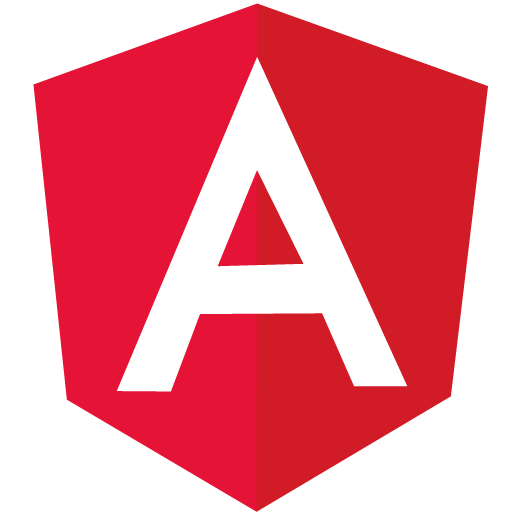
What is Angular?
1. Introduction to Angular
Angular is a popular open-source web application framework developed by Google. It is a TypeScript-based framework used for building dynamic, single-page web applications (SPAs). Angular simplifies the development process by offering tools and features to manage data binding, routing, and application structure.
2. Key Features of Angular
Angular offers several features that make it a powerful and flexible framework:
- Two-Way Data Binding: Automatically synchronizes data between the model and the view.
- Dependency Injection: Simplifies the management of dependencies and services in an application.
- Directives: Extend the HTML syntax to create reusable components and dynamic templates.
- Routing: Enables navigation between different views and modules in a single-page application.
- Modular Architecture: Supports the creation of reusable and maintainable code with modules.
3. Why Use Angular?
Angular is widely used for its ability to build scalable and robust applications. It is particularly beneficial for:
- Creating dynamic, interactive web applications.
- Building enterprise-level applications with complex requirements.
- Ensuring a consistent development experience across teams.
4. Example: Basic Angular Component
Here’s a simple example of an Angular component:
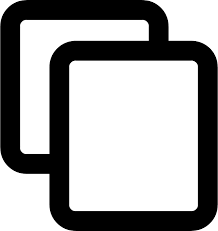
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>Welcome to Angular</h1>`,
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular Tutorial';
}
In this example, the component defines a selector
that acts as a custom HTML tag (app-root
). The template contains the view logic, and styles can be applied using the specified CSS file.
5. Conclusion
Angular is a comprehensive framework that provides developers with a structured and efficient approach to building web applications. With its rich set of features, Angular has become a preferred choice for developers and organizations aiming to create modern, scalable, and maintainable applications.
Features of Angular
1. Two-Way Data Binding
Two-way data binding in Angular ensures that the view and the model are always in sync. When the user updates the view, the model changes automatically and vice versa. This feature reduces the amount of boilerplate code and simplifies handling user input.
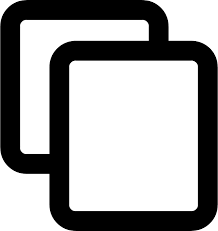
<input [(ngModel)]="name" placeholder="Enter your name">
<p>Hello, {{ name }}!</p>
2. Dependency Injection
Angular's dependency injection system allows developers to inject services into components, making the application more modular and testable. This feature simplifies the management of service instances and their lifecycle.
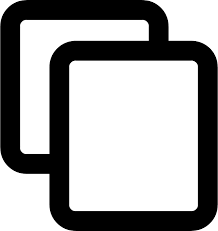
// Example of Dependency Injection
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
getData() {
return "Data from service";
}
}
// Injecting the service into a component
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
template: `<p>{{ data }}</p>`
})
export class AppComponent {
data: string;
constructor(private dataService: DataService) {
this.data = dataService.getData();
}
}
3. Directives
Angular provides structural and attribute directives to manipulate the DOM. Structural directives like *ngIf
and *ngFor
are used to add or remove elements dynamically, while attribute directives like ngClass
and ngStyle
change the appearance of an element.
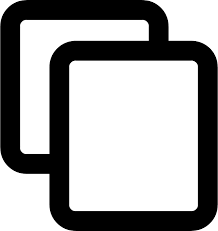
<div *ngIf="isVisible">This text is conditionally displayed.</div>
<p [ngStyle]="{'color': 'blue'}">This text is styled dynamically.</p>
4. Routing
Angular's routing module allows developers to define navigation paths in single-page applications. It provides seamless transitions between different views.
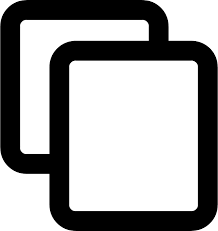
// Example of Routing Configuration
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
5. Modular Architecture
Angular applications are built using a modular structure, where the code is divided into modules that encapsulate components, directives, services, and other resources. This modularity makes the application scalable and maintainable.
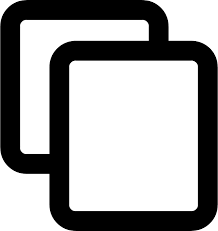
// Example of Angular Module
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
6. Conclusion
Angular's rich set of features makes it a powerful framework for building modern web applications. Its built-in tools like two-way data binding, dependency injection, directives, routing, and modular architecture provide developers with everything needed to create dynamic and scalable applications efficiently.
Advantages of Angular
1. Two-Way Data Binding
Angular's two-way data binding synchronizes the view and the model seamlessly. This reduces boilerplate code and ensures that any changes in the UI are immediately reflected in the application logic, and vice versa.
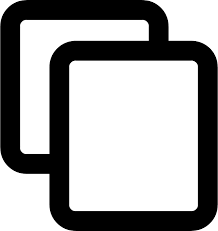
<input [(ngModel)]="userInput" placeholder="Enter text">
<p>You typed: {{ userInput }}</p>
2. Modular Architecture
Angular's modular architecture allows developers to organize code into modules, making applications scalable and easier to maintain. This structure supports lazy loading, which optimizes application performance by loading modules only when needed.
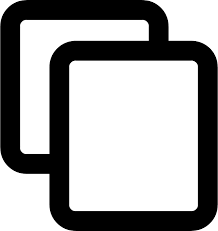
// Example of Lazy Loading a Module
const routes: Routes = [
{ path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) }
];
3. Dependency Injection
Angular’s built-in dependency injection system helps manage service instances and their dependencies efficiently. This makes the application easier to test and maintain.
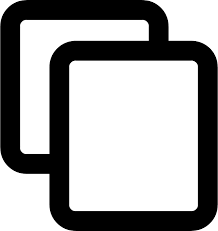
// Example of a Service with Dependency Injection
@Injectable({
providedIn: 'root'
})
export class AuthService {
constructor(private http: HttpClient) {}
login(credentials: any) {
return this.http.post('/api/login', credentials);
}
}
4. High Performance
Angular applications are optimized for high performance with features like Ahead-of-Time (AOT) compilation, which reduces runtime errors and speeds up the application by compiling templates during the build phase.
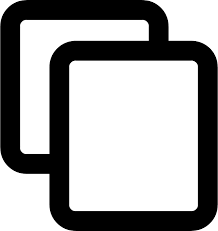
// Enabling AOT in Angular
{
"compilerOptions": {
"enableIvy": true
}
}
5. Cross-Platform Development
Angular allows developers to create applications for web, mobile, and desktop platforms using a single codebase. With Angular Universal, server-side rendering is also supported, improving SEO and load times.
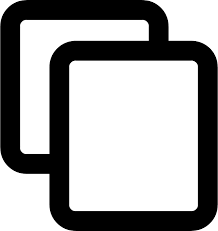
// Example of Angular Universal Server Module
@NgModule({
imports: [
ServerModule,
AppModule
],
bootstrap: [AppComponent]
})
export class AppServerModule {}
6. Strong Community Support
Angular has a vast community of developers and a robust ecosystem. This makes finding solutions, learning resources, and third-party tools easier for developers.
7. Comprehensive Framework
Angular provides a complete solution for application development with built-in tools for routing, state management, HTTP client, and form handling. This reduces the need for external libraries and ensures a consistent development experience.
8. Conclusion
Angular’s extensive feature set and advantages make it a preferred framework for building large-scale, dynamic, and maintainable applications. Its modularity, performance optimization, and wide ecosystem support make it ideal for both enterprise and individual projects.
Angular vs. Other Frameworks
1. Overview
Angular stands out among other frameworks like React and Vue due to its comprehensive nature, offering a complete solution for application development. Here’s a detailed comparison of Angular with other popular frameworks.
2. Angular vs. React
React is a library for building user interfaces, while Angular is a complete framework for developing robust applications. Key differences include:
- Two-Way Binding: Angular provides two-way data binding natively, while React relies on external libraries like Redux for state management.
- Learning Curve: Angular has a steeper learning curve due to its extensive features, while React is easier to get started with.
- Performance: Angular uses Ahead-of-Time (AOT) compilation for optimized performance, while React uses a virtual DOM for efficient updates.
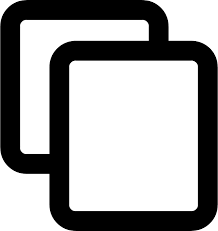
<!-- React Example: Component State Management -->
function App() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
3. Angular vs. Vue.js
Vue.js is known for its simplicity and flexibility, while Angular offers a more opinionated and structured approach. Key differences include:
- Flexibility: Vue allows for more flexible development practices, while Angular enforces strict coding patterns.
- Community Support: Angular has a larger and more established community compared to Vue.
- Tooling: Angular provides an extensive CLI, while Vue's tooling is simpler but less feature-rich.
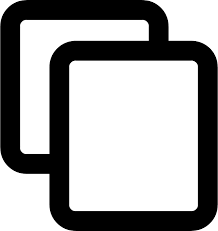
<!-- Vue Example: Two-Way Data Binding -->
<div id="app">
<input v-model="message" placeholder="Enter text">
<p>{{ message }}</p>
</div>
<script>
new Vue({
el: '#app',
data: {
message: ''
}
});
</script>
4. Angular vs. Svelte
Svelte is a relatively new framework that compiles components into efficient JavaScript at build time. Key differences include:
- Performance: Svelte is faster due to its compile-time optimization, while Angular provides runtime features that add overhead.
- Features: Angular includes a comprehensive suite of tools, whereas Svelte focuses on simplicity and minimalism.
- Community: Angular has a well-established ecosystem, while Svelte is gaining popularity but has a smaller community.
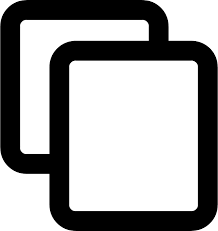
<!-- Svelte Example: Counter Component -->
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<button on:click={increment}>Count: {count}</button>
5. Conclusion
Angular excels as a comprehensive framework suitable for enterprise-level applications, while frameworks like React, Vue, and Svelte cater to different needs such as simplicity, flexibility, and performance. Choosing the right framework depends on the project requirements, team expertise, and long-term goals.
Setting up Angular CLI
1. Introduction
The Angular CLI (Command Line Interface) is a powerful tool for creating, building, and managing Angular projects. It simplifies the development process by providing commands to generate components, services, and more. This guide covers the steps to set up Angular CLI and start your first Angular project.
2. Prerequisites
Ensure that you have the following installed on your system:
- Node.js (version 14.x or higher) and npm (Node Package Manager)
- A code editor (e.g., Visual Studio Code)
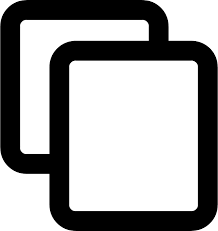
# Check Node.js and npm versions
node -v
npm -v
3. Installing Angular CLI
Use npm to install the Angular CLI globally on your system:
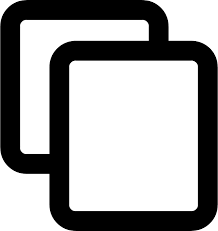
# Install Angular CLI globally
npm install -g @angular/cli
# Verify installation
ng version
4. Creating a New Angular Project
Once the Angular CLI is installed, you can create a new Angular project with the ng new
command:
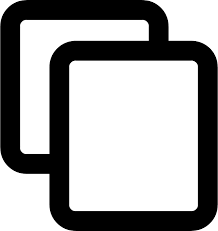
# Create a new Angular project
ng new my-angular-app
# Navigate to the project directory
cd my-angular-app
During project creation, the CLI will prompt you to choose configurations like adding Angular routing and selecting a stylesheet format (CSS, SCSS, etc.).
5. Running the Angular Application
To start the development server and run your application, use the following command:
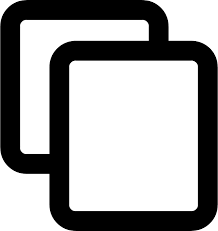
# Start the development server
ng serve
# Open the app in a browser at http://localhost:4200
6. Exploring Angular CLI Commands
Angular CLI provides various commands to streamline development:
- Generate Components:
ng generate component component-name
- Generate Services:
ng generate service service-name
- Build Project:
ng build
- Run Unit Tests:
ng test
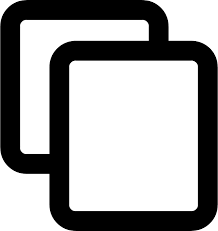
# Example: Generate a new component
ng generate component my-new-component
# Example: Build the project for production
ng build --prod
7. Conclusion
Setting up Angular CLI is straightforward and allows developers to quickly start building Angular applications. Its robust features and commands enhance productivity, making Angular a powerful choice for modern web development.
Creating the First Angular Application
1. Introduction
Building your first Angular application is simple and straightforward. With Angular CLI, you can set up and launch a fully functional app in a few steps. This guide covers the process of creating, running, and exploring your first Angular project.
2. Prerequisites
Before starting, ensure that Angular CLI is installed on your system. If not, refer to the "Setting up Angular CLI" section to install it.
3. Creating a New Angular Project
To create your first Angular project, use the ng new
command:
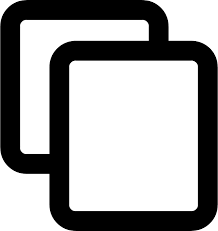
# Create a new Angular application
ng new my-first-app
# Navigate to the project directory
cd my-first-app
You will be prompted to configure the project settings:
- Choose whether to add Angular routing (select
Yes
orNo
). - Select the preferred stylesheet format (CSS, SCSS, etc.).
4. Running the Application
Once the project is created, start the development server to view your application in a browser:
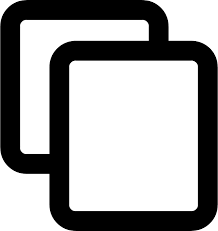
# Start the Angular development server
ng serve
# Open the app in a browser at http://localhost:4200
5. Exploring the Project Structure
The newly created project includes the following structure:
- src/app: Contains the application’s components and modules.
- src/index.html: The main HTML file that loads the Angular app.
- angular.json: Configuration file for Angular CLI.
- package.json: Lists the dependencies and scripts for the project.
6. Modifying the Application
You can start customizing your application by editing the app.component.html
file in the src/app
directory:
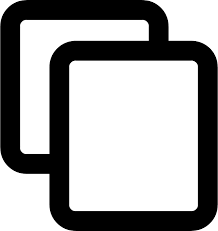
<h1>Welcome to My First Angular App!</h1>
<p>This is a simple Angular application.</p>
7. Adding a New Component
Angular CLI makes it easy to add new components to your application:
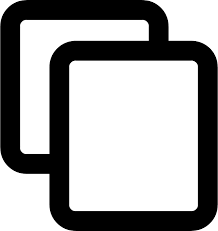
# Generate a new component
ng generate component my-new-component
This command creates a new component with its HTML, CSS, and TypeScript files in the src/app/my-new-component
directory.
8. Building the Application
To prepare your application for production, use the ng build
command:
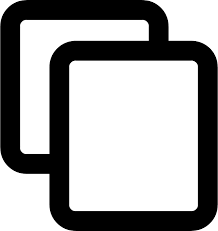
# Build the application for production
ng build --prod
9. Conclusion
Congratulations! You have successfully created and explored your first Angular application. With Angular CLI, you can quickly prototype and build scalable and dynamic web applications.
Project Structure Overview
1. Introduction
When you create a new Angular project using the Angular CLI, it generates a well-organized directory structure. Understanding this structure is essential for effectively working with Angular applications and managing code scalability and maintenance.
2. Root-Level Files
The project’s root directory contains important configuration and metadata files:
- angular.json: Configuration for Angular CLI, including build and test settings.
- package.json: Lists dependencies, scripts, and metadata for the project.
- tsconfig.json: TypeScript configuration file for the project.
- README.md: Contains project documentation.
- node_modules: Directory where all project dependencies are installed.
3. The src
Folder
The src
folder is the heart of the Angular application and contains the following:
- app: Contains the application’s core logic, components, modules, and services.
- assets: Stores static resources like images, fonts, and other files.
- environments: Contains environment-specific configuration files (e.g., development and production).
- index.html: The main HTML file that loads the Angular app.
- main.ts: The entry point for the application. It bootstraps the root module.
- polyfills.ts: Provides compatibility support for older browsers.
- styles.css: Global styles for the application.
- test.ts: Entry point for unit tests.
4. App Folder Structure
The app
folder contains the essential files to build the Angular application:
- app.module.ts: The root module that declares all components, directives, and services.
- app.component.ts: The main component containing the application’s logic.
- app.component.html: The HTML template for the root component.
- app.component.css: Styles specific to the root component.
- app-routing.module.ts: (Optional) Handles the application’s routing configuration.
5. Generated Component Structure
When you generate a new component using Angular CLI, it creates a folder with the following files:
- component-name.component.ts: Contains the component logic.
- component-name.component.html: The HTML template for the component.
- component-name.component.css: The component’s specific styles (or SCSS if selected).
- component-name.component.spec.ts: Unit test file for the component.
6. Build Output Folder
When you build the application for production using ng build
, the compiled output is placed in the dist
folder. This folder contains all the files required to deploy the application.
7. Conclusion
Having a clear understanding of the Angular project structure helps in efficiently managing and scaling the application. Each folder and file serves a specific purpose and contributes to a modular and maintainable architecture.
Running and Debugging the Application
1. Overview
Running and debugging an Angular application is essential to ensure that the application behaves as expected. In this section, we will cover the steps to run an Angular application and the tools available to debug it effectively.
2. Running the Angular Application
To run an Angular application locally, you need to use the Angular CLI. Here are the steps to get your application up and running:
- Step 1: Open a terminal or command prompt.
- Step 2: Navigate to your Angular project directory.
- Step 3: Run the following command to start the application:
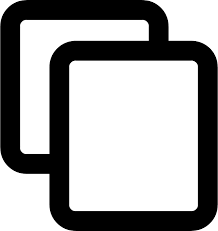
ng serve
Once the application is running, open your browser and go to http://localhost:4200/
to view your Angular app.
3. Debugging the Application
Angular provides various tools for debugging your application. The most common tools and techniques include:
3.1. Using Browser Developer Tools
Most modern browsers come with built-in developer tools that you can use to debug your Angular application. These tools allow you to inspect HTML, CSS, network requests, and JavaScript errors. To open the developer tools in Chrome, right-click anywhere on the page and select "Inspect" or press Ctrl + Shift + I
.
3.2. Using Angular Augury
Angular Augury is a Chrome extension that provides an in-depth view of your Angular application’s structure. It lets you explore component trees, view the state of the application, and interact with the Angular components directly.
To install Angular Augury:
- Step 1: Go to the Chrome Web Store and search for "Angular Augury."
- Step 2: Add the extension to Chrome.
- Step 3: After installing, open the developer tools in Chrome, and you’ll find a new tab called "Augury" where you can inspect your Angular application.
3.3. Using Console Logs
One of the simplest debugging techniques is using console.log()
statements in your TypeScript code. These logs will output information to the browser’s console, helping you track variables, functions, or the flow of your application.
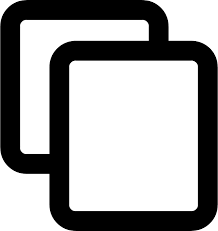
console.log('Component Loaded');
console.log('Current Value:', this.value);
Make sure to remove unnecessary console.log()
statements before deploying your application to production.
3.4. Using Breakpoints in TypeScript
You can set breakpoints in the browser’s developer tools to pause execution of your Angular application at specific lines of code. Once the code execution pauses, you can inspect variables, step through the code, and understand how the application state changes.
To set a breakpoint, simply open the developer tools in Chrome, navigate to the "Sources" tab, locate your TypeScript file, and click on the line number where you want to pause the execution.
4. Common Issues and Solutions
Here are some common issues that developers face when running or debugging Angular applications:
- Issue: Application does not start after running
ng serve
. - Solution: Make sure there are no errors in your console, such as missing dependencies or incorrect configurations.
- Issue: Changes are not reflected in the browser after saving the files.
- Solution: Ensure that the Angular development server is running and the browser is set to auto-refresh when changes are made.
- Issue: Encountering runtime errors in the browser’s console.
- Solution: Check the stack trace in the console for clues, and use breakpoints or
console.log()
to track the cause of the error.
5. Conclusion
Running and debugging an Angular application is crucial for ensuring the application is functioning as expected. By using tools like the Angular CLI, browser developer tools, and Angular Augury, you can easily run, debug, and optimize your application for better performance and reliability.
Modules
1. Overview
Modules are a fundamental part of Angular applications, helping organize your code into functional units. They encapsulate related components, services, pipes, and directives, and provide an efficient way to manage dependencies and encapsulate features of your application.
2. What is an Angular Module?
In Angular, a module is defined using the @NgModule
decorator. It’s a class that contains metadata that Angular uses to organize the application. A module can include:
- Components: The building blocks of the user interface.
- Services: Classes that provide specific functionality, often for data sharing across the application.
- Directives: Functions that extend HTML behavior.
- Pipes: Functions that transform data for display in the user interface.
- Other Modules: You can import other modules to make their features available in your module.
3. Creating a Module
To create a module in Angular, you can use the Angular CLI. Here’s how to generate a new module:
- Step 1: Open the terminal and navigate to your Angular project directory.
- Step 2: Run the following command:
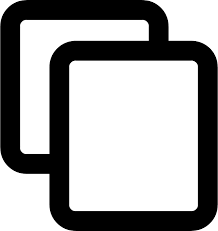
ng generate module my-module
This command will generate a new folder called my-module
with a basic module file inside it.
4. Defining a Module
In the newly created module file, you will see something like this:
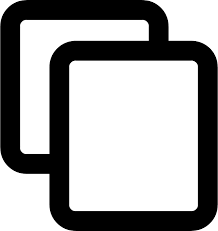
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
@NgModule({
declarations: [],
imports: [
CommonModule
],
exports: []
})
export class MyModule { }
The @NgModule
decorator marks the class as an Angular module. It has several properties:
- declarations: List of components, directives, and pipes that belong to this module.
- imports: List of other modules whose exported components, directives, and pipes are used in this module.
- exports: List of components, directives, and pipes that should be accessible in other modules.
5. Importing Modules
If you want to use components from other modules, you can import them into your module using the imports
array. For example, to use the FormsModule
for template-driven forms, you would do:
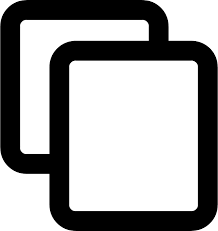
import { FormsModule } from '@angular/forms';
@NgModule({
imports: [
FormsModule
]
})
export class MyModule { }
6. Feature Modules
Feature modules are modules that encapsulate specific functionality within your application. For instance, you could have a feature module for user authentication, another for managing products, etc. These modules help in keeping your application organized, scalable, and maintainable.
6.1. Lazy Loading
Lazy loading allows you to load feature modules only when they are needed, improving the initial loading time of your application. To set up lazy loading, use the loadChildren
property in the routing configuration:
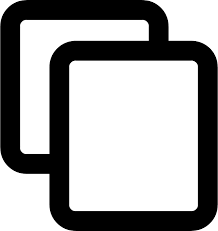
const routes: Routes = [
{
path: 'auth',
loadChildren: () => import('./auth/auth.module').then(m => m.AuthModule)
}
];
This ensures the AuthModule
is only loaded when the user navigates to the /auth
path.
7. Core Module
The CoreModule
is used to define services that should be available throughout the entire application, such as authentication services or logging services. The CoreModule is typically only imported once in the root module (usually AppModule
).
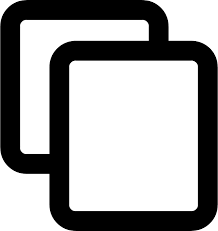
@NgModule({
providers: [AuthService, LoggingService]
})
export class CoreModule { }
8. Conclusion
Modules in Angular provide a way to organize and manage the various parts of your application. By using modules effectively, you can build scalable, maintainable, and efficient Angular applications. Whether you're building small features or large-scale enterprise applications, understanding and utilizing modules is key to a well-structured Angular project.
Components
1. Overview
Components are the building blocks of Angular applications. They define the view for the application and contain the logic to control that view. A component is made up of three key parts: the template (HTML), the class (TypeScript), and the style (CSS).
2. What is an Angular Component?
An Angular component is defined using the @Component
decorator. It contains the following elements:
- Template: The HTML that defines the view for the component.
- Class: The TypeScript class that holds the logic, such as properties and methods.
- Styles: Optional CSS or styles to define the appearance of the component.
3. Creating a Component
To create a new component in Angular, you can use Angular CLI. Run the following command:
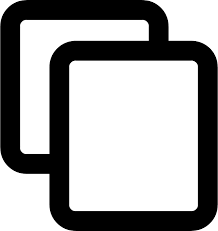
ng generate component my-component
This command generates a new folder with four files for the component: HTML, TypeScript, CSS, and a testing file.
4. Defining a Component
Here’s what the component looks like after it’s generated:
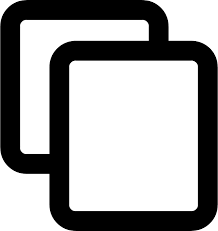
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponent {
message: string = 'Hello, Angular!';
}
In this example:
selector:
The name of the custom HTML tag that represents this component (e.g.,<app-my-component>
).templateUrl:
The path to the HTML template.styleUrls:
The path to the CSS styles.
5. Using a Component
To use the component, you simply add its selector tag (<app-my-component>
) to the template of the parent component. For example, in the AppComponent
template:
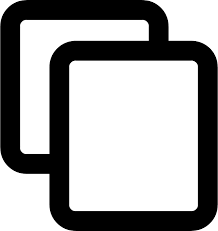
<h1>Welcome to My Angular App</h1>
When this is rendered, Angular will replace the <app-my-component>
tag with the content defined in the MyComponent
template.
6. Component Lifecycle
Components have a lifecycle, which consists of several hooks that allow you to run code at specific points in the component’s life. Some key lifecycle hooks include:
- ngOnInit: Called once the component is initialized, after the first
ngOnChanges
. - ngOnChanges: Called when the input properties of a component change.
- ngOnDestroy: Called just before Angular destroys the component, allowing you to clean up resources.
Here’s an example of using a lifecycle hook in the component class:
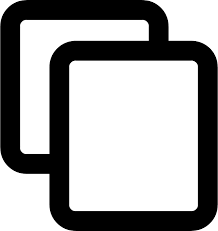
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponent implements OnInit {
message: string;
ngOnInit() {
this.message = 'Component initialized!';
}
}
7. Component Interaction
Components can communicate with each other in several ways:
- Input Properties: A parent component can pass data to a child component through input properties.
- Output Events: A child component can send data or events back to the parent component using output events.
