User created successfully!")
print(response.json()) # Print the response data as JSON
else:
print(f"Failed to create user. Status code: {response.status_code}")
In this example, we send a JSON payload containing user data to the API endpoint https://api.example.com/users
. The server responds with a status code of 201 if the user is created successfully.
Making HTTP PUT Requests
PUT requests are used to update existing data. Here's an example of how to send a PUT request to update a user's information:
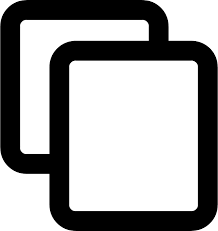
# Data to update
updated_data = {
"email": "newjohn@example.com"
}
# Making a PUT request
response = requests.put("https://api.example.com/users/1", json=updated_data)
# Checking the status code
if response.status_code == 200:
print("User updated successfully!")
print(response.json()) # Print the response data as JSON
else:
print(f"Failed to update user. Status code: {response.status_code}")
In this example, we send a PUT request to update the email of the user with ID 1 at the API endpoint https://api.example.com/users/1
.
Making HTTP DELETE Requests
To delete data from a REST API, you can use a DELETE request. Here's how to send a DELETE request using the requests
library:
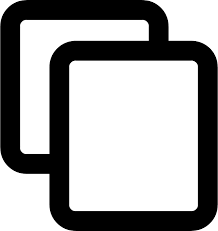
# Making a DELETE request
response = requests.delete("https://api.example.com/users/1")
# Checking the status code
if response.status_code == 204:
print("User deleted successfully!")
else:
print(f"Failed to delete user. Status code: {response.status_code}")
In this example, we send a DELETE request to remove the user with ID 1 at the API endpoint https://api.example.com/users/1
.
Handling Response Data
When consuming REST APIs, the response data is typically returned in JSON format. You can easily parse JSON responses using the response.json()
method:
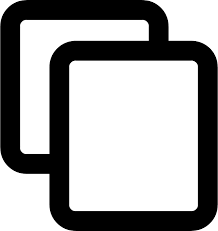
# Making a GET request
response = requests.get("https://api.example.com/data")
# Parsing the JSON response data
data = response.json()
# Accessing specific data in the response
print(data["key1"]) # Access a specific item from the JSON response
In this example, the response.json()
method parses the JSON response, and we access specific data from the response by referring to the keys in the returned dictionary.
Handling Errors
It is essential to handle errors when working with APIs. You can check if the request was successful by examining the status_code
, or you can use the raise_for_status()
method to automatically raise an exception for HTTP errors:
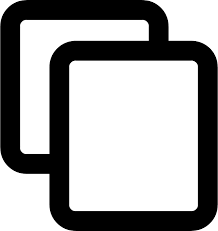
# Making a GET request and handling errors
try:
response = requests.get("https://api.example.com/data")
response.raise_for_status() # Raise an exception for HTTP errors
print(response.json()) # Print the response data
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"An error occurred: {err}")
The raise_for_status()
method will raise a requests.exceptions.HTTPError
if the status code indicates an error (e.g., 404 or 500). This allows you to catch and handle errors more effectively.
Conclusion
Consuming REST APIs with Python is straightforward using the requests
library. Whether you're making GET, POST, PUT, or DELETE requests, the requests
library offers an easy-to-use interface for interacting with APIs. By handling requests and responses properly, you can integrate a wide range of external services and build powerful Python applications.
Creating REST APIs with Flask and Django REST Framework
In this section, we will explore how to create REST APIs using two popular Python frameworks: Flask and Django. Both frameworks provide a simple way to build web services, but they differ in complexity and use cases. Flask is lightweight and flexible, while Django provides a more comprehensive solution with built-in tools for large-scale applications. We will cover the basics of creating REST APIs with both frameworks.
Creating a REST API with Flask
Flask is a micro-framework that is ideal for small to medium-sized applications. It is minimalistic, allowing you to build REST APIs with flexibility. Here's how you can create a simple REST API with Flask:
Step 1: Install Flask
To get started, you need to install Flask:
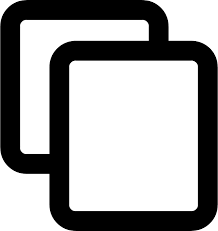
pip install flask
Step 2: Create a Simple API
Now, let's create a basic REST API that returns JSON data:
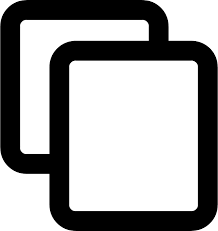
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
data = {"message": "Hello, World!"}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
In this example, we define a Flask route /api/data
that handles GET requests and returns a JSON response with the message "Hello, World!".
Step 3: Run the API
To run the Flask application, execute the following command:
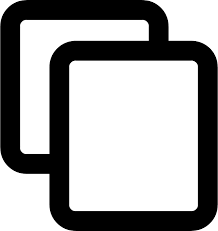
python app.py
The API will be accessible at http://localhost:5000/api/data
.
Creating a REST API with Django REST Framework
Django is a full-fledged web framework, and when combined with the Django REST Framework (DRF), it allows you to build powerful REST APIs. DRF provides a set of tools and features to simplify the process of building APIs, such as serializers, viewsets, and authentication.
Step 1: Install Django and Django REST Framework
First, install Django and DRF:
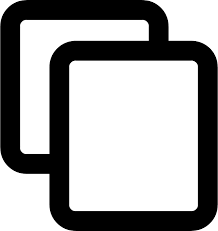
pip install django djangorestframework
Step 2: Create a Django Project
Create a new Django project and app:
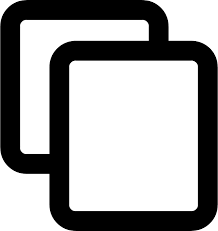
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
Step 3: Define a Model
In your myapp/models.py
file, define a model that will be used to store data for the API:
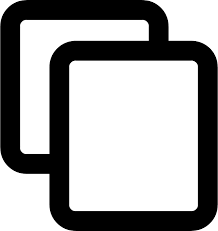
from django.db import models
class Message(models.Model):
content = models.CharField(max_length=200)
def __str__(self):
return self.content
Step 4: Create a Serializer
In myapp/serializers.py
, create a serializer to convert the model data into JSON format:
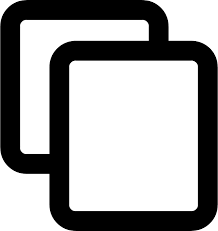
from rest_framework import serializers
from .models import Message
class MessageSerializer(serializers.ModelSerializer):
class Meta:
model = Message
fields = ['id', 'content']
Step 5: Create a Viewset
In myapp/views.py
, create a viewset to handle the API endpoint logic:
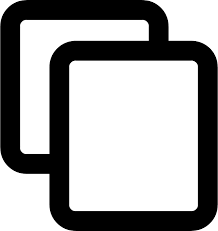
from rest_framework import viewsets
from .models import Message
from .serializers import MessageSerializer
class MessageViewSet(viewsets.ModelViewSet):
queryset = Message.objects.all()
serializer_class = MessageSerializer
Step 6: Define the URL Routes
In myapp/urls.py
, define the URL routes for your API:
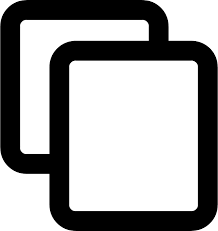
from django.urls import path, include
from rest_framework.routers import DefaultRouter
from .views import MessageViewSet
router = DefaultRouter()
router.register(r'messages', MessageViewSet)
urlpatterns = [
path('api/', include(router.urls)),
]
Step 7: Configure the Django Project
In your myproject/settings.py
, add 'rest_framework'
and 'myapp'
to the INSTALLED_APPS
list:
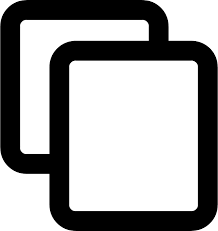
INSTALLED_APPS = [
...
'rest_framework',
'myapp',
]
Step 8: Run the Django API
Run the Django development server:
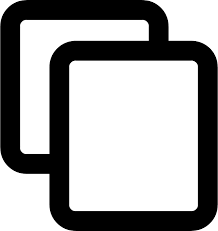
python manage.py runserver
The API will be accessible at http://localhost:8000/api/messages/
, where you can make GET, POST, PUT, and DELETE requests to interact with the data.
Conclusion
Flask and Django REST Framework are both excellent options for creating REST APIs in Python. Flask is great for simple APIs, while Django REST Framework provides a more comprehensive solution with built-in tools for handling complex APIs. Depending on the project size and complexity, you can choose the appropriate framework to suit your needs. Both frameworks are widely used in the industry and offer great flexibility and scalability.
JSON and Python
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. In Python, JSON is often used for data exchange between servers and clients, as well as for storing data in a structured way. Python's json
module provides a simple way to work with JSON data.
Understanding JSON
JSON represents data in a key-value pair format and is widely used in APIs, configuration files, and data storage. It supports basic data types like strings, numbers, and booleans, as well as arrays and objects.
Basic JSON Example
Here’s a basic example of JSON structure:
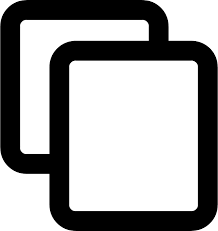
{
"name": "John Doe",
"age": 30,
"is_employee": true,
"skills": ["Python", "JavaScript", "Django"],
"address": {
"street": "123 Main St",
"city": "New York",
"zip": "10001"
}
}
In this JSON example, we have key-value pairs like "name": "John Doe"
and an array "skills": ["Python", "JavaScript"]
, as well as a nested object under the "address"
key.
Working with JSON in Python
Python’s json
module provides methods to parse and generate JSON. The two primary functions are:
json.load()
: Parses a JSON formatted string and converts it into a Python object.json.dump()
: Converts a Python object into a JSON formatted string.
Loading JSON from a String
To parse JSON from a string and convert it into a Python dictionary, we use json.loads()
.
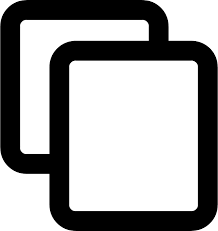
import json
# JSON string
json_string = '{"name": "John Doe", "age": 30, "is_employee": true}'
# Parse JSON string into a Python dictionary
data = json.loads(json_string)
# Accessing data
print(data["name"]) # Output: John Doe
print(data["age"]) # Output: 30
In this example, we use json.loads()
to load the JSON string into a Python dictionary. The key-value pairs can then be accessed like any other dictionary in Python.
Writing JSON to a File
To write JSON data to a file, we can use json.dump()
. Here's an example:
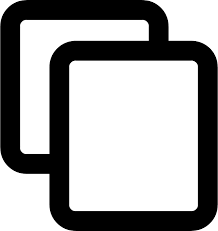
import json
# Data to be written into a JSON file
data = {"name": "John Doe", "age": 30, "is_employee": True}
# Open a file in write mode
with open("data.json", "w") as json_file:
# Write JSON data to the file
json.dump(data, json_file)
In this example, the dictionary data
is written to a file called data.json
. The data will be stored in JSON format.
Reading JSON from a File
To read JSON data from a file and convert it into a Python object, use json.load()
:
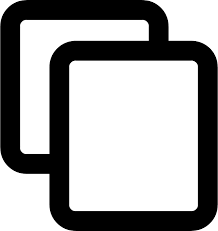
import json
# Open the JSON file in read mode
with open("data.json", "r") as json_file:
# Load the JSON data from the file
data = json.load(json_file)
# Accessing the data
print(data["name"]) # Output: John Doe
print(data["age"]) # Output: 30
The json.load()
function reads the JSON data from the file and converts it back into a Python dictionary, which can be accessed and manipulated as needed.
Common Issues When Working with JSON
While working with JSON, you might encounter common issues such as:
- Invalid JSON format: Ensure that your JSON string is properly formatted with correct syntax, such as using double quotes for keys and values.
- Type mismatches: JSON supports certain data types (string, number, array, etc.), so make sure the data being passed is compatible with JSON.
JSON and Python: Use Cases
JSON is commonly used in Python for the following tasks:
- APIs: Many web APIs provide responses in JSON format, and Python can easily parse and interact with this data.
- Configuration files: JSON is often used in configuration files to store settings in a human-readable format.
- Data exchange: JSON is a widely used format for exchanging data between different systems or programming languages.
Conclusion
JSON is a powerful and widely used format for data interchange, and Python’s json
module makes it easy to work with JSON data. Whether you are building web applications, working with APIs, or automating tasks, understanding how to handle JSON effectively is an essential skill.
Working with GraphQL APIs
GraphQL is a powerful query language for APIs that allows clients to request exactly the data they need, and nothing more. It was developed by Facebook in 2012 and released as an open-source project in 2015. Unlike REST APIs, which rely on multiple endpoints to fetch different data, GraphQL allows clients to interact with a single endpoint to retrieve or modify data based on the query sent.
How GraphQL Works
GraphQL APIs allow you to define the structure of the response data in the query itself, which gives clients more control over what data they receive. This is in contrast to REST APIs, where the server defines the structure of the response.
GraphQL Schema
GraphQL APIs are based on a schema that defines the types of data available. The schema specifies the queries and mutations that clients can make, as well as the data types that can be returned.
Basic GraphQL Example
Here’s an example of a basic GraphQL query:
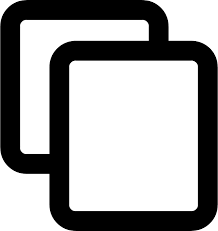
{
"query": "{
user(id: 1) {
name
email
posts {
title
content
}
}
}"
}
This query requests information about a user with the ID of 1, including their name, email, and posts (with title and content). In GraphQL, you specify exactly what fields you want to retrieve.
Setting Up a GraphQL Client in Python
To interact with a GraphQL API from Python, we can use the gql library. This library allows you to send GraphQL queries to an endpoint and process the results easily.
Installing gql
To install the gql
library, use the following command:
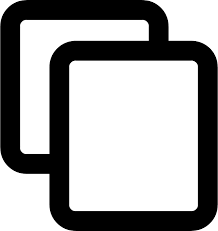
pip install gql
GraphQL Query Example in Python
Here’s how you can send a GraphQL query using Python:
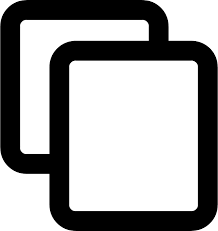
from gql import gql, Client
from gql.transport.requests import RequestsHTTPTransport
# Define the GraphQL endpoint
url = "https://api.example.com/graphql"
# Set up the transport layer
transport = RequestsHTTPTransport(url=url, use_json=True)
# Create the GraphQL client
client = Client(transport=transport, fetch_schema_from_transport=True)
# Define the GraphQL query
query = gql('''
query {
user(id: 1) {
name
email
posts {
title
content
}
}
}
''')
# Execute the query
response = client.execute(query)
# Print the response
print(response)
In this example, we import the necessary components from the gql
library, define the GraphQL endpoint URL, and set up the client. Then, we define the query and use the client.execute()
method to send the query to the server and retrieve the response.
Handling GraphQL Mutations
GraphQL also supports mutations, which are used to modify data on the server. Here’s an example of a mutation that creates a new post:
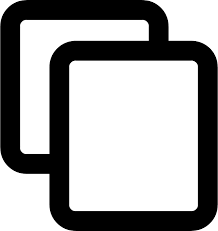
mutation {
createPost(title: "New Post", content: "This is the content of the post") {
title
content
}
}
To send a mutation using Python, the process is similar to sending a query. Here’s how you would handle a mutation in Python:
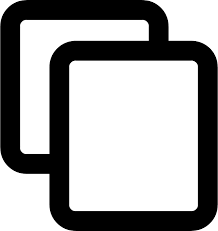
# Define the mutation
mutation = gql('''
mutation {
createPost(title: "New Post", content: "This is the content of the post") {
title
content
}
}
''')
# Execute the mutation
response = client.execute(mutation)
# Print the response
print(response)
Handling Errors
When interacting with a GraphQL API, it's important to handle errors properly. GraphQL responses contain an errors
field that provides information about any issues with the request.
Error Handling Example
Here’s an example of how to check for errors in a GraphQL response:
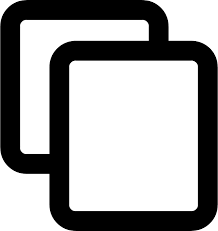
# Check if there are errors in the response
if "errors" in response:
print(f"Error: {response['errors']}")
else:
print(response)
This code checks for the presence of an errors
field in the response and prints any error messages if they exist.
Conclusion
GraphQL provides a more flexible and efficient way to interact with APIs compared to traditional REST APIs. By allowing clients to specify exactly what data they need, GraphQL reduces over-fetching and under-fetching of data, making APIs faster and more efficient. With the gql
library, Python makes it easy to send GraphQL queries and mutations, handle responses, and integrate with GraphQL-based services.
Common Python Errors
When working with Python, errors are bound to occur. Understanding the most common types of errors will help you debug and improve your code. In Python, errors can be categorized into two main types: syntax errors and runtime errors. Let's take a look at the most common Python errors and how to resolve them.
1. SyntaxError
A SyntaxError
occurs when Python cannot parse your code due to incorrect syntax. This usually happens when you forget a colon, parentheses, or quotation mark, or when indentation is incorrect.
Example of SyntaxError
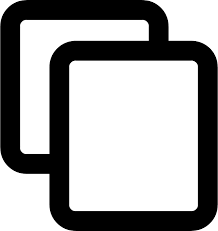
# Incorrect syntax
if x > 10
print("x is greater than 10")
In the above example, a colon is missing after the if
statement, causing a SyntaxError
.
How to Fix
Ensure that all control flow statements end with a colon:
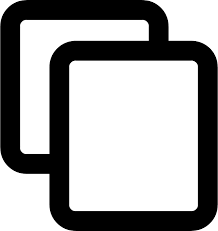
# Corrected code
if x > 10:
print("x is greater than 10")
2. NameError
A NameError
occurs when Python encounters a variable that has not been defined or is misspelled.
Example of NameError
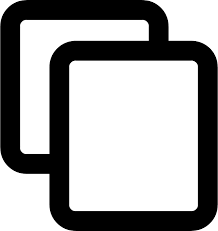
# Incorrect variable name
print(value) # value is not defined
In the above example, value
is not defined before it is used, resulting in a NameError
.
How to Fix
Ensure that all variables are defined before use:
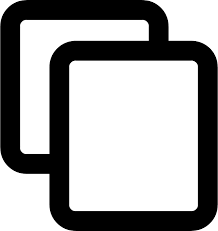
# Corrected code
value = 10
print(value)
3. TypeError
A TypeError
occurs when you try to perform an operation on a value of an inappropriate type. For example, trying to add a string to an integer will raise a TypeError
.
Example of TypeError
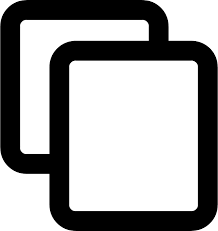
# Adding a string to an integer
result = "Hello" + 5 # TypeError: can only concatenate str (not "int") to str
The above code raises a TypeError
because you cannot concatenate a string and an integer directly.
How to Fix
Ensure that you use the correct types. Convert the integer to a string or use proper type handling:
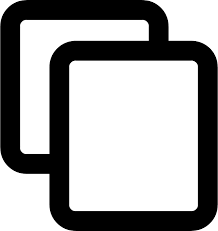
# Corrected code
result = "Hello" + str(5) # No TypeError
4. IndexError
An IndexError
occurs when you try to access an index that is outside the range of a list or other indexable collection.
Example of IndexError
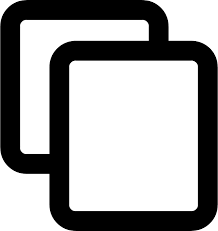
# Accessing an index outside the range of the list
my_list = [1, 2, 3]
print(my_list[5]) # IndexError: list index out of range
The above code raises an IndexError
because index 5 does not exist in the list my_list>.
How to Fix
Ensure that you access valid indices within the range of the collection:
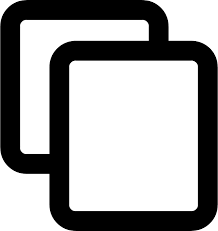
# Corrected code
print(my_list[2]) # No IndexError, outputs 3
5. ValueError
A ValueError
occurs when a function receives an argument of the correct type, but the value is inappropriate.
Example of ValueError
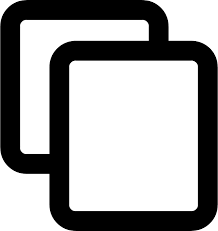
# Converting a string that cannot be converted to an integer
number = int("abc") # ValueError: invalid literal for int() with base 10: 'abc'
In the above example, attempting to convert the string "abc" to an integer raises a ValueError
.
