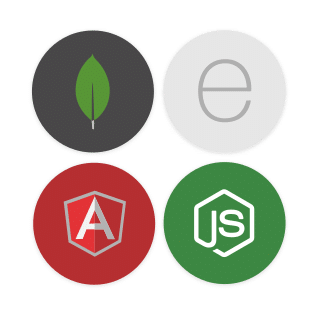
What is the MEAN Stack?
The MEAN stack is a collection of technologies used to develop web applications. It comprises four main technologies, each offering a specific feature in the development process:
- MongoDB: A NoSQL database used to store data in a flexible, JSON-like format.
- Express.js: A web application framework for Node.js that simplifies the creation of server-side applications.
- Angular: A front-end framework for building dynamic and single-page web applications.
- Node.js: A runtime environment that allows you to run JavaScript on the server-side.
Benefits of the MEAN Stack
The MEAN stack offers several advantages for developers building modern web applications:
Benefit | Description |
---|---|
Full JavaScript Stack | The MEAN stack allows you to use JavaScript across both client-side and server-side development, making it easier to manage the codebase. |
Fast Development | With the combination of Express.js, Node.js, and Angular, developers can quickly build and deploy applications without worrying about compatibility issues between technologies. |
Scalability | MongoDB offers scalability and flexibility, allowing developers to handle large amounts of data efficiently as the application grows. |
Active Community | Each technology in the MEAN stack has a large and active community, offering extensive resources, tutorials, and libraries for developers to use. |
Setting Up the MEAN Stack
To get started with the MEAN stack, follow these steps:
- Install Node.js from the official website.
- Install MongoDB by following the instructions on the MongoDB website.
- Set up Angular using the Angular CLI by running
npm install -g @angular/cli
in your terminal. - Set up Express.js by creating a Node.js application and installing the
express
package using npm.
Code Example: Simple MEAN Stack API
Here is a simple example of how to create a RESTful API using Express.js and MongoDB in a MEAN stack application:
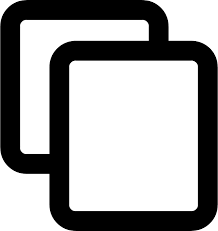
// app.js (Express + MongoDB)
const express = require('express');
const mongoose = require('mongoose');
const app = express();
mongoose.connect('mongodb://localhost:27017/mean-stack', { useNewUrlParser: true, useUnifiedTopology: true });
const Item = mongoose.model('Item', { name: String });
app.get('/items', async (req, res) => {
const items = await Item.find();
res.json(items);
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Diagram: MEAN Stack Architecture
The following diagram illustrates the architecture of a MEAN stack application:

This diagram shows how the MongoDB database, Express.js server, Angular front-end, and Node.js runtime work together to create a dynamic web application.
Benefits of Using MEAN for Web Development
The MEAN stack provides many advantages when developing modern web applications:
- Full JavaScript Stack: All components are written in JavaScript, simplifying development.
- Single Language Development: Developers only need to be proficient in JavaScript for both client-side and server-side development.
- Fast Development: Rapid development with tools like Angular CLI and Express.js.
- Scalability: MongoDB provides a flexible, scalable database solution that grows with your application.
- Large Community: Each MEAN stack technology has a large, active community for support and resources.
Understanding Each Component of the MEAN Stack
MongoDB
MongoDB is a NoSQL, document-based database that stores data in flexible, JSON-like format. It is highly scalable and suitable for applications with large amounts of unstructured data.
Express.js
Express.js is a minimal and flexible Node.js web application framework that simplifies routing and handling HTTP requests. It's designed to build APIs and web applications efficiently.
Angular
Angular is a TypeScript-based front-end framework that helps developers build dynamic, single-page web applications with two-way data binding, components, and modular architecture.
Node.js
Node.js is a JavaScript runtime that allows developers to run JavaScript on the server side. It is asynchronous and event-driven, making it ideal for building scalable network applications.
MEAN vs. MERN vs. Other Stacks
When comparing MEAN with other popular stacks, here are some key differences:
Stack | Key Technologies | Advantages |
---|---|---|
MEAN | MongoDB, Express.js, Angular, Node.js | Great for full JavaScript-based development; Angular provides a powerful front-end framework with two-way data binding. |
MERN | MongoDB, Express.js, React, Node.js | React is more lightweight and flexible than Angular, ideal for building dynamic UIs with a component-based structure. |
LAMP | Linux, Apache, MySQL, PHP | Traditional, open-source stack for building dynamic websites with a mature set of tools. |
JAMstack | JavaScript, APIs, Markup | Optimized for static site generation and fast performance, ideal for modern web apps with minimal back-end complexity. |
Installing Node.js and npm
Node.js is a JavaScript runtime, and npm (Node Package Manager) is used to manage project dependencies. Follow the steps below to install both:
- Visit the official Node.js website and download the installer for your OS.
- Run the installer and follow the prompts to install Node.js and npm.
- After installation, open your terminal or command prompt and type
node -v
andnpm -v
to verify the installation.
Installing MongoDB Locally or Using Cloud (MongoDB Atlas)
MongoDB can be installed locally or used via MongoDB Atlas, a cloud service. Here's how to do both:
Installing MongoDB Locally
- Visit the MongoDB Community Download page.
- Choose your OS and download the installer.
- Follow the installation instructions to set up MongoDB on your local machine.
- Once installed, use
mongod
to start the MongoDB server.
Using MongoDB Atlas (Cloud)
- Go to MongoDB Atlas and sign up for an account.
- Create a new cluster and follow the instructions to configure it.
- Once the cluster is set up, you can connect to it using the provided connection string in your application.
Setting Up Angular CLI
Angular CLI is a command-line interface tool that helps you automate tasks in Angular development. Follow these steps to install and set it up:
- Ensure that you have Node.js installed on your system (see previous section).
- Open your terminal and run the following command to install Angular CLI globally:
- Once installed, you can create a new Angular project by running:
- Navigate to your project directory and serve your app:
- Your Angular app should now be running at http://localhost:4200.
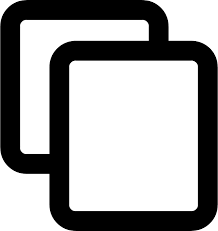
npm install -g @angular/cli
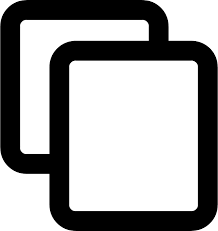
ng new my-angular-app
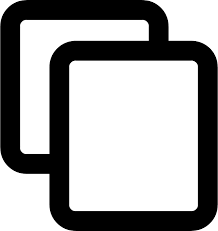
cd my-angular-app
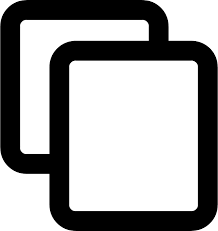
ng serve
Project Structure for MEAN Applications
When building a MEAN stack application, the project structure is crucial for organizing your code. Below is a common structure for MEAN applications:
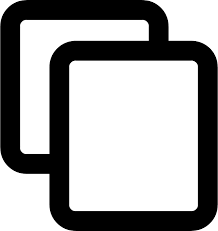
my-mean-app/
├── server/ # Node.js and Express backend
│ ├── config/ # Configuration files (e.g., database connections)
│ ├── controllers/ # Express controllers for handling routes
│ ├── models/ # Mongoose models for MongoDB
│ └── routes/ # Express route definitions
│
├── client/ # Angular front-end
│ ├── src/
│ │ ├── app/ # Angular components and services
│ │ ├── assets/ # Static files (images, styles, etc.)
│ │ └── environments/ # Environment configurations (dev, prod)
│ └── angular.json # Angular project configuration
│
├── node_modules/ # Installed npm packages
├── package.json # Project metadata and dependencies
└── .gitignore # Git ignore file
This structure separates the client and server code, making it easier to manage the application and scale it in the future.
Introduction to Node.js and Non-blocking I/O
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine that allows developers to run JavaScript code outside the browser. It's commonly used for building scalable network applications, particularly web servers and APIs. Node.js is designed to be lightweight and efficient, making it a popular choice for real-time applications like chat apps and live data streaming.
What is Non-blocking I/O?
One of the key features of Node.js is its non-blocking, event-driven I/O model. In traditional, blocking I/O operations, the system must wait for one operation to complete before starting the next one. This can lead to delays and inefficiency, especially in applications that make many I/O requests (such as reading from a database or handling HTTP requests).
In contrast, Node.js uses non-blocking I/O, which means that operations like reading from a file or making HTTP requests do not block the execution of other code. Instead, Node.js initiates the operation and continues executing other code while waiting for the operation to complete. Once the operation is finished, a callback function is called to handle the result.
Benefits of Non-blocking I/O in Node.js
- Efficiency: Node.js can handle multiple operations at the same time, which makes it more efficient in handling concurrent requests.
- Scalability: Non-blocking I/O allows Node.js to handle a large number of requests with minimal overhead, making it ideal for building scalable applications.
- Low Latency: As I/O operations do not block the execution thread, Node.js can respond to requests more quickly, resulting in lower latency.
Example: Synchronous vs. Asynchronous Code
Let’s look at an example to understand how synchronous and asynchronous (non-blocking) code works:
Synchronous (Blocking) Code:
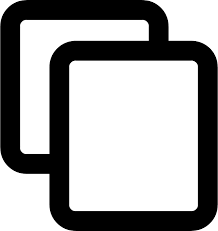
const fs = require('fs');
console.log('Start reading file');
const data = fs.readFileSync('file.txt', 'utf8'); // Blocking operation
console.log('File content:', data);
console.log('End reading file');
In the above example, the program will wait for the file to be read before continuing to the next line of code. This can be inefficient if you're reading multiple files or handling many requests.
Asynchronous (Non-blocking) Code:
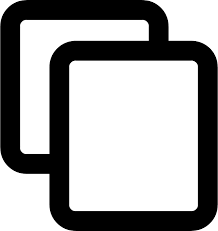
const fs = require('fs');
console.log('Start reading file');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log('File content:', data); // Callback when file is read
});
console.log('End reading file');
With asynchronous code, the program continues execution without waiting for the file to be read. The callback function is called once the file reading operation is complete.
Understanding the Event Loop
The Event Loop is one of the most important concepts in Node.js. It is the mechanism that allows Node.js to perform non-blocking I/O operations, despite being single-threaded. Understanding the Event Loop is crucial for understanding how Node.js handles concurrency and asynchronous operations.
What is the Event Loop?
The Event Loop is a core part of Node.js's architecture. It's responsible for handling multiple operations concurrently, without blocking the execution of the program. Although JavaScript in Node.js runs on a single thread, the Event Loop allows it to handle many tasks at once by delegating operations (such as I/O) to the underlying system, and only executing JavaScript code when necessary.
How the Event Loop Works
The Event Loop works in a sequence of phases. During each phase, specific tasks are processed. These phases are repeated continuously as long as there are operations to be handled.
Phases of the Event Loop:
- Timers: This phase executes callbacks for tasks scheduled by
setTimeout()
andsetInterval()
. - I/O Callbacks: This phase executes almost all callbacks, excluding timers, close callbacks, and a few others.
- Idle, Prepare: Internal phase used for housekeeping tasks.
- Poll: In this phase, the Event Loop checks if there are any I/O events to process. If there are, it processes those, and if not, it waits for them.
- Check: This phase runs callbacks that are scheduled by
setImmediate()
. - Close Callbacks: If there are any close events (like
socket.on('close', ...)
), they are executed in this phase.
The Event Loop continuously cycles through these phases and processes callbacks, managing asynchronous operations efficiently without blocking the main thread.
Example: Understanding the Event Loop
Let’s look at a simple example to understand how the Event Loop handles asynchronous code:
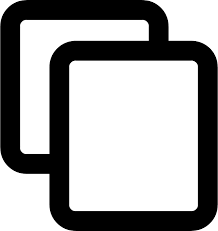
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
setImmediate(() => {
console.log('Immediate');
});
console.log('End');
In the above code, you might expect the output to be:
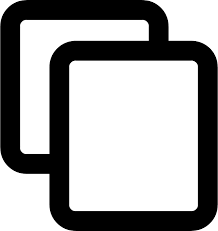
Start
End
Timeout
Immediate
However, the actual output is:
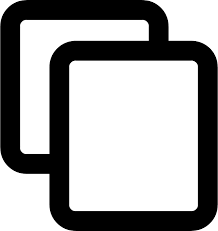
Start
End
Immediate
Timeout
This happens because setImmediate()
is executed in the "Check" phase, while setTimeout()
is executed in the "Timers" phase, which is processed after the I/O callbacks are completed.
Visualizing the Event Loop
The following diagram illustrates the flow of the Event Loop in Node.js:

This diagram shows how Node.js processes different phases of the Event Loop, handling tasks like I/O, timers, and immediate callbacks in a non-blocking manner.
Creating a Simple Node.js Server
Node.js is ideal for building web servers due to its non-blocking, event-driven nature. In this section, we will create a simple web server using Node.js to serve HTTP requests.
Setting Up a Simple HTTP Server
First, we will use Node.js's built-in http
module to create a basic server that listens for HTTP requests and responds with a simple message.
Code Example: Simple Node.js HTTP Server
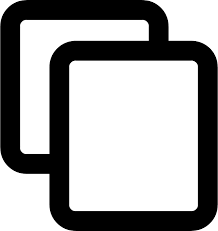
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
This code creates a server that listens on port 3000 and responds with "Hello, World!" when accessed through a browser or using tools like cURL or Postman.
Running the Server
To run the server, save the code in a file called server.js
and run the following command in your terminal:
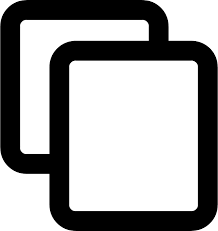
node server.js
Once the server is running, you can access it by opening a browser and navigating to http://127.0.0.1:3000.
Using Modules and Packages with npm
Node.js allows you to extend its functionality using external packages and modules. npm (Node Package Manager) is the default package manager for Node.js and is used to install and manage these external dependencies.
Installing npm and Initializing a Project
To start using npm, you need to initialize a project. Here’s how you can do it:
- Open your terminal and navigate to the project folder.
- Run the following command to initialize a new Node.js project:
- Follow the prompts to fill out project details. This will create a
package.json
file in the project directory, which will track your dependencies.
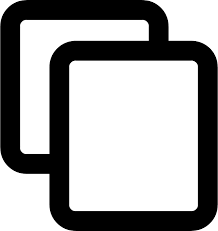
npm init
Installing External Packages
To install a package from the npm registry, use the npm install
command followed by the package name. For example, to install the popular express
package for handling HTTP requests in a Node.js application:
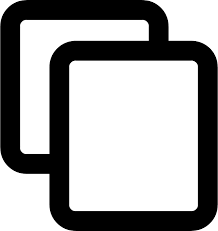
npm install express
This will download the express
package and add it to the node_modules
folder in your project. The dependency will also be added to the package.json
file.
Using Installed Packages
Once installed, you can import and use the package in your Node.js code. For example, to use the express
package to create a simple server:
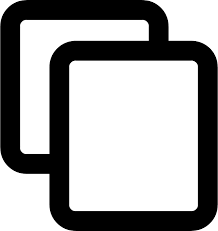
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Server running at http://127.0.0.1:3000/');
});
In this example, we used express
to create a simple web server that responds with "Hello, Express!" when accessed on port 3000.
Listing Installed Packages
To view all the installed packages in your project, you can run the following command:
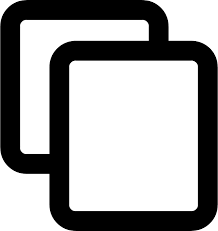
npm list
Uninstalling Packages
If you no longer need a package, you can uninstall it using the following command:
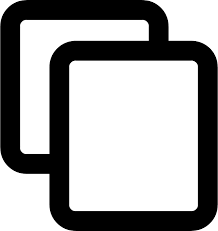
npm uninstall
For example, to uninstall express
, you would run:
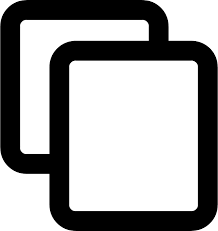
npm uninstall express
Introduction to Express.js
Express.js is a fast, unopinionated, and minimalist web framework for Node.js. It simplifies the process of building web applications by providing a robust set of features to handle HTTP requests, routing, middleware, and more.
What is Express.js?
Express.js is a framework built on top of Node.js that facilitates the creation of web servers and APIs. It abstracts away much of the repetitive code involved in handling HTTP requests and responses, allowing developers to focus on building the core functionality of their applications.
Setting Up Express.js
To get started with Express.js, you first need to install it in your Node.js project.
Step 1: Install Express
Open your terminal and run the following command to install Express in your project:
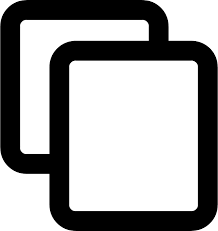
npm install express
Step 2: Create a Simple Express Server
After installing Express, you can create a basic server using the following code:
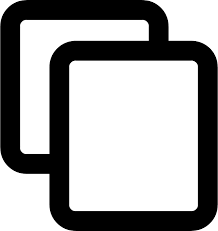
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Server running at http://127.0.0.1:3000/');
});
This code creates a simple Express server that listens on port 3000 and responds with "Hello, Express!" when accessed in a browser or using a tool like cURL or Postman.
Understanding the Code
- express: This imports the Express module.
- app: This creates an instance of an Express application.
- app.get(): This defines a route that listens for GET requests at the root URL (`/`). The callback function sends a response of "Hello, Express!" to the client.
- app.listen(): This starts the server on port 3000 and logs a message once the server is running.
Running the Server
To run the server, save the code in a file called server.js
and run the following command in your terminal:
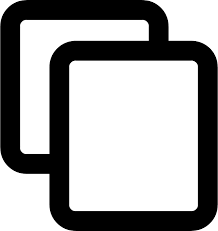
node server.js
Once the server is running, you can access it by navigating to http://127.0.0.1:3000 in your browser.
Creating Routes and Handling Requests
Express.js allows you to define routes that handle different HTTP requests (like GET, POST, PUT, DELETE) and specify how the server should respond to those requests. This is the core functionality of any web application or API.
What Are Routes?
Routes in Express are defined by the combination of an HTTP method (such as GET, POST, etc.) and a URL path. Each route is associated with a callback function that determines how to handle the request and send the response.
Creating Routes in Express
Routes are created using the HTTP methods (e.g., app.get()
, app.post()
, app.put()
, and app.delete()
). Each of these methods takes a path and a callback function that defines the behavior for that route.
Example: Defining a GET Route
Below is an example of defining a GET route that handles requests to the root URL (`/`):
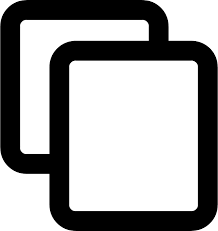
const express = require('express');
const app = express();
// Define a GET route
app.get('/', (req, res) => {
res.send('Welcome to the homepage!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, when a user sends a GET request to the root URL (`/`), the server responds with "Welcome to the homepage!".
Handling POST Requests
POST requests are typically used to submit data to the server. Below is an example of how to handle a POST request:
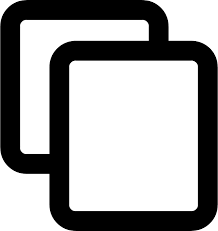
const express = require('express');
const app = express();
app.use(express.json()); // Middleware to parse JSON request body
// Define a POST route
app.post('/submit', (req, res) => {
const { name, email } = req.body;
res.send(`Received name: ${name} and email: ${email}`);
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, when a user sends a POST request to `/submit` with a JSON body, the server extracts the `name` and `email` from the request body and sends a response confirming the received data.
Handling PUT and DELETE Requests
PUT and DELETE requests are used for updating and deleting resources, respectively. Here are examples of both:
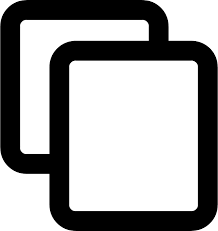
const express = require('express');
const app = express();
app.use(express.json()); // Middleware to parse JSON request body
// Handle PUT request
app.put('/update/:id', (req, res) => {
const { id } = req.params;
res.send(`Updating resource with ID: ${id}`);
});
// Handle DELETE request
app.delete('/delete/:id', (req, res) => {
const { id } = req.params;
res.send(`Deleting resource with ID: ${id}`);
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
The PUT request updates a resource based on the provided `id` in the URL, and the DELETE request deletes the resource with the specified `id`.
Route Parameters and Query Strings
Express allows you to capture dynamic parts of the URL using route parameters and query strings.
Example: Using Route Parameters
Route parameters are specified using `:` before the parameter name in the route path. For example, in the following route, the `id` is a route parameter:
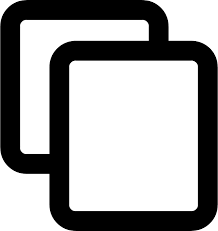
app.get('/user/:id', (req, res) => {
const { id } = req.params;
res.send(`User ID is: ${id}`);
});
In this case, the server will capture the `id` from the URL and send it back in the response.
Example: Using Query Strings
Query strings are appended to the URL after the `?` symbol and are typically used for optional parameters. Here's an example:
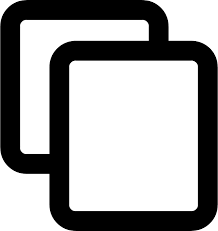
app.get('/search', (req, res) => {
const { query } = req.query;
res.send(`Search query: ${query}`);
});
In this example, the server expects a query string like ?query=express
, and it will return the value of the `query` parameter in the response.
Conclusion
Routes in Express allow you to handle different HTTP requests and perform actions based on the URL and method used. By creating routes for GET, POST, PUT, and DELETE requests, you can build a flexible and dynamic web application or API.
Middleware in Express.js
Middleware functions in Express.js are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application’s request-response cycle. They are used to perform a variety of tasks, such as logging, authentication, validation, error handling, etc.
What is Middleware?
Middleware is essentially a function that is executed during the request-response cycle. It can modify the request, the response, or even end the request-response cycle. Middleware functions can also call the next middleware in the stack by invoking the next()
function.
Types of Middleware
- Application-level middleware: Bound to an Express application instance, it runs for every incoming request.
- Router-level middleware: Bound to an Express router instance, it applies to routes that are handled by that router.
- Built-in middleware: Express provides some built-in middleware functions, like
express.json()
,express.static()
, etc. - Third-party middleware: Middleware functions from external libraries or packages.
Using Middleware in Express
To use middleware in Express, you call app.use()
followed by the middleware function. The middleware function can be written directly or imported from other modules.
Example: Application-Level Middleware
Here’s an example of a basic middleware that logs the request method and URL for every incoming request:
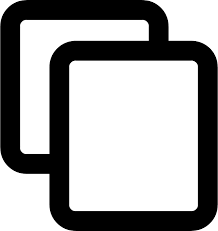
const express = require('express');
const app = express();
// Application-level middleware
app.use((req, res, next) => {
console.log(`${req.method} request to ${req.url}`);
next(); // Pass control to the next middleware or route handler
});
// Define a route
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In the example above, the middleware logs the HTTP method (e.g., GET, POST) and the requested URL each time a request is made to the server. The next()
function is called to pass control to the next middleware or route handler.
Example: Error Handling Middleware
Express provides a special kind of middleware for handling errors. This middleware is defined with four parameters (err
, req
, res
, next
).
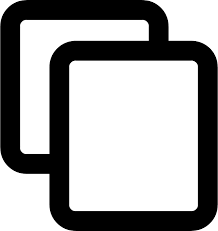
const express = require('express');
const app = express();
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
// A route that triggers an error
app.get('/', (req, res) => {
throw new Error('Oops!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, if an error is thrown in the route, the error handling middleware will catch it and return a 500 status with a message.
Example: Third-Party Middleware (CORS)
Third-party middleware can be added to an Express app by installing external packages. For example, to handle cross-origin requests, you can use the cors
package:
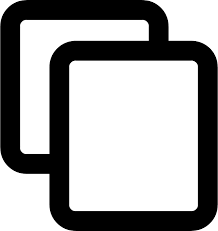
const express = require('express');
const cors = require('cors');
const app = express();
// Use CORS middleware
app.use(cors());
// Define a route
app.get('/', (req, res) => {
res.send('CORS-enabled for all origins!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
The cors()
middleware function enables CORS (Cross-Origin Resource Sharing) for all incoming requests, allowing the server to handle requests from different origins.
Custom Middleware
You can create your own middleware to perform tasks such as logging, authentication, data validation, etc. Here’s an example of creating custom middleware for logging request data:
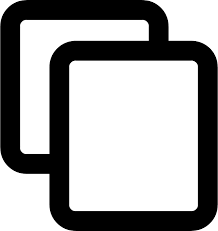
const express = require('express');
const app = express();
// Custom middleware for logging request data
const logRequestData = (req, res, next) => {
console.log(`Request URL: ${req.url}, Request Method: ${req.method}`);
next();
};
// Use custom middleware
app.use(logRequestData);
// Define a route
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
This custom middleware logs details about each incoming request before passing control to the next middleware or route handler.
Conclusion
Middleware is a powerful feature in Express.js that allows you to customize the request-response cycle. You can use built-in, third-party, or custom middleware to handle tasks such as logging, authentication, error handling, and more.
Error Handling in Express Applications
In Express.js, error handling is crucial to ensure that your application responds appropriately to errors, providing useful feedback to the client and preventing the server from crashing. Express provides a built-in mechanism for handling errors, allowing developers to define custom error-handling middleware.
What is Error Handling Middleware?
In Express, error handling middleware is a special type of middleware that has four arguments: err
, req
, res
, and next
. This middleware is used to catch errors that occur during the request-response cycle and respond accordingly.
Basic Error Handling Middleware
A basic error-handling middleware function typically looks like this:
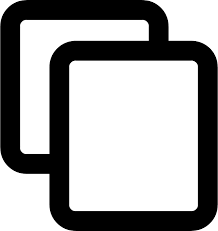
const express = require('express');
const app = express();
// Route that causes an error
app.get('/', (req, res) => {
throw new Error('Something went wrong!');
});
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In the example above, when an error occurs in the route, it is passed to the error-handling middleware using the next()
function. The middleware then logs the error and sends a generic 500 status response to the client.
Handling Different Types of Errors
In a real-world application, different types of errors might need to be handled differently. You can customize your error-handling middleware to handle different HTTP status codes based on the type of error.
Example: Handling Different HTTP Errors
Here’s an example of handling different types of errors with custom messages and status codes:
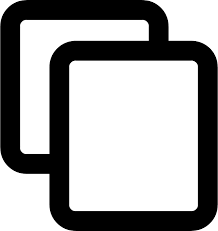
const express = require('express');
const app = express();
// Route that causes a 404 error
app.get('/not-found', (req, res) => {
throw new Error('Page not found!');
});
// Route that causes a 400 error
app.get('/bad-request', (req, res) => {
const error = new Error('Bad request');
error.status = 400; // Set custom status code
throw error;
});
// Error handling middleware
app.use((err, req, res, next) => {
if (err.status) {
res.status(err.status).send(err.message);
} else {
res.status(500).send('Internal Server Error');
}
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, custom error messages and status codes are set for different types of errors. The error-handling middleware checks if the error has a status
property and sends a response with the appropriate status code and message.
Async Error Handling in Express
Asynchronous code in Express (e.g., database queries, API calls) can also cause errors. To handle these errors, you can use async
functions with try-catch
blocks or use a helper function to catch unhandled promise rejections.
Example: Async Error Handling
Here’s an example of how to handle async errors in Express routes:
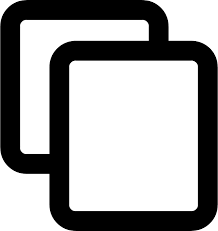
const express = require('express');
const app = express();
// Async route that throws an error
app.get('/async-error', async (req, res, next) => {
try {
const result = await someAsyncFunction();
res.send(result);
} catch (err) {
next(err); // Pass error to the error-handling middleware
}
});
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, the route uses an async function and the try-catch
block to catch errors. If an error occurs, it is passed to the error-handling middleware using next(err)
.
Custom Error Class
For better error handling, you can create a custom error class that extends the built-in Error
class. This allows you to provide more detailed error information, such as error codes and additional properties.
Example: Custom Error Class
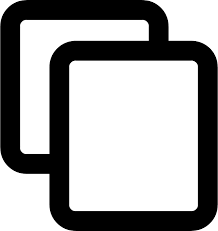
class CustomError extends Error {
constructor(message, statusCode) {
super(message);
this.status = statusCode;
}
}
const express = require('express');
const app = express();
// Route that throws a custom error
app.get('/custom-error', (req, res, next) => {
const error = new CustomError('Custom error occurred!', 400);
next(error); // Pass custom error to the next middleware
});
// Error handling middleware
app.use((err, req, res, next) => {
if (err instanceof CustomError) {
res.status(err.status).send(err.message);
} else {
res.status(500).send('Internal Server Error');
}
});
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, we define a CustomError
class to create errors with a specific status
and message
. The error-handling middleware then checks if the error is an instance of CustomError
and responds accordingly.
Conclusion
Effective error handling is essential for building robust Express applications. By using error-handling middleware, handling async errors, and creating custom error classes, you can ensure that your application handles errors gracefully and provides meaningful feedback to users.
Serving Static Files with Express
Express makes it easy to serve static files, such as images, CSS, and JavaScript files, in web applications. By using the built-in express.static
middleware, you can serve static assets from a directory in your project.
What Are Static Files?
Static files are those that do not change dynamically. These files are usually served as they are, without any processing. Common examples of static files include:
- Images (e.g., JPG, PNG, GIF)
- CSS files
- JavaScript files
- Fonts
Using express.static to Serve Static Files
To serve static files, you can use the express.static
middleware. This middleware serves files from a specified directory and makes them available for direct access via URL.
Example: Serving Static Files
Here is how you can set up Express to serve static files from a directory:
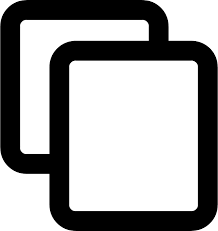
const express = require('express');
const app = express();
const path = require('path');
// Serve static files from the "public" directory
app.use(express.static(path.join(__dirname, 'public')));
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In the example above, we are using express.static
to serve files from the "public"
directory. When the app is running, you can access static files via their URL paths. For example, if there is an image called logo.png
inside the public directory, it can be accessed via http://127.0.0.1:3000/logo.png
.
Serving Static Files from Multiple Directories
Express also allows serving static files from multiple directories. This can be useful if you have different directories for different types of static files, such as images, stylesheets, and scripts.
Example: Multiple Directories
Here is an example of serving static files from multiple directories:
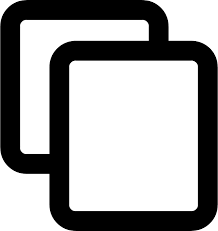
const express = require('express');
const app = express();
const path = require('path');
// Serve static files from "public" directory for images
app.use('/images', express.static(path.join(__dirname, 'public/images')));
// Serve static files from "assets" directory for CSS and JavaScript
app.use('/assets', express.static(path.join(__dirname, 'public/assets')));
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, static files in the "public/images"
directory will be accessible via http://127.0.0.1:3000/images
and files in the "public/assets"
directory will be accessible via http://127.0.0.1:3000/assets
.
Customizing the URL Path for Static Files
You can also customize the URL path for serving static files. For example, you may want your JavaScript files to be accessed via a custom route like /js/
instead of using the default path.
Example: Custom URL Path
Here’s how to customize the URL path for serving static files:
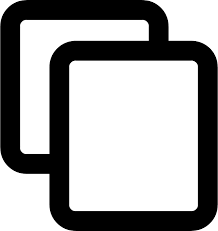
const express = require('express');
const app = express();
const path = require('path');
// Serve static files from "public/js" and access them with "/js"
app.use('/js', express.static(path.join(__dirname, 'public/js')));
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
In this example, files in the "public/js"
directory can be accessed via http://127.0.0.1:3000/js
.
Cache Control for Static Files
To improve performance, you can set up caching for static files using cache-control headers. This allows browsers to cache static files and reduce the number of requests to the server.
