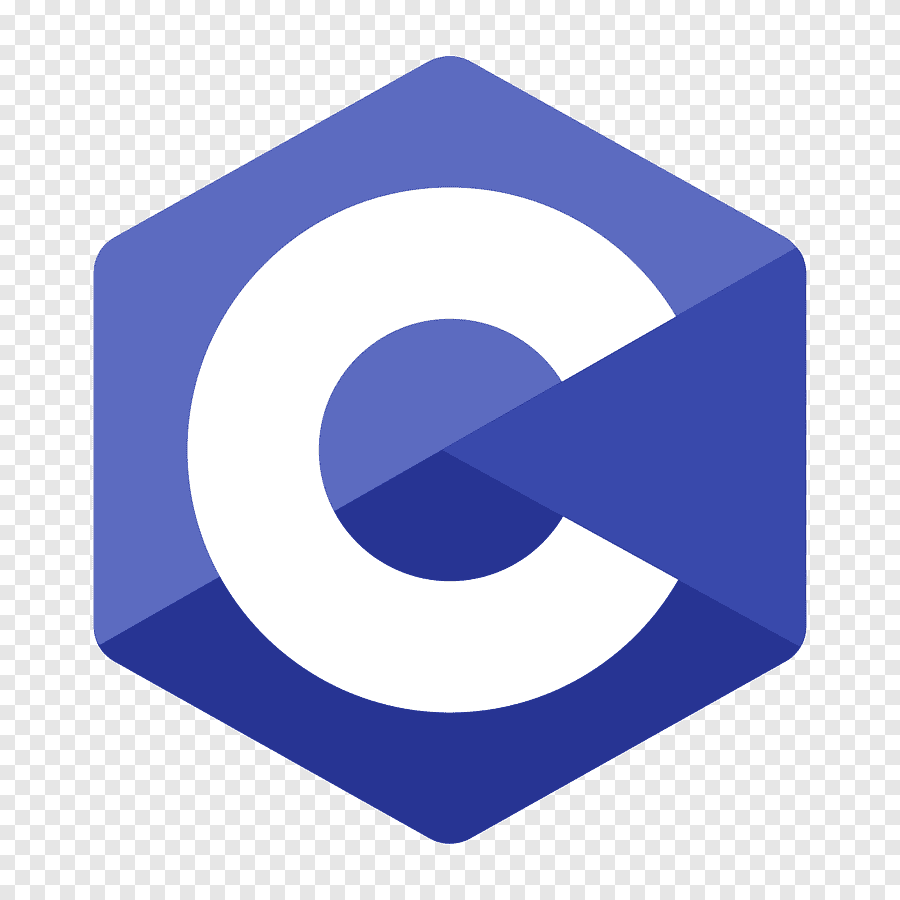
Introduction to C Programming
C is a powerful general-purpose programming language. It was developed in the early 1970s by Dennis Ritchie and has since become a foundational language in computer science. C is known for its efficiency, portability, and wide range of applications, making it an excellent choice for both beginners and experienced developers.
What is C Programming?
C is a structured programming language used to write operating systems, embedded systems, and high-performance applications. It provides low-level access to memory, making it highly efficient. Additionally, it serves as a base for many modern programming languages, such as C++ and Python.
Key Features of C Programming
- Simplicity: C is easy to learn with a simple set of keywords and syntax.
- Efficiency: Programs written in C are fast and require minimal system resources.
- Portability: C programs can run on various hardware platforms with little or no modification.
- Extensibility: C allows users to write libraries and extend functionality.
Why Learn C Programming?
Learning C is important because:
- Foundation: It builds a strong foundation for learning other programming languages.
- Versatility: C is used in various domains like embedded systems, operating systems, and game development.
- Problem-Solving: C helps develop logical thinking and problem-solving skills.
How to Get Started with C Programming
Here are the steps to start programming in C:
- Install a Compiler: Download and install a C compiler like GCC or Turbo C.
- Write Your First Program: Use a text editor or IDE to write your first C program.
- Compile and Run: Compile your program using the compiler and execute it.
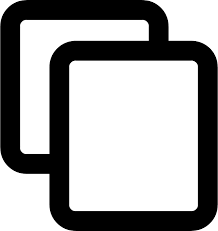
// Installing GCC on Linux
sudo apt update
sudo apt install gcc
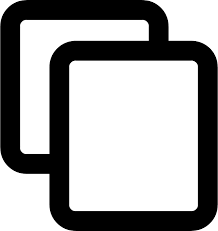
#include <stdio.h>
int main() {
printf("Hello, World!\\n");
return 0;
}
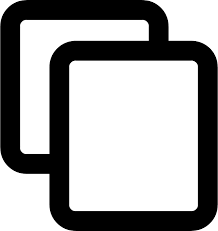
// Compiling a C program
gcc program.c -o program
// Running the program
./program
Basic Structure of a C Program
A typical C program consists of the following parts:
- Headers: Include necessary header files like <stdio.h> and <stdlib.h>.
- Main Function: The entry point of the program, usually written as
int main()
. - Statements: Instructions to perform operations, written inside the main function.
Conclusion
C programming is a powerful skill that opens the door to numerous opportunities in software development, system design, and computer science. Its simplicity, efficiency, and versatility make it a valuable tool for developers of all levels.
Features of C
The C programming language offers a variety of features that make it a widely used and versatile tool in software development. These features contribute to its efficiency, adaptability, and broad applicability in different domains.
Key Features of C Programming
- Simple Syntax: C has a straightforward and easy-to-understand syntax, making it a great choice for beginners.
- Portability: Programs written in C can be executed on different platforms with minimal or no changes, making it a portable language.
- Efficiency: C provides low-level access to memory and system resources, resulting in highly efficient programs.
- Structured Language: C supports structured programming, which allows developers to break their code into smaller, reusable modules for better organization and debugging.
- Rich Library: C comes with a rich set of built-in functions and libraries that simplify complex operations.
- Extensibility: C allows programmers to create custom libraries and functions to extend its capabilities.
- Low-Level Manipulation: C enables direct manipulation of hardware and memory, making it ideal for system-level programming.
- Dynamic Memory Allocation: C provides functions like
malloc()
andfree()
for efficient use of memory.
Why Are These Features Important?
The features of C make it an ideal choice for a wide range of applications, from system programming to application development. Its efficiency and simplicity have contributed to its continued relevance in modern software development.
Applications of C Based on Its Features
- Operating Systems: C’s low-level capabilities make it suitable for developing operating systems like Linux and Windows.
- Embedded Systems: Its efficiency and portability are crucial for developing firmware and embedded applications.
- Game Development: C is used in game engines for its high performance and control over system resources.
- Network Programming: C’s robustness and low-level access are ideal for network protocol implementations.
Conclusion
The features of C have made it a cornerstone of computer science and programming. Its efficiency, portability, and versatility make it a powerful language for developers seeking to create robust and high-performance applications.
Setting Up the C Environment (IDE/Compiler)
To start programming in C, you need to set up a development environment that includes a text editor or Integrated Development Environment (IDE) and a C compiler. This setup allows you to write, compile, and execute C programs efficiently.
Steps to Set Up the C Environment
- Choose a Text Editor or IDE:
Select a tool for writing your C programs. Popular options include:
- Code::Blocks: A free, open-source IDE designed for C and C++ development. It provides an integrated compiler and debugger.
- Visual Studio Code (VS Code): A lightweight, versatile editor with extensions for C/C++ development.
- Dev-C++: An IDE with a built-in compiler and debugger.
- Install a C Compiler:
A compiler converts your C code into machine-readable instructions. Commonly used C compilers include:
- GCC (GNU Compiler Collection): A widely used, open-source compiler available for Linux, Windows, and macOS.
- Clang: A fast and modern compiler compatible with GCC.
- MinGW: A minimalistic GNU for Windows that provides GCC and other tools.
- Set Up the Compiler:
After installing a compiler, configure your environment to use it. For example, ensure the compiler is added to your system’s PATH variable for easy access.
- Write Your First Program:
Open your IDE or text editor and write a simple C program to test your setup:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
- Compile and Run:
Use your compiler to compile the program into an executable file and run it:
// Compile the program gcc program.c -o program // Run the program ./program
Testing Your Setup
Ensure your environment is correctly configured by writing and running a simple program. If you encounter errors, verify the installation paths and compiler settings.
Tips for a Smooth Setup
- Use an All-in-One IDE: Tools like Code::Blocks and Dev-C++ come with a pre-configured compiler, simplifying the setup process.
- Keep Your Tools Updated: Ensure your IDE and compiler are up to date for the latest features and bug fixes.
- Refer to Documentation: Consult the official documentation for your chosen tools if you face issues during installation or configuration.
Conclusion
Setting up the C environment is the first step toward learning C programming. With the right tools and configuration, you can efficiently write, compile, and execute C programs. Once your environment is ready, you can focus on learning and building projects in C.
Writing Your First C Program
Writing your first C program is an exciting step in learning the C programming language. This guide will walk you through creating, compiling, and running a simple "Hello, World!" program in C.
Steps to Write Your First C Program
- Set Up Your Environment:
Ensure your development environment is ready, including a text editor or IDE and a C compiler. Refer to the "Setting Up the C Environment" section if needed.
- Create a New File:
Open your text editor or IDE and create a new file. Save it with a
.c
extension, such ashello.c
. - Write the Code:
Type the following code into your file:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
This program does the following:
#include <stdio.h>:
Includes the standard input/output library for functions likeprintf
.int main():
Defines the main function where the program starts execution.printf("Hello, World!\n");
Prints the message "Hello, World!" to the console.return 0;
Indicates that the program executed successfully.
- Compile the Program:
Open your terminal or command prompt and navigate to the directory where you saved the file. Use the following command to compile the program:
gcc hello.c -o hello
This creates an executable file named
hello
. - Run the Program:
Execute the compiled program with the following command:
./hello
You should see the output:
Hello, World!
Understanding the Program
This simple program introduces key concepts of C programming:
- The
#include
directive for importing libraries. - The
main()
function as the entry point of a C program. - Using
printf
to display text on the console. - Returning a value from the
main()
function to indicate program success or failure.
Common Errors and Troubleshooting
- Compiler Not Found: Ensure your compiler (e.g., GCC) is correctly installed and added to the system PATH.
- Syntax Errors: Double-check your code for typos or missing semicolons (
;
). - File Not Found: Ensure the terminal is in the correct directory where your file is saved.
Conclusion
Congratulations! You’ve written, compiled, and executed your first C program. This foundational step sets the stage for exploring more advanced features and building complex applications in C.
Compiling and Running a C Program
After writing your C program, the next step is to compile it into an executable file and run it. This process converts your source code into machine-readable instructions that your computer can execute.
Steps to Compile and Run a C Program
- Write Your Program:
Create a
.c
file (e.g.,example.c
) and write your C code. For instance:#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
- Open a Terminal or Command Prompt:
Navigate to the directory where your
.c
file is saved. Use thecd
command to change directories:cd path/to/your/file
- Compile the Program:
Use a C compiler like GCC to compile your program. The syntax is:
gcc example.c -o example
Explanation:
gcc:
Invokes the GNU Compiler Collection.example.c:
Specifies the source code file.-o example:
Specifies the name of the output executable file (in this case,example
).
If the compilation is successful, no errors will be displayed, and an executable file will be created.
- Run the Program:
Execute the compiled program using the following command:
./example
You should see the output:
Hello, World!
Common Compilation Errors
- Syntax Errors: Ensure your code follows the C syntax rules. Check for missing semicolons or unmatched braces.
- File Not Found: Verify the filename and ensure you are in the correct directory.
- Undefined Reference: Ensure all required functions are included in the program and necessary libraries are linked.
Compiling Multiple Files
If your program consists of multiple source files, you can compile them together:
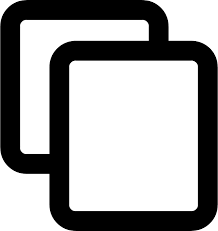
gcc file1.c file2.c -o program
This creates a single executable program
from the source files.
Using Makefiles for Automation
For larger projects, consider using a Makefile to automate the compilation process. A sample Makefile might look like this:
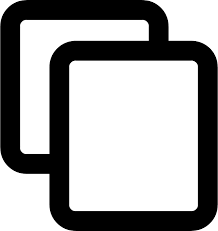
program: file1.o file2.o
gcc file1.o file2.o -o program
file1.o: file1.c
gcc -c file1.c
file2.o: file2.c
gcc -c file2.c
Run make
in the terminal to compile the program.
Conclusion
Compiling and running a C program is a straightforward process once you understand the steps. Mastering this will allow you to test and debug your programs effectively as you progress in C programming.
Syntax and Structure of a C Program
Understanding the syntax and structure of a C program is fundamental to writing efficient and error-free code. This guide explains the basic building blocks and layout of a typical C program.
Basic Structure of a C Program
A C program typically consists of the following components:
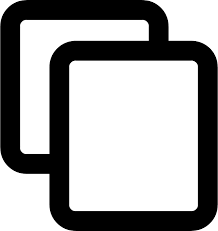
// Header files
#include <stdio.h>
// Main function
int main() {
// Code logic
printf("Hello, World!\n");
return 0;
}
Key Components of a C Program
- Header Files:
Header files contain declarations of standard functions and macros. The
#include
directive is used to include them in the program. For example:#include <stdio.h>
- Main Function:
The
main()
function is the entry point of every C program. It is where the execution starts. For example:int main() { return 0; }
- Statements:
Statements are the instructions that the program executes. Each statement ends with a semicolon (
;
). - Functions:
Functions are blocks of reusable code. The
main()
function is a special function, but you can define your own functions as needed. - Comments:
Comments are used to make the code more readable. They are ignored by the compiler. There are two types of comments:
- Single-line comments:
// This is a comment
- Multi-line comments:
/* This is a multi-line comment */
- Single-line comments:
Syntax Rules in C
- Case Sensitivity: C is case-sensitive. For example,
main
andMain
are different. - Semicolons: Every statement must end with a semicolon (
;
). - Braces: Curly braces (
{}
) are used to group statements into a block. - Identifiers: Names for variables, functions, etc., must start with a letter or an underscore and can contain letters, digits, or underscores.
- Keywords: Reserved words like
int
,return
, andif
cannot be used as identifiers.
Example of a Simple C Program
Here is an example of a simple C program that adds two numbers:
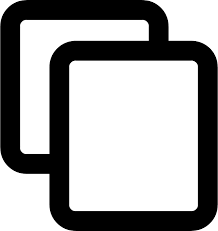
#include <stdio.h>
int main() {
int a = 5, b = 10, sum;
sum = a + b;
printf("Sum = %d\n", sum);
return 0;
}
Output:
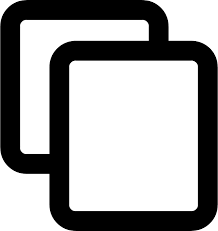
Sum = 15
Common Mistakes to Avoid
- Omitting the semicolon (
;
) at the end of statements. - Using undeclared variables or functions.
- Forgetting to include necessary header files.
- Mismatching braces or parentheses.
Conclusion
By understanding the syntax and structure of a C program, you can write programs that are well-structured and easy to understand. Practice writing small programs to become familiar with these concepts.
Variables and Data Types
In C programming, variables and data types are fundamental concepts. Variables are used to store data, and data types define the type of data a variable can hold.
What is a Variable?
A variable is a named storage location in memory that holds a value. You can declare a variable by specifying its data type and name.
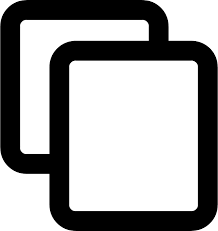
data_type variable_name;
For example:
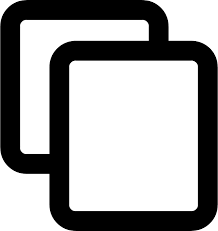
int age;
float height;
char grade;
Here, age
, height
, and grade
are variables of types int
, float
, and char
, respectively.
Rules for Naming Variables
- Variable names must begin with a letter or underscore (
_
). - They can contain letters, digits, and underscores, but no spaces or special characters.
- They cannot be a C keyword (e.g.,
int
,return
). - Variable names are case-sensitive (e.g.,
age
andAge
are different).
What are Data Types?
Data types specify the type of data that a variable can hold. C provides several built-in data types:
Basic Data Types
int
: Used for integers. Size: 2 or 4 bytes.float
: Used for floating-point numbers. Size: 4 bytes.double
: Used for double-precision floating-point numbers. Size: 8 bytes.char
: Used for single characters. Size: 1 byte.
Derived Data Types
- Array: A collection of elements of the same data type.
- Pointer: A variable that stores the address of another variable.
- Structure: A collection of variables of different types.
Void Data Type
void
is used for functions that do not return a value.
Declaring and Initializing Variables
You can declare a variable and assign a value to it at the same time:
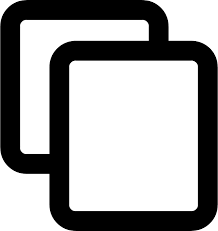
int age = 25;
float height = 5.9;
char grade = 'A';
Or declare it first and assign a value later:
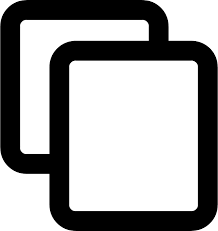
int age;
age = 25;
Example Program
Here’s an example demonstrating variables and data types in C:
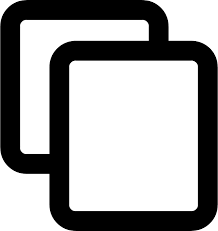
#include <stdio.h>
int main() {
int age = 25;
float height = 5.9;
char grade = 'A';
printf("Age: %d\\n", age);
printf("Height: %.1f\\n", height);
printf("Grade: %c\\n", grade);
return 0;
}
Output:
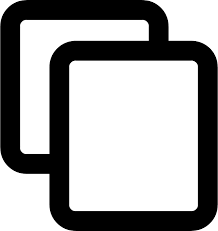
Age: 25
Height: 5.9
Grade: A
Common Mistakes to Avoid
- Using a variable without declaring it.
- Assigning a value of the wrong data type to a variable.
- Exceeding the storage capacity of a data type.
Conclusion
Variables and data types are essential for storing and manipulating data in a C program. Understanding how to use them effectively is crucial for writing efficient and error-free code.
Constants and Literals
In C programming, constants and literals are used to represent fixed values that do not change during the execution of a program. Understanding these concepts is essential for writing clean and efficient code.
What are Constants?
Constants are variables whose values cannot be changed after they are defined. In C, constants are declared using the const
keyword or #define
preprocessor directive.
Using the const
Keyword
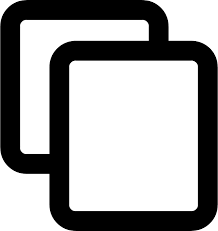
const int MAX_AGE = 100;
Using the #define
Preprocessor Directive
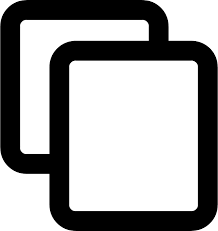
#define PI 3.14159
Types of Constants
- Integer Constants: Whole numbers without a fractional part, e.g.,
10
,-45
. - Floating-Point Constants: Numbers with a fractional part, e.g.,
3.14
,-0.001
. - Character Constants: A single character enclosed in single quotes, e.g.,
'A'
,'9'
. - String Constants: A sequence of characters enclosed in double quotes, e.g.,
"Hello, World!"
.
What are Literals?
Literals are fixed values directly used in the code without being assigned to variables. For example:
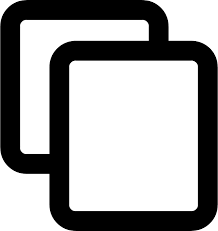
int age = 25; // 25 is an integer literal
float pi = 3.14; // 3.14 is a floating-point literal
char grade = 'A'; // 'A' is a character literal
char str[] = "C Programming"; // "C Programming" is a string literal
Types of Literals
- Integer Literals: Represent whole numbers, e.g.,
10
,0xFF
(hexadecimal). - Floating-Point Literals: Represent decimal numbers, e.g.,
2.5
,1.2e3
(scientific notation). - Character Literals: Represent single characters enclosed in single quotes, e.g.,
'A'
. - String Literals: Represent a sequence of characters enclosed in double quotes, e.g.,
"Hello!"
.
Example Program
The following example demonstrates the use of constants and literals:
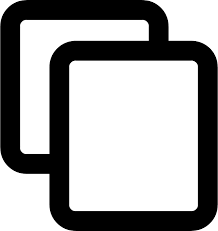
#include <stdio.h>
#define PI 3.14159 // Defining a constant using #define
int main() {
const int MAX_VALUE = 100; // Defining a constant using const
int radius = 5;
float area = PI * radius * radius;
printf("The maximum value is %d\\n", MAX_VALUE);
printf("The area of the circle is %.2f\\n", area);
return 0;
}
Output:
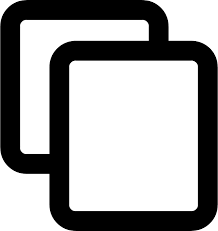
The maximum value is 100
The area of the circle is 78.54
Common Mistakes to Avoid
- Trying to modify the value of a
const
variable. - Using literals without proper data types, e.g., assigning a floating-point literal to an integer variable.
- Forgetting to use quotes for string and character literals.
Conclusion
Constants and literals are essential tools for creating readable and maintainable programs. By using them effectively, you can ensure that your code is both robust and easy to understand.
Input and Output Functions (printf, scanf)
In C programming, printf
and scanf
are the most commonly used functions for outputting data to the screen and taking input from the user. Understanding these functions is essential for interacting with users in a C program.
What is printf
?
The printf
function is used to display output on the screen. It is part of the stdio.h
library. The syntax of printf
is:
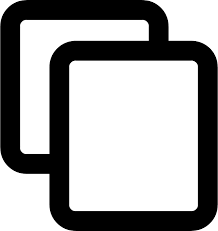
printf("format string", argument_list);
The format string contains text and format specifiers that define how the arguments are displayed.
Common Format Specifiers
%d
: Displays an integer.%f
: Displays a floating-point number.%c
: Displays a single character.%s
: Displays a string.%%
: Displays a percent sign.
Example:
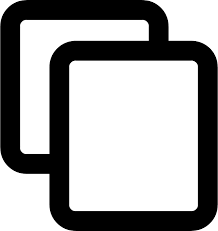
#include <stdio.h>
int main() {
int age = 25;
float height = 5.9;
char grade = 'A';
printf("Age: %d\\n", age);
printf("Height: %.1f\\n", height);
printf("Grade: %c\\n", grade);
return 0;
}
Output:
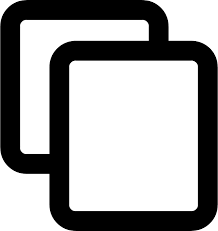
Age: 25
Height: 5.9
Grade: A
What is scanf
?
The scanf
function is used to take input from the user. It reads formatted input from the keyboard and stores it in variables. The syntax of scanf
is:
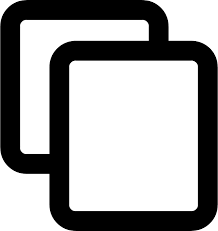
scanf("format string", &variable);
The ampersand (&
) is used to pass the address of the variable where the input will be stored.
Example:
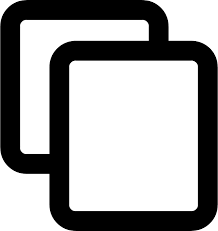
#include <stdio.h>
int main() {
int age;
float height;
char grade;
printf("Enter your age: ");
scanf("%d", &age);
printf("Enter your height: ");
scanf("%f", &height);
printf("Enter your grade: ");
scanf(" %c", &grade); // Note the space before %c to handle newline character
printf("You entered:\\n");
printf("Age: %d\\n", age);
printf("Height: %.2f\\n", height);
printf("Grade: %c\\n", grade);
return 0;
}
Output (example user input):
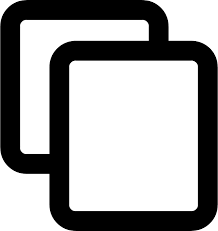
Enter your age: 25
Enter your height: 5.9
Enter your grade: A
You entered:
Age: 25
Height: 5.90
Grade: A
Common Mistakes to Avoid
- Forgetting to include
stdio.h
, which causes errors while usingprintf
andscanf
. - Not using the
&
symbol withscanf
for variables (except for arrays). - Incorrect format specifiers, which can lead to unexpected behavior.
- Ignoring the newline issue when reading a
char
after other inputs inscanf
.
Conclusion
The printf
and scanf
functions are the foundation of input and output in C programs. Mastering their usage will allow you to build interactive and user-friendly applications.
Arithmetic Operators in C
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, division, and modulus on numeric data types (such as integers and floats) in C programming.
List of Arithmetic Operators
- +: Addition - Adds two operands.
- -: Subtraction - Subtracts the second operand from the first.
- *: Multiplication - Multiplies two operands.
- /: Division - Divides the first operand by the second.
- %: Modulus - Returns the remainder of the division of the first operand by the second.
Operator Precedence
In C, arithmetic operators follow the standard mathematical order of precedence. For example, multiplication and division have higher precedence than addition and subtraction. Parentheses can be used to modify the order of operations.
The precedence order from highest to lowest is as follows:
- Multiplication, Division, Modulus (*, /, %)
- Addition, Subtraction (+, -)
Examples
The following example demonstrates the use of arithmetic operators:
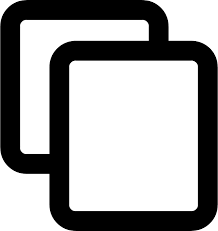
#include <stdio.h>
int main() {
int a = 10, b = 5;
float c = 7.5, d = 2.5;
// Addition
printf("a + b = %d\\n", a + b);
printf("c + d = %.2f\\n", c + d);
// Subtraction
printf("a - b = %d\\n", a - b);
// Multiplication
printf("a * b = %d\\n", a * b);
printf("c * d = %.2f\\n", c * d);
// Division
printf("a / b = %d\\n", a / b);
printf("c / d = %.2f\\n", c / d);
// Modulus
printf("a %% b = %d\\n", a % b); // Double %% to escape in printf
return 0;
}
Output:
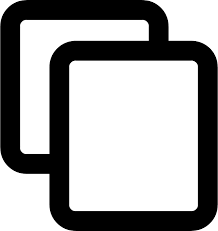
a + b = 15
c + d = 10.00
a - b = 5
a * b = 50
c * d = 18.75
a / b = 2
c / d = 3.00
a % b = 0
Important Notes
- Integer Division: When both operands are integers, division results in an integer value. Any decimal part is discarded.
- Floating-Point Division: If at least one operand is a floating-point number (e.g.,
float
ordouble
), the result is a floating-point value. - Modulus Operator: The modulus operator only works with integers and returns the remainder after division.
Common Mistakes to Avoid
- Trying to use the modulus operator with floating-point numbers, which will result in an error.
- Forgetting to include the correct data type when performing operations (e.g., using
float
for decimals). - Misunderstanding the result of integer division, where the fractional part is truncated.
Conclusion
Arithmetic operators in C are fundamental for performing mathematical calculations. By understanding how each operator works and their precedence, you can perform a wide variety of operations in your C programs efficiently and accurately.
Relational and Logical Operators in C
Relational and logical operators are used in C to perform comparisons and logical tests. They are essential for making decisions and controlling the flow of a program through conditional statements like if
, else
, and loops like while
and for
.
Relational Operators
Relational operators are used to compare two values. The result of a relational operation is either true (1) or false (0), depending on whether the condition is satisfied.
- ==: Equal to - Returns true if the operands are equal.
- !=: Not equal to - Returns true if the operands are not equal.
- >: Greater than - Returns true if the first operand is greater than the second.
- <: Less than - Returns true if the first operand is less than the second.
- >=: Greater than or equal to - Returns true if the first operand is greater than or equal to the second.
- <=: Less than or equal to - Returns true if the first operand is less than or equal to the second.
Logical Operators
Logical operators are used to combine multiple conditions. The result of a logical operation is either true (1) or false (0).
- &&&: Logical AND - Returns true if both conditions are true.
- ||: Logical OR - Returns true if either of the conditions is true.
- !: Logical NOT - Reverses the result of a condition; returns true if the condition is false, and false if the condition is true.
Examples of Relational and Logical Operators
The following program demonstrates the use of relational and logical operators:
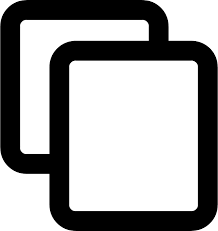
#include <stdio.h>
int main() {
int a = 10, b = 5, c = 20;
// Relational Operators
printf("a == b: %d\\n", a == b); // False (0)
printf("a != b: %d\\n", a != b); // True (1)
printf("a > b: %d\\n", a > b); // True (1)
printf("b < c: %d\\n", b < c); // True (1)
printf("a >= c: %d\\n", a >= c); // False (0)
// Logical Operators
printf("a > b && b < c: %d\\n", a > b && b < c); // True (1)
printf("a > b || a < c: %d\\n", a > b || a < c); // True (1)
printf("!(a == b): %d\\n", !(a == b)); // True (1)
return 0;
}
Output:
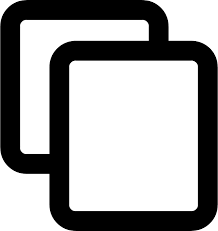
a == b: 0
a != b: 1
a > b: 1
b < c: 1
a >= c: 0
a > b && b < c: 1
a > b || a < c: 1
!(a == b): 1
Why Use Relational and Logical Operators?
- Decision Making: Relational operators are used in conditional statements to make decisions based on comparisons between values.
- Combining Conditions: Logical operators allow you to combine multiple conditions to evaluate more complex expressions.
- Control Flow: These operators are fundamental in controlling the flow of execution in a program, such as determining whether a loop continues or if a specific block of code is executed.
Common Mistakes to Avoid
- Confusing the assignment operator (=) with the equality operator (==). The assignment operator is used to assign a value, while the equality operator checks for equality.
- Using logical operators incorrectly. Ensure that logical expressions are combined properly to avoid unexpected results.
- Neglecting operator precedence. Logical and relational operators have different precedence, so parentheses are sometimes necessary to clarify the order of operations.
Conclusion
Relational and logical operators are critical for controlling program flow and decision-making in C. Understanding how to use these operators effectively enables you to write more complex and dynamic programs that can handle various conditions and scenarios.
Bitwise Operators in C
Bitwise operators in C are used to perform bit-level operations on data. These operators work on individual bits of data, allowing you to manipulate data at the binary level. Bitwise operations are often used in low-level programming, such as device drivers, cryptography, and networking.
List of Bitwise Operators
- &: Bitwise AND - Performs logical AND operation on each corresponding bit of two operands.
- |: Bitwise OR - Performs logical OR operation on each corresponding bit of two operands.
- ^: Bitwise XOR (Exclusive OR) - Performs logical XOR operation on each corresponding bit of two operands. The result is 1 if the bits are different, and 0 if they are the same.
- ~: Bitwise NOT - Inverts all the bits of the operand (flips 0s to 1s and 1s to 0s).
- <<: Left Shift - Shifts the bits of the operand to the left by a specified number of positions. Zeros are shifted in from the right.
- >>: Right Shift - Shifts the bits of the operand to the right by a specified number of positions. The leftmost bits are filled with the sign bit (for signed numbers).
Examples of Bitwise Operators
The following program demonstrates the use of bitwise operators:
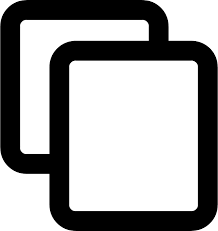
#include <stdio.h>
int main() {
int a = 5, b = 9;
// Bitwise AND
printf("a & b = %d\\n", a & b);
// Bitwise OR
printf("a | b = %d\\n", a | b);
// Bitwise XOR
printf("a ^ b = %d\\n", a ^ b);
// Bitwise NOT
printf("~a = %d\\n", ~a);
// Left Shift
printf("a << 1 = %d\\n", a << 1);
// Right Shift
printf("a >> 1 = %d\\n", a >> 1);
return 0;
}
Output:
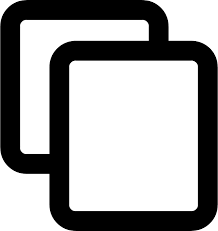
a & b = 1
a | b = 13
a ^ b = 12
~a = -6
a << 1 = 10
a >> 1 = 2
Explanation of the Operations
- Bitwise AND (&): The result is 1 only if both bits are 1. For example, 5 (0101) & 9 (1001) results in 1 (0001).
- Bitwise OR (|): The result is 1 if at least one of the bits is 1. For example, 5 (0101) | 9 (1001) results in 13 (1101).
- Bitwise XOR (^): The result is 1 if the bits are different. For example, 5 (0101) ^ 9 (1001) results in 12 (1100).
- Bitwise NOT (~): This operator inverts all the bits. For example, ~5 (0101) results in -6 (in two's complement representation).
- Left Shift (<<): Shifts the bits of the number to the left, filling the rightmost bits with 0s. For example, 5 (0101) << 1 results in 10 (1010).
- Right Shift (>>): Shifts the bits of the number to the right. For example, 5 (0101) >> 1 results in 2 (0010).
Why Use Bitwise Operators?
- Efficiency: Bitwise operators are often more efficient than arithmetic operators, especially in performance-critical applications like embedded systems and graphics programming.
- Low-level Control: Bitwise operations allow direct manipulation of individual bits, which is useful in hardware programming and optimizing memory usage.
- Data Encoding: Bitwise operators are frequently used in data encryption, compression, and checksums, where data is encoded at the bit level.
Common Mistakes to Avoid
- Confusing bitwise AND with logical AND (&&). Bitwise operators work on individual bits, while logical operators work on boolean expressions.
- Using bitwise operators with non-integer types, as they are designed to work with integers and can produce unexpected results with floating-point types.
- Misunderstanding the effects of the shift operators. Left shifting can result in data loss if bits are shifted out of bounds, and right shifting of signed numbers can lead to undefined behavior in some cases.
Conclusion
Bitwise operators in C provide powerful tools for performing bit-level operations. While they may not be needed in everyday programming, they are essential in low-level programming, such as embedded systems, cryptography, and performance optimization. Understanding these operators is crucial for efficiently manipulating data at the binary level.
Assignment Operators in C
Assignment operators in C are used to assign values to variables. The most basic assignment operator is the equal sign (=
), which assigns the value of the right-hand operand to the left-hand operand. However, C also provides several shorthand assignment operators that combine an arithmetic operation with assignment to make code more concise.
List of Assignment Operators
- =: Simple assignment - Assigns the value of the right operand to the left operand.
- +=: Addition assignment - Adds the right operand to the left operand and assigns the result to the left operand.
- -=: Subtraction assignment - Subtracts the right operand from the left operand and assigns the result to the left operand.
- *=: Multiplication assignment - Multiplies the left operand by the right operand and assigns the result to the left operand.
- /=: Division assignment - Divides the left operand by the right operand and assigns the result to the left operand.
- %=: Modulus assignment - Takes the modulus of the left operand divided by the right operand and assigns the result to the left operand.
