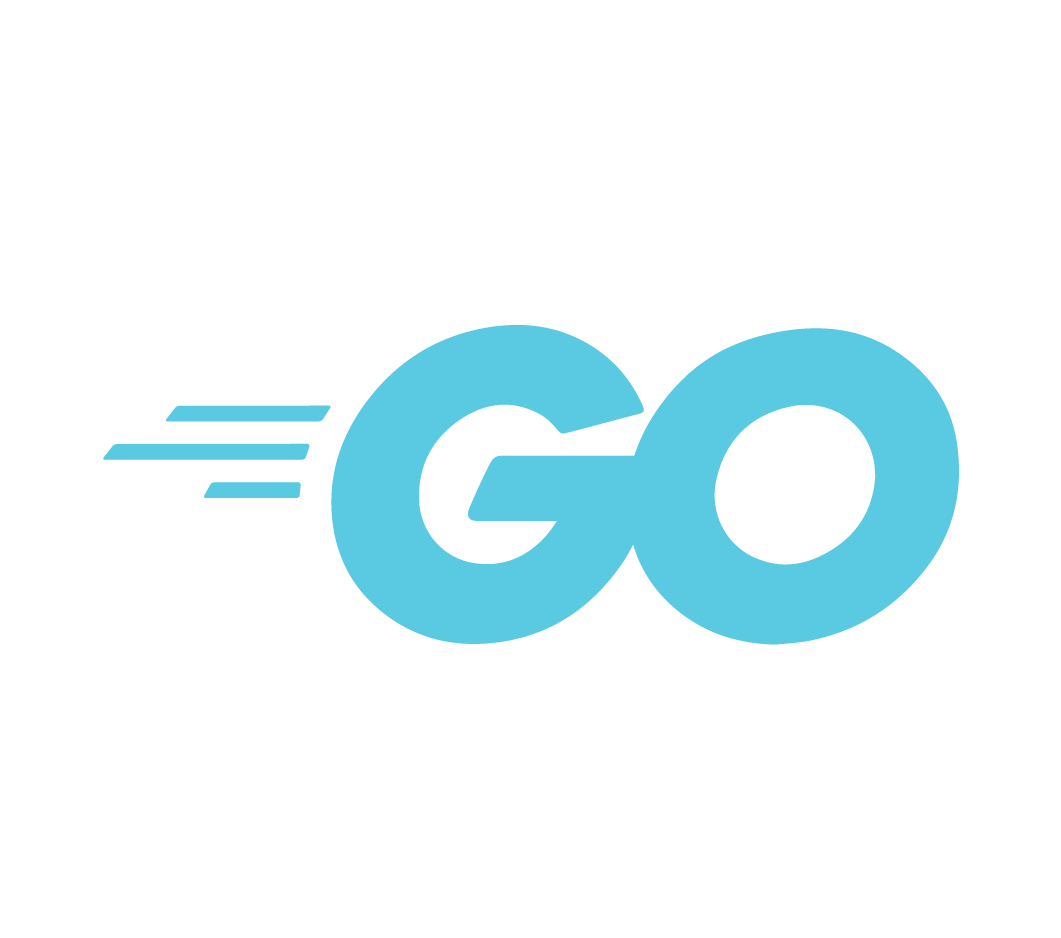
What is Go (Golang)?
1. Introduction to Go
Go, also known as Golang, is an open-source programming language developed by Google in 2009. It is designed to be simple, efficient, and scalable, making it a popular choice for building modern software solutions. Go was created by Robert Griesemer, Rob Pike, and Ken Thompson to address the limitations of existing programming languages while maintaining simplicity and high performance.
2. Key Features of Go
- Concurrent Programming: Go supports built-in concurrency with goroutines and channels, allowing developers to efficiently handle multiple tasks simultaneously.
- Statically Typed: Go is statically typed, meaning variables are bound to specific data types, which helps in catching errors at compile time.
- Garbage Collection: Go comes with automatic memory management through garbage collection, simplifying memory handling for developers.
- Simple Syntax: Go’s syntax is easy to learn and read, enabling developers to write clean and maintainable code.
- Cross-Platform Compilation: Go can compile code for multiple platforms, making it a great choice for building cross-platform applications.
- Standard Library: Go’s extensive standard library provides tools for networking, file I/O, cryptography, and more, reducing the need for external dependencies.
3. Why Use Go?
Go is widely used in various domains due to its unique features and advantages:
- Performance: Go offers performance close to that of C or C++ while being much simpler to use.
- Scalability: The language is designed to handle scalable systems, making it ideal for modern web services, cloud computing, and microservices architectures.
- Community and Ecosystem: Go has a growing community and ecosystem, with numerous libraries and tools available for developers.
- Ease of Deployment: Go programs compile into single, statically linked binaries, making deployment straightforward and efficient.
4. Applications of Go
Go is versatile and is used in various fields, such as:
- Web Development: Frameworks like Gin and Echo simplify building web applications.
- Cloud Computing: Go is a popular choice for building cloud-native applications and working with platforms like Kubernetes and Docker.
- Networking Tools: Go’s efficiency and networking libraries make it suitable for building network-related tools and services.
- Data Science: While not as common as Python, Go is gaining traction in data processing and analysis tasks.
- DevOps: Go is widely used for writing CLI tools and automation scripts for DevOps workflows.
5. Conclusion
Go is a powerful, efficient, and easy-to-learn programming language that bridges the gap between performance and simplicity. Whether you are building web applications, handling large-scale systems, or developing automation scripts, Go provides the tools and flexibility to create robust and scalable solutions. Its rising popularity and strong community support make it a valuable language for developers to learn and use in various projects.
Features of Go Programming Language
1. Simplicity
Go is designed with simplicity in mind, making it easy for developers to learn and use. Its clean syntax and minimalistic design reduce complexity and improve readability.
2. Concurrency Support
Go provides native support for concurrency through goroutines and channels. Goroutines are lightweight threads that enable efficient multitasking, making Go ideal for building scalable systems.
3. Performance
Go is a compiled language, offering performance similar to C and C++. Its efficient memory management and garbage collection ensure fast execution of programs.
4. Cross-Platform Compilation
Go allows developers to compile code for multiple platforms (e.g., Windows, macOS, Linux) without requiring platform-specific modifications. This feature simplifies deployment in diverse environments.
5. Rich Standard Library
The Go standard library includes a wide range of packages for tasks such as networking, file I/O, cryptography, and more, enabling developers to build applications without relying heavily on third-party libraries.
6. Static Typing
Go is a statically typed language, which means variables are bound to specific data types. This helps catch errors at compile time, enhancing code reliability and reducing runtime issues.
7. Garbage Collection
Go includes an efficient garbage collector that handles memory management automatically, freeing developers from the complexities of manual memory allocation and deallocation.
8. Built-in Testing Tools
Go comes with built-in tools for writing, running, and benchmarking tests. The `testing` package simplifies test creation, enabling developers to ensure code quality and reliability.
9. Package Management
Go supports modular programming with its package system. Developers can organize their code into reusable packages and manage dependencies effectively using tools like `go mod`.
10. Open Source
Go is an open-source language, supported by a vibrant community of developers. Its active ecosystem and frequent updates make it a reliable choice for modern software development.
11. Scalability
Go’s lightweight goroutines and efficient concurrency model make it highly scalable, enabling the development of robust systems capable of handling massive workloads.
12. Security
Go enforces strict compilation rules, reducing vulnerabilities. It also includes features like immutable strings and built-in support for cryptographic operations, enhancing application security.
13. Community and Ecosystem
Go has a growing community and ecosystem, with numerous third-party libraries, frameworks, and tools available to extend its capabilities and simplify development.
14. Fast Compilation
Go’s compiler is designed for speed, enabling developers to quickly build and test applications, which enhances the development workflow and productivity.
15. Cloud-Native Development
Go is widely adopted for cloud-native applications, with strong support for platforms like Kubernetes, Docker, and various cloud services, making it a top choice for modern software solutions.
16. Easy Deployment
Go programs compile into standalone binaries with no external dependencies, simplifying deployment and ensuring compatibility across environments.
Installing Go on Your Machine
1. Download Go
To install Go on your machine, start by downloading the appropriate installer for your operating system:
- Visit the official Go website: https://golang.org/dl/.
- Select the installer that matches your operating system (Windows, macOS, or Linux).
2. Installing Go on Windows
Follow these steps to install Go on a Windows machine:
- Run the downloaded MSI installer.
- Follow the on-screen instructions to complete the installation.
- During installation, the installer will set the Go environment variables and add Go to your system's PATH.
- Once installed, open a Command Prompt and type
go version
to verify the installation.
3. Installing Go on macOS
To install Go on macOS:
- Run the downloaded .pkg file.
- Follow the installation wizard to complete the setup.
- Ensure Go is added to your PATH by checking
/etc/paths
. - Open the terminal and type
go version
to verify the installation.
4. Installing Go on Linux
For Linux users, follow these steps:
- Download the tarball (.tar.gz file) for your system architecture from the Go website.
- Extract the tarball to
/usr/local
:sudo tar -C /usr/local -xzf go1.x.x.linux-amd64.tar.gz
- Add Go to your PATH by editing your shell profile (e.g.,
.bashrc
,.zshrc
):export PATH=$PATH:/usr/local/go/bin
- Reload your shell or run
source ~/.bashrc
. - Verify the installation by running
go version
in the terminal.
5. Setting Up Your Go Workspace
After installing Go, configure your workspace:
- Create a directory for your Go workspace, e.g.,
~/go
. - Set the
GOPATH
environment variable to point to this directory:export GOPATH=~/go
- Add the Go
bin
directory to your PATH:export PATH=$PATH:$GOPATH/bin
- Save the changes to your shell profile and reload your shell.
6. Testing Your Installation
Verify that Go is correctly installed and your workspace is set up:
- Create a new Go file in your workspace:
mkdir -p ~/go/src/hello cd ~/go/src/hello nano hello.go
- Add the following code to
hello.go
:package main import "fmt" func main() { fmt.Println("Hello, World!") }
- Run the program:
go run hello.go
- If you see "Hello, World!" printed, your Go installation is complete.
