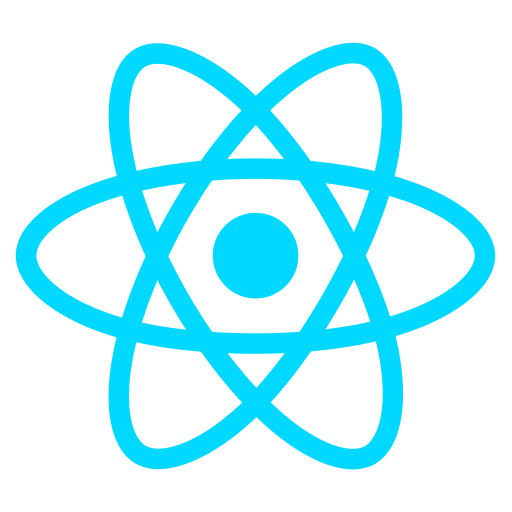
What is React?
React is a popular JavaScript library used for building user interfaces, primarily for single-page applications where you need a fast and dynamic user experience. It was developed by Facebook in 2011 and released as an open-source project in 2013.
History of React
React was created by Jordan Walke, a software engineer at Facebook, to address the complexities of building dynamic UIs. It was initially deployed on Facebook's newsfeed and later on Instagram. Over time, React gained widespread adoption due to its performance optimizations and developer-friendly features.
React Features
Here are the key features that make React a popular choice for front-end development:
Feature | Description |
---|---|
Component-Based Architecture | React uses a component-based architecture, where the UI is divided into reusable components, making the codebase easier to manage and maintain. |
Virtual DOM | React uses a virtual DOM (Document Object Model) that helps optimize rendering. React updates the virtual DOM first, compares it with the actual DOM, and only changes the parts of the UI that need updating, leading to faster performance. |
Unidirectional Data Flow | React enforces a unidirectional data flow, meaning data flows in one direction, making it easier to understand how data changes in the application. |
JSX Syntax | React uses JSX, a syntax extension that allows HTML to be written directly in JavaScript. JSX simplifies the process of creating UI components by providing a clean and readable syntax. |
Setting Up React
To start working with React, you need to set up a development environment. Here are the steps to set up React on your local machine:
- Ensure you have Node.js and npm installed on your system.
- Use
npx create-react-app my-app
to create a new React project. - Navigate to your project directory using
cd my-app
and start the development server withnpm start
.
Code Example: Hello World in React
Here’s a simple React component that displays "Hello, World!" on the screen:
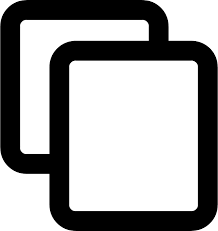
// HelloWorld.js
import React from 'react';
function HelloWorld() {
return Hello, World!
;
}
export default HelloWorld;
Diagram: React Component Lifecycle
The following diagram shows the lifecycle of a React component, from creation to destruction:

This diagram illustrates the various stages of a React component's life, including mounting, updating, and unmounting phases.
Why React is Popular: Component-Based Architecture
One of the main reasons React has become so popular is its component-based architecture. React’s approach to building UIs by breaking them into isolated, reusable components makes development more efficient, modular, and scalable.
What is Component-Based Architecture?
In React, a component is a self-contained unit that defines a piece of the UI. Components can be as simple as a button or a text box, or as complex as a complete page layout. These components can be reused, nested, and composed to form a larger UI. This modularity is what makes React so powerful.
Key Benefits of Component-Based Architecture
Here are some key benefits of using a component-based approach in React:
Benefit | Description |
---|---|
Reusability | Once a component is created, it can be reused across the application. This reduces repetition and improves code maintainability. |
Modularity | With components being self-contained, developers can focus on building and testing individual parts of the UI without worrying about the whole application. |
Maintainability | Since components are independent and encapsulated, making changes to one component doesn’t affect the rest of the application. This makes the app easier to maintain as it grows. |
Separation of Concerns | React promotes the separation of concerns by having each component manage its own logic, state, and UI, making it easier to debug and test individual parts. |
Example of a Simple React Component
Here’s an example of a simple React component that displays a greeting message:
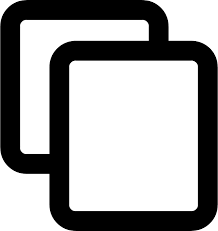
// Greeting.js
import React from 'react';
function Greeting(props) {
return Hello, {props.name}!
;
}
export default Greeting;
How Components Work Together
In a React application, components can be nested inside one another, allowing for complex UIs to be built by combining small, reusable components. For example, a header component might contain a logo component, a navigation component, and a search bar component. These components can be developed independently and then combined into a larger UI.
Diagram: React Component Hierarchy
The following diagram illustrates how components can be nested and composed together in a React application:

This diagram shows how a parent component can contain multiple child components, and how data is passed down via props.
Setting Up React Environment: Node.js, npm, and Create React App
Before you can start building React applications, you need to set up a development environment. This environment typically consists of Node.js, npm (Node Package Manager), and Create React App, which is a tool that helps you quickly set up a React project with minimal configuration.
What You Need to Install
To get started with React, make sure you have the following tools installed on your system:
- Node.js: A JavaScript runtime that allows you to run JavaScript on the server side and manage packages.
- npm: A package manager that comes with Node.js, used for managing libraries and dependencies in your React project.
- Create React App: A tool that helps you quickly set up a React application with all the necessary configuration.
Step 1: Install Node.js and npm
First, you need to install Node.js, which will also install npm automatically. Follow the steps below:
- Go to the official Node.js website.
- Download the latest stable version of Node.js.
- Run the installer and follow the on-screen instructions.
- Once installed, verify the installation by opening your terminal or command prompt and running the following commands:
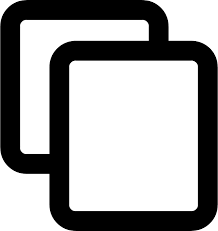
node --version
npm --version
If the installation is successful, both commands will show the installed versions of Node.js and npm.
Step 2: Install Create React App
Create React App is a command-line tool that sets up a new React project with default configurations. To install Create React App globally, run the following command:
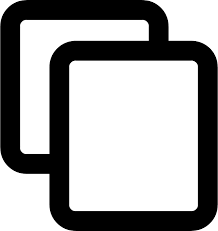
npm install -g create-react-app
This command installs Create React App globally, allowing you to create new React applications from anywhere on your system.
Step 3: Create a New React Application
Once Create React App is installed, you can create a new React project by running the following command:
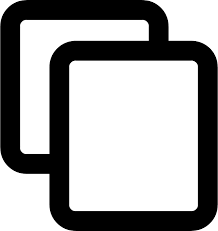
npx create-react-app my-app
This will create a new React project named "my-app" in a folder with the same name. The setup process will install all necessary dependencies and create a basic folder structure.
Step 4: Run Your React Application
Navigate to the newly created project folder and start the development server by running:
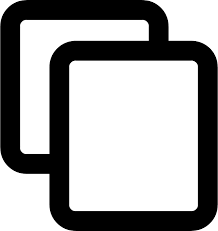
cd my-app
npm start
This will start the React development server and open your default browser with the React app running. By default, the app will be hosted at http://localhost:3000
.
Step 5: Explore the Project Structure
After creating the React app, the project folder will have the following structure:
- public/: Contains static files like the HTML template and icons.
- src/: Contains the main React code (components, App.js, etc.).
- package.json: A configuration file that includes dependencies, scripts, and project metadata.
- node_modules/: Contains the project’s dependencies installed via npm.
Code Example: App.js
The default template generated by Create React App includes a basic App.js
component. Here’s what it looks like:
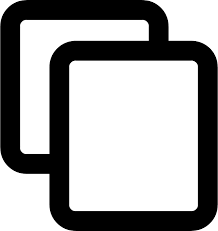
import React from 'react';
import './App.css';
function App() {
return (
Welcome to React!
);
}
export default App;
Conclusion
With Node.js, npm, and Create React App, setting up a React development environment is quick and easy. Once you’ve completed these steps, you’re ready to start building React applications!
Overview of JSX (JavaScript XML)
JSX (JavaScript XML) is a syntax extension for JavaScript commonly used with React to describe what the UI should look like. It looks similar to HTML but comes with some key differences. JSX allows you to write HTML elements and components in a JavaScript file, creating a more readable and declarative way of defining user interfaces in React.
What is JSX?
JSX is a syntax extension that allows you to write HTML-like code inside JavaScript. It's not required to use React, but it is highly recommended because it makes it easier to define and visualize the UI structure. JSX gets compiled into regular JavaScript code during the build process.
Under the hood, JSX gets transformed into React.createElement()
calls, which are used to create React elements (the building blocks of React applications).
Why Use JSX?
JSX allows you to define your UI in a more intuitive and readable way. Some reasons for using JSX include:
- Declarative Syntax: JSX provides a clear, concise syntax that looks similar to HTML, making it easy to define the structure of your UI components.
- Increased Readability: JSX helps keep the logic and the UI in the same place, making code easier to read and maintain.
- Integration with JavaScript: You can easily embed JavaScript expressions inside JSX, making it highly dynamic and interactive.
Basic Syntax of JSX
Here’s an example of JSX syntax:
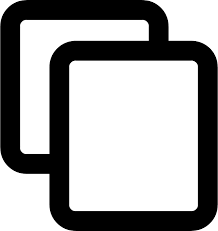
const element = Hello, world!
;
The above code creates a JSX element that represents an h1
tag with the text "Hello, world!". This is a simple JSX element, and it can be rendered to the DOM by React.
Embedding Expressions in JSX
JSX allows you to embed JavaScript expressions inside curly braces { }
. These expressions can be variables, function calls, or any valid JavaScript code. Here’s an example:
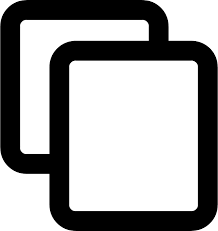
const name = 'React';
const element = Hello, {name}!
;
The value inside curly braces will be evaluated as a JavaScript expression, so {name}
will be replaced with the value of the name
variable, resulting in "Hello, React!" being rendered to the screen.
JSX and HTML Differences
Though JSX looks like HTML, there are some important differences between the two:
- HTML Attributes: In JSX, some HTML attributes are written in camelCase, such as
className
instead ofclass
, andhtmlFor
instead offor
. - Self-closing Tags: In JSX, all tags (like
img
,input
, etc.) need to be self-closed, such as<img src="image.jpg" />
. - JSX Elements Must Be Wrapped: JSX requires that all elements within a component must be wrapped inside a single parent element. For example, you cannot return two sibling elements without wrapping them in a
div
.
Code Example: JSX in a React Component
Here’s a simple example of how JSX is used in a React component:
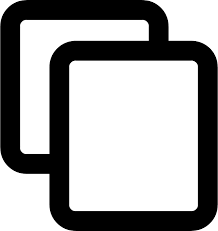
import React from 'react';
function Greeting(props) {
return Hello, {props.name}!
;
}
export default Greeting;
In the example above, the Greeting
component uses JSX to render a dynamic greeting message. The value of props.name
is embedded within the JSX element using curly braces.
JSX Compilation
JSX is not valid JavaScript, so it needs to be transpiled into JavaScript. This is done by tools like Babel. Babel translates JSX into React.createElement()
function calls, which React can process and render to the DOM.
For example, the following JSX:
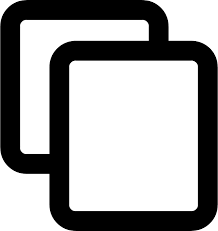
const element = Welcome to React!
;
Gets compiled into the following JavaScript code:
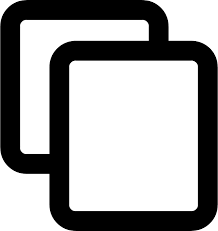
const element = React.createElement('h1', null, 'Welcome to React!');
Conclusion
JSX is an essential part of React development that makes writing React components more intuitive. By combining HTML-like syntax with JavaScript, it enables you to create dynamic, interactive UIs in a declarative way.
Understanding the React Rendering Process
The React rendering process is a core feature of React, determining how and when the UI updates in response to changes in data or state. React efficiently manages DOM updates, ensuring that the application runs optimally and with minimal resource usage. To understand this process, it's important to explore how React uses a virtual DOM and performs reconciliation to update the actual DOM.
What is the Virtual DOM?
The Virtual DOM is a lightweight, in-memory representation of the actual DOM in the browser. It is an essential part of React's rendering process. When a React component’s state or props change, React creates a new Virtual DOM tree, compares it with the previous tree, and calculates the minimal set of changes needed to update the actual DOM. This approach helps improve performance by reducing the number of direct manipulations of the actual DOM, which can be slow.
The React Rendering Lifecycle
React follows a structured lifecycle that defines the order of events during the rendering process. The key steps involved in rendering a React component include:
- Mounting: When a component is created and inserted into the DOM for the first time.
- Updating: When a component's state or props change, causing it to re-render.
- Unmounting: When a component is removed from the DOM.
How the Rendering Process Works
The rendering process can be broken down into the following steps:
1. Initial Rendering (Mounting)
During the initial rendering, React creates the component’s Virtual DOM and compares it to the current state of the actual DOM. React then updates the DOM based on the difference between the Virtual DOM and the actual DOM. This is known as the "reconciliation" process.
2. Updating (Reconciliation)
When a component's state or props change, React triggers the update process. The following steps occur:
- React updates the Virtual DOM to reflect the new state or props.
- React compares the updated Virtual DOM with the previous Virtual DOM using a process called "diffing." This helps identify the minimal set of changes.
- React calculates the most efficient way to update the actual DOM, applying the necessary changes.
3. React Diffing Algorithm
The diffing algorithm is the heart of React's efficient rendering process. It compares two Virtual DOM trees and determines the minimum number of changes required to update the actual DOM. React uses the following strategies to optimize this process:
- Element Type Comparison: React compares the type of elements (e.g.,
div
,span
, etc.). If the types differ, React will replace the entire element. - Reusing Elements: If the elements have the same type, React will only update the properties or attributes, avoiding re-rendering the entire element.
- Key Prop: React uses keys to identify elements in lists. Keys help React optimize updates by ensuring that elements are correctly matched between updates.
Optimizing the Rendering Process
React is designed to be highly efficient, but there are still ways you can optimize the rendering process in your application:
- Pure Components: Use
React.PureComponent
to automatically implement a shallow comparison of props and state, preventing unnecessary re-renders. - Memoization: Use
React.memo()
to memoize functional components and prevent them from re-rendering if their props haven't changed. - shouldComponentUpdate: Implement the
shouldComponentUpdate
lifecycle method (or useReact.memo()
for functional components) to control when a component should re-render. - Code Splitting: Use React's built-in support for code splitting to load components only when they're needed, reducing the initial load time.
Rendering in Different Environments
React's rendering process is not limited to the browser. React can render components on the server (Server-Side Rendering or SSR) or in native mobile applications (React Native). In these environments, the rendering process follows similar principles, but some specifics differ:
- Server-Side Rendering (SSR): React renders components on the server and sends the fully rendered HTML to the client. This improves performance and SEO.
- React Native: React Native uses a different rendering engine tailored to native mobile applications. It still uses the same principles of virtual DOM and reconciliation but renders components to native views instead of the web browser's DOM.
Code Example: React Rendering
Here’s an example of how React updates the UI when state changes:
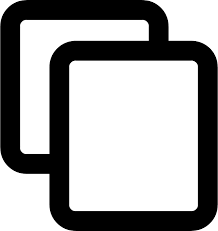
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
{count}
<button onClick={() => setCount(count + 1)}>Increment</button>
);
}
export default Counter;
In the above code, when the button is clicked, the state (count) is updated, triggering a re-render. React will update the Virtual DOM and compare it with the previous state, then apply the minimal set of changes to the actual DOM (updating the h1
element).
Conclusion
The React rendering process is efficient and optimized for performance. By using the Virtual DOM, React minimizes the number of direct manipulations to the actual DOM, improving rendering speed and responsiveness. Understanding how React renders and updates components will help you build faster, more efficient React applications.
React Components: Functional vs Class Components
In React, components are the building blocks of an application. They determine how the user interface (UI) is rendered and updated in response to user interaction or state changes. There are two main types of components in React: functional components and class components. Both serve the same purpose, but they differ in syntax, behavior, and how they manage state and lifecycle methods.
What are Functional Components?
Functional components are simple JavaScript functions that accept props as an argument and return React elements (JSX). They are often referred to as "stateless" components because they do not have their own internal state or lifecycle methods. However, with the introduction of React Hooks, functional components can now manage state and side effects, making them just as powerful as class components.
Functional Component Example
Here’s an example of a basic functional component:
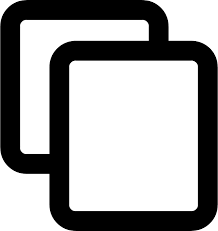
function Welcome(props) {
return Hello, {props.name}!
;
}
export default Welcome;
In this example, the Welcome
component is a function that receives props
and returns a JSX element. Functional components are simple, easy to understand, and are the preferred way to write components in modern React development.
What are Class Components?
Class components are ES6 class-based components that extend from React.Component
. They have additional features compared to functional components, such as the ability to have internal state and lifecycle methods. Class components were the primary way to handle state and side effects in earlier versions of React. However, with the introduction of Hooks, the need for class components has diminished, and functional components have become the more commonly used approach.
Class Component Example
Here’s an example of a basic class component:
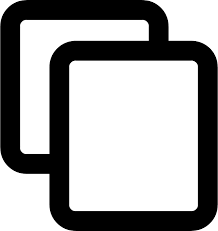
import React, { Component } from 'react';
class Welcome extends Component {
render() {
return Hello, {this.props.name}!
;
}
}
export default Welcome;
In this example, the Welcome
component is a class that extends React.Component
. The component has a render
method that returns JSX. Class components are more verbose compared to functional components, but they provide more features in earlier versions of React.
Key Differences Between Functional and Class Components
Here’s a comparison of the two types of components:
Feature | Functional Components | Class Components |
---|---|---|
Syntax | Simple JavaScript function | ES6 class that extends React.Component |
State | Can use useState (Hooks) to manage state |
Has internal state through this.state |
Lifecycle Methods | Can use useEffect (Hooks) for side effects |
Has lifecycle methods like componentDidMount , componentDidUpdate , etc. |
Readability | Shorter and more concise | More verbose |
Performance | Generally better performance due to simpler structure | Can be less performant due to the overhead of classes |
Usage | Preferred for most modern React apps | Still used, but less common in new React apps |
Using State and Lifecycle in Functional Components (with Hooks)
React Hooks introduced in version 16.8 allow functional components to use state and lifecycle features, making them much more powerful. The useState
hook manages state, while useEffect
handles side effects such as fetching data or subscribing to events. Here's an example:
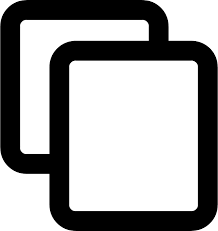
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => setSeconds(prev => prev + 1), 1000);
return () => clearInterval(interval);
}, []);
return {seconds} seconds
;
}
export default Timer;
In this example, the Timer
component uses the useState
hook to maintain the state of the timer and the useEffect
hook to set up an interval when the component mounts, and clean up when it unmounts.
When to Use Functional or Class Components?
In modern React development, functional components are the preferred choice, especially with the advent of Hooks. They are simpler, more concise, and offer the same capabilities as class components in terms of managing state and side effects. Class components are still valid and useful, especially if you are working with legacy code or libraries that use class-based components.
Conclusion
Both functional and class components have their advantages, but functional components with Hooks are now the recommended approach for building React applications. They allow for cleaner, more readable code and align with React’s modern development practices.
Props: Passing Data to Components
In React, props (short for "properties") are used to pass data from a parent component to a child component. Props are read-only, meaning that a child component cannot modify the props it receives from its parent. They allow React components to be dynamic and reusable, making it easier to manage and display data in a structured way.
What Are Props?
Props are plain JavaScript objects that are passed to components. They can contain any type of data, including strings, numbers, arrays, or even functions. Props are used to configure a component, allowing it to render content based on the data it receives.
Props Example
Let’s see an example of how props are used to pass data from a parent component to a child component:
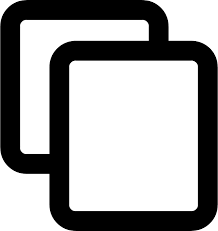
// Parent Component
function Parent() {
const userName = "John Doe";
return ;
}
// Child Component
function Child(props) {
return Hello, {props.name}!
;
}
export default Parent;
In this example, the Parent
component passes the name
prop to the Child
component. The Child
component accesses the prop using props.name
and displays it in an <h1>
element.
How to Pass Props
Props are passed to a component by adding them as attributes when the component is rendered. In the example above, the name
prop is passed to the Child
component like this:
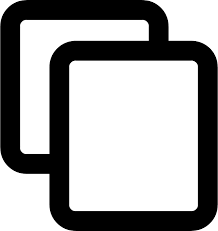
Here, name={userName}
is how props are passed. The value of the prop (userName
) is evaluated and passed to the child component. The child component can then access this value via props.name
.
Default Props
Components can also have default values for their props if a value is not passed by the parent. This is achieved using the defaultProps
property.
Default Props Example
Here’s an example of setting default props in a component:
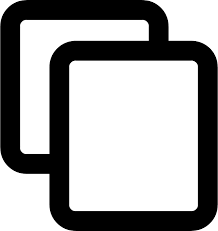
function Greeting(props) {
return Hello, {props.name}!
;
}
Greeting.defaultProps = {
name: "Guest"
};
export default Greeting;
If the parent component does not pass a name
prop to Greeting
, the default value of "Guest" will be used instead. Default props ensure that components have fallback values when necessary.
Prop Types (Type Checking)
React also provides a way to define the expected types of props using the prop-types
package. This helps catch bugs by ensuring that the right type of data is passed to a component.
Prop Types Example
Here’s how you can define prop types for a component:
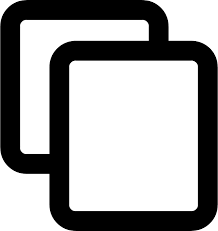
import PropTypes from 'prop-types';
function Greeting(props) {
return Hello, {props.name}!
;
}
Greeting.propTypes = {
name: PropTypes.string.isRequired
};
export default Greeting;
In this example, the Greeting
component expects a name
prop of type string
. The isRequired
modifier ensures that the prop is mandatory, and if it’s not passed, React will issue a warning.
Passing Functions as Props
Props can also be used to pass functions from parent components to child components. This allows child components to trigger actions or modify the state of the parent component.
Function as Prop Example
In the following example, we pass a function as a prop from the parent to the child:
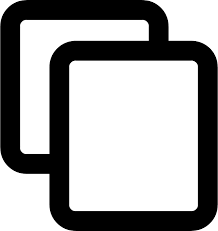
function Parent() {
const handleClick = () => alert("Button clicked!");
return ;
}
function Child(props) {
return ;
}
export default Parent;
In this example, the Parent
component passes the handleClick
function as a prop called onClick
to the Child
component. The Child
component invokes this function when the button is clicked.
Props vs State
While props are used to pass data down to components, state is used to manage data that changes over time within a component. Props are immutable, meaning that a component cannot modify its own props. On the other hand, state is mutable and can be updated using the setState
method (in class components) or the useState
hook (in functional components).
Conclusion
Props are a fundamental concept in React, allowing components to be dynamic, reusable, and configurable. By passing data from parent to child components, React enables the creation of complex UIs that respond to user interaction and data changes. Understanding props and how to effectively use them is essential for building React applications.
State: Managing Component Data
In React, state refers to the data or variables that determine a component’s behavior and rendering. Unlike props, which are passed from parent to child components, state is managed within the component itself and can change over time. When state changes, React re-renders the component to reflect the updated data.
What is State?
State is an object used to store dynamic data in a component. It allows components to respond to user input, server responses, or any other changes in data that should trigger a re-render. State is mutable, meaning it can be updated, unlike props which are immutable.
State Example
Let's see an example of how state is used in a React component:
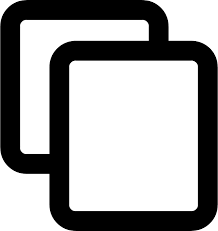
import React, { useState } from 'react';
function Counter() {
// Declare a state variable 'count' with initial value of 0
const [count, setCount] = useState(0);
return (
You clicked {count} times
<button onClick={() => setCount(count + 1)}>Click me</button>
);
}
export default Counter;
In this example, the Counter
component uses the useState
hook to manage the count
state. When the button is clicked, the setCount
function updates the state, and React re-renders the component with the updated value of count
.
Using the useState Hook
In functional components, the useState
hook is used to declare state variables. The useState
function returns an array, where:
- The first element is the current state value.
- The second element is a function used to update the state.
In the Counter
example, [count, setCount]
is returned by useState(0)
, where count
is the current state, and setCount
is the function used to update the state.
State in Class Components
In class components, state is declared within the constructor and updated using the setState
method. Here's an example:
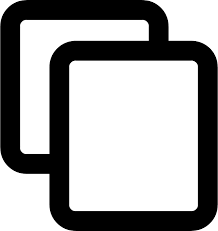
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
// Declare state in the constructor
this.state = { count: 0 };
}
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
You clicked {this.state.count} times
);
}
}
export default Counter;
In class components, state is initialized in the constructor with this.state
, and the setState
method is used to update the state. The handleClick
method updates the count
state when the button is clicked.
Updating State
State can be updated in two ways:
- Direct State Update: You can directly call the state update function (like
setCount
in the functional component) to update the state. - Functional Updates: If the new state depends on the previous state, it's recommended to use a functional update, like this:
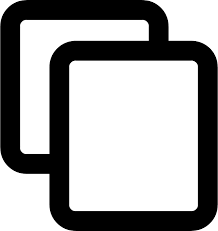
// Functional update to access previous state
setCount(prevCount => prevCount + 1);
This ensures that you're always working with the most up-to-date state value, which is important when state changes asynchronously.
State and Re-rendering
When the state of a component changes, React triggers a re-render of that component and its children. This is one of the key features of React, as it allows the UI to be updated automatically based on changes in data. React uses a virtual DOM to efficiently update only the parts of the UI that have changed, minimizing performance overhead.
State in Functional vs. Class Components
In functional components, state is managed with the useState
hook, while in class components, state is managed using the this.state
object and updated with this.setState
.
Functional Component Example:
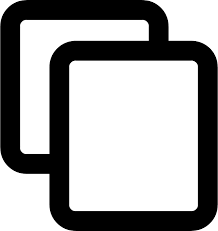
import React, { useState } from 'react';
function Example() {
const [name, setName] = useState("John");
return (
Name: {name}
<button onClick={() => setName("Doe")}>Change Name</button>
);
}
export default Example;
Class Component Example:
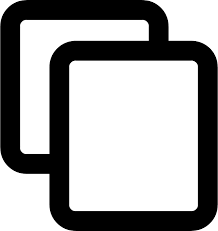
import React, { Component } from 'react';
class Example extends Component {
constructor(props) {
super(props);
this.state = { name: "John" };
}
changeName = () => {
this.setState({ name: "Doe" });
};
render() {
return (
Name: {this.state.name}
);
}
}
export default Example;
Conclusion
State is an essential concept in React, allowing components to manage dynamic data and respond to user interactions or other events. Understanding how to use state effectively is crucial for building interactive and dynamic React applications. Whether using functional components with useState
or class components with setState
, state management plays a key role in React development.
Handling Events in React (onClick, onChange, etc.)
In React, handling events is very similar to handling events in vanilla JavaScript, but with some important differences. React uses a synthetic event system, which is a wrapper around the browser’s native events. This system ensures consistent behavior across different browsers. You can handle events like onClick
, onChange
, and many others in React components.
React Event System
React's synthetic event system normalizes events so that they have consistent properties across different browsers. In React, event names are written in camelCase (e.g., onClick
instead of onclick
), and event handlers are passed as functions, not strings.
Basic Event Handling Example
Here's an example of handling a click
event in a React component:
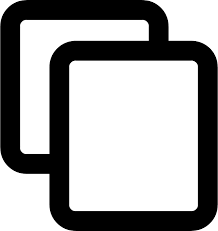
import React from 'react';
function ClickButton() {
const handleClick = () => {
alert("Button was clicked!");
};
return (
);
}
export default ClickButton;
In this example, the handleClick
function is triggered when the button is clicked. The onClick
prop is passed the handleClick
function as a callback.
Event Binding in Class Components
In class components, you need to bind event handler methods to the component instance to ensure that this
refers to the correct context. Here's an example:
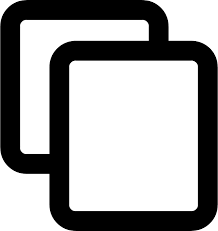
import React, { Component } from 'react';
class ButtonClass extends Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
alert("Button was clicked!");
}
render() {
return (
);
}
}
export default ButtonClass;
In the example above, handleClick
is bound to the class instance in the constructor so that it can properly access this
when triggered by the event.
Event Handling in Functional Components
In functional components, event handlers are usually defined as arrow functions, which automatically bind the function to the component’s context, making it easier to handle events.
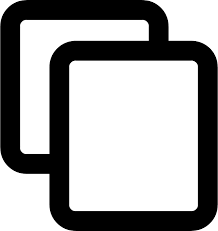
import React, { useState } from 'react';
function InputForm() {
const [value, setValue] = useState("");
const handleChange = (event) => {
setValue(event.target.value);
};
return (
Value: {value}
);
}
export default InputForm;
In this example, the onChange
event is used to track changes in the input field. The handleChange
function updates the component’s state with the new value entered by the user.
Common React Events
Here are some common events that can be handled in React:
onClick
: Triggered when an element is clicked.onChange
: Triggered when the value of an input, textarea, or select element changes.onSubmit
: Triggered when a form is submitted.onMouseEnter
: Triggered when the mouse pointer enters an element.onMouseLeave
: Triggered when the mouse pointer leaves an element.onKeyDown
: Triggered when a keyboard key is pressed down.onFocus
: Triggered when an element gains focus.onBlur
: Triggered when an element loses focus.
Example of Handling Multiple Events
Here’s an example of handling multiple events in a single component:
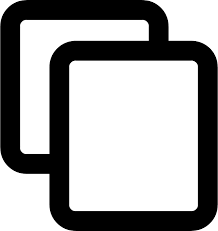
import React, { useState } from 'react';
function EventExample() {
const [message, setMessage] = useState("");
const handleClick = () => {
setMessage("Button was clicked!");
};
const handleMouseEnter = () => {
setMessage("Mouse entered the button!");
};
const handleMouseLeave = () => {
setMessage("Mouse left the button!");
};
return (
{message}
);
}
export default EventExample;
This example demonstrates how to handle multiple events in a single React component, such as onClick
, onMouseEnter
, and onMouseLeave
. The message
state is updated based on the event that occurs.
Event Object
React passes an event
object to the event handler, which contains details about the event. In the example below, we log the event details:
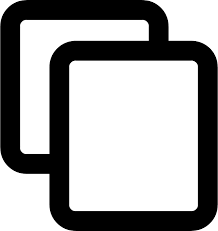
import React from 'react';
function EventLogger() {
const handleClick = (event) => {
console.log(event);
alert("Event logged to console!");
};
return (
);
}
export default EventLogger;
The event
object contains various properties such as target
(which is the element that triggered the event) and type
(which is the type of event, like click
). This can be useful for more complex event handling.
Conclusion
Event handling in React is straightforward and follows similar patterns as in plain JavaScript. React’s synthetic event system ensures consistent behavior across browsers, and state and props can be used to control the flow of data during events. Whether using onClick
, onChange
, or other events, React provides a simple and efficient way to handle user interactions.
Conditional Rendering in React
Conditional rendering in React refers to the ability to render different UI elements based on certain conditions. This is essential when you need to display content dynamically based on the state or props of a component. React provides various ways to implement conditional rendering, allowing you to efficiently control what is displayed on the screen.
Basic Conditional Rendering with if-else
In React, you can use traditional JavaScript if-else
statements to conditionally render components or elements. However, this typically works best when you’re rendering entire components based on conditions.
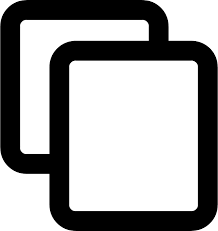
import React, { useState } from 'react';
function WelcomeMessage() {
const [isLoggedIn, setIsLoggedIn] = useState(false);
if (isLoggedIn) {
return Welcome Back!
;
} else {
return Please Log In
;
}
}
export default WelcomeMessage;
In this example, the component renders either "Welcome Back!" or "Please Log In" depending on the value of the isLoggedIn
state.
Conditional Rendering with ternary Operator
The ternary operator
is a concise way to implement conditional rendering. It’s a shorthand for if-else
and is especially useful when you need to render small snippets of JSX based on a condition.
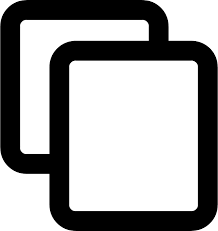
import React, { useState } from 'react';
function WelcomeMessage() {
const [isLoggedIn, setIsLoggedIn] = useState(false);
return (
{isLoggedIn ? "Welcome Back!" : "Please Log In"}
);
}
export default WelcomeMessage;
Here, the ternary operator is used to check the value of isLoggedIn
and display either "Welcome Back!" or "Please Log In" accordingly.
Logical && Operator for Conditional Rendering
Another common pattern for conditional rendering in React is using the logical &&
operator. This allows you to conditionally render an element if the condition is true, and nothing if it's false. This method is helpful when you only want to display something under a specific condition.
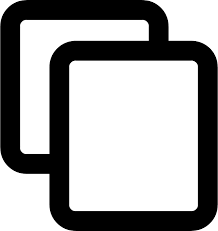
import React, { useState } from 'react';
function Notification() {
const [unreadMessages, setUnreadMessages] = useState(0);
return (
{unreadMessages > 0 && You have {unreadMessages} unread messages
}
);
}
export default Notification;
In this example, the notification message is only rendered if unreadMessages
is greater than 0. If it is 0, nothing is rendered.
Switch-Case for Conditional Rendering
You can also use the switch-case
statement for more complex conditional rendering. This method is especially useful when you have multiple conditions to check and want to render different components or content based on each case.
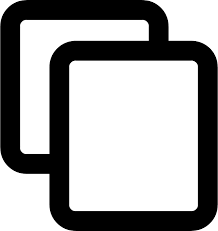
import React, { useState } from 'react';
function UserRole() {
const [role, setRole] = useState('guest');
let message;
switch (role) {
case 'admin':
message = Welcome Admin!
;
break;
case 'member':
message = Welcome Member!
;
break;
default:
message = Welcome Guest!
;
break;
}
return {message};
}
export default UserRole;
In this example, the switch-case
statement checks the value of the role
state and renders different messages depending on whether the user is an "admin", "member", or "guest".
Rendering Lists Conditionally
Sometimes you need to render a list of elements conditionally. The map
function can be used to loop through arrays and render components or elements based on conditions. Here's an example of rendering a list of items only if the array is not empty:
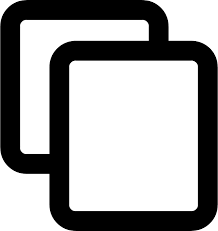
import React, { useState } from 'react';
function ItemList() {
const [items, setItems] = useState(['Apple', 'Banana', 'Orange']);
return (
{items.length > 0 ? (
{items.map(item => - {item}
)}
) : (
No items available
)}
);
}
export default ItemList;
In this example, the list of items is rendered only if the items
array contains elements. Otherwise, a message is displayed indicating that no items are available.
Conditional Rendering with React Hooks
Conditional rendering also works well with React hooks. The state can be used to control the flow of conditional rendering in function components. For instance, you can toggle visibility by updating state based on user interaction:
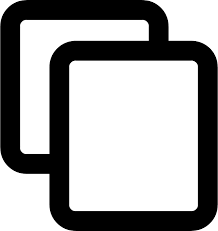
import React, { useState } from 'react';
function ToggleVisibility() {
const [isVisible, setIsVisible] = useState(false);
return (
<button onClick={() => setIsVisible(!isVisible)}>Change Name</button>
{isVisible ? 'Hide' : 'Show'} Content
{isVisible && This is some toggleable content!
}
);
}
export default ToggleVisibility;
In this example, clicking the button toggles the isVisible
state, which controls whether the content is shown or hidden.
Conclusion
Conditional rendering is a powerful feature in React that allows you to display different UI elements based on specific conditions. Whether you use traditional if-else
statements, ternary operators, or logical operators, React gives you flexibility in deciding what gets rendered. You can also use more complex patterns like switch-case
or render lists conditionally. Mastering conditional rendering is key to building dynamic, interactive React applications.
Lists and Keys in React
In React, lists allow you to render multiple components or elements dynamically. To efficiently update and manage lists of items in a React application, React uses keys. Keys are unique identifiers that help React keep track of each element in the list, improving performance and avoiding potential issues when updating the list.
Rendering Lists in React
Rendering lists in React is simple using the JavaScript map()
function. The map()
function allows you to iterate over an array and return an array of components or elements. This is very useful when you need to display a list of dynamic items.
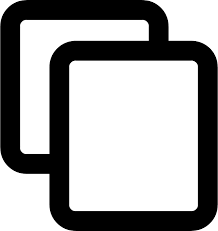
import React from 'react';
function GroceryList() {
const groceries = ['Apples', 'Bananas', 'Oranges'];
return (
{groceries.map((item, index) => (
- {item}
))}
);
}
export default GroceryList;
In this example, the map()
function is used to iterate over the groceries
array and render a li
element for each item. Each li
element is given a key
prop to help React identify and manage the list items.
Why Do We Need Keys?
Keys help React efficiently manage the virtual DOM during updates. When items in a list change, React can use keys to determine which items have changed, been added, or been removed. Without keys, React would have to re-render the entire list, causing unnecessary performance overhead.
Keys should be unique among siblings but don’t need to be globally unique across the entire app. The key
attribute helps React distinguish between elements in an array.
Using Unique Keys
It’s recommended to use a unique identifier for the key
prop. The most common approach is to use an ID from the data you are rendering. Using array indices as keys is not ideal because it can lead to issues when the list is re-ordered or modified.
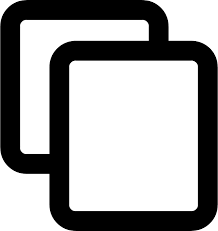
import React from 'react';
function UserList() {
const users = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Alice' },
{ id: 3, name: 'Bob' }
];
return (
{users.map(user => (
- {user.name}
))}
);
}
export default UserList;
In this example, the key
prop is set to the id
of each user. This ensures that each li
element has a unique identifier, which helps React manage the list updates efficiently.
Dynamic Lists with User Input
You can also dynamically add or remove items from a list in React. By updating the state, the component re-renders with the new list of items. Here's an example where users can add items to a shopping list:
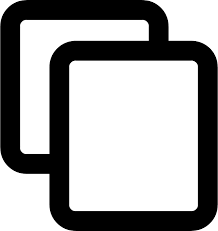
import React, { useState } from 'react';
function ShoppingList() {
const [items, setItems] = useState([]);
const [newItem, setNewItem] = useState('');
const addItem = () => {
setItems([...items, newItem]);
setNewItem('');
};
return (
<input
type="text"
value={newItem}
onChange={(e) => setNewItem(e.target.value)}
placeholder="Add an item"
/>
{items.map((item, index) => (
- {item}
))}
);
}
export default ShoppingList;
In this example, a text input and button are used to add new items to the shopping list. The list is rendered dynamically based on the items stored in the component's state.
Removing Items from the List
You can also remove items from a list by updating the state. Here’s an example where users can delete items from the list:
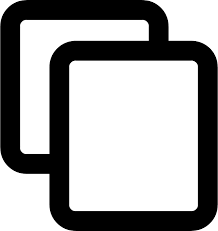
import React, { useState } from 'react';
function ShoppingList() {
const [items, setItems] = useState(['Apples', 'Bananas', 'Oranges']);
const removeItem = (itemToRemove) => {
setItems(items.filter(item => item !== itemToRemove));
};
return (
{items.map((item, index) => (
-
{item}
<button onClick={() => removeItem(item)}>Change Name</button>
))}
);
}
export default ShoppingList;
In this example, each list item is rendered with a delete button. When clicked, the corresponding item is removed from the list by updating the state.
Key Considerations
Here are some key things to consider when using lists and keys in React:
- Keys must be unique among siblings but don’t need to be globally unique.
- Always provide a
key
prop when rendering lists of elements to improve performance and avoid rendering issues. - Don’t use indices as keys if the list can change dynamically (e.g., items are added or removed).
- Keys help React identify which items have changed, been added, or been removed, improving performance during updates.
Conclusion
Lists and keys are a fundamental concept in React. Using keys helps React optimize updates to lists, making sure that only the necessary elements are re-rendered. By using the map()
function to render lists and ensuring each item has a unique key, you can create dynamic, efficient applications that can handle changing data smoothly.
Component Lifecycle Methods (Class Components)
In React, class components provide several lifecycle methods that allow you to hook into different stages of a component's life. These methods are important for managing tasks like fetching data, adding event listeners, or cleaning up resources when a component is no longer needed. The lifecycle methods are divided into three main phases: Mounting, Updating, and Unmounting.
Lifecycle Phases
- Mounting: The phase where the component is being created and inserted into the DOM.
- Updating: The phase where the component is being re-rendered due to changes in props or state.
- Unmounting: The phase where the component is being removed from the DOM.
Common Lifecycle Methods
Here are some of the most commonly used lifecycle methods in class components:
- componentDidMount: Called once the component has been rendered to the screen. This is a good place to initiate AJAX requests or set up subscriptions.
- shouldComponentUpdate: Determines whether a component should re-render when its state or props change. Returns a boolean value.
- componentDidUpdate: Called immediately after updating occurs. It is useful for working with the DOM after updates or making further API calls.
- componentWillUnmount: Called just before the component is removed from the DOM. It is typically used for cleanup tasks like invalidating timers or cancelling network requests.
Code Example: Class Component Lifecycle Methods
Below is an example of a class component with common lifecycle methods:
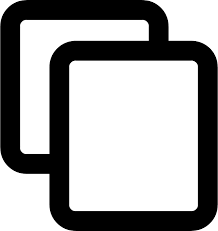
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
componentDidMount() {
console.log('Component has mounted!');
// Simulate an API call
setTimeout(() => {
this.setState({ count: 5 });
}, 2000);
}
shouldComponentUpdate(nextProps, nextState) {
return nextState.count !== this.state.count;
}
componentDidUpdate(prevProps, prevState) {
console.log('Component has updated!');
if (prevState.count !== this.state.count) {
console.log('Count has changed:', this.state.count);
}
}
componentWillUnmount() {
console.log('Component is being removed from the DOM');
}
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>Increment</button>
</div>
);
}
}
export default MyComponent;
In this example, the class component MyComponent
uses the componentDidMount
, shouldComponentUpdate
, componentDidUpdate
, and componentWillUnmount
lifecycle methods:
componentDidMount
: Initializes the state after the component has mounted, simulating an API call by updating the state after 2 seconds.shouldComponentUpdate
: Prevents unnecessary re-renders by only allowing updates when the count state has changed.componentDidUpdate
: Logs a message when the component updates and checks if the count has changed.componentWillUnmount
: Logs a message when the component is about to be removed from the DOM.
Lifecycle Method Usage
Lifecycle methods are helpful for managing side effects and optimizing performance. Here are some typical use cases:
- componentDidMount: Ideal for making API calls, setting up subscriptions, or any task that requires interaction with the DOM or external data once the component is rendered.
- shouldComponentUpdate: Useful for performance optimization by preventing unnecessary re-renders. Only return
true
if the component should re-render. - componentDidUpdate: Useful for handling operations that need to be performed after an update, such as fetching new data based on props or state changes.
- componentWillUnmount: Important for cleanup, such as removing event listeners, invalidating timers, or clearing network requests.
Conclusion
Lifecycle methods in class components are essential for managing side effects, fetching data, and handling cleanup in React. While function components with hooks have become more popular for managing state and side effects, class components are still an important part of React, especially for legacy codebases.
Using useEffect Hook for Side Effects
The useEffect
hook is one of the most commonly used hooks in React. It allows you to perform side effects in function components, such as fetching data, directly manipulating the DOM, or setting up subscriptions. Before the introduction of hooks, class components were the only way to manage side effects using lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
What is a Side Effect?
A side effect is any operation that interacts with something outside the component, such as:
- Fetching data from an API
- Directly manipulating the DOM
- Setting up subscriptions
- Timers (like
setTimeout
orsetInterval
)
Using the useEffect Hook
The useEffect
hook accepts a function that will run after the render is completed. This hook can also clean up after itself when the component unmounts or when specific dependencies change.
Basic Syntax
The basic syntax of useEffect
looks like this:
useEffect(() => {
// Side effect code goes here
}, [dependencies]);
- The first argument is a function where the side effect will be performed.
- The second argument is an optional array of dependencies. The effect will only run when the values in this array change.
Code Example: Using useEffect for Fetching Data
Here is an example of how you can use useEffect
to fetch data from an API when the component mounts:
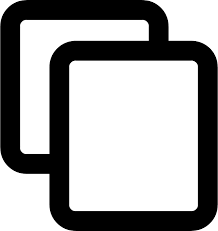
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => setData(data));
}, []); // Empty array means this effect runs only once after the first render
return (
<div>
<h1>Fetched Data</h1>
<ul>
{data ? data.map(item => (
<li key={item.id}>{item.title}</li>
)) : <p>Loading...</p>}
</ul>
</div>
);
}
export default DataFetcher;
In this example, the useEffect
hook is used to fetch data from an API when the component is mounted. The empty dependency array []
ensures that the effect runs only once, similar to the behavior of componentDidMount
in class components.
Cleaning Up with useEffect
In some cases, you may need to clean up resources (e.g., canceling a network request, clearing a timer) when the component unmounts or when a dependency changes. You can return a cleanup function from the useEffect
callback:
useEffect(() => {
// Setup code (side effect)
return () => {
// Cleanup code
};
}, [dependencies]);
Code Example: Setting Up and Cleaning Up a Timer
Here is an example of using useEffect
to set up and clean up a timer:
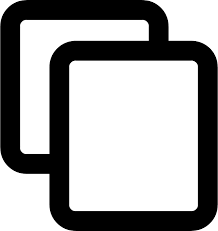
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const timer = setInterval(() => {
setSeconds(prevSeconds => prevSeconds + 1);
}, 1000);
// Cleanup function to clear the timer when the component unmounts
return () => clearInterval(timer);
}, []); // Empty array means this effect runs only once after the first render
return (
<div>
<h1>Timer: {seconds} seconds</h1>
</div>
);
}
export default Timer;
In this example, a timer is created when the component mounts, and the cleanup function clearInterval
is called when the component unmounts to prevent memory leaks.
Dependencies in useEffect
When you provide a second argument to useEffect
, you specify the dependencies of the effect. The effect will run again if any of the dependencies change between renders. If the array is empty, the effect will run only once after the initial render, similar to componentDidMount
.
Code Example: Using Dependencies in useEffect
Here is an example where we fetch data based on a prop that changes:
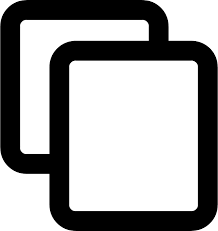
import React, { useState, useEffect } from 'react';
function UserProfile({ userId }) {
const [profile, setProfile] = useState(null);
useEffect(() => {
fetch(`https://jsonplaceholder.typicode.com/users/${userId}`)
.then(response => response.json())
.then(data => setProfile(data));
}, [userId]); // The effect will run every time the userId prop changes
return (
<div>
{profile ? (
<div>
<h1>{profile.name}</h1>
<p>{profile.email}</p>
</div>
) : <p>Loading profile...</p>}
</div>
);
}
export default UserProfile;
In this example, the useEffect
hook fetches data for a user based on the userId
prop. Whenever the userId
changes, the effect will re-run and fetch the new user's data.
Conclusion
The useEffect
hook is a powerful tool for managing side effects in functional components. It allows you to handle tasks like data fetching, subscriptions, and cleanup in a declarative and concise way. By specifying dependencies, you can control when the effect should be triggered, making it an essential part of React functional components.
Using useState Hook for State Management
The useState
hook is one of the most commonly used hooks in React. It allows functional components to have their own state, enabling them to react to user input, make updates, and render new UI based on the state changes. Prior to hooks, state was only available in class components, but with the useState
hook, functional components can now also manage state.
What is State in React?
State in React refers to the data or variables that determine how a component behaves or renders. State is mutable, meaning it can change over time, and when it changes, the component re-renders to reflect the updates.
Basic Syntax of useState
The useState
hook takes an initial state as an argument and returns an array with two elements:
- The current state value
- A function that updates the state
The basic syntax of useState
looks like this:
const [state, setState] = useState(initialState);
state
is the current state value.setState
is the function used to update the state.initialState
is the initial value of the state.
Code Example: Using useState for Managing Counter
Here is an example of how you can use useState
to manage a simple counter:
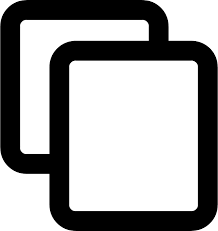
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // Initial state is set to 0
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
}
export default Counter;
In this example, we have a counter that starts with an initial state of 0. When the user clicks the "Increment" button, the state is updated by 1 using the setCount
function, and similarly for the "Decrement" button.
Updating State with a Function
If the new state depends on the previous state, it's better to pass a function to the setState
function. This ensures that React uses the most recent state when updating it:
setCount(prevCount => prevCount + 1);
Code Example: Updating State Based on Previous State
Here's an example where we update the state based on the previous state:
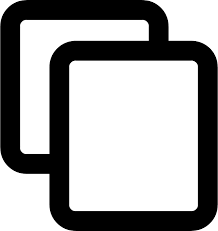
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(prevCount => prevCount + 1); // Update based on previous state
};
const decrement = () => {
setCount(prevCount => prevCount - 1); // Update based on previous state
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
In this example, the increment
and decrement
functions use the previous state to update the count value, which is more reliable in cases where multiple state updates may be triggered in the same render cycle.