Examples of Assignment Operators
The following program demonstrates the use of assignment operators:
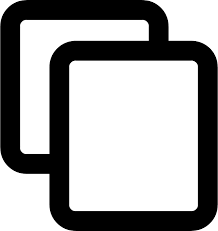
#include <stdio.h>
int main() {
int a = 10, b = 5;
// Simple assignment
a = b;
printf("a = %d\\n", a); // Output: a = 5
// Addition assignment
a += b;
printf("a += b: %d\\n", a); // Output: a = 10
// Subtraction assignment
a -= b;
printf("a -= b: %d\\n", a); // Output: a = 5
// Multiplication assignment
a *= b;
printf("a *= b: %d\\n", a); // Output: a = 25
// Division assignment
a /= b;
printf("a /= b: %d\\n", a); // Output: a = 5
// Modulus assignment
a %= b;
printf("a %%= b: %d\\n", a); // Output: a = 0
return 0;
}
Output:
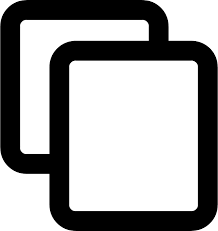
a = 5
a += b: 10
a -= b: 5
a *= b: 25
a /= b: 5
a %= b: 0
Explanation of the Operations
- = (Simple Assignment): The value of the right operand is assigned to the left operand. For example,
a = b;
assigns the value ofb
toa
. - += (Addition Assignment): Adds the value of the right operand to the left operand and assigns the result to the left operand. For example,
a += b;
is equivalent toa = a + b;
. - -= (Subtraction Assignment): Subtracts the value of the right operand from the left operand and assigns the result to the left operand. For example,
a -= b;
is equivalent toa = a - b;
. - *= (Multiplication Assignment): Multiplies the left operand by the right operand and assigns the result to the left operand. For example,
a *= b;
is equivalent toa = a * b;
. - /= (Division Assignment): Divides the left operand by the right operand and assigns the result to the left operand. For example,
a /= b;
is equivalent toa = a / b;
. - %= (Modulus Assignment): Takes the modulus of the left operand divided by the right operand and assigns the result to the left operand. For example,
a %= b;
is equivalent toa = a % b;
.
Why Use Assignment Operators?
- Simplicity: Assignment operators allow you to perform arithmetic operations and assignment in a single, concise statement, improving code readability and maintainability.
- Optimization: They are often used in loops and functions where repeated arithmetic operations are required, making the code more efficient and compact.
- Code Clarity: Using shorthand assignment operators helps clarify the intent of the code, reducing the chance of errors and making the code easier to understand.
Common Mistakes to Avoid
- Using the assignment operator (
=
) when a comparison operator (==
) is needed. For example,a = b
assigns the value ofb
toa
, whereasa == b
checks whethera
is equal tob
. - Forgetting to check for division by zero when using the division or modulus assignment operators. Ensure that the right operand is not zero before performing
/=
or%=
. - Overwriting the original value of a variable before performing an operation that depends on its initial value. Always ensure that the variable's value is updated in the correct order.
Conclusion
Assignment operators in C provide a concise and efficient way to assign values to variables while performing arithmetic operations. Mastering these operators helps improve the readability and compactness of your code, making it easier to manage and maintain. Whether you're performing simple assignments or complex arithmetic operations, these operators are essential for effective programming in C.
Increment and Decrement Operators in C
In C, the increment (++) and decrement (--) operators are unary operators that increase or decrease the value of a variable by one, respectively. These operators are often used in loops and expressions where you need to adjust the value of a variable by a fixed amount.
Types of Increment and Decrement Operators
- Prefix Increment (++): Increases the value of the variable before it is used in the expression.
- Postfix Increment (++): Increases the value of the variable after it is used in the expression.
- Prefix Decrement (--): Decreases the value of the variable before it is used in the expression.
- Postfix Decrement (--): Decreases the value of the variable after it is used in the expression.
Examples of Increment and Decrement Operators
The following program demonstrates the use of increment and decrement operators:
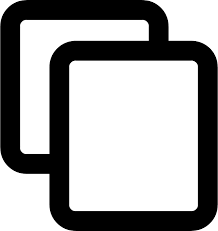
#include <stdio.h>
int main() {
int a = 5, b = 5, c;
// Prefix Increment
c = ++a;
printf("Prefix Increment: a = %d, c = %d\\n", a, c); // Output: a = 6, c = 6
// Postfix Increment
c = b++;
printf("Postfix Increment: b = %d, c = %d\\n", b, c); // Output: b = 6, c = 5
// Prefix Decrement
c = --a;
printf("Prefix Decrement: a = %d, c = %d\\n", a, c); // Output: a = 5, c = 5
// Postfix Decrement
c = b--;
printf("Postfix Decrement: b = %d, c = %d\\n", b, c); // Output: b = 5, c = 6
return 0;
}
Output:
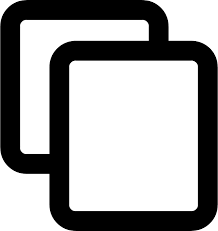
Prefix Increment: a = 6, c = 6
Postfix Increment: b = 6, c = 5
Prefix Decrement: a = 5, c = 5
Postfix Decrement: b = 5, c = 6
Explanation of the Operations
- Prefix Increment (++a): The value of
a
is increased by 1 before it is assigned toc
. So, the value ofa
becomes 6, andc
also gets the value 6. - Postfix Increment (b++): The value of
b
is assigned toc
first, and thenb
is incremented. So,c
gets the old value ofb
(5), and thenb
becomes 6. - Prefix Decrement (--a): The value of
a
is decreased by 1 before it is assigned toc
. So, the value ofa
becomes 5, andc
also gets the value 5. - Postfix Decrement (b--): The value of
b
is assigned toc
first, and thenb
is decremented. So,c
gets the old value ofb
(6), and thenb
becomes 5.
When to Use Increment and Decrement Operators
- In Loops: The increment and decrement operators are commonly used in loop control structures, such as
for
andwhile
, to adjust the loop counter. - In Expressions: These operators can be used in expressions to increase or decrease a variable's value without needing to write additional lines of code.
- In Conditional Statements: You can use them in conditions where a variable needs to be adjusted by one unit before or after the comparison.
Common Mistakes to Avoid
- Confusing the order of operations in prefix and postfix forms. Remember that in prefix form (
++a
), the value is incremented before it is used, while in postfix form (a++
), the value is used before it is incremented. - Using increment and decrement operators in complex expressions where the order of evaluation might not be clear. This can lead to unexpected results and hard-to-debug code.
- Neglecting to initialize the variable before using the increment or decrement operator. Always ensure the variable has a valid value before performing these operations.
Conclusion
Increment and decrement operators are simple yet powerful tools in C. They allow you to manipulate variables by increasing or decreasing their value by one, making your code more concise and efficient. Whether you're working with loops, expressions, or conditional statements, understanding these operators will help you write cleaner and more effective code in C.
Ternary Operator in C
The ternary operator in C is a shorthand for the if-else
statement. It allows you to make a decision and assign values based on a condition in a more concise manner. The ternary operator is a conditional operator that takes three operands, hence the name "ternary."
Syntax of the Ternary Operator
The syntax of the ternary operator is as follows:
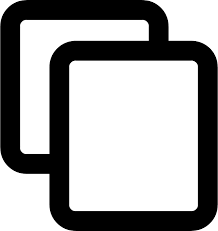
condition ? expression1 : expression2;
- condition: The condition is an expression that evaluates to either true or false (non-zero or zero).
- expression1: This expression is executed if the condition is true (non-zero).
- expression2: This expression is executed if the condition is false (zero).
Example of the Ternary Operator
The following program demonstrates the use of the ternary operator:
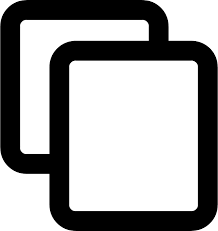
#include <stdio.h>
int main() {
int a = 10, b = 5;
int max;
// Using ternary operator to find the maximum of a and b
max = (a > b) ? a : b;
printf("The maximum value is: %d\\n", max); // Output: The maximum value is: 10
return 0;
}
Output:
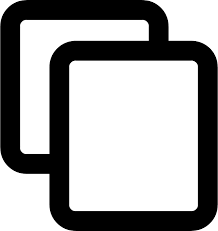
The maximum value is: 10
Explanation of the Example
In this example, the condition (a > b)
is checked. Since a
(10) is greater than b
(5), the ternary operator evaluates to a
, and max
is assigned the value of a
(10).
When to Use the Ternary Operator
The ternary operator is useful in scenarios where you want to write concise code for simple conditional assignments. It can replace simple if-else
statements and make your code more readable when the logic is straightforward.
- Conditional Assignment: Use the ternary operator to assign values to variables based on a condition in one line.
- Shortened If-Else Statements: The ternary operator is ideal for simple conditional checks that return one of two values.
Examples of Ternary Operator
Here are a few more examples:
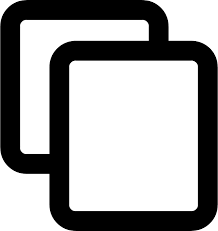
// Example 1: Checking if a number is even or odd
int num = 7;
printf("%d is %s\\n", num, (num % 2 == 0) ? "Even" : "Odd");
// Example 2: Assigning grade based on marks
int marks = 75;
char *grade = (marks >= 50) ? "Pass" : "Fail";
printf("Grade: %s\\n", grade);
Output:
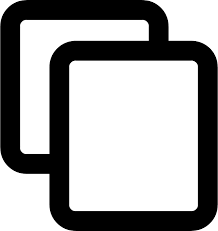
7 is Odd
Grade: Pass
Advantages of Using the Ternary Operator
- Concise Code: It reduces the number of lines compared to writing full
if-else
statements. - Improved Readability: For simple conditions, the ternary operator makes the code more readable, especially when used for variable assignments.
- Inline Operations: The ternary operator can be used inline within expressions or function arguments, making it suitable for compact code.
Limitations of the Ternary Operator
- Complexity: If the condition or the expressions are too complex, using the ternary operator can make the code harder to read and understand.
- Not for Multiple Conditions: When you need to handle multiple conditions, it’s better to use
if-else
statements orswitch
statements for clarity and maintainability.
Conclusion
The ternary operator is a powerful tool in C that allows you to simplify conditional assignments. It is best used for simple conditions where you need to return one of two values based on a condition. However, for more complex logic, traditional if-else
statements may be a better choice for readability.
Decision Making in C: if, if-else, and switch
In C programming, decision-making is crucial for determining the flow of execution based on conditions. The if
, if-else
, and switch
statements are the primary control flow structures used for decision-making.
1. The if
Statement
The if
statement is used to execute a block of code if a specified condition is true. If the condition is false, the code block is skipped.
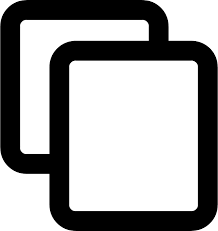
if (condition) {
// Code to execute if the condition is true
}
Example of if
Statement
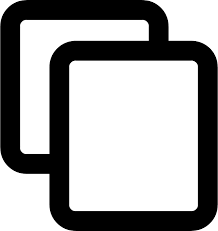
#include <stdio.h>
int main() {
int a = 10;
// If statement to check if the number is positive
if (a > 0) {
printf("The number is positive.\\n");
}
return 0;
}
Output:
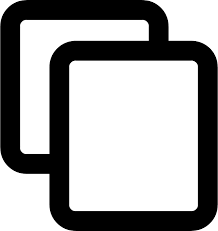
The number is positive.
2. The if-else
Statement
The if-else
statement allows you to execute one block of code if the condition is true and another block of code if the condition is false.
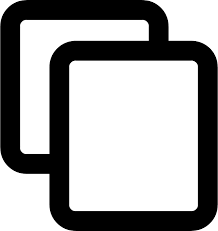
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Example of if-else
Statement
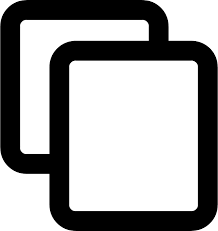
#include <stdio.h>
int main() {
int a = -5;
// If-else statement to check if the number is positive or negative
if (a > 0) {
printf("The number is positive.\\n");
} else {
printf("The number is negative.\\n");
}
return 0;
}
Output:
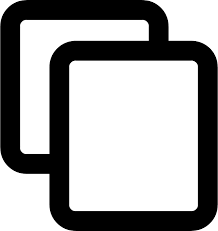
The number is negative.
3. The switch
Statement
The switch
statement allows you to choose between multiple options based on the value of an expression. It is an efficient alternative to multiple if-else
statements when you need to select from a number of possible cases.
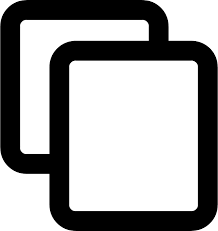
switch (expression) {
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
default:
// Code to execute if no case matches
}
Example of switch
Statement
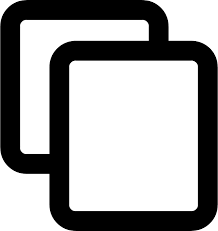
#include <stdio.h>
int main() {
int day = 3;
// Using switch statement to determine the day of the week
switch (day) {
case 1:
printf("Monday\\n");
break;
case 2:
printf("Tuesday\\n");
break;
case 3:
printf("Wednesday\\n");
break;
case 4:
printf("Thursday\\n");
break;
case 5:
printf("Friday\\n");
break;
case 6:
printf("Saturday\\n");
break;
case 7:
printf("Sunday\\n");
break;
default:
printf("Invalid day\\n");
}
return 0;
}
Output:
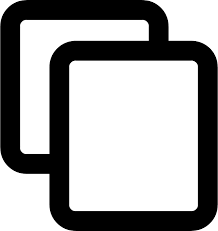
Wednesday
Explanation of the switch
Statement Example
In this example, the value of day
(which is 3) is evaluated in the switch
statement. The program matches case 3
, and the output is "Wednesday". The break
statement ensures that once a case is matched, the program exits the switch block.
When to Use if
, if-else
, and switch
- Use
if
: When you have a simple condition to check, and you only need one block of code to execute if the condition is true. - Use
if-else
: When you need to check a condition and execute one block of code if true and another block if false. - Use
switch
: When you need to evaluate an expression against multiple possible values and execute different code based on the result.
Advantages of Using switch
- Improved readability:
switch
is more readable than multipleif-else
statements when you need to handle many possible cases. - Efficient for large cases: A
switch
statement can be more efficient than multipleif-else
checks, especially for large numbers of conditions.
Limitations of switch
- Only works with integral types:
switch
only works with integer-based types likeint
,char
, andenum
. - Cannot evaluate ranges:
switch
cannot check conditions like "greater than" or "less than" asif
can.
Conclusion
Decision-making structures like if
, if-else
, and switch
are essential for controlling the flow of a C program. Understanding when and how to use each of these constructs will help you write more efficient and readable code.
Loops in C: for, while, and do-while
Loops in C are used to execute a block of code repeatedly based on a condition. The for
, while
, and do-while
loops are the primary loop structures in C programming. They allow you to perform repetitive tasks without writing the same code multiple times.
1. The for
Loop
The for
loop is used when you know in advance how many times you want to execute a statement or a block of statements. It consists of three parts: initialization, condition, and increment/decrement.
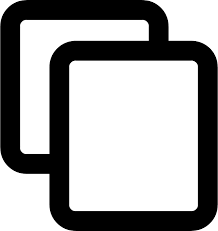
for (initialization; condition; increment/decrement) {
// Code to execute
}
Example of for
Loop
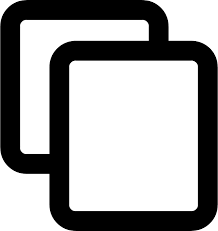
#include <stdio.h>
int main() {
// Using a for loop to print numbers from 1 to 5
for (int i = 1; i <= 5; i++) {
printf("%d\\n", i);
}
return 0;
}
Output:
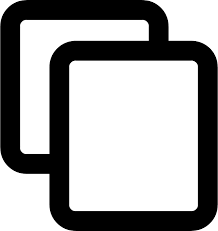
1
2
3
4
5
Explanation of the for
Loop Example
In this example, the loop starts with i = 1
, and as long as i <= 5
is true, the loop executes. After each iteration, i
is incremented by 1, and the loop stops when i
becomes 6.
2. The while
Loop
The while
loop is used when you want to repeat a block of code an unknown number of times, as long as the condition is true. The condition is checked before each iteration.
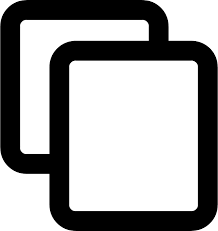
while (condition) {
// Code to execute
}
Example of while
Loop
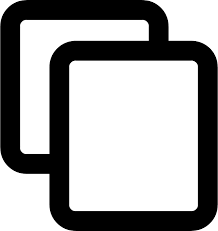
#include <stdio.h>
int main() {
int i = 1;
// Using a while loop to print numbers from 1 to 5
while (i <= 5) {
printf("%d\\n", i);
i++;
}
return 0;
}
Output:
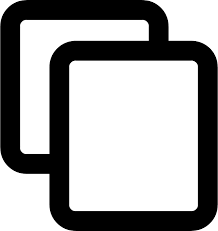
1
2
3
4
5
Explanation of the while
Loop Example
In this example, the loop continues to execute as long as i <= 5
is true. The variable i
starts at 1 and is incremented inside the loop. The loop stops when i
becomes 6.
3. The do-while
Loop
The do-while
loop is similar to the while
loop, but with one key difference: it always executes the block of code at least once before checking the condition. The condition is checked after the execution of the block.
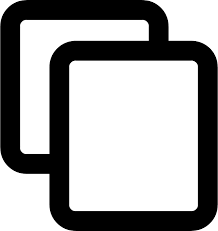
do {
// Code to execute
} while (condition);
Example of do-while
Loop
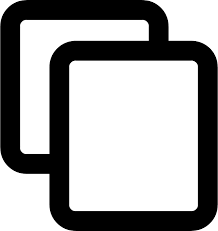
#include <stdio.h>
int main() {
int i = 1;
// Using a do-while loop to print numbers from 1 to 5
do {
printf("%d\\n", i);
i++;
} while (i <= 5);
return 0;
}
Output:
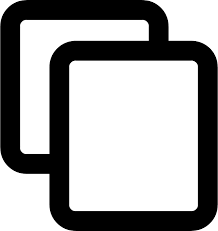
1
2
3
4
5
Explanation of the do-while
Loop Example
In this example, the loop executes at least once before checking the condition. The value of i
is printed first, and then i
is incremented. The loop stops when i
becomes 6.
When to Use for
, while
, and do-while
- Use
for
: When you know beforehand how many times you need to repeat a block of code, such as iterating through an array or a range of numbers. - Use
while
: When the number of iterations is not known in advance, and you need to repeat a block of code while a condition is true. - Use
do-while
: When you want to ensure that the loop executes at least once, regardless of the condition.
Advantages of Using Loops
- Code Reusability: Loops allow you to write code that can be reused multiple times without repetition, making your code cleaner and more efficient.
- Control Over Iteration: You can control the number of times a block of code is executed based on conditions, providing flexibility in your programs.
- Efficient Computation: Loops allow you to perform repetitive tasks in an efficient manner, avoiding manual repetition.
Conclusion
Loops are essential in C programming for performing repetitive tasks efficiently. The for
, while
, and do-while
loops each have their use cases, and understanding when to use each type will help you write more flexible and efficient programs.
Nested Loops in C
In C programming, a nested loop is a loop inside another loop. The inner loop is executed completely every time the outer loop runs once. Nested loops are used when you need to perform a set of operations multiple times for each iteration of another set of operations.
Syntax of Nested Loops
The syntax for nested loops in C is similar to a single loop. You can use any type of loop (for, while, do-while) within another loop.
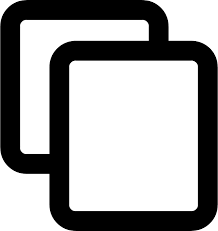
for (initialization; condition; increment/decrement) {
// Outer loop body
for (initialization; condition; increment/decrement) {
// Inner loop body
}
}
Example of Nested for
Loop
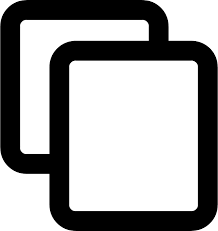
#include <stdio.h>
int main() {
// Using nested for loops to print a multiplication table
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5; j++) {
printf("%d\t", i * j); // Printing the multiplication table
}
printf("\\n"); // Newline after each row
}
return 0;
}
Output:
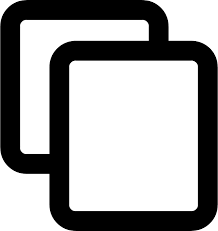
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
Explanation of the Nested for
Loop Example
In this example, there are two for
loops: the outer loop runs 5 times (for values of i
from 1 to 5), and for each iteration of the outer loop, the inner loop runs 5 times (for values of j
from 1 to 5). Each time the inner loop runs, it prints the product of i
and j
in a tabular format.
Example of Nested while
Loop
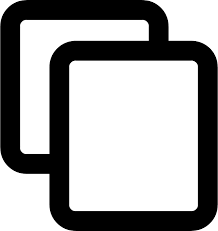
#include <stdio.h>
int main() {
int i = 1;
// Using nested while loops to print a pattern
while (i <= 5) {
int j = 1;
while (j <= 5) {
printf("* ");
j++;
}
printf("\\n");
i++;
}
return 0;
}
Output:
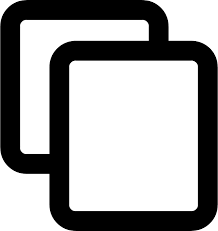
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
Explanation of the Nested while
Loop Example
This example uses two while
loops to print a 5x5 grid of asterisks. The outer while
loop runs 5 times (for values of i
from 1 to 5), and for each iteration of the outer loop, the inner while
loop runs 5 times (for values of j
from 1 to 5), printing an asterisk each time. A new line is printed after each row.
Key Points About Nested Loops
- Multiple Iterations: Nested loops allow you to perform multiple iterations of one loop for each iteration of another loop.
- Time Complexity: The time complexity of nested loops increases exponentially as the number of loops increases. For example, two nested loops that each run
n
times will have a time complexity of O(n²). - Use Cases: Nested loops are commonly used in problems such as matrix manipulations, printing patterns, and handling multi-dimensional arrays.
Example of Nested do-while
Loop
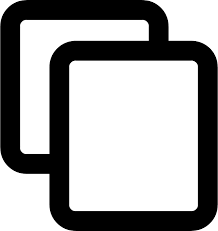
#include <stdio.h>
int main() {
int i = 1;
// Using nested do-while loops to print numbers in a pattern
do {
int j = 1;
do {
printf("%d ", j);
j++;
} while (j <= 5);
printf("\\n");
i++;
} while (i <= 5);
return 0;
}
Output:
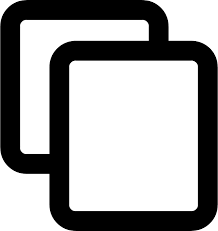
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
Explanation of the Nested do-while
Loop Example
This example uses two do-while
loops to print numbers in a pattern. The outer loop ensures that the inner loop runs 5 times, printing numbers from 1 to 5 in each iteration.
Best Practices for Using Nested Loops
- Limit the Depth: Avoid using more than 3-4 levels of nested loops as it can lead to complex and inefficient code.
- Optimize Conditions: Try to optimize the conditions inside nested loops to reduce unnecessary iterations and improve performance.
- Use Break and Continue: In some cases, you can use the
break
andcontinue
statements to exit or skip iterations of the inner loop if necessary.
Conclusion
Nested loops are a powerful concept in C programming that allows you to perform complex operations involving multiple sets of iterations. While they are useful in many applications, it's important to be mindful of their complexity and performance implications. By using nested loops effectively, you can solve a wide range of problems, from pattern generation to multi-dimensional array processing.
Break, Continue, and Goto Statements in C
In C programming, the break, continue, and goto statements are used to control the flow of execution within loops, conditional statements, and functions. These control flow statements can help you manage the flow of your program and enhance its functionality.
Break Statement
The break
statement is used to exit or "break" out of a loop or switch statement before it completes all its iterations. When encountered, it immediately terminates the loop or switch statement and transfers control to the next statement after the loop or switch block.
Syntax of Break
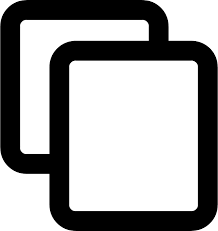
break;
Example of Break in a Loop
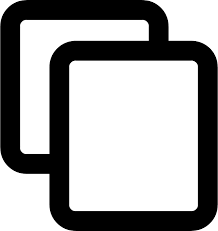
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
printf("%d ", i);
}
return 0;
}
Output:
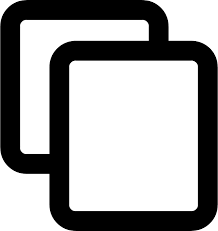
1 2 3 4
In this example, the break
statement stops the loop when i
becomes 5, so the numbers 1 through 4 are printed, and the loop exits.
Continue Statement
The continue
statement is used to skip the current iteration of a loop and proceed to the next iteration. When encountered, it stops the execution of the current iteration and moves to the next iteration of the loop.
Syntax of Continue
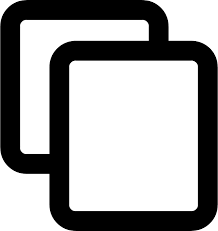
continue;
Example of Continue in a Loop
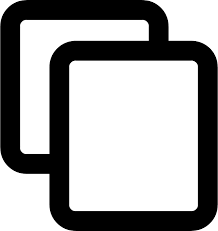
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
continue; // Skip the current iteration when i equals 5
}
printf("%d ", i);
}
return 0;
}
Output:
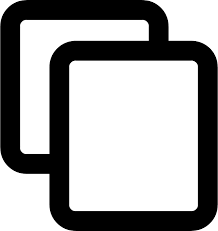
1 2 3 4 6 7 8 9 10
In this example, the continue
statement skips the iteration when i
is 5, so the number 5 is excluded from the output, and the loop continues with the next iteration.
Goto Statement
The goto
statement is used to jump to a specific part of the code, marked by a label. It transfers control to the label, and execution continues from there. While goto
can be useful in certain situations, it is generally discouraged because it can lead to hard-to-read and difficult-to-maintain code (often referred to as "spaghetti code").
Syntax of Goto
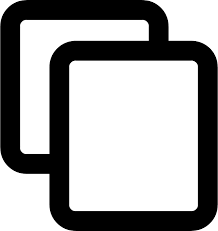
goto label_name;
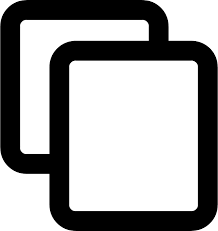
label_name: // Label
// Code to jump to
Example of Goto
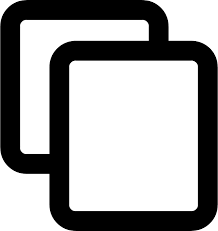
#include <stdio.h>
int main() {
int i = 0;
start: // Label
printf("%d ", i);
i++;
if (i < 5) {
goto start; // Jump back to the start label
}
return 0;
}
Output:
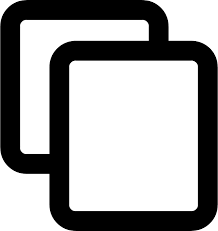
0 1 2 3 4
In this example, the goto
statement causes the program to jump back to the start
label, printing the values of i
from 0 to 4. The loop is repeated until the condition i < 5
is no longer true.
Key Points About Break, Continue, and Goto
- Break: Terminates the loop or switch statement immediately.
- Continue: Skips the current iteration and proceeds to the next iteration of the loop.
- Goto: Transfers control to a specified label in the program, but should be used cautiously due to its potential to reduce code readability and maintainability.
When to Use Break and Continue
- Break: Use
break
when you want to exit a loop or switch early, such as when a specific condition is met. - Continue: Use
continue
when you want to skip the rest of the current iteration and proceed with the next iteration, such as skipping even numbers in a loop.
Best Practices
- Avoid Excessive Use of Goto: Overuse of the
goto
statement can lead to complex and unstructured code. Try to use structured control flow like loops and conditionals instead. - Improve Readability: Always ensure that the use of
break
andcontinue
improves the readability of your code and does not make it unnecessarily complex.
Conclusion
Break, continue, and goto are powerful control flow statements in C that allow you to manipulate the flow of your program. While break
and continue
are typically used in loops, goto
can be used to jump to specific locations in the code, though it should be used sparingly. Understanding how to use these statements effectively will make you more efficient at managing flow control in your programs.
Introduction to Functions in C
In C programming, a function is a block of code that performs a specific task. Functions allow you to break down your program into smaller, manageable pieces of code, making it easier to understand, maintain, and reuse. Functions are essential for modular programming, as they allow you to organize your code into logical chunks.
What is a Function?
A function in C is a group of statements that are executed when the function is called. It can take input in the form of parameters and can return a result to the caller. Functions help to avoid repetition of code, making programs more efficient and easier to maintain.
Function Syntax
The basic syntax of a function in C consists of the following parts:
- Return Type: Specifies the type of value the function will return (e.g.,
int
,float
, etc.). If the function does not return a value, the return type isvoid
. - Function Name: The name of the function that you will use to call it.
- Parameters (Optional): A list of input values that the function can accept. These are enclosed in parentheses.
- Function Body: A block of code enclosed in curly braces (
{ }
) that defines the function's behavior.
Syntax Example
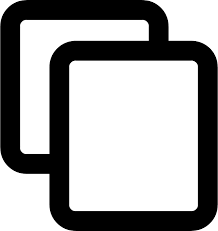
return_type function_name(parameters) {
// Function body
}
Example of a Simple Function
Here’s a simple example of a function in C that adds two integers:
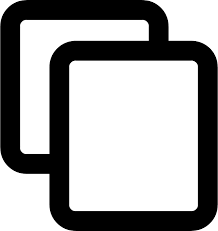
#include <stdio.h>
int add(int a, int b) {
return a + b; // Return the sum of a and b
}
int main() {
int result = add(3, 4); // Call the add function
printf("Sum: %d\n", result); // Output the result
return 0;
}
Output:
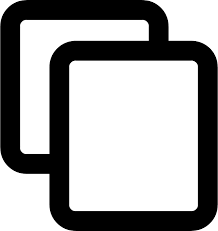
Sum: 7
In this example, the add
function takes two integer parameters, adds them together, and returns the result. The main
function calls the add
function with the arguments 3 and 4, and prints the result.
Types of Functions
In C, functions can be broadly classified into two types:
- Library Functions: These are predefined functions provided by the C standard library, such as
printf()
,scanf()
,strlen()
, etc. - User-defined Functions: These are functions that you define in your program to perform specific tasks. They allow you to customize the behavior of your program and reuse code.
Function Declaration, Definition, and Calling
There are three key steps when working with functions in C:
- Function Declaration: The declaration tells the compiler about the function's name, return type, and parameters before it is used. It can be placed before
main()
or in a header file. - Function Definition: This is where you write the actual code of the function. It provides the logic that is executed when the function is called.
- Function Calling: To call a function, you use its name followed by parentheses containing any arguments (if any).
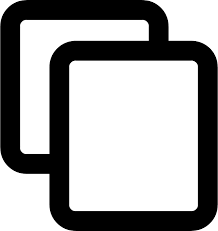
int add(int, int);
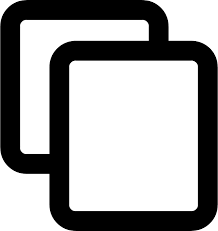
int add(int a, int b) {
return a + b;
}
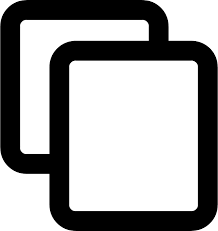
int result = add(3, 4);
Return Statement
The return
statement in a function is used to return a value to the caller. The return type of the function must match the type of value being returned. If the function does not return any value, the return type is void
and the return
statement is optional.
Example of Return Statement
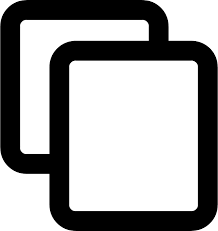
int multiply(int a, int b) {
return a * b; // Returning the product of a and b
}
Function with No Return Value
If a function does not need to return a value, you can define it with the void
return type. The void
return type indicates that the function performs a task but does not return any result to the caller.
Example of Void Function
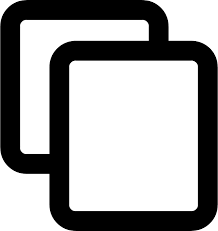
void displayMessage() {
printf("Hello, C Programming!\n");
}
In this case, the displayMessage
function does not return any value but simply prints a message when called.
Function Arguments
Functions can accept one or more arguments (also known as parameters). These arguments are passed to the function when it is called, and they can be used within the function body.
Passing Arguments by Value
In C, function arguments are passed by value by default. This means that a copy of the argument is passed to the function, and any changes made to the parameter inside the function do not affect the original argument in the calling function.
Recursion
Recursion is a technique in which a function calls itself. It can be useful for solving problems that can be broken down into smaller subproblems, such as factorials, Fibonacci series, etc.
Example of Recursion
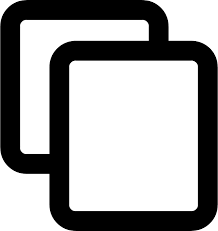
int factorial(int n) {
if (n == 0) {
return 1; // Base case
}
return n * factorial(n - 1); // Recursive call
}
Advantages of Using Functions
- Modularity: Functions allow you to break down complex problems into smaller, easier-to-manage tasks.
- Code Reusability: Functions can be reused multiple times in different parts of the program.
- Improved Maintainability: Functions make it easier to maintain and update your code since changes can be made in one place.
Conclusion
Functions in C are essential for structuring programs, making code more efficient and manageable. Understanding how to define, declare, and call functions is a fundamental skill in C programming. Functions not only promote code reusability but also allow you to organize your code into logical chunks, making it easier to debug and maintain.
Defining and Calling Functions in C
Functions are one of the most important features in C programming. They allow you to group code into reusable, modular blocks that can be easily called and executed from different parts of your program. Understanding how to define and call functions is essential for structuring and organizing your C programs.
Defining a Function
A function is defined by declaring its return type, name, and parameters (if any). The body of the function contains the code that is executed when the function is called.
The syntax to define a function is:
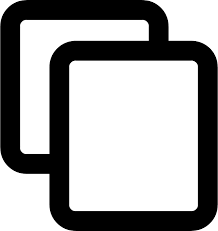
return_type function_name(parameters) {
// Function body
// Code to be executed
}
Example of Function Definition
Here’s an example of defining a function that adds two integers:
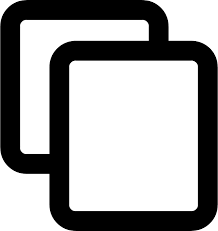
#include <stdio.h>
int add(int a, int b) {
return a + b; // Return the sum of a and b
}
Calling a Function
Once a function is defined, you can call it from another function, typically main()
. Calling a function means invoking the function's name and passing the required arguments (if any). The function executes its defined behavior and returns a result (if applicable).
