What is MongoDB?
MongoDB is a popular, open-source NoSQL database designed for scalability, flexibility, and performance. Unlike traditional relational databases, MongoDB stores data in a JSON-like format called BSON (Binary JSON), which allows for more dynamic and hierarchical data storage. This makes it well-suited for modern applications that require handling large volumes of structured and unstructured data.
History of MongoDB
MongoDB was created by 10gen (now MongoDB, Inc.) in 2007 as a scalable database solution for web applications. The company initially focused on building a cloud-based platform but later pivoted to offering MongoDB as a database service. MongoDB was officially released in 2009 and has since become one of the most widely used NoSQL databases in the world.
MongoDB Features
Below are the key features that make MongoDB a popular choice for modern applications:
Feature | Description |
---|---|
Document-Oriented | MongoDB stores data in BSON format, allowing flexibility in the structure of documents within collections. It doesn’t enforce a fixed schema, which is ideal for dynamic applications. |
Scalable | MongoDB is designed for horizontal scalability. It supports sharding, which enables the distribution of data across multiple machines for high availability and performance. |
Aggregation Framework | MongoDB includes a powerful aggregation framework that enables complex data transformations, filtering, and grouping operations, making it suitable for analytics and reporting. |
High Availability | MongoDB supports replica sets, which provide automatic failover and data redundancy, ensuring high availability even in the event of a server failure. |
Setting Up MongoDB
Before you begin using MongoDB, you need to install it on your system. Follow these steps to set it up:
- Download the MongoDB installer from the official MongoDB website.
- Follow the installation instructions for your operating system (Windows, macOS, or Linux).
- Once installed, open a terminal or command prompt and type
mongo --version
to verify that MongoDB is installed correctly.
Code Example: Connecting to MongoDB with Node.js
Here’s a simple example of how to connect to MongoDB using Node.js:
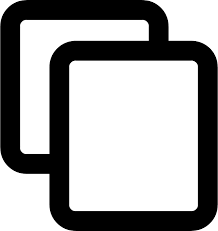
// Node.js code to connect to MongoDB
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('Could not connect to MongoDB...', err));
Diagram: MongoDB Architecture
The following diagram illustrates the key components of MongoDB’s architecture:

This diagram highlights MongoDB’s components such as collections, documents, replica sets, and sharding, which work together to provide a scalable and high-performance database solution.
NoSQL vs. SQL Databases
SQL (Structured Query Language) and NoSQL (Not Only SQL) are two major categories of databases used in modern application development. While SQL databases are widely used for structured data and relational applications, NoSQL databases are preferred for applications that require scalability, flexibility, and the ability to handle unstructured or semi-structured data.
SQL Databases
SQL databases are relational databases that store data in tables with predefined schemas. They use SQL to define and manipulate data. SQL databases are highly structured and are well-suited for applications where data relationships are important, such as finance, banking, and traditional business applications.
NoSQL Databases
NoSQL databases are non-relational and store data in a variety of formats, such as key-value pairs, documents, graphs, or wide-column stores. NoSQL databases are designed for scalability and flexibility, making them ideal for applications that handle large volumes of unstructured or rapidly changing data, such as social media platforms, big data analytics, and real-time web applications.
Key Differences Between SQL and NoSQL
Here are the major differences between SQL and NoSQL databases:
Characteristic | SQL | NoSQL |
---|---|---|
Data Model | Relational (tables, rows, columns) | Non-relational (document-based, key-value, graph, column-family) |
Schema | Fixed schema (structure is predefined) | Dynamic schema (no fixed structure) |
Scalability | Vertical scaling (increasing CPU, RAM, or storage of a single server) | Horizontal scaling (distributing data across multiple servers) |
Transactions | Supports ACID transactions (Atomicity, Consistency, Isolation, Durability) | Supports BASE (Basically Available, Soft state, Eventually consistent) |
Use Cases | Applications requiring complex queries and transactions (banking, ERP, CRM) | Applications requiring scalability and flexibility (real-time analytics, big data, IoT) |
When to Use SQL Databases
SQL databases are best suited for applications that require complex queries, strong consistency, and structured data. Use SQL when:
- Your data has a clear structure and relationships between entities.
- Your application requires complex JOIN operations and transactions.
- You need ACID compliance for data integrity.
- Your application is small to medium-sized and doesn't need extreme scalability.
When to Use NoSQL Databases
NoSQL databases are ideal for applications that need high availability, scalability, and handle large amounts of unstructured data. Use NoSQL when:
- Your data is unstructured, semi-structured, or rapidly changing.
- Your application needs to scale horizontally to handle large volumes of data.
- Your application requires flexibility in data modeling or schema design.
- Your application is focused on real-time analytics, social media, or IoT.
Code Example: SQL Query
Here’s an example of a SQL query to fetch all customers from a table:
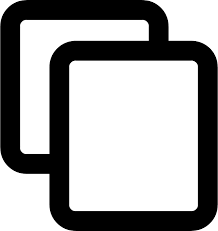
-- SQL Query to fetch all customers
SELECT * FROM customers WHERE city = 'New York';
Code Example: NoSQL Query (MongoDB)
Here’s an example of a NoSQL query to fetch all customers from a MongoDB collection:
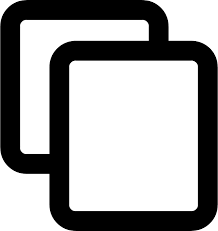
// MongoDB query to fetch all customers
db.customers.find({ city: 'New York' });
Diagram: SQL vs NoSQL
The following diagram compares SQL and NoSQL databases, highlighting their key differences:

This diagram illustrates the structure, scalability, and use cases of SQL and NoSQL databases, helping you understand when to use each type based on your application needs.
Key Features and Benefits of MongoDB
MongoDB is a popular NoSQL database that provides high performance, flexibility, and scalability. It is known for its ability to handle large volumes of unstructured and semi-structured data. MongoDB offers several key features and benefits that make it a preferred choice for modern applications, especially when dealing with big data, real-time analytics, and high-velocity workloads.
Key Features of MongoDB
Here are some of the standout features of MongoDB:
- Document-Oriented Storage: MongoDB stores data in flexible, JSON-like documents, which allows for a dynamic schema. This makes it easier to store and retrieve complex, nested data structures.
- Scalability: MongoDB is designed for horizontal scalability. It can scale across multiple servers, allowing applications to handle large amounts of data and traffic without significant performance loss.
- High Availability: MongoDB provides built-in replication and automatic failover with replica sets, ensuring data availability and fault tolerance even in the case of hardware failure.
- Indexing: MongoDB supports a variety of indexing options, including single-field, compound, geospatial, text, and hashed indexes, to improve query performance.
- Aggregation Framework: MongoDB’s powerful aggregation framework enables the transformation and analysis of data using pipelines. It allows developers to perform complex queries, data filtering, and summarization.
- Flexible Schema: Unlike relational databases, MongoDB does not require a fixed schema. This allows for easy changes to the structure of data as the application evolves.
- Rich Query Language: MongoDB supports a rich query language that provides a variety of operations such as sorting, filtering, joins (via `$lookup`), and more, which enhances its ability to handle complex data retrieval tasks.
- Geospatial Indexing: MongoDB supports geospatial queries and indexing, which is useful for applications that require location-based searches, such as mapping and geolocation services.
Benefits of MongoDB
MongoDB offers several advantages that make it well-suited for modern application development:
- Ease of Use: MongoDB’s document-based structure is intuitive and easy to work with, especially for developers who are familiar with JSON. This also makes it easier to map data in applications directly to MongoDB’s storage format.
- High Performance: MongoDB offers high throughput and low latency by using a flexible data model and allowing for indexing and optimized query execution. It is capable of handling high-velocity workloads and large-scale datasets.
- Seamless Horizontal Scaling: With its ability to shard data across multiple servers, MongoDB can handle increasing data and user loads, making it ideal for applications that need to scale rapidly and efficiently.
- Developer Productivity: MongoDB’s flexible schema and rich query capabilities allow developers to quickly iterate and modify their applications without worrying about rigid database schemas, which boosts productivity.
- Real-Time Analytics: MongoDB’s aggregation framework and support for real-time data processing make it an excellent choice for applications that require quick insights and analytics, such as social media platforms, IoT, and e-commerce websites.
- Cloud-Native: MongoDB integrates well with cloud platforms like AWS, Azure, and Google Cloud. MongoDB Atlas, MongoDB's fully managed cloud service, offers easy deployment, scaling, and monitoring for cloud-based applications.
- Community and Ecosystem: MongoDB has a large community of developers and a rich ecosystem of tools, frameworks, and resources, which helps speed up development and troubleshooting.
Code Example: Inserting Data in MongoDB
Here’s an example of how to insert data into a MongoDB collection using Node.js:
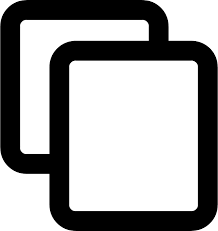
// MongoDB example in Node.js to insert a document into the 'users' collection
const mongoose = require('mongoose');
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('Failed to connect', err));
// Define a Schema
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
// Create a Model
const User = mongoose.model('User', userSchema);
// Create a new user document
const user = new User({
name: 'John Doe',
email: 'john.doe@example.com',
age: 30
});
// Insert the document into the collection
user.save()
.then(() => console.log('User saved'))
.catch(err => console.error('Error saving user:', err));
Diagram: MongoDB Architecture
This diagram explains the architecture of MongoDB, illustrating its components such as the database, collections, documents, and replica sets:

In this diagram, you can see how MongoDB stores and manages data within collections and documents, as well as the replication mechanism for ensuring high availability and fault tolerance.
MongoDB Architecture (Collections, Documents, and Databases)
MongoDB's architecture is designed to be simple, flexible, and scalable. It is a document-oriented NoSQL database that stores data in collections and documents, making it different from traditional relational databases. This section explains the core components of MongoDB architecture, including collections, documents, and databases.
1. MongoDB Databases
A database in MongoDB is a container for collections. Each MongoDB database has its own set of collections and is isolated from other databases. MongoDB supports multiple databases within a single instance, and each database operates independently, with its own data and user access control.
- Default Database: MongoDB provides a default database called
test
, which is created when no database is specified. - Creating a Database: You can create a new database using the
use
command or programmatically when inserting data into a collection.
2. MongoDB Collections
A collection is a grouping of MongoDB documents. Collections are analogous to tables in relational databases. However, unlike tables, collections in MongoDB do not enforce a strict schema, meaning each document can have different fields and structures.
- Unstructured Data: Collections can store documents with varied structures. This flexibility allows MongoDB to handle semi-structured or unstructured data effectively.
- Creating a Collection: Collections are created automatically when you
insert the first document. You can also manually create a collection using the
createCollection()
method.
3. MongoDB Documents
A document in MongoDB is the basic unit of data. It is a JSON-like object consisting of key-value pairs, where the key is a field name and the value is the corresponding data. MongoDB uses BSON (Binary JSON) format for storing documents, which supports additional data types like ObjectId, Date, and more.
- Flexibility: Unlike rows in relational databases, documents in MongoDB can have different fields and even nested structures, which makes MongoDB ideal for flexible and evolving data models.
- Example Document: A document might represent a user and contain fields
such as
name
,email
, andaddress
, where theaddress
field might contain another object with nested fields likestreet
,city
, etc.
Code Example: MongoDB Database, Collection, and Document
The following example demonstrates how to interact with MongoDB databases, collections, and documents using Node.js:
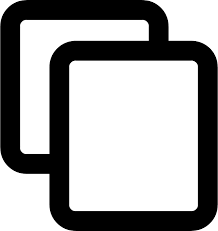
// MongoDB example in Node.js to create a database, collection, and document
const mongoose = require('mongoose');
// Connect to MongoDB (it will automatically create the database if it doesn't exist)
mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('Failed to connect', err));
// Define a Schema for a 'User' collection
const userSchema = new mongoose.Schema({
name: String,
email: String,
address: {
street: String,
city: String,
state: String
}
});
// Create a model for the 'User' collection
const User = mongoose.model('User', userSchema);
// Insert a new document into the 'users' collection
const newUser = new User({
name: 'Jane Doe',
email: 'jane.doe@example.com',
address: {
street: '123 Main St',
city: 'Anytown',
state: 'Anystate'
}
});
// Save the document to the database
newUser.save()
.then(() => console.log('User saved'))
.catch(err => console.error('Error saving user:', err));
Diagram: MongoDB Architecture
The following diagram provides a visual representation of MongoDB’s architecture, showing how databases, collections, and documents are organized:

In this diagram, we can see how a MongoDB instance contains multiple databases, each with collections, which in turn contain documents.
Use Cases and Applications of MongoDB
MongoDB is a versatile and scalable NoSQL database, well-suited for a wide range of applications. Its flexible schema and ability to handle large volumes of unstructured or semi-structured data make it ideal for various use cases. Below are some common use cases and applications where MongoDB excels.
1. Content Management Systems (CMS)
MongoDB is commonly used in content management systems due to its ability to store dynamic content and manage metadata efficiently. It is especially beneficial for handling varied content formats such as articles, images, videos, and documents.
- Benefits: Flexible schema allows easy handling of diverse content types, and high scalability supports content-heavy applications.
- Example: Websites that host blogs, articles, and multimedia content can use MongoDB to store and manage content at scale.
2. Real-Time Analytics
MongoDB's ability to handle large volumes of data in real-time makes it ideal for applications requiring quick analytics and data processing. It supports various data types, including time-series data, which is crucial in real-time analytics.
- Benefits: High-performance read/write operations and support for complex aggregation allow quick insights into live data.
- Example: Monitoring applications that track user behavior, website traffic, or system metrics can benefit from MongoDB’s real-time analytics.
3. E-Commerce Applications
MongoDB is frequently used in e-commerce platforms due to its flexible data model and scalability. It can handle varying product catalogs, large inventories, and customer data efficiently.
- Benefits: Scalability for handling high traffic and large catalogs, and flexibility to store complex product information.
- Example: Online stores can store product details, reviews, user profiles, and order histories in MongoDB, while scaling seamlessly as user demands grow.
4. Mobile and Social Media Applications
MongoDB is well-suited for mobile and social media apps due to its support for large, dynamic datasets and rapid changes in data structure. It is ideal for storing user profiles, media, posts, and other social interactions.
- Benefits: Fast data retrieval and seamless scalability for handling millions of users and interactions in real time.
- Example: Social networking platforms can use MongoDB to store user-generated content, friend lists, posts, and likes, allowing for quick retrieval and updates.
5. Internet of Things (IoT)
IoT applications often require handling vast amounts of sensor and device data, which can be unstructured or semi-structured. MongoDB is well-suited for this use case due to its ability to store diverse data types and scale horizontally.
- Benefits: MongoDB supports high-throughput write operations, which is ideal for storing large volumes of IoT data in real time.
- Example: Applications tracking data from smart devices, sensors, and wearables can leverage MongoDB for storing and analyzing IoT data.
6. Gaming Applications
MongoDB is widely used in gaming applications to manage user data, session data, and game state in real time. Its flexibility and scalability make it a preferred choice for storing game data dynamically.
- Benefits: Real-time data updates, horizontal scaling, and the ability to handle complex game data structures.
- Example: Multiplayer online games can store player profiles, in-game statistics, and real-time data in MongoDB.
7. Financial Applications
MongoDB is well-suited for financial applications that need to store large volumes of transaction data, customer details, and financial records in a scalable and flexible manner.
- Benefits: MongoDB's horizontal scaling allows it to handle high volumes of transactions, while its flexible schema supports financial data's complex and dynamic nature.
- Example: Personal finance apps, cryptocurrency platforms, and banking systems can store transaction histories, account details, and real-time financial data in MongoDB.
8. Catalog Management Systems
Catalog management systems benefit from MongoDB's flexibility in handling large, varied product inventories. MongoDB can efficiently store and query information about products and services, including descriptions, prices, categories, and images.
- Benefits: Schema flexibility to store different product attributes and the ability to scale for large inventories.
- Example: Retailers and wholesalers can use MongoDB to manage product catalogs, pricing, and stock details.
9. Healthcare Applications
MongoDB is increasingly being used in healthcare applications to store patient records, medical histories, and real-time data from health monitoring systems. Its ability to handle a variety of data formats and scale with data growth is essential for healthcare systems.
- Benefits: MongoDB’s flexibility allows for integrating structured and unstructured data sources like text, images, and sensor data.
- Example: Electronic health record (EHR) systems and telemedicine platforms can use MongoDB to store patient information, test results, and diagnostic images.
Code Example: Real-Time Analytics with MongoDB
The following example demonstrates using MongoDB for real-time analytics in a web application:
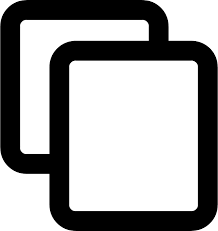
// Example of storing and querying real-time analytics data in MongoDB
const mongoose = require('mongoose');
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/analytics', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('Failed to connect', err));
// Define Schema for storing analytics data
const analyticsSchema = new mongoose.Schema({
userId: String,
action: String,
timestamp: { type: Date, default: Date.now }
});
// Create model for analytics data
const Analytics = mongoose.model('Analytics', analyticsSchema);
// Insert real-time analytics data
const newAction = new Analytics({
userId: 'user123',
action: 'clicked_button'
});
newAction.save()
.then(() => console.log('Action logged'))
.catch(err => console.error('Error logging action:', err));
// Query real-time analytics data
Analytics.find({ userId: 'user123' })
.then(actions => console.log('User actions:', actions))
.catch(err => console.error('Error fetching actions:', err));
Installing MongoDB on Windows, macOS, and Linux
MongoDB is available for installation on multiple operating systems, including Windows, macOS, and Linux. Below are the instructions for installing MongoDB on each platform.
1. Installing MongoDB on Windows
Follow the steps below to install MongoDB on a Windows machine:
- Visit the official MongoDB download page.
- Select the Windows version and download the .msi installer.
- Run the downloaded installer and follow the on-screen instructions.
- During installation, select the "Complete" setup type and choose "Install MongoDB as a Service" to ensure that MongoDB starts automatically when your system boots.
- Once installation is complete, open the Command Prompt and type
mongo
to confirm that MongoDB is installed and running. - If MongoDB does not start automatically, you can start it manually by running
net start MongoDB
in the Command Prompt.
2. Installing MongoDB on macOS
To install MongoDB on macOS, you can use the Homebrew package manager. Follow these steps:
- If you do not have Homebrew installed, open the Terminal and run the following command
to install it:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Once Homebrew is installed, tap the MongoDB formula by running:
brew tap mongodb/brew
- Now, install MongoDB by running:
brew install mongodb-community@6.0
- Start MongoDB by using the following command:
brew services start mongodb/brew/mongodb-community
- Verify that MongoDB is running by typing
mongo
in the terminal.
3. Installing MongoDB on Linux
For Linux distributions, the installation steps may vary depending on the package manager. Below are the steps for installing MongoDB on Ubuntu and CentOS:
For Ubuntu (Debian-based distributions):
- Import the MongoDB public GPG key by running:
wget -qO - https://www.mongodb.org/static/pgp/server-6.0.asc | sudo apt-key add -
- Add the MongoDB repository by running:
echo "deb [ arch=amd64,arm64 ] https://repo.mongodb.org/apt/ubuntu focal/mongodb-org/6.0 multiverse" | sudo tee /etc/apt/sources.list.d/mongodb-org-6.0.list
- Update the local package database:
sudo apt-get update
- Install MongoDB:
sudo apt-get install -y mongodb-org
- Start MongoDB by running:
sudo systemctl start mongod
- Enable MongoDB to start on boot:
sudo systemctl enable mongod
- Verify MongoDB installation by typing:
mongo
For CentOS (RHEL-based distributions):
- Create a MongoDB repository file:
echo "[mongodb-org-6.0] name=MongoDB Repository baseurl=https://repo.mongodb.org/yum/amazon/2/mongodb-org/6.0/x86_64/ gpgcheck=1 enabled=1 gpgkey=https://www.mongodb.org/static/pgp/server-6.0.asc" | sudo tee /etc/yum.repos.d/mongodb-org-6.0.repo
- Install MongoDB:
sudo yum install -y mongodb-org
- Start MongoDB:
sudo systemctl start mongod
- Enable MongoDB to start on boot:
sudo systemctl enable mongod
- Verify MongoDB installation by typing:
mongo
4. Verifying MongoDB Installation
After installation, you can verify that MongoDB is working properly by launching the MongoDB shell. In your terminal or command prompt, type:
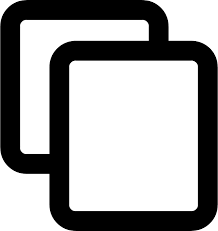
mongo
If MongoDB is running, you should see the MongoDB shell prompt, which looks like:
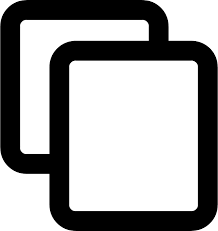
MongoDB shell version v6.0.4
connecting to: mongodb://127.0.0.1:27017
Implicit session: session { "id" : UUID("some-unique-id") }
MongoDB server version: 6.0.4
>
If you see the shell prompt, MongoDB is successfully installed and running.
Troubleshooting
If you encounter any issues during installation, check the following:
- Ensure that your system meets the necessary hardware and software requirements for MongoDB.
- Check that the MongoDB service is running by using
systemctl status mongod
(Linux) or the Windows Services Manager (Windows). - Review log files for error messages. MongoDB logs are located in
/var/log/mongodb/mongod.log
on Linux, and in the MongoDB installation directory on Windows.
Introduction to MongoDB Atlas (Cloud-based MongoDB)
MongoDB Atlas is a fully-managed cloud database service provided by MongoDB, Inc. It offers a cloud-hosted version of MongoDB with all the features of the open-source database, but with the added benefits of scalability, security, and ease of management. Atlas takes care of database operations, including monitoring, backups, and scaling, so you can focus on building your application rather than managing infrastructure.
Key Features of MongoDB Atlas
MongoDB Atlas provides a wide range of features that make it easy to deploy, manage, and scale MongoDB databases in the cloud:
- Fully Managed Database: MongoDB Atlas handles all aspects of database management, including backups, monitoring, and patching, freeing you from the complexity of database administration.
- Scalable: With MongoDB Atlas, you can easily scale your database horizontally or vertically to handle large amounts of data and traffic. It allows you to increase storage capacity, replica sets, and shard clusters with just a few clicks.
- Global Distribution: MongoDB Atlas allows you to deploy your database across multiple cloud regions, providing low-latency access to users worldwide and ensuring high availability.
- Security: MongoDB Atlas includes built-in security features like encryption at rest, network isolation, IP whitelisting, and advanced authentication methods to help keep your data secure.
- Real-Time Monitoring: MongoDB Atlas offers comprehensive monitoring and analytics, including real-time performance metrics, alerting, and custom dashboards to track the health of your database.
- Integrated Backups: Atlas provides automated backups, enabling point-in-time recovery in case of data loss or corruption.
- Cloud Provider Integration: MongoDB Atlas is designed to integrate with major cloud providers, including AWS, Google Cloud, and Microsoft Azure, allowing you to choose your cloud provider of choice for database deployment.
Benefits of Using MongoDB Atlas
Using MongoDB Atlas offers several advantages over self-managed MongoDB deployments:
- Reduced Operational Overhead: MongoDB Atlas automates tasks like patching, backups, and scaling, reducing the time and effort required to manage your database infrastructure.
- High Availability: Atlas provides automatic failover and replica set configuration, ensuring that your database remains available even in the event of failures.
- Automatic Scaling: Atlas automatically scales your database resources based on workload demand, ensuring that you don’t experience performance degradation as your application grows.
- Faster Time to Market: With MongoDB Atlas, you can quickly spin up and deploy databases without having to worry about infrastructure, allowing you to focus on building and deploying your application.
- Global Access: MongoDB Atlas allows you to deploy and access your database from anywhere in the world, providing fast and reliable data access to users in different regions.
Getting Started with MongoDB Atlas
To get started with MongoDB Atlas, follow these steps:
- Create an Atlas Account: Visit the MongoDB Atlas website and sign up for a free account.
- Set Up a Cluster: Once logged in, click on "Build a Cluster" to create a new cloud database cluster. You can choose your cloud provider, region, and cluster tier (there is a free tier available for smaller applications).
- Connect to the Cluster: After the cluster is provisioned, click "Connect" and follow the instructions to connect to your MongoDB Atlas cluster using your preferred method (e.g., MongoDB Shell, Compass, or application drivers).
- Create a Database and Collections: You can now create a database and collections in your cluster and start inserting data.
MongoDB Atlas Free Tier
MongoDB Atlas offers a free tier (M0 cluster) that allows you to deploy a small MongoDB database in the cloud with 512 MB of storage. The free tier is ideal for testing, development, and learning MongoDB. With the free tier, you get the following benefits:
- 1 replica set with 3 nodes for high availability
- 512 MB of storage
- Automated backups and monitoring
- Access to all MongoDB features
- Global distribution (limited regions)
Code Example: Connecting to MongoDB Atlas from Node.js
Here’s a simple example of how to connect to a MongoDB Atlas cluster from a Node.js
application using the mongoose
library:
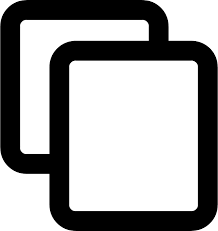
const mongoose = require('mongoose');
const uri = "your-atlas-connection-uri"; // Replace with your Atlas connection URI
mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => {
console.log("Connected to MongoDB Atlas");
})
.catch((err) => {
console.error("Error connecting to MongoDB Atlas:", err);
});
Diagram: MongoDB Atlas Architecture
The following diagram illustrates the architecture of a MongoDB Atlas cluster:

In this diagram, you can see how MongoDB Atlas clusters are distributed across multiple cloud regions for high availability and performance.
Setting Up a Local MongoDB Server
Setting up a local MongoDB server allows you to run MongoDB on your own machine, which is useful for development and testing purposes. In this guide, we will walk you through the process of installing MongoDB on Windows, macOS, and Linux, and setting up a local MongoDB server.
Prerequisites
Before you begin, ensure that you have the following requirements:
- A supported operating system (Windows, macOS, or Linux)
- Internet access to download MongoDB
Installing MongoDB on Windows
Follow these steps to install MongoDB on a Windows machine:
- Download MongoDB: Go to the MongoDB download center and select the appropriate version for Windows.
- Run the Installer: Once the download is complete, run the installer and follow the prompts. Make sure to check the box that says "Install MongoDB as a Service" to have MongoDB run as a background service.
- Choose Installation Options: Select "Complete" to install MongoDB with all features, including the MongoDB Compass GUI.
- Start MongoDB: After installation, MongoDB should start automatically
as a service. To check, open a command prompt and type
mongo
. If everything is set up correctly, you should see the MongoDB shell prompt.
Installing MongoDB on macOS
To install MongoDB on macOS, we will use Homebrew:
- Install Homebrew: If you don't have Homebrew installed, open the terminal and run the following command:
- Tap MongoDB Formula: Run the following command to tap the MongoDB formula in Homebrew:
- Install MongoDB: Install MongoDB using the following command:
- Start MongoDB: After installation, start MongoDB with the following command:
- Verify the Installation: To verify that MongoDB is running, type
mongo
in the terminal. You should be connected to the MongoDB shell.
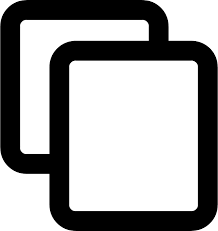
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
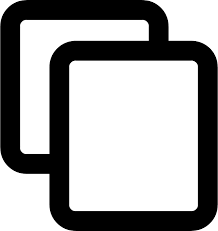
brew tap mongodb/brew
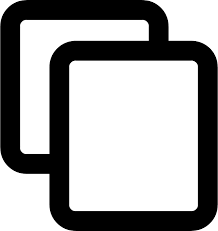
brew install mongodb-community@5.0
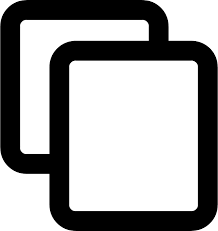
brew services start mongodb/brew/mongodb-community
Installing MongoDB on Linux
On Linux, you can install MongoDB using the package manager (e.g., apt
for
Ubuntu or yum
for CentOS).
For Ubuntu:
- Import MongoDB Public Key: Run the following command to import the MongoDB public key used by the package management system:
- Create the MongoDB List File: Create the /etc/apt/sources.list.d/mongodb-org-5.0.list file for MongoDB 5.0:
- Update the Package Database: Update the package database to include the MongoDB packages:
- Install MongoDB: Install MongoDB using the following command:
- Start MongoDB: To start MongoDB, use the following command:
- Verify the Installation: To verify that MongoDB is running, check the status of the MongoDB service:
- Enable MongoDB to Start on Boot: To ensure MongoDB starts automatically when the system boots, run:
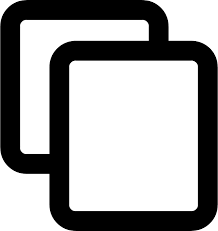
wget -qO - https://www.mongodb.org/static/pgp/server-5.0.asc | sudo apt-key add -
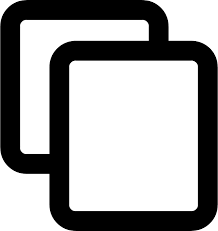
echo "deb [ arch=amd64,arm64 ] https://repo.mongodb.org/apt/ubuntu focal/mongodb-org/5.0 multiverse" | sudo tee /etc/apt/sources.list.d/mongodb-org-5.0.list
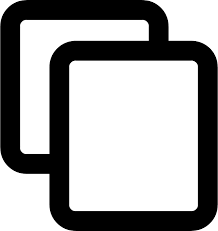
sudo apt-get update
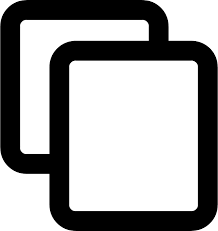
sudo apt-get install -y mongodb-org
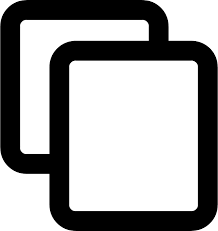
sudo systemctl start mongod
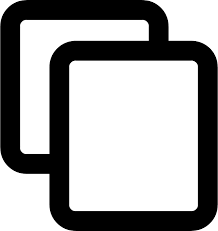
sudo systemctl status mongod
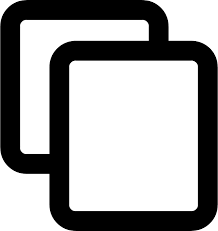
sudo systemctl enable mongod
Configuring MongoDB
After installing MongoDB, you may want to configure it for your specific use case. MongoDB's
configuration file (mongod.conf
) allows you to set options such as:
- Port number and bind IP address
- Storage options (e.g., data directory)
- Security settings, including authentication and authorization
- Replica set configurations (for replication)
The default configuration file is typically located in:
- Windows:
C:\Program Files\MongoDB\Server\5.0\bin\mongod.cfg
- macOS/Linux:
/etc/mongod.conf
Starting and Stopping MongoDB
MongoDB can be started and stopped using the following commands:
- Start MongoDB:
mongod
(for manual starting) orsudo systemctl start mongod
(for systemd) - Stop MongoDB:
sudo systemctl stop mongod
Accessing MongoDB
Once MongoDB is running, you can access it using the MongoDB shell or connect to it via MongoDB Compass, a graphical user interface (GUI) for MongoDB.
- MongoDB Shell: Run the
mongo
command to access the MongoDB shell. - MongoDB Compass: Download MongoDB Compass from the MongoDB website and use it to connect to your local MongoDB instance.
Diagram: Local MongoDB Setup
The following diagram shows a basic architecture of a local MongoDB setup:

This diagram illustrates the components of a local MongoDB setup, including the MongoDB server (mongod), the MongoDB client (mongo shell), and data storage.
Connecting to MongoDB Using the MongoDB Shell
The MongoDB shell is an interactive JavaScript interface to MongoDB, allowing you to perform CRUD (Create, Read, Update, Delete) operations, query data, and manage your MongoDB databases. In this guide, we will show you how to connect to MongoDB using the MongoDB shell.
Prerequisites
Before connecting to MongoDB, ensure that the following prerequisites are met:
- MongoDB is installed and running on your local machine or a remote server.
- You have access to the MongoDB server and its connection details (hostname, port, etc.).
Launching the MongoDB Shell
To start the MongoDB shell, open your terminal or command prompt and run the following command:
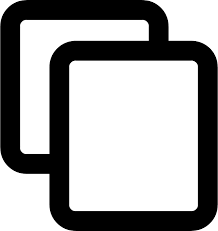
mongo
This will connect you to the MongoDB server running on localhost
(default) and
port 27017
(default). If the connection is successful, you will be greeted with
the MongoDB shell prompt, which looks like this:
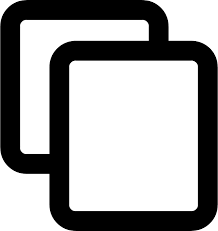
MongoDB shell version v5.0.0
connecting to: mongodb://127.0.0.1:27017
>
Connecting to a Remote MongoDB Server
If MongoDB is running on a remote server, you need to specify the hostname and port number in the connection command. Use the following format:
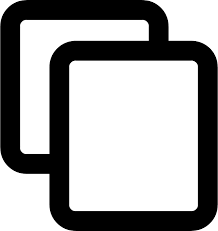
mongo :
For example, if MongoDB is running on a remote server with IP address
192.168.1.100
and port 27017
, the command would be:
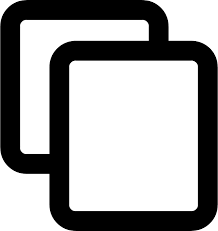
mongo 192.168.1.100:27017
If the MongoDB instance requires authentication, you can add the username and password using the following format:
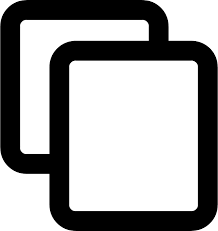
mongo -u -p --authenticationDatabase :
For example:
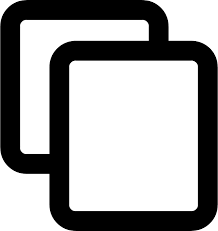
mongo -u admin -p password --authenticationDatabase admin 192.168.1.100:27017
Connecting to MongoDB with Authentication
If MongoDB requires authentication, you can use the -u
and -p
flags
to pass the username and password:
-u
: Specifies the username to authenticate with.-p
: Specifies the password for the username.--authenticationDatabase
: Specifies the database that contains the user's credentials (usually theadmin
database).
Example connection with authentication:
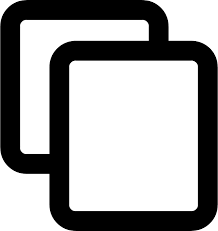
mongo -u myUser -p myPassword --authenticationDatabase admin 192.168.1.100:27017
Switching Databases in MongoDB
Once you are connected to the MongoDB shell, you can switch between databases using the
use
command. For example, to switch to the test
database:
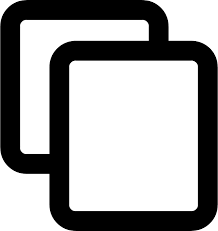
use test
If the database does not exist, MongoDB will create it when you insert data into it.
Performing Basic Operations in MongoDB Shell
Once connected to MongoDB, you can perform basic CRUD operations using the shell. Below are some examples:
Insert a Document
To insert a document into a collection, use the insertOne
or
insertMany
methods:
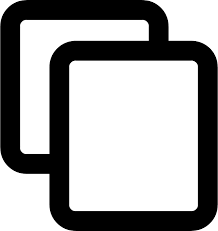
db.users.insertOne({ name: "John Doe", age: 30 })
Find Documents
To find documents in a collection, use the find
method:
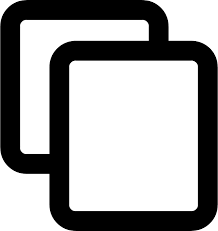
db.users.find({ name: "John Doe" })
Update Documents
To update documents in a collection, use the updateOne
or
updateMany
methods:
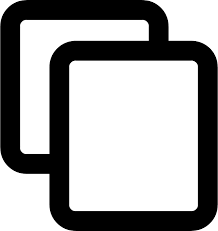
db.users.updateOne({ name: "John Doe" }, { $set: { age: 31 } })
Delete Documents
To delete documents from a collection, use the deleteOne
or
deleteMany
methods:
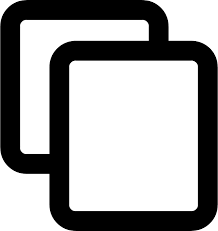
db.users.deleteOne({ name: "John Doe" })
Exiting the MongoDB Shell
To exit the MongoDB shell, simply type exit
at the prompt:
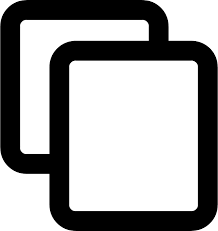
exit
This will close your connection to MongoDB and exit the shell.
Diagram: MongoDB Shell Interaction
The following diagram shows the interaction flow between the MongoDB shell and the MongoDB server:

This diagram illustrates how you can interact with the MongoDB server using the MongoDB shell for executing queries and performing CRUD operations.
Introduction to MongoDB Compass (GUI for MongoDB)
MongoDB Compass is the official graphical user interface (GUI) for MongoDB, designed to provide a simple way to interact with your MongoDB databases. Compass allows you to perform various tasks like managing collections, querying data, analyzing schema, and visualizing your data in an intuitive interface.
Key Features of MongoDB Compass
MongoDB Compass includes several powerful features that make it easier to work with MongoDB:
- Intuitive GUI: Easy-to-use interface for interacting with your MongoDB database.
- Schema Visualization: Visualize the structure of your collections, including field types and data distributions.
- Query Builder: Build complex queries using a visual interface, without writing any MongoDB query syntax.
- Real-Time Performance Metrics: Monitor the performance of your MongoDB deployment in real time.
- Aggregation Pipeline Builder: Construct and test aggregation pipelines using a visual builder.
- Index Management: Easily manage indexes in your MongoDB collections to optimize query performance.
- Data Validation: Enforce data validation rules and ensure data quality.
Installing MongoDB Compass
MongoDB Compass is available for Windows, macOS, and Linux. Follow the steps below to install MongoDB Compass:
1. Download MongoDB Compass
Visit the official MongoDB Compass download page: MongoDB Compass Download.
2. Install MongoDB Compass
Follow the installation steps based on your operating system:
- Windows: Run the downloaded installer and follow the on-screen instructions.
- macOS: Open the downloaded .dmg file and drag MongoDB Compass into the Applications folder.
- Linux: Follow the installation instructions provided for your specific distribution.
3. Launch MongoDB Compass
Once installed, open MongoDB Compass. You’ll be greeted with the connection screen where you can connect to your local or remote MongoDB instance.
Connecting to MongoDB with MongoDB Compass
To connect to your MongoDB server using Compass, follow these steps:
- Open MongoDB Compass.
- Enter the connection details (hostname, port, username, password, etc.) in the connection dialog.
- Click Connect to establish the connection to your MongoDB server.
Exploring Your MongoDB Database in Compass
After connecting to your MongoDB instance, you can start exploring your databases and collections:
- On the left-hand side, you’ll see a list of databases. Click on a database to view its collections.
- Click on a collection to view its documents, index details, and schema.
- Use the Filter bar at the top to query specific documents in the collection.
- Click on the Aggregation tab to build aggregation pipelines using a visual interface.
Using the Query Builder
The query builder in MongoDB Compass allows you to build queries without writing code. You can filter your data, sort it, and project specific fields. Here’s how to use it:
- Select the collection you want to query.
- Click on the Filter field and use the dropdown menus to select the field, operator, and value you want to filter by.
- Click Find to execute the query and view the results.
Aggregation Pipeline Builder
The Aggregation Pipeline Builder in MongoDB Compass allows you to visually create aggregation queries. This tool helps you build and test your aggregation queries step-by-step.
To use the Aggregation Pipeline Builder:
- Click on the Aggregation tab for the desired collection.
- Click Add Stage to start building your aggregation pipeline.
- Select the aggregation operator (e.g., $match, $group, $sort) and add the necessary fields and parameters.
- Click Execute to view the results of your pipeline.
Monitoring Performance with MongoDB Compass
MongoDB Compass provides real-time performance metrics for your MongoDB server. You can monitor key metrics like:
- Current operations
- Database and collection statistics
- Index usage
- Replication status
Diagram: MongoDB Compass Interface
The following diagram illustrates the MongoDB Compass interface, highlighting the key sections and features:

This diagram helps you understand the layout and tools available within MongoDB Compass.
Understanding Databases, Collections, and Documents
In MongoDB, data is stored in a flexible, JSON-like format, which makes it different from traditional relational databases. MongoDB organizes its data into databases, collections, and documents. Understanding how these components work is key to using MongoDB effectively.
What is a Database?
A database in MongoDB is a container for collections. It holds all the data for a specific application or project. Each MongoDB instance can have multiple databases, and each database can have one or more collections. MongoDB does not require a predefined schema, meaning you can have different collections with different structures within the same database.
Example of creating a database in MongoDB:
use myDatabase; // Switch to the "myDatabase" database (it will be created if it does not exist)
In the example above, if the database "myDatabase" does not exist, MongoDB will automatically create it when you insert data or collections into it.
What is a Collection?
A collection in MongoDB is a group of related documents. Collections are similar to tables in relational databases, but unlike tables, they don’t require a predefined schema. This allows different documents in the same collection to have different fields and data types.
Collections are created automatically when you insert a document into them. If you try to query a collection that doesn’t exist, MongoDB will create it for you when you insert data.
Example of creating a collection in MongoDB:
db.createCollection("users"); // Creates a new collection named "users"
In this example, the collection "users" is created. You can then insert documents into it.
What is a Document?
A document in MongoDB is a single record in a collection. It is a set of key-value pairs, where the keys are strings and the values can be various data types, including strings, numbers, arrays, and even other documents (subdocuments). Documents in MongoDB are represented in the BSON (Binary JSON) format, which is a binary-encoded serialization of JSON-like documents.
Documents in MongoDB are flexible, meaning they can have different structures within the same collection. This flexibility allows MongoDB to store complex and hierarchical data in a way that traditional relational databases cannot.
Example of a document in MongoDB:
db.users.insertOne({
name: "John Doe",
age: 30,
email: "johndoe@example.com",
address: {
street: "123 Main St",
city: "New York",
state: "NY"
},
hobbies: ["reading", "traveling", "sports"]
});
In the example above, a document with fields like name
, age
,
email
, and address
is inserted into the "users" collection. The
address
field is a subdocument, and hobbies
is an array.
Key Differences Between Databases, Collections, and Documents
Component | Definition | Example |
---|---|---|
Database | A container for collections in MongoDB. | myDatabase |
Collection | A group of documents within a database. Similar to a table in relational databases. | users, orders, products |
Document | A single record in a collection, represented in BSON format. | { name: "John Doe", age: 30, email: "johndoe@example.com" } |
Diagram: MongoDB Structure
The following diagram illustrates the hierarchical structure of MongoDB's data storage, showing how databases, collections, and documents are related:

This diagram demonstrates how a MongoDB instance can contain multiple databases, each of which can contain multiple collections, and each collection can store multiple documents.
Data Types in MongoDB (Strings, Numbers, Arrays, Objects)
MongoDB, as a NoSQL database, supports a rich set of data types that allows for flexible and dynamic document structures. These data types are used to represent various kinds of data in MongoDB documents. Below, we’ll explore some of the most common data types used in MongoDB: strings, numbers, arrays, and objects.
String
A string in MongoDB is used to represent textual data. Strings are often used to store names, email addresses, descriptions, and other textual fields. MongoDB strings are UTF-8 encoded, which means they can store any characters in most languages.
Example of a string in MongoDB:
db.users.insertOne({
name: "John Doe",
email: "johndoe@example.com"
});
In the example above, the name
and email
fields are strings in the
"users" collection document.
Number
A number in MongoDB can be either an integer or a floating-point number. MongoDB supports different types of numbers, including 32-bit and 64-bit integers and double-precision floating-point numbers. Numbers are used to store numerical data such as age, price, and quantity.
Example of a number in MongoDB:
db.products.insertOne({
name: "Laptop",
price: 999.99,
quantity: 100
});
In the example above, the price
and quantity
fields are numbers in
the "products" collection document.
Array
An array in MongoDB is an ordered list of values. The values in an array can be of any data type, including strings, numbers, subdocuments, or even other arrays. Arrays allow you to represent multiple values for a single field.
Example of an array in MongoDB:
db.users.insertOne({
name: "Jane Doe",
hobbies: ["reading", "traveling", "sports"]
});
In the example above, the hobbies
field is an array containing multiple values
in the "users" collection document.
Object (Subdocument)
An object, also known as a subdocument in MongoDB, is a nested document inside another document. Objects are used to represent more complex data structures, such as addresses, orders, or any other related data. Subdocuments allow you to keep related information together and maintain an organized structure within a single document.
Example of an object in MongoDB:
db.users.insertOne({
name: "John Doe",
address: {
street: "123 Main St",
city: "New York",
state: "NY"
}
});
In the example above, the address
field is an object (subdocument) containing
nested fields like street
, city
, and state
.
Key Differences Between Data Types in MongoDB
Data Type | Description | Example |
---|---|---|
String | Used to represent textual data. UTF-8 encoded. | "John Doe", "johndoe@example.com" |
Number | Used to represent numerical values, including integers and floating-point numbers. | 999.99, 100 |
Array | Used to represent an ordered list of values, which can be of any data type. | ["reading", "traveling", "sports"] |
Object (Subdocument) | Used to represent a nested document within a document. | { street: "123 Main St", city: "New York", state: "NY" } |
Diagram: Data Types in MongoDB
The following diagram illustrates how different data types can be used in MongoDB documents:

This diagram shows how strings, numbers, arrays, and objects can be combined within a MongoDB document, making it highly flexible and suitable for complex data storage needs.
CRUD Operations in MongoDB (Create, Read, Update, Delete)
MongoDB provides a set of operations to perform basic CRUD (Create, Read, Update, Delete) actions on documents within collections. These operations allow for managing and manipulating data in MongoDB. Below is an overview of the most commonly used CRUD operations and their examples.
Create Operations
In MongoDB, data can be inserted into collections using the insertOne()
and
insertMany()
methods.
insertOne()
The insertOne()
method is used to insert a single document into a collection. If
the insertion is successful, the document is added to the collection.
Example of insertOne()
db.users.insertOne({
name: "Alice",
email: "alice@example.com",
age: 28
});
insertMany()
The insertMany()
method is used to insert multiple documents into a collection
at once. It is more efficient when inserting multiple documents.
Example of insertMany()
db.users.insertMany([
{ name: "Bob", email: "bob@example.com", age: 24 },
{ name: "Charlie", email: "charlie@example.com", age: 30 }
]);
Read Operations
MongoDB provides the find()
and findOne()
methods to read data from
collections. These methods allow you to query documents based on certain criteria.
find()
The find()
method is used to retrieve multiple documents that match the given
filter. It returns a cursor to the documents, which can be iterated over.
Example of find()
db.users.find({ age: { $gt: 25 } });
In this example, the find()
method retrieves all users whose age is greater than
25.
findOne()
The findOne()
method is used to retrieve a single document that matches the
given filter. It returns the first document that meets the criteria.
Example of findOne()
db.users.findOne({ email: "alice@example.com" });
In this example, the findOne()
method retrieves the first document that matches
the email "alice@example.com".
Update Operations
MongoDB provides the updateOne()
and updateMany()
methods to modify
existing documents in a collection. These methods allow you to update specific fields of
documents that match the given criteria.
updateOne()
The updateOne()
method is used to update a single document that matches the
specified filter. Only the first matching document will be updated.
Example of updateOne()
db.users.updateOne(
{ email: "alice@example.com" },
{ $set: { age: 29 } }
);
In this example, the updateOne()
method updates the age of the user with the
email "alice@example.com" to 29.
updateMany()
The updateMany()
method is used to update multiple documents that match the
given filter. All matching documents will be updated.
Example of updateMany()
db.users.updateMany(
{ age: { $lt: 30 } },
{ $set: { status: "young" } }
);
In this example, the updateMany()
method updates all users whose age is less
than 30 and sets their status to "young".
Delete Operations
MongoDB provides the deleteOne()
and deleteMany()
methods to remove
documents from a collection.
deleteOne()
The deleteOne()
method is used to delete a single document that matches the
specified filter. Only the first matching document will be deleted.
Example of deleteOne()
db.users.deleteOne({ email: "bob@example.com" });
In this example, the deleteOne()
method deletes the document of the user with
the email "bob@example.com".
deleteMany()
The deleteMany()
method is used to delete multiple documents that match the
specified filter. All matching documents will be deleted.
Example of deleteMany()
db.users.deleteMany({ age: { $lt: 20 } });
In this example, the deleteMany()
method deletes all users whose age is less
than 20.
CRUD Operations Summary
Operation | Method | Description |
---|---|---|
Create | insertOne(), insertMany() | Inserts one or many documents into a collection. |
Read | find(), findOne() | Retrieves documents from a collection based on a filter. |
Update | updateOne(), updateMany() | Updates one or many documents in a collection. |
Delete | deleteOne(), deleteMany() | Deletes one or many documents from a collection. |
Diagram: CRUD Operations
The following diagram illustrates the flow of MongoDB CRUD operations:

This diagram visually represents how CRUD operations work in MongoDB, from creating documents to deleting them.
Query Filters and Projection in MongoDB
In MongoDB, query filters and projection are essential tools for retrieving specific data from collections. Filters allow you to specify criteria for matching documents, and projection enables you to control which fields are included or excluded in the result set.
Query Filters
A query filter in MongoDB is used to specify the conditions that documents must meet to be
returned in the result set. Filters are typically created by using comparison operators such
as $eq
, $gt
, $lt
, and logical operators like
$and
, $or
.
Common Query Filter Operators
Operator | Description | Example |
---|---|---|
$eq |
Matches values that are equal to the specified value. | db.users.find({ age: { $eq: 28 } }) |
$gt |
Matches values that are greater than the specified value. | db.users.find({ age: { $gt: 25 } }) |
$lt |
Matches values that are less than the specified value. | db.users.find({ age: { $lt: 30 } }) |
$in |
Matches any of the values in an array. | db.users.find({ age: { $in: [25, 28] } }) |
$and |
Matches documents that satisfy all the conditions specified in the array. | db.users.find({ $and: [{ age: { $gt: 25 } }, { age: { $lt: 30 } }] })
|
$or |
Matches documents that satisfy at least one of the conditions specified in the array. | db.users.find({ $or: [{ age: { $lt: 25 } }, { age: { $gt: 30 } }] })
|
Example Query Filter
To find all users who are older than 25 but younger than 30:
db.users.find({ age: { $gt: 25, $lt: 30 } });
In this example, the query will return all documents where the age
field is
greater than 25 but less than 30.
Projection
Projection in MongoDB is used to specify which fields should be included or excluded in the query results. By default, MongoDB returns all fields of the documents that match the query filter. With projection, you can limit the fields returned to just those you need.
Including Fields in the Result
To include specific fields in the result, pass a projection document with the field names set
to 1
.
Example: Including Fields
To retrieve only the name
and age
fields of the documents:
db.users.find({}, { name: 1, age: 1 });
This query will return only the name
and age
fields for each
document that matches the query filter (if any).
Excluding Fields from the Result
To exclude specific fields from the result, pass a projection document with the field names
set to 0
.
Example: Excluding Fields
To retrieve all fields except the email
field:
db.users.find({}, { email: 0 });
This query will return all fields of each document except the email
field.
Combining Inclusion and Exclusion
Note that MongoDB does not allow combining inclusion and exclusion in the same projection. You can either include or exclude fields, but not both in the same query.
If you want to retrieve specific fields while excluding others, you must make sure your
projection document only includes fields with 1
or 0
as
appropriate.
Example: Invalid Projection (Inclusion and Exclusion)
Following query is invalid because it combines inclusion and exclusion:
db.users.find({}, { name: 1, email: 0 });
This will result in an error. MongoDB will not allow the combination of both inclusion and exclusion in a single projection document.
Query Filters with Projection Example
To find users whose age is greater than 25 and return only their name
and
age
fields:
db.users.find({ age: { $gt: 25 } }, { name: 1, age: 1 });
This query will return users who are older than 25, including only their name
and age
in the result.
Diagram: Query Filters and Projection
The following diagram illustrates the process of applying filters and projections in MongoDB:

This diagram shows how MongoDB first filters the documents based on the query filter and then applies the projection to limit the fields in the final result.
What are Indexes and Why Are They Important?
Indexes in MongoDB are special data structures that store a small portion of the data set in a way that makes it easier to quickly search and retrieve the documents you need. Indexes are essential for improving the performance of queries, especially when dealing with large datasets. Without indexes, MongoDB has to scan every document in a collection to find the matching documents, which can be slow and inefficient.
How Indexes Work
Indexes are created on specific fields in a MongoDB collection. When a query is executed, MongoDB uses the index to quickly locate the documents that match the query, instead of scanning the entire collection. An index works like a table of contents in a book, allowing the database to locate the relevant data more efficiently.
Types of Indexes
MongoDB supports several types of indexes, each optimized for different use cases:
- Single Field Index: The most basic index type, created on a single field. It allows for fast queries that filter based on that field.
- Compound Index: An index that is created on multiple fields. It is useful when you need to query documents based on multiple fields.
- Text Index: Used for full-text search in MongoDB. It allows you to search for text within string fields.
- Geospatial Index: Used for location-based queries, such as finding nearby places based on coordinates.
- Hashed Index: Used for sharding and distributing documents across a sharded cluster based on the hash of a field.
Creating Indexes
Indexes can be created using the createIndex()
method. Here is an example of
creating a simple index on the name
field:
db.users.createIndex({ name: 1 });
In this example, the 1
indicates ascending order. You can also use
-1
for descending order.
Example: Compound Index
To create a compound index on the name
and age
fields:
db.users.createIndex({ name: 1, age: -1 });
This index will be useful when running queries that filter based on both name
and age
.
Why Are Indexes Important?
Indexes are important because they significantly improve query performance. Without an index, MongoDB would have to perform a collection scan, which can be very slow if the collection contains a large number of documents. Indexes help MongoDB reduce the number of documents it needs to scan, resulting in faster query execution.
Benefits of Indexes
- Faster Queries: Indexes allow MongoDB to quickly locate the documents that match a query, improving query performance and reducing response time.
- Efficient Sorting: Indexes can also improve the performance of sorting operations by avoiding the need to scan the entire collection to order the results.
- Reduced Resource Usage: By reducing the amount of data that MongoDB needs to scan, indexes help save processing power, memory, and storage resources.
- Improved Scalability: Indexes help MongoDB handle large datasets efficiently and can improve scalability as the size of the data grows.
Trade-offs of Using Indexes
While indexes provide significant performance improvements, they also come with some trade-offs:
- Increased Storage Requirements: Indexes consume disk space. For large collections, this can be significant.
- Slower Write Operations: Every time a document is inserted, updated, or deleted, the corresponding indexes need to be updated as well. This can slow down write operations.
Removing Indexes
If an index is no longer needed, it can be dropped using the dropIndex()
method:
db.users.dropIndex({ name: 1 });
In this example, we drop the index created on the name
field.
Diagram: Indexing Process
The following diagram illustrates how MongoDB uses indexes to optimize query performance:

This diagram shows how MongoDB first creates an index on a field and then uses it to quickly locate the matching documents during a query.
Creating and Using Indexes (createIndex())
Indexes in MongoDB are essential for improving the performance of database queries. By
creating an index on a field, MongoDB can use it to speed up search operations, making it
faster to retrieve documents that match specific criteria. The createIndex()
method is used to create indexes on fields in MongoDB collections.
Creating Indexes with createIndex()
The createIndex()
method allows you to create an index on one or more fields in
a collection. This method accepts an object where the keys represent the field(s) to be
indexed and the values specify the index type (e.g., ascending or descending order).
Basic Syntax
The basic syntax of the createIndex()
method is as follows:
db.collection.createIndex({ field: 1 });
In this syntax:
db.collection
: The collection on which the index will be created.{ field: 1 }
: The field to be indexed, with1
for ascending order or-1
for descending order.
Example: Creating a Single Field Index
To create an ascending index on the name
field of a collection called
users
, you would use the following code:
db.users.createIndex({ name: 1 });
This will create an index on the name
field in ascending order. If you wanted to
create a descending index, you would use -1
:
db.users.createIndex({ name: -1 });
Example: Creating a Compound Index
A compound index is an index created on multiple fields. This can be useful when you need to perform queries that filter or sort based on more than one field.
For example, to create an index on both the name
and age
fields:
db.users.createIndex({ name: 1, age: -1 });
In this case, the index is created with the name
field in ascending order and
the age
field in descending order.
Using Indexes to Improve Query Performance
Once indexes are created, MongoDB automatically uses them to optimize the performance of queries. You do not need to explicitly reference indexes in your queries; MongoDB will decide the most efficient index to use based on the query.
Example: Query Using Indexed Field
If you have an index on the name
field, you can perform queries that utilize
this index:
db.users.find({ name: "John" });
MongoDB will use the index on the name
field to quickly locate all documents
where the name
is "John".
Index Options
When creating an index, you can specify additional options to control the behavior of the index. Here are a few common options:
- unique: Ensures that all values in the indexed field are unique. Useful
for fields like
_id
or email addresses. - name: Allows you to specify a custom name for the index.
- sparse: Creates an index that only includes documents that contain the indexed field. This is useful for fields that may not exist in all documents.
- expireAfterSeconds: Used for TTL (Time-To-Live) indexes, which automatically expire documents after a specified time.
Example: Creating a Unique Index
To create a unique index on the email
field in the users
collection:
db.users.createIndex({ email: 1 }, { unique: true });
This ensures that no two documents can have the same email
value.
Example: Creating a TTL Index
To create a TTL index on the createdAt
field to automatically delete documents
after 30 days:
db.users.createIndex({ createdAt: 1 }, { expireAfterSeconds: 2592000 });
The value 2592000
represents the number of seconds in 30 days.
Viewing Existing Indexes
You can view the existing indexes in a collection using the getIndexes()
method:
db.users.getIndexes();
This will return a list of all indexes in the users
collection.
Dropping Indexes
If you no longer need an index, you can drop it using the dropIndex()
method:
db.users.dropIndex({ name: 1 });
This will remove the index on the name
field from the users
collection.
Diagram: Index Creation Process
The following diagram illustrates the process of creating and using indexes in MongoDB:

In this diagram, you can see how an index is created and how MongoDB uses it during query execution to improve performance.
Types of Indexes (Single-Field, Compound, Text, etc.)
MongoDB supports various types of indexes to optimize the performance of database queries. These indexes are designed to handle different types of queries efficiently. Below are the common types of indexes in MongoDB:
1. Single-Field Index
A single-field index is created on a single field of a collection. It is the most basic form of indexing and is typically used when you frequently query on a single field.
Example: Creating an index on the name
field:
db.users.createIndex({ name: 1 });
This index will speed up queries that search for documents with a specific name
.
2. Compound Index
A compound index is an index created on multiple fields in a collection. Compound indexes can improve query performance when queries filter or sort based on more than one field. The order of fields in a compound index matters, as MongoDB uses the index in the order the fields are specified.
Example: Creating a compound index on the name
and age
fields:
db.users.createIndex({ name: 1, age: -1 });
This index will speed up queries that filter by both name
and age
,
and the results will be sorted by age
in descending order.
3. Text Index
A text index is used for full-text search in MongoDB. It allows you to search for words or phrases within string fields. You can create a text index on one or more string fields to enable text search capabilities, such as finding documents that contain specific words or phrases.
Example: Creating a text index on the description
field:
db.products.createIndex({ description: "text" });
With this index, you can perform text search queries like:
db.products.find({ $text: { $search: "laptop" } });
This query will return all products whose description contains the word "laptop".
4. Geospatial Index
Geospatial indexes are used to optimize queries that deal with geographic data, such as
locations and coordinates. MongoDB supports two types of geospatial indexes: 2d
indexes for flat (two-dimensional) data and 2dsphere
indexes for spherical
(earth-like) data.
Example: Creating a geospatial index on the location
field:
db.stores.createIndex({ location: "2dsphere" });
This index allows you to perform queries that calculate distances or search for stores within a specific radius.
5. Hashed Index
A hashed index is used when you want to distribute data evenly across shards in a sharded cluster. The index is based on the hash of the field value rather than the value itself, which helps with load balancing in sharded collections.
Example: Creating a hashed index on the user_id
field:
db.orders.createIndex({ user_id: "hashed" });
This index is often used in sharded clusters to shard data based on the hashed value of a field.
6. Wildcard Index
A wildcard index is a special type of index that indexes all fields in a document, including nested fields. It is useful when you want to create an index on all fields in a collection without specifying each field individually.
Example: Creating a wildcard index on all fields:
db.articles.createIndex({ "$**": 1 });
This index will index all fields in the articles
collection, including fields
nested within subdocuments.
7. TTL (Time-To-Live) Index
A TTL index is used to automatically delete documents after a certain period of time. This is
useful for scenarios where data should expire, such as session data or logs. The
expireAfterSeconds
option specifies how long a document should stay in the
collection before it is automatically deleted.
Example: Creating a TTL index on the createdAt
field that expires documents
after 24 hours:
db.sessions.createIndex({ createdAt: 1 }, { expireAfterSeconds: 86400 });
This index will automatically delete documents in the sessions
collection after
24 hours (86400 seconds).
Choosing the Right Index
Choosing the right type of index depends on the types of queries you perform most often. Here are some guidelines:
- Use single-field indexes when you frequently query a single field.
- Use compound indexes when you query on multiple fields together, especially with sorting.
- Use text indexes when you need to perform full-text search operations.
- Use geospatial indexes for queries involving geographic locations.
- Use hashed indexes in sharded collections to distribute data evenly across shards.
- Use wildcard indexes when you need to index all fields in a collection, including nested fields.
- Use TTL indexes for data that has a limited lifespan, such as session data.
Diagram: Types of Indexes
The following diagram highlights the different types of indexes and when to use them:

This diagram illustrates the different types of indexes in MongoDB and their use cases.
Aggregation Framework Basics
The MongoDB aggregation framework provides a powerful toolset to process and analyze data stored in MongoDB collections. It allows you to perform operations such as filtering, grouping, sorting, and transforming data in a more complex way than simple queries. The aggregation framework processes data in stages, where each stage performs a specific operation on the data.
What is Aggregation?
Aggregation is the process of transforming data in MongoDB to retrieve useful information. It
can be used to perform operations such as grouping data by specific fields, sorting it,
filtering it, or calculating aggregates like sums, averages, or counts. MongoDB provides the
aggregate
method to perform aggregation queries, which is based on pipelines.
Aggregation Pipeline
The aggregation pipeline is a framework that processes data through a series of stages, where each stage transforms the data in a specific way. Each stage in the pipeline takes the input from the previous stage and outputs the modified data to the next stage. This allows you to build complex queries that perform multiple operations in sequence.
The basic structure of an aggregation pipeline consists of an array of stages. Each stage is defined as an object in the array, and each stage uses a MongoDB aggregation operator to specify the operation to be performed.
Common Aggregation Operators
There are several important aggregation operators that are used within the stages of the pipeline:
- $match: Filters documents based on the specified criteria (similar to
the
find()
query). - $group: Groups documents together based on a specified field or expression and performs aggregation operations like sum, average, etc.
- $sort: Sorts the documents in ascending or descending order.
- $project: Specifies which fields to include or exclude in the output documents and can also create new fields based on existing ones.
- $limit: Limits the number of documents to return.
- $skip: Skips a specified number of documents.
- $unwind: Deconstructs an array field and outputs one document for each element in the array.
- $count: Counts the number of documents in a group.
Basic Aggregation Example
Let’s see a basic example of how an aggregation pipeline works. Consider a collection of
orders
with fields such as item
, quantity
, and
price
. You can use an aggregation pipeline to calculate the total sales for
each item.
db.orders.aggregate([
{ $group: { _id: "$item", totalSales: { $sum: { $multiply: ["$quantity", "$price"] } } } },
{ $sort: { totalSales: -1 } }
]);
In this example, the pipeline contains two stages:
- The first stage uses
$group
to group documents by theitem
field and calculate the total sales by multiplying thequantity
with theprice
. - The second stage uses
$sort
to sort the results bytotalSales
in descending order.
Aggregation Stage Examples
$match: Filtering Documents
The $match
stage filters documents based on a specified condition. It is similar
to the find()
query.
db.orders.aggregate([
{ $match: { status: "shipped" } }
]);
$group: Grouping Data
The $group
stage is used to group documents by a specified field and to compute
aggregates like sums, averages, or counts.
db.orders.aggregate([
{ $group: { _id: "$item", totalQuantity: { $sum: "$quantity" } } }
]);
$project: Reshaping Documents
The $project
stage allows you to include or exclude fields from the output
documents or create new fields.
db.orders.aggregate([
{ $project: { item: 1, totalPrice: { $multiply: ["$quantity", "$price"] } } }
]);
$sort: Sorting Data
The $sort
stage sorts documents based on one or more fields.
db.orders.aggregate([
{ $sort: { totalSales: -1 } }
]);
$unwind: Deconstructing Arrays
The $unwind
stage is used to deconstruct an array field and output a separate
document for each element in the array.
db.orders.aggregate([
{ $unwind: "$items" }
]);
Aggregation Pipeline in Action
The aggregation pipeline enables powerful data processing by chaining multiple stages together. Each stage allows you to refine the data step by step. The result is a transformed set of documents that meet the desired criteria.
For example, here is a complete pipeline that filters documents based on a condition, groups them, and sorts them:
db.orders.aggregate([
{ $match: { status: "shipped" } },
{ $group: { _id: "$item", totalQuantity: { $sum: "$quantity" } } },
{ $sort: { totalQuantity: -1 } }
]);
Diagram: Aggregation Pipeline
The following diagram illustrates how data flows through the aggregation pipeline, with each stage transforming the data:

This diagram shows how each stage of the pipeline processes the data and passes it to the next stage.
Using $match, $group, $project, $sort
The MongoDB aggregation framework allows for powerful data processing and transformation
using several operators. Four of the most commonly used operators are $match
,
$group
, $project
, and $sort
. These operators enable
you to filter, group, reshape, and sort your data within an aggregation pipeline.
$match: Filtering Data
The $match
stage filters the documents in the pipeline based on specified
conditions. It works similarly to the find()
query, allowing you to filter
documents that match a specific criterion.
For example, to filter documents where the status
field is equal to "shipped",
you can use the following aggregation:
db.orders.aggregate([
{ $match: { status: "shipped" } }
]);
The $match
stage comes first in the pipeline, as it limits the documents that
are passed to the next stages.
$group: Grouping Data
The $group
stage is used to group documents by a specific field or expression
and to compute aggregates like sums, averages, or counts. It is commonly used for performing
calculations like total sales, total quantity, or average price.
For example, to group orders by item and calculate the total quantity sold for each item, use the following aggregation:
db.orders.aggregate([
{ $group: { _id: "$item", totalQuantity: { $sum: "$quantity" } } }
]);
In this example, the $group
stage groups documents by the item
field and sums the quantity
for each item.
$project: Reshaping Documents
The $project
stage is used to reshape documents by including or excluding
fields, or by adding new fields based on existing fields. This stage is useful when you want
to control the structure of the output documents.
For example, to project only the item
and totalPrice
fields, where
totalPrice
is calculated by multiplying the quantity
by the
price
, use the following aggregation:
db.orders.aggregate([
{ $project: { item: 1, totalPrice: { $multiply: ["$quantity", "$price"] } } }
]);
The $project
stage allows you to control which fields are included in the output
and can also create new fields derived from existing ones.
$sort: Sorting Data
The $sort
stage allows you to sort documents based on one or more fields. You
can specify whether to sort the data in ascending or descending order.
For example, to sort the documents by totalSales
in descending order, use the
following aggregation:
db.orders.aggregate([
{ $project: { totalSales: { $multiply: ["$quantity", "$price"] } } },
{ $sort: { totalSales: -1 } }
]);
The $sort
stage follows the $project
stage in this example to sort
the documents based on the newly calculated totalSales
field.
Combining $match, $group, $project, and $sort
These stages can be combined in a single aggregation pipeline to perform more advanced data processing. For example, to filter documents, group them by item, calculate the total sales, and sort the items by total sales in descending order, use the following aggregation:
db.orders.aggregate([
{ $match: { status: "shipped" } },
{ $group: { _id: "$item", totalSales: { $sum: { $multiply: ["$quantity", "$price"] } } } },
{ $sort: { totalSales: -1 } },
{ $project: { item: "$_id", totalSales: 1, _id: 0 } }
]);
In this example:
- The
$match
stage filters the orders by status. - The
$group
stage groups the orders by item and calculates the total sales. - The
$sort
stage sorts the items by total sales in descending order. - The
$project
stage reshapes the documents to include theitem
andtotalSales
fields, excluding the_id
field.
Diagram: Aggregation Pipeline Flow
The following diagram illustrates how each stage in the aggregation pipeline works together to process the data:

This diagram shows the flow of data through the stages and how each stage performs a specific operation on the data before passing it to the next stage.
Aggregation Pipelines for Data Transformation
In MongoDB, the aggregation framework allows you to process and transform data using an aggregation pipeline. An aggregation pipeline is a series of stages that transform data into a desired format. Each stage in the pipeline performs a specific operation on the data, and the output of one stage becomes the input for the next. This allows for powerful data transformation, aggregation, and analysis.
What is an Aggregation Pipeline?
An aggregation pipeline consists of multiple stages, where each stage performs a transformation on the data. The result of each stage is passed to the next stage in the pipeline. The stages can include operations like filtering, grouping, sorting, reshaping, and more. The data flows through each stage in a specific order, and each stage can modify or transform the data in various ways.
Basic Structure of an Aggregation Pipeline
Each stage in an aggregation pipeline is represented by an object that specifies the
operation to be performed. Common stages include $match
, $group
,
$project
, $sort
, and others. The pipeline can be constructed as an
array of these stages:
db.orders.aggregate([
{ $match: { status: "shipped" } },
{ $group: { _id: "$item", totalSales: { $sum: "$quantity" } } },
{ $sort: { totalSales: -1 } }
]);
In this example, the pipeline consists of three stages:
- The
$match
stage filters the documents to include only those where thestatus
field is "shipped". - The
$group
stage groups the documents byitem
and sums thequantity
field for each group to calculate total sales. - The
$sort
stage sorts the results bytotalSales
in descending order.
Data Transformation Using Aggregation Stages
Aggregation pipelines allow you to transform data into various formats. Some common use cases for data transformation include:
Reshaping Data with $project
The $project
stage is often used to reshape documents by including or excluding
fields, or by adding new fields based on existing ones. It allows you to modify the
structure of the document to match your desired output.
For example, to include only the item
and totalQuantity
fields and
exclude the _id
field, you can use:
db.orders.aggregate([
{ $project: { item: 1, totalQuantity: { $sum: "$quantity" }, _id: 0 } }
]);
This reshapes the document to include only the necessary fields for the output.
Adding New Fields with $addFields
The $addFields
stage allows you to add new fields to documents in the pipeline.
For example, you can add a new field called totalPrice
by multiplying the
quantity
and price
fields:
db.orders.aggregate([
{ $addFields: { totalPrice: { $multiply: ["$quantity", "$price"] } } }
]);
This adds the totalPrice
field to each document based on the existing fields.
Grouping and Aggregating Data with $group
The $group
stage is used for grouping documents based on a specific field and
performing aggregation operations, such as summing or averaging values. For instance, you
can group orders by item
and calculate the total price for each item:
db.orders.aggregate([
{ $group: { _id: "$item", totalPrice: { $sum: { $multiply: ["$quantity", "$price"] } } } }
]);
This groups the documents by item
and calculates the total price for each item
by multiplying quantity
and price
.
Sorting Data with $sort
The $sort
stage is used to sort the documents in ascending or descending order
based on one or more fields. For example, to sort the aggregated total price in descending
order, you can use:
db.orders.aggregate([
{ $group: { _id: "$item", totalPrice: { $sum: { $multiply: ["$quantity", "$price"] } } } },
{ $sort: { totalPrice: -1 } }
]);
This sorts the grouped results based on the totalPrice
field in descending
order.
Complex Data Transformations with Multiple Stages
For more advanced data transformations, you can combine multiple stages in an aggregation pipeline. For example, you might want to filter data, group it, add new fields, and then sort it:
db.orders.aggregate([
{ $match: { status: "shipped" } },
{ $group: { _id: "$item", totalSales: { $sum: "$quantity" }, totalPrice: { $sum: { $multiply: ["$quantity", "$price"] } } } },
{ $project: { item: "$_id", totalSales: 1, totalPrice: 1, _id: 0 } },
{ $sort: { totalSales: -1 } }
]);
This complex aggregation pipeline filters documents by status, groups by item, calculates total sales and total price, reshapes the documents, and finally sorts the results by total sales in descending order.
Diagram: Aggregation Pipeline for Data Transformation
The following diagram illustrates how the data flows through various stages of the aggregation pipeline and how each stage transforms the data:

This diagram shows the sequential flow of data from one transformation stage to the next, providing a visual representation of the entire aggregation process.
Schema Design and Best Practices
Schema design is a crucial part of building a MongoDB application. Unlike relational databases, MongoDB is a NoSQL database, which means it doesn't rely on predefined schemas for tables and relationships. Instead, MongoDB uses flexible, document-based schemas. However, even with this flexibility, there are best practices to follow to ensure efficient storage, fast queries, and maintainable code. In this section, we’ll explore MongoDB schema design principles and best practices.
Understanding Schema Design in MongoDB
In MongoDB, data is stored in the form of documents, which are organized into collections. A schema defines the structure of these documents, such as the fields they contain, the types of data they store, and the relationships between different collections. While MongoDB is schema-less in a strict sense, it's still a good practice to define an expected structure for the documents to maintain consistency and optimize performance.
Designing a MongoDB Schema
When designing a schema for MongoDB, there are a few key considerations that will directly impact performance, scalability, and flexibility:
- Data Modeling: Choose between embedding documents (denormalization) and referencing documents (normalization) based on the application needs.
- Scalability: Consider how the schema design will scale as the application grows, particularly with regard to sharding and indexing.
- Query Patterns: Think about how the data will be queried. Schema design should align with common query patterns to ensure efficient data retrieval.
Best Practices for MongoDB Schema Design
Here are some best practices to follow when designing schemas in MongoDB:
1. Choose Between Embedding and Referencing
MongoDB supports two main ways to model relationships between data:
- Embedding (Denormalization): Embed related data within a document when the related data is frequently accessed together. This is ideal for one-to-few relationships and reduces the need for joins.
- Referencing (Normalization): Use references when data is shared across many documents or when the data is updated frequently. This is ideal for one-to-many or many-to-many relationships, but it may require additional queries or joins.
2. Use Proper Data Types
When defining your schema, ensure that you choose the appropriate data type for each field to optimize storage and query performance. For example, use integers or doubles for numeric data, strings for textual data, and arrays for list-like data structures.
3. Avoid Large Documents
MongoDB has a document size limit (currently 16 MB). Avoid storing large objects or arrays in a single document, as this can lead to performance issues. Instead, break large data into smaller, more manageable chunks and use references if necessary.
4. Use Indexes Wisely
Indexes play a significant role in query performance. Create indexes on fields that are frequently queried or used in sorting. However, avoid over-indexing, as it can lead to increased storage overhead and slower write operations.
5. Plan for Data Growth and Sharding
As your application grows, you may need to scale horizontally. Consider how your schema will perform with sharding in mind. Choose an appropriate shard key based on your application’s query patterns to ensure data is evenly distributed across shards.
6. Use the Correct Schema Validation
Although MongoDB is schema-less, it’s possible to define schema validation rules to enforce
structure and data integrity. Use the validator
option when creating
collections to apply restrictions on document fields, ensuring that your data adheres to the
expected format.
7. Be Mindful of Denormalization
While embedding documents is useful for performance, it can lead to data redundancy and challenges with updates. In cases where you need to frequently update embedded data, consider using references instead to avoid redundancy and make updates easier.
Example Schema Design: Blog Application
Let’s look at an example schema design for a simple blog application, which includes users, posts, and comments. In this case, we’ll use a combination of embedded documents and references:
// Users collection with embedded posts
{
_id: ObjectId("..."),
username: "john_doe",
email: "john.doe@example.com",
posts: [
{
_id: ObjectId("..."),
title: "My First Post",
content: "This is the content of the post...",
comments: [
{
_id: ObjectId("..."),
author: "jane_doe",
content: "Great post!"
},
{
_id: ObjectId("..."),
author: "john_doe",
content: "Thanks for reading!"
}
]
}
]
}
// Posts collection with reference to users
{
_id: ObjectId("..."),
userId: ObjectId("..."), // Reference to Users
title: "My First Post",
content: "This is the content of the post...",
comments: [
{
_id: ObjectId("..."),
author: "jane_doe",
content: "Great post!"
}
]
}
In this design:
- The
users
collection contains embedded posts and comments, as users typically access their posts and comments together. - The
posts
collection contains a reference to the user who created the post, allowing you to query posts based on user information.
Diagram: Example Schema Design
The following diagram illustrates the relationship between the collections and how data is organized in the blog application schema:

This diagram highlights how users, posts, and comments are related in the MongoDB schema and provides a visual representation of the data flow.
Conclusion
MongoDB's flexible schema design allows developers to model data in ways that make sense for their applications. By following best practices, such as choosing between embedding and referencing, using proper data types, and considering scalability, you can design an efficient and scalable MongoDB schema. Always consider your application’s query patterns and future growth to ensure optimal performance and maintainability.
Embedded vs. Referenced Documents
One of the key decisions when designing a MongoDB schema is determining whether to embed documents within other documents or to use references between documents. The choice between embedded and referenced documents depends on the specific use case, data access patterns, and performance considerations. In this section, we'll explore the differences between embedded and referenced documents, their advantages, and when to use each approach.
Embedded Documents
In MongoDB, embedding means storing related data within a single document. This approach is typically used when data is frequently accessed together and the size of the embedded data is manageable. Embedded documents are stored as arrays or sub-documents within the parent document. Embedding is a denormalization technique, which means that all related data is contained within one document, reducing the need for additional queries or joins.
Advantages of Embedded Documents
- Performance: Embedded documents are fast to retrieve because all related data is stored together in one document, reducing the need for additional queries or joins.
- Atomic Operations: When updating or deleting embedded documents, MongoDB guarantees atomicity at the document level, meaning the entire document is updated in a single operation.
- Simplified Data Access: Since all related data is stored together, accessing it requires only a single query, which is more efficient than multiple queries across collections.
When to Use Embedded Documents
- When the data is frequently accessed together and does not require frequent updates.
- For one-to-few relationships where the embedded data is not too large.
- When data consistency is important, and you need all the related data to be updated atomically.
Example of Embedded Documents
Let's consider a "Blog" application where each blog post has a list of comments. In this case, it might make sense to embed the comments within the blog post document:
{
_id: ObjectId("..."),
title: "My First Post",
content: "This is the content of the post...",
comments: [
{
_id: ObjectId("..."),
author: "jane_doe",
content: "Great post!"
},
{
_id: ObjectId("..."),
author: "john_doe",
content: "Thanks for reading!"
}
]
}
Referenced Documents
In MongoDB, referencing means storing data in separate documents and linking them together using references (usually via an ObjectId). This approach is commonly used when data is shared across multiple documents or when the data changes independently of the parent document. Referencing is a normalization technique, where related data is stored separately, and you need to perform multiple queries to retrieve the full set of related data.
Advantages of Referenced Documents
- Data Reusability: Referencing helps avoid data duplication, especially when the same data is used in multiple places (e.g., a user's profile is referenced in multiple posts or comments).
- Reduced Document Size: Since the data is stored in separate documents, the document size stays small, which can help with performance when dealing with large datasets.
- Easier Updates: When referenced data changes (e.g., a user’s profile information), it only needs to be updated once, rather than in every embedded document.
When to Use Referenced Documents
- When data is shared across multiple documents, and storing it multiple times would cause redundancy.
- For many-to-many relationships or one-to-many relationships where data may be updated independently.
- When the embedded data may grow too large or when documents would exceed MongoDB’s 16 MB document size limit.
Example of Referenced Documents
In the same "Blog" application, let's assume that we store comments in a separate collection and reference them in the blog post. This allows us to reuse the same comment across multiple posts:
// Blog Post Collection
{
_id: ObjectId("..."),
title: "My First Post",
content: "This is the content of the post...",
comments: [
ObjectId("..."), // Reference to Comment
ObjectId("...") // Reference to Comment
]
}
// Comment Collection
{
_id: ObjectId("..."),
postId: ObjectId("..."), // Reference to Blog Post
author: "jane_doe",
content: "Great post!"
}
Comparison: Embedded vs. Referenced Documents
Let's summarize the key differences between embedded and referenced documents:
Criteria | Embedded Documents | Referenced Documents |
---|---|---|
Data Size | Can lead to larger document sizes if embedded data is large | Keeps document sizes small by storing data separately |
Data Access | Faster access since data is stored in the same document | Requires multiple queries or joins to retrieve related data |
Data Duplication | Data may be duplicated across documents | Reduces data duplication by storing related data separately |
Updates | Easy atomic updates for data that is embedded | Requires careful management to ensure consistency across references |
Use Case | Best for one-to-few relationships, frequently accessed together data | Best for many-to-many relationships, shared data |
Conclusion
Choosing between embedded and referenced documents depends on the specific needs of your application. Embedded documents are ideal for data that is frequently accessed together and does not change often, while referenced documents are more suitable for shared or large data that needs to be updated independently. By understanding the trade-offs between these two approaches, you can make informed decisions when designing your MongoDB schema to optimize for performance, scalability, and maintainability.
One-to-One, One-to-Many, and Many-to-Many Relationships
When working with MongoDB, it's important to understand how to represent different types of relationships between documents. In traditional relational databases, relationships are often represented using foreign keys and joins. However, in MongoDB, relationships are typically represented using embedded documents or references between documents. In this section, we will explore the three main types of relationships in MongoDB: one-to-one, one-to-many, and many-to-many.
One-to-One Relationship
A one-to-one relationship occurs when a single document in one collection is associated with a single document in another collection. This type of relationship is typically represented by embedding the related document within the parent document or by using references between documents. A common example of a one-to-one relationship is a user's profile, where each user has one profile.
Example of One-to-One Relationship
In this example, we will represent a user's profile as a sub-document embedded within the user document. This way, each user has one profile:
// User Collection
{
_id: ObjectId("..."),
username: "john_doe",
email: "john.doe@example.com",
profile: {
age: 30,
gender: "male",
location: "New York"
}
}
When to Use One-to-One Relationships
- When the data is tightly related and should always be accessed together.
- When the size of the embedded document is small and manageable.
- For data that changes together and requires atomic updates.
One-to-Many Relationship
A one-to-many relationship occurs when a single document in one collection is associated with multiple documents in another collection. This is a common scenario where the parent document can have an array of references or embedded documents. A typical example of a one-to-many relationship is a blog post with many comments.
Example of One-to-Many Relationship
In this example, a blog post contains many comments. We will store the comments as an array of embedded documents within the blog post document:
// Blog Post Collection
{
_id: ObjectId("..."),
title: "My First Blog Post",
content: "This is the content of the post...",
comments: [
{
_id: ObjectId("..."),
author: "jane_doe",
content: "Great post!"
},
{
_id: ObjectId("..."),
author: "john_doe",
content: "Thanks for sharing!"
}
]
}
When to Use One-to-Many Relationships
- When the data is related but not always needed together, and the child data can grow independently of the parent.
- When you want to store multiple items (e.g., comments, orders) that are linked to a parent document.
- If data consistency is required, and atomic updates are needed for the entire parent document and its children.
Many-to-Many Relationship
A many-to-many relationship occurs when multiple documents in one collection are associated with multiple documents in another collection. In MongoDB, this is typically achieved by using references to link documents in both collections. For example, a user can belong to many groups, and a group can have many users.
Example of Many-to-Many Relationship
In this example, we will represent users and groups. The relationship between users and groups is many-to-many, so we store references to each group in the user's document and references to each user in the group's document:
// User Collection
{
_id: ObjectId("..."),
username: "john_doe",
email: "john.doe@example.com",
groups: [
ObjectId("..."), // Reference to Group
ObjectId("...")
]
}
// Group Collection
{
_id: ObjectId("..."),
name: "Tech Enthusiasts",
members: [
ObjectId("..."), // Reference to User
ObjectId("...")
]
}
When to Use Many-to-Many Relationships
- When multiple documents are related to multiple other documents.
- When the data is highly reusable and shared across multiple documents (e.g., users and groups, books and authors).
- When data can grow independently, and you need flexibility in how documents are related.
Comparison: One-to-One, One-to-Many, and Many-to-Many Relationships
Let's compare the key characteristics of each relationship type:
Relationship Type | Example | Data Access | Use Case |
---|---|---|---|
One-to-One | User and Profile | All data is accessed together in one document | For tightly related data that changes together |
One-to-Many | Blog Post and Comments | Parent document stores references to multiple child documents | For hierarchical data where one document can have many child documents |
Many-to-Many | User and Groups | Multiple documents are linked to multiple other documents | For complex relationships where data is shared and can grow independently |
Conclusion
Understanding the different types of relationships in MongoDB helps you design your schema more effectively. One-to-one relationships are useful for tightly coupled data, one-to-many relationships are ideal for hierarchical data, and many-to-many relationships provide flexibility for complex associations. By choosing the right relationship model, you can optimize your MongoDB schema for performance, scalability, and maintainability.
Polymorphic Patterns in MongoDB
In MongoDB, polymorphic patterns refer to scenarios where a document can reference multiple types of related documents, i.e., a single field can point to different types of documents in different collections. This is similar to polymorphism in object-oriented programming, where the same interface can be used for different types of data. Polymorphic patterns are especially useful when you want to store different types of related entities in a flexible way.
What are Polymorphic Patterns?
Polymorphic patterns allow a document to reference multiple kinds of related documents. For example, a comment on a blog post might refer to different types of content, such as blog posts, videos, or images. The polymorphic pattern allows comments to be linked to any content type without creating separate comment collections for each content type.
Types of Polymorphic Patterns
There are different ways to implement polymorphic patterns in MongoDB, but two common approaches are:
- Single Field Reference: A field stores a reference to any document type, and an additional field specifies the type of the referenced document.
- Embedded Documents: Use embedded documents that can store different types of data, potentially using a discriminator field to identify the type of data.
Single Field Reference Pattern
The single field reference pattern involves using a single reference field to point to a document from any collection and an additional field to store the type of the document. This method is useful when you want to reference different types of documents but keep the references flexible.
Example of Single Field Reference Pattern
Let’s say we have a collection of comments
, and these comments can reference
either a blogPost
or a video
. We can use a ref
field
to store the reference to the related document and a type
field to store the
type of the referenced document:
// Comment Collection
{
_id: ObjectId("..."),
content: "Great post!",
ref: ObjectId("..."), // Reference to a blog post or video
type: "blogPost" // Type of the referenced document
}
In the above example, the ref
field stores the ObjectId of the related document
(either a blog post or a video), while the type
field stores a string value
that indicates whether the reference is pointing to a blog post or a video.
When to Use Single Field Reference Pattern
- When you have different types of documents that need to be referenced together in a flexible way.
- When you need to store references to documents from different collections and easily identify the type of the referenced document.
- When you want to minimize the number of fields in a document and keep the schema flexible.
Embedded Documents with Discriminator Pattern
The embedded documents with discriminator pattern involves storing different types of data as embedded documents. You can add a discriminator field to indicate the type of data stored within the document. This pattern is especially useful when you want to store different types of data in the same collection but distinguish them based on their type.
Example of Embedded Documents with Discriminator Pattern
Let’s say we have a collection of media
documents, and we want to store both
images and videos in the same collection. We can store both types of content as embedded
documents, adding a type
field to each document to indicate whether it’s an
image or video:
// Media Collection
[
{
_id: ObjectId("..."),
type: "image",
url: "image1.jpg",
width: 800,
height: 600
},
{
_id: ObjectId("..."),
type: "video",
url: "video1.mp4",
duration: 120
}
]
In the example above, each media document has a type
field that indicates
whether the media is an image or video, and each document contains relevant fields for that
type of content. The image
document has width and height, whereas the
video
document has a duration field.
When to Use Embedded Documents with Discriminator Pattern
- When you want to store different types of data in the same collection while still distinguishing between their types.
- When the different types of data share a common structure and can be embedded together in a single document.
- When you want to query all content types from a single collection, but still retain type-specific fields for each content type.
Comparison: Single Field Reference vs Embedded Documents with Discriminator
Let’s compare the two common polymorphic patterns:
Pattern | Use Case | Advantages | Disadvantages |
---|---|---|---|
Single Field Reference | When you need to store references to different types of documents across collections | Flexible, easy to manage references between collections | Requires additional logic to fetch and join data, may result in extra queries |
Embedded Documents with Discriminator | When different types of data need to be stored in the same collection | Single collection, easy to query all types of data together | May lead to large documents, schema changes can be complex |
Conclusion
Polymorphic patterns in MongoDB offer a flexible way to model relationships between different types of documents. Whether you choose the single field reference pattern or the embedded documents with discriminator pattern depends on your use case and the nature of your data. By understanding these patterns, you can efficiently design schemas that can handle complex relationships while maintaining performance and scalability.
Using Comparison Operators ($gt, $lt, $in, etc.)
In MongoDB, comparison operators are used to filter documents based on specific conditions. They allow you to compare field values to a specified value or another field. These operators are essential for querying data efficiently and are used in MongoDB queries to retrieve documents that meet specific criteria.
Common Comparison Operators
MongoDB provides a variety of comparison operators to perform different kinds of comparisons on the data. Some of the most commonly used operators are:
$gt
: Greater than$lt
: Less than$gte
: Greater than or equal to$lte
: Less than or equal to$eq
: Equal to$ne
: Not equal to$in
: Matches any of the values in an array$nin
: Does not match any of the values in an array
Examples of Comparison Operators
Let’s look at examples of how these comparison operators are used in queries.
Using $gt (Greater Than)
The $gt
operator is used to find documents where the field value is greater than
a specified value.
db.products.find({ price: { $gt: 50 } })
This query will return all products where the price
field is greater than 50.
Using $lt (Less Than)
The $lt
operator is used to find documents where the field value is less than a
specified value.
db.products.find({ price: { $lt: 30 } })
This query will return all products where the price
field is less than 30.
Using $gte (Greater Than or Equal To)
The $gte
operator finds documents where the field value is greater than or equal
to a specified value.
db.products.find({ price: { $gte: 40 } })
This query will return all products where the price
is greater than or equal to
40.
Using $lte (Less Than or Equal To)
The $lte
operator finds documents where the field value is less than or equal to
a specified value.
db.products.find({ price: { $lte: 100 } })
This query will return all products where the price
is less than or equal to
100.
Using $eq (Equal To)
The $eq
operator finds documents where the field value is equal to a specified
value.
db.products.find({ category: { $eq: "electronics" } })
This query will return all products where the category
field is exactly
"electronics".
Using $ne (Not Equal To)
The $ne
operator finds documents where the field value is not equal to a
specified value.
db.products.find({ price: { $ne: 20 } })
This query will return all products where the price
is not equal to 20.
Using $in (Matches Any of the Values in an Array)
The $in
operator is used to match any of the values in an array. It’s helpful
when you want to query for multiple possible values.
db.products.find({ category: { $in: ["electronics", "books"] } })
This query will return all products where the category
is either "electronics"
or "books".
Using $nin (Does Not Match Any of the Values in an Array)
The $nin
operator is used to find documents where the field value does not match
any of the values in an array.
db.products.find({ category: { $nin: ["clothing", "furniture"] } })
This query will return all products where the category
is neither "clothing" nor
"furniture".
Combining Comparison Operators
You can also combine multiple comparison operators in a query to form more complex conditions. For example, if you want to find products that are both expensive and belong to a certain category, you could do something like this:
db.products.find({
price: { $gte: 50, $lte: 200 },
category: { $in: ["electronics", "appliances"] }
})
This query will return all products where the price
is between 50 and 200, and
the category
is either "electronics" or "appliances".
Conclusion
Comparison operators in MongoDB provide powerful ways to filter and retrieve documents based on specific conditions. By combining different operators, you can create complex queries to retrieve precisely the data you need. Understanding and utilizing these operators is fundamental to mastering MongoDB queries.
Logical Operators ($and, $or, $not)
Logical operators in MongoDB are used to combine multiple conditions and filter documents
based on these conditions. These operators allow for more complex queries and are commonly
used to enhance the flexibility of MongoDB queries. The main logical operators in MongoDB
are $and
, $or
, and $not
.
Common Logical Operators
The most commonly used logical operators in MongoDB are:
$and
: Combines multiple conditions and matches documents where all conditions are true.$or
: Combines multiple conditions and matches documents where at least one condition is true.$not
: Negates the condition, returning documents where the condition is false.
Examples of Logical Operators
Let’s look at examples of how these logical operators are used in queries.
Using $and (All Conditions Must Be True)
The $and
operator is used to combine multiple conditions, and documents are
returned only if they satisfy all conditions.
db.products.find({
$and: [
{ price: { $gte: 50 } },
{ category: "electronics" }
]
})
This query will return all products that are both priced at 50 or more and belong to the "electronics" category. In this case, both conditions must be true for the document to be included in the result.
Using $or (At Least One Condition Must Be True)
The $or
operator is used to combine multiple conditions, and documents are
returned if at least one condition is true.
db.products.find({
$or: [
{ category: "electronics" },
{ price: { $lte: 30 } }
]
})
This query will return products where the category
is "electronics" or the
price
is less than or equal to 30. Either condition being true will include the
document in the result.
Using $not (Negating a Condition)
The $not
operator is used to negate a condition, meaning documents will be
returned where the condition is false.
db.products.find({
price: { $not: { $gte: 100 } }
})
This query will return all products where the price
is not greater than or equal
to 100. Essentially, it retrieves products with a price less than 100.
Combining Logical Operators
You can also combine logical operators to form more complex queries. For example, if you want
to find products that are either "electronics" or "appliances" but do not have a price
greater than or equal to 200, you can use both the $or
and $not
operators together:
db.products.find({
$or: [
{ category: "electronics" },
{ category: "appliances" }
],
price: { $not: { $gte: 200 } }
})
This query will return products where the category is either "electronics" or "appliances",
and the price
is less than 200.
Conclusion
Logical operators in MongoDB allow you to build powerful queries by combining multiple
conditions. Whether you're using $and
to require multiple conditions to be
true, $or
to accept multiple conditions, or $not
to negate a
condition, these operators help you retrieve the exact data you need. Mastering these
logical operators is essential for performing complex queries in MongoDB.
Array Queries ($all, $elemMatch)
Array queries in MongoDB allow you to query for documents that contain arrays. MongoDB
provides operators like $all
and $elemMatch
to perform advanced
queries on array fields. These operators give you flexibility when working with arrays in
your documents.
Common Array Query Operators
The most commonly used array query operators in MongoDB are:
$all
: Matches documents where the array field contains all specified elements, regardless of order.$elemMatch
: Matches documents that contain an array with at least one element that satisfies the given query.
Examples of Array Queries
Let’s look at examples of how these array operators can be used in queries.
Using $all (Matching All Specified Elements in an Array)
The $all
operator is used to match documents where the array field contains all
the specified elements, regardless of order. It does not require the elements to be in a
particular order.
db.products.find({
tags: { $all: ["electronics", "sale"] }
})
This query will return documents where the tags
array contains both
"electronics" and "sale" elements, regardless of order. For example, the array could be
["sale", "electronics"]
or ["electronics", "sale"]
, and both would
match.
Using $elemMatch (Matching Array Elements that Satisfy a Query)
The $elemMatch
operator is used to query for documents where at least one
element in the array satisfies the specified query. It is especially useful when querying
for documents with arrays of embedded documents.
db.orders.find({
items: { $elemMatch: { product: "laptop", quantity: { $gte: 2 } } }
})
This query returns documents where the items
array contains at least one element
that has the product "laptop" with a quantity greater than or equal to 2. The
$elemMatch
operator allows you to match documents where the embedded document
in the array meets complex conditions.
Using $elemMatch with Multiple Conditions
You can also use $elemMatch
with multiple conditions to match more specific
array elements. For instance, if you want to find products that have a price less than 50
and are in stock, you can use:
db.products.find({
inventory: { $elemMatch: { price: { $lt: 50 }, inStock: true } }
})
This query will return documents where the inventory
array contains at least one
element where the price
is less than 50 and inStock
is true.
Combining $all and $elemMatch
You can also combine $all
and $elemMatch
to create more complex
queries. For example, if you want to find documents where the tags
array
contains both "electronics" and "sale", and at least one item in the items
array has a quantity greater than 3, you can combine both operators:
db.products.find({
tags: { $all: ["electronics", "sale"] },
items: { $elemMatch: { quantity: { $gt: 3 } } }
})
This query will return documents where the tags
array contains both
"electronics" and "sale", and the items
array contains at least one element
with a quantity greater than 3.
Conclusion
Array queries in MongoDB provide powerful ways to query and filter documents based on array
data. The $all
operator allows you to match documents that contain all
specified elements in an array, while $elemMatch
allows you to query for array
elements that satisfy complex conditions. Mastering these array query operators is essential
for working with array-based data in MongoDB.
Regular Expressions in Queries
In MongoDB, you can use regular expressions (regex) to perform pattern matching on string fields. Regular expressions provide a powerful way to search for documents that match specific patterns, making them useful for tasks like validating data, filtering records, or finding partial matches.
What Are Regular Expressions?
A regular expression (regex) is a sequence of characters that defines a search pattern. MongoDB supports Perl-compatible regular expressions (PCRE), which are widely used in many programming languages. Regular expressions are typically used for searching, replacing, and validating strings.
Using Regular Expressions in MongoDB Queries
You can use regular expressions in MongoDB queries with the $regex
operator.
This operator allows you to search for documents where a field matches a pattern defined by
the regular expression.
Basic Syntax of $regex
The basic syntax for using regular expressions in MongoDB queries looks like this:
db.collection.find({
field: { $regex: /pattern/ }
})
In this syntax, the field
is the name of the field you want to search, and
/pattern/
is the regular expression pattern. You can also include options like
i
for case-insensitive matching or m
for multiline matching.
Examples of Regular Expressions in Queries
Case-Insensitive Search
To perform a case-insensitive search, you can use the i
option. For example, to
find documents where the name
field contains the word "mongodb" regardless of
case, you can use the following query:
db.products.find({
name: { $regex: /mongodb/i }
})
This query will match documents where the name
field contains "mongodb",
"MongoDB", "MONGODB", or any other case variation.
Pattern Matching at the Start of a String
If you want to match documents where the field starts with a certain pattern, you can use the
caret (^
) symbol in the regular expression. For example, to find all products
whose name
starts with "prod", you can use:
db.products.find({
name: { $regex: /^prod/ }
})
This query will return documents where the name
field starts with "prod", such
as "product1" or "producer".
Pattern Matching at the End of a String
Similarly, to match documents where the field ends with a specific pattern, you can use the
dollar sign ($
) symbol. For example, to find all products whose
name
ends with "book", use:
db.products.find({
name: { $regex: /book$/ }
})
This query will return documents where the name
field ends with "book", such as
"storybook" or "notebook".
Matching Any Character
To match any character in a string, you can use the period (.
) symbol. For
example, to find all products whose name
has "phone" followed by any character,
use:
db.products.find({
name: { $regex: /phone./ }
})
This query will match names like "phone1", "phoneX", "phoneA", and so on.
Using $options for Regular Expression Flags
In addition to using regular expressions directly, you can also pass options for
case-insensitivity and multiline matching with the $options
field. For example,
the following query finds all products whose name
contains "prod" regardless of
case:
db.products.find({
name: { $regex: "prod", $options: "i" }
})
The $options
field allows you to pass flags like i
for
case-insensitive matching, m
for multiline matching, or s
for
dotall mode (matching newline characters with .
).
Limitations and Performance Considerations
While regular expressions are powerful, they can have performance implications, especially when used on large datasets. To optimize performance:
- Try to use regular expressions with a specific pattern (e.g., starting or ending characters).
- Avoid using regular expressions on large text fields unless necessary.
- Consider indexing the field you're querying on for faster matching.
Conclusion
Regular expressions provide a flexible way to search for patterns within string fields in MongoDB. They are ideal for matching partial strings, validating data, and performing advanced search queries. However, they should be used carefully with large datasets, as they can impact query performance.
Analyzing Query Performance with explain()
In MongoDB, the explain()
method is a powerful tool for analyzing query
performance. It provides detailed information about how MongoDB executes a query, allowing
developers to optimize queries for better performance. By using explain()
, you
can gain insight into whether MongoDB uses indexes, how long the query takes, and where it
may be inefficient.
What is explain()?
The explain()
method returns a document that contains information about the
query execution plan. This plan includes details about:
- How MongoDB processes the query (e.g., collection scan, index scan).
- Which indexes are used, if any.
- The execution time of the query.
- The number of documents scanned versus the number of documents returned.
Using explain()
helps identify performance bottlenecks and allows you to make
adjustments, such as adding indexes or optimizing queries.
Basic Usage of explain()
You can use explain()
with any MongoDB query to analyze its execution plan.
Here’s an example of how to use it:
db.collection.find({ field: "value" }).explain()
This will return an execution plan that describes how MongoDB would execute the query. The response will include various details about the query's performance.
Types of explain Output
MongoDB'sexplain()
method provides different levels of detail about the query
execution plan. There are three main verbosity levels:
- queryPlanner: Shows details about the query plan, such as the indexes used and whether a collection scan is performed.
- executionStats: Provides additional information, including the number of documents scanned, the number of documents returned, and the execution time.
- allPlansExecution: Shows information about all possible query plans and their execution statistics. This level is useful for comparing different plans to identify the most efficient one.
To specify the verbosity level, you can pass it as an argument to explain()
. For
example:
db.collection.find({ field: "value" }).explain("executionStats")
This will return detailed execution statistics for the query.
Understanding the explain Output
The explain output contains several important fields that help you analyze the query performance. Here are some key fields to look for:- queryPlanner: Describes the stages of query execution, such as whether an index is used or if a collection scan is performed. If an index is used, it will specify which index is being utilized.
- stage: Indicates the type of operation being performed at a given
stage, such as
COLLSCAN
(collection scan) orIXSCAN
(index scan). - nReturned: The number of documents returned by the query.
- nScanned: The number of documents that were scanned during the query
execution. A large difference between
nScanned
andnReturned
could indicate an inefficient query. - executionTimeMillis: The time in milliseconds it took to execute the query.
Example of explain Output
Here's an example of the output you might get from runningexplain("executionStats")
on a query:
{
"queryPlanner": {
"namespace": "mydb.collection",
"indexFilterSet": false,
"parsedQuery": { "field": "value" },
"winningPlan": {
"stage": "IXSCAN",
"keyPattern": { "field": 1 },
"inputStage": {
"stage": "FETCH",
"inputStage": {
"stage": "IXSCAN",
"keyPattern": { "field": 1 },
"direction": "forward"
}
}
}
},
"executionStats": {
"nReturned": 1,
"nScanned": 1,
"executionTimeMillis": 2
}
}
In this example, the query uses an index scan (IXSCAN
) on the field
index to find documents matching the criteria, and the execution time is 2 milliseconds.
Optimizing Queries Using explain()
Here are some common strategies to improve query performance using insights from
explain()
:
- Use Indexes Efficiently: Ensure that queries are using indexes
appropriately. If a collection scan (
COLLSCAN
) is being used, consider adding an index on the fields being queried. - Avoid Full Collection Scans: Full collection scans are inefficient, especially for large collections. Use indexes to avoid scanning entire collections.
- Optimize Query Filters: Ensure that query filters are selective and narrow down the result set as much as possible before returning documents.
- Analyze Query Execution Time: If a query is taking too long, look at
the
executionTimeMillis
field and investigate ways to optimize it.
Conclusion
Using explain()
is a crucial step in understanding how MongoDB executes your
queries and identifying opportunities for optimization. By analyzing the execution plan and
performance statistics, you can make informed decisions about indexing and query design to
improve the efficiency of your MongoDB queries.
Understanding Query Plans
In MongoDB, a query plan is a detailed blueprint that describes how the database engine
executes a query. Understanding query plans is essential for optimizing query performance,
as it helps you identify whether indexes are being used efficiently, how documents are
retrieved, and what operations are involved. MongoDB provides tools like the
explain()
method to analyze query plans and understand how the database
processes your queries.
What is a Query Plan?
A query plan is a set of steps that MongoDB follows to retrieve data that matches your query criteria. The plan includes information on whether MongoDB uses indexes, scans the entire collection, or applies other operations like sorting or filtering. Each query plan will vary depending on the query, available indexes, and the size and structure of the data.
How MongoDB Chooses a Query Plan
MongoDB uses a cost-based query planner to decide which query plan to use. The query planner evaluates different possible plans based on several factors, such as:
- Indexes: Whether an index exists that can be used to optimize the query.
- Query conditions: The conditions specified in the query and whether they can be efficiently matched using an index.
- Collection size: The size of the collection and whether it would be more efficient to scan the entire collection or use an index.
- Sorting: Whether sorting is required and how it can be optimized using an index.
- Join operations: Whether $lookup or other aggregation operators are used, which may require scanning multiple collections.
Explaining Query Plans with explain()
The explain()
method in MongoDB provides valuable insights into how a query is
executed. By using explain()
on a query, MongoDB will return the query
execution plan, which includes information on the stages involved in executing the query,
the indexes used (if any), and performance statistics.
Here’s an example of using explain()
on a query:
db.collection.find({ field: "value" }).explain("executionStats")
This will return detailed information about the query execution, including the stages involved, the indexes used, the number of documents scanned, and the total execution time.
Key Components of a Query Plan
When analyzing a query plan, there are several key components to look at:- Winning Plan: The winning plan is the one chosen by MongoDB's query planner. It shows how the query will be executed, including details on whether an index is used and how documents are retrieved.
- Stage: Each stage in the query execution represents an operation, such
as an index scan (
IXSCAN
) or a collection scan (COLLSCAN
). - Index: If an index is used, the query plan will specify the index being
utilized. Look for the
indexName
field to see which index is involved. - Execution Time: The
executionTimeMillis
field indicates how long the query took to run. This is useful for identifying performance bottlenecks. - Documents Scanned vs Returned: The query plan will indicate how many documents were scanned during the execution and how many were actually returned. A large number of documents scanned compared to those returned may signal inefficiencies.
Example of a Query Plan
Here’s an example of a query plan returned by theexplain()
method:
{
"queryPlanner": {
"namespace": "mydb.collection",
"indexFilterSet": false,
"parsedQuery": { "field": "value" },
"winningPlan": {
"stage": "IXSCAN",
"keyPattern": { "field": 1 },
"inputStage": {
"stage": "FETCH",
"inputStage": {
"stage": "IXSCAN",
"keyPattern": { "field": 1 },
"direction": "forward"
}
}
}
},
"executionStats": {
"nReturned": 1,
"nScanned": 1,
"executionTimeMillis": 2
}
}
In this example, the query uses an index scan (IXSCAN
) on the field
index to find documents matching the query. The query execution time is 2 milliseconds, and
only one document was scanned and returned.
Types of Query Plans
There are several common types of query plans you may encounter in MongoDB:- COLLSCAN (Collection Scan): This plan indicates that MongoDB is scanning the entire collection because no suitable index is available. Collection scans are generally slower, especially for large collections, and should be avoided when possible.
- IXSCAN (Index Scan): This plan indicates that MongoDB is using an index to retrieve documents matching the query criteria. Index scans are much faster than collection scans and should be used whenever possible.
- FETCH: The fetch stage happens after an index scan. It retrieves the actual documents that match the query conditions.
- SORT: If the query involves sorting, MongoDB will apply a sort operation during the execution plan. Sorting can be optimized with indexes on the fields involved in sorting.
- $lookup (Join): If the query involves a join using the $lookup operator, the query plan will show stages related to join operations.
Optimizing Queries Using Query Plans
By understanding query plans, you can identify performance bottlenecks and optimize your queries. Here are some tips for optimizing queries based on the query plan:- Use Indexes: Ensure that your queries are using indexes effectively. If
a query is performing a collection scan (
COLLSCAN
), consider creating an index on the fields being queried. - Avoid Full Collection Scans: Collection scans are inefficient and can slow down your queries significantly, especially on large collections. Always ensure that indexes are used wherever possible.
- Optimize Sorting: If your query involves sorting, make sure that an index exists on the fields being sorted. Sorting without an index can be slow and resource-intensive.
- Analyze Execution Time: If a query is taking too long, check the
executionTimeMillis
field and try to optimize the query by reducing the number of documents scanned or simplifying the query.
Conclusion
Understanding query plans is an essential skill for any MongoDB developer. By using the
explain()
method and analyzing query plans, you can gain valuable insights into
how MongoDB executes your queries and identify areas for improvement. Optimizing query plans
can help ensure that your MongoDB queries run efficiently, even with large datasets.
Compound Indexes and Multi-Key Indexes
MongoDB supports various types of indexes to improve query performance, and compound indexes and multi-key indexes are two essential types for optimizing specific types of queries.
What are Compound Indexes?
A compound index in MongoDB is an index that includes multiple fields. MongoDB uses compound indexes to optimize queries that filter or sort on more than one field. Compound indexes are particularly useful when your queries frequently involve multiple criteria, as they allow MongoDB to handle these queries more efficiently without needing to scan the entire collection.
When to Use Compound Indexes
Compound indexes are most useful when:
- Your queries filter on multiple fields simultaneously.
- Your queries involve sorting on multiple fields.
- The order of fields in the index matches the order in the query.
Example of a Compound Index
Suppose you have a collection of documents representing products, and you often query by both
category
and price
. You can create a compound index on both fields
to optimize these queries:
db.products.createIndex({ category: 1, price: -1 })
This compound index ensures that queries filtering by category
and sorting by
price
will be efficient. The index is created in ascending order for
category
and descending order for price
.
Compound Indexes and Query Execution
MongoDB will only use a compound index when the query uses the leftmost prefix of the index.
This means that the fields must appear in the same order in both the query and the index.
For example, if you have a compound index on { category: 1, price: -1 }
,
MongoDB can use it for queries that filter on category
alone, or both
category
and price
, but not for queries that filter only on
price
.
What are Multi-Key Indexes?
A multi-key index is a special type of index that MongoDB creates automatically when you index a field that contains an array. Multi-key indexes allow MongoDB to index each element of the array as a separate index entry, making it possible to efficiently query for documents that contain specific elements in an array.
When to Use Multi-Key Indexes
Multi-key indexes are particularly useful when:
- You're working with documents that contain arrays and you frequently need to query for specific elements within those arrays.
- Your queries filter or sort based on array elements.
Example of a Multi-Key Index
Consider a collection of documents representing users, where each user has a list of
tags
that they are associated with. You can create a multi-key index on the
tags
field to optimize queries that search for users with specific tags:
db.users.createIndex({ tags: 1 })
This multi-key index allows MongoDB to efficiently query for users who have specific tags in
their tags
array.
Multi-Key Indexes and Query Execution
When you query for a document based on an element in an array, MongoDB will automatically use the multi-key index if it exists. For example, a query like this:
db.users.find({ tags: "developer" })
will use the multi-key index on the tags
field to efficiently locate documents
with the "developer"
tag. Without a multi-key index, MongoDB would need to
perform a full collection scan, which would be much slower.
Differences Between Compound and Multi-Key Indexes
While both compound and multi-key indexes are used to optimize query performance, they serve different purposes:
- Compound Indexes: Used to index multiple fields in a document, allowing efficient querying when multiple fields are involved in the query.
- Multi-Key Indexes: Used to index array fields, allowing efficient querying of specific elements within an array.
In some cases, MongoDB may automatically create a multi-key index when you index an array field. However, compound indexes must be explicitly created by the user.
Example of Using Both Compound and Multi-Key Indexes
Consider a collection of blog posts where each post has a tags
array, and you
frequently query by both the author
field and the tags
field. You
can create a compound index on author
and tags
to optimize these
queries:
db.posts.createIndex({ author: 1, tags: 1 })
If the tags
field is an array, MongoDB will automatically create a multi-key
index for the array elements, allowing efficient queries on both the author
and
tags
fields.
Best Practices for Using Compound and Multi-Key Indexes
- Limit the Number of Indexes: While indexes improve query performance, they also add overhead to write operations (inserts, updates, and deletes). Avoid creating excessive indexes that may degrade performance.
- Choose Index Fields Carefully: Choose fields for compound indexes that are most frequently queried together. Ensure the order of the fields in the index matches the order in the query.
- Use Multi-Key Indexes for Arrays: For fields that contain arrays, use multi-key indexes to optimize queries that search for specific array elements.
- Monitor Index Usage: Use MongoDB's
explain()
method to monitor the effectiveness of your indexes and ensure they are being used as expected.
Conclusion
Both compound and multi-key indexes are powerful tools for optimizing MongoDB queries. Compound indexes allow you to efficiently query multiple fields, while multi-key indexes make it possible to query array elements efficiently. By understanding the differences and use cases for each type of index, you can design your MongoDB schema to optimize query performance and scalability.
Text Search and Full-Text Indexes
MongoDB provides powerful full-text search capabilities through text indexes. Text search allows users to perform queries based on string matching, searching documents for specific words or phrases. Full-text indexes optimize these searches, enabling efficient and powerful text-based queries.
What is Text Search in MongoDB?
Text search in MongoDB allows you to query documents based on text content. This is particularly useful for applications such as search engines, forums, or any application that involves searching through large volumes of text data. MongoDB’s text search allows for operations such as matching words, phrases, and performing text-based queries like prefix search or stemming.
How MongoDB Handles Text Search
MongoDB uses text indexes to support text search functionality. When you create a text index on a field, MongoDB automatically tokenizes the content of that field and stores the tokens (words) in the index. This allows MongoDB to perform efficient text searches by matching the tokens in your search queries with the indexed tokens.
Creating a Text Index
To enable text search on a field or fields, you need to create a text index on that field. MongoDB supports creating text indexes on string fields, and you can create a text index on one or more fields in a collection.
Example: Creating a Text Index
Suppose you have a collection of blog posts, each with a title
and
content
field. You can create a text index on both fields to perform full-text
searches:
db.posts.createIndex({ title: "text", content: "text" })
In this example, MongoDB creates a text index on both the title
and
content
fields, enabling efficient text searches across both fields.
Text Search Queries
Once a text index is created, you can perform text search queries using the
$text
operator. The $text
operator matches documents that contain
a specific word or phrase in the indexed fields.
Example: Searching for Text
Suppose you want to search for blog posts that contain the word "MongoDB"
in
either the title
or content
field. You can execute a query like
this:
db.posts.find({ $text: { $search: "MongoDB" } })
This query will return all blog posts where the word "MongoDB"
appears in either
the title
or content
fields.
Text Search with Multiple Keywords
MongoDB’s text search also supports searching for multiple words in a single query. When you
use multiple words in the $search
value, MongoDB will return documents that
match any of the words in the query.
Example: Searching with Multiple Keywords
Suppose you want to search for blog posts containing both "MongoDB"
and
"database"
. You can modify your query like this:
db.posts.find({ $text: { $search: "MongoDB database" } })
This query will return documents where both words appear in the indexed fields.
Text Search with Phrases
MongoDB’s text search also supports searching for exact phrases. When you enclose multiple words in quotation marks, MongoDB will search for the exact phrase.
Example: Searching for a Phrase
If you want to search for the exact phrase "MongoDB tutorial"
, you can use the
following query:
db.posts.find({ $text: { $search: '"MongoDB tutorial"' } })
This query will only return documents where the exact phrase "MongoDB tutorial"
appears.
Text Search with Exclusions
MongoDB’s text search allows you to exclude certain words from the search results by
prefixing them with a minus sign (-
).
Example: Excluding a Word from the Search
If you want to search for blog posts that contain the word "MongoDB"
but exclude
posts that also contain the word "tutorial"
, you can use:
db.posts.find({ $text: { $search: "MongoDB -tutorial" } })
This query will return documents that contain the word "MongoDB"
, but will
exclude any documents containing "tutorial"
.
Text Search and Sorting
You can also sort the results of a text search query based on the relevance of the matches.
MongoDB assigns a textScore
to each document based on how well it matches the
search query. You can sort the results by textScore
to prioritize more relevant
documents.
Example: Sorting by Text Score
To sort the search results by relevance, use the textScore
field in the
sort
method:
db.posts.find({ $text: { $search: "MongoDB" } })
.sort({ score: { $meta: "textScore" } })
This query will return blog posts that match the search term "MongoDB"
, sorted
by the relevance of the match.
Text Index Options
When creating a text index, MongoDB provides various options to control the behavior of the text search. Some important options include:
- default_language: Specifies the default language for stemming and stop words (e.g., English, Spanish, etc.).
- language_override: Specifies a field in the document that contains the language for the text search.
- weights: Allows you to assign weights to specific fields to influence how much they contribute to the relevance score.
Example: Text Index with Options
Here’s how you can create a text index with custom options, such as setting a default language and assigning weights to fields:
db.posts.createIndex(
{ title: "text", content: "text" },
{ default_language: "english", weights: { title: 5, content: 1 } }
)
Best Practices for Using Text Search
- Use Text Search Sparingly: Full-text search operations can be resource-intensive. Use them only when necessary and ensure your queries are optimized.
- Index Only Relevant Fields: Only index fields that are frequently used for text search queries to avoid unnecessary performance overhead.
- Leverage Sorting and Scoring: Use relevance scoring and sorting to prioritize the most relevant search results for your users.
- Monitor Text Indexes: Monitor the performance of your text indexes
using MongoDB's
explain()
method to ensure they are working efficiently.
Conclusion
Text search and full-text indexes in MongoDB provide powerful capabilities for performing
efficient and flexible text-based queries. By creating text indexes and using the
$text
operator, you can build sophisticated search features for applications
that require text-based search, such as blogs, forums, and search engines. Understanding how
to create and use text indexes effectively will help you optimize your MongoDB applications
for text searching.
Introduction to Transactions
In MongoDB, transactions provide a way to execute a series of operations in a way that guarantees that all operations are completed successfully, or none are applied at all. This is known as "ACID" (Atomicity, Consistency, Isolation, Durability) compliance, which ensures data integrity and consistency even in the case of failures or errors during the execution of operations.
What Are Transactions?
A transaction in MongoDB is a set of operations that are grouped together and executed as a unit. Transactions allow you to perform multiple operations on multiple documents or collections and ensure that either all operations are successful, or none of them are applied, providing a way to handle errors more gracefully.