Example: Cache Control
Here’s an example of how to set caching headers for static files:
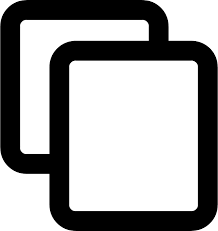
const express = require('express');
const app = express();
const path = require('path');
// Serve static files with cache-control header
app.use(express.static(path.join(__dirname, 'public'), {
maxAge: '1d' // Cache static files for 1 day
}));
app.listen(3000, () => {
console.log('Server running on http://127.0.0.1:3000');
});
The maxAge
option in the express.static
middleware tells the server to cache static files for 1 day. This will reduce the server load and improve client performance by allowing the browser to cache files and avoid repeated requests.
Conclusion
Serving static files is an essential feature in web development, and Express makes it easy to serve them efficiently. By using the express.static
middleware, you can quickly set up static file serving for images, JavaScript, CSS, and other assets. You can also control the URL path, set up caching, and serve files from multiple directories to organize your application better.
Introduction to MongoDB and NoSQL Databases
MongoDB is a popular NoSQL database that stores data in a flexible, JSON-like format known as BSON (Binary JSON). Unlike traditional relational databases, MongoDB does not use tables and rows but instead stores data in collections and documents. This allows for greater flexibility and scalability, making MongoDB ideal for modern web applications that require high performance and handle large amounts of unstructured data.
What is NoSQL?
NoSQL stands for "Not Only SQL," and it represents a category of databases that are designed to handle unstructured or semi-structured data. NoSQL databases are highly scalable and are used for applications that require high performance and real-time access to large amounts of data. Some key characteristics of NoSQL databases include:
- Schema-less data models
- Horizontal scalability (sharding)
- Support for different data formats like JSON, XML, or key-value pairs
- High availability and fault tolerance
Overview of MongoDB
MongoDB is one of the most popular NoSQL databases. It is designed to handle large amounts of data while providing high performance and flexibility. Unlike relational databases that use tables, MongoDB uses collections to store data in documents. Each document is a self-contained unit, typically represented in BSON format.
Key Features of MongoDB
- Document-Oriented: Data is stored in JSON-like documents that can have nested structures and dynamic schemas.
- Scalability: MongoDB supports horizontal scaling through a process called sharding, which distributes data across multiple servers.
- High Availability: MongoDB provides replica sets, which allow for data redundancy and failover in case of server failure.
- Flexible Schema: MongoDB allows documents in a collection to have different structures, making it easy to adapt to changing data requirements.
MongoDB Architecture
MongoDB's architecture is built around three main components:
- Database: A container for collections.
- Collection: A group of documents, similar to a table in relational databases.
- Document: The individual record, similar to a row in relational databases. Each document is stored in BSON format.
Setting Up MongoDB
To get started with MongoDB, you need to install it on your system or use a cloud-based service like MongoDB Atlas.
Steps to Install MongoDB Locally
- Download MongoDB from the official MongoDB website.
- Follow the installation instructions for your operating system (Windows, macOS, or Linux).
- Start the MongoDB server by running the following command in your terminal:
mongod
- To connect to MongoDB, run the following command in another terminal:
mongo
Basic MongoDB Operations
Here are some basic MongoDB operations you can perform after setting up MongoDB:
1. Creating a Database
To create a new database, use the use
command:
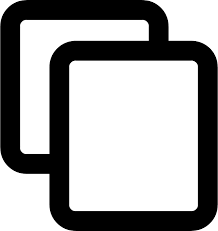
use myDatabase
This will create a new database named myDatabase
if it doesn't already exist.
2. Creating a Collection
To create a new collection, use the db.createCollection()
method:
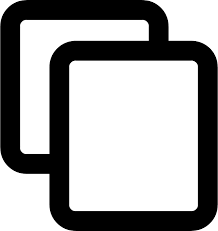
db.createCollection('myCollection')
3. Inserting Data into a Collection
To insert data into a collection, use the insertOne()
or insertMany()
methods:
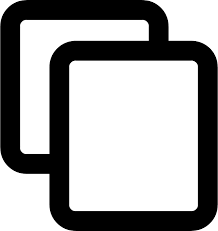
db.myCollection.insertOne({
name: 'John Doe',
age: 30,
email: 'john@example.com'
})
4. Querying Data
To query data from a collection, use the find()
method:
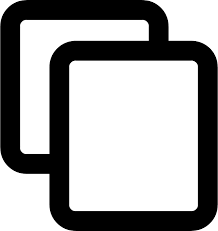
db.myCollection.find({ name: 'John Doe' })
MongoDB vs. Relational Databases
MongoDB and relational databases like MySQL or PostgreSQL differ in several ways:
- Data Model: MongoDB uses a document-based model, while relational databases use a table-based model with rows and columns.
- Schema: MongoDB is schema-less, meaning documents in a collection can have different structures, while relational databases have fixed schemas.
- Scalability: MongoDB supports horizontal scaling (sharding), while relational databases typically scale vertically (adding more power to a single server).
- Relationships: MongoDB does not use joins like relational databases but instead uses embedded documents and references to represent relationships.
Conclusion
MongoDB is a powerful, flexible, and scalable NoSQL database ideal for modern applications. Its document-oriented model allows developers to easily work with complex, unstructured data. By understanding the basics of MongoDB, including its architecture and operations, you'll be able to leverage its full potential for your web applications.
Setting Up a MongoDB Database
Setting up MongoDB involves installing MongoDB on your system or using a cloud-based solution like MongoDB Atlas. In this section, we will go through both local installation and setting up a cloud-based MongoDB database using MongoDB Atlas.
1. Setting Up MongoDB Locally
To set up MongoDB locally, follow the steps below:
Step 1: Download MongoDB
Visit the official MongoDB download page to download the community edition of MongoDB for your operating system (Windows, macOS, or Linux).
Step 2: Install MongoDB
Follow the installation instructions for your operating system:
- Windows: Run the installer and follow the on-screen instructions to install MongoDB.
- macOS: You can use Homebrew to install MongoDB with the command:
brew tap mongodb/brew && brew install mongodb-community
- Linux: Use your system's package manager (e.g.,
apt
for Ubuntu) to install MongoDB.
Step 3: Start MongoDB Server
After installation, start the MongoDB server by running the following command:
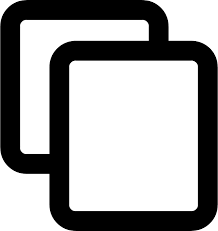
mongod
This starts the MongoDB server and opens the default port (27017) for connections.
Step 4: Connect to MongoDB
To connect to the MongoDB server, open another terminal window and run:
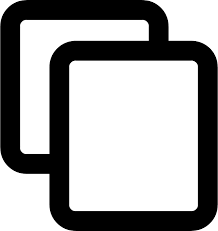
mongo
This opens the MongoDB shell, where you can begin working with your database.
2. Setting Up MongoDB Atlas (Cloud-based Database)
If you prefer a cloud-based solution, you can use MongoDB Atlas, which is a fully managed database service. Below are the steps to set up a MongoDB database on Atlas:
Step 1: Create an Account on MongoDB Atlas
Go to the MongoDB Atlas website and create a free account.
Step 2: Create a New Project
Once logged in, create a new project by clicking on the "Create a New Project" button. Give it a name and click "Next."
Step 3: Build a Cluster
After creating your project, click on the "Build a Cluster" button. MongoDB Atlas will guide you through the process of choosing your cluster configuration (e.g., cloud provider, region, and cluster tier). The free tier (M0) is sufficient for most small projects.
Step 4: Create a Database User
After setting up the cluster, create a database user by clicking on "Database Access" in the left sidebar. Click "Add New Database User," enter a username and password, and assign the user appropriate privileges.
Step 5: Get Connection String
Once your cluster is up and running, click on the "Connect" button. Choose "Connect Your Application" and copy the connection string provided. You’ll need this to connect your application to MongoDB Atlas.
Step 6: Connect to MongoDB Atlas
To connect to the Atlas database from your local machine, use a MongoDB client or your application code. For example, with Node.js, you can use the following code:
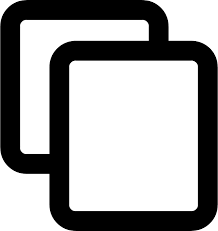
const mongoose = require('mongoose');
const uri = 'your-atlas-connection-string';
mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB Atlas'))
.catch(err => console.error('Error connecting to MongoDB Atlas:', err));
Replace 'your-atlas-connection-string'
with the connection string you copied from MongoDB Atlas. This will establish a connection to your MongoDB Atlas database.
3. MongoDB Atlas vs. Local MongoDB
Using MongoDB Atlas offers several advantages over running MongoDB locally:
- Managed Service: MongoDB Atlas is a fully managed service, so you don’t have to worry about maintenance tasks like backups or scaling.
- Cloud Scalability: MongoDB Atlas provides easy horizontal scaling to meet growing data demands.
- Global Distribution: Atlas allows you to deploy clusters in multiple geographic regions for low-latency data access.
- Security: MongoDB Atlas includes built-in security features like encryption, authentication, and access control.
4. Verifying Your Database Setup
Once MongoDB is set up, verify that everything is working by connecting to the database and performing some basic operations, such as creating a database, inserting documents, and querying data.
Example: Inserting a Document
To insert a document into a collection, use the following command in the MongoDB shell:
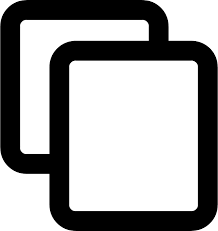
db.users.insertOne({ name: 'Alice', age: 25 })
Conclusion
Setting up a MongoDB database is straightforward, whether you’re using a local instance or a cloud-based solution like MongoDB Atlas. MongoDB’s flexible document-oriented model makes it an excellent choice for modern web applications, and with the right setup, you can begin using it to handle your data efficiently.
Introduction to Mongoose for MongoDB Integration
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It provides a powerful set of tools for interacting with MongoDB databases in a more structured way, allowing you to define schemas, models, and perform operations such as querying, validation, and data manipulation in a more seamless manner.
Why Use Mongoose?
While MongoDB is a flexible NoSQL database, Mongoose provides several features that make it easier to work with MongoDB in Node.js applications:
- Schema Definition: Mongoose allows you to define schemas to enforce structure on your MongoDB documents.
- Modeling: With Mongoose, you can create models from schemas that allow you to interact with data in a more object-oriented way.
- Querying: Mongoose provides a powerful query builder that makes it easy to find, update, and delete documents from MongoDB.
- Validation: Mongoose allows you to define validation rules for fields in your schema, ensuring data consistency and integrity.
- Middleware: Mongoose offers middleware functions that can be run before or after certain operations, such as saving or removing documents.
Installing Mongoose
To get started with Mongoose, first, you need to install it in your Node.js application. Run the following command to install Mongoose via npm:
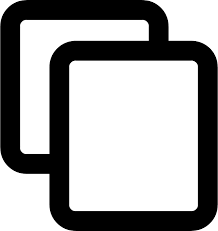
npm install mongoose
Basic Usage of Mongoose
Once Mongoose is installed, you can start using it to interact with MongoDB. Below is a simple example of how to use Mongoose to connect to a MongoDB database and create a model.
1. Connecting to MongoDB
To connect to MongoDB, you need to pass the connection string to Mongoose. Here’s an example of how to connect to a local MongoDB instance:
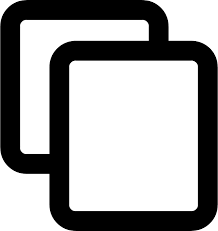
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => console.log('MongoDB connected'))
.catch(err => console.error('MongoDB connection error:', err));
2. Defining a Schema
In Mongoose, a schema defines the structure of your documents. Here’s an example of how to define a schema for a "User" document:
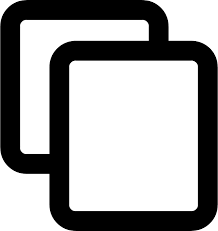
const userSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String, required: true, unique: true },
age: { type: Number, min: 18 }
});
3. Creating a Model
Once you have a schema, you can create a model from it. A model is a wrapper for the schema and provides methods to interact with the documents:
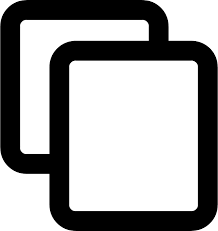
const User = mongoose.model('User', userSchema);
4. Creating and Saving a Document
After defining the model, you can create and save documents to MongoDB. Below is an example of how to create a new user and save it to the database:
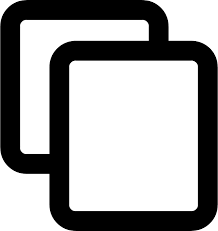
const newUser = new User({
name: 'Alice Johnson',
email: 'alice@example.com',
age: 25
});
newUser.save()
.then(user => console.log('User saved:', user))
.catch(err => console.error('Error saving user:', err));
5. Querying Data
You can use Mongoose to find documents in your MongoDB database with various query methods. Below is an example of finding a user by their email address:
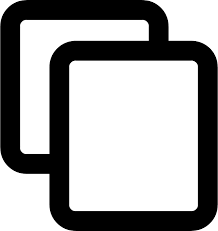
User.findOne({ email: 'alice@example.com' })
.then(user => console.log('Found user:', user))
.catch(err => console.error('Error finding user:', err));
Middleware in Mongoose
Mongoose allows you to define middleware functions that run during certain operations. Middleware is useful for tasks like validation, logging, and modifying data before or after it is saved to the database.
Example: Pre-save Middleware
Here is an example of using a pre-save middleware to hash a password before saving a user:
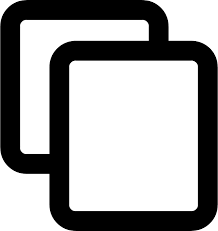
userSchema.pre('save', function(next) {
// Hash the password before saving the user document
if (this.isModified('password')) {
this.password = hashPassword(this.password);
}
next();
});
Conclusion
Mongoose is an essential tool for working with MongoDB in Node.js applications. It helps you structure your data, perform queries, handle validation, and work with MongoDB in an object-oriented manner. With Mongoose, you can easily define schemas, create models, and perform operations on your data.
Defining and Using Schemas and Models
In Mongoose, schemas are used to define the structure of the documents within a collection, while models are used to create, query, and interact with the documents. A schema is a blueprint for the data, and the model provides the tools to work with that data in the database.
Defining a Schema
A schema in Mongoose defines the structure of the documents within a collection. It defines the fields in the document, their types, and any validation rules, default values, and other properties.
Example: Defining a User Schema
Here’s how you can define a simple schema for a "User" model that includes fields for name, email, and age:
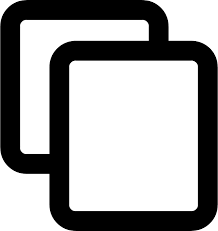
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String, required: true, unique: true },
age: { type: Number, min: 18 }
});
The schema defines three fields: name
, email
, and age
. The name
and email
fields are required, and the email
field must be unique. The age
field must be at least 18.
Creating a Model
Once you’ve defined a schema, you can create a model from it. A model is a wrapper for the schema that gives you methods to interact with the MongoDB collection.
Example: Creating a User Model
Here’s how you can create a model from the userSchema
and use it to interact with the "users" collection in MongoDB:
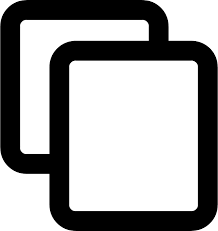
const User = mongoose.model('User', userSchema);
Using Models to Create and Query Documents
Once you have a model, you can use it to create, read, update, and delete documents in the database. Below are examples of how to create a new user and find users based on certain criteria:
1. Creating and Saving a Document
Below is an example of how to create a new user document and save it to the database:
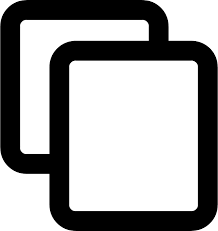
const newUser = new User({
name: 'Alice Johnson',
email: 'alice@example.com',
age: 25
});
newUser.save()
.then(user => console.log('User saved:', user))
.catch(err => console.error('Error saving user:', err));
2. Querying Documents
You can use Mongoose to find documents in your collection. Below is an example of how to find a user by their email address:
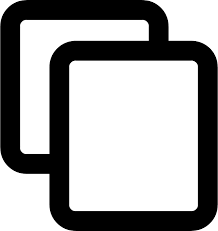
User.findOne({ email: 'alice@example.com' })
.then(user => console.log('Found user:', user))
.catch(err => console.error('Error finding user:', err));
3. Querying All Documents
You can also find all users that match certain criteria. Below is an example of how to find all users who are 18 or older:
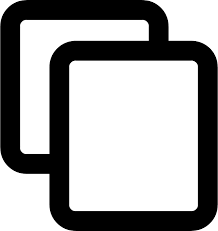
User.find({ age: { $gte: 18 } })
.then(users => console.log('Users 18 or older:', users))
.catch(err => console.error('Error fetching users:', err));
Additional Schema Options
Mongoose schemas allow you to define additional options and behavior. Here are a few examples:
1. Default Values
You can define default values for fields in your schema. Here’s an example of how to set a default value for the createdAt
field:
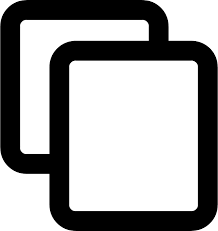
const postSchema = new mongoose.Schema({
title: { type: String, required: true },
content: String,
createdAt: { type: Date, default: Date.now }
});
2. Virtuals
You can define virtual fields that are not stored in the database but are derived from other fields. Here’s an example of a virtual field that concatenates the firstName
and lastName
fields:
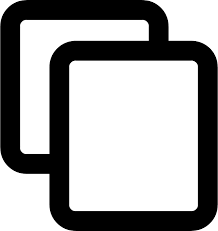
userSchema.virtual('fullName').get(function() {
return this.firstName + ' ' + this.lastName;
});
Conclusion
Defining schemas and models in Mongoose allows you to enforce structure on your MongoDB documents while providing powerful methods to interact with the data. By using schemas to define data types, validation rules, default values, and other options, and by using models to interact with the database, you can build robust and maintainable applications with MongoDB and Node.js.
CRUD Operations with Mongoose (Create, Read, Update, Delete)
In this section, we will cover the basic CRUD operations (Create, Read, Update, Delete) using Mongoose, which is an Object Data Modeling (ODM) library for MongoDB and Node.js.
Create Operation
The Create operation involves adding new documents to the MongoDB collection. In Mongoose, this can be done by creating an instance of a model and saving it to the database.
Example: Creating a New Document
Below is an example of how to create a new user and save it to the MongoDB database:
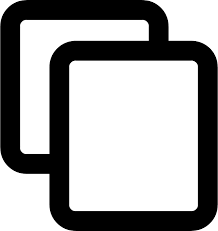
const newUser = new User({
name: 'John Doe',
email: 'johndoe@example.com',
age: 30
});
newUser.save()
.then(user => console.log('User Created:', user))
.catch(err => console.error('Error creating user:', err));
Read Operation
The Read operation is used to retrieve documents from the MongoDB database. Mongoose provides methods like find()
and findOne()
to fetch documents.
Example: Finding All Users
To find all users in the database, you can use the find()
method:
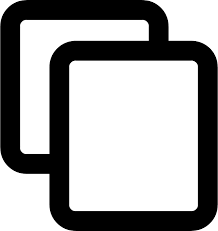
User.find()
.then(users => console.log('All Users:', users))
.catch(err => console.error('Error fetching users:', err));
Example: Finding a Single User
To find a single user by a specific condition (e.g., email), you can use findOne()
:
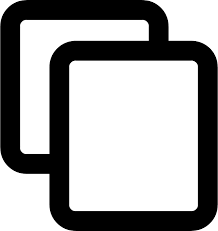
User.findOne({ email: 'johndoe@example.com' })
.then(user => console.log('User Found:', user))
.catch(err => console.error('Error finding user:', err));
Update Operation
The Update operation allows you to modify existing documents. Mongoose provides methods like updateOne()
, updateMany()
, and findByIdAndUpdate()
to update documents.
Example: Updating a User's Age
Below is an example of how to update a user's age:
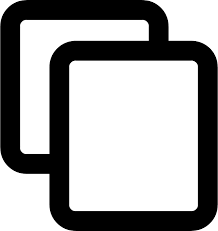
User.findOneAndUpdate({ email: 'johndoe@example.com' }, { age: 35 }, { new: true })
.then(user => console.log('Updated User:', user))
.catch(err => console.error('Error updating user:', err));
Delete Operation
The Delete operation is used to remove documents from the MongoDB collection. Mongoose provides methods like deleteOne()
and deleteMany()
to delete documents.
Example: Deleting a User
Below is an example of how to delete a user from the database:
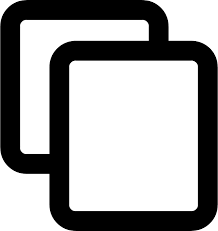
User.findOneAndDelete({ email: 'johndoe@example.com' })
.then(user => console.log('Deleted User:', user))
.catch(err => console.error('Error deleting user:', err));
CRUD Operations Summary
In summary, here are the basic methods for performing CRUD operations in Mongoose:
Operation | Method | Example |
---|---|---|
Create | Model.create() or new Model() and save() |
new User({ name: 'Alice' }).save() |
Read | Model.find() , Model.findOne() |
User.find() , User.findOne({ email: 'alice@example.com' }) |
Update | Model.updateOne() , Model.findByIdAndUpdate() |
User.updateOne({ email: 'alice@example.com' }, { age: 30 }) |
Delete | Model.deleteOne() , Model.findByIdAndDelete() |
User.deleteOne({ email: 'alice@example.com' }) |
Conclusion
CRUD operations in Mongoose are straightforward and provide a simple and efficient way to interact with the MongoDB database. By using these methods, you can easily create, read, update, and delete documents in your MongoDB collections. This is essential for building dynamic applications that require persistent data storage.
Introduction to REST API Concepts
REST (Representational State Transfer) is an architectural style for designing networked applications. It is based on a set of principles and constraints that allow different software systems to communicate with each other over the internet, typically using HTTP.
What is a REST API?
A REST API (Application Programming Interface) is a set of rules that allow different software programs to communicate with each other over the internet using HTTP methods such as GET, POST, PUT, DELETE, etc. It is designed to be stateless and follows a client-server architecture.
Key Principles of REST
- Stateless: Each request from a client to a server must contain all the information the server needs to fulfill the request. The server does not store any session information between requests.
- Client-Server Architecture: The client and server are independent entities that interact over a network. The client sends requests, and the server processes them and sends back responses.
- Uniform Interface: A uniform set of rules and conventions for communication, such as using standard HTTP methods (GET, POST, PUT, DELETE) for operations on resources.
- Resource-Based: REST APIs interact with resources, which are represented by URLs. Each resource is identified by a unique URL, and the operations are performed on these resources.
- Representation of Resources: Resources are typically represented in formats like JSON or XML. The representation of a resource is what the client receives in the response.
Common HTTP Methods Used in REST APIs
HTTP Method | Description |
---|---|
GET | Retrieves data from the server. It is a read-only operation and does not modify any resources. |
POST | Creates a new resource on the server. It is used to send data to the server to be processed. |
PUT | Updates an existing resource on the server. It replaces the current representation with the new one. |
DELETE | Deletes a resource from the server. |
Advantages of REST APIs
- Scalability: REST APIs are stateless, making them highly scalable and easy to manage in distributed systems.
- Flexibility: REST APIs allow communication between different types of clients (web browsers, mobile devices, etc.) and servers, making them flexible for various use cases.
- Performance: Because REST APIs use standard HTTP methods, they are lightweight and perform well under high loads.
- Simplicity: REST APIs use a simple, well-defined set of operations based on HTTP methods, making them easy to understand and implement.
Real-World Example of a REST API
Let’s say you are building an application where users can manage a list of books. The API for this application might look like this:
GET /books
- Retrieves a list of all books.GET /books/{id}
- Retrieves information about a specific book by its ID.POST /books
- Adds a new book to the list.PUT /books/{id}
- Updates a specific book's information by its ID.DELETE /books/{id}
- Deletes a specific book by its ID.
Conclusion
REST APIs are a powerful and widely used method for building and consuming web services. By following the principles of REST, developers can create APIs that are flexible, scalable, and easy to understand and integrate with. Understanding REST API concepts is an essential skill for any web developer working with modern technologies.
Designing and Implementing RESTful Endpoints
Designing RESTful endpoints involves creating URLs that represent resources and defining the actions that can be performed on them using HTTP methods. A well-designed REST API is intuitive, easy to use, and scalable.
Guidelines for Designing RESTful Endpoints
- Use Nouns for Resources: Each endpoint should represent a resource, and resources should be named using nouns. For example,
/users
to represent a collection of users, and/users/{id}
to represent an individual user. - Use HTTP Methods for Actions: Use standard HTTP methods to perform actions on resources. For example:
GET
- Retrieve a resource or a collection of resources.POST
- Create a new resource.PUT
- Update an existing resource.DELETE
- Remove a resource.
- Use Plural Nouns for Collections: When representing a collection of resources, use plural nouns. For example,
/books
for a collection of books, and/books/{id}
for a specific book. - Use Meaningful and Consistent Naming: Endpoints should be clear and self-explanatory. Use consistent naming conventions and avoid unnecessary abbreviations.
Example of RESTful Endpoints
Consider a simple application for managing books. Here is how the RESTful endpoints could be designed:
GET /books
- Retrieves a list of all books.GET /books/{id}
- Retrieves a specific book by its ID.POST /books
- Adds a new book to the collection.PUT /books/{id}
- Updates the details of a specific book.DELETE /books/{id}
- Removes a specific book from the collection.
Implementing RESTful Endpoints in Express
Now, let’s implement these endpoints in an Express.js application:
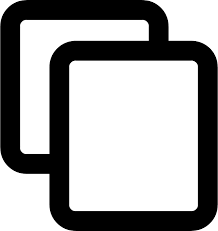
const express = require('express');
const app = express();
app.use(express.json());
let books = [{ id: 1, title: "1984", author: "George Orwell" }];
// Get all books
app.get('/books', (req, res) => {
res.json(books);
});
// Get a specific book by ID
app.get('/books/:id', (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send('Book not found');
res.json(book);
});
// Add a new book
app.post('/books', (req, res) => {
const book = {
id: books.length + 1,
title: req.body.title,
author: req.body.author
};
books.push(book);
res.status(201).json(book);
});
// Update a book by ID
app.put('/books/:id', (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send('Book not found');
book.title = req.body.title;
book.author = req.body.author;
res.json(book);
});
// Delete a book by ID
app.delete('/books/:id', (req, res) => {
const bookIndex = books.findIndex(b => b.id === parseInt(req.params.id));
if (bookIndex === -1) return res.status(404).send('Book not found');
books.splice(bookIndex, 1);
res.status(204).send();
});
app.listen(3000, () => console.log('Server is running on port 3000'));
Best Practices for Implementing RESTful Endpoints
- Use HTTP Status Codes: Make sure to return appropriate HTTP status codes for each response. For example,
200 OK
for successful requests,201 Created
for successful resource creation,404 Not Found
for missing resources, and400 Bad Request
for invalid requests. - Use Request Validation: Always validate incoming requests to ensure they are correctly formatted. This can be done using libraries like
Joi
orexpress-validator
. - Handle Errors Gracefully: Provide meaningful error messages and handle errors effectively to give users feedback about what went wrong.
- Use Pagination: When dealing with large collections of resources, implement pagination to avoid overwhelming the client with too much data. This can be done using query parameters like
?page=1&limit=10
. - Version Your API: It's important to version your API to ensure backward compatibility. This can be done by including the version number in the URL, e.g.,
/api/v1/books
.
Conclusion
Designing and implementing RESTful endpoints is a critical part of building web services. By following best practices and adhering to REST principles, developers can create APIs that are easy to use, maintain, and scale. With Express.js, building RESTful endpoints is straightforward and efficient.
Handling JSON Requests and Responses
In web development, JSON (JavaScript Object Notation) is the most commonly used format for transmitting data between the client and the server. Express.js makes it simple to handle JSON data in requests and responses, making it an essential tool for building RESTful APIs.
Handling JSON Requests
When a client sends data in the body of a request, it is typically formatted as JSON. To handle JSON requests in Express, you need to use the express.json()
middleware, which parses incoming requests with JSON payloads.
Enabling JSON Parsing in Express
To enable Express to parse JSON data, you need to use the express.json()
middleware. This middleware will automatically parse the request body and make it accessible in req.body
.
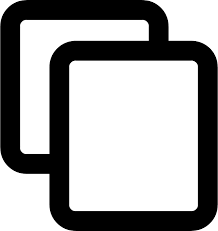
const express = require('express');
const app = express();
// Middleware to handle JSON requests
app.use(express.json());
// A POST route to handle JSON requests
app.post('/submit', (req, res) => {
const data = req.body;
console.log(data);
res.status(200).json({ message: 'Data received successfully', data });
});
app.listen(3000, () => console.log('Server is running on port 3000'));
In this example, we used the express.json()
middleware to handle incoming JSON requests. The req.body
object now contains the JSON data sent by the client.
Handling JSON Responses
In Express, you can send JSON responses using the res.json()
method. This method automatically sets the Content-Type
header to application/json
and converts the provided JavaScript object into a JSON string.
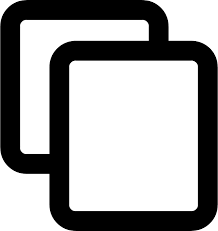
app.get('/data', (req, res) => {
const responseData = {
message: 'Here is your data',
items: ['item1', 'item2', 'item3']
};
res.json(responseData);
});
In this example, the /data
route sends a JSON response containing an object with a message
and items
array. The client will receive this response in JSON format.
Handling JSON Errors
If an error occurs while processing a JSON request, it's important to handle the error gracefully and return a meaningful JSON response. You can use try-catch blocks and send a JSON error response with the appropriate status code.
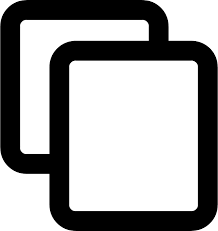
app.post('/submit', (req, res) => {
try {
const data = req.body;
if (!data.name) {
throw new Error('Name is required');
}
res.status(200).json({ message: 'Data received successfully', data });
} catch (error) {
res.status(400).json({ error: error.message });
}
});
In this example, if the client does not provide a name
field, the server will respond with a 400 Bad Request
status and a JSON error message.
Best Practices for Handling JSON Requests and Responses
- Use Middleware for Parsing: Always use
express.json()
middleware to handle JSON requests efficiently and avoid manual parsing. - Send Meaningful Responses: Ensure that the responses sent from the server are clear, descriptive, and consistent. This helps the client understand the status of the request.
- Validate Input: Always validate incoming JSON data to ensure it meets the expected format and structure. Use libraries like
Joi
orexpress-validator
for request validation. - Handle Errors Gracefully: Provide detailed error messages in JSON format, including error codes, descriptions, and possible solutions.
Conclusion
Handling JSON requests and responses is a fundamental aspect of building APIs with Express.js. By using the express.json()
middleware to handle incoming requests and res.json()
to send responses, developers can efficiently work with JSON data in their applications. Proper error handling and input validation ensure that APIs are robust and reliable.
Using Postman or cURL for API Testing
Testing your API is a crucial step in the development process to ensure that it behaves as expected. Two common tools for API testing are Postman and cURL. Both tools allow you to send HTTP requests to your server, inspect the responses, and verify that your API is working correctly.
Introduction to Postman
Postman is a popular tool for testing APIs. It provides a user-friendly interface to send different types of HTTP requests (GET, POST, PUT, DELETE) and view the responses. It also supports features like saving requests, setting headers, managing environments, and running automated tests.
Steps to Use Postman
- Install Postman: Download and install Postman from the official website.
- Create a New Request: Open Postman and click on "New Request" or use the "+" button to create a new tab.
- Set the Request Type: Choose the request method (GET, POST, PUT, DELETE) from the dropdown next to the URL field.
- Enter the API URL: Enter the URL of the API endpoint you want to test in the URL field.