Setting Up the Go Development Environment
1. Install a Text Editor or IDE
To write Go code efficiently, choose a text editor or Integrated Development Environment (IDE) with Go support:
- Visual Studio Code (VS Code): A lightweight, popular editor with an excellent Go extension. Download it from https://code.visualstudio.com/.
- GoLand: A full-featured IDE by JetBrains specifically designed for Go development. Download it from https://www.jetbrains.com/go/.
- Sublime Text: A versatile text editor with Go syntax highlighting and plugins.
2. Install the Go Extension or Plugins
Enable Go-specific features like syntax highlighting, autocompletion, and debugging:
- For VS Code, install the Go extension from the Extensions Marketplace.
- For GoLand, most Go features are built-in. No additional plugins are required.
- For Sublime Text, install GoSublime or GoTools plugins via the Package Control.
3. Configure the Go Environment
Ensure your Go environment is properly configured:
- Verify your Go installation by running:
go version
- Set up the
GOPATH
environment variable:export GOPATH=~/go
Ensure this points to your Go workspace directory (default is
~/go
). - Add Go binaries to your system PATH:
export PATH=$PATH:/usr/local/go/bin:$GOPATH/bin
- Save these settings in your shell profile file (e.g.,
.bashrc
,.zshrc
) to persist them across sessions.
4. Install Essential Go Tools
Go provides a set of tools to enhance development:
- Install tools required by your IDE or editor:
go install golang.org/x/tools/...@latest
- Install gofmt for code formatting (bundled with Go by default).
- Install gopls (Go Language Server) for intelligent code completion and navigation:
go install golang.org/x/tools/gopls@latest
5. Testing the Environment
Verify that your development environment is working correctly:
- Create a new directory for your project:
mkdir -p ~/go/src/myproject
- Create a Go file:
cd ~/go/src/myproject nano main.go
- Add the following code:
package main import "fmt" func main() { fmt.Println("Go environment is set up!") }
- Run your program:
go run main.go
You should see the output:
Go environment is set up!
6. Debugging Go Code
Use debugging tools to troubleshoot your Go programs:
- In VS Code, use the built-in debugger after installing the Go extension.
- In GoLand, set breakpoints and use the debugger features built into the IDE.
- For command-line debugging, use Delve, the official debugger for Go:
go install github.com/go-delve/delve/cmd/dlv@latest
7. Setting Up Version Control
Use Git for version control in your Go projects:
- Initialize a Git repository in your project directory:
git init
- Create a
.gitignore
file to exclude unnecessary files:echo "bin/ pkg/ *.exe *.log" > .gitignore
- Commit your code:
git add . git commit -m "Initial commit"
8. Conclusion
With your Go development environment set up, you’re ready to start building Go applications. By leveraging an IDE or text editor, installing essential tools, and configuring your workspace, you can enhance your productivity and streamline your development process.
Understanding Go’s Compilation Process
1. Overview of Go Compilation
Go is a statically typed, compiled language known for its simplicity and speed. The compilation process converts Go source code into an executable binary, eliminating the need for a separate runtime environment. The compiled binaries are self-contained, including all necessary dependencies.
2. Steps in the Compilation Process
The Go compilation process involves the following steps:
- Parsing: The Go compiler parses the source code into an Abstract Syntax Tree (AST) by analyzing syntax and structure.
- Type Checking: The compiler verifies that all variables, functions, and expressions adhere to Go’s type system.
- Dependency Resolution: The compiler resolves all imported packages and includes their code in the compilation process.
- Code Optimization: The compiler optimizes the code for performance, such as inlining functions and reducing redundant operations.
- Code Generation: The optimized code is translated into machine-level instructions for the target platform.
- Linking: The compiler links all code, libraries, and dependencies into a single executable binary.
3. Compilation Commands
Go provides several commands to manage the compilation process:
go build
: Compiles the source code into a binary without executing it.go build main.go
go run
: Compiles and immediately runs the code.go run main.go
go install
: Compiles the code and installs the binary in the$GOPATH/bin
directory.go install
go clean
: Removes compiled binary files and cached build artifacts.go clean
4. Cross-Compilation
Go supports cross-compilation, allowing you to build binaries for different operating systems and architectures. Use the GOOS
and GOARCH
environment variables:
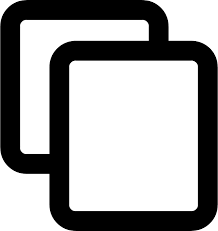
GOOS=linux GOARCH=amd64 go build main.go
In this example, the binary is compiled for a Linux 64-bit system, regardless of the host OS.
5. Compilation Optimization
Go’s compiler applies several optimizations to improve performance:
- Inlining: Small functions are replaced with their body to reduce function call overhead.
- Escape Analysis: Determines whether a variable can be allocated on the stack instead of the heap, reducing garbage collection overhead.
- Dead Code Elimination: Removes unused code to minimize binary size.
6. Benefits of Go’s Compilation Process
Go’s compilation process offers several advantages:
- Fast Compilation: The simplicity of Go’s syntax and the design of its compiler ensure fast build times.
- Static Binaries: Go produces self-contained binaries, simplifying deployment by eliminating external dependencies.
- Cross-Platform Support: Native support for cross-compilation enables building binaries for multiple platforms from a single machine.
- Portability: The resulting binaries can be run on any compatible system without requiring additional setup.
7. Common Issues and Debugging
While compiling Go code, you may encounter errors. Here are some tips for debugging:
- Syntax Errors: Check for typos or missing syntax elements in your code.
- Dependency Issues: Ensure all imported packages are installed and compatible with your Go version.
- Environment Variables: Verify that
GOPATH
andGOROOT
are correctly configured. - Verbose Output: Use the
-x
flag withgo build
for detailed build logs:go build -x main.go
8. Conclusion
Understanding Go’s compilation process is essential for efficient development and deployment. By leveraging Go’s fast and flexible compiler, you can build optimized, portable binaries for a wide range of platforms and applications.
Syntax and Structure of a Go Program
1. Overview
Go (or Golang) has a simple and clean syntax designed to promote readability and ease of use. It emphasizes simplicity and avoids unnecessary complexity. A typical Go program consists of packages, imports, and functions, with the main function being the entry point.
2. Basic Structure of a Go Program
A basic Go program includes the following elements:
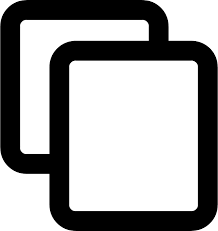
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Let’s break down the components:
- Package Declaration: Every Go file starts with a
package
declaration. Themain
package is required for the entry point of an executable program. - Imports: The
import
statement is used to include built-in or third-party packages. In this example, we import thefmt
package for formatted I/O. - Main Function: The
main()
function is the starting point of the program’s execution.
3. Key Syntax Elements
Here are some key syntax elements in Go:
Variables
Variables in Go can be declared with the var
keyword or using shorthand notation:
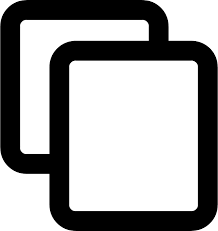
var name string = "Go Language"
age := 10
Constants
Constants are declared using the const
keyword:
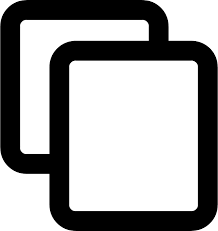
const Pi = 3.14
Functions
Functions are defined using the func
keyword:
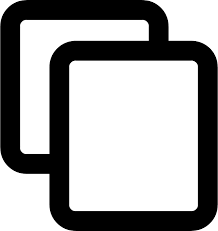
func add(a int, b int) int {
return a + b
}
Control Structures
Go supports standard control structures like if-else, for loops, and switch statements:
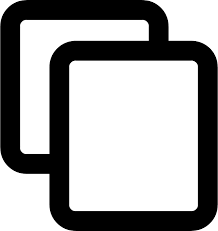
if x > 10 {
fmt.Println("x is greater than 10")
} else {
fmt.Println("x is 10 or less")
}
for i := 0; i < 5; i++ {
fmt.Println(i)
}
switch day := "Monday"; day {
case "Monday":
fmt.Println("Start of the week")
default:
fmt.Println("Another day")
}
Arrays and Slices
Go uses arrays and slices for handling collections:
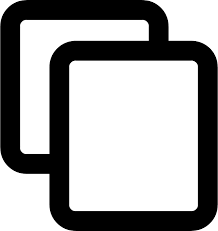
var arr [3]int = [3]int{1, 2, 3}
slice := []int{1, 2, 3}
4. Go’s Formatting Rules
Go enforces a consistent code style using the gofmt
tool, which automatically formats code according to Go’s standards. Some key rules include:
- No semicolons (
;
) are required; line breaks indicate the end of a statement. - Curly braces (
{}
) must be on the same line as the controlling statement. - Indentation uses tabs, not spaces.
5. Error Handling
Go uses explicit error handling instead of exceptions. The error
type is used to indicate and handle errors:
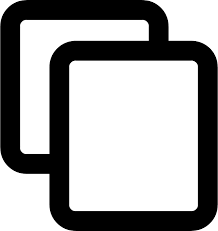
func divide(a, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
6. Example Program
Here’s a complete example of a Go program:
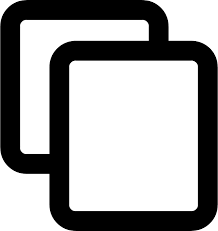
package main
import "fmt"
func main() {
name := "Go"
fmt.Printf("Welcome to %s programming!\n", name)
}
7. Conclusion
The syntax and structure of Go are designed for simplicity, readability, and efficiency. By understanding the basic elements and conventions, you can quickly write clean and idiomatic Go code for various applications.
Hello World in Go
1. Introduction
The "Hello, World!" program is the simplest example to get started with any programming language. In Go, it demonstrates the basic structure of a program, including the package declaration, imports, and the main function.