State for Multiple Values
In cases where you need to manage multiple pieces of state, you can use multiple calls to useState
, each with its own state variable and update function:
const [count, setCount] = useState(0);
const [name, setName] = useState("John");
Code Example: Managing Multiple States
Here is an example of managing multiple state variables:
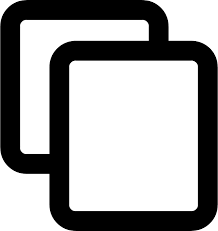
import React, { useState } from 'react';
function User() {
const [name, setName] = useState("John");
const [age, setAge] = useState(25);
return (
<div>
<h1>Name: {name}</h1>
<p>Age: {age}</p>
<button onClick={() => setAge(age + 1)}>Increase Age</button>
<button onClick={() => setName("Jane")}>Change Name</button>
</div>
);
}
export default User;
In this example, we manage the name
and age
states separately. Clicking the buttons updates the respective states without affecting the other.
Conclusion
The useState
hook is a powerful tool in React for managing state in functional components. It allows for easy state management without needing class components. By understanding how to use useState
and its variations, you can efficiently manage dynamic data in your components and build interactive and responsive user interfaces.
Handling Forms in React
Forms are a crucial part of web applications, allowing users to input data. In React, forms are managed using controlled components, where React is in charge of maintaining the state of the form elements. This provides better control and flexibility when working with user input and form submission.
Controlled Components
A controlled component is a form element whose value is controlled by the state in React. In other words, the value of the input field is stored in the component’s state, and React takes care of updating and managing that state.
Basic Syntax for Controlled Components
The basic syntax for a controlled component involves binding the value of the input field to the state and updating the state using an event handler:
const [value, setValue] = useState(''); // Initialize state
const handleChange = (event) => {
setValue(event.target.value); // Update state with input value
};
return (
<input
type="text"
value={value} // Bind value to state
onChange={handleChange} // Handle input change
/>
);
Code Example: Handling User Input
Here’s an example of how you can handle user input in a controlled form component:
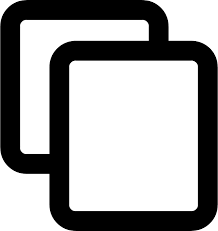
import React, { useState } from 'react';
function UserForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (event) => {
event.preventDefault(); // Prevent form submission
alert(`Name: {name}, Email: {email}`); // Display input values
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div>
<label>Email:</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<button type="submit">Submit</button>
</form>
);
}
export default UserForm;
In this example, we have two controlled inputs: one for the name and one for the email. The onChange
event updates the state as the user types, and the form submission is handled by the handleSubmit
function.
Handling Multiple Form Fields
If your form has multiple fields, you can manage them using multiple useState
calls or by using a single state object that holds all form values. Let’s explore both approaches:
Using Multiple useState Calls
const [name, setName] = useState('');
const [email, setEmail] = useState('');
Using a Single State Object
const [formData, setFormData] = useState({ name: '', email: '' });
const handleInputChange = (event) => {
const { name, value } = event.target;
setFormData(prevState => ({
...prevState,
[name]: value
}));
};
In the second approach, we use a single state object that holds all form values and update the state by spreading the previous state and modifying the specific field that changed.
Code Example: Using a Single State Object for Form
Here’s how you can handle multiple fields using a single state object:
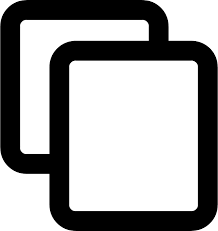
import React, { useState } from 'react';
function UserForm() {
const [formData, setFormData] = useState({ name: '', email: '' });
const handleInputChange = (event) => {
const { name, value } = event.target;
setFormData(prevState => ({
...prevState,
[name]: value
}));
};
const handleSubmit = (event) => {
event.preventDefault();
alert(`Name: ${formData.name}, Email: ${formData.email}`);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleInputChange}
/>
</div>
<div>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleInputChange}
/>
</div>
<button type="submit">Submit</button>
</form>
);
}
export default UserForm;
In this example, we use a single object formData
to store both the name and email, and we update the state dynamically using handleInputChange
.
Handling Form Submission
When a user submits a form, the default action (page reload) is prevented using event.preventDefault()
. You can then process the form data as needed, such as displaying it in an alert, sending it to a backend API, or updating the component state.
Validation and Error Handling
Form validation can be handled within the handleSubmit
function. You can check if the input fields are empty, contain invalid data, or meet certain criteria before submission. Here’s an example of simple validation:
const handleSubmit = (event) => {
event.preventDefault();
if (!formData.name || !formData.email) {
alert('Please fill in all fields');
return;
}
alert(`Name: ${formData.name}, Email: ${formData.email}`);
};
Conclusion
Handling forms in React is simple and powerful with controlled components. By using the useState
hook, you can manage form data, update input values, and perform validation. React provides an easy way to control form elements and ensures that the UI remains in sync with the state.
Controlled vs Uncontrolled Components
In React, components that manage their own state are known as controlled components. Uncontrolled components, on the other hand, allow the DOM to handle the state. The choice between controlled and uncontrolled components depends on the use case, with controlled components offering more flexibility and direct control over the input data.
Controlled Components
A controlled component is a form element whose value is controlled by React state. In this case, React is in charge of maintaining the state of the input field, and any changes to the input are reflected in the state of the component. This gives you complete control over the input and ensures that the UI is always in sync with the state.
Example of a Controlled Component
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
return (
<input
type="text"
value={value}
onChange={handleChange}
/>
);
In this example, the value
of the input field is controlled by the value
state variable. Every time the user types into the field, the handleChange
function is called, updating the state with the new value.
Uncontrolled Components
An uncontrolled component is a form element whose value is not directly controlled by React state. Instead, the DOM handles the form element's value. While React does not directly manage the state of the input field, you can access the value of an uncontrolled component using a ref
.
Example of an Uncontrolled Component
const inputRef = useRef(null);
const handleSubmit = (event) => {
event.preventDefault();
alert('Input value: ' + inputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
ref={inputRef}
/>
<button type="submit">Submit</button>
</form>
);
In this example, the inputRef
is used to access the value of the input field. When the form is submitted, the value of the input is retrieved using the ref
, and an alert is displayed with the current value of the input field.
When to Use Controlled vs Uncontrolled Components
There are a few considerations when deciding whether to use controlled or uncontrolled components:
- Controlled Components: Use when you need to manage and manipulate the form data directly in React state, perform validation, or conditionally render UI based on form values.
- Uncontrolled Components: Use when you do not need to interact with the form data directly or when performance is a concern, especially in large forms where the state may not need to be tracked constantly.
Advantages and Disadvantages
Here’s a quick comparison of controlled and uncontrolled components:
Aspect | Controlled Components | Uncontrolled Components |
---|---|---|
State Management | React state manages the form data. | The DOM manages the form data. |
Flexibility | More flexible and allows for easier manipulation of form data. | Less flexible, but may be simpler for basic forms. |
Performance | Can be less performant in large forms due to frequent state updates. | Better performance in large forms, as React does not need to track the form data constantly. |
Use Cases | Best for complex forms that require validation, conditionally rendered elements, or dynamic behavior. | Best for simple forms with less interaction required. |
Code Example: Controlled vs Uncontrolled Components
Let’s compare both controlled and uncontrolled components in a simple form:
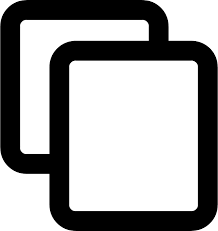
import React, { useState, useRef } from 'react';
// Controlled Component
function ControlledForm() {
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
return (
<form>
<input
type="text"
value={value}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
);
}
// Uncontrolled Component
function UncontrolledForm() {
const inputRef = useRef(null);
const handleSubmit = (event) => {
event.preventDefault();
alert('Input value: ' + inputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
ref={inputRef}
/>
<button type="submit">Submit</button>
</form>
);
}
Conclusion
Controlled components offer complete control over form data and are ideal for complex forms. On the other hand, uncontrolled components can be simpler to implement and may be appropriate for simpler forms where you don’t need to track the data constantly. Understanding when and how to use each type of component can help you make informed decisions when building React applications.
React Refs (useRef, createRef)
Refs in React provide a way to reference and interact with DOM elements or React components directly, bypassing the typical data flow. React offers two main ways to create refs: useRef
(for functional components) and createRef
(for class components). These refs are particularly useful when you need to access a DOM element directly for tasks such as focusing an input, measuring an element, or triggering animations.
useRef in Functional Components
useRef
is a hook introduced in React 16.8 that returns a mutable ref object. This object has a current
property that holds the value of the reference. Unlike state, updates to refs do not trigger re-renders of the component.
Example of useRef
import React, { useRef } from 'react';
function FocusInput() {
const inputRef = useRef(null);
const handleClick = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleClick}>Focus Input</button>
</div>
);
}
In this example, we use useRef
to create a reference to the input element. When the button is clicked, the handleClick
function triggers the focus
method on the input element, giving it focus.
createRef in Class Components
createRef
is used in class components to create a reference to a DOM element or a class component. Like useRef
, the reference object has a current
property that holds the reference value.
Example of createRef
import React, { Component } from 'react';
class FocusInput extends Component {
constructor(props) {
super(props);
this.inputRef = React.createRef();
}
handleClick = () => {
this.inputRef.current.focus();
};
render() {
return (
<div>
<input ref={this.inputRef} type="text" />
<button onClick={this.handleClick}>Focus Input</button>
</div>
);
}
}
In this class component example, we use createRef
in the constructor to create the reference and then use it to focus the input field when the button is clicked.
Common Use Cases for Refs
Refs are commonly used in the following scenarios:
- Accessing DOM Elements: You can use refs to interact with DOM elements directly, like focusing an input field or measuring the size of an element.
- Triggering Animations: Refs can be used to trigger animations on DOM elements without re-rendering the component.
- Integrating with Third-Party Libraries: Refs can be used to integrate React components with non-React libraries that require direct DOM manipulation.
Code Example: Using Refs to Trigger Animations
Here’s an example of using refs to trigger a simple CSS animation:
import React, { useRef } from 'react';
function AnimationComponent() {
const divRef = useRef(null);
const startAnimation = () => {
divRef.current.classList.add('animate');
};
return (
<div>
<div ref={divRef} className="box"></div>
<button onClick={startAnimation}>Start Animation</button>
</div>
);
}
In this example, the startAnimation
function adds the animate
class to the div
element, which can trigger a CSS animation. The reference to the div
is handled using useRef
.
Differences Between useRef and createRef
The key differences between useRef
and createRef
are:
- Usage:
useRef
is used in functional components, whilecreateRef
is used in class components. - Re-creation on Renders:
useRef
persists the reference across re-renders, whilecreateRef
creates a new reference on every render of the component. - Performance:
useRef
is more efficient in functional components as it does not recreate the ref object on each render.
Conclusion
Refs provide a way to access and manipulate DOM elements directly in React. Whether using useRef
in functional components or createRef
in class components, refs offer a powerful tool for handling tasks that require direct interaction with the DOM, like focusing inputs, triggering animations, or integrating third-party libraries.
Introduction to React Router for Navigation
React Router is a powerful library that allows you to implement routing in your React applications. It enables developers to navigate between different views or pages in a single-page application (SPA) without reloading the page. React Router helps in managing the browser history and allows you to conditionally render components based on the URL.
Why Use React Router?
React Router provides a declarative way to manage routing in a React app. It enables single-page applications (SPAs) to handle navigation without full-page reloads, making the user experience smoother. Some of the key benefits of React Router include:
- Dynamic route matching based on URL patterns.
- Easy handling of navigation state and history.
- Conditional rendering of components based on the route.
- Nested routes for structuring complex UIs.
Setting Up React Router
To start using React Router in a React application, follow these steps:
- Install React Router by running
npm install react-router-dom
in your project directory. - Wrap your app with
BrowserRouter
from React Router to enable routing in your app. - Define routes using
Route
components to map URLs to React components.
Example of Setting Up React Router
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function About() {
return <h2>About Page</h2>;
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
export default App;
In this example, we set up React Router in the App
component by using BrowserRouter
(aliased as Router
). We define two routes, one for the Home
page and one for the About
page, and use Link
components to create navigation links.
Understanding Route Matching
The Route
component in React Router is used to define the mapping between a URL path and a React component. It matches the current URL to the defined path
and renders the corresponding component. The exact
prop ensures that the route matches the path exactly, so only when the URL is exactly "/", the Home
component will render. Without the exact
prop, the route will match any path that starts with the specified path.
Example of Route Matching
function App() {
return (
<Router>
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/:id" component={Profile} />
</div>
</Router>
);
}
In this example, the URL path /profile/:id
will match any URL with a dynamic id
segment, rendering the Profile
component. The :id
is a dynamic parameter that can be accessed in the Profile
component using props.match.params.id
.
Navigation with Links
React Router provides the Link
component for navigation, which is preferred over the traditional <a>
tag. The Link
component ensures that navigation occurs without triggering a full-page reload, preserving the SPA behavior.
Example of Using Links
function NavBar() {
return (
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
);
}
Nested Routes
React Router supports nested routes, allowing you to render child components based on the parent route. This is useful for creating complex UIs where some parts of the page change based on the route while the rest remains the same.
Example of Nested Routes
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<Route path="/dashboard/settings" component={Settings} />
</div>
);
}
function Settings() {
return <h3>Settings Page</h3>;
}
function App() {
return (
<Router>
<div>
<Route path="/dashboard" component={Dashboard} />
</div>
</Router>
);
}
In this example, when the user navigates to /dashboard/settings
, the Settings
component will be rendered within the Dashboard
component.
Conclusion
React Router is an essential library for implementing navigation in single-page React applications. It provides powerful features for route matching, nested routes, and navigation, making it easy to build dynamic and responsive applications. By using BrowserRouter
, Route
, and Link
, you can handle complex navigation scenarios efficiently in your React app.
Setting Up React Router (BrowserRouter, Route, Link)
React Router is a powerful library that enables navigation in a React application. To set up React Router, you need to use the components BrowserRouter
, Route
, and Link
. Below are the steps to configure React Router in your app.
Installation
To use React Router, you must first install the react-router-dom
package. Run the following command in your project directory:
npm install react-router-dom
Setting Up BrowserRouter
The BrowserRouter
component is the parent component that provides routing functionality to your entire application. It should wrap the part of your app where you will be using the Route
components.
import React from 'react';
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<h1>Welcome to React Router!</h1>
</div>
</Router>
);
}
export default App;
In this example, we are wrapping the main content of the app with the BrowserRouter
component (aliased as Router
), which enables routing within the app.
Setting Up Route
The Route
component is used to define which component should be rendered when the URL matches a specified path. You can use the path
prop to specify the URL path and the component
prop to define which component should be displayed.
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function About() {
return <h2>About Page</h2>;
}
function App() {
return (
<Router>
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
export default App;
In this example, two routes are defined:
"/"
: Renders theHome
component."/about"
: Renders theAbout
component.
Using Links for Navigation
React Router provides the Link
component to enable navigation between routes without reloading the page. The to
prop of the Link
component specifies the target URL.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function About() {
return <h2>About Page</h2>;
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
export default App;
In this example, we use the Link
component to create navigation links to the Home
and About
pages. Clicking on these links will change the URL and render the respective component without refreshing the page.
Using Exact for Exact Matching
By default, React Router uses partial matching for routes. This means that the Route
component will match any URL that starts with the specified path
. To ensure that a route only matches when the URL is exactly equal to the path
, you can use the exact
prop.
function App() {
return (
<Router>
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
In this example, the exact
prop ensures that the Home
component only renders when the URL is exactly /
.
Conclusion
Setting up React Router is a straightforward process that involves installing the react-router-dom
package, using BrowserRouter
to wrap your app, defining routes using Route
, and enabling navigation with Link
. With these basic components, you can create dynamic and navigable React applications.
Dynamic Routing with React Router
Dynamic routing in React Router allows you to define routes that can change based on data, such as user input or URL parameters. This approach enables you to render different components based on dynamic content, making your app more flexible and responsive to user actions.
What is Dynamic Routing?
Dynamic routing refers to the ability to define routes that change based on data provided in the URL, typically through URL parameters. It allows you to create flexible and reusable components that can adapt based on the data passed to them via the URL, such as displaying different user profiles based on their unique IDs.
Setting Up Dynamic Routes with URL Parameters
React Router makes it easy to set up dynamic routes by using parameters in the path. These parameters are denoted by a colon (:
) followed by the parameter name in the Route
path.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function User({ match }) {
return <h2>User ID: {match.params.id}</h2>;
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/user/1">User 1</Link></li>
<li><Link to="/user/2">User 2</Link></li>
</ul>
</nav>
<Route path="/user/:id" component={User} />
</div>
</Router>
);
}
export default App;
In this example, we define a dynamic route with the path /user/:id
, where :id
is a URL parameter that will be replaced with the actual user ID. The User
component receives the dynamic id
from the match.params
object and displays it as part of the page.
Accessing URL Parameters in the Component
To access the dynamic parameter in the component, you can use the match
prop, which is automatically passed to the component by React Router when the route matches. The match.params
object contains the parameters defined in the route path.
function User({ match }) {
return <h2>User ID: {match.params.id}</h2>;
}
In this example, match.params.id
will contain the value of the user ID from the URL (e.g., 1
or 2
).
Nested Routes and Dynamic Routing
You can also have dynamic routes within other dynamic routes, creating nested routes. This allows you to organize your app's URL structure in a more hierarchical way.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Post({ match }) {
return <h3>Post ID: {match.params.postId}</h3>;
}
function User({ match }) {
return (
<div>
<h2>User ID: {match.params.id}</h2>
<nav>
<Link to={`${match.url}/post/1`}>Post 1</Link> |
<Link to={`${match.url}/post/2`}>Post 2</Link>
</nav>
<Route path={`${match.path}/post/:postId`} component={Post} />
</div>
);
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/user/1">User 1</Link></li>
<li><Link to="/user/2">User 2</Link></li>
</ul>
</nav>
<Route path="/user/:id" component={User} />
</div>
</Router>
);
}
export default App;
In this example, the User
component has a nested route for displaying posts. The match.url
and match.path
properties help create dynamic links and nested routes that depend on the current route parameters.
Redirecting Based on Conditions
In React Router, you can programmatically redirect users based on certain conditions using the Redirect
component or history.push
. This is useful when you want to redirect to a different page based on some logic, such as after form submission or authentication.
import React from 'react';
import { BrowserRouter as Router, Route, Redirect } from 'react-router-dom';
function App() {
const isAuthenticated = false;
return (
<Router>
<div>
<Route
path="/dashboard"
render={() => (
isAuthenticated ? <h2>Welcome to Dashboard</h2> : <Redirect to="/" />
)}
/>
</div>
</Router>
);
}
export default App;
If isAuthenticated
is false
, the user is redirected to the home page (/
) instead of accessing the /dashboard
route.
Conclusion
Dynamic routing in React Router is a powerful feature that allows you to create flexible, data-driven applications. By using URL parameters, nested routes, and conditional redirects, you can build sophisticated routing systems that adapt to user input and data changes. These techniques are essential for creating dynamic and interactive React applications.
Nested Routes and Route Parameters
In React Router, nested routes allow you to define routes inside other routes, enabling a hierarchical structure for your application. This is particularly useful for creating complex layouts where child components depend on the parent route.
What are Nested Routes?
Nested routes are routes that are defined within other routes. In React Router, you can nest Route
components inside each other to create a hierarchy. This is useful when you want to display different content based on the current route but maintain a common layout or structure.
Setting Up Nested Routes
To set up nested routes, you need to define a parent route and include Route
components for the child routes inside the parent component's render method. The child routes will render in a specific location within the parent component, typically inside a <div>
or another container element.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<nav>
<Link to="/dashboard/profile">Profile</Link> |
<Link to="/dashboard/settings">Settings</Link>
</nav>
<Route path="/dashboard/profile" component={Profile} />
<Route path="/dashboard/settings" component={Settings} />
</div>
);
}
function Profile() {
return <h3>User Profile</h3>;
}
function Settings() {
return <h3>Settings Page</h3>;
}
function App() {
return (
<Router>
<div>
<Route path="/dashboard" component={Dashboard} />
</div>
</Router>
);
}
export default App;
In this example, the Dashboard
component contains two nested routes: one for the user's profile and one for settings. The parent route /dashboard
will render the Dashboard
component, and depending on the link clicked, either the Profile
or Settings
component will render inside it.
Using Route Parameters in Nested Routes
Route parameters allow you to pass dynamic values through the URL. These parameters can be accessed within the component via the match.params
object. You can use route parameters in both parent and nested routes, making it possible to render different components based on dynamic data.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<nav>
<Link to="/dashboard/user/1">User 1</Link> |
<Link to="/dashboard/user/2">User 2</Link>
</nav>
<Route path="/dashboard/user/:id" component={User} />
</div>
);
}
function User({ match }) {
return <h3>User ID: {match.params.id}</h3>;
}
function App() {
return (
<Router>
<div>
<Route path="/dashboard" component={Dashboard} />
</div>
</Router>
);
}
export default App;
In this example, the /dashboard/user/:id
route is a nested route that takes a dynamic user ID as a parameter. When you click on the links for "User 1" or "User 2", the User
component will render with the corresponding user ID from the URL.
Accessing Route Parameters in a Nested Component
To access the route parameters in a nested component, you can use the match
prop, which is automatically passed by React Router. The match.params
object contains all the parameters from the route.
function User({ match }) {
return <h3>User ID: {match.params.id}</h3>;
}
Here, match.params.id
gives you access to the user ID passed in the URL, allowing the User
component to render dynamic content based on the parameter.
Nested Routes with Dynamic Parameters
You can also combine nested routes and dynamic parameters. For example, you could create a route that displays a user's profile or settings based on an ID passed through the URL.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Dashboard() {
return (
<div>
<h2>Dashboard</h2>
<nav>
<Link to="/dashboard/user/1/profile">User 1 Profile</Link> |
<Link to="/dashboard/user/2/settings">User 2 Settings</Link>
</nav>
<Route path="/dashboard/user/:id/profile" component={Profile} />
<Route path="/dashboard/user/:id/settings" component={Settings} />
</div>
);
}
function Profile({ match }) {
return <h3>Profile for User ID: {match.params.id}</h3>;
}
function Settings({ match }) {
return <h3>Settings for User ID: {match.params.id}</h3>;
}
function App() {
return (
<Router>
<div>
<Route path="/dashboard" component={Dashboard} />
</div>
</Router>
);
}
export default App;
In this example, the Dashboard
component has nested routes with dynamic user IDs. Depending on the URL, it renders either the Profile
or Settings
component for the corresponding user ID.
Conclusion
Nested routes and route parameters are powerful features in React Router that allow you to build complex, dynamic applications. By combining these techniques, you can easily create reusable, data-driven components that change based on the route parameters, making your app more interactive and user-friendly.
Redirects and Navigation in React
In React applications, navigation allows users to move between different views or pages, while redirects help automatically navigate users to a different route based on certain conditions. React Router provides tools for both, allowing you to manage user navigation and redirection effectively.
What is Navigation in React?
Navigation refers to the process of moving between different parts of an application. In React, this is typically handled using the Link
component from React Router. Link
allows you to create navigation links that update the URL without reloading the page, providing a seamless user experience.
Setting Up Navigation with React Router
To set up navigation in your React app, you can use the Link
component provided by React Router. It works similarly to an anchor tag (<a>
) but prevents the page from reloading.
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function About() {
return <h2>About Page</h2>;
}
function App() {
return (
<Router>
<div>
<nav>
<Link to="/">Home</Link> |
<Link to="/about">About</Link>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
export default App;
In this example, the Link
components are used to navigate between the "Home" and "About" pages. Clicking on either link will update the URL without reloading the page, and the corresponding component will be rendered.
Redirects in React
Redirects are useful when you want to automatically navigate the user to a different route based on certain conditions. For example, you might want to redirect a user after they successfully log in or when they attempt to visit a restricted page.
Using Redirect Component
React Router provides a Redirect
component to handle redirects. You can specify a to
prop with the target route to which you want to navigate the user. The redirect will happen immediately after the component renders.
import React from 'react';
import { BrowserRouter as Router, Route, Redirect } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function Login() {
return <h2>Login Page</h2>;
}
function Dashboard() {
return <h2>Dashboard Page (Protected)</h2>;
}
function App() {
const isAuthenticated = false; // Modify to simulate login status
return (
<Router>
<div>
<Route path="/" exact component={Home} />
<Route path="/login" component={Login} />
{/* Redirect to login if not authenticated */}
<Route
path="/dashboard"
render={() => isAuthenticated ? <Dashboard /> : <Redirect to="/login" />}
/>
</div>
</Router>
);
}
export default App;
In this example, the Dashboard
route will redirect the user to the /login
page if isAuthenticated
is false
. If the user is authenticated, they will be able to access the dashboard.
Using useHistory Hook for Programmatic Navigation
In addition to using Link
for navigation, you can also navigate programmatically using the useHistory
hook. This is useful when you need to perform navigation based on user actions or conditions within your component.
import React from 'react';
import { useHistory } from 'react-router-dom';
function Login() {
const history = useHistory();
const handleLogin = () => {
// Perform login logic
history.push('/dashboard'); // Redirect to dashboard after login
};
return (
<div>
<h2>Login Page</h2>
<button onClick={handleLogin}>Login</button>
</div>
);
}
export default Login;
Here, the useHistory
hook is used to navigate programmatically. After the user clicks the login button, the page will navigate to the /dashboard
route.
Using useNavigate Hook (React Router v6 and above)
In React Router v6 and above, the useHistory
hook has been replaced with useNavigate
. It works similarly, allowing you to navigate programmatically.
import React from 'react';
import { useNavigate } from 'react-router-dom';
function Login() {
const navigate = useNavigate();
const handleLogin = () => {
// Perform login logic
navigate('/dashboard'); // Redirect to dashboard after login
};
return (
<div>
<h2>Login Page</h2>
<button onClick={handleLogin}>Login</button>
</div>
);
}
export default Login;
In this example, the useNavigate
hook is used to navigate the user to the /dashboard
route after they click the login button.
Conclusion
Navigation and redirects are essential parts of building a seamless user experience in a React application. React Router provides several tools like Link
, Redirect
, and the useHistory
/useNavigate
hook to handle navigation and redirection in different scenarios. By leveraging these features, you can create dynamic, user-friendly applications with easy navigation flows.
Local Component State with useState
In React, useState
is a hook that allows you to add state to functional components. Before hooks were introduced, state management in React was only possible in class components. With the introduction of hooks, you can now manage state in functional components, making it easier to write and manage your components.
What is useState?
useState
is a React hook that lets you add state variables to functional components. It returns an array with two elements: the current state value and a function to update that state. This makes it easy to manage and update the state inside your component.
Setting Up useState in a Component
To use the useState
hook, you need to import it from React. You can then call it inside your functional component to define a state variable and a function to update it.
import React, { useState } from 'react';
function Counter() {
// Declare a state variable called 'count', with an initial value of 0
const [count, setCount] = useState(0);
return (
<div>
<h1>Current Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
In this example, we are using useState(0)
to initialize the count
state variable with an initial value of 0. The setCount
function is used to update the state when the button is clicked, which increments the counter by 1.
How useState Works
useState
works by returning an array. The first element of the array is the current state value, and the second element is a function to update the state. When the state is updated, React triggers a re-render of the component with the new state value.
Example: Toggling State with useState
Here is another example where we toggle a state value between true
and false
:
import React, { useState } from 'react';
function Toggle() {
const [isToggled, setIsToggled] = useState(false);
const handleToggle = () => {
setIsToggled(!isToggled); // Toggle between true and false
};
return (
<div>
<h1>The button is {isToggled ? 'ON' : 'OFF'}</h1>
<button onClick={handleToggle}>Toggle</button>
</div>
);
}
export default Toggle;
In this example, the isToggled
state variable starts with a value of false
. When the button is clicked, the handleToggle
function is called, which updates the state to the opposite value (from false
to true
or vice versa).
Multiple State Variables with useState
It’s possible to use multiple state variables in a single component. Each call to useState
creates a separate piece of state, so you can manage different states independently.
import React, { useState } from 'react';
function Form() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
alert(`Name: ${name}, Email: ${email}`);
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<label>Email:</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
);
}
export default Form;
In this example, we have two state variables: name
and email
. Each one is managed by a separate useState
hook. When the form is submitted, the values of both state variables are displayed in an alert.
Resetting State in useState
You can reset the state by calling the state setter function with the initial value or a new value. Here’s an example of resetting the state after an action is performed:
import React, { useState } from 'react';
function ResetCounter() {
const [count, setCount] = useState(0);
const resetCounter = () => {
setCount(0); // Reset the counter to 0
};
return (
<div>
<h1>Current Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={resetCounter}>Reset</button>
</div>
);
}
export default ResetCounter;
In this example, the resetCounter
function is used to reset the count
state back to 0. The button calls this function when clicked.
Conclusion
useState
is a fundamental hook in React that allows you to manage state in functional components. By using useState
, you can keep track of and update state values, manage user input, and create interactive components. The hook also supports using multiple state variables in a single component, making it versatile and powerful for building dynamic React applications.
Lifting State Up (Sharing State Between Components)
In React, "lifting state up" refers to the practice of moving state from a child component to a common parent component in order to share the state between multiple components. This is useful when you have multiple child components that need to access and modify the same piece of state.
Why Lift State Up?
When components need to share state, it’s better to lift the state up to their closest common ancestor rather than duplicating the state or relying on props. This allows the parent component to manage the shared state, and the child components can receive updated state through props.
How to Lift State Up?
Lifting state up involves three steps:
- Define the state in the parent component using
useState
. - Pass the state and the function to update it as props to the child components.
- In the child components, use the props to read and update the state.
Example: Lifting State Up Between Two Components
In this example, we have two child components: ChildA
and ChildB
. We lift the state that is shared between them up to the parent component, Parent
.
import React, { useState } from 'react';
// Child A component
function ChildA({ count, increment }) {
return (
<div>
<h2>Child A</h2>
<p>Count: {count}</p>
<button onClick={increment}>Increment in Child A</button>
</div>
);
}
// Child B component
function ChildB({ count, decrement }) {
return (
<div>
<h2>Child B</h2>
<p>Count: {count}</p>
<button onClick={decrement}>Decrement in Child B</button>
</div>
);
}
// Parent component
function Parent() {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div>
<h1>Lifting State Up Example</h1>
<ChildA count={count} increment={increment} />
<ChildB count={count} decrement={decrement} />
</div>
);
}
export default Parent;
In this example, the count
state is defined in the Parent
component. The increment
and decrement
functions are also passed down as props to ChildA
and ChildB
. Both child components can modify the state by calling the respective functions, and the state is shared between them.
Explanation of the Example
- The Parent
component holds the state (the count
) and the functions to modify that state, increment
and decrement
.
- The Parent
component passes the state and these functions as props to the child components: ChildA
and ChildB
.
- The child components use the props to display the current value of count
and trigger the respective actions on the state when the buttons are clicked.
When to Lift State Up
You should consider lifting state up when:
- Multiple components need access to the same state.
- You want to have a single source of truth for shared state.
- You want to centralize the logic for state updates in a parent component.
Best Practices for Lifting State Up
Here are some best practices for lifting state up:
- Keep the state in the closest common ancestor component.
- Pass only the necessary state and functions to child components as props.
- Consider using
useContext
for deeply nested components where lifting state up becomes cumbersome.
Conclusion
Lifting state up is a powerful concept in React that allows multiple components to share state by moving the state to their common ancestor. This approach helps you maintain a single source of truth and ensures that state is consistently updated across components. By following best practices, you can manage shared state efficiently in your React applications.
Global State Management with Context API
The Context API is a powerful feature in React that allows you to share state across multiple components without having to pass props manually at every level. It provides a way to manage global state in your application, making it easier to handle complex state sharing needs.
Why Use Context API?
The Context API is often used when we have data that needs to be accessed by many components at different levels of the component tree. Passing props down through each level can be cumbersome, especially when the component tree is deep. The Context API helps eliminate "prop drilling" by allowing you to share state globally without passing it explicitly through each component.
How the Context API Works
The Context API is composed of three main parts:
- Context: A container for the data you want to share.
- Provider: A component that supplies the state to its child components.
- Consumer: A component that consumes and uses the data from the context.
Creating a Context
To use the Context API, you first need to create a context object using React.createContext
. This context will hold the data that you want to share globally.
import React, { createContext, useState } from 'react';
// Create a Context
const MyContext = createContext();
// A Provider component
function MyProvider({ children }) {
const [state, setState] = useState('Hello, world!');
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
}
export { MyContext, MyProvider };
In this example, we create a context named MyContext
using createContext
. We define a MyProvider
component which holds the state and provides it to its children via the MyContext.Provider
component.
Using Context in Child Components
To consume the context in a child component, we can use the useContext
hook. This hook allows us to access the value passed from the provider.
import React, { useContext } from 'react';
import { MyContext } from './MyProvider';
function ChildComponent() {
const { state, setState } = useContext(MyContext);
return (
<div>
<h2>Context API Example</h2>
<p>State from Context: {state}</p>
<button onClick={() => setState('State updated!')}>Update State</button>
</div>
);
}
export default ChildComponent;
In this example, we use the useContext
hook to access the state and setState
function from the context. The child component can display the current state and update it when the button is clicked.
Putting It All Together
Now, let's combine the provider and consumer components in the main app to see how they work together.
import React from 'react';
import { MyProvider } from './MyProvider';
import ChildComponent from './ChildComponent';
function App() {
return (
<MyProvider>
<ChildComponent />
</MyProvider>
);
}
export default App;
Here, the MyProvider
wraps the ChildComponent
, providing the context value to it. Now, the ChildComponent
can access and update the state through the context without needing to pass props through intermediate components.
Benefits of Using Context API
- Global State Management: The Context API allows you to manage state globally without prop drilling, making state management easier in large applications.
- Component Decoupling: Components can access the state directly from the context, reducing the need for tightly coupled components.
- Cleaner Code: With the Context API, you can avoid the need to manually pass props at every level, leading to cleaner and more maintainable code.
When to Use Context API
The Context API is useful when:
- You have data that needs to be accessed by many components at different levels in the component tree.
- You want to avoid passing props down through every intermediate component.
- You need a simple and lightweight solution for global state management in small to medium-sized applications.
Limitations of Context API
- Performance Concerns: If the context value is updated frequently, it may cause unnecessary re-renders of all consuming components, leading to performance issues in large applications.
- Limited to Shallow State: Context is suitable for managing simple state. For more complex state management (e.g., with deep nesting, complex logic, etc.), a more robust solution like Redux may be more suitable.
Conclusion
The Context API is a powerful tool for managing global state in React. It allows you to share data across components without the need to pass props through each level of the component tree. While it is a great solution for small to medium-sized applications, it's important to be aware of potential performance issues for larger applications with frequent context updates.
Introduction to Redux for State Management
Redux is a state management library commonly used with React applications for managing global state in a predictable and centralized way. Unlike React's built-in state management, Redux allows you to manage the entire application’s state in a single store, making it easier to manage and debug complex state changes across large applications.
Why Use Redux?
As applications grow in complexity, passing data down through props becomes cumbersome. Redux provides a solution to this problem by centralizing the state and managing updates through actions and reducers, making state management more predictable and easier to debug.
Core Concepts of Redux
- Store: The store holds the entire state of the application in a single object, allowing components to access and update the state.
- Actions: Actions are plain JavaScript objects that describe the type of event and any data that needs to be sent to the reducer to update the state.
- Reducers: Reducers are functions that receive the current state and an action and return a new state based on the action type.
Setting Up Redux in a React Application
To use Redux in a React app, you need to install the following dependencies:
npm install redux react-redux
After installing Redux, you can create a store and connect it to your React application using the Provider
component from react-redux
.
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import App from './App';
// Example Reducer
const reducer = (state = { count: 0 }, action) => {
switch(action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
// Create the Redux store
const store = createStore(reducer);
// Wrap the App component with the Provider and pass the store
function Root() {
return (
<Provider store={store}>
<App />
</Provider>
);
}
export default Root;
Actions, Reducers, and Store
Let's break down the main concepts of Redux:
Actions
Actions are objects that describe what happened in the application. They must have a type
property and may optionally include additional data.
const incrementAction = { type: 'INCREMENT' };
const decrementAction = { type: 'DECREMENT' };
Reducers
Reducers are pure functions that specify how the state changes in response to an action. They receive two parameters: the current state and the action.
const reducer = (state = { count: 0 }, action) => {
switch(action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
Store
The store is an object that holds the entire application’s state. It is created by passing a reducer to the createStore
method.
const store = createStore(reducer);
Using useDispatch and useSelector Hooks
The react-redux
library provides two hooks: useDispatch
and useSelector
to interact with the Redux store in functional components.
useDispatch
The useDispatch
hook is used to dispatch actions to the Redux store.
import { useDispatch } from 'react-redux';
function Counter() {
const dispatch = useDispatch();
const increment = () => {
dispatch({ type: 'INCREMENT' });
};
const decrement = () => {
dispatch({ type: 'DECREMENT' });
};
return (
<div>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
useSelector
The useSelector
hook is used to access the state from the Redux store.
import { useSelector } from 'react-redux';
function Counter() {
const count = useSelector(state => state.count);
return (
<div>
<p>Count: {count}</p>
</div>
);
}
Middleware in Redux (redux-thunk, redux-saga)
Middleware is used in Redux to extend its capabilities, such as handling asynchronous actions. Two commonly used middleware in Redux are redux-thunk
and redux-saga
.
redux-thunk
redux-thunk
allows you to write action creators that return a function (instead of an action), making it easy to handle asynchronous actions like API calls.
import { applyMiddleware, createStore } from 'redux';
import thunk from 'redux-thunk';
// Example action with thunk
const fetchData = () => {
return function(dispatch) {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
dispatch({ type: 'FETCH_DATA_SUCCESS', payload: data });
});
};
};
const store = createStore(reducer, applyMiddleware(thunk));
redux-saga
redux-saga
is another middleware that helps manage side effects in Redux. It uses generator functions to handle asynchronous actions in a more declarative way.
import createSagaMiddleware from 'redux-saga';
import { applyMiddleware, createStore } from 'redux';
const sagaMiddleware = createSagaMiddleware();
const store = createStore(reducer, applyMiddleware(sagaMiddleware));
// Define the saga for handling async actions
function* rootSaga() {
yield takeEvery('FETCH_DATA_REQUEST', fetchDataSaga);
}
sagaMiddleware.run(rootSaga);
Conclusion
Redux provides a powerful way to manage global state across your React applications. By using actions, reducers, and a store, Redux enables you to keep track of your application’s state in a predictable way. The useDispatch
and useSelector
hooks allow for easy integration with functional components, while middleware like redux-thunk
and redux-saga
make handling side effects and asynchronous actions straightforward.
Controlled Components in React Forms
In React, controlled components are components that rely on React state to manage the form input values. When using controlled components, the value of the form element (like an input, select, or textarea) is controlled by the state of the component. This means that React is the "single source of truth" for the form's data.
How Controlled Components Work
In a controlled component, every time a user types into an input field, the value is updated in the component's state. React then re-renders the component with the updated state, making the UI reflect the new input value. This allows you to directly control the form data and implement logic like form validation, conditionally enabling buttons, and more.
Creating a Controlled Component
To create a controlled component in React, you need to:
- Bind the input field’s value to the component's state.
- Use the
onChange
event handler to update the state with the new value whenever the user types in the input.
Here's an example of a controlled component for a simple text input:
import React, { useState } from 'react';
function ControlledForm() {
// State to hold the input value
const [inputValue, setInputValue] = useState('');
// Handle the change event
const handleChange = (event) => {
setInputValue(event.target.value);
};
return (
<form>
<label>Name:</label>
<input
type="text"
value={inputValue}
onChange={handleChange}
/>
<p>You typed: {inputValue}</p>
</form>
);
}
export default ControlledForm;
Explanation of the Code
In the code above, the inputValue
state holds the current value of the input field. The value
attribute of the input
element is set to the state value, making the input field a controlled component. The onChange
event handler updates the state every time the user types in the input, keeping the form data synchronized with the component state.
Benefits of Controlled Components
- Centralized State: Since the form data is stored in React state, it’s easier to manage and manipulate.
- Validation and Logic: You can easily implement validation, conditional logic, and enable/disable form buttons based on the state.
- Consistent UI: React ensures that the UI always matches the state, making it more predictable.
Handling Multiple Inputs in a Controlled Component
If your form has multiple input fields, you can use a single state object to hold all the values and update them dynamically based on the input name.
import React, { useState } from 'react';
function ControlledForm() {
const [formData, setFormData] = useState({
name: '',
email: ''
});
// Handle input changes for multiple inputs
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
return (
<form>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
<p>Name: {formData.name}</p>
<p>Email: {formData.email}</p>
</form>
);
}
export default ControlledForm;
Explanation of the Code
In this example, we have two form fields: name
and email
. We store both values in a single formData
state object. The handleChange
function updates the corresponding value in the state based on the name
attribute of each input field.
Conclusion
Controlled components in React provide a powerful way to manage form input values. By synchronizing the form data with the component’s state, you can easily add functionality like form validation, conditional rendering, and more. Whether you are handling a single input or multiple inputs in a form, controlled components offer a clean and maintainable approach to managing form state in React applications.
Handling Form Inputs and Validation
In React, handling form inputs and validating them is a common task. React provides a way to manage form data with controlled components, and you can easily implement form validation to ensure the submitted data meets specific criteria. This section explains how to handle form inputs, validate user input, and provide feedback to users.
Handling Form Inputs
To handle form inputs in React, you typically use controlled components. Controlled components are form elements whose values are controlled by React state. You can capture user input and update the state using the onChange
event handler.
Here’s an example of handling a simple form input (like a text field) in a controlled component:
import React, { useState } from 'react';
function Form() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
return (
<form>
<label>Name:</label>
<input
type="text"
value={inputValue}
onChange={handleChange}
/>
<p>Entered Name: {inputValue}</p>
</form>
);
}
export default Form;
Form Validation in React
Form validation is an essential part of any form that collects user input. Validation ensures that the data submitted by the user is correct and meets certain criteria (e.g., correct format, required fields, etc.). In React, you can implement form validation by checking the input values when the user submits the form and providing feedback.
Here’s an example of how to validate a form that checks whether a user has entered a name and an email address:
import React, { useState } from 'react';
function ValidationForm() {
const [formData, setFormData] = useState({
name: '',
email: ''
});
const [errors, setErrors] = useState({
name: '',
email: ''
});
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
const validateForm = () => {
let formErrors = {};
if (!formData.name) {
formErrors.name = 'Name is required.';
}
if (!formData.email) {
formErrors.email = 'Email is required.';
} else if (!/\S+@\S+\.\S+/.test(formData.email)) {
formErrors.email = 'Email address is invalid.';
}
setErrors(formErrors);
return Object.keys(formErrors).length === 0;
};
const handleSubmit = (event) => {
event.preventDefault();
if (validateForm()) {
alert('Form submitted successfully!');
}
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
{errors.name && <p style={{ color: 'red' }}>{errors.name}</p>}
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
{errors.email && <p style={{ color: 'red' }}>{errors.email}</p>}
<button type="submit">Submit</button>
</form>
);
}
export default ValidationForm;
Explanation of the Code
In the above example, the form has two fields: name and email. We store the input values in the state using useState
. When the user submits the form, we call the validateForm
function to check for validation errors.
If any field is empty or the email format is invalid, we display an error message below the corresponding field. If no errors are found, the form is submitted successfully, and a success message is shown.
Handling Multiple Inputs with Validation
For forms with multiple inputs, you can dynamically handle validation for all fields by using a single validation function and checking each field’s value in the state.
import React, { useState } from 'react';
function MultiInputForm() {
const [formData, setFormData] = useState({
name: '',
email: '',
age: ''
});
const [errors, setErrors] = useState({});
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
const validateForm = () => {
let formErrors = {};
if (!formData.name) {
formErrors.name = 'Name is required';
}
if (!formData.email) {
formErrors.email = 'Email is required';
} else if (!/\S+@\S+\.\S+/.test(formData.email)) {
formErrors.email = 'Email is invalid';
}
if (!formData.age || isNaN(formData.age)) {
formErrors.age = 'Age must be a number';
}
setErrors(formErrors);
return Object.keys(formErrors).length === 0;
};
const handleSubmit = (event) => {
event.preventDefault();
if (validateForm()) {
alert('Form submitted successfully!');
}
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
{errors.name && <p style={{ color: 'red' }}>{errors.name}</p>}
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
{errors.email && <p style={{ color: 'red' }}>{errors.email}</p>}
<label>Age:</label>
<input
type="text"
name="age"
value={formData.age}
onChange={handleChange}
/>
{errors.age && <p style={{ color: 'red' }}>{errors.age}</p>}
<button type="submit">Submit</button>
</form>
);
}
export default MultiInputForm;
Conclusion
Handling form inputs and validation in React is straightforward with controlled components and state management. By validating user input, you can ensure that the data submitted by the user is correct and meets the required criteria. React makes it easy to implement form validation and provide real-time feedback to the user, ensuring a smooth user experience.
Form Submission in React
In React, submitting a form involves handling the form submission event, preventing the default form submission behavior, and then processing the form data. This section will explain how to handle form submissions, submit data to a server, and display the success or error messages after submission.
Handling Form Submission
In React, form submission is typically handled by adding an onSubmit
event handler to the <form>
element. We use the event.preventDefault()
method to prevent the page from reloading when the form is submitted.
Here’s an example of a basic form submission in React:
import React, { useState } from 'react';
function Form() {
const [formData, setFormData] = useState({
name: '',
email: ''
});
const [message, setMessage] = useState('');
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
const handleSubmit = (event) => {
event.preventDefault();
// Simulate a form submission (e.g., sending data to a server)
setMessage(`Form submitted successfully! Name: ${formData.name}, Email: ${formData.email}`);
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
{message && <p>{message}</p>}
);
}
export default Form;
Explanation of the Code
In the above example, we define a form with two fields: name and email. We use the useState
hook to manage the input values. When the user submits the form, the handleSubmit
function is triggered, and we use event.preventDefault()
to prevent the default form submission behavior (which would reload the page).
After the form is submitted, we simulate sending the form data to a server by updating the message
state and displaying a success message with the entered name and email.
Submitting Form Data to a Server
In a real-world scenario, after handling the form submission, you would typically send the form data to a server using tools like fetch()
, axios
, or XMLHttpRequest
. Here’s an example of submitting form data using fetch()
:
import React, { useState } from 'react';
function Form() {
const [formData, setFormData] = useState({
name: '',
email: ''
});
const [message, setMessage] = useState('');
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
const handleSubmit = (event) => {
event.preventDefault();
// Send form data to server
fetch('https://your-api-endpoint.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(formData),
})
.then((response) => response.json())
.then((data) => {
setMessage('Form submitted successfully!');
})
.catch((error) => {
setMessage('Error submitting the form. Please try again.');
});
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
{message && <p>{message}</p>}
);
}
export default Form;
Explanation of Sending Data to a Server
In this example, the form data is sent to a server using the fetch()
API. The data is sent as JSON in the request body. The fetch()
function returns a promise, which we handle using .then()
and .catch()
to manage the response and errors, respectively.
Handling Form Submission Responses
After the form is successfully submitted, you can display a success message, or in case of an error, you can show an error message. In the example above, we use the message
state to store the response and display it below the form.
Handling Submission with Async/Await
Instead of using .then()
and .catch()
, you can use async/await
syntax to handle asynchronous operations in a more readable way. Here’s how you can refactor the previous example using async/await
:
import React, { useState } from 'react';
function Form() {
const [formData, setFormData] = useState({
name: '',
email: ''
});
const [message, setMessage] = useState('');
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevData) => ({
...prevData,
[name]: value
}));
};
const handleSubmit = async (event) => {
event.preventDefault();
try {
const response = await fetch('https://your-api-endpoint.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(formData),
});
if (response.ok) {
setMessage('Form submitted successfully!');
} else {
setMessage('Error submitting the form. Please try again.');
}
} catch (error) {
setMessage('Error submitting the form. Please try again.');
}
};
return (
<form onSubmit={handleSubmit}>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
{message && <p>{message}</p>}
);
}
export default Form;
Conclusion
Form submission in React is essential for handling user input. React makes it easy to manage form data with controlled components and handle form submissions using onSubmit
event handlers. With React’s state management, you can easily capture and submit form data to a server while providing feedback to users based on the response.
Dynamic Form Fields and Multi-Step Forms
In some cases, you may need to render dynamic form fields based on user input or create multi-step forms where the form is broken down into multiple sections. React makes it easy to manage dynamic form fields and multi-step forms using state and event handling. This section will explain how to implement both dynamic form fields and multi-step forms in React.
Dynamic Form Fields
Dynamic form fields allow users to add or remove fields in a form based on their needs. For example, you could allow users to add multiple email addresses or phone numbers.
Here’s an example of dynamically adding form fields in React:
import React, { useState } from 'react';
function DynamicForm() {
const [fields, setFields] = useState([{ name: '', email: '' }]);
const handleChange = (event, index) => {
const { name, value } = event.target;
const updatedFields = [...fields];
updatedFields[index][name] = value;
setFields(updatedFields);
};
const handleAddField = () => {
setFields([...fields, { name: '', email: '' }]);
};
const handleRemoveField = (index) => {
const updatedFields = fields.filter((_, i) => i !== index);
setFields(updatedFields);
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(fields);
};
return (
<form onSubmit={handleSubmit}>
{fields.map((field, index) => (
<div key={index}>
<label>Name:</label>
<input
type="text"
name="name"
value={field.name}
onChange={(e) => handleChange(e, index)}
/>
<label>Email:</label>
<input
type="email"
name="email"
value={field.email}
onChange={(e) => handleChange(e, index)}
/>
<button type="button" onClick={() => handleRemoveField(index)}>Remove</button>
</div>
))}
<button type="button" onClick={handleAddField}>Add Field</button>
<button type="submit">Submit</button>
</form>
);
}
export default DynamicForm;
Explanation of Dynamic Form Fields
In the above example, we manage an array of form fields using React's useState
hook. The form fields are dynamically rendered based on the fields
state. The user can add new fields by clicking the "Add Field" button, which adds an object with empty values to the fields
array. The user can also remove a field by clicking the "Remove" button, which filters out the field from the state.
Multi-Step Forms
Multi-step forms are used when a form contains multiple sections that require different user inputs. Instead of displaying the entire form at once, you can break the form into smaller, more manageable sections and navigate between them using buttons or pagination.