Here’s an example of a parent-child component interaction using input and output properties:
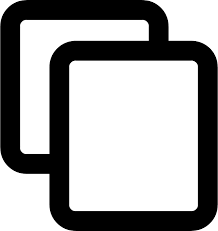
@Component({
selector: 'app-parent',
template: ` `
})
export class ParentComponent {
parentData: string = 'Data from Parent';
onChildEvent(event: any) {
console.log('Event received from child:', event);
}
}
@Component({
selector: 'app-child',
template: `{{ childData }}
<button (click)="sendEvent()">Send Event</button>`
})
export class ChildComponent {
@Input() childData: string;
@Output() childEvent = new EventEmitter();
sendEvent() {
this.childEvent.emit('Hello from Child');
}
}
In this example:
@Input
binds the parent’s data to the child component.@Output
emits an event from the child back to the parent.
8. Conclusion
Components are essential to building Angular applications. They help in breaking down the application into smaller, reusable parts, making your code more maintainable and scalable. Understanding how to create, use, and interact with components is key to mastering Angular development.
Templates
1. Overview
In Angular, templates define the view for a component. They describe what the user will see in the browser. Templates are written in HTML, but they can include Angular-specific syntax to handle dynamic data, events, and more.
2. What is an Angular Template?
An Angular template is an HTML file that contains Angular-specific syntax, such as:
- Data Binding: Binding data from the component class to the template.
- Directives: Instructions for how the DOM should be updated or manipulated.
- Event Binding: Listening for and responding to user events like clicks, input changes, etc.
3. Types of Data Binding
Angular supports several types of data binding:
- Interpolation: Binding component data to the template using {{ expression }} syntax.
- Property Binding: Binding component properties to HTML element attributes using [property] syntax.
- Event Binding: Binding events to methods in the component using (event) syntax.
- Two-Way Binding: A combination of property and event binding, allowing for synchronization between the template and component using [(ngModel)] syntax.
4. Interpolation Example
Interpolation is used to display dynamic values in the template:
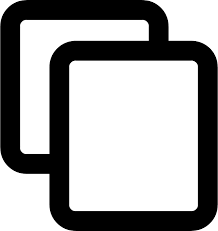
<h1>{{ title }}</h1>
In this example, the value of the title
variable from the component is displayed in the HTML.
5. Property Binding Example
Property binding is used to bind a component property to an HTML element’s property:
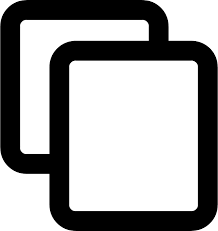
<img [src]="imageUrl" alt="Image" />
Here, the src
property of the <img>
element is bound to the imageUrl
property from the component.
6. Event Binding Example
Event binding allows you to listen to DOM events and call methods in the component class:
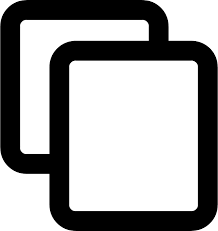
<button (click)="onClick()">Click me</button>
In this example, when the button is clicked, the onClick()
method in the component is triggered.
7. Two-Way Data Binding Example
Two-way data binding allows for synchronization between the component class and the template:
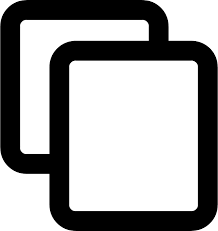
<input [(ngModel)]="name" />
<p>Hello, {{ name }}!</p>
Here, the input field is bound to the name
property. Any changes in the input field will update the component’s name
property and vice versa.
8. Using Directives in Templates
Directives are special markers in Angular templates that extend HTML's capabilities. There are two types of directives: Structural and Attribute.
- Structural Directives: They alter the structure of the DOM, such as
*ngIf
and*ngFor
. - Attribute Directives: They modify the appearance or behavior of an element, such as
ngClass
andngStyle
.
9. Structural Directive Example - *ngIf
The *ngIf
directive conditionally includes or excludes an HTML element based on a boolean expression:
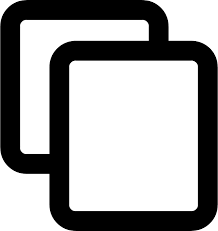
<div *ngIf="isLoggedIn">Welcome, User!</div>
This will display the welcome message only if the isLoggedIn
property is true
.
10. Structural Directive Example - *ngFor
The *ngFor
directive is used to iterate over an array and display elements in the template:
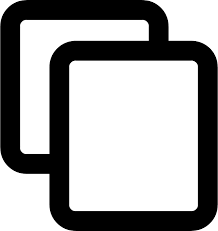
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
Here, the items
array is looped over, and each item is displayed as a list item.
11. Conclusion
Templates in Angular provide a powerful way to bind data, respond to events, and manipulate the DOM. With features like data binding, directives, and event handling, templates make it easy to create dynamic and interactive views. Understanding how to use templates is essential for building Angular applications.
Directives
1. Overview
Directives are special markers or instructions in Angular templates that tell the Angular framework to do something to the DOM (Document Object Model). They allow you to extend HTML capabilities, manipulate the DOM, and add behavior to elements and components.
2. Types of Directives
There are three main types of directives in Angular:
- Structural Directives: These change the structure of the DOM by adding or removing elements. Examples:
*ngIf
,*ngFor
. - Attribute Directives: These change the appearance or behavior of an element. Examples:
ngClass
,ngStyle
. - Custom Directives: These are user-defined directives that extend Angular's built-in functionality.
3. Structural Directives
Structural directives modify the DOM layout by adding or removing elements. They are prefixed with an asterisk (*
) in the template.
4. Example: *ngIf
The *ngIf
directive conditionally includes or excludes an HTML element based on a boolean expression. If the expression evaluates to true, the element is included in the DOM; otherwise, it is removed.
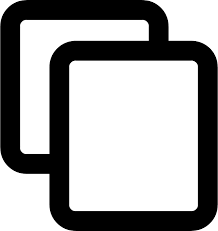
<div *ngIf="isVisible">This content is visible when isVisible is true</div>
In this example, the div
will only be displayed if isVisible
is true
.
5. Example: *ngFor
The *ngFor
directive is used to loop through a list or array and repeat HTML elements for each item in the collection.
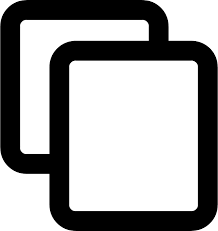
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
This example loops through the items
array and displays each item as a list item in the unordered list.
6. Attribute Directives
Attribute directives change the appearance or behavior of an element. They are not prefixed with an asterisk.
7. Example: ngClass
The ngClass
directive allows you to dynamically add or remove CSS classes to an element based on component data.
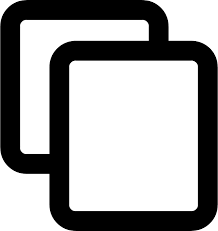
<div [ngClass]="{'highlight': isHighlighted}">This div will be highlighted if isHighlighted is true</div>
In this example, the highlight
class will be applied to the div
if the isHighlighted
property is true
.
8. Example: ngStyle
The ngStyle
directive allows you to set inline styles on an element dynamically based on component data.
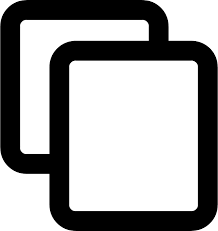
<div [ngStyle]="{'background-color': bgColor}">This div has a dynamic background color</div>
The background color of the div
will change based on the bgColor
property in the component.
9. Creating Custom Directives
Angular allows you to create custom directives to extend its functionality. Custom directives can be structural or attribute directives, depending on how they are intended to be used.
10. Example: Custom Attribute Directive
Here’s an example of a custom attribute directive that changes the background color of an element when hovered:
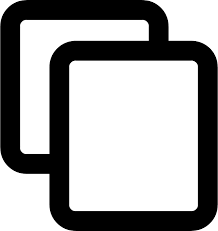
import { Directive, ElementRef, Renderer2, HostListener } from '@angular/core';
@Directive({
selector: '[appHoverEffect]'
})
export class HoverEffectDirective {
constructor(private el: ElementRef, private renderer: Renderer2) { }
@HostListener('mouseenter') onMouseEnter() {
this.renderer.setStyle(this.el.nativeElement, 'background-color', 'yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.renderer.setStyle(this.el.nativeElement, 'background-color', 'transparent');
}
}
This directive changes the background color of the element when the mouse enters or leaves the element.
11. Example: Using Custom Attribute Directive
Here’s how to apply the custom appHoverEffect
directive in a template:
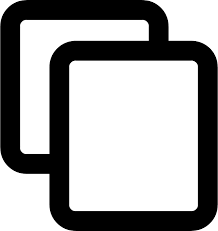
<div appHoverEffect>Hover over me to change my background color!</div>
When you hover over this div
, the background color will change to yellow, and it will revert to transparent when the mouse leaves.
12. Conclusion
Directives are a powerful feature in Angular that allow you to manipulate the DOM and extend HTML with custom behavior. Whether you are using built-in structural and attribute directives or creating your own, directives are essential for creating dynamic and interactive Angular applications.
Data Binding (Interpolation, Property, Event, Two-way)
1. Overview
Data binding in Angular is a mechanism that allows you to connect the component and the view. It enables communication between the component class and its template, allowing data to flow in both directions. Angular supports various types of data binding: Interpolation, Property Binding, Event Binding, and Two-way Binding.
2. Types of Data Binding
There are four main types of data binding in Angular:
- Interpolation: Binding data from the component to the view as part of an expression inside double curly braces (
{{}}
). - Property Binding: Binding an element property to a component property using square brackets (
[]
). - Event Binding: Binding an event (like click or input) to a method in the component using parentheses (
( )
). - Two-way Binding: Combining property binding and event binding using
[( )]
syntax.
3. Interpolation
Interpolation allows you to embed component property values into the template. It’s achieved by enclosing the expression in double curly braces ({{}}
).
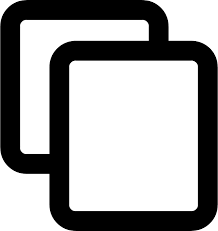
<p>{{ title }}</p>
In this example, the title
property from the component will be displayed inside the paragraph.
4. Property Binding
Property binding allows you to bind an element property to a component property. It is done using square brackets ([]
) around the element's property.
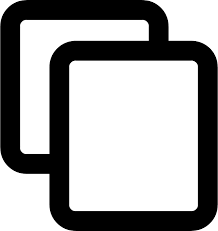
<img [src]="imageUrl" alt="Angular Logo">
In this example, the src
property of the img
element is bound to the imageUrl
property of the component.
5. Event Binding
Event binding allows you to bind a DOM event (like a click or input event) to a method in your component. This is done by using parentheses (( )
) around the event name.
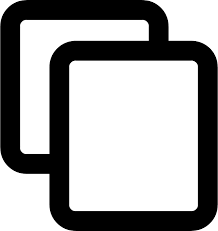
<button (click)="onClick()">Click Me</button>
In this example, the onClick()
method in the component will be triggered when the button is clicked.
6. Two-way Binding
Two-way data binding allows you to bind both the property and the event in a single operation. It is represented using the syntax [( )]
, which is a combination of property and event binding.
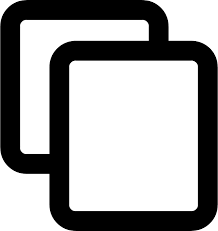
<input [(ngModel)]="name">
<p>Your name: {{ name }}</p>
In this example, the ngModel
directive binds the input element to the name
property in the component. When the user types in the input field, the value of name
is updated, and it is also reflected in the paragraph.
7. Full Example with All Types of Data Binding
Here’s an example that demonstrates all four types of data binding in one component:
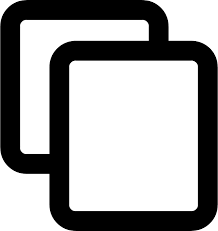
<h1>{{ title }}</h1>
<button (click)="changeTitle()">Change Title</button>
<p>{{ description }}</p>
<img [src]="imageUrl" alt="Image" />
<input [(ngModel)]="inputText">
<p>Your input: {{ inputText }}</p>
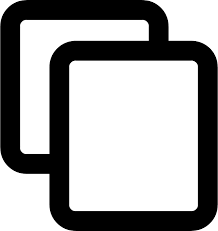
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular Data Binding Example';
description = 'This is an example of how to use data binding in Angular.';
imageUrl = 'https://angular.io/assets/images/logos/angular/angular.png';
inputText = '';
changeTitle() {
this.title = 'Title Changed!';
}
}
In this example:
- Interpolation:
{{ title }}
and{{ description }}
display values from the component. - Event Binding: The
changeTitle()
method is triggered when the button is clicked, changing the title. - Property Binding: The
src
property of theimg
element is bound to theimageUrl
property. - Two-way Binding: The
inputText
property is bound to the input field withngModel
, and changes are reflected immediately in the paragraph.
8. Conclusion
Data binding is a powerful feature in Angular that allows you to synchronize data between the component and the view. Understanding the different types of data binding — Interpolation, Property Binding, Event Binding, and Two-way Binding — is essential for building dynamic and interactive Angular applications. By using these techniques, you can build efficient and user-friendly applications.
Dependency Injection
1. Overview
Dependency Injection (DI) is a core concept in Angular that allows you to inject services and other dependencies into components and other services. This design pattern helps to make your code more modular, testable, and maintainable by decoupling the creation of dependencies from their usage.
2. What is Dependency Injection?
Dependency Injection is a design pattern where an object or function receives its dependencies from an external source rather than creating them internally. In Angular, services and other objects are injected into components, directives, and other services using DI.
3. How Dependency Injection Works in Angular
In Angular, the DI system provides dependencies (such as services) to components and other services. These dependencies are declared in the constructor of a class and are injected automatically by Angular's injector at runtime.
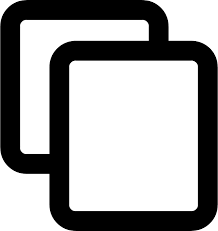
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private dataService: DataService) {
this.dataService.getData().subscribe(data => {
console.log(data);
});
}
}
In the above example, the DataService
is injected into the AppComponent
through the constructor. Angular’s DI system handles the creation and injection of the service.
4. Creating a Service for Dependency Injection
To use Dependency Injection, you first need to create a service that will be injected into your components or other services. A service is a class that contains logic that is needed across multiple components.
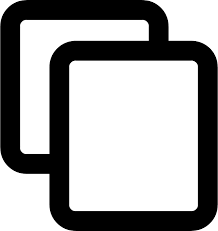
import { Injectable } from '@angular/core';
import { Observable, of } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor() { }
getData(): Observable {
return of(['Item 1', 'Item 2', 'Item 3']);
}
}
In this example, the DataService
is marked with the @Injectable
decorator, and it is provided in the root of the application using the providedIn: 'root'
syntax. This makes it available for DI throughout the application.
5. Providing Services
In Angular, services can be provided at different levels, such as the root level or at the component level. The @Injectable
decorator is used to define how and where the service is provided.
- Root Level: Providing the service at the root level using
providedIn: 'root'
ensures that there is only one instance of the service throughout the application. - Component Level: Providing the service at the component level makes the service available only within that specific component.
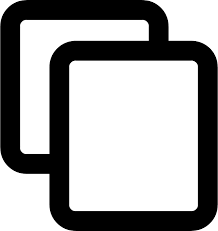
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { DataService } from './data.service';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [DataService],
bootstrap: [AppComponent]
})
export class AppModule { }
6. Benefits of Dependency Injection
- Loose Coupling: Components and services are decoupled, making it easier to manage and test them independently.
- Reusability: Services can be reused across components, ensuring that shared logic is centralized.
- Testability: DI makes it easier to mock dependencies in unit tests, improving testability.
- Flexibility: Dependencies can be injected at different levels, giving flexibility in how services are provided and shared.
7. Conclusion
Dependency Injection is one of the most powerful features of Angular, enabling developers to create highly modular, maintainable, and testable code. By using Angular's DI system, you can simplify the management of services and their dependencies, making your application more scalable and flexible.
Template-driven Forms
1. Overview
Template-driven forms are a simple way to handle forms in Angular. They rely on directives, such as ngModel
, to manage form controls and validations. With template-driven forms, Angular automatically handles the form's data binding and validation logic.
2. What are Template-driven Forms?
Template-driven forms provide a simpler, declarative way to build forms in Angular. They use directives to bind HTML form elements to model properties and automatically apply validation rules.
3. How Template-driven Forms Work in Angular
In a template-driven form, the form model is implicitly created by Angular. The ngModel
directive binds the form input elements to properties in the component class.
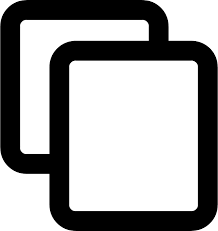
<form #userForm="ngForm" (ngSubmit)="onSubmit(userForm)">
<label for="firstName">First Name:</label>
<input type="text" id="firstName" name="firstName" ngModel required>
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" name="lastName" ngModel required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" ngModel required email>
<button type="submit" [disabled]="!userForm.valid">Submit</button>
</form>
In the above example, the form is bound to the userForm
variable using the #userForm="ngForm"
directive. The ngModel
directive binds each input element to the form model, and validation is automatically handled using the required
and email
attributes.
4. Creating a Component for Template-driven Forms
To handle form submissions, you need to create a method in your component class that processes the form data. In this example, the form data is logged to the console.
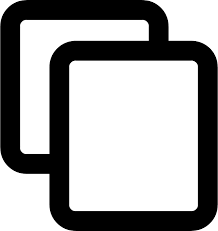
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
onSubmit(form: any) {
console.log('Form Submitted:', form.value);
}
}
The onSubmit
method receives the form data as an argument and logs it. This method is invoked when the user submits the form.
5. Validation in Template-driven Forms
Template-driven forms support both built-in validators (like required
and email
) and custom validators. The form control’s validity can be checked using properties such as valid
and invalid
.
- Built-in Validators: Angular provides several built-in validators like
required
,minlength
, andmaxlength
. - Custom Validators: You can also create your own validators to enforce specific business logic.
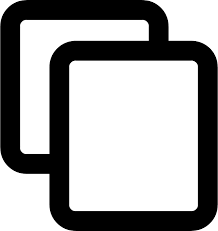
import { Component } from '@angular/core';
import { NgForm } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
onSubmit(form: NgForm) {
if (form.valid) {
console.log('Form Submitted:', form.value);
} else {
console.log('Form is invalid');
}
}
}
6. Benefits of Template-driven Forms
- Simplicity: Template-driven forms are easy to set up and use for simple forms.
- Declarative Syntax: Forms are built using a declarative syntax in the HTML template.
- Automatic Validation: Angular provides automatic validation for form controls with minimal effort.
7. Conclusion
Template-driven forms are a great choice for simple forms in Angular. They provide a concise and declarative way to handle form binding and validation with minimal boilerplate code.
Reactive Forms
1. Overview
Reactive Forms are a more powerful and flexible way of handling forms in Angular. They allow you to define the form model in the component class, giving you more control over the form's behavior. Reactive Forms are better suited for complex forms and for cases where you need to work with dynamic form controls.
2. What are Reactive Forms?
Reactive Forms, also known as model-driven forms, are defined in the component class, and their state is managed by the component. They use the FormControl
, FormGroup
, and FormArray
classes to define the form structure and validation logic.
3. How Reactive Forms Work in Angular
In Reactive Forms, the form model is explicitly created in the component class, and you use observables to track changes in form control values. Unlike template-driven forms, which are declarative, reactive forms are more programmatic and offer more flexibility.
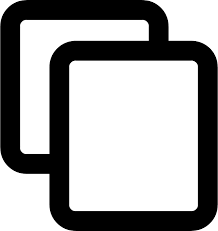
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<label for="firstName">First Name:</label>
<input type="text" id="firstName" formControlName="firstName">
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" formControlName="lastName">
<label for="email">Email:</label>
<input type="email" id="email" formControlName="email">
<button type="submit" [disabled]="userForm.invalid">Submit</button>
</form>
The form is bound to a FormGroup
in the component, and each input element is bound to a FormControl
using the formControlName
directive. The form is submitted using the (ngSubmit)
event handler.
4. Creating a Component for Reactive Forms
To create a reactive form, you need to import the ReactiveFormsModule
and define a FormGroup
and FormControl
in your component class. This allows you to manage the form's state programmatically.
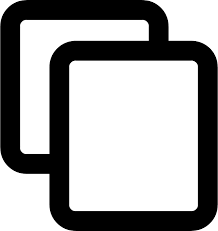
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
userForm: FormGroup;
constructor(private fb: FormBuilder) {}
ngOnInit(): void {
this.userForm = this.fb.group({
firstName: ['', Validators.required],
lastName: ['', Validators.required],
email: ['', [Validators.required, Validators.email]]
});
}
onSubmit(): void {
if (this.userForm.valid) {
console.log('Form Submitted:', this.userForm.value);
} else {
console.log('Form is invalid');
}
}
}
The FormGroup
is created using the FormBuilder
service. Each field is defined as a FormControl
with its respective validations. The onSubmit
method checks whether the form is valid before logging the data.
5. Validation in Reactive Forms
Reactive Forms provide fine-grained control over form validation. Validators can be added to the form controls when defining them in the component class. You can also apply custom validators, handle asynchronous validation, and track the validation status programmatically.
- Built-in Validators: Angular provides built-in validators like
required
,minlength
, andemail
. - Custom Validators: You can create custom validators for more complex scenarios.
- Async Validators: Reactive Forms support asynchronous validation, useful for checking server-side data.
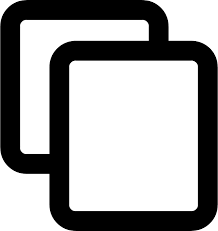
import { AbstractControl } from '@angular/forms';
export function forbiddenNameValidator(control: AbstractControl) {
const forbidden = /admin/i.test(control.value);
return forbidden ? { forbiddenName: { value: control.value } } : null;
}
This is an example of a custom validator that checks if the input contains the word "admin". If the condition is met, it returns an error object.
6. Benefits of Reactive Forms
- Explicit Control: Reactive forms provide explicit control over the form model in the component class, giving more flexibility for complex forms.
- Predictable: Reactive forms are easier to test and debug since the form model is defined in the component class.
- Handling Dynamic Forms: Reactive forms allow you to dynamically add or remove form controls and form groups.
7. Conclusion
Reactive Forms are a great choice when you need more control over your form logic and validation. With the flexibility they offer, they are ideal for complex forms, dynamic form groups, and handling more advanced validation scenarios.
Form Validation in Angular
1. Overview
Form validation is an essential part of any web application to ensure that the data entered by users is correct and adheres to certain rules. Angular provides built-in validators for both template-driven and reactive forms, as well as the ability to create custom validators for more complex scenarios.
2. Types of Form Validation
Angular supports two types of forms: template-driven forms and reactive forms. Both types have their own way of handling validation:
- Template-driven Forms: Validation is done declaratively in the HTML template using directives like
required
,minlength
, etc. - Reactive Forms: Validation is done programmatically in the component class using
Validators
from Angular's ReactiveFormsModule.
3. Form Validation in Template-driven Forms
In template-driven forms, validation is applied directly within the HTML template using attributes like required
, minlength
, and email
. Angular will automatically handle form validation based on these attributes.
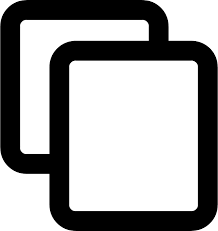
<form #userForm="ngForm" (ngSubmit)="onSubmit(userForm)">
<label for="firstName">First Name:</label>
<input type="text" id="firstName" name="firstName" ngModel required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" ngModel required email>
<button type="submit" [disabled]="!userForm.valid">Submit</button>
</form>
In this example, the required
and email
attributes are used for validation. The form is only submitted if it is valid, thanks to the [disabled]="!userForm.valid"
directive.
4. Form Validation in Reactive Forms
In reactive forms, validation is defined programmatically in the component class. Angular provides a set of built-in validators such as required
, minlength
, and email
that can be applied when defining form controls.
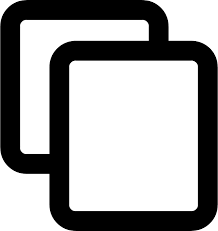
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
userForm: FormGroup;
constructor(private fb: FormBuilder) {}
ngOnInit(): void {
this.userForm = this.fb.group({
firstName: ['', Validators.required],
email: ['', [Validators.required, Validators.email]]
});
}
onSubmit(): void {
if (this.userForm.valid) {
console.log('Form Submitted:', this.userForm.value);
} else {
console.log('Form is invalid');
}
}
}
In the above example, the form controls are defined using the FormBuilder
and validators are applied using Angular’s Validators
5. Built-in Validators
Angular provides several built-in validators that can be used for both template-driven and reactive forms. Some of the most commonly used validators include:
- required: Ensures that the field is not empty.
- minlength: Validates that the value entered is at least the specified length.
- maxlength: Ensures that the value entered does not exceed the specified length.
- email: Validates that the value entered is a valid email address.
- pattern: Validates that the value matches a regular expression pattern.
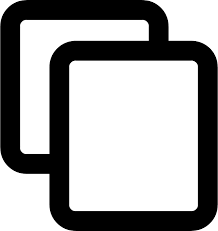
<form #userForm="ngForm" (ngSubmit)="onSubmit(userForm)">
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" name="lastName" ngModel required minlength="3">
<button type="submit" [disabled]="!userForm.valid">Submit</button>
</form>
In this example, the lastName
field is required and must have a minimum length of 3 characters. The form will not be submitted unless these conditions are met.
6. Custom Validators
Angular allows you to create custom validators to handle more complex validation logic. A custom validator is a function that takes a FormControl
and returns an error object if validation fails or null
if validation passes.
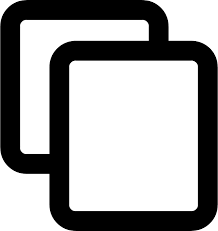
import { AbstractControl } from '@angular/forms';
export function forbiddenNameValidator(control: AbstractControl) {
const forbidden = /admin/i.test(control.value);
return forbidden ? { forbiddenName: { value: control.value } } : null;
}
This is an example of a custom validator that checks if the input contains the word "admin". If it does, the validator returns an error object with the name forbiddenName
.
7. Handling Validation Errors
You can check for validation errors and display appropriate messages to the user. In reactive forms, you can access the control's state (valid, invalid, touched, etc.) to show error messages conditionally.
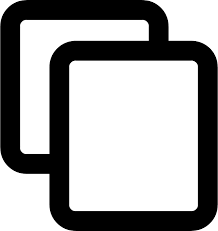
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<label for="email">Email:</label>
<input type="email" id="email" formControlName="email">
<div *ngIf="userForm.get('email')?.invalid && userForm.get('email')?.touched">
<small *ngIf="userForm.get('email')?.hasError('required')">Email is required.</small>
<small *ngIf="userForm.get('email')?.hasError('email')">Please enter a valid email address.</small>
</div>
<button type="submit" [disabled]="userForm.invalid">Submit</button>
This example shows how to display error messages based on the form control's state. If the user has touched the email input and the field is invalid, an error message will be shown.
8. Conclusion
Angular provides a powerful and flexible system for handling form validation. Whether using template-driven or reactive forms, you can easily apply built-in or custom validators to ensure data integrity. Proper validation improves user experience and helps in maintaining clean data for your application.
Setting up Angular Router
1. Overview
Angular Router is the official routing library for Angular applications that allows you to navigate between different views or pages. It helps in developing single-page applications (SPAs) by managing the navigation between components without reloading the entire page. The router enables you to define routes, handle navigation, and manage the state of the application in a flexible and declarative way.