The syntax to call a function is:
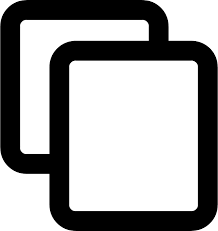
function_name(arguments);
Example of Function Call
Here’s an example of calling the add
function in the main
function:
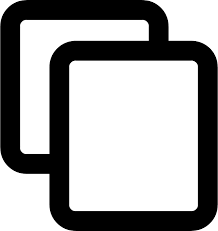
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(3, 4); // Call the add function
printf("Sum: %d\n", result); // Output the result
return 0;
}
Output:
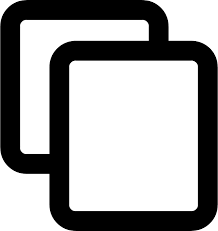
Sum: 7
In this example, the main
function calls the add
function with the arguments 3 and 4, and the result is printed to the screen.
Function with No Return Value (Void Function)
If a function does not need to return a value, it can be defined with the void
return type. A void
function simply performs a task without returning any value to the caller.
Here’s an example of a void
function that prints a message:
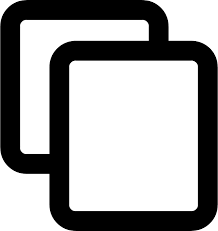
#include <stdio.h>
void displayMessage() {
printf("Hello, C programming!\n");
}
int main() {
displayMessage(); // Call the displayMessage function
return 0;
}
Output:
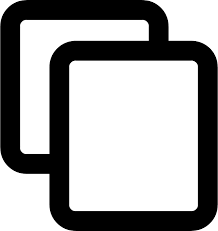
Hello, C programming!
Function Parameters and Arguments
Functions can accept parameters, which are values passed to the function when it is called. These parameters are used within the function to perform its task. The function parameters are specified in the function definition, and the values passed to the function are called arguments.
Example of defining a function with parameters:
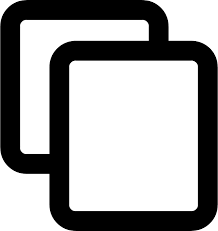
#include <stdio.h>
int multiply(int a, int b) {
return a * b;
}
int main() {
int result = multiply(2, 5); // Pass arguments 2 and 5
printf("Product: %d\n", result); // Output the result
return 0;
}
Output:
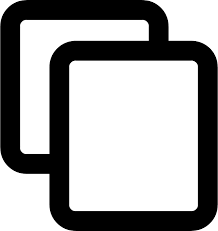
Product: 10
Function with Multiple Parameters
Functions can also accept multiple parameters. You can specify as many parameters as needed, separated by commas.
Example of a function that takes multiple parameters:
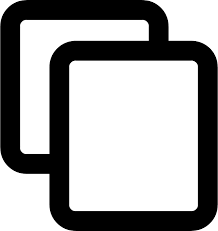
#include <stdio.h>
void printDetails(char name[], int age) {
printf("Name: %s\n", name);
printf("Age: %d\n", age);
}
int main() {
printDetails("Alice", 25); // Pass two arguments
return 0;
}
Output:
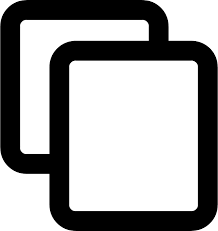
Name: Alice
Age: 25
Function Return Values
Functions can return values to the calling function using the return
statement. The return type of the function must match the type of value returned. If a function does not return any value, its return type should be void
.
Example of returning a value from a function:
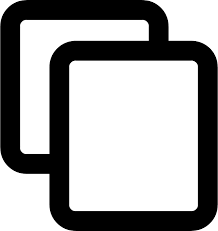
#include <stdio.h>
int subtract(int a, int b) {
return a - b; // Return the difference of a and b
}
int main() {
int result = subtract(10, 5); // Call the subtract function
printf("Difference: %d\n", result); // Output the result
return 0;
}
Output:
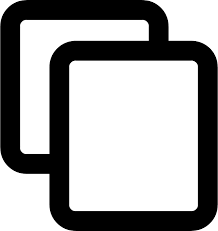
Difference: 5
Function Prototypes
Before using a function in the main()
function or elsewhere, it is a good practice to declare its prototype. This tells the compiler about the function's return type and parameters.
The syntax of a function prototype is:
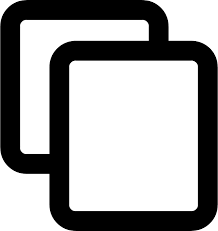
return_type function_name(parameters);
Example of a function prototype:
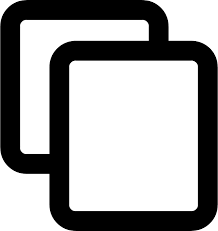
#include <stdio.h>
int add(int a, int b); // Function prototype
int main() {
int result = add(3, 4); // Call the add function
printf("Sum: %d\n", result);
return 0;
}
int add(int a, int b) {
return a + b;
}
Conclusion
Defining and calling functions in C is essential for creating structured, modular, and maintainable programs. Functions provide a way to break down complex problems into smaller, manageable tasks. By defining functions and calling them from main()
or other functions, you can easily reuse code and improve the organization of your C programs.
Function Parameters and Return Types
In C programming, functions can accept parameters and return values to the caller. These parameters are used to pass data into the function, while the return type specifies what type of value the function will return (if any). Understanding function parameters and return types is crucial for writing clear and efficient code in C.
Function Parameters
Function parameters are variables that are passed to a function when it is called. These parameters are used within the function to perform operations. Parameters allow functions to be more flexible by working with different values passed during the function call.
The syntax to define function parameters is as follows:
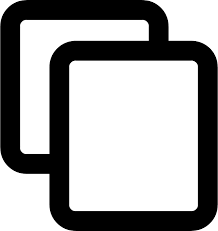
return_type function_name(parameter1, parameter2, ...) {
// Function body
}
Example of Function with Parameters
In this example, the function multiply
takes two integer parameters a
and b
and returns their product:
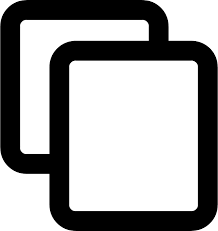
#include <stdio.h>
int multiply(int a, int b) {
return a * b; // Return the product of a and b
}
int main() {
int result = multiply(3, 4); // Call the multiply function with arguments 3 and 4
printf("Product: %d\n", result); // Output the result
return 0;
}
Output:
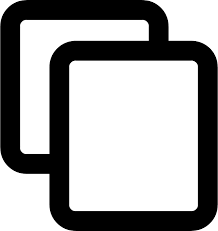
Product: 12
Function Return Types
The return type of a function specifies the type of value the function will return. If a function does not return any value, its return type should be void
. If the function returns a value, the return type must match the data type of that value (e.g., int
, float
, char
, etc.).
The syntax for returning a value from a function is:
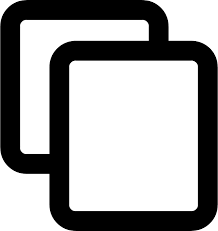
return return_value;
Example of Function with Return Value
In this example, the function add
takes two integer parameters and returns their sum:
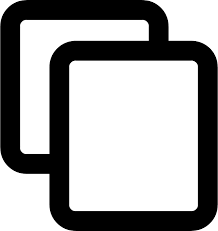
#include <stdio.h>
int add(int a, int b) {
return a + b; // Return the sum of a and b
}
int main() {
int result = add(5, 7); // Call the add function with arguments 5 and 7
printf("Sum: %d\n", result); // Output the result
return 0;
}
Output:
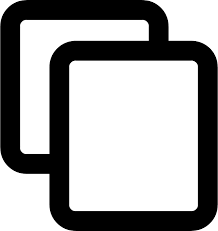
Sum: 12
Function with No Return Value (Void Functions)
If a function does not need to return a value, it is declared with the return type void
. A void
function performs a task but does not return any result to the caller.
Example of a void
function:
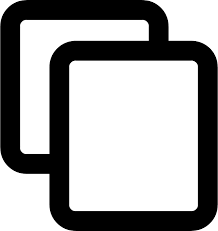
#include <stdio.h>
void printMessage() {
printf("Hello, C Programming!\n"); // Print a message
}
int main() {
printMessage(); // Call the printMessage function
return 0;
}
Output:
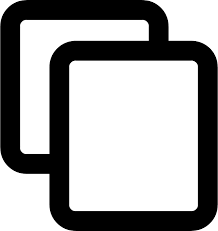
Hello, C Programming!
Multiple Parameters in Functions
Functions can accept multiple parameters, allowing you to pass more than one value to the function. These parameters are separated by commas within the function definition.
Example of a function with multiple parameters:
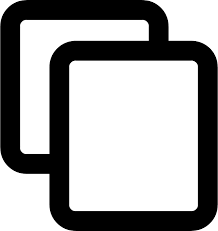
#include <stdio.h>
void printDetails(char name[], int age) {
printf("Name: %s\n", name);
printf("Age: %d\n", age);
}
int main() {
printDetails("Alice", 25); // Pass two arguments to the function
return 0;
}
Output:
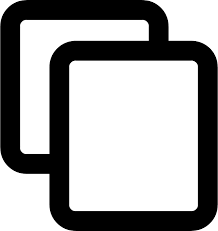
Name: Alice
Age: 25
Return Type and Function Overloading
In C, function overloading is not supported. Function overloading is a feature in some languages like C++ where multiple functions can have the same name but different parameter types. In C, the function name must be unique, and the return type does not affect the function signature.
Passing Arguments by Value vs. Reference
In C, function arguments are passed by value by default. This means that a copy of the argument is passed to the function. If you want to modify the original value, you need to pass the argument by reference using pointers.
Example of Passing Arguments by Value:
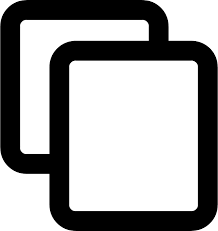
#include <stdio.h>
void modifyValue(int x) {
x = 10; // Modify the local copy of x
}
int main() {
int num = 5;
modifyValue(num); // Pass num by value
printf("Value of num: %d\n", num); // num remains unchanged
return 0;
}
Output:
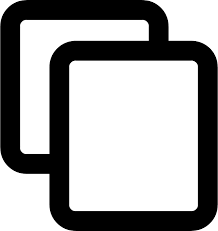
Value of num: 5
Example of Passing Arguments by Reference:
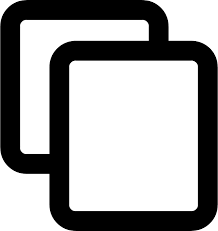
#include <stdio.h>
void modifyValue(int *x) {
*x = 10; // Modify the original value of x
}
int main() {
int num = 5;
modifyValue(&num); // Pass num by reference
printf("Value of num: %d\n", num); // num is changed
return 0;
}
Output:
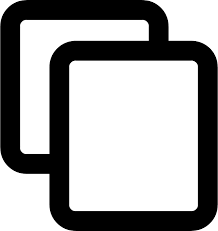
Value of num: 10
Conclusion
Understanding function parameters and return types is a key concept in C programming. Parameters allow functions to operate on different values, and return types determine what value (if any) a function will provide back to the caller. By mastering these concepts, you can create more modular, efficient, and reusable code in your C programs.
Recursion in C
Recursion is a programming technique where a function calls itself in order to solve a problem. It allows problems to be broken down into smaller sub-problems, making it easier to design and implement solutions. In C, a function that calls itself is known as a recursive function.
What is Recursion?
Recursion is a process in which a function calls itself directly or indirectly in order to solve a problem. A recursive function typically has two main parts:
- Base Case: The condition under which the function stops calling itself to prevent an infinite loop.
- Recursive Case: The part where the function calls itself with a modified argument, gradually reducing the problem size.
Example of Recursion: Factorial Function
The factorial of a number n
, denoted as n!
, is defined as:
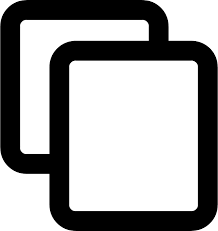
n! = n * (n-1) * (n-2) * ... * 1
Factorial can be calculated recursively as follows:
- Base Case:
0! = 1
(Factorial of 0 is 1). - Recursive Case:
n! = n * (n-1)!
(Factorial of n is n multiplied by the factorial of (n-1)).
Here’s an example of calculating the factorial of a number using recursion in C:
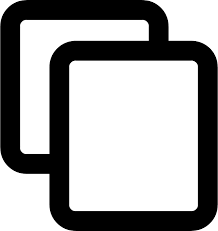
#include <stdio.h>
int factorial(int n) {
if (n == 0) // Base case
return 1;
else // Recursive case
return n * factorial(n - 1);
}
int main() {
int num = 5;
printf("Factorial of %d is %d\n", num, factorial(num)); // Call the recursive function
return 0;
}
Output:
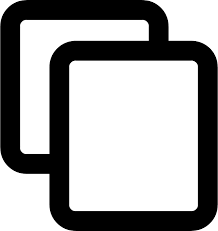
Factorial of 5 is 120
How Recursion Works
When a recursive function is called, the function executes and calls itself with a new argument. This continues until the base case is met, at which point the function stops calling itself and returns a value back to the previous function call. The function then "unwinds" by returning the result back through the chain of function calls.
Recursive Call Stack
Recursion uses the call stack to keep track of each function call. Each time a function calls itself, a new stack frame is pushed onto the call stack. When the base case is reached, the stack begins to unwind as each function call returns its result to the previous one.
Advantages of Recursion
- Simplicity: Recursion can simplify the code for problems that have a natural recursive structure (e.g., tree traversal, factorial calculation, Fibonacci sequence).
- Clarity: Recursive solutions are often easier to understand and implement for problems that inherently require breaking down into smaller sub-problems.
Disadvantages of Recursion
- Memory Usage: Recursive functions require more memory because each function call is added to the call stack. This can lead to stack overflow if the recursion depth is too large.
- Performance: Recursive functions can be less efficient than iterative solutions, especially for problems with overlapping sub-problems.
Example of Recursion: Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. The sequence starts as: 0, 1, 1, 2, 3, 5, 8, 13, and so on. The Fibonacci sequence can be calculated recursively as:
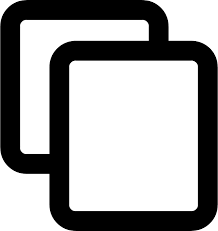
fib(n) = fib(n-1) + fib(n-2)
Here is an example implementation of a recursive Fibonacci function in C:
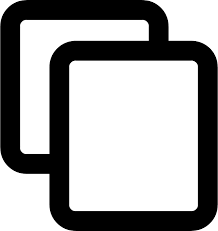
#include <stdio.h>
int fibonacci(int n) {
if (n <= 1) // Base case
return n;
else // Recursive case
return fibonacci(n - 1) + fibonacci(n - 2);
}
int main() {
int num = 6;
printf("Fibonacci number at position %d is %d\n", num, fibonacci(num)); // Call the recursive function
return 0;
}
Output:
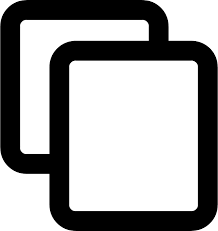
Fibonacci number at position 6 is 8
Recursion vs. Iteration
In many cases, recursion can be replaced with iteration (using loops). While recursion is conceptually simpler for certain problems, iteration is often more efficient in terms of memory usage and execution speed. However, recursion is preferred when the problem has a natural recursive structure, such as in tree and graph traversal.
Conclusion
Recursion is a powerful technique in C programming that allows you to solve problems by breaking them down into smaller sub-problems. While recursion makes code simpler and more elegant, it should be used carefully, as it can lead to excessive memory usage and stack overflow if not properly managed. Understanding recursion is essential for solving many complex problems efficiently.
Storage Classes in Functions (auto, static, register, extern)
In C, storage classes define the lifetime, visibility, and scope of variables. They determine how and where the variable is stored in memory and how long it exists during the execution of a program. C provides four primary storage classes: auto
, static
, register
, and extern
.
1. auto
Storage Class
The auto
storage class is the default storage class for local variables. It is used to declare variables within a function or block. These variables are automatically created when the function is called and destroyed when the function exits. They are stored in the stack memory and do not retain their values between function calls.
- Scope: Local to the function or block where they are defined.
- Lifetime: Exists only during the function call or block execution.
- Default: Local variables are
auto
by default, so explicitly declaring them asauto
is not necessary.
2. static
Storage Class
The static
storage class is used to declare variables that retain their values across function calls. A static variable is initialized only once, and its value is preserved between function calls. It is stored in the data segment of memory rather than the stack. A static variable has a local scope, but it retains its value for the entire duration of the program's execution.
- Scope: Local to the function or file, but retains its value across function calls.
- Lifetime: Exists for the lifetime of the program.
- Example:
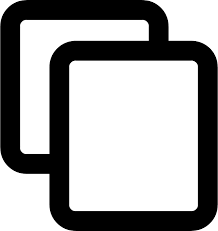
#include <stdio.h>
void counter() {
static int count = 0; // Static variable
count++;
printf("Count: %d\n", count);
}
int main() {
counter(); // Output: Count: 1
counter(); // Output: Count: 2
counter(); // Output: Count: 3
return 0;
}
In the example above, the static variable count
retains its value across multiple calls to the counter()
function.
3. register
Storage Class
The register
storage class is used to declare variables that should be stored in the CPU registers instead of the regular memory. This can improve performance for frequently accessed variables, as accessing registers is faster than accessing memory. However, the compiler may ignore the register
keyword if there are insufficient registers available.
- Scope: Local to the function or block where they are defined.
- Lifetime: Exists only during the function call or block execution.
- Note:
register
variables cannot be passed by reference (i.e., no & operator).
Example:
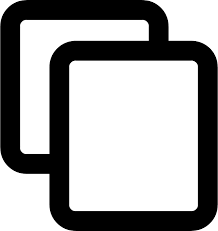
#include <stdio.h>
void sum() {
register int i, sum = 0;
for (i = 1; i <= 5; i++) {
sum += i;
}
printf("Sum: %d\n", sum);
}
int main() {
sum(); // Output: Sum: 15
return 0;
}
In the example, the variable i
and sum
are declared as register
, suggesting that they may be stored in the CPU registers for faster access.
4. extern
Storage Class
The extern
storage class is used to declare variables that are defined in another file or outside the current function. It tells the compiler that the variable exists, but its memory allocation is handled elsewhere. The extern
keyword is commonly used in multi-file programs to reference global variables or functions defined in other files.
- Scope: Global in scope, accessible across multiple files.
- Lifetime: Exists for the lifetime of the program.
- Example:
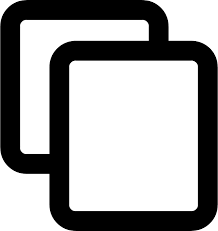
#include <stdio.h>
extern int count; // Declare external variable
void display() {
printf("Count: %d\n", count);
}
int main() {
count = 10; // Define the external variable
display(); // Output: Count: 10
return 0;
}
In the example above, the variable count
is declared as extern
, which means it is defined in another file. In this case, the definition is assumed to be present outside the current function.
Comparison of Storage Classes
Storage Class | Scope | Lifetime | Default Value |
---|---|---|---|
auto |
Local to function/block | Until the function/block exits | Uninitialized (garbage value) |
static |
Local to function/block, but retains value | Throughout the program's execution | Zero (if not explicitly initialized) |
register |
Local to function/block | Until the function/block exits | Uninitialized (garbage value) |
extern |
Global (across multiple files) | Throughout the program's execution | Uninitialized (garbage value) |
Conclusion
Storage classes in C are used to manage the scope, lifetime, and visibility of variables. Understanding these storage classes—auto
, static
, register
, and extern
—helps optimize memory usage and program structure. Choosing the appropriate storage class for a variable depends on the requirements of the program and how long and where the variable needs to persist.
Introduction to Arrays
An array is a collection of variables of the same type that are stored in contiguous memory locations. It is used to store multiple values in a single variable, making it easier to manage large amounts of data. In C, arrays are useful when we need to store a fixed-size sequential collection of elements of the same type.
What is an Array?
An array in C is a data structure that can hold multiple values of the same type under a single name. Each value in the array is called an element, and each element is accessed using an index. The first element of the array is at index 0, the second element is at index 1, and so on.
Declaring and Initializing Arrays
In C, arrays can be declared by specifying the type of the elements and the number of elements (size) in the array. The size is a constant integer value that determines the number of elements the array can hold.
- Declaration:
data_type array_name[array_size];
- Initialization: Arrays can be initialized at the time of declaration by providing values inside curly braces.
Example:
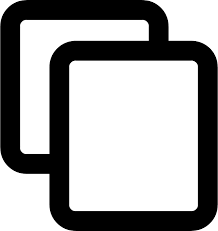
#include <stdio.h>
int main() {
int numbers[5] = {1, 2, 3, 4, 5}; // Array of 5 integers
printf("First element: %d\n", numbers[0]); // Output: First element: 1
printf("Second element: %d\n", numbers[1]); // Output: Second element: 2
return 0;
}
Types of Arrays
There are two main types of arrays in C:
- One-dimensional Arrays: These are simple arrays where each element is accessed using a single index.
- Multi-dimensional Arrays: These arrays contain arrays within arrays, allowing for more complex data storage such as matrices.
One-dimensional Array Example
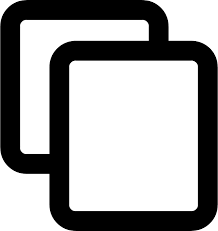
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i, arr[i]);
}
return 0;
}
Multi-dimensional Array Example
Multi-dimensional arrays are arrays of arrays. They can be declared by specifying multiple sizes in the declaration.
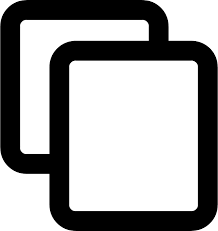
#include <stdio.h>
int main() {
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
printf("Element [%d][%d]: %d\n", i, j, matrix[i][j]);
}
}
return 0;
}
Accessing Array Elements
Array elements are accessed using the array name followed by the index inside square brackets. The index starts from 0 for the first element and goes up to size - 1
for the last element.
- Syntax:
array_name[index]
Array Indexing
In C, array indices start at 0. This means that for an array of size n
, the valid indices range from 0 to n-1
.
Example:
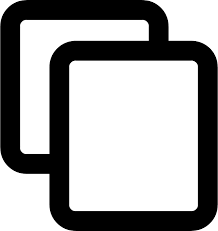
#include <stdio.h>
int main() {
int arr[3] = {100, 200, 300};
printf("First element: %d\n", arr[0]); // Output: 100
printf("Last element: %d\n", arr[2]); // Output: 300
return 0;
}
Common Array Operations
- Traversal: Accessing each element of the array using loops (e.g., for, while).
- Insertion: Adding an element to an array at a specific position.
- Deletion: Removing an element from an array.
- Search: Searching for an element in the array.
- Sorting: Arranging the elements of the array in a specific order (ascending/descending).
Conclusion
Arrays are a powerful and essential feature in C programming. They allow for efficient data storage and management of multiple values. Whether you're dealing with simple lists or more complex multi-dimensional structures, understanding arrays is fundamental to working with C effectively.
One-dimensional Arrays
A one-dimensional array is a linear collection of variables of the same type that are stored in contiguous memory locations. It is the simplest form of an array and can be thought of as a list of elements, all of which are of the same data type. One-dimensional arrays are commonly used to store a collection of data, such as numbers, strings, or characters, that can be accessed using a single index.
Declaring and Initializing One-dimensional Arrays
In C, you can declare a one-dimensional array by specifying the type of the elements and the size of the array. The size is the number of elements the array can hold.
- Declaration:
data_type array_name[array_size];
- Initialization: Arrays can be initialized at the time of declaration using curly braces.
Example:
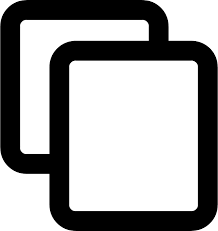
#include <stdio.h>
int main() {
int numbers[5] = {1, 2, 3, 4, 5}; // Array of 5 integers
printf("First element: %d\n", numbers[0]); // Output: First element: 1
printf("Second element: %d\n", numbers[1]); // Output: Second element: 2
return 0;
}
Accessing Elements of a One-dimensional Array
Elements of a one-dimensional array are accessed using an index, starting from 0. The first element is at index 0, the second element is at index 1, and so on. You can access any element by specifying its index inside square brackets.
Example:
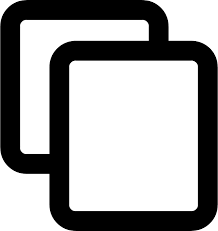
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
printf("Element at index 0: %d\n", arr[0]); // Output: 10
printf("Element at index 1: %d\n", arr[1]); // Output: 20
printf("Element at index 4: %d\n", arr[4]); // Output: 50
return 0;
}
Traversal of One-dimensional Arrays
Traversal refers to accessing all elements of an array one by one. You can use a loop (such as a for
loop) to traverse the array and print each element.
Example:
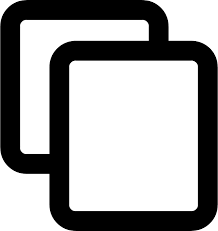
#include <stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
// Traversing the array
for (int i = 0; i < 5; i++) {
printf("Element at index %d: %d\n", i, arr[i]);
}
return 0;
}
Modifying Elements of a One-dimensional Array
You can modify the value of an element in a one-dimensional array by assigning a new value to a specific index.
Example:
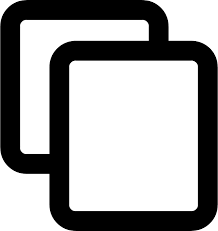
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
// Modifying the third element (index 2)
arr[2] = 100;
printf("Modified array: \n");
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]); // Output: 10 20 100 40 50
}
return 0;
}
Memory Representation of One-dimensional Arrays
In memory, the elements of a one-dimensional array are stored sequentially in contiguous memory locations. The array name refers to the address of the first element, and each element can be accessed using the index along with the base address.
Example of Memory Representation
Consider the following array:
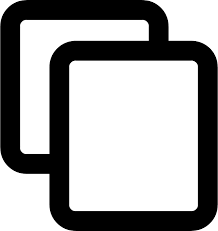
int arr[3] = {10, 20, 30};
The memory representation would look like this:
arr[0]
is stored at the base address of the array,arr[1]
is stored immediately afterarr[0]
, andarr[2]
is stored immediately afterarr[1]
.
Conclusion
One-dimensional arrays are fundamental data structures in C that allow you to store multiple values of the same type in a single variable. They are useful for managing lists or sequences of data, and they can be easily traversed, modified, and accessed using indices. Understanding one-dimensional arrays is crucial for mastering C programming and working with more complex data structures.
Two-dimensional Arrays
A two-dimensional array is an array of arrays. It can be visualized as a table or matrix with rows and columns, where each element is accessed using two indices: one for the row and one for the column. Two-dimensional arrays are useful for storing data in tabular form, such as matrices, grids, or tables.
Declaring and Initializing Two-dimensional Arrays
In C, a two-dimensional array can be declared by specifying the type of elements, followed by the number of rows and columns. The general syntax is:
- Declaration:
data_type array_name[row_size][column_size];
- Initialization: You can initialize a two-dimensional array at the time of declaration using nested curly braces.
Example:
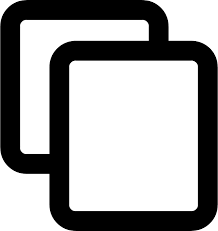
#include <stdio.h>
int main() {
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}}; // 2 rows and 3 columns
printf("Element at [0][0]: %d\n", matrix[0][0]); // Output: 1
printf("Element at [1][2]: %d\n", matrix[1][2]); // Output: 6
return 0;
}
Accessing Elements of a Two-dimensional Array
Elements of a two-dimensional array are accessed using two indices: the first index specifies the row, and the second specifies the column. The indices start from 0.
Example:
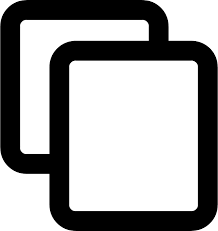
#include <stdio.h>
int main() {
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
// Accessing elements
printf("Element at [0][1]: %d\n", matrix[0][1]); // Output: 2
printf("Element at [1][0]: %d\n", matrix[1][0]); // Output: 4
return 0;
}
Traversing a Two-dimensional Array
Traversal refers to accessing all elements of a two-dimensional array. You can use nested loops (a loop for rows and another for columns) to traverse through all the elements.
Example:
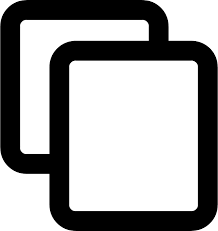
#include <stdio.h>
int main() {
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
// Traversing the array
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
printf("Element at [%d][%d]: %d\n", i, j, matrix[i][j]);
}
}
return 0;
}
Modifying Elements of a Two-dimensional Array
You can modify elements in a two-dimensional array by using the row and column indices. This allows you to change the value of any element in the matrix.
Example:
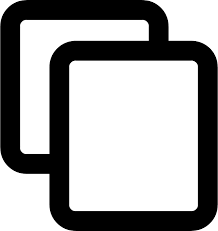
#include <stdio.h>
int main() {
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
// Modifying an element
matrix[0][1] = 10;
printf("Modified matrix:\n");
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
printf("%d ", matrix[i][j]); // Output: 1 10 3 4 5 6
}
printf("\n");
}
return 0;
}
Memory Representation of Two-dimensional Arrays
In memory, two-dimensional arrays are stored in row-major order. This means that the elements of the first row are stored first, followed by the elements of the second row, and so on. The memory is contiguous, but the array is conceptually a "table" with rows and columns.
Example:
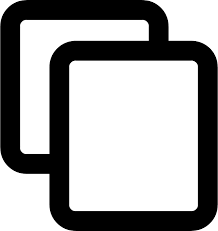
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
In memory, this array would look like:
- Address of matrix[0][0] (1), matrix[0][1] (2), matrix[0][2] (3), matrix[1][0] (4), matrix[1][1] (5), matrix[1][2] (6).
Dynamic Memory Allocation for Two-dimensional Arrays
In C, you can also dynamically allocate memory for two-dimensional arrays using malloc
or calloc
. This is useful when the size of the array is not known at compile time.
Example:
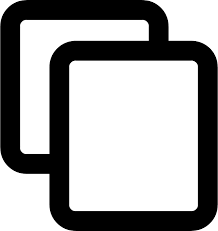
#include <stdio.h>
#include <stdlib.h>
int main() {
int rows = 2, cols = 3;
int **matrix = (int **)malloc(rows * sizeof(int *));
for (int i = 0; i < rows; i++) {
matrix[i] = (int *)malloc(cols * sizeof(int));
}
// Initializing the matrix
matrix[0][0] = 1; matrix[0][1] = 2; matrix[0][2] = 3;
matrix[1][0] = 4; matrix[1][1] = 5; matrix[1][2] = 6;
// Printing the matrix
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
// Freeing the memory
for (int i = 0; i < rows; i++) {
free(matrix[i]);
}
free(matrix);
return 0;
}
Conclusion
Two-dimensional arrays are an important concept in C programming, allowing you to store data in a grid-like format. They are useful for a variety of applications such as matrix operations, image processing, and more. Understanding how to declare, initialize, access, and modify two-dimensional arrays is essential for working with complex data structures in C.
Strings in C
In C programming, a string is an array of characters terminated by a null character ('\0'). Strings are used to store and manipulate text data. Unlike other data types, strings are not a built-in primitive data type in C, but are implemented as arrays of characters.
Declaring and Initializing Strings
In C, strings are declared as arrays of characters. You can initialize a string either by specifying the characters explicitly or by using string literals.
- Declaration:
char string_name[size];
- Initialization: You can initialize a string by providing a string literal:
Example:
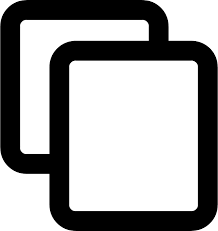
#include <stdio.h>
int main() {
char str[6] = "Hello"; // 6th element is for the null terminator \0
printf("String: %s\n", str); // Output: Hello
return 0;
}
Accessing and Modifying String Elements
Each character of a string can be accessed using an index. The first character is at index 0, the second character at index 1, and so on. You can also modify individual characters of a string.
Example:
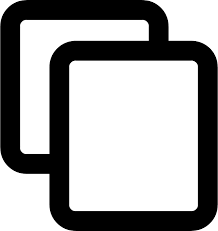
#include <stdio.h>
int main() {
char str[] = "Hello";
// Accessing and modifying string elements
printf("First character: %c\n", str[0]); // Output: H
str[0] = 'h'; // Modify the first character
printf("Modified string: %s\n", str); // Output: hello
return 0;
}
String Handling Functions
C provides a set of standard library functions to manipulate strings, which are defined in the string.h
library. Common functions include:
- strlen(str): Returns the length of the string excluding the null terminator.
- strcmp(str1, str2): Compares two strings lexicographically and returns 0 if they are equal.
- strcpy(dest, src): Copies the string from the source to the destination.
- strcat(dest, src): Concatenates the source string to the destination string.
- strchr(str, c): Returns a pointer to the first occurrence of the character in the string.
Example:
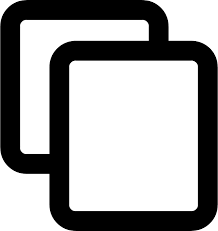
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello";
char str2[] = " World";
// Concatenate strings
strcat(str1, str2);
printf("Concatenated string: %s\n", str1); // Output: Hello World
// Compare strings
int result = strcmp(str1, "Hello World");
if(result == 0) {
printf("Strings are equal.\n");
} else {
printf("Strings are not equal.\n");
}
return 0;
}
String Input and Output
Strings can be read from the user using the scanf
function or the gets
function (though gets
is not recommended due to security concerns). Strings are displayed using the printf
function.
Example:
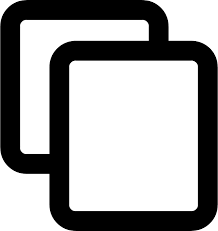
#include <stdio.h>
int main() {
char name[30];
// Getting input using scanf
printf("Enter your name: ");
scanf("%s", name); // Warning: scanf stops at the first space
printf("Hello, %s!\n", name); // Output: Hello, (name entered)
return 0;
}
Multidimensional Arrays and Strings
Strings can also be represented as multidimensional arrays. A two-dimensional array of characters is used to store multiple strings. Each string is stored in a separate row of the array.
Example:
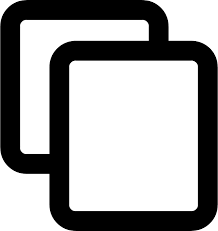
#include <stdio.h>
int main() {
char names[3][20] = {"Alice", "Bob", "Charlie"};
// Accessing individual strings
printf("First name: %s\n", names[0]); // Output: Alice
printf("Second name: %s\n", names[1]); // Output: Bob
printf("Third name: %s\n", names[2]); // Output: Charlie
return 0;
}
String Constants
String constants in C are sequences of characters enclosed in double quotes (""). Each character in the string constant is stored as an individual character in memory, followed by a null terminator ('\0').
Example:
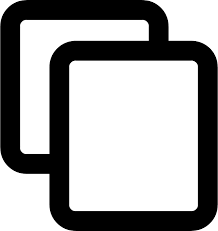
#include <stdio.h>
int main() {
printf("This is a string constant.\n"); // Output: This is a string constant.
return 0;
}
Conclusion
Strings are an essential part of C programming, allowing you to store and manipulate text. With the help of string manipulation functions, you can perform various operations such as comparison, copying, concatenation, and finding the length of strings. Understanding how to work with strings is fundamental for text processing and handling user input in C programs.