How to Fix
Ensure that the value you are trying to convert is valid for the operation:
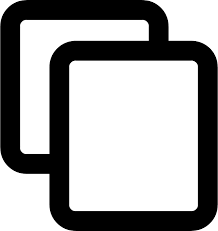
# Corrected code
number = int("123") # No ValueError, outputs 123
6. AttributeError
An AttributeError
occurs when you try to access an attribute or method that does not exist for an object.
Example of AttributeError
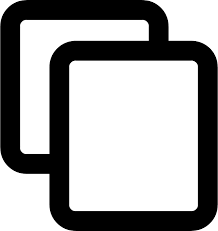
# Calling a method that does not exist
my_string = "Hello"
my_string.append(" World") # AttributeError: 'str' object has no attribute 'append'
In the above example, trying to use the append
method on a string object raises an AttributeError
because strings do not have an append
method.
How to Fix
Ensure that the method or attribute you are calling exists for the object:
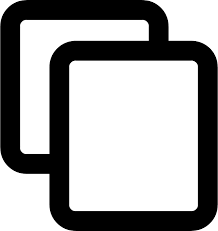
# Corrected code
my_string = "Hello"
my_string += " World" # No AttributeError, outputs "Hello World"
Conclusion
These are some of the most common Python errors that developers encounter. By understanding these errors and knowing how to resolve them, you can improve your debugging skills and write more efficient Python code. Remember to read error messages carefully, as they often provide helpful information about what went wrong and where to fix it.
Using try, except, else, and finally
In Python, exception handling is done using the try
and except
blocks. These blocks allow you to catch exceptions (errors) and handle them gracefully, preventing your program from crashing. Additionally, you can use else
and finally
blocks to specify what happens if no error occurs or after the exception handling is complete.
1. The try Block
The try
block lets you write code that might cause an exception. If no exception occurs, the rest of the code in the block will execute normally. If an exception occurs, Python will stop executing the remaining code in the try
block and jump to the except
block.
Example of try Block
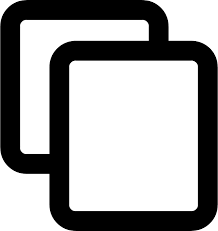
# Using try block to catch errors
try:
x = 10 / 2 # No error
print("Division successful!")
except ZeroDivisionError as e:
print(f"Error: {e}")
If no error occurs (as in the case above), the code inside the try
block executes normally, and the message "Division successful!" is printed.
2. The except Block
The except
block handles the exception. You can specify the type of exception you want to catch, such as ZeroDivisionError
, ValueError
, or a generic exception. If the specified exception occurs, the code inside the except
block will run.
Example of except Block
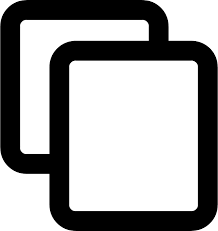
# Using except block to catch specific exception
try:
x = 10 / 0 # This will cause a ZeroDivisionError
except ZeroDivisionError as e:
print(f"Error: {e}")
The code above attempts to divide by zero, which raises a ZeroDivisionError
. The exception is caught by the except
block, and the error message is printed.
3. The else Block
The else
block runs if the code inside the try
block does not raise an exception. It allows you to define actions that should be executed when no error occurs.
Example of else Block
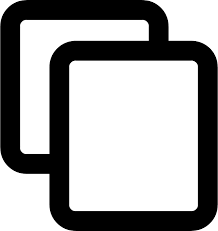
# Using else block when no error occurs
try:
x = 10 / 2 # No error
except ZeroDivisionError as e:
print(f"Error: {e}")
else:
print("No error occurred!")
If no error occurs in the try
block, the else
block will execute, printing "No error occurred!"
4. The finally Block
The finally
block contains code that will always run, regardless of whether an exception occurred or not. It is typically used for cleanup actions such as closing files or releasing resources.
Example of finally Block
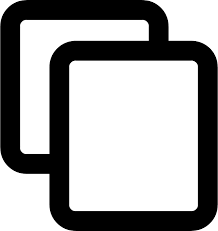
# Using finally block to execute cleanup code
try:
x = 10 / 2 # No error
except ZeroDivisionError as e:
print(f"Error: {e}")
else:
print("No error occurred!")
finally:
print("This will always run!")
In the above example, the message "This will always run!" will be printed regardless of whether an exception occurred.
5. Combining try, except, else, and finally
You can combine all the blocks (try
, except
, else
, and finally
) in a single code structure to handle different scenarios efficiently.
Example of Combining All Blocks
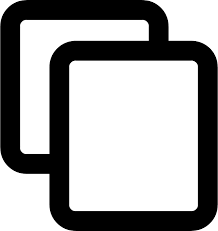
# Combining try, except, else, and finally blocks
try:
x = 10 / 0 # This will cause a ZeroDivisionError
except ZeroDivisionError as e:
print(f"Error: {e}")
else:
print("No error occurred!")
finally:
print("This will always run!")
In this example, the code attempts to divide by zero, which raises a ZeroDivisionError
. The exception is caught by the except
block, and the finally
block runs regardless of whether an error occurred.
Conclusion
Using try
, except
, else
, and finally
blocks provides a powerful way to handle errors and ensure that your program continues running smoothly. The try
and except
blocks help you catch and handle errors, while the else
and finally
blocks give you control over code execution after the exception handling process.
Raising Custom Exceptions
In Python, you can create your own exceptions by defining custom exception classes. These custom exceptions allow you to handle specific error cases in your application more effectively. By using raise
, you can trigger exceptions in your code, including your custom ones.
1. Creating a Custom Exception
To create a custom exception, you define a new class that inherits from the built-in Exception
class. You can also add a custom message to your exception to provide more useful information when it is raised.
Example of a Custom Exception
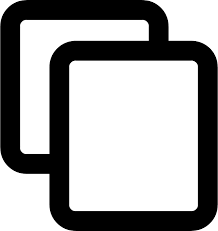
# Custom exception class
class MyCustomError(Exception):
def __init__(self, message):
self.message = message
super().__init__(self.message)
# Raising the custom exception
def my_function():
raise MyCustomError("This is a custom error message.")
try:
my_function()
except MyCustomError as e:
print(f"Error: {e}")
In the above example, we created a custom exception called MyCustomError
that inherits from the Exception
class. When the my_function
function is called, it raises this custom exception, which is caught in the except
block and the error message is printed.
2. Raising the Custom Exception
To raise a custom exception, use the raise
keyword followed by an instance of the exception class. You can also pass custom messages or data to the exception when raising it.
Example of Raising a Custom Exception
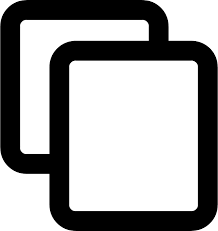
# Function that raises a custom exception
def check_age(age):
if age < 18:
raise MyCustomError("Age must be 18 or older.")
else:
print("Age is valid.")
try:
check_age(15)
except MyCustomError as e:
print(f"Error: {e}")
In this example, the check_age
function raises the MyCustomError
if the age
is less than 18. When the exception is raised, it is caught in the except
block and the custom error message is displayed.
3. Chaining Exceptions
You can also raise a new exception while handling a previous one. This is called "exception chaining," and you can use the from
keyword to chain exceptions together.
Example of Chaining Exceptions
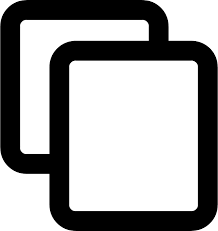
# Custom exception class
class MyCustomError(Exception):
def __init__(self, message):
self.message = message
super().__init__(self.message)
# Raising and chaining exceptions
def function_that_raises():
try:
raise ValueError("A ValueError occurred!")
except ValueError as e:
raise MyCustomError("A custom error occurred due to the ValueError.") from e
try:
function_that_raises()
except MyCustomError as e:
print(f"Error: {e}")
print(f"Caused by: {e.__cause__}")
Here, a ValueError
is raised inside the function_that_raises
function. The MyCustomError
exception is then raised, chaining the two exceptions together. In the except
block, both the custom error message and the original cause of the exception are printed.
4. Customizing Exception Behavior
You can customize the behavior of your custom exceptions further by overriding methods like __str__
or __repr__
, which define how the exception is represented as a string.
Example of Customizing Exception Behavior
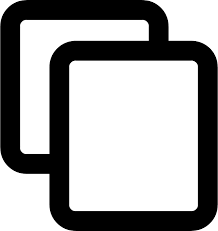
# Custom exception class with custom string representation
class MyCustomError(Exception):
def __init__(self, message, code):
self.message = message
self.code = code
super().__init__(self.message)
def __str__(self):
return f"Error {self.code}: {self.message}"
# Raising the custom exception
try:
raise MyCustomError("Something went wrong!", 404)
except MyCustomError as e:
print(e) # Will print 'Error 404: Something went wrong!'
In this example, the __str__
method is overridden to return a custom string representation of the exception. When the exception is raised, it prints the error message with a custom code.
5. Conclusion
Custom exceptions provide a way to handle specific errors in your application more effectively. By defining your own exception classes and raising them using the raise
keyword, you can create more meaningful error messages and handle specific scenarios in your code.
List Comprehensions and Generator Expressions
List comprehensions and generator expressions are powerful tools in Python that allow you to create and manipulate lists and iterators in a concise, readable manner. They help in reducing the lines of code and making the code more efficient.
1. List Comprehensions
List comprehensions provide a compact way to process all or part of the elements in a list and return a list of results. The syntax for a list comprehension is:
[expression for item in iterable if condition]
Here, the expression
is applied to each item in the iterable
, and the condition
is optional. If provided, only the items that satisfy the condition will be included in the new list.
Example of List Comprehension
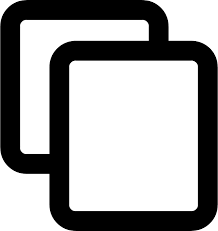
# List comprehension to square numbers from 1 to 5
squares = [x**2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
In this example, the list comprehension creates a list of squares for numbers from 1 to 5. The resulting list is printed to the console.
List Comprehension with Condition
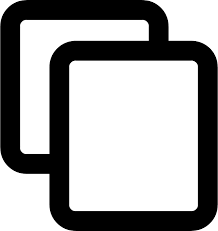
# List comprehension with a condition
even_squares = [x**2 for x in range(1, 6) if x % 2 == 0]
print(even_squares) # Output: [4, 16]
Here, the list comprehension squares only even numbers from the range 1 to 5, resulting in the list [4, 16].
2. Generator Expressions
Generator expressions are similar to list comprehensions, but instead of returning a list, they return a generator object. A generator object is an iterator, which means it yields values one at a time as they are iterated over, making it more memory-efficient for large datasets.
Syntax of a Generator Expression
(expression for item in iterable if condition)
Like list comprehensions, generator expressions can include an optional condition. However, they return a generator object, which is iterated over using a for
loop or by converting it to a list or another collection type.
Example of Generator Expression
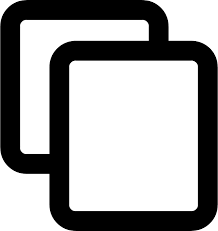
# Generator expression to square numbers from 1 to 5
gen = (x**2 for x in range(1, 6))
print(gen) # Output: at 0x...>
In this example, a generator expression is used to create a generator that yields the squares of numbers from 1 to 5. The output shows that it is a generator object.
Iterating over a Generator
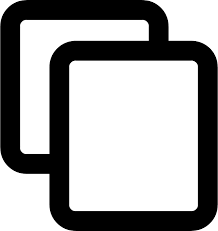
# Iterating over the generator to get values
for value in gen:
print(value)
# Output: 1 4 9 16 25
Here, we iterate over the generator object using a for
loop to print each squared value.
Converting Generator to List
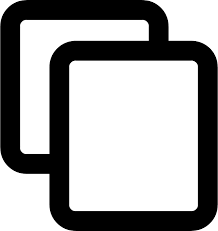
# Converting generator to a list
gen_list = list((x**2 for x in range(1, 6)))
print(gen_list) # Output: [1, 4, 9, 16, 25]
We can convert the generator to a list using the list()
function, which will give us a list of the squares.
3. Key Differences Between List Comprehensions and Generator Expressions
- List comprehensions generate the entire list in memory at once, while generator expressions create an iterator that yields values one by one, making them more memory-efficient.
- List comprehensions return a list, while generator expressions return a generator object, which can be iterated over lazily.
- List comprehensions are typically used when you need to store all the results in memory at once, while generator expressions are useful for large datasets or when you only need to iterate over values without storing them in memory.
4. Conclusion
List comprehensions and generator expressions are both powerful features in Python that help make your code more concise and efficient. List comprehensions are suitable for cases where you need the entire list in memory, while generator expressions are ideal for handling large datasets efficiently by yielding values one at a time.
Decorators: Basics and Use Cases
Decorators in Python are a powerful and elegant way to modify or enhance the behavior of functions or methods without changing their actual code. They are commonly used in scenarios such as logging, access control, memoization, and more. A decorator is essentially a function that takes another function as an argument and returns a new function that adds some functionality.
1. What is a Decorator?
A decorator is a function that wraps another function to extend or alter its behavior. It is applied using the @decorator_name
syntax, right before the function definition. Decorators allow you to write cleaner code by abstracting repetitive tasks into reusable components.
Basic Decorator Syntax
def decorator_function(original_function):
def wrapper_function():
print("Wrapper executed this before {}".format(original_function.__name__))
return original_function()
return wrapper_function
@decorator_function
def display():
return "Display function executed"
print(display()) # Output: Wrapper executed this before display
# Display function executed
In this example, the decorator_function
takes the display
function as an argument, wraps it inside wrapper_function
, and adds some additional logic before executing the original function.
2. How Decorators Work
When the @decorator_function
is applied to the display
function, it is equivalent to calling:
display = decorator_function(display)
This means that the display
function is now replaced by wrapper_function
, which adds extra functionality (like printing a message) before calling the original function.
3. Use Cases for Decorators
Decorators are commonly used in a variety of real-world scenarios. Some common use cases include:
3.1 Logging
Decorators are often used to log function calls, arguments, and return values without modifying the function itself. This is useful for debugging or tracking function execution.
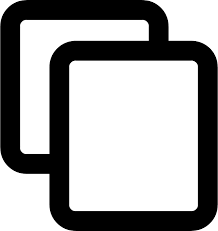
def log_function_call(func):
def wrapper(*args, **kwargs):
print("Function {} was called with arguments: {} and keyword arguments: {}".format(func.__name__, args, kwargs))
return func(*args, **kwargs)
return wrapper
@log_function_call
def add(a, b):
return a + b
add(2, 3) # Output: Function add was called with arguments: (2, 3) and keyword arguments: {}
# 5
In this example, the log_function_call
decorator logs the function name, arguments, and keyword arguments every time the add
function is called.
3.2 Authentication and Access Control
Decorators can also be used for checking user authentication or permissions before executing certain functions, such as in web applications.
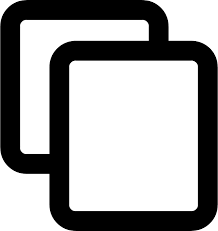
def require_authentication(func):
def wrapper(*args, **kwargs):
if not user_is_authenticated():
raise PermissionError("User is not authenticated")
return func(*args, **kwargs)
return wrapper
@require_authentication
def access_dashboard():
return "Welcome to the dashboard!"
# This will raise PermissionError if user_is_authenticated() is False
The require_authentication
decorator checks if the user is authenticated before executing the access_dashboard
function. If not, it raises a PermissionError
.
3.3 Caching / Memoization
Decorators can be used to store (cache) the results of expensive function calls and return the cached result when the same inputs occur again, thus improving performance.
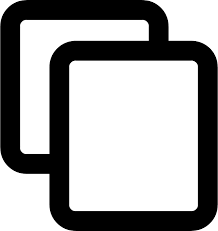
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def slow_function(n):
print(f"Calculating slow_function({n})")
return n * n
print(slow_function(4)) # Output: Calculating slow_function(4)
# 16
print(slow_function(4)) # Output: 16 (result is cached, no calculation)
The memoize
decorator caches the result of slow_function
for each argument so that repeated calls with the same argument don't require recalculation.
4. Chaining Decorators
You can apply multiple decorators to a single function. They will be applied from the inside out (bottom to top). This is useful when you need to apply multiple behaviors to the same function.
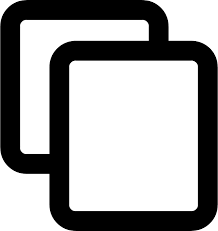
def decorator_one(func):
def wrapper():
print("Decorator One")
return func()
return wrapper
def decorator_two(func):
def wrapper():
print("Decorator Two")
return func()
return wrapper
@decorator_one
@decorator_two
def greet():
return "Hello!"
greet() # Output: Decorator One
# Decorator Two
# Hello!
In this example, both decorators are applied to the greet
function. The decorators are applied in the order of @decorator_one
first, then @decorator_two
.
5. Conclusion
Decorators are a powerful feature in Python that allows you to modify the behavior of functions in a clean and reusable way. They are commonly used for logging, authentication, caching, and more. By learning how to use decorators, you can write more concise, modular, and maintainable code.
Iterators and Generators
In Python, iterators and generators are used to iterate over sequences of data. They provide efficient ways to process large datasets by allowing you to work with one item at a time, rather than loading everything into memory at once. This makes them ideal for scenarios with large amounts of data or when you want to lazily evaluate a sequence.
1. What is an Iterator?
An iterator is an object that implements two key methods: __iter__()
and __next__()
. The __iter__()
method returns the iterator object itself and is required for the object to be iterable. The __next__()
method returns the next item in the sequence. When there are no more items to return, StopIteration
is raised, signaling the end of iteration.
Creating an Iterator
Here's an example of how you can create and use an iterator in Python:
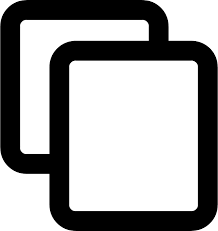
class MyIterator:
def __init__(self, start, end):
self.current = start
self.end = end
def __iter__(self):
return self
def __next__(self):
if self.current < self.end:
value = self.current
self.current += 1
return value
else:
raise StopIteration
# Using the iterator
my_iter = MyIterator(1, 5)
for num in my_iter:
print(num) # Output: 1, 2, 3, 4
In this example, the MyIterator
class is a custom iterator that starts from a given start
value and iterates until the end
value is reached. The __next__()
method keeps returning the next number until it raises the StopIteration
exception.
2. What is a Generator?
A generator is a special type of iterator that is defined using a function with the yield
keyword. Instead of returning all values at once, a generator "yields" values one at a time, pausing its execution and resuming where it left off each time next()
is called. Generators are memory efficient because they generate values on-the-fly and do not store them in memory.
Creating a Generator
Here is how you can create a generator using the yield
keyword:
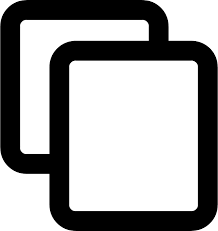
def my_generator(start, end):
while start < end:
yield start
start += 1
# Using the generator
gen = my_generator(1, 5)
for num in gen:
print(num) # Output: 1, 2, 3, 4
In this example, the my_generator
function is a generator that yields numbers from start
to end
. Each time the generator is called, it produces a value and pauses until the next value is requested.
3. Difference Between Iterators and Generators
While both iterators and generators allow you to iterate over a sequence of data, they differ in how they are implemented:
- Iterators: Require explicit class definitions, implementing the
__iter__
and__next__
methods. - Generators: Are defined using a function with the
yield
keyword. They are simpler and more concise than iterators. - Memory Usage: Generators are more memory efficient because they generate values one at a time and do not store the entire sequence in memory.
4. Advantages of Using Generators
Generators have several advantages over other iteration methods:
- Memory Efficiency: Since generators do not store all values in memory, they are ideal for processing large datasets.
- Lazy Evaluation: Generators evaluate values on-the-fly, which can improve performance when working with large sequences.
- Concise Code: Generators are often simpler and shorter than iterators, especially for simple cases.
5. Using Generators with Built-in Functions
Many Python built-in functions work seamlessly with generators. For example, the sum()
function can be used with a generator to calculate the sum of values in a sequence:
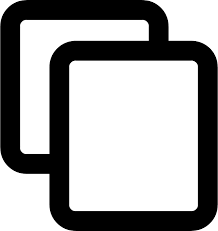
gen = my_generator(1, 5)
print(sum(gen)) # Output: 10
Here, the sum()
function calculates the sum of values generated by my_generator
without storing all values in memory.
6. Generator Expressions
Similar to list comprehensions, Python also provides generator expressions. These expressions allow you to create generators in a concise way:
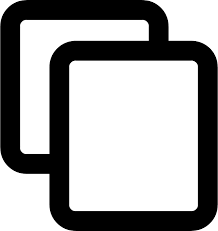
gen_expr = (x * x for x in range(1, 5))
for num in gen_expr:
print(num) # Output: 1, 4, 9, 16
In this example, a generator expression creates a generator that yields the square of numbers from 1 to 4. It works similarly to a list comprehension, but without creating an entire list in memory.