2. Installing Angular Router
Angular Router is included by default when you create a new Angular application using the Angular CLI. However, if you need to install it manually, you can install it via npm:
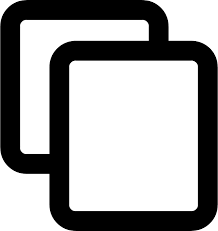
# Install Angular Router
npm install @angular/router
Once installed, you can import it into your application module and configure the routes.
3. Setting Up Routes
To set up routing in your Angular application, you need to define routes in the routing configuration and then configure the router module in the application's root module.
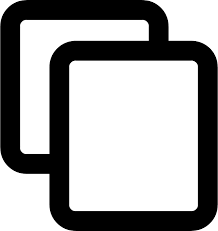
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
In the above example, we define two routes: one for the HomeComponent
and one for the AboutComponent
. The RouterModule.forRoot(routes)
method configures the router with the defined routes and makes it available throughout the application.
4. Adding Router Outlet
The router-outlet
directive is used in the template to mark where the routed components should be displayed. It acts as a placeholder for the components defined in the route configuration.
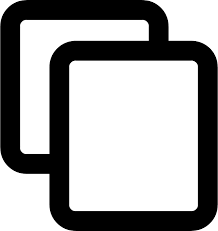
The router-outlet
directive should be added to the main component template (usually app.component.html
) to display the routed components when a user navigates to a specific route.
5. Navigating Between Routes
In Angular, you can navigate between routes using the routerLink
directive in the HTML template or programmatically using the Router
service.
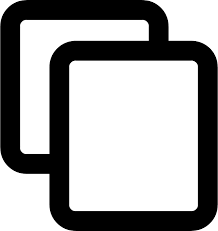
The routerLink
directive is used to create links to different routes, and routerLinkActive
is used to apply a CSS class when the route is active. The router-outlet
directive is where the routed components will be displayed.
6. Programmatic Navigation
You can also navigate programmatically using Angular’s Router
service. This is useful when you need to navigate based on certain conditions or events in your component.
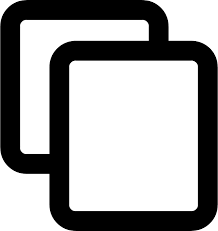
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private router: Router) {}
goToAbout(): void {
this.router.navigate(['/about']);
}
}
In this example, the goToAbout
method navigates to the AboutComponent
programmatically using the Router.navigate()
method.
7. Route Parameters
Routes can accept parameters, allowing you to pass dynamic values to your components. You can define route parameters in the route path and access them in your component using the ActivatedRoute
service.
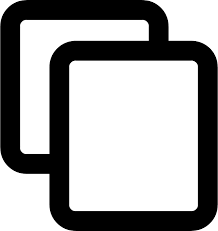
const routes: Routes = [
{ path: 'user/:id', component: UserComponent },
];
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-user',
templateUrl: './user.component.html',
styleUrls: ['./user.component.css']
})
export class UserComponent implements OnInit {
userId: string;
constructor(private route: ActivatedRoute) {}
ngOnInit(): void {
this.userId = this.route.snapshot.paramMap.get('id')!;
}
}
In this example, the route /user/:id
accepts a dynamic id
parameter. The ActivatedRoute
service is used to access the parameter in the component's ngOnInit
method.
8. Route Guards
Route guards are services that can be used to protect routes and control navigation. They allow you to check conditions before allowing access to a route, such as checking authentication or authorization status.
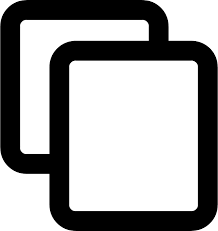
import { Injectable } from '@angular/core';
import { CanActivate } from '@angular/router';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
canActivate(): boolean {
const isAuthenticated = localStorage.getItem('token');
if (!isAuthenticated) {
return false;
}
return true;
}
}
The AuthGuard
service checks if a user is authenticated before allowing them to access a route. You can add the guard to routes by using the canActivate
property.
9. Conclusion
Setting up Angular Router allows you to create a smooth and dynamic navigation experience in your application. With the ability to define routes, pass parameters, and implement guards, Angular Router is a powerful tool for managing your application's navigation and ensuring a great user experience.
Route Parameters in Angular
1. Overview
Route parameters in Angular allow you to pass dynamic values in the URL and access them in your components. This is useful for creating routes that depend on specific values, such as user IDs, product names, or category filters. Route parameters make your application more dynamic by enabling different content to be displayed based on the URL.
2. Defining Route Parameters
You can define route parameters in your route configuration using a colon syntax (:param
) in the path. The parameter is then passed as part of the URL when navigating to the route.
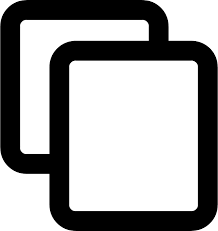
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { UserProfileComponent } from './user-profile/user-profile.component';
const routes: Routes = [
{ path: 'user/:id', component: UserProfileComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
In this example, the route /user/:id
defines a parameter :id
in the path. When you navigate to this route, Angular will capture the value of the id
parameter from the URL.
3. Accessing Route Parameters in the Component
To access the route parameters in the component, you can use Angular's ActivatedRoute
service. This service provides information about the current route, including the parameters that are part of the URL.
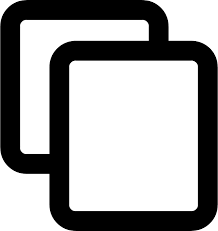
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-user-profile',
templateUrl: './user-profile.component.html',
styleUrls: ['./user-profile.component.css']
})
export class UserProfileComponent implements OnInit {
userId: string;
constructor(private route: ActivatedRoute) {}
ngOnInit(): void {
this.userId = this.route.snapshot.paramMap.get('id')!;
}
}
In the above example, the ActivatedRoute
service is used to access the id
parameter. The snapshot.paramMap.get('id')
method retrieves the value of the id
parameter from the URL when the component is initialized.
4. Route Parameters with Optional Parameters
Route parameters can be optional as well. You can define optional parameters using a question mark in the route configuration (:param?
). Optional parameters can be skipped in the URL, and their absence will not result in an error.
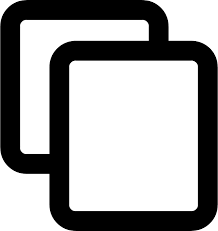
const routes: Routes = [
{ path: 'user/:id/:name', component: UserProfileComponent },
{ path: 'user/:id', component: UserProfileComponent }
];
In this configuration, both /user/:id/:name
and /user/:id
are valid routes. The :name
parameter is optional, and if it's not provided, the route will still work with just the :id
parameter.
5. Retrieving Optional Parameters
When using optional parameters, you can use the ActivatedRoute
service to check if the parameter exists and retrieve its value.
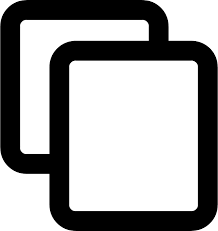
ngOnInit(): void {
this.userId = this.route.snapshot.paramMap.get('id')!;
this.userName = this.route.snapshot.paramMap.get('name') || 'Guest';
}
In this example, if the name
parameter is not provided in the URL, the default value 'Guest' is used.
6. Route Parameters with Multiple Values
Angular allows you to define routes that accept multiple parameters. You can pass multiple values in the URL, and they will be accessible using the ActivatedRoute
service.
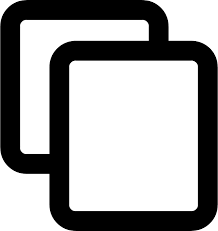
const routes: Routes = [
{ path: 'product/:category/:id', component: ProductDetailComponent },
];
In this example, the route /product/:category/:id
defines two parameters: category
and id
. You can access both parameters in the component as shown:
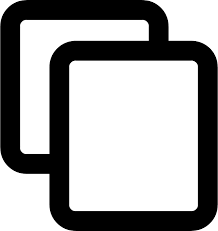
ngOnInit(): void {
this.category = this.route.snapshot.paramMap.get('category')!;
this.productId = this.route.snapshot.paramMap.get('id')!;
}
In this case, both category
and id
parameters will be available in the component.
7. Using Route Parameters for Navigation
You can also use route parameters when programmatically navigating between routes. The Router
service allows you to navigate by passing parameters as part of the route path.
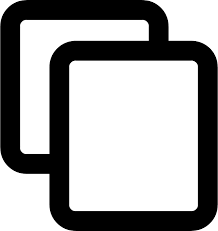
import { Router } from '@angular/router';
constructor(private router: Router) {}
goToProductDetail(category: string, id: string): void {
this.router.navigate(['/product', category, id]);
}
In this example, the goToProductDetail
method navigates to the ProductDetailComponent
with the specified category
and id
parameters.
8. Conclusion
Route parameters in Angular are a powerful feature that allow you to pass dynamic values through the URL, making your application more flexible. By defining route parameters, accessing them in components, and using them for navigation, you can create more dynamic and user-friendly applications.
Child Routes in Angular
1. Overview
Child routes in Angular allow you to create nested views within your application. This concept enables you to organize your application into smaller, reusable components and manage complex navigation scenarios by nesting routes inside other routes. Child routes help to build modular and maintainable applications by keeping the routing logic organized.
2. Defining Child Routes
Child routes are defined inside the children
property of a parent route. A parent route can have multiple child routes, which will be rendered inside a designated
in the parent component's template.
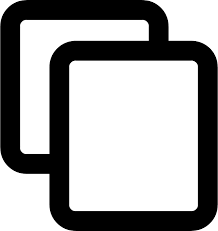
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { DashboardComponent } from './dashboard/dashboard.component';
import { UserProfileComponent } from './user-profile/user-profile.component';
import { SettingsComponent } from './settings/settings.component';
const routes: Routes = [
{
path: 'dashboard',
component: DashboardComponent,
children: [
{ path: 'user-profile', component: UserProfileComponent },
{ path: 'settings', component: SettingsComponent }
]
}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
In this example, the DashboardComponent
is the parent route, and it has two child routes: /dashboard/user-profile
and /dashboard/settings
. These child routes will be displayed inside the
of the DashboardComponent
's template.
3. Setting Up the Parent Component's Template
The parent component must have a
directive in its template to render the child components when their respective routes are activated.
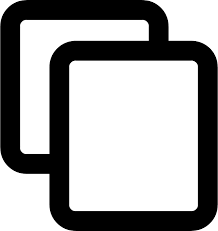
<h2>Dashboard</h2>
<p>Welcome to the dashboard!</p>
In the above example, the child routes will be rendered inside the
tag in the DashboardComponent
's template. When the user navigates to /dashboard/user-profile
or /dashboard/settings
, the respective component will be displayed inside this outlet.
4. Navigating to Child Routes
To navigate to a child route, you can use Angular's RouterLink
directive, just like with parent routes. The child route path is relative to the parent route.
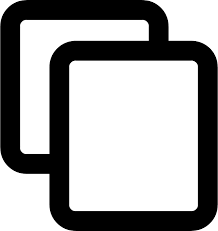
<nav>
<a routerLink="user-profile">User Profile</a>
<a routerLink="settings">Settings</a>
</nav>
In this example, when the user clicks on the "User Profile" or "Settings" links, Angular will navigate to /dashboard/user-profile
or /dashboard/settings
, respectively, and the corresponding child component will be displayed in the
of the parent component.
5. Lazy Loading Child Routes
You can configure lazy loading for child routes to improve the performance of your application by loading child routes only when they are needed.
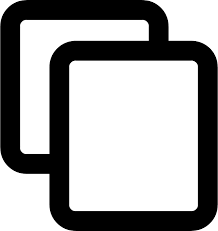
const routes: Routes = [
{
path: 'dashboard',
component: DashboardComponent,
children: [
{ path: 'user-profile', loadChildren: () => import('./user-profile/user-profile.module').then(m => m.UserProfileModule) },
{ path: 'settings', loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule) }
]
}
];
In this configuration, the user-profile
and settings
modules are lazily loaded when the user navigates to the corresponding child routes. This helps reduce the initial bundle size and improves the application's load time.
6. Child Routes with Parameters
Child routes can also accept parameters, just like parent routes. You can pass parameters to a child route by including them in the route path, and then access them in the child component using the ActivatedRoute
service.
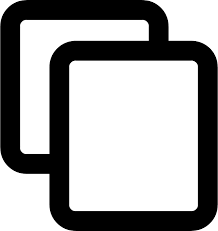
const routes: Routes = [
{
path: 'dashboard',
component: DashboardComponent,
children: [
{ path: 'user-profile/:id', component: UserProfileComponent }
]
}
];
In this configuration, the child route /dashboard/user-profile/:id
accepts an id
parameter. You can access this parameter in the child component as shown:
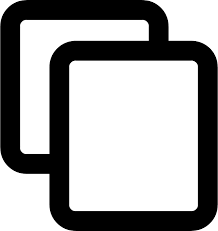
import { ActivatedRoute } from '@angular/router';
export class UserProfileComponent implements OnInit {
userId: string;
constructor(private route: ActivatedRoute) {}
ngOnInit(): void {
this.userId = this.route.snapshot.paramMap.get('id')!;
}
}
In this example, the id
parameter is retrieved from the URL and used in the UserProfileComponent
to display the appropriate user's profile.
7. Conclusion
Child routes in Angular provide a powerful way to organize and manage your application's navigation. By defining child routes, you can create a more modular structure for your application, reduce redundant code, and improve overall maintainability. Additionally, you can use lazy loading to optimize performance and pass parameters to child routes to display dynamic content.
Lazy Loading in Angular
1. Overview
Lazy loading is a design pattern in Angular that allows modules to be loaded on demand, reducing the initial load time of the application. This approach is particularly beneficial for large applications as it helps improve performance by loading only the necessary parts of the application when needed.
2. What is Lazy Loading?
Lazy loading means that specific modules or components are loaded only when the user navigates to a route that requires them. This is achieved by splitting the application into multiple feature modules, each responsible for a specific part of the application, and loading these modules asynchronously.
3. Setting Up Lazy Loading
To implement lazy loading in Angular, follow these steps:
Step 1: Create a Feature Module
Generate a new feature module using the Angular CLI command:
ng generate module feature-name --route feature-path --module app.module
<p>This command creates a new feature module and sets up lazy loading automatically.</p>
<h5>Step 2: Configure the Routes</h5>
<p>Modify the application's routing configuration to use lazy loading for the feature module:</p>
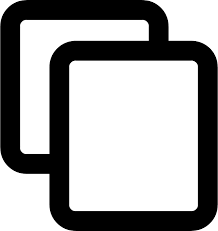
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [
{ path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) },
{ path: '', redirectTo: '/home', pathMatch: 'full' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
In this example, the FeatureModule
is lazily loaded when the user navigates to /feature
.
4. Creating Routes in the Feature Module
Define the routes for the feature module in its routing configuration:
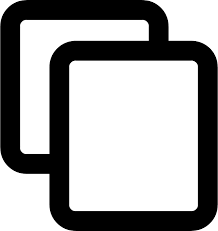
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { FeatureComponent } from './feature.component';
const routes: Routes = [
{ path: '', component: FeatureComponent }
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class FeatureRoutingModule { }
Here, the FeatureComponent
will be displayed when the user navigates to /feature
.
5. Benefits of Lazy Loading
- Improved Performance: Reduces the size of the initial bundle, speeding up the application's initial load time.
- Scalability: Makes it easier to scale large applications by dividing them into smaller, feature-specific modules.
- Optimized Resource Usage: Loads only the required modules, reducing memory usage and improving efficiency.
6. Lazy Loading with Preloading
To further optimize performance, Angular provides preloading strategies that allow you to preload lazy-loaded modules in the background after the initial application load. You can enable preloading by configuring the router as follows:
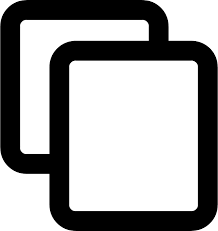
import { NgModule } from '@angular/core';
import { RouterModule, Routes, PreloadAllModules } from '@angular/router';
const routes: Routes = [
{ path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) },
{ path: '', redirectTo: '/home', pathMatch: 'full' }
];
@NgModule({
imports: [RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })],
exports: [RouterModule]
})
export class AppRoutingModule { }
In this configuration, the PreloadAllModules
strategy preloads all lazy-loaded modules in the background, ensuring faster navigation without impacting the initial load time.
7. Conclusion
Lazy loading is a powerful mechanism in Angular that optimizes the performance of large applications by loading feature modules only when needed. Combined with preloading strategies, lazy loading ensures a balance between performance and user experience, making it an essential technique for scalable application development.
Creating Services in Angular
1. Overview
Services in Angular are used to share data, business logic, or other functionalities between components. They enable code reusability and modularity, making your application easier to manage and test. Services are typically used for tasks like fetching data from APIs, managing state, or encapsulating business logic.
2. What is a Service?
A service is a class with a specific purpose, such as handling data logic or interactions with a backend API. Services are commonly injected into components or other services using Angular's Dependency Injection (DI) system.
3. Creating a Service
To create a service in Angular, follow these steps:
Step 1: Generate a Service
Use the Angular CLI to generate a new service:
ng generate service service-name
This command creates two files: service-name.service.ts
and service-name.service.spec.ts
. The service file contains the logic, while the spec file is for unit testing.
Step 2: Implement the Service Logic
Add the required logic to the service. For example, a service that fetches data from an API:
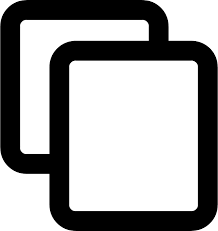
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) { }
getData(): Observable {
return this.http.get(this.apiUrl);
}
}
Here, the DataService
uses Angular's HttpClient
module to fetch data from an API.
4. Injecting a Service into a Component
To use a service in a component, inject it into the component's constructor:
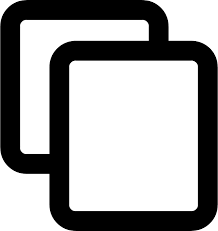
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
data: any;
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.getData().subscribe(response => {
this.data = response;
});
}
}
In this example, the DataService
is injected into the AppComponent
and used to fetch data.
5. Providing Services
Angular services can be provided at different levels:
- Root Level: Use
providedIn: 'root'
in the service's@Injectable
decorator to make it available application-wide. - Module Level: Add the service to the
providers
array in a specific module to make it available only within that module. - Component Level: Add the service to the
providers
array in a specific component's decorator to limit its scope to that component.
6. Benefits of Using Services
- Code Reusability: Encapsulate logic that can be reused across multiple components.
- Modularity: Organize your application into smaller, maintainable pieces.
- Testability: Easily test services in isolation with unit tests.
- Separation of Concerns: Keep components focused on UI logic by delegating business logic to services.
7. Conclusion
Services are an essential part of Angular applications, enabling you to share data and logic between components while adhering to best practices like separation of concerns and dependency injection. By creating and using services effectively, you can build scalable and maintainable applications.
Using Services in Components
1. Overview
In Angular, services are often used to share data, interact with APIs, or perform complex operations. By injecting services into components, you can keep your code modular and maintainable, separating the business logic from the component's presentation logic.
2. Steps to Use a Service in a Component
Follow these steps to use a service in an Angular component:
Step 1: Create or Import a Service
Ensure that you have a service created using the Angular CLI or manually:
ng generate service service-name
For example, consider a service named DataService
:
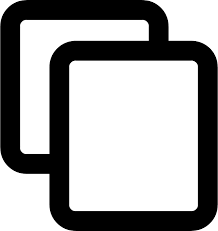
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) { }
getData(): Observable {
return this.http.get(this.apiUrl);
}
}
Step 2: Inject the Service into a Component
To use the service, inject it into the component's constructor:
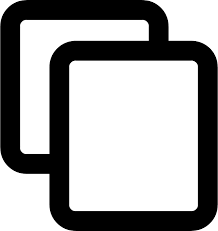
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
data: any;
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.getData().subscribe(response => {
this.data = response;
});
}
}
Here, the DataService
is injected into the component using the constructor. The ngOnInit
lifecycle hook calls the service to fetch data.
Step 3: Use the Service's Data in the Template
Bind the service data to the component's template to display it:
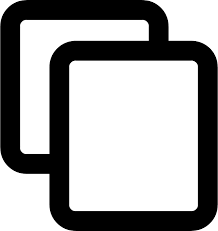
<div *ngIf="data">
<h3>Data from the Service:</h3>
<ul>
<li *ngFor="let item of data">{{ item }}</li>
</ul>
</div>
3. Benefits of Using Services in Components
- Separation of Concerns: Keeps the component focused on UI logic while delegating business logic to the service.
- Reusability: Allows the same service to be used in multiple components.
- Modularity: Makes the codebase easier to maintain and scale.
- Testability: Simplifies testing by mocking the service's functionality during unit tests.
4. Common Use Cases for Services in Components
- Fetching data from APIs using
HttpClient
. - Sharing data between multiple components.
- Handling application-level logic (e.g., authentication or state management).
- Encapsulating business logic or reusable functions.
5. Conclusion
Injecting and using services in Angular components is a fundamental practice that promotes modularity, reusability, and separation of concerns. By leveraging Angular's Dependency Injection system, you can simplify the development process and maintain a clean, scalable codebase.
Using Services in Components
1. Overview
Services in Angular are powerful tools for sharing data, managing application logic, and interacting with APIs. By injecting services into components, you can use their functionality to handle tasks like fetching and managing data, or sharing state between components. This approach adheres to the principles of modularity and separation of concerns.
2. Why Use Services in Components?
- Code Reusability: Avoid duplicating logic by centralizing it in a service.
- State Sharing: Share data and state across multiple components.
- API Interaction: Manage API calls in a service to keep components focused on UI logic.
- Testability: Services can be easily mocked for unit testing components.
3. Steps to Use Services in Components
Step 1: Create a Service
First, generate a service using the Angular CLI:
ng generate service my-service
This creates a new service file (my-service.service.ts
) where you can define logic.
Step 2: Add Logic to the Service
Add the required logic to the service. For example, fetching data from an API:
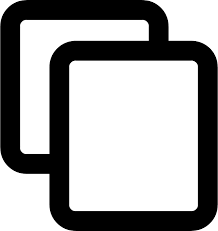
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) { }
getData(): Observable {
return this.http.get(this.apiUrl);
}
}
Step 3: Inject the Service into a Component
Inject the service into the component's constructor:
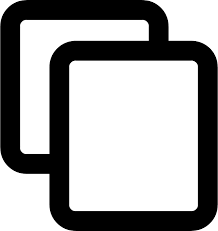
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
data: any;
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.getData().subscribe(response => {
this.data = response;
});
}
}
Here, the DataService
is injected into the AppComponent
. The service's getData()
method is called to fetch data and store it in the component's state.
Step 4: Display the Data in the Template
Bind the data fetched by the service to the component's template:
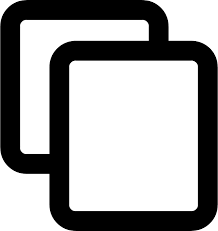
<div *ngIf="data">
<h2>Data from Service</h2>
<ul>
<li *ngFor="let item of data">{{ item }}</li>
</ul>
</div>
4. Providing Services
Angular services are typically provided at the root level using the providedIn: 'root'
syntax. This makes the service available application-wide. Alternatively, you can provide it at the module or component level for a more scoped usage.
5. Benefits of Using Services in Components
- Separation of Concerns: Components handle UI logic, while services manage application logic and data.
- Centralized Logic: Reuse service logic across multiple components.
- Improved Maintenance: Easier to update and debug code when logic is centralized in services.
- Enhanced Testability: Services can be independently tested, reducing the complexity of component tests.
6. Conclusion
Using services in components is a fundamental practice in Angular development. By delegating logic to services and using Dependency Injection, you can create modular, maintainable, and testable applications. This approach also simplifies component design, keeping the focus on UI rendering and interaction.
Dependency Injection Basics
1. Overview
Dependency Injection (DI) is a design pattern used in Angular to provide components and services with the dependencies they need. Angular’s DI system simplifies the process of managing dependencies, making the code more modular, testable, and maintainable.
2. What is Dependency Injection?
Dependency Injection is a technique where an object receives the dependencies it needs from an external source rather than creating them internally. This approach decouples components and services, allowing for more flexible and reusable code.
3. How Dependency Injection Works in Angular
Angular's DI system uses an injector to create and supply dependencies. These dependencies are provided through constructors of components, directives, and other services.
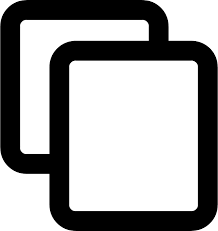
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private dataService: DataService) {
this.dataService.getData().subscribe(data => {
console.log(data);
});
}
}
In this example, the DataService
is injected into the AppComponent
through its constructor. Angular’s DI system manages the creation and provision of the service.
4. Creating a Service
To use DI, start by creating a service. Angular services are decorated with @Injectable
to enable injection.
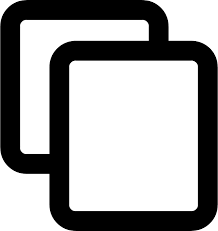
import { Injectable } from '@angular/core';
import { Observable, of } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
getData(): Observable {
return of(['Item 1', 'Item 2', 'Item 3']);
}
}
Here, providedIn: 'root'
ensures that the service is a singleton instance available throughout the application.
5. Providing Dependencies
Angular provides several ways to register and provide dependencies:
- Root Level: Using
providedIn: 'root'
in the service's@Injectable
decorator. - Module Level: Adding services to the
providers
array in a module. - Component Level: Adding services to the
providers
array in a component decorator.
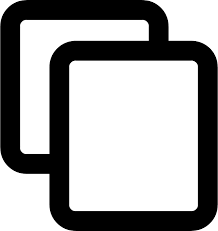
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { DataService } from './data.service';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [DataService],
bootstrap: [AppComponent]
})
export class AppModule { }
6. Benefits of Dependency Injection
- Loose Coupling: Components and services are decoupled, making them independent and reusable.
- Centralized Logic: Shared logic can be centralized in services.
- Testability: Dependencies can be mocked easily for testing.
- Flexibility: Dependencies can be swapped out or updated without changing consuming components.
7. Example Use Case
Consider a scenario where a service fetches data from an API and provides it to a component:
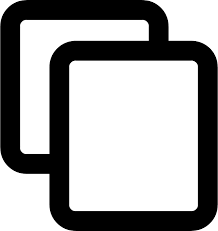
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
data: string[];
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.getData().subscribe(response => {
this.data = response;
});
}
}
In this example, the DataService
is injected into the component. The data fetched by the service is displayed in the component's template.
8. Conclusion
Dependency Injection is a cornerstone of Angular's architecture, enabling developers to write clean, modular, and testable code. By leveraging Angular's DI system, you can manage dependencies efficiently and make your applications more scalable and maintainable.
Making GET, POST, PUT, DELETE Requests
1. Overview
Angular provides the HttpClient
module for making HTTP requests to interact with RESTful APIs. It supports various methods such as GET
, POST
, PUT
, and DELETE
to perform CRUD operations on server resources.
2. Importing HttpClientModule
Before making HTTP requests, you need to import the HttpClientModule
in your application module.
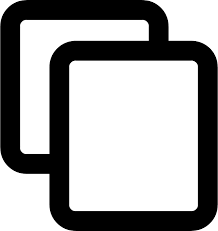
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
HttpClientModule
],
bootstrap: [AppComponent]
})
export class AppModule { }
3. Making HTTP Requests
The HttpClient
service provides methods for performing HTTP operations. Below are examples of each HTTP request type:
3.1 GET Request
Used to retrieve data from a server.