String Manipulation Functions (strcpy, strlen, strcmp, etc.)
C provides a set of standard library functions for manipulating strings, which are defined in the string.h
library. These functions allow you to perform operations such as copying, comparing, concatenating, and finding the length of strings. Below are some commonly used string manipulation functions in C.
1. strcpy()
- Copying Strings
The strcpy()
function is used to copy the contents of one string to another. It takes two arguments: the destination string and the source string. It returns a pointer to the destination string.
Syntax:
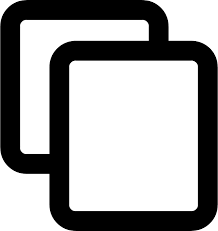
char *strcpy(char *dest, const char *src);
Example:
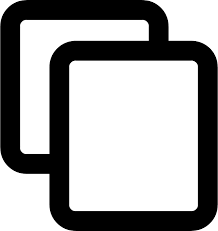
#include <stdio.h>
#include <string.h>
int main() {
char src[] = "Hello, world!";
char dest[50];
// Copying string
strcpy(dest, src);
printf("Source String: %s\n", src); // Output: Hello, world!
printf("Destination String: %s\n", dest); // Output: Hello, world!
return 0;
}
2. strlen()
- Finding the Length of a String
The strlen()
function is used to find the length of a string, excluding the null terminator ('\0'). It returns the number of characters in the string.
Syntax:
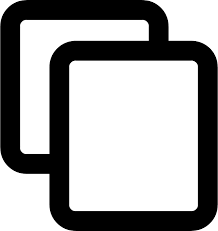
size_t strlen(const char *str);
Example:
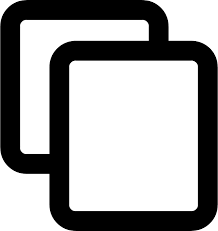
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
// Finding the length of the string
int len = strlen(str);
printf("Length of the string: %d\n", len); // Output: 13
return 0;
}
3. strcmp()
- Comparing Two Strings
The strcmp()
function compares two strings lexicographically. It returns:
- 0 if the strings are equal.
- negative value if the first string is lexicographically less than the second.
- positive value if the first string is lexicographically greater than the second.
Syntax:
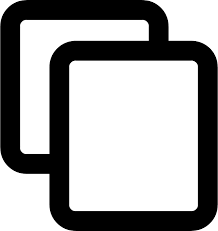
int strcmp(const char *str1, const char *str2);
Example:
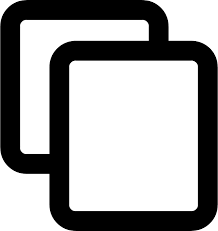
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "apple";
char str2[] = "banana";
// Comparing strings
int result = strcmp(str1, str2);
if(result == 0) {
printf("Strings are equal.\n");
} else if(result < 0) {
printf("str1 is less than str2.\n");
} else {
printf("str1 is greater than str2.\n");
}
return 0;
}
4. strcat()
- Concatenating Strings
The strcat()
function appends the source string to the destination string. The destination string should have enough space to hold the concatenated result, including the null terminator.
Syntax:
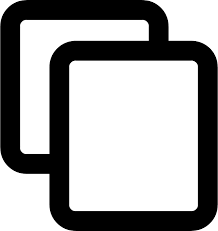
char *strcat(char *dest, const char *src);
Example:
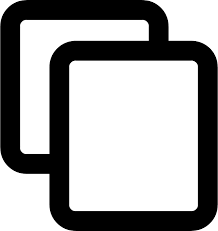
#include <stdio.h>
#include <string.h>
int main() {
char str1[50] = "Hello";
char str2[] = " World!";
// Concatenating strings
strcat(str1, str2);
printf("Concatenated String: %s\n", str1); // Output: Hello World!
return 0;
}
5. strchr()
- Finding a Character in a String
The strchr()
function searches for the first occurrence of a character in a string. It returns a pointer to the first occurrence of the character, or NULL
if the character is not found.
Syntax:
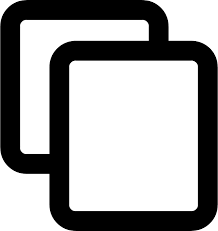
char *strchr(const char *str, int c);
Example:
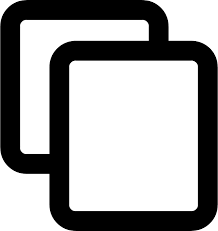
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
// Finding the first occurrence of 'o'
char *ptr = strchr(str, 'o');
if(ptr != NULL) {
printf("Found 'o' at position: %ld\n", ptr - str); // Output: 4
} else {
printf("'o' not found.\n");
}
return 0;
}
6. strstr()
- Finding a Substring in a String
The strstr()
function searches for a substring within a string. It returns a pointer to the first occurrence of the substring, or NULL
if the substring is not found.
Syntax:
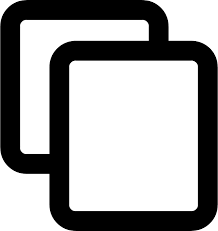
char *strstr(const char *haystack, const char *needle);
Example:
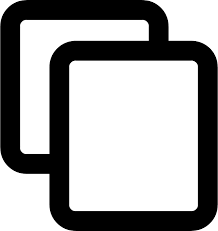
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
// Finding the substring "world"
char *ptr = strstr(str, "world");
if(ptr != NULL) {
printf("Found substring: %s\n", ptr); // Output: world!
} else {
printf("Substring not found.\n");
}
return 0;
}
7. strncat()
- Concatenating a Limited Number of Characters
The strncat()
function is similar to strcat()
, but it only appends the specified number of characters from the source string to the destination string.
Syntax:
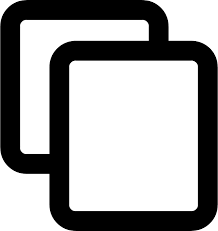
char *strncat(char *dest, const char *src, size_t n);
Example:
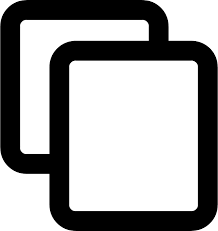
#include <stdio.h>
#include <string.h>
int main() {
char str1[50] = "Hello";
char str2[] = " World!";
// Concatenating first 3 characters of str2 to str1
strncat(str1, str2, 3);
printf("Concatenated String: %s\n", str1); // Output: Hello Wor
return 0;
}
Conclusion
String manipulation functions in C are essential tools for working with text-based data. Functions such as strcpy()
, strlen()
, strcmp()
, and strcat()
allow you to copy, compare, concatenate, and find the length of strings. By mastering these functions, you can efficiently handle string operations in C programming.
Introduction to Pointers
A pointer is a variable that stores the memory address of another variable. Pointers are a powerful feature of C and provide the ability to directly manipulate memory. They allow you to work with dynamic memory allocation, arrays, and functions efficiently. Understanding pointers is crucial for writing efficient and optimized C programs.
What are Pointers?
In C, a pointer is a variable that holds the memory address of another variable. Instead of storing a value directly, a pointer stores the location of the value in memory. This allows you to indirectly access and manipulate the value of the variable using the pointer.
Pointer Syntax
The syntax for declaring a pointer is as follows:
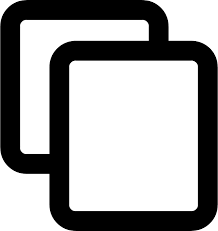
data_type *pointer_name;
Here, data_type
represents the type of data the pointer will point to, and *pointer_name
is the pointer variable.
Example of Declaring Pointers
The following example demonstrates how to declare and initialize a pointer:
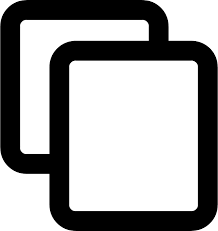
#include <stdio.h>
int main() {
int num = 10;
int *ptr; // Pointer to an integer
ptr = # // Assigning the address of num to the pointer ptr
printf("Value of num: %d\n", num); // Output: 10
printf("Address of num: %p\n", &num); // Output: memory address of num
printf("Value stored at ptr: %d\n", *ptr); // Output: 10 (dereferencing the pointer)
return 0;
}
The & Operator (Address-of Operator)
The &
operator is used to get the memory address of a variable. In the above example, #
is used to get the memory address of the variable num
and assign it to the pointer ptr
.
The * Operator (Dereferencing Operator)
The *
operator is used to access the value at the memory address the pointer is pointing to. This process is called "dereferencing" the pointer. In the example, *ptr
gives us the value stored at the address stored in ptr
.
Pointer Arithmetic
Pointer arithmetic allows you to perform operations on pointers. Since pointers store memory addresses, you can increment or decrement a pointer to point to the next or previous memory location. The size of the increment depends on the type of data the pointer is pointing to.
Example of Pointer Arithmetic:
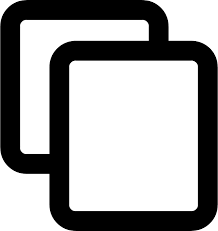
#include <stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int *ptr = arr; // Pointer to the first element of the array
// Accessing array elements using pointer arithmetic
printf("First element: %d\n", *ptr); // Output: 10
ptr++; // Move pointer to the next element
printf("Second element: %d\n", *ptr); // Output: 20
ptr++; // Move pointer to the next element
printf("Third element: %d\n", *ptr); // Output: 30
return 0;
}
Pointer to Pointer
A pointer can also point to another pointer, creating a "pointer to pointer" or a double pointer. This allows you to work with pointers dynamically and manage memory more effectively.
Example of Pointer to Pointer:
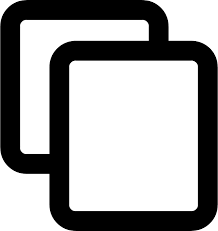
#include <stdio.h>
int main() {
int num = 10;
int *ptr = #
int **ptr2 = &ptr; // Pointer to pointer
printf("Value of num: %d\n", num); // Output: 10
printf("Value of ptr: %p\n", ptr); // Output: memory address of num
printf("Value of ptr2: %p\n", ptr2); // Output: memory address of ptr
printf("Value pointed by ptr2: %d\n", **ptr2); // Output: 10 (dereferencing twice)
return 0;
}
Why Use Pointers?
- Memory Efficiency: Pointers allow efficient memory management by directly accessing and modifying values in memory.
- Dynamic Memory Allocation: Pointers enable the allocation and freeing of memory dynamically using functions like
malloc()
,calloc()
, andfree()
. - Function Arguments: Pointers can be used to pass large structures or arrays efficiently to functions by reference, avoiding the need to copy data.
- Implementing Data Structures: Pointers are essential for implementing linked lists, trees, and other dynamic data structures.
Conclusion
Pointers are a powerful and essential feature of C programming. They allow you to directly manipulate memory, pass arguments efficiently to functions, and work with dynamic data structures. Understanding pointers is crucial to becoming proficient in C programming and writing efficient, optimized code.
Pointer Arithmetic
Pointer arithmetic allows you to perform operations on pointers. Since pointers store memory addresses, you can increment or decrement a pointer to point to the next or previous memory location. Pointer arithmetic is frequently used when working with arrays, as arrays are stored in contiguous memory locations.
What is Pointer Arithmetic?
In C, a pointer can be modified by performing arithmetic operations such as addition, subtraction, and comparison. This is known as pointer arithmetic. It is primarily used to navigate through arrays or dynamically allocated memory.
Pointer Arithmetic Operations
The following are the key operations you can perform with pointers:
- Incrementing a Pointer (
ptr++
): Moves the pointer to the next memory location based on the type of data it points to. If a pointer is pointing to an integer, incrementing it will move it by the size of an integer (typically 4 bytes in most systems). - Decrementing a Pointer (
ptr--
): Moves the pointer to the previous memory location, again depending on the type of data it points to. - Pointer Addition (
ptr + n
): Adds an integer to the pointer, moving it forward byn
elements of the type it points to. - Pointer Subtraction (
ptr - n
): Subtracts an integer from the pointer, moving it backward byn
elements of the type it points to. - Difference Between Two Pointers (
ptr1 - ptr2
): Computes the number of elements between two pointers that point to the same array or block of memory.
Example of Pointer Arithmetic
The following example demonstrates pointer arithmetic with an array:
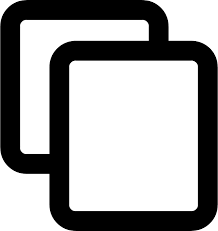
#include <stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int *ptr = arr; // Pointer to the first element of the array
// Accessing array elements using pointer arithmetic
printf("First element: %d\n", *ptr); // Output: 10
ptr++; // Move pointer to the next element
printf("Second element: %d\n", *ptr); // Output: 20
ptr++; // Move pointer to the next element
printf("Third element: %d\n", *ptr); // Output: 30
return 0;
}
Pointer Arithmetic with Arrays
Arrays in C are stored in contiguous memory locations. Pointer arithmetic is useful for traversing an array. If you increment a pointer that points to the first element of an array, it will point to the second element, and so on. Similarly, you can decrement a pointer to move it backward through the array.
Example: Traversing an Array with Pointer Arithmetic
Here's an example that shows how pointer arithmetic can be used to traverse an array:
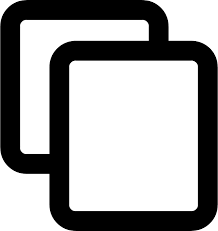
#include <stdio.h>
int main() {
int arr[] = {100, 200, 300, 400, 500};
int *ptr = arr; // Pointer to the first element of the array
printf("Using pointer arithmetic to traverse the array:\n");
// Traverse the array using pointer arithmetic
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i + 1, *ptr);
ptr++; // Increment pointer to next element
}
return 0;
}
Pointer Subtraction
Pointer subtraction can be used to find the difference between two pointers. This operation returns the number of elements between the two pointers, not the byte difference. The pointers must point to the same array or block of memory.
Example: Pointer Subtraction
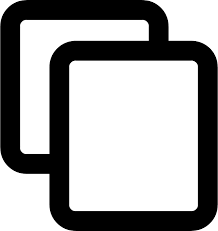
#include <stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int *ptr1 = &arr[0];
int *ptr2 = &arr[4];
// Find the difference between two pointers
int diff = ptr2 - ptr1; // Number of elements between ptr1 and ptr2
printf("Difference between pointers: %d\n", diff); // Output: 4
return 0;
}
Why Use Pointer Arithmetic?
- Efficient Array Traversal: Pointer arithmetic provides a more efficient way to traverse and manipulate arrays, especially when working with large arrays or when using dynamic memory allocation.
- Memory Management: Pointer arithmetic is essential for working with dynamically allocated memory in C, such as memory allocated with functions like
malloc()
orcalloc()
. - Faster Code: Pointer arithmetic can be more efficient than using array indexing in certain scenarios, particularly in tight loops or performance-critical code.
Conclusion
Pointer arithmetic is a powerful and essential feature in C programming. It allows you to manipulate memory locations directly, making it easier to work with arrays and dynamically allocated memory. Mastering pointer arithmetic is an important step in becoming proficient in C programming, enabling you to write efficient and optimized code.
Pointers and Arrays
Pointers and arrays are closely related in C programming. An array is essentially a contiguous block of memory, and a pointer can be used to refer to the address of the first element of an array. Understanding how pointers and arrays work together is crucial for efficiently managing and manipulating data in C.
Understanding Arrays in C
An array in C is a collection of elements of the same type, stored in contiguous memory locations. The name of the array itself is a pointer to the first element in the array. This means that you can use a pointer to access and modify the elements of an array.
How Pointers and Arrays are Related
- Array Name as Pointer: In C, the name of an array is treated as a pointer to the first element of the array. For example, if you have an array
arr[5]
, the expressionarr
is equivalent to&arr[0]
, which is a pointer to the first element of the array. - Array Indexing vs Pointer Arithmetic: You can access array elements using array indexing or pointer arithmetic. Both methods are equivalent. For example,
arr[2]
is the same as*(arr + 2)
, wherearr
is the pointer to the first element.
Accessing Array Elements Using Pointers
You can use pointers to iterate through arrays, just like array indexing. However, using pointers allows for more flexibility and control when working with dynamic arrays or memory manipulation.
Example: Accessing Array Elements Using Pointers
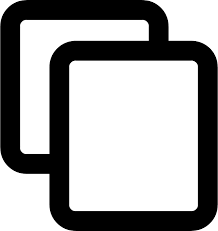
#include <stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int *ptr = arr; // Pointer to the first element of the array
// Accessing array elements using pointer arithmetic
printf("First element: %d\n", *ptr); // Output: 10
ptr++; // Move to the next element
printf("Second element: %d\n", *ptr); // Output: 20
ptr++; // Move to the next element
printf("Third element: %d\n", *ptr); // Output: 30
return 0;
}
Pointer Arithmetic with Arrays
Since arrays are stored in contiguous memory locations, pointer arithmetic is a natural way to traverse an array. When you increment a pointer that points to an array element, the pointer moves to the next element based on the size of the type the pointer is pointing to.
Example: Pointer Arithmetic with Arrays
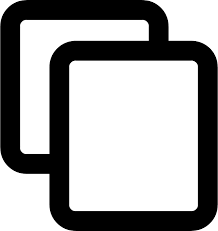
#include <stdio.h>
int main() {
int arr[] = {100, 200, 300, 400, 500};
int *ptr = arr; // Pointer to the first element
// Traverse the array using pointer arithmetic
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i + 1, *ptr);
ptr++; // Move the pointer to the next element
}
return 0;
}
Using Pointers to Modify Array Elements
You can modify the elements of an array by dereferencing the pointer and assigning new values to the memory locations.
Example: Modifying Array Elements Using Pointers
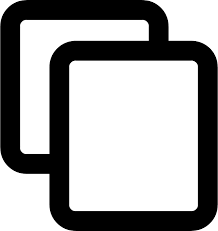
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int *ptr = arr; // Pointer to the first element
// Modify the array elements using pointer arithmetic
*ptr = 10; // Change the first element to 10
*(ptr + 2) = 30; // Change the third element to 30
// Print modified array
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i + 1, arr[i]);
}
return 0;
}
Pointer to Array vs Array of Pointers
It is essential to understand the difference between a pointer to an array and an array of pointers:
- Pointer to an Array: A pointer that points to the first element of an array. It can access the array elements using pointer arithmetic, as shown in the examples above.
- Array of Pointers: An array where each element is a pointer. It is useful when dealing with multiple arrays or strings. Each element of the array holds the address of a different location in memory.
Example: Pointer to Array
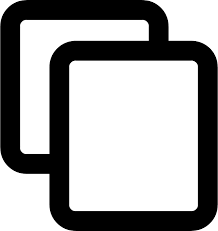
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int (*ptr)[5] = &arr; // Pointer to an array of 5 integers
printf("First element: %d\n", (*ptr)[0]); // Accessing first element using pointer to array
return 0;
}
Example: Array of Pointers
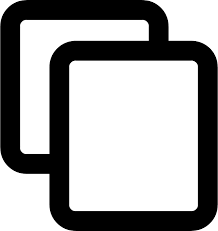
#include <stdio.h>
int main() {
int *arr[5];
int a = 10, b = 20, c = 30, d = 40, e = 50;
arr[0] = &a;
arr[1] = &b;
arr[2] = &c;
arr[3] = &d;
arr[4] = &e;
// Accessing elements through the array of pointers
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i + 1, *arr[i]);
}
return 0;
}
Conclusion
Pointers and arrays are fundamental concepts in C programming. Understanding the relationship between them allows you to efficiently work with arrays, pass arrays to functions, and perform operations like pointer arithmetic. Mastery of pointers and arrays is essential for writing efficient, memory-safe code in C.
Pointers to Functions
Pointers to functions in C allow you to store the address of a function and call it indirectly through the pointer. This technique is useful for dynamic function calls, implementing callback functions, and creating more flexible and reusable code.
What is a Pointer to a Function?
A pointer to a function is a pointer variable that stores the address of a function. You can use this pointer to call the function indirectly. The syntax for declaring a pointer to a function is slightly different from pointers to variables, as you must also specify the function signature (return type and parameter types).
Declaring a Pointer to a Function
The syntax for declaring a pointer to a function is as follows:
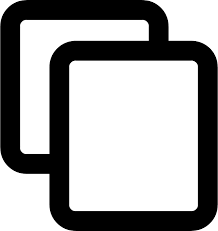
return_type (*pointer_name)(parameter_types);
Where:
- return_type: The return type of the function (e.g.,
int
,void
, etc.). - pointer_name: The name of the pointer variable that will hold the address of the function.
- parameter_types: A list of the types of parameters the function takes (e.g.,
int, float
).
Example: Declaring a Pointer to a Function
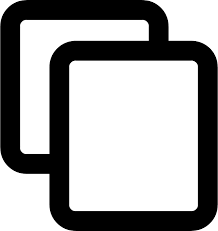
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int main() {
// Declaration of function pointer
int (*func_ptr)(int, int);
// Assigning the address of the function 'add' to the pointer
func_ptr = add;
// Calling the function through the pointer
int result = func_ptr(2, 3);
printf("Result: %d\n", result); // Output: Result: 5
return 0;
}
Calling Functions Using Pointers
Once a function pointer is assigned the address of a function, it can be used to call that function by dereferencing the pointer. Calling a function through a pointer is similar to calling the function directly, but you use the pointer variable instead of the function name.
Example: Calling a Function Using a Pointer
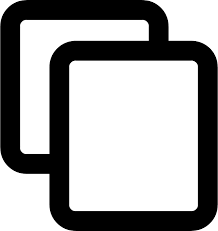
#include <stdio.h>
void greet() {
printf("Hello, world!\n");
}
int main() {
// Function pointer declaration
void (*greet_ptr)();
// Assigning the address of the greet function
greet_ptr = greet;
// Calling the greet function via the pointer
greet_ptr(); // Output: Hello, world!
return 0;
}
Using Function Pointers with Arrays
Function pointers can also be stored in arrays, allowing you to call different functions dynamically based on some condition or input. This is particularly useful when you have a set of similar functions and want to select one at runtime.
Example: Using an Array of Function Pointers
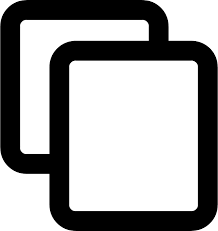
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
int main() {
// Array of function pointers
int (*operations[3])(int, int) = {add, subtract, multiply};
// Calling functions through the array of pointers
int x = 10, y = 5;
printf("Addition: %d\n", operations[0](x, y)); // Output: Addition: 15
printf("Subtraction: %d\n", operations[1](x, y)); // Output: Subtraction: 5
printf("Multiplication: %d\n", operations[2](x, y)); // Output: Multiplication: 50
return 0;
}
Passing Function Pointers as Arguments
You can pass function pointers as arguments to other functions. This is a common technique used in callback functions, where you pass a pointer to a function to another function that will call it at a later time.
Example: Passing Function Pointers as Arguments
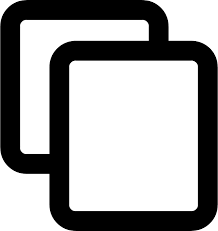
#include <stdio.h>
void execute_operation(int a, int b, int (*operation)(int, int)) {
int result = operation(a, b); // Calling the function via the pointer
printf("Result: %d\n", result);
}
int add(int a, int b) {
return a + b;
}
int main() {
// Passing function pointer to another function
execute_operation(5, 3, add); // Output: Result: 8
return 0;
}
Returning Function Pointers
In C, you can also return function pointers from other functions. This is useful when you need to decide at runtime which function to call based on certain conditions.
Example: Returning a Function Pointer
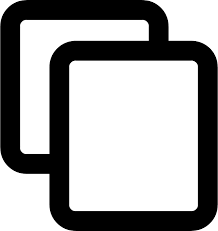
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
// Function that returns a function pointer
int (*get_operation(int choice))(int, int) {
if (choice == 1)
return add;
else
return subtract;
}
int main() {
// Get function pointer based on user input
int (*operation)(int, int) = get_operation(1);
printf("Result: %d\n", operation(5, 3)); // Output: Result: 8
return 0;
}
Conclusion
Pointers to functions are a powerful feature in C that allows for more dynamic and flexible programming. By using function pointers, you can implement callback functions, dynamically choose which functions to execute, and create more modular and reusable code. Mastering function pointers is essential for handling complex situations in C programming, such as when working with event-driven programming or callback mechanisms.
Double Pointers in C
A double pointer in C is a pointer to a pointer. It is a variable that stores the address of another pointer. Double pointers are useful when you need to work with multidimensional arrays, dynamic memory allocation, or modify the value of a pointer from within a function.
What is a Double Pointer?
A double pointer is a pointer that points to another pointer, which in turn points to a value or memory address. The syntax for declaring a double pointer is:
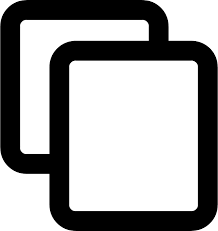
data_type **pointer_name;
Where:
- data_type: The data type the pointer points to (e.g.,
int
,char
, etc.). - pointer_name: The name of the double pointer.
Example: Declaring a Double Pointer
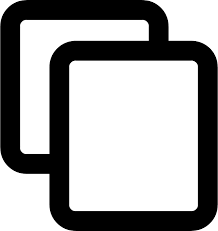
#include <stdio.h>
int main() {
int a = 5;
// Declare a pointer to int
int *ptr = &a;
// Declare a double pointer to pointer to int
int **dbl_ptr = &ptr;
printf("Value of a: %d\n", a); // Output: Value of a: 5
printf("Value of ptr: %d\n", *ptr); // Output: Value of ptr: 5
printf("Value of dbl_ptr: %d\n", **dbl_ptr); // Output: Value of dbl_ptr: 5
return 0;
}
Using Double Pointers
You can access the value stored at a double pointer by dereferencing it twice. The first dereference gives you the pointer it points to, and the second dereference gives you the value that pointer points to.
Example: Accessing Values Using Double Pointers
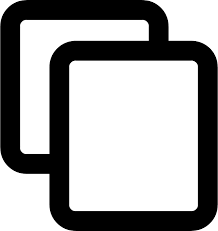
#include <stdio.h>
int main() {
int a = 5;
int *ptr = &a; // Pointer to int
int **dbl_ptr = &ptr; // Double pointer to pointer to int
// Accessing value through double pointer
printf("Value of a: %d\n", **dbl_ptr); // Output: Value of a: 5
return 0;
}
Double Pointers with Arrays
Double pointers are particularly useful when working with multidimensional arrays or dynamically allocated arrays. They can be used to handle arrays of pointers, especially in functions where you want to modify the original array.
Example: Double Pointer with 2D Arrays
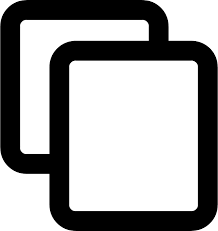
#include <stdio.h>
int main() {
int rows = 2, cols = 3;
// Declare a 2D array dynamically using double pointer
int **arr = (int **)malloc(rows * sizeof(int *));
for (int i = 0; i < rows; i++) {
arr[i] = (int *)malloc(cols * sizeof(int));
}
// Initialize the 2D array
arr[0][0] = 1; arr[0][1] = 2; arr[0][2] = 3;
arr[1][0] = 4; arr[1][1] = 5; arr[1][2] = 6;
// Print the 2D array
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
// Free the allocated memory
for (int i = 0; i < rows; i++) {
free(arr[i]);
}
free(arr);
return 0;
}
Modifying Values Using Double Pointers
Double pointers are useful for modifying values indirectly through a function. You can pass a double pointer to a function and modify the value of the pointer it points to.
Example: Modifying Pointer Values Using a Double Pointer
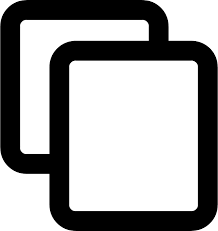
#include <stdio.h>
void modify_pointer(int **ptr) {
int b = 10;
*ptr = &b; // Modify the pointer to point to b
}
int main() {
int a = 5;
int *ptr = &a;
printf("Before: %d\n", *ptr); // Output: Before: 5
modify_pointer(&ptr); // Pass pointer to function
printf("After: %d\n", *ptr); // Output: After: 10
return 0;
}
Conclusion
Double pointers are a powerful feature in C that allows you to work with pointers more flexibly. They are essential when working with multidimensional arrays, dynamic memory allocation, and when you need to modify pointers within a function. Understanding double pointers is crucial for handling more advanced memory management and pointer manipulation in C programming.
Defining Structures in C
A structure in C is a user-defined data type that allows grouping variables of different data types under a single unit. Structures are useful for representing real-world entities with different properties (e.g., a student or a book) in a program. In C, structures are defined using the struct
keyword.
What is a Structure?
A structure is a collection of variables, possibly of different types, that are grouped together under a single name. Each variable inside a structure is called a member or field. Structures are typically used to represent a record, such as a student’s details (name, age, and grade).
Syntax for Defining a Structure
The general syntax for defining a structure is:
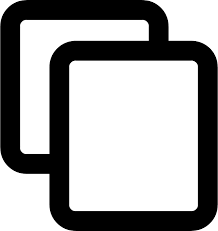
struct structure_name {
data_type member1;
data_type member2;
...
};
Where:
- structure_name: The name of the structure.
- member1, member2, ...: The members of the structure, each with a specified data type.
Example: Defining a Structure
Here is an example of defining a structure to represent a student with name, age, and grade as its members:
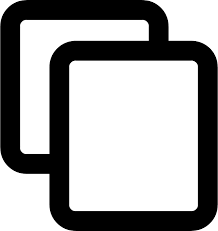
#include <stdio.h>
// Define a structure to represent a student
struct Student {
char name[50];
int age;
float grade;
};
int main() {
// Declare a structure variable
struct Student student1;
// Assign values to members
strcpy(student1.name, "John Doe");
student1.age = 20;
student1.grade = 85.5;
// Print structure members
printf("Name: %s\n", student1.name);
printf("Age: %d\n", student1.age);
printf("Grade: %.2f\n", student1.grade);
return 0;
}
Accessing Structure Members
You can access the members of a structure using the dot operator (.
). The dot operator is used to refer to the members of a structure variable.
Example: Accessing Structure Members
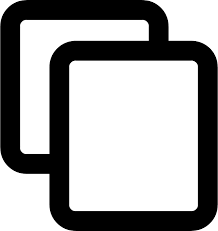
#include <stdio.h>
struct Student {
char name[50];
int age;
float grade;
};
int main() {
struct Student student1 = {"Alice", 22, 92.5};
// Accessing structure members
printf("Name: %s\n", student1.name); // Output: Name: Alice
printf("Age: %d\n", student1.age); // Output: Age: 22
printf("Grade: %.2f\n", student1.grade); // Output: Grade: 92.50
return 0;
}
Defining and Initializing Structures
You can define and initialize the members of a structure at the time of declaration.
Example: Initializing a Structure at Declaration
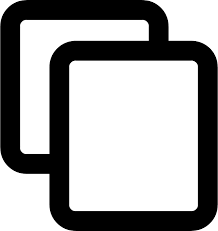
#include <stdio.h>
struct Student {
char name[50];
int age;
float grade;
};
int main() {
// Initialize structure at the time of declaration
struct Student student1 = {"Bob", 19, 88.0};
// Print structure members
printf("Name: %s\n", student1.name); // Output: Name: Bob
printf("Age: %d\n", student1.age); // Output: Age: 19
printf("Grade: %.2f\n", student1.grade); // Output: Grade: 88.00
return 0;
}
Using Structure Pointers
When working with structures, you can also use pointers to structures. The members of a structure can be accessed through a structure pointer using the arrow operator (->
).
Example: Using Structure Pointers
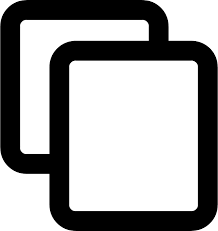
#include <stdio.h>
struct Student {
char name[50];
int age;
float grade;
};
int main() {
struct Student student1 = {"Charlie", 21, 75.5};
struct Student *ptr = &student1;
// Access structure members using pointer
printf("Name: %s\n", ptr->name); // Output: Name: Charlie
printf("Age: %d\n", ptr->age); // Output: Age: 21
printf("Grade: %.2f\n", ptr->grade); // Output: Grade: 75.50
return 0;
}
Conclusion
Structures in C are powerful tools for organizing related data of different types. They allow you to define complex data types and group them together, making programs easier to understand and manage. Understanding how to define and use structures is essential for working with real-world data in C programming.
Accessing Members of a Structure in C
In C, once you have defined a structure, you can access its members using a couple of methods. The two primary methods are using the dot operator (.) for structure variables and the arrow operator (->) for structure pointers.
Using the Dot Operator
The dot operator is used to access the members of a structure variable. This is the most common way to access structure members when you have a structure variable.
Syntax for the Dot Operator
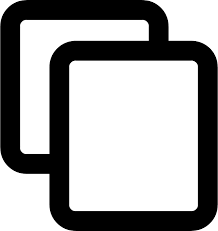
structure_variable.member_name
Where:
- structure_variable: The variable of the structure type.
- member_name: The member you want to access within the structure.
Example: Accessing Members Using the Dot Operator
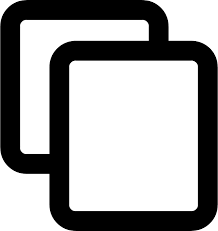
#include <stdio.h>
struct Student {
char name[50];
int age;
float grade;
};
int main() {
struct Student student1 = {"John Doe", 20, 89.5};
// Access structure members using dot operator
printf("Name: %s\n", student1.name); // Output: Name: John Doe
printf("Age: %d\n", student1.age); // Output: Age: 20
printf("Grade: %.2f\n", student1.grade); // Output: Grade: 89.50
return 0;
}
Using the Arrow Operator
The arrow operator (->
) is used to access members of a structure through a pointer to the structure. If you have a pointer to a structure, you cannot use the dot operator. Instead, you use the arrow operator.
Syntax for the Arrow Operator
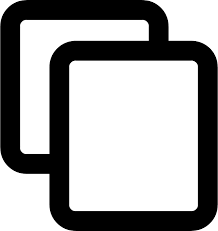
structure_pointer->member_name
Where:
- structure_pointer: A pointer to the structure.
- member_name: The member you want to access within the structure.
Example: Accessing Members Using the Arrow Operator
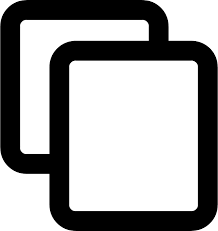
#include <stdio.h>
struct Student {
char name[50];
int age;
float grade;
};
int main() {
struct Student student1 = {"Alice", 22, 92.5};
struct Student *ptr = &student1;
// Access structure members using arrow operator
printf("Name: %s\n", ptr->name); // Output: Name: Alice
printf("Age: %d\n", ptr->age); // Output: Age: 22
printf("Grade: %.2f\n", ptr->grade); // Output: Grade: 92.50
return 0;
}
Important Notes
- When you are accessing members of a structure variable, always use the dot operator.
- If you have a pointer to a structure, use the arrow operator to access its members.