MongoDB introduced multi-document transactions in version 4.0, enabling the ability to perform operations across multiple documents or collections with the same guarantee of ACID compliance.
ACID Properties of Transactions
The four properties that define the behavior of transactions are:
- Atomicity: A transaction is atomic, meaning that all operations within the transaction are completed successfully or none of them are. If one operation fails, the entire transaction is rolled back.
- Consistency: The database is in a consistent state before and after the transaction. Any changes made during the transaction do not violate the integrity of the data.
- Isolation: Each transaction is executed in isolation from other transactions. Operations from one transaction are not visible to others until the transaction is committed.
- Durability: Once a transaction is committed, the changes are permanent. Even in the case of a system failure, the changes will not be lost.
When to Use Transactions
Transactions are particularly useful in situations where multiple operations need to be performed as a single unit of work. Common use cases include:
- Banking Systems: Transactions involving multiple accounts, where money is transferred between accounts. If one part of the transaction fails, the entire transfer should be rolled back.
- Order Processing: Ensuring that an order, payment, and inventory updates are all performed together. If any step fails, the whole order should be rolled back.
- Data Integrity: When performing operations that involve multiple documents or collections, such as updating related documents, transactions can ensure that all changes are consistent.
Types of Transactions
MongoDB supports two types of transactions:
- Single-Document Transactions: These are transactions that involve operations on a single document. Although MongoDB automatically ensures the atomicity of single-document operations, using transactions for single documents is redundant, but it's possible to include them for consistency.
- Multi-Document Transactions: These transactions involve operations on more than one document or across multiple collections. Multi-document transactions are more complex and require explicit start, commit, and rollback operations.
Starting a Transaction
To start a transaction in MongoDB, you need to use a session. A session is an object that
tracks the state of the transaction. Transactions are initiated by calling the
startTransaction
method on a session.
Example: Starting a Transaction
The following example demonstrates how to start a multi-document transaction:
const session = await client.startSession();
session.startTransaction();
try {
// Perform multiple operations within the transaction
await collection1.insertOne({ name: "John", age: 30 }, { session });
await collection2.updateOne({ name: "Doe" }, { $set: { age: 31 } }, { session });
// Commit the transaction
await session.commitTransaction();
} catch (error) {
// If an error occurs, abort the transaction
await session.abortTransaction();
console.error("Transaction failed:", error);
} finally {
session.endSession();
}
This example starts a transaction, performs operations on two collections, and then either commits the transaction (if successful) or aborts it (if an error occurs).
Committing and Aborting Transactions
Once all operations within a transaction have been performed, you can either commit or abort the transaction:
- Commit: If all operations are successful, you call the
commitTransaction
method to make the changes permanent. - Abort: If any operation fails or an error occurs, you can call the
abortTransaction
method to roll back all operations in the transaction.
Example: Committing and Aborting Transactions
Here’s how you can commit or abort a transaction:
if (allOperationsSuccessful) {
await session.commitTransaction();
} else {
await session.abortTransaction();
}
Transaction Best Practices
To ensure efficient use of transactions and avoid potential pitfalls, here are a few best practices to follow:
- Keep Transactions Short: The longer a transaction runs, the higher the chances of conflicts with other operations. Keep transactions as short as possible.
- Handle Errors Properly: Always handle errors in transactions. If any
operation fails, make sure to call
abortTransaction
to ensure the database remains in a consistent state. - Use Transactions for Critical Operations: Transactions should be used for critical operations that require ACID guarantees. For simple operations on single documents, transactions may not be necessary.
- Monitor Transaction Performance: Keep an eye on the performance of transactions. Long-running transactions can reduce database performance and should be optimized where possible.
Limitations of Transactions in MongoDB
Although MongoDB supports transactions, there are certain limitations:
- Replica Set Requirements: Multi-document transactions require a replica set, and they are not supported in standalone MongoDB instances.
- Performance Impact: Transactions can introduce performance overhead, especially in high-volume workloads. Use transactions judiciously and optimize where necessary.
- Nested Transactions: MongoDB does not support nested transactions. You must commit or abort the current transaction before starting another one.
Conclusion
Transactions in MongoDB provide a powerful mechanism for ensuring data consistency and integrity, especially when performing multiple operations on multiple documents or collections. By using transactions, you can ensure that either all operations are successfully completed or none are applied, which is essential for applications that require a high level of data integrity. Understanding when and how to use transactions is crucial for building reliable and robust MongoDB applications.
Multi-document Transactions
Multi-document transactions allow you to execute multiple operations on multiple documents or collections within a single, atomic transaction. This ensures that either all the operations succeed or none of them are applied, providing ACID guarantees across multiple documents or collections in a MongoDB database. Multi-document transactions were introduced in MongoDB 4.0, which made MongoDB suitable for use cases that require complex transactions, such as financial systems or order processing systems.
What Are Multi-Document Transactions?
In MongoDB, a multi-document transaction spans operations across multiple documents, and potentially multiple collections, ensuring the atomicity and consistency of all operations. If any operation in the transaction fails, all the changes are rolled back to maintain data integrity. Multi-document transactions allow you to perform operations on more than one document and treat the entire set of operations as a single unit.
ACID Properties in Multi-Document Transactions
Multi-document transactions in MongoDB provide the same ACID (Atomicity, Consistency, Isolation, Durability) guarantees as traditional relational databases:
- Atomicity: Either all operations in the transaction are applied, or none are. If an error occurs during any operation, all changes are rolled back.
- Consistency: The database remains in a valid state after the transaction, ensuring data integrity.
- Isolation: Transactions are isolated from other concurrent operations. Changes made in the transaction are not visible to other operations until the transaction is committed.
- Durability: Once a transaction is committed, the changes are permanent, even in case of system failures.
When to Use Multi-Document Transactions
Multi-document transactions are helpful in scenarios where multiple documents or collections need to be updated in a way that ensures consistency. Some common use cases include:
- Banking Systems: When transferring funds between multiple bank accounts, a transaction must ensure that money is deducted from one account and added to another, or neither operation is performed if any of them fails.
- Order Management Systems: In e-commerce applications, when processing an order, multiple documents in various collections (e.g., orders, products, inventory) need to be updated. A transaction ensures all changes are applied or none are.
- Inventory Management: When updating inventory after a purchase, the quantity must be decreased for the purchased item, and the order status must be updated. A multi-document transaction ensures these operations are atomic.
How to Use Multi-Document Transactions
To use multi-document transactions in MongoDB, you need to use a session. The session tracks the state of the transaction and allows you to start, commit, or abort the transaction.
Example: Starting a Multi-Document Transaction
The following example demonstrates how to start a multi-document transaction in MongoDB, perform operations on multiple collections, and commit or abort the transaction based on success or failure:
const session = await client.startSession();
session.startTransaction();
try {
// Perform multiple operations within the transaction
await collection1.updateOne(
{ _id: "account1" },
{ $inc: { balance: -100 } },
{ session }
);
await collection2.updateOne(
{ _id: "account2" },
{ $inc: { balance: 100 } },
{ session }
);
// Commit the transaction if all operations succeed
await session.commitTransaction();
} catch (error) {
// If an error occurs, abort the transaction
await session.abortTransaction();
console.error("Transaction failed:", error);
} finally {
session.endSession();
}
In this example, we are transferring money between two accounts. If either update operation fails, the transaction will be aborted, and no changes will be made to the database. If both operations succeed, the transaction will be committed and the changes will be applied permanently.
Commit and Abort Transactions
Once you have completed the operations within the transaction, you can either commit or abort the transaction:
- Commit: If all operations are successful, you commit the transaction,
making the changes permanent using the
commitTransaction
method. - Abort: If any operation fails or an error occurs, you abort the
transaction using the
abortTransaction
method, rolling back all changes made during the transaction.
Example: Committing and Aborting a Transaction
The following code demonstrates how to commit or abort a transaction based on success or failure:
if (allOperationsSuccessful) {
await session.commitTransaction();
} else {
await session.abortTransaction();
}
Best Practices for Multi-Document Transactions
Here are some best practices to ensure efficient and effective use of multi-document transactions:
- Minimize Transaction Scope: Only include the necessary operations in the transaction to reduce the risk of conflicts and improve performance. The fewer operations in the transaction, the less overhead it introduces.
- Handle Errors and Rollbacks: Always implement proper error handling. If any operation fails, abort the transaction to ensure data integrity.
- Use Transactions for Critical Operations: Multi-document transactions should be used for critical operations that require strong consistency. For simple operations on a single document, transactions may not be necessary.
- Monitor Performance: Multi-document transactions can introduce overhead, especially in high-concurrency environments. Monitor transaction performance and optimize where needed.
Limitations of Multi-Document Transactions
While multi-document transactions provide powerful capabilities, there are some limitations:
- Replica Set Requirement: Multi-document transactions are only supported in replica sets. Standalone MongoDB instances do not support multi-document transactions.
- Performance Impact: Transactions introduce overhead, especially when running for extended periods. Make sure to use them judiciously and optimize your operations.
- No Nested Transactions: MongoDB does not support nesting transactions within another transaction. Each transaction must be committed or aborted before starting a new one.
Conclusion
Multi-document transactions provide a powerful way to perform complex operations across multiple documents and collections while maintaining ACID guarantees. By using multi-document transactions, you can ensure that your MongoDB operations are consistent, isolated, and reliable, even in the face of failures. Understanding when and how to use multi-document transactions effectively is key to building robust and reliable applications with MongoDB.
ACID Properties in MongoDB
ACID properties (Atomicity, Consistency, Isolation, Durability) ensure that database transactions are processed reliably and maintain data integrity. MongoDB, starting with version 4.0, introduced support for multi-document transactions, providing ACID guarantees for operations that span multiple documents and collections. These properties are fundamental to ensuring that database operations are safe, even in the event of system failures or errors during transaction execution.
What Are ACID Properties?
ACID properties define the characteristics that a transaction must have to ensure the reliability of the database. The four properties are:
- Atomicity: Ensures that all operations in a transaction are completed successfully. If any part of the transaction fails, all changes are rolled back and the database is left in its original state. Atomicity guarantees that a transaction is treated as a single, indivisible unit of work.
- Consistency: Ensures that the database transitions from one valid state to another. A transaction must bring the database from a valid state (according to the defined schema and business rules) to another valid state. Any transaction that violates the database’s constraints must be rolled back.
- Isolation: Ensures that transactions are executed in isolation from one another. Changes made by a transaction are not visible to other transactions until the transaction is complete. This property prevents interference from concurrently running transactions, ensuring that the results are consistent.
- Durability: Ensures that once a transaction is committed, the changes are permanent, even if the system crashes. MongoDB uses write-ahead logging (WAL) to ensure that changes are recorded to disk before being acknowledged as committed.
Atomicity in MongoDB
Atomicity ensures that a transaction is treated as a single unit, where all its operations are completed successfully or none at all. In MongoDB, atomicity is guaranteed for operations on a single document. However, for operations involving multiple documents or collections, MongoDB uses multi-document transactions to ensure atomicity across multiple entities.
Consistency in MongoDB
Consistency ensures that the database is left in a valid state after a transaction. MongoDB enforces consistency through its schema design and business rules. For instance, if a transaction violates any constraints (such as attempting to insert invalid data or violating a required field constraint), MongoDB will reject the transaction and roll back any changes made.
Isolation in MongoDB
Isolation ensures that the operations of one transaction do not interfere with those of another. In MongoDB, isolation is provided by using locking mechanisms. For multi-document transactions, MongoDB ensures that changes made by one transaction are invisible to other transactions until the transaction is committed. This prevents dirty reads and ensures that transactions are executed in isolation, even in high-concurrency environments.
Durability in MongoDB
Durability guarantees that once a transaction is committed, the changes are permanent, even in the event of a power failure or system crash. MongoDB uses write-ahead logging to ensure that all operations are logged to disk before being acknowledged. This ensures that the database can recover from failures and that committed data is not lost.
How MongoDB Implements ACID Properties
Starting with MongoDB 4.0, multi-document transactions provide full ACID guarantees. The following mechanisms are in place to ensure ACID properties:
- Two-Phase Commit: MongoDB uses the two-phase commit protocol in multi-document transactions to ensure atomicity and consistency. The first phase validates the transaction, and the second phase commits the changes.
- Write-Ahead Log (WAL): MongoDB writes all transaction operations to a durable log before committing them to the database. This ensures that data can be recovered in the event of a failure.
- Snapshot Isolation: MongoDB uses snapshot isolation to provide consistent views of data during a transaction, ensuring that operations do not affect each other.
- Journaling: MongoDB uses journaling to ensure durability. When a transaction is committed, the changes are written to the journal before being fully applied to the database.
Multi-Document Transactions and ACID Compliance
With the introduction of multi-document transactions in MongoDB 4.0, operations on multiple documents or collections can now be wrapped in a single transaction, providing full ACID guarantees across the entire set of operations. This makes MongoDB suitable for applications that require complex, multi-step transactions, such as financial applications, order processing systems, and inventory management systems.
Example of a Transaction with ACID Properties
The following example demonstrates how to start a multi-document transaction in MongoDB, ensuring that all operations are atomic and consistent:
const session = await client.startSession();
session.startTransaction();
try {
await collection1.updateOne({ _id: "account1" }, { $inc: { balance: -100 } }, { session });
await collection2.updateOne({ _id: "account2" }, { $inc: { balance: 100 } }, { session });
await session.commitTransaction(); // Ensures atomicity and consistency
} catch (error) {
await session.abortTransaction(); // Ensures rollback in case of failure
console.error("Transaction failed:", error);
} finally {
session.endSession();
}
Best Practices for Working with ACID Properties
To make the most of ACID properties in MongoDB, here are a few best practices:
- Use Multi-Document Transactions for Complex Operations: For operations that involve multiple documents or collections, use multi-document transactions to ensure atomicity and consistency.
- Minimize Transaction Duration: Keep transactions short to minimize the impact on performance and reduce the risk of conflicts with other operations.
- Handle Failures Gracefully: Always implement proper error handling and ensure that transactions are either committed or aborted based on success or failure.
- Optimize for Performance: While multi-document transactions provide ACID guarantees, they can introduce overhead. Use them judiciously for critical operations and monitor their performance.
Conclusion
ACID properties are fundamental for ensuring the reliability and integrity of database transactions. MongoDB’s support for multi-document transactions in version 4.0 and later allows developers to perform complex, multi-step operations while maintaining full ACID compliance. By understanding and leveraging the ACID properties, you can build robust and reliable applications with MongoDB that handle critical operations like financial transactions, order processing, and more.
Session-based Operations in MongoDB
Session-based operations in MongoDB are an essential feature for managing multi-document transactions and maintaining consistency across multiple operations. A session allows you to group multiple operations into a single context, enabling features like transactions, causal consistency, and the ability to track state across multiple operations and requests. Sessions provide a way to handle operations atomically across multiple collections and databases, ensuring that the operations are treated as a single unit and that changes are committed or rolled back together.
What Is a MongoDB Session?
A session in MongoDB is a context that allows you to group multiple operations together. This context is used to support multi-document transactions and causal consistency. When you perform operations within a session, MongoDB ensures that these operations are executed atomically and consistently. Sessions also provide the ability to track the state of the operations, and they allow for the use of features like retryable writes, causal consistency, and transaction support.
Session Creation and Usage
To begin using sessions in MongoDB, you first need to create a session using the driver’s API. Once the session is created, you can pass it to the methods that perform operations like insert, update, or delete. This ensures that the operations are executed within the context of that session.
Creating a Session
To create a session, you can use the startSession()
method provided by the
MongoDB client. Here’s an example of how to create a session in MongoDB using the Node.js
driver:
const session = await client.startSession();
Session-based Transactions
One of the key benefits of using sessions is the ability to perform multi-document transactions. A transaction is a sequence of operations that are treated as a single unit of work. If any operation within the transaction fails, all changes made during the transaction are rolled back, ensuring that the database remains in a consistent state.
Using Sessions in a Multi-document Transaction
In MongoDB, you can use sessions to perform multi-document transactions. You can begin a
transaction with the startTransaction()
method and commit or abort the
transaction when needed using commitTransaction()
and
abortTransaction()
methods, respectively.
const session = await client.startSession();
session.startTransaction();
try {
// Perform operations within the transaction
await collection1.updateOne({ _id: "account1" }, { $inc: { balance: -100 } }, { session });
await collection2.updateOne({ _id: "account2" }, { $inc: { balance: 100 } }, { session });
// Commit the transaction if all operations are successful
await session.commitTransaction();
} catch (error) {
// Abort the transaction if an error occurs
await session.abortTransaction();
console.error("Transaction failed:", error);
} finally {
// End the session
session.endSession();
}
Key Features of Sessions in MongoDB
- Atomicity: Sessions ensure atomicity by grouping multiple operations into a single transaction. If an error occurs during any operation, the entire transaction can be rolled back.
- Consistency: Sessions maintain consistency by ensuring that all operations within a transaction are executed as a unit. MongoDB guarantees that the database will remain in a consistent state even in the event of failures.
- Isolation: Sessions provide isolation by preventing other operations from interfering with the current transaction. The changes made within a session are only visible after the transaction is committed.
- Durability: Sessions ensure durability by writing changes to a durable log before committing them, so the changes are not lost in case of system failures.
Retryable Writes with Sessions
One of the key advantages of using sessions is the support for retryable writes. MongoDB ensures that write operations can be retried safely in the event of network or server failures. When a write operation is executed within a session, MongoDB automatically retries the operation if it is interrupted, ensuring that no data is lost in the process.
Using Retryable Writes
To enable retryable writes, you simply need to specify the session when performing the write operation. Here’s an example of how to use retryable writes with a session in MongoDB:
const session = await client.startSession();
try {
await collection.updateOne({ _id: "item1" }, { $set: { quantity: 10 } }, { session });
} catch (error) {
console.error("Write failed, retrying:", error);
}
session.endSession();
Causal Consistency
Causal consistency allows you to ensure that the operations are executed in the correct order across distributed MongoDB clusters. MongoDB ensures that the order of operations within a session is maintained, enabling you to read the data in a way that reflects the causal relationship between write operations and subsequent reads.
Enabling Causal Consistency
To enable causal consistency, you need to specify that the operations in the session are causally related. MongoDB ensures that the reads within the session reflect the order of writes, even across distributed nodes.
const session = await client.startSession();
session.startTransaction();
session.advanceClusterTime({ clusterTime: new Date() });
try {
await collection.updateOne({ _id: "user1" }, { $set: { lastLogin: new Date() } }, { session });
const result = await collection.find({ _id: "user1" }).session(session).toArray();
console.log(result);
await session.commitTransaction();
} catch (error) {
await session.abortTransaction();
console.error("Transaction failed:", error);
} finally {
session.endSession();
}
Best Practices for Session-based Operations
- Use Sessions for Multi-document Transactions: Always use sessions to group multiple operations into a single transaction, ensuring atomicity and consistency.
- Keep Transactions Short: Minimize the duration of transactions to reduce the impact on performance and prevent potential locking issues.
- Handle Failures Gracefully: Always implement error handling for sessions, ensuring that transactions are either committed or aborted appropriately.
- Enable Retryable Writes: Make use of retryable writes to ensure that write operations are robust, even in the event of network or server failures.
Conclusion
Session-based operations in MongoDB provide a powerful way to manage multi-document transactions, ensure causal consistency, and support retryable writes. By using sessions, you can group multiple operations into a single transaction, ensuring that the database remains in a consistent and reliable state even in the event of failures. Sessions offer atomicity, consistency, isolation, and durability, making them essential for building robust applications that require complex operations across multiple documents and collections.
Advanced Aggregation Operators in MongoDB
MongoDB's aggregation framework allows you to perform complex transformations and
computations on your data. Among the advanced aggregation operators, the most commonly used
are $unwind
, $lookup
, and $out
. These operators are
used for unrolling arrays, performing joins, and exporting results, respectively.
Understanding how to use them effectively can greatly enhance the flexibility and power of
your aggregation queries.
$unwind Operator
The $unwind
operator deconstructs an array field from the input documents to
output a document for each element in the array. This operator is useful when you need to
"flatten" an array field, treating each array element as a separate document.
Syntax
The syntax for using $unwind
is as follows:
{ $unwind: }
Where
is the name of the array field you want to unwind.
Example
Consider a collection of orders where each order contains an array of items. You can use
$unwind
to break down the items array into individual documents:
db.orders.aggregate([
{ $unwind: "$items" }
])
This query will produce a separate document for each item in the items
array,
allowing you to work with individual items instead of the entire array.
$lookup Operator
The $lookup
operator performs a left outer join between two collections. It
allows you to combine documents from one collection with matching documents from another
collection, based on a specified condition. This is essential for performing relational-like
operations in MongoDB.
Syntax
The syntax for $lookup
is as follows:
{
$lookup: {
from: ,
localField: ,
foreignField: ,
as:
}
}
from
specifies the collection to join, localField
is the field from
the input documents, foreignField
is the field from the joined collection, and
as
is the name of the array field where the results will be stored.
Example
Let’s say you have a collection of orders
and a collection of
products
. You can use $lookup
to combine order documents with
product details:
db.orders.aggregate([
{
$lookup: {
from: "products",
localField: "product_id",
foreignField: "_id",
as: "product_details"
}
}
])
This query will join the orders
collection with the products
collection, matching the product_id
from orders with the _id
from
products, and add the product details in an array called product_details
.
$out Operator
The $out
operator is used to write the results of an aggregation pipeline to a
new or existing collection. This operator is useful when you want to persist the results of
a complex aggregation operation for future use or further processing.
Syntax
The syntax for using $out
is as follows:
{ $out: }
collection_name
is the name of the collection where the results will be stored.
If the collection exists, it will be replaced with the new data; if it doesn’t exist, it
will be created.
Example
Let’s say you want to aggregate data from a collection of orders
and store the
results in a new collection called order_summary
:
db.orders.aggregate([
{ $group: { _id: "$customer_id", total: { $sum: "$amount" } } },
{ $out: "order_summary" }
])
This query will group the orders by customer_id
, calculate the total amount for
each customer, and store the result in the order_summary
collection.
Combining $unwind, $lookup, and $out
These advanced aggregation operators can be combined to create complex queries. For example,
you can unwind an array, perform a join using $lookup
, and then export the
results with $out
.
Example
Here’s an example that combines $unwind
, $lookup
, and
$out
:
db.orders.aggregate([
{ $unwind: "$items" },
{
$lookup: {
from: "products",
localField: "items.product_id",
foreignField: "_id",
as: "product_details"
}
},
{ $out: "processed_orders" }
])
This query will unwind the items
array, join the products
collection to get product details, and store the results in the
processed_orders
collection.
Best Practices for Using Advanced Aggregation Operators
- Optimize Performance: Use
$match
early in the pipeline to filter documents before using$unwind
and$lookup
, as these operations can be expensive. - Use $lookup Efficiently: When using
$lookup
, ensure that the fields involved in the join are indexed to improve performance. - Limit $out Usage: Be cautious when using
$out
, as it overwrites the target collection. Consider using temporary collections or ensuring the output collection is not critical. - Check the Pipeline Order: The order of operations in the aggregation pipeline affects performance. Always try to minimize the number of documents being processed at each stage.
Conclusion
Advanced aggregation operators like $unwind
, $lookup
, and
$out
are powerful tools in MongoDB's aggregation framework, allowing you to
manipulate and transform data in complex ways. By understanding and leveraging these
operators, you can perform sophisticated data processing and querying, making MongoDB a
highly flexible and powerful database for a wide range of applications.
Data Transformations Using Pipelines in MongoDB
In MongoDB, the aggregation pipeline is a powerful framework that allows you to transform and manipulate data in various ways. Pipelines consist of multiple stages, where each stage performs a specific operation on the data, such as filtering, grouping, sorting, and projecting. Data transformations using pipelines enable you to shape and format your data to meet the needs of your application.
What is an Aggregation Pipeline?
An aggregation pipeline is a sequence of stages that process data in a stream-like fashion. Each stage transforms the data as it passes through, and the output of one stage is passed to the next. The stages are executed in order, and the final result of the pipeline is the aggregated output.
Some of the most common stages in a pipeline include:
$match
: Filters the data based on a specified condition.$group
: Groups the data based on a specified field and performs aggregate functions.$project
: Shapes the data by including or excluding fields, or adding new fields.$sort
: Sorts the data based on one or more fields.$limit
: Limits the number of documents passed to the next stage.$skip
: Skips a specified number of documents in the pipeline.
Basic Example of an Aggregation Pipeline
Let’s consider a collection of sales records, where each document contains information about a product, quantity, and price. You can use an aggregation pipeline to calculate the total sales per product.
Example
The following pipeline performs the following operations:
- Filters sales records where the quantity is greater than 10.
- Groups the sales records by
product_name
and calculates the total sales by summing up theprice
multiplied byquantity
. - Sorts the results in descending order of total sales.
db.sales.aggregate([
{ $match: { quantity: { $gt: 10 } } },
{ $group: {
_id: "$product_name",
total_sales: { $sum: { $multiply: ["$price", "$quantity"] } }
}},
{ $sort: { total_sales: -1 } }
])
Using $project for Data Transformation
The $project
stage is used to reshape the documents passing through the
pipeline. You can include or exclude fields, add new computed fields, or rename fields. This
stage is helpful when you want to transform the data into a specific format for the
application.
Example
Let’s say you want to calculate the total cost per item in the sales collection, and only
return the product_name
, the quantity
, and the computed
total_cost
:
db.sales.aggregate([
{ $project: {
product_name: 1,
quantity: 1,
total_cost: { $multiply: ["$price", "$quantity"] }
}}
])
This pipeline will return the product name, quantity, and the total cost for each sale based on the price and quantity.
Using $group for Advanced Data Transformations
The $group
stage allows you to group documents by a specific field and perform
aggregation functions like $sum
, $avg
, $max
,
$min
, and $count
. It is essential for summarizing data, such as
calculating totals, averages, and counts.
Example
Let’s calculate the average price of each product across all sales records:
db.sales.aggregate([
{ $group: {
_id: "$product_name",
average_price: { $avg: "$price" }
}}
])
This query will group the sales records by product name and calculate the average price for each product.
Using $sort for Ordering Data
The $sort
stage is used to sort the documents based on one or more fields. You
can specify the sort order, where 1
is ascending and -1
is
descending.
Example
Let’s say you want to sort the sales records by the date
field in descending
order:
db.sales.aggregate([
{ $sort: { date: -1 } }
])
This query will return the sales records sorted by date in the descending order, with the most recent records appearing first.
Using $limit and $skip for Pagination
The $limit
and $skip
stages are useful for pagination.
$limit
restricts the number of documents passed to the next stage, while
$skip
skips a specified number of documents. These stages are commonly used
together to implement pagination in applications.
Example
Let’s say you want to paginate through a collection of sales records and return only 10 documents starting from the 11th document:
db.sales.aggregate([
{ $skip: 10 },
{ $limit: 10 }
])
This query will skip the first 10 documents and return the next 10 documents from the sales collection.
Combining Multiple Stages for Complex Transformations
MongoDB allows you to combine multiple stages in a pipeline to perform complex data
transformations. By chaining stages like $match
, $group
,
$project
, and $sort
, you can manipulate the data in various ways
to suit your needs.
Example
Here’s an example that combines multiple stages to calculate the total sales per product and sort the results in descending order:
db.sales.aggregate([
{ $match: { quantity: { $gt: 10 } } },
{ $group: {
_id: "$product_name",
total_sales: { $sum: { $multiply: ["$price", "$quantity"] } }
}},
{ $sort: { total_sales: -1 } }
])
This pipeline performs the following steps:
- Filters the sales records where quantity is greater than 10.
- Groups the sales records by product name and calculates the total sales.
- Sorts the results in descending order of total sales.
Best Practices for Using Aggregation Pipelines
- Use $match Early: Always apply
$match
as early as possible in the pipeline to reduce the number of documents passing through subsequent stages. - Minimize the Number of Stages: Keep the pipeline as simple as possible to avoid performance overhead.
- Indexing: Ensure that fields used in
$match
and$sort
are indexed to improve performance. - Use $project for Optimizing Data: Use
$project
to remove unnecessary fields and reduce the amount of data passed through the pipeline.
Conclusion
MongoDB’s aggregation pipeline is a powerful tool for performing data transformations and complex computations. By combining multiple stages in a pipeline, you can filter, group, sort, and reshape data to meet the needs of your application. Mastering these transformations is essential for building efficient and flexible data processing workflows in MongoDB.
Geospatial Queries and Geospatial Indexing in MongoDB
MongoDB provides powerful geospatial features that allow you to query and index geographic data, such as location coordinates, distances, and areas. Geospatial queries enable you to perform operations like finding nearby locations, calculating distances, and searching within geographic boundaries. MongoDB uses geospatial indexes to efficiently execute these types of queries.
What are Geospatial Queries?
Geospatial queries in MongoDB enable you to work with data that represents locations, such as longitude and latitude coordinates, and perform searches based on geographic criteria. These queries are essential for location-based applications like map-based services, delivery tracking, and geolocation-based search.
MongoDB supports two types of geospatial indexing: 2dsphere and 2d.
Types of Geospatial Indexes
MongoDB offers two types of geospatial indexes to optimize geospatial queries:
- 2dsphere Index: A 2dsphere index supports spherical geometry, allowing you to perform queries on data that represents points on the Earth's surface. This index is ideal for handling GPS coordinates (latitude and longitude).
- 2d Index: A 2d index supports flat, planar geometry and is used for legacy geospatial data that does not require spherical geometry. It is less accurate than a 2dsphere index and is typically used for applications that do not require high precision.
Creating Geospatial Indexes
To perform geospatial queries in MongoDB, you need to create an appropriate geospatial index
on the relevant field. For example, to create a 2dsphere
index on a field that
holds location data, you would run the following command:
db.locations.createIndex({ location: "2dsphere" })
This command creates a 2dsphere
index on the location
field of the
locations
collection. The location
field should contain a GeoJSON
object representing a point or other geographic shapes.
Geospatial Query Examples
Once a geospatial index is created, you can perform geospatial queries using MongoDB's geospatial operators. Below are some common examples of geospatial queries:
Find Locations Within a Certain Distance
To find locations within a specified distance from a given point, use the $near
operator. The following query finds all locations within 10 kilometers of a given point:
db.locations.find({
location: {
$near: {
$geometry: { type: "Point", coordinates: [-73.97, 40.77] },
$maxDistance: 10000
}
}
})
This query finds all documents in the locations
collection where the
location
field is within 10 kilometers of the point with coordinates
[-73.97, 40.77]
(latitude and longitude).
Find Locations Within a Polygon
To find locations within a defined polygon, use the $geoWithin
operator with a
GeoJSON polygon object. This example finds locations within a specified polygon:
db.locations.find({
location: {
$geoWithin: {
$geometry: {
type: "Polygon",
coordinates: [
[
[-73.97, 40.77],
[-73.98, 40.75],
[-73.95, 40.74],
[-73.96, 40.76],
[-73.97, 40.77]
]
]
}
}
}
})
This query finds all locations within the polygon defined by the given coordinates (longitude, latitude).
Find Locations Within a Circle
To find locations within a circle, use the $geoWithin
operator with the
$centerSphere
modifier. The following query finds all locations within a
5-kilometer radius of a point:
db.locations.find({
location: {
$geoWithin: {
$centerSphere: [ [-73.97, 40.77], 5 / 3963.2 ] // Radius in radians (5 km)
}
}
})
This query finds all locations within a 5-kilometer radius of the point with coordinates
[-73.97, 40.77]
. The radius is specified in radians, so the value
5 / 3963.2
represents 5 kilometers.
Geospatial Data Types
MongoDB supports GeoJSON objects for representing geospatial data. Here are the common GeoJSON data types:
- Point: Represents a single location on the Earth's surface (e.g., latitude, longitude).
- Polygon: Represents a polygonal area, defined by a set of coordinates that form the boundary.
- LineString: Represents a series of connected line segments.
GeoJSON data types are used in MongoDB queries to represent geographic features such as points, lines, and areas.
Best Practices for Geospatial Indexing
- Use 2dsphere Index for Spherical Geometry: When dealing with GPS
coordinates, always use the
2dsphere
index, as it supports spherical geometry and is ideal for calculating distances on the Earth's surface. - Limit the Scope of Geospatial Queries: To improve performance, try to limit the scope of your geospatial queries by specifying a smaller search radius or using other filters alongside geospatial queries.
- Index Only Relevant Fields: Create geospatial indexes only on fields that will be used in geospatial queries. Indexing unnecessary fields can reduce performance.
- Use GeoJSON Format: Always store geospatial data in GeoJSON format for consistency and compatibility with MongoDB's geospatial operators.
Conclusion
Geospatial queries and geospatial indexing in MongoDB provide powerful tools for working with
geographic data. By using geospatial operators like $near
,
$geoWithin
, and $centerSphere
, you can perform location-based
searches efficiently. Geospatial indexing ensures that these queries are fast and scalable,
making MongoDB a great choice for location-based applications such as mapping, geolocation
services, and spatial analytics.
Connecting MongoDB with Node.js (Mongoose or Native Driver)
Connecting MongoDB with a Node.js application allows you to interact with the database from your backend server. There are two main ways to connect MongoDB with Node.js: using the native MongoDB driver or using an ODM (Object Data Modeling) library like Mongoose. Both methods have their advantages, and the choice depends on your project's needs.
1. Using Mongoose
Mongoose is a popular library that provides a higher-level abstraction to MongoDB, making it easier to interact with MongoDB by providing features like schema validation, middleware, and data modeling. Mongoose is often preferred for its ease of use and the ability to enforce data schemas.
Installing Mongoose
To get started with Mongoose, you need to install it in your Node.js project:
npm install mongoose
Connecting to MongoDB using Mongoose
Once Mongoose is installed, you can connect to MongoDB using the following code:
const mongoose = require('mongoose');
// Connection string to your MongoDB instance
const uri = 'mongodb://localhost:27017/mydatabase'; // Replace with your MongoDB URI
// Connect to MongoDB
mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => {
console.log('Connected to MongoDB');
})
.catch((err) => {
console.error('Error connecting to MongoDB:', err);
});
In the example above, we use Mongoose's connect()
method to establish a
connection to the MongoDB server. The connection string should include the MongoDB URI,
which can be either a local database or a cloud-based MongoDB instance (like MongoDB Atlas).
Defining a Mongoose Schema and Model
Once the connection is established, you can define a Mongoose schema and model to interact with your MongoDB database:
const Schema = mongoose.Schema;
// Define a schema for a "User" collection
const userSchema = new Schema({
name: String,
email: { type: String, unique: true },
age: Number
});
// Create a model based on the schema
const User = mongoose.model('User', userSchema);
// Create a new user instance and save it to the database
const newUser = new User({
name: 'John Doe',
email: 'johndoe@example.com',
age: 30
});
newUser.save()
.then(() => {
console.log('User saved to the database');
})
.catch((err) => {
console.error('Error saving user:', err);
});
In this example, we define a schema for the "User" collection with fields like
name
, email
, and age
. Then, we create a model based
on the schema and use it to insert a new user document into the database.
2. Using the Native MongoDB Driver
The native MongoDB driver provides a more direct way to interact with MongoDB. While it doesn't offer the features that Mongoose does (like schema validation), it gives you more control over your database operations.
Installing the MongoDB Native Driver
To use the native MongoDB driver, install it with the following command:
npm install mongodb
Connecting to MongoDB using the Native Driver
To connect to MongoDB using the native driver, use the following code:
const { MongoClient } = require('mongodb');
// Connection string to your MongoDB instance
const uri = 'mongodb://localhost:27017'; // Replace with your MongoDB URI
// Create a new MongoClient instance
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
// Connect to the MongoDB server
client.connect()
.then(() => {
console.log('Connected to MongoDB');
// Access the "mydatabase" database
const db = client.db('mydatabase');
// Access the "users" collection
const usersCollection = db.collection('users');
// Insert a new user document into the collection
return usersCollection.insertOne({
name: 'Jane Smith',
email: 'janesmith@example.com',
age: 25
});
})
.then((result) => {
console.log('User inserted:', result);
})
.catch((err) => {
console.error('Error connecting to MongoDB:', err);
})
.finally(() => {
// Close the MongoDB connection
client.close();
});
In this example, we use the MongoClient
class from the native driver to connect
to MongoDB. After the connection is established, we access the "mydatabase" database and the
"users" collection to insert a new user document into the collection.
Choosing Between Mongoose and the Native Driver
Here are some points to consider when deciding between Mongoose and the native MongoDB driver:
- Mongoose: Use Mongoose if you want an easier and more feature-rich way to interact with MongoDB. Mongoose provides schema validation, middleware, and built-in methods, making it a great option for complex applications.
- Native Driver: Use the native MongoDB driver if you need more control over your database operations, or if you prefer to handle data modeling and validation manually. The native driver is more lightweight and flexible.
Conclusion
Connecting MongoDB with Node.js is straightforward using either Mongoose or the native MongoDB driver. Mongoose is ideal for developers who prefer an ODM with built-in features like schema validation and middleware, while the native driver provides a lightweight and flexible approach. Depending on your project's needs, either option can help you effectively interact with MongoDB in your Node.js application.
MongoDB Connections in Python, Java, and Other Languages
MongoDB can be connected to various programming languages, each with its own driver or library to interact with the database. Below, we will discuss how to connect MongoDB with Python, Java, and other popular languages.
1. Connecting MongoDB with Python
In Python, MongoDB is typically accessed using the PyMongo library, which provides a simple and efficient way to interact with MongoDB.
Installing PyMongo
To get started with PyMongo, install it using pip
:
pip install pymongo
Connecting to MongoDB using PyMongo
Once PyMongo is installed, you can connect to MongoDB using the following Python code:
from pymongo import MongoClient
# Connection string to MongoDB (localhost or MongoDB Atlas)
uri = 'mongodb://localhost:27017/' # Replace with your URI
# Create a MongoClient instance
client = MongoClient(uri)
# Access a database
db = client['mydatabase']
# Access a collection
collection = db['users']
# Insert a document into the collection
collection.insert_one({'name': 'John Doe', 'email': 'johndoe@example.com'})
print('Document inserted')
The code connects to MongoDB using the MongoClient
class and accesses the
"mydatabase" database and "users" collection. Then, it inserts a simple document into the
collection.
2. Connecting MongoDB with Java
In Java, you can use the MongoDB Java Driver to connect to MongoDB and perform database operations.
Installing the MongoDB Java Driver
To use the MongoDB Java Driver, include the following dependency in your pom.xml
if you're using Maven:
org.mongodb
mongo-java-driver
4.5.1
Connecting to MongoDB using the Java Driver
Once the driver is added to your project, you can connect to MongoDB with the following code:
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
public class MongoDBExample {
public static void main(String[] args) {
// Connection string to MongoDB (localhost or MongoDB Atlas)
String uri = "mongodb://localhost:27017"; // Replace with your URI
// Create a MongoClient instance
MongoClient client = MongoClients.create(uri);
// Access a database
MongoDatabase database = client.getDatabase("mydatabase");
// Access a collection
var collection = database.getCollection("users");
// Create a new document
Document newUser = new Document("name", "Jane Doe")
.append("email", "janedoe@example.com");
// Insert the document into the collection
collection.insertOne(newUser);
System.out.println("Document inserted");
// Close the connection
client.close();
}
}
The Java example shows how to connect to MongoDB using the MongoClients.create()
method, access a database and collection, and insert a document into MongoDB.
3. Connecting MongoDB with Other Languages
MongoDB supports connections in various other languages. Below are some common drivers for other languages:
- Node.js: Use the MongoDB Node.js Driver to connect to MongoDB in Node.js applications.
- C# (.NET): The MongoDB .NET Driver allows you to use MongoDB from C# applications.
- PHP: Use the MongoDB PHP Driver for interacting with MongoDB in PHP.
- Go: The MongoDB Go Driver is used for MongoDB integration in Go applications.
- Ruby: The MongoDB Ruby Driver helps Ruby developers integrate MongoDB.
Example: Connecting MongoDB with Go
Here’s an example of connecting MongoDB in Go using the MongoDB Go Driver:
package main
import (
"fmt"
"log"
"context"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
"go.mongodb.org/mongo-driver/bson"
)
func main() {
// Create a MongoClient instance
client, err := mongo.NewClient(options.Client().ApplyURI("mongodb://localhost:27017"))
if err != nil {
log.Fatal(err)
}
// Connect to MongoDB
err = client.Connect(context.Background())
if err != nil {
log.Fatal(err)
}
defer client.Disconnect(context.Background())
// Access a database
collection := client.Database("mydatabase").Collection("users")
// Insert a document
newUser := bson.D{{"name", "Alice Smith"}, {"email", "alice@example.com"}}
_, err = collection.InsertOne(context.Background(), newUser)
if err != nil {
log.Fatal(err)
}
fmt.Println("Document inserted")
}
In the Go example, we use the MongoDB Go Driver to connect to MongoDB, access a database, and insert a document into the "users" collection.
Conclusion
MongoDB can be easily connected to various programming languages using official drivers. Whether you're using Python, Java, Go, or another language, you can leverage MongoDB's flexible and powerful features in your applications. Each driver provides the necessary tools to interact with MongoDB, perform CRUD operations, and manage connections efficiently.
Using MongoDB Atlas with a Driver
MongoDB Atlas is a fully managed cloud database that provides a scalable and secure MongoDB service. Atlas allows you to easily set up, manage, and scale MongoDB clusters without worrying about hardware and infrastructure. You can use MongoDB Atlas with various drivers in different programming languages to connect to your cloud-based MongoDB instance. Below, we'll show you how to connect MongoDB Atlas with drivers for Python, Java, and Node.js.
1. Creating a MongoDB Atlas Cluster
Before connecting to MongoDB Atlas using a driver, you need to create a cluster on MongoDB Atlas. Follow these steps:
- Go to the MongoDB Atlas website and sign up or log in to your account.
- Click on Create Cluster and choose your cloud provider (AWS, Google Cloud, or Azure) and region.
- Once the cluster is created, go to the Database Access section and create a new database user with the required privileges.
- Next, navigate to the Network Access section and add your IP address to the IP whitelist.
- Finally, in the Clusters section, click on Connect to get your connection string. Choose Connect your application and copy the connection string.
Now that you have your MongoDB Atlas connection string, you can use it to connect MongoDB Atlas with your preferred programming language.
2. Connecting MongoDB Atlas with Python
In Python, you can use the PyMongo library to connect to MongoDB Atlas. Here’s how to do it:
Install PyMongo
First, install the PyMongo package:
pip install pymongo
Connecting to MongoDB Atlas using PyMongo
Use the connection string from MongoDB Atlas to connect to the cluster:
from pymongo import MongoClient
# MongoDB Atlas connection string (replace and with your credentials)
uri = "mongodb+srv://:@cluster0.mongodb.net/?retryWrites=true&w=majority"
# Create a MongoClient instance
client = MongoClient(uri)
# Access a database
db = client['mydatabase']
# Access a collection
collection = db['users']
# Insert a document
collection.insert_one({'name': 'John Doe', 'email': 'johndoe@example.com'})
print('Document inserted')
This code connects to your MongoDB Atlas cluster and inserts a document into the "users" collection of the "mydatabase" database.
3. Connecting MongoDB Atlas with Java
In Java, the MongoDB Java Driver can be used to connect MongoDB Atlas. Here’s how to do it:
Install MongoDB Java Driver
Add the MongoDB Java Driver dependency to your pom.xml
file:
org.mongodb
mongo-java-driver
4.5.1
Connecting to MongoDB Atlas using Java
Use the connection string from MongoDB Atlas to connect to the cluster:
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
public class MongoDBExample {
public static void main(String[] args) {
// MongoDB Atlas connection string
String uri = "mongodb+srv://:@cluster0.mongodb.net/mydatabase?retryWrites=true&w=majority";
// Create a MongoClient instance
MongoClient client = MongoClients.create(uri);
// Access a database
MongoDatabase database = client.getDatabase("mydatabase");
// Access a collection
var collection = database.getCollection("users");
// Create a new document
Document newUser = new Document("name", "Jane Doe")
.append("email", "janedoe@example.com");
// Insert the document into the collection
collection.insertOne(newUser);
System.out.println("Document inserted");
// Close the connection
client.close();
}
}
This Java example connects to MongoDB Atlas using the connection string, accesses a database and collection, and inserts a document.
4. Connecting MongoDB Atlas with Node.js
In Node.js, you can use the MongoDB Node.js Driver or Mongoose library to connect to MongoDB Atlas. Here’s how to do it with the Node.js driver:
Install MongoDB Node.js Driver
Install the MongoDB Node.js driver using npm:
npm install mongodb
Connecting to MongoDB Atlas using Node.js
Use the connection string from MongoDB Atlas to connect to the cluster:
const { MongoClient } = require('mongodb');
// MongoDB Atlas connection string
const uri = "mongodb+srv://:@cluster0.mongodb.net/mydatabase?retryWrites=true&w=majority";
// Create a MongoClient instance
const client = new MongoClient(uri);
async function main() {
try {
// Connect to MongoDB Atlas
await client.connect();
// Access a database
const database = client.db("mydatabase");
// Access a collection
const collection = database.collection("users");
// Insert a document
const result = await collection.insertOne({ name: "Alice Smith", email: "alice@example.com" });
console.log(`Document inserted with ID: ${result.insertedId}`);
} finally {
// Close the connection
await client.close();
}
}
main().catch(console.error);
This Node.js example connects to MongoDB Atlas using the connection string, accesses a database and collection, and inserts a document.
Conclusion
MongoDB Atlas makes it easy to manage and scale your MongoDB clusters in the cloud. By using the connection string provided by Atlas, you can seamlessly connect MongoDB Atlas with drivers in different programming languages such as Python, Java, and Node.js. This allows you to take advantage of MongoDB’s powerful features in your cloud-based applications.
Authentication and User Roles in MongoDB
In MongoDB, authentication is the process of verifying the identity of users, and user roles define the level of access and the actions users are permitted to perform on the MongoDB database. MongoDB offers robust features for managing security, allowing administrators to enforce access controls, ensuring that only authorized users can access or modify sensitive data.
1. Authentication in MongoDB
MongoDB supports several methods of authentication:
- Username and Password Authentication: This is the most basic form of authentication where users are identified by their username and password.
- LDAP Authentication: MongoDB supports integration with LDAP (Lightweight Directory Access Protocol) to authenticate users against an external directory service.
- X.509 Certificate Authentication: This allows users to authenticate using SSL/TLS certificates, providing enhanced security for communication between MongoDB and clients.
- Kerberos Authentication: MongoDB supports Kerberos for centralized authentication, commonly used in enterprise environments.
By default, MongoDB does not enable authentication, and anyone can access the database. However, enabling authentication ensures that only authorized users can interact with the database.
Enabling Authentication
To enable authentication in MongoDB, you need to modify the mongod.conf
configuration file and restart the MongoDB server:
# In mongod.conf, enable authorization
security:
authorization: "enabled"
Once enabled, you can manage users and roles in MongoDB.
2. User Roles in MongoDB
MongoDB uses a role-based access control (RBAC) system to manage permissions. With RBAC, roles define the actions that users can perform on various resources in MongoDB, such as databases and collections. MongoDB provides several built-in roles, but you can also create custom roles to fit your application’s needs.
Built-in Roles
MongoDB comes with predefined roles that provide a range of privileges:
Role | Description |
---|---|
read | Provides read-only access to all data in the database. |
readWrite | Provides read and write access to all data in the database. |
dbAdmin | Provides administrative privileges to manage indexes and other database-level configurations. |
userAdmin | Allows managing users and roles on the database. |
root | Provides full access to all databases and administrative functions in MongoDB. |
clusterAdmin | Provides administrative access to cluster-level operations (e.g., sharding, replication). |
Creating Custom Roles
You can create custom roles tailored to your specific needs using MongoDB’s
createRole()
method. Here’s how to create a custom role:
// Create a custom role
db.createRole({
role: "customRole",
privileges: [
{
resource: { db: "mydatabase", collection: "" },
actions: [ "find", "insert" ]
}
],
roles: []
});
This example creates a role named "customRole" that allows users to find and insert documents in the "mydatabase" database.
3. Managing Users in MongoDB
Users are created and assigned roles in MongoDB to control access to resources. Here’s how you can manage users:
Creating a User
To create a new user and assign roles, use the createUser()
method. The
following example shows how to create a new user with the "readWrite" role:
// Create a user with readWrite access
db.createUser({
user: "myUser",
pwd: "myPassword",
roles: [ { role: "readWrite", db: "mydatabase" } ]
});
This creates a new user named "myUser" with "readWrite" access to the "mydatabase" database. You can replace the username and password with your own values.
Listing Users
To view all users in the current database, use the show users
command:
show users
Modifying a User
To modify a user's roles, use the grantRolesToUser()
or
revokeRolesFromUser()
methods. For example:
// Grant a role to an existing user
db.grantRolesToUser("myUser", [ { role: "dbAdmin", db: "mydatabase" } ]);
This grants the "dbAdmin" role to the user "myUser" on the "mydatabase" database.
Deleting a User
To delete a user, use the dropUser()
method:
// Delete a user
db.dropUser("myUser");
This deletes the user "myUser" from the current database.
4. Authentication and Authorization Best Practices
To ensure the security of your MongoDB deployment, follow these best practices for authentication and authorization:
- Enable Authentication: Always enable authentication to prevent unauthorized access.
- Use Strong Passwords: Ensure all user passwords are complex and hard to guess.
- Use Role-Based Access Control: Assign users only the roles they need to minimize access to sensitive data.
- Audit Access: Regularly review user roles and permissions to ensure they align with your security policies.
- Use TLS/SSL: Encrypt communication between MongoDB and clients using TLS/SSL to prevent man-in-the-middle attacks.
Conclusion
Authentication and user roles are crucial for securing your MongoDB deployment. By enabling authentication and using roles, you can control who has access to your data and what actions they can perform. MongoDB’s flexible RBAC system allows you to create custom roles and assign them to users, ensuring that your database is both secure and tailored to your application’s needs.
Enabling and Using SCRAM and LDAP Authentication in MongoDB
MongoDB supports multiple authentication mechanisms, including SCRAM (Salted Challenge Response Authentication Mechanism) and LDAP (Lightweight Directory Access Protocol). These authentication methods provide additional layers of security and allow MongoDB deployments to integrate with external systems for managing user credentials.
1. SCRAM Authentication in MongoDB
SCRAM is the default authentication mechanism in MongoDB and is based on the challenge-response mechanism. It is secure, efficient, and widely used for authenticating users directly within MongoDB without relying on an external system.
Enabling SCRAM Authentication
To enable SCRAM authentication in MongoDB, you need to modify the mongod.conf
configuration file and restart the MongoDB server:
# In mongod.conf, enable authentication
security:
authorization: "enabled"
authenticationMechanisms: ["SCRAM-SHA-256", "SCRAM-SHA-1"]
The above configuration enables both SCRAM-SHA-256 and SCRAM-SHA-1 as valid authentication mechanisms. You can choose to enable either one depending on your requirements.
Creating a User with SCRAM Authentication
Once SCRAM authentication is enabled, you can create a new user with the
createUser()
method. Here's an example:
// Create a user with SCRAM authentication
db.createUser({
user: "scramUser",
pwd: "securePassword123",
roles: [ { role: "readWrite", db: "mydatabase" } ]
});
This creates a user named "scramUser" with a password "securePassword123" and assigns the "readWrite" role on the "mydatabase" database.
Authenticating with SCRAM
To authenticate using SCRAM, you can use the mongo
shell or connect from a
MongoDB driver. Example of using the shell:
# Connect to the MongoDB instance with SCRAM authentication
mongo --username scramUser --password securePassword123 --authenticationDatabase admin
This command connects to MongoDB as the "scramUser" user and authenticates against the "admin" database.
2. LDAP Authentication in MongoDB
LDAP authentication allows MongoDB to authenticate users based on an external LDAP server. This is useful for organizations that want to centralize user management and authentication across various services using LDAP directories like Microsoft Active Directory or OpenLDAP.
Enabling LDAP Authentication
To enable LDAP authentication in MongoDB, you need to configure the mongod.conf
file to specify the LDAP server details:
# In mongod.conf, enable LDAP authentication
security:
authorization: "enabled"
authenticationMechanisms: ["LDAP"]
ldap:
servers: ["ldap://your-ldap-server:389"]
bind:
method: "simple"
dn: "cn=admin,dc=example,dc=com"
password: "ldapPassword"
userToDNMapping:
- clientField: "username"
ldapField: "uid"
dbField: "user"
groupSearchBase: "ou=groups,dc=example,dc=com"
groupSearchFilter: "(&(objectClass=posixGroup)(memberUid={USER}))"
ssl:
enabled: false
bindAsMappedUser: false
usernameField: "uid"
passwordField: "userPassword"
ldapAuthMechanism: "GSSAPI"
In this configuration:
- servers: Specifies the LDAP server to which MongoDB will connect.
- bind: Defines the method and credentials MongoDB uses to bind to the LDAP server.
- userToDNMapping: Maps MongoDB users to LDAP users based on the provided field mappings.
- ssl: Optionally enables SSL for secure communication with the LDAP server.
Once LDAP authentication is enabled, MongoDB will authenticate users against the LDAP directory instead of using its internal authentication system.
Creating Users for LDAP Authentication
Users who are authenticated through LDAP don’t need to be created manually in MongoDB. Instead, MongoDB will check the LDAP server for users and authenticate them based on the LDAP credentials. However, you still need to assign roles within MongoDB to control access.
Authenticating with LDAP
To authenticate using LDAP, you can use the mongo
shell with the
--authenticationMechanism
option:
# Connect to MongoDB with LDAP authentication
mongo --username ldapUser --password ldapPassword --authenticationDatabase "$external" --authenticationMechanism "LDAP"
This connects to MongoDB as the "ldapUser" user and authenticates using the LDAP directory.
3. Best Practices for SCRAM and LDAP Authentication
- SCRAM Security: Use strong, complex passwords for SCRAM users and opt for SCRAM-SHA-256 for the best security.
- LDAP Security: Ensure that your LDAP server is securely configured, preferably using SSL/TLS to encrypt communication between MongoDB and the LDAP server.
- Role Management: Use role-based access control (RBAC) to define user permissions and restrict access based on the principle of least privilege.
- Secure Connections: Always use encrypted connections (SSL/TLS) when possible to protect user credentials during authentication.
- Audit Logging: Enable audit logging to keep track of authentication events and ensure compliance with security policies.
4. Conclusion
MongoDB offers flexible authentication mechanisms, including SCRAM and LDAP. SCRAM provides a simple and secure way to authenticate users within MongoDB, while LDAP integration allows for centralizing user management in enterprise environments. By enabling and configuring these authentication methods properly, you can secure your MongoDB deployment and control access to sensitive data.
IP Whitelisting and Network Security in MongoDB
IP whitelisting and network security are critical components of securing a MongoDB deployment. By controlling which IP addresses can access your MongoDB server, you can minimize the risk of unauthorized access. MongoDB offers several network security features, such as IP whitelisting, firewalls, and network encryption, to protect your data from external threats.
1. IP Whitelisting in MongoDB
IP whitelisting allows you to specify a list of trusted IP addresses or IP ranges that are allowed to connect to your MongoDB instance. Any IP address not on this list will be denied access, providing an additional layer of security to your deployment.
Enabling IP Whitelisting
IP whitelisting is typically configured on the network or firewall level. If you are using MongoDB Atlas (the cloud-based MongoDB service), you can configure IP whitelisting directly through the Atlas UI. For self-hosted MongoDB instances, you can configure IP whitelisting using your firewall or security groups in cloud environments like AWS, GCP, or Azure.
Configuring IP Whitelisting in MongoDB Atlas
In MongoDB Atlas, setting up IP whitelisting is straightforward:
- Log in to MongoDB Atlas and navigate to your project.
- Click on the "Network Access" tab in the left sidebar.
- Click the "Add IP Address" button to add the IP addresses or ranges that you want to allow.
- Enter the IP address or CIDR block and click "Confirm."
This will whitelist the specified IP addresses, allowing only those IPs to access your MongoDB cluster.
Configuring IP Whitelisting for Self-Hosted MongoDB
If you are running a self-hosted MongoDB instance, you can configure IP whitelisting using a
firewall or cloud security group settings. Here's a basic example using
iptables
on a Linux server:
# Allow access from a specific IP address (e.g., 192.168.1.100)
sudo iptables -A INPUT -p tcp -s 192.168.1.100 --dport 27017 -j ACCEPT
# Deny access to all other IP addresses
sudo iptables -A INPUT -p tcp --dport 27017 -j DROP
In this example, we explicitly allow connections to port 27017 (the default MongoDB port)
from IP
192.168.1.100, and block all other IP addresses.
2. Network Security for MongoDB
Along with IP whitelisting, there are several other network security measures you can take to ensure that your MongoDB deployment is secure:
- Firewall Configuration: Ensure that your MongoDB instance is behind a firewall to protect it from unauthorized access. Firewalls should block all access to MongoDB’s ports (default is 27017) except for trusted IP addresses.
- VPC Peering: If you're running MongoDB on a cloud service like AWS, consider using Virtual Private Cloud (VPC) peering to restrict access to your MongoDB cluster from specific VPCs or subnets.
- SSL/TLS Encryption: Encrypt communication between MongoDB clients and servers using SSL/TLS to prevent eavesdropping and man-in-the-middle attacks. MongoDB supports SSL/TLS encryption out of the box, and you can configure it by modifying the
mongod.conf
file.
Enabling SSL/TLS Encryption
To enable SSL/TLS encryption in MongoDB, you need to modify your mongod.conf
file to include the following settings:
# Enable SSL/TLS encryption
net:
ssl:
mode: requireSSL
PEMKeyFile: /path/to/your/mongodb.pem
PEMKeyPassword: your-password
CAFile: /path/to/your/ca.pem
clusterFile: /path/to/your/cluster-cert.pem
allowConnectionsWithoutCertificates: false
This configuration ensures that MongoDB only allows encrypted connections, requiring SSL/TLS certificates for all client connections.
3. Best Practices for Network Security
- Use Strong Authentication: Always use authentication mechanisms like SCRAM or LDAP to ensure that only authorized users can connect to your MongoDB instance. Enabling authentication is critical for controlling access to your data.
- Use TLS/SSL Encryption: Always use TLS/SSL encryption to secure the communication channel between MongoDB clients and servers. This protects data in transit from being intercepted or tampered with.
- Limit Access with IP Whitelisting: Enable IP whitelisting to restrict access to MongoDB from only trusted IP addresses. This helps prevent unauthorized access from malicious IP addresses.
- Regularly Update MongoDB: Keep your MongoDB installation up to date with the latest patches and security updates to protect against known vulnerabilities.
- Monitor Network Traffic: Regularly monitor network traffic to and from your MongoDB instance. Use monitoring tools like MongoDB Ops Manager or third-party solutions to track access patterns and detect unusual activities.
4. Conclusion
Securing your MongoDB deployment requires a multi-layered approach, and IP whitelisting is one of the most effective methods for controlling access. By combining IP whitelisting with other network security measures like SSL/TLS encryption, firewalls, and strong authentication, you can ensure that your MongoDB instance is protected from unauthorized access and potential threats.
TLS/SSL Configuration in MongoDB
Transport Layer Security (TLS) and Secure Sockets Layer (SSL) are cryptographic protocols designed to provide secure communication over a computer network. In MongoDB, TLS/SSL is used to encrypt the communication between clients and servers to prevent eavesdropping, tampering, and forgery of data. Configuring TLS/SSL in MongoDB ensures that all data exchanged between MongoDB clients and servers is transmitted securely.
1. Why Use TLS/SSL in MongoDB?
Enabling TLS/SSL encryption in MongoDB provides the following benefits:
- Data Encryption: Protects sensitive data from being intercepted during transmission between clients and servers.
- Authentication: Verifies the identity of both the MongoDB server and the client, ensuring they are who they claim to be.
- Integrity: Ensures that the data sent between the client and server is not altered or tampered with during transmission.
2. Enabling TLS/SSL Encryption in MongoDB
To enable TLS/SSL encryption, you need to configure MongoDB to use SSL certificates for both server and client communication. The steps are as follows:
Step 1: Generate SSL Certificates
You need to generate or obtain SSL certificates for your MongoDB server. The process involves generating a public-private key pair and a certificate signing request (CSR) to get an SSL certificate from a certificate authority (CA). Here's an example of how to create a self-signed certificate:
# Generate a private key
openssl genpkey -algorithm RSA -out mongodb.key
# Generate a self-signed certificate
openssl req -new -x509 -key mongodb.key -out mongodb.crt -days 365
These commands create a private key (mongodb.key
) and a self-signed certificate
(mongodb.crt
) that will be used for MongoDB's TLS/SSL encryption.
Step 2: Configure MongoDB to Use SSL Certificates
Edit the mongod.conf
configuration file to enable TLS/SSL and specify the paths
to your SSL certificate and key files. For example:
net:
ssl:
mode: requireSSL
PEMKeyFile: /path/to/mongodb.crt
PEMKeyPassword: your-password
CAFile: /path/to/ca.crt
clusterFile: /path/to/cluster-cert.pem
allowConnectionsWithoutCertificates: false
In this configuration:
mode: requireSSL
ensures that MongoDB only accepts SSL/TLS connections.PEMKeyFile
specifies the path to the server’s SSL certificate.PEMKeyPassword
is the password for the certificate (if applicable).CAFile
is the path to the certificate authority’s certificate for verifying client certificates.allowConnectionsWithoutCertificates
determines whether clients can connect without certificates (set tofalse
for mandatory client certificates).
Step 3: Restart MongoDB
After modifying the mongod.conf
file, restart MongoDB to apply the changes:
sudo systemctl restart mongod
This will restart MongoDB with SSL enabled and your certificates loaded.
3. Enabling TLS/SSL for MongoDB Clients
Once TLS/SSL is configured on the MongoDB server, clients must also be configured to use SSL
for connections. For example, when using the MongoDB shell, you can connect to the server
with the --ssl
option:
mongo --ssl --sslCAFile /path/to/ca.crt --sslPEMKeyFile /path/to/client.pem --host your-mongo-server
In this command:
--ssl
tells the MongoDB shell to use SSL for the connection.--sslCAFile
points to the certificate authority’s certificate.--sslPEMKeyFile
points to the client’s SSL certificate.
For MongoDB drivers (Node.js, Python, etc.), you will also need to specify SSL-related options in the connection string or configuration settings of the driver.
4. Verifying TLS/SSL Encryption
To verify that SSL/TLS is correctly enabled, you can check the MongoDB logs for SSL connection information:
tail -f /var/log/mongodb/mongod.log
The log should indicate that SSL is enabled and that secure connections are being established.
5. Best Practices for TLS/SSL in MongoDB
- Use a Trusted Certificate Authority (CA): While self-signed certificates work, it is recommended to use SSL certificates from a trusted certificate authority for production environments to avoid certificate trust issues.
- Ensure Strong Encryption: Use strong encryption algorithms, such as AES-256, and keep your private keys secure.
- Regularly Rotate Certificates: Periodically rotate your certificates to maintain a high level of security and avoid potential security breaches.
- Limit Access: Use firewalls and network security rules to restrict access to your MongoDB instance to only trusted IP addresses.
- Enable Client Authentication: If required, enable client certificate authentication to ensure that only authorized clients can connect to MongoDB.
6. Conclusion
Enabling TLS/SSL encryption in MongoDB is an essential step in securing your data during transmission. By following best practices and configuring the appropriate certificates, you can protect your MongoDB instance from various security threats and ensure the confidentiality and integrity of your data.
Setting Up Replica Sets in MongoDB
A replica set in MongoDB is a group of mongod instances that maintain the same data set, providing redundancy and high availability. Replica sets are a crucial component of MongoDB's fault tolerance, enabling automatic failover and data replication across multiple nodes. This section guides you through the process of setting up replica sets in MongoDB.
1. Why Use Replica Sets?
Replica sets offer several advantages, including:
- High Availability: In case of a primary node failure, MongoDB automatically promotes a secondary node to be the new primary, ensuring uninterrupted service.
- Data Redundancy: All data is replicated across multiple nodes, preventing data loss in case of a hardware failure.
- Read Scalability: Secondary nodes can handle read operations, distributing the workload across multiple servers and improving performance.
2. Setting Up a Replica Set with 3 Nodes
To set up a basic replica set with 3 nodes (1 primary and 2 secondaries), follow the steps below:
Step 1: Install MongoDB on All Nodes
Ensure that MongoDB is installed on all machines (or virtual machines) that will be part of the replica set. You can install MongoDB by following the installation instructions for your operating system from the official MongoDB website.
Step 2: Configure Each Node
On each node, you need to configure MongoDB to enable replica set functionality. This is done
by editing the mongod.conf
configuration file.
# Edit the mongod.conf file
replication:
replSetName: "rs0"
Here, the replSetName
should be the same for all nodes in the replica set. In
this example, we have named the replica set "rs0".
Step 3: Start MongoDB on All Nodes
Start the MongoDB server on each node. For example:
mongod --config /path/to/mongod.conf
This command starts the MongoDB server using the specified configuration file.
Step 4: Initialize the Replica Set
After starting the MongoDB instances on all nodes, connect to one of the nodes (usually the primary node) using the MongoDB shell:
mongo --host
Once connected, initiate the replica set:
rs.initiate()
The rs.initiate()
command initializes the replica set on the primary node.
Step 5: Add Secondaries to the Replica Set
Next, add the secondary nodes to the replica set. On the primary node, run the following command:
rs.add("")
Repeat this command for each secondary node you want to add to the replica set. This command adds the secondary nodes to the replica set configuration.
Step 6: Check the Replica Set Status
To verify that the replica set is working correctly, check the status of the replica set:
rs.status()
This command provides information about the replica set members, including their status (primary, secondary, etc.), health, and sync status.
3. Replica Set Configuration Details
Each node in a replica set has a role:
- Primary: The primary node is the main node that accepts both read and write operations. Only one primary node exists in a replica set at any given time.
- Secondary: Secondary nodes replicate the data from the primary node. They can also serve read requests if configured to do so.
- Arbiter: An arbiter is a special type of node that does not store data but participates in the election process to determine the new primary node in case the current primary goes down. Arbiters are useful in odd-numbered replica sets to avoid split-brain scenarios.
4. Replica Set Elections and Failover
Replica sets provide automatic failover in case of a failure of the primary node. When the current primary node is unavailable, MongoDB will automatically trigger an election process to elect a new primary node from the available secondaries. This process ensures that your application can continue to operate with minimal downtime.
The election process is initiated by the secondaries, and the node with the most up-to-date data will become the new primary. If necessary, an arbiter can participate in the election to help break ties.
5. Configuring Read and Write Preferences
MongoDB allows you to configure read and write preferences to control how your application interacts with the replica set. Some common configurations include:
- Primary: Directs all read and write operations to the primary node.
- Secondary: Directs all read operations to the secondary nodes, which can be useful for read-heavy applications.
- Nearest: Reads from the node with the lowest network latency.
6. Best Practices for Replica Sets
- Use an Odd Number of Nodes: It is recommended to have an odd number of nodes in your replica set to avoid tie situations during elections. Typically, a 3-node setup is ideal.
- Monitor Replica Set Health: Regularly monitor the health of your
replica set using tools like
rs.status()
and MongoDB Atlas to identify potential issues early. - Deploy in Different Zones: To increase fault tolerance, deploy your replica set members in different availability zones or data centers.
- Enable Read Preferences: Configure read preferences to direct read traffic to secondary nodes, offloading the primary node and improving performance.
7. Conclusion
Setting up a replica set in MongoDB provides high availability, data redundancy, and automatic failover capabilities. By following the steps outlined above, you can configure a replica set with multiple nodes that ensures your MongoDB deployment is robust and fault-tolerant. Replica sets are a critical component of any production-level MongoDB deployment, especially for applications requiring high uptime and data consistency.
How Replication Works in MongoDB
Replication in MongoDB provides high availability and data redundancy by copying data from one server (the primary) to one or more servers (the secondaries). This mechanism ensures that the data is available even if one of the nodes fails. Replication is a key feature of MongoDB's architecture, enabling fault tolerance and automatic failover.
1. Basic Replication Workflow
The basic replication workflow in MongoDB involves the primary node and secondary nodes:
- Primary Node: The primary node is responsible for handling all write operations. It records all changes to its data in an operation log (Oplog) and then replicates the changes to the secondary nodes.
- Secondary Nodes: Secondary nodes replicate the data from the primary node by applying the operations from the Oplog. They maintain an identical copy of the data to ensure redundancy.
2. Oplog (Operation Log)
Each node in a replica set, including the primary and secondary nodes, has an Oplog (operation log). The Oplog is a capped collection that records all changes (inserts, updates, deletes) made to the data in the primary node.
When a write operation is performed on the primary node, it is immediately recorded in the Oplog. The secondary nodes continuously poll the Oplog of the primary node and replicate the changes to their own Oplogs.
Each secondary node keeps an internal pointer to the last operation it applied from the Oplog. This allows secondaries to catch up to the primary node in case they fall behind.
3. Replication Process Overview
The replication process follows these steps:
- Write Operation on Primary: A client performs a write operation (insert, update, or delete) on the primary node.
- Oplog Entry: The primary node records the write operation in its Oplog.
- Replication to Secondaries: The secondary nodes replicate the changes from the Oplog of the primary node. The replication process is asynchronous, meaning the secondaries do not block write operations on the primary.
- Applying Oplog Entries: The secondary nodes apply the operations from the Oplog to their local copies of the data.
- Consistency: Once the secondary node has applied all the operations from the Oplog, it has an identical copy of the primary node's data.
4. Automatic Failover and Election
In the event of a failure of the primary node, MongoDB automatically initiates an election process to determine a new primary. The election process works as follows:
- If the primary node becomes unavailable (due to network issues, hardware failure, etc.), the secondaries will detect the failure and trigger an election.
- The secondaries compare their data states and vote for the node that has the most up-to-date data to be promoted as the new primary.
- The new primary is selected, and the replica set continues to operate with minimal downtime.
5. Replication Lag
Replication in MongoDB is asynchronous, which means that there might be a delay between when a write operation is committed on the primary node and when it appears on the secondary nodes. This delay is known as replication lag.
Replication lag can vary depending on factors like network speed, hardware performance, and
the amount of data being written. MongoDB provides several tools to monitor replication lag,
such as the rs.status()
command and the oplog
size.
6. Types of Replication
MongoDB supports different types of replication modes:
- Master-Slave Replication (Deprecated): In this older model, there is one master node that handles writes, and one or more slave nodes that replicate the master’s data. This model is no longer recommended, as replica sets provide more flexibility and features.
- Replica Sets: Replica sets are the recommended replication model in MongoDB. A replica set consists of a primary node and one or more secondary nodes. All nodes in the replica set maintain the same data set, ensuring data availability and redundancy.
7. Configuring Replication
To configure replication in MongoDB, you need to:
- Ensure that each node has the same replica set name.
- Configure replication settings in the
mongod.conf
configuration file. - Start the MongoDB instances on each node and initiate the replica set using
rs.initiate()
in the MongoDB shell.
8. Best Practices for Replication
- Use Odd Number of Nodes: It is recommended to deploy an odd number of nodes in a replica set (e.g., 3, 5) to ensure that there is always a majority for elections and avoid split-brain scenarios.
- Deploy Across Multiple Availability Zones: To ensure high availability, deploy replica set members across different data centers or availability zones to minimize the risk of a single point of failure.
- Monitor Oplog Size: Regularly monitor the Oplog size to ensure that it is sufficient to handle the volume of write operations. If the Oplog is too small, secondaries may fall behind and fail to replicate all operations.
- Enable Read Preferences: Configure read preferences to distribute read traffic to secondary nodes and offload the primary node, improving performance.
9. Conclusion
Replication in MongoDB is a powerful feature that provides high availability, data redundancy, and automatic failover. By understanding how replication works and following best practices, you can ensure that your MongoDB deployment is resilient, fault-tolerant, and highly available, even in the event of node failures.
Failover Mechanisms in MongoDB
Failover in MongoDB ensures that in the event of a node failure, a new primary node is automatically elected to maintain availability and prevent downtime. This is critical for maintaining the high availability of the database in production environments. MongoDB uses replica sets for automatic failover and provides mechanisms to promote secondary nodes to primary when necessary.
1. Role of Replica Sets in Failover
Replica sets form the core of MongoDB's failover mechanism. A replica set consists of one primary node and multiple secondary nodes. These nodes work together to provide data redundancy and automatic failover capabilities:
- Primary Node: Handles all write operations. It is the only node that accepts write requests and replicates them to secondary nodes.
- Secondary Nodes: Replicate the data from the primary node and serve read operations based on the configured read preferences. In case of primary node failure, one of the secondary nodes is promoted to primary.
Replica sets enable MongoDB to automatically detect node failures and initiate an election process to select a new primary. This ensures that the application can continue to function without manual intervention.
2. Automatic Failover Process
The automatic failover process in MongoDB occurs as follows:
- Primary Node Failure: If the primary node fails (e.g., due to network issues, hardware failure, etc.), the replica set members detect the failure.
- Election Process: An election is triggered among the secondary nodes to select a new primary. This process ensures that one of the secondaries with the most up-to-date data is promoted to primary.
- New Primary Node: Once the election is complete, the new primary node is chosen. The former secondary node becomes the new primary, and it starts accepting write operations.
- Replication Continues: The secondary nodes continue to replicate data from the new primary to maintain data consistency across the replica set.
This failover process is seamless and ensures that the application continues to operate with minimal disruption.
3. Election Process in Detail
The election process involves the following steps:
- Detection of Failure: Each node in the replica set monitors the health of the primary node. If the primary node is not responding within a certain timeframe, the secondary nodes detect the failure.
- Voting: The secondary nodes participate in the election by casting votes. Each node in the replica set has a vote, and the node with the most votes becomes the new primary.
- Priority and Tie-breaking: The election process considers the priority of nodes in the replica set. Nodes with a higher priority are more likely to be elected as the new primary. In case of a tie, MongoDB uses the node's last operation timestamp to break the tie.
- Replication Resumption: Once the election is complete, the new primary node starts accepting writes, and replication resumes as usual.
The election mechanism ensures that MongoDB remains highly available by automatically selecting a new primary when needed.
4. Arbiter Nodes
In some scenarios, you might configure an arbiter node to help with the election process. An arbiter is a special type of node that does not hold any data but participates in elections to break ties in case of a vote split. An arbiter node ensures that there is always a majority in the replica set to decide the primary node.
Arbiters are useful when you need to maintain an odd number of voting members in the replica set, but do not want to consume additional resources by running a full-fledged replica set member.
5. Monitoring Failover and Replica Set Health
MongoDB provides tools and commands to monitor the health of the replica set and track the status of the failover process:
- rs.status(): This command provides detailed information about the replica set, including the state of each node and the current primary. You can use this command to check if the failover process has occurred and to identify which node is the current primary.
- rs.isMaster(): This command returns information about the current state of the replica set, including the primary node and whether the current node is the primary. This is useful to check which node is accepting writes.
- Logs: MongoDB logs events related to replica set elections and failovers. You can check the logs to understand when the failover occurred and which node became the new primary.
6. Impact of Failover on Clients
When a failover occurs, the client application may experience brief downtime while the replica set elects a new primary. However, MongoDB's drivers are designed to automatically reconnect to the new primary without requiring manual intervention. This ensures that the application can continue writing data and serving requests with minimal disruption.
To ensure a smooth failover experience, applications should:
- Use read preferences to allow reading from secondaries during the failover process, which helps minimize the impact on read operations.
- Implement automatic retries for write operations in case of temporary interruptions during failover.
- Be aware of write concerns and configure them to suit the application's consistency requirements during failover.
7. Handling Split-Brain Scenario
A split-brain scenario occurs when a network partition causes multiple nodes in the replica set to believe they are the primary node. This can lead to data inconsistencies and conflicts. MongoDB avoids split-brain situations by requiring a majority of nodes (quorum) to agree on the primary node during elections.
To prevent split-brain scenarios, MongoDB:
- Ensures that the majority of nodes in a replica set are always in communication with each other.
- Uses write concerns and read concerns to ensure that data is written and read from a consistent, majority-acknowledged node.
- Allows configuration of voting members to control the number of nodes that can participate in elections and avoid split-brain situations.
8. Best Practices for Failover
- Deploy Replica Set Members Across Multiple Data Centers: To ensure high availability and disaster recovery, deploy replica set members across different data centers or availability zones. This reduces the risk of a single point of failure.
- Use Odd Number of Nodes: Always deploy an odd number of nodes (e.g., 3, 5) in the replica set to ensure that elections can always occur with a clear majority.
- Monitor Replica Set Health: Regularly monitor the health of the replica set using MongoDB monitoring tools and commands. This helps detect issues before they lead to failover events.
- Configure Proper Write Concerns: Set appropriate write concerns to ensure that write operations are acknowledged by the majority of replica set members, reducing the likelihood of data inconsistency during failover.
9. Conclusion
Failover mechanisms in MongoDB, powered by replica sets and automatic elections, provide high availability and fault tolerance. By understanding how failover works and following best practices, you can ensure that your MongoDB deployment remains resilient and continues to operate smoothly, even during node failures.
Backup Strategies and Point-in-Time Recovery
Backup strategies and point-in-time recovery are critical components of any MongoDB deployment. Regular backups ensure that you can restore your data in the event of a disaster or accidental data loss. Point-in-time recovery (PITR) allows you to restore your database to a specific moment, ensuring minimal data loss in case of a failure. MongoDB provides several options and tools to implement backups and perform PITR.
1. Types of Backups in MongoDB
MongoDB offers different types of backups based on your requirements:
- Full Backups: A full backup captures the entire dataset, including all databases and collections. This is the most common type of backup and is ideal for disaster recovery scenarios.
- Incremental Backups: Incremental backups store only the data that has changed since the last backup. This reduces storage requirements and backup time, but it requires a base full backup to restore properly.
- Oplog Backups: MongoDB's replication model uses an oplog (operation log) to record all changes made to the database. By backing up the oplog, you can capture changes made after a full backup and perform point-in-time recovery.
2. Backup Methods in MongoDB
There are several methods to create backups in MongoDB:
- MongoDB Dumps (mongodump/mongorestore): The
mongodump
andmongorestore
utilities are the most basic tools for backing up and restoring MongoDB data. A dump generates BSON files of your collections, which can be used to restore the data usingmongorestore
. - Filesystem Snapshots: Filesystem snapshots capture the entire data
directory at a particular point in time. This method can be faster than using
mongodump
, but it requires that the MongoDB server is either stopped or that the data is flushed to disk to ensure consistency. - Cloud Backups (MongoDB Atlas): MongoDB Atlas, the managed cloud service, provides built-in backup capabilities. Atlas automatically creates backups and allows you to restore data to a specific point in time. This eliminates the need for manual backup management.
3. Point-in-Time Recovery (PITR)
Point-in-time recovery (PITR) allows you to restore MongoDB to the exact state it was in at a specific moment in time. PITR is crucial for recovering from events such as accidental data deletion or corruption. MongoDB uses the oplog (operation log) to achieve PITR.
Steps for Point-in-Time Recovery:
- Step 1: Perform a Full Backup: First, take a full backup of the
database using one of the available backup methods (e.g.,
mongodump
or filesystem snapshots). - Step 2: Capture Oplog Backups: After taking a full backup, you should periodically back up the oplog. This log records all operations performed on the database.
- Step 3: Restore Full Backup: When performing a PITR, start by restoring
the full backup using
mongorestore
or the appropriate recovery method based on the backup type. - Step 4: Apply Oplog Entries: Once the full backup is restored, apply the oplog entries from the backup to bring the data up to the desired point in time.
- Step 5: Verify Data Integrity: After the oplog is applied, verify that the data is in a consistent state and matches the expected point in time.
4. Backup Considerations
When implementing a backup strategy for MongoDB, you should take the following considerations into account:
- Backup Frequency: Define a backup schedule that meets your recovery point objectives (RPO). You may need to take frequent backups for highly critical data and less frequent backups for less important data.
- Backup Storage: Store backups securely and ensure that they are replicated or stored in a different geographic location to protect against physical damage or disasters. Cloud storage solutions can be used for scalability and reliability.
- Backup Testing: Regularly test your backup and recovery process to ensure that you can restore data quickly and without errors in the event of a disaster.
- Backup Retention: Define a backup retention policy to manage the number of backups you keep. Ensure that you maintain enough history for compliance and auditing purposes.
5. Backup Tools and Utilities
MongoDB provides a variety of tools for managing backups:
- mongodump/mongorestore: These command-line tools are used for full and incremental backups and restores. They support compression and filtering to optimize backup storage and performance.
- MongoDB Atlas Backup: If you are using MongoDB Atlas, the cloud-based MongoDB service provides automatic and on-demand backups. You can easily restore data using the Atlas UI or API.
- Cloud Provider Snapshots: If your MongoDB is hosted in a cloud environment, you can take advantage of cloud storage snapshots for faster backups and cost-effective storage management.
6. Restore Strategies
Restoring MongoDB from backups can be done in several ways, depending on the backup method you used:
- Restoring from mongodump: Use the
mongorestore
utility to restore the data from a dump generated bymongodump
. This method is suitable for smaller datasets or when you need to restore specific collections. - Restoring from Filesystem Snapshots: If you used filesystem snapshots for backup, you can restore the data by copying the snapshot back into the MongoDB data directory. Ensure the server is stopped before performing this operation.
- Restoring from Atlas Backup: If using MongoDB Atlas, you can restore data from backups directly from the Atlas dashboard. PITR is available for up to 30 days in Atlas and can be used to restore to a specific timestamp.
7. Best Practices for Backup and Recovery
- Automate Backups: Automate your backup process to ensure that backups are taken regularly and without human intervention.
- Monitor Backup Health: Use monitoring tools to track the status of your backups and ensure that they are completed successfully. Set up alerts for backup failures.
- Use Encryption: Ensure that your backups are encrypted both at rest and in transit to protect sensitive data.
- Test Backups Regularly: Periodically restore backups to test their integrity and ensure that the recovery process works smoothly in case of an actual disaster.
8. Conclusion
A robust backup strategy and point-in-time recovery process are essential for ensuring the durability and availability of your MongoDB data. By implementing regular backups, using oplog-based PITR, and following best practices for backup storage and testing, you can minimize the risk of data loss and ensure your MongoDB deployment remains resilient to failures.
Understanding Sharding in MongoDB
Sharding is a method used by MongoDB to distribute data across multiple servers, ensuring horizontal scalability and improving the system's ability to handle large datasets and high throughput. By distributing data into smaller, manageable chunks, sharding enables MongoDB to scale out across several machines, balancing the load and optimizing performance.
1. What is Sharding?
Sharding in MongoDB involves splitting a large dataset into smaller, more manageable chunks and distributing those chunks across multiple servers, called shards. Each shard holds a subset of the data, and the sharded cluster collectively holds the entire dataset. Sharding helps MongoDB scale horizontally, meaning it can handle more data and requests by adding more servers to the cluster.
2. Why Use Sharding?
- Horizontal Scalability: Sharding allows MongoDB to scale horizontally by adding more nodes (shards) to the cluster. This eliminates the limitations of vertical scaling (increasing the capacity of a single server) and enables the system to handle large data volumes.
- Improved Performance: With sharding, data is distributed across multiple servers, reducing the load on any single server and improving response times, especially for read-heavy workloads.
- High Availability: Sharded clusters can be configured with replica sets, ensuring data availability even in the case of server failures or network partitions.
3. Components of a Sharded Cluster
A MongoDB sharded cluster consists of the following components:- Shards: Each shard is a replica set that stores a subset of the data. Shards handle data storage and query processing. The data is partitioned into chunks, and each shard holds one or more chunks of the data.
- Config Servers: Config servers store the metadata for the sharded cluster. They keep track of the distribution of data and provide this information to the query router. A sharded cluster requires exactly three config servers for redundancy and high availability.
- Query Routers (mongos): Query routers, also known as mongos processes, act as the interface between the client applications and the sharded cluster. They route client queries to the appropriate shard based on the data distribution information stored in the config servers.
4. Sharding Key
The sharding key is the field or set of fields that MongoDB uses to distribute data across shards. The choice of sharding key is critical because it determines how the data will be partitioned and distributed. A well-chosen sharding key ensures that data is evenly distributed across all shards, preventing hotspots where one shard becomes overloaded.
Sharding Key Considerations:
- Even Distribution: Choose a sharding key that will evenly distribute data across the shards to avoid situations where one shard becomes overloaded with data and others have little data.
- Query Pattern: The sharding key should align with the most common queries to ensure that queries can be routed efficiently to the relevant shard without requiring a scan of all the shards.
- Cardinality: The sharding key should have enough distinct values to evenly distribute data across the shards. Avoid using fields with low cardinality (e.g., a boolean field) as a sharding key.
5. Shard Key Types
There are two common types of shard keys in MongoDB:- Single Field Shard Key: A single field is chosen as the shard key. This is the simplest form of sharding and works well for many use cases where the field has a large number of distinct values.
- Compound Shard Key: A compound shard key consists of multiple fields. This allows for more granular control over how data is distributed and can be used to optimize for specific query patterns.
6. Sharding Strategy
MongoDB provides several sharding strategies for distributing data:- Range-Based Sharding: In this strategy, data is distributed based on the value of the sharding key. Each shard holds data within a certain range of values. Range-based sharding is useful when queries often request data within specific ranges (e.g., dates or numeric ranges).
- Hash-Based Sharding: In hash-based sharding, the value of the sharding key is hashed, and the hash value is used to determine the shard to which the data should be sent. This provides a more even distribution of data but may not optimize for range queries.
- Zone-Based Sharding: Zone-based sharding allows for a more customized distribution of data. You can define specific ranges or zones for a set of shards, which is useful for workloads with specific geographic or business requirements.
7. Balancing and Chunk Migration
MongoDB automatically balances the data across shards by moving chunks of data between shards to maintain an even distribution. The balancing process occurs in the background to ensure that no shard becomes overloaded with data. MongoDB uses the chunk size to determine when data should be moved to maintain even distribution.Chunk Migration Process:
- The balancer identifies imbalanced shards based on the current chunk distribution.
- Chunks that are too large or unevenly distributed are selected for migration.
- The chunk is moved to another shard, and the metadata is updated on the config servers.
- The balancer continues to monitor shard distribution and triggers further migrations when necessary.
8. Shard Key Rebalancing