Here’s an example of a simple multi-step form in React:
import React, { useState } from 'react';
function MultiStepForm() {
const [step, setStep] = useState(1);
const [formData, setFormData] = useState({
name: '',
email: '',
address: ''
});
const handleChange = (event) => {
const { name, value } = event.target;
setFormData({
...formData,
[name]: value
});
};
const handleNextStep = () => {
setStep(step + 1);
};
const handlePreviousStep = () => {
setStep(step - 1);
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
{step === 1 && (
<div>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
</div>
)}
{step === 2 && (
<div>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
</div>
)}
{step === 3 && (
<div>
<label>Address:</label>
<input
type="text"
name="address"
value={formData.address}
onChange={handleChange}
/>
</div>
)}
<div>
{step > 1 && (
<button type="button" onClick={handlePreviousStep}>Previous</button>
)}
{step > 3 && (
<button type="button" onClick={handleNextStep}>Next</button>
)}
{step === 3 && (
<button type="submit">Submit</button>
)}
</div>
</form>
);
}
export default MultiStepForm;
Explanation of Multi-Step Forms
In the above example, we use the step
state to keep track of the current step in the form. The form fields are conditionally rendered based on the value of step
. The user can navigate between steps using the "Next" and "Previous" buttons. When the user reaches the last step, the "Submit" button is displayed.
The handleChange
function updates the formData
state, while the handleNextStep
and handlePreviousStep
functions control the navigation between steps.
Conclusion
Dynamic form fields and multi-step forms are powerful features in React that allow you to create flexible and interactive forms. Dynamic form fields let users add or remove fields based on their needs, while multi-step forms break down a large form into smaller sections for better user experience. Both features can be easily managed using React's state and event handling mechanisms.
Using Libraries for Form Handling (Formik, React Hook Form)
Handling forms in React can be made more efficient and manageable using third-party libraries like Formik and React Hook Form. These libraries simplify form validation, error handling, and form submission. In this section, we will discuss both Formik and React Hook Form, and demonstrate how to use them to handle forms in React.
Formik
Formik is a popular library for managing forms in React. It simplifies handling form state, validation, and submission. Formik reduces the boilerplate code required for handling form inputs, making it easier to manage complex forms.
Installing Formik
To start using Formik in your React project, install it via npm:
npm install formik
Example: Simple Form with Formik
Here’s an example of using Formik for a simple form that handles name and email inputs, with basic validation:
import React from 'react';
import { Formik, Field, Form, ErrorMessage } from 'formik';
function MyForm() {
return (
<Formik
initialValues={{ name: '', email: '' }}
validate={values => {
const errors = {};
if (!values.name) {
errors.name = 'Name is required';
}
if (!values.email) {
errors.email = 'Email is required';
} else if (!/\S+@\S+\.\S+/.test(values.email)) {
errors.email = 'Invalid email address';
}
return errors;
}}
onSubmit={(values) => {
console.log(values);
}}
>
{() => (
<Form>
<div>
<label htmlFor="name">Name</label>
<Field type="text" id="name" name="name" />
<ErrorMessage name="name" component="div" />
</div>
<div>
<label htmlFor="email">Email</label>
<Field type="email" id="email" name="email" />
<ErrorMessage name="email" component="div" />
</div>
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
}
export default MyForm;
Explanation of Formik Example
In the above example, Formik is used to manage the form state and handle validation. The Formik
component takes an initialValues
prop to define the initial state of the form fields. The validate
function is used to validate the form data before submission, and the onSubmit
function handles the form submission.
The <Field>
component is used to link each input field to Formik’s internal state, and the <ErrorMessage>
component displays validation errors associated with each field.
React Hook Form
React Hook Form is another popular library for managing form state and validation. It provides a simpler and more performant approach to form handling in React by using React hooks. React Hook Form avoids unnecessary re-renders, making it more suitable for large forms.
Installing React Hook Form
To use React Hook Form in your project, install it via npm:
npm install react-hook-form
Example: Simple Form with React Hook Form
Here’s an example of a simple form using React Hook Form:
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label htmlFor="name">Name</label>
<input
type="text"
id="name"
{...register('name', { required: 'Name is required' })}
/>
{errors.name && <span>{errors.name.message}</span>}
</div>
<div>
<label htmlFor="email">Email</label>
<input
type="email"
id="email"
{...register('email', {
required: 'Email is required',
pattern: { value: /\S+@\S+\.\S+/, message: 'Invalid email address' }
})}
/>
{errors.email && <span>{errors.email.message}</span>}
</div>
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
Explanation of React Hook Form Example
In this example, React Hook Form is used to manage the form state and validation. The useForm
hook provides methods like register
to register input fields, handleSubmit
to handle form submission, and formState
to access validation errors.
Each input field is registered using the register
method, which takes the field name and validation rules as arguments. If there are validation errors, they are displayed below each input field.
Formik vs React Hook Form
Both Formik and React Hook Form are excellent choices for handling forms in React, but they differ in terms of API design and performance.
- Formik: Provides a more feature-rich API and is easier to use for beginners. It is suitable for smaller forms and provides built-in components like
<Field>
and<ErrorMessage>
. - React Hook Form: Offers better performance for large forms due to its minimal re-rendering approach. It provides a simpler API and uses React hooks for managing form state and validation.
Conclusion
Using libraries like Formik and React Hook Form can simplify form handling in React by abstracting away much of the boilerplate code required to manage form state, validation, and error handling. Both libraries offer unique advantages, so choose the one that best fits your project requirements.
The useEffect Hook and its Use Cases
The useEffect hook is one of the most commonly used hooks in React. It allows you to perform side effects in function components, such as fetching data, subscribing to events, or manually updating the DOM. useEffect is called after the render, making it ideal for operations that require interaction with the outside world, such as network requests and subscriptions.
What is the useEffect Hook?
The useEffect
hook is used to handle side effects in React function components. It is similar to lifecycle methods in class components, such as componentDidMount
, componentDidUpdate
, and componentWillUnmount
. However, it combines these lifecycle methods into one API.
Basic Syntax of useEffect
The basic syntax of the useEffect
hook is as follows:
useEffect(() => {
// Your side effect code here
}, [dependencies]);
Here:
- The first argument is the function that contains the side effect logic.
- The second argument is the dependency array. It specifies when the effect should be run. If the array is empty, the effect runs once after the initial render (like
componentDidMount
).
Common Use Cases of useEffect
1. Fetching Data
One of the most common use cases for useEffect
is fetching data from an API. The effect runs once after the component is mounted, and you can update the component state with the fetched data.
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data))
.catch(error => console.error('Error fetching data:', error));
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
{data ? (
<pre>{JSON.stringify(data, null, 2)}</pre>
) : (
<p>Loading data...</p>
)}
</div>
);
}
export default FetchData;
In this example, the useEffect
hook fetches data from the API once the component is mounted. The empty dependency array ensures that the effect only runs once.
2. Subscribing to Events
You can also use useEffect
to subscribe to events like window resizing, mouse movements, etc. This is useful for setting up event listeners.
import React, { useState, useEffect } from 'react';
function WindowSize() {
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight
});
useEffect(() => {
const handleResize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight
});
};
window.addEventListener('resize', handleResize);
// Cleanup function to remove the event listener
return () => {
window.removeEventListener('resize', handleResize);
};
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
Width: {windowSize.width}, Height: {windowSize.height}
</div>
);
}
export default WindowSize;
This example uses useEffect
to listen for window resizing events. The cleanup function removes the event listener when the component unmounts, preventing memory leaks.
3. Cleanup Operations
Sometimes, side effects may need to be cleaned up when the component is unmounted or when a dependency changes. For example, clearing timers or unsubscribing from events. You can return a cleanup function from the useEffect
hook to handle this.
import React, { useState, useEffect } from 'react';
function Timer() {
const [time, setTime] = useState(0);
useEffect(() => {
const timerId = setInterval(() => {
setTime(prevTime => prevTime + 1);
}, 1000);
// Cleanup function to clear the timer
return () => clearInterval(timerId);
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
Time: {time} seconds
</div>
);
}
export default Timer;
In this example, we set up a timer that updates the time every second. The cleanup function is used to clear the timer when the component unmounts.
4. Dependencies in useEffect
By adding dependencies to the dependency array, you can control when the effect should run. The effect will re-run whenever one of the dependencies changes.
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]); // Effect depends on 'count'
return (
<div>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
In this example, the useEffect
hook updates the document title whenever the count
changes. The effect depends on the count
state, so it will re-run whenever the count is updated.
Conclusion
The useEffect
hook is a powerful tool for managing side effects in React function components. It allows you to handle asynchronous operations, subscribe to events, and clean up resources when components are unmounted or updated. By understanding when and how to use useEffect
, you can build more efficient and organized React applications.
Fetching Data with useEffect
Fetching data from an API is one of the most common use cases for the useEffect
hook in React. The useEffect
hook allows you to run side effects in function components, such as fetching data when the component mounts or when certain dependencies change.
How to Fetch Data with useEffect
To fetch data using useEffect
, you typically make an asynchronous API request inside the effect function. Once the data is fetched, you can store it in the component's state and render it accordingly.
Basic Example of Fetching Data
Here is an example of how to fetch data from an API and display it in your component:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
// Fetch data from an API
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => {
setData(data); // Store the fetched data in state
setLoading(false); // Set loading to false
})
.catch((error) => {
setError(error); // Handle any errors
setLoading(false);
});
}, []); // Empty dependency array ensures the effect runs once after the initial render
if (loading) {
return <p>Loading data...</p>
}
if (error) {
return <p>Error fetching data: {error.message}</p>
}
return (
<div>
<h3>Fetched Data:</h3>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default FetchData;
In this example, the useEffect
hook fetches data from an API once the component mounts. The empty dependency array ensures the effect runs only once. The fetched data is stored in the component's state using the setData
function, and the component renders the data when it's available.
Handling Loading and Error States
In the example above, we also handle the loading and error states. When the data is being fetched, the loading
state is set to true
, and while it's fetching, we show a loading message. If there's an error during the fetch process, we handle it using a catch
block and show the error message to the user.
Refactoring with Async/Await
Instead of using the then
and catch
methods, you can also use the async/await
syntax to make the code cleaner:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
setLoading(false);
} catch (error) {
setError(error);
setLoading(false);
}
};
fetchData(); // Call the async function
}, []); // Empty dependency array ensures the effect runs once after the initial render
if (loading) {
return <p>Loading data...</p>
}
if (error) {
return <p>Error fetching data: {error.message}</p>
}
return (
<div>
<h3>Fetched Data:</h3>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default FetchData;
In this refactored example, we've used the async/await
syntax inside the useEffect
hook. This makes the code more readable and easier to manage, especially for larger async functions.
Conclusion
The useEffect
hook is a powerful tool for fetching data in React components. By using it effectively, you can fetch data when the component mounts, handle loading and error states, and update the UI with the fetched data. With the ability to use async/await, handling asynchronous operations in React has become cleaner and more efficient.
Handling Errors and Loading States in React
When working with asynchronous operations in React, such as fetching data from an API, it's crucial to handle loading and error states effectively to enhance user experience. React provides simple ways to manage these states using the useState
and useEffect
hooks.
Managing Loading States
A loading state is useful when you want to show a loading indicator or message while waiting for asynchronous data to load, such as when fetching data from an API. You can create a state variable to track whether data is being loaded or not and use it to conditionally render content.
Example of Handling Loading State
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
setData(data); // Store the fetched data
setLoading(false); // Set loading to false
})
.catch((error) => {
console.error('Error fetching data:', error);
setLoading(false); // Set loading to false even if there is an error
});
}, []); // Empty dependency array to run the effect once after initial render
if (loading) {
return <p>Loading data...</p>
}
return (
<div>
<h3>Fetched Data:</h3>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default FetchData;
In this example, while the data is being fetched, the loading state is set to true
. Once the data is fetched, the loading state is set to false
, and the fetched data is displayed. If an error occurs, the loading state is also set to false
, and you can handle errors in the catch
block.
Managing Error States
Error handling is crucial when working with APIs or any other asynchronous operations. If something goes wrong during an API call, you can catch the error and display an appropriate message to the user.
Example of Handling Error State
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
setData(data); // Store the fetched data
setLoading(false); // Set loading to false
})
.catch((error) => {
setError(error); // Store the error
setLoading(false); // Set loading to false
});
}, []); // Empty dependency array to run the effect once after initial render
if (loading) {
return <p>Loading data...</p>
}
if (error) {
return <p>Error fetching data: {error.message}</p>
}
return (
<div>
<h3>Fetched Data:</h3>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default FetchData;
In this updated example, we've added an error
state to store the error message. If an error occurs during the fetch operation, it will be caught in the catch
block, and the error message will be displayed to the user.
Combining Loading and Error States
It's common to combine both loading and error states in your components, especially when working with data fetching. This ensures that users are informed about the status of the operation and any potential issues.
Example of Combined Loading and Error Handling
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Failed to fetch');
}
return response.json();
})
.then(data => {
setData(data);
setLoading(false);
})
.catch((error) => {
setError(error);
setLoading(false);
});
}, []);
if (loading) {
return <p>Loading data...</p>
}
if (error) {
return <p>Error fetching data: {error.message}</p>
}
return (
<div>
<h3>Fetched Data:</h3>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default FetchData;
In this enhanced example, we perform an additional check on the response status. If the response is not OK (i.e., if the status code is not in the 200-299 range), an error is thrown. This ensures that even if the fetch request succeeds, we can still catch issues like a bad response from the server.
Conclusion
Handling loading and error states in React is essential for building smooth, user-friendly applications. By using useState
and useEffect
, you can easily manage these states while performing asynchronous operations, ensuring that users are always informed about the progress and issues related to data fetching or other side effects.
Cleaning Up Side Effects in useEffect
The useEffect hook is used to perform side effects in React components, but sometimes you need to clean up those side effects to avoid memory leaks or unintended behavior. This is especially important when dealing with subscriptions, event listeners, timers, or fetching data. React provides a simple way to handle cleanup in the useEffect
hook.
Why Clean Up Side Effects?
When side effects like event listeners, timers, or subscriptions are set up inside a component, they may not automatically be cleaned up when the component is unmounted. This can lead to memory leaks and performance issues. To prevent this, React allows us to define a cleanup function inside the useEffect
hook that will be executed when the component unmounts or when the effect dependencies change.
Basic Cleanup Syntax
The cleanup function is returned from the effect function. It will run when the component is about to unmount or before the effect is re-run due to a change in dependencies.
useEffect(() => {
// Side effect code here
return () => {
// Cleanup code here
};
}, [dependencies]);
Here:
- The first function is the side effect logic that runs after the render.
- The return function is the cleanup function, which runs when the component is unmounted or when the effect dependencies change.
Common Cleanup Use Cases
1. Clearing Timers
If you set up a timer (such as using setTimeout
or setInterval
), it’s important to clear the timer when the component unmounts to avoid memory leaks.
import React, { useState, useEffect } from 'react';
function Timer() {
const [time, setTime] = useState(0);
useEffect(() => {
const timerId = setInterval(() => {
setTime(prevTime => prevTime + 1);
}, 1000);
// Cleanup function to clear the timer
return () => clearInterval(timerId);
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
Time: {time} seconds
</div>
);
}
export default Timer;
In this example, we use setInterval
to increment the time every second. The cleanup function ensures that the timer is cleared when the component unmounts, preventing memory leaks.
2. Removing Event Listeners
When setting up event listeners (such as window.addEventListener
), it's important to remove them when the component unmounts to avoid memory leaks and unnecessary event handling.
import React, { useState, useEffect } from 'react';
function WindowSize() {
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight
});
useEffect(() => {
const handleResize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight
});
};
window.addEventListener('resize', handleResize);
// Cleanup function to remove the event listener
return () => {
window.removeEventListener('resize', handleResize);
};
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
Width: {windowSize.width}, Height: {windowSize.height}
</div>
);
}
export default WindowSize;
This example listens for window resizing events and updates the component state accordingly. The cleanup function removes the event listener when the component unmounts, ensuring no memory leaks or unnecessary event handling.
3. Cleanup with Subscriptions
If your component subscribes to some external data source or service (e.g., WebSocket or Firebase), you should unsubscribe when the component unmounts to avoid memory leaks.
import React, { useState, useEffect } from 'react';
function SubscribeData() {
const [data, setData] = useState(null);
useEffect(() => {
const subscribe = () => {
// Simulating a subscription to data
setInterval(() => {
setData('New Data');
}, 2000);
};
subscribe();
// Cleanup function to unsubscribe
return () => {
// Unsubscribe logic here (e.g., clearing interval)
};
}, []); // Empty dependency array ensures the effect runs once
return (
<div>
{data}
</div>
);
}
export default SubscribeData;
In this example, we simulate subscribing to new data. The cleanup function is where you would unsubscribe from the data source to avoid memory leaks when the component unmounts.
Using Cleanup to Handle Dependencies
If your effect depends on certain variables, you can also clean up before the effect runs again. For example, when the dependency changes, the cleanup function will run first to clean up the previous effect before running the new one.
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
const [interval, setIntervalValue] = useState(1000);
useEffect(() => {
const timerId = setInterval(() => {
setSeconds(prevSeconds => prevSeconds + 1);
}, interval);
// Cleanup function to clear the timer
return () => clearInterval(timerId);
}, [interval]); // The effect will run again if 'interval' changes
return (
<div>
Time: {seconds} seconds
<button onClick={() => setIntervalValue(500)}>Speed Up</button>
</div>
);
}
export default Timer;
In this example, the timer interval is controlled by the interval
state. When the interval
changes, the cleanup function is called to clear the previous timer before setting a new one. This ensures that the effect is properly cleaned up and updated.
Conclusion
Cleaning up side effects in React is crucial for optimizing performance and avoiding memory leaks. By using the cleanup function in the useEffect
hook, you can ensure that resources such as event listeners, timers, or subscriptions are properly cleaned up when the component unmounts or when dependencies change. This leads to more efficient and reliable React applications.
Debouncing and Throttling in React
In React, debouncing and throttling are techniques used to limit the rate at which a function is called, which can improve performance when dealing with events like scrolling, typing, or resizing. These techniques are essential when you need to prevent unnecessary function calls and reduce the number of re-renders in your React applications.
What is Debouncing?
Debouncing is a technique used to ensure that a function is only called after a certain amount of time has passed since the last call. It is useful in situations like handling user input (e.g., typing in a search field) where you want to wait until the user has finished typing before executing a function (e.g., making an API call).
What is Throttling?
Throttling is a technique used to limit the number of times a function is called over time. It ensures that a function is executed no more than once in a specified time interval. This is particularly useful in situations like scrolling, where you want to limit the frequency of function calls to avoid performance issues.
Debouncing Example in React
In this example, we will debounce the input field to delay the API call until the user stops typing for a specified duration.
import React, { useState, useEffect } from 'react';
function DebouncedInput() {
const [query, setQuery] = useState('');
const [debouncedQuery, setDebouncedQuery] = useState(query);
useEffect(() => {
const timer = setTimeout(() => {
setDebouncedQuery(query);
}, 1000); // Debounce for 1 second
return () => clearTimeout(timer); // Cleanup timer on component unmount or query change
}, [query]);
const handleChange = (event) => {
setQuery(event.target.value);
};
return (
<div>
<input
type="text"
value={query}
onChange={handleChange}
placeholder="Search..."
/>
Debounced Query: {debouncedQuery}
</div>
);
}
export default DebouncedInput;
In this example, we use setTimeout
in the useEffect
hook to implement debouncing. The timer is cleared and reset every time the user types, and the debounced query only updates after the user stops typing for 1 second.
Throttling Example in React
In this example, we will throttle the scroll event to limit the number of times the function is called while scrolling.
import React, { useState, useEffect } from 'react';
function ThrottledScroll() {
const [scrollPosition, setScrollPosition] = useState(0);
useEffect(() => {
const handleScroll = () => {
setScrollPosition(window.scrollY);
};
const throttle = () => {
let lastScrollTime = 0;
const throttleInterval = 1000; // Throttle to 1 second
return () => {
const now = Date.now();
if (now - lastScrollTime >= throttleInterval) {
handleScroll();
lastScrollTime = now;
}
};
};
window.addEventListener('scroll', throttle());
return () => {
window.removeEventListener('scroll', throttle());
};
}, []);
return (
<div>
<p>Scroll Position: {scrollPosition}</p>
</div>
);
}
export default ThrottledScroll;
In this example, we throttle the scroll event using a custom throttle
function. This ensures that the handleScroll
function is only called once every 1 second, regardless of how fast the user is scrolling.
Conclusion
Both debouncing and throttling are important techniques to optimize performance in React applications. Debouncing is useful when you want to delay the execution of a function until a certain amount of time has passed, while throttling is useful when you want to limit the frequency of function calls over time. By using these techniques in the right situations, you can improve the performance and responsiveness of your React applications.
Introduction to Context API
The Context API in React is a powerful feature that allows you to manage and share state across your component tree, without having to pass props down manually at every level. It provides a way to share values like authentication status, themes, and preferences globally, making it easier to manage state in a React application.
What is the Context API?
The Context API provides a way to share values between components without having to explicitly pass a prop through every level of the tree. This is especially useful for global state management, such as sharing user authentication data, themes, language preferences, or other global variables.
How to Use the Context API?
The Context API consists of three core components:
- React.createContext: This is used to create a context object that holds the shared state.
- Provider: This component provides the context value to the components that need access to it.
- Consumer: This component allows components to consume and access the context value.
Creating and Using Context
Let's walk through an example where we use the Context API to provide and consume a theme value.
import React, { useState, createContext, useContext } from 'react';
// Step 1: Create the context
const ThemeContext = createContext();
// Step 2: Create a provider component
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(prevTheme => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
// Step 3: Create a component that consumes the context
function ThemedComponent() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
}
// Step 4: Use the provider in the app
function App() {
return (
<ThemeProvider>
<ThemedComponent />
</ThemeProvider>
);
}
export default App;
In this example:
- We first create a context using
createContext
. - Then, we create a
ThemeProvider
component that holds thetheme
state and provides it to child components using theProvider
. - The
ThemedComponent
usesuseContext
to consume the context and display the current theme, along with a button to toggle the theme.
Context API without Consumer
Instead of using a Consumer
component, you can use the useContext
hook to access the context value directly in functional components.
import React, { useState, createContext, useContext } from 'react';
const ThemeContext = createContext();
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(prevTheme => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
function ThemedComponent() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
}
function App() {
return (
<ThemeProvider>
<ThemedComponent />
</ThemeProvider>
);
}
export default App;
Here, the useContext
hook is used to directly access the context value in the ThemedComponent
, eliminating the need for the Consumer
component.
Conclusion
The React Context API is a powerful tool for managing state across your application without having to pass props manually through every level of the component tree. It simplifies global state management and makes it easier to share data like themes, authentication status, and more. By using the Provider
and Consumer
components or the useContext
hook, you can efficiently manage and consume shared state in your React applications.
Creating and Using Context in React
The Context API in React provides a way to share values like themes, authentication status, or settings across your component tree without explicitly passing props down at every level. In this guide, we will walk through the process of creating and using context in a React application.
Steps for Creating and Using Context
We will follow these steps:
- Step 1: Create the context.
- Step 2: Create a provider component to pass the context values.
- Step 3: Consume the context values in a component.
Step 1: Create the Context
First, we create a context using createContext
. This function returns an object that we can use to provide and consume the context.
import React, { createContext } from 'react';
// Create the context
const ThemeContext = createContext('light'); // Default value is 'light'
The createContext
function takes an optional default value, which will be used if a component doesn't have a corresponding provider in the tree above it.
Step 2: Create a Provider Component
The provider component will allow us to pass the context value down to the components that need it. It uses the Provider
component of the context object to wrap the components that will consume the context.
import React, { useState } from 'react';
import { ThemeContext } from './ThemeContext'; // Importing the context
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(prevTheme => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
export default ThemeProvider;
In the above code:
- The
ThemeProvider
component manages the state of the theme and provides the context value. - The context value contains both the
theme
(light or dark) and atoggleTheme
function to change it.
Step 3: Consume the Context in Child Components
Now that we have a provider, we can use the context in any child component by using the useContext
hook or the Context.Consumer
component.
import React, { useContext } from 'react';
import { ThemeContext } from './ThemeContext'; // Import the context
function ThemedComponent() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
}
export default ThemedComponent;
Here, we use the useContext
hook to consume the context value. The component receives the theme
and toggleTheme
function from the context and renders them accordingly.
Step 4: Wrapping the App with the Provider
The ThemeProvider
component needs to wrap the part of the app that needs access to the context. In this case, we wrap the entire app with it so that any component can consume the theme.
import React from 'react';
import ThemeProvider from './ThemeProvider';
import ThemedComponent from './ThemedComponent';
function App() {
return (
<ThemeProvider>
<ThemedComponent />
</ThemeProvider>
);
}
export default App;
Here, the App
component is wrapped with the ThemeProvider
, allowing the ThemedComponent
to access the theme and toggle it.
Conclusion
Using the Context API in React is a great way to manage global state without having to pass props down manually. By creating a context and using the Provider
and useContext
(or Consumer
) components, we can easily share state across multiple components in a component tree. This is particularly useful for managing things like themes, authentication, and user preferences.
Passing Data with Context
The Context API is a powerful tool in React that allows you to pass data through the component tree without explicitly passing props down at every level. In this guide, we'll walk through the process of passing data using Context and how to make it easier to manage state across different components.
Steps for Passing Data with Context
We will follow these steps:
- Step 1: Create the context with a default value.
- Step 2: Provide the context value to the component tree.
- Step 3: Consume the context in child components.
Step 1: Create the Context
The first step is to create a context using the createContext
function. This context will hold the data we want to pass down the component tree.
import React, { createContext } from 'react';
// Create the context with a default value
const UserContext = createContext({ name: 'Guest', age: 0 });
In this example, we create a UserContext
with a default value that includes a name
and age
.
Step 2: Provide the Context Value
To pass data down to child components, we need to use the Provider
component of the context. The provider accepts a value
prop, which will be passed down to any components that consume this context.
import React, { useState } from 'react';
import { UserContext } from './UserContext'; // Import the context
function UserProvider({ children }) {
const [user, setUser] = useState({ name: 'John Doe', age: 25 });
return (
<UserContext.Provider value={user}>
{children}
</UserContext.Provider>
);
}
export default UserProvider;
In the UserProvider
component, we create a state variable user
that holds the current user's data. We pass this data as the value to the Provider
component.
Step 3: Consume the Context in Child Components
Now that the context is provided, we can consume it in child components using the useContext
hook. This allows us to access the context data in any component that is a descendant of the provider.
import React, { useContext } from 'react';
import { UserContext } from './UserContext'; // Import the context
function UserProfile() {
const user = useContext(UserContext);
return (
<div>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
</div>
);
}
export default UserProfile;
In this example, the UserProfile
component uses the useContext
hook to consume the UserContext
and display the name
and age
of the user.
Step 4: Wrapping the App with the Provider
To make the context available throughout the application, we need to wrap the components that need access to the context with the UserProvider
.
import React from 'react';
import UserProvider from './UserProvider';
import UserProfile from './UserProfile';
function App() {
return (
<UserProvider>
<UserProfile />
</UserProvider>
);
}
export default App;
In this example, the App
component is wrapped with the UserProvider
, so the UserProfile
component can access the user data via the context.
Conclusion
Passing data with Context allows you to manage global state in a more efficient way. Instead of drilling props down through multiple layers of components, you can use context to make values available to any component in the tree. This is especially useful for passing data like user information, themes, and settings across an app without prop drilling.
Using useContext
Hook for Accessing Context
In React, the useContext
hook provides an easy way to access context values in functional components. It eliminates the need for a nested structure to consume context, making your code cleaner and more readable.
Steps to Use useContext
Follow these steps to use the useContext
hook:
- Step 1: Create a context using
createContext
. - Step 2: Wrap the component tree with the context provider.
- Step 3: Access the context value using
useContext
.
Step 1: Create the Context
Start by creating a context using the createContext
function:
import React, { createContext } from 'react';
// Create a context for user data
const UserContext = createContext();
export default UserContext;
Step 2: Provide the Context
Wrap your components with the context provider and pass the value you want to share:
import React, { useState } from 'react';
import UserContext from './UserContext';
function UserProvider({ children }) {
const [user, setUser] = useState({ name: 'Jane Doe', role: 'Admin' });
return (
<UserContext.Provider value={user}>
{children}
</UserContext.Provider>
);
}
export default UserProvider;
Step 3: Consume the Context with useContext
Now, you can use the useContext
hook to access the context value inside any child component:
import React, { useContext } from 'react';
import UserContext from './UserContext';
function UserProfile() {
const user = useContext(UserContext);
return (
<div>
<h3>User Profile</h3>
<p>Name: {user.name}</p>
<p>Role: {user.role}</p>
</div>
);
}
export default UserProfile;
Step 4: Wrap the App with the Provider
Ensure that the components consuming the context are wrapped within the provider:
import React from 'react';
import UserProvider from './UserProvider';
import UserProfile from './UserProfile';
function App() {
return (
<UserProvider>
<UserProfile />
</UserProvider>
);
}
export default App;
Benefits of useContext
- Eliminates the need for nested render props or
Consumer
components. - Makes the code cleaner and easier to read.
- Allows you to access context values directly in functional components.
Conclusion
The useContext
hook simplifies accessing context values in React functional components. It improves code readability and reduces complexity, especially in large applications with deeply nested components.
Performance Considerations and Optimization
Optimizing performance in React applications is crucial for creating fast, responsive user interfaces. React provides several tools and techniques to help developers identify performance bottlenecks and implement optimizations effectively. Below, we will discuss key performance considerations and strategies for optimizing React applications.
1. Avoid Unnecessary Re-renders
Unnecessary re-renders can significantly impact performance. React components re-render when their props or state change. To prevent this:
- Use
React.memo
to memoize functional components and skip re-rendering if props haven't changed. - Use
shouldComponentUpdate
orReact.PureComponent
for class components to control re-rendering behavior.
import React, { memo } from 'react';
const ChildComponent = memo(({ value }) => {
console.log('ChildComponent rendered');
return <p>Value: {value}</p>;
});
export default ChildComponent;
2. Use Keys Effectively
When rendering lists, always provide a unique key
prop to each item. This helps React efficiently identify which items have changed, been added, or removed, avoiding unnecessary DOM updates.
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
3. Optimize Component Rendering
React components should be small and focused. For heavy computations or large data rendering, consider splitting components or using pagination. Additionally:
- Use
useMemo
to memoize expensive calculations. - Use
useCallback
to memoize functions passed as props.
import React, { useState, useMemo } from 'react';
function ExpensiveCalculation({ num }) {
const result = useMemo(() => {
console.log('Calculating...');
return num * 2; // Simulate heavy computation
}, [num]);
return <p>Result: {result}</p>;
}
export default ExpensiveCalculation;
4. Lazy Loading Components
Lazy loading helps reduce the initial load time by splitting your app into smaller chunks and loading them as needed. Use React.lazy
and Suspense
for lazy loading components:
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
export default App;
5. Code Splitting
Use tools like React.lazy
and dynamic imports to split your code into smaller chunks that are loaded on demand:
const LazyLoadedComponent = React.lazy(() => import('./LargeComponent'));
return (
<React.Suspense fallback="Loading...">
<LazyLoadedComponent />
</React.Suspense>
);
6. Avoid Inline Functions
Passing inline functions as props can cause re-renders. Use useCallback
to memoize functions:
import React, { useState, useCallback } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount(prevCount => prevCount + 1);
}, []);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
7. Optimize State Updates
Batch state updates to avoid multiple renders:
import React, { useState } from 'react';
function App() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(prev => prev + 1);
setCount(prev => prev + 1); // Batched update
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increase</button>
</div>
);
}
export default App;
8. Use React DevTools for Profiling
The React Developer Tools extension includes a profiler that helps you identify performance bottlenecks by showing how components are rendered and how long they take to render.
Conclusion
By applying these techniques, you can improve the performance of your React applications. Understanding how React updates the UI and identifying unnecessary renders is key to building efficient and scalable apps.
React Rendering and Virtual DOM
React is a powerful JavaScript library for building user interfaces, and one of its key features is the Virtual DOM. Understanding how React rendering works and the role of the Virtual DOM can help you write efficient and performant applications.
1. What is the Virtual DOM?
The Virtual DOM is a lightweight, in-memory representation of the actual DOM. It allows React to perform efficient updates to the UI by minimizing direct interactions with the browser's DOM, which can be slow.
When the state or props of a React component change:
- React updates the Virtual DOM first.
- It then compares the updated Virtual DOM to the previous version (a process called diffing).
- React calculates the minimal set of changes needed to update the real DOM.
- Finally, React applies these changes to the actual DOM efficiently.
2. How Rendering Works in React
Rendering in React involves transforming your application's state and props into a visual representation (UI). React components go through the following steps:
- Render Phase: React calls the
render
method (or the functional component) to generate the Virtual DOM structure. - Commit Phase: React applies changes to the actual DOM and updates the UI.
3. React Diffing Algorithm
The diffing algorithm is the process React uses to compare the previous and updated Virtual DOMs. React assumes that:
- Elements with the same type remain the same.
- Elements with different types are replaced entirely.
- Keys are critical for efficiently identifying elements in lists.
// Example of a key-based diffing optimization
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
4. Reconciliation Process
Reconciliation is React's process for updating the DOM efficiently:
- When a component's state or props change, React creates a new Virtual DOM tree.
- The new Virtual DOM is compared to the previous one.
- React determines the minimal number of changes required and updates the real DOM accordingly.
5. Benefits of Using the Virtual DOM
The Virtual DOM provides several advantages:
- Improved performance: React minimizes direct DOM manipulation, which is costly.
- Predictable updates: The Virtual DOM ensures that updates are consistent and efficient.
- Cross-platform compatibility: The Virtual DOM is platform-independent, making it useful for web, mobile, and server rendering.
6. Avoiding Common Rendering Pitfalls
To ensure React renders efficiently:
- Use
React.memo
to prevent unnecessary re-renders of functional components. - Provide unique
key
props for list items. - Avoid inline functions and objects, as they can create new instances on every render.
import React, { memo } from 'react';
const ListItem = memo(({ item }) => {
console.log('Rendering:', item);
return <li>{item}</li>;
});
export default function ItemList({ items }) {
return (
<ul>
{items.map((item) => (
<ListItem key={item} item={item} />
))}
</ul>
);
}
7. React and Browser Rendering
React optimizes browser rendering in several ways:
- Batching Updates: React batches multiple state updates into a single render to minimize DOM operations.
- Fiber Architecture: React's Fiber architecture improves rendering performance by breaking rendering work into small units, enabling React to pause and resume work as needed.
8. Tools for Debugging and Profiling Rendering
React Developer Tools provide a Profiler to analyze rendering behavior:
- Identify which components re-render unnecessarily.
- Measure the rendering time for components.
Use the Profiler to find performance bottlenecks and optimize rendering.
Conclusion
The Virtual DOM and React's rendering process are core to React's efficiency. By understanding how React updates the UI and leveraging tools like the Profiler, you can build performant and scalable applications.
Memoization with React.memo and useMemo
Memoization is a performance optimization technique in React that prevents unnecessary re-renders of components or recomputation of values. React provides two built-in tools for memoization: React.memo
and useMemo
.
1. What is React.memo?
React.memo
is a higher-order component (HOC) that memoizes a functional component. It ensures that the component only re-renders if its props have changed.
import React from 'react';
// A functional component that renders a message
const Message = ({ text }) => {
console.log('Rendering Message:', text);
return <p>{text}</p>;
};
// Wrapping the component with React.memo
export default React.memo(Message);
In this example:
- If the
text
prop doesn't change, theMessage
component will not re-render. - This optimization is useful for components that receive the same props but are nested within parent components that frequently re-render.
2. When to Use React.memo
Use React.memo
when:
- The component is functional and pure.
- Re-renders are expensive or frequent.
- The props of the component rarely change.
3. What is useMemo?
useMemo
is a React hook that memoizes the result of a computation. It ensures that expensive calculations are only recomputed when their dependencies change.
import React, { useState, useMemo } from 'react';
function ExpensiveCalculation({ num }) {
// A function to simulate an expensive calculation
const calculateFactorial = (n) => {
console.log('Calculating factorial...');
return n <= 0 ? 1 : n * calculateFactorial(n - 1);
};
// Memoize the result of the expensive calculation
const factorial = useMemo(() => calculateFactorial(num), [num]);
return (
<div>
<p>Factorial of {num}: {factorial}</p>
</div>
);
}
export default ExpensiveCalculation;
In this example:
- The factorial is only recomputed when the
num
prop changes. - This prevents unnecessary recomputation, improving performance.
4. When to Use useMemo
Use useMemo
when:
- You have expensive calculations that don’t need to re-run on every render.
- You want to avoid recalculating derived data unnecessarily.
5. Common Pitfalls of Memoization
While memoization can improve performance, it should be used judiciously. Overusing memoization can lead to unnecessary complexity:
- Avoid using
React.memo
oruseMemo
for lightweight or inexpensive calculations. - Ensure dependencies in
useMemo
are correctly specified to avoid stale values or unnecessary re-renders.
6. Combining React.memo and useMemo
You can use both React.memo
and useMemo
together for optimal performance:
import React, { useState, useMemo } from 'react';
const ExpensiveChild = React.memo(({ number }) => {
const isEven = useMemo(() => {
console.log('Calculating isEven...');
return number % 2 === 0;
}, [number]);
return <p>{number} is {isEven ? 'Even' : 'Odd'}</p>;
});
export default function ParentComponent() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<ExpensiveChild number={count} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example:
- The child component is memoized using
React.memo
. - The
isEven
computation inside the child is further optimized usinguseMemo
.
Conclusion
Memoization with React.memo
and useMemo
helps optimize performance by reducing unnecessary re-renders and expensive computations. Use these tools wisely to keep your React applications efficient and maintainable.
Avoiding Unnecessary Re-renders
In React, unnecessary re-renders can affect the performance of your application, especially as it grows. Understanding how React's rendering works and using optimization techniques can help you avoid these re-renders, leading to more efficient apps.
1. How React Rendering Works
React re-renders a component when:
- The component's state changes.
- The component's props change.
- The parent component re-renders.
By default, React re-renders the entire subtree of a component even if only a small part of it has changed. Optimizing this behavior is key to avoiding unnecessary re-renders.
2. Common Causes of Unnecessary Re-renders
- Passing new object or array references as props.
- Parent components re-rendering frequently.
- Not memoizing expensive calculations or components.
- Inline functions or styles causing new references on every render.
3. Techniques to Avoid Unnecessary Re-renders
3.1 Using React.memo
React.memo
is a higher-order component that prevents functional components from re-rendering if their props haven't changed.
import React from 'react';
const ChildComponent = React.memo(({ value }) => {
console.log('ChildComponent rendered');
return <p>Value: {value}</p>;
});
export default ChildComponent;
When to Use: Use React.memo
when the child component is functional, pure, and receives props that rarely change.
3.2 Memoizing Functions with useCallback
Inline functions are created anew with every render, causing components that depend on them to re-render. Use useCallback
to memoize these functions.
import React, { useState, useCallback } from 'react';
function Parent() {
const [count, setCount] = useState(0);
const increment = useCallback(() => setCount(count + 1), [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Parent;
When to Use: Use useCallback
when you pass functions as props to child components that depend on stable references.
3.3 Memoizing Computations with useMemo
Use useMemo
to memoize expensive computations and avoid recalculating them on every render.
import React, { useState, useMemo } from 'react';
function ExpensiveCalculation({ number }) {
const factorial = useMemo(() => {
console.log('Calculating factorial...');
return number <= 0 ? 1 : number * factorial(number - 1);
}, [number]);
return <p>Factorial: {factorial}</p>;
}
export default ExpensiveCalculation;
3.4 Avoid Inline Functions and Styles
Inline functions and styles create new references on every render, leading to unnecessary re-renders. Move them out of the render method or memoize them.
import React from 'react';
function StyledComponent() {
const styles = { color: 'blue', fontSize: '16px' };
return <p style={styles}>Styled Text</p>;
}
export default StyledComponent;
Best Practice: Avoid defining styles or functions directly in the JSX. Instead, define them outside or memoize them.
3.5 Splitting Components
Break down large components into smaller, more focused components. This ensures that only the necessary parts of the UI re-render when state or props change.
import React, { useState } from 'react';
function Parent() {
const [count, setCount] = useState(0);
return (
<div>
<p>Parent Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<Child />
</div>
);
}
function Child() {
console.log('Child rendered');
return <p>I am a child component.</p>;
}
export default Parent;
3.6 Using Key Wisely
React uses key
to identify elements in lists. Using incorrect or unstable keys can lead to unnecessary re-renders. Always use unique and stable keys.
import React from 'react';
function List({ items }) {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
export default List;
4. Conclusion
By understanding React's rendering behavior and using techniques like React.memo
, useCallback
, and useMemo
, you can significantly reduce unnecessary re-renders. Optimizing component structure, avoiding inline functions or styles, and managing props effectively are key practices to build efficient React applications.
Lazy Loading Components with React.lazy
and Suspense
Lazy loading in React is a technique for optimizing the performance of your application by loading components only when they are needed. This reduces the initial load time and improves user experience, especially in large applications.
1. What is Lazy Loading?
Lazy loading is a design pattern that defers the loading of resources until they are actually required. In React, you can use the React.lazy
function and Suspense
component to implement lazy loading for components.
2. Introduction to React.lazy
React.lazy
allows you to load components dynamically. It returns a React component that can be rendered like any other component but loads its code asynchronously.
import React, { lazy, Suspense } from 'react';
// Lazy load the component
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<h1>Welcome to Lazy Loading</h1>
<Suspense fallback=<div>Loading...</div>>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
3. Using Suspense
for Fallback UI
The Suspense
component is used to display a fallback UI while a lazy-loaded component is being loaded. The fallback can be any valid React element, such as a spinner, loading text, or a skeleton loader.
import React, { lazy, Suspense } from 'react';
const UserProfile = lazy(() => import('./UserProfile'));
function App() {
return (
<div>
<h1>User Dashboard</h1>
<Suspense fallback=<div>Fetching user profile...</div>>
<UserProfile />
</Suspense>
</div>
);
}
export default App;
4. Benefits of Lazy Loading
- Reduces the initial bundle size.
- Improves the load time of the application.
- Loads resources only when they are needed.
- Enhances the user experience by prioritizing visible content.
5. Code-Splitting with Lazy Loading
React.lazy is a key tool for implementing code-splitting. Code-splitting allows you to split your app into smaller bundles that can be loaded dynamically.
import React, { lazy, Suspense } from 'react';
const Dashboard = lazy(() => import('./Dashboard'));
const Settings = lazy(() => import('./Settings'));
function App() {
const [view, setView] = React.useState('dashboard');
return (
<div>
<button onClick={() => setView('dashboard')}>Dashboard</button>
<button onClick={() => setView('settings')}>Settings</button>
<Suspense fallback=<div>Loading...</div>>
{view === 'dashboard' ? <Dashboard /> : <Settings />}
</Suspense>
</div>
);
}
export default App;
6. Error Handling for Lazy Loading
When using lazy loading, errors like network issues can occur. Use an error boundary to catch and handle these errors gracefully.
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError() {
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <h2>Something went wrong while loading the component.</h2>;
}
return this.props.children;
}
}
function App() {
return (
<ErrorBoundary>
<Suspense fallback=<div>Loading...</div>>
<LazyComponent />
</Suspense>
</ErrorBoundary>
);
}
export default App;
7. Best Practices
- Group related components and lazy load them together for better performance.
- Use meaningful fallback UI to enhance user experience.
- Combine lazy loading with route-based code-splitting using a library like
React Router
. - Always use error boundaries to handle potential loading issues.
8. Example with React Router
React Router supports lazy-loaded components for route-based code splitting. Here's an example:
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
const Home = lazy(() => import('./Home'));
const About = lazy(() => import('./About'));
function App() {
return (
<Router>
<Suspense fallback=<div>Loading page...</div>>
<Routes>
<Route path="/" element=<Home /> />
<Route path="/about" element=<About /> />
</Routes>
</Suspense>
</Router>
);
}
export default App;
9. Conclusion
Lazy loading with React.lazy
and Suspense
is a powerful way to optimize your React application. By loading components only when needed, you can reduce the initial load time and improve performance, especially in large-scale applications.
Code Splitting and Dynamic Imports
Code splitting is a technique that allows you to split your application code into smaller chunks. This improves performance by loading only the required code for a specific view or feature, reducing the initial load time.
1. What is Code Splitting?
Code splitting is the process of dividing your JavaScript code into multiple smaller bundles that can be loaded on demand. It optimizes the application's performance by delaying the loading of non-critical resources.
React supports code splitting through dynamic imports, which allow you to load modules dynamically at runtime.
2. Dynamic Imports in JavaScript
The import()
function in JavaScript is used for dynamic imports. It returns a promise that resolves to the module object.
// Dynamic import example
function loadModule() {
import('./module.js')
.then(module => {
module.default();
})
.catch(error => {
console.error('Error loading module:', error);
});
}
3. Code Splitting in React
React enables code splitting using React.lazy
and Suspense
. This allows you to load components dynamically when they are needed.
import React, { lazy, Suspense } from 'react';
// Dynamically load the component
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<h1>Code Splitting Example</h1>
<Suspense fallback=<div>Loading...</div>>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
4. Benefits of Code Splitting
- Reduces the initial bundle size, improving page load speed.
- Ensures faster interaction by loading only the required code.
- Optimizes performance for large-scale applications with many features.
5. Code Splitting with React Router
React Router supports code splitting by lazy loading components for specific routes. Here’s an example:
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
// Dynamically import components
const Home = lazy(() => import('./Home'));
const About = lazy(() => import('./About'));
function App() {
return (
<Router>
<Suspense fallback=<div>Loading page...</div>>
<Routes>
<Route path="/" element=<Home /> />
<Route path="/about" element=<About /> />
</Routes>
</Suspense>
</Router>
);
}
export default App;
6. Dynamic Imports for Libraries
Dynamic imports can also be used to load libraries or large utility modules on demand. This ensures that your application doesn't load unnecessary code upfront.
// Example: Loading a library dynamically
function loadLodash() {
import('lodash')
.then(_ => {
console.log(_.shuffle([1, 2, 3, 4]));
})
.catch(error => console.error('Failed to load lodash:', error));
}
7. Error Handling with Dynamic Imports
Dynamic imports might fail due to network issues or other errors. Use error boundaries or try...catch
blocks to handle such scenarios gracefully.
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError() {
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <h2>Failed to load the component.</h2>;
}
return this.props.children;
}
}
function App() {
return (
<ErrorBoundary>
<Suspense fallback=<div>Loading...</div>>
<LazyComponent />
</Suspense>
</ErrorBoundary>
);
}
export default App;
8. Best Practices
- Use meaningful fallback UI for better user experience.
- Group related components into chunks to optimize loading.
- Leverage route-based code splitting for applications with multiple pages.
- Always handle errors in dynamic imports to prevent crashes.
9. Conclusion
Code splitting and dynamic imports are essential tools for optimizing React applications. They enable you to load only the necessary code for each feature, improving both performance and user experience.
Using shouldComponentUpdate
in Class Components
The shouldComponentUpdate
lifecycle method in React is used to optimize the performance of class components by controlling whether a re-render should occur. This method helps prevent unnecessary renders, improving efficiency in your application.
1. What is shouldComponentUpdate
?
The shouldComponentUpdate
method is a lifecycle method available in class components. It is called before the component re-renders when new props or state changes occur. By default, React re-renders a component whenever its props or state change. However, you can override this behavior using shouldComponentUpdate
to return true
or false
, based on specific conditions.
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// Logic to decide whether to re-render
return true; // or false
}
}
2. Parameters
nextProps
: The new props that the component will receive.nextState
: The new state that the component will have.
3. Example Usage
Here’s an example of using shouldComponentUpdate
to prevent re-renders when the component’s props or state have not changed significantly:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
shouldComponentUpdate(nextProps, nextState) {
// Only re-render if the count has changed
return nextState.count !== this.state.count;
}
increment = () => {
this.setState(prevState => ({ count: prevState.count + 1 }));
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
In this example, the component only re-renders if the count
value in the state has changed. This avoids unnecessary re-renders when other unrelated state variables or props are updated.
4. Benefits of Using shouldComponentUpdate
- Improves performance by avoiding unnecessary renders.
- Allows you to implement custom logic for determining re-renders.
- Gives fine-grained control over rendering behavior in class components.
5. Limitations
- Only applicable to class components; functional components use
React.memo
for similar behavior. - Overusing or misusing
shouldComponentUpdate
can make the code more complex and harder to maintain. - Manually comparing complex objects or arrays can be expensive and may negate performance gains.
6. Alternative: Using PureComponent
React provides the PureComponent
class as a simpler alternative to implementing shouldComponentUpdate
. A PureComponent
automatically performs a shallow comparison of props and state to decide whether to re-render.
import React, { PureComponent } from 'react';
class Counter extends PureComponent {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
increment = () => {
this.setState(prevState => ({ count: prevState.count + 1 }));
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
The PureComponent
class is ideal for components that don’t require custom logic in shouldComponentUpdate
. It simplifies the code and ensures performance optimizations.
7. Best Practices
- Use
shouldComponentUpdate
only when necessary to avoid over-complicating your code. - Prefer
PureComponent
for shallow comparisons if no custom logic is required. - For functional components, use
React.memo
to achieve similar optimizations. - Be cautious with deep comparisons; they can be expensive and negate performance benefits.
8. Conclusion
The shouldComponentUpdate
lifecycle method is a powerful tool for optimizing class components in React. By controlling re-renders, you can improve the performance of your application. However, it should be used judiciously and only when necessary to avoid introducing complexity.
Styling with CSS in React Components
Styling React components is a fundamental part of building visually appealing applications. React provides multiple approaches to apply styles, allowing flexibility based on the project's needs.
1. Inline Styling
Inline styles in React are specified using a JavaScript object. Properties are written in camelCase, and values are provided as strings (or numbers for unitless properties).
import React from 'react';
function InlineStyleExample() {
const style = {
color: 'blue',
fontSize: '20px',
margin: '10px'
};
return (
<div style={style}>
This is an example of inline styling.