- Using the arrow operator is equivalent to dereferencing the structure pointer and then using the dot operator, so
*ptr.name
andptr->name
are functionally equivalent.
Conclusion
Accessing members of a structure in C is easy and can be done either using the dot operator for structure variables or the arrow operator for structure pointers. Understanding how to access and manipulate structure members is essential for working with structured data in C programming.
Nested Structures in C
In C, a structure can contain other structures as its members. This concept is called a "nested structure." Nested structures are useful for modeling complex data structures by combining simple structures.
What Are Nested Structures?
A nested structure is a structure that has another structure as a member. This allows you to organize and manage data in a hierarchical manner.
Syntax of Nested Structures
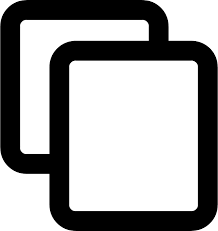
struct outer_structure {
struct inner_structure {
// Members of inner structure
} inner_structure_variable;
// Other members of outer structure
};
Here, the inner structure is defined within the outer structure.
Example of Nested Structures
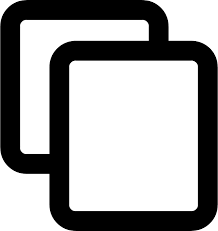
#include <stdio.h>
struct Date {
int day;
int month;
int year;
};
struct Employee {
char name[50];
float salary;
struct Date joiningDate; // Nested structure
};
int main() {
struct Employee emp = {"John Doe", 55000.50, {25, 12, 2020}};
// Accessing members of the nested structure
printf("Employee Name: %s\n", emp.name);
printf("Employee Salary: %.2f\n", emp.salary);
printf("Joining Date: %d/%d/%d\n", emp.joiningDate.day, emp.joiningDate.month, emp.joiningDate.year);
return 0;
}
Accessing Nested Structure Members
To access the members of a nested structure, you can use the dot operator multiple times, once for each level of the structure.
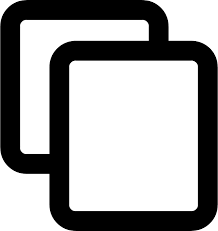
outer_structure_variable.inner_structure_variable.member_name
Example: Accessing Nested Structure Members
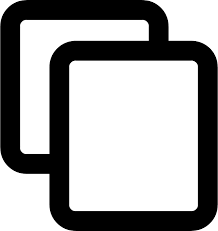
#include <stdio.h>
struct Date {
int day;
int month;
int year;
};
struct Employee {
char name[50];
float salary;
struct Date joiningDate; // Nested structure
};
int main() {
struct Employee emp = {"Alice", 60000.75, {15, 8, 2021}};
// Accessing members of the nested structure using dot operator
printf("Employee Name: %s\n", emp.name);
printf("Employee Salary: %.2f\n", emp.salary);
printf("Joining Date: %d/%d/%d\n", emp.joiningDate.day, emp.joiningDate.month, emp.joiningDate.year);
return 0;
}
Nested Structures with Pointers
If you have a pointer to a structure with nested structures, you can access the members using the arrow operator (->).
Syntax for Accessing Nested Structure Members with Pointers
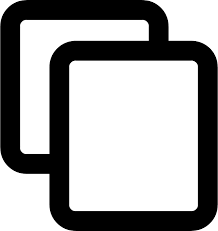
structure_pointer->inner_structure_variable.member_name
Example: Nested Structure with Pointer
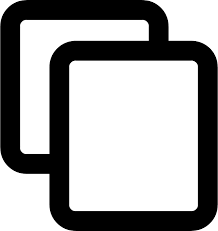
#include <stdio.h>
struct Date {
int day;
int month;
int year;
};
struct Employee {
char name[50];
float salary;
struct Date joiningDate; // Nested structure
};
int main() {
struct Employee emp = {"Bob", 45000.25, {1, 1, 2022}};
struct Employee *emp_ptr = &emp;
// Accessing nested structure members using arrow operator
printf("Employee Name: %s\n", emp_ptr->name);
printf("Employee Salary: %.2f\n", emp_ptr->salary);
printf("Joining Date: %d/%d/%d\n", emp_ptr->joiningDate.day, emp_ptr->joiningDate.month, emp_ptr->joiningDate.year);
return 0;
}
Advantages of Nested Structures
- Modularization: Nested structures allow you to group related data together, leading to better organization and modularity of the program.
- Modeling Complex Data: Complex data structures can be modeled efficiently, like representing an employee with both personal information and employment details.
- Code Readability: Using nested structures makes the code more readable and easier to maintain, especially for complex data types.
Conclusion
Nested structures are an effective way to model complex data in C. By using nested structures, you can group related data together and organize your program more efficiently. Accessing nested structure members requires using the dot or arrow operator, depending on whether you are working with structure variables or pointers.
Introduction to Unions in C
In C programming, a union is a special data type that allows you to store different data types in the same memory location. Unlike structures, where each member has its own memory, in a union, all members share the same memory space. This means that a union can only hold one of its members at a time, but it saves memory by using the same location for all its members.
What is a Union?
A union is a data structure similar to a structure in C, but with a key difference: all members of a union share the same memory space. The memory size of a union is determined by the size of its largest member. This helps conserve memory but limits you to using only one member at a time.
Syntax of a Union
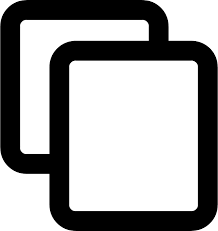
union union_name {
data_type member1;
data_type member2;
data_type member3;
};
In the syntax above, the union is defined with a name (`union_name`), and it has several members (`member1`, `member2`, etc.), each with a data type. All members share the same memory location.
Example of a Union
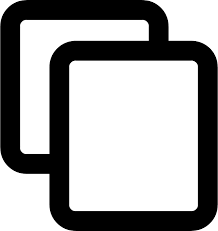
#include <stdio.h>
union Data {
int i;
float f;
char str[20];
};
int main() {
union Data data;
// Storing an integer in the union
data.i = 10;
printf("data.i: %d\n", data.i);
// Storing a float in the union (will overwrite the previous value)
data.f = 3.14;
printf("data.f: %.2f\n", data.f);
// Storing a string in the union (will overwrite the previous value)
strcpy(data.str, "Hello, Union!");
printf("data.str: %s\n", data.str);
return 0;
}
In the above example, the `union Data` is used to store an integer, a float, and a string. However, only the last assigned member will hold a valid value, as all members share the same memory location.
Accessing Union Members
Union members are accessed in the same way as structure members, using the dot operator. However, only the last assigned member will hold a valid value.
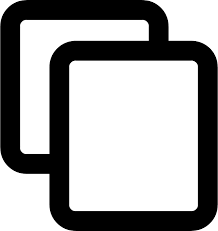
union_variable.member_name
Example: Accessing Union Members
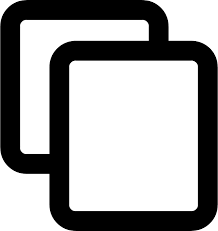
#include <stdio.h>
union Data {
int i;
float f;
char str[20];
};
int main() {
union Data data;
// Assigning an integer value
data.i = 10;
printf("data.i: %d\n", data.i);
// Assigning a float value (overwrites the integer)
data.f = 3.14;
printf("data.f: %.2f\n", data.f);
// Assigning a string (overwrites the float)
strcpy(data.str, "Hello, Union!");
printf("data.str: %s\n", data.str);
return 0;
}
Memory Size of a Union
The memory size of a union is determined by the size of its largest member. This is because all members share the same memory location. In the example above, the union `Data` will have a size of the largest member, which is the string `str` in this case. The size of a union can be determined using the sizeof
operator.
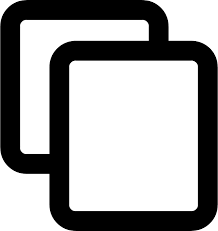
printf("Size of union Data: %lu\n", sizeof(data));
Advantages of Unions
- Memory Efficiency: Unions save memory because all members share the same memory location.
- Flexible Data Representation: Unions allow different types of data to be stored in the same memory location, making them useful for applications that need to handle multiple data types.
Disadvantages of Unions
- Limited Usage: Only one member of a union can hold a value at a time, so it’s not suitable for storing multiple pieces of data simultaneously.
- Memory Overwrite: Assigning a value to one member of a union will overwrite the value of the previously assigned member.
Conclusion
Unions are a useful feature in C when you need to store different types of data in the same memory location. They save memory and offer flexible data representation, but the limitation of only being able to store one member at a time should be kept in mind when using them.
Difference Between Structures and Unions in C
In C programming, both structures and unions are used to group different data types together. However, they differ significantly in how they store the data and their memory usage. Understanding these differences is essential for choosing the right data structure for a particular use case.
What is a Structure?
A structure is a user-defined data type in C that groups different types of data under a single name. Each member of a structure has its own memory location, which means that all members of a structure are stored separately in memory.
What is a Union?
A union is similar to a structure, but with a key difference: all members of a union share the same memory location. This means that at any given time, a union can store only one of its members, but it saves memory by using a single memory block for all its members.
Key Differences Between Structures and Unions
Feature | Structure | Union |
---|---|---|
Memory Allocation | Each member has its own memory location. The total memory required is the sum of the sizes of all members. | All members share the same memory location. The memory required is the size of the largest member. |
Storage | All members of a structure can hold values simultaneously. | Only one member can hold a value at a time; others are overwritten. |
Access to Members | All members can be accessed and used at the same time. | Only the most recently assigned member holds a valid value. |
Memory Usage | Structures use more memory as each member occupies separate memory space. | Unions use less memory since all members share the same memory space. |
Use Case | Used when you need to store multiple different values simultaneously. | Used when you need to store different data types but only need one value at a time. |
Example: Structure
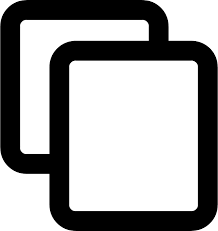
#include <stdio.h>
struct Employee {
int id;
char name[50];
float salary;
};
int main() {
struct Employee emp1 = {101, "John Doe", 50000.50};
printf("Employee ID: %d\n", emp1.id);
printf("Employee Name: %s\n", emp1.name);
printf("Employee Salary: %.2f\n", emp1.salary);
return 0;
}
Example: Union
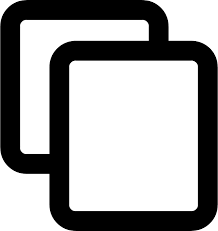
#include <stdio.h>
union Data {
int i;
float f;
char str[20];
};
int main() {
union Data data;
data.i = 10; // Assigning integer value
printf("data.i: %d\n", data.i);
data.f = 3.14; // Assigning float value (overwrites integer)
printf("data.f: %.2f\n", data.f);
strcpy(data.str, "Hello, Union!"); // Assigning string value (overwrites float)
printf("data.str: %s\n", data.str);
return 0;
}
When to Use a Structure vs. a Union
Choosing between a structure and a union depends on your requirements:
- Use a structure when you need to store multiple pieces of data at the same time, and each data member needs to have its own memory location.
- Use a union when you need to store different types of data, but only one value will be used at a time, and you want to conserve memory.
Conclusion
Both structures and unions are important features of C programming, allowing you to work with complex data. Structures are ideal when you need to store multiple values simultaneously, while unions are more memory-efficient when only one value is needed at a time.
Introduction to File Handling in C
File handling in C refers to the process of reading from and writing to files. It allows programs to store data persistently and retrieve it later. File handling is essential when working with large datasets or when you need to save user data between program executions.
What is a File?
A file is a collection of data stored on a disk or other storage device. It can be of various types, such as text files, binary files, and more. C provides functions to interact with these files, enabling operations like opening, reading, writing, and closing.
Types of Files
- Text Files: These files contain human-readable characters, often with line breaks. They are easy to edit and read.
- Binary Files: These files contain data in binary format (0s and 1s), and they are more efficient for storing complex data types.
File Handling Functions in C
C provides several functions for performing file operations. These functions are declared in the stdio.h
header file. The most commonly used file handling functions are:
- fopen(): Opens a file and returns a pointer to it. It takes the file name and mode (e.g., read, write) as arguments.
- fclose(): Closes an opened file.
- fread(): Reads data from a file into a buffer.
- fwrite(): Writes data from a buffer to a file.
- fgets(): Reads a line of text from a file.
- fputs(): Writes a string to a file.
- fprintf(): Writes formatted output to a file.
- fscanf(): Reads formatted input from a file.
Modes for Opening a File
The fopen()
function requires a mode that specifies the operation to be performed on the file. Here are the common file modes:
Mode | Meaning |
---|---|
"r" | Open for reading. The file must exist. |
"w" | Open for writing. If the file does not exist, it will be created. If it exists, its contents will be overwritten. |
"a" | Open for appending. Data will be written to the end of the file. If the file does not exist, it will be created. |
"rb" | Open for reading in binary mode. |
"wb" | Open for writing in binary mode. |
"ab" | Open for appending in binary mode. |
Example of File Handling in C
Reading from a File
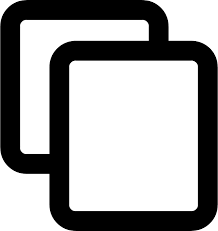
#include <stdio.h>
int main() {
FILE *file;
char line[100];
// Open the file for reading
file = fopen("example.txt", "r");
if (file == NULL) {
printf("Could not open file.\n");
return 1;
}
// Read a line from the file
fgets(line, sizeof(line), file);
printf("File content: %s\n", line);
// Close the file
fclose(file);
return 0;
}
Writing to a File
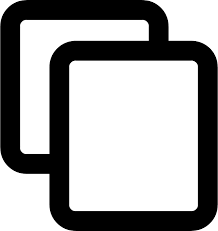
#include <stdio.h>
int main() {
FILE *file;
// Open the file for writing
file = fopen("output.txt", "w");
if (file == NULL) {
printf("Could not open file.\n");
return 1;
}
// Write to the file
fprintf(file, "Hello, File Handling in C!\n");
// Close the file
fclose(file);
printf("Data written to the file successfully.\n");
return 0;
}
File Error Handling
When performing file operations, it's important to check for errors. C provides the ferror()
function to check if an error occurred during the last file operation. Additionally, you can use feof()
to check if the end of the file has been reached.
Closing a File
Once you finish working with a file, it is crucial to close it using the fclose()
function. This ensures that any buffered data is written to the file and that resources are freed.
Conclusion
File handling is an essential concept in C programming, allowing programs to interact with external data stored on a disk. By using functions like fopen()
, fclose()
, fread()
, and fwrite()
, you can read from and write to files in a variety of formats. Understanding file handling can help create programs that persist data, making them more useful and dynamic.
Reading and Writing Files (fopen, fclose, fprintf, fscanf)
In C programming, file handling allows you to perform operations like reading from and writing to files. Functions like fopen()
, fclose()
, fprintf()
, and fscanf()
are commonly used to manage files. These functions help you interact with text and binary files efficiently.
Opening a File - fopen()
The fopen()
function is used to open a file for reading, writing, or appending. It takes two arguments: the name of the file and the mode (the operation you want to perform on the file). It returns a pointer to the file if successful, or NULL
if the file cannot be opened.
Syntax:
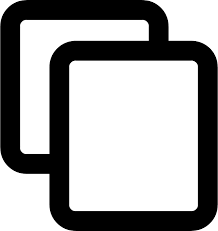
FILE *fopen(const char *filename, const char *mode);
File Modes:
- "r" - Open for reading (file must exist).
- "w" - Open for writing (creates a new file or overwrites an existing file).
- "a" - Open for appending (creates a new file or appends to the existing file).
- "rb" - Open for reading in binary mode.
- "wb" - Open for writing in binary mode.
- "ab" - Open for appending in binary mode.
Closing a File - fclose()
Once you are done with a file, you should close it using the fclose()
function. This ensures that any buffered data is written to the file and that system resources are freed.
Syntax:
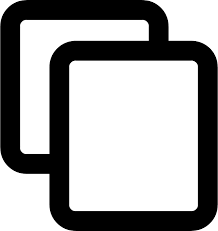
int fclose(FILE *file);
If the file is closed successfully, fclose()
returns 0. If there is an error, it returns EOF.
Writing to a File - fprintf()
The fprintf()
function is used to write formatted data to a file. It is similar to the printf()
function, but instead of printing to the console, it writes to the file.
Syntax:
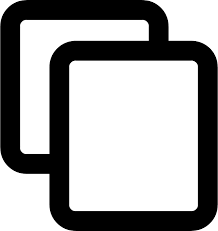
int fprintf(FILE *file, const char *format, ...);
It takes a file pointer, a format string, and the values to write to the file. It returns the number of characters written to the file or a negative value if an error occurs.
Reading from a File - fscanf()
The fscanf()
function is used to read formatted data from a file. It works similarly to the scanf()
function, but instead of reading from the standard input, it reads from a file.
Syntax:
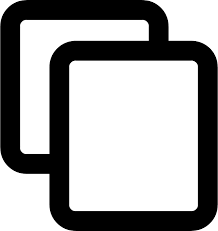
int fscanf(FILE *file, const char *format, ...);
It takes a file pointer, a format string, and variables to store the read values. It returns the number of items successfully read or EOF
if the end of the file is reached or an error occurs.
Example: Writing to a File Using fprintf()
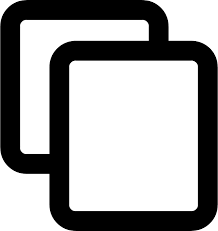
#include <stdio.h>
int main() {
FILE *file;
// Open the file for writing
file = fopen("example.txt", "w");
if (file == NULL) {
printf("Could not open file for writing.\n");
return 1;
}
// Write to the file
fprintf(file, "Hello, World!\n");
fprintf(file, "This is an example of file handling in C.\n");
// Close the file
fclose(file);
printf("Data written to the file successfully.\n");
return 0;
}
Example: Reading from a File Using fscanf()
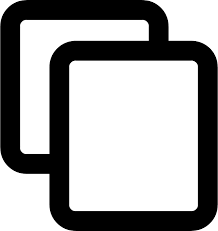
#include <stdio.h>
int main() {
FILE *file;
char str[100];
// Open the file for reading
file = fopen("example.txt", "r");
if (file == NULL) {
printf("Could not open file for reading.\n");
return 1;
}
// Read from the file
fscanf(file, "%s", str);
printf("Read from file: %s\n", str);
// Close the file
fclose(file);
return 0;
}
Error Handling in File Operations
When working with files, it is important to handle errors properly. If fopen()
returns NULL
, it indicates that the file could not be opened. You can also use ferror()
to check if an error occurred during the last file operation.
Syntax for checking file errors:
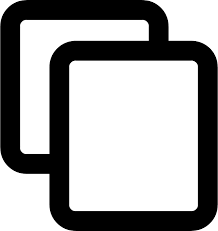
int ferror(FILE *file);
This function returns a non-zero value if an error occurred, and 0 if no error occurred.
Conclusion
File handling in C is an essential skill for working with data that needs to be stored and retrieved. By using functions like fopen()
, fclose()
, fprintf()
, and fscanf()
, you can easily read from and write to files. It's important to remember to handle errors properly and close files to ensure efficient resource management.
Random Access in Files (fseek, ftell, rewind)
In C programming, random access allows you to read or write data at any position within a file, rather than just sequentially. The functions fseek()
, ftell()
, and rewind()
are used to manage file pointers and perform random access operations in files.
Moving the File Pointer - fseek()
The fseek()
function is used to move the file pointer to a specific location in the file. This allows you to read or write data from any position in the file, instead of just the beginning or the end.
Syntax:
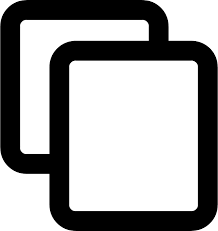
int fseek(FILE *file, long offset, int whence);
Parameters:
file
: A pointer to the file.offset
: The number of bytes to move the pointer (positive or negative).whence
: The starting point for the offset. It can have one of the following values:SEEK_SET
: The beginning of the file.SEEK_CUR
: The current position of the file pointer.SEEK_END
: The end of the file.
If successful, fseek()
returns 0; otherwise, it returns a non-zero value indicating an error.
Getting the Current File Position - ftell()
The ftell()
function is used to get the current position of the file pointer. This is useful after using fseek()
to ensure that the file pointer is at the correct location.
Syntax:
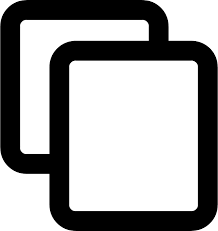
long ftell(FILE *file);
If successful, ftell()
returns the current file pointer position in bytes from the beginning of the file. If there is an error, it returns EOF
.
Resetting the File Pointer - rewind()
The rewind()
function is used to reset the file pointer to the beginning of the file. This is useful when you want to read the file from the start after performing some operations.
Syntax:
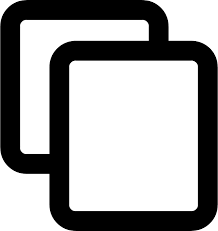
void rewind(FILE *file);
The rewind()
function does not return any value. It resets the file pointer to the beginning of the file and clears the error and end-of-file indicators for the file.
Example: Using fseek()
, ftell()
, and rewind()
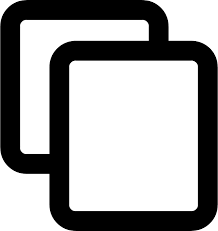
#include <stdio.h>
int main() {
FILE *file;
char ch;
// Open the file for reading
file = fopen("example.txt", "r");
if (file == NULL) {
printf("Could not open file.\n");
return 1;
}
// Move the file pointer to the 10th byte from the beginning
fseek(file, 10, SEEK_SET);
// Get the current position of the file pointer
long position = ftell(file);
printf("Current file position: %ld\n", position);
// Read a character from the new file position
ch = fgetc(file);
printf("Character at position 10: %c\n", ch);
// Move the file pointer 5 bytes from the current position
fseek(file, 5, SEEK_CUR);
// Read a character from the new position
ch = fgetc(file);
printf("Character after moving 5 bytes forward: %c\n", ch);
// Reset the file pointer to the beginning of the file
rewind(file);
// Read and print the first character after rewind
ch = fgetc(file);
printf("First character after rewind: %c\n", ch);
// Close the file
fclose(file);
return 0;
}
Explanation of the Example
- fseek(file, 10, SEEK_SET): Moves the file pointer to the 10th byte from the beginning of the file.
- ftell(file): Returns the current file pointer position (10 bytes from the start in this case).
- fgetc(file): Reads a character from the current position of the file pointer.
- fseek(file, 5, SEEK_CUR): Moves the file pointer 5 bytes forward from the current position.
- rewind(file): Resets the file pointer to the beginning of the file.
Use Cases for Random Access
Random access is particularly useful when you need to:
- Read or write specific parts of a large file without processing the entire file sequentially.
- Implement file-based databases where records can be accessed directly using an index.
- Update parts of a file (such as modifying a specific record in a log file) without re-writing the entire file.
Conclusion
Random access in C allows for efficient reading and writing at any position within a file. By using functions like fseek()
, ftell()
, and rewind()
, you can move the file pointer to desired locations and manage file operations more flexibly. These functions are crucial for tasks that require direct access to specific parts of a file.
Working with Binary Files
In C programming, binary files are files that contain data in binary format (i.e., in the form of 0s and 1s), as opposed to text files, which store data as human-readable characters. Working with binary files allows you to store data in its raw format, which is more efficient for large data and non-textual data like images, videos, or complex data structures.
Why Use Binary Files?
Binary files are used when:
- You need to store large or complex data that is not suitable for plain text (e.g., images, audio, or structured data).
- You want to preserve the exact representation of data without any formatting or encoding conversion.
- You need to read or write data faster than with text files, as binary files avoid the overhead of converting between text and binary formats.
Reading and Writing Binary Files
In C, you can read and write binary files using the fread()
and fwrite()
functions. These functions allow you to directly read and write blocks of data from and to the file, rather than character by character.
Writing to a Binary File - fwrite()
The fwrite()
function is used to write data to a binary file. It writes data in chunks of memory (instead of characters) to the file.
Syntax:
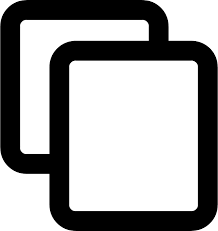
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
Parameters:
ptr
: A pointer to the memory block to write.size
: The size (in bytes) of each element to write.count
: The number of elements to write.stream
: A pointer to the file where data will be written.
fwrite()
returns the total number of elements successfully written.
Reading from a Binary File - fread()
The fread()
function is used to read data from a binary file. It reads chunks of data and stores them in a memory block (instead of reading one character at a time).
Syntax:
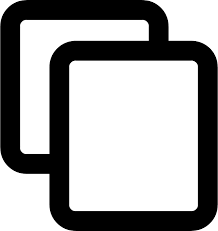
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
Parameters:
ptr
: A pointer to the memory block where data will be stored.size
: The size (in bytes) of each element to read.count
: The number of elements to read.stream
: A pointer to the file from which data will be read.
fread()
returns the total number of elements successfully read.
Example: Writing and Reading a Binary File
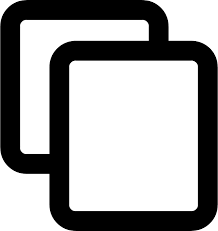
#include <stdio.h>
#include <string.h>
struct Person {
char name[30];
int age;
};
int main() {
FILE *file;
struct Person person = {"John Doe", 25};
// Open the binary file for writing
file = fopen("person.dat", "wb");
if (file == NULL) {
printf("Unable to open file for writing.\n");
return 1;
}
// Write the structure to the binary file
fwrite(&person, sizeof(struct Person), 1, file);
// Close the file
fclose(file);
// Reading the data back from the file
file = fopen("person.dat", "rb");
if (file == NULL) {
printf("Unable to open file for reading.\n");
return 1;
}
// Read the structure from the binary file
struct Person readPerson;
fread(&readPerson, sizeof(struct Person), 1, file);
// Close the file
fclose(file);
// Display the read data
printf("Name: %s\n", readPerson.name);
printf("Age: %d\n", readPerson.age);
return 0;
}
Explanation of the Example
- fopen("person.dat", "wb"): Opens the file "person.dat" in binary write mode. If the file does not exist, it is created.
- fwrite(&person, sizeof(struct Person), 1, file): Writes the entire structure
person
to the file in binary format. - fopen("person.dat", "rb"): Opens the file "person.dat" in binary read mode.
- fread(&readPerson, sizeof(struct Person), 1, file): Reads the structure from the file into the
readPerson
variable. - printf("Name: %s\n", readPerson.name): Prints the name of the person read from the file.
Binary File Advantages
- Efficiency: Binary files are more efficient in terms of both storage space and speed, as no encoding is required.
- Exact Representation: Data is stored exactly as it is in memory, ensuring that no information is lost or altered.
- Handling Complex Data: Binary files are ideal for storing complex data structures (like structs) without the need for text conversion.
Binary File Limitations
- Portability: Binary files may not be portable across different systems, as the binary format may vary based on endianness or architecture.
- Human Readability: Unlike text files, binary files are not human-readable, making debugging or manual inspection more difficult.
Conclusion
Working with binary files in C provides a way to efficiently store and manipulate complex data. Using functions like fwrite()
and fread()
, you can directly read and write data to binary files, which is especially useful for large datasets or non-textual information. While binary files offer performance and data integrity benefits, they come with some portability and readability challenges that need to be considered.
Memory Management Functions (malloc, calloc, realloc, free)
In C programming, memory management refers to the process of dynamically allocating and deallocating memory during the execution of a program. This is done through a set of functions that allow the programmer to control memory usage manually. The four primary memory management functions in C are malloc()
, calloc()
, realloc()
, and free()
.
1. malloc()
- Memory Allocation
The malloc()
function is used to allocate a specified amount of memory at runtime. It returns a pointer to the first byte of the allocated memory block. If the memory allocation fails, it returns NULL
.
Syntax:
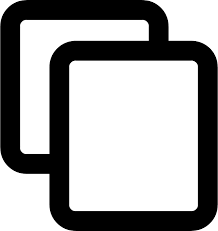
void *malloc(size_t size);
Parameters:
size
: The number of bytes to allocate.
Example:
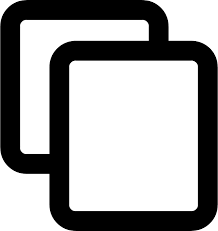
int *arr = (int *)malloc(5 * sizeof(int));
This allocates memory for an array of 5 integers. Remember to always check if malloc()
returns NULL
, indicating that the memory allocation failed.
2. calloc()
- Contiguous Memory Allocation
The calloc()
function allocates memory for an array of elements and initializes all bytes to zero. It is similar to malloc()
but with the added benefit of initialization.
Syntax:
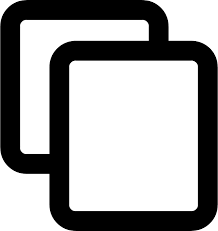
void *calloc(size_t num, size_t size);
Parameters:
num
: The number of elements to allocate.size
: The size of each element in bytes.
Example:
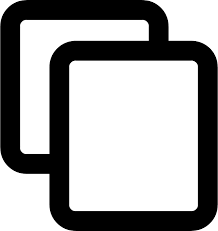
int *arr = (int *)calloc(5, sizeof(int));
This allocates memory for an array of 5 integers and initializes all the values to zero.
3. realloc()
- Reallocation of Memory
The realloc()
function is used to change the size of a previously allocated memory block. It can increase or decrease the size, depending on the specified size. If the memory block cannot be resized, it returns a NULL
pointer, and the original memory block remains unchanged.
Syntax:
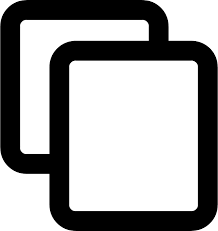
void *realloc(void *ptr, size_t size);
Parameters:
ptr
: A pointer to the previously allocated memory block.size
: The new size for the memory block in bytes.
Example:
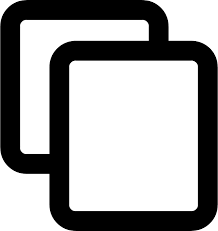
arr = (int *)realloc(arr, 10 * sizeof(int));
This reallocates the memory block pointed to by arr
to hold space for 10 integers.
4. free()
- Deallocating Memory
The free()
function is used to release previously allocated memory. After calling free()
, the pointer becomes invalid and should not be used again unless it is reallocated.
Syntax:
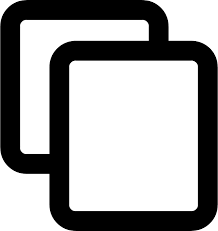
void free(void *ptr);
Parameters:
ptr
: A pointer to the memory block to be freed.
Example:
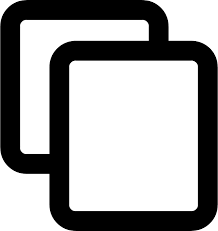
free(arr);
This releases the memory block allocated for the array arr
.
Example of Using Memory Management Functions
Below is an example that demonstrates the usage of malloc()
, calloc()
, realloc()
, and free()
in a program:
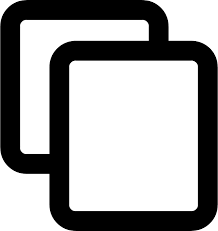
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr;
int i;
// Allocating memory using malloc
arr = (int *)malloc(5 * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
// Initializing array using malloc
for (i = 0; i < 5; i++) {
arr[i] = i + 1;
}
// Reallocating memory using realloc
arr = (int *)realloc(arr, 10 * sizeof(int));
if (arr == NULL) {
printf("Memory reallocation failed.\n");
return 1;
}
// Initializing new elements after realloc
for (i = 5; i < 10; i++) {
arr[i] = i + 1;
}
// Printing array elements
for (i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// Freeing allocated memory
free(arr);
return 0;
}
Explanation of the Example
- malloc(5 * sizeof(int)): Allocates memory for 5 integers using
malloc()
. - realloc(arr, 10 * sizeof(int)): Resizes the array to hold space for 10 integers using
realloc()
. - free(arr): Releases the allocated memory using
free()
.
Common Errors to Avoid
- Not checking for NULL pointers: Always check if memory allocation or reallocation was successful by verifying that the returned pointer is not NULL.
- Not freeing memory: Failing to release memory with
free()
can result in memory leaks, where the program consumes more and more memory over time. - Using freed memory: After freeing a memory block, do not use the pointer again. It becomes invalid.
Conclusion
Memory management is an essential part of C programming that allows you to allocate, resize, and release memory dynamically during program execution. The functions malloc()
, calloc()
, realloc()
, and free()
give you fine-grained control over memory, but they also require careful handling to avoid errors such as memory leaks or segmentation faults.
Difference Between Static and Dynamic Memory Allocation
Memory allocation in C can be broadly classified into two types: Static Memory Allocation and Dynamic Memory Allocation. These two types of memory allocation differ in their mechanism, flexibility, and the time at which memory is allocated and deallocated. Let’s understand each type in detail.
1. Static Memory Allocation
Static memory allocation refers to the process of allocating memory at compile time. The size and type of memory required are known beforehand and remain fixed during the program’s execution. The memory is allocated during the program's startup and deallocated when the program terminates.
Characteristics of Static Memory Allocation:
- Fixed Size: The memory size is fixed at compile time and cannot be changed during runtime.
- Memory Allocation: Memory is allocated at compile time.
- Deallocation: Memory is automatically deallocated when the program terminates.
- Faster Access: Access to statically allocated memory is faster as it is allocated beforehand.
- Limited Flexibility: You cannot change the size of the allocated memory during runtime.
Example of Static Memory Allocation:
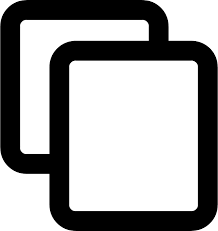
int arr[10]; // Array of 10 integers
In this example, the memory for an array of 10 integers is allocated at compile time, and the size of the array cannot be changed during runtime.
2. Dynamic Memory Allocation
Dynamic memory allocation refers to the process of allocating memory at runtime. In dynamic memory allocation, memory is allocated when needed during the execution of the program. You can allocate memory for variables, arrays, or structures, and the size can be determined during runtime.
Characteristics of Dynamic Memory Allocation:
- Flexible Size: The memory size can be adjusted during runtime based on the program’s needs.
- Memory Allocation: Memory is allocated at runtime using functions like
malloc()
,calloc()
,realloc()
, andfree()
. - Deallocation: Memory must be explicitly deallocated using the
free()
function. Failure to do so can result in memory leaks. - Slower Access: Dynamic memory allocation is generally slower than static memory allocation because it requires runtime management.
- Increased Flexibility: You can allocate and deallocate memory at any point during the program's execution.
Example of Dynamic Memory Allocation:
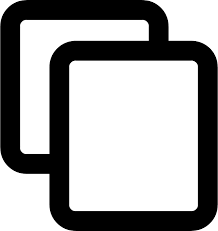
int *arr = (int *)malloc(10 * sizeof(int)); // Dynamically allocate memory for 10 integers
In this example, memory for an array of 10 integers is allocated dynamically at runtime using malloc()
.