- Set Request Headers (if needed): You can add headers such as
Content-Type
,Authorization
, etc. - Send the Request: Click on the "Send" button to send the request and view the response in the lower panel.
Example: Testing a GET Request
Let's assume you have a simple API endpoint that returns a list of users. Here's how you can use Postman to test this endpoint:
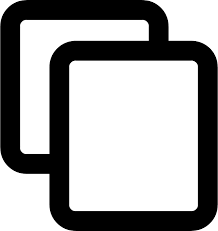
GET http://localhost:3000/users
Click "Send" in Postman to test the endpoint. The response should contain a list of users in JSON format.
Introduction to cURL
cURL is a command-line tool for transferring data with URLs. It's widely used for API testing and can be used in scripts or directly from the terminal. cURL supports all HTTP methods and can send both GET and POST requests with various headers and data formats.
Steps to Use cURL
- Install cURL: cURL comes pre-installed on most operating systems. If it's not installed, you can download it from the official website.
- Open the Terminal or Command Prompt: On Windows, open Command Prompt or PowerShell. On macOS or Linux, open the Terminal.
- Send a GET Request: To test a GET request, use the following syntax:
curl http://localhost:3000/users
- Send a POST Request: To send a POST request with data, use the following syntax:
curl -X POST http://localhost:3000/users -H "Content-Type: application/json" -d '{"name": "John", "email": "john@example.com"}'
Example: Testing a POST Request with cURL
Let's test the same API endpoint as before, but this time we will send a POST request with JSON data to create a new user:
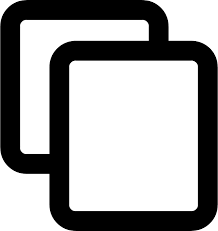
curl -X POST http://localhost:3000/users -H "Content-Type: application/json" -d '{"name": "John", "email": "john@example.com"}'
The above cURL command sends a POST request to the server with a JSON payload. If the request is successful, the server should respond with the newly created user data.
Postman vs cURL
Both Postman and cURL are excellent tools for testing APIs, but each has its strengths:
- Postman: Ideal for developers who prefer a graphical user interface. It's perfect for debugging and testing complex APIs with advanced features like authentication, environment variables, and automated tests.
- cURL: Great for quick testing directly from the terminal. It's lightweight, scriptable, and works well in CI/CD pipelines or automated workflows.
Best Practices for API Testing
- Always test your API with multiple request types (GET, POST, PUT, DELETE) to ensure all routes are working correctly.
- Check the response status codes to verify that your API is returning the correct HTTP status (200, 400, 404, etc.).
- Test both valid and invalid inputs to see how your API handles edge cases and errors.
- Verify the structure of the response to ensure it matches your API documentation or expected format.
Conclusion
API testing is an essential part of the development process, and tools like Postman and cURL make it easy to test your API endpoints. Whether you're using Postman for a more user-friendly interface or cURL for quick terminal-based testing, both tools are invaluable for ensuring your API works as expected and delivers reliable responses.
Pagination and Sorting in REST APIs
Pagination and sorting are essential techniques when working with large sets of data in REST APIs. Pagination helps to divide data into smaller, manageable chunks, while sorting allows for ordering the data based on specific fields (e.g., alphabetical order, date, etc.). Implementing both in your RESTful API enhances performance, user experience, and scalability.
Introduction to Pagination
Pagination is used to break down large datasets into smaller, more manageable pieces, called pages. Instead of returning all results in a single response, you send a subset of results. This is particularly useful when dealing with large collections of data, like user lists or product catalogs.
How Pagination Works
Pagination is typically implemented using query parameters to specify the page number and the number of results per page. Common query parameters used for pagination are:
- page: Specifies which page of results to retrieve (e.g., page 1, page 2, etc.).
- limit: Specifies how many results to return per page.
Example: Pagination in a GET Request
Let's assume you have an API endpoint that returns a list of users. To implement pagination, you can modify the endpoint to accept the page
and limit
query parameters:
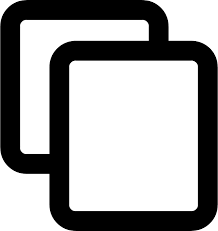
GET http://localhost:3000/users?page=2&limit=10
In this example, the API will return the second page of users with a maximum of 10 users per page. The response might include metadata such as the total number of users, the current page, and the total number of pages.
Handling Pagination in Express.js
In Express.js, you can implement pagination by retrieving the page
and limit
from the query string and applying them to your database query. Here's an example using MongoDB and Mongoose:
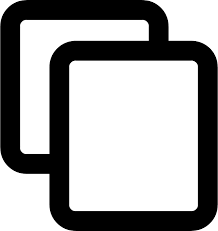
app.get('/users', async (req, res) => {
const page = parseInt(req.query.page) || 1;
const limit = parseInt(req.query.limit) || 10;
const skip = (page - 1) * limit;
const users = await User.find().skip(skip).limit(limit);
const totalUsers = await User.countDocuments();
res.json({
data: users,
total: totalUsers,
pages: Math.ceil(totalUsers / limit),
currentPage: page
});
});
In this example, we calculate the skip
value based on the current page and limit, then use Mongoose to fetch the appropriate data. We also return metadata in the response, including the total number of users, the current page, and the total number of pages.
Introduction to Sorting
Sorting is another important feature in REST APIs. It allows users to retrieve data in a specific order based on one or more fields. Sorting is typically done using query parameters that specify the field to sort by and the order (ascending or descending).
How Sorting Works
Sorting is commonly implemented using query parameters like:
- sort: Specifies the field by which to sort the results (e.g.,
name
,createdAt
, etc.). - order: Specifies the sorting order—use
asc
for ascending ordesc
for descending.
Example: Sorting in a GET Request
To sort users by their name in ascending order, you can modify your API request like this:
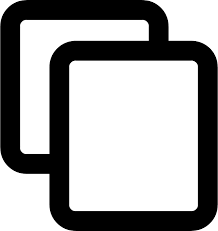
GET http://localhost:3000/users?sort=name&order=asc
In this case, the API will return a list of users sorted alphabetically by the name
field in ascending order.
Handling Sorting in Express.js
You can handle sorting in Express.js by retrieving the sort
and order
query parameters and applying them to your database query. Here's an example using MongoDB and Mongoose:
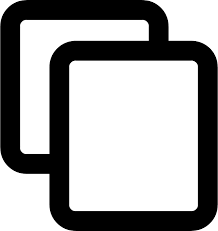
app.get('/users', async (req, res) => {
const sortField = req.query.sort || 'name'; // Default to sorting by name
const sortOrder = req.query.order === 'desc' ? -1 : 1; // Default to ascending order
const users = await User.find().sort({ [sortField]: sortOrder });
res.json(users);
});
In this example, the API sorts the users by the specified field and order. If no sort field is specified, it defaults to sorting by the name
field in ascending order.
Combining Pagination and Sorting
You can also combine pagination and sorting in a single API request. For example, to get the second page of users, sorted by name in descending order, you can use the following request:
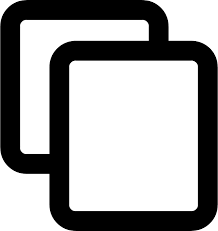
GET http://localhost:3000/users?page=2&limit=10&sort=name&order=desc
In this case, the API will return the second page of users, with 10 users per page, sorted by their name in descending order.
Best Practices for Pagination and Sorting
- Limit the number of results: Always set a reasonable limit for the number of records returned per page to avoid overloading the server.
- Use indexes in the database: Ensure that the fields you sort by are indexed in the database for better performance.
- Provide metadata: Return metadata such as the total number of results, current page, and total pages to help clients implement pagination smoothly.
- Validate inputs: Always validate and sanitize the pagination and sorting parameters to prevent errors or malicious inputs.
Conclusion
Pagination and sorting are essential features for optimizing API performance and improving user experience when working with large datasets. By implementing these techniques in your REST API, you can ensure that your API remains efficient, scalable, and responsive to user requests.
Introduction to Angular and Its Features
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. Developed and maintained by Google, Angular provides a comprehensive set of tools for developing modern web applications. It is known for its powerful features like two-way data binding, dependency injection, and a modular architecture.
What is Angular?
Angular is a TypeScript-based open-source framework for building dynamic, high-performance web applications. It is a complete package that includes tools, libraries, and best practices for building complex applications. Angular supports building single-page applications (SPAs) where the application loads a single HTML page and dynamically updates as the user interacts with the app.
Core Features of Angular
- Component-based architecture: Angular applications are built using components, which are reusable units of the interface. Each component has its own HTML, CSS, and logic.
- Two-way data binding: Angular provides two-way data binding, which ensures that changes in the model update the view, and changes in the view update the model automatically.
- Dependency Injection: Angular uses dependency injection to manage the relationships between components and services, promoting modularity and testability.
- Directives: Angular directives allow you to add behavior to DOM elements. Examples include
*ngIf
for conditional rendering and*ngFor
for looping through data. - Routing: Angular's router allows you to define routes and navigate between different views or pages within your application without reloading the page.
- RxJS and Observables: Angular uses RxJS (Reactive Extensions for JavaScript) for handling asynchronous events and data streams with Observables.
Angular Modules
Angular applications are modular, meaning they are divided into smaller, manageable parts called modules. A module is a container for different parts of the application, such as components, directives, services, and pipes. Each module can have its own functionality and can be imported into other modules to build the complete application.
Angular CLI
The Angular Command Line Interface (CLI) is a powerful tool that simplifies the development process. It helps in generating components, services, and other Angular entities, running tests, bundling the application for production, and more. The CLI makes setting up and maintaining Angular projects faster and easier.
Angular Lifecycle Hooks
Angular components have lifecycle hooks that allow developers to tap into key events during the component's life. These hooks include:
- ngOnInit: Called once the component is initialized and inputs are bound.
- ngOnChanges: Called when any input property of the component changes.
- ngOnDestroy: Called just before Angular destroys the component.
Creating an Angular Application
To create a new Angular application, you can use the Angular CLI. Here’s the command to create a new Angular app:
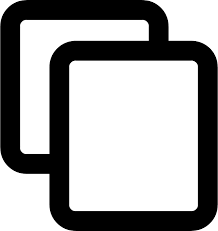
ng new my-angular-app
This will generate a new project with all the necessary configurations and files for starting an Angular application. Once the project is created, you can navigate into the project directory and start the development server:
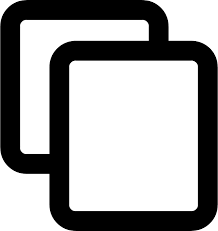
cd my-angular-app
ng serve
Your application will be accessible at http://localhost:4200
by default.
Angular CLI Commands
Here are some essential Angular CLI commands you will use during development:
- ng generate: Generates components, services, and other Angular entities. For example,
ng generate component my-component
. - ng serve: Starts the development server and serves your Angular application locally.
- ng build: Compiles your application for production and bundles it into static files for deployment.
Conclusion
Angular is a powerful and versatile framework for building dynamic web applications. With its component-based architecture, two-way data binding, and rich set of features, Angular is widely used for creating scalable and maintainable applications. Whether you're building a simple application or a complex enterprise-level system, Angular provides the tools and capabilities to get the job done effectively and efficiently.
Installing and Setting Up an Angular Project
Setting up an Angular project involves installing the necessary tools and dependencies, creating a new Angular project, and running the development server. The Angular CLI (Command Line Interface) is the main tool used for this purpose. The following steps will guide you through the process of installing and setting up an Angular project.
Step 1: Installing Node.js and npm
Before you can use Angular, you need to have Node.js and npm (Node Package Manager) installed on your system. These are the core tools that Angular uses for managing packages and dependencies.
To install Node.js and npm:
- Visit the official Node.js website: https://nodejs.org/.
- Download and install the latest stable version of Node.js for your operating system.
- Once installed, verify the installation by running the following commands in your terminal or command prompt:
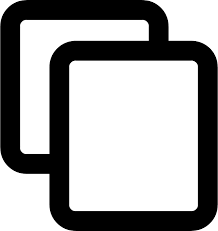
node -v
npm -v
If the installation is successful, these commands should display the versions of Node.js and npm installed on your system.
Step 2: Installing Angular CLI
Once Node.js and npm are installed, you can install Angular CLI globally on your machine. Angular CLI is a powerful command-line tool that helps you automate tasks such as creating projects, generating components, and running tests.
To install Angular CLI, run the following command in your terminal or command prompt:
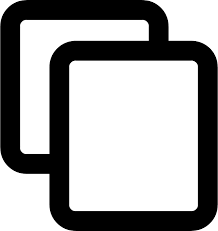
npm install -g @angular/cli
This command installs Angular CLI globally, which means you can use the ng
command anywhere on your system.
Step 3: Creating a New Angular Project
Now that Angular CLI is installed, you can create a new Angular project. To create a new project, run the following command:
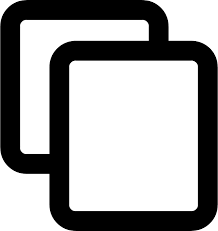
ng new my-angular-app
Replace my-angular-app
with your preferred project name. This command creates a new directory with the project name and initializes an Angular application in that directory.
During the creation process, you will be prompted to choose some configuration options, such as whether to include routing and which stylesheets to use (CSS, SCSS, etc.). You can choose the default options or customize them based on your preferences.
Step 4: Navigating to the Project Directory
Once the project is created, navigate to the project directory using the following command:
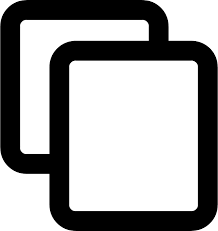
cd my-angular-app
Step 5: Running the Development Server
With the project set up and the project directory navigated to, you can now run the Angular development server. Use the following command to start the server:
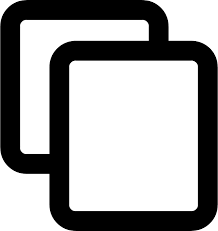
ng serve
This will compile your Angular project and serve it locally. You can open your browser and go to http://localhost:4200
to view your application.
Step 6: Opening the Project in a Code Editor
You can open your Angular project in a code editor like Visual Studio Code. To open your project in Visual Studio Code, run the following command in your project directory:
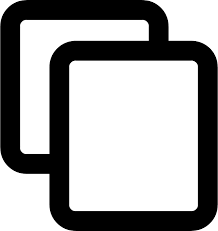
code .
This will open the entire project in Visual Studio Code, allowing you to modify the source code, add components, and configure other aspects of your application.
Conclusion
By following these steps, you have successfully installed Node.js, npm, and Angular CLI, created a new Angular project, and started the development server. Angular CLI simplifies the process of setting up and managing Angular applications, allowing you to focus on building your application rather than dealing with configuration details.
Understanding Angular Components, Templates, and Modules
Angular is built around the concept of components, which are the building blocks of an Angular application. Components are used to define the view (what the user sees) and the logic (what the application does) for a specific part of the UI. Templates are HTML structures that define the layout and structure of components, and modules help organize and manage the different parts of an Angular application. Let’s dive deeper into each concept.
Angular Components
A component in Angular is a TypeScript class that contains the logic for a specific part of the user interface. Each component is associated with a template, which defines the layout and view for that component.
Components are defined using the @Component
decorator. The decorator provides metadata about the component, such as the selector (which is used to insert the component into HTML), the template, and the styles.
Example of a simple Angular component:
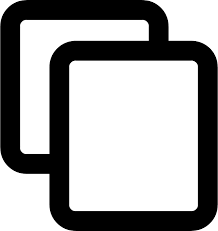
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: 'Hello, {{ name }}!
',
styles: ['h1 { color: green; }']
})
export class AppComponent {
name = 'Angular';
}
In this example:
selector
: The component can be used in the HTML code with the tag<app-root>
.template
: The view for the component is defined here. In this case, it displays a simple heading with a dynamic value.styles
: The styles for this component are defined inline.
Angular Templates
The template defines the layout and structure of the component's view. It uses HTML, along with Angular-specific syntax for dynamic content binding. You can bind properties, events, and apply directives like *ngIf
and *ngFor
to control the view.
Here’s an example of a component template using property binding and event binding:
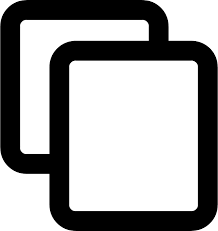
@Component({
selector: 'app-user',
template: `
{{ user.name }}
`
})
export class UserComponent {
user = { name: 'John Doe' };
showDetails() {
alert('Showing user details...');
}
}
In this example:
{{ user.name }}
: Property binding is used to display the user's name dynamically.(click)="showDetails()"
: Event binding is used to call theshowDetails
method when the button is clicked.
Angular Modules
Modules in Angular help organize an application into cohesive blocks of functionality. A module is a container for different parts of an application, such as components, services, directives, and pipes. The NgModule
decorator is used to define a module, and it contains metadata to configure the module, such as the components it declares and the modules it imports.
Example of an Angular module:
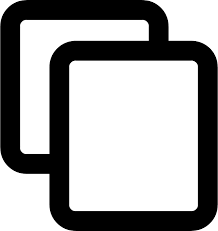
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
In this example:
declarations
: Lists all the components, directives, and pipes that belong to this module (e.g.,AppComponent
).imports
: Lists other modules that are needed by this module (e.g.,BrowserModule
).bootstrap
: Specifies the root component that Angular should bootstrap when the application starts (e.g.,AppComponent
).
Conclusion
Components, templates, and modules are the core building blocks of any Angular application. Components define the logic and structure of the application, templates provide the view, and modules help organize the application into cohesive units. Understanding how these three elements work together is fundamental to becoming proficient in Angular.
Two-Way Data Binding and Event Handling
Two-way data binding and event handling are essential concepts in Angular for creating interactive and dynamic applications. Two-way data binding allows seamless synchronization of data between the component class and the view, while event handling enables user interactions with the application. Let’s explore these concepts in detail.
Two-Way Data Binding
Two-way data binding in Angular allows changes in the model (component class) to automatically reflect in the view (template), and vice versa. This is especially useful for forms where the input values need to be synchronized with the component's properties.
In Angular, two-way data binding is achieved using the [(ngModel)]
directive. This directive creates a binding between the input element and the component property, allowing for both data flow directions.
Example of two-way data binding:
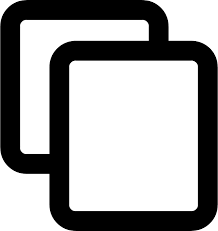
import { Component } from '@angular/core';
@Component({
selector: 'app-two-way-data-binding',
template: `
Hello, {{ name }}!
`
})
export class TwoWayDataBindingComponent {
name: string = '';
}
In this example:
[(ngModel)]="name"
: This creates a two-way data binding between the input field and thename
property in the component class.{{ name }}
: The value of thename
property is dynamically displayed in the paragraph, reflecting changes made in the input field.
Event Handling
Event handling in Angular allows you to capture user actions like clicks, keypresses, and other DOM events. You can bind an event to a method in your component by using the (event)
syntax, where event
is the name of the DOM event (e.g., click
, input
, etc.).
Example of event handling:
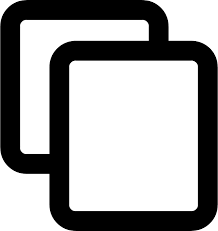
import { Component } from '@angular/core';
@Component({
selector: 'app-event-handling',
template: `
{{ message }}
`
})
export class EventHandlingComponent {
message: string = '';
greet() {
this.message = 'Hello, Angular!';
}
}
In this example:
(click)="greet()"
: This binds theclick
event of the button to thegreet()
method in the component class.{{ message }}
: Themessage
property is dynamically updated when the button is clicked, and the new value is displayed in the paragraph.
Combining Two-Way Data Binding and Event Handling
You can combine two-way data binding and event handling to create interactive forms and user interfaces. For example, you can update a model when an event is triggered, and vice versa. This allows for more complex interactions and dynamic content in the application.
Example of combining both concepts:
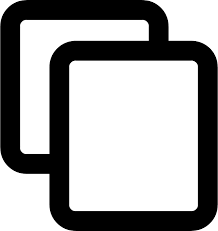
import { Component } from '@angular/core';
@Component({
selector: 'app-combined-example',
template: `
{{ message }}
`
})
export class CombinedExampleComponent {
userInput: string = '';
message: string = '';
updateMessage() {
this.message = `You typed: ${this.userInput}`;
}
}
In this example:
[(ngModel)]="userInput"
: Two-way binding synchronizes the input value with theuserInput
property in the component.(input)="updateMessage()"
: Theinput
event triggers theupdateMessage()
method, which updates themessage
property.{{ message }}
: The message is dynamically displayed based on the user's input.
Conclusion
Two-way data binding and event handling are powerful features in Angular that allow you to build dynamic, interactive applications. Two-way data binding simplifies synchronization of data between the view and the model, while event handling enables you to capture user interactions and update the application state accordingly. Understanding these concepts is key to mastering Angular.
Using Directives (*ngIf, *ngFor, etc.)
In Angular, directives are special markers attached to elements in the DOM that modify their behavior. Directives can be structural (like *ngIf
and *ngFor
) or attribute-based. Structural directives are used to add or remove elements from the DOM based on conditions or iterations.
1. *ngIf
- Conditional Rendering
The *ngIf
directive allows you to conditionally include or exclude an element from the DOM. If the expression evaluates to true
, the element will be included in the DOM; otherwise, it will be removed.
Example of using *ngIf
:
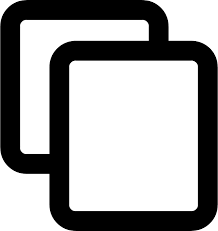
import { Component } from '@angular/core';
@Component({
selector: 'app-ngif-example',
template: `
This content is visible when isVisible is true
`
})
export class NgIfExampleComponent {
isVisible: boolean = true;
toggleVisibility() {
this.isVisible = !this.isVisible;
}
}
In this example:
*ngIf="isVisible"
: IfisVisible
istrue
, theelement will be displayed. Otherwise, it will be hidden.(click)="toggleVisibility()"
: When the button is clicked, thetoggleVisibility()
method changes theisVisible
value, thus toggling the visibility of the content.2.
*ngFor
- Looping Through DataThe
*ngFor
directive is used for iterating over a collection of items and displaying them dynamically. It is similar to afor
loop in other languages, but it works directly in the template.Example of using
*ngFor
:import { Component } from '@angular/core'; @Component({ selector: 'app-ngfor-example', template: `
- {{ item }}
In this example:
*ngFor="let item of items"
: This loops over theitems
array and renders a- for each element in the array.
- The
let item
syntax is used to define a local variable (item
) that holds each element of the array during each iteration.
3.
*ngFor
with IndexYou can also access the index of the current iteration when using
*ngFor
. This is useful when you need to display the index along with the item.Example:
import { Component } from '@angular/core'; @Component({ selector: 'app-ngfor-index-example', template: `
- {{ i + 1 }}. {{ item }}
In this example:
let i = index
: This syntax allows access to the index of the current element in the loop. The index is 0-based, so we add 1 to display a 1-based index.- The
i + 1
displays the item number along with the item name.
4.
*ngIf
with*ngFor
CombinedYou can also combine structural directives like
*ngIf
and*ngFor
to conditionally render lists or content based on certain conditions.Example:
import { Component } from '@angular/core'; @Component({ selector: 'app-ngif-ngfor-combined', template: `
- 0">
- {{ item }}
No items available.
` }) export class NgIfNgForCombinedComponent { items: string[] = ['Apple', 'Banana', 'Orange']; }In this example:
- The
*ngIf="items.length > 0"
conditionally renders the list of items only if the array has elements. If the array is empty, the messageNo items available.
is displayed.
Conclusion
Directives like
*ngIf
and*ngFor
are powerful tools for controlling the structure and behavior of your templates in Angular.*ngIf
is useful for conditionally rendering elements, while*ngFor
allows you to dynamically create elements based on an array or collection. By mastering these directives, you can create highly dynamic and responsive Angular applications.Creating and Using Angular Services
In Angular, services are used to provide reusable logic and functionality that can be shared across components. Services are typically used for tasks such as making HTTP requests, managing state, or handling business logic. Angular uses Dependency Injection (DI) to provide services to components or other services.
1. What is an Angular Service?
An Angular service is simply a class that encapsulates functionality or data that can be used across different components in an Angular application. Services are ideal for code that needs to be reused in multiple places, such as fetching data from an API or managing user authentication.
2. Creating an Angular Service
To create a service in Angular, you can use the Angular CLI to generate it. Here's the command:
ng generate service user
This command will create a service called
UserService
in thesrc/app
directory. The service file will look like this:import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class UserService { constructor() { } getUser() { return { id: 1, name: 'John Doe' }; } }
In this example:
@Injectable({ providedIn: 'root' })
: This decorator marks the class as injectable and specifies that it should be provided at the root level, making it available throughout the application.- The
getUser
method returns a dummy user object.
3. Using the Service in a Component
To use a service in a component, you need to inject the service into the component's constructor. Here's how you can use the
UserService
in a component:import { Component, OnInit } from '@angular/core'; import { UserService } from './user.service'; @Component({ selector: 'app-user', template: `
User Information
Id: {{ user.id }}
Name: {{ user.name }}
In this example:
constructor(private userService: UserService)
: The service is injected into the component via the constructor.ngOnInit()
: ThegetUser
method from theUserService
is called when the component is initialized, and the result is stored in theuser
property.
4. Service Methods and State Management
Services can also hold state that can be accessed and modified by multiple components. Here's an example of a service managing a list of users:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class UserService { private users = [ { id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Smith' } ]; constructor() { } getUsers() { return this.users; } addUser(user: { id: number, name: string }) { this.users.push(user); } }
In this example:
getUsers
: Returns the list of users stored in the service.addUser
: Adds a new user to the list of users.
5. Using the Updated Service in a Component
Now, we can use the updated
UserService
in a component to display and manage the list of users:import { Component, OnInit } from '@angular/core'; import { UserService } from './user.service'; @Component({ selector: 'app-user-list', template: `
User List
- {{ user.name }}
In this example:
this.users = this.userService.getUsers()
: The component gets the list of users from the service when it is initialized.(click)="addNewUser()"
: When the button is clicked, a new user is added to the list using theaddUser
method.
Conclusion
Services in Angular are a powerful way to share functionality and data across components. They help organize code that needs to be reused, such as API calls, state management, or business logic. By using Angular's Dependency Injection system, services are easy to manage and can be injected into any component that requires them.
Dependency Injection in Angular
Dependency Injection (DI) is a design pattern used to implement Inversion of Control (IoC), allowing for better code modularity and easier testing. In Angular, DI is used to provide services or other objects to components and other services. It allows components to focus on their primary responsibilities while the DI framework takes care of the creation and management of dependent services or objects.
1. What is Dependency Injection?
Dependency Injection is a pattern in which an object's dependencies are provided to it rather than the object creating the dependencies itself. Angular uses DI to manage the services, making them available to the components and other services that require them.
2. How Dependency Injection Works in Angular
In Angular, DI is used to inject services into components, directives, pipes, and other services. Angular has a hierarchical DI system which allows you to define the scope of services at various levels, such as at the root level or within a specific module or component.
3. Using Dependency Injection in Angular
To use DI in Angular, you need to inject services into your components or services using the constructor. Here's how to do that:
import { Component, OnInit } from '@angular/core'; import { UserService } from './user.service'; @Component({ selector: 'app-user', template: `
User Information
Id: {{ user.id }}
Name: {{ user.name }}
In this example:
constructor(private userService: UserService)
: TheUserService
is injected into the component via the constructor. Angular's DI framework automatically provides an instance of the service.ngOnInit()
: ThegetUser()
method from the injectedUserService
is called to fetch the user data.
4. Injectable Services
To use DI with services, the service must be marked with the
@Injectable
decorator. This decorator tells Angular that the service can be injected into other components or services. TheprovidedIn
property determines where the service is provided in the DI tree. Here's an example of an injectable service:import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' // This ensures the service is available across the entire application }) export class UserService { constructor() { } getUser() { return { id: 1, name: 'John Doe' }; } }
In this example:
@Injectable({ providedIn: 'root' })
: This decorator is used to make the service available application-wide. The service will be provided at the root level, ensuring that only one instance of the service exists throughout the application.getUser()
: This method returns a dummy user object, which can be injected and used in components.
5. Hierarchical Injectors
Angular provides a hierarchical injector system, which means that services can be scoped to different levels of the application. Services can be provided at the root level, module level, or component level.
Providing Services at Different Levels
- Root Level: The service is available globally throughout the application. This is the most common usage and is defined with
providedIn: 'root'
. - Module Level: The service is scoped to a specific Angular module. Use this approach if you only need the service in a specific module.
- Component Level: The service is scoped to a specific component. This is useful when you need different instances of the service for different components.
6. Example of Scoped Services
Here's how to provide a service at the component level:
import { Component, OnInit } from '@angular/core'; import { UserService } from './user.service'; @Component({ selector: 'app-user', template: `
User Information
Id: {{ user.id }}
Name: {{ user.name }}
In this example:
providers: [UserService]
: TheUserService
is provided at the component level. This means that each instance of theUserComponent
will have its own instance of theUserService
.
7. Benefits of Dependency Injection
- Separation of Concerns: DI allows components to focus on their core functionality while delegating the creation of dependencies to the DI system.
- Improved Testability: DI makes it easier to test components and services by allowing you to inject mock or fake dependencies.
- Maintainability: DI leads to cleaner and more maintainable code by reducing tight coupling between components and services.
Conclusion
Dependency Injection is a key feature of Angular that makes it easier to manage and inject services into components and other services. It improves code modularity, maintainability, and testability, making Angular applications more scalable and easier to manage.
Using HTTP Client for API Requests
Angular provides a built-in service called the HttpClient that allows you to make HTTP requests to remote servers or APIs. It is used for retrieving and sending data to the server in a RESTful way. The HttpClient module provides an easy-to-use API to interact with REST APIs and handle responses, making it a crucial part of working with APIs in Angular.
1. Importing the HttpClientModule
To use the HttpClient service, first, you need to import the
HttpClientModule
in your Angular application's main module. This module enables Angular's HttpClient service throughout your app.import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { HttpClientModule } from '@angular/common/http'; import { AppComponent } from './app.component'; @NgModule({ declarations: [AppComponent], imports: [BrowserModule, HttpClientModule], // Import HttpClientModule here providers: [], bootstrap: [AppComponent] }) export class AppModule { }
In this example:
HttpClientModule
is imported in theAppModule
so that you can use theHttpClient
service in any component throughout your application.
2. Injecting HttpClient into a Component
To make HTTP requests, you need to inject the
HttpClient
service into the component or service where you want to use it. Here's how to do that:import { Component, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-data', template: `
Data from API
- {{ item.name }}
('https://api.example.com/data') .subscribe(response => { this.data = response; }); } } In this example:
HttpClient
is injected into the component via the constructor.- The
get()
method is used to make a GET request to an API endpoint. The response is subscribed to, and the data is stored in thedata
property.
3. Making Different Types of HTTP Requests
In addition to GET requests, the
HttpClient
service supports other HTTP methods such as POST, PUT, DELETE, etc. Here are examples of how to use them:// POST request example this.http.post('https://api.example.com/data', { name: 'New Item' }) .subscribe(response => { console.log('Item created:', response); }); // PUT request example this.http.put('https://api.example.com/data/1', { name: 'Updated Item' }) .subscribe(response => { console.log('Item updated:', response); }); // DELETE request example this.http.delete('https://api.example.com/data/1') .subscribe(response => { console.log('Item deleted:', response); });
In these examples:
- The
post()
method sends data to the server to create a new resource. - The
put()
method updates an existing resource on the server. - The
delete()
method removes a resource from the server.
4. Handling API Responses
Angular’s HttpClient automatically parses the response data into a JavaScript object, which you can work with directly. However, you can also handle the response in different formats (e.g., JSON, text, or blobs) using the
responseType
option.// Handling different response types this.http.get('https://api.example.com/data', { responseType: 'text' }) .subscribe(response => { console.log('Response as text:', response); });
In this example:
responseType: 'text'
specifies that the response should be treated as plain text, rather than JSON (the default format).