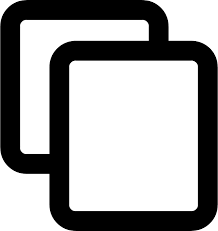
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://jsonplaceholder.typicode.com/posts';
constructor(private http: HttpClient) { }
getPosts(): Observable {
return this.http.get(this.apiUrl);
}
}
3.2 POST Request
Used to send data to the server and create a new resource.
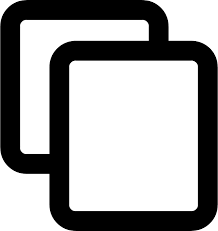
postPost(data: any): Observable {
return this.http.post(this.apiUrl, data);
}
3.3 PUT Request
Used to update an existing resource on the server.
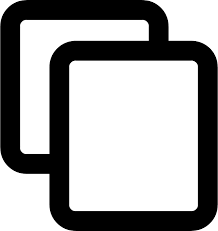
updatePost(id: number, data: any): Observable {
return this.http.put(`${this.apiUrl}/${id}`, data);
}
3.4 DELETE Request
Used to delete an existing resource from the server.
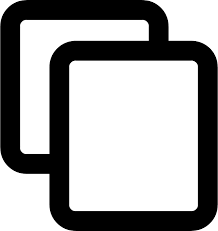
deletePost(id: number): Observable {
return this.http.delete(`${this.apiUrl}/${id}`);
}
4. Using the Service in a Component
Inject the service into a component to call these methods.
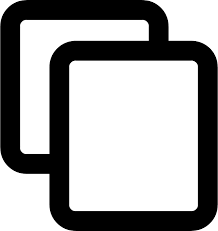
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
posts: any[] = [];
constructor(private dataService: DataService) { }
ngOnInit(): void {
// GET Request
this.dataService.getPosts().subscribe(data => {
this.posts = data;
});
// POST Request
const newPost = { title: 'New Post', body: 'This is a new post' };
this.dataService.postPost(newPost).subscribe(response => {
console.log('Post created:', response);
});
// PUT Request
const updatedPost = { title: 'Updated Post', body: 'Updated content' };
this.dataService.updatePost(1, updatedPost).subscribe(response => {
console.log('Post updated:', response);
});
// DELETE Request
this.dataService.deletePost(1).subscribe(response => {
console.log('Post deleted:', response);
});
}
}
5. Error Handling
Use the catchError
operator from RxJS to handle errors in HTTP requests.
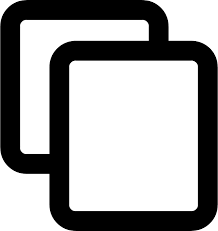
import { catchError } from 'rxjs/operators';
import { throwError } from 'rxjs';
getPosts(): Observable {
return this.http.get(this.apiUrl).pipe(
catchError(error => {
console.error('Error occurred:', error);
return throwError(error);
})
);
}
6. Conclusion
Using the HttpClient
module, Angular makes it easy to perform HTTP operations. By understanding how to use GET
, POST
, PUT
, and DELETE
requests, you can interact effectively with APIs and build dynamic, data-driven applications.
Handling HTTP Errors
1. Overview
Handling HTTP errors is an essential part of building robust applications. Angular's HttpClient
provides a way to handle errors gracefully using RxJS operators like catchError
, throwError
, and more. Proper error handling improves user experience and application reliability.
2. Common HTTP Errors
HTTP errors can occur due to various reasons, such as:
- Client-side errors (4xx): Invalid requests, unauthorized access, or resource not found.
- Server-side errors (5xx): Internal server issues or service unavailability.
- Network errors: Issues with connectivity or timeouts.
3. Using catchError
and throwError
In Angular, you can use the catchError
operator to intercept and handle errors in HTTP requests. The throwError
function is used to rethrow the error after logging or transforming it.
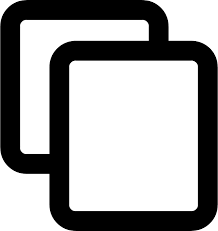
import { Injectable } from '@angular/core';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://jsonplaceholder.typicode.com/posts';
constructor(private http: HttpClient) { }
getPosts(): Observable {
return this.http.get(this.apiUrl).pipe(
catchError(this.handleError)
);
}
private handleError(error: HttpErrorResponse): Observable {
if (error.error instanceof ErrorEvent) {
// Client-side error
console.error('Client-side error:', error.error.message);
} else {
// Server-side error
console.error(`Server-side error: Code ${error.status}, Message: ${error.message}`);
}
return throwError('Something went wrong. Please try again later.');
}
}
4. Example: Handling Errors in Components
Inject the service into a component and display error messages to the user when an error occurs.
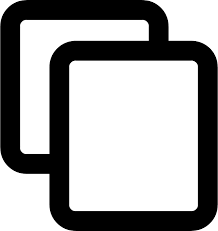
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
posts: any[] = [];
errorMessage: string = '';
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.getPosts().subscribe({
next: (data) => {
this.posts = data;
},
error: (error) => {
this.errorMessage = error;
console.error('Error fetching posts:', error);
}
});
}
}
5. Global HTTP Error Handling
You can handle errors globally using Angular's HttpInterceptor
. This allows you to catch and process errors across the entire application.
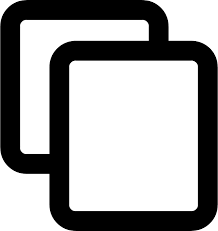
import { Injectable } from '@angular/core';
import { HttpEvent, HttpInterceptor, HttpHandler, HttpRequest, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
@Injectable()
export class ErrorInterceptor implements HttpInterceptor {
intercept(req: HttpRequest, next: HttpHandler): Observable> {
return next.handle(req).pipe(
catchError((error: HttpErrorResponse) => {
console.error('HTTP Error:', error);
return throwError('A global error occurred. Please try again later.');
})
);
}
}
6. Registering the Interceptor
Register the interceptor in the providers array of your application module.
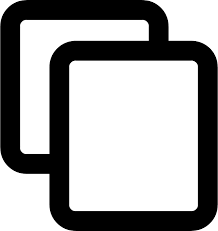
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { AppComponent } from './app.component';
import { ErrorInterceptor } from './error.interceptor';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
HttpClientModule
],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: ErrorInterceptor, multi: true }
],
bootstrap: [AppComponent]
})
export class AppModule { }
7. Conclusion
By handling HTTP errors effectively, you can ensure a better user experience and more resilient applications. Angular's HttpClient
and RxJS operators provide powerful tools for managing errors in both individual requests and globally across your application.
Interceptors
1. Overview
Interceptors in Angular are a powerful way to intercept and modify HTTP requests or responses globally. They allow you to add custom logic, such as adding authentication tokens, logging, or transforming data, to all HTTP requests and responses without modifying individual service methods.
2. What Are Interceptors?
Interceptors are part of Angular's HttpClient
module and implement the HttpInterceptor
interface. They act as middleware for HTTP requests and responses, enabling developers to:
- Modify or add headers to HTTP requests.
- Handle authentication tokens.
- Log or monitor HTTP traffic.
- Process or transform HTTP responses.
- Handle errors globally.
3. Creating an Interceptor
To create an interceptor, implement the HttpInterceptor
interface and define the intercept
method.
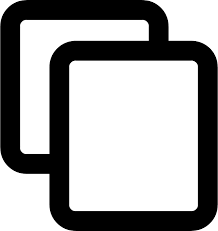
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest, next: HttpHandler): Observable> {
const authToken = 'Bearer your-auth-token'; // Replace with your actual token
const clonedRequest = req.clone({
setHeaders: {
Authorization: authToken
}
});
console.log('Interceptor added token:', clonedRequest);
return next.handle(clonedRequest);
}
}
4. Registering an Interceptor
Once created, register the interceptor in your app module by adding it to the providers
array.
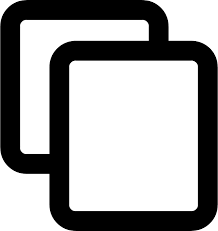
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { AppComponent } from './app.component';
import { AuthInterceptor } from './auth.interceptor';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, HttpClientModule],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true }
],
bootstrap: [AppComponent]
})
export class AppModule { }
5. Adding Multiple Interceptors
You can add multiple interceptors to handle different aspects of HTTP requests or responses. The interceptors are executed in the order they are provided in the providers
array.
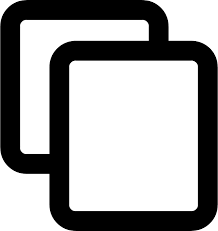
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
import { tap } from 'rxjs/operators';
@Injectable()
export class LoggingInterceptor implements HttpInterceptor {
intercept(req: HttpRequest, next: HttpHandler): Observable> {
console.log('Request made:', req);
return next.handle(req).pipe(
tap(event => {
console.log('Response received:', event);
})
);
}
}
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true },
{ provide: HTTP_INTERCEPTORS, useClass: LoggingInterceptor, multi: true }
]
6. Benefits of Using Interceptors
- Centralized Logic: Handle common tasks like authentication and logging in one place.
- Reusability: Share the same interceptor across multiple modules or services.
- Ease of Maintenance: Modify a single interceptor to update behavior across the application.
7. Example: Error Handling with Interceptors
Interceptors are also useful for handling HTTP errors globally. For example:
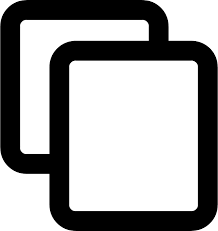
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
@Injectable()
export class ErrorInterceptor implements HttpInterceptor {
intercept(req: HttpRequest, next: HttpHandler): Observable {
return next.handle(req).pipe(
catchError((error: HttpErrorResponse) => {
console.error('HTTP Error:', error);
alert('An error occurred: ' + error.message);
return throwError(error);
})
);
}
}
8. Conclusion
Interceptors are a powerful feature in Angular that enables developers to handle HTTP requests and responses at a global level. By using interceptors effectively, you can simplify your application code, enhance security, and improve maintainability.
Using RxJS for State Management
1. Overview
RxJS (Reactive Extensions for JavaScript) is a powerful library for reactive programming using observables. It provides tools to handle asynchronous data streams efficiently. In Angular, RxJS can be used for state management to manage shared state across components in a reactive and predictable manner.
2. Why Use RxJS for State Management?
- Reactive Updates: Automatically update components when the state changes.
- Decoupled Logic: Separate state management logic from UI components.
- Stream Control: Handle multiple streams of data efficiently using operators like
map
,filter
, andcombineLatest
. - Lightweight Alternative: Use RxJS as a lightweight alternative to full-fledged state management libraries like NgRx or Akita.
3. Setting Up a State Management Service
Create a dedicated service to manage the application state. This service will use RxJS BehaviorSubject
or ReplaySubject
for maintaining and broadcasting the state.
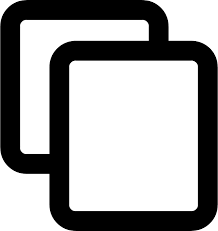
import { Injectable } from '@angular/core';
import { BehaviorSubject, Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class StateService {
private stateSubject = new BehaviorSubject({}); // Initial state
state$: Observable = this.stateSubject.asObservable();
constructor() {}
// Update state
updateState(newState: any): void {
this.stateSubject.next({ ...this.stateSubject.value, ...newState });
}
// Get current state
getState(): any {
return this.stateSubject.value;
}
}
4. Using the State Service in Components
Inject the state service into components to manage or subscribe to the state.
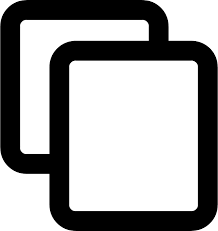
import { Component } from '@angular/core';
import { StateService } from './state.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
state: any;
constructor(private stateService: StateService) {
// Subscribe to state changes
this.stateService.state$.subscribe(newState => {
this.state = newState;
console.log('State updated:', this.state);
});
}
// Update state
updateState(): void {
this.stateService.updateState({ user: 'John Doe', age: 30 });
}
}
5. Handling Complex State with RxJS Operators
Use RxJS operators like map
, filter
, and combineLatest
for complex state transformations.
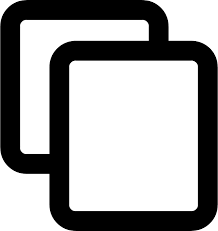
import { Injectable } from '@angular/core';
import { BehaviorSubject, Observable, combineLatest } from 'rxjs';
import { map } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class StateService {
private userSubject = new BehaviorSubject(null);
private preferencesSubject = new BehaviorSubject({ theme: 'light' });
user$ = this.userSubject.asObservable();
preferences$ = this.preferencesSubject.asObservable();
// Combine user and preferences streams
combinedState$: Observable = combineLatest([this.user$, this.preferences$]).pipe(
map(([user, preferences]) => ({ user, preferences }))
);
updateUser(user: any): void {
this.userSubject.next(user);
}
updatePreferences(preferences: any): void {
this.preferencesSubject.next(preferences);
}
}
6. Example Usage in a Component
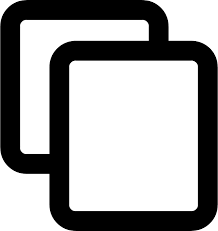
import { Component } from '@angular/core';
import { StateService } from './state.service';
@Component({
selector: 'app-user',
template: `
<div *ngIf="combinedState">
<h3>User: {{ combinedState.user?.name }}</h3>
<p>Theme: {{ combinedState.preferences?.theme }}</p>
</div>
<button (click)="changeTheme()">Toggle Theme</button>
`
})
export class UserComponent {
combinedState: any;
constructor(private stateService: StateService) {
this.stateService.combinedState$.subscribe(state => {
this.combinedState = state;
});
}
changeTheme(): void {
const newTheme = this.combinedState.preferences?.theme === 'light' ? 'dark' : 'light';
this.stateService.updatePreferences({ theme: newTheme });
}
}
7. Benefits of RxJS for State Management
- Real-time Updates: Automatically propagate changes to all subscribers.
- Modular and Scalable: Manage complex state transformations using RxJS operators.
- Lightweight: Avoid introducing additional state management libraries.
- Reactive Design: Seamlessly handle asynchronous data streams.
8. Conclusion
Using RxJS for state management in Angular provides a reactive, lightweight, and efficient way to handle shared state across components. By leveraging RxJS operators, you can manage complex state logic and ensure your application remains maintainable and scalable.
Observables and Subscriptions
1. Overview
Observables are a key feature of RxJS and Angular. They represent data streams that can emit multiple values over time. Subscriptions allow us to listen to these data streams and react to emitted values. Together, they are fundamental to handling asynchronous programming in Angular.
2. What are Observables?
An Observable is a data producer that emits values to its subscribers. Observables can emit data synchronously or asynchronously, and they are often used to handle HTTP requests, user input events, or other streams of data.
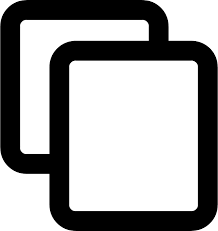
import { Observable } from 'rxjs';
const observable = new Observable(observer => {
observer.next('First value');
observer.next('Second value');
setTimeout(() => observer.next('Third value'), 1000);
setTimeout(() => observer.complete(), 2000);
});
observable.subscribe({
next: value => console.log(value),
complete: () => console.log('Observable complete'),
});
3. Subscribing to Observables
To consume values from an Observable, you need to subscribe to it. A subscription represents the execution of an Observable.
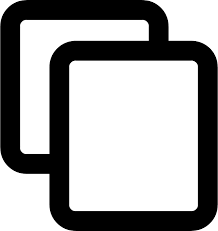
import { Observable } from 'rxjs';
const numbers$ = new Observable(observer => {
observer.next(1);
observer.next(2);
observer.complete();
});
const subscription = numbers$.subscribe({
next: value => console.log('Received value:', value),
complete: () => console.log('All values received'),
});
// Unsubscribe to avoid memory leaks
subscription.unsubscribe();
4. Common Use Cases in Angular
- HTTP Requests: Use Observables to handle data from HTTP requests.
- Event Streams: Listen to user interactions like clicks or keypresses.
- WebSocket Communication: Handle real-time updates.
- Form Value Changes: Watch form control values change over time.
5. Using Observables in Angular
Angular integrates Observables into many of its core features, such as HTTPClient and Reactive Forms.
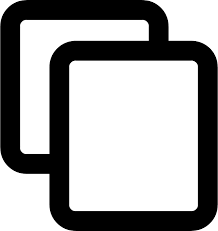
import { HttpClient } from '@angular/common/http';
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-data',
template: 'Data: {{ data | json }}
',
})
export class DataComponent implements OnInit {
data: any;
constructor(private http: HttpClient) {}
ngOnInit(): void {
this.http.get('https://api.example.com/data').subscribe(response => {
this.data = response;
});
}
}
6. Managing Subscriptions
Managing subscriptions is crucial to avoid memory leaks. Angular provides tools like the takeUntil
operator and the async
pipe to simplify subscription management.
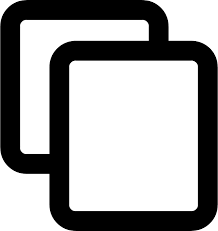
import { Component, OnDestroy } from '@angular/core';
import { Subject } from 'rxjs';
import { takeUntil } from 'rxjs/operators';
@Component({
selector: 'app-example',
template: 'Example component
',
})
export class ExampleComponent implements OnDestroy {
private destroy$ = new Subject();
constructor() {
const observable$ = new Observable(observer => {
observer.next('Value');
});
observable$.pipe(takeUntil(this.destroy$)).subscribe(value => {
console.log(value);
});
}
ngOnDestroy(): void {
this.destroy$.next();
this.destroy$.complete();
}
}
7. Benefits of Using Observables
- Asynchronous Handling: Observables are designed to work seamlessly with asynchronous data streams.
- Powerful Operators: Use RxJS operators like
map
,filter
, andmergeMap
for complex data transformations. - Flexibility: Observables can handle multiple values over time, unlike Promises.
- Integration: Deep integration with Angular’s core features like HTTPClient and Reactive Forms.
8. Conclusion
Observables and subscriptions form the backbone of reactive programming in Angular. By leveraging RxJS, you can handle asynchronous operations efficiently and build highly interactive and responsive applications. Proper subscription management ensures optimal performance and avoids memory leaks.
Angular NgRx Basics
1. Overview
NgRx is a powerful state management library for Angular applications, based on Redux principles. It provides a reactive approach to managing the state, actions, and side effects of your app. NgRx uses the Observable pattern to manage state, which makes it a perfect fit for Angular's reactive programming model.
2. What is NgRx?
NgRx is a state management solution inspired by Redux but specifically designed for Angular. It helps maintain consistent, predictable state across your application by managing the flow of data and updates in a centralized store. The state is immutable, and updates to the state are made only through actions, which are processed by reducers.
3. Core Concepts of NgRx
NgRx is built around several core concepts:
- Store: A single, immutable state tree that holds the application state.
- Actions: Payloads of information that express a state change.
- Reducers: Functions that specify how the state should change in response to an action.
- Selectors: Functions to query the store for specific pieces of state.
- Effects: Handle side effects like HTTP requests or other asynchronous operations.
4. Setting up NgRx in Angular
To set up NgRx in your Angular project, you need to install the NgRx packages and import them into your app module.
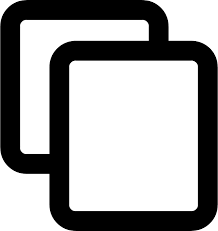
# Install NgRx packages
npm install @ngrx/store @ngrx/effects @ngrx/store-devtools
5. Creating Actions in NgRx
Actions are events that describe a state change. In NgRx, actions are defined using the createAction
function. They can optionally carry a payload.
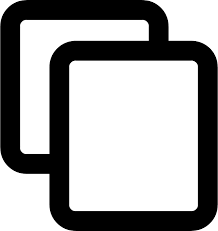
import { createAction, props } from '@ngrx/store';
export const loadData = createAction('[Data] Load Data');
export const loadDataSuccess = createAction(
'[Data] Load Data Success',
props<{ data: any[] }>()
);
export const loadDataFailure = createAction(
'[Data] Load Data Failure',
props<{ error: any }>()
);
6. Creating Reducers in NgRx
Reducers are pure functions that specify how the state changes in response to an action. They take the current state and an action as arguments, and return a new state.
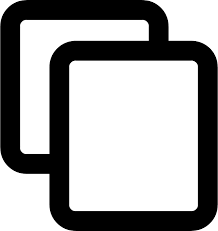
import { createReducer, on } from '@ngrx/store';
import { loadData, loadDataSuccess, loadDataFailure } from './data.actions';
export const initialState = {
data: [],
error: null,
};
const _dataReducer = createReducer(
initialState,
on(loadData, state => ({ ...state, data: [] })),
on(loadDataSuccess, (state, { data }) => ({ ...state, data })),
on(loadDataFailure, (state, { error }) => ({ ...state, error }))
);
export function dataReducer(state, action) {
return _dataReducer(state, action);
}
7. Selecting State from the Store
Selectors allow you to query the store for specific slices of state. They are often used to retrieve data that is needed in your components.
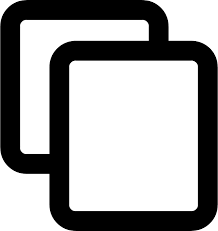
import { createFeatureSelector, createSelector } from '@ngrx/store';
export const selectData = createFeatureSelector('data');
export const selectDataItems = createSelector(
selectData,
state => state.data
);
8. Using Effects in NgRx
Effects are used to handle side effects like HTTP requests, navigation, or other asynchronous tasks. They listen to specific actions and can dispatch new actions based on the result of the side effect.
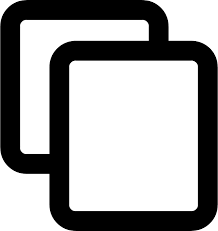
import { Injectable } from '@angular/core';
import { Actions, ofType } from '@ngrx/effects';
import { Observable } from 'rxjs';
import { map, catchError, switchMap } from 'rxjs/operators';
import { DataService } from './data.service';
import { loadData, loadDataSuccess, loadDataFailure } from './data.actions';
@Injectable()
export class DataEffects {
loadData$ = createEffect(() =>
this.actions$.pipe(
ofType(loadData),
switchMap(() =>
this.dataService.getData().pipe(
map(data => loadDataSuccess({ data })),
catchError(error => [loadDataFailure({ error })])
)
)
)
);
constructor(private actions$: Actions, private dataService: DataService) {}
}
9. Registering Store, Reducers, and Effects
In the Angular module, you need to register the store and effects so that NgRx can manage the state and actions.
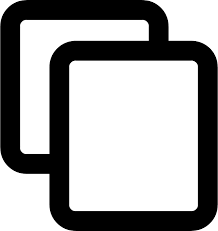
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { StoreModule } from '@ngrx/store';
import { EffectsModule } from '@ngrx/effects';
import { AppComponent } from './app.component';
import { dataReducer } from './state/data.reducer';
import { DataEffects } from './state/data.effects';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
StoreModule.forRoot({ data: dataReducer }),
EffectsModule.forRoot([DataEffects]),
],
bootstrap: [AppComponent],
})
export class AppModule {}
10. Benefits of NgRx
- Centralized State Management: NgRx centralizes your application's state, making it easier to manage and debug.
- Predictable State Changes: State changes are predictable and traceable through actions and reducers.
- Reactive Programming: NgRx integrates seamlessly with Angular's reactive programming model, making it a natural choice for Angular apps.
- Side Effects Management: Effects handle side effects (like HTTP requests) in a clean, organized manner.
11. Conclusion
NgRx provides a robust state management solution for Angular applications, making it easier to manage complex states and side effects. By adopting NgRx, you can build scalable, predictable, and maintainable Angular applications.
Built-in Directives (ngIf, ngFor, ngSwitch, etc.)
1. Overview
Angular provides several built-in directives to help you control the layout and behavior of the elements in your templates. Directives are special markers on elements in the DOM that allow you to attach behavior to them. The most commonly used built-in directives in Angular are ngIf
, ngFor
, and ngSwitch
.
2. ngIf Directive
The ngIf
directive conditionally includes an element in the DOM based on the value of an expression. If the expression evaluates to true, the element is included, otherwise, it is excluded.
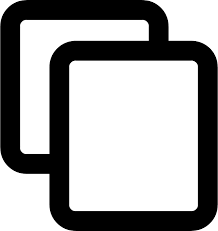
<div *ngIf="isVisible">This message is visible</div>
In this example, the ngIf
directive checks the isVisible
property. If it’s true, the message "This message is visible" will be displayed.
3. ngFor Directive
The ngFor
directive is used to repeat an element for each item in an array or list. It is commonly used to display lists of data dynamically.
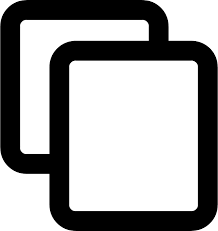
<ul>
<li *ngFor="let item of items">{{ item }}></li>
</ul>
In this example, the ngFor
directive loops through the items
array and displays each item as a list element.
4. ngSwitch Directive
The ngSwitch
directive is used to conditionally display elements based on a matching expression. It works similarly to the switch
statement in JavaScript. You use ngSwitch
on a container element and ngSwitchCase
on individual elements to specify which element to display based on the value of the expression.
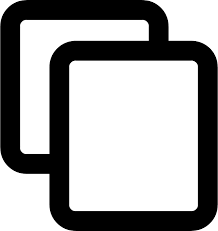
<div [ngSwitch]="color">
<div *ngSwitchCase="'red'">The color is red</div>
<div *ngSwitchCase="'blue'">The color is blue</div>
<div *ngSwitchDefault>The color is unknown</div>
</div>
In this example, the content is displayed based on the value of the color
variable. If color
is 'red', the first div
will be shown, and if it's 'blue', the second will be shown. If it doesn’t match any case, the ngSwitchDefault
will be used.
5. ngClass Directive
The ngClass
directive is used to dynamically add or remove CSS classes from an element. It can be used with either an object, array, or string to control which classes are applied.
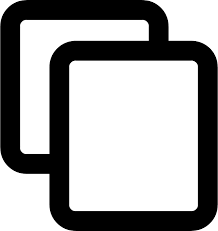
<div [ngClass]="{ 'active': isActive, 'disabled': isDisabled }">This element's classes are dynamic</div>
In this example, the ngClass
directive applies the 'active'
class if isActive
is true, and the 'disabled'
class if isDisabled
is true.
6. ngStyle Directive
The ngStyle
directive is used to dynamically set inline styles for an element based on an expression.
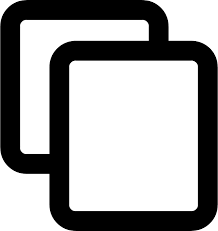
<div [ngStyle]="{ 'background-color': color, 'font-size': fontSize + 'px' }">This element has dynamic styles</div>
In this example, the background color and font size are set dynamically based on the values of color
and fontSize
.
7. ngModel Directive
The ngModel
directive is used for two-way data binding in Angular. It allows you to bind the value of an input field to a variable and keep them in sync.
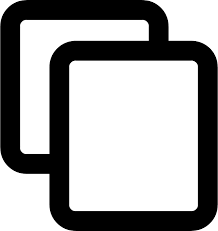
<input [(ngModel)]="userName" placeholder="Enter your name">
<p>Your name is: {{ userName }}</p>
In this example, the ngModel
directive binds the userName
variable to the input field. Any changes made in the input field will automatically update userName
, and vice versa.