Key Differences Between Static and Dynamic Memory Allocation
Property | Static Memory Allocation | Dynamic Memory Allocation |
---|---|---|
Memory Allocation Time | At compile time | At runtime |
Memory Size | Fixed size | Variable size |
Memory Management | Managed by the compiler | Managed by the programmer (using malloc, calloc, realloc, free) |
Speed | Faster access | Slower access |
Flexibility | Low flexibility (size cannot be changed) | High flexibility (size can change during runtime) |
Memory Deallocation | Automatic at program termination | Must be manually deallocated using free() |
Advantages of Static Memory Allocation
- Faster access to memory.
- No need for explicit memory management or deallocation.
- Less prone to memory leaks or errors due to improper memory handling.
Advantages of Dynamic Memory Allocation
- More flexible, as memory size can be adjusted during runtime.
- Efficient use of memory, as memory is allocated only when needed.
- Useful for applications where the data size is not known in advance.
Conclusion
Both static and dynamic memory allocation have their advantages and disadvantages. Static memory allocation is simpler, but less flexible, while dynamic memory allocation provides greater flexibility, but requires careful management of memory to prevent leaks. Understanding the differences between the two types of memory allocation helps in making the right choice based on the needs of your program.
Common Errors in Dynamic Memory Allocation
Dynamic memory allocation allows for flexible and efficient memory usage, but it can introduce several common errors if not handled properly. Below are the most common errors encountered while working with dynamic memory allocation in C, along with explanations and solutions.
1. Memory Leak
A memory leak occurs when dynamically allocated memory is not deallocated properly using the free()
function. This results in memory being "lost" and unavailable for future use, leading to reduced system performance and, in some cases, a program crash.
Example of Memory Leak:
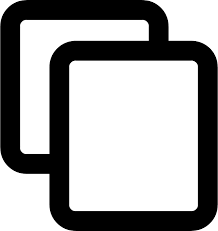
int *arr = (int *)malloc(10 * sizeof(int));
// Memory is allocated but never freed, causing a memory leak.
Solution: Always ensure that memory allocated dynamically is properly deallocated using the free()
function when it is no longer needed.
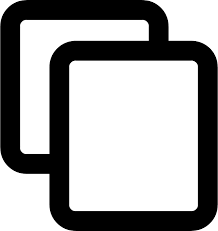
free(arr); // Properly deallocating memory
2. Dangling Pointer
A dangling pointer occurs when a pointer points to a memory location that has been deallocated. Accessing or dereferencing a dangling pointer leads to undefined behavior and can cause program crashes.
Example of Dangling Pointer:
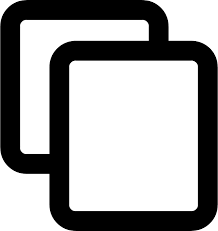
int *arr = (int *)malloc(10 * sizeof(int));
free(arr);
// arr is now a dangling pointer and should not be used.
Solution: After freeing memory, it is a good practice to set the pointer to NULL
to avoid referencing freed memory.
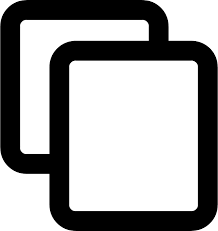
free(arr);
arr = NULL; // Prevents dangling pointer
3. Double Free
Double free occurs when the free()
function is called on the same memory location more than once. This can lead to memory corruption, crashes, and unpredictable behavior.
Example of Double Free:
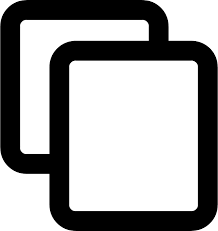
int *arr = (int *)malloc(10 * sizeof(int));
free(arr);
free(arr); // Double free error
Solution: Avoid calling free()
on the same pointer more than once. After freeing memory, set the pointer to NULL
to prevent double freeing.
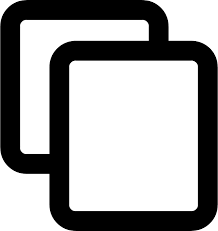
free(arr);
arr = NULL; // Ensures no double free
4. Memory Fragmentation
Memory fragmentation occurs when free memory is split into small, non-contiguous blocks. As a result, it becomes increasingly difficult to allocate large contiguous blocks of memory, leading to inefficient memory usage.
Solution: To avoid memory fragmentation, use memory allocation functions efficiently and try to allocate larger blocks of memory at once. Additionally, reallocate memory carefully using realloc()
.
5. Allocating Insufficient Memory
Allocating insufficient memory can lead to buffer overflows, memory corruption, and undefined behavior. It occurs when the amount of memory requested is too small for the intended data.
Example of Insufficient Memory Allocation:
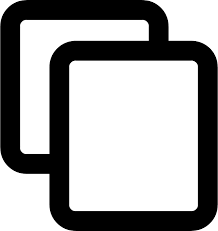
int *arr = (int *)malloc(5 * sizeof(int));
// Allocates space for only 5 integers, but the program may try to store more.
Solution: Ensure the correct size is calculated and allocated based on the data being stored. Always check that the allocation is successful by verifying the pointer is not NULL
.
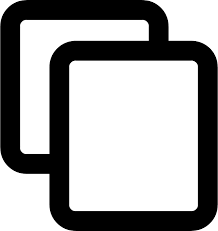
int *arr = (int *)malloc(10 * sizeof(int));
if (arr == NULL) {
// Handle memory allocation failure
}
6. Failure to Check Memory Allocation Success
It is important to check whether memory allocation was successful. If malloc()
, calloc()
, or realloc()
fails, they return NULL
, and attempting to dereference a NULL
pointer leads to crashes or undefined behavior.
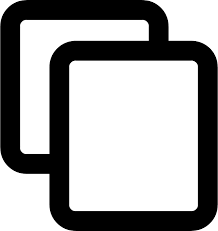
Example of Failure to Check Allocation Success:
int *arr = (int *)malloc(10 * sizeof(int));
arr[0] = 1; // Unsafe if malloc failed and arr is NULL
Solution: Always check that the pointer returned by memory allocation functions is not NULL
before using it.
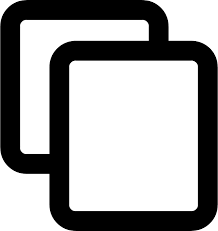
int *arr = (int *)malloc(10 * sizeof(int));
if (arr == NULL) {
// Handle allocation failure
printf("Memory allocation failed\n");
exit(1);
}
7. Incorrect Use of Realloc
The realloc()
function is used to resize a previously allocated block of memory. However, if not used carefully, it can cause memory leaks or undefined behavior. If realloc()
fails, it returns NULL
, and the original memory block is not freed.
Example of Incorrect Use of Realloc:
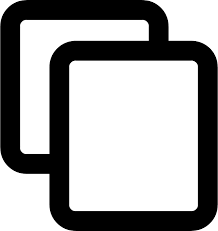
int *arr = (int *)malloc(10 * sizeof(int));
arr = realloc(arr, 20 * sizeof(int));
// If realloc fails, arr will be NULL, and the original memory is leaked.
Solution: Always assign the result of realloc()
to a temporary pointer and check if realloc was successful before overwriting the original pointer.
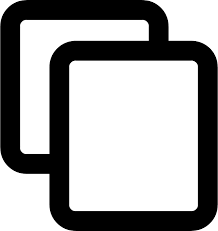
int *temp = realloc(arr, 20 * sizeof(int));
if (temp == NULL) {
// Handle realloc failure
printf("Reallocation failed\n");
free(arr);
exit(1);
} else {
arr = temp;
}
Conclusion
Dynamic memory allocation provides flexibility but requires careful management to avoid errors. By understanding and addressing the common errors mentioned above, you can write more efficient and error-free programs. Always ensure memory is allocated, used, and deallocated correctly to prevent memory leaks, crashes, and undefined behavior.
Command-Line Arguments
Command-line arguments allow you to pass external inputs to a C program when it is executed. These arguments can be used to modify the behavior of the program based on user input from the command line, enabling more flexibility and user control.
What are Command-Line Arguments?
Command-line arguments are values or options provided to a program when it is run from the command line (or terminal). In C, these arguments are passed to the main()
function through parameters argc
and argv
.
Syntax of Command-Line Arguments
In C, the main()
function can be defined as:
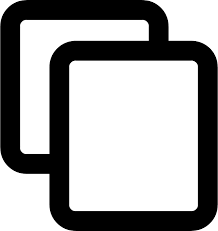
int main(int argc, char *argv[]) {
Here, argc
is the argument count, which represents the number of arguments passed to the program, including the program name. argv
is an array of strings (character pointers), each storing a command-line argument.
How to Use Command-Line Arguments
You can access the arguments passed to your program by using argv
array and its index. The first argument (argv[0]
) is always the name of the program itself, and the remaining arguments are the ones passed by the user.
Example of Command-Line Arguments
Here is a simple example demonstrating how to use command-line arguments in C:
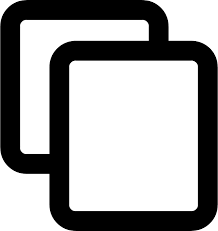
#include <stdio.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Please provide at least one argument.\n");
return 1;
}
printf("Program name: %s\n", argv[0]);
printf("First argument: %s\n", argv[1]);
printf("Second argument: %s\n", argv[2]);
return 0;
}
In this example, the program prints the name of the program and the first two arguments passed from the command line. If fewer than two arguments are provided, it displays a message requesting the user to provide one.
Running the Program
To compile and run this program, use the following commands:
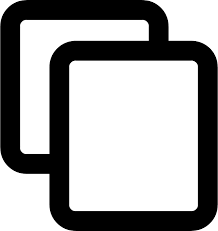
gcc -o example program.c
./example Hello World
The output will be:
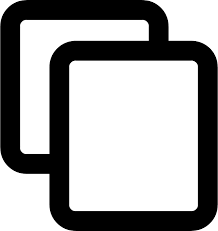
Program name: ./example
First argument: Hello
Second argument: World
Handling Multiple Arguments
To handle multiple arguments, you can loop through the argv
array, using argc
to determine how many arguments were passed.
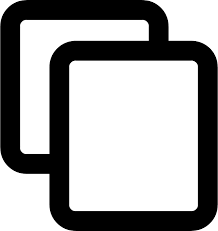
#include <stdio.h>
int main(int argc, char *argv[]) {
printf("Total number of arguments: %d\n", argc);
for (int i = 0; i < argc; i++) {
printf("Argument %d: %s\n", i, argv[i]);
}
return 0;
}
Running this program with the following command:
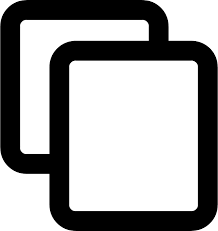
./example argument1 argument2 argument3
Would produce the output:
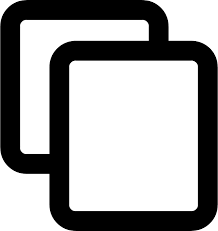
Total number of arguments: 4
Argument 0: ./example
Argument 1: argument1
Argument 2: argument2
Argument 3: argument3
Common Use Cases of Command-Line Arguments
- File Handling: Command-line arguments are often used to pass filenames to the program for reading or writing.
- Flags and Options: Programs can accept flags or options (e.g.,
-h
for help) to modify their behavior. - User Input: Instead of prompting the user inside the program, arguments can be passed when executing the program to provide input data.
Conclusion
Command-line arguments are a powerful feature in C that allows for user interaction with a program without modifying the code. They enable flexible and dynamic behavior for programs, making them more versatile and user-friendly. Always ensure to check argc
to handle missing arguments and avoid errors in your program.
Preprocessor Directives (#define, #include, #ifdef)
Preprocessor directives are special instructions to the C compiler that are processed before the actual compilation begins. These directives are used for various purposes such as defining constants, including header files, and conditional compilation. They are identified by the #
symbol at the beginning of the line.
1. #define Directive
The #define
directive is used to define a constant value or a macro in a program. It replaces occurrences of a specified identifier with a defined value or expression.
- Syntax:
#define name value
- Example:
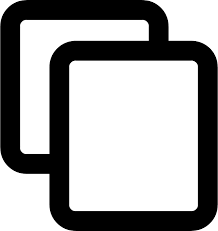
#define PI 3.14159
#define SQUARE(x) ((x) * (x))
In this example, PI
is defined as a constant, and SQUARE(x)
is a macro that returns the square of x
.
Using Defined Constants
Once defined, the constant can be used throughout the program:
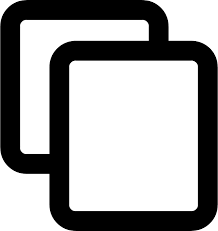
#include <stdio.h>
#define PI 3.14159
int main() {
printf("Value of PI: %f\n", PI);
return 0;
}
This will output:
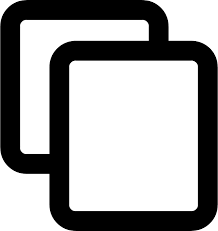
Value of PI: 3.141590
2. #include Directive
The #include
directive is used to include external files, typically header files, into the program. It helps to organize the code and reuse functions, constants, and other declarations across multiple files.
- Syntax:
#include <header_file>
(for system libraries) or#include "file_name"
(for user-defined header files). - Example:
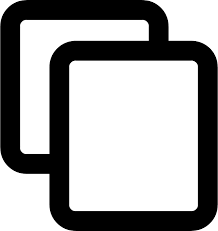
#include <stdio.h>
#include "myheader.h"
The #include <stdio.h>
includes the standard input/output library, while #include "myheader.h"
includes a user-defined header file.
Common Header Files
<stdio.h>
: Standard input and output functions (e.g.,printf
,scanf
)<stdlib.h>
: Memory allocation, process control, and conversion functions<math.h>
: Mathematical functions (e.g.,sin
,cos
,sqrt
)
3. #ifdef Directive
The #ifdef
directive is used for conditional compilation. It allows the compiler to include or exclude parts of the code based on whether a specific macro or constant is defined.
- Syntax:
- Example:
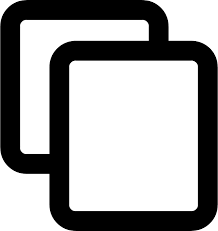
#ifdef macro_name
// Code to include if macro is defined
#endif
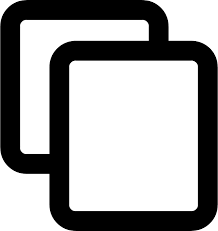
#define DEBUG
#ifdef DEBUG
printf("Debugging enabled!\n");
#endif
If DEBUG
is defined, the code inside the #ifdef
block will be included during compilation. If it's not defined, the code inside the block will be ignored.
Conditional Compilation
Preprocessor directives like #ifdef
are useful for debugging, platform-specific code, or any situation where certain parts of the code should be included only under specific conditions.
Other Conditional Directives
There are other related directives that help with conditional compilation:
#ifndef
: Checks if a macro is not defined.#else
: Specifies an alternative block of code if the#ifdef
condition is false.#endif
: Ends an#if
or#ifdef
block.
Example of Combined Conditional Directives
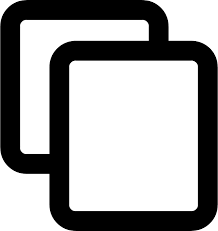
#define VERSION 2
#ifdef VERSION
#if VERSION == 1
printf("Version 1\n");
#elif VERSION == 2
printf("Version 2\n");
#else
printf("Unknown version\n");
#endif
#endif
Conclusion
Preprocessor directives in C provide powerful mechanisms to define constants, include files, and manage conditional compilation. By using directives like #define
, #include
, and #ifdef
, you can write more maintainable, modular, and flexible code that can be easily adapted to different environments or debugging scenarios.
Working with Macros
In C programming, macros are preprocessor directives that are used to define constants, expressions, or blocks of code that can be reused multiple times throughout a program. They are defined using the #define
directive and are typically used for making code more readable, maintainable, and efficient.
What is a Macro?
A macro is essentially a rule that tells the preprocessor to replace a specific identifier with a value or expression before the program is compiled. Macros can be used to define constants or create inline functions or code snippets that are reused throughout the program.
Defining Macros
Macros are defined using the #define
directive followed by the name of the macro and its corresponding value or code. The syntax is:
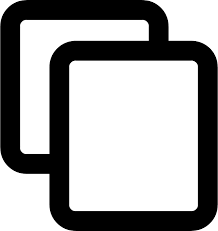
#define macro_name replacement_value
Example:
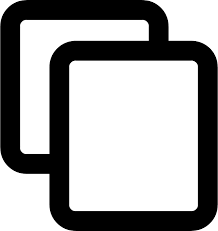
#define PI 3.14159
In this example, every occurrence of the identifier PI
will be replaced with 3.14159
during compilation.
Using Macros in Code
Once defined, macros can be used in the code wherever their name appears. They are replaced by the preprocessor before actual compilation.
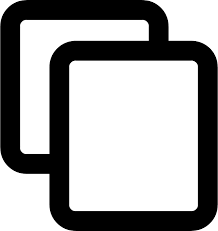
#include <stdio.h>
#define PI 3.14159
int main() {
printf("Value of PI: %f\n", PI);
return 0;
}
This will output:
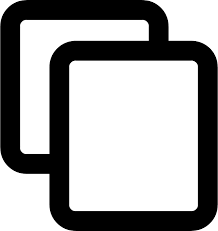
Value of PI: 3.141590
Function-like Macros
Macros can also take arguments, allowing you to define code snippets that act like functions. These are called function-like macros. The syntax for function-like macros is:
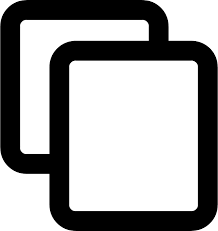
#define macro_name(argument(s)) expression
Example:
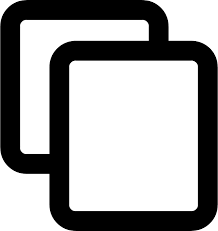
#define SQUARE(x) ((x) * (x))
This macro calculates the square of a number. You can use it like a function, passing arguments to it:
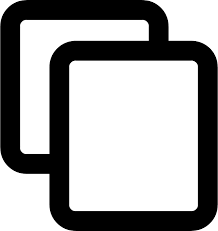
#include <stdio.h>
#define SQUARE(x) ((x) * (x))
int main() {
int num = 5;
printf("Square of %d: %d\n", num, SQUARE(num));
return 0;
}
This will output:
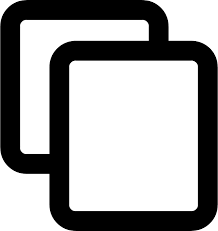
Square of 5: 25
Advantages of Using Macros
- Code Reusability: Macros help avoid repetitive code by allowing code snippets to be reused wherever they are needed.
- Efficiency: Macros are replaced during preprocessing, so there is no performance overhead during runtime.
- Readability: They can make code more readable by using meaningful names instead of repetitive literal values or expressions.
Disadvantages of Using Macros
- Lack of Type Checking: Macros don’t perform type checking, which can lead to unexpected results if the types don’t match.
- Debugging Difficulty: Since macros are replaced before compilation, they can make debugging harder because the code in the debugger may not reflect the original macro code.
- No Scope: Macros do not have variable scope, which means they are globally available and can potentially cause conflicts.
Best Practices for Using Macros
Here are some tips to follow when working with macros:
- Use parentheses: Always surround the entire expression in parentheses when defining function-like macros to avoid precedence issues. For example,
#define SQUARE(x) ((x) * (x))
. - Prefix macro names: Use a naming convention that clearly identifies macros. Prefixing macros with
MACRO_
or similar can help avoid naming conflicts. - Limit macro usage: While macros are useful, overusing them can make the code harder to maintain. Consider using functions when appropriate for better type safety and clarity.
Macro Example: Conditional Compilation
Macros are also commonly used for conditional compilation, where certain parts of the code are included or excluded based on the definition of a macro. This is useful for debugging or platform-specific code.
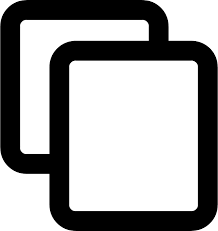
#define DEBUG
#ifdef DEBUG
printf("Debugging mode enabled\n");
#endif
If the DEBUG
macro is defined, the code inside the #ifdef
block will be included. Otherwise, it will be ignored.
Conclusion
Macros are a powerful feature in C programming that allow for code reuse and efficiency. However, they should be used with caution due to their lack of type safety and potential for making debugging more difficult. By following best practices, you can leverage macros effectively in your programs.
Enum and Typedef
In C programming, enum and typedef are two important features that help in improving code readability, maintainability, and organization. The enum
type is used to define a set of named integer constants, while the typedef
keyword allows you to create new names (aliases) for existing data types.
What is Enum?
An enum (short for enumerated type) is a data type that allows you to define a variable that can only have one of a set of predefined constant values. This is useful when you want to represent options like days of the week, months, or any other collection of related values.
Syntax of Enum
The syntax for defining an enum is as follows:
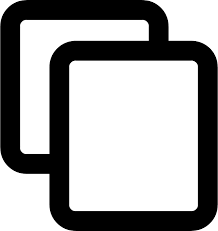
enum enum_name {
value1,
value2,
value3,
...
};
Example:
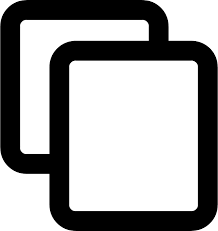
enum day { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday };
In this example, the enum
named day
represents the days of the week, where Sunday
has a value of 0, Monday
has a value of 1, and so on. By default, the first value is assigned 0, and the following values are incremented by 1.
Using Enum
You can declare a variable of an enum type, and assign one of the predefined values to it. Example:
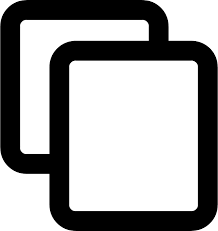
enum day today;
today = Wednesday;
Here, the variable today
is of type enum day
, and the value assigned is Wednesday
.
Assigning Custom Values to Enum Constants
By default, enum values start from 0 and increase by 1, but you can assign custom values to enum constants:
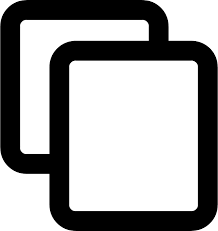
enum day { Sunday = 1, Monday = 5, Tuesday = 10, Wednesday = 15, Thursday = 20, Friday = 25, Saturday = 30 };
In this case, Sunday
is assigned the value 1, Monday
is assigned 5, and so on.
What is Typedef?
typedef is a keyword in C that is used to create new data type names (aliases) for existing types. This can make complex type declarations more readable and easier to manage. It’s commonly used with structs, pointers, and function pointers.
Syntax of Typedef
The syntax for defining a typedef is as follows:
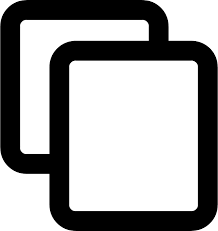
typedef existing_type new_type_name;
Example:
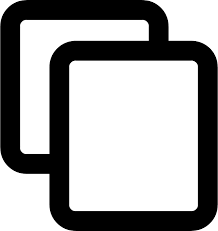
typedef unsigned long ulong;
In this example, ulong
is now an alias for unsigned long
. You can now use ulong
in place of unsigned long
throughout your program.
Using Typedef
Once you have defined a typedef, you can use the new name as if it were a regular data type:
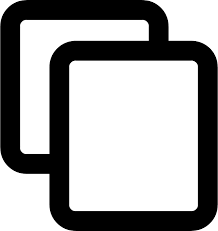
ulong num1, num2; // Equivalent to unsigned long num1, num2;
Typedef with Structures
Typedef is particularly useful when working with structures. When you define a structure, you often need to use the struct
keyword every time you declare a variable. Using typedef
, you can avoid this repetition:
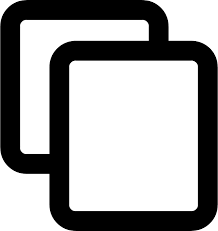
typedef struct {
int x;
int y;
} Point;
Now you can declare variables of type Point
without needing to use the struct
keyword:
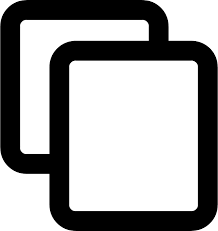
Point p1, p2;
Typedef with Pointers
Typedef can also be used to create aliases for pointers, making pointer declarations more readable:
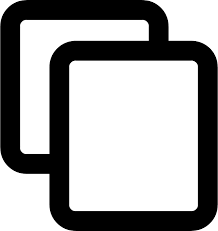
typedef int* IntPtr;
IntPtr ptr1, ptr2; // Equivalent to int* ptr1, ptr2;
Typedef with Function Pointers
Another powerful use of typedef
is with function pointers. It allows you to define a type for a function pointer, making it easier to use:
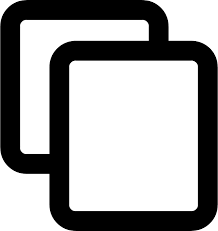
typedef int (*MathFunction)(int, int);
MathFunction add, subtract;
In this example, MathFunction
is now a type for function pointers that point to functions taking two int
arguments and returning an int
.
Differences Between Enum and Typedef
- Purpose:
enum
is used to define a set of named constants, whiletypedef
is used to create an alias for an existing data type. - Functionality:
enum
helps in defining a type with a predefined set of values, making code more readable, whereastypedef
simplifies type declarations.
Conclusion
enum
and typedef
are powerful tools in C programming that help you make your code more readable and maintainable. enum
is useful when you want to define a set of named constants, while typedef
helps in creating type aliases for existing data types to simplify code.
The Volatile Keyword in C
The volatile keyword in C is a type qualifier used to indicate that a variable’s value may be changed unexpectedly, outside of the program's control, such as by hardware or a concurrent thread. The volatile
keyword tells the compiler to avoid optimizing the variable, ensuring that the program always reads the actual value of the variable from memory instead of using a cached or optimized version.
What is the Volatile Keyword?
The volatile keyword is primarily used for variables that can be modified by external factors, such as:
- Hardware registers
- Interrupt service routines (ISRs)
- Shared memory in multithreaded programs
When the compiler detects a volatile
variable, it ensures that every read or write to that variable happens directly from or to memory, avoiding any caching or optimization techniques that could lead to incorrect results.
Why Use Volatile?
In certain situations, the value of a variable can be modified outside the normal program flow. For example, in embedded systems, hardware might update a memory-mapped register, or an interrupt may update a variable while the program is running. If the compiler does not know about these changes, it might optimize the code incorrectly, leading to unexpected behavior.
By using the volatile
keyword, you inform the compiler that the value of the variable can change at any time, ensuring that every access to the variable is done in real-time.
Syntax of Volatile
The syntax for declaring a volatile variable is as follows:
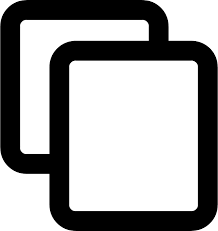
volatile data_type variable_name;
Example:
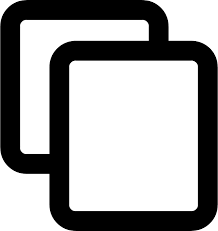
volatile int sensorData;
In this example, sensorData
is a variable that can be modified by external sources (e.g., hardware or an interrupt service routine), and the compiler will not optimize it.
When to Use Volatile?
Some typical situations where you might use the volatile
keyword include:
- Memory-mapped I/O: When working with hardware registers that can change independently of the program flow.
- Interrupts: When a variable is modified inside an interrupt service routine (ISR), the compiler may optimize the variable access, leading to inconsistent values if
volatile
is not used. - Multithreading: When shared variables are accessed from different threads or processes, marking a variable as
volatile
ensures that each thread sees the latest value.
Example of Volatile in Interrupts
In embedded systems programming, an interrupt service routine (ISR) may update a flag that the main program uses. Without volatile
, the compiler might optimize the flag check, causing the main program to miss the update.
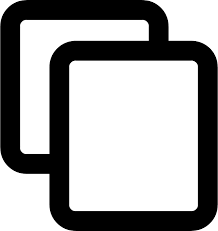
volatile int interruptFlag = 0;
void ISR() {
interruptFlag = 1; // ISR sets the flag
}
int main() {
while (1) {
if (interruptFlag) {
// Handle interrupt
interruptFlag = 0;
}
}
}
In this example, the interruptFlag
is marked as volatile
to ensure that the main program always checks the actual value set by the interrupt service routine.
Example of Volatile with Hardware Registers
In embedded systems, hardware registers are often mapped to specific memory locations. These registers can change independently of the program’s flow, and the volatile
keyword ensures that the compiler does not optimize accesses to these registers.
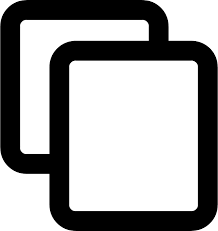
#define STATUS_REGISTER (*(volatile unsigned int *)0x40000000)
int main() {
while (1) {
if (STATUS_REGISTER & 0x01) {
// Handle some condition based on hardware status
}
}
}
In this example, STATUS_REGISTER
refers to a hardware register, and using volatile
ensures that the compiler always reads its latest value from memory.
Volatile vs Const
The volatile
keyword differs from const
in that const
tells the compiler that the variable’s value should not be modified by the program, while volatile
tells the compiler that the variable’s value may change at any time due to external factors.
For example, a const
variable cannot be modified by the program, while a volatile
variable can be modified, but the compiler must always fetch its value from memory.
Common Pitfalls
- Overusing Volatile: While
volatile
is useful, overusing it can lead to inefficient code. It should only be used when necessary, such as when working with hardware or multi-threaded applications. - Misunderstanding Volatile: The
volatile
keyword does not make a variable thread-safe. It simply prevents the compiler from optimizing it, but proper synchronization mechanisms (like mutexes) are still needed in multi-threaded programs.
Conclusion
The volatile keyword is an important tool in C programming, especially when working with embedded systems, hardware programming, interrupts, and multi-threaded applications. It ensures that the compiler does not optimize the access to variables whose values can change at any time due to factors outside the program’s control.
Arrays as Data Structures
Arrays are one of the most fundamental and commonly used data structures in C. An array is a collection of elements, all of the same type, stored in contiguous memory locations. Arrays provide an efficient way to store and access data, especially when the size of the data is known in advance. They are widely used to represent data in a structured way, making them a key component in many algorithms and applications.
What is an Array?
An array in C is a data structure that can hold a fixed number of values, all of the same type, in a contiguous block of memory. Each element in an array is identified by an index, which is used to access its value. The index in C arrays starts at 0, meaning the first element is accessed using index 0, the second element with index 1, and so on.
Array Declaration and Initialization
In C, arrays are declared by specifying the type of elements, followed by the array name, and the size (number of elements) in square brackets. Here's an example of declaring and initializing an array:
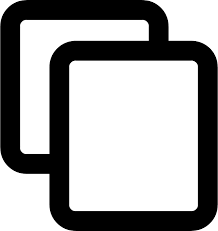
int arr[5] = {1, 2, 3, 4, 5};
This declares an array of integers with 5 elements and initializes it with values. If not explicitly initialized, the array elements will contain garbage values.
Advantages of Using Arrays
- Efficient Memory Usage: Arrays use contiguous memory locations, making them highly efficient for accessing elements based on their index.
- Random Access: Arrays allow random access to elements, meaning that any element can be accessed directly using its index in constant time.
- Simplicity: Arrays are simple to implement and use, making them easy to work with in most applications.
- Cache Friendliness: Since arrays store elements in contiguous memory locations, they are cache-friendly, making them faster for accessing elements in sequence.
Disadvantages of Using Arrays
- Fixed Size: Once an array is declared with a size, its size cannot be changed. This can be limiting in situations where the number of elements is not known in advance.
- Memory Waste: If an array is larger than necessary, it can lead to wasted memory. Conversely, if it is too small, it may not be able to hold all the required elements.
- Difficulty in Insertion/Deletion: Inserting or deleting elements in an array is not as straightforward as in other data structures like linked lists, as it may require shifting elements to maintain continuity.
Types of Arrays
- One-dimensional Arrays: A simple array containing a single row of elements. It is the most basic form of array.
- Two-dimensional Arrays: Arrays containing rows and columns, often used to represent matrices or tables.
- Multi-dimensional Arrays: Arrays that have more than two dimensions, used for complex data representations such as 3D matrices.
Example of Using Arrays
Here’s an example of how you might use an array in C to store and print values:
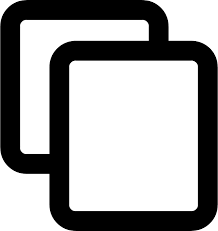
#include
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
// Accessing and printing array elements
for (int i = 0; i < 5; i++) {
printf("Element at index %d: %d\n", i, numbers[i]);
}
return 0;
}
This program declares an array of 5 integers, initializes it with values, and then prints each element using a for loop.
Arrays as Data Structures
Arrays can be considered as a basic data structure because they provide a means of storing and organizing data. As a data structure, arrays are used in a variety of applications, including:
- Storing Lists: Arrays are ideal for storing lists of items, such as a list of numbers, strings, or other objects, when the size of the list is known.
- Representing Matrices: Arrays are used to represent matrices in mathematical computations, which can be useful for applications in scientific computing, graphics, and simulations.
- Implementing Other Data Structures: Arrays are used as building blocks for more complex data structures such as stacks, queues, heaps, and hash tables.
Memory Layout of Arrays
In C, arrays are stored in contiguous memory locations, meaning that the elements are placed one after another in memory. The first element of the array is at the base address, and each subsequent element is placed in the next memory slot. This allows for efficient access to array elements using their indices.
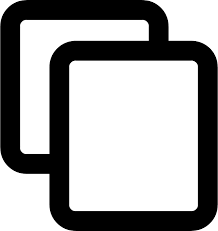
int arr[5] = {10, 20, 30, 40, 50};
The memory layout of the array could look something like this:
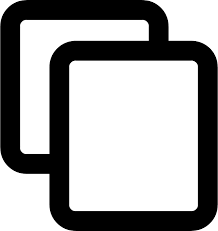
arr[0] -> 10
arr[1] -> 20
arr[2] -> 30
arr[3] -> 40
arr[4] -> 50
Conclusion
Arrays are a fundamental data structure in C that provide an efficient way to store and access data. While they have some limitations, such as fixed size and difficulty in insertion or deletion, their benefits in terms of memory efficiency, random access, and simplicity make them essential for many applications. Understanding arrays is crucial for becoming proficient in C programming and for working with more advanced data structures.
Linked Lists (Singly, Doubly, Circular)
A linked list is a linear data structure where elements (known as nodes) are stored in non-contiguous memory locations. Each node contains a data element and a reference (or link) to the next node in the sequence. Linked lists offer dynamic memory allocation, making them more flexible than arrays. They are widely used in situations where the size of the data structure is not known in advance, or where frequent insertion and deletion operations are required.
Types of Linked Lists
- Singly Linked List: In a singly linked list, each node contains a data element and a reference to the next node. The last node points to null, indicating the end of the list.
- Doubly Linked List: In a doubly linked list, each node contains a data element, a reference to the next node, and a reference to the previous node, allowing traversal in both directions.
- Circular Linked List: A circular linked list is similar to a singly or doubly linked list, but the last node points back to the first node instead of null, creating a circular structure.
Singly Linked List
A singly linked list is the simplest type of linked list. It consists of nodes, where each node contains two parts: the data element and a reference to the next node. The list has a head pointer that points to the first node, and the last node points to null to mark the end of the list.