Over time, as your data grows and query patterns evolve, you may need to adjust your sharding strategy. MongoDB provides options for rebalancing the data across shards by modifying the shard key or adjusting the chunk distribution.9. Considerations for Sharding
- Complexity: Sharding introduces complexity in terms of deployment, maintenance, and monitoring. It is important to carefully plan your sharding strategy and shard key selection to avoid performance issues.
- Write-Heavy Workloads: Sharding is particularly beneficial for write-heavy workloads, as it allows MongoDB to distribute the write load across multiple shards.
- Data Access Patterns: Sharding works best when your data access patterns are well understood. Ensure that your sharding key aligns with your most frequent queries to achieve the best performance.
10. Sharded Cluster Deployment Example
# Example of configuring a sharded cluster
# Start config servers
mongod --configsvr --replSet configReplSet --port 27019 --dbpath /data/configdb
# Start shard servers
mongod --shardsvr --replSet shard1 --port 27018 --dbpath /data/shard1
# Start query routers
mongos --configdb configReplSet/localhost:27019 --port 27017
# Add shard to cluster
sh.addShard("shard1/localhost:27018")
11. Conclusion
Sharding is a powerful technique in MongoDB that allows for horizontal scaling and the ability to handle large datasets and high-throughput workloads. By dividing data into chunks and distributing it across multiple shards, MongoDB can ensure better performance, availability, and scalability. However, choosing the right shard key, understanding your data access patterns, and managing the complexity of a sharded cluster are key factors for successful implementation.
Configuring Sharded Clusters in MongoDB
Configuring a sharded cluster in MongoDB involves setting up the key components of the cluster: shards, config servers, and query routers (mongos). The configuration of these components ensures that MongoDB can distribute data across multiple servers for horizontal scalability and high availability. In this section, we will walk through the steps to configure a sharded cluster in MongoDB.
1. Prerequisites for Configuring Sharded Clusters
Before configuring a sharded cluster, ensure that the following prerequisites are met:- MongoDB Version: Ensure that you are using a version of MongoDB that supports sharding (usually MongoDB 3.4 or later).
- Multiple Machines: You will need multiple machines or virtual instances for the config servers, shards, and mongos routers. These can be separate physical machines or virtual machines in a cloud environment.
- Replica Sets: Each shard and config server should be configured as a replica set to ensure high availability.
2. Components of a Sharded Cluster
A MongoDB sharded cluster consists of three main components:- Shards: Each shard stores a subset of the data and is typically a replica set.
- Config Servers: Config servers store the metadata for the cluster, including the distribution of data across shards.
- Query Routers (mongos): mongos processes act as the interface between client applications and the sharded cluster. They route queries to the appropriate shard based on the shard key.
3. Setting Up Config Servers
Config servers store the configuration and metadata for the sharded cluster. MongoDB requires at least three config servers for a sharded cluster to provide redundancy and high availability. These config servers should be configured as replica sets.Steps to Set Up Config Servers:
- Start the MongoDB instances for config servers on different machines (or ports) with the `--configsvr` option.
- Initialize a replica set for the config servers using the `--replSet` option.
- Use the `rs.initiate()` command to initiate the replica set on the config servers.
mongod --configsvr --replSet configReplSet --port 27019 --dbpath /data/configdb
4. Setting Up Shards
Each shard is a replica set that stores a subset of the data. MongoDB requires at least one shard, but in practice, you typically have multiple shards for a large deployment.Steps to Set Up Shards:
- Start each MongoDB instance for a shard with the `--shardsvr` option.
- Initialize a replica set for each shard using the `--replSet` option.
- Use the `rs.initiate()` command to initiate the replica set for each shard.
mongod --shardsvr --replSet shard1 --port 27018 --dbpath /data/shard1
5. Setting Up Query Routers (mongos)
Query routers (mongos) are the entry point for client applications. They route the queries to the appropriate shard based on the shard key. A sharded cluster can have multiple mongos instances to handle large volumes of traffic.Steps to Set Up Query Routers:
- Start the mongos process on a machine that will serve as the query router.
- Specify the config server replica set as part of the mongos configuration using the `--configdb` option.
mongos --configdb configReplSet/localhost:27019 --port 27017
6. Adding Shards to the Cluster
Once your config servers and mongos routers are running, you can add shards to the sharded cluster. MongoDB allows you to add shards dynamically as your data grows.Steps to Add Shards:
- Use the `sh.addShard()` command to add a shard to the cluster.
- Repeat the process for each additional shard you want to add to the cluster.
sh.addShard("shard1/localhost:27018")
7. Enabling Sharding for a Database
After setting up the sharded cluster, you need to enable sharding for specific databases. MongoDB allows you to shard individual databases to distribute their data across the shards.Steps to Enable Sharding for a Database:
- Use the `sh.enableSharding()` command to enable sharding for a database.
sh.enableSharding("myDatabase")
8. Sharding a Collection
After enabling sharding for a database, you need to choose a shard key for the collections you want to shard. The shard key determines how data is distributed across the shards.Steps to Shard a Collection:
- Use the `sh.shardCollection()` command to shard a collection, specifying the collection name and the shard key.
sh.shardCollection("myDatabase.myCollection", { "shardKeyField": 1 })
9. Verifying the Sharded Cluster
After configuring the sharded cluster, verify that it is working as expected. Use the following commands to check the status of the cluster:- Check Shard Status: Use the `sh.status()` command to display the status of the sharded cluster, including the shards, databases, and collections.
- Check Shard Balancing: Use the `sh.isBalancerRunning()` command to check if the balancer is running and ensuring data is evenly distributed across the shards.
10. Conclusion
Configuring a sharded cluster in MongoDB requires careful planning and setup of the various components, including shards, config servers, and query routers. Once configured, the sharded cluster enables MongoDB to scale horizontally, improving performance and supporting large datasets and high-throughput workloads. Properly configuring and managing sharded clusters ensures that MongoDB can meet the demands of your applications as they grow.Understanding Sharding in MongoDB
Sharding is a method used by MongoDB to distribute data across multiple servers, ensuring horizontal scalability and improving the system's ability to handle large datasets and high throughput. By distributing data into smaller, manageable chunks, sharding enables MongoDB to scale out across several machines, balancing the load and optimizing performance.
1. What is Sharding?
Sharding in MongoDB involves splitting a large dataset into smaller, more manageable chunks and distributing those chunks across multiple servers, called shards. Each shard holds a subset of the data, and the sharded cluster collectively holds the entire dataset. Sharding helps MongoDB scale horizontally, meaning it can handle more data and requests by adding more servers to the cluster.
2. Why Use Sharding?
- Horizontal Scalability: Sharding allows MongoDB to scale horizontally by adding more nodes (shards) to the cluster. This eliminates the limitations of vertical scaling (increasing the capacity of a single server) and enables the system to handle large data volumes.
- Improved Performance: With sharding, data is distributed across multiple servers, reducing the load on any single server and improving response times, especially for read-heavy workloads.
- High Availability: Sharded clusters can be configured with replica sets, ensuring data availability even in the case of server failures or network partitions.
3. Components of a Sharded Cluster
A MongoDB sharded cluster consists of the following components:- Shards: Each shard is a replica set that stores a subset of the data. Shards handle data storage and query processing. The data is partitioned into chunks, and each shard holds one or more chunks of the data.
- Config Servers: Config servers store the metadata for the sharded cluster. They keep track of the distribution of data and provide this information to the query router. A sharded cluster requires exactly three config servers for redundancy and high availability.
- Query Routers (mongos): Query routers, also known as mongos processes, act as the interface between the client applications and the sharded cluster. They route client queries to the appropriate shard based on the data distribution information stored in the config servers.
4. Sharding Key
The sharding key is the field or set of fields that MongoDB uses to distribute data across shards. The choice of sharding key is critical because it determines how the data will be partitioned and distributed. A well-chosen sharding key ensures that data is evenly distributed across all shards, preventing hotspots where one shard becomes overloaded.
Sharding Key Considerations:
- Even Distribution: Choose a sharding key that will evenly distribute data across the shards to avoid situations where one shard becomes overloaded with data and others have little data.
- Query Pattern: The sharding key should align with the most common queries to ensure that queries can be routed efficiently to the relevant shard without requiring a scan of all the shards.
- Cardinality: The sharding key should have enough distinct values to evenly distribute data across the shards. Avoid using fields with low cardinality (e.g., a boolean field) as a sharding key.
5. Shard Key Types
There are two common types of shard keys in MongoDB:- Single Field Shard Key: A single field is chosen as the shard key. This is the simplest form of sharding and works well for many use cases where the field has a large number of distinct values.
- Compound Shard Key: A compound shard key consists of multiple fields. This allows for more granular control over how data is distributed and can be used to optimize for specific query patterns.
6. Sharding Strategy
MongoDB provides several sharding strategies for distributing data:- Range-Based Sharding: In this strategy, data is distributed based on the value of the sharding key. Each shard holds data within a certain range of values. Range-based sharding is useful when queries often request data within specific ranges (e.g., dates or numeric ranges).
- Hash-Based Sharding: In hash-based sharding, the value of the sharding key is hashed, and the hash value is used to determine the shard to which the data should be sent. This provides a more even distribution of data but may not optimize for range queries.
- Zone-Based Sharding: Zone-based sharding allows for a more customized distribution of data. You can define specific ranges or zones for a set of shards, which is useful for workloads with specific geographic or business requirements.
7. Balancing and Chunk Migration
MongoDB automatically balances the data across shards by moving chunks of data between shards to maintain an even distribution. The balancing process occurs in the background to ensure that no shard becomes overloaded with data. MongoDB uses the chunk size to determine when data should be moved to maintain even distribution.Chunk Migration Process:
- The balancer identifies imbalanced shards based on the current chunk distribution.
- Chunks that are too large or unevenly distributed are selected for migration.
- The chunk is moved to another shard, and the metadata is updated on the config servers.
- The balancer continues to monitor shard distribution and triggers further migrations when necessary.
8. Shard Key Rebalancing
Over time, as your data grows and query patterns evolve, you may need to adjust your sharding strategy. MongoDB provides options for rebalancing the data across shards by modifying the shard key or adjusting the chunk distribution.9. Considerations for Sharding
- Complexity: Sharding introduces complexity in terms of deployment, maintenance, and monitoring. It is important to carefully plan your sharding strategy and shard key selection to avoid performance issues.
- Write-Heavy Workloads: Sharding is particularly beneficial for write-heavy workloads, as it allows MongoDB to distribute the write load across multiple shards.
- Data Access Patterns: Sharding works best when your data access patterns are well understood. Ensure that your sharding key aligns with your most frequent queries to achieve the best performance.
10. Sharded Cluster Deployment Example
# Example of configuring a sharded cluster
# Start config servers
mongod --configsvr --replSet configReplSet --port 27019 --dbpath /data/configdb
# Start shard servers
mongod --shardsvr --replSet shard1 --port 27018 --dbpath /data/shard1
# Start query routers
mongos --configdb configReplSet/localhost:27019 --port 27017
# Add shard to cluster
sh.addShard("shard1/localhost:27018")
11. Conclusion
Sharding is a powerful technique in MongoDB that allows for horizontal scaling and the ability to handle large datasets and high-throughput workloads. By dividing data into chunks and distributing it across multiple shards, MongoDB can ensure better performance, availability, and scalability. However, choosing the right shard key, understanding your data access patterns, and managing the complexity of a sharded cluster are key factors for successful implementation.
Shard Keys and Choosing the Right Shard Key
In MongoDB, sharding is the process of distributing data across multiple machines to support horizontal scaling. The shard key is a critical part of this process, as it determines how data is distributed across the shards. Selecting the right shard key is essential to ensure the performance and efficiency of a sharded cluster.
1. What Is a Shard Key?
A shard key is a field or set of fields in a MongoDB collection that is used to partition the data into chunks. MongoDB uses the shard key to determine which shard will store a particular document. The shard key must be chosen carefully because it will directly impact the performance of data distribution and query routing.
2. Importance of Choosing the Right Shard Key
Choosing the right shard key is critical for maintaining the efficiency and performance of your sharded cluster. An inappropriate shard key can lead to several issues, including:
- Uneven Data Distribution: A poorly chosen shard key can lead to uneven distribution of data across the shards, causing some shards to become overloaded while others remain underutilized.
- Poor Query Performance: If queries often target a single shard, the load on that shard may increase, leading to slower query times and reduced overall performance.
- Chunk Migration Overhead: If the shard key is not chosen to ensure balanced data, MongoDB may need to move chunks between shards, which can cause performance degradation.
3. Characteristics of a Good Shard Key
A good shard key should have the following characteristics:- High Cardinality: The shard key should have a wide range of possible values. This ensures that the data is evenly distributed across the shards. For example, using a Boolean field with only two possible values (true/false) would result in uneven distribution of data.
- Even Distribution of Data: The shard key should help distribute data evenly across the shards, avoiding "hot spots" where one shard holds a disproportionate amount of data.
- Frequent Usage in Queries: The shard key should be a field that is frequently used in queries, as this will help optimize query routing. Queries that target the shard key can be routed directly to the correct shard, improving performance.
- Immune to Hot Spots: The shard key should avoid causing frequent updates to a small set of documents, which could create hot spots in the system. This could degrade performance significantly.
- Low Update/Write Skew: The shard key should not cause a disproportionate number of writes or updates to a single shard. Such skew can lead to bottlenecks in one shard while others remain idle.
4. Types of Shard Keys
MongoDB supports different types of shard keys based on the chosen strategy for distributing data. The main options include:- Single Field Shard Key: This is the most common type of shard key. It involves selecting a single field in the document to act as the shard key. Examples include using a user ID, timestamp, or geographic location.
- Compound Shard Key: A compound shard key is composed of multiple fields. This approach is useful when a single field does not provide sufficient cardinality or distribution, but a combination of fields can. For example, combining "region" and "timestamp" can provide better data distribution than either field alone.
- Hashed Shard Key: A hashed shard key uses a hash of the shard key value to distribute documents evenly across shards. This is useful when the shard key has low cardinality or is not naturally evenly distributed. Hashed sharding ensures an even distribution of documents, but it does not allow for efficient range queries.
- Range Shard Key: Range sharding allows MongoDB to partition data into ranges based on the shard key values. This can be useful for scenarios where you expect to perform range-based queries on the shard key (e.g., finding all records within a date range).
5. Common Patterns for Choosing a Shard Key
The choice of a shard key often depends on the data structure and query patterns of your application. Here are some common patterns to consider when choosing a shard key:- Time-based Sharding: If your application stores time-series data (e.g., logs, events), a timestamp field is often a good choice for a shard key. However, it’s important to ensure that your timestamp values are distributed over time to avoid hot spots.
- Geospatial Sharding: If your application deals with geographic data (e.g., locations of users or devices), a location-based shard key, such as latitude and longitude, might be appropriate. MongoDB supports geospatial indexing, which can optimize queries based on location.
- User-based Sharding: If your application involves user data, a user ID field is often a good shard key. This allows you to route all the data for a given user to a specific shard, improving query performance for user-specific queries.
- Content-based Sharding: If your application involves media or content (e.g., videos, articles), consider using metadata like content type or category as the shard key to partition data logically.
6. Example of Choosing a Shard Key
Let’s assume we have a collection of user activity logs in an e-commerce application, where each document contains a user ID, timestamp, and activity type. To choose the best shard key, we consider the following:- We frequently query logs by user ID (e.g., viewing the history of a particular user).
- We also query logs by time range (e.g., fetching logs from a specific day).
- If we use only the "timestamp" as the shard key, we could end up with hot spots where data for the same time range is concentrated on a few shards.
- Using a compound shard key of "user ID" and "timestamp" provides a good distribution of data and allows efficient queries by both user and time range.
sh.shardCollection("ecommerce.userActivityLogs", { "userID": 1, "timestamp": 1 })
7. Conclusion
Choosing the right shard key is a critical step in designing a sharded MongoDB cluster. A well-chosen shard key can improve data distribution, query performance, and scalability, while a poorly chosen one can lead to performance bottlenecks and imbalanced clusters. By carefully considering your data patterns, query behavior, and cardinality of the potential shard keys, you can ensure that your sharded cluster operates efficiently and scales effectively.Balancing Shards
In MongoDB, sharding is used to distribute data across multiple servers or clusters, ensuring horizontal scaling and high availability. However, as the data grows, it's crucial to ensure that the data is evenly distributed across the shards to avoid performance bottlenecks or uneven workloads. This process is known as balancing shards.
1. What Is Shard Balancing?
Shard balancing in MongoDB refers to the mechanism that ensures that the data is distributed evenly across all the shards in a sharded cluster. MongoDB automatically manages this process, moving chunks of data between shards to ensure that no single shard is overloaded while others remain underutilized. The balancing process is done automatically by the MongoDB balancer, which runs in the background to maintain an even distribution of data.
2. How Shard Balancing Works
The balancing process works by moving chunks of data between shards based on a number of factors, such as:
- Chunk Size: MongoDB splits collections into chunks, each containing a range of shard key values. Once a chunk exceeds a certain size (typically 64MB), the balancer will attempt to move it to another shard to balance the load.
- Shard Distribution: The balancer ensures that the chunks are evenly distributed across the shards. If a shard holds more data than others, chunks will be moved from it to underutilized shards.
- Write Operations: If a shard becomes overloaded with write operations, the balancer will attempt to redistribute the chunks to maintain performance and avoid a single shard bottleneck.
3. When Does MongoDB Trigger Balancing?
MongoDB triggers the balancing process in the following scenarios:- When Chunks Become Too Large: As mentioned earlier, chunks are moved when their size exceeds the 64MB threshold. MongoDB will attempt to move chunks to balance the data distribution across the shards.
- When New Data Is Added: If new data is added to the collection and results in an uneven distribution, MongoDB will trigger the balancer to move chunks and ensure the data is balanced.
- When Shards Are Added or Removed: If a new shard is added to the cluster or an existing shard is removed, MongoDB will automatically initiate the balancing process to redistribute the data evenly across the remaining shards.
- When a Shard is Overloaded: If one shard is experiencing high load or excessive writes, the balancer will move chunks to other shards to ensure that the load is distributed evenly.
4. Balancer Process in Detail
The balancer operates in several stages:- Chunk Splitting: When a chunk exceeds the maximum size limit (64MB), MongoDB will split the chunk into two smaller ones. This process helps maintain manageable chunk sizes and supports the balancing process.
- Chunk Migration: MongoDB uses chunk migration to move chunks between shards. The balancer selects chunks based on shard key ranges and moves them to the appropriate shard to maintain data distribution.
- Targeted Shard Selection: The balancer selects which shard to move the chunk to based on the shard's data distribution and current load. The goal is to avoid overloading any particular shard while ensuring the data is evenly distributed.
5. Monitoring the Balancer
MongoDB provides several tools and methods to monitor the status of the balancer and the chunk migration process:- balancerStatus: You can use the
balancerStatus
command to check the current status of the balancer, including whether it is running and whether any chunks are being moved. - sh.status(): The
sh.status()
command provides information about the sharded cluster, including the number of shards, chunks, and data distribution. This can help identify any imbalances in the data distribution. - Logs: MongoDB logs contain detailed information about the balancer's actions, including chunk migrations and status changes. Reviewing the logs can help identify issues or bottlenecks in the balancing process.
6. Controlling the Balancer
MongoDB allows you to control the balancer's behavior by enabling or disabling it temporarily and adjusting its settings:- Enabling/Disabling the Balancer: The balancer can be enabled or disabled using the
sh.stopBalancer()
andsh.startBalancer()
commands, respectively. This is useful for maintenance tasks or when you want to prevent balancing during periods of high load. - Balancing Window: MongoDB allows you to configure a balancing window, which is a specific time range when the balancer can move chunks. This can help prevent the balancer from interfering with normal operations during peak usage times.
- Throttling Balancing Operations: You can control the rate at which the balancer moves chunks by adjusting the
balancer.chunkMigrationThrottle
setting. This can help prevent the balancer from consuming too many resources.
7. Troubleshooting Balancing Issues
If you encounter issues with shard balancing, consider the following troubleshooting steps:- Check Data Distribution: Use the
sh.status()
command to verify the data distribution across shards. If the data is not evenly distributed, MongoDB may need to move chunks to balance the load. - Monitor Chunk Size: Ensure that chunks are not exceeding the maximum size of 64MB. If chunks are too large, they will be split, and balancing will be triggered.
- Ensure Sufficient Resources: Make sure your system has sufficient resources (e.g., disk space, network bandwidth) to handle chunk migrations. Insufficient resources can cause the balancing process to fail or become slow.
- Check Balancer Logs: Review the balancer logs for errors or warnings. MongoDB logs can provide valuable insights into the status and performance of the balancer.
8. Conclusion
Balancing shards in MongoDB is an essential process to ensure the even distribution of data across a sharded cluster. By using MongoDB's automatic balancing features and actively monitoring and managing the balancer, you can ensure that your sharded cluster operates efficiently and scales effectively. Proper shard balancing minimizes performance bottlenecks and ensures high availability, making it a critical component of maintaining a healthy sharded MongoDB deployment.Monitoring MongoDB Performance
Monitoring performance is a crucial part of ensuring that your MongoDB deployment operates efficiently. By tracking key performance metrics, you can identify potential issues early and optimize your MongoDB setup to ensure high availability, low latency, and optimal resource usage.
1. Key Performance Metrics to Monitor
To effectively monitor MongoDB performance, it’s important to focus on several key metrics that reflect the health and performance of your database:- CPU Usage: High CPU utilization can indicate that MongoDB is under heavy load, which may affect query performance. Monitoring CPU usage helps ensure that your server has sufficient processing power.
- Memory Usage: MongoDB relies heavily on RAM for storing frequently accessed data. Monitoring memory usage helps detect memory bottlenecks or inefficient use of resources. Keep an eye on the WiredTiger cache and operating system memory usage.
- Disk I/O: MongoDB reads and writes data to disk, so monitoring disk I/O is essential. High disk I/O can lead to slow query performance and should be addressed by optimizing queries or adding more storage.
- Network Utilization: High network traffic can indicate inefficient queries, replication issues, or a high number of clients accessing the database. Monitoring network usage ensures that MongoDB is not overwhelmed by network requests.
- Replication Lag: In a replica set, replication lag indicates the delay between the primary and secondary nodes. A high replication lag can affect read consistency and application performance.
- Query Performance: Monitoring query performance is essential to ensure that queries are executing efficiently. Slow queries can be optimized by creating indexes, adjusting query structure, or analyzing database schema.
2. Monitoring Tools for MongoDB
Several tools are available to help monitor and analyze MongoDB performance:- MongoDB Atlas: MongoDB Atlas is a fully managed cloud database that provides comprehensive monitoring tools and dashboards. It offers built-in performance metrics, such as latency, throughput, and disk usage, in real-time. Atlas also includes automated alerts and performance optimization recommendations.
- MongoDB Ops Manager: Ops Manager is a monitoring solution for on-premises and hybrid MongoDB deployments. It provides detailed performance metrics, backups, and automated maintenance. Ops Manager can track database health, query performance, replication, and storage usage.
- mongostat: The
mongostat
command-line tool provides a real-time view of MongoDB’s performance, including metrics such as operations per second, memory usage, and network activity. This tool is useful for monitoring live performance in a MongoDB instance. - mongotop: The
mongotop
tool shows the read and write activity for each collection in a MongoDB instance. It helps identify which collections are most active and can be used to spot potential performance bottlenecks. - Profiler: MongoDB’s built-in query profiler allows you to log slow-running queries and analyze their performance. You can enable profiling with different levels of granularity (e.g., logging slow queries or all queries) to gain insights into query performance.
3. Using the MongoDB Atlas Dashboard
If you’re using MongoDB Atlas, the dashboard provides several key metrics that can help you monitor your cluster’s performance:- Cluster Overview: The dashboard provides an overview of your cluster’s health, including CPU, memory, and disk usage, along with any active connections and replication status.
- Real-Time Monitoring: Atlas offers real-time performance monitoring of various metrics, such as operations per second, network traffic, and latency. You can view these metrics for individual nodes or the entire cluster.
- Slow Query Tracking: The Atlas performance panel shows slow-running queries, allowing you to identify performance issues and optimize them by adding indexes or refactoring the queries.
- Alerts: Atlas allows you to set up custom alerts for various performance thresholds, such as CPU usage or disk space. Alerts can be sent via email, Slack, or other notification systems.
4. Performance Tuning Tips
Monitoring performance is only half of the solution. Optimizing MongoDB requires continuous effort. Here are some tips to help you optimize MongoDB performance:- Indexing: Ensure that your most frequently queried fields are indexed. Indexes drastically improve query performance by reducing the amount of data MongoDB needs to scan.
- Optimize Queries: Use the
explain()
method to analyze your queries and identify performance bottlenecks. Refactor slow or inefficient queries to reduce resource consumption. - Sharding: For large datasets, consider implementing sharding to distribute data across multiple servers. Sharding helps prevent performance degradation by balancing the load and improving scalability.
- Use WiredTiger: MongoDB’s default storage engine, WiredTiger, provides better performance than the previous MMAPv1 engine. It supports document-level locking and compression, which can improve performance in both read-heavy and write-heavy environments.
- Data Modeling: Design your MongoDB schema to ensure efficient data access patterns. Embedding documents can be faster for certain use cases than using references, as it minimizes the need for joins or lookups.
- Limit Lock Contention: MongoDB uses locks to ensure consistency. To avoid lock contention, ensure that long-running or write-heavy operations are minimized, and scale out with replica sets and sharding where necessary.
5. Troubleshooting Performance Issues
When you experience performance problems, it’s important to follow a systematic troubleshooting approach:- Check System Resources: Start by checking system resources (CPU, memory, disk) to ensure that your hardware is not underpowered. If resources are exhausted, consider upgrading your server or optimizing your MongoDB configuration.
- Analyze Slow Queries: Use the profiler and
explain()
to identify slow queries and optimize them by adding indexes or rewriting them to be more efficient. - Check for Locking Issues: If MongoDB is experiencing locking issues, consider reducing the frequency of long-running operations or using sharding to distribute the load.
- Monitor Replication Lag: In replica sets, monitor replication lag to ensure that secondary nodes are catching up with the primary. High replication lag can impact read consistency and cause performance degradation.
6. Conclusion
Monitoring MongoDB performance is essential to ensure that your database operates efficiently and scales effectively. By tracking key metrics, using the right monitoring tools, and optimizing your database based on performance insights, you can maintain a high-performance MongoDB environment. Regular monitoring and proactive optimization will help you prevent bottlenecks, minimize downtime, and ensure that your MongoDB deployment continues to meet the demands of your application.Query Optimization Strategies
Query optimization is a crucial part of MongoDB performance tuning. Efficiently written queries ensure faster response times, reduce resource consumption, and improve the overall performance of your MongoDB deployment. By following the right strategies, you can optimize your queries to handle large datasets and complex workloads more effectively.
1. Use Indexes Efficiently
Indexes are one of the most important tools for optimizing queries in MongoDB. Without indexes, MongoDB has to perform a collection scan, which can be slow for large datasets.- Choose the Right Index: Ensure you’re indexing fields that are frequently queried, used in sorting, or involved in joining operations. For example, index fields used in the
$match
or$sort
stages of an aggregation pipeline. - Compound Indexes: If you often query multiple fields together, create compound indexes. Compound indexes allow MongoDB to use multiple fields to optimize query performance.
- Use Covered Queries: Covered queries allow MongoDB to retrieve the data directly from the index, avoiding a collection scan. Make sure the query uses only the fields that are part of the index.
- Indexing Arrays: MongoDB can automatically create multi-key indexes for arrays, but be mindful of the size of array values, as it could impact performance.
2. Optimize Query Structure
The structure of your query can impact its performance. Writing optimized queries can help MongoDB execute them faster.- Limit the Fields: Use projections to return only the necessary fields in your query result. Retrieving unnecessary fields wastes memory and CPU resources.
- Use $in for Range Queries: If you’re querying a range of values, use the
$in
operator instead of multiple$or
clauses. This can reduce the number of documents MongoDB has to scan. - Use $exists for Missing Values: Use the
$exists
operator to filter documents where a field is missing or null. This can be more efficient than querying for null values directly. - Use $regex with Caution: Regular expressions in queries can be costly. If possible, avoid using them in performance-critical queries, especially without anchoring the regular expression to the beginning of the string.
3. Optimize Aggregation Pipelines
Aggregation pipelines offer powerful data transformation capabilities, but they also need to be optimized to ensure fast execution.- Use $match Early: Place the
$match
stage as early as possible in your aggregation pipeline to filter out unnecessary documents before processing them further. - Use $project to Limit Fields: Use the
$project
stage to remove unnecessary fields early in the pipeline. This reduces the amount of data that MongoDB needs to process in subsequent stages. - Avoid $unwind on Large Arrays: The
$unwind
stage can be expensive, especially when dealing with large arrays. Consider using alternatives such as$arrayElemAt
or restructuring the data to minimize the need for unwinding. - Avoid $lookup with Large Collections: The
$lookup
stage in aggregation performs a left join, which can be very resource-intensive. When working with large collections, ensure that both collections being joined are indexed appropriately.
4. Use Query Profiling
MongoDB’s query profiler helps you identify slow-running queries that may need optimization.- Enable Query Profiling: Enable query profiling to log slow queries. You can set the profiler level to log slow queries or all queries, depending on your needs. This will allow you to identify and optimize inefficient queries.
- Analyze Slow Queries: Use the
explain()
method to analyze the execution plan of slow queries. The execution plan will show how MongoDB is processing the query and where potential bottlenecks might exist. - Track Query Execution Time: Regularly monitor query execution time using monitoring tools like MongoDB Atlas. This helps you spot performance degradation and identify areas for improvement.
5. Sharding and Data Distribution
For large datasets, sharding can be an effective strategy to distribute the data across multiple servers, improving query performance by balancing the load.- Choose the Right Shard Key: The choice of shard key is critical. An inefficient shard key can result in data imbalances and slow queries. The shard key should distribute data evenly across the shards to avoid hotspots.
- Monitor Shard Distribution: Regularly monitor how data is distributed across shards. If data is not evenly distributed, consider changing the shard key or reorganizing the data.
- Leverage Zone Sharding: Zone sharding allows you to define specific ranges of data to reside on certain shards. This can be helpful for optimizing queries that are frequently run against a specific range of data.
6. Use the Right Hardware and Configuration
Optimizing the hardware and configuration of your MongoDB deployment can lead to significant performance improvements.- Upgrade Hardware: Ensure that your MongoDB server has enough RAM, CPU, and disk space to handle the expected workload. Inadequate hardware resources can result in slower query performance.
- Optimize Disk I/O: Use fast SSD drives to store your MongoDB data files. Disk I/O can be a significant bottleneck in performance, so upgrading to SSDs can drastically improve query times.
- Configure WiredTiger Storage Engine: MongoDB’s default storage engine, WiredTiger, offers better performance in terms of concurrency and compression. Ensure that the WiredTiger storage engine is optimized for your workload.
7. Conclusion
Query optimization in MongoDB is an ongoing process that involves selecting the right indexes, structuring your queries efficiently, optimizing aggregation pipelines, and ensuring that your database configuration and hardware are up to the task. By following the strategies outlined above and regularly monitoring query performance, you can significantly improve the speed and scalability of your MongoDB deployment.Cache Management in MongoDB
Cache management is an important strategy for improving the performance of MongoDB queries, particularly when working with frequently accessed data. By caching query results or frequently used documents, MongoDB can reduce the load on the database and speed up data retrieval times. Effective cache management in MongoDB can minimize disk I/O, reduce query latency, and improve the overall performance of your application.
1. Understanding MongoDB's Built-in Caching
MongoDB automatically uses an internal cache, which is managed by the WiredTiger storage engine. This cache stores frequently accessed data in memory to improve query performance. However, as the dataset grows, the cache may not be large enough to store all frequently accessed data, making manual cache management necessary.- WiredTiger Cache: The WiredTiger storage engine uses a memory cache to store frequently accessed data. This cache is automatically managed by MongoDB, and its size can be adjusted based on the available system memory.
- Cache Size Limit: The default cache size for the WiredTiger engine is 50% of system memory, but this can be adjusted by setting the
storage.wiredTiger.engineConfig.cacheSizeGB
parameter in the MongoDB configuration file. - In-memory Storage: For workloads that require fast access to data, consider using an in-memory storage engine. This engine keeps all data in memory, eliminating the need for disk I/O and dramatically improving performance for read-heavy applications.
2. Manual Cache Management Strategies
While MongoDB’s built-in cache is effective, there are several manual cache management strategies you can use to improve performance further:- Application-Level Caching: Implement caching in your application layer using tools like Redis or Memcached. These caching systems store frequently queried data in memory and reduce the load on MongoDB by serving cached results for repeated queries.
- Cache Hot Documents: If there are documents that are frequently accessed, you can cache them in memory either at the application level or using in-memory databases like Redis. This minimizes the need to query MongoDB for popular data.
- Query Result Caching: For frequently executed queries, you can cache the result set in memory and reuse it for subsequent requests. Be mindful of cache expiration strategies to ensure that the cache does not serve outdated data.
3. Cache Invalidation and Expiry
Effective cache management also involves ensuring that cached data remains fresh and accurate. Cache invalidation and expiry are crucial to preventing stale data from being served to users.- Time-based Expiry: Set a time-to-live (TTL) on cached data to automatically expire and refresh the cache after a certain period. This ensures that the cache does not hold outdated data for too long.
- Event-based Invalidation: Use events in your application to trigger cache invalidation when underlying data changes. For example, when a document is updated in MongoDB, invalidate the cache for that document to ensure the next query fetches the latest data.
- Cache Preloading: In some cases, you may want to preload frequently accessed data into the cache during application startup. This can help reduce latency for the first request to access certain data.
4. Using MongoDB’s TTL Indexes
MongoDB provides Time-To-Live (TTL) indexes to automatically manage the expiration of documents in a collection. TTL indexes are ideal for caching scenarios where you want documents to be deleted after a certain period, reducing the need for manual cache management.- TTL Index Setup: To create a TTL index, you must define an index on a date or timestamp field, and MongoDB will automatically remove documents after the specified time has passed.
- TTL Index Example: Here’s an example of creating a TTL index that expires documents after 3600 seconds (1 hour):
db.cacheCollection.createIndex({ "createdAt": 1 }, { expireAfterSeconds: 3600 });
- Use Cases: TTL indexes are useful for caching session data, temporary files, or user activity logs where the data should only be kept for a limited time.
5. Memory Considerations
Managing memory efficiently is essential for cache performance. MongoDB’s in-memory cache can be limited by the available system memory, and when the cache size exceeds the available memory, MongoDB may experience performance degradation.- Monitor Memory Usage: Regularly monitor the memory usage of your MongoDB instance to ensure that the cache is not consuming excessive resources. Tools like
mongostat
and MongoDB Atlas monitoring can help you track memory consumption in real-time. - Optimize Data Size: Store only the most critical and frequently accessed data in the cache. Avoid caching large documents or unnecessary data that doesn’t significantly benefit from being cached.
- Cache Warmup: When MongoDB restarts, the cache is cleared, which can lead to a cold start with slower query performance. Implement a cache warmup strategy to populate the cache with frequently accessed data after a restart.
6. Hybrid Cache Solutions
In complex applications, combining MongoDB’s built-in cache with external caching systems can provide the best of both worlds.- MongoDB + Redis: Use Redis as an in-memory cache to handle hot data and MongoDB for persistent storage. Redis can significantly speed up read-heavy workloads by serving data from memory, while MongoDB provides durable storage for the data.
- Cache-aside Pattern: In a cache-aside pattern, your application fetches data from the cache first. If the data is not in the cache, it retrieves it from MongoDB and stores it in the cache for future use.
- Write-through Caching: In this approach, whenever data is written to MongoDB, it’s also written to the cache immediately, ensuring that the cache is always up-to-date.
7. Conclusion
Effective cache management in MongoDB can significantly improve query performance, reduce response times, and decrease the load on the database. By leveraging MongoDB’s built-in caching, implementing application-level caching with tools like Redis, and using TTL indexes, you can ensure that your application remains fast and scalable. Proper cache invalidation and memory management strategies are crucial to ensure that the cache serves fresh and accurate data while maintaining optimal performance.Profiling Slow Queries in MongoDB
Profiling slow queries in MongoDB is essential to identifying performance bottlenecks and optimizing query efficiency. Slow queries can significantly impact the overall performance of your application, especially as the dataset grows. MongoDB provides tools to help analyze and profile slow-running queries, enabling you to fine-tune your database operations and improve response times.
1. Enabling the Profiler
MongoDB’s database profiler allows you to capture data on slow queries and operations. The profiler can log information about queries, including execution times, read/write operations, and any other performance-related data.To enable profiling in MongoDB, use the db.setProfilingLevel()
command:
db.setProfilingLevel(1); // Logs slow queries with execution time > 100ms
- Profiling Levels:
- Level 0: No profiling. MongoDB does not track any operations.
- Level 1: Logs slow queries that exceed the specified threshold (default is 100ms).
- Level 2: Logs all operations, regardless of their execution time.
2. Query Performance Threshold
When enabling profiling, you can set a threshold to track queries that take longer than a specified time. By default, MongoDB logs operations that take longer than 100 milliseconds. You can adjust the threshold to suit your application's needs.To set a custom threshold, use the slowms
parameter:
db.setProfilingLevel(1, { slowms: 200 }); // Logs queries slower than 200ms
This command ensures that only queries that take more than 200 milliseconds will be logged for profiling.
3. Profiling Query Data
MongoDB stores the profiling information in thesystem.profile
collection, which contains documents that describe the operations that took place and their execution times.
To view the profile data, run the following query:
db.system.profile.find().pretty();
This will return a detailed list of operations that MongoDB has logged, including query execution times, the type of operation (e.g., query, update), and the namespace (collection) affected.
4. Understanding Profiling Output
Profiling data includes several important fields that help you understand the performance of each operation:- op: The type of operation (query, insert, update, delete).
- ns: The namespace (database and collection) where the operation occurred.
- query: The query criteria used in the operation.
- millis: The execution time in milliseconds.
- nreturned: The number of documents returned by the query.
- keysExamined: The number of index keys scanned during the query.
- docsExamined: The number of documents scanned during the query execution.
Understanding these fields helps identify which queries are taking longer than expected and why they might be inefficient (e.g., performing full collection scans instead of using indexes).
5. Analyzing Slow Queries
After collecting profiling data, analyze the queries that are taking the longest to execute. Consider the following strategies to improve slow queries:- Check Index Usage: Ensure that your queries are using indexes efficiently. If a query is performing a full collection scan (i.e., not using an index), consider adding the appropriate index to speed up the query.
- Optimize Query Criteria: Avoid using inefficient query operators that require MongoDB to scan the entire collection (e.g.,
$regex
or$nin
on large datasets). - Limit Data Retrieved: Use projections to only return the fields necessary for your application, reducing the amount of data transferred and processed.
- Reduce Complex Aggregations: If you’re using aggregation pipelines, try to break down complex queries into simpler stages or use intermediate collections to reduce the workload.
6. Disabling the Profiler
Once you’ve collected enough profiling data, you can disable the profiler to reduce the overhead on your system. To disable profiling, set the profiling level back to 0:
db.setProfilingLevel(0); // Disables profiling
Disabling profiling ensures that MongoDB will no longer log operations to the system.profile
collection, reducing any unnecessary performance overhead.
7. MongoDB Atlas Profiler
If you’re using MongoDB Atlas, the cloud-based version of MongoDB, you can leverage its built-in performance monitoring tools to profile slow queries:- Performance Advisor: MongoDB Atlas provides a Performance Advisor that suggests optimizations for slow queries, including index recommendations and query execution plans.
- Real-time Performance Metrics: Atlas allows you to monitor real-time query performance, so you can identify and address slow queries as they occur.
- Query Profiler: You can enable the query profiler in Atlas to log slow queries and analyze them through the Atlas UI.
8. Conclusion
Profiling slow queries in MongoDB is a crucial step in maintaining database performance and optimizing query execution. By enabling the profiler, analyzing query performance, and making necessary improvements such as adding indexes and adjusting query patterns, you can reduce query latency and improve the overall efficiency of your MongoDB instance. Whether you’re using MongoDB on-premises or through MongoDB Atlas, understanding and managing slow queries is key to ensuring that your database performs well under load.Local Deployment of MongoDB
Local deployment refers to setting up MongoDB on your local machine to manage and access your database directly. This setup is ideal for development, testing, and learning purposes before moving to a production environment. By deploying MongoDB locally, you can have full control over the database and its configurations, without needing a cloud-based solution.
1. Prerequisites
Before starting with MongoDB's local deployment, ensure your system meets the following prerequisites:- Operating System: MongoDB supports Windows, macOS, and Linux. Ensure your system is compatible with MongoDB’s requirements.
- System Resources: MongoDB requires at least 2GB of RAM for smooth operation, though more is recommended for larger datasets.
- Disk Space: MongoDB stores data on disk, so ensure sufficient space is available, especially for larger collections.
2. Downloading MongoDB
To deploy MongoDB locally, download the installer from the official MongoDB website: Choose the appropriate version for your operating system (Windows, macOS, or Linux) and download the installer.3. Installation Process
The installation process varies depending on your operating system:Windows
- Run the downloaded installer (.msi file).
- During installation, choose the option to install MongoDB as a service (this allows MongoDB to run in the background).
- Specify the installation directory or leave the default path.
- Once installation is complete, MongoDB should start automatically as a service.
macOS
- Install MongoDB using Homebrew:
brew tap mongodb/brew
brew install mongodb-community@5.0
brew services start mongodb/brew/mongodb-community
Linux
- Follow the package manager instructions for your distribution (Ubuntu, CentOS, etc.).
- For Ubuntu, use the following commands:
sudo apt-get update
sudo apt-get install -y mongodb
sudo service mongodb start
4. Starting MongoDB
Once MongoDB is installed, you need to start the MongoDB server. Depending on your operating system, the command will differ:- Windows: MongoDB starts automatically as a service, but you can manually start it from the command line by running
net start MongoDB
. - macOS/Linux: Use the following command to start MongoDB:
mongod
This starts the MongoDB server on the default port (27017) and waits for incoming connections.
5. Connecting to MongoDB
After starting the MongoDB server, you can connect to it using the Mongo shell or a MongoDB client like MongoDB Compass or a programming language driver (e.g., Node.js, Python).To connect via the Mongo shell, open another terminal window and run:
mongo
This will connect to the local MongoDB instance running on localhost:27017