5. Handling Errors
When making HTTP requests, it’s important to handle errors properly. You can use the
catchError
operator from RxJS to handle errors and provide a fallback response.import { catchError } from 'rxjs/operators'; import { throwError } from 'rxjs'; this.http.get('https://api.example.com/data') .pipe( catchError(error => { console.error('Error occurred:', error); return throwError('Something went wrong'); }) ) .subscribe(response => { console.log('Data:', response); });
In this example:
catchError
is used to catch any errors that occur during the HTTP request.throwError
is used to throw a custom error message after logging the original error.
6. Conclusion
Using the HttpClient service in Angular allows you to make HTTP requests to interact with external APIs or server-side services. The service provides an easy-to-use API to make requests, handle responses, and manage errors, making it an essential part of working with APIs in Angular applications.
Handling Promises and Observables
In Angular, both Promises and Observables are used to handle asynchronous operations such as HTTP requests, user input, and timers. While they share similarities, they differ in how they are used and the features they offer. Let's dive into both of them and understand how they work in Angular.
1. Introduction to Promises
A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises are part of JavaScript and are widely used for handling asynchronous tasks.
Promise Example
Here is an example of how you can use a Promise to handle an asynchronous operation such as an HTTP request:
const promise = new Promise((resolve, reject) => { setTimeout(() => { resolve('Data fetched successfully!'); }, 2000); }); promise.then(response => { console.log(response); }).catch(error => { console.log('Error:', error); });
In this example:
- A new Promise is created that resolves with a success message after a 2-second delay.
- The
then()
method is used to handle the resolved value, whilecatch()
handles any errors.
2. Introduction to Observables
Observables are a key part of the reactive programming paradigm and are provided by RxJS in Angular. Observables represent a stream of values over time and can emit multiple values, unlike Promises, which only emit a single value.
Observable Example
Here is an example of how you can use an Observable to handle a stream of asynchronous data:
import { Observable } from 'rxjs'; const observable = new Observable(subscriber => { setTimeout(() => { subscriber.next('Data fetched successfully!'); subscriber.complete(); }, 2000); }); observable.subscribe({ next(response) { console.log(response); }, complete() { console.log('Observable completed!'); } });
In this example:
- An Observable is created using the
Observable
constructor, which emits values using thenext()
method. - The
subscribe()
method is used to listen for emitted values and handle them in thenext()
method. Thecomplete()
method is called once the Observable has finished emitting values.
3. Difference Between Promises and Observables
While both Promises and Observables handle asynchronous operations, there are key differences between them:
Feature Promise Observable Emission Single value (resolved or rejected) Multiple values over time Lazy or Eager Eager (executes immediately) Lazy (executes when subscribed) Cancellation Cannot be cancelled once started Can be cancelled using unsubscribe()
Operators No operators like RxJS Support for many operators like map()
,filter()
,mergeMap()
, etc.4. Using Promises in Angular with HttpClient
Angular's
HttpClient
service returns Observables by default, but you can also convert them into Promises if needed. Here's how to use Promises with HTTP requests:import { HttpClient } from '@angular/common/http'; export class DataComponent { constructor(private http: HttpClient) {} fetchData() { this.http.get('https://api.example.com/data') .toPromise() .then(response => { console.log(response); }) .catch(error => { console.log('Error:', error); }); } }
In this example:
- The
toPromise()
method is used to convert the Observable returned byhttp.get()
into a Promise. - The
then()
method is used to handle the resolved value, andcatch()
handles errors.
5. Using Observables in Angular with HttpClient
In Angular, the more common approach is to use Observables with the
HttpClient
service. Here is how to handle HTTP requests using Observables:import { HttpClient } from '@angular/common/http'; export class DataComponent { data: any[]; constructor(private http: HttpClient) {} fetchData() { this.http.get
('https://api.example.com/data') .subscribe(response => { this.data = response; console.log('Data fetched:', this.data); }); } } In this example:
- The
http.get()
method is used directly with the Observable pattern. - The
subscribe()
method is used to listen for the emitted data, and handle it in the success callback function.
6. Conclusion
Promises and Observables are both useful tools for handling asynchronous operations in JavaScript and Angular. While Promises are suited for single-value asynchronous operations, Observables are more powerful and flexible, allowing for multiple emissions, cancellation, and various operators for transformation and combination of values. Angular's HttpClient service by default returns Observables, which is the recommended way to handle HTTP requests and responses in Angular applications.
Setting Up Angular Router
Angular Router is a powerful tool that helps navigate between views or pages in a single-page application (SPA). It allows users to move between different views without reloading the entire page. Let's explore how to set up Angular Router in your Angular application.
1. Installing Angular Router
Angular Router is included by default when you create a new Angular application. If for some reason it’s not included in your project, you can install it using the following command:
ng add @angular/router
This command installs the Angular Router and configures your application to use routing.
2. Configuring Routes
After installing the Angular Router, you need to configure your application’s routes. A route is a mapping between a URL path and a component. To configure routes, follow these steps:
- Open the app-routing.module.ts file. If it doesn’t exist, create a new one.
- Import the necessary Angular Router modules, such as
RouterModule
andRoutes
. - Define the routes as an array of route objects, specifying the path and the corresponding component for each route.
- Use
RouterModule.forRoot()
to register the routes in the application module.
Example of Route Configuration
Here’s how you can configure the routes in the app-routing.module.ts file:
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; import { ContactComponent } from './contact/contact.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, { path: 'contact', component: ContactComponent } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
In this example:
- We’ve defined three routes: the home route (
''
), the about route ('about'
), and the contact route ('contact'
). - Each route points to a corresponding component (
HomeComponent
,AboutComponent
, andContactComponent
). - We import and configure the routes in the
AppRoutingModule
, and useRouterModule.forRoot(routes)
to make the routes available throughout the application.
3. Adding Router Links in the Template
Once the routes are configured, you can use the
routerLink
directive to create navigation links in your application’s templates. This allows users to navigate between different views by clicking on links:In this example:
- The
routerLink
directive is used to bind a link to a route. For example,routerLink="/about"
will navigate to the About page.
4. Using
<router-outlet>
for Displaying ViewsTo display the routed components, you need to add a
<router-outlet>
element in your main template file (usuallyapp.component.html
). This acts as a placeholder for the routed component views.The
<router-outlet>
will dynamically load and display the component associated with the active route based on the URL.5. Handling Wildcard Routes
Angular Router also supports wildcard routes, which allow you to catch all invalid URLs or handle unknown routes:
const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, { path: 'contact', component: ContactComponent }, { path: '**', component: PageNotFoundComponent } // Wildcard route ];
The wildcard route
**
will match any route that does not correspond to an existing path, such as invalid URLs. In this case, it will redirect users to thePageNotFoundComponent
.6. Conclusion
By setting up Angular Router in your application, you can create a navigation system for users to interact with different views. Configuring routes, using
routerLink
, and adding a<router-outlet>
placeholder are key steps to creating a dynamic single-page application. Additionally, wildcard routes help handle invalid URLs, ensuring a smooth user experience.Defining and Navigating Between Routes
In Angular, defining routes and enabling navigation between different components is a key feature of building single-page applications (SPAs). By using Angular Router, you can define routes and allow users to navigate between views seamlessly. In this section, we will explore how to define routes and navigate between them.
1. Defining Routes in Angular
Routes are defined as a set of path-component pairs. Each route has a URL path and a corresponding component. The route configuration is typically done in the
app-routing.module.ts
file.Example of Defining Routes:
In this example, we define three basic routes: Home, About, and Contact.
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; import { ContactComponent } from './contact/contact.component'; const routes: Routes = [ { path: '', component: HomeComponent }, // Home route { path: 'about', component: AboutComponent }, // About route { path: 'contact', component: ContactComponent } // Contact route ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
In this example:
- The
path
is the URL you want to match, and thecomponent
is the Angular component to display for that route. - We use
RouterModule.forRoot()
to configure the routes at the root level of the application.
2. Navigating Between Routes
Once routes are defined, you can navigate between them using the
routerLink
directive in the template. TherouterLink
directive is used to bind a link to a route. When users click the link, the application will navigate to the associated route.Example of Navigating Between Routes:
Here’s how to create navigation links to the Home, About, and Contact pages in your template:
In this example:
- Each link uses the
routerLink
directive to navigate to the corresponding route when clicked. For example,routerLink="/about"
will navigate to the About component. - The
/
in the Home link corresponds to the root route, which is mapped to theHomeComponent
.
3. Navigating Programmatically
Besides using links, you can also navigate programmatically within your component’s TypeScript code using the Angular Router’s
navigate()
method. This method allows you to navigate to a route based on some logic in your component.Example of Programmatic Navigation:
Here’s an example of navigating to a different route when a button is clicked:
import { Component } from '@angular/core'; import { Router } from '@angular/router'; @Component({ selector: 'app-root', template: `` }) export class AppComponent { constructor(private router: Router) {} navigateToAbout() { this.router.navigate(['/about']); } }
In this example:
- We inject the
Router
service into the component’s constructor. - We call the
navigate()
method on theRouter
to programmatically navigate to the About component when the button is clicked.
4. Wildcard Routes for Catching Invalid Routes
You can also define a wildcard route that will catch any invalid or unmatched routes. This is useful for redirecting users to a “Page Not Found” component.
Example of a Wildcard Route:
const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, { path: 'contact', component: ContactComponent }, { path: '**', component: PageNotFoundComponent } // Wildcard route ];
In this example:
- The
**
wildcard route will catch any route that does not match the defined paths and will navigate the user to thePageNotFoundComponent
.
5. Conclusion
Defining and navigating between routes is a fundamental part of building Angular applications. Using Angular Router, you can define routes, create navigation links with
routerLink
, and navigate programmatically with thenavigate()
method. Additionally, wildcard routes help manage invalid URLs and provide a smooth user experience.Route Parameters and Query Strings
In Angular, route parameters and query strings are essential for passing dynamic values between routes, enhancing the user experience by enabling more personalized content or behavior. In this section, we will explore how to use route parameters and query strings in Angular applications.
1. Route Parameters
Route parameters allow you to pass dynamic values in the URL. These parameters are typically used for things like user profiles, product IDs, or any other item that requires specific information in the URL.
Defining Route Parameters in Routes:
To define route parameters, you use a colon (:) followed by the parameter name in the route path.
const routes: Routes = [ { path: 'user/:id', component: UserComponent }, // Route with parameter ];
In this example, the route
/user/:id
defines a route parameterid
that can be used to fetch a specific user.Accessing Route Parameters in the Component:
To access the route parameters in the component, you use the
ActivatedRoute
service, which allows you to retrieve parameters from the URL.import { Component, OnInit } from '@angular/core'; import { ActivatedRoute } from '@angular/router'; @Component({ selector: 'app-user', templateUrl: './user.component.html', styleUrls: ['./user.component.css'] }) export class UserComponent implements OnInit { userId: string; constructor(private route: ActivatedRoute) {} ngOnInit(): void { // Access the route parameter 'id' this.userId = this.route.snapshot.paramMap.get('id'); } }
In this example:
- We inject the
ActivatedRoute
service into the component. - We use
this.route.snapshot.paramMap.get('id')
to retrieve the value of theid
route parameter.
2. Query Strings
Query strings are used to pass additional data in the URL, typically in the form of key-value pairs. They can be used alongside route parameters or independently.
Defining Query Strings in the URL:
Query strings are added to the URL after the
?
symbol and are followed by key-value pairs separated by the&
symbol.const routes: Routes = [ { path: 'products', component: ProductsComponent }, ];
An example of a URL with query strings:
/products?category=electronics&priceRange=100-500
Accessing Query Strings in the Component:
To access query strings, we use the
ActivatedRoute
service'squeryParamMap
method.import { Component, OnInit } from '@angular/core'; import { ActivatedRoute } from '@angular/router'; @Component({ selector: 'app-products', templateUrl: './products.component.html', styleUrls: ['./products.component.css'] }) export class ProductsComponent implements OnInit { category: string; priceRange: string; constructor(private route: ActivatedRoute) {} ngOnInit(): void { // Access the query parameters 'category' and 'priceRange' this.category = this.route.snapshot.queryParamMap.get('category'); this.priceRange = this.route.snapshot.queryParamMap.get('priceRange'); } }
In this example:
- We use
this.route.snapshot.queryParamMap.get('category')
to access thecategory
query string. - We use
this.route.snapshot.queryParamMap.get('priceRange')
to access thepriceRange
query string.
3. Combining Route Parameters and Query Strings
In Angular, you can use route parameters and query strings together in a single route. This allows you to pass both dynamic data through the URL path and additional data through the query string.
Example of Using Both Route Parameters and Query Strings:
Here’s an example where both route parameters and query strings are used in the same route:
const routes: Routes = [ { path: 'user/:id/details', component: UserDetailsComponent }, ];
An example of a URL with both route parameters and query strings:
/user/123/details?showDetails=true
In the above URL:
:id
is a route parameter that identifies the user.showDetails=true
is a query string that controls additional logic, such as whether to show the detailed view.
4. Conclusion
Route parameters and query strings are powerful tools in Angular for passing dynamic data between routes. Route parameters are used for identifying resources, while query strings are useful for passing additional, optional data. By combining both, you can create flexible and dynamic Angular applications.
Route Guards for Authentication and Authorization
Route guards in Angular are used to control access to certain routes based on conditions like user authentication or authorization. This ensures that only authorized users can access specific parts of the application.
1. Introduction to Route Guards
Route guards are services that implement one or more lifecycle hooks to check conditions before a route is activated, deactivated, or can be loaded. There are several types of route guards in Angular:
- CanActivate: Prevents navigation to a route if the user is not authorized.
- CanActivateChild: Prevents navigation to child routes if the user is not authorized.
- CanDeactivate: Confirms whether the user can leave the current route.
- CanLoad: Prevents the lazy loading of a module if the user is not authorized.
2. Creating a Route Guard
To create a route guard, we use Angular's CLI to generate a service that implements one or more of the lifecycle hooks. Here we will create a simple guard for authentication using the
CanActivate
interface.Generating the Route Guard:
Use the Angular CLI command to generate a guard:
ng generate guard auth/auth
Implementing the Guard:
In the generated guard service, we implement the
CanActivate
interface to check if the user is authenticated.import { Injectable } from '@angular/core'; import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, Router } from '@angular/router'; import { AuthService } from './auth.service'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private authService: AuthService, private router: Router) {} canActivate( next: ActivatedRouteSnapshot, state: RouterStateSnapshot): boolean { if (this.authService.isAuthenticated()) { return true; } else { this.router.navigate(['/login']); return false; } } }
In this example:
- We inject the
AuthService
to check if the user is authenticated. - If the user is authenticated, the route is activated, otherwise, the user is redirected to the login page.
3. Protecting Routes with the Guard
Once the guard is created, we can use it to protect routes by adding the
canActivate
property to the route configuration.const routes: Routes = [ { path: 'dashboard', component: DashboardComponent, canActivate: [AuthGuard] }, { path: 'login', component: LoginComponent }, ];
In this example:
- The
AuthGuard
is applied to the/dashboard
route. - If the user is not authenticated, they will be redirected to the
/login
route.
4. Authorization with Route Guards
In addition to authentication, route guards can also handle authorization. For example, a user may be authenticated, but they may not have the necessary role or permission to access a route. Here’s how we can add an authorization check to the guard:
Modifying the Guard to Check User Role:
canActivate( next: ActivatedRouteSnapshot, state: RouterStateSnapshot): boolean { if (this.authService.isAuthenticated() && this.authService.hasRole('admin')) { return true; } else { this.router.navigate(['/access-denied']); return false; } }
In this example:
- The user is required to be authenticated and have the 'admin' role to access the route.
- If the user doesn’t meet these conditions, they are redirected to the
/access-denied
route.
5. Conclusion
Route guards are a powerful feature in Angular that allow you to control access to routes based on authentication and authorization. They provide a way to protect parts of your application from unauthorized access, ensuring that only users who meet the required conditions can navigate to protected routes.
Setting Up JWT (JSON Web Tokens) in Node.js
JSON Web Tokens (JWT) are used for securely transmitting information between parties as a JSON object. In Node.js, JWTs are commonly used for authentication, enabling stateless authentication mechanisms where a server does not need to store session information.
1. What is JWT?
A JWT is a compact, URL-safe token that represents claims to be transferred between two parties. It consists of three parts:
- Header: Contains information about how the JWT is signed, typically using an algorithm like HMAC SHA256 or RSA.
- Payload: Contains the claims (data) being transmitted, such as the user’s ID, roles, or expiration information.
- Signature: Ensures the integrity of the token and verifies that the sender is legitimate.
2. Installing Necessary Packages
To set up JWT in a Node.js application, you need to install two packages:
jsonwebtoken
anddotenv
(for managing environment variables).npm install jsonwebtoken dotenv
3. Creating a JWT Token
To create a JWT token, you need to sign it using a secret key. Here’s an example of how you can create a JWT token in your Node.js application.
const jwt = require('jsonwebtoken'); // Secret key for signing JWT const secretKey = process.env.JWT_SECRET; // Function to generate JWT token function generateToken(user) { const payload = { userId: user.id, username: user.username, }; const options = { expiresIn: '1h' }; // Token expires in 1 hour return jwt.sign(payload, secretKey, options); }
In this example:
- The payload contains the user's ID and username.
- The token is signed using a secret key (
JWT_SECRET
) and set to expire in 1 hour.
4. Verifying the JWT Token
To verify the JWT token on subsequent requests, you need to decode and verify the signature of the token using the same secret key.
function verifyToken(token) { try { const decoded = jwt.verify(token, secretKey); return decoded; // Returns the decoded payload if the token is valid } catch (error) { throw new Error('Invalid Token'); } }
In this example:
- The
verifyToken
function attempts to decode the token using thejwt.verify
method. - If the token is valid, it returns the decoded payload; otherwise, it throws an error.
5. Using JWT in Express.js Routes
To secure routes in your application, you can create a middleware that checks for the JWT token in the request header and verifies it before allowing access to protected routes.
const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); // Secret key for signing JWT const secretKey = process.env.JWT_SECRET; // Middleware to check JWT function authenticateToken(req, res, next) { const token = req.headers['authorization']?.split(' ')[1]; // Bearer token if (!token) { return res.status(403).send('Access Denied'); } jwt.verify(token, secretKey, (err, user) => { if (err) { return res.status(403).send('Invalid Token'); } req.user = user; // Attach the user info to the request next(); }); } // Protected route app.get('/protected', authenticateToken, (req, res) => { res.send('This is a protected route'); });
In this example:
- The
authenticateToken
middleware extracts the token from theAuthorization
header. - If the token is valid, the user information is added to the request object, and the request proceeds to the next middleware or route handler.
- If the token is missing or invalid, the server responds with a 403 status code (Forbidden).
6. Storing the JWT Token in Frontend
Once the JWT token is generated on the backend, it is typically sent to the client (frontend) and stored in
localStorage
orsessionStorage
for subsequent requests.localStorage.setItem('token', 'YOUR_JWT_TOKEN');
7. Conclusion
Setting up JWT in Node.js is a simple and effective way to secure your application by enabling stateless authentication. With JWTs, you can authenticate users, protect routes, and ensure secure communication between the client and server.
Implementing Login and Registration APIs
Login and registration APIs are essential for user authentication. In a Node.js application, you can implement these APIs to allow users to register an account and log in using credentials, often with JWT for authentication.
1. Setting Up the Environment
To implement login and registration APIs, you'll need a few essential packages:
- express for setting up the server and handling HTTP requests.
- bcryptjs for hashing passwords before storing them in the database.
- jsonwebtoken for generating JWT tokens to authenticate users.
- mongoose for database interaction (assuming MongoDB is used).
npm install express bcryptjs jsonwebtoken mongoose
2. Creating the User Model
We will create a User model using Mongoose to store user details such as username and password.
const mongoose = require('mongoose'); // User Schema const userSchema = new mongoose.Schema({ username: { type: String, required: true, unique: true }, password: { type: String, required: true }, }); // Create and export User model const User = mongoose.model('User', userSchema); module.exports = User;
3. Registration API
The registration API will allow a new user to create an account. The password will be hashed using
bcryptjs
before saving it to the database.const express = require('express'); const bcrypt = require('bcryptjs'); const User = require('./models/User'); const app = express(); app.use(express.json()); // Middleware to parse JSON // Registration API app.post('/register', async (req, res) => { const { username, password } = req.body; try { // Check if user already exists const existingUser = await User.findOne({ username }); if (existingUser) { return res.status(400).json({ message: 'User already exists' }); } // Hash password const hashedPassword = await bcrypt.hash(password, 10); // Create new user const newUser = new User({ username, password: hashedPassword }); await newUser.save(); res.status(201).json({ message: 'User registered successfully' }); } catch (error) { res.status(500).json({ message: 'Server error' }); } });
In this example:
- The user’s password is hashed using
bcrypt.hash
before storing it in the database. - If a user with the same username already exists, the server returns a 400 status code with an error message.
- If the registration is successful, a 201 status code is returned with a success message.
4. Login API
The login API will authenticate a user by verifying their credentials. If the credentials are valid, a JWT token will be generated and returned to the client for further authentication.
const jwt = require('jsonwebtoken'); // Login API app.post('/login', async (req, res) => { const { username, password } = req.body; try { // Find user by username const user = await User.findOne({ username }); if (!user) { return res.status(400).json({ message: 'Invalid credentials' }); } // Check if password matches const isPasswordValid = await bcrypt.compare(password, user.password); if (!isPasswordValid) { return res.status(400).json({ message: 'Invalid credentials' }); } // Generate JWT token const token = jwt.sign({ userId: user._id }, process.env.JWT_SECRET, { expiresIn: '1h' }); res.json({ token }); } catch (error) { res.status(500).json({ message: 'Server error' }); } });
In this example:
- The server first checks if the user exists in the database by their username.
- The password is compared with the hashed password stored in the database using
bcrypt.compare
. - If the credentials are valid, a JWT token is generated using
jwt.sign
and returned to the client.
5. Conclusion
With the registration and login APIs set up, you can now allow users to register an account and authenticate using JWT tokens. These tokens can be used for secure access to protected routes in your application. This setup is a key part of most modern applications, providing a secure and efficient way to handle user authentication.
Protecting Routes with Middleware
In a Node.js application, protecting routes is an essential part of ensuring that only authenticated users can access specific resources. This is commonly done by using middleware that checks if a user has a valid authentication token (usually a JWT). If the token is valid, the user is granted access to the requested route. If not, access is denied, and the user is redirected to a login page or shown an error message.
1. Setting Up JWT Authentication Middleware
First, we'll create a middleware function that will verify JWT tokens in requests and ensure that only authenticated users can access protected routes.
const jwt = require('jsonwebtoken'); // Middleware to protect routes const authenticate = (req, res, next) => { const token = req.header('Authorization'); if (!token) { return res.status(401).json({ message: 'Access denied. No token provided.' }); } try { // Verify token const decoded = jwt.verify(token, process.env.JWT_SECRET); req.user = decoded; // Attach user information to the request object next(); // Proceed to the next middleware or route handler } catch (error) { res.status(400).json({ message: 'Invalid token.' }); } }; module.exports = authenticate;
In this middleware:
- The token is expected to be sent in the
Authorization
header of the request. - If no token is provided, the user will receive a 401 Unauthorized error.
- If the token is invalid or expired, a 400 Bad Request error will be returned.
- If the token is valid, the user's information (usually the user ID) is decoded from the token and attached to the request object, allowing the route handler to access it.
2. Protecting Routes Using the Middleware
Once we have the authentication middleware in place, we can use it to protect specific routes. Here’s how to apply it to routes that should only be accessible by authenticated users.
const express = require('express'); const authenticate = require('./middleware/authenticate'); const app = express(); // Protected route app.get('/protected', authenticate, (req, res) => { // Only authenticated users can access this route res.json({ message: 'This is a protected route', user: req.user }); }); // Example of an open route app.get('/open', (req, res) => { res.json({ message: 'This is an open route, anyone can access it.' }); });
In this example:
- The
/protected
route is protected by theauthenticate
middleware, meaning that only requests with a valid JWT token will be allowed to access it. - The
/open
route is open to everyone, and does not require authentication.
3. How the Middleware Works
When a request is made to the
/protected
route, the following steps occur:- The middleware is executed first, checking if the request contains a valid JWT token.
- If the token is valid, the request proceeds to the route handler, and the user data (e.g., user ID) is available in
req.user
. - If the token is missing or invalid, the request is halted, and an error message is returned to the client.
4. Conclusion
Protecting routes with middleware is an essential technique for securing sensitive data and ensuring that only authorized users can access certain parts of your application. With JWT authentication, it is easy to implement this kind of security by verifying tokens and attaching user information to the request. This method is highly scalable and can be used to protect routes in both REST APIs and single-page applications (SPAs).
Creating Authentication Guards in Angular
Authentication guards are used to protect routes in Angular applications by determining whether a user is authenticated before granting access to a specific route. If the user is not authenticated, they can be redirected to a login page or an unauthorized page. Guards can be applied to routes in the Angular routing module to prevent unauthorized access to protected routes.
1. Generating an Authentication Guard
First, we need to generate an authentication guard using Angular CLI. This will create a TypeScript file that contains the logic for protecting routes.
ng generate guard auth/auth
After running this command, Angular CLI creates a new guard in the
auth
folder. The guard will look like this:import { Injectable } from '@angular/core'; import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, Router } from '@angular/router'; import { Observable } from 'rxjs'; import { AuthService } from './auth.service'; // Assume AuthService handles authentication logic @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private authService: AuthService, private router: Router) {} canActivate( route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable
| Promise | boolean { if (this.authService.isAuthenticated()) { return true; // Allow access to the route } else { this.router.navigate(['/login']); // Redirect to login if not authenticated return false; } } } In this guard:
- The
canActivate
method checks if the user is authenticated using a service (e.g.,AuthService
). - If the user is authenticated, the guard returns
true
and allows access to the route. - If the user is not authenticated, the guard returns
false
and redirects the user to the login page.
2. Protecting Routes with the Guard
Once the guard is created, we need to apply it to the routes that should be protected. This is done by adding the
canActivate
property to the route definition in the routing module.import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { AuthGuard } from './auth/auth.guard'; import { DashboardComponent } from './dashboard/dashboard.component'; import { LoginComponent } from './login/login.component'; const routes: Routes = [ { path: 'dashboard', component: DashboardComponent, canActivate: [AuthGuard] }, // Protected route { path: 'login', component: LoginComponent }, // Open route { path: '', redirectTo: '/login', pathMatch: 'full' }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
In this routing configuration:
- The
DashboardComponent
is protected by theAuthGuard
. Only authenticated users can access this route. - The
LoginComponent
is an open route and does not require authentication.
3. Handling Unauthorized Access
When an unauthenticated user attempts to access a protected route, they will be redirected to the login page. You can display a message or notification to inform the user about the authentication requirement.
import { Injectable } from '@angular/core'; import { CanActivate, Router } from '@angular/router'; import { Observable } from 'rxjs'; import { AuthService } from './auth.service'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private authService: AuthService, private router: Router) {} canActivate(): Observable
| Promise | boolean { if (!this.authService.isAuthenticated()) { alert('You must be logged in to access this page'); this.router.navigate(['/login']); return false; } return true; } } In this updated guard, we added an alert to notify the user that they must be logged in to access the page. After the alert, they are redirected to the login page.
4. Conclusion
Authentication guards are a critical feature in Angular to control access to routes based on user authentication status. By using
canActivate
in combination with services likeAuthService
, you can protect sensitive routes and redirect unauthorized users to login pages or other relevant sections.Storing and Managing Tokens in Local Storage
In modern web applications, JSON Web Tokens (JWT) are commonly used for user authentication. After a user logs in, the server sends a JWT to the client, which is typically stored in either local storage or session storage. In this section, we will discuss how to store and manage JWT tokens in the browser's local storage and the best practices for using them in your Angular application.
1. Storing JWT in Local Storage
Once the user successfully logs in, the server sends a JWT to the client. We can store this token in local storage so that it persists even after the browser is closed or refreshed. Here's how you can store the token in local storage:
// Storing token after successful login localStorage.setItem('authToken', response.token);
In this example, the token is stored in local storage under the key
authToken
. Theresponse.token
is the JWT returned from the server after the user logs in successfully.2. Retrieving the Token from Local Storage
When making authenticated API requests, you need to retrieve the stored token from local storage. Here’s how you can get the token:
// Retrieving token from local storage const token = localStorage.getItem('authToken');
By calling
localStorage.getItem('authToken')
, you can retrieve the token that was previously stored. If the token does not exist, it will returnnull
.3. Using the Token for API Requests
Once you have the token, you can use it in the HTTP Authorization header to authenticate requests to protected routes. Here's an example of how to include the token in an HTTP request in Angular:
import { HttpClient, HttpHeaders } from '@angular/common/http'; const token = localStorage.getItem('authToken'); const headers = new HttpHeaders().set('Authorization', `Bearer ${token}`); this.httpClient.get('https://example.com/protected-route', { headers }) .subscribe(response => { console.log(response); });
In this example, the token is added to the
Authorization
header using theHttpHeaders
class. The token is prefixed with theBearer
keyword, as required by the standard for passing JWTs in HTTP headers.4. Removing the Token from Local Storage
When the user logs out, it's important to clear the token from local storage to prevent unauthorized access. You can remove the token from local storage like this:
// Removing the token upon logout localStorage.removeItem('authToken');
This will completely remove the
authToken
from local storage, effectively logging the user out and preventing the token from being used in further API requests.5. Handling Expired Tokens
JWT tokens typically have an expiration time. When a token expires, your application should handle this situation gracefully. You can check the token's expiration by decoding it or by using the server response to notify the user. Here's how you can check for token expiration:
// Checking the token's expiration const token = localStorage.getItem('authToken'); if (token) { const decodedToken = JSON.parse(atob(token.split('.')[1])); const expirationTime = decodedToken.exp; const currentTime = Math.floor(Date.now() / 1000); if (expirationTime < currentTime) { // Token is expired, log out or refresh the token localStorage.removeItem('authToken'); alert('Session expired, please log in again.'); } }
This example decodes the JWT and checks the expiration timestamp. If the token is expired, it removes the token from local storage and informs the user about the session expiration.
6. Conclusion
Storing and managing JWT tokens in local storage provides a simple way to maintain user authentication across different pages and sessions. However, it is important to ensure that tokens are stored securely and that they are removed when no longer needed (e.g., on logout or session expiration). Always follow best practices for managing authentication tokens to ensure your application's security.
Creating and Managing Reactive Forms
Reactive forms in Angular are a powerful way to manage form controls and validations. Unlike template-driven forms, reactive forms are defined in the component class, which provides more control over form validation, form submission, and dynamic form updates. In this section, we will go over how to create and manage reactive forms in Angular.
1. Setting Up Reactive Forms
To work with reactive forms, you need to import the
ReactiveFormsModule
into your Angular module. Here's how to set it up:import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ imports: [ReactiveFormsModule], ... }) export class AppModule { }
Importing
ReactiveFormsModule
enables reactive form functionality in your application.2. Creating a Form Group
In reactive forms, you use a
FormGroup
to represent the form andFormControl
to represent each individual input. Here's how to create a simple reactive form with a single text input field:import { Component } from '@angular/core'; import { FormGroup, FormControl } from '@angular/forms'; @Component({ selector: 'app-login-form', templateUrl: './login-form.component.html', styleUrls: ['./login-form.component.css'] }) export class LoginFormComponent { loginForm = new FormGroup({ username: new FormControl(''), }); onSubmit() { console.log(this.loginForm.value); } }
In this example, we’ve created a
FormGroup
with a single form control (username
) and a methodonSubmit()
that logs the form data when submitted.3. Binding the Form to the Template
To bind the reactive form in the template, use the
formGroup
directive to bind the form group and theformControlName
directive to bind each form control:Here, the
formGroup
directive binds theloginForm
from the component to the template. TheformControlName
directive binds theusername
form control to the corresponding input field.4. Adding Validation to Forms
You can add validation to your form controls using the built-in validators available in Angular. Below is an example of adding a required validator to the username input:
import { Validators } from '@angular/forms'; this.loginForm = new FormGroup({ username: new FormControl('', [Validators.required]), });
In this example, the
Validators.required
validator is added to theusername
form control to ensure that the field is not empty when the form is submitted.5. Handling Form Submission
When the form is submitted, you can access the form values through the
value
property of theFormGroup
. Here's how you handle form submission:onSubmit() { if (this.loginForm.valid) { console.log('Form Submitted!', this.loginForm.value); } else { console.log('Form is not valid'); } }
This checks if the form is valid before submitting and logs the form values to the console. If the form is not valid, an error message is shown.