7. Conclusion
Iterators and generators are essential tools for handling large datasets in Python. Generators, in particular, offer a memory-efficient way to iterate over data, making them ideal for working with large files or streams of data. By using these tools, you can write more efficient and scalable Python code.
Context Managers (with Statement)
In Python, the with
statement is used to simplify the management of resources, such as file handling, database connections, or network sockets. It ensures that resources are properly acquired and released, even in the case of errors or exceptions. The with
statement works with objects that implement context management, which defines how to set up and tear down resources.
1. What is a Context Manager?
A context manager is a Python object that implements the __enter__()
and __exit__()
methods. The __enter__()
method is executed when the context is entered, and __exit__()
is executed when the context is exited. This allows for proper resource management, such as opening and closing a file, acquiring and releasing locks, etc.
Creating a Context Manager
Here’s how you can create a custom context manager by implementing the __enter__()
and __exit__()
methods:
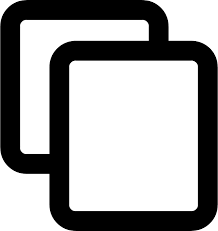
class MyContextManager:
def __enter__(self):
print("Entering the context")
return self # The value returned by __enter__ can be accessed in the 'with' block
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context")
# Handle exceptions if any
if exc_type:
print(f"An error occurred: {exc_value}")
return True # Suppresses the exception if True
# Using the context manager
with MyContextManager() as cm:
print("Inside the context")
# Uncomment the next line to raise an exception and see how it's handled
# raise ValueError("An error occurred")
In this example, the MyContextManager
class defines a context manager that prints messages when entering and exiting the context. If an exception occurs inside the with
block, the __exit__()
method will handle it and suppress the exception if necessary.
2. Using the with
Statement for File Handling
The most common use of context managers is for file handling. The with
statement ensures that files are properly opened and closed, even if an exception occurs while reading or writing the file.
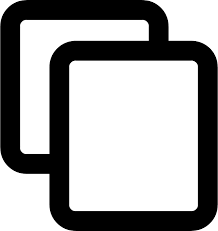
# Using 'with' statement to open and automatically close a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
print("File written successfully")
# No need to explicitly call file.close(), it is done automatically
In this example, the file example.txt
is opened in write mode using the with
statement. The file is automatically closed when the block is exited, even if an error occurs during the writing process.
3. Handling Exceptions in a Context Manager
Context managers can handle exceptions that occur inside the with
block. The __exit__()
method receives information about the exception, such as its type, value, and traceback. You can use this information to manage exceptions appropriately.
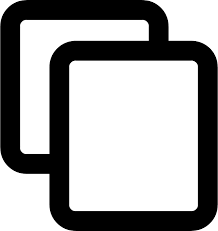
class ErrorHandlingContextManager:
def __enter__(self):
print("Entering context")
def __exit__(self, exc_type, exc_value, traceback):
if exc_type:
print(f"Exception caught: {exc_value}")
print("Exiting context")
return True # Suppresses the exception
with ErrorHandlingContextManager():
print("Inside the context")
# Raising an exception to test error handling
raise ValueError("Something went wrong")
In this example, the ErrorHandlingContextManager
catches the exception and prints the error message. By returning True
from the __exit__()
method, the exception is suppressed and doesn't propagate beyond the with
block.
4. Using Contextlib for Simpler Context Managers
Python provides the contextlib
module, which simplifies the creation of context managers. The contextlib.contextmanager
decorator allows you to write a context manager using a generator function, which is more concise than implementing the __enter__()
and __exit__()
methods manually.
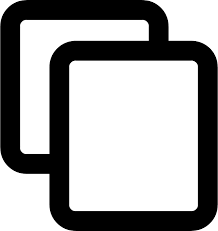
from contextlib import contextmanager
@contextmanager
def my_simple_context():
print("Entering context")
yield
print("Exiting context")
# Using the simple context manager
with my_simple_context():
print("Inside the context")
In this example, the my_simple_context
function is a context manager created using the contextmanager
decorator. The yield
keyword is used to mark the point where the code inside the with
block is executed. The code after the yield
is executed when the block is exited.
5. Use Cases for Context Managers
Context managers are useful in various scenarios where resource management is required:
- File Handling: Ensure files are properly opened and closed after reading or writing.
- Database Connections: Automatically open and close database connections.
- Network Connections: Manage network resources, ensuring connections are properly closed.
- Thread Locks: Manage locks in multi-threaded programs to avoid race conditions.
6. Conclusion
The with
statement and context managers provide an elegant and efficient way to manage resources in Python. By handling the setup and teardown of resources automatically, they reduce boilerplate code and help ensure that resources are properly cleaned up, even in the presence of errors. For simple use cases, the contextlib
module offers an easy way to create context managers using generator functions.
Regular Expressions (re Module)
Regular expressions (regex) are a powerful tool for working with text data. They allow you to search, match, and manipulate strings based on specific patterns. The re
module in Python provides functions to work with regular expressions, enabling you to perform complex string operations such as searching, replacing, and splitting strings based on patterns.
1. What Are Regular Expressions?
A regular expression is a sequence of characters that forms a search pattern. Regular expressions are used for pattern matching with strings, which can help with tasks like validating input, extracting information, and performing search-and-replace operations.
For example, a regular expression can be used to check if a string is an email address, a phone number, or a URL. The re
module in Python provides a way to work with regular expressions and apply them to strings.
2. Basic Syntax of Regular Expressions
Here are some basic components of regular expressions:
- .: Matches any single character except newline.
- \d: Matches any digit (0-9).
- \D: Matches any non-digit character.
- \w: Matches any alphanumeric character (letters and digits) and underscore.
- \W: Matches any non-alphanumeric character.
- \s: Matches any whitespace character (space, tab, newline).
- \S: Matches any non-whitespace character.
- []: Defines a character class (e.g., [a-z] matches any lowercase letter).
- ^: Anchors the match at the beginning of the string.
- $: Anchors the match at the end of the string.
- *: Matches zero or more occurrences of the preceding element.
- +: Matches one or more occurrences of the preceding element.
- ?: Matches zero or one occurrence of the preceding element.
- {} : Specifies the exact number of occurrences (e.g., {2} matches exactly two occurrences).
- |: Acts as an OR operator.
3. Functions in the re
Module
The re
module provides several functions to work with regular expressions:
- re.match(): Matches a pattern at the beginning of a string.
- re.search(): Searches for a pattern anywhere in the string.
- re.findall(): Returns a list of all occurrences of the pattern in the string.
- re.sub(): Replaces occurrences of a pattern with a specified string.
- re.split(): Splits the string by occurrences of the pattern.
4. Examples of Using Regular Expressions
Example 1: Using re.match()
re.match()
checks for a match only at the beginning of the string:
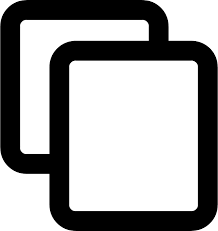
import re
# Checking if the string starts with 'Hello'
result = re.match(r"Hello", "Hello, World!")
if result:
print("Match found:", result.group())
else:
print("No match found")
This code checks if the string starts with the word "Hello". If it does, the match is found; otherwise, no match is returned.
Example 2: Using re.search()
re.search()
searches for a pattern anywhere in the string:
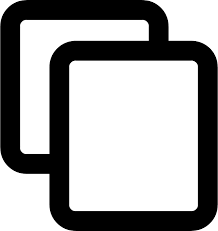
import re
# Searching for a pattern anywhere in the string
result = re.search(r"World", "Hello, World!")
if result:
print("Pattern found:", result.group())
else:
print("Pattern not found")
This code searches for the word "World" anywhere in the string. If found, it prints the matched pattern.
Example 3: Using re.findall()
re.findall()
returns a list of all matches found in the string:
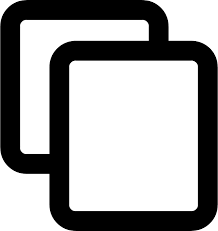
import re
# Finding all occurrences of digits in the string
result = re.findall(r"\d+", "There are 15 apples and 20 oranges.")
print("Numbers found:", result)
This code finds all sequences of digits in the string and returns them in a list.
Example 4: Using re.sub()
re.sub()
replaces occurrences of a pattern with a specified string:
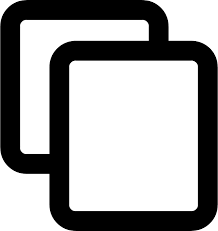
import re
# Replacing 'apples' with 'oranges' in the string
result = re.sub(r"apples", "oranges", "There are 15 apples and 20 apples.")
print("Modified string:", result)
This code replaces the word "apples" with "oranges" in the string.
Example 5: Using re.split()
re.split()
splits the string by the occurrences of a pattern:
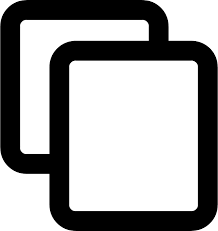
import re
# Splitting the string by spaces
result = re.split(r"\s+", "This is a test string.")
print("Split string:", result)
This code splits the string into a list of words by spaces.
5. Pattern Matching Examples
Here are some common use cases for regular expressions:
- Email Validation: Use a regular expression to validate email addresses.
- Phone Number Validation: Validate phone numbers with specific formats.
- Extracting Data: Use regular expressions to extract specific patterns like dates, URLs, etc.
6. Conclusion
The re
module in Python is a powerful tool for working with strings and pattern matching. Regular expressions can be used for a variety of tasks, such as validating user input, extracting data, and performing complex string manipulations. Understanding the basic syntax and functions in the re
module will help you harness the full potential of regular expressions in your Python programs.
Introduction to Databases in Python
Databases are essential for storing and managing large amounts of data. In Python, you can interact with databases using various libraries and modules. This section introduces how to work with databases in Python, focusing on relational databases like SQLite and MySQL, and how to use Python to interact with them effectively.
1. What Are Databases?
A database is a structured collection of data that can be easily accessed, managed, and updated. It is typically stored in tables with rows and columns. Databases are widely used in applications to store data such as user information, product details, and transaction history.
There are two main types of databases:
- Relational Databases (SQL): These databases store data in tables with predefined relationships between them (e.g., MySQL, PostgreSQL, SQLite).
- Non-Relational Databases (NoSQL): These databases store data in formats like key-value pairs, documents, or graphs (e.g., MongoDB, Redis).
2. Connecting to a Database in Python
Python provides libraries to connect to relational databases, allowing you to execute queries, retrieve data, and manage records. Some commonly used libraries for database interaction include:
- SQLite: A lightweight, serverless database that is part of the Python standard library.
- MySQL and PostgreSQL: Popular relational databases that can be connected to using external libraries like
mysql-connector-python
andpsycopg2
.
3. Working with SQLite in Python
SQLite is a serverless, self-contained, and lightweight database engine. Python’s sqlite3
module allows you to work with SQLite databases directly within Python.
To connect to an SQLite database, use the sqlite3.connect()
function:
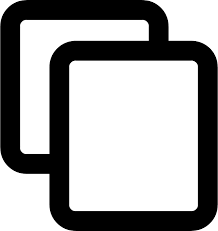
import sqlite3
# Connect to an SQLite database (or create one if it doesn't exist)
connection = sqlite3.connect('example.db')
# Create a cursor object to interact with the database
cursor = connection.cursor()
# Create a table
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
# Commit the changes and close the connection
connection.commit()
connection.close()
This example shows how to create a new SQLite database and a table called users
with three columns: id
, name
, and age
.
4. Inserting Data into a Database
To insert data into a database, you can use the INSERT INTO
SQL statement. The following example demonstrates how to insert records into the users
table:
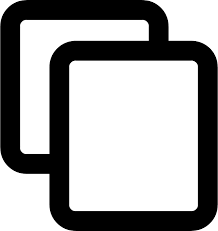
# Connect to the database
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Insert data into the users table
cursor.execute("INSERT INTO users (name, age) VALUES ('Alice', 30)")
# Commit the changes and close the connection
connection.commit()
connection.close()
This code inserts a new user named "Alice" with an age of 30 into the database.
5. Retrieving Data from a Database
You can retrieve data from a database using the SELECT
SQL query. The following example shows how to fetch all users from the users
table:
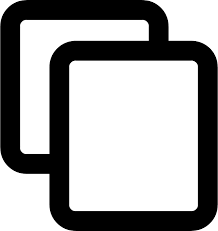
# Connect to the database
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Retrieve all users from the users table
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
# Print the retrieved data
for row in rows:
print(row)
# Close the connection
connection.close()
This code fetches all users from the table and prints each record. The fetchall()
method retrieves all the rows from the query result.
6. Updating Data in a Database
You can update records in a database using the UPDATE
SQL statement. Here's how to update a user's information in the users
table:
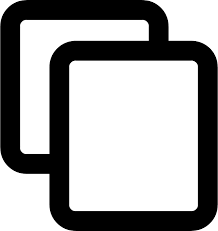
# Connect to the database
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Update a user's age
cursor.execute("UPDATE users SET age = 31 WHERE name = 'Alice'")
# Commit the changes and close the connection
connection.commit()
connection.close()
This example updates Alice's age to 31 in the database.
7. Deleting Data from a Database
To delete data from a database, you can use the DELETE
SQL statement. Here's an example of deleting a user from the users
table:
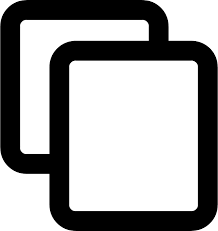
# Connect to the database
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Delete a user by name
cursor.execute("DELETE FROM users WHERE name = 'Alice'")
# Commit the changes and close the connection
connection.commit()
connection.close()
This code deletes the user named "Alice" from the table.
8. Using MySQL with Python
To work with MySQL databases in Python, you can use the mysql-connector-python
library. First, install the library using pip
:
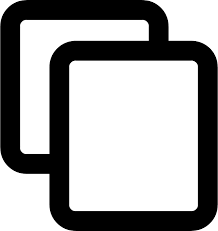
pip install mysql-connector-python
Once installed, you can connect to a MySQL database and execute queries using similar methods as with SQLite:
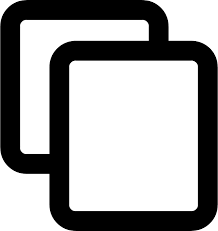
import mysql.connector
# Connect to the MySQL database
connection = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="mydatabase"
)
cursor = connection.cursor()
# Execute a query
cursor.execute("SELECT * FROM users")
# Fetch all rows and print them
rows = cursor.fetchall()
for row in rows:
print(row)
# Close the connection
connection.close()
This example shows how to connect to a MySQL database and retrieve data from it using Python.
9. Conclusion
Working with databases in Python is straightforward and powerful. Whether you're using SQLite for lightweight, file-based storage or MySQL for more complex, server-based applications, Python provides libraries and tools that simplify database interaction. By mastering database connections, SQL queries, and basic data manipulation techniques, you can build robust applications that efficiently manage large datasets.
Using SQLite with sqlite3
SQLite is a lightweight, serverless, and self-contained database engine that is widely used for small to medium-sized applications. Python includes a built-in library sqlite3
that makes it easy to interact with SQLite databases. In this section, we will learn how to use the sqlite3
module to create databases, tables, and manipulate data within an SQLite database.
1. What is SQLite?
SQLite is a relational database management system (RDBMS) that stores data in a file format. It is self-contained, which means there is no need for a separate database server. This makes SQLite a great choice for embedded applications, small projects, or local data storage. It is serverless and provides a simple API for developers to interact with the database in a file-based format.
2. Setting Up SQLite with Python
SQLite comes pre-installed with Python, so you don’t need to install any external libraries to use it. You can start by importing the sqlite3
module, which is part of the standard Python library.
Here's how you can import the module:
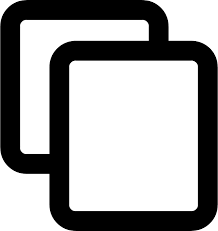
import sqlite3
3. Connecting to an SQLite Database
To work with an SQLite database, you first need to connect to it. If the specified database does not exist, SQLite will create a new database file. The connect()
method is used to establish the connection.
Here’s how to connect to an SQLite database:
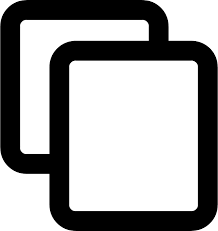
# Connect to an SQLite database (or create one if it doesn't exist)
connection = sqlite3.connect('mydatabase.db')
# Create a cursor object to interact with the database
cursor = connection.cursor()
This code creates a connection to an SQLite database file named mydatabase.db
and creates a cursor object to interact with the database.
4. Creating a Table
Once connected to the database, you can create tables to store data. You can use SQL commands like CREATE TABLE
to define the structure of the table.
Here’s an example of how to create a table in SQLite:
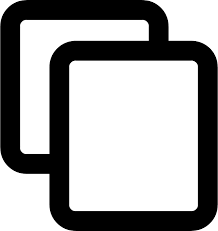
# Create a table in the database
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
# Commit the changes
connection.commit()
This code creates a table named users
with three columns: id
(integer, primary key), name
(text), and age
(integer).
5. Inserting Data into a Table
To insert data into an SQLite table, you can use the INSERT INTO
SQL statement. Here’s how you can insert a record into the users
table:
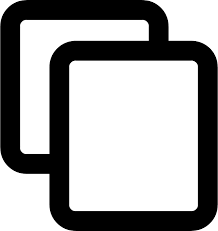
# Insert a new user into the users table
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('Alice', 30))
# Commit the changes
connection.commit()
In this example, a new record is inserted into the users
table with the name "Alice" and age 30. The ?
placeholders are used to safely insert values into the SQL query to prevent SQL injection attacks.
6. Retrieving Data from the Database
You can retrieve data from the database using the SELECT
SQL statement. The fetchall()
method retrieves all the rows from the query result.
Here’s how to retrieve data from the users
table:
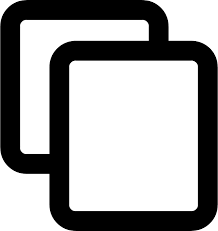
# Retrieve all users from the users table
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
# Print the retrieved data
for row in rows:
print(row)
This code fetches all the records from the users
table and prints each row. Each row is returned as a tuple containing the values from the table columns.
7. Updating Data in the Database
To update existing data in the database, you can use the UPDATE
SQL statement. Here’s an example that updates a user's age:
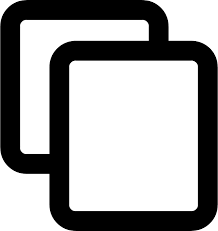
# Update a user's age
cursor.execute("UPDATE users SET age = ? WHERE name = ?", (31, 'Alice'))
# Commit the changes
connection.commit()
This code updates the age of the user "Alice" to 31 in the users
table.
8. Deleting Data from the Database
If you want to delete records from the database, use the DELETE
SQL statement. Here's how you can delete a user:
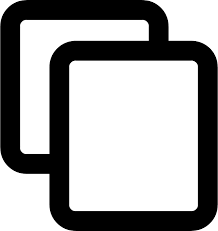
# Delete a user by name
cursor.execute("DELETE FROM users WHERE name = ?", ('Alice',))
# Commit the changes
connection.commit()
This code deletes the user named "Alice" from the users
table.
9. Closing the Connection
Once you’re done interacting with the database, it’s important to close the connection to free up resources:
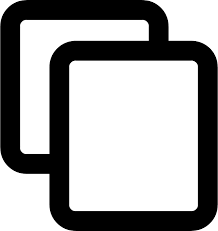
# Close the connection
connection.close()
10. Conclusion
SQLite provides a simple and efficient way to interact with relational databases in Python. The sqlite3
module offers all the necessary tools to create databases, tables, and perform basic operations like inserting, updating, and deleting data. It is a great tool for small projects, testing, and applications where a full-fledged database server is not necessary.
Connecting to MySQL and PostgreSQL
In Python, you can use different libraries to connect to relational databases like MySQL and PostgreSQL. For MySQL, the most common library is mysql-connector-python
, and for PostgreSQL, you can use psycopg2
. In this section, we will explore how to connect to both MySQL and PostgreSQL databases, execute queries, and fetch results.