8. Conclusion
Angular's built-in directives like ngIf
, ngFor
, and ngSwitch
are essential for controlling the behavior and layout of elements in your templates. They allow for dynamic rendering of content, conditional displays, and efficient iteration over collections, making Angular an incredibly powerful framework for building interactive web applications.
Custom Directives
1. Overview
In Angular, directives are used to manipulate the DOM, and they can be either built-in or custom. Custom directives allow you to create reusable behavior that can be applied to DOM elements. You can create custom directives to encapsulate custom functionality, such as changing the appearance of an element, handling user events, or applying complex logic to an element.
2. Types of Custom Directives
Angular supports three types of custom directives:
- Attribute Directives: These directives are used to change the appearance or behavior of an existing element. Example:
ngClass
,ngStyle
. - Structural Directives: These directives change the structure of the DOM, such as adding or removing elements. Example:
ngIf
,ngFor
. - Component Directives: These are not custom directives but are essentially Angular components that are a special type of directive.
3. Creating an Attribute Directive
Let’s create a simple custom directive that changes the background color of an element on mouse hover. This will be an attribute directive.
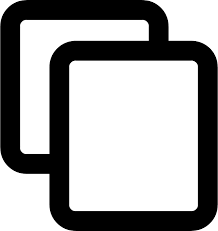
import { Directive, ElementRef, Renderer2, HostListener } from '@angular/core';
@Directive({
selector: '[appHoverBackground]'
})
export class HoverBackgroundDirective {
constructor(private el: ElementRef, private renderer: Renderer2) {}
@HostListener('mouseenter') onMouseEnter() {
this.renderer.setStyle(this.el.nativeElement, 'background-color', 'yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.renderer.removeStyle(this.el.nativeElement, 'background-color');
}
}
In this example, the HoverBackgroundDirective
changes the background color of an element to yellow when the mouse enters the element and removes the background color when the mouse leaves.
4. Using the Attribute Directive
To use the appHoverBackground
directive, you simply add it as an attribute to an element in your template.
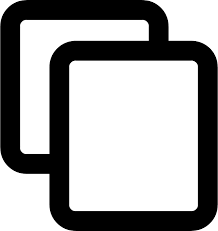
<div appHoverBackground>
Hover over me to change my background color!
</div>
In this example, the directive is applied to a div
element. When the mouse hovers over the element, its background color changes to yellow.
5. Creating a Structural Directive
Now let’s create a custom structural directive. A structural directive is used to modify the structure of the DOM. For example, let’s create a directive that shows an element only if the user is logged in.
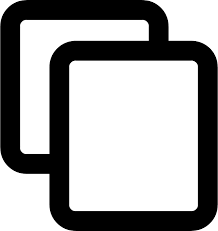
import { Directive, Input, TemplateRef, ViewContainerRef } from '@angular/core';
@Directive({
selector: '[appShowIfLoggedIn]'
})
export class ShowIfLoggedInDirective {
@Input() set appShowIfLoggedIn(isLoggedIn: boolean) {
if (isLoggedIn) {
this.viewContainer.createEmbeddedView(this.templateRef);
} else {
this.viewContainer.clear();
}
}
constructor(private templateRef: TemplateRef, private viewContainer: ViewContainerRef) {}
}
In this example, the ShowIfLoggedInDirective
will only display the content inside the element if the isLoggedIn
condition is true. If it’s false, the content is removed from the DOM.
6. Using the Structural Directive
To use the appShowIfLoggedIn
directive, you can apply it to any element and pass the condition as an argument.
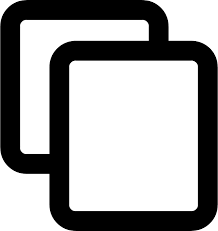
<div *appShowIfLoggedIn="isLoggedIn">
You are logged in!
</div>
In this example, the content inside the div
will only be displayed if the isLoggedIn
property is true. If the user is not logged in, the content will not appear in the DOM.
7. Conclusion
Custom directives in Angular allow you to extend the functionality of your application and create reusable, encapsulated logic that can be applied to your templates. By creating custom attribute and structural directives, you can significantly improve code reuse, maintainability, and customization of your application’s UI behavior.
Using Built-in Pipes
1. Overview
Pipes in Angular are used to transform data in templates. They provide a convenient way to modify data before displaying it to the user. Angular offers several built-in pipes, which are commonly used for formatting data, such as dates, numbers, currencies, and more. These pipes can be used directly in the templates without needing additional logic in the component class.
2. Common Built-in Pipes
Angular provides the following built-in pipes:
- CurrencyPipe: Formats a number as currency.
- DatePipe: Formats a date object into a human-readable string.
- DecimalPipe: Formats a number to a specified decimal format.
- PercentPipe: Converts a number into a percentage string.
- LowerCasePipe: Transforms a string to lowercase.
- UpperCasePipe: Transforms a string to uppercase.
- JsonPipe: Converts an object into a JSON-formatted string.
- SlicePipe: Creates a sub-array or substring from a given array or string.
- AsyncPipe: Unwraps values from asynchronous operations like Observables and Promises.
3. Using the DatePipe
The DatePipe
allows you to format dates into different string formats. It is very useful for displaying date information in a user-friendly format.
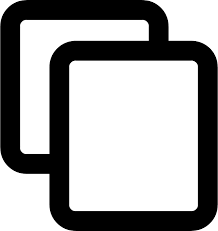
<p>The current date is: {{ currentDate | date:'fullDate' }}</p>
<p>Short date format: {{ currentDate | date:'shortDate' }}</p>
<p>Time format: {{ currentDate | date:'shortTime' }}</p>
In this example, the currentDate
will be displayed in various formats, such as 'fullDate'
, 'shortDate'
, and 'shortTime'
.
4. Using the CurrencyPipe
The CurrencyPipe
formats a number as a currency value. You can also specify the currency code and locale to tailor the output.
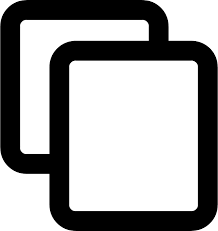
<p>Product price: {{ productPrice | currency:'USD':'symbol' }}</p>
<p>Product price (localized): {{ productPrice | currency:'EUR':'code':'1.2-2' }}</p>
In this example, productPrice
is formatted as USD and EUR currency, with different symbols and formatting.
5. Using the UpperCasePipe and LowerCasePipe
The UpperCasePipe
and LowerCasePipe
are used to transform strings to uppercase or lowercase, respectively.
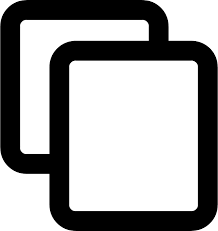
<p>Uppercase: {{ 'hello world' | uppercase }}</p>
<p>Lowercase: {{ 'HELLO WORLD' | lowercase }}</p>
In this example, the text 'hello world' is transformed to uppercase, and 'HELLO WORLD' is transformed to lowercase.
6. Using the JsonPipe
The JsonPipe
is useful for converting complex objects or arrays into JSON strings for debugging or displaying raw data.
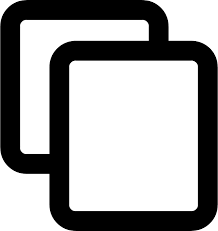
<p>JSON Object: {{ user | json }}</p>
In this example, the user
object will be displayed as a JSON string in the template.
7. Using the PercentPipe
The PercentPipe
formats a number as a percentage. It multiplies the input by 100 and adds a percent sign at the end.
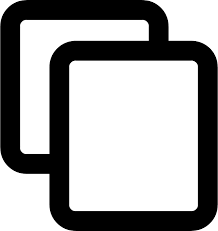
<p>Completion: {{ completionPercentage | percent }}</p>
In this example, completionPercentage
will be displayed as a percentage (e.g., 0.75 becomes 75%).
8. Using the SlicePipe
The SlicePipe
is used to slice a string or array into a subset of its elements. It can be useful for pagination or trimming long texts.
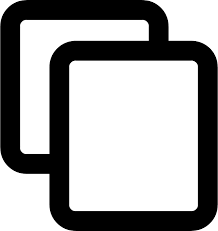
<p>First 5 characters: {{ 'Angular is awesome!' | slice:0:5 }}</p>
<p>Array slice: {{ [1, 2, 3, 4, 5] | slice:2:4 }}</p>
In this example, the slice
pipe is used to extract a portion of a string and an array.
9. Conclusion
Built-in pipes in Angular provide a powerful and flexible way to transform data in templates. They allow you to format dates, numbers, strings, and more without writing custom logic in your components. By understanding and utilizing these pipes, you can easily enhance the user experience with well-formatted and dynamic data presentation.
Creating Custom Pipes
1. Overview
In Angular, custom pipes allow you to transform data in ways that are not covered by the built-in pipes. Creating your own pipes gives you the flexibility to implement specific data transformations that are unique to your application. Custom pipes can be used for various tasks, such as formatting data, filtering lists, or performing calculations in templates.
2. How to Create a Custom Pipe
To create a custom pipe, you need to follow these steps:
- Generate a pipe using Angular CLI or manually create a pipe class.
- Implement the transformation logic in the
transform
method of the pipe class. - Register the pipe in the
declarations
array of the module.
3. Creating a Simple Custom Pipe
Let's create a simple custom pipe that formats a string by appending a suffix.
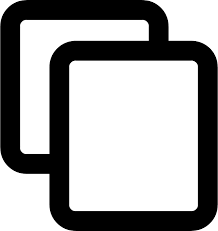
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'suffix'
})
export class SuffixPipe implements PipeTransform {
transform(value: string, suffix: string = '!!!'): string {
return value + suffix;
}
}
In this example, the SuffixPipe
appends a suffix (default is '!!!') to the input string.
4. Using the Custom Pipe in Templates
Once the custom pipe is created, you can use it in any template just like built-in pipes. Here's an example of using the suffix
pipe:
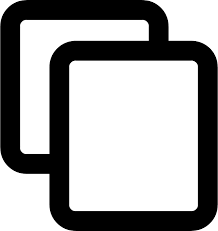
<p>{{ 'Hello Angular' | suffix }}</p>
<p>{{ 'Welcome' | suffix:'!' }}</p>
In this example, the string 'Hello Angular' will be transformed to 'Hello Angular!!!', and the string 'Welcome' will be transformed to 'Welcome!'.
5. Registering the Custom Pipe in a Module
After creating your custom pipe, you need to add it to the module’s declarations
array to make it available throughout your application.
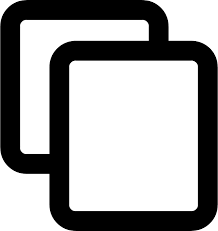
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { SuffixPipe } from './suffix.pipe';
@NgModule({
declarations: [
AppComponent,
SuffixPipe
],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
In this example, we register the SuffixPipe
in the declarations
array of the AppModule
, making it available throughout the application.
6. Custom Pipe with Multiple Arguments
Custom pipes can accept multiple arguments. Let's modify the suffix
pipe to allow the user to specify both a prefix and a suffix.
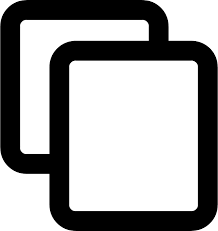
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'prefixSuffix'
})
export class PrefixSuffixPipe implements PipeTransform {
transform(value: string, prefix: string = '', suffix: string = ''): string {
return prefix + value + suffix;
}
}
In this example, the PrefixSuffixPipe
allows you to add both a prefix and a suffix to the string.
7. Using the Custom Pipe with Multiple Arguments
Here is how you can use the prefixSuffix
pipe in a template:
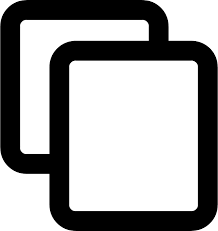
<p>{{ 'Angular' | prefixSuffix:'Learn ':' Rocks' }}</p>
In this example, the string 'Angular' will be transformed to 'Learn Angular Rocks'.
8. Optimizing Custom Pipes
Custom pipes can be optimized by implementing the Pure
and Impure
pipe strategies:
- Pure Pipes: The default behavior, where the transformation is done only when the input value changes.
- Impure Pipes: Always run the transformation when the component’s change detection runs, even if the input value hasn't changed.
To make a pipe impure, set the pure
property to false
in the @Pipe
decorator.
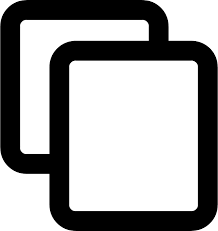
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'impureSuffix',
pure: false
})
export class ImpureSuffixPipe implements PipeTransform {
transform(value: string, suffix: string = ''): string {
return value + suffix;
}
}
In this example, the ImpureSuffixPipe
will always transform the value whenever change detection runs, even if the input hasn't changed.
9. Conclusion
Custom pipes are a powerful feature in Angular that allow you to encapsulate and reuse data transformation logic in templates. By creating custom pipes, you can extend Angular's built-in functionality to meet the specific needs of your application. Custom pipes are easy to create and use, and they provide a clean and efficient way to manage complex data transformations in your templates.
Setting up Angular Material
1. Overview
Angular Material is a UI component library for Angular applications that implements Google's Material Design principles. It provides a set of reusable, well-tested, and accessible UI components that follow Material Design guidelines. Setting up Angular Material in your Angular project allows you to quickly build responsive, modern, and user-friendly interfaces.
2. Prerequisites
Before setting up Angular Material, make sure you have the following:
- Angular CLI installed. If you don't have it, you can install it using
npm install -g @angular/cli
. - An Angular project created using Angular CLI. If you don't have an Angular project, create one with
ng new your-project-name
.
3. Installing Angular Material
The first step in setting up Angular Material is to install it using npm. Run the following command in your project directory:
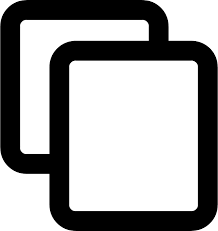
npm install @angular/material @angular/cdk @angular/animations
This command installs Angular Material, the Component Dev Kit (CDK), and Angular Animations, which are required for Material components.
4. Adding Angular Material Modules
Once Angular Material is installed, you need to import the necessary Material modules into your application. Start by importing the MatButtonModule
as an example:
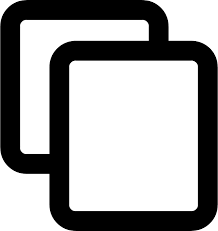
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { MatButtonModule } from '@angular/material/button'; // Importing MatButtonModule
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
MatButtonModule // Adding MatButtonModule to imports
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
In this example, we import the MatButtonModule
and add it to the imports
array in the AppModule
so that we can use Material buttons in our application.
5. Importing Other Angular Material Modules
Angular Material provides a wide range of components such as buttons, forms, cards, dialogs, etc. Here’s how you can import additional components:
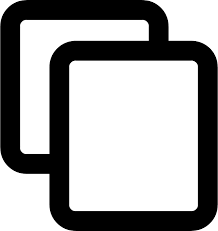
import { MatCardModule } from '@angular/material/card';
import { MatInputModule } from '@angular/material/input';
import { MatIconModule } from '@angular/material/icon';
@NgModule({
imports: [
MatCardModule, // For Material Cards
MatInputModule, // For Material Inputs
MatIconModule, // For Material Icons
],
})
export class AppModule { }
In this example, we import MatCardModule
, MatInputModule
, and MatIconModule
to use Material cards, input fields, and icons in our application.
6. Using Angular Material Components
Once the necessary modules are imported, you can start using Material components in your templates. Here’s an example using the Material Button and Card:
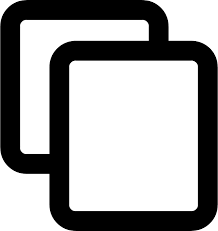
Angular Material Card
This is a card content. You can add any content you want here.
In this example, we use the Material Card component to display a simple card, and a Material Button inside the card's actions section.
7. Adding a Theme
Angular Material requires a theme to style the components. You can choose one of the pre-built themes or create your own. To add a pre-built theme, include the following line in your styles file (styles.css
or styles.scss
):
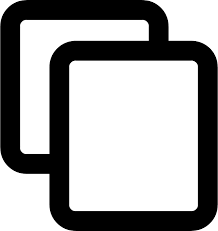
/* styles.css or styles.scss */
@import '~@angular/material/prebuilt-themes/indigo-pink.css';
In this example, we import the indigo-pink
pre-built theme. You can replace it with other themes such as deeppurple-amber.css
, pink-bluegrey.css
, or purple-green.css
.
8. Setting Up the Angular Material Typography
To enable Material typography, you need to import the Material Typography module in your styles:
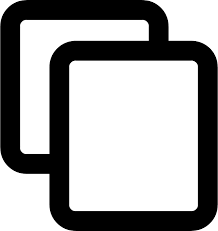
/* styles.css or styles.scss */
@import '~@angular/material/core/theming/all-theme';
@include mat-core();
This will automatically apply Material Design typography styles to your application.
9. Conclusion
Setting up Angular Material in your project is a simple process that can greatly improve the look and feel of your application. With pre-built components and themes, Angular Material allows you to create modern, responsive user interfaces quickly and efficiently. You can further customize the components and styles to suit your project's needs.
Using Angular Material Components (Buttons, Cards, Forms, etc.)
1. Overview
Angular Material provides a wide range of pre-built UI components that implement Google's Material Design principles. These components are designed to be easy to use and customizable, helping developers create beautiful and responsive UIs quickly. Some of the most commonly used components include buttons, cards, forms, inputs, and more.
2. Importing Angular Material Components
Before using any Angular Material components, you need to import the corresponding modules into your AppModule
. Here’s how to import the modules for buttons, cards, and form controls:
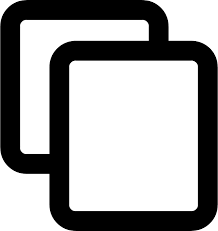
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { MatButtonModule } from '@angular/material/button'; // Importing MatButtonModule
import { MatCardModule } from '@angular/material/card'; // Importing MatCardModule
import { MatInputModule } from '@angular/material/input'; // Importing MatInputModule
import { MatFormFieldModule } from '@angular/material/form-field'; // Importing MatFormFieldModule
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
MatButtonModule,
MatCardModule,
MatInputModule,
MatFormFieldModule
],
bootstrap: [AppComponent]
})
export class AppModule { }
In this example, we’ve imported the necessary modules for the Material Button, Card, Input, and Form Field components.
3. Using Material Buttons
Material buttons are used to trigger actions in your application. You can create a simple button using the mat-button
directive:
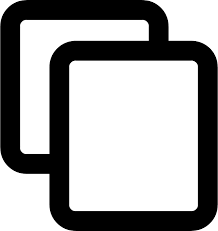
<button mat-button>Click Me</button>
The mat-button
directive styles the button to follow Material Design principles. You can also add additional properties such as color="primary"
to change the button's color:
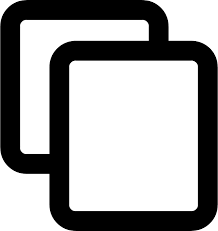
<button mat-button color="primary">Primary Button</button>
4. Using Material Cards
Material cards are used to display content in a flexible and consistent layout. A basic card can be created using the mat-card
component:
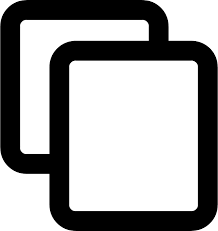
Card Title
<p>This is some content inside a material card. You can add text, images, and other components here.</p>
<button mat-button>Action</button>
This example shows how to add a header, content, and actions to a Material Card, along with a Material Button inside the actions section.
5. Using Material Inputs and Forms
Material inputs provide a consistent, styled way of capturing user input. You can use the mat-form-field
and mat-input
components to create Material-style input fields:
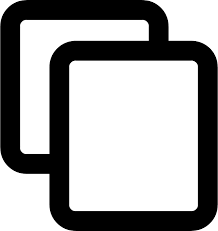
Enter your name
<input matInput placeholder="Name">
In this example, the mat-form-field
is used to wrap the input field, and the matInput
directive is applied to the input
element to style it according to Material Design.
6. Using Material Selects
Material Select components allow you to create dropdown menus for selecting options. Here’s an example of how to use the mat-select
component:
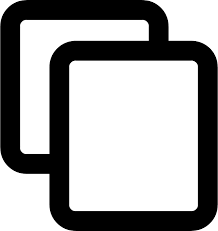
Select an option
Option 1
Option 2
Option 3
This example demonstrates how to create a dropdown using the mat-select
component and mat-option
for each item.
7. Using Material Dialogs
Material Dialogs are used to display modal windows. To use dialogs, you need to import the MatDialogModule
and inject the dialog service into your component:
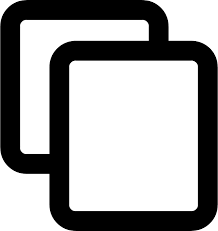
import { Component } from '@angular/core';
import { MatDialog } from '@angular/material/dialog';
import { DialogContentComponent } from './dialog-content/dialog-content.component';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(public dialog: MatDialog) {}
openDialog(): void {
this.dialog.open(DialogContentComponent);
}
}
In this example, we inject the MatDialog
service into the component and use the open()
method to open the dialog. You’ll also need to define a component for the dialog content.
8. Conclusion
Angular Material provides a rich set of UI components that follow Material Design principles. These components help you build modern, responsive web applications quickly. From buttons to forms and dialogs, Angular Material components are easy to integrate into your Angular applications. You can customize these components as per your requirements and ensure that your application adheres to Material Design guidelines.
Theming and Customization
1. Overview
Angular Material provides a robust theming system that allows you to define custom color palettes and apply consistent styles across your application. The theming system is based on the concepts of Material Design and supports both light and dark themes. You can also customize individual components to meet specific design requirements.
2. Setting Up a Theme
Angular Material comes with pre-built themes, such as indigo-pink
, deeppurple-amber
, and more. To use these, simply include the theme CSS in your project:
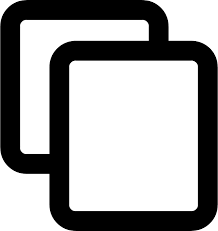
/* angular.json */
"styles": [
"src/styles.css",
"./node_modules/@angular/material/prebuilt-themes/indigo-pink.css"
]
This example uses the indigo-pink
theme. Replace it with another pre-built theme if needed.
3. Customizing Themes
For more control, you can create your own custom theme by defining a SCSS file. Here's an example:
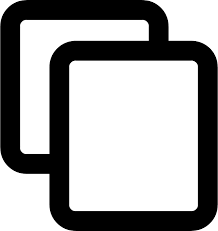
/* custom-theme.scss */
@use '@angular/material' as mat;
@include mat.core();
// Define custom color palettes
$primary-palette: mat.define-palette(mat.$indigo-palette);
$accent-palette: mat.define-palette(mat.$pink-palette, A200, A100, A400);
$warn-palette: mat.define-palette(mat.$red-palette);
// Define the theme
$custom-theme: mat.define-light-theme((
color: (
primary: $primary-palette,
accent: $accent-palette,
warn: $warn-palette,
)
));
// Include Angular Material styles
@include mat.all-component-themes($custom-theme);
Save this file as custom-theme.scss
, then include it in your project:
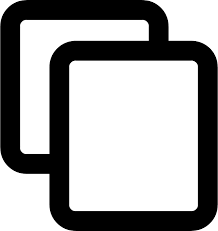
/* angular.json */
"styles": [
"src/styles.scss",
"src/custom-theme.scss"
]
4. Switching Between Light and Dark Themes
Angular Material also supports toggling between light and dark themes. You can define both themes in your SCSS file:
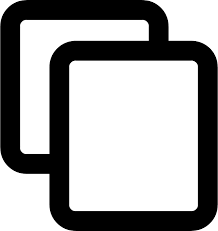
/* custom-theme.scss */
$dark-theme: mat.define-dark-theme((
color: (
primary: $primary-palette,
accent: $accent-palette,
warn: $warn-palette,
)
));
You can toggle themes dynamically in your application using CSS classes or Angular logic.
5. Customizing Individual Components
To customize individual Angular Material components, you can override their styles in your global styles or component-specific stylesheets:
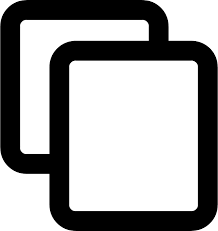
/* styles.css */
.mat-button {
background-color: #1976d2;
color: white;
}
.mat-card {
border-radius: 8px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
6. Applying Themes Dynamically
To apply themes dynamically, use Angular's binding capabilities to switch between themes based on user preferences:
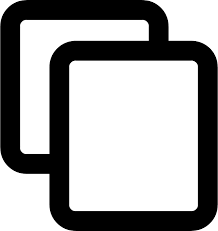
<button (click)="toggleTheme()">Toggle Theme</button>
<div [ngClass]="currentTheme>
<This content will reflect the current theme.</>
</div>
export class AppComponent {
currentTheme = 'light-theme';
toggleTheme() {
this.currentTheme = this.currentTheme === 'light-theme' ? 'dark-theme' : 'light-theme';
}
}
Define your light and dark theme styles in your global stylesheet and bind the currentTheme
class to the root element.
7. Conclusion
Angular Material's theming and customization system provides a flexible way to implement consistent and visually appealing designs. By leveraging pre-built themes or creating custom ones, you can ensure that your application aligns with branding requirements while maintaining a modern look and feel.
Testing Components
1. Overview
Testing is a crucial part of Angular development to ensure the reliability and correctness of components. Angular provides a robust testing framework integrated with Jasmine and Karma to create and execute tests efficiently. This section focuses on writing unit tests for components using Angular’s testing utilities.
2. Setting Up the Testing Environment
Angular CLI automatically sets up the testing environment when you create a project. The essential files for testing include:
karma.conf.js
: Configuration file for the Karma test runner.src/test.ts
: Entry point for the test environment.- Spec files (
.spec.ts
): Contain test cases for components, services, and more.
3. Writing a Basic Test Case for a Component
A component spec file typically includes the setup logic and test cases. Here's an example:
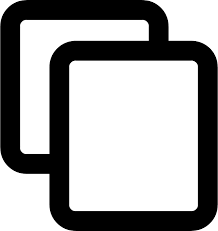
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { MyComponent } from './my-component.component';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [MyComponent],
}).compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create the component', () => {
expect(component).toBeTruthy();
});
it('should display the correct title', () => {
component.title = 'Test Title';
fixture.detectChanges();
const compiled = fixture.nativeElement;
expect(compiled.querySelector('h1').textContent).toContain('Test Title');
});
});
4. Simulating User Interactions
To test user interactions like button clicks or input events, use Angular's DebugElement
or native DOM methods:
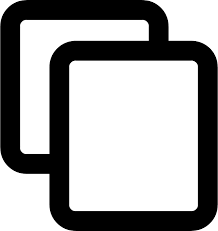
it('should increment the counter on button click', () => {
const button = fixture.nativeElement.querySelector('button');
button.click();
fixture.detectChanges();
expect(component.counter).toBe(1);
});
5. Testing Component Dependencies
If the component relies on services or other dependencies, you can mock them in your tests:
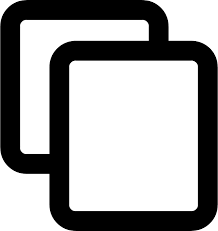
import { MyService } from './my-service.service';
class MockMyService {
getData() {
return of(['Mock Data']);
}
}
it('should display mock data', () => {
TestBed.overrideProvider(MyService, { useValue: new MockMyService() });
fixture.detectChanges();
const compiled = fixture.nativeElement;
expect(compiled.querySelector('ul').textContent).toContain('Mock Data');
});
6. Testing Asynchronous Code
For components with asynchronous logic, use Angular's fakeAsync
or async
utilities:
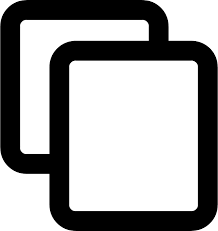
import { fakeAsync, tick } from '@angular/core/testing';
it('should update data after async operation', fakeAsync(() => {
component.loadData();
tick(1000); // Simulate passage of time
fixture.detectChanges();
expect(component.data).toEqual(['Async Data']);
}));
7. Testing with Angular Material Components
To test Angular Material components, import the required modules and interact with them like standard components:
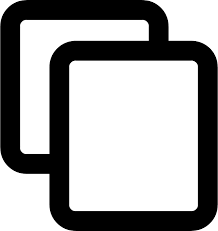
import { MatInputModule } from '@angular/material/input';
beforeEach(async () => {
await TestBed.configureTestingModule({
imports: [MatInputModule],
declarations: [MyComponent],
}).compileComponents();
});
it('should capture input value', () => {
const input = fixture.nativeElement.querySelector('input');
input.value = 'Test Input';
input.dispatchEvent(new Event('input'));
fixture.detectChanges();
expect(component.inputValue).toBe('Test Input');
});
8. Conclusion
Testing components in Angular ensures that your UI behaves as expected and integrates well with other parts of the application. By leveraging Angular's powerful testing utilities, you can create robust and maintainable test cases, improving the quality and reliability of your application.