Structure of a Singly Linked List Node
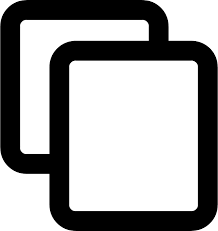
struct Node {
int data;
struct Node* next;
};
Basic Operations in Singly Linked List
- Insertion: Adding a new node to the list, either at the beginning, at the end, or at a specific position.
- Deletion: Removing a node from the list, either from the beginning, the end, or a specific position.
- Traversal: Visiting each node in the list to display its data.
Doubly Linked List
A doubly linked list is more complex than a singly linked list. Each node contains three parts: the data element, a reference to the next node, and a reference to the previous node. This allows for traversal in both directions (forward and backward).
Structure of a Doubly Linked List Node
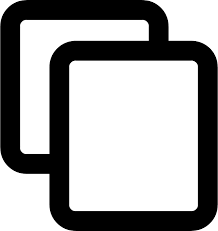
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
Basic Operations in Doubly Linked List
- Insertion: Adding a new node at the beginning, the end, or at a specific position.
- Deletion: Removing a node from the list, either from the beginning, the end, or a specific position.
- Traversal: Traversing the list in both directions: from the beginning (head to tail) or from the end (tail to head).
Circular Linked List
A circular linked list is a variation of a singly or doubly linked list where the last node points back to the first node, creating a circular structure. This makes it easier to perform operations like looping through the list repeatedly without needing to reset the pointer to the head.
Structure of a Circular Linked List Node
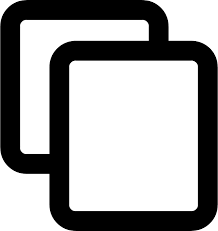
struct Node {
int data;
struct Node* next;
};
In a circular singly linked list, the last node’s next pointer points to the head node instead of null. In a circular doubly linked list, both the next pointer of the last node and the previous pointer of the first node point to each other.
Basic Operations in Circular Linked List
- Insertion: Adding a new node to the list, either at the beginning, at the end, or anywhere in the circular structure.
- Deletion: Removing a node from the list, either from the beginning, the end, or a specific position.
- Traversal: Traversing the circular list, which can be done repeatedly, as the last node points back to the first node.
Advantages of Linked Lists
- Dynamic Size: Linked lists do not require a predefined size, making them more flexible than arrays in terms of memory allocation.
- Efficient Insertions/Deletions: Inserting or deleting a node in a linked list is more efficient than in arrays, especially for large datasets, as no shifting of elements is required.
- Memory Efficient: Linked lists allocate memory dynamically as nodes are added, unlike arrays, which may require preallocating a fixed amount of memory.
Disadvantages of Linked Lists
- Extra Memory for Pointers: Each node in a linked list requires extra memory for storing pointers (next and possibly previous), which can be inefficient for small datasets.
- Sequential Access: Accessing elements in a linked list requires traversing the list from the head to the desired node, which can be slower than array-based access.
- Complexity: Linked lists are more complex to implement and manage than arrays, especially when it comes to pointer manipulation.
Example Code for Singly Linked List
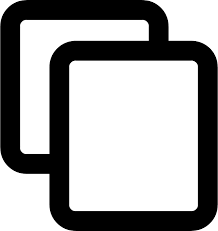
#include
#include
struct Node {
int data;
struct Node* next;
};
// Function to print the linked list
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
// Allocate memory for 3 nodes
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
// Assign data values and link nodes
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
printList(head); // Output: 1 -> 2 -> 3 -> NULL
return 0;
}
Conclusion
Linked lists are a versatile and powerful data structure that can be used in various scenarios where dynamic data storage and efficient insertion/deletion operations are required. Understanding the differences between singly, doubly, and circular linked lists, as well as their advantages and limitations, is essential for becoming proficient in C programming and for solving complex problems efficiently.
Stacks and Queues
Stacks and queues are both fundamental data structures used to store and manage data in a specific order. They are linear data structures, meaning that their elements are arranged in a sequential manner. The difference between the two lies in the order in which elements are added and removed.
Stacks
A stack is a collection of elements that follows the LIFO (Last In, First Out) principle. This means that the last element added to the stack is the first one to be removed. It operates similarly to a stack of plates, where you add a plate to the top and also remove a plate from the top.
Basic Operations of a Stack
- Push: Adds an element to the top of the stack.
- Pop: Removes the element from the top of the stack.
- Peek/Top: Returns the element at the top without removing it from the stack.
- isEmpty: Checks whether the stack is empty.
- Size: Returns the number of elements in the stack.
Implementation of a Stack
Stacks can be implemented using arrays or linked lists. Here is a basic stack implementation using an array:
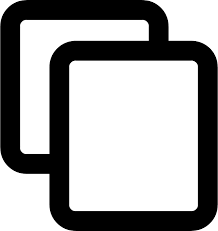
#include
#define MAX 5
int stack[MAX];
int top = -1;
// Push operation
void push(int value) {
if (top == MAX - 1) {
printf("Stack Overflow\n");
} else {
stack[++top] = value;
printf("%d pushed to stack\n", value);
}
}
// Pop operation
int pop() {
if (top == -1) {
printf("Stack Underflow\n");
return -1;
} else {
int poppedValue = stack[top--];
return poppedValue;
}
}
// Peek operation
int peek() {
if (top == -1) {
printf("Stack is empty\n");
return -1;
} else {
return stack[top];
}
}
int main() {
push(10);
push(20);
push(30);
printf("Top element: %d\n", peek());
printf("%d popped from stack\n", pop());
printf("%d popped from stack\n", pop());
return 0;
}
Queues
A queue is a collection of elements that follows the FIFO (First In, First Out) principle. This means that the first element added to the queue will be the first one to be removed. It operates similarly to a queue at a ticket counter, where the first person in line is the first to be served.
Basic Operations of a Queue
- Enqueue: Adds an element to the end (rear) of the queue.
- Dequeue: Removes an element from the front of the queue.
- Front: Returns the element at the front of the queue without removing it.
- Rear: Returns the element at the rear of the queue.
- isEmpty: Checks whether the queue is empty.
- Size: Returns the number of elements in the queue.
Implementation of a Queue
Queues can be implemented using arrays or linked lists. Here is a basic queue implementation using an array:
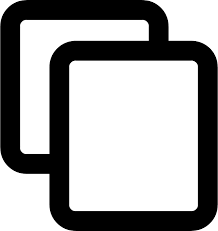
#include
#define MAX 5
int queue[MAX];
int front = -1, rear = -1;
// Enqueue operation
void enqueue(int value) {
if (rear == MAX - 1) {
printf("Queue Overflow\n");
} else {
if (front == -1) { // If queue is empty
front = 0;
}
queue[++rear] = value;
printf("%d enqueued to queue\n", value);
}
}
// Dequeue operation
int dequeue() {
if (front == -1 || front > rear) {
printf("Queue Underflow\n");
return -1;
} else {
int dequeuedValue = queue[front++];
return dequeuedValue;
}
}
// Front operation
int frontElement() {
if (front == -1 || front > rear) {
printf("Queue is empty\n");
return -1;
} else {
return queue[front];
}
}
int main() {
enqueue(10);
enqueue(20);
enqueue(30);
printf("Front element: %d\n", frontElement());
printf("%d dequeued from queue\n", dequeue());
printf("%d dequeued from queue\n", dequeue());
return 0;
}
Types of Queues
- Simple Queue: The basic form of a queue where elements are added at the rear and removed from the front.
- Circular Queue: A type of queue where the last element is connected back to the first element, making it a circular structure. This helps in efficiently utilizing the space in the queue.
- Priority Queue: A type of queue where each element is associated with a priority. Elements with higher priority are dequeued before elements with lower priority, regardless of their order in the queue.
Applications of Stacks and Queues
- Stacks: Used in function calls (call stack), undo operations in editors, syntax parsing, and reversing data.
- Queues: Used in scheduling tasks (like CPU scheduling), handling requests in a queue, breadth-first search (BFS) in graphs, and buffering data (e.g., printer queues).
Advantages and Disadvantages
Stacks
- Advantages: Simple to implement, efficient for LIFO operations, and useful in recursion and backtracking problems.
- Disadvantages: Fixed size (if implemented using arrays), limited access (only the top element can be accessed). Stack overflow/underflow can occur.
Queues
- Advantages: Simple to implement, efficient for FIFO operations, and useful in scheduling and buffering.
- Disadvantages: Fixed size (if implemented using arrays), limited access (only front and rear elements can be accessed). Queue overflow/underflow can occur.
Conclusion
Stacks and queues are essential data structures used in many programming tasks. Stacks are ideal for problems that require LIFO ordering, such as function calls and backtracking. Queues are useful for FIFO ordering, such as task scheduling and buffering. Mastering these data structures is crucial for solving a wide range of computational problems efficiently.
Trees (Binary Tree, Binary Search Tree)
A tree is a hierarchical data structure consisting of nodes connected by edges. It is widely used in various computer science applications such as databases, file systems, and searching algorithms. In this section, we will cover two fundamental types of trees: Binary Tree and Binary Search Tree.
Binary Tree
A Binary Tree is a tree where each node has at most two children referred to as the left child and the right child. The top node is called the root, and nodes with no children are called leaf nodes.
Basic Terminology in Binary Tree
- Root: The topmost node in the tree.
- Parent: A node that has one or more children.
- Child: A node that is a descendant of another node.
- Leaf: A node with no children.
- Subtree: A tree that is part of a larger tree.
- Level: The level of a node is defined by the number of edges from the root to the node.
Representation of Binary Tree
A binary tree can be represented using a structure in C. Here's a basic implementation for a binary tree node:
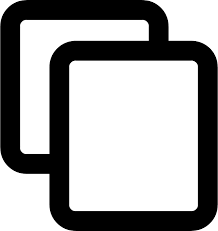
#include
#include
// Structure for a binary tree node
struct Node {
int data;
struct Node* left;
struct Node* right;
};
// Function to create a new node
struct Node* newNode(int data) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->left = node->right = NULL;
return node;
}
// Inorder traversal of the tree
void inorder(struct Node* root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
int main() {
struct Node* root = newNode(1);
root->left = newNode(2);
root->right = newNode(3);
root->left->left = newNode(4);
root->left->right = newNode(5);
printf("Inorder Traversal: ");
inorder(root);
return 0;
}
Binary Search Tree (BST)
A Binary Search Tree (BST) is a binary tree with the following property: for each node, the value of the left child is smaller than the parent node, and the value of the right child is greater than the parent node. This property makes BSTs very efficient for searching, insertion, and deletion operations.
Basic Operations on a BST
- Insertion: Insert a new node into the tree while maintaining the BST property.
- Deletion: Remove a node from the tree and restructure the tree to maintain the BST property.
- Searching: Find a node with a specific value.
- Inorder Traversal: Visit the nodes in ascending order.
Implementation of a Binary Search Tree
The following code demonstrates basic operations such as insertion and inorder traversal in a Binary Search Tree:
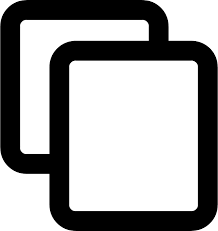
#include
#include
struct Node {
int data;
struct Node* left;
struct Node* right;
};
// Function to create a new node
struct Node* newNode(int data) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->left = node->right = NULL;
return node;
}
// Function to insert a node in a BST
struct Node* insert(struct Node* root, int data) {
if (root == NULL) {
return newNode(data);
}
if (data < root->data) {
root->left = insert(root->left, data);
} else if (data > root->data) {
root->right = insert(root->right, data);
}
return root;
}
// Inorder traversal of the BST
void inorder(struct Node* root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
int main() {
struct Node* root = NULL;
root = insert(root, 50);
insert(root, 30);
insert(root, 20);
insert(root, 40);
insert(root, 70);
insert(root, 60);
insert(root, 80);
printf("Inorder Traversal: ");
inorder(root);
return 0;
}
Advantages of Binary Search Tree
- Efficient Searching: The binary search property allows for fast searching, with a time complexity of O(log n) in average cases.
- Efficient Insertion/Deletion: Insertion and deletion operations can also be done in O(log n) time on average.
- Ordered Data: Inorder traversal of a BST gives the data in sorted order.
Disadvantages of Binary Search Tree
- Unbalanced Trees: If the tree becomes unbalanced (e.g., when nodes are inserted in sorted order), the time complexity for search, insertion, and deletion may degrade to O(n), where n is the number of nodes in the tree.
- Balancing Issues: Maintaining a balanced tree requires additional algorithms such as AVL trees or Red-Black trees.
Applications of Trees
- Searching: Used in search engines, database indexing, and file systems.
- Sorting: Used in sorting algorithms like tree sort.
- Decision Making: Used in decision trees, which are used in artificial intelligence and machine learning.
- Expression Parsing: Used for parsing expressions in compilers (e.g., syntax trees).
Conclusion
Trees, especially Binary Trees and Binary Search Trees, are critical data structures in computer science. They provide efficient ways for storing and organizing data and are widely used in various applications such as databases, search engines, and AI. Understanding binary trees and BSTs helps in solving problems related to searching, sorting, and decision-making efficiently.
Graphs (Adjacency Matrix, List)
A graph is a non-linear data structure that consists of a set of vertices (or nodes) connected by edges. Graphs can represent various real-world problems such as social networks, maps, and web pages. In this section, we will focus on two representations of graphs: the Adjacency Matrix and the Adjacency List.
Introduction to Graphs
Graphs are classified into two types based on the direction of edges:
- Directed Graphs (Digraphs): The edges have a direction, i.e., they go from one vertex to another.
- Undirected Graphs: The edges do not have a direction; they simply connect two vertices.
Basic Terminology
- Vertex (Node): A fundamental unit of the graph that holds data.
- Edge: A connection between two vertices.
- Degree of a vertex: The number of edges connected to it.
- Path: A sequence of vertices where each adjacent pair is connected by an edge.
- Cycle: A path that starts and ends at the same vertex, without repeating any edges or vertices.
Adjacency Matrix
The Adjacency Matrix is a 2D array used to represent a graph. If the graph has V
vertices, the matrix will be of size V × V
. The matrix stores a value indicating whether two vertices are connected or not.
Properties of Adjacency Matrix
- For an undirected graph, the matrix is symmetric.
- For a directed graph, the matrix is not necessarily symmetric.
- The matrix entry at position
[i][j]
is non-zero (typically 1) if there is an edge from vertexi
to vertexj
, otherwise 0.
Adjacency Matrix Representation in C
Here’s an example of how to represent a graph using an adjacency matrix in C:
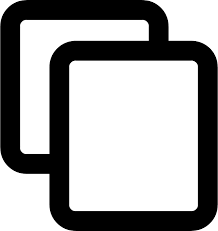
#include
#define V 5 // Number of vertices
// Function to print the adjacency matrix
void printAdjMatrix(int graph[V][V]) {
for (int i = 0; i < V; i++) {
for (int j = 0; j < V; j++) {
printf("%d ", graph[i][j]);
}
printf("\n");
}
}
int main() {
// Example graph represented as an adjacency matrix
int graph[V][V] = {
{0, 1, 0, 0, 1},
{1, 0, 1, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 1, 0, 1},
{1, 0, 0, 1, 0}
};
printf("Adjacency Matrix of the Graph:\n");
printAdjMatrix(graph);
return 0;
}
Adjacency List
An Adjacency List is a more space-efficient way to represent a graph. It consists of an array of lists. Each index in the array represents a vertex, and the list at that index contains the adjacent vertices (i.e., vertices that are directly connected to it).
Properties of Adjacency List
- For an undirected graph, each edge is represented twice—once for each vertex it connects.
- The adjacency list is more space-efficient than an adjacency matrix, especially for sparse graphs.
Adjacency List Representation in C
Here’s an example of how to represent a graph using an adjacency list in C:
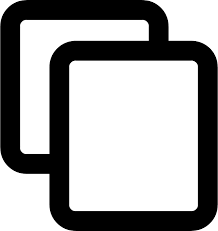
#include
#include
#define V 5 // Number of vertices
// Structure for an adjacency list node
struct Node {
int vertex;
struct Node* next;
};
// Structure for an adjacency list
struct Graph {
struct Node* adjList[V];
};
// Function to create a new adjacency list node
struct Node* newNode(int vertex) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->vertex = vertex;
node->next = NULL;
return node;
}
// Function to create a graph
void createGraph(struct Graph* graph) {
for (int i = 0; i < V; i++) {
graph->adjList[i] = NULL;
}
}
// Function to add an edge to the graph
void addEdge(struct Graph* graph, int src, int dest) {
// Add edge from src to dest
struct Node* node = newNode(dest);
node->next = graph->adjList[src];
graph->adjList[src] = node;
// Add edge from dest to src (for undirected graph)
node = newNode(src);
node->next = graph->adjList[dest];
graph->adjList[dest] = node;
}
// Function to print the adjacency list
void printGraph(struct Graph* graph) {
for (int i = 0; i < V; i++) {
struct Node* temp = graph->adjList[i];
printf("Vertex %d: ", i);
while (temp) {
printf("%d -> ", temp->vertex);
temp = temp->next;
}
printf("NULL\n");
}
}
int main() {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
createGraph(graph);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 3, 4);
printf("Adjacency List of the Graph:\n");
printGraph(graph);
return 0;
}
Comparison Between Adjacency Matrix and Adjacency List
Feature | Adjacency Matrix | Adjacency List |
---|---|---|
Space Complexity | O(V^2) | O(V + E) |
Time Complexity for Checking if Edge Exists | O(1) | O(V) in the worst case |
Time Complexity for Adding an Edge | O(1) | O(1) |
Best for Sparse Graphs | No | Yes |
Best for Dense Graphs | Yes | No |
Applications of Graphs
- Social Networks: Representing users and their relationships.
- Computer Networks: Representing routers and their connections.
- Navigation: Representing maps and routes.
- Recommendation Systems: Representing items and their relationships.
- Web Crawling: Representing web pages and links.
Conclusion
Graphs are versatile data structures used to represent relationships between objects. The choice of representation (adjacency matrix or adjacency list) depends on the type of graph (dense or sparse) and the operations you need to perform. Understanding graphs and their representations is crucial for solving complex problems such as pathfinding, network routing, and social network analysis.
Introduction to Error Handling in C
Error handling is an important concept in programming, as it allows the program to gracefully handle unexpected situations, like invalid user inputs, file errors, memory allocation failures, and many others. In C, error handling is primarily done using return codes, error codes, and the standard library functions. In this section, we'll explore how errors can be handled in C and what tools are available to handle them effectively.
Types of Errors in C
- Syntax Errors: These occur when the code violates the syntax rules of the C language. For example, missing semicolons or mismatched parentheses.
- Runtime Errors: These happen during the execution of the program, such as division by zero or invalid memory access.
- Logical Errors: These are errors in the logic of the program, where the syntax is correct, but the output is not as expected. For example, using incorrect formulas.
Basic Error Handling in C
C does not have built-in exception handling (like try-catch
in languages like C++ or Java). Instead, C uses return values and error codes to indicate errors and handle them appropriately.
Return Codes
One common way of handling errors in C is through the use of return codes. Functions that may encounter an error will return a special value (often -1
, NULL
, or another error-specific value) to indicate the failure. The caller is expected to check the return value and act accordingly.
Example: Checking for Errors Using Return Codes
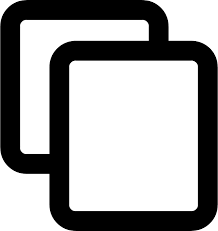
#include
// Function that may fail
int divide(int a, int b) {
if (b == 0) {
return -1; // Error: Division by zero
}
return a / b;
}
int main() {
int result = divide(10, 0);
if (result == -1) {
printf("Error: Division by zero\n");
} else {
printf("Result: %d\n", result);
}
return 0;
}
Error Handling with errno
and perror()
C provides a global variable errno
which is set by system calls and library functions when an error occurs. The value of errno
is a positive integer that corresponds to a specific error code. To print an error message, you can use the perror()
function, which uses errno
to print a relevant error message to the standard error stream.
Example: Using errno
and perror()
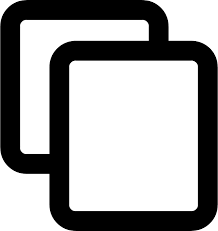
#include
#include
int main() {
FILE *file = fopen("nonexistent_file.txt", "r");
if (!file) {
perror("Error opening file");
}
return 0;
}
In the above example, if the file does not exist, perror()
will output an error message like:
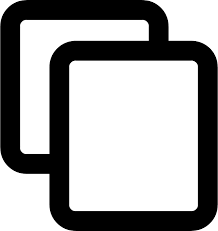
Error opening file: No such file or directory
Error Handling with exit()
and abort()
C provides functions like exit()
and abort()
to terminate the program when an error occurs. The difference is that exit()
terminates the program cleanly, while abort()
terminates the program abruptly, without cleaning up resources (e.g., closing files).
Example: Using exit()
and abort()
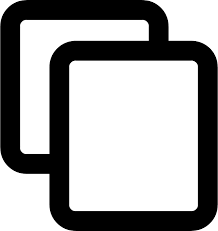
#include
#include
int main() {
int condition = 1;
if (condition) {
printf("Error occurred, program will exit.\n");
exit(1); // Exit with error code
}
printf("This will not be printed.\n");
return 0;
}
In this example, the program will terminate immediately after printing the error message. The exit code 1
is used to indicate an error.
Example: Using abort()
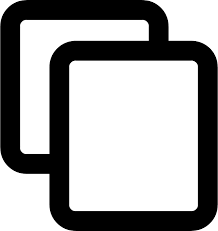
#include
#include
int main() {
printf("Error occurred, program will abort.\n");
abort(); // Abrupt termination without cleanup
return 0;
}
In this example, the program terminates abruptly and does not clean up resources (such as closing opened files).
Custom Error Handling
In some cases, you may need to define custom error handling mechanisms. One way to do this is by creating an error handling function that displays a custom message and either continues execution or terminates the program based on the error type.
Example: Custom Error Handling
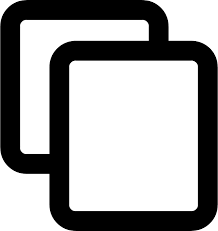
#include
#include
// Custom error function
void handleError(const char *msg) {
printf("Error: %s\n", msg);
exit(1); // Terminate the program
}
int main() {
int x = -5;
if (x < 0) {
handleError("Negative value encountered");
}
printf("Value: %d\n", x);
return 0;
}
In this example, the handleError()
function is used to display an error message and terminate the program when a negative value is encountered.
Conclusion
In C, error handling is a crucial aspect of writing robust programs. While C does not support exceptions like other languages, you can handle errors effectively using return codes, errno
, perror()
, exit()
, and abort()
. You can also define custom error-handling mechanisms to suit your program's needs. Proper error handling ensures that your program can handle unexpected situations gracefully, improving its reliability and user experience.
Handling Errors Using errno
errno
is a global variable in C that is set by system calls and library functions to indicate what error occurred when a function fails. The value of errno
is an integer that corresponds to an error code, which can then be interpreted using predefined constants. You can use this error code to identify the specific error and take appropriate action.
Understanding errno
errno
is defined in the header file errno.h
. When a library function encounters an error, it sets errno
to a specific value, which can then be examined. It's important to note that errno
is only set if an error occurs; if a function succeeds, errno
remains unchanged.
Common errno
Values
The errno
variable can be set to a variety of error codes. Some common error values defined in the errno.h
header are:
- ENOMEM: Insufficient memory available.
- EINVAL: Invalid argument passed to the function.
- EPERM: Operation not permitted.
- ENOENT: No such file or directory.
- EBADF: Bad file descriptor.
- ESRCH: No such process.
Using errno
with perror()
The perror()
function is used to print a descriptive error message based on the current value of errno
. It outputs the error string followed by a colon and a description of the error.
Example: Using perror()
with errno
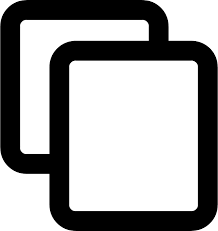
#include
#include
int main() {
FILE *file = fopen("nonexistent_file.txt", "r");
if (!file) {
perror("Error opening file");
}
return 0;
}
In the above example, if the file does not exist, perror()
will output:
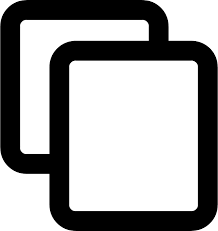
Error opening file: No such file or directory
Checking errno
After Function Calls
When checking for errors, you should examine errno
immediately after a function fails, as subsequent function calls may overwrite its value.
Example: Checking errno
After malloc()
Failure
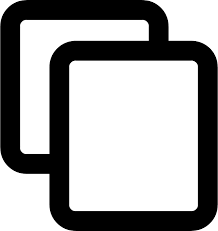
#include
#include
#include
int main() {
int *ptr = malloc(sizeof(int) * 1000000000); // Attempt to allocate a huge amount of memory
if (ptr == NULL) {
printf("Memory allocation failed\n");
perror("Error");
} else {
// Memory allocated successfully
free(ptr);
}
return 0;
}
If memory allocation fails, errno
will be set to a relevant error code, and perror()
will display the error message.
Resetting errno
errno
is not automatically cleared between function calls. If you need to check for errors in multiple function calls, it's a good idea to reset errno
to 0
before each call to ensure accurate error tracking.
Example: Resetting errno
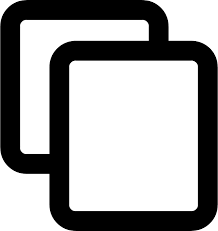
#include
#include
int main() {
errno = 0; // Reset errno
// Example of a function call that might set errno
FILE *file = fopen("nonexistent_file.txt", "r");
if (!file) {
if (errno == ENOENT) {
printf("File not found\n");
}
perror("Error opening file");
}
return 0;
}
Conclusion
errno
is a valuable tool in C for error handling. By using errno
in combination with functions like perror()
, you can easily diagnose and respond to errors in your programs. Remember to check errno
immediately after a function call that might fail and reset it when necessary to avoid confusion with previous errors.
Exception-like Mechanisms in C
C does not have built-in exception handling like languages such as C++ or Java. However, C provides mechanisms that can simulate exception-like behavior. These mechanisms allow for error handling and control flow similar to exceptions, even though they are not true exceptions. In this section, we will explore how to simulate exception handling in C using techniques like setjmp()
and longjmp()
, as well as other error handling strategies.
Using setjmp()
and longjmp()
for Simulating Exceptions
The setjmp()
and longjmp()
functions in C, defined in the setjmp.h
header, provide a way to jump back to a specific point in the program, similar to how exceptions work in languages with native exception handling. The setjmp()
function is used to save the program's state, and longjmp()
is used to restore that state, causing the program to continue execution from the point where setjmp()
was called.
Syntax of setjmp()
and longjmp()
setjmp()
sets a checkpoint in the program, and longjmp()
is used to jump back to that checkpoint. Here is the syntax:
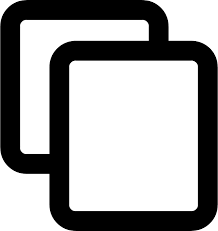
#include
int setjmp(jmp_buf env);
void longjmp(jmp_buf env, int val);
jmp_buf env
is an array that stores information about the program's state, including the stack pointer and program counter. The setjmp()
function returns 0
when it is called directly, and longjmp()
returns a non-zero value when it is used to jump back to the point where setjmp()
was called.
Example: Simulating Exception Handling Using setjmp()
and longjmp()
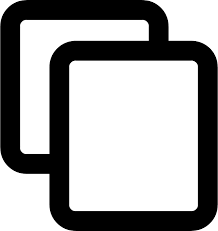
#include
#include
jmp_buf buf;
void functionWithError() {
printf("An error occurred. Jumping back...\n");
longjmp(buf, 1); // Jump back to the point where setjmp() was called
}
int main() {
if (setjmp(buf) != 0) {
// This block is executed when longjmp() is called
printf("Error handled. Execution continues...\n");
} else {
// This block is executed when setjmp() is first called
printf("No error, proceeding with normal execution.\n");
functionWithError(); // Simulates an error
}
return 0;
}
In this example, the setjmp()
function is used to set a checkpoint in the program. If an error occurs, the longjmp()
function is called to jump back to the checkpoint, and the program continues execution after the error is handled.
How setjmp()
and longjmp()
Work
setjmp()
: Saves the program's state (including register values, program counter, and stack pointer) into the buffer. It returns0
when called directly.longjmp()
: Restores the program's state from the buffer saved bysetjmp()
and causessetjmp()
to return a non-zero value, allowing the program to jump to the point wheresetjmp()
was called.
While this may simulate exception handling, it is important to note that these functions should be used carefully, as they can lead to complex control flow that is difficult to manage and debug.
Limitations of setjmp()
and longjmp()
- Resource Management: When using
longjmp()
, local variables in the function wheresetjmp()
was called are not cleaned up properly, which can lead to resource leaks. - Non-Local Jumps:
longjmp()
can jump across function calls, which can make the flow of the program harder to follow and debug. - Not a True Exception Mechanism: This is not a true exception mechanism like in C++ or Java, as it does not provide structured exception handling with catch blocks or detailed error information.
Alternative Error Handling Techniques in C
In addition to using setjmp()
and longjmp()
, there are other error handling strategies in C that can be used depending on the use case:
- Return Values: Functions can return error codes or status values that can be checked by the caller to determine if an error occurred.
- Global Error Variables: Many C library functions use global error codes (e.g.,
errno
) to report errors, which can be checked by the program to handle errors appropriately. - Logging and Debugging: Implementing logging mechanisms to capture error details, including function calls, error codes, and other useful information, can be an effective way to track and handle errors in C programs.
Conclusion
While C does not have built-in exception handling, techniques like setjmp()
and longjmp()
can be used to simulate exception-like behavior. However, it is important to use these mechanisms carefully to avoid making the program flow difficult to understand and maintain. Alternatively, using return codes, global error variables, and logging can be effective ways to manage errors in C programs. Although these techniques are not as robust as exception handling in other programming languages, they can still provide a way to handle errors in complex C applications.
Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for ensuring that software projects are easy to understand, extend, and debug. Clean code adheres to best practices, uses clear and consistent naming conventions, and is organized in a way that makes it easy for others (or yourself) to maintain and update. In this section, we will explore some key principles for writing clean and maintainable code in C, which can be applied to other programming languages as well.
Principles of Clean and Maintainable Code
- Readability: Code should be easy to read and understand. Use meaningful variable, function, and constant names that convey the purpose of the code.
- Consistency: Consistent naming conventions and formatting help ensure that code is predictable and easy to follow. Follow consistent patterns for function definitions, variable names, and code indentation.
- Modularity: Divide code into smaller, reusable functions or modules. Each function should have a single responsibility and be as independent as possible.
- Documentation: Proper documentation, including comments and README files, helps others (and your future self) understand the purpose of the code and how it works.
- Avoiding Redundancy: Eliminate repeated code by creating reusable functions or macros. This reduces the chance of errors and makes updates easier.
- Error Handling: Ensure that errors are handled correctly and consistently. Use appropriate error codes, return values, or logging mechanisms to capture and manage errors.
- Testing: Write unit tests or other forms of automated tests to ensure that your code functions as expected and handles edge cases properly.
Tips for Writing Clean Code in C
- Use Descriptive Variable and Function Names: Choose names that clearly describe the purpose of the variable or function. For example,
calculateSum()
is better thanfunc1()
. - Keep Functions Small: Each function should do only one thing and do it well. If a function is too large or does too many things, break it down into smaller, more manageable pieces.
- Use Constants for Magic Numbers: Avoid using "magic numbers" (numeric values without explanation) in your code. Instead, define constants with meaningful names to improve readability. For example:
- Comment Wisely: Use comments to explain the "why" behind your code, not the "how." Good code should be self-explanatory, but comments can help clarify complex logic or decisions that might not be obvious to others.
- Maintain Consistent Formatting: Use consistent indentation, spacing, and brace placement. This helps others (and your future self) quickly understand your code's structure. For example, always use 4 spaces for indentation and place opening braces on the same line as the function or control statement:
- Avoid Global Variables: Minimize the use of global variables as they can introduce complexity and make your code harder to understand. Instead, pass data between functions using parameters and return values.
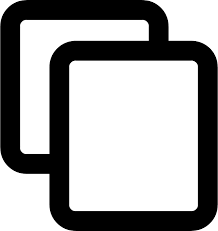
#define MAX_BUFFER_SIZE 1024
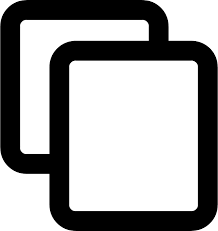
if (condition) {
// Code block
}
Example of Clean Code in C
Here’s an example of clean and maintainable C code:
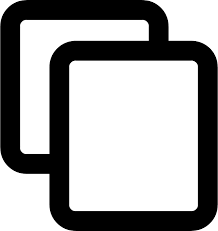
#include
#define MAX_NAME_LENGTH 100
// Function to print a greeting message
void printGreeting(const char *name) {
printf("Hello, %s!\n", name);
}
// Function to read a name from the user
void readName(char *name) {
printf("Enter your name: ");
fgets(name, MAX_NAME_LENGTH, stdin);
}
int main() {
char name[MAX_NAME_LENGTH];
readName(name);
printGreeting(name);
return 0;
}
In this example, the code is clean, modular, and easy to understand. The functions are small and focused on a single responsibility, constants are used for magic numbers, and the naming conventions are descriptive.
Common Mistakes to Avoid
- Excessive Nesting: Deeply nested loops or conditionals can make code hard to read and understand. Try to avoid deep nesting by breaking up complex conditions or loops into smaller functions.
- Hardcoding Values: Avoid hardcoding values directly in the code. Use constants, configuration files, or input from users to make your code more flexible and maintainable.
- Lack of Error Handling: Failing to handle errors can lead to unpredictable behavior in your program. Always check for potential errors, such as memory allocation failures, file read/write errors, or invalid input.
- Poor Documentation: While code should be as self-explanatory as possible, failing to document complex logic or important decisions can lead to confusion for others working with your code.
Conclusion
Writing clean and maintainable code is a crucial skill for any software developer. By following best practices such as using meaningful names, keeping functions small, avoiding redundancy, and writing proper documentation, you can ensure that your code is readable, understandable, and easy to maintain. Clean code not only improves the quality of your software but also makes it easier for others to collaborate and contribute to your project.
Debugging Techniques in C
Debugging is a crucial skill for any programmer. It helps identify and fix errors or bugs in your code, ensuring the program runs as expected. In C programming, debugging can be challenging due to the language's low-level nature and manual memory management. In this section, we will explore some common debugging techniques and tools that can help you find and resolve issues in your C programs.
Common Types of Bugs in C Programs
- Syntax Errors: These occur when the program does not follow the correct syntax rules of the C language. Examples include missing semicolons, unmatched parentheses, or incorrect keyword usage.
- Runtime Errors: These occur while the program is running, such as accessing invalid memory, dividing by zero, or using uninitialized variables.
- Logical Errors: These occur when the program runs without crashing but produces incorrect results. These errors are often hard to detect because the program executes normally but does not behave as expected.
- Memory Leaks: These occur when memory that was allocated dynamically is not properly freed, leading to wasted memory and performance issues.