1. Connecting to MySQL
To connect to a MySQL database in Python, you need to install the mysql-connector-python
library. You can install it using pip
:
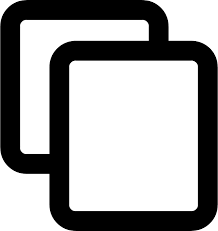
pip install mysql-connector-python
Once installed, you can use the following code to connect to your MySQL database:
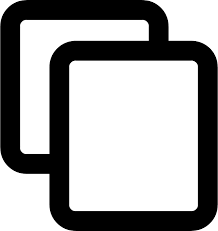
import mysql.connector
# Connect to the MySQL database
connection = mysql.connector.connect(
host="localhost", # Database host
user="your_username", # Database username
password="your_password",# Database password
database="your_database" # Database name
)
# Create a cursor object to interact with the database
cursor = connection.cursor()
# Execute a query
cursor.execute("SELECT * FROM users")
# Fetch all results
rows = cursor.fetchall()
for row in rows:
print(row)
# Close the connection
cursor.close()
connection.close()
This code demonstrates how to connect to a MySQL database, execute a query, and fetch results. You can replace the host
, user
, password
, and database
with your own database credentials.
2. Handling MySQL Errors
When working with MySQL, it's important to handle errors properly. You can use try-except
blocks to catch exceptions and handle any errors that occur during the connection or query execution process:
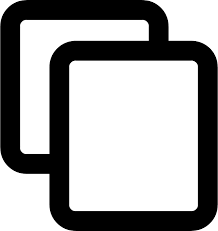
try:
# Attempt to connect to MySQL database
connection = mysql.connector.connect(
host="localhost",
user="your_username",
password="your_password",
database="your_database"
)
cursor = connection.cursor()
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
for row in rows:
print(row)
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
This code will catch any exceptions that occur during the connection or query execution and print an error message. Finally, it ensures that the connection is closed.
3. Connecting to PostgreSQL
To connect to a PostgreSQL database in Python, you need to install the psycopg2
library. You can install it using pip
:
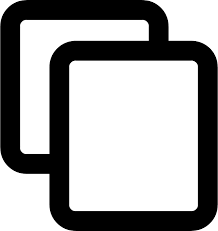
pip install psycopg2
Once installed, you can use the following code to connect to your PostgreSQL database:
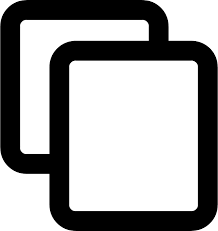
import psycopg2
# Connect to the PostgreSQL database
connection = psycopg2.connect(
host="localhost", # Database host
user="your_username", # Database username
password="your_password",# Database password
dbname="your_database" # Database name
)
# Create a cursor object to interact with the database
cursor = connection.cursor()
# Execute a query
cursor.execute("SELECT * FROM users")
# Fetch all results
rows = cursor.fetchall()
for row in rows:
print(row)
# Close the connection
cursor.close()
connection.close()
This code demonstrates how to connect to a PostgreSQL database, execute a query, and fetch results. You can replace the host
, user
, password
, and dbname
with your own database credentials.
4. Handling PostgreSQL Errors
Similar to MySQL, you should handle errors in PostgreSQL using try-except
blocks:
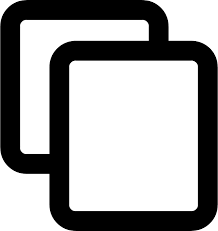
try:
# Attempt to connect to PostgreSQL database
connection = psycopg2.connect(
host="localhost",
user="your_username",
password="your_password",
dbname="your_database"
)
cursor = connection.cursor()
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
for row in rows:
print(row)
except psycopg2.Error as err:
print(f"Error: {err}")
finally:
if connection:
cursor.close()
connection.close()
This code will catch any exceptions that occur during the connection or query execution and print an error message. The connection is closed in the finally
block to ensure proper cleanup.
5. Conclusion
Connecting to MySQL and PostgreSQL databases in Python is straightforward using the mysql-connector-python
and psycopg2
libraries. Both libraries provide methods for establishing a connection, executing queries, handling errors, and closing the connection. These libraries can be used in various types of applications to interact with relational databases in Python.
ORMs: Introduction to SQLAlchemy and Django ORM
Object-Relational Mappers (ORMs) are tools that allow developers to interact with relational databases using object-oriented programming (OOP) concepts. Two popular ORMs in Python are SQLAlchemy
and Django ORM
. These ORMs abstract away the need to write raw SQL queries, enabling developers to work with database tables as Python objects.
1. Introduction to SQLAlchemy
SQLAlchemy is a powerful and flexible ORM that supports both simple and complex database interactions. It provides an efficient way to map Python classes to database tables and allows you to perform CRUD operations without writing raw SQL queries. It works with many relational databases like MySQL, PostgreSQL, SQLite, and more.
Installing SQLAlchemy
To install SQLAlchemy, use pip
:
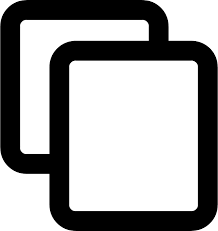
pip install sqlalchemy
Basic SQLAlchemy Example
Here’s a simple example that demonstrates how to use SQLAlchemy to define a database model and interact with a database:
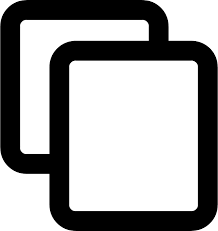
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Create a base class for your models
Base = declarative_base()
# Define a model (table)
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String, nullable=False)
age = Column(Integer)
# Create an engine to connect to the database
engine = create_engine('sqlite:///users.db')
# Create tables in the database
Base.metadata.create_all(engine)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
# Create a new user
new_user = User(name="John Doe", age=30)
# Add the user to the session and commit to the database
session.add(new_user)
session.commit()
# Query the database
users = session.query(User).all()
for user in users:
print(f"{user.id}: {user.name}, {user.age} years old")
# Close the session
session.close()
This code demonstrates how to define a class that maps to a database table, insert new records, and query the database using SQLAlchemy.
2. Introduction to Django ORM
Django ORM is the built-in ORM for the Django web framework. It allows you to interact with the database using Python classes and objects, just like SQLAlchemy, but it is specifically designed for Django applications. Django ORM supports multiple database backends like PostgreSQL, MySQL, SQLite, and Oracle.
Installing Django
To install Django, use pip
:
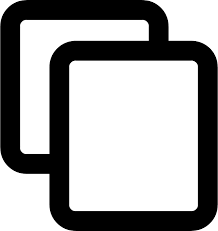
pip install django
Basic Django ORM Example
Here’s a simple example that demonstrates how to use Django ORM to create models and perform database operations:
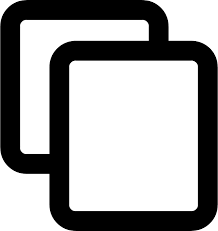
# models.py in a Django app
from django.db import models
# Define a model (table)
class User(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
# To create the table in the database
# Run: python manage.py makemigrations
# Run: python manage.py migrate
# Create a new user (inside a Django shell)
new_user = User.objects.create(name="Jane Doe", age=25)
# Query the database
users = User.objects.all()
for user in users:
print(f"{user.id}: {user.name}, {user.age} years old")
This code demonstrates how to define a model in Django, create a new record, and query the database using Django ORM. You’ll need to run makemigrations
and migrate
commands to create the corresponding table in the database.
3. SQLAlchemy vs. Django ORM
Both SQLAlchemy and Django ORM are excellent tools for interacting with relational databases, but there are some differences to consider:
- SQLAlchemy is more flexible and can be used in any Python project, including web frameworks like Flask or standalone applications. It allows for more control over the database and query generation.
- Django ORM is tightly integrated with the Django web framework and is best suited for Django-based applications. It provides a high-level interface for interacting with the database and is simpler to use within the Django ecosystem.
4. Conclusion
Both SQLAlchemy and Django ORM are powerful tools for working with databases in Python. SQLAlchemy is more flexible and can be used in various types of projects, while Django ORM is specifically designed for Django web applications. Depending on your project requirements, you can choose the ORM that best fits your needs.
Working with Cloud APIs
Cloud APIs allow you to interact with cloud services such as AWS, Google Cloud, and Microsoft Azure programmatically. These APIs provide functionality for accessing and managing cloud resources like computing instances, storage, databases, and machine learning models.
1. Introduction to Cloud APIs
Cloud providers offer APIs that expose the functionality of their platforms. Cloud APIs are typically RESTful and allow developers to perform actions like:
- Provisioning virtual machines or containers
- Managing cloud storage (e.g., uploading and downloading files)
- Configuring databases and networking
- Monitoring cloud resources
- Integrating machine learning models and services
2. Working with AWS Cloud API
AWS provides a comprehensive set of APIs through the AWS SDKs, including the Python SDK called Boto3. Boto3 allows you to interact with AWS services like EC2, S3, DynamoDB, and more.
Installing Boto3
To install Boto3, use pip
:
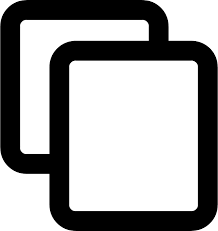
pip install boto3
Basic AWS S3 Example
Here’s an example of using Boto3 to upload a file to an S3 bucket:
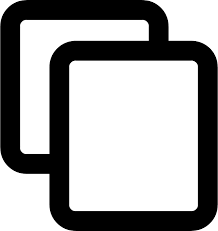
import boto3
# Initialize a session using Amazon S3
s3 = boto3.client('s3')
# Upload a new file
file_name = 'my_file.txt'
bucket_name = 'my-bucket'
s3.upload_file(file_name, bucket_name, file_name)
print(f"File {file_name} uploaded to {bucket_name}.")
# List all files in the bucket
response = s3.list_objects_v2(Bucket=bucket_name)
for obj in response.get('Contents', []):
print(f"File: {obj['Key']}")
This example shows how to upload a file to an S3 bucket and list all the objects stored in that bucket using Boto3.
3. Working with Google Cloud APIs
Google Cloud provides APIs for services like Compute Engine, Cloud Storage, BigQuery, and more. You can interact with Google Cloud resources using the Google Cloud Client Libraries.
Installing Google Cloud Client Libraries
To interact with Google Cloud services, you can install the necessary client libraries using pip
:
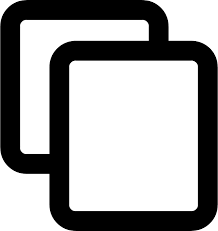
pip install google-cloud-storage
Basic Google Cloud Storage Example
This example demonstrates how to upload a file to Google Cloud Storage using the google-cloud-storage client library:
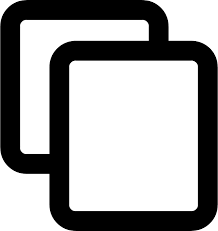
from google.cloud import storage
# Initialize a Cloud Storage client
client = storage.Client()
# Specify the bucket name and file
bucket_name = 'my-gcs-bucket'
file_name = 'my_file.txt'
# Upload the file
bucket = client.get_bucket(bucket_name)
blob = bucket.blob(file_name)
blob.upload_from_filename(file_name)
print(f"File {file_name} uploaded to {bucket_name}.")
In this example, we use the Google Cloud Storage client library to upload a file to a Google Cloud Storage bucket. You will need a valid Google Cloud project and appropriate authentication setup to run this code.
4. Working with Azure Cloud APIs
Microsoft Azure provides APIs through the Azure SDKs, including the Python SDK. Azure APIs allow you to manage resources like virtual machines, databases, and storage accounts.
Installing Azure SDK
To interact with Azure resources, you can install the Azure SDK for Python:
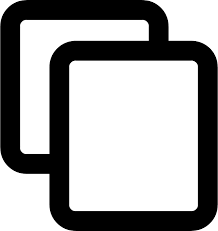
pip install azure-storage-blob
Basic Azure Blob Storage Example
This example shows how to upload a file to Azure Blob Storage:
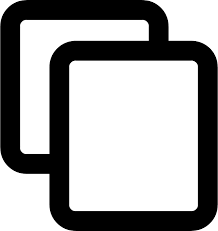
from azure.storage.blob import BlobServiceClient
# Initialize the BlobServiceClient
blob_service_client = BlobServiceClient.from_connection_string("your_connection_string")
# Specify the container and file
container_name = 'my-container'
file_name = 'my_file.txt'
# Upload the file
container_client = blob_service_client.get_container_client(container_name)
with open(file_name, "rb") as data:
container_client.upload_blob(file_name, data)
print(f"File {file_name} uploaded to Azure Blob Storage.")
This example demonstrates how to upload a file to an Azure Blob Storage container using the Azure SDK for Python. You need a valid Azure account and connection string to run this code.
5. Best Practices for Working with Cloud APIs
When working with cloud APIs, there are some best practices to follow:
- Authentication and Authorization: Use secure methods for authentication (e.g., API keys, OAuth, service accounts). Never hard-code credentials in your code.
- Error Handling: Handle exceptions and errors properly to account for network issues, API limits, and other potential failures.
- Rate Limiting: Be aware of API rate limits and implement backoff strategies to avoid hitting these limits.
- Monitoring: Use logging and monitoring to track API calls, responses, and errors for debugging and performance optimization.
- Use SDKs: Prefer using official cloud SDKs as they are optimized, provide better error handling, and are easier to work with compared to raw HTTP requests.
6. Conclusion
Cloud APIs provide powerful ways to interact with cloud services programmatically. By using the appropriate SDKs for AWS, Google Cloud, or Azure, you can automate cloud resource management, storage operations, and more. Always follow best practices for security, error handling, and resource management to ensure smooth and efficient cloud API integration.
Using Python for Server Automation
Python is a powerful tool for automating server tasks and managing infrastructure. It can automate repetitive tasks, such as server deployment, configuration management, backups, and monitoring. By using Python scripts, system administrators can streamline workflows and reduce manual intervention.
1. Introduction to Server Automation with Python
Server automation refers to the process of automating tasks on servers, such as starting/stopping services, managing users, performing backups, monitoring system health, and more. Python simplifies automation through a wide range of libraries, APIs, and frameworks, making it an ideal language for this purpose.
2. Popular Python Libraries for Server Automation
Python has several libraries that make server automation easy. Some of the most commonly used libraries include:
- Fabric: A high-level Python library for automating SSH-based tasks. Fabric allows you to run commands on remote servers, deploy software, and manage server configurations.
- Paramiko: A Python library that provides an interface for working with SSH. Paramiko is commonly used for secure remote server connections and automating command execution.
- Ansible (Python API): A widely used automation tool with a Python API for managing configurations and deployments across multiple servers in a network.
- Psutil: A library that allows you to retrieve system and process information, making it useful for monitoring system health and resources.
- Subprocess: A built-in Python module that allows you to spawn new processes and interact with the operating system’s shell, making it useful for automating command-line tasks.
3. Automating Server Tasks: Examples
Here are some examples of common server automation tasks that can be accomplished using Python:
Example 1: Running Remote Commands with Fabric
Fabric allows you to run commands on remote servers over SSH. Here’s an example of how to use Fabric to run commands on a remote server:
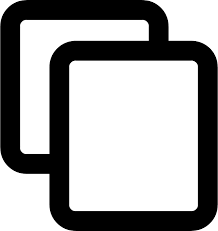
from fabric import Connection
# Connect to the remote server using SSH
conn = Connection('username@remote_server_ip')
# Run a command on the remote server
result = conn.run('uptime', hide=True)
# Print the result of the command
print(result.stdout.strip())
This example connects to a remote server using SSH and runs the uptime
command to check the server's uptime.
Example 2: Automating Backups with Python
Python can automate backups by creating scripts that copy server files to a remote location or cloud storage. Here's how you can automate backups using Python's shutil library:
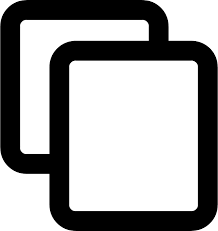
import shutil
import os
from datetime import datetime
# Define backup directory and destination
backup_dir = "/path/to/important/data"
backup_dest = "/path/to/backup/location"
# Create a timestamp for the backup
timestamp = datetime.now().strftime("%Y%m%d%H%M%S")
# Create a backup folder with the timestamp
backup_folder = os.path.join(backup_dest, f"backup_{timestamp}")
os.makedirs(backup_folder)
# Copy files to the backup folder
shutil.copytree(backup_dir, backup_folder)
print(f"Backup completed: {backup_folder}")
This script creates a backup of a specified directory and appends a timestamp to the backup folder name to ensure each backup is unique.
Example 3: Monitoring System Health with Psutil
The Psutil library allows you to retrieve information about CPU usage, memory usage, disk usage, and more. Here’s how you can monitor CPU and memory usage:
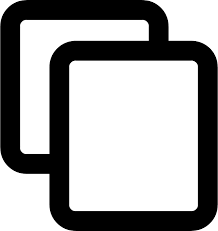
import psutil
# Get the CPU usage
cpu_usage = psutil.cpu_percent(interval=1)
# Get the memory usage
memory_usage = psutil.virtual_memory().percent
print(f"CPU Usage: {cpu_usage}%")
print(f"Memory Usage: {memory_usage}%")
This script monitors the system's CPU and memory usage and prints the values to the console.
4. Managing Services and Processes
Python can also be used to manage services and processes. For example, you can start, stop, or restart services on a server using the subprocess module:
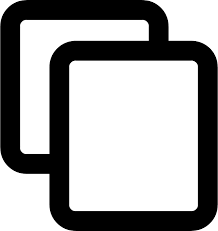
import subprocess
# Restart a service on a Linux server
subprocess.run(['sudo', 'systemctl', 'restart', 'nginx'])
This code restarts the Nginx service on a server using the systemctl
command, utilizing Python's subprocess module.
5. Automating Cloud Infrastructure
Python is also used to automate tasks in cloud environments, such as provisioning virtual machines, configuring storage, and managing cloud resources. Tools like Boto3 for AWS, google-cloud-python for Google Cloud, and azure-sdk for Azure provide APIs for automating cloud infrastructure tasks. For example, you can use Python to launch EC2 instances on AWS:
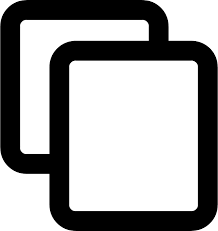
import boto3
# Initialize AWS EC2 client
ec2 = boto3.client('ec2')
# Launch a new EC2 instance
response = ec2.run_instances(
ImageId='ami-0c55b159cbfafe1f0',
InstanceType='t2.micro',
MinCount=1,
MaxCount=1
)
print("EC2 Instance launched:", response['Instances'][0]['InstanceId'])
This script uses Boto3 to launch a new EC2 instance on AWS.
6. Best Practices for Server Automation with Python
When automating server tasks with Python, it’s important to follow best practices to ensure security, reliability, and efficiency:
- Security: Always use secure methods for authentication (e.g., SSH keys, environment variables) and avoid hardcoding sensitive information in your scripts.
- Error Handling: Ensure proper error handling is implemented in your scripts to manage failures gracefully and provide useful logs for debugging.
- Logging: Use logging to track the actions performed by the automation scripts. This will help in troubleshooting and auditing.
- Idempotency: Ensure that your automation tasks are idempotent, meaning they can be executed multiple times without causing unintended effects.
- Testing: Test your scripts thoroughly in a staging environment before deploying them to production to avoid potential issues.
7. Conclusion
Python is an excellent choice for automating server tasks due to its simplicity and the powerful libraries available. Whether you are automating system administration tasks, managing cloud infrastructure, or monitoring server health, Python can help streamline operations and reduce manual effort.
Writing Scripts for Continuous Integration/Continuous Deployment (CI/CD)
Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in modern software development that allow teams to deliver code changes frequently and reliably. Python is commonly used to write scripts for automating the CI/CD pipeline, from testing and building to deployment and monitoring.
1. Introduction to CI/CD
CI/CD is a methodology that focuses on automating the software development lifecycle. Continuous Integration ensures that code changes are integrated and tested frequently, while Continuous Deployment automates the process of delivering the integrated code to production. Python can be used to write scripts that manage various stages of the CI/CD process.
2. Components of a CI/CD Pipeline
A typical CI/CD pipeline consists of the following stages:
- Build: The process of compiling or packaging the code for deployment.
- Test: Running automated tests to ensure the code is working as expected.
- Deploy: Deploying the code to the staging or production environment.
- Monitor: Monitoring the deployed application for issues.
3. Writing Python Scripts for CI/CD
Python is often used to automate tasks in the CI/CD pipeline. Below are examples of how you can use Python in different stages of the pipeline:
Example 1: Automating Tests with Python
Automated testing is a critical part of CI. You can write Python scripts to run tests and report results. For example, if you are using unittest for testing, the following script can automate the testing process:
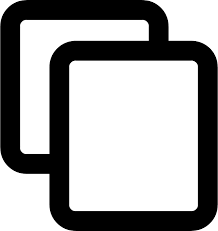
import unittest
# Define a simple test case
class TestExample(unittest.TestCase):
def test_addition(self):
self.assertEqual(2 + 2, 4)
# Run the test cases
if __name__ == '__main__':
unittest.main()
This script defines a simple unit test and runs it. You can integrate this script into your CI pipeline, which will automatically run tests whenever changes are made to the codebase.