</div>
);
}
export default InlineStyleExample;
2. CSS Stylesheets
You can create a separate CSS file and import it into your React component. This approach keeps styles modular and reusable.
/* styles.css */
.container {
color: green;
font-size: 18px;
padding: 10px;
}
import React from 'react';
import './styles.css';
function StylesheetExample() {
return (
<div className="container">
This is styled using a CSS stylesheet.
</div>
);
}
export default StylesheetExample;
3. CSS Modules
CSS Modules scope styles locally to the component, preventing class name conflicts in larger applications.
/* styles.module.css */
.container {
color: red;
font-size: 22px;
border: 1px solid black;
}
import React from 'react';
import styles from './styles.module.css';
function CSSModulesExample() {
return (
<div className={styles.container}>
This is styled using CSS Modules.
</div>
);
}
export default CSSModulesExample;
4. Styled Components
Styled Components is a popular library for styling React components using tagged template literals.
import React from 'react';
import styled from 'styled-components';
const StyledDiv = styled.div`
color: purple;
font-size: 24px;
background-color: #f0f0f0;
padding: 15px;
`;
function StyledComponentsExample() {
return (
<StyledDiv>
This is styled using Styled Components.
</StyledDiv>
);
}
export default StyledComponentsExample;
5. Dynamic Styling
React allows dynamic styling based on component state or props, enabling conditional application of styles.
import React, { useState } from 'react';
function DynamicStylingExample() {
const [isActive, setIsActive] = useState(false);
const style = {
color: isActive ? 'orange' : 'gray',
fontWeight: isActive ? 'bold' : 'normal'
};
return (
<div>
<p style={style}>
This text changes style dynamically.
</p>
<button onClick={() => setIsActive(!isActive)}>
Toggle Style
</button>
</div>
);
}
export default DynamicStylingExample;
6. Third-Party Libraries
React supports various third-party libraries like Material-UI, Ant Design, or Tailwind CSS for advanced and pre-designed components and styling.
7. Best Practices
- Choose a styling approach based on project size and complexity.
- Use CSS Modules or Styled Components for scoped styles in larger applications.
- Leverage dynamic styling for components with changing states or props.
- Minimize inline styles for better maintainability and readability.
8. Conclusion
React offers multiple options for styling components, each with its own strengths and use cases. By understanding these approaches, you can choose the most suitable method for your application and ensure clean, scalable, and maintainable code.
Inline Styles in React
Inline styles in React are a quick and straightforward way to apply styles directly to elements. Unlike traditional inline styles in HTML, React styles are written as JavaScript objects where property names use camelCase notation.
1. Basic Syntax
In React, the style
attribute accepts an object with key-value pairs. The keys represent CSS property names in camelCase, and the values are strings or numbers.
import React from 'react';
function InlineStyleExample() {
return (
<div style={{ color: 'blue', fontSize: '20px', padding: '10px' }}>
This is an example of inline styles in React.
</div>
);
}
export default InlineStyleExample;
2. Using a Style Object
For better readability and reusability, you can define the styles in a separate object and apply them to the style
attribute.
import React from 'react';
function InlineStyleWithObject() {
const styles = {
color: 'green',
backgroundColor: '#f0f0f0',
fontSize: '18px',
border: '1px solid black',
borderRadius: '5px',
padding: '10px'
};
return (
<div style={styles}>
This div is styled using a style object.
</div>
);
}
export default InlineStyleWithObject;
3. Dynamic Inline Styles
You can apply styles dynamically based on a component's state or props. This approach allows you to create interactive and responsive designs.
import React, { useState } from 'react';
function DynamicInlineStyles() {
const [isActive, setIsActive] = useState(false);
const styles = {
color: isActive ? 'white' : 'black',
backgroundColor: isActive ? 'blue' : 'lightgray',
fontSize: '18px',
padding: '10px',
cursor: 'pointer'
};
return (
<div>
<div style={styles} onClick={() => setIsActive(!isActive)}>
Click me to toggle styles!
</div>
</div>
);
}
export default DynamicInlineStyles;
4. Combining Inline Styles
When multiple style objects need to be applied, you can merge them using the spread operator or other techniques.
import React from 'react';
function CombinedInlineStyles() {
const baseStyle = {
fontSize: '16px',
padding: '10px',
margin: '5px'
};
const successStyle = {
...baseStyle,
color: 'white',
backgroundColor: 'green'
};
const errorStyle = {
...baseStyle,
color: 'white',
backgroundColor: 'red'
};
return (
<div>
<div style={successStyle}>Success Message</div>
<div style={errorStyle}>Error Message</div>
</div>
);
}
export default CombinedInlineStyles;
5. Advantages of Inline Styles
- Useful for dynamic styles that depend on state or props.
- Scoped to the specific element, avoiding global CSS conflicts.
- Quick to implement for small or one-off components.
6. Disadvantages of Inline Styles
- Not suitable for complex or reusable styles.
- Cannot use pseudo-classes like
:hover
or media queries directly. - May lead to cluttered JSX if overused.
7. Best Practices
- Use inline styles sparingly for dynamic or one-time styling needs.
- Keep the style object separate for better readability and maintainability.
- For complex styling, consider using CSS files, CSS Modules, or styled-components.
Conclusion
Inline styles in React provide a simple and effective way to style components dynamically and avoid global conflicts. While they are not ideal for all scenarios, they are a great option for localized and state-dependent styling.
CSS Modules for Scoped Styling
CSS Modules allow you to write styles scoped to a specific component, avoiding the global scope issues that come with traditional CSS. With CSS Modules, each style is automatically scoped to the component in which it is used, ensuring that styles do not leak or cause conflicts between components.
1. What is CSS Modules?
CSS Modules are a way to write CSS that is scoped locally to the component, unlike global CSS where styles can inadvertently affect other parts of the application. Each class name is automatically transformed into a unique identifier, ensuring that it only applies to the component in which it is defined.
2. Setting Up CSS Modules
To use CSS Modules in a React project, you need to ensure that the file extension is .module.css
or .module.scss
for SCSS. Most React setups (like Create React App) automatically support CSS Modules out of the box.
Example of a CSS Module file, Button.module.css
:
.button {
padding: 10px 20px;
background-color: blue;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.button:hover {
background-color: darkblue;
}
3. Importing and Using CSS Modules in React Components
Once you have your CSS Module set up, you can import the styles into your component and use them in JSX. The imported styles will be scoped to that component.
import React from 'react';
import styles from './Button.module.css';
function Button() {
return (
<button className={styles.button}>
Click me
</button>
);
}
export default Button;
In this example, the button
class is scoped to the Button
component and won’t affect other components even if they have a class called button
.
4. Dynamic Class Names with CSS Modules
You can dynamically apply class names using the styles from CSS Modules. This is useful if you want to modify the appearance of a component based on its state or props.
import React, { useState } from 'react';
import styles from './Button.module.css';
function Button() {
const [isClicked, setIsClicked] = useState(false);
return (
<button
className={isClicked ? styles.clicked : styles.button}
onClick={() => setIsClicked(!isClicked)}
>
{isClicked ? 'Clicked' : 'Click me'}
</button>
);
}
export default Button;
In this example, the button’s style changes when it is clicked, using the appropriate class from the CSS Module based on the isClicked
state.
5. Advantages of CSS Modules
- Scoped Styles: Styles are scoped to the component, preventing global style conflicts.
- Automatic Name Generation: Class names are automatically generated, ensuring uniqueness.
- Maintainability: Easier to manage styles for individual components, improving maintainability in large projects.
- CSS Features: Supports all CSS features like media queries, pseudo-classes, and more.
6. Disadvantages of CSS Modules
- Learning Curve: Requires some learning to understand the module system, especially if transitioning from global CSS.
- Limited Global Styles: For styles that need to apply globally (e.g., resets or themes), a separate global stylesheet is still required.
7. Best Practices
- Use CSS Modules for component-level styles and avoid global styles as much as possible.
- When using dynamic class names, prefer the combination of multiple classes rather than inline styles for better performance.
- For global styles (e.g., resets, typography), you can still use global CSS files alongside CSS Modules.
8. Conclusion
CSS Modules offer a great way to manage styles in React applications by scoping them to individual components and preventing style conflicts. They enable more maintainable and modular CSS that can scale well in large projects. While CSS Modules are ideal for component-specific styles, global styles can still be managed through traditional CSS or other CSS-in-JS solutions.
Styled Components: Using JavaScript for Styling
Styled Components is a popular library that allows you to write CSS in JavaScript. By using tagged template literals, you can create styled components that are scoped to the component itself. This approach enables a more dynamic and reusable way of applying styles to your React components without using external stylesheets.
1. What is Styled Components?
Styled Components is a library for styling React components using tagged template literals. It allows you to define styles directly within your JavaScript code and automatically handles scoping to avoid conflicts between components. Styled components are actual React components, and you can define their styles inline, using JavaScript.
2. Setting Up Styled Components
To use Styled Components in your React project, you need to install it via npm or yarn:
npm install styled-components
Once installed, you can import the styled
object from the styled-components
package to start creating styled components.
3. Creating Styled Components
Here is a simple example of creating and using a styled component:
import styled from 'styled-components';
// Create a styled button component
const Button = styled.button`
padding: 10px 20px;
background-color: blue;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
&:hover {
background-color: darkblue;
}
`;
function App() {
return (
<div>
<Button>Click me</Button>
</div>
);
}
export default App;
In this example, we created a Button
styled component using tagged template literals. The styles are defined inside the backticks, and React will render the styled button with the specified styles.
4. Props for Dynamic Styles
Styled Components allows you to pass props to modify the styling dynamically. For instance, you can pass props to change the colors or sizes of components based on the component's state or any other prop values.
import styled from 'styled-components';
const Button = styled.button`
padding: 10px 20px;
background-color: ${props => props.primary ? 'blue' : 'gray'};
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
&:hover {
background-color: ${props => props.primary ? 'darkblue' : 'darkgray'};
}
`;
function App() {
return (
<div>
<Button primary>Primary Button</Button>
<Button>Secondary Button</Button>
</div>
);
}
export default App;
In this example, the button color changes based on the primary
prop passed to the component. If primary
is true, the button gets a blue background; otherwise, it gets a gray background.
5. Nesting Styles with Styled Components
Styled Components supports nesting styles using CSS syntax. This allows you to apply styles to child elements inside the parent component's styles.
import styled from 'styled-components';
const Card = styled.div`
background-color: lightgray;
padding: 20px;
border-radius: 5px;
h2 {
font-size: 24px;
color: darkblue;
}
p {
font-size: 16px;
color: darkgray;
}
`;
function App() {
return (
<Card>
<h2>Styled Card Title</h2>
<p>This is a description inside the card</p>
</Card>
);
}
export default App;
Here, we have a Card
styled component, and we use nested styles to style the h2
and p
tags inside the Card
.
6. Theming with Styled Components
Styled Components also supports theming, allowing you to define global styles for your entire application. Themes help you manage and centralize design variables like colors, fonts, and spacing.
import styled, { ThemeProvider } from 'styled-components';
const Button = styled.button`
padding: 10px 20px;
background-color: ${props => props.theme.primaryColor};
color: ${props => props.theme.textColor};
border: none;
border-radius: 5px;
cursor: pointer;
`;
const theme = {
primaryColor: 'blue',
textColor: 'white'
};
function App() {
return (
<ThemeProvider theme={theme}>
<div>
<Button>Themed Button</Button>
</div>
</ThemeProvider>
);
}
export default App;
In this example, we used the ThemeProvider
to pass a theme object containing the primary and text colors. These theme values are then accessed inside the styled component using props.theme
.
7. Advantages of Styled Components
- Scoped Styles: Styled Components automatically scope styles to the component, avoiding global CSS issues.
- Dynamic Styles: You can use props to dynamically apply styles based on component state or other variables.
- Theming: Centralize design variables and manage them with themes for consistency across your application.
- Composability: Styled Components are React components themselves, so you can easily compose them together to build complex UIs.
8. Disadvantages of Styled Components
- Performance: Styled Components use runtime CSS injection, which could affect performance on large applications with many styled components.
- Learning Curve: For developers new to JavaScript-based styling, there may be an initial learning curve.
9. Best Practices
- Use Styled Components for component-level styles and avoid global styles as much as possible.
- For highly dynamic styles, use props to pass values and modify styles accordingly.
- Leverage theming to maintain a consistent design system across your entire application.
10. Conclusion
Styled Components provide a powerful and flexible way to manage styles in React applications using JavaScript. By allowing you to define styles within your components, you can create more maintainable, modular, and dynamic UIs. Whether you're building small components or large applications, Styled Components offer a unique approach to styling in React.
Tailwind CSS with React
Tailwind CSS is a utility-first CSS framework that allows you to quickly build custom designs without writing custom CSS. In a React application, Tailwind can be used to style your components by applying pre-defined utility classes directly in JSX. This approach helps maintain a consistent design system and promotes a clean and efficient workflow.
1. What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that provides low-level utility classes to style elements. Unlike traditional CSS frameworks like Bootstrap, Tailwind doesn’t come with predefined components. Instead, it offers a set of utilities that can be combined to create custom designs. The beauty of Tailwind is its ability to let you write your styles inline, keeping your components clean and maintainable.
2. Setting Up Tailwind CSS in React
To use Tailwind CSS in your React application, you need to follow these steps:
- Install Tailwind via npm or yarn.
- Configure Tailwind by creating a configuration file.
- Set up PostCSS to handle Tailwind's CSS processing.
Here’s how to set it up:
# Install Tailwind and its dependencies
npm install tailwindcss postcss-cli autoprefixer
# Create a tailwind.config.js file
npx tailwindcss init
Next, you need to configure your PostCSS setup:
# Create a postcss.config.js file
touch postcss.config.js
Inside postcss.config.js
, add the following configuration:
module.exports = {
plugins: [
require('tailwindcss'),
require('autoprefixer'),
],
};
Then, create a src/index.css
file and add the following Tailwind directives:
/* src/index.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
Finally, import this CSS file into your index.js
:
import './index.css';
3. Using Tailwind CSS Classes in React Components
Now that Tailwind CSS is set up in your project, you can start using its utility classes in your React components. Tailwind provides a wide range of utility classes like text-center
, p-4
, bg-blue-500
, etc., to style your elements directly in JSX.
function App() {
return (
<div className="bg-blue-500 text-white p-4 rounded-md">
<h1 className="text-3xl">Hello, Tailwind CSS in React!</h1>
</div>
);
}
export default App;
In this example, we applied Tailwind’s utility classes like bg-blue-500
, text-white
, p-4
, and rounded-md
directly to the JSX elements.
4. Customizing Tailwind CSS
Tailwind is highly customizable. You can modify the default configuration in the tailwind.config.js
file to adjust colors, spacing, fonts, and more.
For example, you can customize the colors like this:
// tailwind.config.js
module.exports = {
theme: {
extend: {
colors: {
customBlue: '#1DA1F2',
customGray: '#F5F5F5',
},
},
},
};
Once you’ve customized Tailwind, you can use your custom colors in the same way as the default ones:
function App() {
return (
<div className="bg-customBlue text-white p-4">
<h1>Welcome to Custom Tailwind Colors!</h1>
</div>
);
}
5. Responsiveness with Tailwind
Tailwind CSS makes it easy to build responsive layouts with its built-in breakpoints. You can apply different styles based on screen size by using the responsive utilities.
function App() {
return (
<div className="bg-blue-500 p-4 text-white sm:bg-green-500 md:bg-red-500 lg:bg-yellow-500">
<h1>Responsive Tailwind Example</h1>
</div>
);
}
In this example, the background color will change depending on the screen size:
sm:
applies styles for small screens (min-width: 640px)md:
applies styles for medium screens (min-width: 768px)lg:
applies styles for large screens (min-width: 1024px)
6. Using Tailwind with CSS-in-JS Libraries
Tailwind can be used in combination with CSS-in-JS libraries like styled-components or emotion. You can use Tailwind classes alongside JavaScript-based styles for more dynamic styling in React.
import tw from 'twin.macro'; // Twin.macro is a popular library for using Tailwind with CSS-in-JS
const Button = styled.button`
${tw`bg-blue-500 text-white p-4 rounded-lg`}
`;
function App() {
return (
<Button>Click Me</Button>
);
}
7. Advantages of Using Tailwind CSS in React
- Utility-first: Tailwind provides utility classes that make it easy to style components without writing custom CSS.
- Customizable: Tailwind is highly configurable, allowing you to define your design system.
- Responsive: Tailwind includes built-in responsive utilities that enable easy adaptation to different screen sizes.
- Maintainable: Tailwind's utility classes promote readability and reduce the need for long custom styles.
8. Disadvantages of Using Tailwind CSS
- Verbose HTML: The markup can become cluttered with many utility classes, which might affect readability for some developers.
- Initial Learning Curve: There’s a bit of a learning curve when it comes to understanding the utility-first approach and the variety of available classes.
9. Best Practices
- Use Tailwind’s
@apply
directive to create reusable styles, reducing repetition in your JSX code. - Leverage Tailwind’s responsive utilities to ensure your app is mobile-friendly and adapts to different screen sizes.
- Keep your HTML structure clean and avoid adding too many classes to a single element for better readability.
10. Conclusion
Tailwind CSS is a powerful and flexible utility-first CSS framework that integrates seamlessly with React applications. By using Tailwind, you can build highly customizable and responsive user interfaces without writing much custom CSS. Whether you're building small components or large, complex applications, Tailwind offers a great approach to styling with a focus on speed, scalability, and maintainability.
Using SCSS/SASS with React
SCSS (Sassy CSS) or SASS (Syntactically Awesome Stylesheets) is a CSS preprocessor that extends CSS with features like variables, nested rules, mixins, and more. In a React application, you can use SCSS/SASS to write more maintainable and organized styles for your components. This guide will show you how to integrate SCSS/SASS with React and use the power of these preprocessors to enhance your stylesheets.
1. What is SCSS/SASS?
SASS is a CSS preprocessor that allows you to use features not available in regular CSS, such as variables, nesting, mixins, and more. SCSS is a more recent syntax for SASS that is fully compatible with CSS and features a more familiar curly brace syntax.
Here are some key features of SCSS/SASS:
- Variables: Store values such as colors, fonts, or spacing to reuse throughout your stylesheet.
- Nesting: Nest CSS rules inside one another to reflect the HTML structure, making the styles more readable.
- Mixins: Create reusable pieces of code for properties that need to be reused across multiple selectors.
- Partials and Imports: Split your CSS into smaller files and import them into one main stylesheet.
2. Setting Up SCSS/SASS in a React Project
To get started with SCSS/SASS in a React application, you need to install the necessary dependencies and configure your project accordingly.
Here’s how to set it up:
# Install SASS as a development dependency
npm install sass
Once you’ve installed SASS, you can start using SCSS in your React components. Rename your CSS files with the .scss
extension, and React will automatically compile them.
3. Using SCSS/SASS in React Components
Once SCSS/SASS is installed, you can start using it to style your React components. Here’s how to structure your SCSS files and use them in your React components:
// src/App.js
import './App.scss'; // Import your SCSS file into your component
function App() {
return (
Hello, SCSS in React!
);
}
export default App;
In the App.scss
file, you can use SCSS syntax:
// src/App.scss
.app-container {
background-color: #f0f0f0;
padding: 20px;
}
.heading {
font-size: 2rem;
color: #333;
}
Once you save your SCSS file, React will automatically compile it into the corresponding CSS file and apply the styles to your components.
4. SCSS Features in React
Here are some features of SCSS/SASS that you can take advantage of when styling your React components:
Variables
SCSS allows you to define variables that can store values like colors, fonts, and sizes to reuse throughout your stylesheets.
// src/styles/variables.scss
$primary-color: #3498db;
$font-size: 16px;
// src/App.scss
.heading {
color: $primary-color;
font-size: $font-size;
}
Nesting
SCSS allows you to nest your CSS selectors in a way that mirrors the HTML structure, making your styles more organized and easier to read.
.container {
padding: 20px;
.heading {
color: $primary-color;
}
.content {
font-size: $font-size;
}
}
Mixins
Mixins allow you to define reusable styles that can be included in multiple selectors.
// src/styles/mixins.scss
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
border-radius: $radius;
}
// src/App.scss
.button {
@include border-radius(5px);
padding: 10px 15px;
background-color: $primary-color;
}
Partials and Imports
SCSS supports partials, which allow you to break up your styles into smaller, more manageable files. You can then import these partials into your main SCSS file.
// _variables.scss
$primary-color: #3498db;
// _button.scss
.button {
background-color: $primary-color;
}
// main.scss
@import 'variables';
@import 'button';
5. Benefits of Using SCSS/SASS with React
- Improved Readability: SCSS syntax allows for cleaner and more readable CSS, especially with features like nesting and variables.
- Maintainability: SCSS makes your styles more maintainable with reusable mixins and variables.
- Modularization: With partials, you can break up your styles into smaller, more manageable files and import them when needed.
- Advanced Features: SCSS comes with advanced features like loops, conditionals, and functions, which allow you to write more dynamic stylesheets.
6. Best Practices for Using SCSS/SASS in React
- Use BEM Convention: Consider using the BEM (Block Element Modifier) naming convention to structure your SCSS classes in a readable and predictable manner.
- Modularize Your Styles: Break up your SCSS into smaller files using partials for better maintainability.
- Use Variables and Mixins: Utilize variables to store common values like colors and fonts, and use mixins for reusable patterns like border-radius or button styles.
- Keep Styles Component-Specific: When using SCSS with React, it's important to scope your styles to the components they belong to. This helps avoid styles leaking between components.
7. Conclusion
Using SCSS/SASS in a React application enhances the maintainability, readability, and scalability of your styles. By leveraging features like variables, nesting, mixins, and partials, you can write clean, organized, and reusable CSS for your React components. Whether you're building small components or large applications, SCSS/SASS makes it easier to manage complex styles in a React project.
Introduction to Testing in React
Testing is a crucial part of modern software development, ensuring that your application works as expected and preventing regressions. React provides powerful tools and libraries to help you test your components, logic, and interactions efficiently. In this guide, we will introduce the key concepts behind testing in React and how to get started with testing your React components.
1. Why Test in React?
Testing React applications is important for several reasons:
- Ensure code correctness: Tests help verify that your application works as expected and that updates do not break existing functionality.
- Catch bugs early: Testing allows you to catch potential bugs early in the development process, saving time and effort in the long run.
- Improve code quality: Well-tested applications have better maintainability and reliability, making collaboration and future changes easier.
- Document behavior: Tests serve as a form of documentation, describing how components are expected to behave.
2. Types of Tests in React
There are several types of tests you can write for React applications:
- Unit Tests: Test individual functions or components in isolation. These tests are fast and focus on a single unit of code.
- Integration Tests: Test the interaction between multiple components or functions to verify they work together as expected.
- End-to-End (E2E) Tests: Test the entire application flow, simulating user interactions from start to finish. These tests are more comprehensive but slower than unit and integration tests.
3. Tools and Libraries for Testing in React
There are various libraries and tools available for testing React applications. The most popular ones include:
Jest
Jest is a JavaScript testing framework developed by Facebook, commonly used with React. It provides a test runner, assertion library, and mocking capabilities. Jest comes pre-configured with Create React App, making it easy to get started.
React Testing Library
React Testing Library (RTL) focuses on testing React components in a way that simulates how users interact with the app. It encourages writing tests based on the component’s behavior rather than its internal implementation, promoting better testing practices.
Enzyme
Enzyme is another popular testing utility for React, developed by Airbnb. It provides utilities for shallow rendering, full DOM rendering, and static rendering of React components. However, React Testing Library is now the more widely recommended tool for testing React components.
4. Writing Tests for React Components
Let’s start by writing a simple test for a React component using Jest and React Testing Library.
Step 1: Install Dependencies
# If you're using Create React App, Jest and React Testing Library are already installed
npm install @testing-library/react @testing-library/jest-dom
Step 2: Write a Simple Component
// src/components/Hello.js
import React from 'react';
const Hello = ({ name }) => {
return Hello, {name}!
;
};
export default Hello;
Step 3: Write a Test for the Component
// src/components/Hello.test.js
import { render, screen } from '@testing-library/react';
import Hello from './Hello';
test('renders the correct greeting message', () => {
render( );
const heading = screen.getByText(/hello, john!/i);
expect(heading).toBeInTheDocument();
});
In this example, we’re using React Testing Library’s render
function to render the component and the screen.getByText
to find the heading element. Finally, we assert that the heading is in the document using Jest’s expect
function.
5. Running Tests
To run the tests, simply use the following command:
npm test
Jest will automatically find the test files in your project and run them, reporting any failed tests in the console.
6. Best Practices for Testing in React
- Test the component's behavior, not implementation: Write tests that focus on how the component behaves from the user's perspective rather than its internal implementation details.
- Write small, isolated tests: Ensure your tests are small and focused on a single piece of functionality to make debugging easier.
- Test edge cases: Test your components with different inputs, edge cases, and error conditions to ensure robustness.
- Keep tests fast: Slow tests can hinder the development process, so prioritize fast unit and integration tests. Reserve end-to-end tests for critical workflows.
- Mock external dependencies: Use Jest’s mocking functions to mock external services or APIs to isolate the component behavior during tests.
7. Conclusion
Testing is an essential part of React development, helping you ensure that your components work as expected and preventing bugs from being introduced as your application evolves. By using tools like Jest and React Testing Library, you can write effective tests that focus on your components' behavior. Following best practices such as testing behavior, writing small tests, and mocking dependencies will ensure your tests are efficient and maintainable.
Setting Up Jest for Unit Testing
Jest is a popular JavaScript testing framework that is widely used for unit testing React applications. It provides a simple and powerful way to write tests for your JavaScript code, with features like a built-in test runner, assertion library, and mocking capabilities. In this guide, we will walk through the steps to set up Jest for unit testing in your React project.
1. Installing Jest
If you are using Create React App, Jest is already pre-configured, and you can start writing tests right away. However, if you're setting up Jest in a custom project, you need to install it manually.
Step 1: Install Jest
To get started with Jest in a React project, first install the necessary dependencies:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
Here, jest
is the test runner, @testing-library/react
is for rendering React components in the test environment, and @testing-library/jest-dom
provides custom Jest matchers for assertions on the DOM.
Step 2: Add a Test Script
Next, add a test script to your package.json
file, so you can run Jest from the command line:
"scripts": {
"test": "jest"
}
With this, you can run npm test
to execute your tests using Jest.
2. Writing Unit Tests in Jest
Jest allows you to write unit tests for your components and functions. Here's an example of how to write a simple unit test for a function and a React component.
Example 1: Writing a Unit Test for a Function
Let's start by writing a unit test for a simple function that adds two numbers:
// src/utils/math.js
function add(a, b) {
return a + b;
}
export default add;
Now, let's write a Jest test to check if the add
function works correctly:
// src/utils/math.test.js
import add from './math';
test('adds two numbers correctly', () => {
expect(add(2, 3)).toBe(5);
});
In this test, we use test
to define a test case, and expect
to make assertions about the behavior of our code. The matcher toBe
checks if the result of add(2, 3)
equals 5.
Example 2: Writing a Unit Test for a React Component
Next, let's write a test for a simple React component:
// src/components/Hello.js
import React from 'react';
const Hello = ({ name }) => {
return Hello, {name}!
;
};
export default Hello;
Now, let's write a unit test for this component using Jest and React Testing Library:
// src/components/Hello.test.js
import { render, screen } from '@testing-library/react';
import Hello from './Hello';
test('renders the correct greeting message', () => {
render( );
const heading = screen.getByText(/hello, john!/i);
expect(heading).toBeInTheDocument();
});
This test renders the Hello
component with the name prop set to "John", and then checks if the rendered text contains "Hello, John!" using screen.getByText
.
3. Running Jest Tests
To run the tests, use the following command:
npm test
Jest will automatically find all files with the .test.js
or .spec.js
extension and execute the tests. It will also watch for file changes and rerun the tests automatically.
4. Configuring Jest
Jest can be configured using a jest.config.js
file or by adding Jest-specific settings in your package.json
. Here are some common configuration options:
Example Jest Configuration
// jest.config.js
module.exports = {
testEnvironment: 'jsdom', // Use jsdom for testing React components
setupFilesAfterEnv: ['@testing-library/jest-dom/extend-expect'], // Extend expect with jest-dom matchers
transform: {
'^.+\\.jsx?$': 'babel-jest', // Use babel-jest to transpile JavaScript files
},
};
This configuration sets up Jest to use the jsdom
environment (which simulates a browser environment), adds custom jest-dom matchers, and sets up Babel for transpiling JSX files.
5. Best Practices for Unit Testing in Jest
- Write isolated tests: Ensure your tests focus on a single piece of functionality, such as one function or component. This makes tests easier to maintain and debug.
- Use descriptive test names: Write clear and concise test names that explain what the test is checking for. This improves the readability of your test suite.
- Mock external dependencies: Use Jest's mocking features to mock external services or APIs to isolate your units of code during tests.
- Test edge cases: Consider testing edge cases, such as empty inputs or invalid values, to ensure your code handles all scenarios properly.
- Keep tests fast: Write fast unit tests that can be run frequently without slowing down your development process.
6. Conclusion
Setting up Jest for unit testing in your React project is a straightforward process. By following the steps in this guide, you can start writing unit tests for your functions and components. Jest’s powerful features, like mocking, coverage reporting, and an easy-to-use API, make it an excellent choice for testing in React. Remember to follow best practices such as writing isolated tests, using descriptive names, and testing edge cases to ensure your application remains reliable and bug-free.
Testing React Components with Enzyme or React Testing Library
Testing is an essential part of any React application to ensure components work as expected. Two popular libraries for testing React components are Enzyme and React Testing Library. Both libraries provide tools for rendering components and interacting with them in tests, but they differ in their approaches and philosophy. In this guide, we'll explore both libraries and how to use them for testing React components.
1. Introduction to Enzyme
Enzyme is a testing utility developed by Airbnb for React that allows you to manipulate, traverse, and assert the output of React components. It provides shallow rendering, full DOM rendering, and static rendering, giving you flexibility in how you write your tests.
Installing Enzyme
To get started with Enzyme, you need to install it along with an adapter that matches your version of React:
npm install --save-dev enzyme enzyme-adapter-react-16
Make sure to replace react-16
with the appropriate version if you are using a different version of React.
Setting up Enzyme
Next, you need to configure Enzyme to use the React adapter. Create a setupTests.js
file in your src
folder and add the following code:
// src/setupTests.js
import { configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
Enzyme requires this setup to work with your React version.
Rendering and Testing Components with Enzyme
Here’s an example of how to test a simple React component using Enzyme:
// src/components/Hello.js
import React from 'react';
const Hello = ({ name }) => {
return Hello, {name}!
;
};
export default Hello;
Now, let’s write a test for this component using Enzyme:
// src/components/Hello.test.js
import React from 'react';
import { shallow } from 'enzyme';
import Hello from './Hello';
test('renders the correct greeting message', () => {
const wrapper = shallow( );
expect(wrapper.text()).toBe('Hello, John!');
});
In this test, we use shallow
rendering to render the component and check if the component's text matches the expected greeting message.
2. Introduction to React Testing Library
React Testing Library is another popular library for testing React components. Unlike Enzyme, React Testing Library encourages developers to test components as users would interact with them. This means focusing on the component's rendered output rather than its internal implementation.
Installing React Testing Library
To get started with React Testing Library, install it along with Jest (which is typically already installed in most React projects):
npm install --save-dev @testing-library/react @testing-library/jest-dom
React Testing Library relies on @testing-library/jest-dom
for custom Jest matchers like toBeInTheDocument
.
Rendering and Testing Components with React Testing Library
Here's how you can test the same Hello
component using React Testing Library:
// src/components/Hello.test.js
import { render, screen } from '@testing-library/react';
import Hello from './Hello';
test('renders the correct greeting message', () => {
render( );
const heading = screen.getByText(/hello, john!/i);
expect(heading).toBeInTheDocument();
});
In this test, we use render
to render the component and screen.getByText
to query for the text content. We then assert that the text is present using the toBeInTheDocument
matcher from Jest DOM.
3. Key Differences Between Enzyme and React Testing Library
- Testing Philosophy: Enzyme allows for testing a component’s internal implementation, while React Testing Library focuses on testing the component’s behavior from a user’s perspective, encouraging you to query elements like a user would (via text, labels, etc.).
- Querying the DOM: React Testing Library provides a set of queries for interacting with the DOM, such as
getByText
,getByLabelText
, andgetByRole
, which focus on how users would interact with the DOM. Enzyme provides methods likefind
for traversing the component tree. - Shallow Rendering vs. Full DOM Rendering: Enzyme offers shallow rendering, which renders only the component itself and not its child components. React Testing Library renders the entire component tree, including child components.
- Testing Utilities: Enzyme provides utilities like
shallow
,mount
, andrender
, whereas React Testing Library focuses on rendering components and using queries to interact with the DOM.
4. Best Practices for Testing React Components
- Test Behavior, Not Implementation: Focus on testing how your component behaves rather than its internal implementation. This ensures your tests are more resilient to code changes.
- Query Elements Like a User: Use queries like
getByText
,getByRole
, orgetByLabelText
in React Testing Library to find elements based on how users interact with them. - Keep Tests Isolated: Each test should focus on a specific behavior, component, or function. Avoid testing multiple components or functions in a single test.
- Mock External Dependencies: Use mocking utilities to mock external services, APIs, or functions during tests to avoid unnecessary complexity and ensure tests are isolated.
- Write Readable and Maintainable Tests: Tests should be easy to understand and maintain. Use descriptive test names and comments when necessary.
5. Conclusion
Both Enzyme and React Testing Library are powerful tools for testing React components, but they have different philosophies and approaches. Enzyme gives you more control over the component's internals, while React Testing Library encourages testing components from the user's perspective, which can result in more robust tests. Depending on your needs and project requirements, you can choose the library that best fits your testing strategy.
By following best practices such as testing component behavior, using appropriate queries, and keeping tests isolated, you can ensure your React components are thoroughly tested and reliable.
Writing Snapshot Tests with Jest
Snapshot testing is a powerful feature provided by Jest that allows you to test React components (or any part of your code) by comparing their rendered output with a saved "snapshot" of that output. This ensures that the component's output remains consistent over time. In this guide, we’ll explore how to write and use snapshot tests in Jest.
1. Introduction to Snapshot Testing
Snapshot testing allows you to take a snapshot of the component's rendered output and compare it to previous snapshots. If the output changes unexpectedly, Jest will alert you to the difference, and you can decide whether to update the snapshot or investigate the cause of the change.
Snapshot tests are typically used for testing the rendered output of components, ensuring that the UI remains consistent and doesn’t unintentionally change.
2. Setting Up Jest for Snapshot Testing
Jest comes with built-in support for snapshot testing, so no additional installation is required. If you already have Jest set up in your project, you can immediately start writing snapshot tests. If not, you can install Jest as follows:
npm install --save-dev jest
Setting Up the Test Environment
In most React applications, you’ll need to install the react-test-renderer
package to render React components for snapshot testing. It’s not bundled with Jest by default, so you can install it with:
npm install --save-dev react-test-renderer
3. Writing Snapshot Tests
Once you have Jest and react-test-renderer
installed, you can start writing snapshot tests for your components. Here's an example:
// src/components/Hello.js
import React from 'react';
const Hello = ({ name }) => {
return Hello, {name}!
;
};
export default Hello;
To write a snapshot test for this component, you can use react-test-renderer
to render the component and then use Jest’s toMatchSnapshot
matcher to compare the output with a saved snapshot.
// src/components/Hello.test.js
import React from 'react';
import renderer from 'react-test-renderer';
import Hello from './Hello';
test('renders correctly', () => {
const tree = renderer.create( ).toJSON();
expect(tree).toMatchSnapshot();
});
In this example, we use react-test-renderer.create()
to render the component, and the toJSON()
method is called to get the rendered output in a JSON format. The toMatchSnapshot()
assertion will save the rendered output as a snapshot and compare it to the previous snapshot on subsequent test runs.
4. Understanding Snapshot Files
When you run the test for the first time, Jest will create a snapshot file in the __snapshots__
directory alongside the test file. The snapshot file contains the rendered output of the component:
src/components/Hello.test.js
├── Hello.js
└── __snapshots__
└── Hello.test.js.snap
The snapshot file will look like this:
exports[`renders correctly 1`] = `
Hello, John!
`;
This snapshot is essentially a serialized version of the component's rendered output. On subsequent test runs, Jest will compare the current output to the saved snapshot and alert you if there are any differences.
5. Updating Snapshots
If the component's rendered output changes intentionally (such as during a UI update), you may need to update the snapshot. To do this, you can run the following command:
npm test -- -u
Jest will update the snapshot files to reflect the new output of the component. Be cautious when updating snapshots, as you should ensure the changes are intentional before doing so.
6. Snapshot Testing Best Practices
- Use for UI Components: Snapshot tests are most effective when used for UI components where the rendered output is stable and predictable.
- Don’t Overuse Snapshots: Avoid using snapshots for non-UI logic or for testing highly dynamic content. It’s best suited for static or semi-static parts of your UI.
- Keep Snapshots Small: When writing snapshot tests, try to keep the snapshots small and focused on specific parts of the component’s output. Large snapshots can make it harder to identify the root cause of failed tests.
- Review Snapshot Changes Carefully: Always review changes to snapshots to ensure that the modifications are intentional and not caused by unintended bugs.
7. Best Practices for Snapshot Tests
- Test User Interactions: While snapshot tests are helpful for comparing the rendered output, you should also write tests to verify how your components behave with user interactions (e.g., button clicks, form submissions).
- Don’t Use Snapshots for Dynamic Content: Avoid using snapshot tests for highly dynamic content that changes frequently, such as data fetched from APIs. Instead, use other testing techniques (e.g., mocking data or using React Testing Library).
- Use for Regression Testing: Snapshot tests are helpful for catching regressions in the UI. If the UI changes unexpectedly, Jest will notify you so that you can investigate the cause.
- Keep Snapshots Versioned: Make sure to version control your snapshot files along with your source code. This ensures that other developers on your team can update and review snapshots when necessary.
8. Conclusion
Snapshot testing with Jest is a powerful tool for ensuring your React components produce consistent output. By writing snapshot tests, you can easily detect unintended UI changes and catch regressions early in the development process. Just be sure to follow best practices to avoid overusing snapshots or introducing unnecessary complexity into your tests.
Snapshot tests are an invaluable tool for maintaining the integrity of your components’ UI, and when used correctly, they can help you catch bugs and prevent regressions in your application.
Mocking API Calls and Dependencies in Tests
When writing tests for your React application, you often need to isolate the component or functionality under test. Mocking API calls and dependencies ensures that your tests are fast, deterministic, and independent of external systems. This guide explains how to mock API calls and other dependencies effectively in your tests.
1. Why Mock API Calls?
Mocking API calls in tests is crucial for several reasons:
- Isolation: It ensures that the test focuses only on the component's logic, not the behavior of external APIs.
- Speed: Tests run faster since no actual network requests are made.
- Deterministic Results: Mock data ensures consistent results, making tests more reliable and easier to debug.
- No Dependency on API Availability: Your tests won't fail due to external API downtime or changes.
2. Setting Up Mocking Tools
Several libraries and tools can help you mock API calls and dependencies in JavaScript. Commonly used tools include:
- Jest: Jest has built-in support for mocking functions, modules, and timers.
- MSW (Mock Service Worker): Mocks API calls at the network level, simulating an actual backend.
- Sinon.js: Provides standalone test spies, stubs, and mocks.
3. Mocking API Calls with Jest
Jest provides a simple way to mock API calls using its jest.mock
function. Here’s an example:
Component to Test
// src/components/UserList.js
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const UserList = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
axios.get('/api/users').then(response => setUsers(response.data));
}, []);
return (
{users.map(user => (
- {user.name}
))}
);
};
export default UserList;
Mocking Axios in Tests
// src/components/UserList.test.js
import React from 'react';
import { render, screen } from '@testing-library/react';
import axios from 'axios';
import UserList from './UserList';
// Mock the axios module
jest.mock('axios');
test('renders user list', async () => {
// Mock API response
axios.get.mockResolvedValue({
data: [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
],
});
render( );
// Wait for the users to be rendered
const userItems = await screen.findAllByRole('listitem');
expect(userItems).toHaveLength(2);
expect(userItems[0]).toHaveTextContent('John Doe');
expect(userItems[1]).toHaveTextContent('Jane Smith');
});
In this example, jest.mock('axios')
replaces the actual axios
module with a mock. The mockResolvedValue
method defines the behavior of the mocked axios.get
call.
4. Mocking Fetch API
If you're using the Fetch API instead of libraries like Axios, you can mock it with Jest's jest.fn
or libraries like whatwg-fetch
. Here’s an example:
global.fetch = jest.fn(() =>
Promise.resolve({
json: () => Promise.resolve([{ id: 1, name: 'John Doe' }]),
})
);
test('fetches and displays data', async () => {
render( );
const userItems = await screen.findAllByRole('listitem');
expect(userItems).toHaveLength(1);
expect(userItems[0]).toHaveTextContent('John Doe');
});
5. Using MSW (Mock Service Worker)
MSW allows you to mock API calls at the network level, providing a realistic mock environment. Here’s how to set it up:
Install MSW
npm install msw --save-dev
Setup Handlers
// src/mocks/handlers.js
import { rest } from 'msw';
export const handlers = [
rest.get('/api/users', (req, res, ctx) => {
return res(
ctx.json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
])
);
}),
];
Start the Worker
// src/setupTests.js
import { setupServer } from 'msw/node';
import { handlers } from './mocks/handlers';
const server = setupServer(...handlers);
// Establish API mocking before all tests.
beforeAll(() => server.listen());
// Reset any request handlers that are declared as a part of our tests (i.e. for testing one-time scenarios).
afterEach(() => server.resetHandlers());
// Clean up after the tests are finished.
afterAll(() => server.close());
6. Mocking Dependencies
Besides API calls, you may also need to mock dependencies like utility functions, date/time libraries, or third-party components. Here's an example of mocking a utility function:
// utils/calculate.js
export const calculateSum = (a, b) => a + b;
// Mocking in tests
jest.mock('./utils/calculate', () => ({
calculateSum: jest.fn(() => 42),
}));
test('uses mocked calculateSum', () => {
const result = calculateSum(1, 2);
expect(result).toBe(42);
});
7. Best Practices for Mocking
- Mock Only What’s Necessary: Avoid over-mocking, as it can make your tests brittle and less meaningful.
- Use MSW for Integration-Like Tests: MSW provides a more realistic environment by mocking API calls at the network level.
- Reset Mocks Between Tests: Always reset mocks using
jest.clearAllMocks()
or similar methods to avoid test interference. - Test Edge Cases: Mock API errors and edge cases to ensure your components handle them gracefully.
8. Conclusion
Mocking API calls and dependencies is a vital skill for writing effective, reliable tests. By isolating the component or functionality under test, you can focus on validating its behavior without external interference. Whether you use Jest's built-in mocking capabilities or tools like MSW, the key is to ensure that your tests are fast, reliable, and easy to maintain.
End-to-End Testing with Cypress or Puppeteer
End-to-End (E2E) testing ensures that your application behaves as expected from the user's perspective. It simulates real user interactions and verifies that all parts of the system work together seamlessly. This guide explains how to set up and use two popular tools for E2E testing: Cypress and Puppeteer.
1. What is End-to-End Testing?
End-to-End testing focuses on testing the application as a whole, from the user interface to the backend and database. It is particularly useful for:
- Validating critical user workflows, such as login, checkout, or form submissions.
- Ensuring that different parts of the application (frontend, backend, and services) integrate well.
- Catching bugs that unit or integration tests might miss.
2. Cypress Overview
Cypress is a modern testing framework built specifically for the web. It is known for its simplicity, fast execution, and real-time reloading.
- Key Features:
- Interactive test runner with real-time feedback.
- Automatic waiting for DOM elements and assertions.
- Built-in time-travel debugging.
- Rich API for simulating user interactions.
Setting Up Cypress
# Install Cypress
npm install cypress --save-dev
# Open Cypress Test Runner
npx cypress open
Writing a Cypress Test
// cypress/e2e/login.spec.js
describe('Login Flow', () => {
it('should allow users to log in', () => {
cy.visit('http://localhost:3000/login');
cy.get('input[name="email"]').type('user@example.com');
cy.get('input[name="password"]').type('password123');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
cy.contains('Welcome, User');
});
});
Running Cypress Tests
You can run Cypress tests in the interactive mode or headless mode:
# Run tests in interactive mode
npx cypress open
# Run tests in headless mode
npx cypress run
3. Puppeteer Overview
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium using the DevTools Protocol. It is ideal for testing UI interactions and automating browser tasks.
- Key Features:
- Full control over the browser environment.
- Support for screenshots, PDF generation, and performance analysis.
- Can be used for both testing and web scraping.
Setting Up Puppeteer
# Install Puppeteer
npm install puppeteer --save-dev
Writing a Puppeteer Test
// tests/login.test.js
const puppeteer = require('puppeteer');
describe('Login Flow', () => {
let browser, page;
beforeAll(async () => {
browser = await puppeteer.launch();
page = await browser.newPage();
});
afterAll(async () => {
await browser.close();
});
test('should allow users to log in', async () => {
await page.goto('http://localhost:3000/login');
await page.type('input[name="email"]', 'user@example.com');
await page.type('input[name="password"]', 'password123');
await page.click('button[type="submit"]');
await page.waitForNavigation();
const url = await page.url();
expect(url).toContain('/dashboard');
const content = await page.content();
expect(content).toMatch(/Welcome, User/);
});
});
Running Puppeteer Tests
Run your Puppeteer tests using Jest or any test runner of your choice:
npx jest tests/login.test.js
4. Cypress vs. Puppeteer: Which One to Use?
The choice between Cypress and Puppeteer depends on your testing needs:
Feature | Cypress | Puppeteer |
---|---|---|
Ease of Use | Simple and beginner-friendly | Requires more setup and coding |
Test Runner | Built-in interactive test runner | Requires external test runner (e.g., Jest) |
Browser Support | Limited to modern browsers | Supports Chrome/Chromium |
Performance | Best for UI testing | Better for performance analysis and advanced tasks |
5. Best Practices for E2E Testing
- Test Critical Flows: Focus on workflows like login, checkout, and form submissions.
- Use Realistic Data: Simulate real user interactions with meaningful data.
- Run Tests in CI/CD: Integrate E2E tests into your CI/CD pipeline to catch issues early.
- Keep Tests Isolated: Avoid dependencies between tests for better reliability.
- Optimize for Speed: Use headless mode for faster test execution in CI environments.
6. Conclusion
End-to-End testing is an essential part of ensuring the quality of your application. Both Cypress and Puppeteer offer powerful tools for creating reliable E2E tests. Cypress is ideal for developers looking for simplicity and a robust test runner, while Puppeteer provides more control for advanced use cases. Choose the tool that best fits your project’s requirements and start building confidence in your application’s workflows.
Fetching Data with fetch
API
The fetch
API is a modern, promise-based way to make HTTP requests in JavaScript. It is widely used for fetching data from APIs in web applications. This guide explains how to use the fetch
API effectively in your projects.
1. Introduction to the fetch
API
The fetch
API provides a global function fetch()
that allows you to make network requests. It returns a Promise
that resolves to the Response
object.
// Basic syntax of fetch
fetch(url, options)
.then(response => {
// Handle the response
})
.catch(error => {
// Handle errors
});
2. Fetching Data from an API
Here's an example of fetching data from a REST API using the fetch
API:
// Example: Fetching data from a public API
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse JSON data
})
.then(data => {
console.log('Fetched data:', data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
3. Sending Data with POST Requests
The fetch
API can also be used to send data to a server using POST requests. You need to specify the HTTP method and include the data in the request body.
// Example: Sending a POST request
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
})
.then(response => {
if (!response.ok) {
throw new Error('Failed to send data');
}
return response.json();
})
.then(data => {
console.log('Data sent successfully:', data);
})
.catch(error => {
console.error('Error sending data:', error);
});
4. Handling Errors
Error handling is crucial when using the fetch
API. You should check the response status and handle network errors properly.
// Example: Error handling
fetch('https://jsonplaceholder.typicode.com/invalid-endpoint')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log('Data:', data);
})
.catch(error => {
console.error('Error fetching data:', error.message);
});
5. Using Async/Await with fetch
For cleaner and more readable code, you can use async
and await
with the fetch
API:
// Example: Using async/await with fetch
async function fetchData() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
console.log('Fetched data:', data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
6. Handling Timeouts
The fetch
API does not natively support request timeouts, but you can implement a timeout using Promise.race
:
// Example: Handling timeouts
const fetchWithTimeout = (url, timeout = 5000) => {
return Promise.race([
fetch(url),
new Promise((_, reject) =>
setTimeout(() => reject(new Error('Request timed out')), timeout)
),
]);
};
fetchWithTimeout('https://jsonplaceholder.typicode.com/posts', 3000)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log('Fetched data:', data);
})
.catch(error => {
console.error('Error:', error.message);
});
7. Best Practices
- Check Response Status: Always check the
ok
property of the response. - Use Async/Await: Write more readable and maintainable asynchronous code.
- Handle Errors Gracefully: Use try-catch blocks and provide user-friendly error messages.
- Set Timeouts: Implement timeouts to handle unresponsive servers.
- Use Appropriate Headers: Set headers like
Content-Type
when sending data.
8. Conclusion
The fetch
API is a powerful and versatile tool for making HTTP requests in JavaScript. Whether you are fetching data, sending data, or handling errors, the fetch
API provides a simple and efficient way to interact with APIs. By following best practices and leveraging features like async/await and timeouts, you can build robust and reliable data-fetching logic for your applications.
Using Axios for API Requests
Axios is a popular JavaScript library used for making HTTP requests. It is promise-based, supports async/await, and provides several features that make it a powerful tool for handling API requests in web applications.
1. Introduction to Axios
Axios is a lightweight HTTP client that works in both browser and Node.js environments. It offers a clean API for making requests, handling responses, and managing errors.