Testing Services
1. Overview
Services in Angular are used to encapsulate business logic and share data across components. Testing services ensures that this logic is functioning as expected, especially for asynchronous operations and data interactions. Angular provides a robust testing framework to facilitate unit testing of services.
2. Setting Up the Test Environment
When testing services, you typically use the TestBed
to configure and provide the service. This allows you to isolate the service and test it in a controlled environment.
3. Writing a Basic Test Case for a Service
The following example demonstrates how to write a simple test for an Angular service:
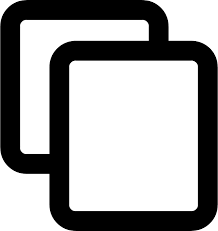
import { TestBed } from '@angular/core/testing';
import { DataService } from './data.service';
describe('DataService', () => {
let service: DataService;
beforeEach(() => {
TestBed.configureTestingModule({});
service = TestBed.inject(DataService);
});
it('should be created', () => {
expect(service).toBeTruthy();
});
it('should return a list of items', () => {
const data = service.getData();
expect(data).toEqual(['Item 1', 'Item 2', 'Item 3']);
});
});
4. Testing Asynchronous Methods
For services with asynchronous methods, such as HTTP calls, you can use Angular's fakeAsync
or async
utilities:
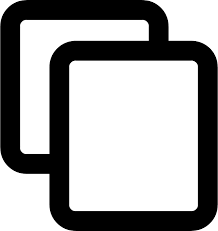
import { fakeAsync, tick } from '@angular/core/testing';
it('should fetch data asynchronously', fakeAsync(() => {
let result: any;
service.getAsyncData().subscribe(data => (result = data));
tick(1000); // Simulate passage of time
expect(result).toEqual(['Async Item 1', 'Async Item 2']);
}));
5. Mocking HTTP Requests
To test services that make HTTP requests, use Angular's HttpTestingController
from the @angular/common/http/testing
package:
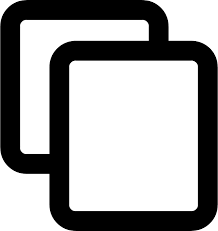
import { HttpClientTestingModule, HttpTestingController } from '@angular/common/http/testing';
describe('DataService with HTTP', () => {
let service: DataService;
let httpMock: HttpTestingController;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [DataService],
});
service = TestBed.inject(DataService);
httpMock = TestBed.inject(HttpTestingController);
});
it('should fetch data from API', () => {
const mockData = ['API Item 1', 'API Item 2'];
service.fetchData().subscribe(data => {
expect(data).toEqual(mockData);
});
const req = httpMock.expectOne('https://api.example.com/data');
expect(req.request.method).toBe('GET');
req.flush(mockData);
});
afterEach(() => {
httpMock.verify();
});
});
6. Mocking Dependencies
If a service depends on other services, you can mock those dependencies for testing:
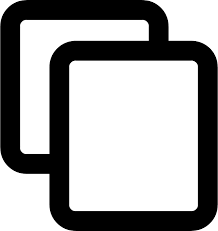
class MockDependencyService {
getDependencyData() {
return ['Mock Dependency Data'];
}
}
it('should use mock dependency data', () => {
TestBed.overrideProvider(DependencyService, { useValue: new MockDependencyService() });
service = TestBed.inject(DataService);
const result = service.getDataFromDependency();
expect(result).toEqual(['Mock Dependency Data']);
});
7. Testing Error Handling
To test how the service handles errors, mock error responses or simulate failure scenarios:
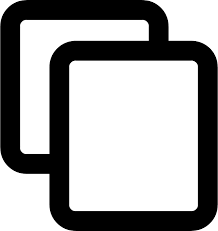
it('should handle HTTP errors gracefully', () => {
service.fetchData().subscribe(
() => fail('Should have failed with an error'),
(error) => {
expect(error.status).toBe(500);
}
);
const req = httpMock.expectOne('https://api.example.com/data');
req.flush('Error message', { status: 500, statusText: 'Internal Server Error' });
});
8. Conclusion
Testing services in Angular ensures that your business logic and data interactions work as expected. By using Angular's testing tools, you can handle various scenarios such as asynchronous operations, HTTP requests, dependencies, and error handling, making your services reliable and maintainable.
Testing Directives
1. Overview
Directives in Angular are used to extend the functionality of HTML elements and encapsulate custom behavior. Testing directives ensures that their behavior works as expected across different scenarios. Angular provides tools like TestBed
and ComponentFixture
to simplify directive testing.
2. Setting Up the Test Environment
To test a directive, you need to configure it in a testing module using TestBed
. A host component is often used to apply the directive and verify its behavior.
3. Writing a Basic Test for a Directive
The following example demonstrates how to test a simple directive that changes the background color of an element:
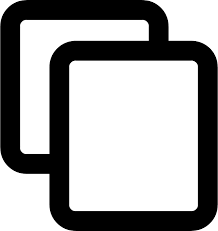
import { Directive, ElementRef, HostListener } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef) {}
@HostListener('mouseenter') onMouseEnter() {
this.el.nativeElement.style.backgroundColor = 'yellow';
}
@HostListener('mouseleave') onMouseLeave() {
this.el.nativeElement.style.backgroundColor = '';
}
}
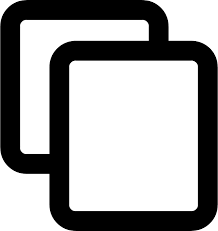
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { Component } from '@angular/core';
import { HighlightDirective } from './highlight.directive';
@Component({
template: 'Test Highlight Directive
'
})
class TestHostComponent {}
describe('HighlightDirective', () => {
let fixture: ComponentFixture;
let paragraphEl: HTMLElement;
beforeEach(() => {
TestBed.configureTestingModule({
declarations: [HighlightDirective, TestHostComponent],
});
fixture = TestBed.createComponent(TestHostComponent);
paragraphEl = fixture.nativeElement.querySelector('p');
});
it('should change background color on mouse enter', () => {
const event = new Event('mouseenter');
paragraphEl.dispatchEvent(event);
fixture.detectChanges();
expect(paragraphEl.style.backgroundColor).toBe('yellow');
});
it('should remove background color on mouse leave', () => {
const event = new Event('mouseleave');
paragraphEl.dispatchEvent(event);
fixture.detectChanges();
expect(paragraphEl.style.backgroundColor).toBe('');
});
});
4. Testing Structural Directives
Structural directives, such as those that add or remove elements from the DOM, can also be tested. The following example demonstrates a custom structural directive:
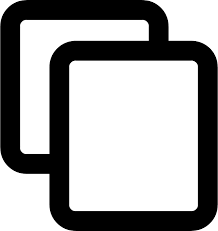
import { Directive, Input, TemplateRef, ViewContainerRef } from '@angular/core';
@Directive({
selector: '[appUnless]'
})
export class UnlessDirective {
@Input() set appUnless(condition: boolean) {
if (!condition) {
this.vcRef.createEmbeddedView(this.templateRef);
} else {
this.vcRef.clear();
}
}
constructor(
private templateRef: TemplateRef,
private vcRef: ViewContainerRef
) {}
}
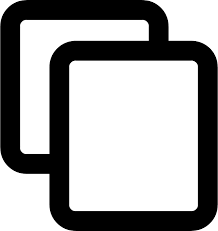
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { Component } from '@angular/core';
import { UnlessDirective } from './unless.directive';
@Component({
template: 'Test Unless Directive
'
})
class TestHostComponent {
condition = false;
}
describe('UnlessDirective', () => {
let fixture: ComponentFixture;
let testComponent: TestHostComponent;
beforeEach(() => {
TestBed.configureTestingModule({
declarations: [UnlessDirective, TestHostComponent],
});
fixture = TestBed.createComponent(TestHostComponent);
testComponent = fixture.componentInstance;
fixture.detectChanges();
});
it('should display the paragraph when condition is false', () => {
const paragraph = fixture.nativeElement.querySelector('p');
expect(paragraph).toBeTruthy();
});
it('should not display the paragraph when condition is true', () => {
testComponent.condition = true;
fixture.detectChanges();
const paragraph = fixture.nativeElement.querySelector('p');
expect(paragraph).toBeFalsy();
});
});
5. Mocking Dependencies in Directives
If your directive depends on services, you can mock those services in your tests:
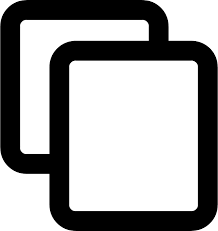
class MockService {
performAction() {
return 'Mocked Action';
}
}
it('should use the mock service', () => {
TestBed.overrideProvider(SomeService, { useValue: new MockService() });
const service = TestBed.inject(SomeService);
expect(service.performAction()).toBe('Mocked Action');
});
6. Conclusion
Testing directives ensures their custom behavior functions as expected. Angular's testing utilities, such as TestBed
, ComponentFixture
, and mock services, make it easier to test attribute and structural directives under various scenarios, ensuring robust and reusable directives.
Using Jasmine and Karma
1. Overview
Jasmine and Karma are popular tools used for testing Angular applications. Jasmine is a JavaScript testing framework that provides functions for writing and structuring tests, while Karma is a test runner that executes the tests in various browsers. Together, they form a robust testing setup for Angular projects.
2. Setting Up Jasmine and Karma
Angular CLI comes with Jasmine and Karma preconfigured. When you create a new Angular project using ng new
, the necessary files and configurations are automatically set up. The key configuration files are:
- karma.conf.js: Configuration file for the Karma test runner.
- tsconfig.spec.json: TypeScript configuration for the test environment.
3. Writing Tests with Jasmine
Jasmine provides a suite of functions for writing tests, including describe
, it
, beforeEach
, and expect
. Here’s a basic example:
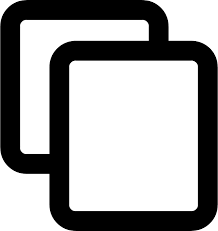
describe('Basic Jasmine Test', () => {
let value: number;
beforeEach(() => {
value = 10;
});
it('should add two numbers', () => {
const sum = value + 5;
expect(sum).toBe(15);
});
it('should subtract two numbers', () => {
const difference = value - 3;
expect(difference).toBe(7);
});
});
4. Running Tests with Karma
To execute tests, use the Angular CLI command:
ng test
This command launches the Karma test runner, which opens a browser and executes the tests. The results are displayed in the terminal and the browser.
5. Key Features of Jasmine
- Matchers: Jasmine provides a variety of matchers like
toBe
,toEqual
,toContain
, and more. - Spies: Jasmine allows you to mock functions using spies. For example:
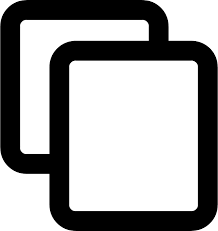
it('should call the function', () => {
const spy = jasmine.createSpy('spyFunction');
spy();
expect(spy).toHaveBeenCalled();
});
6. Configuring Karma
The karma.conf.js
file allows you to customize the test runner. Key configurations include:
- Browsers: Specifies which browsers to use (e.g., Chrome, Firefox).
- Reporters: Configures how test results are displayed.
- Preprocessors: Configures file transformations, such as TypeScript compilation.
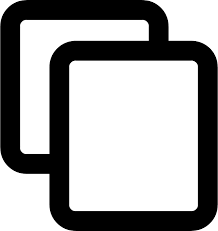
// karma.conf.js
module.exports = function (config) {
config.set({
frameworks: ['jasmine', '@angular-devkit/build-angular'],
browsers: ['Chrome'],
reporters: ['progress', 'kjhtml'],
autoWatch: true,
singleRun: false,
restartOnFileChange: true,
});
};
7. Debugging Tests
Use the following tips for debugging failing tests:
- Console Logs: Add
console.log
statements to inspect values during tests. - Disable Headless Mode: Run Karma in a visible browser by disabling headless mode in the
karma.conf.js
file. - Focus Tests: Use
fdescribe
orfit
to run a specific test suite or test case.
8. Advantages of Using Jasmine and Karma
- End-to-End Integration: Preconfigured with Angular CLI for seamless usage.
- Real Browser Testing: Tests run in real browsers, ensuring compatibility and reliability.
- Extensibility: Both tools are highly configurable to suit project-specific needs.
9. Conclusion
Jasmine and Karma together provide a comprehensive setup for testing Angular applications. Jasmine’s rich API for assertions and spies, combined with Karma’s real-browser execution, ensures robust and reliable testing for your application.
Introduction to Animations
1. Overview
Animations in Angular provide a powerful way to create visually appealing and dynamic applications. Angular's animation system is built on top of the Web Animations API and enables you to define complex transitions and effects with minimal code.
2. Setting Up Animations
Angular animations are part of the @angular/animations
package. To use animations, import the BrowserAnimationsModule
in your application module:
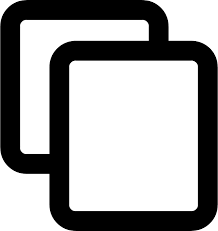
// app.module.ts
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserAnimationsModule],
bootstrap: [AppComponent]
})
export class AppModule { }
3. Basics of Angular Animations
Angular animations are defined in the @Component
decorator using the animations
property. Animations are defined using functions such as trigger
, state
, style
, transition
, and animate
.
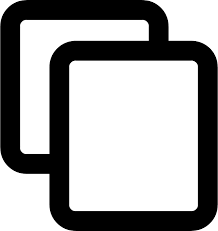
// app.component.ts
import { Component } from '@angular/core';
import { trigger, state, style, animate, transition } from '@angular/animations';
@Component({
selector: 'app-root',
template: `
<div [@fadeInOut]="isVisible ? 'visible' : 'hidden'" class="animated-box">
Animated Box
</div>
<button (click)="toggle()">Toggle Animation</button>
`,
styles: [`
.animated-box {
width: 200px;
height: 100px;
background-color: lightblue;
margin: 20px;
text-align: center;
line-height: 100px;
}
`],
animations: [
trigger('fadeInOut', [
state('visible', style({ opacity: 1 })),
state('hidden', style({ opacity: 0 })),
transition('visible <=> hidden', [animate('500ms ease-in-out')])
])
]
})
export class AppComponent {
isVisible = true;
toggle() {
this.isVisible = !this.isVisible;
}
}
4. Key Animation Functions
- trigger: Defines an animation trigger linked to an element.
- state: Describes different states for an element.
- style: Sets CSS styles for an element.
- transition: Defines transitions between states.
- animate: Specifies the duration and easing of the transition.
5. Using Animation Triggers
Animation triggers are linked to elements using the [@triggerName]
syntax. The trigger's state is dynamically controlled by component properties.
6. Advanced Animations
You can create more complex animations using sequences, groups, and keyframes:
- Sequences: Define animations that run one after the other.
- Groups: Run multiple animations simultaneously.
- Keyframes: Define animations with intermediate styles.
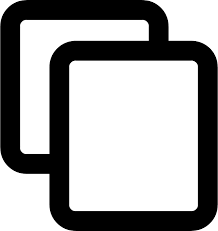
// Example of keyframes animation
import { trigger, style, animate, keyframes, transition } from '@angular/animations';
trigger('bounce', [
transition('* => *', [
animate('1s', keyframes([
style({ transform: 'translateY(0)', offset: 0 }),
style({ transform: 'translateY(-30px)', offset: 0.5 }),
style({ transform: 'translateY(0)', offset: 1.0 })
]))
])
]);
7. Benefits of Angular Animations
- Performance: Built on the Web Animations API for smooth performance.
- Integration: Seamlessly integrates with Angular's component-based architecture.
- Flexibility: Supports a wide range of animation styles and effects.
8. Conclusion
Angular animations enable you to enhance the user experience by adding smooth and engaging transitions to your application. By leveraging Angular's animation APIs, you can easily create and manage animations that are both performant and visually appealing.
Creating Animations
1. Overview
Animations bring life to Angular applications by adding smooth transitions and effects. With Angular's animation API, you can create powerful and engaging animations using triggers, states, styles, transitions, and other built-in methods.
2. Steps to Create Animations
To create animations in Angular, follow these steps:
- Import the
BrowserAnimationsModule
into your Angular module. - Define an animation using
trigger
,state
,style
, andtransition
. - Link the animation to an element in the template using the
[@triggerName]
syntax. - Control the animation state dynamically using component properties.
3. Example: Creating a Slide-In Animation
In this example, we'll create a slide-in animation for a panel:
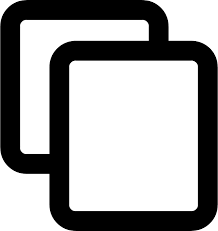
// app.component.ts
import { Component } from '@angular/core';
import { trigger, state, style, animate, transition } from '@angular/animations';
@Component({
selector: 'app-root',
template: `
<div class="panel" [@slideInOut]="isOpen ? 'open' : 'closed'">
Animated Panel
</div>
<button (click)="toggle()">Toggle Panel</button>
`,
styles: [`
.panel {
width: 300px;
height: 100px;
background-color: lightcoral;
overflow: hidden;
margin: 20px;
}
`],
animations: [
trigger('slideInOut', [
state('open', style({ transform: 'translateX(0)' })),
state('closed', style({ transform: 'translateX(-100%)' })),
transition('open <=> closed', [
animate('400ms ease-in-out')
])
])
]
})
export class AppComponent {
isOpen = false;
toggle() {
this.isOpen = !this.isOpen;
}
}
4. Key Animation Concepts
- Trigger: Defines the animation and is linked to an element in the template.
- State: Represents a specific style configuration for an element.
- Style: Applies CSS styles to elements at a specific point in the animation.
- Transition: Specifies how and when a state change occurs.
- Animate: Defines the duration and timing of the animation.
5. Advanced Animation Features
Angular animations allow you to create complex animations using advanced features like:
- Keyframes: Define multiple intermediate styles within an animation.
- Groups: Run multiple animations simultaneously.
- Sequences: Chain animations to run one after another.
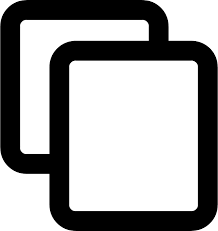
// Example of keyframes in an animation
import { trigger, style, animate, keyframes, transition } from '@angular/animations';
trigger('bounce', [
transition('* => *', [
animate('1s', keyframes([
style({ transform: 'translateY(0)', offset: 0 }),
style({ transform: 'translateY(-30px)', offset: 0.5 }),
style({ transform: 'translateY(0)', offset: 1.0 })
]))
])
]);
6. Tips for Creating Effective Animations
- Keep animations simple and intuitive for a better user experience.
- Use easing functions like
ease-in-out
for smooth transitions. - Test animations across devices to ensure performance consistency.
- Use
query
andstagger
for animating multiple elements.
7. Conclusion
Creating animations in Angular can significantly enhance the user experience by adding visual appeal and interactivity. With Angular's flexible animation API, you can craft animations that are both efficient and engaging, making your application stand out.
Triggering Animations with Events
1. Overview
In Angular, animations can be dynamically triggered based on user interactions or other events. By combining Angular's animation API with event bindings, you can control animations effectively to respond to clicks, hovers, or any other custom events.
2. How to Trigger Animations with Events
- Define an animation using the
trigger
method. - Attach the animation to an element using the
[@triggerName]
syntax. - Update the animation state dynamically through event handlers in your component.
3. Example: Animating on Click
In this example, we will animate a box to expand and collapse when clicked:
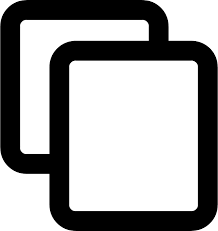
// app.component.ts
import { Component } from '@angular/core';
import { trigger, state, style, transition, animate } from '@angular/animations';
@Component({
selector: 'app-root',
template: `
<div class="box" [@expandCollapse]="isExpanded ? 'expanded' : 'collapsed'" (click)="toggle()">
Click to Toggle
</div>
`,
styles: [`
.box {
width: 200px;
height: 100px;
background-color: lightblue;
margin: 20px;
text-align: center;
line-height: 100px;
cursor: pointer;
}
`],
animations: [
trigger('expandCollapse', [
state('expanded', style({ height: '200px', backgroundColor: 'lightgreen' })),
state('collapsed', style({ height: '100px', backgroundColor: 'lightblue' })),
transition('expanded <=> collapsed', animate('300ms ease-in-out'))
])
]
})
export class AppComponent {
isExpanded = false;
toggle() {
this.isExpanded = !this.isExpanded;
}
}
4. Triggering Animations with Hover
You can use mouse events to trigger animations. For example, changing the style of an element when hovered:
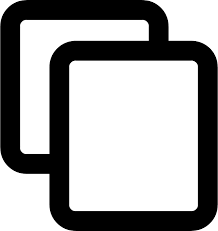
// app.component.ts
@Component({
selector: 'app-hover-box',
template: `
<div class="hover-box" [@hoverEffect]="isHovered ? 'hovered' : 'normal'"
(mouseenter)="onMouseEnter()"
(mouseleave)="onMouseLeave()">
Hover over me!
</div>
`,
styles: [`
.hover-box {
width: 200px;
height: 100px;
background-color: lightcoral;
text-align: center;
line-height: 100px;
transition: transform 0.3s;
}
`],
animations: [
trigger('hoverEffect', [
state('normal', style({ transform: 'scale(1)' })),
state('hovered', style({ transform: 'scale(1.1)' })),
transition('normal <=> hovered', animate('200ms ease-in'))
])
]
})
export class HoverBoxComponent {
isHovered = false;
onMouseEnter() {
this.isHovered = true;
}
onMouseLeave() {
this.isHovered = false;
}
}
5. Best Practices
- Use meaningful animation states (e.g.,
'open'
,'closed'
,'active'
,'inactive'
). - Optimize performance by limiting the number of animated elements on the screen.
- Test animations for responsiveness across different devices and screen sizes.
- Combine animations with ARIA attributes to ensure accessibility.
6. Advanced Techniques
Combine Angular animations with custom event emitters or RxJS to create highly dynamic animations based on complex user interactions or data changes.
7. Conclusion
Triggering animations with events allows you to create interactive and dynamic user experiences. By leveraging Angular's animation API and event bindings, you can efficiently control animations to respond to user actions, enhancing the overall engagement of your application.
Lazy Loading Modules
1. Overview
Lazy loading is an Angular feature that allows you to load modules on demand instead of loading them all at once. This optimizes the performance of your application by reducing the initial load time, especially for large applications with multiple modules.
2. Why Use Lazy Loading?
- Improves application performance by reducing the bundle size loaded at the start.
- Allows loading only the modules required for a specific feature or route.
- Enhances scalability by organizing the application into smaller, more manageable modules.
3. Setting Up Lazy Loading
To implement lazy loading, follow these steps:
- Create a feature module for the part of the application you want to load lazily.
- Use the
loadChildren
property in the route configuration to specify the module to load. - Ensure the feature module uses
RouterModule.forChild()
for its routing configuration.
4. Example: Lazy Loading a Module
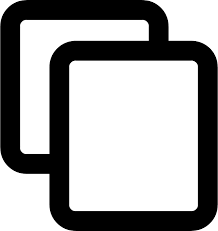
// app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomeModule) },
{ path: 'about', loadChildren: () => import('./about/about.module').then(m => m.AboutModule) }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
5. Creating a Feature Module
Create a feature module (e.g., HomeModule
) and configure its routing with RouterModule.forChild()
:
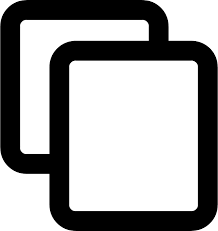
// home-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home.component';
const routes: Routes = [
{ path: '', component: HomeComponent }
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class HomeRoutingModule { }
Next, set up the feature module:
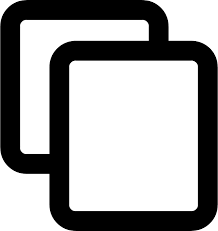
// home.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { HomeComponent } from './home.component';
import { HomeRoutingModule } from './home-routing.module';
@NgModule({
declarations: [HomeComponent],
imports: [
CommonModule,
HomeRoutingModule
]
})
export class HomeModule { }
6. Loading Modules on Demand
With the above setup, the HomeModule
will only be loaded when the user navigates to the /home
route.
7. Benefits of Lazy Loading
- Faster Initial Load: Reduces the initial bundle size by loading only essential modules.
- Better Performance: Improves runtime performance by loading feature modules only when needed.
- Modular Codebase: Organizes the application into smaller, self-contained modules.
8. Best Practices
- Use lazy loading for all feature modules to optimize performance.
- Ensure that the feature modules' routes do not conflict with other routes in the application.
- Keep feature modules focused on a single feature or functionality.
- Test lazy-loaded modules thoroughly to ensure that all dependencies are correctly imported.
9. Conclusion
Lazy loading is an essential optimization technique for Angular applications, especially as they grow in size and complexity. By implementing lazy loading, you can significantly enhance the performance and maintainability of your application.
AOT (Ahead of Time) Compilation
1. Overview
Ahead of Time (AOT) compilation is a process where Angular compiles your application during the build process, before the browser downloads and runs the application. This approach improves application performance and reduces runtime errors.
2. What is AOT Compilation?
AOT compilation transforms Angular HTML templates and TypeScript code into efficient JavaScript code during the build process. This compiled code is optimized for browsers, resulting in faster load times and improved execution speed.
3. Benefits of AOT Compilation
- Faster Rendering: Templates are precompiled into JavaScript, so the browser doesn't need to compile them at runtime.
- Smaller Bundles: Unnecessary Angular metadata is removed, reducing the bundle size.
- Early Error Detection: Syntax and template errors are caught during the build process.
- Improved Security: AOT compilation prevents injection attacks by pre-compiling templates and reducing reliance on runtime HTML evaluation.
4. How to Enable AOT Compilation
AOT compilation is enabled by default when you build your Angular application for production using the Angular CLI:
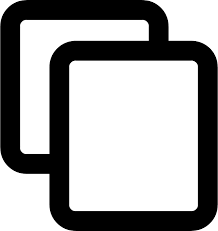
# Build the application with AOT enabled
ng build --prod
5. Checking AOT Compilation
To explicitly enable or disable AOT during development or testing, use the --aot
flag:
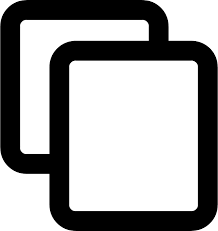
# Enable AOT during development
ng build --aot
# Disable AOT (use JIT - Just in Time compilation)
ng build --aot=false
6. How AOT Works
The AOT compilation process includes three main steps:
- Template Parsing: Angular parses the HTML templates and validates them.