Example 2: Automating Deployment with Python
Automating deployment to a server can also be achieved with Python. For example, you can use the Fabric library to automate deployment to a remote server:
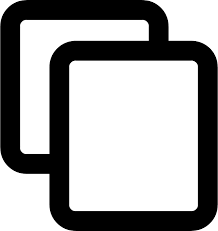
from fabric import Connection
# Connect to the remote server
conn = Connection('username@remote_server_ip')
# Pull the latest code from the repository and restart the service
conn.run('git pull origin main')
conn.run('sudo systemctl restart myapp.service')
print("Deployment successful!")
This script connects to a remote server, pulls the latest code from the Git repository, and restarts the application service. This can be integrated into the CD pipeline for automatic deployments.
Example 3: Monitor Deployment Status
Once the code is deployed, it's important to monitor the application for any issues. Python can be used to check the health of the application by monitoring logs or APIs. Here's an example that checks if a web service is running:
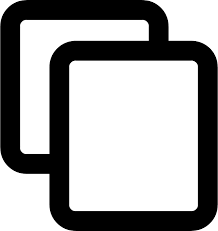
import requests
# Check if the web service is running
response = requests.get('http://your_web_service_url/health')
if response.status_code == 200:
print("Service is running smoothly.")
else:
print(f"Service is down! Status code: {response.status_code}")
This script sends an HTTP request to the health check endpoint of the service and reports whether the service is running correctly. You can integrate this script into your CI/CD pipeline to monitor the health of your application after deployment.
4. Integrating Python with CI/CD Tools
Many CI/CD platforms support Python scripts and can be configured to run them during different stages of the pipeline. Some popular CI/CD tools include:
- Jenkins: Jenkins can execute Python scripts as part of the build or deployment process using the sh or python commands in the build configuration.
- GitLab CI/CD: GitLab allows you to define CI/CD pipelines using a
.gitlab-ci.yml
file, where you can include Python scripts for testing, building, and deployment. - Travis CI: Travis CI can run Python scripts as part of the build process by specifying Python in the
.travis.yml
configuration file. - CircleCI: CircleCI supports Python and allows you to create workflows that include running Python scripts during the build and deployment stages.
5. Best Practices for Writing CI/CD Scripts
When writing Python scripts for CI/CD, it's important to follow best practices to ensure that the scripts are efficient, maintainable, and secure:
- Modularize Your Code: Break down your scripts into smaller, reusable functions to improve readability and maintainability.
- Keep It DRY (Don't Repeat Yourself): Avoid redundant code by using functions and scripts that can be reused across different stages of the pipeline.
- Use Environment Variables: For sensitive data like API keys, use environment variables rather than hardcoding them in your scripts.
- Handle Errors Gracefully: Implement proper error handling in your scripts to ensure that failures are caught and logged appropriately.
- Write Logs: Add logging to your scripts to track the execution of tasks and to facilitate troubleshooting in case of failures.
- Test Locally: Test your Python scripts locally before integrating them into the CI/CD pipeline to catch any errors early.
6. Conclusion
Python is an excellent language for automating the CI/CD pipeline. It can be used to automate testing, deployment, monitoring, and many other tasks in the pipeline. By writing efficient and modular Python scripts, you can ensure that your CI/CD processes are streamlined, reliable, and secure, leading to faster and more efficient development cycles.
Unit Testing with unittest
Unit testing is a software testing technique where individual units or components of a program are tested in isolation to ensure that they work as expected. Python provides the unittest module, which is a powerful tool for writing and executing unit tests. It is part of the Python standard library and follows the xUnit style of testing frameworks.
1. Introduction to Unit Testing
Unit tests are small, isolated tests that focus on a single function or method. Writing unit tests helps ensure that the code behaves as expected and makes it easier to detect bugs early in the development process. The unittest module provides a test framework, assertions, and utilities to write and run tests.
2. Setting Up the Test Class
In Python, unit tests are written by creating a subclass of unittest.TestCase
. This class provides various assertion methods to check the behavior of your code. Here's how you can set up a basic unit test:
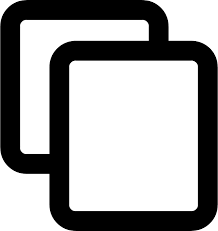
import unittest
# Function to be tested
def add(a, b):
return a + b
# Test case class
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5) # Assert that 2 + 3 equals 5
# Run the tests
if __name__ == '__main__':
unittest.main()
In this example, we have a simple function add(a, b)
that adds two numbers. The test class TestMathOperations
contains a method test_add
that tests whether the add
function works as expected by asserting that the sum of 2 and 3 is 5.
3. Common Assertions in unittest
unittest provides several assertion methods to check different conditions. Below are some commonly used assertions:
- assertEqual(a, b): Checks if
a == b
. - assertNotEqual(a, b): Checks if
a != b
. - assertTrue(x): Checks if
x
is True. - assertFalse(x): Checks if
x
is False. - assertIsNone(x): Checks if
x
isNone
. - assertIsNotNone(x): Checks if
x
is notNone
. - assertRaises(exception, func): Checks if the specified exception is raised when calling
func
.
Example: Using assertRaises
In some cases, you may want to test whether a specific error is raised. You can use assertRaises
to check that:
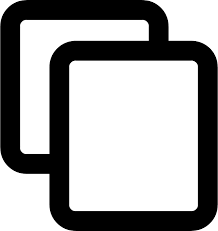
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
class TestMathOperations(unittest.TestCase):
def test_divide(self):
with self.assertRaises(ValueError):
divide(10, 0) # Should raise ValueError
if __name__ == '__main__':
unittest.main()
In this example, we test the divide
function to ensure it raises a ValueError
when dividing by zero.
4. Running Tests
Once you've written your unit tests, you can run them using the unittest.main()
function. This will automatically find all the test methods (methods starting with test_
) and execute them. You can run the tests from the command line by executing the Python script:
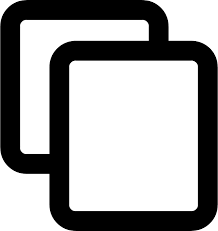
python test_script.py
If all tests pass, you'll see an output indicating that the tests passed. If any tests fail, you'll get an error message describing the failure.
5. Organizing Test Suites
As your project grows, you might have many tests. You can organize tests into test suites to manage them better. A test suite is a collection of test cases that are executed together. Here's an example:
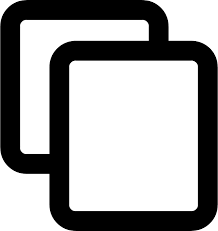
# Test case class
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
def test_subtract(self):
self.assertEqual(5 - 3, 2)
# Create a test suite
def suite():
suite = unittest.TestSuite()
suite.addTest(TestMathOperations('test_add'))
suite.addTest(TestMathOperations('test_subtract'))
return suite
# Run the tests
if __name__ == '__main__':
runner = unittest.TextTestRunner()
runner.run(suite())
In this example, we create a test suite that includes both the test_add
and test_subtract
tests. The suite is executed using a TextTestRunner
.
6. Conclusion
Unit testing with unittest helps ensure that your code works as expected and reduces the likelihood of introducing bugs. By writing unit tests, you can verify that each unit of your program performs correctly, making it easier to maintain and extend. With the unittest module, you can automate the testing process, organize tests into suites, and handle various test scenarios efficiently.
Writing Test Cases for Functions and Classes
Writing test cases for your functions and classes ensures that your code works as expected and handles edge cases. In Python, you can use the unittest module to write test cases for both functions and classes. This section will walk you through the process of writing test cases for both.
1. Test Cases for Functions
When writing test cases for functions, you want to test the function's output for various inputs. This helps ensure that the function behaves correctly in different scenarios. Here’s an example of testing a simple add()
function:
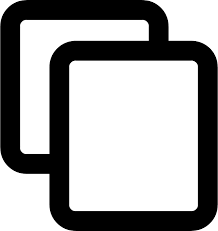
import unittest
# Function to be tested
def add(a, b):
return a + b
# Test case class for the add function
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5) # Regular test case
self.assertEqual(add(-1, 1), 0) # Test case with negative numbers
self.assertEqual(add(0, 0), 0) # Test case with zeros
self.assertEqual(add(1.5, 2.5), 4.0) # Test case with floats
if __name__ == '__main__':
unittest.main()
In the above example, the add()
function is tested with various types of inputs, including positive numbers, negative numbers, zeros, and floats. The assertEqual
method checks if the function produces the expected result for each input.
2. Test Cases for Classes
When writing test cases for classes, you need to test methods, attributes, and class behavior. You can create instances of the class and test how the methods interact with the class attributes. Below is an example of testing a simple Person
class:
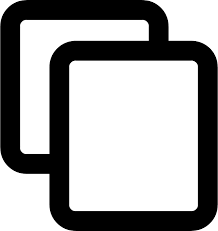
# Class to be tested
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, my name is {self.name} and I am {self.age} years old."
# Test case class for the Person class
class TestPerson(unittest.TestCase):
def test_person_creation(self):
person = Person("Alice", 30)
self.assertEqual(person.name, "Alice") # Check that name is set correctly
self.assertEqual(person.age, 30) # Check that age is set correctly
def test_greet(self):
person = Person("Bob", 25)
self.assertEqual(person.greet(), "Hello, my name is Bob and I am 25 years old.") # Check the greet method
if __name__ == '__main__':
unittest.main()
In this example, the Person
class is tested with two methods: test_person_creation
and test_greet
. The first method checks if the attributes name
and age
are set correctly, while the second method checks if the greet
method produces the expected greeting message.
3. Testing Edge Cases
It is important to test edge cases to ensure that your functions and classes handle extreme or boundary values. For example, when testing the add()
function, you should also test for very large numbers or very small numbers, and ensure that the behavior is as expected.
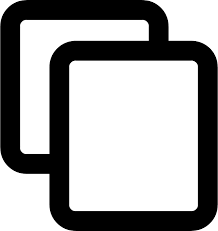
class TestMathOperations(unittest.TestCase):
def test_large_numbers(self):
self.assertEqual(add(10**6, 10**6), 2 * 10**6) # Test case with large numbers
def test_small_numbers(self):
self.assertEqual(add(0.0001, 0.0002), 0.0003) # Test case with small numbers
if __name__ == '__main__':
unittest.main()
In this case, edge cases such as adding large numbers and small numbers are tested to ensure the function can handle different ranges of values.
4. Mocking Dependencies in Unit Tests
In some cases, your function or method might depend on external systems or resources, such as a database or a web service. To isolate the functionality you want to test, you can use mocking. Python’s unittest.mock module allows you to replace parts of your code with mock objects during testing.
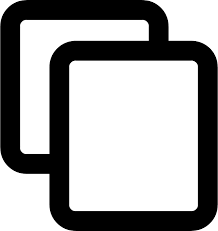
from unittest.mock import patch
# Function that makes an API call (example)
def fetch_data_from_api():
# Imagine this function fetches data from an external API
pass
# Test case class for mocking an external call
class TestExternalAPI(unittest.TestCase):
@patch('module_name.fetch_data_from_api')
def test_fetch_data(self, mock_fetch):
mock_fetch.return_value = {'data': 'mocked data'} # Mocked return value
self.assertEqual(fetch_data_from_api(), {'data': 'mocked data'}) # Test with mocked data
if __name__ == '__main__':
unittest.main()
In this example, the fetch_data_from_api()
function is mocked using @patch
. The mock replaces the actual external call, allowing you to test how your code behaves when the API returns predefined data.
5. Conclusion
Writing test cases for functions and classes is essential in ensuring the correctness of your code. By covering different scenarios, including edge cases and mocking dependencies, you can confidently test your code for robustness and functionality. The unittest module in Python provides powerful tools to write structured and effective test cases for both simple functions and complex classes.
Using pytest for Testing
pytest is a popular testing framework for Python that simplifies writing and running tests. It provides a more flexible and expressive way to write tests compared to the built-in unittest module. In this section, you’ll learn how to use pytest for writing tests, running them, and leveraging its features.
1. Installing pytest
To start using pytest, you need to install it first. You can install it using the Python package manager pip
:
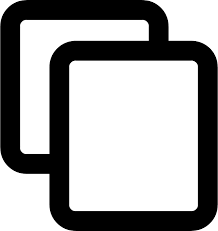
pip install pytest
Once installed, you can start writing and running tests using pytest.
2. Writing Test Cases with pytest
pytest makes it easy to write tests. Tests are typically written in files starting with test_
or ending with _test.py
. Test functions should be named starting with test_
as well. Below is an example of writing test cases for a simple function using pytest:
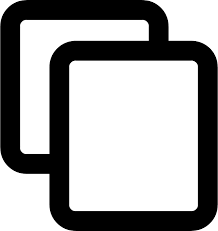
# Function to be tested
def add(a, b):
return a + b
# Test cases using pytest
def test_add():
assert add(2, 3) == 5 # Regular test case
assert add(-1, 1) == 0 # Test case with negative numbers
assert add(0, 0) == 0 # Test case with zeros
assert add(1.5, 2.5) == 4.0 # Test case with floats
In this example, we define a simple add
function and write tests using assert
statements. The assert
statement checks if the result of the function matches the expected output. If the condition is false, pytest will report the test as failed.
3. Running Tests with pytest
To run tests with pytest, simply run the following command in your terminal:
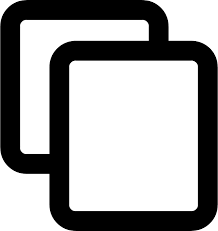
pytest
Pytest will automatically discover all files that match the pattern test_*.py
and execute the test functions that start with test_
. If all tests pass, you will see output indicating that everything is okay. If any test fails, pytest will show detailed information about the failure.
4. Advanced pytest Features
pytest offers many advanced features that make it more powerful for testing:
- Fixtures: Fixtures are used to set up and tear down resources before and after tests run. They can be used to create reusable test setups, such as database connections, API calls, or file operations.
- Parametrize: You can use the
@pytest.mark.parametrize
decorator to run a test with multiple sets of input values, improving test coverage and reducing redundancy. - Assertions: Pytest supports rich assertions, including checking exception handling, comparing objects, and more.
Example: Using Fixtures in pytest
Here’s an example of using a fixture to set up data for your tests:
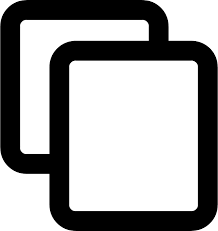
import pytest
# Fixture to set up data
@pytest.fixture
def setup_data():
return [1, 2, 3]
# Test case using the fixture
def test_sum(setup_data):
assert sum(setup_data) == 6 # Sum of 1 + 2 + 3
In this example, the setup_data
fixture is used to provide a list of numbers to the test function. The test case then checks if the sum of the numbers is correct.
Example: Using Parametrize in pytest
Using @pytest.mark.parametrize
, you can run a single test with multiple sets of data:
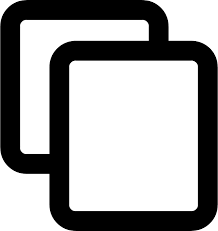
import pytest
# Parametrize decorator to run the test with multiple inputs
@pytest.mark.parametrize("a, b, expected", [
(2, 3, 5),
(-1, 1, 0),
(0, 0, 0),
(1.5, 2.5, 4.0)
])
def test_add(a, b, expected):
assert add(a, b) == expected # Check if add function produces the expected result
In this example, the test_add
function is run multiple times with different inputs, allowing you to test the same function with various data sets.
5. Pytest Output and Reporting
Pytest provides detailed output, including which tests passed or failed, and why a test failed. When running tests, you can use various options to control the verbosity of the output:
-v
: Increased verbosity (shows test names and results).--maxfail=1
: Stops after the first failure (useful for debugging).--disable-warnings
: Disables warnings in the output.
Example of running pytest with increased verbosity:
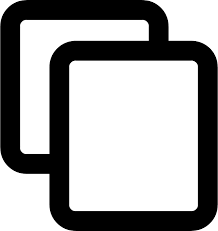
pytest -v
6. Conclusion
Pytest is a powerful and flexible testing framework for Python that simplifies writing, running, and organizing tests. With features like fixtures, parametrization, and rich assertions, pytest makes it easier to write comprehensive tests for your Python code. Whether you are testing simple functions or complex classes, pytest allows you to do so with ease and efficiency.
Profiling Python Code with cProfile
cProfile is a built-in Python module that allows you to profile your Python code and analyze its performance. Profiling provides detailed insights into how your code executes, helping you identify bottlenecks and areas for optimization. In this section, we will explore how to use cProfile to profile Python code and analyze the results.
1. Introduction to Profiling
Profiling is the process of measuring the performance of a program by recording various statistics about how time is spent during execution. With cProfile, you can see details such as:
- The number of function calls
- The time spent in each function
- The cumulative time spent across the entire program
- Other relevant performance metrics
Profiling helps you understand which parts of your code are slowing down and need improvement. Let's look at how to use cProfile for this purpose.
2. Using cProfile to Profile Python Code
To profile Python code with cProfile, you can run your script with the -m cProfile
option from the command line:
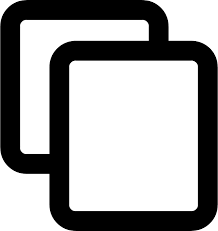
python -m cProfile your_script.py
This command will output the profiling statistics in the terminal. cProfile will show how many times each function was called, the total time spent in the function, and other details.
3. Profiling a Python Script
Here is an example Python script that we will profile using cProfile:
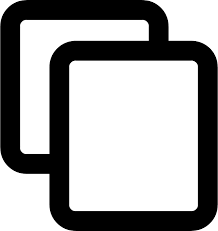
import time
def slow_function():
time.sleep(2)
def fast_function():
time.sleep(0.5)
def main():
slow_function()
fast_function()
if __name__ == "__main__":
main()
This script defines two functions: slow_function
(which sleeps for 2 seconds) and fast_function
(which sleeps for 0.5 seconds). The main
function calls both of these functions.
To profile this script, run the following command:
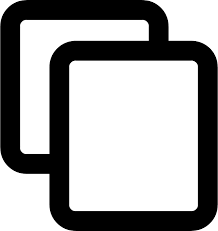
python -m cProfile script_name.py
The profiling output will show you the number of calls and the amount of time spent in each function.
4. Profiling Output Explained
The output from cProfile will look like this:
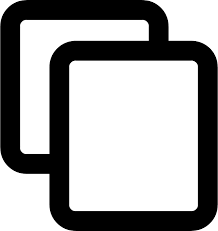
4 function calls in 2.502 seconds
Ordered by: standard name
ncalls tottime percall cumtime percall filename:lineno(function)
1 0.000 0.000 2.502 2.502 script_name.py:8(main)
1 0.000 0.000 2.000 2.000 script_name.py:4(slow_function)
1 0.000 0.000 0.500 0.500 script_name.py:7(fast_function)
1 0.000 0.000 0.000 0.000 {built-in method builtins.exec}
1 0.000 0.000 0.000 0.000 {method 'disable' of '_lsprof.Profiler' objects}
Here’s what the columns mean:
- ncalls: The number of calls made to the function.
- tottime: The total time spent in the function, excluding time spent in sub-functions.
- percall: The time per call, calculated as
tottime / ncalls
. - cumtime: The cumulative time spent in the function and all sub-functions.
- filename:lineno(function): The location of the function in the code.
From this output, you can see that the slow_function
took 2 seconds and the fast_function
took 0.5 seconds, which matches our expectations.
5. Profiling Specific Functions
If you want to profile specific parts of your code instead of the entire script, you can use cProfile within your code. Here’s how you can do that:
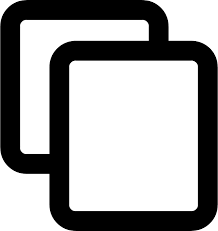
import cProfile
def slow_function():
time.sleep(2)
def fast_function():
time.sleep(0.5)
# Profiling specific code block
cProfile.run('slow_function()')
cProfile.run('fast_function()')
In this example, we use cProfile.run()
to profile the execution of the slow_function
and fast_function
individually.
6. Saving Profiling Results
To save the profiling results to a file, you can use the -o
option when running cProfile from the command line:
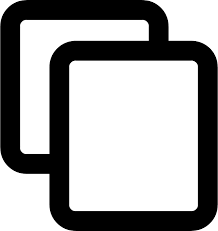
python -m cProfile -o profile_output.prof script_name.py
This will create a file called profile_output.prof
that contains the profiling data. You can later analyze this file using tools like snakeviz
or py-spy
for a more visual representation of the profiling results.
7. Conclusion
Profiling is a crucial step in optimizing Python code for performance. By using cProfile, you can gain valuable insights into how your code is performing and identify areas for improvement. Whether you're profiling a simple function or an entire script, cProfile provides the necessary tools to analyze your code's execution and make it faster and more efficient.