6. Example: Full Login Form with Validation
Here is an example of a complete login form with validation in a reactive form:
this.loginForm = new FormGroup({ username: new FormControl('', [Validators.required]), password: new FormControl('', [Validators.required, Validators.minLength(6)]), });
In this case, both the
username
andpassword
fields are required, and the password must be at least 6 characters long.7. Conclusion
Reactive forms in Angular provide a more flexible and scalable approach for managing forms. With reactive forms, you can easily handle complex validations, form control updates, and dynamic form changes. By utilizing
FormGroup
,FormControl
, and Angular’s built-in validators, you can create robust and efficient forms in your Angular application.Form Validation and Error Handling
Form validation is a crucial part of any web application. Angular provides powerful tools to handle form validation both on the template side (template-driven forms) and the component side (reactive forms). In this section, we will explore how to validate forms, provide error messages, and handle errors effectively in reactive forms.
1. Setting Up Form Validation
In Angular, you can use built-in validators like
Validators.required
,Validators.minLength
, andValidators.pattern
to enforce validation rules on form controls. Here's how to set up validation for a simple form with two fields: username and password.import { Validators, FormGroup, FormControl } from '@angular/forms'; this.loginForm = new FormGroup({ username: new FormControl('', [Validators.required]), password: new FormControl('', [Validators.required, Validators.minLength(6)]), });
In this example, both the
username
andpassword
fields have validation rules:username
is required, andpassword
is required with a minimum length of 6 characters.2. Displaying Validation Errors
To display validation errors on the form, you can use the
touched
andinvalid
properties of the form controls. Here’s how to show error messages below the input fields:In this example, error messages will appear below the respective input fields if the user interacts with the input fields (touched) and the fields are invalid.
3. Disable Submit Button on Invalid Form
You can also disable the submit button when the form is invalid. This can be done by binding the
disabled
property of the button to the form's invalid state:Here, the submit button will be disabled as long as the form is invalid, ensuring that users cannot submit incomplete or incorrect data.
4. Custom Validation
Angular also allows you to create custom validators for more complex validation logic. Here’s an example of a custom validator that checks if the password contains both letters and numbers:
import { AbstractControl, ValidationErrors } from '@angular/forms'; export function passwordStrengthValidator(control: AbstractControl): ValidationErrors | null { const value = control.value; if (!/[A-Za-z]/.test(value) || !/[0-9]/.test(value)) { return { passwordStrength: 'Password must contain both letters and numbers.' }; } return null; } this.loginForm = new FormGroup({ password: new FormControl('', [Validators.required, passwordStrengthValidator]), });
In this example, the custom validator checks if the password contains both letters and numbers, and returns an error message if the validation fails.
5. Handling Form Submission with Validation
When submitting the form, you should first check if the form is valid before processing the submission. Here's how to handle form submission while ensuring the form is valid:
onSubmit() { if (this.loginForm.valid) { console.log('Form Submitted!', this.loginForm.value); } else { console.log('Form is invalid'); } }
If the form is valid, the form values are logged to the console. If the form is invalid, a message is shown indicating that the form is invalid.
6. Conclusion
Form validation and error handling in Angular are essential for ensuring that users provide the correct input. With reactive forms, you can easily manage validation rules, handle errors, and ensure that forms are submitted only when valid. By using built-in validators, custom validators, and handling error messages in the template, you can create a seamless user experience.
Handling File Uploads in Angular Forms
File uploads are a common feature in web applications, allowing users to send files such as images, documents, or other resources. Angular provides an easy way to handle file uploads in forms through the use of the
File
input type and FormData objects. In this section, we will explore how to handle file uploads in Angular forms effectively.1. Setting Up the File Input in HTML
To handle file uploads in Angular, you first need to create an input field of type
file
. This allows users to select files from their local system. Here's how to set up a basic file input field in your Angular form:In the above example, the file input is bound to a form control named
file
. The(change)
event calls theonFileChange()
method whenever a user selects a file.2. Creating the Form Group in the Component
Next, in your component, you need to create a form group with a file control. You will also handle the file change event to update the form with the selected file.
import { FormGroup, FormControl, Validators } from '@angular/forms'; export class FileUploadComponent { uploadForm: FormGroup; constructor() { this.uploadForm = new FormGroup({ file: new FormControl('', Validators.required) }); } onFileChange(event: any) { const file = event.target.files[0]; // Get the selected file if (file) { this.uploadForm.patchValue({ file: file }); } } onSubmit() { if (this.uploadForm.valid) { const formData = new FormData(); formData.append('file', this.uploadForm.get('file')?.value); this.uploadFile(formData); } } uploadFile(formData: FormData) { // Implement the HTTP request to upload the file console.log('File ready for upload:', formData); } }
In this example, the
onFileChange()
method captures the selected file from the file input and updates the form control with the selected file. TheonSubmit()
method then prepares the file for upload by appending it to aFormData
object and calling theuploadFile()
method.3. Handling the File Upload HTTP Request
Once the file is selected and added to the
FormData
, you can send it to the server using Angular'sHttpClient
. Here's how you can send the file as a POST request:import { HttpClient } from '@angular/common/http'; import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class FileUploadService { constructor(private http: HttpClient) {} uploadFile(formData: FormData) { const uploadUrl = 'https://your-api-endpoint.com/upload'; this.http.post(uploadUrl, formData).subscribe(response => { console.log('File uploaded successfully:', response); }, error => { console.error('Error uploading file:', error); }); } }
In this example, we create a
FileUploadService
that uses Angular'sHttpClient
to send aPOST
request to the server with theFormData
object containing the selected file. On successful upload, a success message is logged, and on failure, the error is logged.4. Handling Multiple File Uploads
If you want to allow the upload of multiple files, you can modify the file input to allow multiple selections and adjust the form control logic accordingly:
In the component, handle the multiple files by using an array to store the selected files:
onFilesChange(event: any) { const files = event.target.files; if (files) { this.uploadForm.patchValue({ files: files }); } } onSubmit() { if (this.uploadForm.valid) { const formData = new FormData(); const files = this.uploadForm.get('files')?.value; for (let i = 0; i < files.length; i++) { formData.append('files', files[i]); } this.uploadFile(formData); } }
In this case, the
onFilesChange()
method captures all selected files, and theonSubmit()
method appends each file to theFormData
object before sending it to the server.5. Conclusion
Handling file uploads in Angular forms is easy with the use of the
file
input type,FormData
objects, and Angular'sHttpClient
. By following the steps outlined in this section, you can implement both single and multiple file uploads in your Angular applications.Custom Validators for Advanced Form Validation
Angular provides built-in validators for common validation scenarios, such as required fields, minimum length, and pattern matching. However, in many cases, you might need to create custom validators to meet specific validation requirements. This section covers how to create and use custom validators for advanced form validation in Angular.
1. Creating a Custom Validator
A custom validator is a function that takes a form control as an argument and returns either an error object or
null
if the validation passes. Here's how to create a simple custom validator that checks if a password contains at least one number:<!-- Custom validator function --> import { AbstractControl, ValidationErrors, ValidatorFn } from '@angular/forms'; export function passwordStrengthValidator(): ValidatorFn { return (control: AbstractControl): ValidationErrors | null => { const value = control.value; const hasNumber = /\d/.test(value); return hasNumber ? null : { passwordStrength: 'Password must contain at least one number' }; }; }
This validator checks if the password contains at least one number. If the condition is not met, it returns an error object with the key
passwordStrength
.2. Using the Custom Validator in a Form
Once the custom validator is created, you can use it in your Angular forms just like any other built-in validator. Here's how to apply the
passwordStrengthValidator
to a form control:import { FormGroup, FormControl, Validators } from '@angular/forms'; import { passwordStrengthValidator } from './password-strength-validator'; export class RegistrationComponent { registrationForm: FormGroup; constructor() { this.registrationForm = new FormGroup({ username: new FormControl('', Validators.required), password: new FormControl('', [Validators.required, passwordStrengthValidator()]), }); } onSubmit() { if (this.registrationForm.valid) { console.log(this.registrationForm.value); } } }
In this example, the
password
form control is validated using both the built-inrequired
validator and the custompasswordStrengthValidator
.3. Displaying Validation Errors
To provide feedback to the user, you can display validation error messages when the form control is invalid. Here's an example of displaying the custom validation error message:
<form [formGroup]="registrationForm" (ngSubmit)="onSubmit()"> <label for="username">Username</label> <input type="text" formControlName="username"> <div *ngIf="registrationForm.get('username').invalid && registrationForm.get('username').touched"> <small>Username is required</small> </div> <label for="password">Password</label> <input type="password" formControlName="password"> <div *ngIf="registrationForm.get('password').invalid && registrationForm.get('password').touched"> <small *ngIf="registrationForm.get('password').errors?.passwordStrength"> {{ registrationForm.get('password').errors.passwordStrength }} </small> <small *ngIf="registrationForm.get('password').errors?.required"> Password is required </small> </div> <button type="submit" [disabled]="registrationForm.invalid">Submit</button> </form>
In this example, the error message for the password control is displayed only if the control is invalid and has been touched. The custom error message is shown if the password does not contain a number.
4. Async Validators
Sometimes, you may need to perform asynchronous validation, such as checking if an email address is already taken. You can create async validators by returning an observable or a promise from the validator function. Here's an example of an async validator that checks if an email is available:
import { AbstractControl, ValidationErrors, AsyncValidatorFn } from '@angular/forms'; import { Observable, of } from 'rxjs'; import { debounceTime, map, switchMap } from 'rxjs/operators'; <!-- Async validator function --> export function emailAsyncValidator(): AsyncValidatorFn { return (control: AbstractControl): Observable
=> { const email = control.value; // Simulate an API request to check if the email is taken return of(email).pipe( debounceTime(500), switchMap(value => { const isTaken = value === 'taken@example.com'; // Simulated check return isTaken ? of({ emailTaken: 'This email is already taken' }) : of(null); }) ); }; } This async validator simulates an email availability check. It uses RxJS operators like
debounceTime
andswitchMap
to simulate an asynchronous request and return an error object if the email is already taken.5. Using the Async Validator in a Form
To use the async validator, apply it to a form control in the same way as a synchronous validator, but with the
asyncValidators
option:import { emailAsyncValidator } from './email-async-validator'; export class RegistrationComponent { registrationForm: FormGroup; constructor() { this.registrationForm = new FormGroup({ username: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email], [emailAsyncValidator()]), }); } onSubmit() { if (this.registrationForm.valid) { console.log(this.registrationForm.value); } } }
In this case, the
email
form control uses theemailAsyncValidator
to check whether the email is already taken.6. Conclusion
Custom validators provide flexibility for advanced form validation scenarios in Angular. You can use both synchronous and asynchronous custom validators to meet your application's specific requirements. By combining Angular's built-in validators with custom ones, you can create powerful, responsive forms that guide users and ensure data integrity.
Logging and Error Handling in Express.js
Effective logging and error handling are crucial for any web application. They help diagnose issues, track user behavior, and ensure that the application runs smoothly. In Express.js, logging and error handling can be implemented using middleware and third-party libraries. This section covers how to set up logging and handle errors in an Express.js application.
1. Setting Up Logging with Morgan
One of the most common logging libraries for Express.js is
morgan
. It is a middleware that logs HTTP requests in a predefined format. Here's how to integratemorgan
into your Express app:<!-- Install Morgan --> npm install morgan <!-- Express app setup --> const express = require('express'); const morgan = require('morgan'); const app = express(); // Use morgan to log requests app.use(morgan('dev')); // 'dev' format is a concise colored log app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
In this example, we install and use
morgan
to log all incoming HTTP requests in the 'dev' format, which is a concise and colored log format. You can replace `'dev'` with other formats such as `'combined'` or `'tiny'` depending on your logging needs.2. Custom Logging with Winston
For more advanced logging, you can use
winston
, a flexible logging library. It allows you to log messages to different transports such as files, databases, or even remote servers. Below is an example of setting up custom logging usingwinston
:<!-- Install Winston --> npm install winston <!-- Express app setup with Winston --> const express = require('express'); const winston = require('winston'); const app = express(); // Create a winston logger const logger = winston.createLogger({ level: 'info', format: winston.format.combine( winston.format.colorize(), winston.format.simple() ), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'app.log' }) ] }); // Log requests app.use((req, res, next) => { logger.info(`${req.method} ${req.url}`); next(); }); app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(3000, () => { logger.info('Server running on port 3000'); });
In this example, we set up a basic
winston
logger that logs messages both to the console and to a file calledapp.log
. The logging format includes colorization for the console logs and simple text formatting.3. Error Handling Middleware
In Express.js, you can handle errors globally by using error-handling middleware. The error-handling middleware should be placed after all the route handlers. Here's how to set up basic error handling in Express:
<!-- Error handling middleware --> app.use((err, req, res, next) => { // Log the error logger.error(err.stack); // Send a response to the client res.status(500).send('Something went wrong!'); });
This middleware catches errors that occur in the route handlers and logs the error stack using
winston
. Then it sends a generic 500 status code response to the client with the message "Something went wrong!".4. Handling Specific Errors
You can also handle specific types of errors, such as validation errors, by customizing the error-handling middleware. For example, if you want to handle validation errors, you can do the following:
<!-- Custom error handling for validation errors --> app.use((err, req, res, next) => { if (err.name === 'ValidationError') { return res.status(400).json({ error: err.message }); } next(err); // pass on to the next error handler });
In this case, if the error is a validation error, the server responds with a 400 status code and the error message. Otherwise, it passes the error to the next error handler.
5. Conclusion
Logging and error handling are essential for building maintainable Express.js applications. By using libraries like
morgan
andwinston
, you can log important events and errors effectively. Additionally, proper error handling ensures that your application can gracefully handle unexpected issues and provide meaningful feedback to the user.Debugging Techniques for Node.js and Angular
Debugging is an essential part of the development process. It helps developers identify and resolve issues in their applications. Both Node.js and Angular provide various tools and techniques for efficient debugging. This section covers some of the most effective debugging strategies for Node.js and Angular applications.
1. Debugging in Node.js
Node.js offers several methods for debugging, including built-in debugging tools, logging libraries, and interactive debugging. Below are some common approaches:
1.1. Using the Node.js Debugger
Node.js comes with a built-in debugger that can be used to step through your code and inspect variables. To use the debugger, add the
debugger
keyword in your code where you want the debugger to pause:// Example of using the Node.js debugger function greet(name) { debugger; // Program will pause here console.log('Hello, ' + name); } greet('Alice');
After adding the
debugger
statement, run the Node.js application with theinspect
flag:node inspect app.js
When you run this command, Node.js will start in debugging mode, and you can use the
repl
(Read-Eval-Print Loop) to inspect variables and step through the code.1.2. Using Console Logs
One of the simplest ways to debug your Node.js application is by using
console.log()
statements. These allow you to output values at various points in your code to track the flow and identify issues.console.log('Debugging variable value:', variable);
While not as sophisticated as using the debugger, logging is often useful for quick and temporary debugging during development.
1.3. Using Visual Studio Code Debugger
Visual Studio Code (VS Code) provides an integrated debugger for Node.js applications. To use the debugger, set breakpoints in your code by clicking next to the line numbers, and then run the application in debug mode:
{ "version": "0.2.0", "configurations": [ { "type": "node", "request": "launch", "name": "Launch Program", "skipFiles": ["
/**"], "program": "${workspaceFolder}/app.js" } ] } With this configuration in place, you can launch the debugger directly from VS Code.
2. Debugging in Angular
Angular provides several techniques for debugging, including using the browser’s developer tools, logging, and the Angular DevTools extension. Below are some common methods:
2.1. Using Browser Developer Tools
The browser’s developer tools are a powerful debugging tool for Angular applications. You can use the
Console
tab to log errors and inspect output, and theSources
tab to set breakpoints directly in your application’s JavaScript code.2.2. Using Angular’s Error Handling
Angular has built-in error handling mechanisms that allow you to catch and handle errors in your application. You can use the
HttpClient
to catch HTTP request errors and display useful error messages to the user:import { HttpClient } from '@angular/common/http'; import { catchError } from 'rxjs/operators'; import { throwError } from 'rxjs'; constructor(private http: HttpClient) {} getData() { this.http.get('/api/data') .pipe( catchError(error => { console.error('Error occurred:', error); return throwError(error); }) ) .subscribe(data => console.log(data)); }
In this example, we use the
catchError
operator from RxJS to catch any errors that occur during the HTTP request and log them to the console.2.3. Using Angular DevTools
Angular DevTools is a browser extension that provides advanced debugging features for Angular applications. It allows you to inspect your application's component tree, view change detection cycles, and profile performance. You can install Angular DevTools from the Chrome or Firefox extension stores.
2.4. Using Console Logs
Just like in Node.js, you can use
console.log()
statements in your Angular components and services to track the flow of your application and inspect variable values:console.log('Debugging Angular component:', this.myComponent);
3. Conclusion
Effective debugging is an essential skill for developers. By using the built-in debugging tools in Node.js and Angular, along with external tools like Visual Studio Code and Angular DevTools, you can quickly identify and fix issues in your applications. Remember to also use logging techniques, error handling, and breakpoints to streamline your debugging process.
Handling API Errors and Showing User-Friendly Messages
When interacting with APIs, errors can occur due to various reasons such as network issues, server failures, or invalid user inputs. It's important to handle these errors gracefully and provide clear, user-friendly messages. This section covers how to handle API errors and display meaningful messages to users in both Node.js and Angular applications.
1. Handling API Errors in Node.js
In Node.js, errors can occur when making API requests, interacting with databases, or processing user inputs. Proper error handling ensures that the server responds with appropriate messages, helping developers and users troubleshoot issues.
1.1. Using Try-Catch for Synchronous Errors
For synchronous code, use the
try-catch
block to handle errors. This allows you to catch any errors that occur and respond with an appropriate error message:try { const data = someSyncFunction(); } catch (error) { console.error('An error occurred:', error.message); res.status(500).json({ message: 'Something went wrong! Please try again later.' }); }
In this example, if an error occurs during the execution of
someSyncFunction
, the server responds with a 500 status code and a user-friendly error message.1.2. Handling Asynchronous Errors with Promises
When dealing with asynchronous code, use
.catch()
to handle errors in promises:someAsyncFunction() .then(result => { res.json(result); }) .catch(error => { console.error('API request failed:', error.message); res.status(500).json({ message: 'Error fetching data from the server. Please try again later.' }); });
Here, if the asynchronous function
someAsyncFunction
fails, an error message is sent to the client indicating that something went wrong.1.3. Handling Errors in Express Middleware
To handle errors globally, you can use error-handling middleware in Express. This middleware will catch any unhandled errors and send a user-friendly response:
app.use((err, req, res, next) => { console.error('Unhandled error:', err.message); res.status(500).json({ message: 'Internal Server Error. Please try again later.' }); });
This global error handler will catch any errors that are not specifically handled in your routes and send a generic error response to the client.
2. Handling API Errors in Angular
In Angular, when making HTTP requests to APIs, it's crucial to handle errors effectively and display meaningful messages to users. Angular's
HttpClient
module provides built-in mechanisms to handle HTTP errors.2.1. Using the
catchError
Operator for HTTP ErrorsAngular’s
catchError
operator from RxJS allows you to catch HTTP errors and handle them in a user-friendly way. Here's an example of catching an error from an API call:import { HttpClient } from '@angular/common/http'; import { catchError } from 'rxjs/operators'; import { throwError } from 'rxjs'; constructor(private http: HttpClient) {} getData() { this.http.get('/api/data') .pipe( catchError(error => { console.error('API error:', error); const errorMessage = error.status === 404 ? 'Resource not found' : 'An unexpected error occurred'; return throwError(errorMessage); }) ) .subscribe( data => console.log(data), errorMessage => this.handleError(errorMessage) ); } handleError(errorMessage: string) { alert(errorMessage); // Display user-friendly message }
In this example, if an error occurs while fetching data from the API, the
catchError
operator catches the error, logs it, and returns a user-friendly message based on the error status code. The message is then passed to thehandleError
method, where it can be displayed to the user.2.2. Handling Different HTTP Status Codes
Different HTTP status codes indicate different types of errors. It's important to handle these appropriately by returning messages specific to the error type. For example, a 404 error indicates a "resource not found" issue, while a 500 error indicates a server problem:
this.http.get('/api/data') .pipe( catchError(error => { let errorMessage = 'An unexpected error occurred'; if (error.status === 404) { errorMessage = 'The requested resource was not found'; } else if (error.status === 500) { errorMessage = 'Internal server error. Please try again later.'; } return throwError(errorMessage); }) ) .subscribe( data => console.log(data), errorMessage => this.handleError(errorMessage) );
In this case, different error messages are displayed based on the HTTP status code.
3. Conclusion
Handling API errors and providing user-friendly messages is crucial for ensuring a smooth user experience. In both Node.js and Angular, there are various techniques for catching errors and displaying clear, helpful messages. By implementing proper error handling and showing relevant feedback to users, you can make your application more robust and user-friendly.
Using Angular Pipes and Custom Pipes
Angular pipes provide an easy way to transform data in templates. They can be used to format data, filter lists, or perform other transformations. Angular also allows you to create custom pipes to meet specific transformation needs. This section covers how to use built-in pipes and create custom pipes in Angular.
1. Introduction to Angular Pipes
Pipes are a useful feature in Angular that allow you to transform data in templates. They can be applied to expressions in templates to perform operations like formatting dates, currencies, or even filtering lists.
1.1. Built-in Angular Pipes
Angular provides several built-in pipes for common tasks. Some of the most commonly used pipes include:
date
: Formats a date value according to a given format.currency
: Formats a number as a currency value.percent
: Formats a number as a percentage.uppercase
: Transforms a string to uppercase.lowercase
: Transforms a string to lowercase.json
: Converts an object or array into a JSON string.
1.2. Using Built-in Pipes
To use a built-in pipe, apply it to an expression in the template with the
|
(pipe) operator. Here's an example of using thedate
andcurrency
pipes:Today's date is: {{ today | date:'fullDate' }}
Price: {{ price | currency:'USD' }}
In this example, the
today
variable is formatted as a full date, and theprice
variable is formatted as a currency in USD.2. Creating Custom Pipes in Angular
In addition to built-in pipes, you can create custom pipes to perform specific transformations on data. Here's how to create and use a custom pipe in Angular.
2.1. Creating a Custom Pipe
To create a custom pipe, you'll need to implement the
PipeTransform
interface. A custom pipe consists of a class decorated with the@Pipe
decorator and the logic to transform the input data.import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'reverse' }) export class ReversePipe implements PipeTransform { transform(value: string): string { return value.split('').reverse().join(''); } }
This
ReversePipe
takes a string and reverses it. Thetransform
method is implemented to perform the transformation.2.2. Registering the Pipe
Once the custom pipe is created, you need to register it in an Angular module. This allows Angular to use the pipe in your templates:
// app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { ReversePipe } from './reverse.pipe'; @NgModule({ declarations: [ AppComponent, ReversePipe // Registering the custom pipe ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
The
ReversePipe
is registered in thedeclarations
array of the module to make it available in the application.2.3. Using the Custom Pipe in Templates
Now that the custom pipe is created and registered, you can use it in your templates just like any built-in pipe:
Reversed String: {{ 'Hello, Angular!' | reverse }}
In this example, the text "Hello, Angular!" will be reversed by the
reverse
pipe and displayed as "!ralugnA ,olleH".3. Pipe Chaining
In Angular, you can chain multiple pipes together to apply multiple transformations to data. Here's an example of chaining the
uppercase
andreverse
pipes:{{ 'Hello, Angular!' | reverse | uppercase }}
In this case, the string "Hello, Angular!" will first be reversed and then converted to uppercase, resulting in "RALUGNA ,OLLEH".
4. Conclusion
Angular pipes provide an easy and powerful way to transform data in templates. By using built-in pipes or creating custom ones, you can perform a wide range of transformations, such as formatting dates, reversing strings, or modifying numbers. Custom pipes allow you to encapsulate logic and reuse it throughout your application, making your code cleaner and more maintainable.
Creating and Using Angular Directives
Angular directives are powerful tools that allow you to manipulate the DOM, modify the behavior of elements, or add custom functionality. There are three types of directives in Angular: structural directives, attribute directives, and custom directives. This section explains how to use built-in directives and create your own custom directives in Angular.
1. Introduction to Angular Directives
Directives in Angular are used to extend the functionality of HTML elements in your templates. They can be used to:
- Change the appearance or behavior of elements.
- Manipulate DOM elements dynamically.
- Create reusable and customizable components.
1.1. Types of Directives
Angular directives are classified into three main categories:
- Structural Directives: These directives change the structure of the DOM by adding or removing elements. Examples include
*ngIf
,*ngFor
, and*ngSwitch
. - Attribute Directives: These directives change the appearance or behavior of an element without altering the DOM structure. Examples include
ngClass
,ngStyle
, and custom attribute directives. - Custom Directives: Custom directives allow you to add your own functionality to elements, such as applying custom logic or behavior.
2. Using Built-in Structural Directives
Angular provides several built-in structural directives that allow you to conditionally add or remove elements from the DOM.
2.1. Using
*ngIf
The
*ngIf
directive is used to add or remove an element from the DOM based on a condition. Here's an example:This is visible if isVisible is true.In this example, the
div
element will only be displayed if theisVisible
property istrue
.2.2. Using
*ngFor
The
*ngFor
directive is used to loop through a list of items and display them in the DOM. Here's an example:- {{ item }}
This will loop through the
items
array and display each item in an unordered list.3. Using Built-in Attribute Directives
Attribute directives allow you to change the appearance or behavior of elements. One commonly used attribute directive is
ngClass
, which applies CSS classes dynamically.3.1. Using
ngClass
The
ngClass
directive allows you to add or remove CSS classes from an element conditionally. Here's an example:This element will be highlighted if isHighlighted is true.In this example, the
highlight
class will be applied to thediv
element if theisHighlighted
property istrue
.4. Creating Custom Directives
In addition to built-in directives, Angular allows you to create custom directives to add custom behavior to elements. Custom directives consist of a directive class decorated with the
@Directive
decorator, and they are used to modify the behavior of DOM elements.4.1. Creating a Custom Attribute Directive
Here’s how to create a custom attribute directive that changes the background color of an element when the user hovers over it:
import { Directive, ElementRef, HostListener } from '@angular/core'; @Directive({ selector: '[appHighlight]' }) export class HighlightDirective { constructor(private el: ElementRef) {} @HostListener('mouseenter') onMouseEnter() { this.el.nativeElement.style.backgroundColor = 'yellow'; } @HostListener('mouseleave') onMouseLeave() { this.el.nativeElement.style.backgroundColor = null; } }
This
HighlightDirective
listens formouseenter
andmouseleave
events and changes the background color of the element accordingly.4.2. Registering the Custom Directive
To use a custom directive, you need to declare it in an Angular module. Here’s how to register the
HighlightDirective
:// app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { HighlightDirective } from './highlight.directive'; @NgModule({ declarations: [ AppComponent, HighlightDirective // Registering the custom directive ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
4.3. Using the Custom Directive in Templates
Once the custom directive is created and registered, you can use it in the template just like a built-in directive:
Hover over this text to change its background color.
The
appHighlight
directive will change the background color of thep
element when the mouse hovers over it.5. Conclusion
Angular directives are a powerful way to extend the behavior of DOM elements in your application. By using built-in directives such as
*ngIf
and*ngFor
, and creating your own custom directives, you can add dynamic behavior to your templates and build highly interactive UIs.Angular Modules for Large Applications
As your Angular application grows in size and complexity, it becomes essential to organize your code into smaller, more manageable pieces. Angular's module system provides a way to group related functionality together, making it easier to maintain and scale your application. In this section, we’ll explore how to use Angular modules effectively in large applications.
1. Introduction to Angular Modules
Angular modules are containers for related components, directives, pipes, and services. They help you organize your application into cohesive blocks of functionality. Every Angular application has at least one module, the
AppModule
, which is the root module. You can create additional feature modules to organize your application further.1.1. Why Use Angular Modules?
- Modularity: Group related functionality into distinct units, making the application easier to maintain and scale.
- Lazy Loading: Load feature modules only when needed, improving the initial loading performance of your application.
- Separation of Concerns: Keep different parts of your application (e.g., user management, orders, etc.) in separate modules to increase clarity and reduce coupling.
- Reusability: Create reusable and shareable feature modules that can be imported into different parts of your application.
2. Creating Feature Modules
Feature modules are responsible for encapsulating a specific area of functionality in your application. For example, if you have a user management section in your app, you can create a module just for that feature.
2.1. Creating a Feature Module
You can create a feature module using Angular CLI with the following command:
ng generate module user
This will generate a new module called
UserModule
in your application.2.2. Declaring Components, Directives, and Pipes in Feature Modules
Once you have created a feature module, you can declare components, directives, and pipes that are specific to that module. For example, the
UserModule
may have aUserListComponent
and aUserDetailComponent
.import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { UserListComponent } from './user-list/user-list.component'; import { UserDetailComponent } from './user-detail/user-detail.component'; @NgModule({ declarations: [ UserListComponent, UserDetailComponent ], imports: [ CommonModule ], exports: [ UserListComponent, UserDetailComponent ] }) export class UserModule { }
This example shows the
UserModule
with two components:UserListComponent
andUserDetailComponent
. TheCommonModule
is imported to provide common Angular directives such asngIf
andngFor
. The components are also exported so they can be used in other modules.3. Importing Feature Modules into the Root Module
Once a feature module is created, you need to import it into the root module or any other module that needs its functionality. For example, you can import the
UserModule
into theAppModule
to use its components:import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { UserModule } from './user/user.module'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, UserModule // Importing the UserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
By importing the
UserModule
into theAppModule
, you can now use theUserListComponent
andUserDetailComponent
in the templates of any component inAppModule
.4. Lazy Loading Modules
Lazy loading is a technique used to load feature modules only when they are needed, which helps improve the performance of your Angular application. To implement lazy loading, you must configure the module routes in the root module.
4.1. Setting Up Lazy Loading
First, create the feature module (e.g.,
UserModule
) and set up routing for it. Then, modify theAppRoutingModule
to load the module lazily.import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'users', loadChildren: () => import('./user/user.module').then(m => m.UserModule) } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
In this example, the
UserModule
will be loaded only when the user navigates to the/users
route, improving the initial loading performance of the application.5. Shared Modules
In large applications, you might have some functionality that needs to be reused across multiple feature modules, such as common components, directives, or pipes. Instead of declaring these items in each feature module, you can create a shared module.
5.1. Creating a Shared Module
The shared module is where you can declare and export commonly used components, directives, and pipes. For example:
import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { HeaderComponent } from './header/header.component'; import { FooterComponent } from './footer/footer.component'; @NgModule({ declarations: [ HeaderComponent, FooterComponent ], imports: [ CommonModule ], exports: [ HeaderComponent, FooterComponent ] }) export class SharedModule { }
This shared module declares the
HeaderComponent
andFooterComponent
components, which can be reused across various feature modules by importing theSharedModule
.6. Conclusion
Angular modules are a powerful tool for organizing large applications. They help break down your application into smaller, more manageable pieces, allowing for better maintainability, scalability, and performance optimization. By using feature modules, lazy loading, and shared modules, you can build efficient and well-structured Angular applications that are easy to scale as they grow.
Change Detection and Lifecycle Hooks
Change detection and lifecycle hooks are fundamental concepts in Angular that allow you to manage and respond to changes in your application’s state and the lifecycle of components and directives. Understanding how Angular performs change detection and when lifecycle hooks are triggered is key to building efficient and responsive applications.
1. Introduction to Change Detection
Angular uses a change detection mechanism to track changes in the data model and update the view accordingly. When a component or directive’s state changes, Angular checks the component tree to determine if the view should be updated. The process of detecting changes and updating the view is called change detection.