- TypeScript Compilation: Angular compiles the TypeScript code and validates it.
- Code Generation: Angular generates highly optimized JavaScript code from the templates and TypeScript.
7. Example: AOT vs JIT
The difference between AOT and JIT (Just in Time) compilation lies in when the templates are compiled:
- AOT: Compilation happens during the build process.
- JIT: Compilation happens in the browser at runtime.
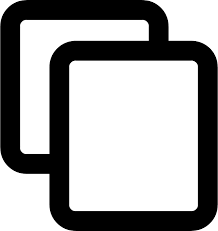
// AOT Example: Precompiled Template
@Component({
selector: 'app-root',
template: 'Hello, {{name}}
'
})
export class AppComponent {
name = 'Angular';
}
// JIT Example: Template Compiled in Browser
@Component({
selector: 'app-root',
template: `Hello, {{name}}
`
})
export class AppComponent {
name = 'Angular';
}
8. Best Practices
- Use AOT for all production builds to maximize performance and security.
- Ensure your templates are free of errors and follow Angular's syntax rules.
- Avoid dynamic HTML content that cannot be precompiled.
- Minimize the use of inline styles and templates in components for better optimization.
9. Debugging AOT Issues
Sometimes, AOT compilation may fail due to incorrect template syntax or unsupported features. Use these tips to debug:
- Check the error messages during the build process for details.
- Ensure all components and modules are properly declared and imported.
- Validate that all dependencies are installed and compatible with your Angular version.
10. Conclusion
AOT compilation is a crucial optimization for Angular applications, ensuring faster load times, better performance, and enhanced security. By leveraging AOT, developers can catch errors early and deliver more efficient applications to users.
Tree Shaking
1. Overview
Tree Shaking is a technique used in modern JavaScript bundlers (such as Webpack) to remove unused code from your final bundle. In Angular, tree shaking helps reduce the size of the application by eliminating dead code that is not being used in the application.
2. What is Tree Shaking?
Tree Shaking refers to the process of removing unused code during the build process. It is based on the concept of "static analysis," where the bundler analyzes the code and removes parts that are not required. This results in smaller, optimized bundles and faster load times for your Angular applications.
3. How Tree Shaking Works in Angular
Angular uses Webpack as its bundler, and Webpack automatically performs tree shaking when the application is built in production mode. It eliminates unused code in both the application and third-party libraries, ensuring only the necessary code is bundled and served to the client.
4. Enabling Tree Shaking in Angular
Tree Shaking is enabled by default when building an Angular application for production. You can enable it by running the following command:
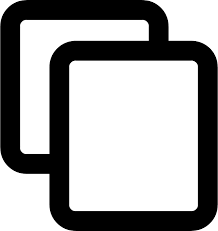
# Build the Angular application for production
ng build --prod
5. How Does Tree Shaking Remove Unused Code?
Tree shaking works by analyzing the imports and exports of JavaScript modules. If a module or function is imported but not used anywhere in the code, Webpack removes it from the final bundle. This process is especially beneficial when using large libraries, where only a small subset of the functionality is needed.
6. Example: Tree Shaking in Action
Consider the following example where we import a large utility library but only use a small part of it. Tree Shaking will ensure that only the used parts of the library are included in the final bundle.
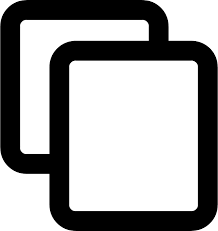
// Importing the entire lodash library (not optimal)
import * as _ from 'lodash';
console.log(_.isEmpty([])); // Only this function will be included in the final bundle
In the above example, the entire Lodash library is imported, but only the isEmpty
function is used. If tree shaking is applied, only the isEmpty
function will be included in the final bundle, and the rest of the unused Lodash code will be removed.
7. Best Practices for Tree Shaking
- Use ES Modules (ESM): Tree Shaking works best when the code is written using ES modules (import/export). Avoid using CommonJS modules as they are not tree-shakable.
- Avoid Dynamic Imports: If possible, avoid using dynamic imports, as they can sometimes prevent effective tree shaking.
- Use Libraries with Tree Shaking Support: Choose libraries that are designed to be tree-shakable, such as Lodash or RxJS. These libraries offer modular imports that allow unused parts to be removed.
- Split Code for Better Results: Implement lazy loading and code splitting to further optimize your application's size.
8. Common Issues with Tree Shaking
Tree Shaking might not work as expected in certain cases, especially when using non-ES module libraries or dynamic code. Common issues include:
- Non-ES Modules: Some third-party libraries do not support ES modules, making tree shaking less effective.
- Dynamic Imports: Using dynamic imports (e.g.,
import()
) can sometimes prevent Webpack from performing effective tree shaking. - Side Effects: Code that has side effects (such as modifying global variables or calling functions that affect the application state) may not be tree-shaken correctly.
9. Debugging Tree Shaking Issues
If tree shaking is not working as expected, here are a few steps to debug:
- Ensure that you're building in production mode using
ng build --prod
. - Check your imports to ensure you're using ES modules and not CommonJS.
- Use the Webpack Bundle Analyzer to inspect the final bundle and identify unused code.
10. Conclusion
Tree Shaking is a powerful technique for optimizing the size of your Angular application by removing unused code. By following best practices and using tree-shakable libraries, you can significantly reduce your bundle size and improve the performance of your app.
Best Practices for Performance
1. Overview
Performance optimization is key to delivering a fast, responsive Angular application. Following best practices ensures that your application runs efficiently, providing an improved user experience and reducing load times. This section covers essential performance tips and techniques to implement in your Angular apps.
2. Minimize Bundle Size
Large bundles can significantly impact the performance of your Angular application. Keeping the bundle size small helps in faster loading times and better performance. Some techniques include:
- Lazy Loading: Lazy load feature modules so they are only loaded when needed, reducing the initial bundle size.
- Tree Shaking: Remove unused code from the final bundle by enabling tree shaking in production builds.
- Code Splitting: Split large bundles into smaller, more manageable chunks, which can be loaded as needed.
3. Use Ahead-of-Time (AOT) Compilation
Ahead-of-Time (AOT) compilation pre-compiles your Angular templates and components during the build process rather than at runtime. This reduces the amount of work the browser has to do and speeds up the application startup time. To enable AOT, use the --prod
flag when building your application:
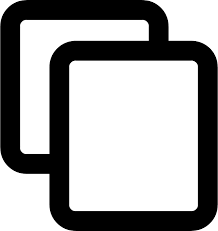
# Build with AOT enabled
ng build --prod
4. Optimize Change Detection
Angular’s change detection mechanism checks for updates in the application state and updates the DOM accordingly. Excessive checks can lead to performance issues. To optimize change detection:
- Use OnPush Change Detection: Set the change detection strategy to
OnPush
for components that don’t change often. This tells Angular to check for changes only when input properties change or events are triggered. - Track By in ngFor: When using
ngFor
, use thetrackBy
function to help Angular efficiently track and update DOM elements.
5. Reduce HTTP Requests
Reducing the number of HTTP requests your Angular application makes can significantly improve performance. Here are some strategies:
- Batch Requests: Combine multiple HTTP requests into a single request to reduce network overhead.
- HTTP Caching: Use HTTP caching strategies to avoid unnecessary requests to the server for data that doesn't change frequently.
- Use HTTP Interceptors: Use interceptors to manage requests, responses, and errors efficiently across the app.
6. Optimize Assets and Images
Large assets, especially images, can slow down your application. Optimize assets to improve performance:
- Image Optimization: Compress images and serve them in modern formats like
WebP
for smaller file sizes. - Lazy Load Images: Implement lazy loading for images so they are only loaded when they come into view.
- Use SVGs for Icons: SVGs are lightweight and resolution-independent, making them ideal for icons and small images.
7. Use Web Workers for Heavy Computation
Web Workers allow you to run scripts in the background, offloading heavy computation tasks from the main thread and improving application responsiveness. In Angular, you can use Web Workers for tasks like data processing or complex calculations:
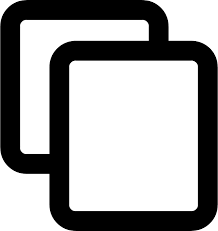
// In your app.component.ts
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class WorkerService {
runWorker(data: any): Observable {
const worker = new Worker('./worker.js');
worker.postMessage(data);
return new Observable(observer => {
worker.onmessage = (event) => {
observer.next(event.data);
};
worker.onerror = (error) => {
observer.error(error);
};
});
}
}
8. Use Server-Side Rendering (SSR)
Server-Side Rendering (SSR) allows you to render Angular applications on the server before sending them to the browser. This can improve the first paint time and SEO of your application. Angular Universal is a tool that enables SSR for Angular apps.
To add Angular Universal to your project, you can use the following command:
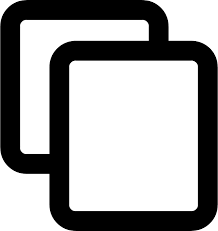
# Add Angular Universal to the app
ng add @nguniversal/express-engine
9. Optimize Mobile Performance
Mobile performance is crucial for delivering a smooth user experience on mobile devices. Some techniques for optimizing mobile performance include:
- Responsive Design: Ensure the layout adapts to different screen sizes, using media queries and Angular’s built-in support for responsive design.
- Touch Gestures: Optimize touch gestures and interactions, as they can impact performance on mobile devices.
- Minimize JavaScript: Reduce JavaScript execution time and avoid rendering bottlenecks on mobile devices.
10. Use Browser Performance Tools
To measure and identify performance bottlenecks, use browser performance tools like:
- Google Chrome DevTools: Use the Performance tab to analyze rendering performance and identify slow parts of your app.
- Web Vitals: Measure real-world user experience metrics like First Contentful Paint (FCP) and Largest Contentful Paint (LCP).
11. Conclusion
By following these best practices, you can significantly improve the performance of your Angular application. Optimizing bundle size, reducing HTTP requests, improving change detection, and using tools like Web Workers and SSR will lead to a faster, more responsive app that provides a great user experience.
Building Angular Applications for Production
1. Overview
Building Angular applications for production involves several steps that ensure your app is optimized for performance, security, and scalability. This section covers the key considerations and steps for building an Angular application that is ready for deployment in a production environment.
2. Use the Production Build
When building your Angular application for production, always use the --prod
flag. This enables several optimizations, such as Ahead-of-Time (AOT) compilation, minification, and tree shaking, which significantly improve your app’s performance.
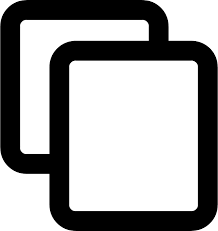
# Create a production build
ng build --prod
3. Enable Ahead-of-Time (AOT) Compilation
Ahead-of-Time (AOT) compilation is an essential optimization for production builds. It pre-compiles the Angular components and templates, reducing the runtime compilation overhead. This speeds up application startup and reduces the bundle size. The --prod
flag automatically enables AOT compilation.
AOT compilation helps:
- Reduce the size of the JavaScript bundles.
- Improve runtime performance by compiling templates and components ahead of time.
- Enhance security by compiling templates and reducing the potential for code injection attacks.
4. Tree Shaking and Minification
Tree shaking and minification are techniques used to reduce the final size of your JavaScript bundles:
- Tree Shaking: This removes unused code from your application to reduce the bundle size. Angular uses tree shaking during production builds to remove unused code from libraries and components.
- Minification: This reduces the size of the JavaScript code by removing whitespace, renaming variables, and shortening function names, making the code more efficient for browsers to load.
5. Use Lazy Loading for Modules
Lazy loading allows you to load Angular modules only when they are needed, reducing the initial load time of your application. This is especially useful for large applications where only a subset of the app is required initially. Lazy loading can be configured in your routes by using the loadChildren
property:
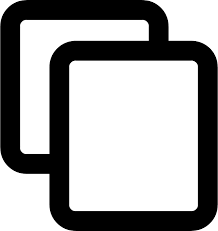
// app-routing.module.ts
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
6. Optimize Assets
Optimizing assets like images, fonts, and styles is essential for building an efficient production application. Here are some strategies:
- Image Optimization: Compress images using modern formats like
WebP
andAVIF
for better performance. - Font Optimization: Use only the required font weights and styles to reduce the load size.
- Use CSS Minification: Minify CSS to reduce the size and improve the loading time of stylesheets.
7. Environment Configuration
Angular allows you to define different configurations for various environments like development, production, and staging. The configuration files, such as environment.ts
and environment.prod.ts
, help manage environment-specific settings like API URLs, logging, and other environment-dependent values.
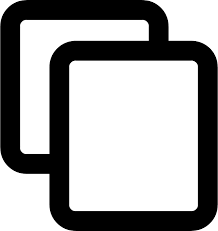
// environment.prod.ts
export const environment = {
production: true,
apiUrl: 'https://api.example.com'
};
8. Configure Service Workers for Offline Support
Service workers enable offline capabilities and caching for your Angular app. By using Angular’s built-in service worker support, your app can continue to function even when there is no network connection. To enable service workers, follow these steps:
- Run the following command to add service worker support:
- Enable the service worker in
ngsw-config.json
to define which resources should be cached.
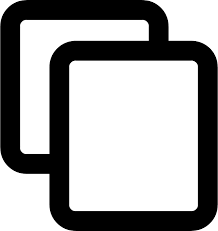
# Add Angular PWA (Progressive Web App) support
ng add @angular/pwa
9. Use Production-Ready Server and Hosting
When deploying your Angular application, ensure that you use a production-ready server and hosting solution. Some recommendations:
- Use HTTPS: Serve your application over HTTPS to ensure security and protect user data.
- Use a CDN (Content Delivery Network): Host static assets like images, stylesheets, and JavaScript files on a CDN to reduce latency and improve load times globally.
- Enable HTTP Caching: Configure caching headers for your assets to reduce unnecessary downloads on repeated visits.
10. Monitor and Optimize Performance in Production
After deploying your Angular application, it’s essential to monitor its performance and usage. You can use tools like:
- Google Lighthouse: Audits your application for performance, accessibility, SEO, and best practices.
- Web Vitals: Collect metrics like First Contentful Paint (FCP) and Largest Contentful Paint (LCP) to monitor real-world user experience.
- Angular Performance Monitoring: Use Angular’s built-in tools like
ng.profiler
to track performance bottlenecks.
11. Conclusion
Building Angular applications for production involves optimizing for performance, security, and scalability. By using the production build, enabling AOT, tree shaking, lazy loading, and optimizing assets, you can ensure your Angular app performs efficiently in a production environment. Additionally, configuring service workers, using a production-ready server, and monitoring application performance are essential steps to deliver an optimal user experience.
Deploying Angular Apps
1. Overview
Deploying an Angular application involves preparing the app for production and choosing a suitable hosting platform. This section covers the steps for deploying Angular applications on various platforms like Firebase, AWS, and other popular hosting services.
2. Preparing the Angular App for Deployment
Before deploying your Angular app, you need to create a production-ready build. Use Angular CLI to build the app with optimizations like Ahead-of-Time (AOT) compilation, minification, and tree shaking.
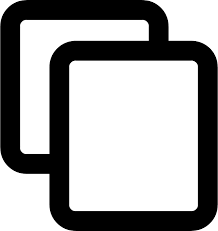
# Build the Angular app for production
ng build --prod
This will create a dist/
folder containing all the files needed for deployment. You can then upload these files to your hosting platform.
3. Deploying on Firebase Hosting
Firebase Hosting provides fast and secure hosting for web apps. To deploy your Angular app on Firebase, follow these steps:
- Install Firebase CLI: Install the Firebase CLI globally on your system.
- Login to Firebase: Authenticate with your Firebase account using the CLI.
- Initialize Firebase Project: Initialize your Firebase project in the root of your Angular project.
- Deploy the App: After the setup, deploy the app using the Firebase CLI.
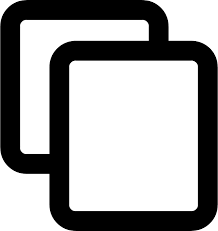
# Install Firebase CLI
npm install -g firebase-tools
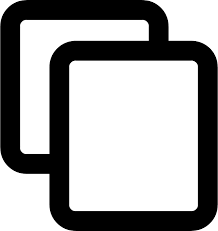
# Login to Firebase
firebase login
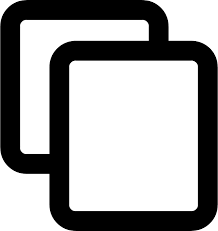
# Initialize Firebase project
firebase init
Select Firebase Hosting from the list of features and follow the prompts to set up the project.
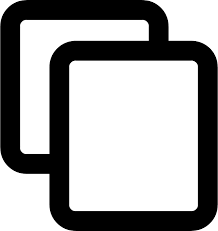
# Deploy to Firebase Hosting
firebase deploy
Your Angular app will now be live on Firebase Hosting, with a URL generated by Firebase.
4. Deploying on AWS (Amazon Web Services)
AWS provides several services for hosting Angular apps, such as S3 for static file hosting and CloudFront for Content Delivery Network (CDN) services. Here’s how to deploy your Angular app on AWS:
- Build the Angular App: First, create a production build using the
ng build --prod
command. - Create an S3 Bucket: Go to the AWS Management Console and create an S3 bucket. Make sure the bucket is publicly accessible and enable static website hosting in the settings.
- Upload the Build Files: Upload all the files from the
dist/
folder to your S3 bucket. - Set up CloudFront (Optional): You can set up AWS CloudFront as a CDN to improve the performance and reduce latency for users worldwide. CloudFront will cache your app’s assets closer to the user.
- Update Bucket Permissions: Set the permissions for the bucket so that the files are publicly accessible by configuring the bucket policy to allow public read access.
- Access Your App: Once the files are uploaded and the bucket is configured, you can access your Angular app via the S3 bucket’s static website URL or through CloudFront if configured.
5. Deploying on Other Platforms (Heroku, Netlify, Vercel, etc.)
There are several other platforms that you can use to deploy your Angular applications. Here’s a quick overview of how to deploy on some of the popular platforms:
- Heroku: Heroku is a platform-as-a-service (PaaS) that allows you to deploy web applications easily. You can deploy your Angular app by setting up a Node.js server to serve the app, and then pushing your app to Heroku using Git.
- Netlify: Netlify provides continuous deployment from Git repositories (GitHub, GitLab, Bitbucket). You can connect your repository to Netlify and it will automatically build and deploy your Angular app whenever you push changes to your repository.
- Vercel: Vercel is another excellent platform for deploying Angular apps. Like Netlify, it integrates with Git repositories and automatically deploys your app with every commit to the repository.
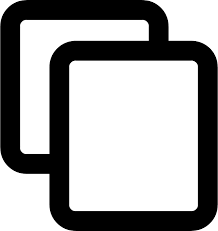
# Deploy Angular app on Netlify
npm run build --prod
# Drag the 'dist/' folder to Netlify dashboard or use their CLI to deploy
6. Setting up Continuous Deployment (CI/CD)
To automate the deployment process, you can set up Continuous Integration and Continuous Deployment (CI/CD) pipelines using platforms like GitHub Actions, GitLab CI, or Bitbucket Pipelines. These platforms can automatically trigger builds and deployments whenever changes are pushed to the repository.
7. Security and Performance Considerations
When deploying your Angular application to a production environment, ensure that you follow best practices for security and performance:
- Enable HTTPS: Always serve your app over HTTPS to ensure data security and privacy for users.
- Set up Caching: Configure HTTP caching to cache static assets such as images, JavaScript, and CSS files to improve performance.
- Use Content Security Policy (CSP): Implement a strong CSP to mitigate XSS (Cross-Site Scripting) attacks.
- Enable GZIP Compression: Enable GZIP compression on your server to reduce the size of the assets transferred over the network.
8. Conclusion
Deploying an Angular app is a straightforward process, but choosing the right hosting platform and configuration can have a big impact on the performance, security, and scalability of your app. Whether you choose Firebase, AWS, or another platform, follow the steps outlined in this guide to ensure a smooth deployment process.
Dynamic Components
1. Overview
Dynamic components in Angular allow you to load components dynamically at runtime, based on the application state or user interactions. This is useful for creating flexible and highly modular applications where certain components are not known upfront but need to be loaded dynamically.
2. Why Use Dynamic Components?
Dynamic components are useful when you need to:
- Load components based on user interaction (e.g., opening a modal or displaying a form dynamically).
- Reduce the initial load time by only loading components when needed.
- Enable highly reusable code that can be dynamically injected based on the context.
3. Steps to Create Dynamic Components
To create and load dynamic components in Angular, you need to follow these steps:
- Step 1: Create the Component to be Loaded Dynamically
Create the component that you want to load dynamically. For example, a modal or a tooltip component.
import { Component } from '@angular/core'; @Component({ selector: 'app-dynamic-modal', template: `<div class="modal-content">This is a dynamically loaded modal!</div>`, }) export class DynamicModalComponent {}
- Step 2: Create a Host Component
This component will act as the container for the dynamic component. It will contain the
ng-template
where the dynamic component will be inserted.import { Component, ViewContainerRef, ComponentFactoryResolver, OnInit } from '@angular/core'; import { DynamicModalComponent } from './dynamic-modal.component'; @Component({ selector: 'app-host', template: `<button (click)="loadDynamicComponent()">Load Dynamic Component</button> <ng-template #dynamicContainer></ng-template>`, }) export class HostComponent implements OnInit { constructor(private viewContainerRef: ViewContainerRef, private componentFactoryResolver: ComponentFactoryResolver) {} ngOnInit() {} loadDynamicComponent() { const componentFactory = this.componentFactoryResolver.resolveComponentFactory(DynamicModalComponent); this.viewContainerRef.clear(); this.viewContainerRef.createComponent(componentFactory); } }
In the
HostComponent
, the dynamic component is loaded into the<ng-template>
element using theViewContainerRef
andComponentFactoryResolver
. - Step 3: Add the Dynamic Component to the Module
Don’t forget to declare the dynamic component in the module's
declarations
array and include it in theentryComponents
array, so Angular knows to compile it dynamically.import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { HostComponent } from './host.component'; import { DynamicModalComponent } from './dynamic-modal.component'; @NgModule({ declarations: [HostComponent, DynamicModalComponent], imports: [BrowserModule], entryComponents: [DynamicModalComponent], bootstrap: [HostComponent] }) export class AppModule {}
- Step 4: Trigger the Dynamic Component Load
In the
HostComponent
, clicking on the button triggers the dynamic loading of theDynamicModalComponent
.
4. Dynamic Components with Inputs and Outputs
You can pass inputs and handle outputs from dynamically loaded components just as you would with statically loaded components. To pass inputs to a dynamic component, use the instance
property of the dynamically created component.
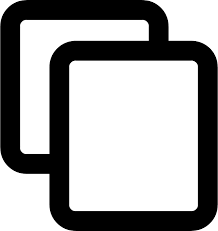
import { Component, ViewContainerRef, ComponentFactoryResolver, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-dynamic-modal',
template: `Dynamic Modal with Input: {{data}}`,
})
export class DynamicModalComponent {
@Input() data: string = '';
@Output() close = new EventEmitter();
closeModal() {
this.close.emit();
}
}
@Component({
selector: 'app-host',
template: `
`,
})
export class HostComponent {
constructor(private viewContainerRef: ViewContainerRef, private componentFactoryResolver: ComponentFactoryResolver) {}
loadDynamicComponent() {
const componentFactory = this.componentFactoryResolver.resolveComponentFactory(DynamicModalComponent);
const componentRef = this.viewContainerRef.createComponent(componentFactory);
componentRef.instance.data = 'Hello, Dynamic Component!';
componentRef.instance.close.subscribe(() => {
componentRef.destroy();
});
}
}
In this example, the DynamicModalComponent
receives an input (data
) and emits an output (close
) event. The HostComponent
passes the input and handles the output.
5. Conclusion
Dynamic components in Angular provide a powerful way to load components at runtime, making it possible to create more flexible and interactive applications. By using ViewContainerRef
and ComponentFactoryResolver
, you can load components dynamically, pass inputs, and handle outputs just like with static components.
Change Detection Strategy
1. Overview
Change Detection is a core concept in Angular that tracks changes in the application’s state and updates the view accordingly. Angular provides different change detection strategies to control how and when the view is updated. The default strategy, `Default`, checks all components in the component tree for changes. However, Angular allows developers to optimize performance by using the `OnPush` change detection strategy.
2. What is Change Detection?
Change detection in Angular is the process of ensuring that the view reflects the current state of the model. Angular uses a mechanism that compares the current values of the model with previous values and updates the view when it detects any changes.
3. Default Change Detection Strategy
The default change detection strategy is `Default`. With this strategy, Angular checks all components in the component tree for any change and updates the view if necessary. This can lead to performance issues in larger applications because Angular performs a check for all components on every event cycle (e.g., user input, HTTP request, etc.).
4. OnPush Change Detection Strategy
The `OnPush` strategy optimizes change detection by limiting the components that need to be checked for changes. With this strategy, Angular only checks the component when one of the following occurs:
- Input properties of the component change (i.e., new reference is passed to the component).
- An event is fired within the component (e.g., a button click, form input change).
- Manual triggering of change detection via `ChangeDetectorRef`.
5. Using the `OnPush` Strategy
To use the `OnPush` strategy, you need to set it in the `@Component` decorator for a component. This tells Angular to check the component only when one of the mentioned conditions occurs. Here's an example of how to enable `OnPush`:
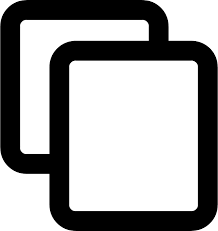
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
// Component logic
}
6. When to Use `OnPush`?
The `OnPush` strategy is ideal in scenarios where:
- The component’s state is mostly immutable or changes only when new data is passed via inputs.
- Performance optimization is needed in large applications with many components.
- You want to manually control when change detection is triggered (e.g., using `ChangeDetectorRef` or `ngOnChanges`).
7. How `OnPush` Affects Change Detection
With `OnPush`, Angular performs change detection only in the following cases:
- Input Property Change: When an input property value changes (via a new reference), Angular will check the component.
- Event Trigger: When an event inside the component triggers change detection (e.g., a button click).
- Manual Trigger: Change detection can also be manually triggered using `ChangeDetectorRef.detectChanges()` or `ApplicationRef.tick()`.
8. Example: Default vs OnPush
Consider the following example to understand the difference between `Default` and `OnPush` change detection:
Default Strategy
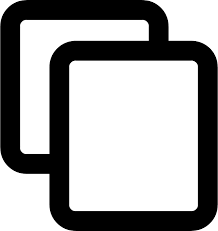
@Component({
selector: 'app-default-strategy',
template: `{{ data }}`,
})
export class DefaultStrategyComponent {
data = 'Initial Data';
updateData() {
this.data = 'Updated Data'; // Angular checks all components in the tree for changes
}
}
OnPush Strategy
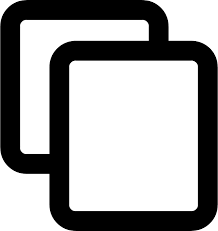
@Component({
selector: 'app-onpush-strategy',
template: `{{ data }}`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class OnPushStrategyComponent {
data = 'Initial Data';
updateData() {
this.data = 'Updated Data'; // Angular only checks the component if the input changes or event fires
}
}
9. Manual Change Detection
In Angular, you can manually trigger change detection using the `ChangeDetectorRef` service. This is especially useful when using the `OnPush` strategy or when you need to trigger a change detection cycle outside Angular's default event handling.