2. Writing the Program
Here is the complete "Hello, World!" program in Go:
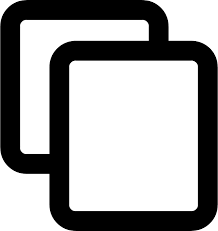
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
3. Explanation
Let’s break down the program:
- package main: Declares the package as
main
, which is required for an executable Go program. Themain
package contains the entry point of the program. - import "fmt": Imports the
fmt
package, which provides functions for formatted I/O operations. - func main(): The
main()
function is the entry point of the program. It is where the program starts execution. - fmt.Println("Hello, World!"): The
Println
function from thefmt
package prints the string "Hello, World!" to the console.
4. Running the Program
Follow these steps to run the program:
- Create the file: Save the program in a file named
hello.go
. - Open the terminal: Navigate to the directory where the file is saved.
- Run the program: Execute the following commands:
go run hello.go
Hello, World!
- Build the program: To create an executable, run:
go build hello.go
hello
), which you can run directly.
5. Key Points
- All Go programs start with a
package main
declaration for executables. - The
main()
function is mandatory for the program to run. - Go uses built-in packages like
fmt
to handle basic operations like printing to the console.
6. Conclusion
The "Hello, World!" program is a great starting point for learning Go. It introduces the essential components of a Go program and provides a foundation for exploring more advanced topics.
Declaring Variables and Constants in Go
1. Introduction
Variables and constants are fundamental to any programming language. In Go, variables are used to store data that can be changed during program execution, while constants store data that remains fixed. Go offers a variety of ways to declare variables and constants.
2. Declaring Variables
In Go, variables can be declared using the var
keyword or through shorthand declaration.
- Using
var
keyword:var name string name = "John"
- Declaring with initialization:
var age int = 30
- Type inference:
var score = 95.5 // Go infers the type as float64
- Shorthand declaration:
message := "Hello, Go!"
3. Declaring Multiple Variables
Go allows declaring multiple variables in a single statement:
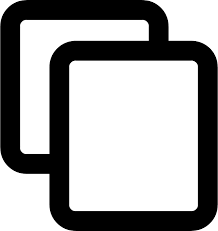
var x, y, z int = 1, 2, 3
You can also use shorthand for multiple variables:
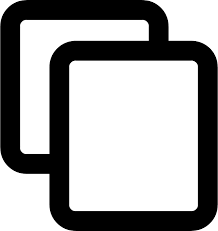
a, b, c := "Alice", "Bob", "Charlie"
4. Declaring Constants
Constants are declared using the const
keyword. They cannot be changed after declaration:
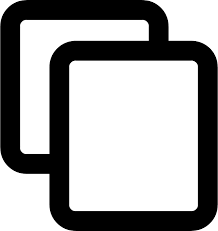
const Pi = 3.14159
Multiple constants can be declared in a group:
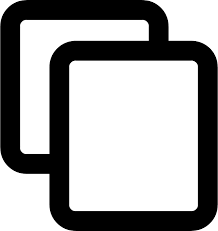
const (
Monday = "Mon"
Tuesday = "Tue"
)
5. Zero Values
If a variable is declared without an initial value, Go assigns a default zero value based on its type:
- Integer: 0
- Float: 0.0
- Boolean:
false
- String:
""
(empty string)
6. Example Program
Here’s a complete example that demonstrates variable and constant declaration:
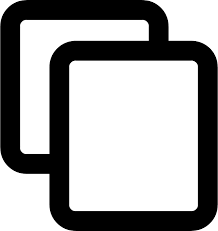
package main
import "fmt"
func main() {
// Variable declarations
var name string = "John"
age := 25
var x, y, z int = 1, 2, 3
// Constant declaration
const Pi = 3.14159
// Printing values
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Coordinates:", x, y, z)
fmt.Println("Value of Pi:", Pi)
}
7. Key Points
- Variables can be declared with or without initial values.
- Constants are immutable and must be assigned a value at declaration.
- Shorthand declaration
:=
is convenient but limited to function scope.
8. Conclusion
Understanding how to declare and use variables and constants in Go is crucial for writing efficient and readable code. With a strong type system and flexible declaration methods, Go ensures both simplicity and clarity in variable management.
Data Types in Go
1. Introduction
Data types in Go define the type of data a variable can hold. Go is a statically typed language, which means the data type of a variable must be known at compile time. Go provides a range of built-in data types to handle different types of data efficiently.
2. Basic Data Types
- Boolean: The
bool
type represents true or false values.var isActive bool = true
- Numeric: Go supports several numeric types:
- Integers:
int
,int8
,int16
,int32
,int64
- Unsigned Integers:
uint
,uint8
,uint16
,uint32
,uint64
- Floating-point:
float32
,float64
- Complex:
complex64
,complex128
var age int = 30 var salary float64 = 45000.50
- Integers:
- String: The
string
type represents a sequence of characters.var message string = "Hello, Go!"
3. Composite Data Types
Go provides composite data types to work with collections or more complex structures:
- Array: A fixed-size sequence of elements of the same type.
var numbers [5]int = [5]int{1, 2, 3, 4, 5}
- Slice: A dynamically-sized array.
var fruits []string = []string{"apple", "banana", "cherry"}
- Map: A collection of key-value pairs.
var studentGrades map[string]int = map[string]int{"Alice": 90, "Bob": 85}
- Struct: A composite data type that groups variables under one name.
type Person struct { Name string Age int }
4. Special Data Types
- Pointer: Holds the memory address of a variable.
var ptr *int
- Interface: Represents a set of method signatures.
var emptyInterface interface{}
5. Type Conversion
Go allows explicit type conversion between compatible types:
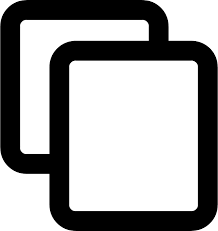
var x int = 42
var y float64 = float64(x)
6. Example Program
Here’s an example demonstrating various data types in Go:
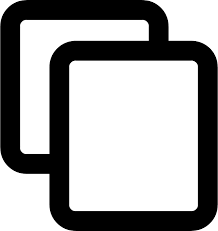
package main
import "fmt"
func main() {
// Basic types
var isActive bool = true
var age int = 30
var message string = "Hello, Go!"
// Composite types
var numbers [3]int = [3]int{1, 2, 3}
var fruits []string = []string{"apple", "banana"}
var studentGrades map[string]int = map[string]int{"Alice": 90, "Bob": 85}
// Print values
fmt.Println("Is Active:", isActive)
fmt.Println("Age:", age)
fmt.Println("Message:", message)
fmt.Println("Numbers:", numbers)
fmt.Println("Fruits:", fruits)
fmt.Println("Student Grades:", studentGrades)
}
7. Key Points
- Go is statically typed, and types must be declared or inferred at compile time.
- Composite types like slices, maps, and structs enable working with more complex data.
- Type conversion in Go is explicit and must be handled by the developer.
8. Conclusion
Understanding data types is essential for working effectively in Go. By leveraging its rich type system, you can write robust and efficient programs.
Type Inference and Type Conversion in Go
1. Introduction
Go provides mechanisms for both type inference and type conversion, making it easier to work with variables and ensure compatibility between types while maintaining type safety.
2. Type Inference
Type inference allows the Go compiler to automatically determine the type of a variable based on the value assigned to it. This is achieved using the shorthand :=
declaration syntax.
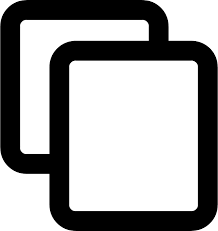
package main
import "fmt"
func main() {
// Type inference
message := "Hello, Go!" // Compiler infers the type as string
value := 42 // Compiler infers the type as int
pi := 3.14 // Compiler infers the type as float64
fmt.Println(message)
fmt.Println(value)
fmt.Println(pi)
}
Key Points:
- Type inference is only available within functions.
- It simplifies code by eliminating the need to explicitly declare variable types.
3. Type Conversion
Type conversion in Go is explicit, meaning you must manually convert one type to another when needed. This ensures type safety and prevents unexpected behavior.
3.1 Numeric Type Conversion
You can convert between numeric types like int
, float64
, etc., using explicit conversion syntax.
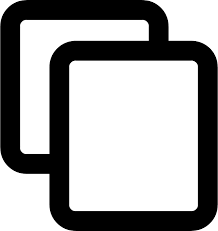
package main
import "fmt"
func main() {
var x int = 42
var y float64 = float64(x) // Convert int to float64
var z int = int(y) // Convert float64 to int (truncates the fractional part)
fmt.Println("x:", x, "y:", y, "z:", z)
}
3.2 String and Byte Conversion
Go allows conversion between strings and byte slices to facilitate encoding and decoding operations.
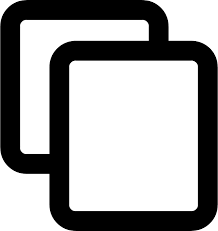
package main
import "fmt"
func main() {
str := "Hello"
bytes := []byte(str) // Convert string to byte slice
newStr := string(bytes) // Convert byte slice back to string
fmt.Println("Bytes:", bytes)
fmt.Println("String:", newStr)
}
4. Common Use Cases for Type Conversion
- Arithmetic with Mixed Types: Convert types to ensure compatibility in operations.
- Formatting and Output: Convert non-string types to strings for printing or concatenation.
- Interfacing with Libraries: Ensure compatibility with function parameters and return types.
5. Example Program
The following program demonstrates type inference and type conversion in action:
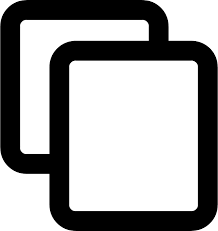
package main
import "fmt"
func main() {
// Type inference
name := "Alice"
age := 25
height := 5.6
fmt.Printf("Name: %s, Age: %d, Height: %.2f\n", name, age, height)
// Type conversion
var total int = 150
var discount float64 = 15.5
var finalAmount float64 = float64(total) - discount
fmt.Printf("Final Amount: %.2f\n", finalAmount)
}
6. Key Points
- Type inference simplifies variable declarations by letting the compiler determine types.