Using Multiprocessing for Parallel Execution
In Python, the multiprocessing module allows you to create multiple processes, enabling parallel execution of tasks. This can significantly improve performance, especially when working with CPU-bound tasks. In this section, we will explore how to use the multiprocessing module to execute Python code in parallel and take advantage of multiple CPU cores.
1. Introduction to Multiprocessing
The multiprocessing module provides a way to create separate processes that run concurrently. This is particularly useful for CPU-bound tasks, where Python’s Global Interpreter Lock (GIL) might limit the performance of multithreaded programs. By using multiple processes, each can run on a separate core, allowing full utilization of the CPU.
Multiprocessing is ideal for tasks such as:
- Data processing
- Image or video processing
- Scientific simulations
- Heavy computations that benefit from parallelism
2. Basic Multiprocessing Example
Let’s start by using the multiprocessing module to run multiple tasks in parallel. In this example, we will create two processes that execute a simple function concurrently:
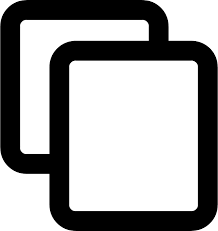
import multiprocessing
import time
def worker(name):
print(f"Worker {name} starting")
time.sleep(2)
print(f"Worker {name} finished")
if __name__ == "__main__":
# Create two processes
process1 = multiprocessing.Process(target=worker, args=("A",))
process2 = multiprocessing.Process(target=worker, args=("B",))
# Start the processes
process1.start()
process2.start()
# Wait for both processes to finish
process1.join()
process2.join()
print("Both workers have finished.")
In this example, we define a worker
function that simulates some work by sleeping for 2 seconds. We then create two separate processes that run the worker
function concurrently. Finally, we use join()
to wait for both processes to finish before printing the completion message.
3. Explanation of Key Concepts
- Process: A process is an independent execution unit with its own memory space. You can create a process using
multiprocessing.Process
. - start(): Starts the execution of the process.
- join(): Waits for the process to finish before continuing with the next step in the main program.
In the above example, the two workers run in parallel, reducing the total execution time as compared to running them sequentially.
4. Using Pool for Parallel Execution
For scenarios where you need to run the same function on multiple inputs, the Pool
class in the multiprocessing module can be used to easily manage multiple processes. Here’s an example that shows how to use a pool of workers to execute a function on a list of inputs:
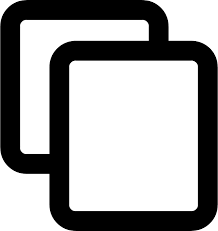
import multiprocessing
import time
def square(number):
print(f"Squaring {number}")
time.sleep(1)
return number * number
if __name__ == "__main__":
# Create a pool of 4 worker processes
with multiprocessing.Pool(4) as pool:
results = pool.map(square, [1, 2, 3, 4, 5])
print("Results:", results)
In this example, we use a pool of 4 worker processes to calculate the square of each number in the list. The map()
function allows you to apply the square
function to each item in the list in parallel.
Key points about using Pool
:
- Pool: A pool of worker processes that can execute tasks concurrently. The number of workers is specified when creating the pool (e.g.,
Pool(4)
for 4 workers). - map(): A function that distributes the input data among the workers and collects the results. It is similar to Python’s built-in
map()
but executes in parallel. - with statement: Ensures that the pool is properly closed and all processes are joined when done.
5. Process Synchronization
In some cases, processes may need to share data or synchronize their execution. The multiprocessing module provides several mechanisms for synchronizing processes, such as:
- Lock: Used to ensure that only one process can access a shared resource at a time.
- Queue: A thread-safe queue that allows processes to communicate with each other.
- Value and Array: Shared memory objects that allow data to be shared between processes.
Here’s an example of using a Lock
to prevent race conditions when accessing a shared resource:
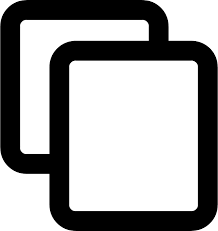
import multiprocessing
def increment(counter, lock):
with lock:
counter.value += 1
print(f"Counter: {counter.value}")
if __name__ == "__main__":
# Create a shared counter and a lock
counter = multiprocessing.Value('i', 0)
lock = multiprocessing.Lock()
# Create multiple processes that increment the counter
processes = [multiprocessing.Process(target=increment, args=(counter, lock)) for _ in range(5)]
# Start all processes
for p in processes:
p.start()
# Wait for all processes to finish
for p in processes:
p.join()
print("Final counter value:", counter.value)
In this example, we use a Value
to store a shared counter and a Lock
to ensure that only one process can access the counter at a time. This prevents race conditions and ensures that the counter is incremented safely.
6. Conclusion
Using the multiprocessing module allows you to parallelize tasks in Python, leading to better performance for CPU-bound tasks. Whether you are executing multiple independent functions concurrently or running the same function on a large dataset, multiprocessing helps you make full use of your system’s CPU cores.
Remember, multiprocessing is most useful for CPU-bound operations. For I/O-bound tasks, consider using multithreading or asynchronous programming to avoid unnecessary overhead.
Async Programming with asyncio
Async programming allows you to write concurrent code that runs more efficiently, especially for I/O-bound tasks. Python's asyncio module is a powerful tool for writing asynchronous programs using async/await syntax. In this section, we'll explore how to use asyncio for asynchronous programming and how it can improve the performance of I/O-bound operations.
1. Introduction to Async Programming
Traditional synchronous programming executes one task at a time, while asynchronous programming allows multiple tasks to run concurrently. The primary benefit of async programming is that it is more efficient for I/O-bound tasks, such as making HTTP requests, reading/writing files, or querying databases, by not blocking the program while waiting for an I/O operation to complete.
In Python, asyncio is a library for writing concurrent code using async
and await
. It allows you to write code that runs asynchronously without the need for threads or processes.
2. Basic Asyncio Example
Let's start with a simple example to demonstrate how async programming works with asyncio. In this example, we will create two async functions and run them concurrently:
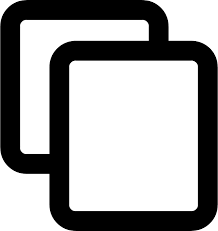
import asyncio
async def task1():
print("Task 1 started")
await asyncio.sleep(2)
print("Task 1 finished")
async def task2():
print("Task 2 started")
await asyncio.sleep(1)
print("Task 2 finished")
async def main():
# Run both tasks concurrently
await asyncio.gather(task1(), task2())
# Run the event loop
asyncio.run(main())
In this example, the task1
and task2
functions are defined as async functions using the async def
syntax. Inside these functions, we use await
to pause the function execution while waiting for an I/O operation (in this case, asyncio.sleep()
). The main
function uses asyncio.gather()
to run both tasks concurrently.
Key Concepts in the Example:
- async def: Defines an asynchronous function that can be paused and resumed with
await
. - await: Pauses the function and yields control back to the event loop while waiting for an I/O-bound operation to complete.
- asyncio.gather(): Runs multiple async functions concurrently and waits for them to finish.
3. Event Loop
The event loop is the core of asynchronous programming in Python. It is responsible for executing asynchronous tasks, managing scheduling, and handling I/O operations. The event loop runs in the background and manages the execution of tasks when they are ready to run.
In the example above, asyncio.run(main())
is used to start the event loop and run the main
function. It ensures that all asynchronous tasks are executed and completed before the program exits.
4. Asyncio for I/O Bound Operations
Async programming shines in scenarios where tasks involve waiting for I/O operations. For example, making multiple HTTP requests, querying a database, or reading files. With async programming, these tasks can be performed concurrently without blocking the execution of the program.
Example: Asynchronous HTTP Requests
Let’s look at an example of using asyncio along with aiohttp, a popular library for making asynchronous HTTP requests:
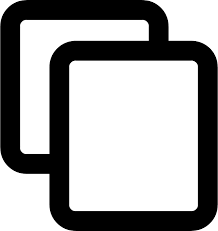
import asyncio
import aiohttp
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
urls = ["https://example.com", "https://example.org", "https://example.net"]
tasks = [fetch_url(url) for url in urls]
results = await asyncio.gather(*tasks)
print(results)
# Run the event loop
asyncio.run(main())
In this example, we define an async
function fetch_url
to make an asynchronous HTTP request using aiohttp
. The main
function creates a list of tasks to fetch multiple URLs concurrently. Using asyncio.gather()
, we gather all tasks and execute them in parallel.
5. Handling Exceptions in Asyncio
Handling exceptions in asynchronous code is similar to handling them in synchronous code. You can use try/except
blocks within async functions to catch and handle errors. Here's an example of handling exceptions in async programming:
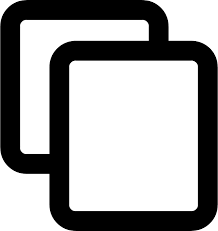
import asyncio
async def task_with_error():
print("Task started")
await asyncio.sleep(1)
raise ValueError("An error occurred in the task")
async def main():
try:
await task_with_error()
except ValueError as e:
print(f"Caught an exception: {e}")
# Run the event loop
asyncio.run(main())
In this example, we simulate an error in an asynchronous task and catch it using a try/except
block in the main
function. This allows us to handle exceptions gracefully in asynchronous code.
6. Conclusion
Async programming with asyncio is a powerful tool for writing concurrent Python code, especially for I/O-bound tasks. By using the async
and await
syntax, you can write efficient programs that handle multiple tasks concurrently without blocking execution. It provides an excellent solution for tasks like web scraping, making HTTP requests, and working with databases that involve waiting for I/O operations.
Remember, async programming is most beneficial when working with tasks that involve I/O waiting. For CPU-bound tasks, consider using multiprocessing or multithreading instead.
Optimizing Code with Numba
Numba is a Just-In-Time (JIT) compiler for Python that can significantly improve the performance of numerical and scientific computing. By compiling Python code to machine code at runtime, Numba accelerates the execution of functions that involve loops, numerical operations, and array manipulations, often making them as fast as C or Fortran.
1. Introduction to Numba
Numba is a powerful tool designed to optimize Python code, especially for functions that are heavily based on numerical computations. It uses the LLVM (Low-Level Virtual Machine) compiler infrastructure to translate Python code into optimized machine code. Numba works seamlessly with libraries like NumPy and SciPy, making it easy to accelerate numerical calculations.
Numba is particularly useful in scenarios where Python’s inherent slowness becomes a bottleneck, such as in loops or mathematical operations. It provides a simple and efficient way to speed up your code without needing to rewrite it in a lower-level language like C or C++.
2. Installing Numba
To get started with Numba, you first need to install it. You can install Numba using pip
:
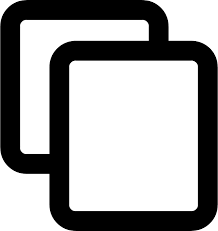
pip install numba
If you're using conda, you can install Numba with:
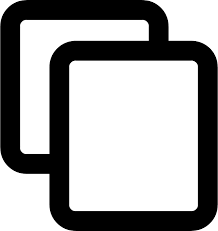
conda install numba
3. Basic Usage of Numba
Numba can be used to optimize functions by adding the @jit
decorator to them. The @jit
decorator tells Numba to compile the function just before it is called, which can lead to significant performance improvements.
Here’s a simple example demonstrating how to use @jit
:
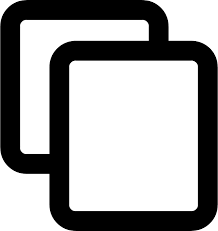
import numba
import numpy as np
@numba.jit
def sum_of_squares(arr):
total = 0
for i in range(len(arr)):
total += arr[i] ** 2
return total
arr = np.array([1, 2, 3, 4, 5])
print(sum_of_squares(arr))
In this example, we define the sum_of_squares
function that calculates the sum of squares of elements in an array. By using the @numba.jit
decorator, we tell Numba to compile this function into optimized machine code. The speedup is noticeable when working with larger arrays.
4. Numba's Types and Specifying Types
Numba automatically infers the types of function arguments and return values. However, you can also specify types explicitly to further optimize performance. To specify types, you can pass a signature to the @jit
decorator. This helps Numba generate more efficient machine code by providing additional type information.
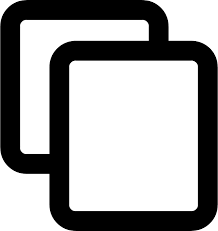
from numba import jit
import numpy as np
@jit("float64(float64[:])")
def sum_of_squares(arr):
total = 0.0
for i in range(len(arr)):
total += arr[i] ** 2
return total
arr = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
print(sum_of_squares(arr))
In this example, we explicitly specify that the function sum_of_squares
takes a NumPy array of float64
values and returns a float64
. This allows Numba to optimize the function more effectively.
5. Numba's Parallelism and GPU Support
Numba also supports parallel execution and GPU acceleration for certain types of functions. By using the prange
function and the nopython=True
flag, you can enable automatic parallelization of loops. This can make a significant difference when dealing with large datasets or computationally intensive tasks.
Here’s an example of using parallelism with Numba:
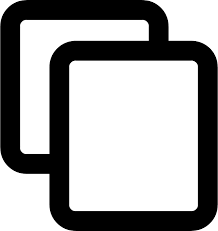
from numba import jit, prange
import numpy as np
@jit(parallel=True)
def parallel_sum_of_squares(arr):
total = 0
for i in prange(len(arr)):
total += arr[i] ** 2
return total
arr = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
print(parallel_sum_of_squares(arr))
In this example, the @jit(parallel=True)
decorator tells Numba to parallelize the loop and split the computation across multiple cores. This can improve performance significantly for large arrays.
GPU Acceleration with Numba
Numba also supports GPU acceleration for certain computations through the cuda.jit
decorator. This allows you to offload computations to a GPU, which can dramatically speed up processing for large datasets or complex mathematical operations.
6. When to Use Numba
Numba is most effective when used in performance-critical sections of code, especially those that involve:
- Numerical computations (e.g., scientific simulations, matrix operations).
- Loops, especially those processing large arrays or datasets.
- Functions that rely on libraries like NumPy for array manipulation.
However, Numba is not suitable for all types of code. For instance, it does not provide significant performance improvements for I/O-bound tasks like web scraping or database queries. It is also not effective for complex Python code that heavily relies on dynamic features, like reflection or metaprogramming.
7. Conclusion
Numba is a powerful tool for optimizing Python code, especially when dealing with heavy numerical computations and loops. By using JIT compilation, Numba can significantly speed up your code while allowing you to write in Python without needing to resort to a lower-level language. For tasks involving large datasets, scientific simulations, or machine learning, Numba is an excellent choice to improve the performance of your Python programs.
Simple Calculator
A simple calculator is a basic program that allows users to perform arithmetic operations such as addition, subtraction, multiplication, and division. Below is an example of how to implement a simple calculator in Python that performs these operations based on user input.
1. Simple Calculator Code
Here’s a Python program that implements a simple calculator using basic conditional statements and arithmetic operations:
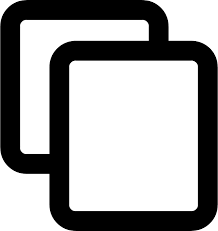
# Simple Calculator Program
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error! Division by zero."
return x / y
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
# Take user input
choice = input("Enter choice (1/2/3/4): ")
# Check if the user choice is valid
if choice in ['1', '2', '3', '4']:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(f"{num1} + {num2} = {add(num1, num2)}")
elif choice == '2':
print(f"{num1} - {num2} = {subtract(num1, num2)}")
elif choice == '3':
print(f"{num1} * {num2} = {multiply(num1, num2)}")
elif choice == '4':
print(f"{num1} / {num2} = {divide(num1, num2)}")
else:
print("Invalid input! Please select a valid operation.")
2. How It Works
This simple calculator program offers the user the following operations:
- Addition: Adds two numbers.
- Subtraction: Subtracts the second number from the first.
- Multiplication: Multiplies two numbers.
- Division: Divides the first number by the second. If the second number is zero, it returns an error message.
The program first prompts the user to select an operation by entering a number between 1 and 4. Then, the user enters two numbers, and the corresponding operation is performed. The result is displayed to the user.
3. Validating User Input
The program checks if the user's choice is valid (i.e., it is one of the options: 1, 2, 3, or 4). If an invalid option is selected, it prompts the user to enter a valid choice.
4. Handling Division by Zero
In the division operation, the program checks whether the second number (divisor) is zero. If it is, an error message is displayed instead of attempting to divide by zero, which would cause an error.
5. Running the Program
To run this program, simply copy the code into a Python file (e.g., calculator.py
) and execute it. The program will prompt the user for input and then display the result of the chosen operation.
6. Example Output
Here’s an example of how the calculator works:
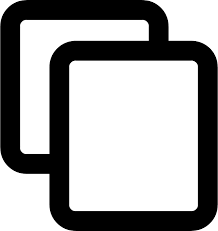
Select operation:
1. Add
2. Subtract
3. Multiply
4. Divide
Enter choice (1/2/3/4): 1
Enter first number: 12
Enter second number: 8
12.0 + 8.0 = 20.0
In this example, the user chose the addition operation, entered 12 and 8, and the result is displayed as 20.0.
7. Extending the Calculator
You can extend this simple calculator by adding more complex operations such as:
- Exponentiation: Raise a number to the power of another number.
- Square root: Calculate the square root of a number using Python's
math.sqrt()
function. - Modulo: Find the remainder of the division of two numbers.
To add any of these features, you simply need to define additional functions and modify the user interface to include those new operations.
8. Conclusion
This simple calculator is a great starting point for learning how to work with basic input, output, and arithmetic operations in Python. By using functions, conditional statements, and loops, you can build more complex programs and explore additional features to enhance your calculator.
To-Do List Application
A To-Do List Application allows users to add, update, and delete tasks they need to accomplish. It's a great way to manage daily tasks, and it's commonly built using Python with a simple interface. Below is an example of a To-Do List application built using Python.
1. Simple To-Do List Code
Here’s a Python program that implements a simple To-Do list application. This program lets the user add tasks, display all tasks, and delete tasks from the list:
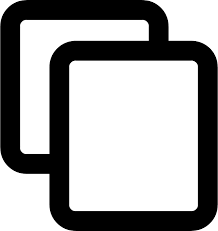
# Simple To-Do List Application
tasks = []
def add_task(task):
tasks.append(task)
print(f"Task '{task}' added successfully!")
def delete_task(task):
if task in tasks:
tasks.remove(task)
print(f"Task '{task}' deleted successfully!")
else:
print("Task not found!")
def display_tasks():
if not tasks:
print("No tasks in the To-Do list.")
else:
print("To-Do List:")
for task in tasks:
print(f"- {task}")
# User interface
while True:
print("\nTo-Do List Application")
print("1. Add Task")
print("2. Delete Task")
print("3. Display Tasks")
print("4. Exit")
choice = input("Enter choice (1/2/3/4): ")
if choice == '1':
task = input("Enter task: ")
add_task(task)
elif choice == '2':
task = input("Enter task to delete: ")
delete_task(task)
elif choice == '3':
display_tasks()
elif choice == '4':
print("Exiting To-Do List Application.")
break
else:
print("Invalid choice! Please select a valid option.")
2. How It Works
This To-Do List application allows the user to:
- Add Tasks: Users can add tasks to the list that need to be completed.
- Delete Tasks: Users can delete tasks that are no longer relevant.
- Display Tasks: Displays all the tasks that are currently in the list.
- Exit: Exits the application.
The application runs in a loop until the user selects the option to exit. The user is presented with a menu of choices, and they can interact with the To-Do list by entering the corresponding number.
3. Example Usage
Here’s an example of how the To-Do List application works:
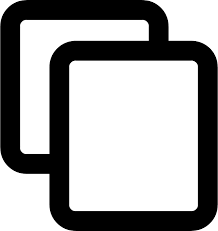
To-Do List Application
1. Add Task
2. Delete Task
3. Display Tasks
4. Exit
Enter choice (1/2/3/4): 1
Enter task: Buy groceries
Task 'Buy groceries' added successfully!
To-Do List Application
1. Add Task
2. Delete Task
3. Display Tasks
4. Exit
Enter choice (1/2/3/4): 3
To-Do List:
- Buy groceries
To-Do List Application
1. Add Task
2. Delete Task
3. Display Tasks
4. Exit
Enter choice (1/2/3/4): 2
Enter task to delete: Buy groceries
Task 'Buy groceries' deleted successfully!
To-Do List Application
1. Add Task
2. Delete Task
3. Display Tasks
4. Exit
Enter choice (1/2/3/4): 3
No tasks in the To-Do list.
4. Running the Program
To run this application, copy and paste the code into a Python file (e.g., todo_list.py
). After running the program, the user can interact with the To-Do list through the menu options.
5. Adding More Features
You can enhance this To-Do list application by adding more features such as:
- Task Due Dates: Add deadlines for tasks and display tasks that are overdue.