To install Axios, use the following command:
npm install axios
2. Making GET Requests
The axios.get
method is used to fetch data from a server. Here’s an example:
// Example: Making a GET request
import axios from 'axios';
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log('Fetched data:', response.data);
})
.catch(error => {
console.error('Error fetching data:', error.message);
});
3. Making POST Requests
The axios.post
method is used to send data to a server. You can include headers and a request body:
// Example: Making a POST request
import axios from 'axios';
axios.post('https://jsonplaceholder.typicode.com/posts', {
title: 'foo',
body: 'bar',
userId: 1,
})
.then(response => {
console.log('Data sent successfully:', response.data);
})
.catch(error => {
console.error('Error sending data:', error.message);
});
4. Using Async/Await
Axios works seamlessly with async/await for cleaner and more readable code:
// Example: Using async/await with Axios
import axios from 'axios';
async function fetchData() {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts');
console.log('Fetched data:', response.data);
} catch (error) {
console.error('Error fetching data:', error.message);
}
}
fetchData();
5. Configuring Axios Requests
Axios allows you to configure requests by passing an object with options:
// Example: Configuring a request
import axios from 'axios';
axios({
method: 'get',
url: 'https://jsonplaceholder.typicode.com/posts',
headers: {
'Authorization': 'Bearer YOUR_TOKEN_HERE',
},
})
.then(response => {
console.log('Fetched data:', response.data);
})
.catch(error => {
console.error('Error:', error.message);
});
6. Interceptors for Requests and Responses
Axios provides interceptors to process requests or responses before they are handled:
// Example: Using Axios interceptors
import axios from 'axios';
// Adding a request interceptor
axios.interceptors.request.use(config => {
console.log('Request sent:', config);
return config;
}, error => {
return Promise.reject(error);
});
// Adding a response interceptor
axios.interceptors.response.use(response => {
console.log('Response received:', response);
return response;
}, error => {
return Promise.reject(error);
});
7. Canceling Requests
You can cancel an Axios request using a CancelToken
:
// Example: Canceling a request
import axios from 'axios';
const CancelToken = axios.CancelToken;
const source = CancelToken.source();
axios.get('https://jsonplaceholder.typicode.com/posts', {
cancelToken: source.token,
})
.then(response => {
console.log('Fetched data:', response.data);
})
.catch(error => {
if (axios.isCancel(error)) {
console.log('Request canceled:', error.message);
} else {
console.error('Error:', error.message);
}
});
// Cancel the request
source.cancel('Request canceled by the user.');
8. Global Axios Configuration
You can set default configurations for all Axios requests:
// Example: Setting global defaults
import axios from 'axios';
axios.defaults.baseURL = 'https://jsonplaceholder.typicode.com';
axios.defaults.headers.common['Authorization'] = 'Bearer YOUR_TOKEN_HERE';
axios.defaults.headers.post['Content-Type'] = 'application/json';
axios.get('/posts')
.then(response => {
console.log('Fetched data:', response.data);
})
.catch(error => {
console.error('Error:', error.message);
});
9. Error Handling
Axios provides detailed error objects, making it easier to handle errors:
// Example: Error handling with Axios
import axios from 'axios';
axios.get('https://jsonplaceholder.typicode.com/invalid-endpoint')
.then(response => {
console.log('Fetched data:', response.data);
})
.catch(error => {
if (error.response) {
console.error('Server responded with an error:', error.response.status);
} else if (error.request) {
console.error('No response received:', error.request);
} else {
console.error('Error setting up request:', error.message);
}
});
10. Conclusion
Axios is a powerful library for handling HTTP requests. It simplifies fetching and sending data, offers features like interceptors, and works well with async/await. By using Axios, you can build robust and maintainable data-fetching logic in your applications.
Handling HTTP Requests with useEffect
The useEffect
hook in React is a powerful tool for managing side effects, including making HTTP requests. By combining useEffect
with an HTTP client like fetch
or axios
, you can efficiently fetch or send data when components mount or update.
1. Basic Use of useEffect
for HTTP Requests
The useEffect
hook is often used to fetch data when a component mounts. Here's an example using the built-in fetch
API:
// Example: Fetching data with useEffect
import React, { useEffect, useState } from 'react';
function DataFetcher() {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
})
.catch(error => {
console.error('Error fetching data:', error);
setLoading(false);
});
}, []); // Empty dependency array ensures this runs only once when the component mounts.
return (
{loading ? (
Loading...
) : (
{data.map(post => (
- {post.title}
))}
)}
);
}
export default DataFetcher;
2. Using axios
with useEffect
Here's an example of using Axios to fetch data:
// Example: Fetching data with Axios and useEffect
import React, { useEffect, useState } from 'react';
import axios from 'axios';
function DataFetcher() {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
setData(response.data);
setLoading(false);
})
.catch(error => {
console.error('Error fetching data:', error);
setLoading(false);
});
}, []);
return (
{loading ? (
Loading...
) : (
{data.map(post => (
- {post.title}
))}
)}
);
}
export default DataFetcher;
3. Cleanup with useEffect
for Aborting Requests
When making HTTP requests, it's important to handle cleanup to avoid memory leaks, especially when a component unmounts before a request completes.
Here's an example of using the AbortController
with fetch
:
// Example: Cleanup with AbortController
import React, { useEffect, useState } from 'react';
function DataFetcher() {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
const controller = new AbortController();
const signal = controller.signal;
fetch('https://jsonplaceholder.typicode.com/posts', { signal })
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Fetch aborted');
} else {
console.error('Error fetching data:', error);
}
setLoading(false);
});
// Cleanup function to abort the request
return () => controller.abort();
}, []);
return (
{loading ? (
Loading...
) : (
{data.map(post => (
- {post.title}
))}
)}
);
}
export default DataFetcher;
4. Dependency Array and Conditional Fetching
The dependency array in useEffect
determines when the effect runs. You can conditionally fetch data based on dependencies:
// Example: Conditional fetching with dependency array
import React, { useEffect, useState } from 'react';
function UserFetcher({ userId }) {
const [user, setUser] = useState(null);
useEffect(() => {
if (!userId) return;
fetch(`https://jsonplaceholder.typicode.com/users/${userId}`)
.then(response => response.json())
.then(data => setUser(data))
.catch(error => console.error('Error fetching user:', error));
}, [userId]); // Effect runs when userId changes
return (
{user ? (
{user.name}
) : (
No user selected
)}
);
}
export default UserFetcher;
5. Error Handling and Loading States
Always include error handling and loading state management for a better user experience:
- Display a loading indicator while fetching data.
- Handle and display error messages if requests fail.
In the examples above, these states are managed using useState
.
6. Best Practices
- Use a dedicated HTTP client like Axios for cleaner and more feature-rich handling.
- Always handle errors and provide fallback UI.
- Clean up effects to prevent memory leaks.
- Avoid running effects too often by carefully managing the dependency array.
7. Conclusion
By using useEffect
with HTTP requests, you can fetch or send data effectively in React. Understanding cleanup, dependency arrays, and proper error handling will help you build robust applications.
Authentication with REST APIs (JWT, OAuth)
Authentication is a critical aspect of securing REST APIs. Two common approaches for implementing authentication are JSON Web Tokens (JWT) and OAuth. This guide covers how these methods work and their implementation in REST APIs.
1. JSON Web Tokens (JWT)
JWT is a compact, self-contained token format that is widely used for authentication and information exchange. It consists of three parts: Header
, Payload
, and Signature
, encoded as a single string.
How JWT Works:
- The client sends a login request with their credentials (username and password).
- If the credentials are valid, the server generates a JWT and sends it to the client.
- The client includes the JWT in the
Authorization
header for subsequent requests. - The server verifies the JWT to authenticate the user.
JWT Example:
// Server-side: Generating a JWT
const jwt = require('jsonwebtoken');
const secretKey = 'your-secret-key';
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Validate user credentials (e.g., check database)
if (username === 'user' && password === 'pass') {
const token = jwt.sign({ username }, secretKey, { expiresIn: '1h' });
res.json({ token });
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
// Middleware: Verifying the JWT
function authenticateJWT(req, res, next) {
const token = req.header('Authorization')?.split(' ')[1];
if (!token) return res.status(403).json({ message: 'Token required' });
jwt.verify(token, secretKey, (err, user) => {
if (err) return res.status(403).json({ message: 'Invalid token' });
req.user = user;
next();
});
}
// Protected route
app.get('/protected', authenticateJWT, (req, res) => {
res.json({ message: `Hello, ${req.user.username}` });
});
Benefits of JWT:
- Stateless: No need to store session data on the server.
- Scalable: Suitable for distributed systems and microservices.
- Flexible: Can store additional claims (e.g., roles, permissions).
2. OAuth
OAuth is a protocol for secure authorization, enabling users to grant third-party applications limited access to their resources without sharing credentials. OAuth 2.0 is the most widely used version.
How OAuth Works:
- The client requests authorization from the user (e.g., via a login form or redirect).
- The user authenticates with the authorization server (e.g., Google or Facebook).
- The authorization server issues an access token to the client.
- The client includes the access token in API requests to access protected resources.
OAuth Example with Passport.js:
// Setting up Google OAuth with Passport.js
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth20').Strategy;
passport.use(new GoogleStrategy({
clientID: 'your-google-client-id',
clientSecret: 'your-google-client-secret',
callbackURL: 'http://localhost:3000/auth/google/callback'
}, (accessToken, refreshToken, profile, done) => {
// Save user profile or handle login logic
return done(null, profile);
}));
// Routes
app.get('/auth/google', passport.authenticate('google', { scope: ['profile', 'email'] }));
app.get('/auth/google/callback',
passport.authenticate('google', { failureRedirect: '/' }),
(req, res) => {
res.redirect('/dashboard');
});
);
Benefits of OAuth:
- Delegated Access: Users can allow third-party apps to access specific resources.
- Secure: Tokens are short-lived and can be revoked.
- Widely Supported: Works with major identity providers (Google, Facebook, etc.).
3. Key Differences Between JWT and OAuth
Feature | JWT | OAuth |
---|---|---|
Purpose | Authentication and data exchange | Authorization and delegated access |
Usage | Stateless authentication | Secure third-party access |
Scalability | Highly scalable | Depends on the implementation |
4. Best Practices for API Authentication
- Use HTTPS to secure communication.
- Store tokens securely (e.g., in HTTP-only cookies).
- Set token expiration and implement refresh tokens.
- Use strong secret keys and regularly rotate them.
- Limit the scope and permissions of tokens.
5. Conclusion
Both JWT and OAuth are powerful tools for securing REST APIs. JWT is ideal for stateless authentication, while OAuth excels in scenarios requiring delegated access. By choosing the right approach and following best practices, you can build secure and scalable applications.
Managing Global Data with React Context or Redux
In modern React applications, managing global data efficiently is essential for scalable and maintainable code. Two popular approaches for managing global state are the React Context API and Redux. This guide explores when and how to use each approach.
1. React Context API
The React Context API is built into React and provides a simple way to manage global state across components. It eliminates the need for prop drilling by making data available to any component in the tree.
How React Context Works:
- Create a Context using
React.createContext
. - Wrap the component tree with a
Provider
to supply the context value. - Access the context value using the
useContext
hook or theConsumer
component.
Example: Using React Context for Theme Management
// Creating a Context
const ThemeContext = React.createContext();
// Providing Context Value
function ThemeProvider({ children }) {
const [theme, setTheme] = React.useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
{children}
);
}
// Consuming Context Value
function ThemeToggler() {
const { theme, toggleTheme } = React.useContext(ThemeContext);
return (
);
}
// Using the Provider
function App() {
return (
);
}
Benefits of React Context:
- Simple and easy to implement.
- No external dependencies required.
- Ideal for small to medium-sized applications.
2. Redux
Redux is a state management library that provides a predictable state container for JavaScript applications. It is particularly useful for managing complex global state across large applications.
Core Concepts of Redux:
- Store: Centralized state container.
- Actions: Plain JavaScript objects describing what happened.
- Reducers: Pure functions that update the state based on actions.
Example: Managing Counter State with Redux
// Actions
const increment = () => ({ type: 'INCREMENT' });
const decrement = () => ({ type: 'DECREMENT' });
// Reducer
function counterReducer(state = 0, action) {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
// Store
const { createStore } = require('redux');
const store = createStore(counterReducer);
// Dispatching Actions
store.dispatch(increment());
store.dispatch(decrement());
console.log(store.getState()); // Logs the updated state
Benefits of Redux:
- Predictable state updates with strict action-based flow.
- DevTools support for debugging and time-traveling.
- Scalable for large applications with complex state.
3. Comparison: React Context vs Redux
Feature | React Context | Redux |
---|---|---|
Use Case | Small to medium apps with simple state. | Large apps with complex state logic. |
Learning Curve | Low | Moderate to high |
Performance | May re-render unnecessary components. | Optimized with middleware and selectors. |
Dependencies | None | Requires redux and react-redux packages. |
4. Choosing the Right Tool
- React Context: Use for simple state management tasks like themes, authentication, or user preferences.
- Redux: Use for large-scale applications with complex state logic, asynchronous operations, and debugging needs.
5. Conclusion
Both React Context and Redux are powerful tools for managing global state. The choice depends on the complexity of your application and your specific requirements. React Context is simple and lightweight, while Redux offers robust tools for handling more challenging state management scenarios.
Working with WebSockets in React
WebSockets provide a full-duplex communication channel over a single, long-lived connection between a client and a server. In React applications, WebSockets are commonly used for real-time features like live chats, notifications, and live updates. This guide explains how to integrate WebSockets into your React projects.
1. Understanding WebSockets
WebSockets enable real-time, two-way communication between the client and server. Unlike traditional HTTP requests, WebSockets keep the connection open, allowing data to be sent and received at any time without the overhead of repeated HTTP requests.
Common Use Cases for WebSockets:
- Real-time chat applications.
- Live sports score updates.
- Collaborative tools (e.g., shared document editing).
- Stock market price updates.
2. Setting Up WebSocket Communication
Follow these steps to establish WebSocket communication in a React application:
Step 1: Create a WebSocket Connection
import React, { useEffect, useState } from 'react';
function WebSocketComponent() {
const [messages, setMessages] = useState([]);
const [socket, setSocket] = useState(null);
useEffect(() => {
// Create a WebSocket connection
const ws = new WebSocket('wss://example.com/socket');
// Handle incoming messages
ws.onmessage = (event) => {
const data = JSON.parse(event.data);
setMessages((prev) => [...prev, data]);
};
// Handle WebSocket errors
ws.onerror = (error) => {
console.error('WebSocket Error:', error);
};
// Cleanup the WebSocket connection on component unmount
return () => {
ws.close();
};
}, []);
return (
WebSocket Messages
{messages.map((message, index) => (
- {message.content}
))}
);
}
export default WebSocketComponent;
Step 2: Sending Messages through WebSocket
// Add a function to send messages
function WebSocketComponent() {
const [message, setMessage] = useState('');
const [socket, setSocket] = useState(null);
useEffect(() => {
const ws = new WebSocket('wss://example.com/socket');
setSocket(ws);
ws.onmessage = (event) => {
console.log('Message received:', event.data);
};
return () => {
ws.close();
};
}, []);
const sendMessage = () => {
if (socket && socket.readyState === WebSocket.OPEN) {
socket.send(JSON.stringify({ content: message }));
setMessage('');
} else {
console.error('WebSocket is not open.');
}
};
return (
<input
type="text"
value={message}
onChange={(e) => setMessage(e.target.value)}
placeholder="Type a message"
/>
);
}
3. Using Third-Party Libraries
For advanced WebSocket functionality, third-party libraries like socket.io-client
or stompjs
are often used:
Example: Using socket.io-client
import React, { useEffect, useState } from 'react';
import { io } from 'socket.io-client';
const socket = io('https://example.com');
function SocketIOComponent() {
const [messages, setMessages] = useState([]);
useEffect(() => {
socket.on('message', (data) => {
setMessages((prev) => [...prev, data]);
});
return () => {
socket.off('message');
};
}, []);
const sendMessage = () => {
socket.emit('message', { content: 'Hello, Server!' });
};
return (
Messages:
{messages.map((msg, index) => (
- {msg.content}
))}
);
}
export default SocketIOComponent;
4. Best Practices
- Always handle WebSocket connection errors and retries.
- Close WebSocket connections when the component unmounts to prevent memory leaks.
- Use secure WebSocket connections (
wss://
) for production environments. - Implement server-side rate-limiting to prevent abuse.
5. Conclusion
WebSockets are a powerful way to implement real-time features in your React applications. Whether you use the native WebSocket API or a library like socket.io-client
, understanding how to manage connections and handle messages effectively is key to building responsive and interactive applications.
Introduction to React Hooks
React Hooks, introduced in React 16.8, are functions that let you use state and other React features in functional components. They simplify component logic by eliminating the need for class components while providing a cleaner and more reusable way to manage state, lifecycle, and side effects.
1. Why Hooks?
Hooks address several challenges in React development:
- Reuse Logic: Hooks make it easier to share stateful logic between components without resorting to higher-order components (HOCs) or render props.
- Simplify Components: Functional components with hooks are often easier to read and maintain compared to class components.
- Eliminate Boilerplate: No need to manage
this
or use lifecycle methods likecomponentDidMount
andcomponentDidUpdate
manually.
2. Basic Hooks
React provides several built-in hooks, each serving a specific purpose:
2.1. useState
: Managing State
The useState
hook allows you to add state to functional components:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
2.2. useEffect
: Managing Side Effects
The useEffect
hook replaces lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
:
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => setSeconds((prev) => prev + 1), 1000);
return () => clearInterval(interval); // Cleanup
}, []); // Empty dependency array means it runs once on mount
return <p>Seconds elapsed: {seconds}</p>;
}
2.3. useContext
: Accessing Context
The useContext
hook lets you consume context values directly in functional components:
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function ThemedComponent() {
const theme = useContext(ThemeContext);
return <div>Current theme: {theme}</div>;
}
3. Advanced Hooks
React also provides advanced hooks for more specific use cases:
3.1. useReducer
: Complex State Management
import React, { useReducer } from 'react';
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
3.2. useRef
: Accessing DOM and Persistent Values
import React, { useRef } from 'react';
function InputFocus() {
const inputRef = useRef();
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={() => inputRef.current.focus()}>Focus Input</button>
</div>
);
}
4. Rules of Hooks
Hooks come with two rules to ensure they work correctly:
- Call Hooks at the Top Level: Do not call hooks inside loops, conditions, or nested functions.
- Call Hooks Only in React Functions: Hooks should only be called in functional components or custom hooks.
5. Custom Hooks
You can create custom hooks to encapsulate reusable logic:
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch(url)
.then((response) => response.json())
.then((data) => {
setData(data);
setLoading(false);
});
}, [url]);
return { data, loading };
}
// Usage
function App() {
const { data, loading } = useFetch('https://api.example.com/data');
if (loading) return <p>Loading...</p>;
return <div>{JSON.stringify(data)}</div>;
}
6. Conclusion
React Hooks revolutionize how we build components by combining the simplicity of functional components with powerful state and lifecycle management. By mastering hooks, you can write cleaner, more modular, and more maintainable React code.
Commonly Used Hooks
React provides several built-in hooks that are commonly used for various tasks in functional components. These hooks allow developers to manage state, side effects, context, and more, in a clean and efficient way.
1. useState
: Managing State
The useState
hook is used for adding state to functional components. It returns a state variable and a function to update it.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
2. useEffect
: Side Effects
The useEffect
hook manages side effects like fetching data, subscribing to services, and manually modifying the DOM. It replaces lifecycle methods in class components like componentDidMount
and componentDidUpdate
.
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => setSeconds((prev) => prev + 1), 1000);
return () => clearInterval(interval); // Cleanup
}, []); // Empty dependency array means it runs once on mount
return <p>Seconds elapsed: {seconds}</p>;
}
3. useContext
: Accessing Context
The useContext
hook provides a way to access values from a React context without needing to use the Context.Consumer
component.
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function ThemedComponent() {
const theme = useContext(ThemeContext);
return <div>Current theme: {theme}</div>;
}
4. useReducer
: Advanced State Management
The useReducer
hook is an alternative to useState
for managing more complex state. It is often used in scenarios where state depends on previous state or multiple actions update the state.
import React, { useReducer } from 'react';
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
5. useMemo
: Memoization for Optimization
The useMemo
hook is used to optimize performance by memoizing the result of a computation. It only recomputes the value if the dependencies have changed.
import React, { useMemo, useState } from 'react';
function ExpensiveComponent() {
const [count, setCount] = useState(0);
const expensiveValue = useMemo(() => {
return count * 1000; // Expensive calculation
}, [count]);
return (
<div>
<p>Expensive calculation result: {expensiveValue}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
6. useCallback
: Preventing Unnecessary Re-renders
The useCallback
hook is used to memoize functions so that they do not get recreated on every render. This is useful when passing functions as props to child components that rely on reference equality.
import React, { useCallback, useState } from 'react';
function Button({ onClick }) {
return <button onClick={onClick}>Click Me</button>;
}
function ParentComponent() {
const [count, setCount] = useState(0);
const memoizedCallback = useCallback(() => {
console.log('Button clicked!');
}, []); // Empty dependency array means it will not change
return (
<div>
<p>Count: {count}</p>
<Button onClick={memoizedCallback} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
7. useRef
: Accessing DOM Elements
The useRef
hook is used to access and interact with DOM elements directly. It can also be used to persist values across renders without triggering re-renders.
import React, { useRef } from 'react';
function InputFocus() {
const inputRef = useRef();
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={() => inputRef.current.focus()}>Focus Input</button>
</div>
);
}
Custom Hooks: Creating Reusable Logic
Custom hooks in React allow you to extract reusable logic from your components. They are JavaScript functions whose names start with use
and can use other React hooks inside them. Custom hooks make your code cleaner and more modular.
Why Use Custom Hooks?
Custom hooks help in encapsulating logic that can be reused across multiple components. They can help reduce code duplication and improve maintainability.
Basic Example of a Custom Hook
In this example, we will create a custom hook that manages the window size. The hook will return the width and height of the window:
import { useState, useEffect } from 'react';
// Custom Hook to track window size
function useWindowSize() {
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight,
});
useEffect(() => {
const handleResize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight,
});
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []); // Empty dependency array to run only once
return windowSize;
}
function MyComponent() {
const { width, height } = useWindowSize();
return (
<div>
<p>Window Width: {width}</p>
<p>Window Height: {height}</p>
</div>
);
}
How to Use Custom Hooks
To use a custom hook, simply call it like a regular function in your component. Custom hooks can return any value, including state, functions, or objects, that your component can use.
Example: Custom Hook for Local Storage
Here is another example of a custom hook that interacts with localStorage
to manage persistent state across page reloads:
import { useState } from 'react';
// Custom Hook to manage localStorage
function useLocalStorage(key, initialValue) {
const [storedValue, setStoredValue] = useState(() => {
try {
const item = window.localStorage.getItem(key);
return item ? JSON.parse(item) : initialValue;
} catch (error) {
console.error(error);
return initialValue;
}
});
const setValue = (value) => {
try {
setStoredValue(value);
window.localStorage.setItem(key, JSON.stringify(value));
} catch (error) {
console.error(error);
}
};
return [storedValue, setValue];
}
function MyComponent() {
const [name, setName] = useLocalStorage('name', 'John Doe');
return (
<div>
<p>Name: {name}</p>
<button onClick={() => setName('Jane Doe')}>Change Name</button>
</div>
);
}
Rules for Creating Custom Hooks
When creating custom hooks, it's important to follow these rules:
- Custom hooks must start with
use
to ensure React can identify them as hooks. - Custom hooks can call other hooks (like
useState
,useEffect
, etc.), but they should not be called conditionally or in loops. - Custom hooks should be used in the same way as built-in hooks—only inside functional components or other hooks.
Advanced Example: Custom Hook for Fetching Data
Here's a more advanced example where we create a custom hook to fetch data from an API:
import { useState, useEffect } from 'react';
// Custom Hook to fetch data
function useFetch(url) {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (error) {
setError(error);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]); // Re-fetch data when the URL changes
return { data, error, loading };
}
function MyComponent() {
const { data, error, loading } = useFetch('https://api.example.com/data');
if (loading) return <p>Loading...</p>
if (error) return <p>Error: {error.message}</p>
return (
<div>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
Introduction to TypeScript and Benefits
TypeScript is a superset of JavaScript that adds static typing to the language. It allows developers to catch type-related errors during development and provides better tooling and editor support. TypeScript compiles to plain JavaScript, which means it runs anywhere JavaScript runs, but with additional benefits like type checking, interfaces, and more.
Why Use TypeScript?
TypeScript offers several key benefits that make it appealing for developers:
- Static Typing: TypeScript allows you to define types for variables, function parameters, and return values, helping catch type-related errors early in the development process.
- Improved Developer Experience: TypeScript offers better editor support, including autocompletion, navigation, and refactoring tools, which enhances productivity.
- Strong Type Inference: Even without explicitly defining types, TypeScript can infer types based on context, reducing the need for manual type annotations.
- Better Tooling and IDE Support: TypeScript integrates well with modern IDEs, providing features like inline error checking, code suggestions, and type information.
- Scalability: TypeScript helps manage large codebases by providing a clear structure and enforcing type safety across the application.
Setting Up TypeScript
To get started with TypeScript, you can install it globally using npm:
npm install -g typescript
After installing TypeScript, you can create a configuration file for TypeScript by running the following command:
tsc --init
Once you've set up TypeScript, you can start writing TypeScript files with the .ts
extension (for normal files) or .tsx
(for files that contain JSX).
Basic TypeScript Syntax
TypeScript introduces type annotations to JavaScript. Here’s a basic example:
let message: string = "Hello, TypeScript!";
message = 42; // Error: Type 'number' is not assignable to type 'string'
In the example above, the variable message
is declared with a type annotation of string
, so assigning a number
to it results in an error.
Defining Types in TypeScript
TypeScript allows you to define types for variables, function parameters, and return values. Here’s how you can define custom types:
type Person = {
name: string;
age: number;
};
const person: Person = {
name: "John",
age: 30
};
In this example, we define a Person
type with two properties: name
and age
. We then create an object that conforms to this type.
Interfaces in TypeScript
Interfaces in TypeScript are used to define the shape of an object. They can be used to enforce the structure of classes or objects:
interface Employee {
id: number;
name: string;
position: string;
}
const employee: Employee = {
id: 1,
name: "Alice",
position: "Developer"
};
In this example, we use an interface
to define the shape of an Employee
object. The employee
object must have an id
, name
, and position
as per the interface definition.
TypeScript and Functions
TypeScript also allows you to specify the types for function parameters and return values:
function greet(name: string): string {
return "Hello, " + name;
}
console.log(greet("John")); // "Hello, John"
In this example, the function greet
accepts a string
as its argument and returns a string
.
Generics in TypeScript
Generics allow you to write flexible functions and classes that work with any data type while maintaining type safety. Here's an example:
function identity(value: T): T {
return value;
}
console.log(identity("Hello, TypeScript!")); // "Hello, TypeScript!"
console.log(identity(42)); // 42
In this example, the identity
function works with any type T
, and TypeScript ensures that the function returns the same type as the argument passed.
Conclusion
TypeScript is a powerful tool that adds type safety to JavaScript, helping developers write more reliable and maintainable code. With its rich feature set, including static typing, interfaces, and generics, TypeScript is especially useful for large-scale applications and teams. By adopting TypeScript, you can catch errors earlier in the development process, improve your tooling, and write cleaner code.
Setting Up TypeScript in a React Project
TypeScript can be easily added to a React project to provide type safety and better tooling support. In this section, we will guide you through the steps of setting up TypeScript in a React application.
Step 1: Create a New React Project
If you haven't created a React project yet, you can do so using Create React App. Here’s the command to create a new React project:
npx create-react-app my-app
Once the project is created, navigate to the project directory:
cd my-app
Step 2: Install TypeScript and Type Definitions
To add TypeScript to your React project, you need to install TypeScript and the necessary type definitions for React:
npm install --save typescript @types/react @types/react-dom
This command installs TypeScript and the type definitions for React and React DOM, which allow TypeScript to understand the React-specific syntax and APIs.
Step 3: Rename Files to .tsx
Once TypeScript is installed, you need to rename your React component files from .js
to .tsx
(for files containing JSX) or .ts
(for regular TypeScript files). For example:
src/App.js → src/App.tsx
This allows TypeScript to process your files and check them for type errors.
Step 4: Add a tsconfig.json File
TypeScript requires a configuration file called tsconfig.json
to specify compiler options. Create this file in the root of your project and add the following basic configuration:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "es6"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"jsx": "react"
},
"include": ["src"]
}
This configuration tells TypeScript to:
- Target ECMAScript 5 for compatibility.
- Include the necessary
dom
andes6
libraries. - Allow JavaScript files to be compiled alongside TypeScript files.
- Skip library checks for faster compilation.
- Enable JSX support for React components.
- Include all files in the
src
directory for compilation.
Step 5: Start the Development Server
Once you have completed the setup, you can start the development server by running:
npm start
The application should now be running with TypeScript support! TypeScript will begin checking your code for any type errors and provide suggestions based on the types you define.
Step 6: Add Type Annotations to Your Code
With TypeScript set up, you can start adding type annotations to your components and functions. Here's an example:
import React, { useState } from 'react';
type CounterProps = {
initialCount: number;
};
const Counter: React.FC = ({ initialCount }) => {
const [count, setCount] = useState(initialCount);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
In this example, we define a CounterProps
type that specifies the expected type for the initialCount
prop. We also provide a type for the useState
hook to ensure that count
is always a number.
Step 7: Optional TypeScript Features
There are additional TypeScript features that you can use to enhance your code, such as:
- Interfaces: Define the shape of objects and enforce structure in your components.
- Enums: Create named constants to represent a set of values.
- Generics: Create reusable components and functions that work with any data type.
- Type Aliases: Create custom types for more complex data structures.
Conclusion
With TypeScript, you can add type safety to your React project, improving code quality and reducing the risk of bugs. By following the steps above, you can set up TypeScript in your React project and start benefiting from its features right away.
Typing Props, State, and Events in TypeScript
TypeScript enhances your React code by allowing you to add types to props, state, and events. In this section, we will explore how to type these elements to improve type safety and tooling support.
Typing Props
Props are an essential part of React components. In TypeScript, you can type props by defining a type or interface. Here’s how you can do it:
import React from 'react';
type GreetingProps = {
name: string;
};
const Greeting: React.FC = ({ name }) => {
return (
<div>
Hello, {name}!
</div>
);
};
export default Greeting;
In this example, we define a GreetingProps
type that expects a name
property of type string
.
Typing State
You can type the state using the useState
hook. Here's an example of how to type state in TypeScript:
import React, { useState } from 'react';
const Counter: React.FC = () => {
const [count, setCount] = useState<number>(0);
return (
<div>
Count: {count}
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Here, we use useState<number>
to ensure that the count
state is of type number
.
Typing Events
In React, event handlers are typed differently based on the event type. TypeScript can help you type events for better safety. Here’s an example of typing an event handler:
import React, { useState } from 'react';
const ClickButton: React.FC = () => {
const [clicked, setClicked] = useState<boolean>(false);
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
setClicked(!clicked);
console.log(event.target);
};
return (
<div>
<button onClick={handleClick}>
{clicked ? 'Clicked!' : 'Click me'}
</button>
</div>
);
};
export default ClickButton;
In the handleClick
function, we type the event
parameter as React.MouseEvent<HTMLButtonElement>
, specifying that the event is a mouse click on a button element.
Conclusion
Typing props, state, and events in TypeScript can significantly improve the development experience by providing type safety and making it easier to catch errors early. By using the examples above, you can start typing your React components to gain the benefits of TypeScript's static typing.
TypeScript with React Hooks
React hooks allow you to add state and lifecycle features to functional components, and with TypeScript, you can add type safety to these hooks. In this section, we will explore how to use TypeScript with React hooks like useState
, useEffect
, useReducer
, and more.
Typing useState Hook
The useState
hook is used to manage state in functional components. You can type the state by providing a type argument to useState
.
import React, { useState } from 'react';
const Counter: React.FC = () => {
const [count, setCount] = useState<number>(0);
return (
<div>
Count: {count}
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
In this example, we use useState<number>
to ensure that the count
state is of type number
.
Typing useEffect Hook
The useEffect
hook is used for side effects such as data fetching, subscriptions, or DOM updates. TypeScript can infer the types of the effect, but you can also specify types for the dependencies or state changes within the effect.
import React, { useState, useEffect } from 'react';
const FetchData: React.FC = () => {
const [data, setData] = useState<string>('');
useEffect(() => {
fetch('https://api.example.com')
.then(response => response.json())
.then(data => setData(data.message));
}, []);
return (
<div>
{data ? data : 'Loading...'}
</div>
);
};
export default FetchData;
Here, TypeScript automatically infers the type of the data
state as string
, and the effect doesn't require any additional type annotations.
Typing useReducer Hook
The useReducer
hook is used for more complex state logic, similar to Redux. You can type the state and actions in a similar way as you do for useState
.
import React, { useReducer } from 'react';
type State = {
count: number;
};
type Action = {
type: string;
};
const reducer = (state: State, action: Action): State => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
const Counter: React.FC = () => {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we define a State
type for the state and an Action
type for the dispatched actions. The reducer function is typed to ensure the correct state and action types are used.
Typing useRef Hook
The useRef
hook is used to persist values across renders without causing re-renders. You can type the reference object to specify the type of DOM element or value it references.
import React, { useRef } from 'react';
const FocusInput: React.FC = () => {
const inputRef = useRef<HTMLInputElement | null>(null);
const focusInput = () => {
if (inputRef.current) {
inputRef.current.focus();
}
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={focusInput}>Focus Input</button>
</div>
);
};
export default FocusInput;
Here, we use useRef<HTMLInputElement | null>
to type the reference to the input element, ensuring that the reference is either null
or an HTMLInputElement
.
Conclusion
By combining React hooks with TypeScript, you can enhance your development workflow with type safety. You can type all the hooks used in your components—useState
, useEffect
, useReducer
, and useRef
—to catch potential errors and improve code maintainability. Start typing your hooks today to take full advantage of TypeScript in your React applications!
Using TypeScript with Redux and Context API
TypeScript works seamlessly with both Redux and the Context API in React to provide type safety and better tooling. In this section, we'll explore how to use TypeScript with Redux and the Context API to manage state in your React applications.
Using TypeScript with Redux
Redux is a popular state management library, and TypeScript can help you ensure the correctness of your store, actions, and reducers. Let's walk through how to integrate TypeScript with Redux.
Step 1: Define Types for State and Actions
Start by defining types for the state and actions in your Redux store. Here's how you can define a simple counter state and actions:
import { Action } from 'redux';
// Define types for state
interface CounterState {
count: number;
}
// Define types for actions
interface IncrementAction extends Action {
type: 'INCREMENT';
}
interface DecrementAction extends Action {
type: 'DECREMENT';
}
// Union type for actions
type CounterAction = IncrementAction | DecrementAction;
Step 2: Create the Reducer
Next, create a reducer that takes the state and actions as arguments and returns the new state:
const initialState: CounterState = {
count: 0,
};
const counterReducer = (state = initialState, action: CounterAction): CounterState => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
Step 3: Set Up Redux Store
Finally, create the Redux store and use the reducer to manage the state:
import { createStore } from 'redux';
const store = createStore(counterReducer);
Step 4: Connecting Redux with React Components
Use useSelector
and useDispatch
hooks to interact with the Redux store in your React components:
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
const Counter: React.FC = () => {
const count = useSelector((state: CounterState) => state.count);
const dispatch = useDispatch();
const increment = () => dispatch({ type: 'INCREMENT' });
const decrement = () => dispatch({ type: 'DECREMENT' });
return (
<div>
Count: {count}
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we use the useSelector
hook to get the state and the useDispatch
hook to dispatch actions to the Redux store. TypeScript ensures that the state and actions conform to the defined types.
Using TypeScript with Context API
The Context API in React provides a way to share state across the component tree without having to pass props down manually at every level. TypeScript can help you type the context value and the provider correctly.
Step 1: Define Types for Context
Define a type for the context value, which will be shared across components:
import React, { createContext, useState, useContext } from 'react';
// Define types for the context value
interface CounterContextType {
count: number;
increment: () => void;
decrement: () => void;
}
// Create context with a default value of undefined
const CounterContext = createContext<CounterContextType | undefined>(undefined);
Step 2: Create a Provider Component
Create a provider component that will use the context and provide the shared state to the component tree:
const CounterProvider: React.FC = ({ children }) => {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<CounterContext.Provider value={{ count, increment, decrement }}>
{children}
</CounterContext.Provider>
);
};
Step 3: Access Context Values in Components
Use the useContext
hook to access the context values in your components:
const Counter: React.FC = () => {
const context = useContext(CounterContext);
if (!context) {
throw new Error('Counter must be used within a CounterProvider');
}
const { count, increment, decrement } = context;
return (
<div>
Count: {count}
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
In this example, we use useContext
to access the context and destructure the state and functions. TypeScript ensures that we only access the values defined in the context type.
Conclusion
By using TypeScript with Redux and the Context API, you can enhance the type safety of your state management logic. Redux provides more advanced state management capabilities, while the Context API is a simpler solution for passing data across components. With TypeScript, you can avoid runtime errors and improve the maintainability of your code by ensuring the correct types are used throughout your application.
Animating Components with React Spring
React Spring is a powerful library for animating React components. It allows you to create fluid, interactive animations that integrate well with React’s declarative style. In this section, we will explore how to animate components using React Spring.
Step 1: Install React Spring
First, you need to install the React Spring library. You can do this by running the following command in your project directory:
npm install react-spring
Step 2: Importing React Spring Hooks
React Spring provides several hooks to create animations. The most commonly used hook is useSpring
, which allows you to animate the properties of a component.
import { useSpring, animated } from 'react-spring';
Step 3: Basic Animation with useSpring
Here’s a simple example of animating the opacity and transform properties of a component:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const FadeIn: React.FC = () => {
const props = useSpring({
opacity: 1,
transform: 'translateY(0px)',
from: { opacity: 0, transform: 'translateY(100px)' },
});
return (
<animated.div style={props}>
<h1>Hello, React Spring!</h1>
</animated.div>
);
};
export default FadeIn;
In this example, the component <h1>
fades in and slides up when it is rendered. The useSpring
hook defines the animation's starting point with from
and the final state. The animated.div
component is a wrapper that applies the animation styles to the component.
Step 4: Multiple Animations and Staggered Animations
You can animate multiple properties at once. Here’s an example of animating both the opacity and color of two elements in a staggered fashion:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const StaggeredAnimations: React.FC = () => {
const props1 = useSpring({
opacity: 1,
color: 'red',
from: { opacity: 0, color: 'blue' },
});
const props2 = useSpring({
opacity: 1,
color: 'green',
from: { opacity: 0, color: 'yellow' },
delay: 500, // delay for second animation
});
return (
<div>
<animated.div style={props1}>First Element</animated.div>
<animated.div style={props2}>Second Element</animated.div>
</div>
);
};
export default StaggeredAnimations;
In this example, two elements are animated with different delay times. The first element fades in and changes color immediately, while the second one starts after a delay of 500ms.
Step 5: Animating with useTrail
If you need to create a sequence of animations for a list of elements, you can use the useTrail
hook. It animates multiple elements in a staggered sequence:
import React from 'react';
import { useTrail, animated } from 'react-spring';
const TrailAnimation: React.FC = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
const trail = useTrail(items.length, {
opacity: 1,
transform: 'translateY(0px)',
from: { opacity: 0, transform: 'translateY(20px)' },
delay: 200,
});
return (
<div>
{items.map((item, index) => (
<animated.div key={index} style={trail[index]}>
{item}
</animated.div>
))}
</div>
);
};
export default TrailAnimation;
Here, the useTrail
hook is used to animate a list of items. Each item will fade in and slide up in sequence, creating a smooth staggered animation.
Step 6: Configuring Easing and Duration
React Spring allows you to configure the easing function and the duration of your animations. This allows you to control the acceleration and deceleration of the animation. Here's an example:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const EasedAnimation: React.FC = () => {
const props = useSpring({
opacity: 1,
transform: 'translateX(0px)',
from: { opacity: 0, transform: 'translateX(-100px)' },
config: { tension: 200, friction: 20 }, // custom configuration
});
return (
<animated.div style={props}>
<h1>Eased Animation Example</h1>
</animated.div>
);
};
export default EasedAnimation;
The config
property allows you to control the tension and friction of the animation, which affects how fast the animation accelerates and decelerates. You can experiment with different values for tension
and friction
to achieve the desired effect.
Conclusion
React Spring is a powerful library that allows you to create smooth, interactive animations in React. By using hooks like useSpring
, useTrail
, and configuring animation properties, you can create complex animations that enhance the user experience of your React application.
CSS Animations in React
CSS animations are a powerful way to add animated effects to your React components. You can use them for smooth transitions, movement, fading effects, and more. In this section, we will explore how to use CSS animations in React components.
Step 1: Adding CSS Animations
First, you need to define your CSS animations in a CSS file. Here’s an example of a simple fade-in animation:
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.fade-in {
animation: fadeIn 2s ease-in-out;
}
In this example, we define a keyframe animation called fadeIn
that animates the opacity
from 0 to 1. The class .fade-in
is used to apply the animation to any element.
Step 2: Applying CSS Animations to React Components
Once you have defined the CSS animation, you can apply it to your React components. Here’s how you can apply the fade-in
animation to a component:
import React from 'react';
import './App.css'; // Import the CSS file
const FadeInComponent: React.FC = () => {
return (
<div className="fade-in">
<h1>Welcome to React Animations!</h1>
</div>
);
};
export default FadeInComponent;
In this example, the fade-in
class is applied to the <div>
element, which triggers the animation when the component is rendered.
Step 3: Using Inline Styles for Animations
If you prefer to use inline styles for your animations, you can define them directly in your React component. Here’s an example:
import React from 'react';
const FadeInInline: React.FC = () => {
const fadeInStyle = {
animation: 'fadeIn 2s ease-in-out',
};
return (
<div style={fadeInStyle}>
<h1>Inline CSS Animation in React</h1>
</div>
);
};
export default FadeInInline;
In this example, the fadeInStyle
object contains the animation properties, and it is applied using the style
attribute in the <div>
element.
Step 4: Using CSS Transitions for Animations
CSS transitions are another way to animate properties in React. They are particularly useful for animating changes in state. Here’s an example of using CSS transitions to animate the background color of a component when it is hovered:
.transition-effect {
transition: background-color 0.5s ease;
}
.transition-effect:hover {
background-color: lightblue;
}
This CSS defines a transition for the background-color
property. When the <div>
element is hovered, its background color will change smoothly over 0.5 seconds.
Step 5: Applying Transitions in React
You can apply the transition effect to your React component like this:
import React, { useState } from 'react';
import './App.css'; // Import the CSS file
const TransitionComponent: React.FC = () => {
const [hovered, setHovered] = useState(false);
return (
<div
className={`transition-effect ${hovered ? 'hovered' : ''}`}
onMouseEnter={() => setHovered(true)}
onMouseLeave={() => setHovered(false)}
>
<h1>Hover to Change Color</h1>
</div>
);
};
export default TransitionComponent;
In this example, the onMouseEnter
and onMouseLeave
events are used to trigger the hover effect. The transition-effect
class is applied, and when the component is hovered, the background-color
will transition smoothly.
Step 6: Keyframe Animation with React and CSS
For more complex animations, you can use keyframes. Here’s an example of animating a component using keyframes for a bouncing effect:
@keyframes bounce {
0% {
transform: translateY(0);
}
50% {
transform: translateY(-20px);
}
100% {
transform: translateY(0);
}
}
.bounce {
animation: bounce 1s infinite;
}
This CSS defines a keyframe animation called bounce
that makes the element move up and down. The bounce
class applies the animation, and it runs indefinitely with a 1-second duration for each cycle.
Step 7: Using Keyframes in React
Now you can apply the bounce animation to a React component:
import React from 'react';
import './App.css'; // Import the CSS file
const BounceComponent: React.FC = () => {
return (
<div className="bounce">
<h1>I am bouncing!</h1>
</div>
);
};
export default BounceComponent;
The bounce
class is applied to the <div>
element, which triggers the bouncing animation when the component is rendered.
Conclusion
CSS animations are an excellent way to enhance the visual appeal of your React applications. By combining CSS animations, transitions, and keyframes with React’s declarative style, you can create engaging and interactive user interfaces.
Introduction to Framer Motion for React Animations
Framer Motion is a popular library for creating animations in React applications. It provides a simple, declarative API to create complex animations with minimal setup. In this section, we will introduce Framer Motion and demonstrate how to integrate it into your React projects.
Step 1: Installing Framer Motion
To start using Framer Motion, you need to install it in your React project. You can add it using npm or yarn:
npm install framer-motion
Or with yarn:
yarn add framer-motion
Step 2: Basic Animation with Framer Motion
Framer Motion makes it easy to animate elements in your React app. Here’s an example of a simple animation using the motion.div
component. This animates the opacity and position of a <div>
element:
import { motion } from 'framer-motion';
const AnimatedComponent: React.FC = () => {
return (
<motion.div
initial={{ opacity: 0, x: -100 }}
animate={{ opacity: 1, x: 0 }}
transition={{ duration: 1 }}
>
<h1>Hello, Framer Motion!</h1>
</motion.div>
);
};
export default AnimatedComponent;
In this example, we use the motion.div
component to animate the <div>
element. The initial
prop defines the starting state (opacity 0 and x position -100), while the animate
prop specifies the final state (opacity 1 and x position 0). The transition
prop sets the duration of the animation to 1 second.
Step 3: Animating on Hover
Framer Motion allows you to trigger animations based on user interactions, such as hover. Here’s how you can animate an element when the user hovers over it:
import { motion } from 'framer-motion';
const HoverEffect: React.FC = () => {
return (
<motion.div
whileHover={{ scale: 1.2 }}
transition={{ type: 'spring', stiffness: 300 }}
>
<h1>Hover over me!</h1>
</motion.div>
);
};
export default HoverEffect;
In this example, the element scales up by 20% when hovered, using the whileHover
prop. The transition
prop adds a spring effect with stiffness set to 300 for a smooth animation.
Step 4: Animating on Scroll
Framer Motion also supports scroll-based animations. Here’s an example of an animation that triggers when the element comes into view:
import { motion } from 'framer-motion';
const ScrollAnimation: React.FC = () => {
return (
<motion.div
initial={{ opacity: 0 }}
whileInView={{ opacity: 1 }}
transition={{ duration: 1 }}
viewport={{ once: true }}
>
<h1>Scroll to reveal me!</h1>
</motion.div>
);
};
export default ScrollAnimation;
In this example, the motion.div
element starts with an opacity of 0, and when it scrolls into view, it animates to opacity 1. The whileInView
prop triggers the animation when the element is in the viewport, and the transition
prop sets the duration to 1 second. The viewport
prop with once: true
ensures the animation runs only once when the element first enters the viewport.
Step 5: Keyframe Animations with Framer Motion
Framer Motion also supports keyframe animations, which allow you to create more complex animations. Here’s an example of animating the position of an element along a path:
import { motion } from 'framer-motion';
const KeyframeAnimation: React.FC = () => {
return (
<motion.div
animate={{
x: [0, 100, 0],
y: [0, -100, 0],
}}
transition={{
duration: 2,
repeat: Infinity,
repeatType: 'loop',
}}
>
<h1>Keyframe Animation!</h1>
</motion.div>
);
};
export default KeyframeAnimation;
In this example, the element moves along a path defined by the keyframes for both x
and y
axes. The animation repeats indefinitely with the repeat: Infinity
setting, and the transition duration is set to 2 seconds.
Step 6: Orchestrating Multiple Animations
Framer Motion makes it easy to orchestrate multiple animations to run together or in sequence. Here’s an example of running multiple animations in parallel:
import { motion } from 'framer-motion';
const ParallelAnimations: React.FC = () => {
return (
<motion.div
initial={{ opacity: 0, x: -100 }}
animate={{ opacity: 1, x: 0 }}
transition={{ duration: 1 }}
>
<motion.div
initial={{ scale: 0 }}
animate={{ scale: 1 }}
transition={{ delay: 0.5, duration: 1 }}
>
<h1>Parallel Animations</h1>
</motion.div>
</motion.div>
);
};
export default ParallelAnimations;
In this example, two animations run in parallel: one animates the opacity and position, while the other animates the scale of the inner <div>
. The delay
prop on the second animation makes sure it starts slightly after the first animation.
Conclusion
Framer Motion is a powerful and flexible library for creating animations in React. Its simple API and support for complex animations like keyframes, scroll-based triggers, and parallel animations make it a great choice for adding rich, interactive animations to your React applications.
Creating Complex Animations with React Transition Group
React Transition Group is a powerful library for handling animations and transitions in React. It provides simple components for transitioning elements in and out of the DOM. In this section, we will explore how to create complex animations using React Transition Group.
Step 1: Installing React Transition Group
To begin, you need to install React Transition Group in your project. You can install it using npm or yarn:
npm install react-transition-group
Or with yarn:
yarn add react-transition-group
Step 2: Basic Transition with CSSTransition
The CSSTransition
component is used to animate elements with CSS classes. Here's an example of a simple fade-in animation:
import { CSSTransition } from 'react-transition-group';
import { useState } from 'react';
const FadeInComponent: React.FC = () => {
const [show, setShow] = useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Fade</button>
<CSSTransition
in={show}
timeout={300}
classNames="fade"
unmountOnExit
>
<div className="fade-box">Fading In and Out!</div>
</CSSTransition>
</div>
);
};
export default FadeInComponent;
In this example, the CSSTransition
component wraps the <div>
element that we want to animate. The in
prop controls whether the element should be visible, timeout
sets the duration of the animation, and classNames
specifies the base class for the animation. The unmountOnExit
prop ensures that the element is removed from the DOM when the animation completes.
Step 3: CSS for Transitions
To style the transitions, you need to define CSS classes that will be applied at different stages of the transition. Here’s an example of the necessary CSS for the fade-in animation:
.fade-enter {
opacity: 0;
}
.fade-enter-active {
opacity: 1;
transition: opacity 300ms ease-in;
}
.fade-exit {
opacity: 1;
}
.fade-exit-active {
opacity: 0;
transition: opacity 300ms ease-in;
}
The fade-enter
and fade-enter-active
classes define the starting and ending states for the element when it is entering the DOM. Similarly, the fade-exit
and fade-exit-active
classes define the transition for when the element is exiting the DOM.