- Type conversion in Go is explicit to ensure clarity and prevent type-related bugs.
- Always handle potential data loss when converting between types (e.g., float to int).
7. Conclusion
Type inference and conversion are powerful features in Go that enhance developer productivity while maintaining type safety. By understanding and applying these features effectively, you can write cleaner and more robust Go programs.
If-Else Statements in Go
1. Introduction
The if
and else
statements in Go allow you to perform conditional branching in your programs. These constructs evaluate boolean expressions and execute code blocks based on the result.
2. Basic Syntax of If-Else
The basic syntax of an if-else
statement in Go is as follows:
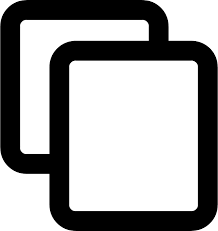
if condition {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Example:
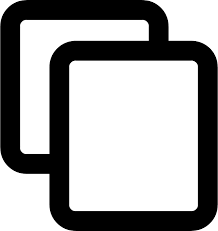
package main
import "fmt"
func main() {
age := 20
if age >= 18 {
fmt.Println("You are an adult.")
} else {
fmt.Println("You are a minor.")
}
}
3. If-Else-If Ladder
The if-else-if
ladder is used to test multiple conditions. The first true condition's block is executed, and the rest are ignored.
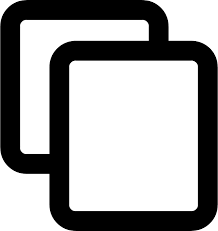
if condition1 {
// Code to execute if condition1 is true
} else if condition2 {
// Code to execute if condition2 is true
} else {
// Code to execute if none of the above conditions are true
}
Example:
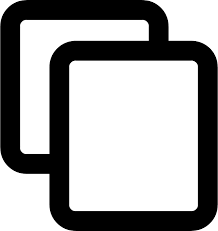
package main
import "fmt"
func main() {
score := 85
if score >= 90 {
fmt.Println("Grade: A")
} else if score >= 75 {
fmt.Println("Grade: B")
} else if score >= 50 {
fmt.Println("Grade: C")
} else {
fmt.Println("Grade: F")
}
}
4. Short Variable Declaration in If Statements
Go allows you to declare and initialize a variable within the if
statement itself. The scope of this variable is limited to the if
and else
blocks.
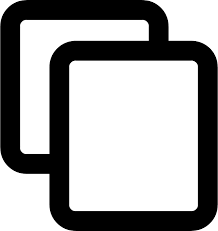
if variable := expression; condition {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Example:
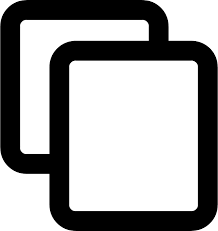
package main
import "fmt"
func main() {
if age := 20; age >= 18 {
fmt.Println("You are eligible to vote.")
} else {
fmt.Println("You are not eligible to vote.")
}
}
5. Nested If Statements
You can nest if
statements within another if
block to test multiple conditions.
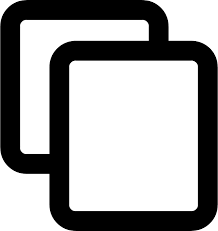
package main
import "fmt"
func main() {
number := 10
if number > 0 {
if number%2 == 0 {
fmt.Println("The number is positive and even.")
} else {
fmt.Println("The number is positive but odd.")
}
} else {
fmt.Println("The number is not positive.")
}
}
6. Key Points
- Conditions in
if
statements must evaluate to a boolean (true
orfalse
). - Curly braces
{}
are mandatory, even for single statements. - Short variable declarations in
if
statements make code concise and limit variable scope. - Always use an
else
block if there is a fallback action to handle.
7. Example Program
The following program demonstrates the use of if-else
and if-else-if
constructs:
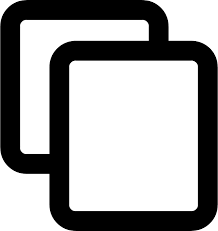
package main
import "fmt"
func main() {
temperature := 30
if temperature > 35 {
fmt.Println("It's very hot.")
} else if temperature >= 25 {
fmt.Println("The weather is warm.")
} else {
fmt.Println("It's cold.")
}
}
8. Conclusion
The if-else
construct is a fundamental tool for decision-making in Go. By mastering this concept, you can implement complex conditional logic in your programs efficiently.
Switch Case Statements in Go
1. Introduction
The switch
statement in Go is a powerful tool for conditional branching. It allows you to evaluate a single expression and execute different code blocks based on its value. Unlike switch
statements in some other languages, Go's switch
does not require explicit break
statements to exit cases, making it cleaner and less error-prone.
2. Basic Syntax
The basic structure of a switch
statement in Go is as follows:
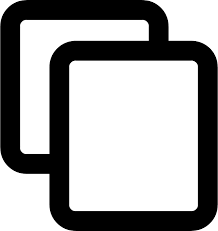
switch expression {
case value1:
// Code to execute if expression == value1
case value2:
// Code to execute if expression == value2
default:
// Code to execute if no case matches
}
Example:
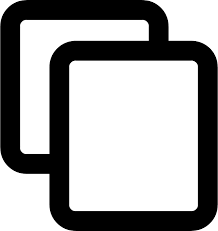
package main
import "fmt"
func main() {
day := "Monday"
switch day {
case "Monday":
fmt.Println("Start of the work week!")
case "Friday":
fmt.Println("End of the work week!")
default:
fmt.Println("It's a regular day.")
}
}
3. Multiple Cases in a Single Branch
You can group multiple case values together by separating them with commas. This is useful when multiple values require the same handling.
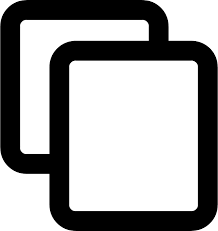
switch expression {
case value1, value2, value3:
// Code to execute if expression matches any of these values
default:
// Fallback code
}
Example:
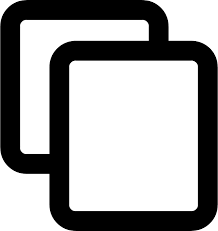
package main
import "fmt"
func main() {
fruit := "apple"
switch fruit {
case "apple", "banana", "cherry":
fmt.Println("This is a common fruit.")
case "kiwi", "mango":
fmt.Println("This is a tropical fruit.")
default:
fmt.Println("Unknown fruit.")
}
}
4. Switch Without an Expression
In Go, you can use a switch
statement without an expression. This allows you to evaluate conditions directly within each case
.
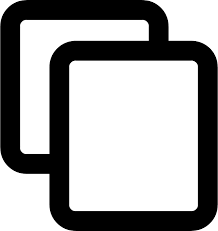
switch {
case condition1:
// Code if condition1 is true
case condition2:
// Code if condition2 is true
default:
// Fallback code
}
Example:
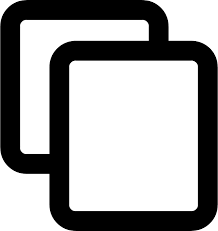
package main
import "fmt"
func main() {
age := 18
switch {
case age < 13:
fmt.Println("You are a child.")
case age >= 13 && age < 20:
fmt.Println("You are a teenager.")
default:
fmt.Println("You are an adult.")
}
}
5. Using Fallthrough
By default, Go's switch
does not automatically fall through to subsequent cases. However, you can explicitly use the fallthrough
keyword to continue to the next case.
Example:
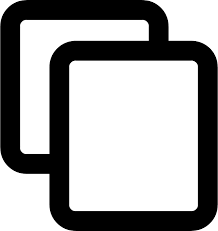
package main
import "fmt"
func main() {
number := 1
switch number {
case 1:
fmt.Println("One")
fallthrough
case 2:
fmt.Println("Two")
case 3:
fmt.Println("Three")
default:
fmt.Println("Unknown number")
}
}
6. Type Switch
A type switch is used to compare the type of an interface value. This is particularly useful when working with variables of the interface{}
type.
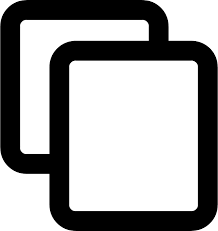
switch value := interfaceValue.(type) {
case type1:
// Code if value is of type1
case type2:
// Code if value is of type2
default:
// Fallback code
}
Example:
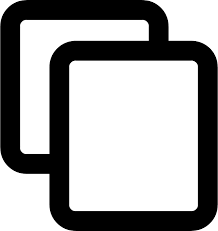
package main
import "fmt"
func main() {
var data interface{} = 42
switch value := data.(type) {
case int:
fmt.Printf("The value is an integer: %d\n", value)
case string:
fmt.Printf("The value is a string: %s\n", value)
default:
fmt.Println("Unknown type")
}
}
7. Key Points
- Go’s
switch
statement is cleaner and avoids fallthrough by default. - Each
case
must be unique; duplicate cases result in a compile-time error. - Default case handles situations where no
case
matches. - Using
fallthrough
allows you to explicitly execute the next case block. - Type switches simplify handling variables of different types.
8. Example Program
The following program demonstrates various uses of switch
statements:
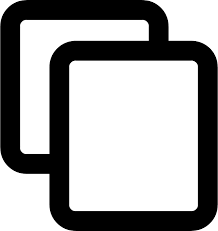
package main
import "fmt"
func main() {
value := 10
switch {
case value < 0:
fmt.Println("Negative number")
case value == 0:
fmt.Println("Zero")
case value > 0:
fmt.Println("Positive number")
}
// Type switch example
var data interface{} = "Hello"
switch data.(type) {
case int:
fmt.Println("Integer type")
case string:
fmt.Println("String type")
default:
fmt.Println("Unknown type")
}
}
9. Conclusion
The switch
statement in Go is a versatile construct that supports various use cases, from evaluating expressions to checking variable types. Its concise syntax and default non-fallthrough behavior make it a valuable tool for writing clear and maintainable code.