Debugging Techniques
- Print Statements: One of the simplest and most common debugging techniques is to insert
printf
statements at different points in your code to print variable values and track the flow of execution. This helps you understand where the program may be going wrong. For example: - Using a Debugger: A debugger is a tool that allows you to step through your code line by line, examine variable values, and control the program's execution. Popular debuggers for C include
gdb
(GNU Debugger) and integrated debuggers in IDEs like Code::Blocks and Visual Studio. Common debugger commands include:break
Sets a breakpoint at a specific line number.: run:
Starts the program from the beginning.step:
Executes the program line by line, entering functions if needed.print
Displays the value of a variable.: continue:
Resumes program execution until the next breakpoint.
- Static Analysis Tools: Static analysis tools examine your code without running it and can catch common errors, such as uninitialized variables, memory leaks, and buffer overflows. Popular static analysis tools for C include
lint
andcppcheck
. - Valgrind: Valgrind is a powerful tool for detecting memory-related issues in C programs, such as memory leaks, invalid memory access, and uninitialized memory reads. It provides detailed reports on memory allocation and deallocation.
- Code Reviews: Sometimes the best way to find bugs is to have someone else look at your code. Code reviews by peers can help spot logical errors and provide new perspectives on solving problems.
- Unit Testing: Writing unit tests for individual components or functions of your program helps ensure that each part works correctly. Testing frameworks like
CUnit
orUnity
can automate unit testing and help catch bugs early in the development process.
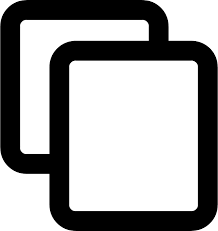
printf("Value of x: %d\n", x);
Debugging Example Using GDB
Here is a simple example of how you can use gdb
to debug a C program:
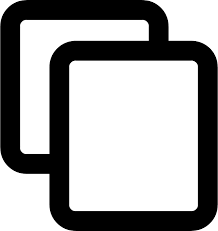
#include
int main() {
int x = 5;
int y = 0;
printf("Result: %d\n", x / y); // This will cause a division by zero error
return 0;
}
To debug this program using gdb
, follow these steps:
- Compile the program with debugging information:
gcc -g program.c -o program
- Start
gdb
with the compiled program:gdb ./program
- Set a breakpoint at the line where the division occurs:
break 6
- Run the program:
run
- When the breakpoint is hit, examine the variables:
print x
,print y
- Step through the program to identify the issue.
Advanced Debugging Tips
- Use Assertions: Assertions are checks you can add to your code to ensure that certain conditions hold true during execution. If an assertion fails, the program will terminate with an error message. For example:
- Inspect Core Dumps: A core dump is a file that contains the memory image of a program at the time it crashes. You can use a debugger to inspect the core dump and investigate what caused the crash. To enable core dumps, use the command
ulimit -c unlimited
in the terminal. - Break Down Complex Functions: If you are debugging a large function, try breaking it down into smaller, simpler functions. This can help isolate the problem and make debugging easier.
- Check for Memory Corruption: Memory corruption can cause erratic behavior in programs. Use tools like
valgrind
to check for memory corruption issues and ensure that you are properly allocating and freeing memory.
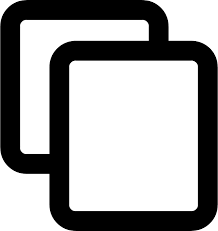
#include
int main() {
int x = 5;
assert(x != 0); // Check that x is not zero
return 0;
}
Conclusion
Debugging is an essential skill for any programmer, and C provides many tools and techniques to help identify and resolve issues in your code. By using print statements, debuggers like gdb
, static analysis tools, and memory management tools like Valgrind
, you can effectively debug your C programs. Remember to keep your code clean, write unit tests, and leverage debugging tools to catch errors early in the development process.
Optimizing C Programs
Optimizing C programs is a critical step in improving the performance, speed, and resource utilization of your applications. Efficient code not only enhances performance but also reduces memory consumption and CPU usage. In this section, we will explore various techniques and strategies for optimizing C programs.
Types of Optimization
- Time Optimization: Reducing the time complexity of algorithms to make the program execute faster.
- Space Optimization: Reducing the memory footprint of the program and using memory more efficiently.
- Code Readability and Maintainability: Writing clean, modular, and maintainable code, which indirectly contributes to optimization through easier refactoring and debugging.
Techniques for Optimizing C Programs
- Choosing the Right Algorithm: The most important factor in optimization is selecting efficient algorithms. Before optimizing code, analyze the algorithm’s time and space complexity. Using more efficient algorithms (e.g., quicksort instead of bubble sort) can significantly improve performance.
- Minimize Memory Usage: Efficient memory management reduces overhead. Avoid unnecessary memory allocations and deallocate memory as soon as it is no longer needed. Consider using stack memory instead of heap memory where possible for faster allocation and deallocation.
- Use Efficient Data Structures: Choosing the right data structure can drastically optimize the performance of your program. For example, using hash tables for fast lookups or linked lists for dynamic data sizes can improve performance.
- In-place Computation: In-place algorithms avoid the need for additional memory by modifying the data directly. For example, using the same array for sorting rather than creating a copy of it can save memory and improve performance.
- Avoid Unnecessary Function Calls: Function calls can add overhead, especially when called repeatedly in tight loops. Inline functions (using the
inline
keyword) or using macros for small functions can reduce overhead. - Loop Optimization: Loops are often a significant part of a program’s execution time. Optimizing loops can have a large impact on performance. Techniques include:
- Minimizing the number of iterations.
- Unrolling loops to reduce loop control overhead.
- Avoiding repetitive calculations inside the loop (e.g., moving computations outside the loop if they don't change on each iteration).
- Use Compiler Optimizations: Most compilers provide optimization flags that can improve performance automatically. For example, using the
-O2
or-O3
flags with GCC enables optimizations like loop unrolling, function inlining, and constant folding. - Profile the Code: Profiling tools like
gprof
andvalgrind
can identify performance bottlenecks in your program. By profiling your code, you can pinpoint areas that require optimization and avoid unnecessary optimizations on parts of the code that are not performance-critical. - Optimize I/O Operations: I/O operations (reading from/writing to files, user input/output) can be slow. Reducing the number of I/O operations, buffering input/output, and using efficient I/O functions can help improve performance.
Compiler Optimizations
Modern compilers offer several optimization levels that can automatically improve the performance of your program. These optimizations include:
- -O1: Basic optimizations such as removing dead code and simplifying expressions.
- -O2: More aggressive optimizations, including inlining functions and loop optimizations.
- -O3: Maximum optimizations, including vectorization, inlining of all functions, and other advanced techniques.
- -Os: Optimizations for size, which reduce the memory footprint of the program.
- -Ofast: Aggressive optimizations, potentially with trade-offs in correctness (e.g., ignoring strict compliance with the C standard).
Optimizing Memory Usage
- Avoid Memory Leaks: Ensure that all dynamically allocated memory is freed when no longer needed. Use tools like
valgrind
to detect memory leaks. - Use Memory Pools: Memory pools can help allocate and free memory efficiently when dealing with many small objects.
- Minimize Stack Usage: Stack memory is limited, so avoid allocating large arrays or structures on the stack. Instead, allocate large objects on the heap.
Example: Optimizing a Simple Program
Consider this simple program that sums the elements of an array:
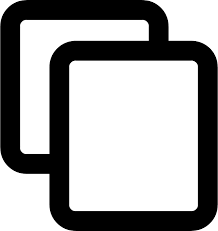
#include
#define SIZE 1000
int main() {
int arr[SIZE];
int sum = 0;
// Fill the array with values
for (int i = 0; i < SIZE; i++) {
arr[i] = i;
}
// Sum the elements of the array
for (int i = 0; i < SIZE; i++) {
sum += arr[i];
}
printf("Sum: %d\n", sum);
return 0;
}
This program can be optimized by reducing repetitive calculations and using better memory access patterns:
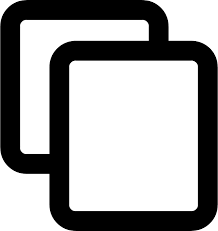
#include
#define SIZE 1000
int main() {
int arr[SIZE];
int sum = 0;
// Fill the array with values
for (int i = 0; i < SIZE; i++) {
arr[i] = i;
}
// Optimized loop (unrolling the loop)
for (int i = 0; i < SIZE; i += 2) {
sum += arr[i] + arr[i + 1];
}
printf("Sum: %d\n", sum);
return 0;
}
In this optimized version, we unroll the loop to reduce the overhead of the loop control. This will lead to fewer iterations and potentially better performance.
Advanced Optimization Techniques
- Vectorization: Modern processors can perform multiple operations simultaneously using SIMD (Single Instruction, Multiple Data). Using compiler flags or libraries like
OpenMP
orIntel MKL
, you can take advantage of these features. - Concurrency and Parallelism: For multi-core processors, parallelizing tasks using threads (with
pthread
orOpenMP
) can significantly speed up computation-heavy programs. - Cache Optimization: Optimizing memory access patterns to take advantage of the CPU cache can reduce memory latency and improve performance. This can be achieved by using cache-friendly algorithms and data structures.
Conclusion
Optimizing C programs requires a balance between speed, memory usage, and code readability. By selecting the right algorithms, managing memory effectively, using compiler optimizations, and profiling your code, you can significantly improve the performance of your C programs. Always remember to measure the impact of optimizations to ensure they actually improve performance and don't introduce new issues.
Common Mistakes to Avoid in C Programming
C programming offers great power and flexibility, but with that comes the responsibility to be careful with certain aspects of the language. Even experienced programmers can make mistakes that can lead to bugs, crashes, or inefficient code. In this section, we will go over some of the most common mistakes in C programming and how to avoid them.
1. Forgetting to Initialize Variables
Uninitialized variables can contain garbage values, leading to unpredictable behavior and bugs that are difficult to track down. Always initialize your variables before using them.
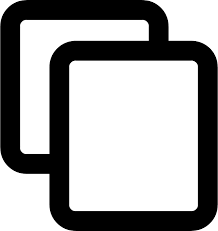
int x; // Uninitialized variable
printf("%d", x); // This may print a garbage value
Correct approach:
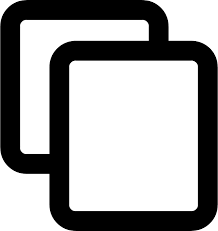
int x = 0; // Initialize variable
printf("%d", x); // This will print 0
2. Using Undefined Behavior (e.g., Dereferencing NULL Pointers)
Accessing memory that is not properly allocated, or dereferencing null pointers, leads to undefined behavior. Always ensure pointers are properly initialized before accessing memory.
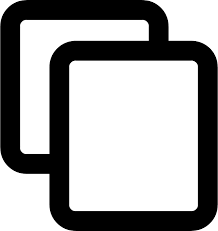
int *ptr = NULL;
*ptr = 10; // Dereferencing a NULL pointer is undefined behavior
Correct approach:
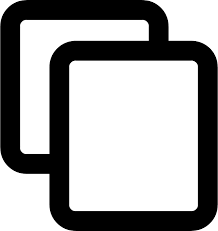
int *ptr = malloc(sizeof(int));
if (ptr != NULL) {
*ptr = 10; // Safe dereference
}
3. Not Freeing Allocated Memory
When you use dynamic memory allocation (e.g., with malloc()
or calloc()
), it is crucial to free the memory using free()
to prevent memory leaks.
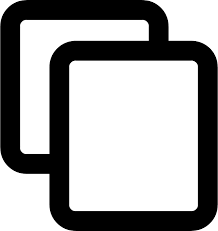
int *arr = malloc(10 * sizeof(int));
// Forgetting to free memory
// This leads to memory leaks
Correct approach:
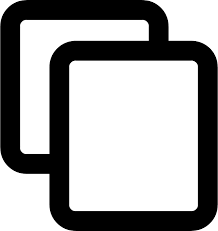
int *arr = malloc(10 * sizeof(int));
// Use arr
free(arr); // Free the allocated memory
4. Buffer Overflow
Buffer overflows occur when you attempt to write more data to a buffer than it can hold, leading to memory corruption and security vulnerabilities. Always ensure you do not exceed the buffer size.
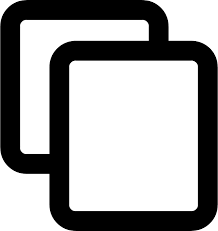
char buffer[10];
strcpy(buffer, "This is a very long string"); // Buffer overflow
Correct approach:
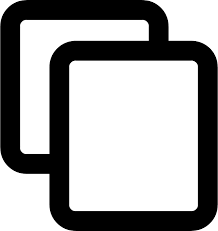
char buffer[10];
strncpy(buffer, "This is a very long string", sizeof(buffer) - 1);
buffer[sizeof(buffer) - 1] = '\0'; // Ensure null termination
5. Using Magic Numbers
Hardcoding values (magic numbers) directly in your code can make it difficult to understand and maintain. Use symbolic constants or #define
to make the code more readable.
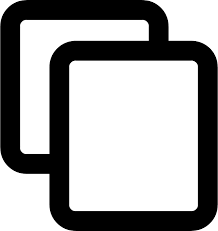
if (x == 10) { // Magic number 10
// Do something
}
Correct approach:
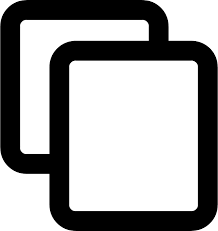
#define MAX_SIZE 10
if (x == MAX_SIZE) { // Use a defined constant
// Do something
}
6. Ignoring Compiler Warnings
Compiler warnings are there to point out potential issues in your code, such as type mismatches, unused variables, or deprecated functions. Always fix or investigate warnings to avoid subtle bugs.
Example warning:
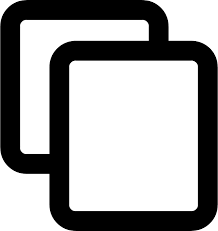
int x = 5;
x = "Hello"; // Compiler will warn about type mismatch
Correct approach:
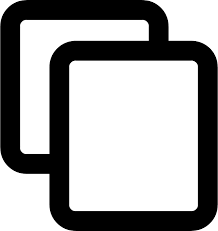
int x = 5;
x = 10; // Correct type assignment
7. Misusing the "==" and "=" Operators
The ==
operator is used for comparison, while =
is used for assignment. Confusing these two can lead to bugs that are hard to detect.
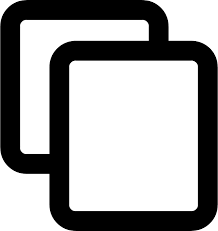
if (x = 5) { // Assignment instead of comparison
// This will assign 5 to x and execute the block
}
Correct approach:
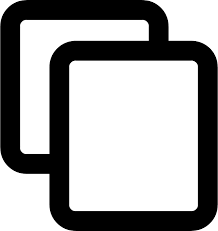
if (x == 5) { // Correct comparison
// This will compare x with 5
8. Using the Wrong Format Specifier with printf()
and scanf()
Using the incorrect format specifier when printing or reading values can cause undefined behavior and incorrect results. Always use the correct format specifier for the variable types.
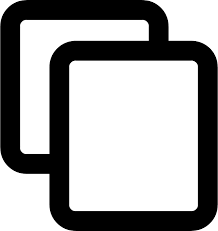
int x = 10;
printf("%f", x); // Incorrect format specifier (x is an integer)
Correct approach:
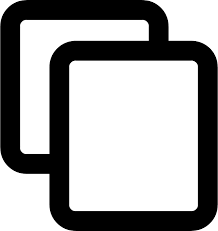
int x = 10;
printf("%d", x); // Correct format specifier for integers
9. Failing to Handle Edge Cases
Edge cases, such as empty strings, large numbers, or boundary conditions, should always be considered to ensure your program behaves correctly in all situations. Test your code with various inputs, including edge cases.
10. Not Using Constants for Fixed Values
Using fixed values directly in the code can lead to errors and make the program less maintainable. Constants should be used for values that do not change during program execution.
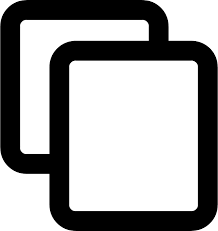
int area = 3.14159 * radius * radius; // Using a "magic number" for PI
Correct approach:
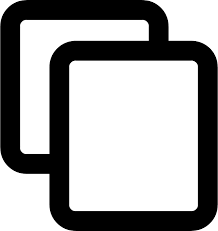
#define PI 3.14159
int area = PI * radius * radius; // Use a defined constant
11. Not Considering Platform-Specific Behavior
C programs can behave differently on different platforms. Ensure your code is portable and test it on multiple platforms when possible. This includes platform-specific libraries, file path formats, and byte ordering.
Conclusion
By avoiding these common mistakes, you can write more efficient, reliable, and maintainable C code. Always be mindful of initialization, memory management, and platform-specific behavior, and make sure to thoroughly test your code to catch errors early.
Building a Simple Calculator in C
In this section, we will walk through how to create a simple calculator in C that can perform basic arithmetic operations like addition, subtraction, multiplication, and division. This will help you practice using conditional statements, loops, and functions in C.
Step 1: Plan the Calculator
Our calculator will take two numbers and an operator as input. Based on the operator, it will perform the corresponding arithmetic operation and display the result. The supported operations will be:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
Step 2: Write the Code
Now, let's write the code for the calculator in C.
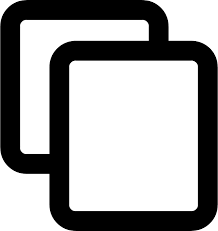
#include <stdio.h>
int main() {
double num1, num2, result;
char operator;
// Get user input
printf("Enter first number: ");
scanf("%lf", &num1);
printf("Enter an operator (+, -, *, /): ");
scanf(" %c", &operator); // Note the space before %c to consume any leftover newline character
printf("Enter second number: ");
scanf("%lf", &num2);
// Perform the calculation
switch (operator) {
case '+':
result = num1 + num2;
printf("Result: %.2f\n", result);
break;
case '-':
result = num1 - num2;
printf("Result: %.2f\n", result);
break;
case '*':
result = num1 * num2;
printf("Result: %.2f\n", result);
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
printf("Result: %.2f\n", result);
} else {
printf("Error! Division by zero.\n");
}
break;
default:
printf("Invalid operator.\n");
}
return 0;
}
Step 3: Explanation of the Code
- Input: The program first asks the user to input two numbers and an operator. The numbers are stored in the variables
num1
andnum2
, and the operator is stored in theoperator
variable. - Switch Case: A
switch
statement is used to check which operator the user entered. Based on the operator, the corresponding arithmetic operation is performed. - Error Handling: The program checks if the user tries to divide by zero and displays an error message in that case.
- Output: The result of the operation is displayed to the user with two decimal points.
Step 4: Sample Output
Here is an example of how the program works:
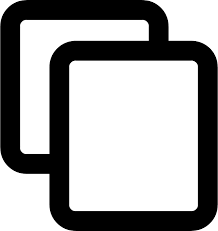
Enter first number: 10
Enter an operator (+, -, *, /): +
Enter second number: 5
Result: 15.00
Step 5: Enhancements
This simple calculator can be extended with additional features, such as:
- Handling multiple operations (e.g., calculating the result of 2 + 3 * 4).
- Adding a loop to let users perform multiple calculations without restarting the program.
- Including more advanced operations like modulus (%) or exponentiation.
Conclusion
Building a simple calculator in C is a great exercise to practice using basic programming concepts. By using conditional statements, loops, and functions, you can extend this calculator to perform more complex tasks. This project provides a foundation for working with arithmetic operations and user input in C.
Creating a Basic File Encryption Program in C
In this section, we will create a simple file encryption program in C. The program will read the contents of a file, encrypt it using a basic encryption algorithm (XOR in this case), and write the encrypted content back to a new file. This will introduce you to file handling and basic encryption techniques in C.
Step 1: Plan the Encryption
We will use a simple encryption method called XOR encryption. XOR is a bitwise operator that performs an exclusive OR operation on two binary digits. The XOR operation is reversible, meaning if we apply XOR to the encrypted file with the same key, we will get back the original content.
The steps for encryption are as follows:
- Open the input file (the file to be encrypted).
- Read the contents of the file byte by byte.
- Apply the XOR operation to each byte using a key.
- Write the encrypted data to a new file.
Step 2: Write the Code
Here’s the C code that implements the basic file encryption program using XOR encryption:
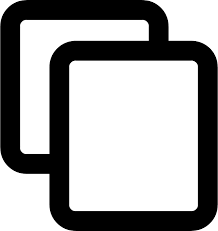
#include <stdio.h>
#include <stdlib.h>>
void encryptFile(FILE *inputFile, FILE *outputFile, char key) {
char buffer;
while ((buffer = fgetc(inputFile)) != EOF) {
// XOR encryption
buffer ^= key;
fputc(buffer, outputFile);
}
}
int main() {
FILE *inputFile, *outputFile;
char key = 'K'; // Encryption key
// Open input file
inputFile = fopen("input.txt", "rb");
if (inputFile == NULL) {
printf("Error: Could not open input file.\n");
return 1;
}
// Open output file
outputFile = fopen("encrypted.txt", "wb");
if (outputFile == NULL) {
printf("Error: Could not create output file.\n");
fclose(inputFile);
return 1;
}
// Encrypt the file
encryptFile(inputFile, outputFile, key);
// Close files
fclose(inputFile);
fclose(outputFile);
printf("File encrypted successfully!\n");
return 0;
}
Step 3: Explanation of the Code
- File Handling: The program uses
fopen
to open the input and output files. The input file is opened in binary mode ("rb"), and the output file is created in binary write mode ("wb"). - Encryption Logic: The
encryptFile
function reads each byte from the input file, applies the XOR operation with the key, and writes the result to the output file. - Key: The encryption key is a single character (
'K'
) used for the XOR operation. You can change this key to any other character or even make it user-defined. - Output: Once the encryption is complete, the program outputs a success message and closes both files using
fclose
.
Step 4: Sample Output
Here is how the output looks once the file is successfully encrypted:
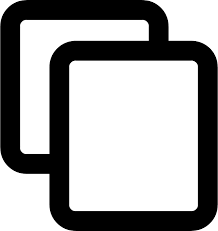
File encrypted successfully!
The content of the file "input.txt" will be encrypted and saved in "encrypted.txt".
Step 5: Decryption
To decrypt the file, we can use the same XOR key. Since XOR is a reversible operation, applying the same key to the encrypted file will return the original content. Here’s the code for decryption:
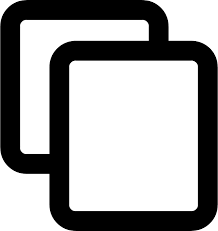
#include <stdio.h>
#include <stdlib.h>
void decryptFile(FILE *inputFile, FILE *outputFile, char key) {
char buffer;
while ((buffer = fgetc(inputFile)) != EOF) {
// XOR decryption (same as encryption)
buffer ^= key;
fputc(buffer, outputFile);
}
}
int main() {
FILE *inputFile, *outputFile;
char key = 'K'; // Decryption key
// Open encrypted file
inputFile = fopen("encrypted.txt", "rb");
if (inputFile == NULL) {
printf("Error: Could not open encrypted file.\n");
return 1;
}
// Open output file to write decrypted content
outputFile = fopen("decrypted.txt", "wb");
if (outputFile == NULL) {
printf("Error: Could not create output file for decrypted content.\n");
fclose(inputFile);
return 1;
}
// Decrypt the file
decryptFile(inputFile, outputFile, key);
// Close files
fclose(inputFile);
fclose(outputFile);
printf("File decrypted successfully!\n");
return 0;
}
Step 6: Conclusion
In this tutorial, we created a basic file encryption program using XOR encryption in C. We also demonstrated how to decrypt the file using the same key. This is a simple example of encryption, and while XOR encryption is not very secure for real-world applications, it provides a basic understanding of how encryption works. You can explore more advanced encryption algorithms such as AES for stronger security.
Designing a Mini Banking System in C
In this section, we will design a basic banking system that allows users to create an account, deposit money, withdraw money, check their balance, and view account details. The program will use basic concepts of C such as functions, structures, and file handling to store and manage account data.
Step 1: Define the Structure for Account
We will start by defining a structure to store account information such as account number, account holder's name, balance, and account type.
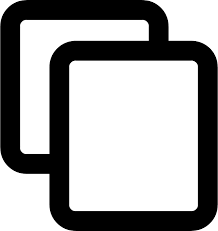
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Account {
int accountNumber;
char name[100];
float balance;
char accountType[20];
};
Step 2: Functions for Banking Operations
We will write functions to perform the following operations:
- Create an account
- Deposit money
- Withdraw money
- View account details
- Exit the program
Here are the functions for each operation:
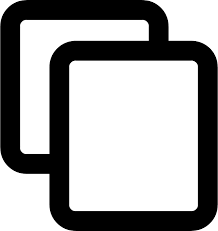
void createAccount(struct Account *acc) {
printf("Enter Account Number: ");
scanf("%d", &acc->accountNumber);
printf("Enter Account Holder's Name: ");
getchar(); // To consume the newline character left by scanf
fgets(acc->name, 100, stdin);
acc->name[strcspn(acc->name, "\n")] = 0; // Remove newline character
printf("Enter Account Type (Saving/Current): ");
fgets(acc->accountType, 20, stdin);
acc->accountType[strcspn(acc->accountType, "\n")] = 0; // Remove newline
acc->balance = 0.0;
printf("Account Created Successfully!\n");
}
void depositMoney(struct Account *acc) {
float amount;
printf("Enter amount to deposit: ");
scanf("%f", &amount);
acc->balance += amount;
printf("Deposit successful! New balance: %.2f\n", acc->balance);
}
void withdrawMoney(struct Account *acc) {
float amount;
printf("Enter amount to withdraw: ");
scanf("%f", &amount);
if (amount > acc->balance) {
printf("Insufficient funds! Current balance: %.2f\n", acc->balance);
} else {
acc->balance -= amount;
printf("Withdrawal successful! New balance: %.2f\n", acc->balance);
}
}
void viewAccountDetails(struct Account *acc) {
printf("\nAccount Number: %d\n", acc->accountNumber);
printf("Account Holder: %s\n", acc->name);
printf("Account Type: %s\n", acc->accountType);
printf("Current Balance: %.2f\n", acc->balance);
}
void exitProgram() {
printf("Exiting the program. Have a great day!\n");
exit(0);
}
Step 3: Main Program Flow
The main program will display a menu to the user and prompt them to choose an operation. Based on the user's choice, the corresponding function will be called.
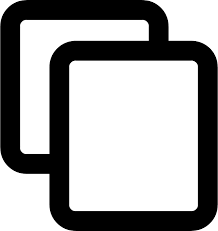
int main() {
struct Account userAccount;
int choice;
while (1) {
printf("\nMini Banking System\n");
printf("1. Create Account\n");
printf("2. Deposit Money\n");
printf("3. Withdraw Money\n");
printf("4. View Account Details\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
createAccount(&userAccount);
break;
case 2:
depositMoney(&userAccount);
break;
case 3:
withdrawMoney(&userAccount);
break;
case 4:
viewAccountDetails(&userAccount);
break;
case 5:
exitProgram();
break;
default:
printf("Invalid choice! Please try again.\n");
break;
}
}
return 0;
}
Step 4: Explanation of the Code
- Structure: We use the
struct Account
to define an account with fields for account number, name, balance, and account type. - createAccount: This function collects data from the user and initializes a new account with default values.
- depositMoney: It allows the user to deposit money into their account by increasing the balance.
- withdrawMoney: This function allows the user to withdraw money, but only if sufficient funds are available.
- viewAccountDetails: It displays the account details such as account number, holder’s name, balance, and account type.
- exitProgram: Exits the program gracefully.
- Menu: The main function presents a menu to the user and directs them to the appropriate function based on the user's choice.
Step 5: Sample Output
Here’s what the program's output looks like when you run it:
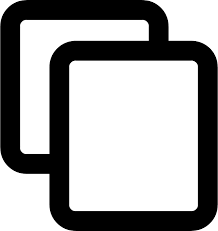
Mini Banking System
1. Create Account
2. Deposit Money
3. Withdraw Money
4. View Account Details
5. Exit
Enter your choice: 1
Enter Account Number: 12345
Enter Account Holder's Name: John Doe
Enter Account Type (Saving/Current): Saving
Account Created Successfully!
Mini Banking System
1. Create Account
2. Deposit Money
3. Withdraw Money
4. View Account Details
5. Exit
Enter your choice: 2
Enter amount to deposit: 500
Deposit successful! New balance: 500.00
Mini Banking System
1. Create Account
2. Deposit Money
3. Withdraw Money
4. View Account Details
5. Exit
Enter your choice: 4
Account Number: 12345
Account Holder: John Doe
Account Type: Saving
Current Balance: 500.00
Step 6: Conclusion
This program demonstrates the basic functionality of a mini banking system where users can create an account, deposit and withdraw money, and view their account details. You can enhance this system by adding features such as storing account data in a file, adding more account types, or implementing a system for transferring money between accounts.
Developing a Student Management System in C
In this section, we will develop a simple Student Management System using C programming. This system will allow the user to add student records, view student details, update student information, and delete records. We will utilize structures to store student data and functions to implement CRUD operations (Create, Read, Update, Delete).
Step 1: Define the Structure for Student
We will define a structure to store student information such as student ID, name, age, and marks.
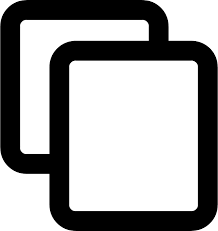
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
int studentID;
char name[100];
int age;
float marks;
};
Step 2: Functions for Student Management Operations
We will write functions to perform the following operations:
- Add a new student record
- Display student records
- Update student information
- Delete a student record
- Exit the program
Here are the functions for each operation:
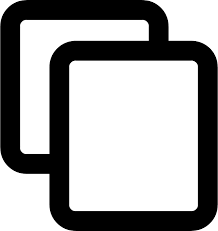
void addStudent(struct Student *student) {
printf("Enter Student ID: ");
scanf("%d", &student->studentID);
printf("Enter Student Name: ");
getchar(); // To consume the newline character left by scanf
fgets(student->name, 100, stdin);
student->name[strcspn(student->name, "\n")] = 0; // Remove newline character
printf("Enter Student Age: ");
scanf("%d", &student->age);
printf("Enter Student Marks: ");
scanf("%f", &student->marks);
printf("Student Added Successfully!\n");
}
void displayStudent(struct Student student) {
printf("\nStudent ID: %d\n", student.studentID);
printf("Student Name: %s\n", student.name);
printf("Student Age: %d\n", student.age);
printf("Student Marks: %.2f\n", student.marks);
}
void updateStudent(struct Student *student) {
printf("Enter new Name: ");
getchar(); // To consume the newline character left by scanf
fgets(student->name, 100, stdin);
student->name[strcspn(student->name, "\n")] = 0; // Remove newline character
printf("Enter new Age: ");
scanf("%d", &student->age);
printf("Enter new Marks: ");
scanf("%f", &student->marks);
printf("Student Information Updated Successfully!\n");
}
void deleteStudent(struct Student *student) {
student->studentID = 0;
strcpy(student->name, "");
student->age = 0;
student->marks = 0.0;
printf("Student Record Deleted Successfully!\n");
}
void exitProgram() {
printf("Exiting the program. Goodbye!\n");
exit(0);
}
Step 3: Main Program Flow
The main program will display a menu to the user and prompt them to choose an operation. Depending on the user's choice, the corresponding function will be called. The program will handle a simple array of students for demonstration purposes.
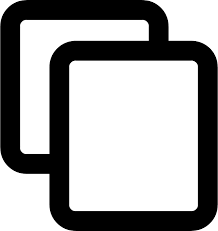
int main() {
struct Student students[10];
int choice, index = 0;
while (1) {
printf("\nStudent Management System\n");
printf("1. Add Student\n");
printf("2. Display Students\n");
printf("3. Update Student\n");
printf("4. Delete Student\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
if (index < 10) {
addStudent(&students[index]);
index++;
} else {
printf("Student list is full!\n");
}
break;
case 2:
for (int i = 0; i < index; i++) {
displayStudent(students[i]);
}
break;
case 3:
printf("Enter Student ID to update: ");
int id;
scanf("%d", &id);
int found = 0;
for (int i = 0; i < index; i++) {
if (students[i].studentID == id) {
updateStudent(&students[i]);
found = 1;
break;
}
}
if (!found) {
printf("Student ID not found!\n");
}
break;
case 4:
printf("Enter Student ID to delete: ");
scanf("%d", &id);
found = 0;
for (int i = 0; i < index; i++) {
if (students[i].studentID == id) {
deleteStudent(&students[i]);
found = 1;
break;
}
}
if (!found) {
printf("Student ID not found!\n");
}
break;
case 5:
exitProgram();
break;
default:
printf("Invalid choice! Please try again.\n");
break;
}
}
return 0;
}
Step 4: Explanation of the Code
- Structure: We use the
struct Student
to define a student with fields for student ID, name, age, and marks. - addStudent: This function takes input from the user to add a student record to the system.
- displayStudent: It displays the details of a student, including their ID, name, age, and marks.
- updateStudent: This function allows updating the details of an existing student.
- deleteStudent: This function deletes a student's record by resetting their details to default values.
- exitProgram: Exits the program gracefully.
- Menu: The main function presents a menu to the user and directs them to the appropriate function based on the user's choice. The program handles a simple array of students and performs CRUD operations on it.
Step 5: Sample Output
Here’s what the program's output looks like when you run it:
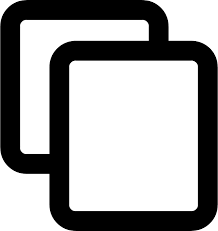
Student Management System
1. Add Student
2. Display Students
3. Update Student
4. Delete Student
5. Exit
Enter your choice: 1
Enter Student ID: 101
Enter Student Name: John Doe
Enter Student Age: 20
Enter Student Marks: 85.5
Student Added Successfully!
Student Management System
1. Add Student
2. Display Students
3. Update Student
4. Delete Student
5. Exit
Enter your choice: 2
Student ID: 101
Student Name: John Doe
Student Age: 20
Student Marks: 85.50
Student Management System
1. Add Student
2. Display Students
3. Update Student
4. Delete Student
5. Exit
Enter your choice: 3
Enter Student ID to update: 101
Enter new Name: Jane Doe
Enter new Age: 21
Enter new Marks: 90.0
Student Information Updated Successfully!
Step 6: Conclusion
This program demonstrates the basic functionality of a student management system where users can add, view, update, and delete student records. You can extend this system by adding more features such as saving student data to a file, sorting students by marks or name, and searching for students by ID.
Comments in C
Comments in C are used to provide explanations or notes about the code, making it easier to understand for anyone reading it, including the programmer themselves. Comments are ignored by the compiler and do not affect the execution of the program.
Types of Comments in C
C supports two types of comments:
1. Single-Line Comments
Single-line comments start with
//
. Anything written after//
on the same line is treated as a comment.2. Multi-Line Comments
Multi-line comments start with
/*
and end with*/
. These can span multiple lines and are useful for longer explanations.Why Use Comments?
Best Practices for Writing Comments
Example Program
The following example demonstrates the use of single-line and multi-line comments:
Output:
Common Mistakes to Avoid
Conclusion
Comments are an essential part of programming in C. They help make your code more readable and maintainable, especially in collaborative projects or when revisiting old code. Use comments wisely to create well-documented and understandable programs.