- Save and Load Tasks: Save tasks to a file so they persist between program runs, and load them when the program starts.
- Task Priorities: Add priorities to tasks, allowing users to filter or sort tasks based on importance.
6. Conclusion
This simple To-Do list application is a great way to practice working with Python functions, user input, and conditional statements. By adding more features, you can extend the program to create a fully functional task management system.
Weather App Using APIs
A Weather App allows users to check the current weather of a specific location. By using an API like OpenWeatherMap, users can get real-time weather data, including temperature, humidity, and weather conditions. Below is an example of how you can create a simple weather app using Python and the OpenWeatherMap API.
1. Setting Up the OpenWeatherMap API
Before you can use the OpenWeatherMap API, you need to sign up and get your API key. Follow these steps:
- Go to the OpenWeatherMap API page.
- Sign up for a free account.
- Get your API key after logging in.
2. Example Weather App Code
Here’s a Python program that fetches weather data using the OpenWeatherMap API and displays it to the user:
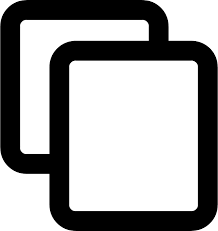
import requests
def get_weather(city, api_key):
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric"
response = requests.get(url)
data = response.json()
if response.status_code == 200:
main = data['main']
weather = data['weather'][0]
temperature = main['temp']
humidity = main['humidity']
description = weather['description']
print(f"Weather in {city}:")
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Condition: {description.capitalize()}")
else:
print("Error fetching the weather data. Please try again.")
# User interface
api_key = "your_api_key_here" # Replace with your API key
city = input("Enter city: ")
get_weather(city, api_key)
3. How It Works
This weather app works by calling the OpenWeatherMap API and parsing the returned JSON data. Here's a breakdown of how the code works:
- The function
get_weather
takes a city name and API key as input. - The
requests.get()
method sends a GET request to the OpenWeatherMap API. - The JSON response is parsed using
response.json()
, extracting the temperature, humidity, and weather condition. - The weather data is then displayed in a user-friendly format.
4. Example Output
Here's an example of what the output looks like when you run the app:
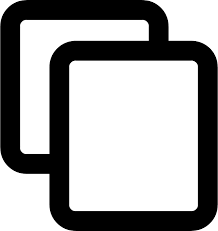
Enter city: London
Weather in London:
Temperature: 12°C
Humidity: 81%
Condition: Clear sky
5. Running the Program
To run the weather app, follow these steps:
- Install the
requests
library if you don't have it already by runningpip install requests
. - Copy the code above into a Python file (e.g.,
weather_app.py
). - Replace
"your_api_key_here"
with your actual OpenWeatherMap API key. - Run the Python script by typing
python weather_app.py
in your terminal. - Enter the city you want to check the weather for, and the app will display the current weather conditions.
6. Enhancing the Weather App
You can add more features to the weather app, such as:
- Weather Forecast: Fetch a 5-day or 7-day weather forecast using the OpenWeatherMap API.
- Better UI: Create a graphical user interface (GUI) using Tkinter or a web-based interface using Flask or Django.
- Unit Conversion: Allow users to switch between Celsius, Fahrenheit, and Kelvin for temperature.
- Error Handling: Improve error handling for invalid cities or API connection issues.
7. Conclusion
By building this weather app, you have learned how to make API calls, handle JSON data, and display the results to the user. This is a foundational skill for building many Python-based applications that interact with APIs.
Web Scraper for eCommerce Websites
A Web Scraper is a tool used for extracting data from websites. By using libraries like BeautifulSoup and requests in Python, you can build a scraper to collect product details from eCommerce websites like Amazon, eBay, or any other online store. Below is an example of how to create a simple web scraper to collect product data from an eCommerce website.
1. Installing Required Libraries
To create a web scraper, you need to install two important libraries: requests
(for sending HTTP requests) and BeautifulSoup
(for parsing HTML content). You can install them using pip
:
pip install requests beautifulsoup4
2. Example Web Scraper Code
Here’s a Python program that scrapes product names, prices, and descriptions from an eCommerce website (e.g., a sample online store).
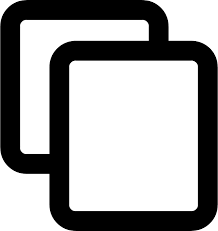
import requests
from bs4 import BeautifulSoup
def get_product_data(url):
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36"
}
# Send HTTP request to the eCommerce website
response = requests.get(url, headers=headers)
# Check if request was successful
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# Extract product information (adjust these based on the website's HTML structure)
products = soup.find_all('div', class_='product-item')
for product in products:
name = product.find('h2', class_='product-title').get_text()
price = product.find('span', class_='product-price').get_text()
description = product.find('p', class_='product-description').get_text()
print(f"Product Name: {name}")
print(f"Price: {price}")
print(f"Description: {description}")
print("-" * 50)
else:
print(f"Failed to retrieve data from {url}")
# Example usage
url = "http://example.com/products" # Replace with the actual URL of the eCommerce website
get_product_data(url)
3. How It Works
This web scraper works by:
- Sending an HTTP GET request to the eCommerce website using the
requests.get()
method. - Parsing the HTML content using BeautifulSoup to extract specific elements, such as product names, prices, and descriptions.
- Looping through the scraped products and printing out their details.
4. Example Output
Here’s an example of the output you might see when running the scraper:
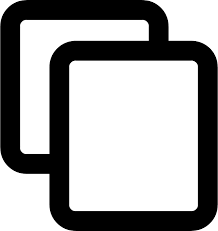
Product Name: Wireless Headphones
Price: $99.99
Description: High-quality wireless headphones with noise-cancellation.
--------------------------------------------------
Product Name: Smart Watch
Price: $199.99
Description: A smartwatch with fitness tracking and heart rate monitoring.
--------------------------------------------------
5. Running the Web Scraper
To run the web scraper, follow these steps:
- Install the required libraries by running
pip install requests beautifulsoup4
in your terminal. - Copy the code into a Python file (e.g.,
web_scraper.py
). - Replace the
url
variable with the actual URL of the eCommerce website you want to scrape. - Run the script by typing
python web_scraper.py
in your terminal. - The scraper will print out the product details from the website.
6. Enhancing the Web Scraper
You can enhance the web scraper by adding the following features:
- Pagination: If the website has multiple pages of products, you can add functionality to scrape data from all pages by following pagination links.
- Storing Data: Instead of just printing the data, store it in a CSV file or a database for later analysis.
- Error Handling: Add better error handling to manage issues like network failures or invalid URLs.
- Data Filtering: Filter products based on specific criteria, such as price range or category.
7. Legal and Ethical Considerations
Before scraping any website, make sure to:
- Check the website’s robots.txt file to see if scraping is allowed.
- Respect the website's terms of service, and do not overload the website’s servers with too many requests in a short period.
- Ensure that you are using the scraped data ethically and for legitimate purposes.
8. Conclusion
By building this web scraper, you have learned how to extract structured data from websites and process it using Python. Web scraping is an essential skill in data collection, especially for eCommerce, competitive analysis, and market research.
Chatbot with Python
A chatbot is an AI program designed to simulate conversation with users. Python is a popular language for creating chatbots due to its ease of use and robust libraries. In this section, we will explore how to build a simple chatbot using Python and the nltk
(Natural Language Toolkit) library.
1. Installing Required Libraries
To create a chatbot, you need to install the nltk
library, which provides tools for working with human language data. You can install it using pip
:
pip install nltk
2. Example Chatbot Code
Here’s an example of a simple chatbot implemented using the nltk
library:
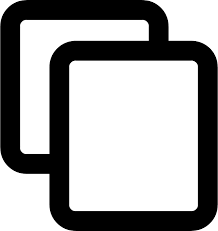
import nltk
from nltk.chat.util import Chat, reflections
# Define pairs of patterns and responses
pairs = [
(r'Hi|Hello|Hey', ['Hello!', 'Hi there!', 'Hey!']),
(r'How are you?', ['I am good, thank you!', 'I am doing great, how about you?']),
(r'What is your name?', ['I am a chatbot created using Python and NLTK.']),
(r'Quit', ['Goodbye! Have a great day!']),
]
# Create a chatbot with the defined pairs
def chatbot():
print("Chatbot: Hello! Type 'Quit' to end the conversation.")
chat = Chat(pairs, reflections)
chat.converse()
# Start the chatbot
if __name__ == "__main__":
chatbot()
3. How It Works
This chatbot works by matching user inputs with predefined patterns and responding with corresponding answers. Here's how it functions:
- The user input is matched with the regular expressions defined in
pairs
. - If a match is found, the chatbot provides a response from the predefined list.
- If the user types "Quit", the chatbot ends the conversation.
4. Example Interaction
Here’s an example of how the conversation with the chatbot might look:
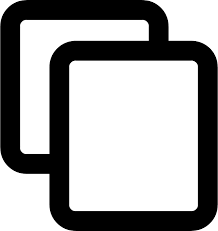
Chatbot: Hello! Type 'Quit' to end the conversation.
User: Hi
Chatbot: Hello!
User: How are you?
Chatbot: I am good, thank you!
User: What is your name?
Chatbot: I am a chatbot created using Python and NLTK.
User: Quit
Chatbot: Goodbye! Have a great day!
5. Running the Chatbot
To run the chatbot, follow these steps:
- Install the
nltk
library by runningpip install nltk
. - Copy the Python code into a file (e.g.,
chatbot.py
). - Run the file by executing
python chatbot.py
in your terminal. - The chatbot will start running, and you can begin chatting with it.
6. Enhancing the Chatbot
You can enhance the chatbot by adding the following features:
- More Complex Responses: Add more complex patterns and responses, including handling multiple user inputs.
- Integration with APIs: Integrate the chatbot with external APIs, such as weather or news APIs, to provide dynamic responses based on real-time data.
- Machine Learning: Use machine learning models, such as those from the
transformers
library, to make the chatbot more intelligent and capable of understanding a wide range of queries. - GUI Interface: Build a graphical user interface (GUI) using libraries like
tkinter
to make the chatbot more interactive.
7. Legal and Ethical Considerations
When building and deploying chatbots, it's important to keep in mind the following:
- Ensure transparency by informing users that they are interacting with a chatbot, not a human.
- Respect user privacy and data security by implementing appropriate measures to protect user data.
- Make sure the chatbot provides accurate and responsible information, especially when dealing with sensitive topics.
8. Conclusion
Building a chatbot with Python is a great way to learn about natural language processing (NLP) and machine learning. By using the nltk
library, you can create simple yet effective chatbots to automate interactions and provide assistance to users. With more advanced tools and techniques, you can create highly intelligent and dynamic chatbots capable of handling complex conversations.
Writing Clean Python Code (PEP 8 Guidelines)
Writing clean and readable code is essential for maintaining software projects, especially in collaborative environments. The PEP 8 guidelines are the de facto standard for writing Python code in a clear and consistent style. PEP 8 ensures that Python code is easy to read and understand by all developers.
1. Introduction to PEP 8
PEP 8 is the Python Enhancement Proposal that provides a set of guidelines for writing Python code. It covers aspects such as indentation, variable naming, line length, and code structure to make code more readable and maintainable. By following PEP 8, you ensure that your code adheres to a well-established standard recognized by the Python community.
2. Key Guidelines from PEP 8
2.1. Indentation
Proper indentation is crucial in Python since it is used to define code blocks. PEP 8 recommends using 4 spaces per indentation level.
# Correct indentation
def my_function():
if True:
print("Hello")
Do not use tabs for indentation. Always use spaces to maintain consistent indentation across your code.
2.2. Line Length
Limit all lines to a maximum of 79 characters. This ensures that the code remains readable, especially on devices with smaller screens or when reviewing code in diff tools.
# Line exceeds 79 characters - avoid this:
def my_function_that_does_a_lot_of_work_and_needs_to_be_split_into_multiple_lines():
pass
# Correct approach:
def my_function():
"""
This function does something important, but it's split across multiple lines
to ensure readability.
"""
pass
2.3. Blank Lines
Use blank lines to separate functions, classes, and larger blocks of code inside functions. This improves readability.
# Correct use of blank lines
def function_one():
pass
def function_two():
pass
2.4. Imports
Imports should be on separate lines. Group imports into three categories: standard library imports, third-party imports, and local imports. Each group should be separated by a blank line.
# Correct import structure
import os
import sys
import numpy as np
import pandas as pd
from mymodule import my_function
2.5. Naming Conventions
PEP 8 recommends specific naming conventions for variables, functions, classes, and modules to improve code readability:
- Variable and Function Names: Use lowercase letters and separate words with underscores (e.g.,
my_variable
,calculate_total_price
). - Class Names: Use CapitalizedWords (e.g.,
MyClass
). - Module and Package Names: Use lowercase letters and separate words with underscores (e.g.,
my_module
). - Constants: Use all uppercase letters with underscores separating words (e.g.,
MAX_SIZE
).
2.6. Function and Method Arguments
When defining functions or methods, avoid using too many arguments. Aim for clarity and simplicity by keeping the number of arguments to a reasonable amount.
# Function with clear and simple arguments
def calculate_area(radius, pi=3.14):
return pi * radius ** 2
2.7. Docstrings
Use docstrings to describe the purpose of functions, methods, and classes. The docstring should be enclosed in triple quotes and placed immediately after the function or class definition. This helps developers understand the functionality of your code.
def my_function():
"""
This function prints a greeting message to the user.
It takes no arguments and returns nothing.
"""
print("Hello, world!")
3. Additional Tips for Writing Clean Code
- Be Consistent: Consistency in code formatting is key. Stick to the guidelines throughout your project.
- Use Meaningful Names: Give variables, functions, and classes meaningful names that clearly describe their purpose.
- Avoid Duplicate Code: If you find yourself repeating code, consider refactoring it into a reusable function.
- Write Tests: Write unit tests for your functions and classes to ensure they behave as expected and to prevent future bugs.
4. Tools for Enforcing PEP 8
There are several tools available to automatically check your code against the PEP 8 guidelines:
- PyLint: A popular tool for checking Python code against PEP 8 and other coding standards.
- Flake8: A tool that checks for style violations, including PEP 8, and can be integrated into editors and IDEs.
- autopep8: A tool that automatically formats Python code according to PEP 8 guidelines.
5. Conclusion
By following the PEP 8 guidelines, you can write Python code that is clean, readable, and maintainable. These principles help ensure that your code is consistent and understandable by other developers, leading to better collaboration and fewer bugs. Adhering to PEP 8 is not just about following rules—it's about writing Python code that others can easily work with and improve over time.
Using Virtual Environments (venv)
Virtual environments in Python allow you to create isolated environments for your projects. This ensures that dependencies for one project do not interfere with those of another. Using virtual environments is a best practice for managing dependencies in Python projects and avoiding version conflicts.
1. Introduction to Virtual Environments
A virtual environment is a self-contained directory that contains a Python installation for a specific version of Python, along with additional packages. With virtual environments, you can keep dependencies required by different projects in separate places, ensuring that they don't clash.
2. Why Use Virtual Environments?
- Dependency Management: Each project can have its own set of dependencies, independent of other projects.
- Avoid Version Conflicts: Different projects may require different versions of the same package. Virtual environments help prevent version conflicts.
- Clean Environment: Virtual environments ensure that you don't clutter your global Python installation with unnecessary packages.
- Portability: You can easily recreate the environment on another machine by sharing the environment's dependency list.
3. Creating a Virtual Environment
To create a virtual environment, you can use the venv
module, which is included with Python 3.3 and later. Follow these steps:
- Open a terminal (or command prompt) and navigate to your project folder.
- Run the following command to create a virtual environment:
python -m venv myenv
This will create a directory named myenv
that contains the virtual environment.
4. Activating the Virtual Environment
After creating the virtual environment, you need to activate it. The method for activation depends on your operating system:
- On Windows: Run the following command in the command prompt:
myenv\Scripts\activate
source myenv/bin/activate
After activation, your terminal prompt will change to indicate that you're working inside the virtual environment. The environment's name will appear in parentheses, like so: (myenv)
.
5. Installing Packages in a Virtual Environment
Once the virtual environment is activated, you can use pip
to install packages. For example, to install requests
, you would run:
pip install requests
This will install the package only within the virtual environment, not globally.
6. Deactivating the Virtual Environment
When you're done working within the virtual environment, you can deactivate it by running:
deactivate
This will return you to your global Python environment.
7. Managing Dependencies with requirements.txt
To share the dependencies of your project, you can create a requirements.txt
file. This file lists all the packages and their versions that the project depends on. To create this file, run:
pip freeze > requirements.txt
To install the dependencies from this file in another environment, run:
pip install -r requirements.txt
8. Virtual Environment Best Practices
- Use a .gitignore file: Avoid committing the virtual environment to version control (e.g., Git). Add the virtual environment directory (e.g.,
myenv/
) to your.gitignore
file. - Keep environments isolated: Each project should have its own virtual environment to avoid conflicts.
- Use a consistent naming convention: Name your virtual environment directories something meaningful, such as
venv
,env
, ormyenv
. - Update dependencies regularly: Periodically update the packages in your virtual environment to keep them up-to-date with the latest versions.
9. Conclusion
Using virtual environments is an essential practice for Python developers, as it helps manage dependencies, avoid conflicts, and maintain clean development environments. By using venv
, you can ensure that each of your Python projects remains isolated and portable, making it easier to work on multiple projects at the same time.
Dependency Management with pip and requirements.txt
In Python, managing dependencies is essential for ensuring that your projects have the necessary packages and libraries to function properly. One of the most common ways to manage dependencies in Python projects is by using pip
(Python's package installer) along with a requirements.txt
file. This allows you to install, update, and share project dependencies efficiently.
1. Introduction to pip
pip
is the standard tool for installing Python packages from the Python Package Index (PyPI). It is included with Python versions 3.4 and later. You can use pip
to install, upgrade, or uninstall Python packages within your virtual environment or globally on your system.
2. Installing Packages with pip
To install a package using pip
, simply run the following command in your terminal:
pip install package_name
For example, to install the requests
library, use:
pip install requests
This will download and install the latest version of the requests
package from PyPI.
3. Checking Installed Packages
You can check which packages are installed in your environment using the command:
pip list
This will display a list of all installed packages along with their versions.
4. Upgrading Packages
If you want to upgrade an installed package to the latest version, you can use the following command:
pip install --upgrade package_name
For example, to upgrade the requests
library, run:
pip install --upgrade requests
5. Uninstalling Packages
If you no longer need a package, you can uninstall it with:
pip uninstall package_name
For example, to uninstall requests
, run:
pip uninstall requests
6. Using requirements.txt for Dependency Management
The requirements.txt
file is a plain text file that lists the dependencies (and their versions) required for a Python project. This file allows others to easily recreate the environment with the same dependencies.
7. Creating a requirements.txt File
To generate a requirements.txt
file, use the following pip freeze
command:
pip freeze > requirements.txt
This will create a requirements.txt
file in the current directory with all the installed packages and their versions. For example, the contents of requirements.txt
might look like this:
requests==2.25.1
flask==1.1.2
This file can now be shared with others or used to replicate the environment later.
8. Installing Dependencies from requirements.txt
To install the dependencies listed in a requirements.txt
file, use the following command:
pip install -r requirements.txt
This will install all the packages listed in requirements.txt
at the specified versions. It’s a great way to ensure consistency across different environments.
9. Version Control with requirements.txt
It is a good practice to include the requirements.txt
file in version control (e.g., Git), so that anyone working on the project can install the same set of dependencies. However, avoid adding the virtual environment directory (e.g., venv/
) to version control. You can do this by adding the virtual environment directory to your .gitignore
file.
10. Updating Dependencies in requirements.txt
If you add new packages or update existing packages, you should regenerate the requirements.txt
file by running:
pip freeze > requirements.txt
This ensures that your requirements.txt
file is up-to-date and reflects the current state of your environment.
11. Best Practices for Managing Dependencies
- Use Virtual Environments: Always use virtual environments to isolate dependencies for each project.
- Pin Dependencies: In
requirements.txt
, always specify the exact version of each package to avoid unexpected changes. - Regularly Update Packages: Periodically check for package updates and keep your dependencies up-to-date for security and stability.
- Use a .gitignore File: Add virtual environment directories (e.g.,
venv/
) to your.gitignore
file to avoid committing them to version control.
12. Conclusion
Managing dependencies with pip
and requirements.txt
is an essential part of Python project development. By using these tools, you can ensure that your project has the correct packages and versions, and that it can be easily shared or deployed in other environments. It also helps maintain consistency and avoid conflicts between different projects.
Version Control with Git and Python Projects
Version control is an essential practice for managing code changes and collaborating in a software development environment. Git is a widely used version control system that helps track changes to your codebase over time. By integrating Git with your Python projects, you can easily manage code versions, collaborate with others, and maintain a history of your project’s development.