For Loop: Traditional and Go-Specific
1. Introduction
The for
loop is the only loop construct in Go. It is more flexible than traditional loops in many other programming languages because it can be used in different ways. Go's for
loop can be used as a traditional for
loop, a while loop, or even an infinite loop, providing multiple forms of iteration.
2. Traditional For Loop (C-style)
The traditional for
loop in Go resembles the loop in languages like C, C++, or Java. It consists of three parts: the initialization, the condition, and the post-expression (increment/decrement).
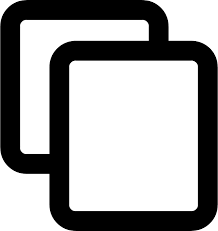
for initialization; condition; post-expression {
// Code to execute on each iteration
}
Example:
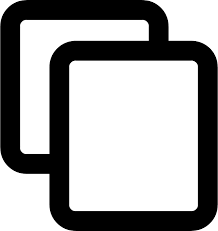
package main
import "fmt"
func main() {
// Traditional for loop
for i := 0; i < 5; i++ {
fmt.Println(i)
}
}
This loop will print the numbers 0 through 4, as the condition i < 5
is checked before each iteration and the post-expression i++
increments the value of i
.
3. Go-Specific For Loop (While Loop)
Go allows you to omit the initialization and post-expression sections, turning the for
loop into a while loop. This is done by just providing the condition part.
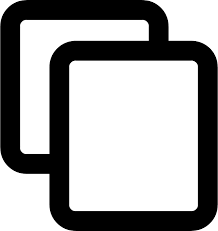
for condition {
// Code to execute while the condition is true
}
Example:
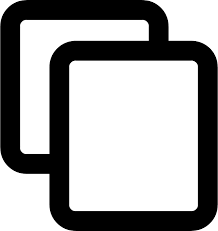
package main
import "fmt"
func main() {
// Go-specific for loop as a while loop
i := 0
for i < 5 {
fmt.Println(i)
i++
}
}
This loop works like a traditional while loop, where i
is incremented in each iteration and the loop continues until i >= 5
.
4. Go-Specific For Loop (Infinite Loop)
In Go, an infinite loop can be created by omitting all three parts of the for
loop. This is useful when you want the loop to run endlessly until some condition is met inside the loop body.
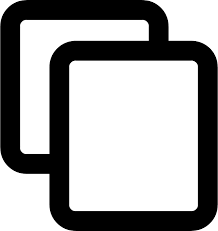
for {
// Code to execute infinitely
}
Example:
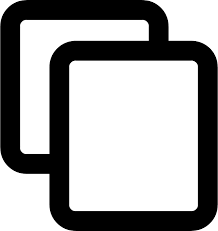
package main
import "fmt"
func main() {
// Infinite loop in Go
i := 0
for {
fmt.Println(i)
i++
if i == 5 {
break // Exit the loop when i reaches 5
}
}
}
This loop will continuously print the value of i
until i == 5
, at which point the break
statement terminates the loop.
5. Nested For Loops
Go allows for nesting for
loops, which can be useful when you need to iterate over multiple dimensions or perform multiple actions in succession.
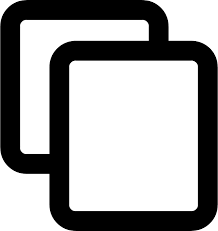
for i := 0; i < 3; i++ {
for j := 0; j < 3; j++ {
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
Example:
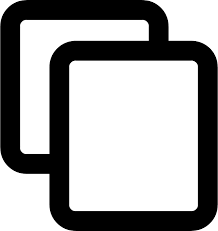
package main
import "fmt"
func main() {
// Nested for loops
for i := 0; i < 3; i++ {
for j := 0; j < 3; j++ {
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
}
This loop will print pairs of i
and j
from 0 to 2, showing all combinations of these values.
6. Using Break and Continue in For Loops
Go supports break
and continue
statements to control the flow of loops:
- Break: Exits the loop entirely.
- Continue: Skips the current iteration and proceeds to the next one.
Example with break
and continue
:
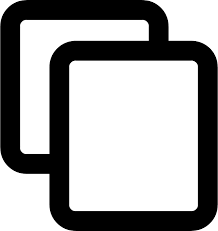
package main
import "fmt"
func main() {
// Using break and continue
for i := 0; i < 5; i++ {
if i == 2 {
continue // Skip iteration when i is 2
}
if i == 4 {
break // Exit the loop when i is 4
}
fmt.Println(i)
}
}
This loop will print the values 0, 1, and 3. When i == 2
, the continue
statement skips the iteration, and when i == 4
, the break
statement exits the loop.
7. Key Points
- Go’s
for
loop is the only loop construct and can be used in multiple forms: traditional, while, and infinite loops. - Go’s
for
loop does not require parentheses, making the syntax more concise. - Go uses
break
andcontinue
to control the flow of loops. - Loops can be nested to handle multidimensional tasks.
8. Example Program
The following program demonstrates the different variations of the for
loop:
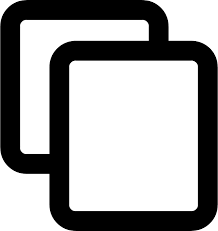
package main
import "fmt"
func main() {
// Traditional for loop
fmt.Println("Traditional for loop:")
for i := 0; i < 3; i++ {
fmt.Println(i)
}
// Go-specific for loop as a while loop
fmt.Println("Go-specific for loop (while loop):")
i := 0
for i < 3 {
fmt.Println(i)
i++
}
// Infinite loop with break
fmt.Println("Infinite for loop with break:")
i = 0
for {
fmt.Println(i)
i++
if i == 3 {
break
}
}
// Nested for loop
fmt.Println("Nested for loop:")
for i := 0; i < 2; i++ {
for j := 0; j < 2; j++ {
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
}
9. Conclusion
The for
loop in Go is versatile and can be used in various ways to handle different types of iteration. Understanding its flexibility will help you write more efficient and readable Go code. Whether you need a simple loop, a while-like loop, or an infinite loop, Go’s for
loop can handle it all.
Break and Continue Statements in Go
1. Introduction
In Go, the break
and continue
statements are used to control the flow of loops. These statements allow you to exit a loop or skip an iteration based on certain conditions. They are essential tools for managing loop execution in Go programs.
2. Break Statement
The break
statement is used to terminate the innermost loop or switch statement. Once the break
statement is executed, the program continues with the code that follows the loop or switch.
Example of break
in a loop:
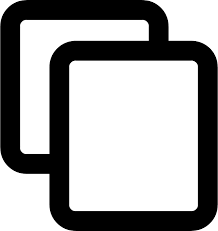
package main
import "fmt"
func main() {
// Break example in a loop
for i := 0; i < 5; i++ {
if i == 3 {
break // Exit the loop when i is 3
}
fmt.Println(i)
}
}
In this example, the loop will print the values 0, 1, 2
and will stop once i == 3
because the break
statement is executed.
3. Continue Statement
The continue
statement skips the current iteration of the loop and moves to the next iteration. It is useful when you want to ignore certain conditions within a loop but continue processing the remaining iterations.
Example of continue
in a loop:
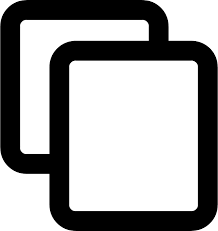
package main
import "fmt"
func main() {
// Continue example in a loop
for i := 0; i < 5; i++ {
if i == 3 {
continue // Skip iteration when i is 3
}
fmt.Println(i)
}
}
In this example, the loop will print the values 0, 1, 2, 4
. When i == 3
, the continue
statement is executed, causing the loop to skip that iteration.
4. Using Break and Continue in Switch Statements
Both the break
and continue
statements can also be used inside switch
statements to control the flow of execution.
Example of break
in a switch
:
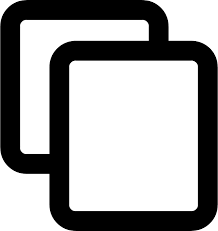
package main
import "fmt"
func main() {
// Break example in a switch statement
day := 3
switch day {
case 1:
fmt.Println("Monday")
case 2:
fmt.Println("Tuesday")
case 3:
fmt.Println("Wednesday")
break // Exit the switch statement
case 4:
fmt.Println("Thursday")
default:
fmt.Println("Invalid day")
}
}
In this example, when day == 3
, the program prints Wednesday
and then exits the switch
statement due to the break
.
Example of continue
in a switch
:
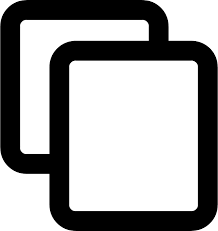
package main
import "fmt"
func main() {
// Continue example in a switch statement
for i := 1; i <= 5; i++ {
switch i {
case 3:
continue // Skip iteration when i is 3
default:
fmt.Println(i)
}
}
}
In this example, the loop will print the values 1, 2, 4, 5
, skipping the iteration when i == 3
due to the continue
statement.
5. Key Points
- Break: Exits the current loop or switch statement entirely.
- Continue: Skips the current iteration of the loop and moves to the next iteration.
- Both
break
andcontinue
can be used infor
loops andswitch
statements. - Control Flow: The use of these statements can help control the flow of execution and improve the readability of code by handling specific conditions efficiently.
6. Example Program
The following program demonstrates the use of both break
and continue
statements in Go:
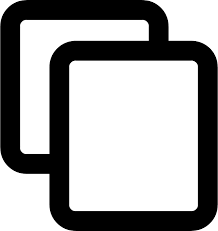
package main
import "fmt"
func main() {
// Using break in a loop
fmt.Println("Break Example:")
for i := 0; i < 5; i++ {
if i == 3 {
break // Exit the loop when i is 3
}
fmt.Println(i)
}
// Using continue in a loop
fmt.Println("\nContinue Example:")
for i := 0; i < 5; i++ {
if i == 3 {
continue // Skip iteration when i is 3
}
fmt.Println(i)
}
}
7. Conclusion
The break
and continue
statements are essential tools for controlling the flow of loops and switch statements in Go. They allow you to exit loops early or skip specific iterations, making your code more efficient and easier to read. Understanding how to use these statements effectively will help you write cleaner, more optimized Go programs.