.
6. Verifying the Installation
To verify that MongoDB is running correctly on your local machine, you can check the status by running a simple command in the Mongo shell:
db.version();
This will return the version of MongoDB that is currently running. You can also check the server’s status using the following command:
db.serverStatus();
7. Configuring MongoDB (Optional)
MongoDB’s configuration file allows you to modify settings such as port numbers, data directory paths, and more. The default configuration file is usually located in the MongoDB installation directory, and you can edit it to adjust settings as needed.To edit the configuration, open the mongod.conf
file and update the desired settings. You can specify options such as:
- port: Change the port number on which MongoDB listens.
- dbpath: Specify a custom path to store MongoDB’s data files.
- logpath: Specify a custom path for MongoDB’s log file.
8. Stopping MongoDB
To stop the MongoDB server, you can use the following commands:- Windows: Open a Command Prompt window and run
net stop MongoDB
. - macOS/Linux: Use the following command to stop MongoDB:
mongod --shutdown
9. Conclusion
Local deployment of MongoDB allows you to have a fully functional MongoDB instance running on your machine for development, testing, and learning purposes. By following the installation and configuration steps outlined above, you can set up a local MongoDB instance and begin interacting with the database through the Mongo shell or any MongoDB-supported client.Hosting MongoDB on AWS, GCP, and Azure
Hosting MongoDB in the cloud offers scalability, availability, and flexibility. Major cloud platforms such as Amazon Web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure provide managed services or infrastructure to host and manage MongoDB instances. Here's how you can set up MongoDB on each of these platforms:
1. Hosting MongoDB on AWS
On AWS, MongoDB can be hosted using either a self-managed EC2 instance or a fully managed service like MongoDB Atlas. Here's how you can set it up:
Self-managed MongoDB on EC2
- Create an EC2 instance from the AWS Management Console. Choose an Amazon Linux or Ubuntu AMI (Amazon Machine Image).
- Configure security groups to allow inbound traffic on port 27017 (MongoDB default port).
- SSH into the EC2 instance and follow the installation steps to install MongoDB on your instance:
sudo apt-get update
sudo apt-get install -y mongodb
sudo service mongodb start
/etc/mongodb.conf
file.MongoDB Atlas on AWS
MongoDB Atlas is a fully managed database as a service that runs on AWS, GCP, and Azure. It takes care of infrastructure, backups, scaling, and monitoring. To host MongoDB on AWS using Atlas:
- Visit the MongoDB Atlas website and sign up for an account.
- Create a new cluster and choose AWS as the cloud provider.
- Select your preferred region for hosting the cluster.
- Configure security settings like IP whitelisting and user roles.
- Once the cluster is created, you can connect to it using MongoDB Compass, the Mongo shell, or a MongoDB driver.
2. Hosting MongoDB on GCP
Google Cloud Platform (GCP) offers various ways to host MongoDB, including self-managed instances on Google Compute Engine or using MongoDB Atlas. Here's how you can set up MongoDB on GCP:
Self-managed MongoDB on Google Compute Engine
- Create a Google Compute Engine instance from the Google Cloud Console. Select your preferred operating system (Ubuntu, Debian, or CentOS).
- Configure firewall rules to allow traffic on port 27017 for MongoDB.
- SSH into the instance and install MongoDB using the following commands:
sudo apt-get update
sudo apt-get install -y mongodb
sudo systemctl start mongodb
sudo systemctl enable mongodb
MongoDB Atlas on GCP
Just like on AWS, MongoDB Atlas can be used to host MongoDB on GCP. Follow these steps:
- Go to the MongoDB Atlas website and log in or sign up.
- Create a new cluster and choose GCP as the cloud provider.
- Choose your region and the desired cluster configuration.
- Set up security rules such as IP whitelisting and user authentication.
- Once the cluster is ready, connect to it using MongoDB Compass, the Mongo shell, or your application’s MongoDB driver.
3. Hosting MongoDB on Azure
Azure provides multiple ways to host MongoDB, including through self-managed virtual machines or using MongoDB Atlas. Here's how to set it up:
Self-managed MongoDB on Azure Virtual Machines
- Create a new Virtual Machine (VM) on the Azure Portal. You can choose Ubuntu, CentOS, or any other Linux distribution.
- During the VM setup, configure networking and ensure that port 27017 is open for MongoDB traffic.
- SSH into the virtual machine and install MongoDB:
sudo apt-get update
sudo apt-get install -y mongodb
sudo service mongodb start
/etc/mongodb.conf
.MongoDB Atlas on Azure
Similar to the other cloud platforms, MongoDB Atlas can be used to host MongoDB on Azure. Here’s how to do it:
- Head over to MongoDB Atlas and create a new account or log in.
- Create a new cluster and select Azure as the cloud provider.
- Choose the region and set up your desired configuration.
- Configure security settings such as IP whitelisting and user authentication.
- Once your cluster is created, you can connect to it using MongoDB Compass, the Mongo shell, or a programming language driver.
4. Conclusion
Hosting MongoDB on cloud platforms like AWS, GCP, and Azure provides flexibility and scalability to meet the demands of growing applications. Whether you choose to manage your own MongoDB instances using virtual machines or opt for a fully managed service like MongoDB Atlas, cloud hosting ensures that your MongoDB database can be easily scaled and maintained. MongoDB Atlas simplifies cloud deployment, offering automated backups, scaling, and monitoring, while self-managed instances give you complete control over configuration and management.
MongoDB Atlas for Cloud Deployment
MongoDB Atlas is a fully managed database-as-a-service that runs MongoDB in the cloud. It simplifies cloud deployment by handling infrastructure provisioning, backups, scaling, monitoring, and security. MongoDB Atlas is available on major cloud platforms such as AWS, Google Cloud Platform (GCP), and Microsoft Azure, offering a seamless experience for developers looking to deploy MongoDB in the cloud.
Features of MongoDB Atlas
MongoDB Atlas offers a variety of features that make it an ideal choice for cloud deployment:
- Fully Managed Service: MongoDB Atlas takes care of deployment, patching, backups, monitoring, and scaling, allowing developers to focus on building applications.
- Global Distribution: MongoDB Atlas provides the ability to deploy clusters across multiple regions and cloud providers (AWS, GCP, Azure), ensuring global availability and low-latency access.
- Automated Backups: Atlas offers automated backups with point-in-time restoration, so you can protect your data and recover from failures easily.
- Scalability: MongoDB Atlas supports horizontal scaling, allowing you to scale your database as your application grows without worrying about infrastructure complexities.
- Security: Atlas provides built-in security features, including encryption at rest, network isolation, and fine-grained access control through role-based access control (RBAC).
- Monitoring and Alerts: Built-in monitoring and performance metrics with customizable alerts help you track the health of your database and optimize performance.
- Integrated Search: MongoDB Atlas includes full-text search capabilities built on the open-source search engine, Apache Lucene, allowing you to perform complex queries with ease.
Setting Up MongoDB Atlas
To get started with MongoDB Atlas, follow these steps:
- Create an Atlas Account: Visit the MongoDB Atlas website and sign up for an account.
- Create a Cluster: After signing up, you can create a new cluster. Choose your preferred cloud provider (AWS, GCP, or Azure) and select the region where your cluster will be hosted.
- Configure Cluster Settings: Atlas provides several configuration options, such as selecting the instance size, number of nodes (sharded clusters or replica sets), and backup options.
- Set Up Database User and Permissions: Create a user with specific roles and permissions to access your MongoDB cluster. You can configure role-based access control (RBAC) for fine-grained permissions.
- Whitelist IP Address: Add your IP address or the IP addresses of the machines that need to connect to the Atlas cluster. This step ensures secure access to your database.
- Connect to Your Cluster: Once your cluster is set up, you can connect to it using MongoDB Compass, the Mongo shell, or your application’s MongoDB driver. Atlas provides connection strings and a connection wizard for easy setup.
Scaling with MongoDB Atlas
MongoDB Atlas supports both vertical and horizontal scaling to handle increasing application demands:
Vertical Scaling
Vertical scaling allows you to increase the resources (CPU, RAM, disk space) for your MongoDB cluster. You can scale up your cluster easily from the Atlas interface by selecting a larger instance size without any downtime.
Horizontal Scaling
Horizontal scaling (sharding) allows you to distribute data across multiple nodes to handle larger datasets and higher traffic loads. MongoDB Atlas automatically manages sharding for you, ensuring data is evenly distributed and balanced across nodes.
Security in MongoDB Atlas
MongoDB Atlas provides several layers of security to protect your database:
- Encryption at Rest: Data is encrypted at rest using AES-256 encryption to protect sensitive information.
- Network Isolation: You can configure network isolation with VPC peering, ensuring secure access to your clusters.
- Authentication and Access Control: MongoDB Atlas supports role-based access control (RBAC), ensuring that only authorized users can access specific resources in the database.
- Audit Logs: Atlas offers audit logging to track database activity and comply with regulatory requirements.
Monitoring and Performance Optimization
MongoDB Atlas provides built-in monitoring tools to help you track performance and optimize your database:
- Real-Time Metrics: View key performance metrics such as CPU usage, memory, disk I/O, and query performance in real-time.
- Slow Query Analysis: Atlas identifies slow-running queries and provides detailed information for optimization.
- Index Suggestions: Atlas suggests indexes to improve query performance based on the workload.
- Alerts: You can set up custom alerts for various database events such as high resource utilization, slow queries, and more.
Benefits of MongoDB Atlas
Here are the main benefits of using MongoDB Atlas for cloud deployment:
- Zero Maintenance: Atlas handles all aspects of database management, including backups, monitoring, and upgrades.
- High Availability: Atlas provides replica sets and automatic failover, ensuring your database is always available.
- Global Distribution: With clusters distributed across multiple regions, you can ensure low latency for users worldwide.
- Scalability: Easily scale your database as your application grows without any infrastructure management.
- Security: Atlas ensures your data is protected with industry-standard security practices, such as encryption, access control, and auditing.
Conclusion
MongoDB Atlas simplifies deploying MongoDB in the cloud by offering a fully managed service with powerful features like automated backups, horizontal and vertical scaling, security, and real-time monitoring. Whether you're building a small app or a global enterprise solution, MongoDB Atlas takes care of the operational overhead, allowing you to focus on developing your application.
Best Practices for MongoDB in Production Environments
When deploying MongoDB in production environments, it is essential to follow best practices to ensure high availability, performance, and security. These practices help ensure that your MongoDB deployments are efficient, reliable, and scalable as your application grows.
1. Replica Sets for High Availability
Replica sets provide high availability by maintaining multiple copies of the data across different nodes. This ensures that if one node fails, another can take over. Here are some key points to consider:
- Use an Odd Number of Members: Always use an odd number of replica set members to ensure a majority vote for elections and avoid split-brain scenarios.
- Deploy in Different Availability Zones: Distribute replica set members across different availability zones (AZs) or regions for fault tolerance and reduced risk of downtime.
- Monitor Replica Set Health: Regularly monitor the health of replica sets using MongoDB's built-in monitoring tools to ensure that secondaries are in sync with the primary.
2. Sharding for Horizontal Scaling
Sharding allows MongoDB to distribute data across multiple servers (shards), enabling horizontal scaling to handle large datasets and high throughput. To implement sharding effectively:
- Choose the Right Shard Key: Select a shard key that distributes data evenly across all shards and avoids hotspots. A good shard key should be frequently queried and have high cardinality.
- Monitor Shard Balancing: Regularly monitor the balancing process to ensure even data distribution across the shards. Unbalanced shards can lead to performance degradation.
- Use Chunk Splitting: Use chunk splitting to divide large chunks into smaller, more manageable pieces. This ensures that data is spread evenly across all shards.
3. Indexing for Query Performance
Proper indexing is essential for maintaining fast query performance. To optimize query performance:
- Use Appropriate Indexes: Index fields that are frequently used in queries, such as search or filter criteria. Compound indexes should be used for queries involving multiple fields.
- Monitor Index Usage: Use the
explain()
method to analyze query performance and identify unused or redundant indexes. - Limit Indexes to Necessary Fields: Avoid over-indexing, as too many indexes can affect write performance. Index only the fields that are required for your queries.
4. Backup and Disaster Recovery
Backup and disaster recovery planning are crucial to protect your data. MongoDB provides various ways to back up your data and ensure you can recover in the event of failure:
- Automated Backups: Use MongoDB Atlas or other backup solutions to automate backups and ensure that you have regular snapshots of your data.
- Point-in-Time Recovery: Take advantage of point-in-time recovery in case of accidental data loss or corruption. This allows you to restore your database to a specific moment in time.
- Store Backups in Multiple Locations: Store backups in different geographic locations to protect against regional failures or disasters.
5. Security Best Practices
Maintaining the security of your MongoDB deployment is critical to prevent unauthorized access and protect sensitive data:
- Use Role-Based Access Control (RBAC): Define user roles with specific permissions to control access to different database operations. Ensure that only authorized users can perform sensitive actions.
- Enable TLS/SSL Encryption: Encrypt data in transit using TLS/SSL to protect sensitive information while it is being transmitted between clients and the database.
- Enable Encryption at Rest: Use MongoDB’s built-in encryption at rest to encrypt data stored on disk. This ensures that data is protected in case of physical server theft or unauthorized access.
- IP Whitelisting: Use IP whitelisting to limit access to your MongoDB deployment, allowing only trusted IP addresses to connect to your cluster.
- Audit Logging: Enable auditing to track and log database activity, ensuring you can review access patterns and detect suspicious behavior.
6. Monitor and Optimize Performance
Ongoing monitoring and optimization are essential for maintaining performance in a production environment:
- Use Monitoring Tools: MongoDB provides built-in monitoring tools such as
mongostat
,mongotop
, and Atlas monitoring to track performance metrics such as CPU usage, memory, disk I/O, and query performance. - Set Up Alerts: Set up alerts for key performance indicators such as slow queries, high CPU usage, or resource exhaustion. This will allow you to identify issues before they escalate into outages.
- Optimize Queries: Review slow queries and optimize them by adding appropriate indexes, restructuring queries, or using the aggregation framework.
7. Proper Resource Allocation
Ensuring your MongoDB deployment has the right resources is crucial for performance and stability:
- Monitor Resource Usage: Track resource usage such as CPU, memory, and disk space to ensure that your MongoDB servers are not under-provisioned or over-provisioned.
- Adjust Resource Allocation: Based on usage patterns, adjust the memory and CPU allocation for your MongoDB instances to ensure they can handle the expected workload.
- Scale Up or Out: Scale vertically by upgrading your instance size or horizontally by adding more nodes to the replica set or sharded cluster as your demands grow.
8. Regular Maintenance
Regular maintenance is key to ensuring that your MongoDB deployment runs smoothly:
- Upgrade MongoDB: Regularly upgrade to the latest version of MongoDB to benefit from new features, bug fixes, and security improvements.
- Clean Up Unused Data: Periodically clean up old or unused data to free up disk space and improve performance.
- Rebuild Indexes: Rebuild indexes regularly to ensure that they are optimized for query performance.
Conclusion
Following best practices for MongoDB in production environments ensures that your deployment is secure, scalable, and optimized for performance. By implementing high availability with replica sets, scaling with sharding, securing data with encryption and access control, and regularly monitoring and maintaining the system, you can ensure the long-term success and stability of your MongoDB deployment.
Building a Blog Application with MongoDB
In this section, we'll walk through the steps of building a simple blog application using MongoDB as the database. The blog will allow users to create, read, update, and delete blog posts, and MongoDB will be used to store the posts and user data.
1. Setting Up the Project
Before starting, set up the necessary tools and frameworks for the blog application:
- Node.js and Express: Use Node.js for the backend and Express for handling HTTP requests.
- MongoDB Database: MongoDB will serve as the NoSQL database to store blog posts and user data.
- Mongoose: Mongoose is an ODM (Object Data Modeling) library that simplifies interacting with MongoDB from Node.js.
- Frontend: You can use any frontend framework such as React or Vue.js, but for simplicity, we will focus on the backend and API for this example.
2. Setting Up MongoDB
Start by setting up a MongoDB database. You can use a local MongoDB server or MongoDB Atlas for cloud deployment:
- Local MongoDB: Install MongoDB locally and ensure it is running on your system.
- MongoDB Atlas: Alternatively, create a free MongoDB Atlas cluster for cloud-based database hosting.
3. Defining the Blog Post Schema
Using Mongoose, define a schema for the blog posts. A basic blog post schema will include the following fields:
const mongoose = require('mongoose');
const blogPostSchema = new mongoose.Schema({
title: { type: String, required: true },
content: { type: String, required: true },
author: { type: String, required: true },
date: { type: Date, default: Date.now }
});
const BlogPost = mongoose.model('BlogPost', blogPostSchema);
module.exports = BlogPost;
This schema defines the structure of each blog post, with fields for the title, content, author, and date of creation.
4. Building the API Endpoints
Next, define the routes and API endpoints to handle CRUD operations for the blog posts:
- Create: To create a new blog post, send a POST request to
/posts
. - Read: To retrieve all blog posts, send a GET request to
/posts
. To retrieve a specific post, send a GET request to/posts/:id
. - Update: To update an existing post, send a PUT request to
/posts/:id
. - Delete: To delete a blog post, send a DELETE request to
/posts/:id
.
API Example Code:
const express = require('express');
const mongoose = require('mongoose');
const BlogPost = require('./models/BlogPost');
const app = express();
app.use(express.json());
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/blogApp', { useNewUrlParser: true, useUnifiedTopology: true });
// Create a new blog post
app.post('/posts', async (req, res) => {
const { title, content, author } = req.body;
const newPost = new BlogPost({ title, content, author });
await newPost.save();
res.status(201).json(newPost);
});
// Get all blog posts
app.get('/posts', async (req, res) => {
const posts = await BlogPost.find();
res.json(posts);
});
// Get a specific blog post by ID
app.get('/posts/:id', async (req, res) => {
const post = await BlogPost.findById(req.params.id);
res.json(post);
});
// Update a blog post by ID
app.put('/posts/:id', async (req, res) => {
const { title, content, author } = req.body;
const updatedPost = await BlogPost.findByIdAndUpdate(
req.params.id,
{ title, content, author },
{ new: true }
);
res.json(updatedPost);
});
// Delete a blog post by ID
app.delete('/posts/:id', async (req, res) => {
await BlogPost.findByIdAndDelete(req.params.id);
res.status(204).send();
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code defines the routes for creating, reading, updating, and deleting blog posts. The MongoDB model is used to interact with the database.
5. Testing the API
Use a tool like Postman or Insomnia to test the API endpoints:
- Create: Send a POST request with a JSON body containing the
title
,content
, andauthor
. - Read: Send a GET request to
/posts
to see all posts or/posts/:id
to retrieve a specific post. - Update: Send a PUT request with the updated data to
/posts/:id
. - Delete: Send a DELETE request to
/posts/:id
to delete a blog post.
6. Adding User Authentication
For a blog application, user authentication is often required for actions like creating, updating, and deleting posts. You can use JWT (JSON Web Tokens) for authentication:
- Implement JWT Authentication: Use Passport.js or a similar library to authenticate users and generate tokens.
- Protect Routes: Secure your routes by verifying the JWT token before allowing actions like creating or deleting posts.
7. Frontend Integration
If you're building a frontend for your blog, you can use any modern JavaScript framework (React, Vue, Angular, etc.) to interact with the API. Use HTTP requests to interact with the backend and display blog posts dynamically.
8. Conclusion
With MongoDB, Node.js, and Express, building a simple blog application is straightforward and scalable. MongoDB's flexibility allows you to easily store and manage blog data, while MongoDB Atlas can help with cloud deployment. With proper authentication and API setup, you can create a fully functional blog application that can grow with your project needs.
Building an Inventory Management System with MongoDB
In this section, we will walk through the process of building an inventory management system using MongoDB as the database. The system will allow users to manage products, track stock quantities, and handle orders, with MongoDB serving as the database to store product details, inventory levels, and order information.
1. Setting Up the Project
To begin, set up the necessary tools for the inventory management system:
- Node.js and Express: Use Node.js for the backend and Express for handling API routes and HTTP requests.
- MongoDB Database: MongoDB will store product details, inventory levels, and order records.
- Mongoose: Mongoose is used to interact with MongoDB from Node.js and simplifies database operations.
- Frontend: You can use any frontend framework (e.g., React or Vue.js) to create a UI for managing inventory, viewing products, and processing orders.
2. Setting Up MongoDB
Start by setting up your MongoDB database. You can either use a local MongoDB server or MongoDB Atlas for cloud-based deployment:
- Local MongoDB: Install MongoDB locally on your system.
- MongoDB Atlas: Alternatively, create a free MongoDB Atlas cluster to host your database in the cloud.
3. Defining the Product and Order Schemas
Use Mongoose to define the schemas for products and orders. The product schema will store details like product name, description, price, and stock quantity. The order schema will store information like product ID, quantity ordered, and order status.
const mongoose = require('mongoose');
// Product Schema
const productSchema = new mongoose.Schema({
name: { type: String, required: true },
description: { type: String },
price: { type: Number, required: true },
stock: { type: Number, required: true }
});
const Product = mongoose.model('Product', productSchema);
// Order Schema
const orderSchema = new mongoose.Schema({
productId: { type: mongoose.Schema.Types.ObjectId, ref: 'Product', required: true },
quantity: { type: Number, required: true },
status: { type: String, default: 'Pending' }, // e.g., Pending, Shipped, Delivered
orderDate: { type: Date, default: Date.now }
});
const Order = mongoose.model('Order', orderSchema);
module.exports = { Product, Order };
This schema setup defines the structure of product and order records in the inventory system. Each product has a name, description, price, and stock count, while each order contains a reference to a product, the quantity ordered, and the status of the order.
4. Building the API Endpoints
Now, create the necessary API routes for managing products and processing orders:
- Create Product: Send a POST request to
/products
to add a new product to the inventory. - Get All Products: Send a GET request to
/products
to retrieve all products. - Update Product: Send a PUT request to
/products/:id
to update product information (e.g., stock quantity or price). - Delete Product: Send a DELETE request to
/products/:id
to remove a product from the inventory. - Create Order: Send a POST request to
/orders
to create a new order. - Get All Orders: Send a GET request to
/orders
to retrieve all orders. - Update Order Status: Send a PUT request to
/orders/:id
to update the status of an order (e.g., from "Pending" to "Shipped").
API Example Code:
const express = require('express');
const mongoose = require('mongoose');
const { Product, Order } = require('./models');
// Initialize app
const app = express();
app.use(express.json());
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/inventorySystem', { useNewUrlParser: true, useUnifiedTopology: true });
// Create a new product
app.post('/products', async (req, res) => {
const { name, description, price, stock } = req.body;
const newProduct = new Product({ name, description, price, stock });
await newProduct.save();
res.status(201).json(newProduct);
});
// Get all products
app.get('/products', async (req, res) => {
const products = await Product.find();
res.json(products);
});
// Update a product
app.put('/products/:id', async (req, res) => {
const updatedProduct = await Product.findByIdAndUpdate(req.params.id, req.body, { new: true });
res.json(updatedProduct);
});
// Delete a product
app.delete('/products/:id', async (req, res) => {
await Product.findByIdAndDelete(req.params.id);
res.status(204).send();
});
// Create a new order
app.post('/orders', async (req, res) => {
const { productId, quantity } = req.body;
const product = await Product.findById(productId);
if (product && product.stock >= quantity) {
const newOrder = new Order({ productId, quantity });
await newOrder.save();
product.stock -= quantity; // Reduce stock
await product.save();
res.status(201).json(newOrder);
} else {
res.status(400).json({ message: 'Insufficient stock' });
}
});
// Get all orders
app.get('/orders', async (req, res) => {
const orders = await Order.find().populate('productId');
res.json(orders);
});
// Update order status
app.put('/orders/:id', async (req, res) => {
const updatedOrder = await Order.findByIdAndUpdate(req.params.id, { status: req.body.status }, { new: true });
res.json(updatedOrder);
});
// Start server
app.listen(3000, () => {
console.log('Inventory management system is running on port 3000');
});
This code defines the routes for managing products and orders. It includes endpoints for creating, updating, and deleting products, as well as processing orders and updating their statuses.
5. Testing the API
Use Postman or a similar API testing tool to test the following endpoints:
- Create Product: Send a POST request with a JSON body containing
name
,description
,price
, andstock
. - Get All Products: Send a GET request to
/products
to see all available products. - Update Product: Send a PUT request with the updated data to
/products/:id
. - Delete Product: Send a DELETE request to
/products/:id
to remove a product. - Create Order: Send a POST request to
/orders
with product ID and quantity. - Get All Orders: Send a GET request to
/orders
to view all orders. - Update Order Status: Send a PUT request to
/orders/:id
to change the status (e.g., "Pending" to "Shipped").
6. Adding User Authentication (Optional)
For securing the application, you can add user authentication using JWT (JSON Web Tokens). This will allow users to authenticate before managing inventory data:
- JWT Authentication: Use Passport.js or another library to handle user authentication and generate JWT tokens.
- Protect Routes: Use middleware to secure routes for managing products and orders, ensuring only authorized users can perform these actions.
7. Frontend Integration
You can use a frontend framework like React or Vue.js to build an interactive UI where users can manage products and view orders. Use HTTP requests to interact with the backend API for product management and order processing.
8. Conclusion
With MongoDB, Node.js, and Express, building an inventory management system is efficient and scalable. MongoDB provides flexibility in managing product data, stock levels, and orders. By integrating with a frontend, you can create a complete inventory management solution for your business or project.
Building an E-commerce Product Catalog with MongoDB
In this section, we will walk through the process of building an e-commerce product catalog using MongoDB. The catalog will store information about products such as name, description, price, category, and image, and will allow users to search and filter products by various attributes such as price, category, and brand.
1. Setting Up the Project
Begin by setting up the necessary tools for the e-commerce product catalog:
- Node.js and Express: Use Node.js for the backend and Express to handle API routes and HTTP requests.
- MongoDB Database: MongoDB will store the product catalog data, including product details, categories, prices, and inventory information.
- Mongoose: Mongoose will be used to define the product schema and interact with MongoDB from Node.js.
- Frontend: Use a frontend framework (e.g., React, Vue.js, or Angular) to display the product catalog and allow users to filter products based on different attributes.
2. Setting Up MongoDB
Start by setting up your MongoDB database. You can either use a local MongoDB instance or MongoDB Atlas for cloud-based deployment.
- Local MongoDB: Install MongoDB locally on your system.
- MongoDB Atlas: Create a free MongoDB Atlas cluster to host your database in the cloud.
3. Defining the Product Schema
Use Mongoose to define the schema for products. The product schema will include attributes such as name, description, price, category, image, and stock.
const mongoose = require('mongoose');
// Product Schema
const productSchema = new mongoose.Schema({
name: { type: String, required: true },
description: { type: String },
price: { type: Number, required: true },
category: { type: String, required: true },
brand: { type: String },
image: { type: String },
stock: { type: Number, default: 0 },
dateAdded: { type: Date, default: Date.now }
});
const Product = mongoose.model('Product', productSchema);
module.exports = Product;
The schema defines the structure of a product document in MongoDB. Each product has a name, description, price, category, brand, image URL, stock quantity, and the date it was added.
4. Building the API Endpoints
Next, create the necessary API routes for managing products and interacting with the product catalog:
- Create Product: Send a POST request to
/products
to add a new product to the catalog. - Get All Products: Send a GET request to
/products
to retrieve all products in the catalog. - Get Products by Category: Send a GET request to
/products/category/:category
to filter products by category. - Get Product Details: Send a GET request to
/products/:id
to retrieve details of a specific product. - Search Products: Send a GET request to
/products/search
to search for products based on query parameters like name, price range, or category. - Update Product: Send a PUT request to
/products/:id
to update product details (e.g., price, stock, description). - Delete Product: Send a DELETE request to
/products/:id
to remove a product from the catalog.
API Example Code:
const express = require('express');
const mongoose = require('mongoose');
const Product = require('./models/product');
// Initialize app
const app = express();
app.use(express.json());
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/ecommerce', { useNewUrlParser: true, useUnifiedTopology: true });
// Create a new product
app.post('/products', async (req, res) => {
const { name, description, price, category, brand, image, stock } = req.body;
const newProduct = new Product({ name, description, price, category, brand, image, stock });
await newProduct.save();
res.status(201).json(newProduct);
});
// Get all products
app.get('/products', async (req, res) => {
const products = await Product.find();
res.json(products);
});
// Get products by category
app.get('/products/category/:category', async (req, res) => {
const products = await Product.find({ category: req.params.category });
res.json(products);
});
// Search products
app.get('/products/search', async (req, res) => {
const { name, priceMin, priceMax, category } = req.query;
const filter = {};
if (name) filter.name = { $regex: name, $options: 'i' };
if (priceMin && priceMax) filter.price = { $gte: priceMin, $lte: priceMax };
if (category) filter.category = category;
const products = await Product.find(filter);
res.json(products);
});
// Get product details by ID
app.get('/products/:id', async (req, res) => {
const product = await Product.findById(req.params.id);
if (!product) return res.status(404).json({ message: 'Product not found' });
res.json(product);
});
// Update a product
app.put('/products/:id', async (req, res) => {
const updatedProduct = await Product.findByIdAndUpdate(req.params.id, req.body, { new: true });
res.json(updatedProduct);
});
// Delete a product
app.delete('/products/:id', async (req, res) => {
await Product.findByIdAndDelete(req.params.id);
res.status(204).send();
});
// Start server
app.listen(3000, () => {
console.log('E-commerce product catalog is running on port 3000');
});
This code defines the API routes for adding, retrieving, updating, and deleting products, as well as the ability to search products based on different filters such as name, price, and category.
5. Testing the API
Use Postman or a similar API testing tool to test the following endpoints:
- Create Product: Send a POST request with a JSON body containing product details such as
name
,description
,price
,category
,brand
, andstock
. - Get All Products: Send a GET request to
/products
to see all available products. - Get Products by Category: Send a GET request to
/products/category/:category
to filter products by category. - Search Products: Send a GET request to
/products/search?name=shirt&priceMin=10&priceMax=50
to search for products. - Get Product Details: Send a GET request to
/products/:id
to view a specific product. - Update Product: Send a PUT request with the updated data to
/products/:id
. - Delete Product: Send a DELETE request to
/products/:id
to remove a product from the catalog.
6. Frontend Integration
For the frontend, you can use a framework like React or Vue.js to create a product catalog page. The frontend will use HTTP requests to interact with the backend API to display products, allow filtering, and view details of each product.
7. Conclusion
With MongoDB, Node.js, and Express, building a dynamic and scalable e-commerce product catalog is straightforward. MongoDB provides flexibility in managing product data and offers powerful querying capabilities for filtering and searching products. The catalog can easily scale to accommodate a growing number of products and provide a rich user experience for browsing and purchasing products.
Implementing a Chat Application Using MongoDB
In this section, we will walk through the process of building a chat application using MongoDB. This application will support real-time messaging and chat history storage. MongoDB will be used to store messages, user details, and chat rooms, allowing for easy scalability and data retrieval. We will use Node.js, Express, and MongoDB (with Mongoose) to implement the backend, and WebSockets for real-time communication.
1. Setting Up the Project
Start by setting up a Node.js project with the necessary dependencies:
- Node.js and Express: Use Node.js for the backend and Express for API routing.
- MongoDB: MongoDB will store messages, users, and chat rooms.
- Mongoose: Mongoose will be used for interacting with MongoDB from Node.js.
- Socket.io: Socket.io will be used for real-time communication between users.
2. Setting Up MongoDB
Set up MongoDB to store chat data. You can either use a local instance of MongoDB or MongoDB Atlas for cloud-based hosting.
- Local MongoDB: Install MongoDB locally on your system if you're using it for local development.
- MongoDB Atlas: Create a MongoDB Atlas account and set up a database cluster for cloud-based hosting.
3. Defining the Schema
Use Mongoose to define schemas for the chat application. The main schemas will include users, messages, and chat rooms.
const mongoose = require('mongoose');
// User Schema
const userSchema = new mongoose.Schema({
username: { type: String, required: true, unique: true },
email: { type: String, required: true, unique: true }
});
// Message Schema
const messageSchema = new mongoose.Schema({
sender: { type: mongoose.Schema.Types.ObjectId, ref: 'User', required: true },
chatRoom: { type: mongoose.Schema.Types.ObjectId, ref: 'ChatRoom', required: true },
message: { type: String, required: true },
timestamp: { type: Date, default: Date.now }
});
// ChatRoom Schema
const chatRoomSchema = new mongoose.Schema({
name: { type: String, required: true },
users: [{ type: mongoose.Schema.Types.ObjectId, ref: 'User' }]
});
const User = mongoose.model('User', userSchema);
const Message = mongoose.model('Message', messageSchema);
const ChatRoom = mongoose.model('ChatRoom', chatRoomSchema);
module.exports = { User, Message, ChatRoom };
These schemas define the structure of user data, messages, and chat rooms in the MongoDB database. The Message schema stores the sender, chat room, message content, and timestamp. The ChatRoom schema stores the name of the room and a list of users who are part of the room.
4. Setting Up the API
Next, create the necessary API routes to handle user registration, message sending, and retrieving chat history:
- Register User: POST to
/api/users
to register a new user. - Create Chat Room: POST to
/api/chatrooms
to create a new chat room. - Send Message: POST to
/api/messages
to send a message to a chat room. - Get Messages: GET to
/api/messages/:chatRoomId
to retrieve chat history for a specific room.
API Example Code:
const express = require('express');
const mongoose = require('mongoose');
const { User, Message, ChatRoom } = require('./models');
// Initialize app
const app = express();
app.use(express.json());
// MongoDB connection
mongoose.connect('mongodb://localhost:27017/chatApp', { useNewUrlParser: true, useUnifiedTopology: true });
// Register user
app.post('/api/users', async (req, res) => {
const { username, email } = req.body;
const newUser = new User({ username, email });
await newUser.save();
res.status(201).json(newUser);
});
// Create a chat room
app.post('/api/chatrooms', async (req, res) => {
const { name, users } = req.body;
const newChatRoom = new ChatRoom({ name, users });
await newChatRoom.save();
res.status(201).json(newChatRoom);
});
// Send a message
app.post('/api/messages', async (req, res) => {
const { sender, chatRoom, message } = req.body;
const newMessage = new Message({ sender, chatRoom, message });
await newMessage.save();
res.status(201).json(newMessage);
});
// Get messages for a chat room
app.get('/api/messages/:chatRoomId', async (req, res) => {
const messages = await Message.find({ chatRoom: req.params.chatRoomId })
.populate('sender', 'username')
.sort('timestamp');
res.json(messages);
});
// Start server
app.listen(3000, () => {
console.log('Chat application is running on port 3000');
});
This code defines the API routes for registering users, creating chat rooms, sending messages, and retrieving messages from a specific chat room. It uses Mongoose to interact with MongoDB.
5. Real-Time Chat with Socket.io
Socket.io will be used to handle real-time communication in the chat application. It allows for sending and receiving messages instantly between clients. Here's how to integrate Socket.io into the application:
- Set up Socket.io on the server: Install and configure Socket.io in your server to emit and listen for messages.
- Emit messages: When a message is sent by a user, emit it to all users in the chat room.
- Listen for incoming messages: On the client side, listen for incoming messages and display them in real time.
Server-side Socket.io Code:
const http = require('http');
const socketIo = require('socket.io');
// Create an HTTP server
const server = http.createServer(app);
// Initialize Socket.io
const io = socketIo(server);
// Real-time communication
io.on('connection', (socket) => {
console.log('A user connected');
// Listen for new messages
socket.on('sendMessage', async (data) => {
const { sender, chatRoom, message } = data;
const newMessage = new Message({ sender, chatRoom, message });
await newMessage.save();
// Emit the message to the chat room
io.to(chatRoom).emit('newMessage', newMessage);
});
// Disconnect event
socket.on('disconnect', () => {
console.log('A user disconnected');
});
});
// Start server
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code sets up a Socket.io server that listens for new messages from clients and emits them to the appropriate chat room. The server also handles user connections and disconnections.
6. Frontend Integration
For the frontend, you can use a framework like React to display the chat interface and handle real-time messaging. The frontend will communicate with the backend API to send and receive messages using Socket.io.
Example Frontend Code for Socket.io:
import io from 'socket.io-client';
const socket = io('http://localhost:3000');
// Listen for new messages
socket.on('newMessage', (message) => {
console.log('New message received:', message);
});
// Send a message
const sendMessage = (chatRoom, sender, message) => {
socket.emit('sendMessage', { chatRoom, sender, message });
};
This code connects the frontend to the Socket.io server and listens for new messages. It also allows users to send messages in real time.
7. Testing the Application
Use Postman to test the API endpoints for user registration, chat room creation, and message sending. Use the frontend to test real-time messaging and ensure that users can send and receive messages instantly.
8. Conclusion
With MongoDB, Node.js, Express, and Socket.io, you can build a scalable and responsive real-time chat application. MongoDB provides flexibility for managing user data, messages, and chat rooms, while Socket.io allows for smooth and instantaneous communication between users. This architecture can easily scale to accommodate a growing number of users and chat rooms, making it an ideal solution for building chat applications.
MongoDB Compass Overview
MongoDB Compass is a powerful graphical user interface (GUI) tool for MongoDB. It provides a user-friendly interface to interact with MongoDB databases, making it easier to visualize, analyze, and manipulate data. Compass allows developers and database administrators to explore database contents, run queries, manage collections, and optimize performance without writing complex commands in the MongoDB shell.
Key Features of MongoDB Compass
- Visualize Data: Compass allows you to view your MongoDB collections and documents in a visually intuitive way, helping to understand and analyze your database structure.
- Query Builder: The built-in query builder enables you to construct complex queries using a point-and-click interface, making it easy to search and filter documents.
- Aggregation Pipeline Builder: Compass provides an interactive aggregation pipeline builder that simplifies the process of creating advanced aggregation queries without needing to write complex code.
- Schema Explorer: The Schema Explorer helps visualize the schema of your collections, displaying data types and structures, which is useful for understanding your data and planning schema changes.
- Index Management: Compass allows you to create, view, and manage indexes, enabling you to optimize query performance.
- Real-Time Performance Metrics: Compass provides real-time performance metrics, helping you identify and address performance bottlenecks by analyzing query performance, memory usage, and other key metrics.
- Data Import/Export: Compass allows you to import and export data in JSON, CSV, or BSON formats, making it easier to migrate data between MongoDB and other systems.
Installing MongoDB Compass
MongoDB Compass is available for Windows, macOS, and Linux. You can download it from the official MongoDB website:
- Visit the official MongoDB Compass download page.
- Select the appropriate version for your operating system (Windows, macOS, or Linux).
- Follow the installation instructions provided on the website to install MongoDB Compass.
Connecting to MongoDB with Compass
Once MongoDB Compass is installed, you can connect to your MongoDB instance by entering the connection details:
- Open MongoDB Compass.
- Enter the connection string (e.g.,
mongodb://localhost:27017
for a local MongoDB instance or your MongoDB Atlas connection string). - Click Connect to establish the connection.
After successfully connecting to the database, you can start exploring your collections and documents in the MongoDB Compass interface.
Working with Data in MongoDB Compass
MongoDB Compass provides an easy interface for managing your data:
- View Documents: You can view the documents within a collection in a tabular format. MongoDB Compass provides features like pagination, sorting, and filtering to help you explore your data.
- Insert Documents: You can insert new documents into a collection directly from the Compass interface. This is particularly useful for quickly adding test data or managing small datasets.
- Edit Documents: Compass allows you to edit existing documents in real time. You can modify fields, add new fields, and update values directly in the UI.
- Delete Documents: Deleting documents is as easy as selecting the document and clicking on the delete option. You can also filter documents to delete multiple entries at once.
Running Queries in MongoDB Compass
One of the key features of MongoDB Compass is its ability to run queries and filter data using a point-and-click interface. You can:
- Build Queries: Use the query builder to construct queries without needing to write MongoDB query syntax manually. You can filter documents by field, apply conditions (like
$gt
,$lt
, and$in
), and sort the results. - Save Queries: Save frequently used queries for easy reuse, and load them later as needed.
- Run Aggregation Pipelines: You can use the aggregation pipeline builder to create complex aggregation queries that transform and analyze your data. Compass provides an interactive interface to build and test the pipeline stages.
Schema Visualization and Analysis
MongoDB Compass automatically analyzes the schema of your collections and presents it in a visual format. The Schema Explorer shows:
- Field Types: See the types of fields in your documents, such as strings, integers, and arrays.
- Field Frequency: View the frequency of field appearances in your documents, helping you understand the distribution of data.
- Data Distribution: View histograms of field values to help identify patterns and outliers in your data.
Index Management in MongoDB Compass
MongoDB Compass provides an interface for managing indexes:
- Create Indexes: You can create indexes to optimize query performance. MongoDB Compass provides a simple interface to select the fields you want to index.
- View Indexes: Compass displays all the indexes on a collection, including their types and usage statistics.
- Delete Indexes: You can delete unnecessary indexes from a collection to reduce overhead and optimize performance.
Performance Optimization in MongoDB Compass
MongoDB Compass provides real-time performance metrics that can help you monitor and optimize your MongoDB instance:
- Monitor Queries: View detailed information on slow-running queries, including execution time, and identify potential performance bottlenecks.
- Analyze Index Usage: Compass shows statistics on how often indexes are used, helping you identify unused indexes and optimize your schema.
- Storage Metrics: View storage metrics, including disk space usage and storage engine statistics, to monitor the health of your MongoDB instance.
Best Practices for Using MongoDB Compass
- Use for Development and Testing: MongoDB Compass is ideal for use during development and testing, where you can quickly iterate on data changes and query performance.
- Monitor Performance: Regularly use Compass to monitor your MongoDB instance's performance and address any slow queries or resource bottlenecks.
- Backup Data: Ensure that you back up your MongoDB data regularly, especially before making significant schema changes or running complex queries.
Conclusion
MongoDB Compass is a powerful, user-friendly tool that makes it easier to interact with MongoDB databases. Whether you're a developer building an application or a database administrator managing large datasets, Compass provides everything you need to visualize, manage, and optimize your MongoDB data. With its intuitive interface and powerful features, MongoDB Compass simplifies database operations and helps you work more efficiently with MongoDB.
Using mongodump and mongorestore for Backups
Backing up MongoDB data is critical for ensuring that your data remains safe in case of failures or data corruption. MongoDB provides two powerful command-line tools—mongodump
and mongorestore
—for creating and restoring backups of MongoDB databases. These tools allow you to efficiently back up your entire database or specific collections, and restore them when needed.
What is mongodump?
mongodump
is used to create a backup of a MongoDB database. It generates a binary export of the database’s contents, which can then be stored or transferred to another location. The output is typically saved in BSON format, which is MongoDB's native binary format for storing data.
What is mongorestore?
mongorestore
is used to restore data from a backup created by mongodump
. It can be used to restore an entire database or specific collections from the BSON dump files created by mongodump
.
Creating a Backup with mongodump
To create a backup of a MongoDB database, you can use the mongodump
command. The most basic usage is:
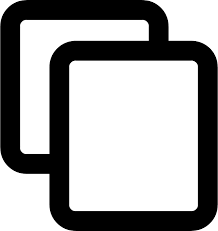
mongodump --host --port --out
- --host: The hostname or IP address of the MongoDB server. If you are running MongoDB locally, you can omit this option or use
localhost
. - --port: The port number where MongoDB is running. The default MongoDB port is 27017.
- --out: Specifies the directory where the backup should be saved. The backup will be stored in subdirectories named after the databases.
Example: Backup a Local MongoDB Database
If you want to back up a local MongoDB instance:
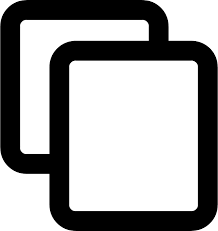
mongodump --out /path/to/backup/
This command creates a backup of all databases in the specified /path/to/backup/
directory.
Backing Up a Specific Database
If you want to back up a specific database, you can use the --db
option:
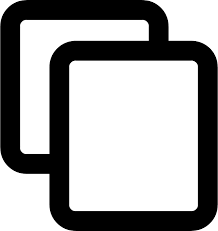
mongodump --db mydatabase --out /path/to/backup/
This command will back up only the mydatabase
database.
Backing Up a Specific Collection
You can also back up a specific collection from a database with the --collection
option:
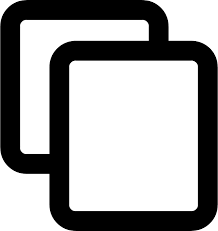
mongodump --db mydatabase --collection mycollection --out /path/to/backup/
This will back up the mycollection
collection from the mydatabase
database.
Restoring Data with mongorestore
To restore data from a backup, you can use the mongorestore
command. The basic usage is:
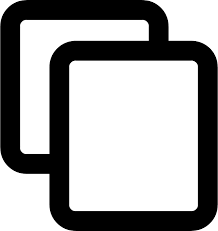
mongorestore --host --port --dir
- --host: The hostname or IP address of the MongoDB server where the data should be restored.
- --port: The port number of the MongoDB instance.
- --dir: The directory where the backup is located. This is the folder where the
mongodump
output was saved.
Example: Restore a Local MongoDB Database