1.1. How Change Detection Works
Angular's change detection operates on the concept of a "change detection cycle." The framework checks for changes in the component's state by comparing the current state with the previous state. If any changes are detected, Angular updates the view accordingly.
Angular runs change detection automatically in the following scenarios:
- When an event is triggered (e.g., user input, button click).
- When an HTTP request completes.
- When a `setTimeout` or `setInterval` function is called.
- When Angular detects changes in observables or promises.
1.2. Change Detection Strategies
Angular provides two change detection strategies:
- Default: The default strategy checks every component in the component tree for changes every time the change detection cycle runs.
- OnPush: The
OnPush
strategy reduces the frequency of checks by only checking a component when its input properties change, or when an event is triggered inside the component.
2. Lifecycle Hooks in Angular
Lifecycle hooks are methods that get called at specific points in a component’s or directive’s life. These hooks allow you to run custom logic at various stages of the lifecycle, such as initialization, change detection, and cleanup.
2.1. Common Lifecycle Hooks
Here are some of the most commonly used lifecycle hooks in Angular:
- ngOnChanges: Called when an input property of the component changes.
- ngOnInit: Called once, after the first
ngOnChanges
is called. It’s a good place for initialization logic. - ngDoCheck: Called during every change detection cycle. It’s a place for custom change detection logic.
- ngAfterContentInit: Called after Angular projects external content into the component’s view (e.g., `
`). - ngAfterContentChecked: Called after every check of the component’s content.
- ngAfterViewInit: Called after Angular initializes the component’s view and child views.
- ngAfterViewChecked: Called after every check of the component’s view and child views.
- ngOnDestroy: Called just before Angular destroys the component or directive. It’s a good place to clean up resources like subscriptions or timers.
2.2. Example of Using Lifecycle Hooks
Here’s an example demonstrating how to use some of the lifecycle hooks in a component:
import { Component, OnInit, OnChanges, SimpleChanges, DoCheck, OnDestroy } from '@angular/core'; @Component({ selector: 'app-lifecycle-demo', template: '
Lifecycle Demo Component
' }) export class LifecycleDemoComponent implements OnInit, OnChanges, DoCheck, OnDestroy { constructor() { console.log('Constructor: Component is created'); } ngOnChanges(changes: SimpleChanges): void { console.log('ngOnChanges: Input properties changed', changes); } ngOnInit(): void { console.log('ngOnInit: Component initialized'); } ngDoCheck(): void { console.log('ngDoCheck: Custom change detection logic'); } ngOnDestroy(): void { console.log('ngOnDestroy: Component is about to be destroyed'); } }In this example, the component logs messages during different lifecycle stages:
ngOnChanges
: Logs when input properties change.ngOnInit
: Logs when the component is initialized.ngDoCheck
: Logs during every change detection cycle.ngOnDestroy
: Logs when the component is about to be destroyed.
3. Change Detection and Performance Optimization
Efficient change detection is critical in large Angular applications. If not optimized, Angular’s default change detection strategy can result in performance issues, especially with complex component trees and frequent updates.
3.1. Optimizing with OnPush
Using the
OnPush
change detection strategy can improve performance by reducing the number of components checked during each change detection cycle. You can set the change detection strategy toOnPush
at the component level like this:@Component({ selector: 'app-onpush-demo', template: '
OnPush Change Detection
', changeDetection: ChangeDetectionStrategy.OnPush }) export class OnPushDemoComponent {}This ensures that Angular will only check the component when one of its input properties changes, or when an event is triggered within the component.
4. Conclusion
Change detection and lifecycle hooks are core concepts in Angular that help you manage the state and behavior of components in a structured way. By understanding when and how change detection occurs, and by using lifecycle hooks effectively, you can optimize the performance of your Angular applications and create more efficient, maintainable code.
Uploading Files with Multer in Express.js
File uploading is a common feature in web applications, and in Express.js, the
multer
middleware is commonly used to handle multipart/form-data, which is used for uploading files. This section covers how to use Multer to upload files in an Express.js application.1. Installing Multer
Before you can use Multer, you need to install it in your project. You can install Multer via npm by running the following command:
npm install multer
2. Setting Up Multer in Express.js
After installing Multer, you can set it up to handle file uploads in your Express.js app. Here’s an example of how to configure Multer to store uploaded files in a specific directory:
const express = require('express'); const multer = require('multer'); const path = require('path'); const app = express(); // Set storage engine const storage = multer.diskStorage({ destination: './uploads/', // Store files in 'uploads' folder filename: (req, file, cb) => { cb(null, file.fieldname + '-' + Date.now() + path.extname(file.originalname)); // Create unique filename } }); // Initialize multer const upload = multer({ storage: storage, limits: { fileSize: 1000000 }, // Limit file size to 1MB }).single('file'); // Handle single file upload with the field name 'file' // Create an endpoint to handle file upload app.post('/upload', (req, res) => { upload(req, res, (err) => { if (err) { res.status(400).send({ message: 'File upload failed', error: err.message }); } else { res.status(200).send({ message: 'File uploaded successfully', file: req.file }); } }); }); app.listen(3000, () => { console.log('Server started on http://localhost:3000'); });
In this example, Multer is configured to store uploaded files in the
uploads
directory with a unique filename based on the original file name and the current timestamp. The file size is limited to 1MB to prevent overly large uploads.3. Handling File Uploads in HTML Form
To upload a file, you need an HTML form that allows users to select a file. Here’s an example of a simple file upload form:
This form sends a POST request to the
/upload
endpoint with the selected file. Theenctype="multipart/form-data"
attribute is required to handle file uploads in forms.4. Handling Multiple File Uploads
In some cases, you may want to upload multiple files at once. You can use the
array
method of Multer to handle multiple files. Here’s an example of how to upload multiple files:app.post('/uploadMultiple', (req, res) => { upload.array('files', 5)(req, res, (err) => { // Max 5 files if (err) { res.status(400).send({ message: 'File upload failed', error: err.message }); } else { res.status(200).send({ message: 'Files uploaded successfully', files: req.files }); } }); });
In this example, the
upload.array('files', 5)
method handles the upload of multiple files with the field namefiles
and limits the number of files to 5. The uploaded files are stored in thereq.files
array.5. Handling File Upload Errors
Multer can throw errors during file uploads, such as when the file exceeds the size limit or the file type is not allowed. You can handle these errors by using the error callback in your route handlers. Here’s an example of error handling:
const upload = multer({ storage: storage, limits: { fileSize: 1000000 }, // 1MB limit fileFilter: (req, file, cb) => { const filetypes = /jpeg|jpg|png/; const extname = filetypes.test(path.extname(file.originalname).toLowerCase()); const mimetype = filetypes.test(file.mimetype); if (extname && mimetype) { return cb(null, true); } cb(new Error('File type not allowed')); } }).single('file'); app.post('/upload', (req, res) => { upload(req, res, (err) => { if (err) { if (err.message === 'File type not allowed') { return res.status(400).send({ message: 'Only image files are allowed' }); } else { return res.status(400).send({ message: 'File upload failed', error: err.message }); } } res.status(200).send({ message: 'File uploaded successfully', file: req.file }); }); });
In this example, the file filter function checks if the uploaded file type is an image (JPEG, JPG, or PNG). If the file type is not allowed, an error is thrown, and the appropriate message is sent to the client.
6. Conclusion
Multer is a powerful and flexible middleware for handling file uploads in Express.js. By configuring storage options, file size limits, and file type filtering, you can easily manage file uploads in your web applications. Whether you’re handling single or multiple file uploads, Multer provides a simple and effective solution for managing file uploads in your backend.
Serving and Downloading Files from the Server
In a web application, you might need to serve files (such as images, documents, or other resources) to the client or allow users to download files from the server. This section explains how to serve static files and handle file downloads in an Express.js application.
1. Serving Static Files
To serve static files (like images, CSS, or JavaScript files) in Express.js, you can use the built-in
express.static
middleware. This middleware allows you to serve files from a specific directory. Here's how you can set it up:const express = require('express'); const app = express(); const path = require('path'); // Serve static files from the 'public' directory app.use(express.static(path.join(__dirname, 'public'))); app.listen(3000, () => { console.log('Server started on http://localhost:3000'); });
In this example, the
express.static
middleware is used to serve files from thepublic
directory. When a user requests a file (like an image or a CSS file), Express will look for the file in thepublic
folder and serve it if found.2. Serving Files from a Specific Path
If you need to serve files from a specific path, you can specify the path directly in the
express.static
middleware. For example:app.use('/images', express.static(path.join(__dirname, 'uploads')));
This will serve all files in the
uploads
directory under the/images
route. For example, if a user requests/images/photo.jpg
, Express will serve thephoto.jpg
file from theuploads
directory.3. Downloading Files from the Server
If you want to allow users to download files from the server, you can use the
res.download()
method. This method sends a file as an attachment and prompts the user to download it. Here's an example of how to implement it:app.get('/download/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'uploads', filename); res.download(filePath, (err) => { if (err) { res.status(404).send({ message: 'File not found' }); } }); });
In this example, the user can download a file by accessing the
/download/:filename
route. The server looks for the file in theuploads
directory, and if the file exists, it is sent as a download. If the file does not exist, a 404 error is returned.4. File Download with Custom Filename
You can also specify a custom filename for the downloaded file. The second argument to the
res.download()
method allows you to define a different name for the downloaded file. Here's an example:app.get('/download/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'uploads', filename); // Provide a custom filename for the download res.download(filePath, 'custom-name.pdf', (err) => { if (err) { res.status(404).send({ message: 'File not found' }); } }); });
In this case, the file is downloaded with the name
custom-name.pdf
regardless of the actual file name on the server.5. Handling Errors and Missing Files
To ensure users don't encounter issues when downloading files, it's important to handle errors gracefully. For example, if a file doesn't exist, you can return an appropriate message instead of crashing the application:
app.get('/download/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'uploads', filename); // Check if file exists fs.access(filePath, fs.constants.F_OK, (err) => { if (err) { return res.status(404).send({ message: 'File not found' }); } res.download(filePath); }); });
In this example, we use
fs.access()
to check if the file exists before attempting to serve it. If the file is not found, we send a 404 error with a custom message.6. Conclusion
Serving and downloading files in Express.js is straightforward with the
express.static
middleware for serving static files and theres.download()
method for handling file downloads. By using these methods, you can easily manage file serving and downloading in your Express.js applications, while also handling errors and ensuring a smooth user experience.Managing Large File Uploads
Handling large file uploads in a web application can present challenges related to server performance, memory usage, and timeouts. It's essential to configure your server and use appropriate libraries to manage large file uploads efficiently. In this section, we will go over how to handle large file uploads in Node.js using Multer, a popular middleware for handling multipart/form-data.
1. Setting Up Multer for File Uploads
Multer is a middleware for handling
multipart/form-data
, which is primarily used for uploading files. It provides an easy way to handle large file uploads in Express.js. Here's how you can set up Multer in your Express.js application:const express = require('express'); const multer = require('multer'); const app = express(); // Set up Multer storage options const storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'uploads/'); // Destination folder for uploaded files }, filename: function (req, file, cb) { cb(null, Date.now() + '-' + file.originalname); // Unique filename } }); // Set up file size limit (e.g., 10MB) const upload = multer({ storage: storage, limits: { fileSize: 10 * 1024 * 1024 } // Max file size: 10MB }); app.post('/upload', upload.single('file'), (req, res) => { if (!req.file) { return res.status(400).send('No file uploaded.'); } res.send('File uploaded successfully.'); }); app.listen(3000, () => { console.log('Server started on http://localhost:3000'); });
In this example, we set up Multer to handle file uploads to the
uploads/
directory. Thelimits
option is used to specify a maximum file size (in this case, 10MB). If the file exceeds the limit, Multer will throw an error.2. Handling Large Files Efficiently
For large file uploads, it's important to manage memory usage and server load. Here are some best practices:
- Set a reasonable file size limit: Limiting the size of uploaded files helps avoid memory overload on the server. Use the
limits
option in Multer to enforce this. - Stream files: Rather than loading entire files into memory, stream files to disk as they're being uploaded. This reduces the memory footprint and improves performance.
- Handle timeouts: For large file uploads, you might need to adjust the server's timeout settings to prevent the server from timing out during long uploads.
3. Example: Streaming Large Files
If you're dealing with large files (e.g., videos, large images), it's a good practice to stream the file instead of saving it all at once. Multer can handle this efficiently by writing the file directly to disk during the upload. Here's an example:
const fs = require('fs'); // Set up file upload stream app.post('/upload-large', upload.single('file'), (req, res) => { if (!req.file) { return res.status(400).send('No file uploaded.'); } const filePath = 'uploads/' + req.file.filename; const fileStream = fs.createWriteStream(filePath); req.file.stream.pipe(fileStream); fileStream.on('finish', () => { res.send('Large file uploaded successfully!'); }); fileStream.on('error', (err) => { res.status(500).send('Error uploading file.'); }); });
This example shows how to handle large file uploads by streaming the file directly to disk, reducing the memory load on the server. The file is piped to a writable stream and saved to disk as it’s uploaded.
4. Handling Upload Timeouts
Large file uploads can take time, and it’s essential to prevent the server from timing out during the upload process. Here's how you can configure your server to handle long uploads:
// Increase server timeout for large uploads app.use((req, res, next) => { req.setTimeout(0); // No timeout next(); }); app.listen(3000, () => { console.log('Server started on http://localhost:3000'); });
In this example, we set the timeout to 0, meaning that the server will not time out during a file upload. However, be cautious when using this in production, as it can cause issues if left unconfigured for extended periods.
5. Handling Errors and Feedback
It's important to provide feedback to the user during file uploads, particularly for large files. You can send progress updates and handle errors gracefully to ensure a smooth user experience. Here's an example of how to handle upload errors:
app.post('/upload', upload.single('file'), (req, res) => { if (!req.file) { return res.status(400).send('No file uploaded.'); } // Handle file size limit exceeded error upload.single('file').on('error', (err) => { if (err.code === 'LIMIT_FILE_SIZE') { return res.status(400).send('File size exceeds the limit.'); } res.status(500).send('Error uploading file.'); }); res.send('File uploaded successfully.'); });
In this example, we handle errors such as exceeding the file size limit and provide appropriate feedback to the user. You can customize the error handling based on the needs of your application.
6. Conclusion
Managing large file uploads requires careful configuration to ensure efficient handling and minimize the impact on server performance. By using Multer's configuration options like
limits
, streaming files, and handling timeouts, you can ensure a seamless upload experience for your users. Additionally, providing clear feedback and handling errors will improve user satisfaction and reduce confusion during the upload process.Introduction to WebSockets and Real-Time Communication
WebSockets provide a full-duplex communication channel over a single, long-lived connection, allowing servers and clients to send messages to each other in real-time. Unlike HTTP, which is request-response based, WebSockets allow for continuous communication with low latency. This makes them ideal for use cases such as real-time chat applications, live notifications, and collaborative applications. In this section, we'll explore the basics of WebSockets and how to implement real-time communication using WebSockets in Node.js.
1. What is WebSocket?
WebSocket is a protocol that provides full-duplex communication channels over a single TCP connection. It is designed to be used in situations where servers need to send messages to clients without waiting for a request. WebSocket is different from HTTP in that it allows for persistent connections, which makes it more efficient for applications requiring real-time communication.
2. Setting Up WebSocket in Node.js
In Node.js, WebSocket connections can be easily handled using the
ws
library. This library allows you to create a WebSocket server and manage connections. Here's how to set up a basic WebSocket server:const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080 }); server.on('connection', (ws) => { console.log('A new client connected'); // Send a message to the client ws.send('Welcome to the WebSocket server!'); // Listen for messages from the client ws.on('message', (message) => { console.log('Received:', message); }); // Handle client disconnection ws.on('close', () => { console.log('Client disconnected'); }); }); console.log('WebSocket server started on ws://localhost:8080');
This WebSocket server listens on port 8080 and sends a welcome message to any client that connects. It also listens for incoming messages and handles client disconnections.
3. Connecting to WebSocket from the Client
To connect to a WebSocket server from the client side, you can use the WebSocket API available in modern browsers. Here's an example of how to connect to the WebSocket server we just created:
// Connect to the WebSocket server const socket = new WebSocket('ws://localhost:8080'); // Listen for messages from the server socket.onmessage = (event) => { console.log('Message from server:', event.data); }; // Send a message to the server socket.onopen = () => { socket.send('Hello, server!'); }; // Handle WebSocket errors socket.onerror = (error) => { console.error('WebSocket error:', error); }; // Handle WebSocket close event socket.onclose = () => { console.log('WebSocket connection closed'); };
In this example, the client connects to the WebSocket server, sends a message, and listens for responses. The
onmessage
event listener is used to handle messages from the server, while theonopen
event ensures the socket is open before sending messages.4. Broadcasting Messages to Multiple Clients
WebSockets are particularly useful for real-time communication with multiple clients. If you want to broadcast a message to all connected clients, you can iterate over all the WebSocket connections and send a message to each one. Here's an example:
server.on('connection', (ws) => { console.log('A new client connected'); // Broadcast a message to all connected clients server.clients.forEach((client) => { if (client !== ws && client.readyState === WebSocket.OPEN) { client.send('A new client has joined the chat!'); } }); // Listen for messages from the client ws.on('message', (message) => { console.log('Received:', message); // Broadcast the message to all clients server.clients.forEach((client) => { if (client !== ws && client.readyState === WebSocket.OPEN) { client.send(message); } }); }); // Handle client disconnection ws.on('close', () => { console.log('Client disconnected'); }); });
In this example, when a new client connects or sends a message, the server broadcasts it to all other connected clients. This is useful for creating chat applications or real-time collaboration tools.
5. Real-Time Communication Use Cases
WebSockets are ideal for applications that require real-time communication. Some common use cases include:
- Chat Applications: WebSockets allow messages to be sent and received in real-time without refreshing the page, making them perfect for chat apps.
- Live Notifications: WebSockets can be used to send live notifications to users, such as updates on social media or alerts for new messages.
- Collaborative Applications: WebSockets enable real-time collaboration between users, such as in document editing apps.
- Live Gaming: WebSockets are used in multiplayer games to handle real-time interactions between players.
6. Conclusion
WebSockets provide a powerful and efficient way to implement real-time communication in web applications. By maintaining a persistent connection, WebSockets allow for instant message delivery with low latency. Whether you're building a chat application, live notifications, or collaborative tools, WebSockets can help you provide a seamless real-time experience for your users.
Setting Up Socket.io with Express and Angular
Socket.io is a powerful library for real-time communication between the server and the client. It simplifies working with WebSockets and provides fallback options for clients that do not support WebSockets. In this section, we'll set up Socket.io with an Express server for backend communication and integrate it with an Angular frontend to enable real-time updates between the client and server.
1. Installing and Setting Up Socket.io in Express
To get started, you first need to install
socket.io
on the server-side (Express) and set it up to handle WebSocket connections. Here's how you can install and configure it:# Install Express and Socket.io npm install express socket.io
After installing the necessary packages, you can set up the Express server with Socket.io:
const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIo(server); // Serve static files (if needed) app.use(express.static('public')); // Handle client connection io.on('connection', (socket) => { console.log('A client connected'); // Send a welcome message to the client socket.emit('message', 'Welcome to the server!'); // Listen for messages from the client socket.on('send-message', (msg) => { console.log('Received message:', msg); // Broadcast the message to all connected clients io.emit('message', msg); }); // Handle client disconnection socket.on('disconnect', () => { console.log('A client disconnected'); }); }); // Start the server server.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
In this example, the server listens for incoming WebSocket connections and sends a message to the connected client. It also listens for messages from the client and broadcasts them to all connected clients.
2. Setting Up Socket.io in Angular
To integrate Socket.io on the Angular frontend, you need to install the
socket.io-client
package:# Install Socket.io client in Angular project npm install socket.io-client
Once installed, you can integrate Socket.io into your Angular application by creating a service to manage the WebSocket connection:
import { Injectable } from '@angular/core'; import { Socket } from 'socket.io-client'; @Injectable({ providedIn: 'root' }) export class WebSocketService { private socket: Socket; constructor() { this.socket = io('http://localhost:3000'); // Server URL } // Listen to messages from the server onMessage() { return new Observable((observer) => { this.socket.on('message', (message) => { observer.next(message); }); }); } // Send a message to the server sendMessage(message: string) { this.socket.emit('send-message', message); } }
The
WebSocketService
manages the connection to the server, listens for incoming messages, and sends messages to the server.3. Using Socket.io in an Angular Component
To use the WebSocket service in an Angular component, you can inject it into the component and listen for messages or send messages to the server:
import { Component, OnInit } from '@angular/core'; import { WebSocketService } from './web-socket.service'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', styleUrls: ['./chat.component.css'] }) export class ChatComponent implements OnInit { message: string; messages: string[] = []; constructor(private webSocketService: WebSocketService) {} ngOnInit() { // Listen for messages from the server this.webSocketService.onMessage().subscribe((message: string) => { this.messages.push(message); }); } // Send a message to the server sendMessage() { if (this.message.trim()) { this.webSocketService.sendMessage(this.message); this.message = ''; // Clear the input field } } }
In this example, the component listens for messages from the server and displays them in a list. It also provides a text input for the user to send messages to the server.
4. HTML Template for the Chat Component
The HTML template for the chat component can be used to display the messages and provide an input field for sending messages:
Chat
{{ msg }}
This template binds the input field to the
message
variable and displays the list of messages received from the server.5. Running the Application
To run the application, follow these steps:
- Start the Express server by running
node server.js
. - Start the Angular frontend by running
ng serve
. - Open
http://localhost:4200
in your browser to view the Angular app.
Once the server and frontend are running, you can open multiple browser tabs to simulate real-time communication. Messages sent from one client will be broadcasted to all other clients connected to the server.
6. Conclusion
By setting up Socket.io with Express and Angular, you can easily implement real-time communication in your web applications. Socket.io handles the complexities of WebSocket communication and provides fallback mechanisms, making it a reliable choice for building chat applications, live notifications, and other real-time features.
Creating a Simple Real-Time Chat Application
In this section, we will create a simple real-time chat application using Socket.io. The application will allow multiple users to connect and send messages to each other in real-time. We will use Express.js for the server and Angular for the frontend to demonstrate how to establish a WebSocket connection and communicate in real-time.
1. Setting Up the Backend with Express and Socket.io
We will first set up the Express server and integrate Socket.io for handling WebSocket connections. The server will listen for incoming connections, accept messages from clients, and broadcast those messages to all connected clients.
// server.js const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIo(server); // Serve static files (optional) app.use(express.static('public')); // Handle incoming WebSocket connections io.on('connection', (socket) => { console.log('A client connected'); // Listen for messages from the client socket.on('sendMessage', (msg) => { console.log('Received message:', msg); // Broadcast the message to all connected clients io.emit('newMessage', msg); }); // Handle disconnection socket.on('disconnect', () => { console.log('A client disconnected'); }); }); // Start the server server.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
In the above code, the server listens for a
sendMessage
event, receives the message from the client, and broadcasts it to all connected clients using thenewMessage
event. The server is set to run on port 3000.2. Setting Up the Frontend with Angular
Next, we will integrate Socket.io into the Angular application. We will create a service to manage the WebSocket connection and send/receive messages. We will also create a component to handle the chat interface.
# Install Socket.io client in Angular npm install socket.io-client
Once the package is installed, we will create a service to manage the WebSocket connection:
// web-socket.service.ts import { Injectable } from '@angular/core'; import { Observable } from 'rxjs'; import { Socket } from 'socket.io-client'; @Injectable({ providedIn: 'root' }) export class WebSocketService { private socket: Socket; constructor() { this.socket = io('http://localhost:3000'); // Connect to the server } // Listen for incoming messages onMessage(): Observable
{ return new Observable((observer) => { this.socket.on('newMessage', (message: string) => { observer.next(message); }); }); } // Send a message to the server sendMessage(message: string): void { this.socket.emit('sendMessage', message); } } This service manages the connection to the server and provides methods to send and receive messages. The
onMessage()
method listens for incoming messages from the server, while thesendMessage()
method sends messages to the server.3. Creating the Chat Component
The chat component will display the chat messages and allow users to send new messages. We will bind the input field to a variable and listen for messages using the WebSocket service:
// chat.component.ts import { Component, OnInit } from '@angular/core'; import { WebSocketService } from './web-socket.service'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', styleUrls: ['./chat.component.css'] }) export class ChatComponent implements OnInit { message: string = ''; // Store the current message messages: string[] = []; // Store the list of messages constructor(private webSocketService: WebSocketService) {} ngOnInit(): void { // Listen for new messages this.webSocketService.onMessage().subscribe((message: string) => { this.messages.push(message); // Add the new message to the list }); } // Send a message to the server sendMessage(): void { if (this.message.trim()) { this.webSocketService.sendMessage(this.message); // Send message via WebSocket this.message = ''; // Clear the input field after sending } } }
The component listens for new messages using the
WebSocketService
and displays them in a list. It also provides an input field to allow users to send new messages.4. Creating the HTML Template
The HTML template for the chat component will display the messages and provide an input field for users to type and send messages:
Real-Time Chat
{{ msg }}
The input field is bound to the
message
variable, and the messages are displayed in a loop using the*ngFor
directive. When the user clicks the "Send" button, the message is sent to the server via WebSocket.5. Running the Application
- Start the server by running
node server.js
. - Start the Angular frontend by running
ng serve
. - Open
http://localhost:4200
in your browser to view the chat application.
With both the server and frontend running, open multiple browser tabs to simulate multiple users. Messages sent from one tab will be broadcasted to all other open tabs in real-time.
6. Conclusion
In this tutorial, we built a simple real-time chat application using Socket.io with Express.js and Angular. Socket.io allows for real-time bidirectional communication between the server and clients, making it a perfect fit for chat applications. By combining the power of Express and Angular, we were able to create a fully functional chat app with live message updates.
Protecting Against SQL Injection and NoSQL Injection
SQL Injection and NoSQL Injection are types of security vulnerabilities that allow attackers to manipulate the queries sent to your database, potentially gaining unauthorized access to sensitive data. This section explains how to protect your applications from these types of injections, both for SQL-based and NoSQL databases.
1. Understanding SQL Injection
SQL injection occurs when an attacker is able to manipulate an SQL query by injecting malicious SQL code into user input fields. This can allow attackers to view or alter the contents of your database, or even execute administrative commands.
2. Preventing SQL Injection
To protect against SQL injection, follow these best practices:
- Use Prepared Statements and Parameterized Queries: This is the most effective way to prevent SQL injection. Prepared statements separate SQL logic from the data input, ensuring that user input is treated as data rather than executable code.
- Escape User Input: If you are constructing queries manually, ensure that user input is properly escaped, which means that special characters are treated as literal characters rather than part of the SQL query.
- Limit Database Permissions: Restrict the database account's permissions to only the operations needed by your application (e.g., SELECT, INSERT). Avoid giving excessive permissions, such as DROP or DELETE, unless absolutely necessary.
- Use ORM (Object Relational Mapper) Frameworks: ORM frameworks abstract SQL queries and help prevent injection risks. Popular frameworks like Sequelize (Node.js) and Hibernate (Java) can help you interact with databases without manually writing SQL queries.
- Validate and Sanitize Input: Always validate input to ensure it matches the expected format (e.g., numbers, emails) and sanitize input to remove unwanted characters.
3. Example: Preventing SQL Injection with Parameterized Queries
Here’s how you can prevent SQL injection when working with MySQL in Node.js using the
mysql2
package and parameterized queries:// Node.js example using mysql2 with parameterized queries const mysql = require('mysql2'); // Create a connection const connection = mysql.createConnection({ host: 'localhost', user: 'root', password: 'password', database: 'mydb' }); // Using parameterized queries to prevent SQL injection const username = 'someuser'; const password = 'somepassword'; connection.execute( 'SELECT * FROM users WHERE username = ? AND password = ?', [username, password], // Parameters are automatically sanitized (err, results) => { if (err) { console.error(err); return; } console.log(results); } );
In this example, the
execute()
function ensures that the user-provided values forusername
andpassword
are treated as parameters, protecting against SQL injection.4. Understanding NoSQL Injection
NoSQL injection is a similar attack vector that targets NoSQL databases such as MongoDB. In NoSQL injection, attackers manipulate NoSQL queries to access or modify data. This usually occurs when user input is directly inserted into NoSQL queries without proper validation or sanitization.
5. Preventing NoSQL Injection
To prevent NoSQL injection, follow these best practices:
- Use Parameterized Queries: Just like with SQL injection, use parameterized queries and avoid directly inserting user input into NoSQL queries. Many NoSQL databases, including MongoDB, support parameterized queries.
- Input Validation: Always validate user input to ensure it is in the correct format before using it in queries. For example, if you expect a number, ensure the input is a valid number.
- Sanitize Input: Sanitize user input by removing special characters that could be interpreted as part of the query. For example, avoid inserting user input directly into MongoDB query objects.
- Use NoSQL Libraries with Safety Features: Use libraries and frameworks that abstract the database queries and automatically protect against injection attacks. For example, Mongoose for MongoDB automatically escapes query parameters.
- Limit Privileges: Just like with SQL, limit the privileges of the account accessing the NoSQL database. Ensure the account has the minimum required permissions.
6. Example: Preventing NoSQL Injection in MongoDB
Here’s an example of how to prevent NoSQL injection in MongoDB using Mongoose:
// Node.js example using Mongoose to prevent NoSQL injection const mongoose = require('mongoose'); // Define a User schema const userSchema = new mongoose.Schema({ username: String, password: String }); const User = mongoose.model('User', userSchema); // Using parameterized query to prevent NoSQL injection const username = 'someuser'; const password = 'somepassword'; // Mongoose query with safe parameters User.findOne({ username: username, password: password }) .then(user => { console.log(user); }) .catch(err => { console.error(err); });
In this example, Mongoose automatically prevents NoSQL injection by safely handling user input. The
findOne()
method accepts the parameters as an object, ensuring that user input is sanitized.7. General Tips for Preventing SQL and NoSQL Injection
- Always use prepared or parameterized queries.
- Validate and sanitize user input to ensure it is in the expected format.
- Use ORM/ODM libraries that abstract raw SQL or NoSQL queries.
- Limit database permissions to reduce the impact of a successful injection attack.
- Enable logging to detect unusual or malicious query patterns.
8. Conclusion
SQL injection and NoSQL injection are serious security risks that can have devastating consequences if not properly mitigated. By using parameterized queries, validating and sanitizing user input, and employing ORM/ODM libraries, you can effectively protect your application from these vulnerabilities. Always follow security best practices to safeguard sensitive data and ensure the integrity of your database.
Setting Up CORS in Express.js
CORS (Cross-Origin Resource Sharing) is a security feature implemented by web browsers to prevent unauthorized access to resources from different origins. When building APIs or web applications, you may need to allow cross-origin requests, especially when your frontend and backend are hosted on different domains or ports. This section explains how to set up CORS in an Express.js application.
1. What is CORS?
CORS is a mechanism that allows web browsers to make requests to a domain other than the one that served the original web page. For example, your frontend might be hosted on
http://localhost:4200
, but your API is hosted onhttp://localhost:3000
. Without proper CORS configuration, the browser will block these requests for security reasons.2. Installing the CORS Middleware
To enable CORS in an Express.js application, you can use the
cors
middleware. This package provides an easy way to set up CORS for your API. First, install thecors
package:npm install cors
3. Basic CORS Setup
After installing the
cors
package, you can set it up in your Express.js application. Here's how to enable CORS for all routes and origins:// Import required modules const express = require('express'); const cors = require('cors'); const app = express(); // Enable CORS for all routes and origins app.use(cors()); // Example route app.get('/api/data', (req, res) => { res.json({ message: 'Hello from the API' }); }); // Start the server app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); });
In this example, the
app.use(cors())
statement enables CORS for all routes and origins. This means any frontend, regardless of its origin, can make requests to your Express.js API.4. Configuring CORS for Specific Origins
You can also configure CORS to only allow requests from specific origins. For example, if you want to allow requests only from
http://localhost:4200
, you can pass an options object to thecors
middleware:// Enable CORS for a specific origin const corsOptions = { origin: 'http://localhost:4200', // Allow only this origin }; app.use(cors(corsOptions)); // Example route app.get('/api/data', (req, res) => { res.json({ message: 'Hello from the API' }); });
With this setup, only requests from
http://localhost:4200
will be allowed to access your API. All other origins will be blocked.5. Enabling CORS for Multiple Origins
If you want to allow multiple specific origins to access your API, you can pass an array of origins or a function to the
origin
property:// Enable CORS for multiple origins const corsOptions = { origin: ['http://localhost:4200', 'http://example.com'], // Allow multiple origins }; app.use(cors(corsOptions)); // Example route app.get('/api/data', (req, res) => { res.json({ message: 'Hello from the API' }); });
In this setup, requests from both
http://localhost:4200
andhttp://example.com
will be allowed. Other origins will be blocked.6. Enabling CORS for Dynamic Origins
Sometimes, you might want to allow CORS for origins dynamically based on the request. You can do this by using a function for the
origin
property:// Enable CORS dynamically based on the origin const corsOptions = { origin: (origin, callback) => { if (origin === 'http://localhost:4200' || origin === 'http://example.com') { callback(null, true); // Allow the origin } else { callback(new Error('Not allowed by CORS')); // Reject the origin } }, }; app.use(cors(corsOptions)); // Example route app.get('/api/data', (req, res) => { res.json({ message: 'Hello from the API' }); });
This dynamic approach allows you to conditionally allow or reject origins based on the incoming request.