Defer, Panic, and Recover in Go
1. Introduction
In Go, the defer
, panic
, and recover
functions are used to manage error handling, resource cleanup, and recovery from unexpected situations during program execution. They provide a robust way to handle exceptions and ensure that resources are cleaned up properly, even when errors occur.
2. The defer
Statement
The defer
statement is used to schedule a function call to be executed after the function completes. Deferred function calls are executed in Last In, First Out (LIFO) order, meaning the last deferred function is executed first. defer
is commonly used for cleanup tasks like closing files or releasing resources.
Example of defer
in action:
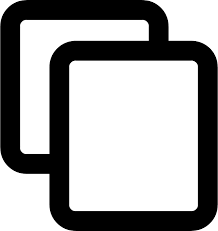
package main
import "fmt"
func example() {
fmt.Println("Before defer")
defer fmt.Println("Deferred 1")
defer fmt.Println("Deferred 2")
fmt.Println("After defer")
}
func main() {
example()
}
In this example, the output will be:
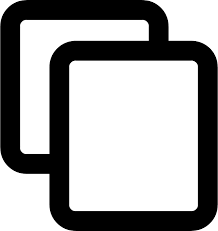
Before defer
After defer
Deferred 2
Deferred 1
The deferred functions are executed after the main function completes, and in LIFO order.
3. The panic
Function
In Go, the panic
function is used to cause a program to stop execution and print an error message. It is typically used for unexpected conditions that cannot be handled gracefully. Once a panic
is triggered, the program starts unwinding, and deferred functions are executed in the reverse order before the program terminates.
Example of panic
in action:
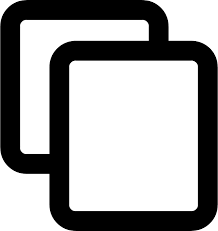
package main
import "fmt"
func triggerPanic() {
panic("Something went wrong!")
}
func main() {
defer fmt.Println("This will be printed after panic.")
triggerPanic()
}
In this example, the program will print:
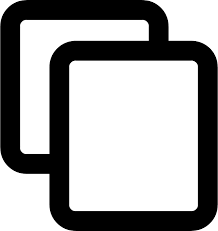
This will be printed after panic.
panic: Something went wrong!
Once panic
is called, the program stops execution, and deferred functions are executed as the stack unwinds.
4. The recover
Function
The recover
function is used to regain control over a panic
and prevent the program from terminating. It can only be called inside a deferred function, and it allows you to handle a panic and recover from it, resuming normal execution of the program. If recover
is not called, the program will terminate.
Example of recover
in action:
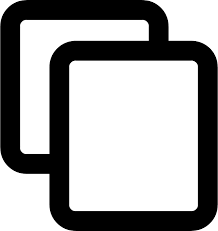
package main
import "fmt"
func recoverFromPanic() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}
func causePanic() {
panic("Critical error occurred!")
}
func main() {
defer recoverFromPanic()
causePanic()
fmt.Println("This will not be printed.")
}
In this example, the program will print:
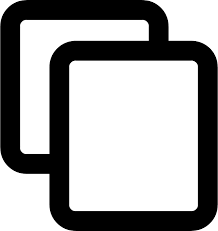
Recovered from panic: Critical error occurred!
After recovering from the panic, the program continues its execution after the deferred function.
5. Key Points
- Defer: Used to schedule a function call to be executed after the current function completes. Deferred functions are executed in LIFO order.
- Panic: Used to trigger a runtime error and stop the execution of the program. It causes the program to unwind and execute deferred functions before terminating.
- Recover: Used to handle a panic and regain control, allowing the program to continue executing.
recover
can only be used inside a deferred function. - Graceful Error Handling: The combination of
defer
,panic
, andrecover
allows Go programs to handle unexpected situations and clean up resources without crashing.
6. Example Program
The following program demonstrates the use of defer
, panic
, and recover
to handle an error:
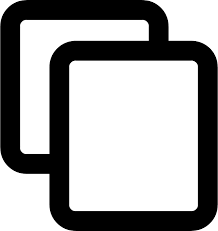
package main
import "fmt"
func recoverFromPanic() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}
func causePanic() {
panic("Something went wrong!")
}
func main() {
defer recoverFromPanic()
fmt.Println("Program started.")
causePanic()
fmt.Println("This line will not be printed due to panic.")
}
Output:
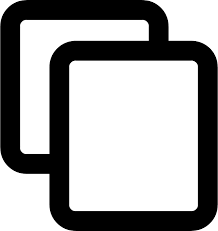
Program started.
Recovered from panic: Something went wrong!
7. Conclusion
Go provides powerful mechanisms for error handling and resource management with the defer
, panic
, and recover
functions. These features help you manage unexpected runtime conditions, clean up resources, and ensure your program continues to run smoothly after handling errors. By understanding and using defer
, panic
, and recover
properly, you can write more robust and reliable Go programs.
Defining and Calling Functions in Go
1. Introduction
Functions in Go are blocks of code designed to perform a specific task. They are a fundamental part of Go's modular approach to programming. Functions help you break down tasks into smaller, reusable units, improving code readability and maintainability. In Go, you can define and call functions with specific parameters and return values.
2. Defining a Function
To define a function in Go, you use the func
keyword, followed by the function name, a list of parameters (if any), and an optional return type.
The basic syntax for defining a function is as follows:
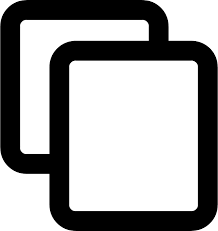
func functionName(parameter1 type1, parameter2 type2) returnType {
// Function body
// Code goes here
}
Example of a simple function definition:
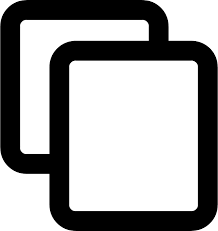
package main
import "fmt"
func greet(name string) {
fmt.Println("Hello, " + name)
}
func main() {
greet("Alice")
}
In this example, the function greet
takes a string
parameter and prints a greeting message. The main
function calls the greet
function with the argument "Alice".
3. Function with Return Value
Functions in Go can also return values. You can define a return type after the parameters in the function signature, and use the return
keyword inside the function body to return a value.
Example of a function with a return value:
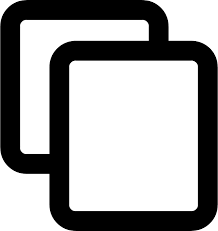
package main
import "fmt"
func add(a int, b int) int {
return a + b
}
func main() {
result := add(3, 4)
fmt.Println("The sum is:", result)
}
In this example, the add
function returns an int
value, which is the sum of the two parameters a
and b
. The result is then printed in the main
function.
4. Multiple Return Values
Go allows functions to return multiple values. This is useful when you need to return more than one result from a function, such as a value and an error.
Example of a function with multiple return values:
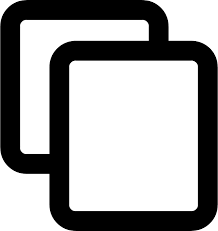
package main
import "fmt"
func divide(a int, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 2)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the divide
function returns two values: the result of the division and an error
. If the divisor b
is zero, the function returns an error message. Otherwise, it returns the result of the division.
5. Function Parameters and Arguments
Function parameters define the types of values that can be passed to a function when it is called. When calling a function, you pass arguments that match the types of the corresponding parameters.
Example of a function with parameters and how to call it:
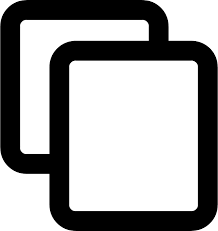
package main
import "fmt"
func greetPerson(name string, age int) {
fmt.Printf("Hello, %s! You are %d years old.\n", name, age)
}
func main() {
greetPerson("Bob", 30)
}
In this example, the greetPerson
function takes two parameters: name
(a string
) and age
(an int
). The function is called with the arguments "Bob" and 30, which are passed to the corresponding parameters.
6. Variadic Functions
Go supports variadic functions, which allow you to pass a variable number of arguments to a function. The variadic parameter is specified by using an ellipsis (...
) before the type name.
Example of a variadic function:
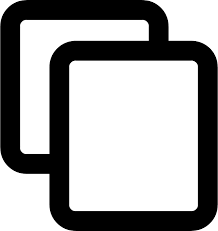
package main
import "fmt"
func sum(numbers ...int) int {
total := 0
for _, num := range numbers {
total += num
}
return total
}
func main() {
result := sum(1, 2, 3, 4, 5)
fmt.Println("Sum:", result)
}
In this example, the sum
function accepts a variable number of int
arguments, which are summed up and returned as the result. The function is called with five arguments, but it can also handle any number of arguments.
7. Anonymous Functions
Go also supports anonymous functions, which are functions that are defined without a name. These functions are often used as arguments to other functions or as inline callback functions.
Example of an anonymous function:
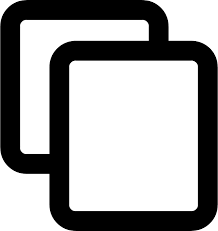
package main
import "fmt"
func main() {
greet := func(name string) {
fmt.Println("Hello, " + name)
}
greet("Charlie")
}
In this example, the anonymous function is assigned to the variable greet
, and then called with the argument "Charlie".
8. Conclusion
Functions are a core building block in Go programming. Understanding how to define and call functions, as well as how to use parameters, return values, variadic functions, and anonymous functions, will help you write more modular and maintainable Go code. Functions allow you to break down complex tasks into smaller, manageable units that can be reused throughout your programs.
Function Parameters and Return Types in Go
1. Introduction
In Go, functions are a key part of the language’s modular approach, allowing you to define reusable blocks of code. Every function in Go can have one or more parameters and return types. The parameters are used to pass values into the function, and the return type specifies the value that the function will output.
2. Function Parameters
Function parameters define the types of values that can be passed to a function when it is called. These parameters act as placeholders for the values (arguments) passed to the function during its invocation. Go requires explicit type declarations for function parameters.