Step 4: Animating Lists with TransitionGroup
React Transition Group also provides the TransitionGroup
component, which allows you to animate lists or groups of elements. This is useful for adding animations when items are added or removed from a list:
import { TransitionGroup, CSSTransition } from 'react-transition-group';
import { useState } from 'react';
const ListComponent: React.FC = () => {
const [items, setItems] = useState([]);
const addItem = () => {
setItems([...items, `Item ${items.length + 1}`]);
};
const removeItem = (index: number) => {
setItems(items.filter((_, i) => i !== index));
};
return (
<div>
<button onClick={addItem}>Add Item</button>
<TransitionGroup component="ul">
{items.map((item, index) => (
<CSSTransition key={index} timeout={500} classNames="item">
<li>
{item}
<button onClick={() => removeItem(index)}>Remove</button>
</li>
</CSSTransition>
))}
</TransitionGroup>
</div>
);
};
export default ListComponent;
In this example, we use TransitionGroup
to animate the list of items. When an item is added or removed, the CSSTransition
component animates its appearance or disappearance. Each item is given a unique key
to ensure that React can correctly manage the transition of individual elements.
Step 5: CSS for List Transitions
Here's the CSS required to animate the list items:
.item-enter {
opacity: 0;
}
.item-enter-active {
opacity: 1;
transition: opacity 500ms ease-in;
}
.item-exit {
opacity: 1;
}
.item-exit-active {
opacity: 0;
transition: opacity 500ms ease-in;
}
The CSS classes follow the same pattern as the fade-in example but are specific to the list items. The item-enter
and item-enter-active
classes handle the animation when an item is added, and the item-exit
and item-exit-active
classes handle the animation when an item is removed.
Step 6: Advanced Animations with CSSTransition
and State
You can use CSSTransition
with complex states to create more advanced animations. For example, animating the size of an element based on state changes:
import { CSSTransition } from 'react-transition-group';
import { useState } from 'react';
const SizeTransitionComponent: React.FC = () => {
const [isLarge, setIsLarge] = useState(false);
return (
<div>
<button onClick={() => setIsLarge(!isLarge)}>Toggle Size</button>
<CSSTransition
in={isLarge}
timeout={300}
classNames="size"
unmountOnExit
>
<div className="size-box">Size Transition!</div>
</CSSTransition>
</div>
);
};
export default SizeTransitionComponent;
In this example, the size of the element changes based on the isLarge
state. The animation is triggered when the state changes, and the classNames
prop ensures that the correct CSS classes are applied for the transition.
Step 7: CSS for Size Transitions
Here’s the CSS to animate the size change:
.size-enter {
transform: scale(0.5);
}
.size-enter-active {
transform: scale(1);
transition: transform 300ms ease-in;
}
.size-exit {
transform: scale(1);
}
.size-exit-active {
transform: scale(0.5);
transition: transform 300ms ease-in;
}
The size-enter
and size-enter-active
classes define the starting and ending size of the element, while the size-exit
and size-exit-active
classes define the transition when the element is removed.
Conclusion
React Transition Group provides an easy way to add animations and transitions to your React components. By using CSSTransition
and TransitionGroup
, you can create complex animations for elements entering and exiting the DOM. With the flexibility to define custom CSS transitions, React Transition Group allows you to create rich and interactive animations in your applications.
What is SSR?
Server-Side Rendering (SSR) is a technique used in web development where HTML pages are rendered on the server rather than in the browser. This allows for faster initial page load times and better SEO (Search Engine Optimization) because the content is delivered as fully rendered HTML, which search engine crawlers can index more easily.
How Does SSR Work?
In a traditional client-side rendered (CSR) application, the browser makes a request to the server, receives a blank HTML document, and then loads JavaScript to dynamically generate the content. In SSR, the server generates the HTML content for each page and sends it to the browser, which improves the user experience by reducing the time it takes for the page to be visible.
Benefits of SSR
- Faster Initial Load: Since the HTML is already pre-rendered on the server, the page can be displayed more quickly in the browser.
- Better SEO: Search engines can easily index server-rendered content, which can improve search rankings.
- Improved Performance: SSR can offload the rendering work from the client to the server, making it easier to handle complex pages and large data sets.
- Better for Social Media Sharing: Pre-rendered content ensures that social media platforms can accurately display links with rich preview data.
Challenges of SSR
- Increased Server Load: Server-side rendering requires the server to render the page for every request, which can put additional load on the server.
- Complex Setup: Setting up SSR can be more complex than traditional CSR and may require additional tools and frameworks.
- Slower Interactivity: After the initial HTML is loaded, the JavaScript must still be loaded to enable full interactivity, which can delay user interaction.
How is SSR Implemented?
To implement SSR, frameworks like Next.js
and Nuxt.js
are commonly used for React and Vue.js, respectively. These frameworks handle server-side rendering and provide features that improve the development experience, such as automatic code splitting, routing, and optimization for SEO.
Example of SSR with React
Here’s a simple example of how SSR can be implemented in a React application using Express.js as the server:
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import express from 'express';
import App from './App';
const server = express();
server.get('*', (req, res) => {
const content = ReactDOMServer.renderToString(<App />);
res.send(`
<html>
<head><title>SSR Example</title></head>
<body>
<div id="root">${content}</div>
<script src="/main.js"></script>
</body>
</html>
`);
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, the ReactDOMServer.renderToString()
method is used to render the App
component to a string of HTML, which is then injected into the server response. This allows the browser to receive a fully rendered page on the initial request.
Conclusion
Server-Side Rendering (SSR) can significantly improve the performance and SEO of a web application by pre-rendering the HTML on the server. While it comes with some challenges, frameworks like Next.js make implementing SSR easier, and the benefits it brings to page load speed and search engine visibility can be well worth the effort.
Benefits of SSR for SEO and Performance
Server-Side Rendering (SSR) provides several advantages when it comes to improving SEO and performance for web applications. By pre-rendering HTML content on the server and delivering a fully rendered page to the client, SSR can enhance the user experience and make it easier for search engines to index content.
Benefits for SEO
- Improved Crawlability: Search engines like Google can index SSR content more effectively since the HTML is pre-rendered and available when the page is loaded. This ensures that all the content is crawlable by search engine bots, even if the site relies heavily on JavaScript.
- Faster Indexing: SSR allows search engines to quickly parse the HTML content, making it easier for them to index the page and update search results more quickly. This is particularly important for dynamic content that changes frequently.
- Better Metadata Visibility: Since SSR pages include metadata (such as titles, descriptions, and social media tags) in the initial HTML, search engines can better understand the content of your page and use this information to generate rich snippets and improve visibility in search results.
- Enhanced Social Media Sharing: SSR ensures that social media platforms like Facebook and Twitter can accurately retrieve metadata and content from your page, leading to better previews and richer link sharing.
Benefits for Performance
- Faster Initial Page Load: Since the HTML is pre-rendered on the server, the browser can display the content immediately without waiting for JavaScript to load and execute. This results in a faster perceived loading time for users.
- Reduced Time to First Byte (TTFB): SSR reduces the time it takes for the browser to receive the initial HTML response, as the server already processes the page content. This results in a lower TTFB, contributing to faster page loads.
- Improved User Experience (UX): With SSR, users see the content more quickly, even if the JavaScript bundle is large or takes time to load. This helps provide a smoother user experience, especially on slower networks or devices.
- Better Performance on Low-Powered Devices: SSR can offload rendering from the client's device to the server, which is especially useful for users with low-powered devices or slow internet connections that may struggle to render complex client-side applications.
Real-World Example of SSR Benefits
Consider an e-commerce website that uses SSR. When the product pages are pre-rendered on the server, search engines can immediately index the product listings, descriptions, and prices. As a result, these pages are more likely to appear in relevant search queries, improving SEO rankings. Additionally, users can see the products and information faster, leading to a better overall shopping experience.
Example of SSR for SEO
In a typical React SSR setup, the rendered HTML content is sent to the browser with all necessary metadata. This ensures that search engines can extract the title and description for better search results. Here’s an example of what the server-side code might look like:
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import express from 'express';
import App from './App';
const server = express();
server.get('*', (req, res) => {
const content = ReactDOMServer.renderToString(<App />);
res.send(`
<html>
<head>
<title>E-Commerce Store</title>
<meta name="description" content="Shop the best products at great prices!" />
</head>
<body>
<div id="root">${content}</div>
<script src="/main.js"></script>
</body>
</html>
`);
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, the metadata for the page (such as title and description) is included in the initial HTML, making it easily accessible to search engines for better SEO performance.
Conclusion
Server-Side Rendering (SSR) provides significant benefits for both SEO and performance. By delivering fully rendered HTML from the server, SSR improves crawlability, indexing speed, and metadata visibility for SEO, while also enhancing the user experience through faster initial page loads and better performance on low-powered devices. Implementing SSR can be a valuable strategy for web developers looking to optimize their websites for both search engines and end users.
Setting Up Server-Side Rendering with React and Express
Server-Side Rendering (SSR) is a technique where the HTML is rendered on the server and sent to the browser instead of relying on the client-side JavaScript to render the content. In this section, we will guide you through setting up SSR with React and Express.
Step 1: Create a New React Project
Start by creating a new React project. If you don’t have one already, you can use Create React App:
npx create-react-app ssr-app
Navigate to the project directory:
cd ssr-app
Step 2: Install Required Dependencies
For SSR, we need a few additional dependencies. Install Express and ReactDOMServer:
npm install express react-dom
Step 3: Set Up the Express Server
Create a new file named server.js
in the root of your project. This file will act as the server for handling SSR.
const express = require('express');
const React = require('react');
const ReactDOMServer = require('react-dom/server');
const App = require('./src/App');
const server = express();
server.use('^/$', (req, res, next) => {
res.send(`
<html>
<head>
<title>Server-Side Rendering with React</title>
</head>
<body>
<div id="root">${ReactDOMServer.renderToString(<App />)}</div>
<script src="main.js"></script>
</body>
</html>
`);
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this setup, when the client accesses the root URL, we render the App
component to HTML using ReactDOMServer.renderToString
and send it as the response. We also include the main.js
script that will run on the client side once the page is loaded.
Step 4: Update the React App to Be SSR-Compatible
In your React app, ensure that there are no direct DOM manipulations. React components should be renderable on both the client and the server. A simple App.js
file might look like this:
import React from 'react';
const App = () => {
return (
<div>
<h1>Hello, Server-Side Rendering with React!</h1>
</div>
);
};
export default App;
Step 5: Bundle Your React Application
To bundle your React application for the server, you’ll need to update the build process. One way to do this is by using Webpack to compile and bundle both server-side and client-side code. You may need to set up a Webpack configuration to support this, but the Create React App setup does not include Webpack configurations out of the box for SSR. In such a case, you can either eject the app or use a custom setup for SSR.
Step 6: Serve the Client-Side JavaScript
Make sure the client-side JavaScript bundle is served on the server by adding the following to your Express server setup:
server.use(express.static('build'));
server.get('*', (req, res) => {
res.sendFile(path.resolve('build', 'index.html'));
});
Step 7: Run the Server
Once everything is set up, you can run the server with the following command:
node server.js
Your server will now render the React app on the server and serve it with all the assets. The client will then take over and hydrate the app on the browser.
Conclusion
By following these steps, you have set up Server-Side Rendering (SSR) with React and Express. SSR improves performance by rendering the HTML on the server and sending it to the client, which can result in faster initial page loads and better SEO. With Express handling the server-side rendering logic and React providing the components, you’ve successfully set up a basic SSR application.
Introduction to Next.js for SSR
Next.js is a popular React framework that makes it easy to implement Server-Side Rendering (SSR) with minimal configuration. It provides built-in support for SSR, static site generation, routing, and much more. In this section, we will explore how Next.js simplifies the process of setting up SSR with React.
What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) is the process of rendering your React components on the server, rather than in the browser. This allows the HTML to be generated on the server and sent to the client, improving performance and SEO. Next.js provides a built-in method to enable SSR for your React applications.
Why Use Next.js for SSR?
Next.js offers several advantages for SSR, including:
- Automatic SSR: Next.js automatically handles SSR for pages that need it, simplifying the setup process.
- File-based Routing: Next.js uses a file-based routing system, where each file in the
pages
directory automatically maps to a route. - Optimized Performance: Next.js automatically optimizes your application for better performance, including code splitting and preloading critical resources.
- API Routes: You can build API endpoints directly within your Next.js app using API routes.
Step 1: Setting Up a Next.js Project
To get started with Next.js, you'll need to create a new Next.js application. You can use the following command to create a new Next.js project:
npx create-next-app ssr-nextjs-app
Once the project is created, navigate to the project directory:
cd ssr-nextjs-app
Step 2: Enabling SSR in Next.js
Next.js automatically enables SSR for React components within the pages
directory. To create a page that uses SSR, simply create a new file in the pages
folder. For example, create a file called index.js
in the pages
directory:
import React from 'react';
const HomePage = () => {
return (
<div>
<h1>Welcome to SSR with Next.js!</h1>
</div>
);
};
export default HomePage;
Next.js will automatically render this page on the server and send it to the client as HTML. When you navigate to http://localhost:3000
, the page will be pre-rendered on the server.
Step 3: Fetching Data with getServerSideProps
Next.js allows you to fetch data on the server before rendering the page using the getServerSideProps
function. This function runs on the server during each request and fetches the necessary data before rendering the page.
export async function getServerSideProps() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return {
props: { data }
};
}
const HomePage = ({ data }) => {
return (
<div>
<h1>Fetched Data: {data.title}</h1>
</div>
);
};
export default HomePage;
In this example, getServerSideProps
fetches data from an API and passes it as props to the component. The page will be pre-rendered on the server with the fetched data.
Step 4: Running the Next.js App
To run your Next.js app, use the following command:
npm run dev
The app will be available at http://localhost:3000
. When you visit this URL, you'll see the page rendered on the server and sent to the client.
Step 5: Static Site Generation (SSG) in Next.js
In addition to SSR, Next.js also supports Static Site Generation (SSG) using the getStaticProps
function. This allows you to generate static pages at build time, which can further improve performance.
export async function getStaticProps() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return {
props: { data }
};
}
When you use getStaticProps
, Next.js will pre-render the page at build time, making it extremely fast when served to the client. To build your app with SSG, run:
npm run build
npm run start
Conclusion
Next.js simplifies the process of implementing Server-Side Rendering (SSR) with React. With minimal configuration, you can take advantage of SSR and Static Site Generation (SSG) to improve performance and SEO. By using features like getServerSideProps
and getStaticProps
, you can fetch data on the server and deliver pre-rendered pages to the client quickly and efficiently.
Static Site Generation (SSG) with Next.js
Static Site Generation (SSG) is a method of pre-rendering pages at build time in Next.js. This allows your site to be served as static files, making it faster and more SEO-friendly. In this section, we will explore how to implement SSG with Next.js and take advantage of its powerful features for better performance.
What is Static Site Generation (SSG)?
Static Site Generation (SSG) is a technique where HTML pages are generated at build time rather than at runtime. The pages are pre-rendered with the data they need, and the static files are served to the client when requested. This results in faster load times and better SEO because the content is available immediately.
Why Use SSG in Next.js?
Next.js provides built-in support for Static Site Generation (SSG), and it offers several benefits:
- Faster Load Times: Since pages are pre-rendered, they are served as static HTML files, which load quickly.
- Better SEO: Search engines can easily crawl pre-rendered HTML, improving the visibility of your content.
- Automatic Static Optimization: Next.js automatically optimizes pages that do not require server-side rendering, serving them as static files.
Step 1: Setting Up SSG in Next.js
In Next.js, you can enable Static Site Generation (SSG) by using the getStaticProps
function. This function is run at build time and allows you to fetch data and pass it as props to your component. Here's how you can set it up:
import React from 'react';
export async function getStaticProps() {
// Fetch data at build time
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return {
props: { data }, // Pass the fetched data to the component as props
};
}
const HomePage = ({ data }) => {
return (
<div>
<h1>Fetched Data: {data.title}</h1>
</div>
);
};
export default HomePage;
In this example, getStaticProps
fetches data from an API during build time and passes it as props to the HomePage
component. The page is pre-rendered and served as static HTML with the fetched data.
Step 2: Running the Next.js App with SSG
After setting up getStaticProps
, you can build your Next.js application and start the server using the following commands:
npm run build
npm run start
Next.js will generate the static HTML files during the build process. These files will be served when you access the application, ensuring fast load times and improved SEO.
Step 3: Dynamic Routes with SSG
You can also use SSG with dynamic routes in Next.js. To do this, you can use the getStaticPaths
function along with getStaticProps
to pre-render dynamic pages at build time. Here's an example:
export async function getStaticPaths() {
const response = await fetch('https://api.example.com/posts');
const posts = await response.json();
const paths = posts.map(post => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false, // Return 404 for any paths not generated at build time
};
}
export async function getStaticProps({ params }) {
const response = await fetch(`https://api.example.com/posts/${params.id}`);
const post = await response.json();
return {
props: { post },
};
}
const PostPage = ({ post }) => {
return (
<div>
<h1>{post.title}</h1>
<p>{post.body}</p>
</div>
);
};
export default PostPage;
In this example, getStaticPaths
fetches a list of posts and generates dynamic paths for each post. The getStaticProps
function fetches data for each post at build time and passes it to the page component.
Step 4: Incremental Static Regeneration (ISR)
Next.js also supports Incremental Static Regeneration (ISR), which allows you to update static pages after build time without rebuilding the entire site. This is useful for pages that need to be updated frequently but do not require full SSR. You can use the revalidate
option in getStaticProps
to specify how often the page should be regenerated.
export async function getStaticProps() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return {
props: { data },
revalidate: 60, // Regenerate the page every 60 seconds
};
}
Conclusion
Static Site Generation (SSG) with Next.js provides a powerful way to pre-render pages at build time, resulting in faster load times and improved SEO. By using getStaticProps
and getStaticPaths
, you can create static pages with dynamic content. Additionally, Next.js offers Incremental Static Regeneration (ISR) for updating static pages without rebuilding the entire site.
What is a PWA?
A Progressive Web App (PWA) is a type of application built using web technologies such as HTML, CSS, and JavaScript that behaves like a native mobile app. PWAs aim to provide users with an app-like experience while being accessible through the web. They combine the best features of web and mobile apps, offering high performance, offline capabilities, and the ability to be installed on a user's device.
Key Features of PWAs
- Responsive: PWAs are responsive and adapt to different screen sizes and devices, ensuring a seamless experience across desktops, tablets, and smartphones.
- Offline Support: PWAs can work offline or in low-network conditions by caching assets and data, enabling users to continue interacting with the app even when they lose internet connectivity.
- App-Like Experience: PWAs provide a native-like experience with smooth animations, fast load times, and the ability to be added to the home screen of a device, just like a native app.
- Installable: PWAs can be installed on a user's device and launched from the home screen, without needing to go through an app store.
- Push Notifications: PWAs can send push notifications to users, keeping them engaged even when the app is not open.
- Secure: PWAs are served over HTTPS, ensuring that all data exchanged between the client and server is encrypted.
How Do PWAs Work?
PWAs rely on several key web technologies to function effectively:
- Service Workers: Service workers are scripts that run in the background and manage caching, network requests, and push notifications. They enable PWAs to function offline by caching important assets and data.
- Web App Manifest: The web app manifest is a JSON file that defines how the app should appear when installed on a device. It includes details such as the app's name, icon, theme color, and start URL.
- HTTPS: PWAs require HTTPS to ensure secure communication between the client and server, protecting user data and preventing man-in-the-middle attacks.
Example of a Basic PWA Setup
Here’s an example of the basic setup for a Progressive Web App:
{
"name": "My PWA",
"short_name": "PWA",
"description": "A simple Progressive Web App",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
This is the manifest.json
file, which defines the web app’s properties like name, icon, theme color, and start URL. This file is linked to the HTML file as follows:
<link rel="manifest" href="/manifest.json">
Service Worker Example
To implement offline capabilities, a service worker script is used. Here’s a basic example of a service worker that caches assets:
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open('my-cache').then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/app.js',
]);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((cachedResponse) => {
return cachedResponse || fetch(event.request);
})
);
});
How to Make Your Website a PWA?
To convert your website into a PWA, you need to implement the following steps:
- Create a Web App Manifest: Include a
manifest.json
file with the necessary properties and link it in your HTML file. - Implement a Service Worker: Write a service worker script to manage caching and offline functionality, and register it in your app.
- Make Your Site HTTPS-Only: PWAs require HTTPS for security, so ensure that your site is served over HTTPS.
- Test and Deploy: Test your PWA using tools like Lighthouse and deploy it to a server.
Benefits of PWAs
PWAs offer several benefits over traditional web apps and native mobile apps:
- Improved Performance: PWAs load faster, even on slow networks, due to caching and background data fetching.
- Cross-Platform Compatibility: PWAs work across all platforms, including desktops, tablets, and smartphones, without the need for separate codebases.
- Lower Development and Maintenance Costs: PWAs eliminate the need to maintain separate versions of an app for iOS, Android, and the web.
- Better User Engagement: With push notifications and installability, PWAs can keep users engaged and drive more interactions.
Conclusion
Progressive Web Apps (PWAs) are an excellent way to deliver app-like experiences using web technologies. They offer several advantages, including offline support, fast load times, and cross-platform compatibility. By leveraging service workers, web app manifests, and HTTPS, you can easily create a PWA that provides users with a reliable and engaging experience.
Benefits of PWAs for Offline Access and Mobile Users
Progressive Web Apps (PWAs) offer numerous advantages for offline access and mobile users. By leveraging modern web technologies, PWAs provide an experience similar to native mobile apps while retaining the accessibility and flexibility of the web. In this section, we will explore the key benefits of PWAs for offline access and mobile users.
1. Offline Access
One of the standout features of PWAs is the ability to function offline or in low-network conditions. This is achieved through the use of service workers, which enable the app to cache assets and data so that users can continue interacting with the app even when they lose internet connectivity.
When a user visits a PWA, the app's service worker caches the necessary files (e.g., HTML, CSS, JavaScript) and data. If the user loses their internet connection, the app can still load from the cache, providing a seamless experience.
Example of Service Worker Caching for Offline Access:
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open('my-cache').then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/app.js',
]);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((cachedResponse) => {
return cachedResponse || fetch(event.request);
})
);
});
2. Improved Performance for Mobile Users
PWAs offer superior performance, especially for mobile users. Since PWAs are designed to be lightweight, they load quickly even on slower networks, providing a smooth experience on mobile devices. By caching assets and data, PWAs reduce the need for repeated network requests, which leads to faster loading times, lower data usage, and better battery life.
In comparison to traditional websites, PWAs often load faster due to their ability to cache files and access them locally. This is particularly important for mobile users who may have limited or unreliable network connectivity.
Advantages of PWAs for Mobile Users:
- Faster Load Times: PWAs load quickly, even on slower mobile networks, because they cache assets and data locally.
- Reduced Data Usage: PWAs reduce the need to download the same resources repeatedly, minimizing data usage, which is especially beneficial for users with limited data plans.
- Better Battery Efficiency: PWAs consume less power than native apps by avoiding frequent network calls and relying on cached data.
3. Installation and Home Screen Access
PWAs can be installed on a mobile device's home screen, just like native apps. Once installed, the PWA can be launched directly from the home screen without the need to open a browser. This provides mobile users with an app-like experience without the need to download the app from an app store.
PWAs are accessible directly through the web, meaning users don’t have to go through the process of searching for and installing an app from the app store. This makes PWAs more convenient for mobile users who prefer a quicker and simpler process.
Example of a Basic Web App Manifest for Installation:
{
"name": "My PWA",
"short_name": "PWA",
"description": "A simple Progressive Web App",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
4. Push Notifications for Engagement
PWAs can also send push notifications, which help to keep users engaged even when the app is not open. This is a powerful feature for mobile users, as it allows businesses to send timely updates, reminders, and promotions directly to the user's device.
Push notifications are particularly useful for mobile users, as they are more likely to have their devices with them at all times. PWAs can send notifications for various events, such as new content, chat messages, or important alerts.
Example of Sending a Push Notification from a Service Worker:
self.addEventListener('push', (event) => {
const options = {
body: event.data.text(),
icon: '/icons/icon-192x192.png',
badge: '/icons/badge-192x192.png'
};
event.waitUntil(
self.registration.showNotification('New Update', options)
);
});
5. Seamless Updates and User Experience
With PWAs, updates can be pushed directly to users without requiring them to download updates from an app store. This ensures that users always have the latest version of the app without the need for manual intervention. The update process is seamless and happens in the background, so users don't even notice it.
Benefits of Seamless Updates:
- Automatic Updates: PWAs can automatically fetch and apply updates without interrupting the user experience.
- No App Store Approval Required: Developers can push updates directly to the app without waiting for approval from app stores.
- Reduced Friction: Users don’t have to manually update the app, which reduces friction and ensures a consistent experience.
6. Cost-Effective for Mobile Users
Developing a PWA is more cost-effective than building separate native apps for iOS and Android. With a PWA, developers only need to create a single codebase that works across all platforms, which results in lower development and maintenance costs.
For mobile users, this means that businesses can provide a consistent experience across different devices and platforms, without the need to download multiple apps or worry about compatibility issues.
Conclusion
Progressive Web Apps (PWAs) offer significant benefits for offline access and mobile users. By leveraging service workers, caching, and push notifications, PWAs provide a fast, reliable, and engaging experience for users, regardless of their network conditions. The ability to install the app on mobile devices and receive updates seamlessly makes PWAs an attractive option for businesses looking to improve user engagement and retention.
Converting a React App to a PWA
Converting an existing React app to a Progressive Web App (PWA) is a straightforward process that can significantly enhance the user experience by providing offline access, push notifications, and faster load times. In this section, we will guide you through the steps to convert your React app into a PWA.
Step 1: Create a React App (If you don't have one)
If you haven’t already created a React app, you can easily set one up using Create React App:
npx create-react-app my-app
Navigate to your app directory:
cd my-app
Step 2: Install Dependencies for Service Workers
To enable PWA features like offline access, you need to set up a service worker in your app. Create React App provides a built-in service worker setup that you can enable. First, make sure your app is using the latest version of Create React App:
npm install --save react-scripts@latest
Step 3: Enable Service Worker in your React App
Open the src/index.js
file in your React app and modify it to register the service worker. By default, Create React App generates a service worker in the public
folder, but it is not registered by default. To enable it, you need to change serviceWorker.unregister()
to serviceWorker.register()
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// Register the service worker
serviceWorker.register();
This will allow your app to cache assets and provide offline functionality. The service worker will manage the caching of resources so that the app can be used even without an internet connection.
Step 4: Add a Web App Manifest
A web app manifest is a JSON file that defines how your app will appear when installed on a user's device. Create a file called manifest.json
in the public
directory of your React app (if it doesn’t already exist). Here's an example manifest:
{
"name": "My PWA",
"short_name": "PWA",
"description": "A simple Progressive Web App",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
The manifest.json
file allows users to add your app to their home screen and provides metadata such as the app name, icons, and theme colors.
Step 5: Add Icons for Your PWA
To make your app look more like a native app when installed on users' devices, add icons for different screen sizes. Place the icon images in the public/icons
directory. For example, you can create icons of various sizes such as 192x192px and 512x512px.
Make sure to reference these icons in the manifest.json
file as shown in the previous step.
Step 6: Test Your PWA
Once you have made the changes, you can test your app locally. To do this, run the app in production mode:
npm run build
This will create a build
folder with a production version of your app. You can then serve the app locally using a static server such as serve
:
npm install -g serve
serve -s build
After serving the app, open it in your browser and check if it works offline and displays the PWA installation prompt. You can also use Chrome DevTools to test the PWA features:
- Open Chrome DevTools, go to the "Application" tab, and look for the "Service Workers" section to check if the service worker is registered.
- Check the "Manifest" section to see if the web app manifest is correctly loaded.
- Test offline functionality by disabling the network in the "Network" tab of DevTools and reloading the page.
Step 7: Deploy Your PWA
Once you're satisfied with the PWA setup, you can deploy your app to a hosting service. Popular choices for deploying PWAs include:
- Netlify
- Vercel
- GitHub Pages
- Firebase Hosting
Simply deploy your production build to one of these services, and your React app will be a fully functional PWA!
Conclusion
Converting a React app to a Progressive Web App is a simple process that provides numerous benefits, including offline access, app-like experiences, and the ability to install the app on mobile devices. By following the steps above, you can quickly transform your React app into a PWA and provide a better experience for users.
Service Workers and Caching in React
Service workers are a powerful feature of Progressive Web Apps (PWAs) that allow you to manage caching, handle background tasks, and enable offline capabilities. In this section, we will explore how to use service workers for caching in your React app and improve performance.
What is a Service Worker?
A service worker is a JavaScript file that runs in the background, separate from the main browser thread. It can intercept network requests, cache responses, and enable features like offline access and push notifications. Service workers are essential for creating a Progressive Web App (PWA).
Step 1: Setting Up the Service Worker
Create React App comes with built-in service worker support, but it is not enabled by default. To set up service workers in your React app, follow these steps:
First, ensure that your app is created using Create React App:
npx create-react-app my-app
Navigate to your project folder:
cd my-app
Step 2: Enable the Service Worker
In the src/index.js
file, locate the following line:
serviceWorker.unregister();
Change it to:
serviceWorker.register();
By calling serviceWorker.register()
, the service worker is registered and can begin managing network requests and caching resources.
Step 3: Understanding the Caching Strategy
Once the service worker is registered, it can use different caching strategies to improve performance. A common approach is to cache the assets of the app so that it can be accessed offline. The service worker can be set up to cache static assets like HTML, CSS, JavaScript files, images, and other resources.
The default service worker provided by Create React App includes a basic caching strategy, which caches assets on the first visit and serves them from the cache on subsequent visits. You can find this logic inside the src/serviceWorker.js
file.
Step 4: Customizing the Caching Logic
If you want to customize the caching strategy, you can modify the service worker code. For example, you can cache API responses or implement a more advanced caching strategy like "stale-while-revalidate" or "cache-first". Here's an example of a custom caching strategy in the service worker:
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((cachedResponse) => {
// Return cached response if available, else fetch from the network
return cachedResponse || fetch(event.request).then((response) => {
return caches.open('my-cache').then((cache) => {
cache.put(event.request, response.clone());
return response;
});
});
})
);
});
This custom strategy checks if the requested resource is in the cache. If it is, the cached version is returned. If not, the request is made to the network, and the response is cached for future use.
Step 5: Testing the Service Worker
Once the service worker is set up and customized, you can test its functionality:
- Build your app for production:
npm run build
npm install -g serve
serve -s build
Step 6: Handling Updates to the Service Worker
When you make changes to your service worker or your app, you may need to update the service worker to reflect those changes. The service worker will automatically update when the client reloads, but you can also manually trigger updates using the following code:
navigator.serviceWorker.ready.then((registration) => {
registration.update();
});
This will force the service worker to check for any updates and apply them if necessary.
Step 7: Considerations for Advanced Caching
For more advanced caching strategies, consider using libraries like Workbox. Workbox simplifies the process of managing caching strategies, background sync, and other service worker features. You can integrate Workbox into your Create React App project to further optimize caching and performance.
Conclusion
Service workers are a powerful tool for improving performance and enabling offline access in React apps. By enabling the service worker and customizing caching strategies, you can ensure that your app works seamlessly even when the user is offline. With the right caching strategy in place, you can provide a faster, more reliable experience for your users.
Using create-react-app for PWA Setup
Create React App (CRA) provides a simple way to set up a Progressive Web App (PWA). By default, CRA includes basic support for service workers, caching, and offline capabilities, which are core features of a PWA. In this section, we will walk through the steps to set up a PWA using Create React App.
Step 1: Create a New React App
If you don't have a React app yet, you can easily create one using Create React App. Open a terminal and run the following command to create a new React project:
npx create-react-app my-pwa
Navigate to the project directory:
cd my-pwa
Step 2: Enable Service Worker
By default, Create React App includes a service worker file, but it is not enabled. To enable the service worker, open the src/index.js
file and find the following line:
serviceWorker.unregister();
Change it to:
serviceWorker.register();
By calling serviceWorker.register()
, the service worker is activated, allowing your app to cache assets and work offline.
Step 3: Modify the Manifest File
Create React App includes a manifest.json
file, which is essential for turning your app into a PWA. The manifest.json
file contains metadata about your app, such as its name, icons, and theme color.
Open the public/manifest.json
file and customize the values to match your app:
{
"short_name": "My PWA",
"name": "My Progressive Web App",
"icons": [
{
"src": "favicon.ico",
"sizes": "16x16 32x32 64x64",
"type": "image/x-icon"
},
{
"src": "logo192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "logo512.png",
"sizes": "512x512",
"type": "image/png"
}
],
"start_url": ".",
"background_color": "#ffffff",
"display": "standalone",
"theme_color": "#000000"
}
The short_name
is used when there is limited space for the app name (e.g., on the home screen). The icons
section defines the icons that will be used by the browser for the PWA installation prompt. The background_color
and theme_color
are used to define the look of the app when it's launched.
Step 4: Build the App for Production
After enabling the service worker and modifying the manifest, you need to build your app for production. Run the following command:
npm run build
This will create an optimized production build of your React app. The service worker will start caching assets, and your app will be ready for offline use.
Step 5: Test the PWA
You can now test your app as a PWA. To do this, you need to serve the app locally using a static file server. First, install the serve
package globally:
npm install -g serve
Then, run the following command to serve the build folder:
serve -s build
Visit the app in your browser (typically at http://localhost:5000
) and check if it works offline. You can also check the "Application" tab in Chrome Developer Tools to confirm that the service worker is registered and caching assets.
Step 6: Optional - Customize the Service Worker
The service worker provided by Create React App is set up for basic caching. If you need more control over the caching strategy, you can customize the service worker file. You can find the service worker logic in the src/service-worker.js
file.
For example, you can modify the caching strategy to use a "stale-while-revalidate" approach or cache API responses. Here's a simple example of customizing the fetch event handler in the service worker:
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((cachedResponse) => {
return cachedResponse || fetch(event.request).then((response) => {
return caches.open('my-cache').then((cache) => {
cache.put(event.request, response.clone());
return response;
});
});
})
);
});
This custom service worker caches assets and serves them from cache when possible, falling back to the network when necessary.
Step 7: Deploy Your PWA
Once your app is ready, you can deploy it to your preferred hosting provider. Popular options for hosting PWAs include:
- Netlify: Simple deployment with automatic SSL and PWA support.
- Vercel: Another easy-to-use platform with support for PWAs.
- Firebase Hosting: Firebase offers excellent support for serving PWAs with caching and service workers out of the box.
Conclusion
By following the steps above, you can easily set up a Progressive Web App using Create React App. Service workers and caching will help make your app work offline, and the app can be installed on users' devices for a native-like experience. PWAs offer significant performance and accessibility improvements, especially for mobile users.
Introduction to React Native for Mobile Development
React Native is a powerful framework for building cross-platform mobile applications using JavaScript and React. With React Native, developers can create mobile apps for iOS and Android using a single codebase, making it a popular choice for mobile development. In this section, we will introduce React Native and its core concepts, along with the steps to get started with building mobile apps.
What is React Native?
React Native is an open-source framework developed by Facebook. It allows developers to build mobile apps using JavaScript and React, but instead of rendering HTML, React Native renders native components on iOS and Android. This makes React Native a great option for building high-performance mobile apps without the need for learning native programming languages like Swift or Java/Kotlin.
Key Features of React Native
- Cross-Platform Development: Write once, run anywhere. With React Native, you can use the same codebase for both iOS and Android applications.
- Native Components: React Native uses actual native components like
View
,Text
, andImage
to build UIs, offering better performance than traditional hybrid apps. - Hot Reloading: React Native supports hot reloading, allowing developers to instantly view changes in the app without rebuilding it.
- Large Community: React Native has a large and active community, which means you can find plenty of tutorials, libraries, and resources to help you along the way.
Setting Up a React Native Development Environment
To get started with React Native, you need to set up your development environment. React Native supports both macOS and Windows, with some additional setup required for macOS to develop for iOS. Follow these steps to get started:
Step 1: Install Node.js and npm
React Native requires Node.js, which includes npm (Node Package Manager) to install packages. You can download and install Node.js from the official website:
https://nodejs.org/
Step 2: Install React Native CLI
React Native offers two different ways to set up a project: the Expo CLI and the React Native CLI. For this guide, we will use the React Native CLI. To install it globally on your system, run the following command:
npm install -g react-native-cli
Step 3: Install Xcode (macOS Only)
If you are on macOS and want to develop for iOS, you need to install Xcode. You can download Xcode from the Mac App Store:
https://apps.apple.com/us/app/xcode/id497799835?mt=12
Step 4: Install Android Studio
To develop for Android, you need to install Android Studio, which includes Android SDK and an emulator. You can download it from the official website:
https://developer.android.com/studio
Creating a New React Native Project
After setting up the development environment, you can create a new React Native project. To do this, run the following command:
react-native init MyFirstApp
This will create a new directory called MyFirstApp
with all the necessary files and dependencies for a React Native app.
Running Your React Native App
Once your project is created, you can run it on an emulator or a physical device.
Running on iOS
If you are developing for iOS, you can run the app on an iOS simulator by running the following command:
react-native run-ios
Running on Android
If you are developing for Android, you can run the app on an Android emulator or a physical device by running:
react-native run-android
Basic React Native App Structure
A React Native app is similar to a React web app in terms of structure, but it uses native components instead of HTML elements. Here’s an example of a basic React Native app:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, React Native!</Text>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
},
text: {
fontSize: 20,
color: '#333',
},
});
export default App;
In this example, we use the View
component to create a container and the Text
component to display text. The StyleSheet
is used to define styles for the components.
React Native Components
React Native provides a set of core components that you can use to build your mobile app. Here are some commonly used components:
- View: A container component that is used to wrap other components.
- Text: Used to display text on the screen.
- Image: Used to display images.
- TextInput: A component for handling text input from the user.
- Button: A simple button component for user interaction.
Conclusion
React Native is a powerful framework for building mobile apps with JavaScript and React. It allows developers to build cross-platform apps with native performance, making it an excellent choice for mobile development. With React Native, you can leverage your existing React knowledge to build mobile applications for iOS and Android, and take advantage of its rich ecosystem and community.
Setting Up a React Native Development Environment
Setting up a React Native development environment involves installing several tools and configuring your system for mobile app development. In this section, we will guide you through the steps to set up your environment for React Native on both macOS and Windows.
Prerequisites
Before you start, ensure that your system meets the following requirements:
- Node.js: React Native relies on Node.js for managing dependencies and running the development server.
- npm: npm is the package manager for Node.js and is used to install React Native and other dependencies.
- Watchman: Watchman is a tool that helps watch file changes. It is recommended for macOS users.
- Java Development Kit (JDK): Required to build Android apps.
- Xcode (macOS only): Required for iOS development.
- Android Studio: Required for Android development.
Step 1: Install Node.js and npm
React Native requires Node.js and npm. You can download and install the latest stable version of Node.js from the official website:
https://nodejs.org/
After installing Node.js, verify that it has been installed correctly by running the following commands in your terminal:
node -v
npm -v
Step 2: Install React Native CLI
React Native offers two different ways to set up a project: the React Native CLI and Expo CLI. In this guide, we will use the React Native CLI, which offers more flexibility for native code customization.
To install the React Native CLI globally, run the following command:
npm install -g react-native-cli
Step 3: Install Watchman (macOS only)
For macOS users, it is recommended to install Watchman to optimize file watching during development. You can install Watchman using Homebrew:
brew install watchman
Step 4: Install Xcode (macOS only)
If you are working on macOS and want to build iOS apps, you need to install Xcode. Xcode is available for free on the Mac App Store:
https://apps.apple.com/us/app/xcode/id497799835?mt=12
Once you have Xcode installed, open it and agree to the license terms. You will also need to install Xcode command-line tools by running:
xcode-select --install
Step 5: Install Android Studio
To develop for Android, you need to install Android Studio, which includes the Android SDK and emulator. Download and install Android Studio from the following link:
https://developer.android.com/studio
After installing Android Studio, open it and follow the setup instructions. Make sure to install the Android SDK and Android Virtual Device (AVD) during the setup process.
Step 6: Set Up Android Environment Variables
After installing Android Studio, you need to set up the Android development environment variables:
- ANDROID_HOME: The path to the Android SDK.
- PATH: Add the Android SDK tools to the PATH environment variable.
On macOS, you can add these lines to your .bash_profile
or .zshrc
file:
export ANDROID_HOME=$HOME/Library/Android/sdk
export PATH=$ANDROID_HOME/tools:$ANDROID_HOME/platform-tools:$PATH
Step 7: Verify the Installation
Once you have installed all the required tools, you can verify that everything is set up correctly by running the following command:
react-native doctor
The react-native doctor
command checks for common issues and tells you if anything is missing or needs to be fixed. Follow the recommended fixes if necessary.
Step 8: Create a New React Native Project
After setting up your environment, you can create a new React Native project by running the following command:
react-native init MyNewApp
This will create a new directory called MyNewApp
with all the necessary files and dependencies for a React Native app.
Step 9: Run Your React Native App
Now that your project is created, you can run the app on an emulator or a physical device.
Running on iOS
If you are developing for iOS, you can run the app on an iOS simulator by running:
react-native run-ios
Running on Android
If you are developing for Android, you can run the app on an Android emulator or a physical device by running:
react-native run-android
Conclusion
By following these steps, you can set up a React Native development environment and start building mobile apps for iOS and Android. React Native offers a fast and efficient way to develop mobile apps with JavaScript, and it allows you to reuse your existing web development skills to create cross-platform apps. Happy coding!
Creating a Simple Mobile App with React Native
React Native allows you to build mobile apps for both iOS and Android using JavaScript. In this section, we will guide you through the process of creating a simple mobile app using React Native.
Step 1: Set Up Your React Native Development Environment
Before you begin, ensure that you have set up your development environment for React Native. If you haven’t done so, refer to the Setting Up a React Native Development Environment section for detailed instructions.
Step 2: Create a New React Native Project
Once your environment is ready, you can create a new React Native project by running the following command:
react-native init MySimpleApp
This will create a new directory called MySimpleApp
with all the necessary files and dependencies. After the project is created, navigate to the project folder:
cd MySimpleApp
Step 3: Modify the App Component
Open the App.js
file in your project directory. This file is the main entry point for your app and contains the default component. Let's modify it to display a simple "Hello, World!" message.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
},
text: {
fontSize: 24,
color: 'blue',
},
});
export default App;
In this code:
- We import
View
andText
fromreact-native
to build the UI components. - We use
StyleSheet.create
to define styles for the app, including centering the content and setting the text color to blue. - We display a simple "Hello, World!" message inside a
Text
component.
Step 4: Run the App on an Emulator or Device
Now that you have modified the app, you can run it on an emulator or a physical device.
Running on iOS
If you are using macOS and have Xcode installed, you can run the app on an iOS simulator:
react-native run-ios
Running on Android
If you are using Android Studio and have an Android emulator set up, you can run the app on an Android device:
react-native run-android
Step 5: Modify the App to Include a Button
Let's now modify the app to include a button that changes the displayed text when pressed.
import React, { useState } from 'react';
import { View, Text, Button, StyleSheet } from 'react-native';
const App = () => {
const [message, setMessage] = useState('Hello, World!');
const changeMessage = () => {
setMessage('Hello, React Native!');
};
return (
<View style={styles.container}>
<Text style={styles.text}>{message}</Text>
<Button title="Change Message" onPress={changeMessage} />
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#fff',
},
text: {
fontSize: 24,
color: 'blue',
marginBottom: 20,
},
});
export default App;
In this code:
- We use the
useState
hook to manage the state of themessage
. - The
changeMessage
function updates the state when the button is pressed. - We display the message inside the
Text
component and add aButton
component that triggers thechangeMessage
function.
Step 6: Run the Modified App
After making the changes, you can re-run the app to see the updated functionality. Press the button to change the displayed message.
Conclusion
Congratulations! You have successfully created a simple mobile app with React Native. You can now start building more complex apps by adding more features like navigation, data fetching, and more. React Native allows you to use your web development skills to create powerful cross-platform mobile apps.
Navigation and State Management in React Native
In this section, we will learn how to implement navigation in a React Native app and manage state using React's built-in hooks or a state management library like Redux. React Navigation is commonly used for managing navigation in React Native apps, and we'll go through how to set it up and use it for navigation.
Step 1: Install React Navigation
First, we need to install the necessary libraries for navigation. Run the following commands to install React Navigation and the required dependencies:
npm install @react-navigation/native
npm install @react-navigation/stack
npm install react-native-screens react-native-safe-area-context
Additionally, you need to install the dependencies for linking:
npx pod-install ios
Step 2: Set Up Navigation
Next, we can set up a basic stack navigation using React Navigation. Create a file called App.js
and modify it as follows:
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import { View, Text, Button } from 'react-native';
const HomeScreen = ({ navigation }) => {
return (
Home Screen
<Button title="Go to Details" onPress={() => navigation.navigate('Details')} />
);
};
const DetailsScreen = () => {
return (
Details Screen
);
};
const Stack = createStackNavigator();
const App = () => {
return (
);
};
export default App;
In this code:
- We use
NavigationContainer
to wrap the entire navigation structure. - We create a
Stack.Navigator
to define the navigation stack. - We define two screens:
HomeScreen
andDetailsScreen
, and navigate between them using thenavigation.navigate()
method.
Step 3: Install and Set Up State Management
For state management, we can use React's built-in useState
hook or a more advanced solution like Redux. Let's start with using useState
to manage state in the app.
import React, { useState } from 'react';
import { View, Text, Button } from 'react-native';
const HomeScreen = ({ navigation }) => {
const [count, setCount] = useState(0);
return (
Home Screen
Count: {count}
<Button title="Increment" onPress={() => setCount(count + 1)} />
<Button title="Go to Details" onPress={() => navigation.navigate('Details')} />
);
};
In this code:
- We use the
useState
hook to manage the state of acount
variable. - We display the value of
count
and update it when the "Increment" button is pressed. - The app still includes a navigation button that takes the user to the
Details
screen.
Step 4: Using Redux for State Management (Optional)
If you need more advanced state management, you can use Redux. To set it up, install the necessary dependencies:
npm install redux react-redux
Next, create a simple Redux setup for managing the app's state. In this case, we will manage a counter value with Redux:
import React from 'react';
import { View, Text, Button } from 'react-native';
import { useDispatch, useSelector } from 'react-redux';
import { increment } from './actions';
const HomeScreen = () => {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
Home Screen
Count: {count}
<Button title="Increment" onPress={() => dispatch(increment())} />
);
};
export default HomeScreen;
In this code:
- We use the
useSelector
hook to retrieve the state from the Redux store. - We use the
useDispatch
hook to dispatch theincrement
action when the "Increment" button is pressed.
You'll need to set up the Redux store and actions in separate files as well. Here's an example of how to set up the Redux store and an increment action:
// actions.js
export const increment = () => {
return {
type: 'INCREMENT',
};
};
// reducer.js
const initialState = {
count: 0,
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
export default rootReducer;
Finally, wrap your app in the Redux Provider
to make the store available to your components:
import React from 'react';
import { Provider } from 'react-redux';
import { createStore } from 'redux';
import rootReducer from './reducer';
import HomeScreen from './HomeScreen';
const store = createStore(rootReducer);
const App = () => {
return (
);
};
export default App;
Step 5: Run the App with Navigation and State Management
Now that you have set up both navigation and state management, run your app on an emulator or device to test the functionality. You should be able to navigate between screens and manage state either with React's useState
or Redux.
Conclusion
In this section, we covered how to implement navigation in React Native using React Navigation, as well as how to manage state using useState
and Redux. With this setup, you can create more complex apps with multiple screens and efficient state management.
Integrating APIs in React Native
In this section, we will explore how to integrate APIs into your React Native app. APIs allow your app to fetch data from external servers, and integrating them is a crucial part of building dynamic mobile applications.
Step 1: Using the Fetch API
React Native provides the fetch()
function, which is used to make HTTP requests to external APIs. Here's how to fetch data from an API and display it in a React Native app:
import React, { useState, useEffect } from 'react';
import { View, Text, ActivityIndicator } from 'react-native';
const App = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => {
setData(data);
setLoading(false);
})
.catch((error) => {
setError(error);
setLoading(false);
});
}, []);
if (loading) {
return (
);
}
if (error) {
return (
Error: {error.message}
);
}
return (
Data: {JSON.stringify(data)}
);
};
export default App;
In this example:
- We use the
fetch()
function to make an API request to an external URL. - We manage the data state using
useState
, and we handle loading and error states as well. - The data is displayed once it's fetched, and an
ActivityIndicator
is shown while the data is loading.
Step 2: Handling API Calls with Axios
Although fetch()
is a simple and native way to make HTTP requests, you can also use Axios, a popular HTTP client that provides additional features like automatic JSON parsing and interceptors.
To use Axios, you first need to install it:
npm install axios
After installing Axios, you can use it to make API requests in the following way:
import React, { useState, useEffect } from 'react';
import { View, Text, ActivityIndicator } from 'react-native';
import axios from 'axios';
const App = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
axios
.get('https://api.example.com/data')
.then((response) => {
setData(response.data);
setLoading(false);
})
.catch((error) => {
setError(error);
setLoading(false);
});
}, []);
if (loading) {
return (
);
}
if (error) {
return (
Error: {error.message}
);
}
return (
Data: {JSON.stringify(data)}
);
};
export default App;
In this code:
- We use Axios to make a GET request to the API and retrieve the data.
- We handle loading and error states similarly as with the
fetch()
example. - The response data is stored in the
data
state variable.
Step 3: Handling POST Requests
To send data to an API, you can use a POST request. Here's an example using Axios to send data to an API:
import React, { useState } from 'react';
import { View, Text, Button } from 'react-native';
import axios from 'axios';
const App = () => {
const [response, setResponse] = useState(null);
const sendData = () => {
axios
.post('https://api.example.com/submit', {
name: 'John Doe',
email: 'johndoe@example.com',
})
.then((response) => {
setResponse(response.data);
})
.catch((error) => {
console.error(error);
});
};
return (
{response && Response: {JSON.stringify(response)} }
);
};
export default App;
In this code:
- We use a POST request to send data to the API.
- The data is sent as an object in the body of the request.
- The server's response is stored in the
response
state variable and displayed on the screen.