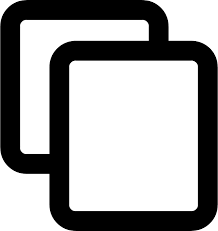
import { Component, ChangeDetectorRef } from '@angular/core';
@Component({
selector: 'app-manual-detection',
template: `<div>{{ data }}</div>`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ManualDetectionComponent {
data = 'Initial Data';
constructor(private cdRef: ChangeDetectorRef) {}
updateData() {
this.data = 'Updated Data';
this.cdRef.detectChanges(); // Manually triggers change detection
}
}
10. Benefits of Change Detection Strategies
- Performance Optimization: Reduces unnecessary checks on components, improving application performance.
- Control Over Change Detection: Allows developers to control when and where change detection occurs.
- Scalability: Helps Angular applications scale better with more components and complex states.
11. Conclusion
Change detection strategies are essential tools for optimizing Angular applications. By using the `OnPush` strategy, you can significantly improve performance, especially in large applications. Understanding when and how to use different strategies can help you build more efficient and scalable Angular applications.
Web Workers in Angular
1. Overview
Web Workers in Angular are used to run code in the background without blocking the main UI thread. They allow Angular applications to offload heavy computations, data processing, or other tasks that would otherwise slow down the UI. Web Workers enable a more responsive application by allowing the browser to continue executing other tasks while the worker runs in parallel.
2. What is a Web Worker?
A Web Worker is a JavaScript thread that runs in parallel to the main browser thread. It can perform tasks like computationally expensive calculations, data fetching, or manipulating large datasets without causing UI freezing or blocking the user interaction. Web Workers communicate with the main thread using a messaging system.
3. Types of Web Workers
- Dedicated Workers: These workers are created for a single page and can handle tasks specifically for that page. They are not shared between multiple pages.
- Shared Workers: These workers are shared between multiple browser windows or tabs, enabling them to communicate and share data.
- Service Workers: Special type of workers that act as a proxy between the web app and the network. Service workers are used for caching resources, handling background sync, and enabling offline functionality.
4. Setting Up Web Workers in Angular
Angular provides built-in support for Web Workers. To set up a Web Worker in Angular, follow these steps:
Step 1: Create a Web Worker
In Angular, you can generate a Web Worker using the Angular CLI. The `ng generate web-worker` command automatically creates the necessary worker files for you.
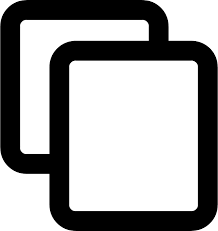
ng generate web-worker worker
This will create a worker file, typically named `worker.worker.ts`. This file will contain the code that is to be run in the Web Worker.
Step 2: Write the Web Worker Code
In your generated worker file, you can write the logic you want to run in the background. The Web Worker will listen to messages from the main thread and send back responses using `postMessage`.
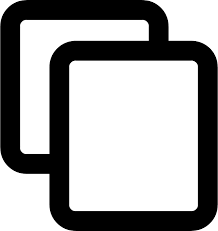
// worker.worker.ts
addEventListener('message', ({ data }) => {
const result = performHeavyTask(data);
postMessage(result); // Sending the result back to the main thread
});
function performHeavyTask(data: any) {
// Heavy computation or data processing
return data * 2; // Example: doubling the data
}
Step 3: Using the Web Worker in the Component
Once the worker is set up, you can instantiate and communicate with the worker from your Angular component. You can send messages to the worker using `postMessage` and listen for results using the `message` event.
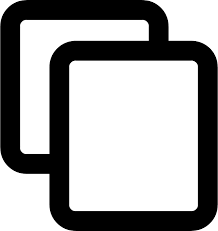
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
result: any;
// Create a new Worker instance
private worker = new Worker(new URL('./worker.worker', import.meta.url));
constructor() {
this.worker.onmessage = ({ data }) => {
this.result = data; // Handle the result from the worker
};
}
// Send data to the worker
sendDataToWorker() {
this.worker.postMessage(10); // Example: sending a number to the worker
}
}
5. Benefits of Using Web Workers in Angular
- Improved Performance: Web Workers help to offload intensive tasks from the main thread, resulting in better UI responsiveness.
- Non-blocking Execution: Tasks run in parallel, so the main UI thread remains free to handle user interactions and rendering.
- Better User Experience: By preventing the UI from freezing, Web Workers ensure a smooth and responsive experience, even during heavy computations.
6. Handling Web Worker Errors
Web Workers can throw errors that need to be caught and handled. Use the `onerror` event handler to manage errors that occur inside the Web Worker.
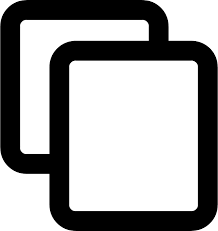
this.worker.onerror = (error) => {
console.error('Error in worker:', error);
};
7. Terminating a Web Worker
Once the task is complete, you can terminate the Web Worker to free up resources. This can be done using the `terminate` method.
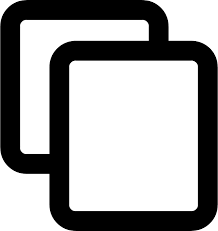
this.worker.terminate(); // Terminates the worker when no longer needed
8. Example: Using Web Workers for Heavy Computation
Consider using Web Workers for tasks such as sorting large datasets or performing complex calculations. The following example demonstrates offloading a sorting task to a Web Worker:
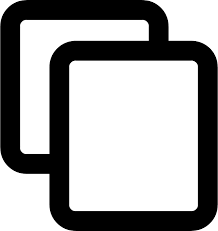
// worker.worker.ts
addEventListener('message', ({ data }) => {
const sortedData = data.sort((a, b) => a - b); // Sorting data
postMessage(sortedData);
});
9. Limitations of Web Workers
- Web Workers cannot directly manipulate the DOM. They can only communicate with the main thread via messages.
- Web Workers do not have access to the window object or document.
- Web Workers run in a separate context, meaning they do not share state with the main thread unless data is passed through messaging.
10. Conclusion
Web Workers are a powerful tool for improving the performance of Angular applications by offloading heavy tasks from the main thread. By using Web Workers, you can ensure that the UI remains responsive even during complex computations. Angular provides seamless support for Web Workers, making it easy to implement background processing for better user experience.
Server-Side Rendering (Angular Universal)
1. Overview
Server-Side Rendering (SSR) in Angular is made possible with Angular Universal. It allows Angular applications to be rendered on the server rather than in the browser. This provides several benefits, such as improved SEO, faster initial page load, and better performance for users with slow internet connections. By rendering the application on the server, Angular Universal sends the fully rendered HTML to the client, reducing the time it takes for the user to see the content.
2. What is Angular Universal?
Angular Universal is a set of technologies used to enable server-side rendering for Angular applications. It can be used to pre-render the application on the server so that the content is immediately visible to the user, even before the Angular application is fully bootstrapped on the client side.
Angular Universal also supports features like lazy loading, which enables the server to render only the necessary parts of the application, improving performance and SEO even further.
3. Benefits of Server-Side Rendering
- Improved SEO: Server-Side Rendering ensures that the HTML content is available to search engines immediately, improving the indexing and ranking of your application.
- Faster Initial Load: The server sends fully rendered HTML, which reduces the time users wait for content to display in the browser, especially on slower networks or devices.
- Better User Experience: By rendering content on the server, users can see the content faster, improving the overall user experience.
- Social Media Sharing: Pre-rendered content makes it easier for social media platforms to properly display previews when users share links to your application.
4. Setting Up Angular Universal
Setting up Angular Universal requires a few steps to integrate the server-side rendering into your Angular application. Here’s how you can set it up:
Step 1: Add Angular Universal to Your Project
You can use the Angular CLI to add Angular Universal to your existing Angular project. The CLI will automatically configure the necessary server-side rendering setup.
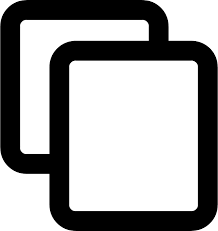
ng add @nguniversal/express-engine
This command adds the necessary dependencies and creates a new server-side rendering module and server file (typically using Express.js for handling server-side requests).
Step 2: Configure Angular Universal
Once you’ve added Angular Universal, you’ll need to configure your application. The CLI will automatically create a new file called `server.ts` for server-side configuration. This file is responsible for bootstrapping the Angular application on the server.
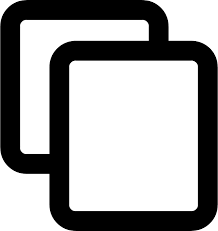
// server.ts
import 'zone.js'; // Importing zone.js for Angular Universal
import { enableProdMode } from '@angular/core';
import { app } from './main.server'; // Main server-side Angular module
import { environment } from './environments/environment';
if (environment.production) {
enableProdMode();
}
export { app };
Step 3: Update the Angular Application
Next, update the application’s `app.module.ts` and create a new `app.server.module.ts`. The `app.server.module.ts` will provide a platform for rendering the application on the server side.
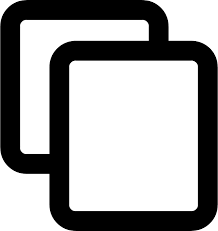
// app.server.module.ts
import { NgModule } from '@angular/core';
import { ServerModule } from '@angular/platform-server';
import { AppModule } from './app.module';
import { AppComponent } from './app.component';
@NgModule({
imports: [AppModule, ServerModule],
bootstrap: [AppComponent]
})
export class AppServerModule {}
Step 4: Running the Application with SSR
Once the configuration is complete, you can build and run the application with server-side rendering. Use the following commands to build and serve the application:
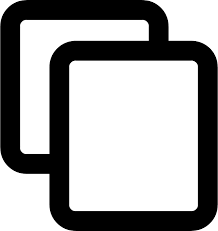
ng build --prod
ng run your-app-name:server
node dist/your-app-name/server/main.js
The application will now be served with server-side rendering, sending fully rendered HTML to the browser upon initial request.
5. Handling Lazy Loading with Angular Universal
Angular Universal supports lazy loading, meaning only the necessary parts of the application will be loaded by the server. This improves performance by reducing the amount of data that needs to be rendered initially. When you set up lazy-loaded routes, Angular Universal will render only the routes that are required at the time of the server request.
6. SEO Considerations
Angular Universal ensures that the content is available for search engines to crawl. Since the server sends fully rendered HTML, search engines can easily index the content. This is especially important for Single Page Applications (SPAs) that rely on JavaScript for rendering, which often causes issues with SEO if not configured properly.
7. Example: Pre-rendering with Angular Universal
Angular Universal also supports pre-rendering of pages. You can pre-render routes at build time instead of dynamically rendering them at runtime. This is useful for static content that doesn’t change often, such as landing pages or blog posts.
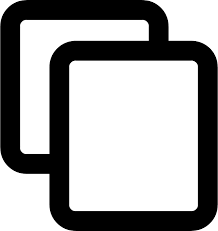
ng run your-app-name:prerender
8. Conclusion
Angular Universal enables Server-Side Rendering for Angular applications, improving SEO, performance, and user experience. By setting up SSR with Angular Universal, you can ensure that your Angular applications are fully optimized for both search engines and users, especially those with slow internet connections. With easy integration through Angular CLI, adding server-side rendering to your Angular application is straightforward and highly beneficial for performance and SEO.
Progressive Web Apps (PWAs) with Angular
1. Overview
Progressive Web Apps (PWAs) are web applications that provide a native app-like experience on the web. They combine the best of web and mobile apps, offering offline capabilities, push notifications, and faster loading times. PWAs are built using standard web technologies like HTML, CSS, and JavaScript, and they can be installed on a user's device, making them accessible even when offline.
2. What Makes a PWA?
PWAs have several key characteristics that make them stand out from traditional web applications:
- Responsive: PWAs are designed to work on any device, whether it is a desktop or a mobile device, and they adapt to different screen sizes.
- Connectivity Independent: PWAs can work offline or on low-quality networks, thanks to service workers that cache resources.
- App-like Interface: PWAs offer a native app-like experience with smooth animations, navigation, and interactions.
- Push Notifications: PWAs can send push notifications to users even when the app is not open, allowing for real-time updates.
- Installable: PWAs can be installed on a user's home screen, just like native mobile apps, without needing an app store.
3. Benefits of PWAs
- Improved Performance: PWAs load faster than traditional web apps, even on slow or unreliable networks, due to caching and service workers.
- Increased Engagement: PWAs can send push notifications to users, keeping them engaged and informed about updates or new content.
- Offline Support: PWAs can work offline by caching resources, making them usable even without an internet connection.
- Cost-Effective: PWAs eliminate the need for separate mobile app development for iOS and Android, reducing development and maintenance costs.
- SEO Benefits: PWAs are indexed by search engines, which helps improve discoverability and SEO rankings.
4. Setting Up a PWA in Angular
Angular makes it easy to create Progressive Web Apps using the Angular Service Worker. You can quickly transform an Angular application into a PWA by following these steps:
Step 1: Install Angular PWA Package
Use the Angular CLI to add PWA functionality to your existing Angular application.
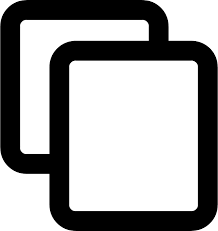
ng add @angular/pwa
This command installs the necessary dependencies and configures the Angular app to support PWA features, such as service workers and app manifest.
Step 2: Modify the Manifest File
After adding the PWA package, Angular will generate a `manifest.webmanifest` file in your `src` directory. This file contains metadata about your app, such as its name, icons, and theme colors, which will be used when the app is installed on a device.
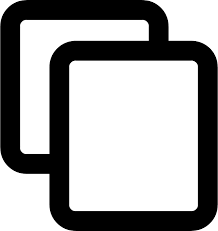
{
"name": "My PWA",
"short_name": "PWA",
"description": "A Progressive Web App built with Angular",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#1976d2",
"icons": [
{
"src": "assets/icons/icon-72x72.png",
"sizes": "72x72",
"type": "image/png"
},
{
"src": "assets/icons/icon-96x96.png",
"sizes": "96x96",
"type": "image/png"
}
]
}
Step 3: Enable Service Workers
Angular uses service workers to handle caching and offline functionality. The service worker will cache your app's assets and serve them when the user is offline. To enable service workers, make sure that the `ngsw-config.json` file is present, and the service worker is registered in the `app.module.ts` file.
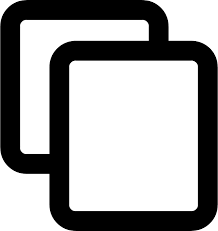
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { ServiceWorkerModule } from '@angular/service-worker';
import { AppComponent } from './app.component';
import { environment } from '../environments/environment';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
ServiceWorkerModule.register('ngsw-worker.js', { enabled: environment.production })
],
bootstrap: [AppComponent]
})
export class AppModule {}
Step 4: Building the PWA
Once you've set up the PWA, you can build the application for production. This will include the necessary service worker and manifest file.
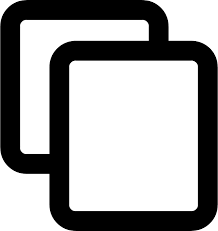
ng build --prod
5. Testing the PWA
After building your PWA, you can test it locally using a web server. This is important because service workers only work in a secure context (HTTPS or localhost). You can use the following command to serve your app locally:
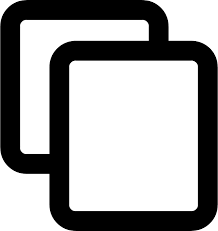
npx http-server -p 8080 -c-1
Then, navigate to `http://localhost:8080` to check the PWA features, such as offline functionality and installation prompts.
6. Push Notifications
One of the key features of PWAs is the ability to send push notifications to users. Angular’s service worker can handle push notifications, allowing the app to send updates even when the user is not actively using the app.
Step 1: Set Up Push Notifications
To use push notifications, you need to integrate Firebase Cloud Messaging (FCM) or any other push notification service into your Angular app. Once integrated, you can subscribe to push notifications and display them in your app.
Step 2: Handle Notifications in the Service Worker
You can handle background push notifications inside the `ngsw-worker.js` file and display them using the Notification API.
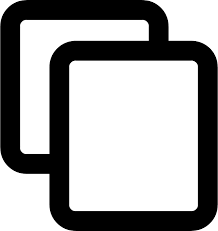
self.addEventListener('push', event => {
const options = {
body: event.data.text(),
icon: 'assets/icons/icon-72x72.png',
badge: 'assets/icons/icon-96x96.png'
};
event.waitUntil(
self.registration.showNotification('New Update', options)
);
});
7. Conclusion
Progressive Web Apps (PWAs) offer a powerful way to create fast, reliable, and engaging web applications with offline capabilities, push notifications, and the ability to be installed on a user’s device. With Angular, it's easy to add PWA features to your application using the Angular Service Worker. By following the steps outlined above, you can create a PWA that works seamlessly across all devices and platforms, providing a native app-like experience without the need for app store distribution.
Creating a To-Do App in Angular
1. Overview
A To-Do App is a simple task management application that helps users organize their daily tasks. In this guide, we will learn how to build a basic To-Do app using Angular, covering essential features such as adding tasks, marking tasks as complete, and removing tasks.
2. Setting Up the Angular Project
First, we need to create a new Angular project. You can do this using the Angular CLI (Command Line Interface).
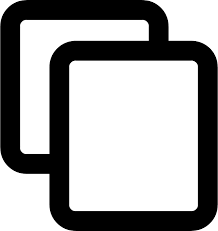
ng new todo-app
cd todo-app
This will create a new Angular project named `todo-app` and navigate to the project directory.
3. Creating the To-Do Model
We need to create a model to represent each task in our To-Do list. This model will have properties like `id`, `task`, and `completed`.
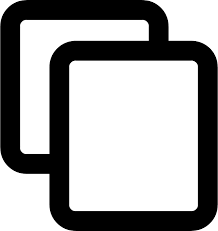
// src/app/todo.model.ts
export class Todo {
constructor(
public id: number,
public task: string,
public completed: boolean = false
) {}
}
The `Todo` class has three properties: `id` (a unique identifier), `task` (the task description), and `completed` (a boolean value to track whether the task is finished).
4. Creating the To-Do Service
Next, we will create a service that will handle the logic for adding, deleting, and updating tasks. The service will manage an array of tasks and provide methods to interact with it.
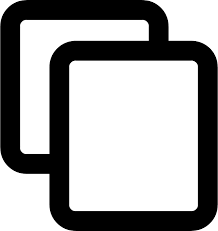
// src/app/todo.service.ts
import { Injectable } from '@angular/core';
import { Todo } from './todo.model';
@Injectable({
providedIn: 'root'
})
export class TodoService {
private todos: Todo[] = [];
constructor() {}
getTodos(): Todo[] {
return this.todos;
}
addTodo(task: string): void {
const newTodo = new Todo(this.todos.length + 1, task);
this.todos.push(newTodo);
}
removeTodo(id: number): void {
this.todos = this.todos.filter(todo => todo.id !== id);
}
toggleComplete(id: number): void {
const todo = this.todos.find(todo => todo.id === id);
if (todo) {
todo.completed = !todo.completed;
}
}
}
The `TodoService` class provides methods to: - `getTodos()` to retrieve the current list of tasks. - `addTodo()` to add a new task to the list. - `removeTodo()` to delete a task by its ID. - `toggleComplete()` to mark a task as completed or not completed.
5. Creating the To-Do Component
Now, we will create a component to display and interact with the To-Do list. This component will use the `TodoService` to manage tasks and bind the data to the template.
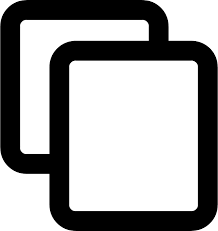
// src/app/todo/todo.component.ts
import { Component } from '@angular/core';
import { TodoService } from '../todo.service';
import { Todo } from '../todo.model';
@Component({
selector: 'app-todo',
templateUrl: './todo.component.html',
styleUrls: ['./todo.component.css']
})
export class TodoComponent {
todos: Todo[] = [];
newTask: string = '';
constructor(private todoService: TodoService) {
this.todos = this.todoService.getTodos();
}
addTodo(): void {
if (this.newTask.trim()) {
this.todoService.addTodo(this.newTask);
this.newTask = '';
}
}
removeTodo(id: number): void {
this.todoService.removeTodo(id);
}
toggleComplete(id: number): void {
this.todoService.toggleComplete(id);
}
}
The `TodoComponent` class uses the `TodoService` to fetch tasks, add new tasks, delete tasks, and toggle task completion. The template will bind to the `todos` array and provide buttons for user interaction.
6. Creating the To-Do Template
The template for the To-Do component will display the list of tasks and provide options for adding, completing, and removing tasks.
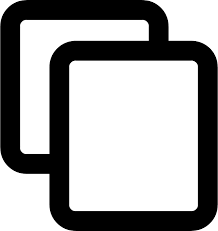
// src/app/todo/todo.component.html
<div class="todo-container">
<h2>To-Do List</h2>
<input [(ngModel)]="newTask" placeholder="Enter new task" />
<button (click)="addTodo()">Add Task</button>
<ul>
<li *ngFor="let todo of todos">
<input type="checkbox" [checked]="todo.completed" (change)="toggleComplete(todo.id)" />
<span [class.completed]="todo.completed">{{ todo.task }}</span>
<button (click)="removeTodo(todo.id)">Remove</button>
</li>
</ul>
</div>
The template includes: - An input field for entering new tasks. - A button to add new tasks to the list. - A list (`
- `) of tasks, where each task has a checkbox to mark it as complete, a button to remove it, and a styled task name.
7. Styling the To-Do App
We will add some basic styles to make the To-Do app visually appealing. This includes styling the completed tasks, input fields, and buttons.
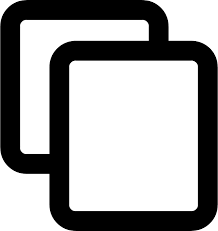
// src/app/todo/todo.component.css
.todo-container {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #f9f9f9;
}
input[type="text"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border-radius: 3px;
border: 1px solid #ccc;
}
button {
padding: 5px 10px;
margin-left: 10px;
border: none;
background-color: #4CAF50;
color: white;
cursor: pointer;
border-radius: 3px;
}
button:hover {
background-color: #45a049;
}
.completed {
text-decoration: line-through;
color: grey;
}
8. Conclusion
In this tutorial, we have created a simple To-Do app using Angular. The app allows users to add, remove, and mark tasks as completed. We used Angular services to manage the state of the tasks and Angular's two-way data binding to connect the component's logic with the template. This basic app can be expanded with additional features like editing tasks, saving tasks to local storage, or integrating with a backend API.
Building a CRUD Application in Angular
1. Overview
CRUD stands for Create, Read, Update, and Delete — the four basic operations for managing data in an application. In this tutorial, we'll build a simple CRUD application using Angular, where users can manage a list of items. We will create a service to handle data operations and a component to interact with that service.
2. Setting Up the Angular Project
First, create a new Angular project using Angular CLI. This project will be used to demonstrate CRUD operations.
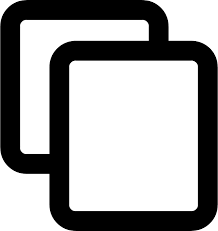
ng new crud-app
cd crud-app
This will create a new Angular project called `crud-app` and navigate to the project directory.
3. Creating the Item Model
We need to define a model for the items we will manage in our CRUD app. The model will include properties like `id`, `name`, and `description`.
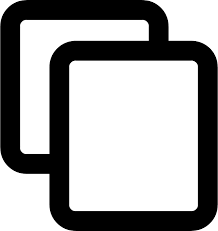
// src/app/item.model.ts
export class Item {
constructor(
public id: number,
public name: string,
public description: string
) {}
}
The `Item` class represents each item with an `id`, `name`, and `description`.
4. Creating the CRUD Service
The service will handle all data operations such as creating, reading, updating, and deleting items. We will use an array to simulate a database for simplicity.
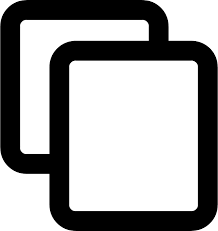
// src/app/item.service.ts
import { Injectable } from '@angular/core';
import { Item } from './item.model';
@Injectable({
providedIn: 'root'
})
export class ItemService {
private items: Item[] = [
new Item(1, 'Item 1', 'Description of Item 1'),
new Item(2, 'Item 2', 'Description of Item 2'),
];
constructor() {}
getItems(): Item[] {
return this.items;
}
addItem(item: Item): void {
this.items.push(item);
}
updateItem(id: number, updatedItem: Item): void {
const item = this.items.find(i => i.id === id);
if (item) {
item.name = updatedItem.name;
item.description = updatedItem.description;
}
}
deleteItem(id: number): void {
this.items = this.items.filter(i => i.id !== id);
}
}
The `ItemService` class provides methods to: - `getItems()` to fetch all items. - `addItem()` to add a new item. - `updateItem()` to update an existing item. - `deleteItem()` to delete an item by its ID.
5. Creating the CRUD Component
The component will interact with the service to perform the CRUD operations. It will display a list of items and provide forms to create or update items.
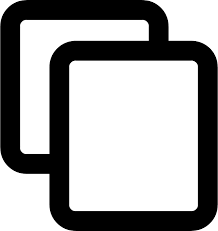
// src/app/item/item.component.ts
import { Component } from '@angular/core';
import { ItemService } from '../item.service';
import { Item } from '../item.model';
@Component({
selector: 'app-item',
templateUrl: './item.component.html',
styleUrls: ['./item.component.css']
})
export class ItemComponent {
items: Item[] = [];
newItem: Item = new Item(0, '', '');
editMode: boolean = false;
editId: number | null = null;
constructor(private itemService: ItemService) {
this.items = this.itemService.getItems();
}
addItem(): void {
if (this.newItem.name.trim() && this.newItem.description.trim()) {
this.itemService.addItem(this.newItem);
this.newItem = new Item(0, '', '');
}
}
editItem(item: Item): void {
this.editMode = true;
this.newItem = { ...item };
this.editId = item.id;
}
updateItem(): void {
if (this.editId !== null && this.newItem.name.trim() && this.newItem.description.trim()) {
this.itemService.updateItem(this.editId, this.newItem);
this.newItem = new Item(0, '', '');
this.editMode = false;
this.editId = null;
}
}
deleteItem(id: number): void {
this.itemService.deleteItem(id);
}
}
The `ItemComponent` class manages the item list and provides methods for adding, editing, updating, and deleting items. It also handles toggling between create and edit modes.
6. Creating the CRUD Template
The template will display the list of items and provide a form to create or update an item. There will also be buttons to perform delete and edit operations.
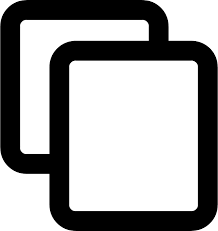
// src/app/item/item.component.html
<div class="crud-container">
<h2>CRUD Application</h2>
<div>
<h3 *ngIf="editMode">Edit Item</h3>
<h3 *ngIf="!editMode">Create Item</h3>
<input [(ngModel)]="newItem.name" placeholder="Item Name" />
<input [(ngModel)]="newItem.description" placeholder="Item Description" />
<button (click)="editMode ? updateItem() : addItem()">
{{ editMode ? 'Update' : 'Add' }} Item
</button>
</div>
-
{{ item.name }}: {{ item.description }}