The syntax for declaring function parameters is:
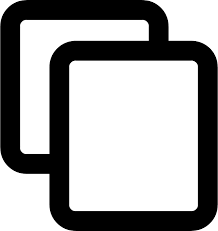
func functionName(parameter1 type1, parameter2 type2) {
Here, parameter1
and parameter2
are the names of the parameters, and type1
and type2
are their corresponding data types.
Example of a function with parameters:
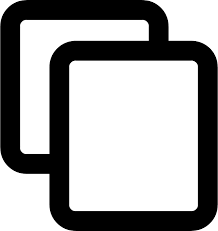
package main
import "fmt"
func greet(name string, age int) {
fmt.Printf("Hello, %s! You are %d years old.\n", name, age)
}
func main() {
greet("Alice", 25)
}
In this example, the function greet
accepts two parameters: name
of type string
and age
of type int
. The function is called in main
with the arguments "Alice" and 25.
3. Multiple Parameters
Go allows you to define a function with multiple parameters. You can specify as many parameters as necessary by separating them with commas.
Example with multiple parameters:
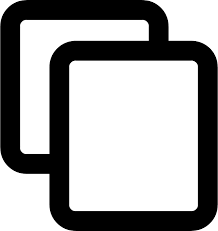
package main
import "fmt"
func add(a int, b int, c int) int {
return a + b + c
}
func main() {
result := add(1, 2, 3)
fmt.Println("The sum is:", result)
}
In this example, the add
function accepts three parameters (a
, b
, and c
) and returns their sum.
4. Function Return Types
Return types specify the type of value that a function will return. Go allows functions to return one or more values. You define the return type(s) after the parameters in the function signature.
The syntax for defining a return type is:
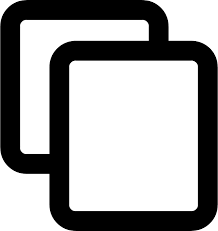
func functionName(parameter1 type1) returnType {
For example, a function returning an int
:
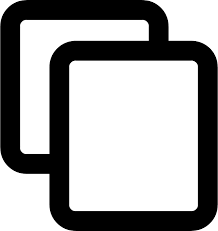
package main
import "fmt"
func multiply(a int, b int) int {
return a * b
}
func main() {
result := multiply(4, 5)
fmt.Println("The result is:", result)
}
In this example, the multiply
function returns an int
value, which is the product of a
and b
.
5. Multiple Return Values
Go allows functions to return multiple values. This feature is useful when you need to return both a result and an error, or when you need to return more than one value from a function.
Here’s how you can return multiple values from a function:
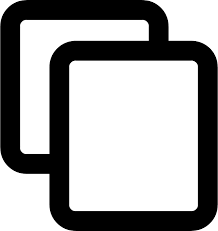
package main
import "fmt"
func divide(a int, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 2)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the divide
function returns two values: the result of the division and an error
value. If the divisor is zero, an error is returned.
6. Named Return Values
You can also provide names for the return values in Go. Named return values allow you to assign a name to the return variable(s) in the function signature. This can make the code more readable and self-documenting.
Example of named return values:
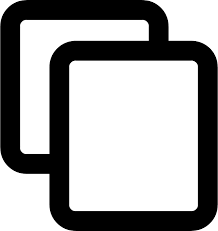
package main
import "fmt"
func calculate(a int, b int) (sum int, product int) {
sum = a + b
product = a * b
return
}
func main() {
resultSum, resultProduct := calculate(4, 5)
fmt.Println("Sum:", resultSum)
fmt.Println("Product:", resultProduct)
}
In this example, the calculate
function returns two named values: sum
and product
. These variables are automatically returned at the end of the function.
7. Type of Return Values
Go is statically typed, so you must specify the type of the return value(s). The return type of a function is declared after the parameters in the function signature.
For example, a function returning a string
:
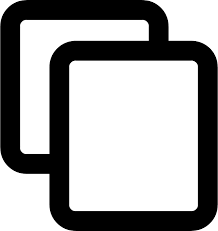
package main
import "fmt"
func greet(name string) string {
return "Hello, " + name
}
func main() {
message := greet("John")
fmt.Println(message)
}
In this example, the greet
function returns a string
value, which is a greeting message. The return type is defined as string
in the function signature.
8. Conclusion
Understanding function parameters and return types is crucial for writing efficient and reusable Go code. Whether you’re working with single or multiple parameters and return types, Go’s syntax ensures that your functions are clear and explicit. By using named return values, handling multiple return values, and defining appropriate parameter types, you can create clean, maintainable functions in Go.
Multiple Return Values in Go
1. Introduction
Go allows functions to return multiple values, which is a unique feature compared to many other programming languages. This is particularly useful when you need to return more than one result from a function, such as a result and an error, or multiple pieces of related data.
2. Returning Multiple Values
In Go, you can return multiple values from a function by separating them with commas in the function signature. When calling the function, you can capture each returned value in a separate variable.
Example of returning multiple values:
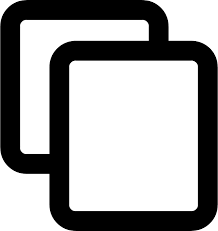
package main
import "fmt"
func addAndSubtract(a int, b int) (int, int) {
return a + b, a - b
}
func main() {
sum, difference := addAndSubtract(10, 5)
fmt.Println("Sum:", sum)
fmt.Println("Difference:", difference)
}
In this example, the addAndSubtract
function returns two values: the sum and the difference of the numbers. These values are captured in the sum
and difference
variables in the main
function.
3. Returning Multiple Values with Different Types
Go’s multiple return values are not limited to values of the same type. You can return values of different types from a function, which is quite useful when you need to return multiple related pieces of data.
Example of returning multiple values with different types:
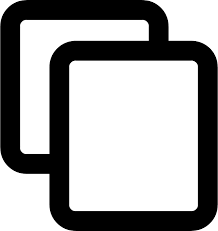
package main
import "fmt"
func getPersonInfo() (string, int, bool) {
return "Alice", 30, true
}
func main() {
name, age, isActive := getPersonInfo()
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Active:", isActive)
}
Here, the getPersonInfo
function returns three values: a string
(name), an int
(age), and a bool
(isActive). The values are captured in the respective variables name
, age
, and isActive
.
4. Ignoring Return Values
If you do not need all the returned values, you can use the blank identifier (_
) to ignore specific return values.
Example of ignoring a return value:
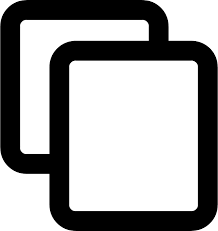
package main
import "fmt"
func divide(a int, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide by zero")
}
return a / b, nil
}
func main() {
result, _ := divide(10, 0) // Ignoring error
fmt.Println("Result:", result)
}
In this example, the divide
function returns two values: a result and an error. In the main
function, the error is ignored by using the blank identifier, and only the result is captured.
5. Named Return Values
Another feature of Go’s multiple return values is that you can name the return values. This allows you to omit the return
statement entirely if you want to return the named variables, making the function simpler and more readable.
Example with named return values:
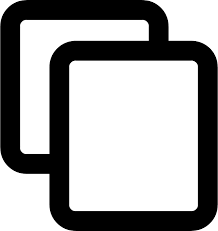
package main
import "fmt"
func calculate(a int, b int) (sum int, product int) {
sum = a + b
product = a * b
return
}
func main() {
resultSum, resultProduct := calculate(3, 4)
fmt.Println("Sum:", resultSum)
fmt.Println("Product:", resultProduct)
}
In this example, the calculate
function returns two named values: sum
and product
. The return statement is implicit, and the function returns the named variables directly.
6. Returning Multiple Values and Error Handling
Returning multiple values is especially useful in error handling. Go does not have exceptions, so functions often return an error value as one of their return values. This approach allows you to handle errors explicitly and in a controlled manner.
Example of multiple return values with error handling:
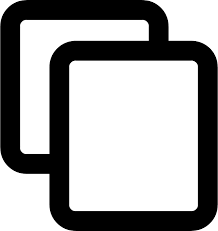
package main
import "fmt"
func safeDivide(a int, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
func main() {
result, err := safeDivide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the safeDivide
function returns two values: the result of the division and an error. If the division is by zero, an error is returned; otherwise, the result is returned along with a nil
error.
7. Conclusion
In Go, returning multiple values is a powerful feature that allows you to handle more complex scenarios efficiently. Whether you are returning multiple results, handling errors, or working with named return values, Go’s approach to multiple return values promotes clear, readable, and maintainable code.
Named Return Values in Go
1. Introduction
In Go, you can define named return values for a function. This allows you to assign names to the return values directly in the function signature, and you can return these values without explicitly mentioning them in the return
statement. Named return values can make your code more readable and eliminate the need to repeat variable names in the return
statement.
2. Declaring Named Return Values
When declaring a function, you can give names to the return values in the function signature. This makes the return values available inside the function and allows you to set their values directly without needing to declare new variables.
Example of declaring and using named return values:
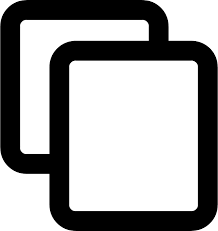
package main
import "fmt"
func calculate(a int, b int) (sum int, product int) {
sum = a + b
product = a * b
return
}
func main() {
resultSum, resultProduct := calculate(3, 4)
fmt.Println("Sum:", resultSum)
fmt.Println("Product:", resultProduct)
}
In this example, the calculate
function has two named return values: sum
and product
. These variables are assigned values within the function, and the return
statement does not need to explicitly mention them. The return values are automatically returned when the function exits.
3. Why Use Named Return Values?
Named return values provide several advantages:
- Improved Readability: Named return values make the code more readable by clearly indicating what values the function is returning.
- Eliminating Redundancy: You don’t need to repeat the names of the return variables in the
return
statement. It can help to reduce redundancy in the code. - Implicit Return: If you omit the
return
values, Go will automatically return the named variables with their values assigned within the function.