7. Handling Preflight Requests
When making requests with certain HTTP methods (such as
PUT
,DELETE
, etc.) or custom headers, browsers send a preflight request (an HTTPOPTIONS
request) to check if the actual request is allowed. CORS middleware automatically handles preflight requests by responding with the necessary headers.If you need to configure specific behavior for preflight requests, you can customize the response headers in your CORS middleware settings:
// Configure CORS to handle preflight requests const corsOptions = { origin: 'http://localhost:4200', methods: ['GET', 'POST', 'PUT'], // Allowed HTTP methods allowedHeaders: ['Content-Type'], // Allowed headers }; app.use(cors(corsOptions)); // Example route app.get('/api/data', (req, res) => { res.json({ message: 'Hello from the API' }); });
In this example, only the
GET
,POST
, andPUT
methods are allowed fromhttp://localhost:4200
, and only theContent-Type
header is accepted in the request.8. Conclusion
Setting up CORS in an Express.js application is essential for enabling cross-origin requests in a secure and controlled manner. By using the
cors
middleware, you can easily configure your API to allow requests from trusted origins, specify allowed HTTP methods and headers, and handle preflight requests. This ensures that your application is both secure and accessible to the frontend.Implementing CSRF Protection
Cross-Site Request Forgery (CSRF) is an attack where a malicious actor tricks a user into performing unwanted actions on a web application in which they are authenticated. To prevent CSRF attacks, you need to implement CSRF protection in your web applications. This section explains how to implement CSRF protection in an Express.js application.
1. What is CSRF?
CSRF attacks occur when an attacker tricks a user into making a request (e.g., form submission, API call) on a website where the user is authenticated. This can result in unintended actions such as changing account details, transferring funds, or performing other sensitive operations. CSRF exploits the trust that a web application has in the user's browser.
2. CSRF Protection Strategy
To protect your Express.js application from CSRF attacks, you can implement a CSRF token-based strategy. The idea is to generate a unique token for each session and include it in the requests made by the client. The server then verifies that the token sent with the request matches the one stored in the session or cookie. If the tokens match, the request is processed; otherwise, it is rejected.
3. Installing CSRF Protection Middleware
To implement CSRF protection in Express.js, we can use the
csurf
middleware. First, install thecsurf
package:npm install csurf
4. Setting Up CSRF Protection Middleware
Once the
csurf
package is installed, you can set up CSRF protection middleware in your Express.js application. Here’s how to do it:// Import required modules const express = require('express'); const csrf = require('csurf'); const session = require('express-session'); const app = express(); // Set up session middleware to store CSRF token app.use(session({ secret: 'your-secret-key', resave: false, saveUninitialized: true })); // Set up CSRF protection middleware const csrfProtection = csrf({ cookie: true }); // Example route to get CSRF token app.get('/form', csrfProtection, (req, res) => { res.send(` `); }); // Example route to handle form submission app.post('/submit', csrfProtection, (req, res) => { res.send('Form successfully submitted!'); }); // Start the server app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); });
In this example, the CSRF token is generated by
req.csrfToken()
and included in the form as a hidden input field. The CSRF token is then validated when the form is submitted. If the tokens don’t match, the request will be rejected.5. Protecting API Routes
If you are building an API and want to protect POST, PUT, and DELETE requests, you can apply the CSRF protection middleware to these routes as well. Here’s an example of protecting API routes:
// Protecting API routes with CSRF protection app.post('/api/data', csrfProtection, (req, res) => { res.json({ message: 'Data successfully received' }); });
In this case, any POST request to the
/api/data
route will require the CSRF token to be sent with the request. If the token is missing or invalid, the server will respond with an error.6. Sending CSRF Token with AJAX Requests
If you are sending requests via AJAX (e.g., with
fetch
oraxios
), you need to include the CSRF token in the request headers. Here’s an example usingaxios
:import axios from 'axios'; // Get the CSRF token from the hidden input field or a cookie const csrfToken = document.querySelector('meta[name="csrf-token"]').getAttribute('content'); // Send an AJAX request with the CSRF token axios.post('/api/data', { data: 'example' }, { headers: { 'csrf-token': csrfToken } }) .then(response => { console.log(response.data); }) .catch(error => { console.error(error); });
This example assumes the CSRF token is stored in a
meta
tag or cookie and includes it in theheaders
of the AJAX request. The server will verify the token before processing the request.7. Handling CSRF Token Expiration
CSRF tokens may expire after a certain period. If you want to handle token expiration, you can configure the
csurf
middleware to automatically expire tokens after a specified time. You can also handle expired tokens by checking for the error and responding appropriately:// Handle CSRF token expiration error app.use((err, req, res, next) => { if (err.code === 'EBADCSRFTOKEN') { res.status(403).send('CSRF token expired or invalid'); } else { next(err); } });
This middleware captures the
EBADCSRFTOKEN
error and responds with a 403 status code and an error message if the CSRF token is invalid or expired.8. Conclusion
Implementing CSRF protection in your Express.js application is crucial for safeguarding your users from malicious attacks. By using the
csurf
middleware, you can easily generate and validate CSRF tokens to ensure that requests are coming from legitimate sources. This adds an important layer of security to your web application and protects sensitive user data.Securely Storing Passwords with bcrypt.js
Storing passwords securely is one of the most important aspects of web security. Plain-text passwords are vulnerable to theft if an attacker gains access to your database. To prevent this, you should hash passwords before storing them.
bcrypt.js
is a popular library for hashing passwords and securely comparing them during authentication. This section explains how to securely store and compare passwords usingbcrypt.js
in your Node.js application.1. What is bcrypt.js?
bcrypt.js
is a JavaScript implementation of the bcrypt password hashing algorithm. It is designed to be slow in order to prevent brute-force and dictionary attacks. The algorithm includes a salting mechanism, which ensures that even if two users have the same password, their hashed password will be different.2. Installing bcrypt.js
To use
bcrypt.js
in your Node.js application, you first need to install it. Run the following command to install the package:npm install bcryptjs
3. Hashing a Password
To securely store a password, you need to hash it using
bcrypt.js
. The hashing process involves creating a salt (a random string) and combining it with the password before applying the bcrypt algorithm. Here's how to hash a password:// Import bcryptjs const bcrypt = require('bcryptjs'); // Function to hash a password function hashPassword(password) { const saltRounds = 10; // Number of salt rounds bcrypt.hash(password, saltRounds, (err, hash) => { if (err) { console.error('Error hashing password:', err); } else { console.log('Hashed password:', hash); } }); } // Example usage hashPassword('mySecurePassword');
In this example, the password is hashed using 10 salt rounds. The more salt rounds you use, the more computationally expensive the hashing process will be, making it harder for attackers to guess the password.
4. Storing the Hashed Password
Once the password is hashed, you can store the resulting hash in your database instead of the plain-text password. Here's an example of how you might store the hash in a MongoDB database using Mongoose:
// Import necessary modules const mongoose = require('mongoose'); const bcrypt = require('bcryptjs'); // Define a user schema const userSchema = new mongoose.Schema({ username: { type: String, required: true }, password: { type: String, required: true } }); // Pre-save hook to hash password before saving to the database userSchema.pre('save', function(next) { const user = this; if (user.isModified('password')) { bcrypt.hash(user.password, 10, (err, hash) => { if (err) return next(err); user.password = hash; next(); }); } else { next(); } }); // Create a user model const User = mongoose.model('User', userSchema); // Example usage: create a new user const newUser = new User({ username: 'johndoe', password: 'mySecurePassword' }); newUser.save() .then(user => console.log('User saved:', user)) .catch(err => console.error('Error saving user:', err));
In this example, the password is hashed before being saved to the database using the
pre('save')
middleware in Mongoose. This ensures that the password is never stored in plain text.5. Comparing a Password with a Hash
When a user logs in, you need to compare the entered password with the stored hashed password in the database. You can do this using
bcrypt.compare()
. Here's an example of how to compare a password with a hash:// Function to compare a password with its hash function comparePassword(password, hashedPassword) { bcrypt.compare(password, hashedPassword, (err, isMatch) => { if (err) { console.error('Error comparing passwords:', err); } else if (isMatch) { console.log('Password is correct'); } else { console.log('Password is incorrect'); } }); } // Example usage const storedHash = '$2a$10$0I0jQ0bWcJ6J9Bz8LezpgO4BopX0fQg3f7l8NdVQ5z2J72z1Jfmyu'; // Example hash comparePassword('mySecurePassword', storedHash);
In this example,
bcrypt.compare()
is used to compare the entered password with the stored hash. If the passwords match, the user is authenticated successfully.6. Handling Errors and Optimizing Performance
While hashing passwords, you might encounter errors such as hash generation failure or comparison failure. Be sure to handle these errors gracefully in your application. Additionally, consider optimizing the hashing process by using a higher number of salt rounds if the application demands higher security. However, remember that more salt rounds increase the time it takes to hash the password, which could impact performance.
7. Conclusion
Using
bcrypt.js
is an effective way to securely store passwords in your Node.js application. By hashing passwords before storing them and comparing them during authentication, you can greatly improve the security of your application and protect sensitive user data from unauthorized access.Preparing a MEAN Stack Application for Production
When you're ready to deploy your MEAN (MongoDB, Express.js, Angular, Node.js) stack application to production, there are several important steps to take to ensure its performance, security, and scalability. This section walks through key steps to prepare your MEAN stack application for a successful production deployment.
1. Optimize Angular for Production
Before deploying your Angular application to production, you should optimize it for better performance. Angular provides built-in tools to do this, such as tree-shaking, minification, and ahead-of-time (AOT) compilation. Here's how to build your Angular app for production:
ng build --prod
The
--prod
flag enables production optimizations, such as minification, dead code elimination, and AOT compilation. This results in smaller bundle sizes and faster loading times for your application.2. Configure Environment Variables
Using environment variables is a good practice for managing configuration settings in production. You should separate your development and production environments. For example, you may want different database URLs, API keys, and authentication settings for each environment.
// Example of environment configuration in Angular export const environment = { production: true, apiUrl: 'https://api.example.com' };
In your Angular project, you can configure different environments by modifying the
environment.ts
andenvironment.prod.ts
files. Similarly, in your Node.js/Express application, you can use libraries likedotenv
to manage environment variables:// Example .env file for Node.js DB_URI=mongodb://localhost:27017/mydb SECRET_KEY=mySuperSecretKey
Ensure that sensitive information such as API keys and database URLs are stored in environment variables and are not hard-coded in your application.
3. Enable Security Features in Express
Express is a powerful framework, but it's important to secure your application before deploying it to production. Some essential security measures to implement include:
- Helmet: A middleware that helps secure your Express app by setting various HTTP headers.
- Rate Limiting: Protect your application from brute-force attacks by limiting the number of requests a user can make within a certain time frame.
- Data Validation and Sanitization: Ensure that user input is validated and sanitized to prevent injection attacks.
Here’s how to use
helmet
andexpress-rate-limit
in your Express app:const express = require('express'); const helmet = require('helmet'); const rateLimit = require('express-rate-limit'); const app = express(); // Use helmet to secure HTTP headers app.use(helmet()); // Rate limiter middleware to limit requests const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // Limit each IP to 100 requests per windowMs }); app.use(limiter); app.listen(3000, () => console.log('App running on port 3000'));
By adding
helmet
and setting up rate limiting, you can significantly improve the security of your application.4. Optimize MongoDB for Production
For production environments, you should also optimize MongoDB. Some key considerations include:
- Indexing: Ensure that your MongoDB collections have proper indexes for frequently queried fields to improve performance.
- Connection Pooling: Enable connection pooling to reduce the overhead of establishing connections to the database.
- Replica Sets: Set up MongoDB replica sets for high availability and fault tolerance in production.
Here’s an example of how to connect to MongoDB with connection pooling in your Node.js app:
const mongoose = require('mongoose'); mongoose.connect(process.env.DB_URI, { useNewUrlParser: true, useUnifiedTopology: true, poolSize: 10, // Set connection pool size }) .then(() => console.log('Connected to MongoDB')) .catch(err => console.error('MongoDB connection error:', err));
5. Configure Logging
In production, it’s crucial to have proper logging to track errors and monitor the application’s behavior. You can use logging libraries like
winston
ormorgan
for logging requests and errors.const winston = require('winston'); const logger = winston.createLogger({ level: 'info', transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'app.log' }) ] }); logger.info('This is an info message'); logger.error('This is an error message');
By using
winston
, you can log application events and errors in both the console and a file for future reference.6. Set Up a Reverse Proxy with Nginx
When deploying your MEAN stack application to production, it's a good idea to set up a reverse proxy with Nginx. This will allow you to forward HTTP requests to your Node.js server and enable HTTPS for secure communication.
Here’s an example of how to set up Nginx as a reverse proxy:
server { listen 80; server_name example.com; location / { proxy_pass http://localhost:3000; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
This configuration forwards incoming requests on port 80 to your Node.js server running on port 3000.
7. Use HTTPS in Production
It’s important to secure your application with HTTPS in production. You can obtain an SSL certificate from a trusted certificate authority or use tools like Let’s Encrypt to get a free certificate. Once you have the certificate, configure your server (e.g., Nginx or Apache) to serve your app over HTTPS.
8. Deploy to a Cloud Provider
Once your application is ready for production, you can deploy it to a cloud provider like AWS, DigitalOcean, or Google Cloud. These platforms offer various tools to manage your app's deployment, scaling, and monitoring. Be sure to automate deployments with tools like Docker or Kubernetes for better management and scalability.
9. Set Up Monitoring and Alerts
To ensure that your application runs smoothly in production, you should set up monitoring and alerts. Use tools like
PM2
for process management, or services likeNew Relic
orDatadog
for application monitoring. Set up alerts for critical issues like high CPU usage, memory usage, or application crashes.10. Conclusion
Preparing a MEAN stack application for production involves optimizing the frontend and backend, securing your app, configuring production settings, and ensuring high availability and scalability. By following these steps, you can ensure that your application performs well and remains secure in a production environment.
Using PM2 to Run a Node.js Server in Production
PM2 is a popular process manager for Node.js applications that simplifies running, managing, and monitoring your server in a production environment. It provides features like process monitoring, auto-restarts, load balancing, and easy deployment. This guide explains how to use PM2 to run a Node.js server in production.
1. Install PM2
To use PM2, you need to install it globally on your system. Use npm to install PM2:
npm install -g pm2
Once installed, verify the installation by running:
pm2 --version
2. Start Your Node.js Server with PM2
To run your Node.js server using PM2, navigate to your project directory and start your application:
pm2 start server.js
Replace
server.js
with the name of your application's main file. PM2 will start your server and keep it running, even if the application crashes or the system restarts.3. Manage PM2 Processes
PM2 provides commands to manage your application processes. Here are some commonly used commands:
- List running processes:
- Stop a process:
- Restart a process:
- Delete a process:
pm2 list
pm2 stop
pm2 restart
pm2 delete
4. Enable Auto-Restart on Server Reboot
To ensure your Node.js server restarts automatically after a system reboot, use the following command to generate a startup script:
pm2 startup
Follow the instructions displayed by PM2 to enable the script. Then, save your current process list so that PM2 restarts them on reboot:
pm2 save
5. Monitor Your Application
PM2 provides real-time monitoring and logs for your application:
- View real-time logs:
- Monitor resource usage:
pm2 logs
pm2 monit
6. Load Balancing with PM2
PM2 allows you to scale your application by running multiple instances to handle more traffic. Use the following command to scale your application:
pm2 scale
For example, to scale an application to 4 instances:
pm2 scale server 4
7. Deploying Applications with PM2
PM2 also simplifies deployment with its ecosystem file. Create a file named
ecosystem.config.js
with your application's configuration:module.exports = { apps: [ { name: 'my-app', script: 'server.js', instances: 'max', exec_mode: 'cluster', env: { NODE_ENV: 'development', }, env_production: { NODE_ENV: 'production', }, }, ], };
Deploy your application using the ecosystem file:
pm2 start ecosystem.config.js --env production
8. Conclusion
Using PM2 to run your Node.js server in production ensures better performance, reliability, and scalability. It simplifies process management, application monitoring, and deployment, making it an essential tool for Node.js developers.
Deploying to Heroku, AWS, or DigitalOcean
Deploying your application to a cloud provider is an essential step in making it accessible to users worldwide. This guide covers deployment to three popular platforms: Heroku, AWS, and DigitalOcean. Follow these steps to get your application live.
1. Deploying to Heroku
Heroku is a platform-as-a-service (PaaS) that makes deployment simple and quick.
Steps:
- Install the Heroku CLI: Download and install the CLI from the Heroku CLI documentation.
- Login to Heroku:
- Create a Heroku app:
- Push your code to Heroku:
- Access your application: Visit the URL provided by Heroku, such as
https://your-app-name.herokuapp.com
.
heroku login
heroku create
git push heroku main
2. Deploying to AWS
AWS offers various services for deployment, including Elastic Beanstalk and EC2.
Deploying to AWS Elastic Beanstalk:
- Install the AWS CLI: Download and install the CLI from the AWS CLI documentation.
- Configure the CLI: Set up your credentials using:
- Initialize Elastic Beanstalk:
- Create an environment and deploy:
- Access your application: Elastic Beanstalk will provide a public URL for your application.
aws configure
eb init
eb create
Deploying to an AWS EC2 Instance:
- Launch an EC2 instance: Go to the AWS Management Console and launch an instance with a suitable configuration.
- SSH into your instance:
- Install Node.js and Nginx: Set up your environment to host your application.
- Upload your application: Use SCP or Git to transfer your application to the instance.
- Run your application: Use a process manager like PM2 or run it manually.
ssh -i your-key.pem ec2-user@your-instance-ip
3. Deploying to DigitalOcean
DigitalOcean offers a virtual private server (VPS) solution with its Droplets.
Steps:
- Create a Droplet: Log in to the DigitalOcean dashboard and create a Droplet with your desired configuration.
- SSH into your Droplet:
- Set up the environment:
- Install Node.js:
- Install Nginx:
- Transfer your application: Use SCP or Git to upload your application files.
- Configure Nginx: Set up a reverse proxy to forward traffic to your Node.js application.
- Run your application: Use PM2 or another process manager to ensure it stays online.
ssh root@your-droplet-ip
sudo apt update sudo apt install nodejs npm
sudo apt install nginx
4. Conclusion
Each platform has its unique advantages and suits different use cases. Heroku is ideal for quick deployments, AWS offers extensive features for scalability, and DigitalOcean provides flexibility for a VPS setup. Choose the platform that best fits your application's requirements and budget.
Using Nginx as a Reverse Proxy for Node.js
Nginx is a powerful web server that can act as a reverse proxy for your Node.js applications, providing features such as load balancing, SSL termination, and caching. This guide explains how to configure Nginx to serve your Node.js application.
1. Prerequisites
- A server with Nginx installed. If not installed, use:
- A running Node.js application (e.g., on port 3000).
- Domain name (optional but recommended).
sudo apt update sudo apt install nginx
2. Basic Nginx Configuration
Create a new Nginx server block to act as a reverse proxy for your Node.js application.
Steps:
- Navigate to Nginx configuration directory:
- Create a new configuration file:
- Add the following configuration:
- Enable the configuration:
- Test the configuration:
- Restart Nginx:
cd /etc/nginx/sites-available
sudo nano your-domain.conf
server { listen 80; server_name your-domain.com www.your-domain.com; location / { proxy_pass http://localhost:3000; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
sudo ln -s /etc/nginx/sites-available/your-domain.conf /etc/nginx/sites-enabled/
sudo nginx -t
sudo systemctl restart nginx
3. Adding SSL (Optional but Recommended)
Secure your Node.js application using HTTPS with a free SSL certificate from Let's Encrypt.
Steps:
- Install Certbot:
- Obtain and configure SSL:
- Verify SSL configuration: Test your HTTPS setup by visiting
https://your-domain.com
. - Enable auto-renewal: Certbot automatically renews SSL certificates. Ensure it's working by running:
sudo apt install certbot python3-certbot-nginx
sudo certbot --nginx -d your-domain.com -d www.your-domain.com
sudo certbot renew --dry-run
4. Verifying the Setup
To ensure everything works as expected, visit your domain or server's IP in a browser. If the Node.js application is correctly set up and running, you should see it served through Nginx.
5. Troubleshooting
- Check Nginx logs:
- Ensure Node.js is running: Verify your Node.js application is active using
pm2
orsystemctl
.
sudo tail -f /var/log/nginx/error.log
6. Conclusion
Using Nginx as a reverse proxy for your Node.js application enhances performance, security, and scalability. With the optional addition of SSL, your application will be ready for production environments.
Configuring Environment Variables for Security
Environment variables are a crucial part of managing application configurations securely. They enable you to keep sensitive data like API keys, database credentials, and secret keys out of your source code. This section covers how to configure and use environment variables securely in your Node.js or Angular applications.
1. Why Use Environment Variables?
- Prevents sensitive information from being hardcoded in your application.
- Allows different configurations for development, testing, and production environments.
- Easy to update without changing the application code.
2. Setting Up Environment Variables in Node.js
In Node.js, you can use the built-in
process.env
object to access environment variables.Steps:
- Install the
dotenv
package: - Create a
.env
file: In the root directory of your project, create a file named.env
. - Add environment variables:
- Load the variables in your application:
npm install dotenv
# .env file DB_HOST=localhost DB_USER=root DB_PASS=securepassword API_KEY=your_api_key
// Load environment variables require('dotenv').config(); const dbHost = process.env.DB_HOST; const apiKey = process.env.API_KEY; console.log(`Database Host: ${dbHost}`); console.log(`API Key: ${apiKey}`);
Ensure that the
.env
file is added to your.gitignore
file to prevent it from being pushed to version control:# Add .env to .gitignore .env
3. Using Environment Variables in Angular
In Angular applications, environment variables are typically stored in the
src/environments
folder.Steps:
- Create environment files: The Angular CLI generates
environment.ts
andenvironment.prod.ts
files by default. - Add configuration values:
- Use the variables in your application:
// src/environments/environment.ts export const environment = { production: false, apiUrl: 'http://localhost:3000/api' }; // src/environments/environment.prod.ts export const environment = { production: true, apiUrl: 'https://yourdomain.com/api' };
import { environment } from '../environments/environment'; export class AppComponent { apiUrl = environment.apiUrl; constructor() { console.log('API URL:', this.apiUrl); } }
4. Best Practices for Environment Variables
- Never hardcode sensitive information: Always use environment variables for secrets.
- Keep the
.env
file out of version control: Add it to.gitignore
. - Use separate files for different environments: For example,
.env.dev
,.env.test
, and.env.prod
. - Use secure storage for production secrets: Tools like AWS Secrets Manager or HashiCorp Vault are ideal for managing secrets securely.
- Validate environment variables: Use a library like
joi
to validate that required variables are set.
5. Conclusion
By using environment variables, you can securely manage sensitive information and configurations across different environments. Following best practices ensures that your application is both secure and maintainable.
Caching with Redis or In-Memory Solutions
Caching is a powerful technique to improve the performance and scalability of your applications by storing frequently accessed data in a faster storage medium. This section covers how to set up caching using Redis and in-memory solutions in Node.js applications.
1. What is Caching?
Caching temporarily stores data for quick access, reducing the need to fetch the same data repeatedly from a slower source, such as a database or an external API. It helps in:
- Reducing latency and improving response times.
- Decreasing the load on your database or API.
- Enhancing the scalability of your application.
2. Setting Up Redis for Caching
Redis is a popular, open-source, in-memory data store that is ideal for caching.
Steps:
- Install Redis: Install Redis on your system or use a hosted service like AWS ElastiCache or Redis Cloud.
- Install the Redis client library for Node.js:
- Connect to Redis:
- Implement caching:
# On Linux or Mac sudo apt install redis-server # Start Redis redis-server
npm install redis
const redis = require('redis'); // Create a Redis client const client = redis.createClient(); client.on('connect', () => { console.log('Connected to Redis'); }); client.on('error', (err) => { console.error('Redis error:', err); });
Here’s an example of caching API responses:
const express = require('express'); const axios = require('axios'); const app = express(); const client = redis.createClient(); const CACHE_DURATION = 3600; // Cache duration in seconds app.get('/data', async (req, res) => { const cacheKey = 'apiData'; // Check Redis cache client.get(cacheKey, async (err, cachedData) => { if (cachedData) { console.log('Cache hit'); return res.json(JSON.parse(cachedData)); } console.log('Cache miss'); try { const response = await axios.get('https://api.example.com/data'); const data = response.data; // Save data to cache client.setex(cacheKey, CACHE_DURATION, JSON.stringify(data)); res.json(data); } catch (error) { res.status(500).json({ error: 'Failed to fetch data' }); } }); }); app.listen(3000, () => console.log('Server running on port 3000'));
3. Using In-Memory Caching
In-memory caching stores data in the application’s memory. While faster than Redis for local access, it is limited by the memory of the application server and is not suitable for distributed systems.
Example:
const cache = {}; app.get('/data', async (req, res) => { const cacheKey = 'apiData'; // Check in-memory cache if (cache[cacheKey]) { console.log('Cache hit'); return res.json(cache[cacheKey]); } console.log('Cache miss'); try { const response = await axios.get('https://api.example.com/data'); const data = response.data; // Save data to cache cache[cacheKey] = data; res.json(data); } catch (error) { res.status(500).json({ error: 'Failed to fetch data' }); } });
4. Best Practices for Caching
- Cache strategically: Cache data that is frequently accessed and changes infrequently.
- Set an expiration time: Always define a TTL (time-to-live) to prevent stale data.
- Invalidate cache when necessary: Clear cache after data updates to maintain consistency.
- Secure your Redis instance: Use authentication and restrict access using firewalls or private networks.
- Monitor cache usage: Track cache performance and hit/miss ratios.
5. Conclusion
Caching with Redis or in-memory solutions can significantly enhance the performance and scalability of your applications. Redis is ideal for distributed systems, while in-memory caching is suitable for single-server setups. By following best practices, you can ensure efficient and secure caching in your application.
Using Compression Middleware in Express.js
Compression middleware is used in Express.js applications to reduce the size of the response body. This can significantly improve the performance of your application, especially for users with slower network connections. By compressing responses, you reduce the amount of data that needs to be transferred, leading to faster load times and improved user experience.
1. What is Compression Middleware?
Compression middleware in Express.js uses gzip or Brotli compression to shrink the size of HTTP responses. It is particularly useful for compressing text-based content like HTML, CSS, JavaScript, and JSON.
2. Installing Compression Middleware
To use compression middleware in your Express.js application, you need to install the
compression
package.# Install compression middleware npm install compression
3. Setting Up Compression Middleware
Once installed, you can include the compression middleware in your Express.js application. Here's how to set it up:
const express = require('express'); const compression = require('compression'); const app = express(); // Enable compression middleware app.use(compression()); // Example route app.get('/', (req, res) => { res.send('This response is compressed!'); }); app.listen(3000, () => console.log('Server running on port 3000'));
With this setup, all responses from your server will be compressed automatically.
4. Configuring Compression Middleware
The
compression()
function accepts an options object to customize its behavior. Some of the commonly used options include:- threshold: Minimum response size (in bytes) to compress. Default is 1,024 bytes.
- filter: A custom function to decide whether a response should be compressed.
Here’s an example of configuring the compression middleware:
app.use(compression({ threshold: 0, // Compress all responses filter: (req, res) => { if (req.headers['x-no-compression']) { // Skip compression if this header is present return false; } return compression.filter(req, res); } }));
5. Verifying Compression
To ensure that your responses are compressed, use browser developer tools or a tool like Postman. Look for the following headers in the response:
Content-Encoding
: Indicates the compression algorithm used (e.g., gzip or br).Content-Length
: Shows the reduced size of the response.
Example Response Headers:
Content-Encoding: gzip Content-Length: 2345
6. Best Practices for Using Compression Middleware
- Use compression for text-based responses like HTML, CSS, JavaScript, and JSON.
- Exclude already compressed files like images, videos, and PDFs.
- Set an appropriate
threshold
to avoid compressing very small responses. - Test the impact of compression on your server's CPU usage and response time.
- Combine compression with other performance optimizations like caching for better results.
7. Conclusion
Using compression middleware in Express.js is a simple yet effective way to enhance the performance of your application. By reducing response sizes, you can improve load times and user experience, especially for clients with limited bandwidth. Make sure to configure the middleware appropriately to maximize its benefits.
Lazy Loading and Ahead-of-Time Compilation in Angular
Angular applications can benefit significantly from performance optimizations such as lazy loading and Ahead-of-Time (AOT) compilation. These techniques help reduce load times and enhance the overall user experience. This section explains how to implement lazy loading and AOT compilation in Angular applications.
1. Lazy Loading in Angular
Lazy loading allows you to load modules only when they are needed, reducing the initial bundle size and improving load times for your application.
Steps to Implement Lazy Loading:
Step 1: Create a Feature Module
Generate a feature module using the Angular CLI:
ng generate module feature --route feature --module app.module
This command creates a feature module with routing configured for lazy loading.
Step 2: Configure Routes
Ensure the lazy-loaded module is correctly configured in the
AppRoutingModule
:import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) }, { path: '', redirectTo: '/home', pathMatch: 'full' } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule {}
With this configuration, the feature module will be loaded only when the user navigates to the
/feature
route.Step 3: Verify Lazy Loading
Use browser developer tools to verify that the feature module is loaded as a separate bundle when navigating to its route.
2. Ahead-of-Time (AOT) Compilation
AOT compilation precompiles your Angular templates and components during the build process, resulting in faster rendering, smaller bundle sizes, and early detection of template errors.
Steps to Enable AOT Compilation:
Step 1: Build with AOT
By default, Angular CLI enables AOT in production builds. Use the following command to create a production build:
ng build --prod
This command automatically compiles your application with AOT.
Step 2: Verify AOT Compilation
Inspect the generated JavaScript files in the
/dist
folder. AOT compilation produces optimized and precompiled JavaScript code for templates.Step 3: Enable AOT in Development (Optional)
You can enable AOT in development mode for testing purposes by running:
ng serve --aot
3. Benefits of Lazy Loading and AOT
- Lazy Loading: Reduces initial load time by splitting the application into smaller bundles.
- AOT Compilation: Improves application performance and security by precompiling templates and detecting errors early.
4. Best Practices
- Organize your application into feature modules to maximize the benefits of lazy loading.
- Use AOT in production to ensure optimal performance and avoid runtime template errors.
- Combine lazy loading with preloading strategies to balance performance and user experience.
5. Conclusion
By implementing lazy loading and Ahead-of-Time compilation, you can significantly enhance the performance and maintainability of your Angular application. These techniques are essential for building scalable and efficient web applications that deliver a seamless user experience.
Query Optimization in MongoDB
Query optimization in MongoDB ensures efficient data retrieval and better performance. MongoDB provides various tools and techniques to optimize queries, making it suitable for handling large datasets and complex operations. This section covers strategies for optimizing queries in MongoDB.