If you want to restore a backup to a local MongoDB instance:
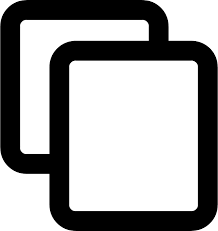
mongorestore --dir /path/to/backup/
This will restore all databases that were backed up in the specified directory.
Restoring a Specific Database
To restore a specific database from the backup:
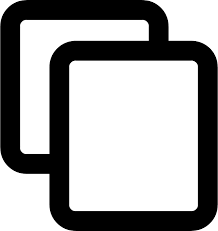
mongorestore --db mydatabase /path/to/backup/mydatabase/
This will restore the mydatabase
database from the backup.
Restoring a Specific Collection
If you want to restore only a specific collection, you can use the --collection
option:
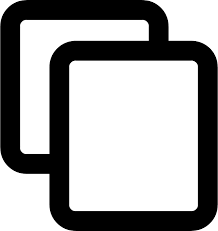
mongorestore --db mydatabase --collection mycollection /path/to/backup/mydatabase/mycollection.bson
This will restore the mycollection
collection from the backup.
Additional mongodump and mongorestore Options
- --gzip: Use this option if your backup files are compressed with gzip.
- --drop: When restoring, this option drops the target database or collection before restoring the data. Use this carefully to avoid unintentional data loss.
- --authenticationDatabase: Use this option if your MongoDB instance requires authentication, specifying the database that holds the user credentials.
Automating Backups
To automate backups, you can use a cron job (Linux/macOS) or Task Scheduler (Windows) to schedule regular mongodump
commands. This ensures that backups are taken at regular intervals without manual intervention.
Restoring from a Backup in Case of Failure
In case of a database failure or data corruption, you can restore from the latest backup by using mongorestore
. Having regular backups ensures that you can quickly recover your data and minimize downtime.
Best Practices for Backups
- Schedule Regular Backups: Set up automated backups to ensure that data is backed up at regular intervals (e.g., daily or weekly).
- Store Backups in Multiple Locations: Keep backup copies in different physical or cloud locations to protect against data loss due to hardware failure or disasters.
- Test Backups Regularly: Periodically test your backups by restoring them to ensure that they are valid and can be used for recovery if needed.
- Encrypt Backups: Use encryption to protect sensitive data in your backups, especially if they are stored in cloud environments.
Conclusion
Using mongodump
and mongorestore
provides an efficient way to back up and restore MongoDB data. These tools give you flexibility and control over your backups, allowing you to back up entire databases, specific collections, or apply advanced options for compression and authentication. By incorporating regular backups into your workflow and following best practices, you can ensure that your MongoDB data is protected and recoverable in case of failure.
Exporting and Importing Data with mongoexport and mongoimport
In addition to creating backups and restoring data with mongodump
and mongorestore
, MongoDB provides two other tools—mongoexport
and mongoimport
—for exporting and importing data in JSON or CSV formats. These tools are particularly useful when you need to move data between MongoDB and external systems or when you want to perform data migrations or integrations.
What is mongoexport?
mongoexport
is a command-line utility that allows you to export data from MongoDB collections to JSON or CSV files. This is useful for creating backups in a readable format, transferring data to other systems, or performing analytics outside of MongoDB.
What is mongoimport?
mongoimport
is a tool used to import data from JSON, CSV, or TSV files into a MongoDB database. It is helpful when you need to load external data into MongoDB, such as data from another database, a file dump, or a CSV file.
Exporting Data with mongoexport
To export data from a MongoDB collection, you can use the mongoexport
command. The basic syntax is:
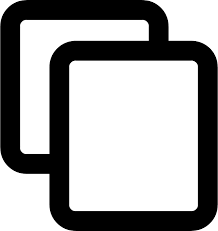
mongoexport --host --port --db --collection --out --type
- --host: The hostname or IP address of the MongoDB server.
- --port: The port number of the MongoDB server.
- --db: The name of the MongoDB database from which to export data.
- --collection: The name of the collection to export.
- --out: The path to the output file where the exported data will be saved.
- --type: The format of the exported file. The supported formats are
json
(default) andcsv
.
Example: Export a Collection to JSON
If you want to export the users
collection from the mydatabase
database to a JSON file:
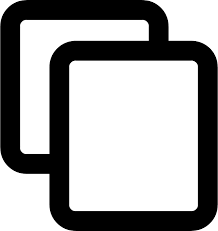
mongoexport --db mydatabase --collection users --out users.json
This command will export the data from the users
collection into a file named users.json
.
Exporting Data to CSV
If you want to export data in CSV format, you need to specify the fields you want to export using the --fields
option:
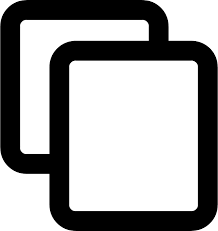
mongoexport --db mydatabase --collection users --out users.csv --type csv --fields "name,email,age"
This will export the name
, email
, and age
fields of the users
collection into a CSV file.
Exporting Data with Query Filters
You can also apply filters to export only specific data using the --query
option:
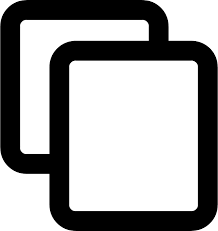
mongoexport --db mydatabase --collection users --out young_users.json --query '{"age": {"$lt": 30}}'
This command will export only the documents where the age
field is less than 30.
Importing Data with mongoimport
To import data into MongoDB, use the mongoimport
tool. The basic syntax for importing data is:
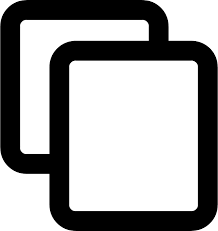
mongoimport --host --port --db --collection --file --type
- --host: The hostname or IP address of the MongoDB server.
- --port: The port number of the MongoDB server.
- --db: The name of the MongoDB database where the data will be imported.
- --collection: The name of the collection to import the data into.
- --file: The path to the input file containing the data to be imported.
- --type: The format of the input file. The supported formats are
json
(default),csv
, andtsv
.
Example: Import a JSON File
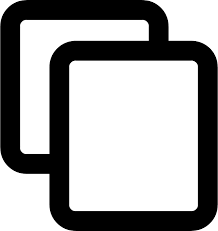
mongoimport --db mydatabase --collection users --file users.json --type json
This command will import the users.json
file into the users
collection of the mydatabase
database.
Importing CSV Data
To import data from a CSV file, specify the --type
option as csv
and provide a list of field names using the --headerline
option:
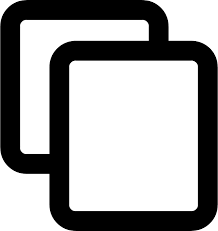
mongoimport --db mydatabase --collection users --file users.csv --type csv --headerline
The --headerline
option tells MongoDB to use the first row of the CSV file as field names.
Importing Data with Query Filters
When importing data, MongoDB can automatically insert documents or update existing ones based on a query filter using the --upsert
option:
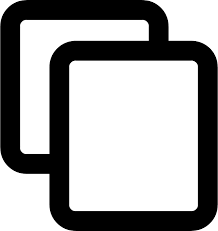
mongoimport --db mydatabase --collection users --file updated_users.json --type json --upsert
This will insert new documents and update existing ones if a match is found based on the document’s unique identifier.
Additional mongoexport and mongoimport Options
- --authenticationDatabase: Specify the database containing the user credentials when MongoDB authentication is enabled.
- --drop: Use the
--drop
option withmongoimport
to drop the collection before importing the data. This is useful to ensure that old data is replaced with the new data. - --jsonArray: When exporting JSON data, use this option to treat the data as a JSON array. This is useful if your data is structured as an array of objects.
Best Practices for Exporting and Importing Data
- Data Validation: Ensure that the data you're importing matches the expected structure of your MongoDB collections to avoid errors.
- Data Integrity: When exporting or importing data, make sure that the file contains complete and accurate data. Use
--query
to filter out incomplete or invalid records before exporting. - Test Before Importing: Always test the import process in a development or staging environment before importing data into production.
- Backup Data: Before importing large datasets, it’s good practice to back up your current MongoDB data to prevent data loss in case of import errors.
Conclusion
Using mongoexport
and mongoimport
provides a simple yet powerful way to export and import data to and from MongoDB. These tools support multiple data formats (JSON, CSV) and offer advanced options for filtering, upserting, and automating the process. By leveraging these tools, you can easily integrate MongoDB with external systems, move data between environments, and perform migrations with minimal effort.
Monitoring with MongoDB Ops Manager and Cloud Manager
MongoDB Ops Manager and Cloud Manager are powerful tools for monitoring, managing, and automating MongoDB deployments. These tools allow you to monitor the health and performance of your MongoDB clusters, receive real-time alerts, and automate administrative tasks like backups, upgrades, and scaling. Both solutions offer robust features to help ensure your MongoDB deployment runs smoothly in a production environment.
What is MongoDB Ops Manager?
MongoDB Ops Manager is a comprehensive management platform for MongoDB deployments, providing full control over the lifecycle of MongoDB clusters. It enables on-premise management of MongoDB instances, ensuring high availability, automated backups, monitoring, and performance optimization. Ops Manager is typically used for self-hosted MongoDB clusters and can be deployed in your data center or private cloud.
What is MongoDB Cloud Manager?
MongoDB Cloud Manager is a cloud-based version of Ops Manager that offers similar functionality but is hosted by MongoDB, Inc. It allows you to monitor and manage MongoDB instances deployed on cloud platforms like AWS, Azure, and Google Cloud. Cloud Manager is suitable for those who prefer a fully managed solution without the need to maintain the infrastructure for the monitoring platform itself.
Key Features of MongoDB Ops Manager and Cloud Manager
- Real-Time Performance Monitoring: Both Ops Manager and Cloud Manager provide real-time monitoring of MongoDB deployments, including metrics like operations per second, memory usage, disk I/O, and more. This helps you identify performance bottlenecks and optimize your clusters.
- Alerting and Notifications: You can set up custom alerts and notifications to be notified of any issues with your MongoDB deployment. Alerts can be triggered based on performance thresholds, replication lag, disk space, and more.
- Automated Backups: Both Ops Manager and Cloud Manager provide automated backup solutions that ensure your data is regularly backed up and easily restorable in case of failures. You can schedule backups and configure retention policies.
- Database Automation: With MongoDB Ops Manager and Cloud Manager, you can automate common administrative tasks such as deployment, scaling, upgrades, and patching. This helps reduce manual intervention and the risk of human error.
- Backup and Restore: Both platforms include options for managing backups and restoring data when necessary. You can perform point-in-time restores, and Ops Manager and Cloud Manager ensure that backups are consistent with your MongoDB clusters.
- Security and Access Control: MongoDB Ops Manager and Cloud Manager allow you to configure advanced security features, including encryption, access control, and audit logging. You can manage user roles, permissions, and access rights to ensure secure database operations.
- Cluster Management: You can create, configure, and manage sharded clusters, replica sets, and standalone instances from within both platforms. You can also scale your MongoDB clusters vertically or horizontally as needed.
Setting Up MongoDB Ops Manager
To begin using MongoDB Ops Manager, you'll need to install it on a server and configure it for your MongoDB instances. The setup process typically involves the following steps:
- Install Ops Manager: Download and install the Ops Manager software on a dedicated server in your data center.
- Connect MongoDB Cluster: Configure your MongoDB instances or replica sets to connect to Ops Manager. You can use the Ops Manager agent to allow communication between Ops Manager and your MongoDB deployment.
- Monitor and Configure Alerts: Once connected, you can begin monitoring your clusters using the Ops Manager dashboard. Set up alerts to notify you of any performance or health issues.
- Automate Backups and Upgrades: Set up automated backups and schedule upgrades through the Ops Manager interface. You can also configure maintenance windows for non-intrusive updates.
Setting Up MongoDB Cloud Manager
MongoDB Cloud Manager is a fully hosted solution, so the setup process is simpler compared to Ops Manager. Here's how to get started:
- Create a MongoDB Cloud Account: Sign up for a MongoDB Cloud account at MongoDB Cloud.
- Connect MongoDB Clusters: After logging in to Cloud Manager, use the connection wizard to connect your MongoDB clusters to the platform. Cloud Manager integrates with cloud providers like AWS, Azure, and GCP.
- Configure Monitoring and Alerts: Use the Cloud Manager interface to monitor your MongoDB clusters, configure alerts, and set up automated tasks.
- Manage Backups: Schedule automatic backups and configure retention policies. Cloud Manager will handle the backup process in the cloud.
Monitoring MongoDB Performance with Ops Manager and Cloud Manager
Both Ops Manager and Cloud Manager provide powerful monitoring tools to track the health and performance of your MongoDB deployment. The dashboard displays key performance indicators (KPIs) such as:
- Operations per Second: The number of read and write operations being performed on the MongoDB cluster.
- CPU and Memory Usage: CPU and memory utilization of the MongoDB server or replica set.
- Disk I/O: The number of disk operations occurring, which can help identify disk bottlenecks.
- Replication Lag: The time delay between the primary and secondary nodes in a replica set.
- Query Performance: The time taken to execute queries and their impact on the system's performance.
Setting Up Alerts and Notifications
Ops Manager and Cloud Manager support alerting based on custom thresholds for various metrics. You can configure alerts for:
- High disk usage
- Replication lag
- Degraded performance
- Failed backups
Alerts can be sent via email, SMS, or integrated with third-party tools like Slack or PagerDuty for real-time notifications.
Backup and Restore with Ops Manager and Cloud Manager
Both platforms support automated backups and provide tools for restoring data in case of failure. Backups are typically performed on a scheduled basis, and you can configure point-in-time restores to recover data to a specific moment in time. This ensures that your MongoDB deployment is resilient and can recover from disasters quickly.
Conclusion
MongoDB Ops Manager and Cloud Manager are essential tools for effectively managing and monitoring MongoDB clusters. They provide comprehensive monitoring, alerting, automation, and backup features that help ensure high availability and optimal performance in production environments. Whether you are managing on-premise clusters with Ops Manager or cloud-hosted clusters with Cloud Manager, these tools offer a seamless and efficient management experience for MongoDB deployments.
Using MongoDB with Express.js in the MERN Stack
The MERN stack is a powerful combination of technologies used to build modern, full-stack web applications. It consists of MongoDB, Express.js, React, and Node.js. MongoDB serves as the database, Express.js handles the backend API, Node.js powers the server-side runtime, and React builds the frontend user interface. In this section, we will focus on how to integrate MongoDB with Express.js in the MERN stack to build robust, data-driven applications.
What is Express.js?
Express.js is a minimal, unopinionated web application framework for Node.js. It simplifies the creation of RESTful APIs, handling routing, middleware, and HTTP requests. Express.js is lightweight and flexible, making it an ideal choice for building scalable server-side applications in the MERN stack.
Setting Up MongoDB with Express.js
To use MongoDB with Express.js, you need to establish a connection between the two. This process typically involves the following steps:
- Install Dependencies: Install the necessary packages, including
mongoose
(an ODM library for MongoDB) andexpress
: - Create an Express Application: Initialize an Express server and set up routes to handle API requests:
- Connect to MongoDB: Use Mongoose to connect your Express.js application to MongoDB:
- Define Mongoose Models: Create Mongoose models to interact with MongoDB collections. A model defines the structure of the data and provides methods for interacting with MongoDB:
- Create API Routes: Define Express routes that interact with MongoDB through the Mongoose models. For example, creating and retrieving users from the database:
- Start the Server: Finally, start the Express.js server to listen for incoming requests:
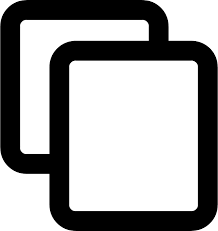
npm install express mongoose
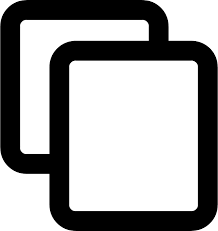
const express = require('express');
const app = express();
app.use(express.json());
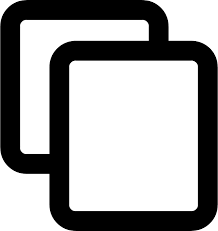
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydb', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch((err) => console.log('MongoDB connection error:', err));
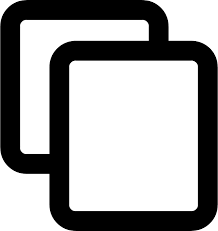
const UserSchema = new mongoose.Schema({
name: String,
email: String,
password: String,
});
const User = mongoose.model('User', UserSchema);
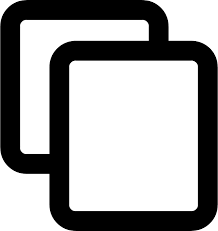
app.post('/users', async (req, res) => {
const newUser = new User(req.body);
try {
const savedUser = await newUser.save();
res.status(201).json(savedUser);
} catch (err) {
res.status(400).json({ message: err.message });
}
});
app.get('/users', async (req, res) => {
try {
const users = await User.find();
res.json(users);
} catch (err) {
res.status(500).json({ message: err.message });
}
});
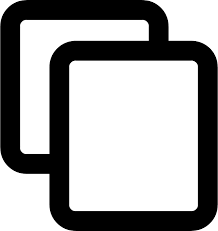
app.listen(5000, () => {
console.log('Server is running on port 5000');
});
Example: Building a Simple User Management API
Let’s walk through a simple example of building an API for managing users with MongoDB and Express.js:
- Create a new Express app.
- Set up Mongoose to connect to a local MongoDB database.
- Define a Mongoose schema and model for the user.
- Create routes to add, retrieve, and update users.
- Test the API using tools like Postman or CURL.
Here is an example of what the API could look like:
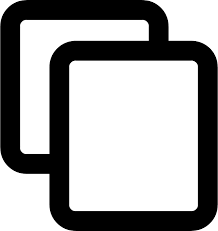
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect('mongodb://localhost:27017/usersdb', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch((err) => console.log('MongoDB connection error:', err));
const UserSchema = new mongoose.Schema({
name: String,
email: { type: String, unique: true },
password: String,
});
const User = mongoose.model('User', UserSchema);
// Route to create a new user
app.post('/users', async (req, res) => {
const newUser = new User(req.body);
try {
const savedUser = await newUser.save();
res.status(201).json(savedUser);
} catch (err) {
res.status(400).json({ message: err.message });
}
});
// Route to fetch all users
app.get('/users', async (req, res) => {
try {
const users = await User.find();
res.json(users);
} catch (err) {
res.status(500).json({ message: err.message });
}
});
// Start the server
app.listen(5000, () => {
console.log('Server running on http://localhost:5000');
});
Using MongoDB with Express.js in Production
When deploying your MERN stack application to production, you'll need to consider additional aspects such as:
- Environment Variables: Store sensitive information like database credentials and server URLs in environment variables rather than hard-coding them into your application.
- Security Best Practices: Implement measures like input validation, JWT authentication, and HTTPS to secure your API endpoints.
- Scaling and Load Balancing: As your application grows, consider using sharding in MongoDB to distribute data across multiple servers and handle high traffic volumes efficiently.
- Monitoring and Logging: Use monitoring tools like MongoDB Atlas, Prometheus, or Grafana to track your application's performance and health. Implement logging to keep track of errors and debug issues.
Conclusion
Integrating MongoDB with Express.js in the MERN stack provides an efficient and scalable architecture for developing modern web applications. With MongoDB's flexibility and Express.js's lightweight framework, you can build powerful APIs that handle data storage, retrieval, and manipulation seamlessly. Whether you're building simple applications or complex data-driven platforms, MongoDB and Express.js are an excellent choice for backend development in the MERN stack.
MongoDB with GraphQL
GraphQL is a powerful query language for APIs and runtime for executing those queries by using a type system you define for your data. MongoDB, a NoSQL database, can integrate seamlessly with GraphQL to provide a flexible and efficient way to query and manipulate data. This section will cover how to set up a GraphQL server with MongoDB, the benefits of using GraphQL with MongoDB, and how to build GraphQL queries that interact with MongoDB collections.
What is GraphQL?
GraphQL is a data query language developed by Facebook that allows clients to request exactly the data they need and nothing more. Unlike REST APIs, which expose fixed endpoints for each resource, GraphQL exposes a single endpoint that can handle all types of queries, mutations, and subscriptions. It allows clients to specify the structure of the response they need, providing more flexibility and efficiency in data fetching.
Why Use MongoDB with GraphQL?
Integrating MongoDB with GraphQL allows you to combine the flexibility of MongoDB's schema-less structure with the power of GraphQL's declarative query language. Here are some reasons to consider using MongoDB with GraphQL:
- Flexible Data Representation: MongoDB's dynamic schema allows storing data in JSON-like documents, which pairs well with GraphQL's flexible query capabilities.
- Efficient Data Fetching: GraphQL allows clients to request only the data they need, reducing over-fetching and improving performance.
- Single Endpoint for All Operations: GraphQL provides a single endpoint for queries, mutations, and subscriptions, simplifying API design.
- Real-time Capabilities: GraphQL supports subscriptions, allowing for real-time data updates over WebSocket connections.
Setting Up MongoDB with GraphQL
To integrate MongoDB with GraphQL, you need to set up a GraphQL server and connect it to MongoDB. Here’s a step-by-step guide:
- Install Dependencies: First, you need to install the required libraries for Express, MongoDB, GraphQL, and Mongoose:
- Set up Mongoose and MongoDB Connection: Use Mongoose to connect to your MongoDB database:
- Define a Mongoose Model: Define a Mongoose schema and model for the data you want to query through GraphQL. For example, let’s create a simple model for a "User":
- Set up GraphQL Schema: Define the GraphQL schema, including types, queries, and mutations. The schema should specify the operations available for interacting with MongoDB:
- Set up GraphQL Server: Use the
express-graphql
package to create a GraphQL endpoint that will handle all GraphQL queries:
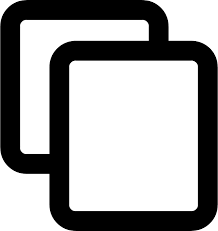
npm install express mongoose graphql express-graphql
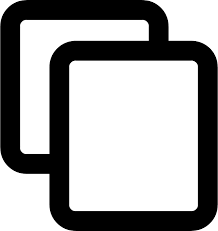
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/graphql_db', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch((err) => console.log('MongoDB connection error:', err));
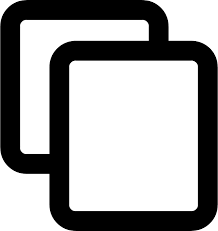
const UserSchema = new mongoose.Schema({
name: String,
email: String,
age: Number,
});
const User = mongoose.model('User', UserSchema);
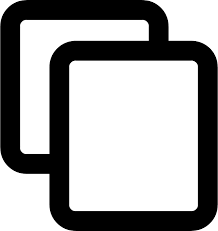
const { GraphQLObjectType, GraphQLSchema, GraphQLString, GraphQLInt } = require('graphql');
const UserType = new GraphQLObjectType({
name: 'User',
fields: () => ({
id: { type: GraphQLString },
name: { type: GraphQLString },
email: { type: GraphQLString },
age: { type: GraphQLInt }
})
});
const RootQuery = new GraphQLObjectType({
name: 'RootQueryType',
fields: {
user: {
type: UserType,
args: { id: { type: GraphQLString } },
resolve(parent, args) {
return User.findById(args.id);
}
}
}
});
const Mutation = new GraphQLObjectType({
name: 'Mutation',
fields: {
addUser: {
type: UserType,
args: {
name: { type: GraphQLString },
email: { type: GraphQLString },
age: { type: GraphQLInt },
},
resolve(parent, args) {
const newUser = new User({
name: args.name,
email: args.email,
age: args.age,
});
return newUser.save();
}
}
}
});
const schema = new GraphQLSchema({
query: RootQuery,
mutation: Mutation
});
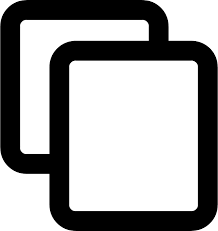
const express = require('express');
const graphqlHTTP = require('express-graphql');
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
graphiql: true, // Enable GraphiQL interface for testing queries
}));
app.listen(4000, () => {
console.log('Server running on http://localhost:4000/graphql');
});
Example: Querying and Mutating Data with GraphQL
Once your server is set up, you can interact with MongoDB through GraphQL queries and mutations:
Query Example
To fetch a user by ID:
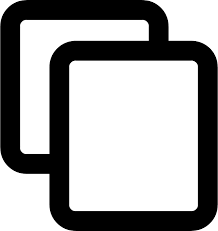
query {
user(id: "5f8d0d55b54764421b7156e7") {
id
name
email
age
}
}
Mutation Example
To add a new user to MongoDB:
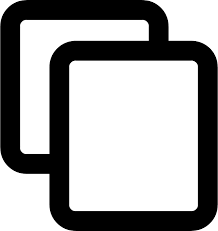
mutation {
addUser(name: "John Doe", email: "john.doe@example.com", age: 30) {
id
name
email
age
}
}
Real-time Data with GraphQL Subscriptions
GraphQL supports subscriptions, which allow clients to receive real-time updates about data changes. This is particularly useful for applications such as chat apps, live updates, and notifications. With MongoDB, you can use subscriptions to notify clients whenever data changes, for example, when a new user is added:
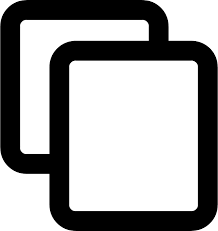
const { PubSub } = require('graphql-subscriptions');
const pubsub = new PubSub();
// Inside the mutation to add a user
pubsub.publish('USER_ADDED', { userAdded: newUser });
// In the subscription resolver
const Subscription = {
userAdded: {
subscribe: () => pubsub.asyncIterator(['USER_ADDED'])
}
};
Best Practices for Using MongoDB with GraphQL
- Use Mongoose to Define Models: Mongoose provides an elegant way to define your data models, ensuring that data interacts correctly with MongoDB.
- Optimize Queries: Use filtering, pagination, and limiting in your GraphQL queries to optimize performance and reduce the load on the server.
- Secure Your API: Implement authorization and authentication for your GraphQL API to ensure that only authorized users can access or modify data.
- Handle Errors Gracefully: Make sure to handle errors in your GraphQL resolvers and provide meaningful error messages to clients.
Conclusion
MongoDB and GraphQL together provide a powerful combination for building flexible, efficient, and scalable APIs. By using GraphQL with MongoDB, you can take advantage of MongoDB's flexible schema and GraphQL's declarative query language to create modern, data-driven applications. Whether you're building a simple CRUD application or a complex real-time platform, the MongoDB and GraphQL stack can meet the needs of a variety of use cases.
MongoDB and Redis for Caching
Using Redis alongside MongoDB can greatly improve the performance and scalability of your application by adding a caching layer. Redis is an in-memory data store that can be used to cache frequently accessed data, reducing the load on MongoDB and speeding up data retrieval. This section will explore how to integrate Redis with MongoDB for caching purposes, the benefits of caching, and how to implement an effective caching strategy.
What is Redis?
Redis is an open-source, in-memory data structure store that is widely used as a caching solution. It supports various data structures such as strings, hashes, lists, sets, and more. Redis is known for its speed and efficiency because it stores data in memory, making it much faster than traditional disk-based databases.
Why Use MongoDB and Redis Together?
MongoDB provides a flexible, scalable, and persistent data store, but it may not always provide the fastest performance for frequently queried data. Redis, being an in-memory data store, can act as a caching layer between your application and MongoDB to speed up data access. Here are the key benefits of using MongoDB and Redis together:
- Improved Performance: Redis can cache frequently accessed data, reducing the number of database queries to MongoDB and decreasing response times.
- Reduced Load on MongoDB: By caching results in Redis, you reduce the load on MongoDB, allowing it to handle more complex queries and operations without being overwhelmed.
- Scalability: Redis provides horizontal scalability, allowing you to easily add more Redis nodes to handle larger volumes of cached data.
- Cost Efficiency: Redis is a low-cost way to speed up data retrieval, as you can avoid expensive database queries by serving data from memory.
Setting Up Redis with MongoDB
To integrate Redis with MongoDB, you'll first need to set up both Redis and MongoDB servers. Then, you’ll implement a caching layer in your application that checks Redis first for cached data and falls back to MongoDB if the data is not found in Redis.
Step 1: Install Redis and MongoDB
Ensure that both Redis and MongoDB are installed and running on your system. You can install Redis using the following command:
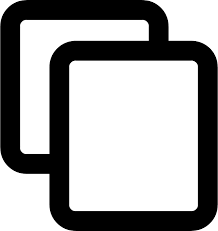
sudo apt-get install redis-server
MongoDB installation can be done from the official MongoDB website or using package managers based on your operating system.
Step 2: Install Redis and MongoDB Client Libraries
In your Node.js application, install the Redis and MongoDB client libraries:
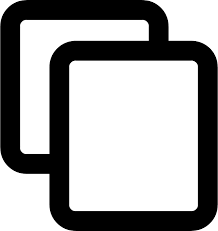
npm install redis mongoose
Step 3: Set Up Redis and MongoDB Connections
Set up connections for both Redis and MongoDB in your application:
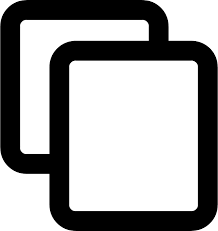
const redis = require('redis');
const mongoose = require('mongoose');
const redisClient = redis.createClient({ host: 'localhost', port: 6379 });
const mongoURI = 'mongodb://localhost:27017/mydb';
mongoose.connect(mongoURI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch((err) => console.log('MongoDB connection error:', err));
Implementing Caching Logic
Now that Redis and MongoDB are set up, you can implement caching logic in your application. The basic idea is to first check if the data exists in Redis. If it does, return it from the cache. If it doesn’t, retrieve the data from MongoDB, store it in Redis, and then return it to the client.
Step 4: Caching Data
Here’s how you can implement a function to fetch data from MongoDB and cache it in Redis:
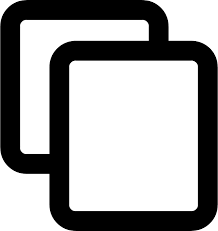
const getData = async (key) => {
// Check if data exists in Redis
redisClient.get(key, async (err, data) => {
if (err) throw err;
if (data) {
// Return data from cache
console.log('Cache hit');
return JSON.parse(data);
} else {
// Fetch data from MongoDB
console.log('Cache miss');
const result = await MyModel.findOne({ _id: key });
// Cache the result in Redis
redisClient.setex(key, 3600, JSON.stringify(result)); // Cache for 1 hour
return result;
}
});
};
Step 5: Storing Data in MongoDB
If you need to store data in MongoDB after a cache miss, you can also update the Redis cache after inserting or updating data in MongoDB. This ensures that the cache is always up to date:
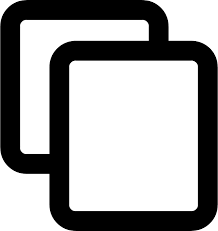
const saveData = async (key, newData) => {
// Save data to MongoDB
const result = await MyModel.updateOne({ _id: key }, newData, { upsert: true });
// Update cache in Redis
redisClient.setex(key, 3600, JSON.stringify(result));
return result;
};
Cache Expiration and Invalidations
One important aspect of caching is managing cache expiration and invalidation. Redis provides built-in support for setting expiration times on cached data, ensuring that stale data is automatically removed. You can use the setex
function to set an expiration time when storing data in Redis, as shown earlier. Additionally, you can manually remove or update the cache when data in MongoDB changes.
Example: Cache Invalidation
When updating data in MongoDB, you should also invalidate or update the corresponding cached data in Redis:
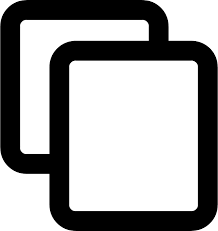
const updateCache = (key, newData) => {
// Update data in MongoDB
MyModel.updateOne({ _id: key }, newData, (err, result) => {
if (err) throw err;
// Invalidate cache
redisClient.del(key, (err) => {
if (err) throw err;
// Optionally, re-cache the updated data
redisClient.setex(key, 3600, JSON.stringify(result));
});
});
};
Best Practices for MongoDB and Redis Caching
- Cache Frequently Accessed Data: Cache the data that is requested often and does not change frequently. This can include user profiles, product listings, or search results.
- Set Appropriate Expiration Times: Cache data with appropriate expiration times to avoid serving stale data. For frequently updated data, choose shorter cache times.
- Handle Cache Misses Efficiently: Design your application to handle cache misses gracefully by falling back to MongoDB and caching the result for subsequent requests.
- Invalidate Cache on Data Changes: Ensure that the cache is invalidated or updated when the underlying data in MongoDB changes to maintain consistency.
Conclusion
By combining MongoDB and Redis, you can significantly improve the performance of your application. Redis serves as a fast, in-memory cache for frequently accessed data, while MongoDB provides a persistent data store. This combination allows for faster data retrieval, reduced load on MongoDB, and better scalability for high-traffic applications. Implementing a caching layer with Redis can be an effective way to optimize your MongoDB-based application.
Common Errors and Solutions in MongoDB
When working with MongoDB, you may encounter some common errors or issues. Understanding these errors and how to resolve them is crucial for maintaining the health and performance of your database. This section covers some of the most frequent MongoDB errors and offers solutions to troubleshoot and fix them.
1. MongoDB Connection Errors
Issue: Unable to connect to MongoDB server.
Solution: This error can occur for a variety of reasons, such as incorrect connection strings or issues with the MongoDB server itself. Here are a few solutions:
- Verify that MongoDB is running on the correct host and port. By default, MongoDB runs on
localhost:27017>.
- Ensure that your connection string is correctly formatted and includes the correct credentials (if authentication is enabled).
- Check your firewall settings to ensure that the MongoDB port is not blocked.
- If you're using MongoDB Atlas, verify that your IP address is whitelisted in the Atlas network settings.
2. Authentication Errors
Issue: Authentication failed, incorrect username/password.
Solution: This error occurs when the provided credentials do not match the ones stored in MongoDB. To resolve it:
- Double-check the username and password you're using to authenticate.
- If you're using MongoDB Atlas, ensure that you have set up a user with the correct roles and permissions.
- If you have recently changed your password, ensure that your application is using the updated credentials.
- Ensure that the authentication database is specified correctly in the connection string (e.g.,
authSource
parameter in MongoDB URI).
3. Replica Set Connection Issues
Issue: Unable to connect to a MongoDB replica set.
Solution: This error typically occurs when MongoDB cannot find or connect to the replica set members. Solutions include:
- Ensure that all replica set members are running and are reachable from the client.
- Check the replica set configuration with
rs.status()
in the MongoDB shell to verify the status of each member. - If the replica set configuration has been changed recently, restart all replica set members to apply the new configuration.
- Verify that the
replSet
parameter is correctly configured in the MongoDB connection string.
4. Out of Memory Errors
Issue: MongoDB processes consume excessive memory or crash due to memory limits.
Solution: Out of memory errors can be caused by large queries or insufficient system resources. To resolve this:
- Optimize your queries to reduce memory consumption by using indexes, limiting the number of returned documents, and using pagination.
- Ensure that your system has sufficient RAM to handle the size of the MongoDB dataset.
- Adjust the
wiredTigerCacheSizeGB
parameter in the MongoDB configuration file to control how much memory MongoDB uses for its cache. - Monitor memory usage with tools like
mongostat
ortop
to identify potential issues.
5. Duplicate Key Errors
Issue: Attempting to insert a document with a duplicate value for a unique field.
Solution: MongoDB will throw an error if you try to insert a document with a duplicate value for a field that has a unique index. Here's how to solve it:
- Ensure that your application logic prevents inserting documents with duplicate values into fields that require uniqueness (e.g., user emails, product SKUs).
- Check the index definition to ensure the unique constraint is applied to the correct field.
- If you need to insert a document with a duplicate value, consider removing the unique index or adjusting your schema design.
6. Timeout Errors
Issue: MongoDB query or connection times out.
Solution: Timeout errors can happen when MongoDB is unable to complete a query or establish a connection within the specified time limit. To troubleshoot:
- Ensure that the MongoDB server is not overloaded and is responding to requests in a timely manner.
- Increase the connection timeout period in your MongoDB connection string using the
connectTimeoutMS
parameter. - Check for network latency or firewall issues that could be slowing down the connection.
- Optimize your queries to reduce the time it takes for MongoDB to execute them, such as by adding appropriate indexes.
7. Index Errors
Issue: Errors related to creating or using indexes.
Solution: Index-related errors can occur when there are issues with creating or using indexes in MongoDB. To resolve:
- Ensure that your index definitions are correct and match the fields used in your queries.
- Check for duplicate or conflicting indexes by running
db.collection.getIndexes()
. - If you're facing issues with index creation, check for sufficient disk space or resource constraints on the server.
8. Disk Space Errors
Issue: MongoDB runs out of disk space.
Solution: Running out of disk space can cause MongoDB to stop functioning properly. To fix this:
- Monitor disk usage regularly using system tools like
df
or MongoDB'sdb.stats()
. - Increase disk space on the server or move the data files to a larger disk.
- Consider enabling data compression to reduce the disk space used by your MongoDB data files.
- Regularly archive or delete old data that is no longer necessary.
9. Unresponsive MongoDB Server
Issue: MongoDB becomes unresponsive or crashes unexpectedly.
Solution: Unresponsiveness or crashes can result from resource exhaustion, misconfiguration, or bugs. Here are some steps to resolve it:
- Check MongoDB logs for error messages or stack traces that might indicate the cause of the issue.
- Ensure that the server has adequate resources (CPU, RAM, disk space) to handle MongoDB's workload.
- Upgrade MongoDB to the latest stable version to avoid bugs or performance issues that have been fixed in newer releases.
- Consider implementing resource limits or sharding to distribute the load across multiple servers if needed.
Conclusion
MongoDB is a powerful and flexible database, but like any system, it can encounter issues. Understanding common errors and their solutions can help you quickly diagnose and resolve problems, ensuring that your MongoDB setup runs smoothly. Regular monitoring, efficient query design, and proper resource management are key to minimizing errors and maintaining a healthy MongoDB environment.
Debugging Performance Issues in MongoDB
Performance issues in MongoDB can arise due to a variety of factors, including inefficient queries, lack of proper indexing, hardware limitations, or even misconfiguration. In this section, we'll explore how to debug common performance issues and optimize MongoDB for better performance.
1. Monitor System Resources
Issue: MongoDB is consuming excessive CPU, memory, or disk resources, leading to poor performance.
Solution: The first step in debugging performance issues is to monitor system resources:
- Use tools like
top
,htop
, ormongostat
to monitor MongoDB's resource usage. - If MongoDB is consuming too much CPU or memory, check if there are queries or operations that are using more resources than expected.
- If disk I/O is high, ensure that your disk has sufficient speed and space for MongoDB's data files.
2. Check Slow Queries
Issue: Certain queries are running slowly and affecting performance.
Solution: MongoDB provides a slowOpThresholdMs
parameter to log slow queries. To identify slow queries:
- Enable the
slowOpThresholdMs
parameter to log queries that take longer than a specified time (e.g., 100ms). - Use
db.currentOp()
in the MongoDB shell to view currently running operations and identify long-running queries. - Analyze the slow query logs and review the query execution plans using
explain()
to identify possible optimizations.
3. Analyze Query Execution Plans
Issue: Queries are not performing as expected, potentially due to missing indexes or inefficient query patterns.
Solution: MongoDB provides the explain()
method to analyze how queries are executed:
- Run queries with
explain()
to obtain detailed information about how MongoDB plans to execute them. - Look for stages in the execution plan that may be inefficient, such as collection scans or sorting without an index.
- If necessary, create indexes to optimize the query performance, especially for fields involved in filtering or sorting.
4. Ensure Proper Indexing
Issue: MongoDB queries are slow due to missing or inefficient indexes.
Solution: Indexes are crucial for fast query performance. Here's how to ensure proper indexing:
- Use
db.collection.getIndexes()
to list all existing indexes and verify if they support your queries. - Create indexes on fields that are frequently queried or used in sorting operations.
- Review compound indexes for queries that filter on multiple fields.
- Use the
indexStats
command to check the efficiency of each index. - Ensure that indexes are not too large or causing performance overhead for write operations.
5. Review Aggregation Pipeline Performance
Issue: Aggregation pipelines are taking too long to execute.
Solution: Aggregation operations can be resource-intensive. To improve aggregation performance:
- Use the
$match
stage early in the pipeline to filter out unnecessary documents. - Use
$project
to exclude unnecessary fields from the pipeline to reduce memory usage. - Make sure to use indexes for filtering and sorting in aggregation pipelines, especially with
$match
and$sort
stages. - Use
explain()
on the aggregation pipeline to check its execution plan and identify bottlenecks.
6. Optimize Write Operations
Issue: Write operations are too slow or causing performance issues.
Solution: Write performance issues can be caused by factors such as unoptimized writes, large documents, or high write load. To optimize write operations:
- Batch write operations to reduce the number of requests to the server.
- Use
writeConcern
appropriately to balance consistency and performance. For example, usewriteConcern: "majority"
only when necessary. - Consider using the
bulkWrite()
method to perform multiple write operations in a single request. - Ensure that the documents being written are not too large, as large documents can slow down write performance.
7. Leverage Caching
Issue: Repeated queries are affecting performance due to high load on MongoDB.
Solution: Caching repeated query results can reduce the load on MongoDB and improve response times:
- Use an external caching layer like Redis or Memcached to cache frequently accessed data.
- Implement caching at the application layer for common queries that do not change often.
- Cache aggregation results or complex queries that involve multiple stages.
- Ensure that caches are invalidated appropriately when underlying data changes.
8. Monitor Logs and Profiling
Issue: Performance issues are hard to trace without detailed logging and profiling.
Solution: MongoDB provides several tools for logging and profiling performance issues:
- Enable MongoDB profiling to log queries that exceed a certain threshold (e.g.,
profilingLevel
andslowOpThresholdMs
). - Use
db.getProfilingStatus()
anddb.system.profile
to view profiling data and identify slow queries. - Review MongoDB logs for errors, warnings, and other performance-related information.
9. Check for Resource Contention
Issue: Performance bottlenecks due to resource contention (e.g., CPU, memory, I/O).
Solution: Resource contention can arise from other processes or services competing for system resources. To resolve it:
- Monitor the server's overall resource usage to identify any competing processes that may be using too much CPU or memory.
- Ensure that MongoDB has adequate resources (e.g., CPU, RAM) for the workload it is handling.
- Consider moving MongoDB to a dedicated server or separating MongoDB from other resource-intensive processes.
- Use
mongostat
to monitor MongoDB's internal operations and identify any areas of contention.
10. Scale MongoDB for Better Performance
Issue: MongoDB is struggling to handle increasing load or data volume.
Solution: If your database is under heavy load, scaling MongoDB horizontally or vertically can improve performance:
- Consider deploying a replica set to improve read scalability and redundancy.
- Use sharding to distribute data across multiple servers for horizontal scaling, particularly for large datasets.
- Monitor shard distribution and ensure that the data is evenly distributed across the shards.
- If necessary, scale up by upgrading the hardware (e.g., more RAM, better disks) to improve MongoDB's performance.
Conclusion
Debugging performance issues in MongoDB requires a systematic approach to identify bottlenecks and optimize queries, indexing, and system resources. By monitoring performance, analyzing query execution plans, and applying best practices, you can ensure that MongoDB performs efficiently, even under heavy loads. Regularly profile your database, optimize write operations, and scale your infrastructure as needed to maintain optimal performance.
Handling Large Data Sets Efficiently in MongoDB
Handling large data sets efficiently in MongoDB requires a combination of proper schema design, indexing strategies, and optimized queries. In this section, we'll explore best practices for managing large data volumes while ensuring optimal performance.
1. Use Indexing to Optimize Queries
Issue: Queries on large data sets can become slow if they are not properly indexed.
Solution: Indexing is crucial for fast data retrieval, especially with large data sets. To optimize queries:
- Use
db.collection.getIndexes()
to check the existing indexes and ensure that the most frequently queried fields are indexed. - Create compound indexes for queries that filter on multiple fields.
- For large datasets, make use of
TTL (Time-To-Live)
indexes for automatically expiring old data, reducing the data size over time. - Ensure that indexes are covering the fields used in the query to avoid full collection scans.
2. Use Pagination to Handle Large Result Sets
Issue: Returning large result sets in a single query can overload both the server and the client.
Solution: Pagination allows for breaking large result sets into smaller, more manageable chunks:
- Implement pagination with the
skip()
andlimit()
methods to return subsets of data instead of the entire dataset. - Use
find()
to retrieve data in smaller chunks to reduce memory and CPU load on MongoDB and the client. - Consider using
range queries
(e.g., date ranges) as an alternative toskip()
for better performance with large datasets.
3. Shard Your Data
Issue: A single MongoDB instance may struggle to handle large data sets due to resource limitations.
Solution: Sharding distributes data across multiple servers, allowing MongoDB to handle larger data sets more effectively:
- Enable sharding on your collection by selecting an appropriate shard key.
- Ensure that the shard key distributes data evenly across shards to avoid hotspots (uneven data distribution).
- Monitor the distribution of data across shards with
sh.status()
to ensure that the system is balanced. - Scale out by adding more shards as the dataset grows to improve performance and storage capacity.
4. Optimize Data Model for Large Data
Issue: A poorly designed data model can lead to inefficiencies when dealing with large data sets.
Solution: Design your data model to minimize data duplication and optimize for read-heavy or write-heavy workloads:
- Use embedded documents when related data is often accessed together to avoid costly joins.
- Use references (or
DBRefs
) when data is accessed separately and should remain normalized. - Avoid storing large binary data (e.g., images or videos) directly in MongoDB. Use GridFS for managing large files.
- Consider using the
aggregation framework
to process data efficiently instead of loading large amounts of data into memory for post-processing.
5. Use Compression and Storage Optimization
Issue: Large data sets consume significant storage space, especially if the data is not compressed.
Solution: MongoDB provides several ways to optimize storage for large data sets:
- Enable
WiredTiger
storage engine with compression for data at rest. - Use
zlib
compression to reduce the storage footprint of documents. - Periodically run
compact
to reclaim disk space and optimize storage. - Consider archiving or deleting old data if it is no longer necessary for operational purposes.
6. Use Bulk Write Operations
Issue: Writing large amounts of data can be inefficient if done in many individual operations.
Solution: MongoDB supports bulk write operations, which allow you to perform multiple write operations in a single request:
- Use
bulkWrite()
to perform multiple insert, update, and delete operations in a single batch, reducing network overhead. - Batch your writes into manageable chunks to avoid overwhelming MongoDB with too many requests.
- Optimize the
writeConcern
for bulk operations based on your consistency requirements (e.g., setwriteConcern
to1
for improved performance in non-critical operations).
7. Monitor and Profile Large Data Queries
Issue: Certain queries may cause performance issues due to inefficient operations on large data sets.
Solution: Use MongoDB's profiling and monitoring tools to identify slow queries and optimize them:
- Enable query profiling with
db.setProfilingLevel()
to log slow queries and analyze their execution times. - Use
explain()
to analyze query plans and identify inefficiencies like full collection scans or missing indexes. - Use
mongostat
andmongotop
to monitor the overall performance of the MongoDB instance.
8. Use Data Archiving Strategies
Issue: Large data sets can become unwieldy over time, leading to storage issues and slower queries.
Solution: Implement data archiving strategies to move older or less frequently accessed data to separate storage:
- Use
TTL (Time-To-Live)
indexes to automatically remove documents that are no longer needed. - Periodically archive old data to a different MongoDB instance or external storage, using batch processing to maintain optimal performance.
- Consider using a dedicated archival system for data that is rarely accessed but still needs to be stored long-term.
9. Optimize Read Performance with Caching
Issue: Frequent queries on large datasets may result in high read load, slowing down performance.
Solution: Caching frequently accessed data can reduce the load on MongoDB and improve response times:
- Implement external caching systems like Redis or Memcached to store the results of frequently queried data.
- Cache results of expensive aggregation operations or large result sets to avoid querying MongoDB repeatedly.
- Ensure that cached data is invalidated when the underlying data changes to keep the cache up-to-date.
10. Scale MongoDB for High Volume Data
Issue: MongoDB struggles to handle large volumes of data due to hardware limitations.
Solution: When handling high-volume data, scaling horizontally or vertically is essential:
- Use sharding to horizontally scale MongoDB by distributing data across multiple servers.
- Scale up by upgrading the hardware (e.g., more RAM, faster disks) to handle larger data volumes.
- Monitor the system's performance regularly and add more resources as needed to maintain optimal performance.
Conclusion
Handling large data sets in MongoDB requires a combination of strategic data modeling, indexing, sharding, and efficient querying. By following best practices such as using pagination, leveraging bulk operations, and optimizing read and write performance, you can ensure that MongoDB remains scalable and efficient as your data grows. Regularly monitoring and profiling your system will help you identify and address performance bottlenecks, ensuring that MongoDB can handle large data sets effectively.