Step 4: Using Async/Await for API Calls
Instead of using then()
and catch()
, you can use async/await
to make your code more readable. Here's an example using async/await
with Axios:
import React, { useState, useEffect } from 'react';
import { View, Text, ActivityIndicator } from 'react-native';
import axios from 'axios';
const App = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
setError(error);
} finally {
setLoading(false);
}
};
useEffect(() => {
fetchData();
}, []);
if (loading) {
return (
);
}
if (error) {
return (
Error: {error.message}
);
}
return (
Data: {JSON.stringify(data)}
);
};
export default App;
In this code:
- We define an
async
functionfetchData
that usesawait
to fetch data from the API. - We use a
try/catch/finally
block to handle loading, errors, and completion of the API request.
Step 5: Error Handling and Retry Logic
Error handling is essential when working with APIs. You can handle errors with catch()
or use try/catch
in an async function. Additionally, implementing retry logic can help in case of network failures or timeouts:
const fetchDataWithRetry = async (retries = 3) => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
if (retries === 0) {
setError(error);
} else {
fetchDataWithRetry(retries - 1);
}
}
};
In this example, if an API call fails, it will retry up to 3 times before throwing an error.
Conclusion
In this section, we covered how to integrate APIs into a React Native app using both the fetch()
API and Axios. We also discussed how to handle GET and POST requests, manage loading and error states, and implement retry logic for resilience. Integrating APIs is a fundamental skill for building mobile apps that interact with external data sources.
Debugging and Testing React Native Apps
Debugging and testing are crucial parts of the development process to ensure your React Native app works as expected. In this section, we will explore various techniques and tools you can use to debug and test your React Native apps effectively.
Step 1: Using the React Native Debugger
The React Native Debugger is a powerful tool that integrates with Chrome Developer Tools and allows you to inspect your app's state, network requests, and more. To start using it, you need to enable debugging in your app:
# Run your React Native app
react-native run-android
# Enable debugging
Cmd + D (iOS) or Cmd + M (Android) -> Enable Debugging
Once debugging is enabled, you can open the React Native Debugger by following these steps:
- Open the developer menu by pressing
Cmd + M
(Android) orCmd + D
(iOS). - Click on Debug JS Remotely.
- In Chrome, open the developer tools to inspect the app’s network requests, console logs, and performance.
Step 2: Using Console Logs for Debugging
Console logs are a simple and effective way to debug issues in your app. You can use console.log()
to log values and trace the flow of your app's execution.
import React from 'react';
import { View, Text, Button } from 'react-native';
const App = () => {
const handleClick = () => {
console.log('Button clicked!');
};
return (
Debugging with console.log
);
};
export default App;
In this example, console.log()
will output "Button clicked!" to the console when the button is pressed. You can use this method to log variables, objects, and other relevant information during app execution.
Step 3: Debugging with React Native’s Remote Debugging
React Native provides a powerful feature called Remote Debugging, which allows you to debug JavaScript code running in your app in Chrome’s Developer Tools.
- Open the developer menu in your app (
Cmd + M
for Android,Cmd + D
for iOS). - Enable Debug JS Remotely.
- This will open a new tab in Chrome, where you can inspect your app’s JavaScript code and set breakpoints.
Step 4: Testing with Jest
Jest is a popular testing framework for JavaScript applications, and it works seamlessly with React Native. To get started with Jest, you need to install it and set up your environment.
# Install Jest for testing
npm install --save-dev jest
Once Jest is installed, you can write unit tests for your components and functions. Here’s an example of how to test a simple React Native component:
import React from 'react';
import { render } from '@testing-library/react-native';
import App from './App';
test('renders learn react link', () => {
const { getByText } = render( );
const linkElement = getByText(/learn react/i);
expect(linkElement).toBeTruthy();
});
In this example, we use the @testing-library/react-native
library to render the App
component and check whether the "learn react" text is present in the output. The test will pass if the text is rendered correctly.
Step 5: Writing Snapshot Tests with Jest
Snapshot testing with Jest allows you to test UI components by capturing their rendered output and comparing it to previous snapshots. This can be useful for detecting unexpected changes in your components.
import React from 'react';
import { render } from '@testing-library/react-native';
import App from './App';
test('App renders correctly', () => {
const { toJSON } = render( );
expect(toJSON()).toMatchSnapshot();
});
In this example, the toJSON()
method is used to capture the rendered output of the App
component and compare it with the saved snapshot. If the UI changes, Jest will alert you to the difference.
Step 6: Using Detox for End-to-End Testing
Detox is an end-to-end testing framework for React Native that allows you to simulate user interactions and test how your app behaves from start to finish. To use Detox, follow these steps:
# Install Detox CLI and dependencies
npm install --save-dev detox
# Link Detox with your React Native project
detox init -r jest
Once Detox is set up, you can write end-to-end tests to interact with your app and verify its behavior:
describe('App', () => {
beforeAll(async () => {
await device.launchApp();
});
it('should show the welcome screen', async () => {
await expect(element(by.id('welcome-screen'))).toBeVisible();
});
});
In this example, we use Detox to launch the app and check whether the welcome screen is visible. Detox simulates user interactions and verifies the expected results.
Step 7: Debugging Performance Issues
Performance issues can significantly affect your app’s usability. React Native provides some tools to help you identify performance bottlenecks:
- React DevTools: Use React DevTools to inspect the component tree and measure performance.
- Performance Monitor: Enable the Performance Monitor from the developer menu to view the app’s FPS and memory usage in real-time.
- Flipper: Flipper is a platform for debugging iOS and Android apps. It offers tools for inspecting the app’s layout, network requests, and performance.
Conclusion
Debugging and testing are essential for ensuring that your React Native apps are bug-free and perform well. By using tools like the React Native Debugger, Jest, Detox, and performance monitors, you can effectively debug and test your apps, leading to better app quality and user experience.
Connecting React with Backend APIs (Node.js, Express, etc.)
Connecting a React frontend with a backend API is a common task in modern web development. In this section, we will explore how to connect your React application to a backend server built with Node.js and Express, allowing you to make HTTP requests and retrieve data.
Step 1: Setting Up the Backend (Node.js and Express)
First, let's set up a simple Node.js and Express server. Create a new directory for your backend server:
mkdir backend
cd backend
npm init -y
npm install express
Once the packages are installed, create a file named server.js
in the root of your backend directory:
const express = require('express');
const app = express();
const port = 5000;
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello from the backend!' });
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
This server listens on port 5000 and has a simple GET route /api/data
that returns a JSON response.
Step 2: Running the Backend Server
To start the backend server, run the following command:
node server.js
Your backend server should now be running at http://localhost:5000
.
Step 3: Setting Up the React Frontend
Next, let's set up the React frontend. If you haven't already created a React app, you can create one using Create React App:
npx create-react-app my-app
cd my-app
Once the app is created, you can start the React development server by running:
npm start
Step 4: Making API Requests from React
Now that both your backend and frontend are set up, you can make API requests from React using the fetch
API or an HTTP client like Axios.
Using the Fetch API
Here’s an example of how to fetch data from the backend API using the fetch
API:
import React, { useEffect, useState } from 'react';
const App = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('http://localhost:5000/api/data')
.then((response) => response.json())
.then((data) => setData(data.message));
}, []);
return (
{data ? data : 'Loading...'}
);
};
export default App;
In this example, the app makes a GET request to the backend API at http://localhost:5000/api/data
and displays the message returned from the server.
Using Axios
Alternatively, you can use Axios, which is a popular library for making HTTP requests. First, install Axios:
npm install axios
Then, modify your component to use Axios instead of fetch
:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const App = () => {
const [data, setData] = useState(null);
useEffect(() => {
axios.get('http://localhost:5000/api/data')
.then((response) => setData(response.data.message))
.catch((error) => console.error('Error fetching data:', error));
}, []);
return (
{data ? data : 'Loading...'}
);
};
export default App;
In this version, Axios is used to make the GET request, and the response data is stored in the data
state.
Step 5: Handling CORS (Cross-Origin Resource Sharing)
If your React app and backend are running on different ports, you might encounter CORS issues. To resolve this, you can install and configure the cors
middleware in your Express server:
npm install cors
Then, update your server.js
file to use cors
:
const express = require('express');
const cors = require('cors');
const app = express();
const port = 5000;
app.use(cors());
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello from the backend!' });
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
By using app.use(cors())
, you allow cross-origin requests from any domain, which resolves the CORS issue when your React frontend makes requests to your backend.
Step 6: Handling POST Requests
In addition to GET requests, you can handle POST requests in Express to send data from your frontend to the backend. For example, let's add a new POST route to the backend:
app.post('/api/data', (req, res) => {
const { message } = req.body;
res.json({ receivedMessage: message });
});
Now, you can send a POST request from React using either fetch
or Axios:
const sendData = () => {
axios.post('http://localhost:5000/api/data', { message: 'Hello, backend!' })
.then((response) => console.log(response.data))
.catch((error) => console.error('Error sending data:', error));
};
In this example, the frontend sends a POST request with a message to the backend, and the backend responds with the received message.
Conclusion
By following these steps, you can easily connect your React frontend to a backend API built with Node.js and Express. This allows your frontend to send and receive data from the backend, enabling you to build dynamic web applications. You can also handle CORS issues, send and receive both GET and POST requests, and manage state in React based on the data from the backend.
Authentication and Authorization in Full-Stack Apps
Authentication and authorization are essential components of web applications to ensure that only authorized users can access certain resources. In this section, we'll explore how to implement authentication and authorization in a full-stack app using technologies like JWT (JSON Web Tokens), OAuth, and role-based access control (RBAC).
Step 1: Understanding Authentication vs Authorization
Before we dive into the implementation, it's important to understand the difference between authentication and authorization:
- Authentication: The process of verifying the identity of a user. In a typical web app, this is done by checking the username and password or using OAuth providers like Google or GitHub.
- Authorization: The process of determining what actions or resources a user can access. This is typically done by checking the user's roles or permissions.
Step 2: Setting Up Authentication with JWT
JWT is a popular method for securely transmitting information between a client and server. It is commonly used for authentication in full-stack applications. In this example, we'll use Node.js and Express for the backend, and React for the frontend.
Backend Setup (Node.js and Express)
First, let's set up the backend to handle JWT authentication. Install the necessary dependencies:
npm install express jsonwebtoken bcryptjs dotenv
Next, create a simple Express server that generates a JWT upon successful login:
const express = require('express');
const jwt = require('jsonwebtoken');
const bcrypt = require('bcryptjs');
const dotenv = require('dotenv');
dotenv.config();
const app = express();
const port = 5000;
app.use(express.json());
const users = []; // In a real app, use a database
// Endpoint to register a new user
app.post('/api/register', async (req, res) => {
const { username, password } = req.body;
const hashedPassword = await bcrypt.hash(password, 10);
users.push({ username, password: hashedPassword });
res.status(201).send('User registered');
});
// Endpoint to login and generate JWT
app.post('/api/login', async (req, res) => {
const { username, password } = req.body;
const user = users.find((u) => u.username === username);
if (!user) return res.status(400).send('User not found');
const validPassword = await bcrypt.compare(password, user.password);
if (!validPassword) return res.status(400).send('Invalid password');
const token = jwt.sign({ username }, process.env.JWT_SECRET, { expiresIn: '1h' });
res.json({ token });
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
In this setup, when a user logs in, the backend generates a JWT using the jsonwebtoken
package. The token is sent back to the client and can be used for subsequent requests to authenticate the user.
Frontend Setup (React)
Now, let's set up the React app to send the login credentials to the server and store the JWT for subsequent requests. First, install the Axios library for making HTTP requests:
npm install axios
Next, create a login form in React and handle the login logic:
import React, { useState } from 'react';
import axios from 'axios';
const Login = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [token, setToken] = useState('');
const handleLogin = async (e) => {
e.preventDefault();
try {
const response = await axios.post('http://localhost:5000/api/login', { username, password });
setToken(response.data.token);
localStorage.setItem('token', response.data.token);
} catch (error) {
console.error('Login failed', error);
}
};
return (
{token && Logged in with token: {token}
}
);
};
export default Login;
In this React component, the user enters their credentials and submits the form. The credentials are sent to the backend, and if successful, a JWT is received and stored in localStorage.
Step 3: Adding Authorization (Role-Based Access Control)
Once the user is authenticated, we can implement authorization to control access to certain parts of the app based on the user's role. For this, we can add roles to the JWT payload and verify them on the backend.
Backend Role-based Authorization
Modify the login endpoint to include a role in the JWT payload:
app.post('/api/login', async (req, res) => {
const { username, password } = req.body;
const user = users.find((u) => u.username === username);
if (!user) return res.status(400).send('User not found');
const validPassword = await bcrypt.compare(password, user.password);
if (!validPassword) return res.status(400).send('Invalid password');
const role = 'admin'; // Hardcoded role for this example
const token = jwt.sign({ username, role }, process.env.JWT_SECRET, { expiresIn: '1h' });
res.json({ token });
});
Now the JWT contains a role
claim. On the backend, you can verify the user's role before granting access to certain routes:
const authenticateRole = (role) => {
return (req, res, next) => {
const token = req.header('Authorization')?.replace('Bearer ', '');
if (!token) return res.status(401).send('Access denied');
jwt.verify(token, process.env.JWT_SECRET, (err, decoded) => {
if (err) return res.status(400).send('Invalid token');
if (decoded.role !== role) return res.status(403).send('Forbidden');
req.user = decoded;
next();
});
};
};
app.get('/api/admin', authenticateRole('admin'), (req, res) => {
res.send('Welcome, admin!');
});
In this example, the authenticateRole
middleware checks if the user has the required role before allowing access to the route.
Step 4: Protecting Routes in React
On the frontend, we can use the JWT token to protect routes that require authentication or specific roles. For example, you can create a protected route component:
import React from 'react';
import { Redirect } from 'react-router-dom';
const ProtectedRoute = ({ children, requiredRole }) => {
const token = localStorage.getItem('token');
if (!token) {
return ;
}
const decoded = JSON.parse(atob(token.split('.')[1]));
if (decoded.role !== requiredRole) {
return ;
}
return children;
};
export default ProtectedRoute;
In this ProtectedRoute
component, the app checks if the token exists and whether the user's role matches the required role. If the conditions are not met, the user is redirected to the login page or an unauthorized page.
Conclusion
Authentication and authorization are crucial for securing full-stack applications. By using JWTs for authentication and role-based access control for authorization, you can ensure that only authenticated users with the appropriate permissions can access certain parts of your app. This approach is scalable and can be extended with more advanced features like OAuth authentication, user permissions, and more.
Managing Sessions and Cookies in React
Managing sessions and cookies is essential for preserving user data, authentication, and preferences across page reloads. In this section, we'll explore how to handle sessions and cookies in a React application to ensure a smooth user experience.
Step 1: Understanding Cookies and Sessions
Before diving into the implementation, it's important to understand the difference between cookies and sessions:
- Cookies: Small pieces of data stored in the browser that are sent with every HTTP request to the server. Cookies can be used to store information like authentication tokens or user preferences.
- Sessions: A session is a server-side storage mechanism that is used to store user-specific data during a user’s interaction with the application. The session typically relies on a session ID that is stored in a cookie.
Step 2: Setting Up Cookies in React
In React, you can set and manage cookies using libraries like js-cookie
. This library makes it easy to read, write, and delete cookies on the client-side.
Install js-cookie
To get started, install the js-cookie
package:
npm install js-cookie
Setting a Cookie
Once you have the library installed, you can set cookies in your React components. Here's an example of how to set a cookie for storing an authentication token:
import React, { useState } from 'react';
import Cookies from 'js-cookie';
const Login = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const handleLogin = () => {
// Assume authentication is successful
const token = 'your-jwt-token';
// Set a cookie with the token
Cookies.set('authToken', token, { expires: 7 }); // Expires in 7 days
alert('Login successful!');
};
return (
<input
type="text"
placeholder="Username"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
<input
type="password"
placeholder="Password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
);
};
export default Login;
In this example, when the user logs in, we create a cookie named authToken
to store the JWT token. The expires
option ensures that the cookie will expire after 7 days.
Reading a Cookie
To read the cookie, you can use the Cookies.get()
method:
import React, { useEffect, useState } from 'react';
import Cookies from 'js-cookie';
const Dashboard = () => {
const [authToken, setAuthToken] = useState('');
useEffect(() => {
const token = Cookies.get('authToken');
if (token) {
setAuthToken(token);
}
}, []);
return (
{authToken ? Welcome, you are logged in!
: Please log in.
}
);
};
export default Dashboard;
In this example, we check if the authToken
cookie exists and display a welcome message if the user is logged in.
Step 3: Deleting a Cookie
If you want to log out the user and remove the cookie, you can use the Cookies.remove()
method:
import React from 'react';
import Cookies from 'js-cookie';
const Logout = () => {
const handleLogout = () => {
// Remove the authToken cookie
Cookies.remove('authToken');
alert('You have been logged out!');
};
return (
);
};
export default Logout;
In this example, when the user clicks the logout button, the authToken
cookie is deleted, effectively logging the user out.
Step 4: Managing Sessions with Cookies
In addition to storing authentication tokens in cookies, you can also store session-related data in cookies. For example, you might want to store the user's preferences or theme settings.
Storing Session Data in Cookies
Here's an example of how to store user preferences like theme settings in a cookie:
import React, { useState, useEffect } from 'react';
import Cookies from 'js-cookie';
const Preferences = () => {
const [theme, setTheme] = useState('light');
useEffect(() => {
const savedTheme = Cookies.get('theme');
if (savedTheme) {
setTheme(savedTheme);
}
}, []);
const handleThemeChange = (newTheme) => {
setTheme(newTheme);
Cookies.set('theme', newTheme, { expires: 30 }); // Save theme for 30 days
};
return (
Current Theme: {theme}
<button onClick={() => handleThemeChange('light')}>Light Theme</button>
<button onClick={() => handleThemeChange('dark')}>Dark Theme</button>
);
};
export default Preferences;
In this example, we store the user's theme preference in a cookie and load it when the component mounts. The user's preference is saved for 30 days.
Step 5: Using Cookies for Session Expiry
Cookies can also be used to manage session expiration. For example, you might want to automatically log the user out after a certain period of inactivity.
import React, { useEffect } from 'react';
import Cookies from 'js-cookie';
const SessionExpiry = () => {
useEffect(() => {
const token = Cookies.get('authToken');
if (token) {
const expiryTime = new Date().getTime() + 3600000; // 1 hour
Cookies.set('expiryTime', expiryTime, { expires: 1 });
}
}, []);
const checkSession = () => {
const expiryTime = Cookies.get('expiryTime');
if (expiryTime && new Date().getTime() > expiryTime) {
Cookies.remove('authToken');
Cookies.remove('expiryTime');
alert('Session expired. Please log in again.');
}
};
useEffect(() => {
const interval = setInterval(checkSession, 10000); // Check every 10 seconds
return () => clearInterval(interval);
}, []);
return Session management is active.;
};
export default SessionExpiry;
In this example, we store an expiryTime
cookie to track when the session should expire. The app checks the session every 10 seconds, and if the session has expired, the user is logged out automatically.
Conclusion
Managing sessions and cookies in React is a crucial part of building secure and user-friendly applications. By using libraries like js-cookie
, you can easily store authentication tokens, manage user preferences, and handle session expiration. Proper cookie management ensures a smooth experience for users and helps maintain the security of your app.
Using WebSockets for Real-Time Features
WebSockets allow you to establish a two-way communication channel between the client and server, enabling real-time features in your application. In this section, we will explore how to use WebSockets to add real-time features like live notifications, chat functionality, and more in your React applications.
Step 1: Understanding WebSockets
WebSockets are a protocol that enables bidirectional communication between a client and a server over a long-lived connection. Unlike traditional HTTP requests, where the client makes a request and waits for a response, WebSockets allow the server to push data to the client without requiring a request. This makes WebSockets ideal for real-time applications.
Step 2: Installing a WebSocket Library
To integrate WebSockets into your React application, you can use the socket.io-client
library, which simplifies working with WebSockets. First, install it using npm:
npm install socket.io-client
Step 3: Setting Up WebSocket Connection in React
Once the WebSocket library is installed, you can establish a WebSocket connection in your React components. Here's an example of how to connect to a WebSocket server and listen for incoming messages:
import React, { useEffect, useState } from 'react';
import { io } from 'socket.io-client';
const RealTimeChat = () => {
const [messages, setMessages] = useState([]);
const [newMessage, setNewMessage] = useState('');
const socket = io('http://localhost:4000'); // Replace with your server URL
useEffect(() => {
// Listen for incoming messages from the server
socket.on('newMessage', (message) => {
setMessages((prevMessages) => [...prevMessages, message]);
});
// Cleanup on component unmount
return () => {
socket.off('newMessage');
};
}, [socket]);
const sendMessage = () => {
if (newMessage.trim()) {
socket.emit('sendMessage', newMessage);
setNewMessage('');
}
};
return (
Real-Time Chat
{messages.map((msg, index) => (
- {msg}
))}
<input
type="text"
value={newMessage}
onChange={(e) => setNewMessage(e.target.value)}
placeholder="Type a message"
/>
);
};
export default RealTimeChat;
In this example, we establish a WebSocket connection to a server running on http://localhost:4000
. We listen for incoming messages on the newMessage
event and update the state with the new message. The sendMessage
function emits a message to the server when the user sends a new message.
Step 4: Setting Up the WebSocket Server
To handle the WebSocket connection on the server side, you can use socket.io
in a Node.js server. Here’s how you can set up a WebSocket server:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
io.on('connection', (socket) => {
console.log('New client connected');
socket.on('sendMessage', (message) => {
// Broadcast the message to all clients
io.emit('newMessage', message);
});
socket.on('disconnect', () => {
console.log('Client disconnected');
});
});
server.listen(4000, () => {
console.log('Server listening on port 4000');
});
In this example, we use socket.io
to listen for incoming connections on the server. When the server receives a sendMessage
event, it broadcasts the message to all connected clients using io.emit
. We also handle client disconnections.
Step 5: Handling Real-Time Notifications
WebSockets are often used for real-time notifications. You can implement notifications in React by listening for specific events from the server. Here's an example of how to display a notification when a new message is received:
import React, { useEffect } from 'react';
import { io } from 'socket.io-client';
const Notification = () => {
useEffect(() => {
const socket = io('http://localhost:4000');
socket.on('newMessage', (message) => {
alert(`New message received: ${message}`);
});
// Cleanup on component unmount
return () => socket.disconnect();
}, []);
return Waiting for new messages...;
};
export default Notification;
In this example, when a new message is received from the server, an alert is shown to the user. You can customize this to display notifications in the UI in a more user-friendly way, such as using a toast or modal.
Step 6: Optimizing WebSocket Connections
When working with WebSockets, it’s important to consider performance and resource management. Here are some best practices:
- Reconnect logic: Implement automatic reconnection in case the connection is lost.
- Throttling: Limit the frequency of messages sent to the server to avoid overwhelming the connection.
- Connection pooling: Reuse WebSocket connections instead of creating new ones for every event or message.
Step 7: WebSocket Security Considerations
Ensure that your WebSocket connections are secure by using wss://
(WebSocket Secure) and implementing proper authentication mechanisms. Here are some tips:
- Use
wss://
for encrypted WebSocket communication. - Authenticate users before allowing them to connect to the WebSocket server.
- Handle errors and disconnections gracefully to prevent security vulnerabilities.
Conclusion
WebSockets are a powerful tool for implementing real-time features in your React applications. By using libraries like socket.io-client
and socket.io
on the server side, you can easily add real-time functionality such as chat, notifications, and live data updates. With proper connection management and security practices, WebSockets can significantly enhance user experience in your apps.
Deploying Full-Stack Applications (Heroku, Vercel, etc.)
Deploying full-stack applications involves making both the backend and frontend available on the internet for users to interact with. In this section, we will guide you through the process of deploying a full-stack application using platforms like Heroku and Vercel.
Step 1: Preparing Your Full-Stack Application
Before deploying your full-stack application, ensure that both the backend (e.g., Node.js, Express) and the frontend (e.g., React) are properly configured. Make sure your application is production-ready by following these steps:
- Ensure that your backend server is listening on the correct port (usually 80 or 443).
- Configure environment variables for production, such as database credentials and API keys.
- Build your frontend application for production using the
npm run build
oryarn build
command.
Step 2: Deploying to Heroku
Heroku is a popular platform-as-a-service (PaaS) that makes it easy to deploy full-stack applications. Follow these steps to deploy your app to Heroku:
2.1: Create a Heroku Account
If you haven’t already, create a Heroku account at heroku.com.
2.2: Install the Heroku CLI
Install the Heroku CLI by following the instructions on the Heroku CLI documentation.
2.3: Initialize a Git Repository
Ensure your full-stack application is in a Git repository. If it’s not already initialized, run the following commands:
git init
git add .
git commit -m "Initial commit"
2.4: Create a Heroku Application
Create a new Heroku app using the following command:
heroku create my-app-name
2.5: Configure Environment Variables
Set environment variables for your application, such as the database URL or secret keys, using the following command:
heroku config:set DATABASE_URL=your-database-url
2.6: Deploy Your Application
Deploy your application to Heroku by pushing your code to the Heroku remote repository:
git push heroku master
Heroku will automatically detect the buildpack for your app (e.g., Node.js for the backend and React for the frontend) and deploy it. After deployment, you can open your app with:
heroku open
Step 3: Deploying to Vercel
Vercel is another popular platform for deploying frontend applications, especially those built with React. It also supports backend APIs for full-stack applications. Follow these steps to deploy to Vercel:
3.1: Create a Vercel Account
Create a Vercel account at vercel.com if you don’t have one already.
3.2: Install the Vercel CLI
Install the Vercel CLI by running the following command:
npm install -g vercel
3.3: Deploy the Frontend
Navigate to your frontend directory (e.g., the client
folder) and run the following command to deploy it:
vercel
Vercel will ask you some questions about the project, such as the project name and whether to link it to an existing Vercel project. Once deployed, Vercel will provide you with a URL where your frontend is live.
3.4: Deploy the Backend
If your backend is a serverless function or API, you can deploy it to Vercel as well. Just ensure that your backend code is in the api
folder of your project. Vercel automatically treats files inside the api
folder as serverless functions.
vercel --prod
This command will deploy both the frontend and backend. Your full-stack app will now be accessible via the URLs provided by Vercel.
Step 4: Managing Environment Variables in Vercel
To manage environment variables for your Vercel project, follow these steps:
- Go to your project dashboard on Vercel.
- Click on the Settings tab.
- Scroll down to the Environment Variables section.
- Add your environment variables here (e.g.,
DATABASE_URL
,SECRET_KEY
, etc.).
Step 5: Continuous Deployment with GitHub/GitLab/Bitbucket
Both Heroku and Vercel support continuous deployment from Git repositories. Here’s how you can set it up:
5.1: Heroku Continuous Deployment
To set up continuous deployment on Heroku, go to your Heroku app's dashboard and click on the Deploy tab. Connect your GitHub repository and enable automatic deployment.
5.2: Vercel Continuous Deployment
To set up continuous deployment on Vercel, go to your project’s dashboard and click on the Git tab. Connect your GitHub or GitLab repository, and Vercel will automatically deploy any changes pushed to the connected branch.
Step 6: Conclusion
Deploying full-stack applications to platforms like Heroku and Vercel allows you to easily make your app available to users. With Heroku, you can deploy traditional Node.js apps, while Vercel is perfect for static sites and serverless backends. Both platforms support continuous deployment, making it easy to keep your application up to date with the latest changes.
Building a React App for Production
When you're ready to deploy your React application to production, you need to optimize it for performance and ensure that it runs smoothly. In this section, we will guide you through the steps to build a React app for production.
Step 1: Preparing the Project
Before building the application for production, ensure that your project is properly configured:
- Ensure that all dependencies are up to date by running
npm install
oryarn install
. - Remove any unused dependencies or files.
- Ensure that your application is working in development mode before building it for production.
Step 2: Building the Application
To create a production build of your React app, use the build script provided by Create React App. Run the following command in your project’s root directory:
npm run build
This command creates a build
directory containing an optimized version of your app. The build folder will have the following optimizations:
- Minification: All JavaScript and CSS files are minified to reduce their size.
- Code Splitting: Your app’s code is split into smaller bundles, ensuring that the user only loads the necessary code.
- Optimized Assets: Images and other assets are optimized to improve load times.
- Environment Variables: Any environment-specific variables (e.g.,
process.env.NODE_ENV
) are set for production.
Step 3: Serving the Build Locally
Once the build is complete, you can serve the production version of your app locally to test it. You can use a static file server like serve> to do this:
npm install -g serve
serve -s build
This will serve the build folder on a local server (usually on http://localhost:5000
). You can test your production build here before deploying it to a live server.
Step 4: Optimizing Performance
To further optimize your React app for production, consider the following tips:
- Lazy Loading: Use React’s
React.lazy()
andSuspense
to load components only when they are needed, reducing the initial load time. - Tree Shaking: Remove unused code by ensuring that your project is properly configured to support tree shaking. This is done automatically by Create React App but can be enhanced with tools like Webpack and Babel.
- Image Optimization: Optimize images by using modern formats like WebP and ensuring that they are served in the correct sizes based on device resolutions (responsive images).
- Minimize Re-renders: Use React’s
memo
anduseMemo
hooks to prevent unnecessary re-renders and optimize performance in large apps. - Font Optimization: Use
font-display: swap
for faster font loading.
Step 5: Using Environment Variables
When deploying your app to production, you'll likely need to set different environment variables for different environments (e.g., development, staging, production). Use the REACT_APP_
prefix for environment variables in Create React App, like so:
REACT_APP_API_URL=https://api.example.com
You can create an .env
file in your project’s root directory for local development and set environment variables for different environments:
REACT_APP_API_URL=https://api.example.com
When building for production, these variables will automatically be replaced in the build output.
Step 6: Deploying the Application
Once your app is built and optimized, it's time to deploy it to a hosting provider. There are several platforms available for deploying React apps:
- Netlify: A simple and powerful platform for deploying static websites. Just connect your Git repository, and it will automatically deploy your app.
- Vercel: Another easy-to-use platform for deploying React apps, offering features like serverless functions and automatic deployments from Git.
- GitHub Pages: You can use GitHub Pages to host your React app by building it and pushing the build folder to the
gh-pages
branch. - AWS, DigitalOcean, or Heroku: For more control over your deployment, you can use services like AWS, DigitalOcean, or Heroku to host your app and API.
Step 7: Conclusion
Building a React app for production involves optimizing for performance, building the app using npm run build
, and deploying it to a hosting platform. By following these steps, you ensure that your app is fast, efficient, and ready for a production environment.
Hosting React on Static Web Hosts (Netlify, Vercel)
Once you have built your React app for production, it's time to deploy it. Static web hosts like Netlify and Vercel are popular platforms for hosting React applications. These platforms provide continuous deployment, automated builds, and globally distributed content delivery. In this section, we will guide you through the steps to host your React app on Netlify and Vercel.
Hosting on Netlify
Netlify is a platform for deploying static websites and web applications. It makes hosting a React app easy and offers features like serverless functions, continuous deployment, and SSL certificates. Here's how to host your React app on Netlify:
Step 1: Create a Netlify Account
If you don't already have a Netlify account, go to Netlify and sign up.
Step 2: Connect Your Git Repository
After logging in to Netlify, click the "New site from Git" button. Netlify supports GitHub, GitLab, and Bitbucket. Select your repository and grant Netlify access to it.
Step 3: Configure Build Settings
Once your repository is connected, you’ll be asked to configure the build settings:
- Build Command: Enter
npm run build
(oryarn build
if you're using Yarn). - Publish Directory: Enter
build
, which is the folder generated bynpm run build
.
Step 4: Deploy the App
Click "Deploy Site" and Netlify will automatically build and deploy your app. Once the deployment is complete, you will be given a URL to access your app.
Hosting on Vercel
Vercel is another powerful platform for hosting static and dynamic web applications. It integrates seamlessly with React and offers features like serverless functions and automatic optimizations. Here's how to host your React app on Vercel:
Step 1: Create a Vercel Account
If you don't already have a Vercel account, go to Vercel and sign up.
Step 2: Connect Your Git Repository
After logging in to Vercel, click on the "New Project" button. Vercel will prompt you to link your GitHub, GitLab, or Bitbucket account. Once linked, select the repository you want to deploy.
Step 3: Configure Project Settings
Vercel automatically detects that you are using React and will suggest the appropriate settings. If not, you can manually configure them:
- Framework Preset: Select "React" as the framework.
- Build Command: Vercel will automatically detect the command as
npm run build
. - Output Directory: The default output directory is
build
.
Step 4: Deploy the App
Click "Deploy" and Vercel will start building and deploying your app. Once the deployment is complete, you will be given a custom URL to access your app.
Benefits of Using Netlify and Vercel
- Continuous Deployment: Both Netlify and Vercel automatically build and deploy your app whenever changes are pushed to your Git repository.
- Global CDN: Both platforms distribute your app across a global content delivery network (CDN), ensuring fast loading times worldwide.
- Custom Domains: You can easily set up custom domains for your app with SSL certificates, improving security and branding.
- Serverless Functions: Both platforms offer serverless functions, allowing you to run backend code in response to HTTP requests without managing infrastructure.
Step 5: Managing Deployments
Both Netlify and Vercel provide easy-to-use dashboards where you can manage your deployments, view build logs, and configure environment variables. You can also roll back to previous versions of your app if necessary.
Conclusion
Hosting your React app on static web hosts like Netlify and Vercel makes the deployment process fast and seamless. These platforms offer powerful features like continuous deployment, global CDNs, and serverless functions, making them ideal for hosting React apps.
Deploying React on a Custom Server with Nginx
Deploying a React application on a custom server with Nginx is a common approach for developers who want full control over their server environment. Nginx is a powerful web server that can serve static files, reverse proxy requests, and handle a variety of other tasks. In this section, we will guide you through the steps to deploy your React app on a custom server using Nginx.
Step 1: Build Your React App
Before deploying, you need to create a production build of your React app. This can be done by running the following command:
npm run build
This will create a build
folder containing the optimized production version of your app. The files in this folder can be served by Nginx.
Step 2: Set Up Nginx on Your Server
If Nginx is not already installed on your server, you can install it using the following commands:
sudo apt update
sudo apt install nginx
Once Nginx is installed, you can start it with:
sudo systemctl start nginx
Enable Nginx to start on boot:
sudo systemctl enable nginx
Step 3: Configure Nginx to Serve Your React App
Next, you need to configure Nginx to serve the static files from your React app’s build folder. Open the default Nginx configuration file:
sudo nano /etc/nginx/sites-available/default
Modify the configuration file to point to your React app’s build folder. Update the server
block as follows:
server {
listen 80;
server_name yourdomain.com;
root /path/to/your/react/app/build;
index index.html;
location / {
try_files $uri /index.html;
}
# Additional configurations for error handling or other proxies can go here
}
In the above configuration:
- listen 80; - Ensures Nginx listens on port 80 (HTTP).
- server_name yourdomain.com; - Replace with your domain or IP address.
- root /path/to/your/react/app/build; - Points to the
build
directory of your React app. - try_files $uri /index.html; - Ensures that React Router works by serving
index.html
for all routes.
Save and exit the file, then check the Nginx configuration for syntax errors:
sudo nginx -t
If there are no errors, reload Nginx to apply the changes:
sudo systemctl reload nginx
Step 4: Set Up a Reverse Proxy (Optional)
If you are using a backend server (such as Node.js with Express) and want to set up a reverse proxy, you can add the following configuration to the Nginx server block:
location /api/ {
proxy_pass http://localhost:5000/;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
This will forward requests to /api/
to your backend server running on port 5000. You can adjust this based on your backend server setup.
Step 5: Enable SSL (Optional)
To secure your site with HTTPS, you can use Let's Encrypt to obtain a free SSL certificate. First, install Certbot:
sudo apt install certbot python3-certbot-nginx
Then, run the following command to obtain and install the SSL certificate:
sudo certbot --nginx -d yourdomain.com
Follow the prompts to configure SSL. Once completed, Certbot will automatically update your Nginx configuration to serve your site over HTTPS.
Step 6: Testing and Verifying the Deployment
To verify that your React app is being served correctly, open a browser and navigate to your server’s IP address or domain. You should see the React app running as expected. If you set up SSL, ensure the connection is secure (HTTPS).
Conclusion
Deploying a React app on a custom server with Nginx provides full control over your hosting environment. With Nginx, you can serve static files, set up reverse proxies, and configure SSL for secure connections. This approach is ideal for developers who want to manage their own server while using a powerful web server like Nginx to serve their React application.
Continuous Deployment with GitHub Actions or CircleCI
Continuous Deployment (CD) is a key practice in modern software development that automates the deployment of applications to production. By integrating with CI/CD tools like GitHub Actions or CircleCI, you can automate the process of deploying your React app to various environments every time code is pushed to your repository. In this section, we will walk through how to set up continuous deployment using GitHub Actions or CircleCI.
Continuous Deployment with GitHub Actions
GitHub Actions is a powerful CI/CD tool that allows you to automate the software development lifecycle directly within GitHub. To set up Continuous Deployment with GitHub Actions, follow these steps:
Step 1: Create a GitHub Actions Workflow
First, create a workflow file in your GitHub repository. This file will define the steps required to build, test, and deploy your React app. In your repository, create a folder called .github/workflows
and add a new file called deploy.yml
.
name: Deploy React App
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Build the app
run: npm run build
- name: Deploy to Server
run: |
scp -r ./build/* user@your-server:/path/to/your/server
ssh user@your-server 'sudo systemctl restart nginx'
This configuration will:
- Trigger the workflow when changes are pushed to the
main
branch. - Set up a Node.js environment.
- Install dependencies and build the React app.
- Deploy the app using
scp
to a remote server and restart Nginx to serve the new build.
Step 2: Set Up Secrets for SSH Access
To securely deploy your React app, you can store secrets like your SSH private key in GitHub's encrypted secrets. Go to your GitHub repository settings, then to Secrets, and add a new secret for your SSH private key (e.g., SSH_PRIVATE_KEY
).
Next, update your workflow file to use this secret:
- name: Deploy to Server
run: |
echo "${{ secrets.SSH_PRIVATE_KEY }}" > ~/.ssh/id_rsa
chmod 600 ~/.ssh/id_rsa
scp -r ./build/* user@your-server:/path/to/your/server
ssh user@your-server 'sudo systemctl restart nginx'
This ensures that your SSH private key is securely used during the deployment process.
Step 3: Test the Workflow
Once you have set up the workflow, you can test it by pushing changes to the main
branch. GitHub Actions will automatically trigger the deployment process, and you should see your React app deployed on your server.
Continuous Deployment with CircleCI
CircleCI is another popular CI/CD service that integrates well with GitHub repositories. To set up Continuous Deployment with CircleCI, follow these steps:
Step 1: Create a CircleCI Configuration File
In your GitHub repository, create a directory called .circleci
and add a configuration file called config.yml
.
version: 2.1
jobs:
build:
docker:
- image: cimg/node:14.17
steps:
- checkout
- run:
name: Install dependencies
command: npm install
- run:
name: Build the app
command: npm run build
deploy:
docker:
- image: cimg/python:3.8
steps:
- checkout
- run:
name: Deploy to Server
command: |
scp -r ./build/* user@your-server:/path/to/your/server
ssh user@your-server 'sudo systemctl restart nginx'
workflows:
version: 2
deploy:
jobs:
- build
- deploy:
requires:
- build
This configuration defines two jobs:
- build - Installs dependencies and builds the React app.
- deploy - Deploys the build to a remote server and restarts Nginx.
Step 2: Set Up SSH Keys for CircleCI
Like with GitHub Actions, you will need to store SSH private keys in CircleCI's environment variables for secure deployment. Go to your CircleCI project settings and add the SSH private key as an environment variable.
In the config file, use this environment variable to deploy securely:
- run:
name: Deploy to Server
command: |
echo "${SSH_PRIVATE_KEY}" > ~/.ssh/id_rsa
chmod 600 ~/.ssh/id_rsa
scp -r ./build/* user@your-server:/path/to/your/server
ssh user@your-server 'sudo systemctl restart nginx'
Step 3: Trigger the Workflow
When you push changes to the GitHub repository, CircleCI will automatically trigger the workflow. The app will be built and deployed to the server as part of the continuous deployment process.
Conclusion
Using GitHub Actions or CircleCI for Continuous Deployment automates the process of deploying your React app to production every time you push changes. This reduces manual intervention, increases deployment frequency, and ensures that your app is always up-to-date. Both GitHub Actions and CircleCI provide robust CI/CD pipelines that you can customize to fit your deployment needs.
Optimizing React for Production (Tree Shaking, Minification)
Optimizing your React app for production is crucial to enhance performance, reduce load times, and improve the overall user experience. Two key techniques for optimizing React applications are Tree Shaking and Minification. In this section, we will explore these techniques and how to implement them in your React project.
What is Tree Shaking?
Tree Shaking is a term commonly used in JavaScript to describe the process of removing unused code from your application. In modern JavaScript bundlers like Webpack, Tree Shaking analyzes the import/export statements and eliminates code that is not used in your app, reducing the final bundle size.
How to Enable Tree Shaking in React
Tree Shaking is enabled by default when you create a React app using create-react-app
with the production build configuration. This is because Webpack, which is used by create-react-app
, automatically performs Tree Shaking during the build process.
However, you can ensure that Tree Shaking is working effectively by following these best practices:
- Use ES6 modules: Tree Shaking works best with ES6
import
andexport
syntax. Avoid using CommonJSrequire
statements, as they are not tree-shakable. - Remove unused imports: Ensure that unused imports are removed from your React components to reduce the final bundle size.
What is Minification?
Minification is the process of removing unnecessary characters from your code (such as whitespace, comments, and newline characters) to reduce the file size. This helps improve the performance of your app by reducing the amount of JavaScript that needs to be transferred over the network.
How to Enable Minification in React
Minification is automatically enabled when you build your React app for production using create-react-app
. The production build process uses terser
, a JavaScript minifier, to minify your code.
To create a minified version of your React app, run the following command:
npm run build
This command will create a build
directory with minified and optimized files for production.
Best Practices for Optimizing React Apps for Production
In addition to Tree Shaking and Minification, here are some other best practices to optimize your React app for production:
- Code Splitting: Code splitting allows you to split your app into smaller bundles that can be loaded on-demand. This reduces the initial load time of your app. You can use React’s built-in
React.lazy
andSuspense
for code splitting.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => (
<Suspense fallback={Loading...}>
);
export default App;
npm run build
and not the development build. The production build performs optimizations like minification, Tree Shaking, and more.ImageOptim
and TinyPNG
can help with image optimization.<link rel="preload">
to preload important assets like fonts, CSS, or JavaScript files that are essential for the initial rendering of the page.Conclusion
Optimizing your React app for production is essential to ensure it performs well in real-world scenarios. By implementing Tree Shaking, Minification, and other best practices like code splitting and image optimization, you can reduce bundle sizes, improve load times, and provide a better user experience.
Building a To-Do List App
In this section, we will guide you through building a simple To-Do List app using React. The app will allow users to add tasks, mark tasks as completed, and delete tasks. This is a great way to get hands-on experience with React's core concepts such as state management, handling user input, and component reusability.
Step 1: Setting Up the Project
First, create a new React app using create-react-app
:
npx create-react-app todo-app
After the project is created, navigate into the project directory:
cd todo-app
Step 2: Create the To-Do List Component
We will create a TodoList
component to display the tasks. In this component, we'll map over the tasks array and display each task along with a checkbox to mark it as completed, and a delete button to remove the task.
import React, { useState } from 'react';
const TodoList = () => {
const [tasks, setTasks] = useState([]);
const [taskInput, setTaskInput] = useState('');
const addTask = () => {
if (taskInput) {
setTasks([...tasks, { text: taskInput, completed: false }]);
setTaskInput('');
}
};
const toggleTask = (index) => {
const updatedTasks = [...tasks];
updatedTasks[index].completed = !updatedTasks[index].completed;
setTasks(updatedTasks);
};
const deleteTask = (index) => {
const updatedTasks = tasks.filter((_, i) => i !== index);
setTasks(updatedTasks);
};
return (
<input
type="text"
value={taskInput}
onChange={(e) => setTaskInput(e.target.value)}
placeholder="Enter a task"
/>
{tasks.map((task, index) => (
-
<input
type="checkbox"
checked={task.completed}
onChange={() => toggleTask(index)}
/>
<span style={{ textDecoration: task.completed ? 'line-through' : 'none' }}>
{task.text}
<button onClick={() => deleteTask()} >Delete</button>
))}
);
};
export default TodoList;
Step 3: Handling User Input
In the code above, we use the useState
hook to manage the state of the tasks and the input field. When the user types in the input field, the onChange
event updates the taskInput
state. When the user clicks the "Add Task" button, the addTask
function adds the new task to the tasks
array.
Step 4: Marking Tasks as Completed
The checkbox is used to mark tasks as completed. When a user clicks the checkbox, the toggleTask
function is triggered. This function toggles the completed
property of the task, and React re-renders the component to reflect the change. We also conditionally apply a line-through style to the task's text if it is completed.
Step 5: Deleting Tasks
The "Delete" button next to each task allows users to remove tasks from the list. The deleteTask
function uses the filter
method to create a new array without the deleted task, and updates the state with the new array of tasks.
Step 6: Putting It All Together
Now that we have the core functionality, let’s integrate everything into the App
component:
import React from 'react';
import TodoList from './TodoList';
const App = () => {
return (
To-Do List
);
};
export default App;
Step 7: Styling the App
Now that we have the basic functionality, you can add some styles to make the app more visually appealing. For example, you can add the following CSS in your App.css
file:
ul {
list-style-type: none;
padding: 0;
}
li {
display: flex;
align-items: center;
justify-content: space-between;
margin: 10px 0;
}
button {
margin-left: 10px;
}
input[type="text"] {
padding: 5px;
margin-right: 10px;
}
Conclusion
Congratulations! You've just built a simple To-Do List app using React. This app allows users to add tasks, mark them as completed, and delete them. This project demonstrates the core principles of React, such as state management, event handling, and component reusability. You can now expand on this app by adding features like task persistence (e.g., using localStorage), task prioritization, or even task categories.
Building a Weather App with an API
In this section, we will build a simple weather app using React that fetches weather data from a public API. The app will allow users to check the weather of any city by entering a city name. This project will help you practice fetching data from an API, managing state, and displaying dynamic content in React.
Step 1: Set Up the Project
Create a new React app using create-react-app
:
npx create-react-app weather-app
After the project is created, navigate into the project directory:
cd weather-app
Step 2: Install Axios for API Requests
We'll use Axios
to make HTTP requests to the weather API. Install Axios by running the following command:
npm install axios
Step 3: Get an API Key
To fetch weather data, we'll use the OpenWeatherMap API. First, sign up at OpenWeatherMap and get an API key.
Step 4: Create the Weather App Component
Now let's create a basic weather app. In the App.js
file, we will create a form to enter the city name and display the weather data.
import React, { useState } from 'react';
import axios from 'axios';
const WeatherApp = () => {
const [city, setCity] = useState('');
const [weather, setWeather] = useState(null);
const [error, setError] = useState('');
const apiKey = 'YOUR_API_KEY'; // Replace with your OpenWeatherMap API key
const handleSubmit = async (e) => {
e.preventDefault();
setError('');
try {
const response = await axios.get(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`);
setWeather(response.data);
} catch (error) {
setError('City not found. Please try again.');
}
};
return (
Weather App
{error && {error}
}
{weather && (
{weather.name}
Temperature: {weather.main.temp}°C
Weather: {weather.weather[0].description}
)}
);
};
export default WeatherApp;
Step 5: Explanation of the Code
In the code above, we use the useState
hook to manage the city name, weather data, and error messages. The handleSubmit
function is triggered when the user submits the form. It makes an API request to OpenWeatherMap using Axios, and if successful, stores the weather data in the weather
state. If there is an error (e.g., the city is not found), the error message is displayed.
Step 6: Styling the App
You can add some basic styles to make the weather app more presentable. For example, in your App.css
file, you can add the following CSS:
form {
margin-bottom: 20px;
}
input {
padding: 8px;
margin-right: 10px;
}
button {
padding: 8px 12px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
h2 {
margin-top: 20px;
}
Step 7: Running the App
Now that we have the basic functionality and styling, you can start the development server by running the following command:
npm start
The app should now be running, and you can enter a city name to see the weather forecast!
Step 8: Enhancements and Features
Once the basic app is working, you can enhance it further by adding additional features such as:
- Displaying additional weather data (e.g., humidity, wind speed, etc.).
- Allowing users to search for weather forecasts of multiple cities.
- Adding weather icons and animations based on the weather conditions.
- Saving recent search results locally using
localStorage
.
Conclusion
In this tutorial, we built a simple weather app using React and Axios to fetch data from the OpenWeatherMap API. We learned how to manage state, handle form submissions, and display dynamic weather information. This is a great starting point, and you can continue to add more features and functionality to your app!
Real-Time Chat App with WebSocket
In this section, we will build a real-time chat application using WebSocket. WebSocket allows for two-way communication between the client and the server over a single, long-lived connection, which is perfect for real-time applications like chat apps.
Step 1: Set Up the Project
Create a new React app using create-react-app
:
npx create-react-app chat-app
Navigate into the project directory:
cd chat-app
Step 2: Install Dependencies
We will need the socket.io-client
library on the client side to connect to our WebSocket server. Install it by running:
npm install socket.io-client
Step 3: Setting Up the WebSocket Server
For the backend, we’ll use express
and socket.io
to create the WebSocket server. Create a new server
folder inside the project directory.
mkdir server
cd server
npm init -y
npm install express socket.io
Next, create the server.js
file in the server
folder:
const express = require('express');
const socketIo = require('socket.io');
const http = require('http');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
app.get('/', (req, res) => {
res.send('Server is running');
});
io.on('connection', (socket) => {
console.log('New client connected');
socket.on('disconnect', () => {
console.log('Client disconnected');
});
socket.on('message', (message) => {
io.emit('message', message); // Broadcast the message to all clients
});
});
server.listen(4000, () => {
console.log('Server running on port 4000');
});
This WebSocket server listens for incoming connections on port 4000. When a client sends a message, it broadcasts the message to all connected clients.