1. Understanding Query Execution
MongoDB uses a query planner to determine the most efficient way to execute a query. You can analyze query performance using the
explain()
method, which provides details about the query execution plan.// Example: Analyzing a query execution plan db.collection.find({ age: { $gt: 30 } }).explain("executionStats");
The
executionStats
option provides detailed information about the query, including the number of documents scanned and execution time.2. Indexing for Faster Queries
Indexes are crucial for optimizing query performance. Without indexes, MongoDB performs a full collection scan, which is inefficient for large datasets.
Creating an Index
Use the
createIndex
method to create an index on a field:// Creating an index on the "age" field db.collection.createIndex({ age: 1 });
Here,
1
indicates ascending order, and-1
indicates descending order.Compound Indexes
For queries involving multiple fields, use compound indexes:
// Creating a compound index on "name" and "age" db.collection.createIndex({ name: 1, age: -1 });
Compound indexes optimize queries that filter or sort by multiple fields.
3. Query Projection
To reduce the amount of data retrieved, use projection to select only the fields you need:
// Query with projection db.collection.find({ age: { $gt: 30 } }, { name: 1, age: 1 });
This query retrieves only the
name
andage
fields, reducing the data transferred.4. Using Query Operators Efficiently
MongoDB provides a wide range of query operators. Use them efficiently to optimize queries:
- $eq: For equality checks.
- $in: To match any value from a list.
- $exists: To check for the existence of a field.
// Example: Using $in operator db.collection.find({ status: { $in: ["active", "pending"] } });
5. Aggregation Framework
The aggregation framework is a powerful tool for performing complex data transformations and calculations. Use it to optimize queries that require grouping, filtering, or sorting.
// Example: Aggregation pipeline db.collection.aggregate([ { $match: { age: { $gt: 30 } } }, { $group: { _id: "$status", total: { $sum: 1 } } }, { $sort: { total: -1 } } ]);
6. Avoiding Common Pitfalls
- Limit Full Scans: Always use indexes to prevent full collection scans.
- Optimize Regular Expressions: Use anchored patterns (e.g.,
^pattern
) to make regex queries faster. - Pagination: Use
limit
andskip
efficiently, or consider range queries for better performance.
7. Monitoring and Tuning
Use monitoring tools like MongoDB Compass or the MongoDB Atlas Performance Advisor to identify slow queries and suggest optimizations. Regularly analyze query performance and update indexes as needed.
8. Conclusion
Query optimization in MongoDB is essential for building high-performance applications. By using indexes, efficient query operators, projection, and the aggregation framework, you can significantly enhance query execution speed and reduce resource consumption. Regularly monitor and tune your queries to maintain optimal performance as your data grows.
Unit Testing with Jasmine and Karma in Angular
Unit testing ensures that individual components of your Angular application work as expected. Angular uses Jasmine as its testing framework and Karma as the test runner. This section covers how to set up and write unit tests with Jasmine and Karma in Angular.
1. Setting Up the Test Environment
When you create an Angular project using the Angular CLI, it comes pre-configured with Jasmine and Karma. The
angular.json
file contains configurations for running tests. To run tests, use the following command:ng test
This command launches Karma, which runs the tests in a browser and reports the results.
2. Writing a Unit Test for a Component
To write a unit test, Angular provides the
TestBed
utility. Here's an example of a simple unit test for a component:// Example: Testing a component import { ComponentFixture, TestBed } from '@angular/core/testing'; import { MyComponent } from './my.component'; describe('MyComponent', () => { let component: MyComponent; let fixture: ComponentFixture
; beforeEach(() => { TestBed.configureTestingModule({ declarations: [MyComponent], }).compileComponents(); fixture = TestBed.createComponent(MyComponent); component = fixture.componentInstance; fixture.detectChanges(); }); it('should create the component', () => { expect(component).toBeTruthy(); }); it('should display the correct title', () => { component.title = 'Hello, Angular!'; fixture.detectChanges(); const compiled = fixture.nativeElement as HTMLElement; expect(compiled.querySelector('h1')?.textContent).toContain('Hello, Angular!'); }); }); In this example, the
TestBed
sets up the testing module, and the tests verify the component's creation and DOM rendering.3. Testing Services
To test a service, use Angular's dependency injection system to provide the service in a test module:
// Example: Testing a service import { TestBed } from '@angular/core/testing'; import { MyService } from './my.service'; describe('MyService', () => { let service: MyService; beforeEach(() => { TestBed.configureTestingModule({ providers: [MyService], }); service = TestBed.inject(MyService); }); it('should return the correct value from a method', () => { const result = service.getData(); expect(result).toEqual('Expected Value'); }); });
This test ensures that the service's method behaves as expected.
4. Mocking Dependencies
When a component or service depends on other services, you can mock those dependencies to isolate the unit being tested:
// Example: Mocking a dependency import { TestBed } from '@angular/core/testing'; import { MyComponent } from './my.component'; import { MyService } from './my.service'; class MockMyService { getData() { return 'Mocked Value'; } } describe('MyComponent', () => { let component: MyComponent; beforeEach(() => { TestBed.configureTestingModule({ declarations: [MyComponent], providers: [{ provide: MyService, useClass: MockMyService }], }); const fixture = TestBed.createComponent(MyComponent); component = fixture.componentInstance; }); it('should use the mocked service', () => { expect(component.getData()).toEqual('Mocked Value'); }); });
Mocking dependencies ensures that the test focuses on the unit being tested and not its dependencies.
5. Running and Debugging Tests
To debug a test, run Karma in watch mode by using:
ng test --watch
You can set breakpoints in your test files or source files to debug the application using browser developer tools.
6. Code Coverage
Angular CLI provides built-in support for code coverage analysis. To generate a coverage report, use:
ng test --code-coverage
The coverage report is generated in the
coverage/
folder. Open theindex.html
file in a browser to view the report.7. Best Practices
- Write tests for every component, service, and pipe to ensure comprehensive coverage.
- Keep tests isolated by mocking dependencies.
- Run tests frequently during development to catch issues early.
8. Conclusion
Unit testing with Jasmine and Karma ensures your Angular application is robust and free of regressions. By testing components, services, and other application parts, you can maintain a high level of code quality and reliability.
Testing REST APIs with Mocha and Chai
Mocha and Chai are powerful tools for testing REST APIs in Node.js applications. Mocha serves as a feature-rich testing framework, while Chai provides an assertion library to write expressive test cases. This section covers how to set up, write, and run tests for REST APIs using these tools.
1. Setting Up Mocha and Chai
To begin, install Mocha, Chai, and other necessary packages:
npm install --save-dev mocha chai chai-http
Here,
chai-http
is an extension to make HTTP requests and assertions for REST APIs.2. Creating a Test File
Create a test file in your project, such as
test/api.test.js
. Structure the file using Mocha'sdescribe
andit
blocks for organizing and writing test cases.3. Example: Testing a REST API
Below is an example of testing a simple REST API endpoint for managing users:
// Import dependencies const chai = require('chai'); const chaiHttp = require('chai-http'); const app = require('../app'); // Replace with the path to your Express app const expect = chai.expect; // Use chai-http chai.use(chaiHttp); describe('User API Tests', () => { // Test GET /users it('should retrieve all users', (done) => { chai.request(app) .get('/users') .end((err, res) => { expect(res).to.have.status(200); expect(res.body).to.be.an('array'); done(); }); }); // Test POST /users it('should create a new user', (done) => { const newUser = { name: 'John Doe', email: 'john@example.com' }; chai.request(app) .post('/users') .send(newUser) .end((err, res) => { expect(res).to.have.status(201); expect(res.body).to.have.property('id'); expect(res.body.name).to.equal('John Doe'); done(); }); }); // Test GET /users/:id it('should retrieve a single user by ID', (done) => { const userId = 1; // Replace with a valid ID from your database chai.request(app) .get(`/users/${userId}`) .end((err, res) => { expect(res).to.have.status(200); expect(res.body).to.have.property('id').that.equals(userId); done(); }); }); // Test DELETE /users/:id it('should delete a user by ID', (done) => { const userId = 1; // Replace with a valid ID from your database chai.request(app) .delete(`/users/${userId}`) .end((err, res) => { expect(res).to.have.status(200); expect(res.body).to.have.property('message').that.equals('User deleted'); done(); }); }); });
This example tests basic CRUD operations for a user API. Adjust the routes and data according to your application's structure.
4. Running the Tests
To run the tests, add the following script to your
package.json
file:"scripts": { "test": "mocha" }
Execute the tests using:
npm test
Mocha will run all test files in the
test
directory and display the results.5. Handling Asynchronous Tests
When testing asynchronous operations, use the
done
callback, as shown in the examples above. Alternatively, you can return a Promise:// Using Promises it('should retrieve all users', async () => { const res = await chai.request(app).get('/users'); expect(res).to.have.status(200); expect(res.body).to.be.an('array'); });
6. Best Practices
- Isolate the test environment by using a dedicated test database or mocking database calls.
- Use meaningful test data to cover various edge cases.
- Test both successful and failing scenarios, such as invalid input or server errors.
- Run tests regularly during development to catch issues early.
7. Conclusion
Testing REST APIs with Mocha and Chai ensures that your API works as intended and can handle different scenarios gracefully. By writing comprehensive tests, you improve the reliability and maintainability of your application.
End-to-End Testing with Protractor
End-to-end (E2E) testing ensures that your entire application works as expected by simulating real user interactions. Protractor is a powerful framework that helps you perform E2E testing for Angular applications. It provides easy integration with Selenium WebDriver and allows you to run tests in a real browser environment. This section covers how to set up and use Protractor for E2E testing in Angular applications.
1. Setting Up Protractor
To get started with Protractor, you need to install it as a development dependency:
npm install --save-dev protractor
Additionally, you need to install WebDriver, which is required for Protractor to interact with the browser:
npx webdriver-manager update
2. Configuring Protractor
Protractor is configured through a configuration file (e.g.,
protractor.conf.js
). This file defines how tests should run, which browser to use, and other important settings. Here's an example configuration:// protractor.conf.js exports.config = { directConnect: true, // Use a direct connection to the browser capabilities: { browserName: 'chrome', // Set the browser to Chrome }, framework: 'jasmine', // Use Jasmine as the testing framework specs: ['e2e/**/*.spec.js'], // Location of the test files onPrepare: () => { browser.manage().window().maximize(); // Maximize the browser window before tests }, };
In this configuration:
directConnect: true
tells Protractor to connect directly to the browser without using Selenium Server.capabilities
defines which browser to use (Chrome in this case).framework
specifies Jasmine as the testing framework.specs
points to the location of the test files.
3. Writing Protractor Tests
Protractor tests are written using Jasmine syntax. Here's an example of a simple test that opens a page and checks if the page title is correct:
// e2e/app.spec.js describe('My Angular App', () => { it('should have the correct title', () => { browser.get('http://localhost:4200'); // Navigate to the app expect(browser.getTitle()).toBe('My Angular App'); // Check the title }); });
This test opens the application in the browser and verifies that the page title matches "My Angular App".
4. Running Protractor Tests
To run the Protractor tests, use the following command:
npx protractor protractor.conf.js
This command will start Protractor, execute the tests in the specified configuration file, and report the results in the terminal.
5. Interacting with Web Elements
Protractor provides several methods to interact with web elements, such as
element()
,by
, and actions likeclick()
andsendKeys()
. Here's an example that tests a login form:// e2e/login.spec.js describe('Login Form', () => { it('should login successfully with valid credentials', () => { browser.get('http://localhost:4200/login'); // Navigate to the login page const usernameField = element(by.id('username')); const passwordField = element(by.id('password')); const submitButton = element(by.id('submit')); usernameField.sendKeys('testuser'); // Enter username passwordField.sendKeys('password123'); // Enter password submitButton.click(); // Click the submit button const successMessage = element(by.css('.success-message')); expect(successMessage.isDisplayed()).toBe(true); // Check if login was successful }); });
In this test, Protractor interacts with the form fields and clicks the submit button. It then checks if the success message is displayed.
6. Handling Asynchronous Operations
Protractor automatically handles asynchronous operations in Angular applications. You don't need to explicitly wait for elements to load; Protractor will wait for Angular to finish loading and for elements to become available. However, you can also use
browser.wait()
for custom waits:// e2e/delayed-action.spec.js describe('Delayed Action', () => { it('should wait for a delayed element', () => { browser.get('http://localhost:4200/delayed'); // Navigate to the page const delayedElement = element(by.id('delayedElement')); browser.wait(protractor.ExpectedConditions.visibilityOf(delayedElement), 5000); // Wait for the element to be visible expect(delayedElement.isDisplayed()).toBe(true); // Check if the element is displayed }); });
In this case, Protractor waits for the element to be visible before continuing with the test.
7. Running Tests in Multiple Browsers
Protractor supports running tests in multiple browsers simultaneously. You can configure this in the
capabilities
section of the Protractor configuration:// protractor.conf.js exports.config = { capabilities: [ { browserName: 'chrome', }, { browserName: 'firefox', } ], // Other configurations... };
This will allow Protractor to run tests in both Chrome and Firefox at the same time.
8. Conclusion
Protractor is an excellent tool for end-to-end testing of Angular applications. It integrates seamlessly with Angular, provides easy-to-use APIs for interacting with elements, and supports parallel execution for faster test runs. By using Protractor for E2E testing, you can ensure that your Angular application behaves as expected in real-world scenarios and provides a great user experience.
Using Jest for Fast Unit Tests in Node.js
Jest is a JavaScript testing framework that is widely used for unit testing, especially in Node.js applications. It provides a fast and efficient way to write and run tests with great built-in features like mocking, code coverage, and snapshot testing. This section covers how to set up and use Jest for unit testing in your Node.js applications.
1. Installing Jest
First, you need to install Jest as a development dependency in your Node.js project:
npm install --save-dev jest
After installation, you can configure Jest to run tests in your project.
2. Configuring Jest
To run Jest tests, you need to add a script in your
package.json
file. This script will allow you to run Jest with thenpm test
command:"scripts": { "test": "jest" }
With this configuration, you can run your tests using:
npm test
3. Writing Unit Tests with Jest
Jest makes writing unit tests simple and intuitive. Below is an example of a simple test suite for a function that adds two numbers:
// add.js (function to test) function add(a, b) { return a + b; } module.exports = add; // add.test.js (unit test for the function) const add = require('./add'); test('adds 1 + 2 to equal 3', () => { expect(add(1, 2)).toBe(3); });
In this example, the
add
function is being tested. Thetest()
function defines the test case, andexpect()
is used to assert the expected outcome. Jest will automatically run the test and report the result in the terminal.4. Running Jest Tests
To run your unit tests, execute the following command:
npm test
Jest will look for files with the
.test.js
suffix or files in the__tests__
directory and run all the test cases within them. The results will be displayed in the terminal.5. Mocking Dependencies
Jest allows you to mock dependencies to isolate the unit being tested. Here's an example of how to mock a database call in a Node.js application:
// database.js (mocked module) const getUser = (id) => { // Simulate a database call return { id, name: 'John Doe' }; }; module.exports = getUser; // userService.js (function that calls the database) const getUser = require('./database'); function getUserInfo(id) { return getUser(id); } module.exports = getUserInfo; // userService.test.js (unit test with mocking) jest.mock('./database'); // Mock the database module const getUserInfo = require('./userService'); const getUser = require('./database'); test('fetches user info from database', () => { // Mock the return value of the database function getUser.mockReturnValue({ id: 1, name: 'Jane Doe' }); expect(getUserInfo(1)).toEqual({ id: 1, name: 'Jane Doe' }); });
In this example, Jest’s
jest.mock()
function is used to mock thegetUser
function. The mock implementation returns a custom value, allowing us to test thegetUserInfo
function in isolation without hitting the actual database.6. Asynchronous Testing
Jest provides support for testing asynchronous code, such as promises or async/await functions. Here’s an example of testing an asynchronous function:
// fetchData.js (async function to test) function fetchData() { return new Promise((resolve) => { setTimeout(() => { resolve('data fetched'); }, 1000); }); } module.exports = fetchData; // fetchData.test.js (unit test for async function) const fetchData = require('./fetchData'); test('fetches data asynchronously', async () => { const data = await fetchData(); expect(data).toBe('data fetched'); });
In this case, the test is using
async/await
to handle the asynchronous operation. Jest will wait for the promise to resolve before making the assertion.7. Code Coverage
Jest has built-in code coverage reporting, which helps you track the percentage of your code that is covered by tests. To enable code coverage, simply run Jest with the
--coverage
flag:npm test -- --coverage
This will generate a code coverage report in the terminal and an HTML report in the
coverage/
directory. The report will show you which lines, functions, and branches are covered by your tests.8. Snapshot Testing
Jest also supports snapshot testing, which allows you to capture the output of a function or a component and compare it against a saved snapshot. If the output changes, Jest will notify you. Here’s an example:
// snapshot.js (function to test) function getUserInfo() { return { id: 1, name: 'John Doe' }; } module.exports = getUserInfo; // snapshot.test.js (snapshot test) const getUserInfo = require('./snapshot'); test('user info matches snapshot', () => { expect(getUserInfo()).toMatchSnapshot(); });
In this example, Jest will save the output of the
getUserInfo
function as a snapshot. If the function’s output changes in the future, Jest will notify you, helping you detect unintended changes in your code.9. Conclusion
Jest is a fast, reliable, and feature-rich testing framework that is well-suited for unit testing in Node.js applications. Its rich set of features, including mocking, asynchronous testing, code coverage, and snapshot testing, make it a great choice for ensuring the quality and correctness of your code. By integrating Jest into your development workflow, you can easily write and maintain unit tests for your Node.js applications, ensuring that they work as expected.
Integrating Payment Gateways (Stripe, PayPal)
Integrating payment gateways like Stripe and PayPal allows you to securely process payments on your website or application. In this section, we'll cover how to integrate both Stripe and PayPal into your Node.js application for seamless online transactions.
1. Integrating Stripe
Stripe is a popular payment gateway that allows you to process payments easily and securely. Follow these steps to integrate Stripe into your Node.js application.
1.1. Setting Up Stripe
To get started with Stripe, you need to create a Stripe account and get your API keys. Once you have the keys, install the Stripe Node.js library:
npm install stripe
Now, configure Stripe in your Node.js server by importing the Stripe package and initializing it with your secret key:
const stripe = require('stripe')('your-secret-key'); // Use your secret API key // Example route to create a payment intent app.post('/create-payment-intent', async (req, res) => { try { const paymentIntent = await stripe.paymentIntents.create({ amount: 1000, // Amount in cents currency: 'usd', payment_method_types: ['card'], }); res.send({ clientSecret: paymentIntent.client_secret, }); } catch (error) { res.status(500).send({ error: error.message }); } });
In this example, a Payment Intent is created when the route is accessed. The client secret is sent back to the front end, where it will be used to complete the payment.
1.2. Handling the Frontend (React Example)
On the frontend, you need to integrate Stripe's JavaScript library to handle the payment flow. First, install the Stripe.js library:
npm install @stripe/stripe-js
Then, create a component that initializes the Stripe Elements for a checkout form:
import React, { useState, useEffect } from 'react'; import { loadStripe } from '@stripe/stripe-js'; import { Elements, CardElement, useStripe, useElements } from '@stripe/react-stripe-js'; const stripePromise = loadStripe('your-public-key'); // Use your public API key const CheckoutForm = () => { const [clientSecret, setClientSecret] = useState(''); const stripe = useStripe(); const elements = useElements(); useEffect(() => { // Fetch the client secret from the server fetch('/create-payment-intent', { method: 'POST', }) .then((res) => res.json()) .then((data) => setClientSecret(data.clientSecret)); }, []); const handleSubmit = async (event) => { event.preventDefault(); if (!stripe || !elements) return; const { error, paymentIntent } = await stripe.confirmCardPayment(clientSecret, { payment_method: { card: elements.getElement(CardElement), }, }); if (error) { console.log('Payment failed:', error.message); } else if (paymentIntent.status === 'succeeded') { console.log('Payment succeeded:', paymentIntent); } }; return ( ); }; const Checkout = () => (
In the frontend code, we use Stripe’s React library to display a card input form. When the user submits the form, it sends the payment information to Stripe for processing, and the result is displayed in the console.
2. Integrating PayPal
PayPal is another widely used payment gateway. To integrate PayPal, you need a PayPal business account and your API credentials. Below is the guide for integrating PayPal into your Node.js application.
2.1. Setting Up PayPal
First, install the PayPal Node SDK:
npm install @paypal/checkout-server-sdk
Next, configure the PayPal environment using your client ID and secret key:
const paypal = require('@paypal/checkout-server-sdk'); const environment = new paypal.core.SandboxEnvironment( 'your-client-id', 'your-client-secret' ); const paypalClient = new paypal.core.PayPalHttpClient(environment);
2.2. Creating a PayPal Payment
Once the PayPal client is set up, you can create a payment route in your server. Below is an example of creating a payment with PayPal:
app.post('/pay', async (req, res) => { const orderPayload = { intent: 'CAPTURE', purchase_units: [{ amount: { currency_code: 'USD', value: '10.00', }, }], application_context: { return_url: 'http://localhost:3000/payment-success', cancel_url: 'http://localhost:3000/payment-cancelled', }, }; try { const request = new paypal.orders.OrdersCreateRequest(); request.requestBody(orderPayload); const order = await paypalClient.execute(request); res.json({ id: order.result.id }); } catch (error) { res.status(500).json(error); } });
This route creates a PayPal order with a payment amount of $10. When the user completes the payment, PayPal will redirect to either the success or cancellation URL.
2.3. Handling the Frontend (PayPal Buttons)
For the frontend, you need to include PayPal’s JavaScript SDK and create a PayPal button that users can click to initiate the payment process:
import React, { useEffect } from 'react'; const PayPalButton = () => { useEffect(() => { // Load the PayPal script const script = document.createElement('script'); script.src = 'https://www.paypal.com/sdk/js?client-id=your-client-id'; script.addEventListener('load', () => { window.paypal.Buttons({ createOrder: (data, actions) => { return actions.order.create({ purchase_units: [{ amount: { value: '10.00', }, }], }); }, onApprove: (data, actions) => { return actions.order.capture().then(function(details) { alert('Payment Successful: ' + details.payer.name.given_name); }); }, onError: (err) => { console.error('PayPal error:', err); }, }).render('#paypal-button-container'); }); document.body.appendChild(script); }, []); return ; }; export default PayPalButton;
In this frontend code, we dynamically load the PayPal SDK script and render the PayPal button. When the user clicks the button, it initiates the payment flow and handles both success and failure cases.
3. Conclusion
Integrating payment gateways such as Stripe and PayPal into your Node.js application allows you to securely process payments. Stripe provides a simple API for handling credit card payments, while PayPal offers a flexible solution for broader payment methods. By following the steps outlined above, you can easily integrate these payment gateways and offer a smooth checkout experience to your users.
Using Cloud Storage (AWS S3, Google Cloud Storage)
Cloud storage services like AWS S3 and Google Cloud Storage offer scalable, secure, and cost-effective solutions for storing and serving files. In this section, we'll explore how to integrate these cloud storage services into your application for uploading, managing, and retrieving files.
1. Using AWS S3 for File Storage
Amazon S3 (Simple Storage Service) is a scalable and highly available cloud storage service. It allows you to upload, store, and manage large amounts of data. Below is the guide for integrating S3 into your Node.js application.
1.1. Setting Up AWS S3
First, sign in to your AWS account and create an S3 bucket. After creating the bucket, generate your AWS access keys (Access Key ID and Secret Access Key) from the AWS IAM service. You will need these keys to authenticate API requests to S3.
1.2. Installing AWS SDK
To interact with AWS services, including S3, you’ll need to install the AWS SDK:
npm install aws-sdk
Then, configure the AWS SDK in your Node.js server:
const AWS = require('aws-sdk'); // Configure AWS SDK AWS.config.update({ accessKeyId: 'your-access-key-id', secretAccessKey: 'your-secret-access-key', region: 'us-east-1', // Replace with your bucket region }); const s3 = new AWS.S3();
1.3. Uploading Files to S3
Now that you’ve set up the AWS SDK, you can upload files to your S3 bucket. Here’s how to upload a file from your Node.js server:
const fs = require('fs'); const uploadFile = async (filePath, bucketName) => { const fileContent = fs.readFileSync(filePath); // Read the file const params = { Bucket: bucketName, Key: 'your-file-name.jpg', // File name in the bucket Body: fileContent, ACL: 'public-read', // Set file permissions }; try { const data = await s3.upload(params).promise(); console.log(`File uploaded successfully. ${data.Location}`); } catch (err) { console.error('Error uploading file:', err); } }; // Call the function to upload a file uploadFile('path/to/local/file.jpg', 'your-bucket-name');
This code reads a file from the local filesystem and uploads it to S3. You can modify the parameters to customize the file name and permissions.
1.4. Downloading Files from S3
To retrieve a file from S3, you can use the following code:
const downloadFile = async (bucketName, fileName) => { const params = { Bucket: bucketName, Key: fileName, // Name of the file to download }; try { const data = await s3.getObject(params).promise(); console.log('File downloaded successfully:', data.Body.toString()); } catch (err) { console.error('Error downloading file:', err); } }; // Call the function to download a file downloadFile('your-bucket-name', 'your-file-name.jpg');
This code fetches a file from S3 and prints its content. You can adapt the function to save the file locally or serve it directly to users.
2. Using Google Cloud Storage
Google Cloud Storage is another widely used cloud storage service. It offers similar functionality to AWS S3 but is integrated with the Google Cloud Platform services. Below is the guide for integrating Google Cloud Storage into your Node.js application.
2.1. Setting Up Google Cloud Storage
To use Google Cloud Storage, first create a Google Cloud Project and enable the Cloud Storage API. After that, create a storage bucket and generate your service account credentials (JSON format). You will need these credentials to authenticate your application.
2.2. Installing Google Cloud Storage Client
Install the Google Cloud Storage client library for Node.js:
npm install @google-cloud/storage
Then, configure the Google Cloud Storage client in your Node.js server:
const { Storage } = require('@google-cloud/storage'); const path = require('path'); // Instantiate a Google Cloud Storage client const storage = new Storage({ keyFilename: path.join(__dirname, 'path/to/your-service-account-file.json'), }); const bucket = storage.bucket('your-bucket-name');
2.3. Uploading Files to Google Cloud Storage
To upload files to Google Cloud Storage, use the following code:
const uploadFileToGCS = async (filePath) => { const options = { destination: 'your-file-name.jpg', // Destination file name public: true, // Set file permissions }; try { await bucket.upload(filePath, options); console.log('File uploaded to Google Cloud Storage'); } catch (err) { console.error('Error uploading file:', err); } }; // Call the function to upload a file uploadFileToGCS('path/to/local/file.jpg');
This code uploads a file from your local filesystem to Google Cloud Storage. You can modify the destination and permissions as needed.
2.4. Downloading Files from Google Cloud Storage
To retrieve a file from Google Cloud Storage, use the following code:
const downloadFileFromGCS = async (fileName) => { const options = { destination: 'path/to/downloaded/file.jpg', // Local path to save the file }; try { await bucket.file(fileName).download(options); console.log('File downloaded from Google Cloud Storage'); } catch (err) { console.error('Error downloading file:', err); } }; // Call the function to download a file downloadFileFromGCS('your-file-name.jpg');
This code downloads a file from Google Cloud Storage and saves it locally. You can also modify this code to serve the file directly to users.
3. Conclusion
Both AWS S3 and Google Cloud Storage are excellent choices for scalable, secure, and reliable file storage in the cloud. By following the steps outlined in this section, you can easily integrate either of these cloud storage services into your Node.js application for managing and serving files efficiently.
Integrating Email Services with Nodemailer
Nodemailer is a popular module for sending emails from a Node.js application. It supports multiple email services, including Gmail, SendGrid, Amazon SES, and others. In this section, we'll walk through how to set up and use Nodemailer to send emails through your preferred email service.
1. Installing Nodemailer
To get started, you need to install Nodemailer in your Node.js application. Run the following command to install it via npm:
npm install nodemailer
2. Sending Emails Using Nodemailer
Once Nodemailer is installed, you can use it to send emails. Below is an example of how to set up a basic email sending function:
const nodemailer = require('nodemailer'); // Create a transporter object using your email service's configuration const transporter = nodemailer.createTransport({ service: 'gmail', // You can replace this with your email service (e.g., 'sendgrid', 'outlook') auth: { user: 'your-email@gmail.com', // Your email address pass: 'your-email-password', // Your email password or app-specific password }, }); // Set up email data const mailOptions = { from: 'your-email@gmail.com', // Sender's email address to: 'recipient-email@example.com', // Recipient's email address subject: 'Test Email', // Subject line text: 'Hello, this is a test email sent using Nodemailer!', // Email body in plain text html: '
Hello, this is a test email sent using Nodemailer!
', // Optional: HTML version of the email body }; // Send the email transporter.sendMail(mailOptions, (error, info) => { if (error) { return console.log('Error:', error); } console.log('Email sent: ' + info.response); });This code sends a test email using Gmail as the email service. You can replace the Gmail configuration with other services like SendGrid, Amazon SES, or others as per your requirements.
3. Using SMTP with Nodemailer
If you want to use your own SMTP server (e.g., for custom email services or your web hosting provider), you can set up Nodemailer with SMTP as follows:
const transporter = nodemailer.createTransport({ host: 'smtp.your-email-provider.com', // SMTP server address port: 587, // Typically 587 for TLS, 465 for SSL secure: false, // Set to true if using SSL (Port 465) auth: { user: 'your-email@provider.com', // Your email address pass: 'your-email-password', // Your email password }, });
This configuration allows you to use an SMTP server to send emails. Adjust the SMTP server settings according to your email service provider's documentation.
4. Using Email Services (e.g., SendGrid, Amazon SES)
Nodemailer also works with third-party email services like SendGrid, Amazon SES, Mailgun, etc. Below is an example of how to configure Nodemailer with SendGrid:
const nodemailer = require('nodemailer'); const sendgridTransport = require('nodemailer-sendgrid-transport'); // Set up SendGrid transport const transporter = nodemailer.createTransport(sendgridTransport({ auth: { api_key: 'your-sendgrid-api-key', // Your SendGrid API key }, })); // Set up email data const mailOptions = { from: 'your-email@example.com', to: 'recipient-email@example.com', subject: 'Test Email from SendGrid', text: 'This email was sent through SendGrid using Nodemailer!', }; // Send the email transporter.sendMail(mailOptions, (error, info) => { if (error) { return console.log('Error:', error); } console.log('Email sent: ' + info.response); });
In this example, we used the `nodemailer-sendgrid-transport` module to connect Nodemailer with SendGrid's email service. Replace the `api_key` with your actual SendGrid API key.
5. Sending HTML and Attachments
If you need to send rich HTML emails or include attachments, Nodemailer makes it easy. Here's how you can send HTML content and add attachments:
const mailOptions = { from: 'your-email@example.com', to: 'recipient-email@example.com', subject: 'Test Email with HTML and Attachment', text: 'This is a plain text version of the email.', html: '
This is a test email with HTML content and an attachment!
', attachments: [ { filename: 'test.txt', // Attachment file name path: 'path/to/your/file.txt', // Path to the file }, ], }; // Send the email transporter.sendMail(mailOptions, (error, info) => { if (error) { return console.log('Error:', error); } console.log('Email sent: ' + info.response); });Here, we've added an attachment along with both plain text and HTML versions of the email body. You can include multiple attachments by adding them to the `attachments` array.
6. Handling Errors and Response
When sending an email using Nodemailer, it's important to handle errors and responses properly:
transporter.sendMail(mailOptions, (error, info) => { if (error) { console.error('Error sending email:', error); return; } console.log('Email sent: ' + info.response); });
In this example, we log errors and responses to the console. You can extend this to send error messages to your logging system or notify users about email delivery failures.
7. Conclusion
Nodemailer is a powerful and flexible email sending library for Node.js. It supports a wide variety of email services and allows you to send emails with text, HTML, and attachments. You can also integrate it with third-party services like SendGrid and Amazon SES for scalable email delivery.
By following the steps in this guide, you can easily integrate email services into your Node.js application and send emails programmatically for notifications, password resets, or any other purpose.