1. Introduction to Version Control
Version control systems (VCS) are tools that help manage changes to code and allow multiple developers to work on the same codebase. Git is a distributed version control system that is widely used in the software development industry. It allows developers to manage their code history efficiently, revert to earlier versions, and collaborate on projects with others.
2. Setting Up Git for Your Python Project
To start using Git in your Python project, you'll need to initialize a Git repository in your project directory. Here's how you can do it:
- Install Git if it’s not already installed. You can download it from git-scm.com.
- Navigate to your Python project directory in the terminal.
- Run the following command to initialize a Git repository:
git init
This command creates a hidden .git
directory in your project, allowing you to start tracking changes.
3. Creating a .gitignore File
When using Git with Python projects, it's important to ignore certain files and directories that shouldn't be tracked, such as virtual environments, compiled files, and temporary files. You can create a .gitignore
file to specify which files and directories to ignore. Here’s an example of a .gitignore
file for Python projects:
# Python bytecode files
*.pyc
*.pyo
__pycache__/
# Virtual environment
venv/
env/
# IDE settings
.idea/
.vscode/
Once you’ve created the .gitignore
file, Git will ignore the specified files when you commit changes.
4. Making Your First Commit
After initializing the Git repository and adding a .gitignore
file, the next step is to commit your changes:
- Add your files to the staging area using the following command:
- Make your first commit with the following command:
git add .
git commit -m "Initial commit"
The git add .
command stages all the changes in your project, and the git commit -m
command commits those changes with a message describing the changes made.
5. Creating a Remote Repository (GitHub, GitLab, Bitbucket)
If you want to collaborate with others or back up your project, you can create a remote repository on a platform like GitHub, GitLab, or Bitbucket. After creating a remote repository, you can link it to your local repository by adding a remote URL:
git remote add origin https://github.com/your-username/your-repo.git
Next, push your changes to the remote repository:
git push -u origin master
6. Branching and Merging
One of Git's most powerful features is branching. Branches allow you to work on different features or fixes in isolation without affecting the main codebase. Here’s how to create and switch between branches:
- Create a new branch:
- Switch to an existing branch:
- Merge a branch into another:
git checkout -b new-feature
git checkout master
git checkout master
git merge new-feature
Branching enables you to work on multiple features at once and merge them back into the main branch (usually master
) once they’re complete.
7. Resolving Merge Conflicts
Merge conflicts occur when two developers make changes to the same part of a file, and Git can't automatically merge the changes. When a merge conflict occurs, Git will mark the conflicting areas in the file, and you’ll need to manually resolve the conflict. Once resolved, add the changes to the staging area and commit them:
git add conflicted-file.py
git commit -m "Resolved merge conflict"
8. Cloning a Repository
If you want to clone an existing project from a remote repository, use the following command:
git clone https://github.com/username/repository.git
This will create a copy of the repository on your local machine, and you can start working on it right away.
9. Collaborating with Others
If you're collaborating on a project with other developers, you'll often need to pull the latest changes from the remote repository before pushing your changes. To pull the latest changes, use:
git pull origin master
This ensures that your local repository is up-to-date with the remote repository before you push your changes.
10. Best Practices for Version Control in Python Projects
- Commit Early and Often: Commit your changes frequently with clear, descriptive commit messages to track the history of your project.
- Use Branches for Features: Create separate branches for new features, bug fixes, or experiments to keep the main branch stable.
- Write Meaningful Commit Messages: Make your commit messages clear and descriptive to explain the changes made.
- Keep .gitignore Updated: Regularly update your
.gitignore
file to ensure that unnecessary files (such as virtual environments or IDE settings) are not tracked in Git. - Collaborate Effectively: Communicate with your team, resolve conflicts, and frequently pull updates from the remote repository to stay in sync.
11. Conclusion
Using Git for version control in Python projects is crucial for managing code, collaborating with others, and ensuring that you can revert to previous versions of your code. By following best practices such as using branches, writing meaningful commit messages, and using a .gitignore
file, you can keep your project organized and collaborate effectively with others. Version control also helps maintain a history of your project, making it easier to track progress and debug issues.
Introduction to Python's Metaclasses
Metaclasses in Python are a complex yet powerful concept that allow you to control the creation of classes. A metaclass is essentially a class of a class, meaning it defines how a class behaves. In Python, everything is an object, including classes, and metaclasses give you the ability to customize class creation and behavior. They are typically used to automate class creation, enforce certain patterns, or manipulate class attributes and methods.
1. What is a Metaclass?
A metaclass is a class that defines how other classes are constructed. In Python, classes themselves are instances of metaclasses. By default, the metaclass for all classes is type
, but you can define your own metaclasses to customize class creation. This means you can influence the behavior of the class itself, such as adding methods, modifying attributes, or even preventing class creation under certain conditions.
2. How Metaclasses Work
When a class is defined in Python, the interpreter performs the following steps:
- Looks up the metaclass of the class.
- Creates a new class object using the metaclass.
- Initializes the class object with the attributes and methods defined in the class body.
- Returns the class object to be used for instantiation.
Essentially, metaclasses allow you to intervene at the point when the class is being created, giving you the opportunity to modify its structure.
3. Defining a Metaclass
You can define a metaclass by subclassing the type
class. The type
class itself is the default metaclass for all classes in Python. Here's an example of how to define a simple metaclass:
class MyMeta(type):
def __new__(cls, name, bases, dct):
# Modify the class definition here
dct['meta_added'] = True
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
obj = MyClass()
print(obj.meta_added) # Output: True
In this example, the MyMeta
metaclass adds an attribute meta_added
to the class definition. When we create an instance of MyClass
, the attribute is accessible, demonstrating the power of metaclasses in modifying class behavior.
4. Key Methods in Metaclasses
In a metaclass, there are several important methods that can be overridden to customize class creation:
__new__(cls, name, bases, dct)
: This method is called when a new class is created. It's used to modify the class before it's created.__init__(cls, name, bases, dct)
: This method is called after the class has been created. It allows further customization after the initial class creation.__call__(cls, *args, **kwargs)
: This method is invoked when the class is called to create a new instance. It can be used to control instance creation.
5. When to Use Metaclasses?
Metaclasses are a powerful tool, but they should be used with care. Some common use cases for metaclasses include:
- Enforcing Coding Standards: You can use metaclasses to enforce coding standards across all classes, such as ensuring that certain methods are implemented in every class.
- Automatic Method Addition: Metaclasses can automatically add methods to classes or modify existing methods during class creation.
- Singleton Pattern Implementation: Metaclasses can be used to implement the Singleton design pattern, ensuring that only one instance of a class exists.
- Validating Class Attributes: Metaclasses can validate the attributes of a class at creation time, such as checking for specific naming conventions or required fields.
6. Example Use Case: Singleton Pattern
One classic use case for metaclasses is implementing the Singleton design pattern, which ensures that only one instance of a class exists. Here's how you can do it:
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super().__call__(*args, **kwargs)
return cls._instances[cls]
class SingletonClass(metaclass=SingletonMeta):
pass
# Testing the Singleton
obj1 = SingletonClass()
obj2 = SingletonClass()
print(obj1 is obj2) # Output: True
In this example, the SingletonMeta
metaclass ensures that only one instance of SingletonClass
exists, even when multiple objects are instantiated.
7. Metaclass Best Practices
While metaclasses provide powerful functionality, they can make the code harder to understand and maintain. It’s important to follow best practices when working with metaclasses:
- Use Metaclasses Sparingly: Metaclasses should be used only when necessary. For most cases, regular classes and inheritance are sufficient.
- Document Your Metaclasses: Since metaclasses can modify class behavior in non-obvious ways, it's crucial to thoroughly document your metaclasses to make your code more understandable to others.
- Test Thoroughly: Metaclasses introduce complexity, so it's important to write unit tests to ensure that they behave as expected.
8. Conclusion
Metaclasses in Python allow you to take full control over class creation and behavior. While they are an advanced feature, they can be invaluable in situations where you need to enforce rules, add functionality, or manipulate classes in ways that are not easily achieved through regular inheritance. By understanding how to define and use metaclasses, you can enhance your Python projects with powerful and flexible behavior.
Working with Python C Extensions
Python is a high-level language that excels in simplicity and readability, but sometimes performance becomes a critical factor, or you might need to interface with existing C libraries. In such cases, writing Python C extensions allows you to combine the speed of C with the usability of Python. C extensions let you write native code that Python can call directly, improving performance and enabling integration with low-level C libraries.
1. What Are Python C Extensions?
Python C extensions are shared libraries written in C or C++ that can be imported into a Python program like a standard Python module. These extensions allow you to:
- Optimize performance-critical parts of your Python code using C.
- Access low-level system functionality not directly available in Python.
- Interface with existing C/C++ libraries.
2. Setting Up a Basic C Extension
To create a Python C extension, you'll need to:
- Write the C code defining the functions you want to expose to Python.
- Use the
Python.h
header file to interface with Python's API. - Compile the C code into a shared library that Python can import.
Example: Creating a Simple C Extension
Here's an example of a C extension that provides a function to add two numbers:
#include <Python.h>
// Function to add two numbers
static PyObject* add(PyObject* self, PyObject* args) {
double a, b;
if (!PyArg_ParseTuple(args, "dd", &a, &b)) {
return NULL;
}
return Py_BuildValue("d", a + b);
}
// Method table
static PyMethodDef MyMethods[] = {
{"add", add, METH_VARARGS, "Add two numbers."},
{NULL, NULL, 0, NULL} // Sentinel
};
// Module definition
static struct PyModuleDef mymodule = {
PyModuleDef_HEAD_INIT,
"mymodule", // Module name
"A simple C extension", // Module description
-1, // Size of per-interpreter state, or -1
MyMethods // Methods
};
// Module initialization
PyMODINIT_FUNC PyInit_mymodule(void) {
return PyModule_Create(&mymodule);
}
3. Compiling the C Extension
To compile the above code into a shared library, you can use Python's setuptools
. Create a setup.py
file:
from setuptools import setup, Extension
module = Extension('mymodule', sources=['mymodule.c'])
setup(
name='mymodule',
version='1.0',
description='A simple C extension',
ext_modules=[module],
)
Compile and build the module using the following command:
python setup.py build
The shared library will be created in the build
directory. You can move it to your project directory and import it like a normal Python module:
import mymodule
print(mymodule.add(3.5, 2.5)) # Output: 6.0
4. Error Handling in C Extensions
Error handling in C extensions is crucial to ensure stability. If an error occurs, you can set a Python exception using PyErr_SetString
or PyErr_Format
. For example:
if (some_error_condition) {
PyErr_SetString(PyExc_ValueError, "An error occurred");
return NULL;
}
5. Performance Considerations
While C extensions provide significant performance benefits, they should be used judiciously. Key considerations include:
- Minimizing the number of transitions between Python and C, as this introduces overhead.
- Ensuring memory safety by properly managing memory allocation and deallocation.
- Profiling your code to identify bottlenecks before deciding to write a C extension.
6. Alternatives to Writing C Extensions
If writing a C extension feels too complex, you can explore alternatives:
- ctypes: A built-in Python library for calling C functions from shared libraries without writing an extension.
- Cython: A language that compiles Python-like code into C for performance gains.
- Pybind11: A modern C++ library for creating Python bindings for C++ code.
7. Conclusion
Python C extensions provide a powerful way to optimize performance-critical code and interface with low-level C libraries. While they require careful implementation and error handling, they can significantly enhance the capabilities of your Python projects. By understanding the basics of creating and compiling C extensions, you can leverage the best of both Python and C in your applications.
Writing Python Code for IoT Devices
The Internet of Things (IoT) has revolutionized the way devices communicate and interact with each other. Python, with its simplicity and extensive library support, is one of the most popular programming languages for developing IoT applications. Python can be used to write code for IoT devices, enabling them to gather data, perform computations, and communicate with other devices or servers.
1. Why Use Python for IoT?
Python is well-suited for IoT projects due to:
- Ease of Use: Its simple syntax makes it accessible for beginners and professionals alike.
- Extensive Libraries: Libraries like
GPIO
,Adafruit_Blinka
,MQTT
, andrequests
simplify working with hardware and communication protocols. - Cross-Platform Support: Python runs on various IoT platforms such as Raspberry Pi, ESP32, and BeagleBone.
- Community Support: A large community ensures access to tutorials, forums, and pre-built libraries for IoT development.
2. Setting Up Python for IoT
To begin developing Python-based IoT applications, you'll need:
- A microcontroller or single-board computer (e.g., Raspberry Pi, ESP32).
- Python installed on the device (usually pre-installed on Raspberry Pi).
- Required Python libraries for your project. Install them using
pip
, for example:
pip install RPi.GPIO paho-mqtt adafruit-blinka
3. Working with Sensors and Actuators
Python can interface with hardware components like sensors and actuators through GPIO pins. Below is an example of controlling an LED using Raspberry Pi:
import RPi.GPIO as GPIO
import time
# Pin setup
GPIO.setmode(GPIO.BCM)
GPIO.setup(18, GPIO.OUT)
try:
while True:
GPIO.output(18, GPIO.HIGH) # Turn on LED
time.sleep(1) # Wait 1 second
GPIO.output(18, GPIO.LOW) # Turn off LED
time.sleep(1) # Wait 1 second
except KeyboardInterrupt:
GPIO.cleanup() # Clean up GPIO resources on exit
4. Communication Protocols
IoT devices often communicate using protocols like MQTT, HTTP, or WebSocket. Here's an example of sending data to an MQTT broker:
import paho.mqtt.client as mqtt
# MQTT setup
broker = "test.mosquitto.org"
port = 1883
topic = "iot/devices/temperature"
# Publish temperature data
client = mqtt.Client()
client.connect(broker, port, 60)
client.publish(topic, "25.6°C")
print("Data sent to MQTT broker!")
5. Accessing Cloud Platforms
IoT devices often send data to cloud platforms for storage and analysis. Using Python, you can send data to services like AWS IoT, Google Cloud IoT, or Azure IoT. For example, sending data to a RESTful API:
import requests
url = "https://example.com/api/temperature"
data = {"device_id": "sensor01", "temperature": 25.6}
response = requests.post(url, json=data)
if response.status_code == 200:
print("Data successfully sent to the cloud!")
else:
print("Failed to send data.")
6. Real-Time Data Visualization
Python libraries like Matplotlib and Flask can be used to visualize IoT data in real-time. For example, setting up a simple Flask web server:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/temperature', methods=['GET'])
def get_temperature():
temperature = 25.6
return jsonify({"temperature": temperature})
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
Access the data by navigating to http://
in a web browser.
7. Security Considerations
When writing Python code for IoT devices, ensure your application is secure:
- Use secure communication protocols like HTTPS and MQTT over TLS.
- Validate and sanitize all inputs to prevent injection attacks.
- Keep your Python version and libraries up to date.
- Encrypt sensitive data stored on the device or transmitted over networks.
8. Conclusion
Python provides a robust and flexible foundation for IoT development. Whether you're reading sensor data, controlling devices, or sending data to the cloud, Python libraries and tools make it easier to bring your IoT projects to life. By combining Python's simplicity with IoT hardware capabilities, you can build innovative and connected solutions for a variety of applications.
Building CLI Applications with argparse
Command-line interfaces (CLI) are essential for creating tools that can be executed in terminal environments. Python’s argparse
module simplifies the process of building robust CLI applications by providing easy-to-use tools for parsing command-line arguments.
1. Why Use argparse for CLI Applications?
The argparse
module is built into Python’s standard library and offers several benefits:
- Ease of Use: Simplifies command-line argument parsing.
- Automatic Help Messages: Generates
--help
output for users. - Customizable: Supports required and optional arguments, default values, and more.
- Input Validation: Automatically validates and converts input types.
2. Setting Up a Basic CLI Application
The simplest CLI application takes arguments from the user and performs an operation based on them. Below is an example of a basic Python script using argparse
:
import argparse
# Initialize the parser
parser = argparse.ArgumentParser(description="A simple CLI calculator.")
# Add arguments
parser.add_argument("num1", type=float, help="First number")
parser.add_argument("num2", type=float, help="Second number")
parser.add_argument("--operation", type=str, choices=["add", "subtract", "multiply", "divide"], default="add", help="Operation to perform")
# Parse arguments
args = parser.parse_args()
# Perform the operation
if args.operation == "add":
result = args.num1 + args.num2
elif args.operation == "subtract":
result = args.num1 - args.num2
elif args.operation == "multiply":
result = args.num1 * args.num2
elif args.operation == "divide":
result = args.num1 / args.num2
print(f"Result: {result}")
Run the script from the terminal like this:
python cli_calculator.py 10 5 --operation multiply
The output will be:
Result: 50.0
3. Adding Positional and Optional Arguments
In argparse
, arguments can be:
- Positional Arguments: Required arguments specified without prefixes.
- Optional Arguments: Arguments prefixed with
--
or-
.
For example:
import argparse
parser = argparse.ArgumentParser(description="File management CLI tool.")
parser.add_argument("filename", help="Name of the file to process")
parser.add_argument("--verbose", "-v", action="store_true", help="Enable verbose output")
args = parser.parse_args()
if args.verbose:
print(f"Processing file: {args.filename} (verbose mode enabled)")
else:
print(f"Processing file: {args.filename}")
4. Using Argument Groups
Argument groups help organize related arguments for clarity:
parser = argparse.ArgumentParser(description="Database CLI tool.")
# Create groups
db_group = parser.add_argument_group("Database Options")
db_group.add_argument("--host", required=True, help="Database host")
db_group.add_argument("--port", type=int, default=3306, help="Database port")
auth_group = parser.add_argument_group("Authentication")
auth_group.add_argument("--user", required=True, help="Database user")
auth_group.add_argument("--password", required=True, help="Database password")
5. Generating Help and Usage Messages
The argparse
module automatically generates help messages based on the arguments provided. For instance:
python cli_tool.py --help
Will output:
usage: cli_tool.py [-h] --host HOST --port PORT --user USER --password PASSWORD
Database CLI tool.
optional arguments:
-h, --help Show this help message and exit
--host HOST Database host
--port PORT Database port (default: 3306)
--user USER Database user
--password PASSWORD Database password
6. Advanced Usage
For more complex applications, argparse
supports:
- Subparsers: For subcommands (e.g.,
git add
,git commit
). - Custom Actions: For custom argument handling logic.
Example of subcommands:
parser = argparse.ArgumentParser(description="CLI with subcommands.")
subparsers = parser.add_subparsers(dest="command")
# Add subcommands
create_parser = subparsers.add_parser("create", help="Create a new resource")
create_parser.add_argument("name", help="Name of the resource")
delete_parser = subparsers.add_parser("delete", help="Delete a resource")
delete_parser.add_argument("id", help="ID of the resource")
args = parser.parse_args()
if args.command == "create":
print(f"Creating resource: {args.name}")
elif args.command == "delete":
print(f"Deleting resource with ID: {args.id}")
7. Conclusion
Building CLI applications with argparse
enables you to create user-friendly and powerful tools. By leveraging its features like automatic help messages, argument validation, and subcommands, you can develop versatile applications suitable for various use cases.
Comments and Documentation Strings
In Python, comments and documentation strings (docstrings) are essential for writing clean, readable, and maintainable code. They help explain the purpose and behavior of code segments, making it easier for others (or yourself) to understand and modify the code in the future.
1. Comments in Python
Comments are ignored by the Python interpreter and are used to explain what a specific part of the code does. Comments can be placed on their own line or at the end of a line of code.
Single-Line Comments
Single-line comments begin with the
#
symbol. Everything after the#
on that line is considered a comment.Multi-Line Comments
For multi-line comments, you can use the
#
symbol at the beginning of each line:2. Documentation Strings (Docstrings)
Documentation strings, or docstrings, are used to describe the functionality of a function, class, or module. Docstrings are placed inside triple quotes (either
"""
or'''
) and can span multiple lines.Function Docstrings
A docstring at the beginning of a function provides a brief description of what the function does, its parameters, and its return value. It is a good practice to include docstrings for every function you write.
Class Docstrings
Similarly, classes also have docstrings to explain their purpose and usage. The docstring is placed immediately after the class definition.
Module Docstrings
At the top of a Python module (a .py file), you can include a docstring that provides information about the module, what it does, and how to use it. This is especially useful for larger projects with multiple files.
3. Accessing Docstrings
You can access a function, class, or module's docstring using the built-in
help()
function or the.__doc__
attribute.Using the
help()
FunctionThe
help()
function displays the docstring of a function, class, or module:Using
.__doc__
AttributeYou can also access the docstring directly by referencing the
.__doc__
attribute of the function, class, or module:4. Best Practices for Writing Comments and Docstrings
Here are some best practices to keep in mind when using comments and docstrings:
5. Conclusion
Comments and documentation strings are essential for writing readable and maintainable Python code. By using single-line comments, multi-line comments, and docstrings effectively, you can ensure that your code is easy to understand and well-documented for others.