Step 4: Implement the Chat UI in React
In the React app, we will create a simple chat interface with a list of messages and an input field to send new messages. In the src/App.js
file, add the following code:
import React, { useState, useEffect } from 'react';
import io from 'socket.io-client';
const socket = io('http://localhost:4000');
const ChatApp = () => {
const [message, setMessage] = useState('');
const [messages, setMessages] = useState([]);
useEffect(() => {
socket.on('message', (message) => {
setMessages((prevMessages) => [...prevMessages, message]);
});
return () => {
socket.off('message');
};
}, []);
const handleSubmit = (e) => {
e.preventDefault();
if (message.trim()) {
socket.emit('message', message);
setMessage('');
}
};
return (
Real-Time Chat
{messages.map((msg, index) => (
{msg}
))}
);
};
export default ChatApp;
In the code above:
- We initialize a socket connection to the server using
socket.io-client
. - We listen for incoming messages with
socket.on('message', ...)
and append them to themessages
state. - When the user submits a new message, we emit the message to the server using
socket.emit('message', message)
.
Step 5: Run the Server and Client
Now that both the client and server are set up, we need to run the server and client. In the server
folder, run:
node server.js
Then, in the client folder, run:
npm start
Now you should be able to open your chat app in the browser and start sending messages. If you open the app in multiple browser windows or tabs, you will see the messages in real time.
Step 6: Deploying the App
You can deploy the client-side React app to services like Netlify or Vercel. The WebSocket server can be deployed on services like Heroku or your own server.
Conclusion
In this tutorial, we built a real-time chat app using WebSockets. We set up a WebSocket server with socket.io
, connected to it from a React app using socket.io-client
, and implemented real-time messaging. This is a powerful way to add live features to your applications!
Blog Application with React and Node.js
In this section, we will build a simple blog application that allows users to create, read, update, and delete posts. The frontend will be built using React, and the backend will be powered by Node.js and Express. We will also use MongoDB to store the blog posts.
Step 1: Set Up the Project
First, create a new directory for the project and set up both the backend and frontend.
mkdir blog-app
cd blog-app
Inside the blog-app
directory, create two subdirectories: client
for the React frontend and server
for the Node.js backend.
mkdir client server
Step 2: Set Up the Backend (Node.js and Express)
Navigate to the server
directory and initialize a new Node.js project:
cd server
npm init -y
Then, install the required dependencies:
npm install express mongoose cors
Now, create a server.js
file in the server
directory:
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const app = express();
app.use(cors());
app.use(express.json());
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/blog', {
useNewUrlParser: true,
useUnifiedTopology: true,
}).then(() => {
console.log('Connected to MongoDB');
}).catch((err) => {
console.log('Error connecting to MongoDB:', err);
});
// Define a Blog Post model
const Post = mongoose.model('Post', new mongoose.Schema({
title: String,
content: String,
}));
// API routes
app.get('/api/posts', async (req, res) => {
const posts = await Post.find();
res.json(posts);
});
app.post('/api/posts', async (req, res) => {
const { title, content } = req.body;
const post = new Post({ title, content });
await post.save();
res.status(201).json(post);
});
app.put('/api/posts/:id', async (req, res) => {
const { title, content } = req.body;
const post = await Post.findByIdAndUpdate(req.params.id, { title, content }, { new: true });
res.json(post);
});
app.delete('/api/posts/:id', async (req, res) => {
await Post.findByIdAndDelete(req.params.id);
res.status(204).end();
});
// Start server
const port = 5000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
This server connects to a MongoDB database, defines a Post
model for blog posts, and provides routes for CRUD operations (Create, Read, Update, Delete) on blog posts.
Step 3: Set Up the Frontend (React)
Now, navigate to the client
directory and create a new React app:
cd ../client
npx create-react-app .
Once the React app is set up, install axios
to make HTTP requests to the backend:
npm install axios
In the src/App.js
file, add the following code to display and manage the blog posts:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const App = () => {
const [posts, setPosts] = useState([]);
const [newPost, setNewPost] = useState({ title: '', content: '' });
useEffect(() => {
axios.get('http://localhost:5000/api/posts')
.then((response) => {
setPosts(response.data);
})
.catch((error) => {
console.error('Error fetching posts:', error);
});
}, []);
const handleSubmit = (e) => {
e.preventDefault();
axios.post('http://localhost:5000/api/posts', newPost)
.then((response) => {
setPosts([...posts, response.data]);
setNewPost({ title: '', content: '' });
})
.catch((error) => {
console.error('Error creating post:', error);
});
};
return (
Blog Application
{posts.map((post) => (
-
{post.title}
{post.content}
))}
);
};
export default App;
This React app fetches the list of blog posts from the backend and displays them. Users can also create new posts by submitting the form.
Step 4: Run the Server and Client
To run the backend server, navigate to the server
directory and run:
node server.js
To run the React frontend, navigate to the client
directory and run:
npm start
Now, your blog application should be up and running. You can create new posts and view them in real-time.
Step 5: Deploying the Application
You can deploy the frontend React app to services like Netlify or Vercel, and the backend to services like Heroku or your own server. Make sure to configure the proper environment variables and update the API URLs accordingly.
Conclusion
In this tutorial, we have created a simple blog application with React, Node.js, and MongoDB. We set up the backend with Express and MongoDB, built the frontend with React, and connected the two with HTTP requests using axios
. This is a basic full-stack application that you can expand upon by adding features like authentication, comments, and more!
E-Commerce Application with React and Redux
In this section, we will build a simple e-commerce application where users can browse products, add them to a shopping cart, and complete a checkout process. The frontend will be built using React and managed with Redux for state management.
Step 1: Set Up the Project
First, create a new React project using Create React App:
npx create-react-app e-commerce-app
cd e-commerce-app
Then, install the necessary dependencies for Redux and React-Redux:
npm install redux react-redux
Step 2: Set Up Redux for State Management
Next, we will create a Redux store to manage the application state. Create a new directory called redux
inside the src
folder. Inside this directory, create two files: actions.js
and reducers.js
.
Creating the Actions
In src/redux/actions.js
, define the action types and action creators for adding and removing items from the cart:
export const ADD_TO_CART = 'ADD_TO_CART';
export const REMOVE_FROM_CART = 'REMOVE_FROM_CART';
export const addToCart = (product) => ({
type: ADD_TO_CART,
payload: product,
});
export const removeFromCart = (productId) => ({
type: REMOVE_FROM_CART,
payload: productId,
});
Creating the Reducers
In src/redux/reducers.js
, define the reducer for managing the cart state:
import { ADD_TO_CART, REMOVE_FROM_CART } from './actions';
const initialState = {
cart: [],
};
const cartReducer = (state = initialState, action) => {
switch (action.type) {
case ADD_TO_CART:
return { ...state, cart: [...state.cart, action.payload] };
case REMOVE_FROM_CART:
return { ...state, cart: state.cart.filter(item => item.id !== action.payload) };
default:
return state;
}
};
export default cartReducer;
Setting Up the Store
In src/redux/store.js
, configure the Redux store:
import { createStore } from 'redux';
import cartReducer from './reducers';
const store = createStore(cartReducer);
export default store;
Step 3: Connect Redux to the React Application
In the src/index.js
file, wrap the app with the Provider
component from react-redux
to make the Redux store available to the entire app:
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import './index.css';
import App from './App';
import store from './redux/store';
ReactDOM.render(
,
document.getElementById('root')
);
Step 4: Creating the UI Components
Next, we will create the UI components for displaying products, adding items to the cart, and displaying the shopping cart.
Product List Component
In src/components/ProductList.js
, create a component to display the list of products:
import React from 'react';
import { useDispatch } from 'react-redux';
import { addToCart } from '../redux/actions';
const ProductList = ({ products }) => {
const dispatch = useDispatch();
const handleAddToCart = (product) => {
dispatch(addToCart(product));
};
return (
Products
{products.map((product) => (
{product.name}
{product.description}
${product.price}
<button onClick={() => handleAddToCart(product)}>Add to Cart</button>
))}
);
};
export default ProductList;
Shopping Cart Component
In src/components/ShoppingCart.js
, create a component to display the items in the cart:
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { removeFromCart } from '../redux/actions';
const ShoppingCart = () => {
const cart = useSelector((state) => state.cart);
const dispatch = useDispatch();
const handleRemoveFromCart = (productId) => {
dispatch(removeFromCart(productId));
};
return (
Shopping Cart
{cart.length === 0 ? (
Your cart is empty
) : (
{cart.map((item) => (
-
{item.name}
{item.description}
${item.price}
<button onClick={() => handleRemoveFromCart(item.id)}>Remove</button>
))}
)}
);
};
export default ShoppingCart;
Step 5: Add Products and Render Components
In the src/App.js
file, define a list of products and render the ProductList
and ShoppingCart
components:
import React from 'react';
import ProductList from './components/ProductList';
import ShoppingCart from './components/ShoppingCart';
const products = [
{ id: 1, name: 'Product 1', description: 'Description for product 1', price: 100 },
{ id: 2, name: 'Product 2', description: 'Description for product 2', price: 150 },
{ id: 3, name: 'Product 3', description: 'Description for product 3', price: 200 },
];
const App = () => {
return (
Welcome to the E-Commerce App
);
};
export default App;
Step 6: Run the Application
To run the React application, use the following command:
npm start
The application should display a list of products and allow users to add them to the shopping cart. The cart will also display the added items with the option to remove them.
Step 7: Deploying the Application
You can deploy the frontend React app to services like Netlify or Vercel, and the backend (if you add one) to services like Heroku or your own server. Make sure to configure the proper environment variables and update the API URLs accordingly.
Conclusion
In this tutorial, we've built a simple e-commerce app with React and Redux. The app allows users to view products, add them to the cart, and manage the cart items. Using Redux, we've managed the state of the cart across the app. This is a basic e-commerce app that you can expand by adding features like user authentication, product categories, and a checkout process.
Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for any software project, especially when working in teams or on long-term projects. In this section, we will explore key principles and best practices for writing code that is easy to read, understand, and modify.
1. Follow Consistent Naming Conventions
One of the most important aspects of clean code is using clear and consistent naming conventions. This helps others (and your future self) understand the purpose of variables, functions, and components at a glance.
- Use descriptive names: Avoid single-letter variables like
x
ory
. Instead, use names likeuserAge
,transactionAmount
, orisLoggedIn
. - Use camelCase for variable and function names in JavaScript:
userProfile
,getUserDetails()
. - Use PascalCase for React component names:
App
,UserCard
.
2. Keep Functions Small and Focused
Functions should be small and focused on one thing. If a function is doing too much, it becomes harder to understand, test, and maintain.
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
In the above example, the function calculateTotal
has a single responsibility: calculating the total price of the items. Keeping functions small also makes them easier to test and debug.
3. Use Comments Wisely
While clean code should be self-explanatory, there are times when a well-placed comment can provide additional context or explain why something is done in a particular way.
- Write comments to explain complex logic or decisions that may not be immediately clear.
- Avoid obvious comments that state the obvious, like
// Incrementing the counter
above an increment operation. - Keep comments up-to-date. Outdated comments can be more harmful than no comments at all.
// This function calculates the total by adding up the prices of all items in the cart
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
4. Avoid Hardcoding Values
Hardcoding values in your code can make it difficult to change and maintain. Instead, use constants or configuration files to store values that may change over time or vary across environments.
const TAX_RATE = 0.07;
function calculateTotal(items) {
const subtotal = items.reduce((total, item) => total + item.price, 0);
return subtotal + (subtotal * TAX_RATE);
}
Here, the TAX_RATE
constant is used instead of hardcoding the tax rate throughout the code, making it easier to modify in the future.
5. Use Descriptive and Consistent Formatting
Consistent formatting helps improve readability and ensures that your code looks uniform across the project. Use a linter or code formatter to enforce consistent formatting rules such as indentation, spaces around operators, and placement of curly braces.
// Good formatting:
function getUserProfile(userId) {
return fetch(`/api/user/${userId}`)
.then(response => response.json())
.then(data => data);
}
In the example above, the code uses consistent indentation and clear formatting, making it easy to understand the structure of the function.
6. DRY Principle (Don't Repeat Yourself)
The DRY principle emphasizes avoiding repetition in your code. When you notice that the same code is being repeated multiple times, it might be a good idea to refactor that code into a reusable function or component.
// Without DRY:
function calculateSubtotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
function calculateTax(items) {
return items.reduce((total, item) => total + item.price, 0) * 0.07;
}
// Applying DRY:
function calculateTotal(items) {
const subtotal = items.reduce((total, item) => total + item.price, 0);
const tax = subtotal * 0.07;
return { subtotal, tax, total: subtotal + tax };
}
By refactoring the two separate functions into one, the code is now more efficient and maintainable.
7. Organize Your Files and Directories
As your project grows, organizing your code into logical modules and directories will help make it easier to maintain. A well-structured project encourages scalability and helps new developers navigate the codebase quickly.
src/
|-- components/
| |-- Header.js
| |-- Footer.js
|-- pages/
| |-- Home.js
|-- services/
| |-- api.js
|-- App.js
|-- index.js
In the above directory structure, each component, page, and service is placed in its respective folder, making the project easy to manage.
8. Write Tests for Your Code
Writing tests for your code ensures that it behaves as expected and helps catch bugs early. It also makes it easier to refactor code in the future since you can run the tests to verify the changes.
import { render, screen } from '@testing-library/react';
import App from './App';
test('renders learn react link', () => {
render( );
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
In the example above, a simple test is written using @testing-library/react
to check if the "learn react" link appears on the screen.
Conclusion
Writing clean and maintainable code is crucial for the success of any software project. By following best practices such as consistent naming, keeping functions small, avoiding repetition, and organizing your code, you can ensure that your codebase is easy to understand, modify, and scale. Additionally, writing tests and documenting complex logic will help improve the long-term maintainability of your application.
Folder Structure and File Organization
Proper folder structure and file organization are essential for maintainable and scalable software projects. A well-organized codebase helps improve collaboration, debugging, and scalability. In this section, we'll discuss best practices for organizing your project files.
1. Use a Modular Approach
Divide your project into logical modules, each focused on a specific feature or functionality. This makes it easier to manage and scale the project, especially as it grows.
src/
|-- components/
|-- pages/
|-- services/
|-- assets/
|-- utils/
In this example, each folder is dedicated to a specific concern:
- components/: Contains reusable UI components like buttons, headers, footers, etc.
- pages/: Contains components representing full pages or views, such as Home, About, etc.
- services/: Contains logic for interacting with external APIs or databases.
- assets/: Stores images, fonts, and other static files.
- utils/: Contains utility functions or helper functions used across the project.
2. Group by Feature (Feature-Based Structure)
Rather than organizing files by type (e.g., separating components, services, etc.), group them by feature. This is especially useful for large projects with multiple features.
src/
|-- home/
| |-- components/
| |-- services/
| |-- pages/
|-- user/
| |-- components/
| |-- services/
| |-- pages/
This structure groups everything related to a specific feature into its own folder, making it easier to locate and manage the code for that feature.
3. Use Meaningful Names for Files and Folders
Names should be descriptive and clearly convey the purpose of the file or folder. Avoid using vague names like temp.js
or testFile.js
.
src/
|-- components/
| |-- Button.js
| |-- Navbar.js
|-- services/
| |-- api.js
| |-- auth.js
In this example, the names clearly indicate the purpose of each component and service, making it easy to understand their function in the project.
4. Separate Configuration Files
Keep configuration files such as environment variables, build configurations, and third-party integrations separate from your main codebase. Store them in a dedicated folder like config/
or env/
.
config/
|-- apiConfig.js
|-- authConfig.js
This keeps your project organized and ensures that configuration files are easy to locate and modify when necessary.
5. Use Index Files for Simplified Imports
Use index.js
files inside folders to simplify imports and reduce the need for long relative paths. This is especially useful for modular components or services.
// In src/components/index.js
export { default as Button } from './Button';
export { default as Navbar } from './Navbar';
// In another file
import { Button, Navbar } from './components';
This allows you to import components or services from a folder in a cleaner, more concise way.
6. Keep Styles and Test Files Together
For consistency and easier maintenance, consider keeping styles and test files alongside the components they belong to. This reduces the need to navigate between multiple folders to find related files.
src/
|-- components/
| |-- Button/
| |-- Button.js
| |-- Button.css
| |-- Button.test.js
By grouping related files together, you can avoid confusion and keep everything in one place for easier access and modification.
7. Avoid Deep Nesting
Avoid deeply nesting files and folders. Deep nesting can make the project harder to navigate and understand. Keep the folder structure as flat as possible while still maintaining logical organization.
src/
|-- components/
|-- pages/
|-- services/
In this example, the folder structure is kept relatively flat, with only a few levels of nesting for each feature.
8. Use Aliases for Simpler Imports
As your project grows, relative imports can become cumbersome. Setting up path aliases allows you to simplify imports and avoid long relative paths.
// In tsconfig.json or jsconfig.json
{
"compilerOptions": {
"baseUrl": "src",
"paths": {
"@components/*": ["components/*"],
"@services/*": ["services/*"]
}
}
}
With path aliases, you can import files like so:
import { Button } from '@components/Button';
import { api } from '@services/api';
9. Document the Folder Structure
Documenting your folder structure helps new developers or collaborators understand how the project is organized. Include a README file that explains the folder structure and the purpose of each directory.
# Folder Structure
- src/
contains all source files
- components/
contains reusable UI components
- pages/
contains page components
- services/
contains API and business logic
- assets/
contains static files like images and fonts
- utils/
contains utility functions
Including this documentation helps ensure that everyone is on the same page about the project structure.
Conclusion
Maintaining a clean, organized folder structure is vital for the success of any software project. By following best practices such as using a modular approach, grouping files by feature, and avoiding deep nesting, you can create a project that is easy to navigate, maintain, and scale. Additionally, using path aliases, simplifying imports, and documenting your folder structure will improve collaboration and long-term maintainability.
Code Splitting and Lazy Loading
Code splitting and lazy loading are techniques used to optimize the performance of web applications by loading only the necessary code when required. These techniques can help reduce the initial load time and improve the overall user experience. In this section, we will explore code splitting and lazy loading in React.
1. What is Code Splitting?
Code splitting refers to the practice of dividing your codebase into smaller bundles, which are loaded as needed. This helps reduce the initial load time by ensuring that only the code required for the current view is loaded, rather than the entire application.
React provides built-in support for code splitting using React.lazy()
and Suspense
. With code splitting, each route or component can be loaded on demand, only when it's needed.
2. Setting Up Code Splitting in React
In React, you can easily implement code splitting by using React.lazy()
to dynamically import components. Here's how to set it up:
import React, { Suspense } from 'react';
// Use React.lazy to load the component only when it's required
const MyComponent = React.lazy(() => import('./MyComponent'));
function App() {
return (
Code Splitting with React
<Suspense fallback={Loading...}>
{/* MyComponent will only be loaded when required */}
);
}
export default App;
In this example, the MyComponent
is loaded only when it is rendered in the app. The Suspense
component is used to show a fallback UI (e.g., "Loading...") while the component is being loaded.
3. What is Lazy Loading?
Lazy loading is a technique used to delay the loading of non-essential resources until they are actually needed. With lazy loading, components or routes are only loaded when they are about to be rendered, which can significantly improve the initial loading time of your application.
In React, lazy loading is commonly implemented using React.lazy()
in combination with Suspense
.
4. Implementing Lazy Loading for Routes
In a React app with routing (e.g., using react-router-dom
), you can implement lazy loading for routes to load components only when the user navigates to them. Here’s how to set it up:
import React, { Suspense } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
// Lazy load components
const Home = React.lazy(() => import('./Home'));
const About = React.lazy(() => import('./About'));
function App() {
return (
<Suspense fallback={Loading...}>
);
}
export default App;
In this example, the Home
and About
components are loaded only when the user navigates to their respective routes. The Suspense
component shows the fallback UI while the components are loading.
5. Benefits of Code Splitting and Lazy Loading
- Improved Performance: Code splitting reduces the initial bundle size, which improves load times and overall performance, especially for large applications.
- Faster Initial Load: By loading only the necessary code at the beginning, the app becomes more responsive, providing a better user experience.
- On-Demand Loading: Code is loaded only when needed, which means that users only pay the cost of downloading code for what they are currently using.
- Smaller Bundles: Code splitting helps keep bundle sizes small, reducing the number of resources the browser needs to download.
6. Best Practices for Code Splitting
Here are some best practices to keep in mind when implementing code splitting:
- Split by Route: The most common and effective way to split code is by route. This ensures that each page loads only the necessary code for that page.
- Split by Component: For larger applications, you can also split by component, ensuring that components are loaded only when required.
- Use
Suspense
for Fallbacks: Always useSuspense
to display a loading state while the component is being fetched. - Avoid Over-Splitting: While code splitting is beneficial, over-splitting your code can lead to too many network requests and impact performance. Be mindful of how you split your code.
7. Tools for Code Splitting
React’s built-in React.lazy()
and Suspense
components work well for most use cases, but there are additional tools and libraries that can help optimize code splitting further:
- React Loadable: A higher-level API for code splitting that provides additional features, such as loading states and error handling.
- Webpack: Webpack, the bundler used by Create React App, also supports advanced code splitting strategies such as splitting vendor libraries, dynamic imports, and more.
8. Conclusion
Code splitting and lazy loading are powerful techniques for improving the performance of your React applications. By loading code only when it's needed, you can reduce initial load times and improve the user experience. By following best practices and using tools like React.lazy()
and Suspense
, you can efficiently manage code splitting in your React app.
Handling Errors Gracefully in React
Handling errors gracefully in React is essential for creating a smooth user experience. Whether it’s a network request failure or unexpected behavior in the UI, handling errors effectively can prevent crashes and provide users with helpful feedback. In this section, we will explore strategies for handling errors in React applications.
1. Using try...catch
for Synchronous Errors
For synchronous errors, you can use a try...catch
block to handle exceptions. This is useful when you expect some code to throw an error, such as invalid inputs or unexpected conditions in your functions.
function handleSubmit(data) {
try {
// Some logic that might throw an error
if (!data) throw new Error('Data is required');
console.log('Form submitted with data:', data);
} catch (error) {
console.error('Error occurred:', error.message);
}
}
In this example, if data
is not provided, an error is thrown and caught within the catch
block. This allows you to handle the error gracefully without crashing the app.
2. Using Error Boundaries for Uncaught Errors in Components
React provides a feature called Error Boundaries
that allows you to catch errors in any part of the component tree and display a fallback UI. This is especially useful for handling errors that may occur during rendering, in lifecycle methods, or in constructors of class components.
To create an error boundary, you need to define a class component that implements componentDidCatch
and getDerivedStateFromError
lifecycle methods.
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false, errorMessage: '' };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, info) {
this.setState({ errorMessage: error.message });
console.error('Error caught in error boundary:', error, info);
}
render() {
if (this.state.hasError) {
return Something went wrong: {this.state.errorMessage}
;
}
return this.props.children;
}
}
export default ErrorBoundary;
Now, you can wrap your components with the ErrorBoundary
to catch any errors they might throw and display a fallback UI:
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
import MyComponent from './MyComponent';
function App() {
return (
);
}
export default App;
If an error occurs in MyComponent
, the ErrorBoundary
will catch it and render a fallback UI instead of crashing the whole app.
3. Handling Errors in Asynchronous Code (Promises)
For asynchronous operations like fetching data from an API, you can handle errors using try...catch
inside an async
function or by using .catch()
on promises.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) throw new Error('Failed to fetch data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error.message);
}
}
In this example, if the fetch operation fails (e.g., due to a network issue or an invalid response), the error is caught and logged in the catch
block.
4. Displaying Error Messages in the UI
When an error occurs, you may want to show an error message to the user instead of just logging it to the console. You can maintain an error state in your component and display the error message conditionally in the UI.
import React, { useState, useEffect } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) throw new Error('Failed to fetch data');
const result = await response.json();
setData(result);
} catch (error) {
setError(error.message);
}
};
fetchData();
}, []);
if (error) {
return Error: {error};
}
return Data: {data ? JSON.stringify(data) : 'Loading...'};
}
export default MyComponent;
In this example, if the fetch operation fails, the error message is stored in the error
state and rendered in the UI. Otherwise, the data is shown or a loading message is displayed.
5. Using a Global Error Handler
In larger applications, it may be useful to have a global error handler to catch unexpected errors across the entire app. This can be achieved by using window.onerror
for unhandled JavaScript errors or window.addEventListener('unhandledrejection')
for unhandled promise rejections.
window.onerror = function (message, source, lineno, colno, error) {
console.error('Global error handler:', message, source, lineno, colno, error);
return true; // Prevents the default browser error handling
};
window.addEventListener('unhandledrejection', function (event) {
console.error('Unhandled promise rejection:', event.reason);
});
This approach provides a catch-all mechanism for handling errors that are not caught by other error boundaries or try...catch
blocks.
6. Conclusion
Gracefully handling errors is essential for building a robust and user-friendly React application. By using techniques like error boundaries, try...catch
, displaying helpful UI messages, and implementing global error handlers, you can prevent crashes and provide a better user experience. Always ensure that your app responds to errors in a way that is clear, informative, and non-disruptive to the user.
Version Control Best Practices (Git, GitHub)
Version control is an essential part of modern software development, allowing developers to track changes, collaborate with others, and maintain a history of their codebase. Git is one of the most popular version control systems, and GitHub is a widely used platform for hosting Git repositories. In this section, we’ll explore best practices for using Git and GitHub to manage your projects effectively.
1. Commit Often and with Meaningful Messages
Commits should be frequent and meaningful. Each commit should represent a logical unit of work, making it easy to understand what was changed and why. A commit message should be concise but descriptive enough to explain the change.
git commit -m "Fix bug in user authentication flow"
Good commit messages follow a convention like:
- Short and to the point: A brief summary of what was changed (around 50 characters).
- Use the imperative mood: "Fix bug" rather than "Fixed bug" (this makes the message sound like a command).
- Why and how: Optionally, include more details about why the change was made or how it was implemented, especially for complex changes.
2. Create Feature Branches
It’s a good practice to create a new branch for each feature or bug fix rather than working directly on the main
branch. This keeps the main
branch stable and allows you to work on multiple features in parallel without interfering with each other.
git checkout -b feature/login-form-validation
After completing the work, merge the feature branch back into the main
branch via a pull request (PR) to keep the history clean and easy to manage.
3. Keep Your Commit History Clean
Keep your commit history clean by avoiding unnecessary or "work-in-progress" commits. Before pushing your changes, it’s a good idea to squash commits that are small, unrelated to the feature, or just fixing minor issues.
git rebase -i HEAD~5 # Squash the last 5 commits
This ensures that the commit history remains concise and meaningful, and it also prevents the repository from becoming cluttered with irrelevant commits.
4. Use .gitignore to Exclude Unnecessary Files
Make sure to use the .gitignore
file to exclude files and directories that don’t need to be tracked by Git, such as node_modules
, build artifacts, and IDE configuration files.
# .gitignore
node_modules/
build/
*.log
.idea/
This keeps the repository clean and reduces the size of the Git history.
5. Sync Your Fork with the Main Repository
If you’re working with a forked repository, it’s important to regularly sync your fork with the original repository to avoid conflicts and keep your fork up to date. This can be done by adding the original repository as a remote and pulling the changes from it.
git remote add upstream https://github.com/original-owner/repository.git
git fetch upstream
git merge upstream/main
This ensures that you’re working with the latest code and helps prevent large merge conflicts later on.
6. Pull Requests and Code Reviews
When your feature or bug fix is complete, submit a pull request (PR) to merge your changes into the main
branch. A PR allows others to review your code before it’s merged, ensuring that the changes are correct, follow the project’s coding standards, and don’t introduce any new bugs.
During a code review, make sure to:
- Explain the context of the changes and why they were made.
- Be open to feedback and suggestions for improvement.
- Test the changes thoroughly before submitting the PR.
7. Resolve Merge Conflicts Carefully
Merge conflicts occur when changes to the same part of a file have been made in different branches. When a conflict arises, Git will mark the conflicting sections of the file, and you’ll need to manually resolve them.
git merge main
# After resolving conflicts
git add .
git commit
When resolving conflicts, make sure to test the functionality thoroughly to ensure that the changes are applied correctly. Once resolved, commit the changes and push them to the repository.
8. Use Git Tags for Releases
Git tags are useful for marking specific points in your repository’s history, such as version releases. This makes it easy to reference, check out, or roll back to specific versions of your code.
git tag -a v1.0.0 -m "Initial release"
git push origin v1.0.0
Tags should be used to denote important milestones, such as production releases or major updates.
9. Use Branch Protection Rules in GitHub
To ensure the integrity of your codebase, use branch protection rules in GitHub. These rules can enforce requirements such as requiring pull request reviews, enforcing a specific status check (e.g., build passing), and preventing direct pushes to the main
branch.
To set branch protection rules in GitHub:
- Go to the repository on GitHub.
- Click on the
Settings
tab. - Navigate to
Branches
and configure the protection rules for themain
branch.
10. Document Your Git Workflow
Every team or project may have a different Git workflow, so it’s important to document your team’s version control practices. This helps new contributors understand how to work with the repository, the branching strategy to follow, and the steps for submitting changes.
Common workflows include:
- Git Flow: A branching model that uses separate branches for features, releases, and hotfixes.
- GitHub Flow: A simplified workflow focused on continuous delivery using pull requests for all changes.
11. Conclusion
By following version control best practices, you can ensure that your Git repositories remain clean, organized, and easy to manage. Proper use of Git and GitHub will help you collaborate more effectively, track the history of your codebase, and maintain a stable production environment. Keep commits meaningful, work with feature branches, and use pull requests for code reviews to maintain a high-quality codebase.
Higher-Order Components (HOC)
Higher-Order Components (HOC) are a powerful pattern in React for reusing component logic. An HOC is a function that takes a component as an argument and returns a new component with additional props or behavior. It allows you to add functionality to a component without modifying its structure, making your codebase more reusable and modular.
1. What is a Higher-Order Component?
A Higher-Order Component is a function that takes a component and returns a new component. The new component can modify the original component's props or behavior, which allows you to add common functionality to multiple components without duplicating code.
function withLoading(Component) {
return function LoadingHOC(props) {
if (props.isLoading) {
return Loading...;
}
return ;
};
}
In the example above, withLoading
is a higher-order component that adds loading functionality to the given component. The new component renders a loading message if the isLoading
prop is true, otherwise it renders the original component.
2. When to Use HOCs
HOCs are useful in the following scenarios:
- Code reuse: If you need to add the same functionality to multiple components (e.g., logging, authentication checks, etc.), HOCs can encapsulate that logic and keep your components clean.
- Conditionally render content: HOCs can alter the rendering logic based on props, such as showing a loading spinner or handling error states.
- Enhancing components: HOCs can add new behavior to components without modifying their original code.
3. Benefits of HOCs
Using Higher-Order Components offers several benefits:
- Separation of concerns: HOCs allow you to separate concerns and enhance components with shared functionality, without cluttering your component code.
- Reusability: HOCs allow you to reuse logic across multiple components, improving maintainability.
- Composability: Multiple HOCs can be composed together to create complex behavior in a declarative way.
4. Common Examples of HOCs
Below are some common examples of HOCs in React:
4.1. withAuth HOC
This HOC checks if the user is authenticated and either renders the requested component or redirects to a login page.
function withAuth(Component) {
return function AuthHOC(props) {
if (!props.isAuthenticated) {
return ;
}
return ;
};
}
4.2. withErrorBoundary HOC
This HOC catches JavaScript errors in components and displays an error message instead of crashing the entire app.
function withErrorBoundary(Component) {
return class ErrorBoundary extends React.Component {
state = { hasError: false };
static getDerivedStateFromError() {
return { hasError: true };
}
componentDidCatch(error, info) {
console.error("Error caught in HOC:", error, info);
}
render() {
if (this.state.hasError) {
return Something went wrong!;
}
return ;
}
};
}
5. Composing Multiple HOCs
HOCs can be composed together to build more complex behavior. When composing multiple HOCs, the order in which they are applied matters. The HOCs are applied from the outermost to the innermost, so the outer HOCs wrap the inner ones.
const enhance = compose(
withLoading,
withAuth,
withErrorBoundary
);
const EnhancedComponent = enhance(MyComponent);
The compose
function is often used to apply multiple HOCs in a readable and maintainable way. It allows you to combine behaviors such as loading, authentication, and error handling into a single component.
6. Caveats and Considerations
While HOCs are powerful, there are a few things to keep in mind when using them:
- Props collision: If an HOC adds props to the component, ensure they don't conflict with the original component’s props.
- Ref forwarding: If the component wrapped by an HOC needs to forward refs, you’ll need to use
React.forwardRef
to ensure the ref is passed down correctly. - Performance issues: HOCs can introduce performance overhead if not carefully implemented, especially if they are deeply nested.
7. Conclusion
Higher-Order Components are a powerful and flexible way to reuse component logic in React. They allow you to add functionality to your components without modifying their original code, improving maintainability and reusability. However, it’s important to be cautious of potential issues like props collision and performance overhead. By following best practices and keeping your HOCs simple and composable, you can take full advantage of this pattern to build more modular and scalable React applications.
React Suspense for Data Fetching
React Suspense is a powerful feature that allows you to handle asynchronous operations such as data fetching in a declarative way. It enables you to display loading states and manage the rendering of components that depend on data without blocking the entire UI. In this section, we will explore how React Suspense can be used for data fetching and how it improves the user experience.
1. What is React Suspense?
React Suspense is a feature that enables components to "wait" for something before rendering. It allows you to manage asynchronous operations like data fetching, code-splitting, and more, by using a <Suspense>
component to specify a loading fallback UI. When the data is ready, the component will re-render with the fetched data.
const MyComponent = React.lazy(() => import("./MyComponent"));
function App() {
return (
<React.Suspense fallback={Loading...}>
);
}
In this example, <React.Suspense>
wraps the MyComponent
and displays a loading message while waiting for the component to load asynchronously.
2. Data Fetching with Suspense
React Suspense can also be used for data fetching by using the Suspense
component in combination with a special data-fetching mechanism called a resource. A resource is an object that knows how to fetch data and can be used to suspend rendering until the data is ready.
function fetchData() {
let status = "pending";
let result = null;
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
status = "success";
result = "Fetched Data";
resolve(result);
}, 2000);
});
return {
read() {
if (status === "pending") {
throw promise;
} else if (status === "error") {
throw new Error("Data fetching error");
} else {
return result;
}
}
};
}
const resource = fetchData();
function MyComponent() {
const data = resource.read();
return {data};
}
function App() {
return (
<React.Suspense fallback={Loading...}>
);
}
In this example, fetchData
simulates an asynchronous data-fetching operation. The resource.read()
method either throws the promise (which suspends rendering) or returns the fetched data. The <Suspense>
component shows a loading message while the data is being fetched.
3. Benefits of Using Suspense for Data Fetching
- Declarative data fetching: Suspense allows you to declaratively specify loading states in your components, making the code more readable and easier to manage.
- Automatic handling of loading states: Suspense automatically manages loading states and renders fallback UIs without the need for manual state management.
- Improved user experience: With Suspense, you can avoid rendering incomplete or inconsistent UIs and provide users with a smooth experience while data is being fetched.
- Better code splitting: Suspense works seamlessly with React's
React.lazy
for code splitting, enabling you to load components and data asynchronously and improve app performance.
4. Error Boundaries with Suspense
When using Suspense, you should also consider error boundaries to handle any errors that may occur during data fetching. You can use the <ErrorBoundary>
component to catch errors and display an error message instead of crashing the app.
class ErrorBoundary extends React.Component {
state = { hasError: false };
static getDerivedStateFromError() {
return { hasError: true };
}
componentDidCatch(error, info) {
console.error("Error caught in Suspense:", error, info);
}
render() {
if (this.state.hasError) {
return Error occurred during data fetching!;
}
return this.props.children;
}
}
function App() {
return (
<React.Suspense fallback={Loading...}>
);
}
In this example, the ErrorBoundary
component catches any errors that occur within the Suspense tree and displays an error message if something goes wrong during data fetching.
5. Future of Suspense for Data Fetching
Currently, React Suspense for data fetching is still an experimental feature, and it requires additional libraries like react-cache
for managing resources. However, in the future, React plans to fully integrate Suspense for data fetching into the core library, making it easier to use without external dependencies.
6. Conclusion
React Suspense for data fetching is a game-changing feature that simplifies asynchronous rendering in React. By using Suspense, you can declaratively handle loading states, improve performance, and enhance the user experience without complex state management. While still in an experimental phase, Suspense has the potential to revolutionize how we fetch and display data in React applications.
Understanding React Fiber Architecture
React Fiber is a complete rewrite of React's core algorithm. It provides more efficient rendering and a more flexible architecture, which allows React to handle complex updates with better performance. In this section, we will explore what React Fiber is and how it improves React’s rendering process.
1. What is React Fiber?
React Fiber is the new reconciliation algorithm in React. It was introduced in React 16 to improve the rendering process and allow for more control over the component rendering lifecycle. Fiber enables React to work asynchronously and prioritize updates based on importance, which results in a smoother user experience, especially in complex applications.
2. The Motivation Behind React Fiber
The primary motivation behind React Fiber was to address the limitations of the old React rendering algorithm (also known as the "stack reconciler"). The stack reconciler was synchronous and could not handle complex UI updates efficiently, especially for large applications or components with heavy animations and transitions.
React Fiber enables asynchronous rendering, which means React can break down large updates into smaller chunks and prioritize the most important tasks, such as user interactions, over less critical ones like background updates.
3. How React Fiber Works
React Fiber introduces a new data structure called the "fiber" to represent the component tree. A fiber is a lightweight object that holds information about a component’s state, props, and the actions that need to be performed when the component is rendered or updated.
The Fiber reconciliation process can be broken down into several phases:
- Render Phase: During this phase, React builds the fiber tree. The components are updated based on the new state and props.
- Commit Phase: After the render phase, React commits the changes to the DOM or native views. This is when the actual rendering happens.
- Reconciliation: React compares the new fiber tree with the previous one to identify what has changed and what needs to be updated.
4. Asynchronous Rendering with Fiber
One of the key features of React Fiber is asynchronous rendering. This allows React to break down rendering work into chunks and spread it out over multiple frames, preventing the UI from blocking during heavy updates.
For example, if a user is interacting with the UI and an update is being processed, React Fiber can split the work into smaller units, allowing the user interaction to remain smooth while the update continues in the background.
const MyComponent = () => {
const [count, setCount] = useState(0);
// Fiber can prioritize updates based on user interaction.
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
};
In this example, React Fiber helps React prioritize the user interaction (clicking the button) over other updates, such as background data fetching or animations.
5. Prioritization of Updates in Fiber
React Fiber allows you to assign priorities to different updates. React uses a priority system to decide which updates should be processed first, depending on their importance.
- High Priority: User interactions, animations, and urgent updates are processed first.
- Low Priority: Background tasks, like data fetching or certain non-urgent updates, are handled later.
const fetchData = async () => {
// Low priority background task.
const response = await fetch("https://api.example.com/data");
const data = await response.json();
setData(data);
};
useEffect(() => {
fetchData();
}, []);
In this example, React Fiber ensures that the fetch request (a background task) will not block the UI, and user interactions or animations will be prioritized.
6. Benefits of React Fiber
- Improved Performance: Fiber allows React to break down rendering into smaller tasks, improving performance, especially for large applications.
- Asynchronous Rendering: React can perform updates in the background, preventing the UI from freezing or becoming unresponsive.
- Better User Experience: By prioritizing updates based on importance, React Fiber ensures smooth user interactions and animations.
- Concurrent Mode: React Fiber lays the groundwork for Concurrent Mode, a future feature that will allow React to pause, interrupt, and resume rendering tasks for even better performance and responsiveness.
7. Conclusion
React Fiber is a groundbreaking improvement to React's core architecture that allows for more efficient rendering, better performance, and a smoother user experience. By enabling asynchronous rendering and prioritizing updates, React Fiber ensures that your application stays fast and responsive, even during complex updates. The introduction of Fiber also paves the way for future features like Concurrent Mode, which will further enhance the React experience.
Custom Renderers in React
React's rendering system is highly flexible, and one of the advanced features of React is the ability to create custom renderers. A custom renderer allows you to build React applications for non-browser environments, such as mobile apps, native platforms, or even for rendering to a custom medium (like a canvas or a virtual reality environment). In this section, we will explore what custom renderers are and how to create one in React.
1. What is a Custom Renderer?
A custom renderer in React is a system that allows you to render React components in a custom target environment, such as a non-HTML DOM or a mobile device. It takes advantage of React's core rendering and reconciliation logic while allowing you to define how components should be rendered in a different environment.
The most common example of a custom renderer is React Native, which uses a custom renderer to render React components as native mobile components rather than HTML elements. Similarly, React 360 (for VR applications) also uses a custom renderer to render components in a 3D environment.
2. Why Use Custom Renderers?
Custom renderers are beneficial when you want to leverage React’s component model and efficient rendering in non-standard environments. Some use cases include:
- Mobile Apps: Creating native mobile apps using React Native.
- Virtual Reality (VR): Rendering React components for 3D or VR environments using React 360 or similar tools.
- Canvas or WebGL: Rendering React components on a canvas element for game development or visualizations.
3. How Custom Renderers Work
A custom renderer interacts with React’s internal rendering pipeline to process the component tree and update the environment accordingly. React's rendering process consists of three main phases:
- Reconciliation: React compares the new virtual DOM tree with the previous one to calculate the minimal set of changes (called "diffing").
- Rendering: React converts the virtual DOM nodes into actual elements (like native UI components or DOM nodes) in the target environment.
- Commit: React applies the changes to the environment (e.g., updates the screen or UI elements in the target platform).
In a custom renderer, you are responsible for implementing the rendering phase and commit phase, deciding how React should render and commit the changes in the custom environment.
4. Creating a Custom Renderer
To create a custom renderer, you need to implement a few essential methods that React’s rendering system relies on. Here’s an overview of the steps:
- Install React Reconciler: Use the
react-reconciler
package, which provides the core reconciliation and rendering logic for building custom renderers. - Define Host Configurations: Define the environment-specific operations, such as how to create, update, and commit components in the target environment.
- Implement the Custom Renderer: Implement the necessary methods to handle rendering in your custom environment.
5. Example: A Simple Custom Renderer
Here’s a simplified example of creating a custom renderer that renders React components onto the console (just for demonstration purposes):
import { createInstance, commitUpdate, commitRoot } from "react-reconciler";
import React, { createElement } from "react";
const hostConfig = {
now: Date.now,
scheduleCallback: requestAnimationFrame,
createInstance(type, props, rootContainerInstance) {
return { type, props };
},
commitUpdate(instance, updatePayload) {
instance.props = updatePayload;
console.log("Updated:", instance);
},
commitRoot(root) {
console.log("Committing root:", root);
},
// Other necessary methods for custom renderer...
};
const reconciler = createInstance(hostConfig);
const MyComponent = () => {
return createElement("div", {}, "Hello from custom renderer!");
};
const container = {}; // This would be your custom container, like a canvas or native view.
reconciler.render(MyComponent, container);
In this example, the custom renderer simply logs the updates to the console instead of rendering to the DOM. The key takeaway is the use of createInstance
, commitUpdate
, and commitRoot
, which are necessary methods for rendering and committing changes to the target environment.
6. Custom Renderer for React Native (Overview)
React Native uses a custom renderer to map React components to native mobile components. The fundamental process is similar to the console example but more complex due to the native environment’s specific requirements.
In React Native, components like View
, Text
, and Image
are the equivalents of HTML elements. The custom renderer ensures these components are properly instantiated and updated in the native mobile environment.
import { AppRegistry } from 'react-native';
import React from 'react';
const MyComponent = () => {
return Hello from React Native! ;
};
AppRegistry.registerComponent('MyApp', () => MyComponent);
In this React Native example, React Native’s custom renderer takes care of rendering the Text
component onto the screen instead of using the browser's DOM.
7. Benefits of Custom Renderers
- Target Different Platforms: Custom renderers allow you to use React's declarative component model for applications across multiple platforms, including mobile, VR, and canvas-based apps.
- Reusability: Custom renderers let you reuse the same React components across different platforms with different rendering logic.
- Performance Optimization: Custom renderers can be optimized for specific environments to achieve better performance and responsiveness.
8. Conclusion
Custom renderers in React provide a powerful way to build React applications for non-standard environments. By leveraging React’s core rendering and reconciliation logic, custom renderers allow you to target any platform and render React components efficiently. Whether you're building mobile apps with React Native, VR apps with React 360, or rendering on a canvas, custom renderers enable you to leverage React's declarative UI model in new and exciting ways.
Serverless Framework with React
Serverless architecture allows you to build and run applications without managing servers. The Serverless Framework is one of the most popular tools for deploying serverless applications. In this section, we’ll explore how to integrate React with the Serverless Framework for building modern, scalable applications that leverage cloud services.
1. What is the Serverless Framework?
The Serverless Framework is an open-source tool designed to simplify the deployment of serverless applications. It provides a way to define the backend components of your application (such as AWS Lambda functions, API Gateway endpoints, and database resources) in a configuration file, allowing for easier deployment and management.
With the Serverless Framework, you can deploy functions to cloud providers like AWS, Google Cloud, and Azure without managing the underlying infrastructure. It abstracts away much of the complexity of setting up server environments, scaling, and handling server maintenance.
2. Why Use Serverless with React?
Using the Serverless Framework with React brings several benefits:
- Scalability: Serverless functions scale automatically based on demand. No need to worry about provisioning servers or managing infrastructure.
- Cost Efficiency: You only pay for the resources you use. Serverless is often cheaper than traditional hosting models because you don’t pay for idle time.
- Quick Deployment: Serverless applications typically require less setup and can be deployed with minimal configuration.
- Focus on the Frontend: React developers can focus more on writing code for the frontend, with the serverless backend handling the heavy lifting.
3. Setting Up the Serverless Framework
Before integrating React with the Serverless Framework, you need to set up the Serverless Framework in your environment. Here are the steps to get started:
- Install Node.js and NPM: Make sure that you have Node.js and npm installed on your machine. If not, download and install them from nodejs.org.
- Install the Serverless Framework: You can install the Serverless Framework globally using npm:
npm install -g serverless
Once installed, you can verify the installation by running:
serverless --version
4. Creating a Serverless Backend
Once the Serverless Framework is set up, you can create a new Serverless service (project) that includes AWS Lambda functions, API Gateway, and other resources like DynamoDB or S3.
- Create a Serverless Service: Run the following command to create a new serverless service:
serverless create --template aws-nodejs --path my-service
cd my-service
This will create a new directory with the serverless service setup, including a serverless.yml
file that defines the service configuration.
5. Creating Lambda Functions
In the Serverless Framework, backend logic is handled by Lambda functions. Let’s create a simple Lambda function that can handle HTTP requests through API Gateway. In the serverless.yml
configuration file, define the function as follows:
functions:
hello:
handler: handler.hello
events:
- http:
path: hello
method: get
This configuration defines a GET endpoint at the path /hello
, which triggers the hello
Lambda function defined in the handler.js
file.
In the handler.js
file, define the Lambda function like so:
module.exports.hello = async (event) => {
return {
statusCode: 200,
body: JSON.stringify({ message: "Hello from Lambda!" })
};
};
Now, deploy the function to AWS using the Serverless Framework:
serverless deploy
This will deploy the Lambda function and API Gateway. After deployment, you’ll receive an endpoint URL that you can use to test the function.
6. Integrating Serverless with React
Now that you have a serverless backend set up, let’s integrate it with a React frontend. In your React app, you can use fetch
or a library like axios
to make HTTP requests to the deployed API Gateway endpoint.
import React, { useEffect, useState } from 'react';
const App = () => {
const [message, setMessage] = useState('');
useEffect(() => {
// Replace with the actual API Gateway endpoint URL
fetch('https://your-api-id.execute-api.us-east-1.amazonaws.com/dev/hello')
.then(response => response.json())
.then(data => setMessage(data.message))
.catch(error => console.error('Error:', error));
}, []);
return (
{message}
);
};
export default App;
In this example, the React app fetches data from the serverless backend and displays the message returned by the Lambda function. Replace the URL with your own API Gateway endpoint URL after deploying the serverless service.
7. Deploying the Full-Stack Application
To deploy the React app and the serverless backend, you can deploy the React app to a static hosting service like Vercel, Netlify, or AWS S3, and deploy the backend using the Serverless Framework as shown earlier.
8. Benefits of Using Serverless with React
- Cost Efficiency: Serverless platforms are pay-as-you-go, meaning you only pay for the execution time and resources you use, which can save costs for backend infrastructure.
- Scalability: Serverless applications scale automatically without requiring manual configuration of servers, making them ideal for high-traffic applications.
- Focus on Frontend Development: Developers can focus on building the frontend with React while the serverless backend handles the logic and data storage automatically.
- Quick Deployment: Serverless applications can be deployed quickly and with minimal configuration.
9. Conclusion
Combining React with the Serverless Framework offers a powerful solution for building scalable, cost-effective applications. By leveraging the Serverless Framework's ease of deployment and React's component-driven architecture, you can create full-stack applications that are fast to deploy, cost-efficient, and scalable based on demand. Whether you're building a simple app or a complex system, serverless with React can streamline your development process and reduce operational overhead.
Social Media Dashboard with React and Chart.js
In this section, we will build a social media dashboard where you can visualize key metrics like user engagement, follower growth, and more using React and Chart.js. The dashboard will use mock data to simulate social media performance and display various charts.
Step 1: Set Up the Project
First, create a new React project using Create React App:
Then, install the necessary dependencies for Chart.js and React-Chartjs-2:
Step 2: Create the Dashboard Component
In your project, create a new component called
Dashboard.js
inside thesrc
folder. This component will hold the charts and visualizations of the social media data.Step 3: Integrate the Dashboard Component into App
Now, integrate the
Dashboard.js
component into your mainApp.js
file.Step 4: Customize the Styling
You can style the dashboard using CSS to make it look more visually appealing. For simplicity, we will add a basic style in
src/App.css
:Step 5: Run the Application
To run your React application, use the following command:
Your social media dashboard will be available at
http://localhost:3000
, displaying the user engagement chart, followers per platform, and engagement breakdown with interactive visualizations using Chart.js.Step 6: Deploying the Application
You can deploy your dashboard to platforms such as Netlify, Vercel, or Heroku for production use. Simply follow the deployment instructions provided by the platform of your choice.
Conclusion
In this tutorial, we've built a simple social media dashboard using React and Chart.js. We created visualizations for user engagement over time, followers per platform, and engagement breakdown. With this foundation, you can expand the dashboard to include more data, additional charts, and interactive features like filtering and dynamic data updates.