4. How Named Return Values Work
When you use named return values, Go automatically returns the variables with the names specified in the function signature. If the function reaches a return
statement without explicitly specifying the return values, Go will return the named variables that were set inside the function.
Example of implicit return with named return values:
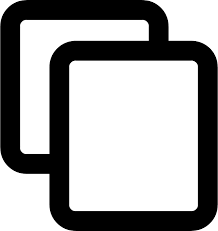
package main
import "fmt"
func divide(a int, b int) (result int, errorMsg string) {
if b == 0 {
errorMsg = "division by zero"
return
}
result = a / b
return
}
func main() {
result, err := divide(10, 0)
if err != "" {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In the example above, the divide
function has two named return values: result
and errorMsg
. If division by zero occurs, the error message is set, and the function returns without specifying the values explicitly, relying on Go’s implicit return behavior.
5. Using Named Return Values with Multiple Return Values
Named return values can be particularly useful when working with functions that return multiple values, such as a result and an error. By naming the return values, you can make the function’s purpose clearer and avoid repetition in the code.
Example with named return values and multiple return values:
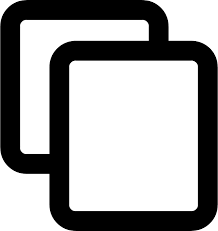
package main
import "fmt"
func getPersonInfo() (name string, age int, isActive bool) {
name = "Alice"
age = 30
isActive = true
return
}
func main() {
name, age, active := getPersonInfo()
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Active:", active)
}
The getPersonInfo
function returns three named values: name
, age
, and isActive
. The values are automatically returned in the return
statement without needing to mention them explicitly.
6. Considerations for Using Named Return Values
While named return values can improve readability, they should be used carefully:
- Clarity: Named return values should be used when they add clarity to the function. Overuse or unnecessary naming can lead to confusion.
- Consistency: Be consistent in your use of named return values, especially in a large codebase. Following a style guide can help maintain readability.
- No Side Effects: Avoid side effects in named return values. Modifying named return values outside the function body can lead to unintended consequences.
7. Conclusion
Named return values in Go offer a convenient and readable way to work with functions that return multiple values. They simplify the return
statement and improve the overall clarity of the function. However, it’s important to use them judiciously to maintain clean and readable code.
Anonymous Functions and Closures in Go
1. Introduction to Anonymous Functions
In Go, anonymous functions (also known as lambda functions or function literals) are functions that do not have a name. They can be defined inline and assigned to variables or passed as arguments to other functions. Anonymous functions are often used when a short function is needed for a specific task or in higher-order functions.
Example of an anonymous function:
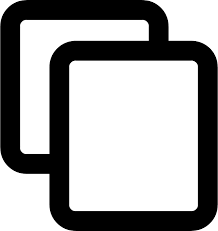
package main
import "fmt"
func main() {
// Define and call an anonymous function
func() {
fmt.Println("Hello from an anonymous function!")
}()
}
In the example above, an anonymous function is defined and called immediately. The function does not have a name and is invoked within the main
function.
2. Assigning Anonymous Functions to Variables
Anonymous functions can also be assigned to variables for later use. This is useful when you need to pass a function as a parameter or store it for later execution.
Example of assigning an anonymous function to a variable:
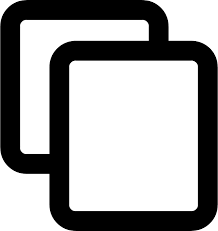
package main
import "fmt"
func main() {
// Assigning an anonymous function to a variable
greet := func(name string) {
fmt.Println("Hello, " + name)
}
// Calling the anonymous function via the variable
greet("Alice")
}
In this example, the anonymous function is assigned to the variable greet
, which is then called with the argument "Alice"
.
3. Passing Anonymous Functions as Arguments
Anonymous functions can be passed as arguments to other functions, which is a common pattern in Go. This is useful for providing short, one-off functions that are not required elsewhere in your code.
Example of passing an anonymous function as an argument:
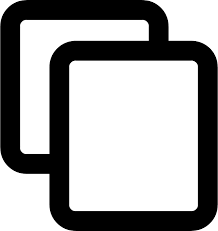
package main
import "fmt"
// Function that accepts a function as an argument
func applyOperation(a int, b int, operation func(int, int)) {
operation(a, b)
}
func main() {
// Passing an anonymous function as an argument
applyOperation(10, 20, func(a int, b int) {
fmt.Println("Sum:", a+b)
})
}
Here, the applyOperation
function accepts two integers and a function as its arguments. The anonymous function is passed as the operation
argument and performs the sum of the two integers.
4. Closures in Go
A closure is a function that captures its surrounding environment, meaning it can access variables from the outer function even after the outer function has returned. Closures are useful for implementing functions that maintain state between calls.
Example of a closure:
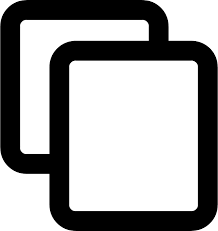
package main
import "fmt"
// Function that returns a closure
func counter() func() int {
count := 0
return func() int {
count++
return count
}
}
func main() {
// Create a counter closure
increment := counter()
// Calling the closure multiple times
fmt.Println(increment()) // 1
fmt.Println(increment()) // 2
fmt.Println(increment()) // 3
}
In this example, the counter
function returns an anonymous function that acts as a closure. The inner function has access to the count
variable from the outer function counter
, and it can modify and return its value each time it's called.
5. How Closures Work
Closures work by "closing over" variables from their surrounding environment, which means they "remember" the values of variables from the outer function even after the outer function has finished executing. This allows closures to maintain state between calls.
Example of a closure maintaining state:
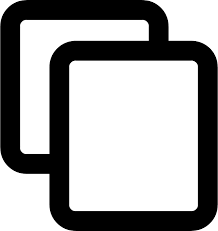
package main
import "fmt"
func main() {
// Define a closure that remembers its state
addX := func(x int) func(int) int {
return func(y int) int {
return x + y
}
}
// Create a closure that adds 5 to its argument
addFive := addX(5)
// Call the closure
fmt.Println(addFive(3)) // 8
fmt.Println(addFive(10)) // 15
}
In this example, the closure addX
remembers the value of x
even after it has been returned. When addFive
is called, it adds 5 to the argument passed to it.
6. Benefits of Closures
Closures provide several benefits:
- Stateful Functions: Closures can maintain state between function calls, making them useful for creating stateful functions like counters, accumulators, and more.
- Encapsulation: Closures allow you to encapsulate functionality and keep the implementation details hidden from the outside world while still allowing access to certain variables.
- Flexible and Concise: Closures enable writing concise and flexible code for situations where you need a function to access specific variables or states that are not passed as arguments.
7. Considerations When Using Closures
While closures are powerful, there are a few things to consider:
- Memory Usage: Closures can retain references to variables, leading to potential memory leaks if not handled properly. Be cautious when dealing with closures in long-running programs.
- Performance: Since closures involve additional function calls, they can introduce some performance overhead. However, this is usually not a significant concern for most use cases.
8. Conclusion
Anonymous functions and closures are powerful features in Go that allow you to create compact, flexible, and stateful functions. By leveraging anonymous functions and closures, you can write more concise and readable code, especially when dealing with higher-order functions or functions that need to maintain state across calls.
Variadic Functions in Go
1. Introduction to Variadic Functions
Variadic functions in Go are functions that can accept a variable number of arguments. This allows you to call the function with any number of arguments of the specified type, making your functions more flexible and adaptable to different use cases. Variadic functions are commonly used when the exact number of arguments is not known in advance, such as in formatting functions or performing operations on a list of items.
In Go, variadic functions are defined by using an ellipsis (...
) before the type of the last parameter. This allows you to pass a slice of values of the specified type.
2. Defining a Variadic Function
To define a variadic function in Go, you place an ellipsis (...
) before the type of the last parameter. This parameter will accept multiple values, which will be treated as a slice of that type.
Example of a variadic function:
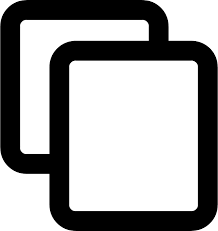
package main
import "fmt"
// Variadic function that sums any number of integers
func sum(numbers ...int) int {
total := 0
for _, number := range numbers {
total += number
}
return total
}
func main() {
// Call the variadic function with different numbers of arguments
fmt.Println(sum(1, 2, 3)) // Output: 6
fmt.Println(sum(10, 20, 30, 40)) // Output: 100
}
In this example, the sum
function accepts a variadic parameter numbers
, which is a slice of integers. The function sums all the integers passed to it and returns the total.
3. Calling a Variadic Function
When calling a variadic function, you can pass any number of arguments of the specified type. If you pass no arguments, the function will receive an empty slice.
Example of calling a variadic function:
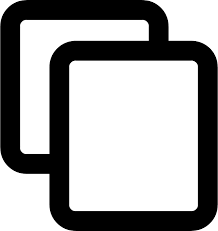
package main
import "fmt"
func greet(names ...string) {
for _, name := range names {
fmt.Println("Hello, " + name)
}
}
func main() {
greet("Alice", "Bob", "Charlie") // Output: Hello, Alice\nHello, Bob\nHello, Charlie
greet("David") // Output: Hello, David
greet() // No output (empty call)
}
The greet
function here takes a variadic parameter names
of type string
. It prints a greeting for each name passed to it. You can call greet
with any number of names or without any names at all.
4. Passing a Slice to a Variadic Function
If you already have a slice of values and want to pass it to a variadic function, you need to use the ...
syntax to unpack the slice into individual arguments.
Example of passing a slice to a variadic function:
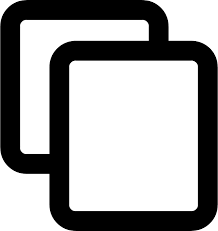
package main
import "fmt"
func sum(numbers ...int) int {
total := 0
for _, number := range numbers {
total += number
}
return total
}
func main() {
nums := []int{5, 10, 15, 20}
fmt.Println(sum(nums...)) // Output: 50 (unpacking the slice)
}
In this example, the slice nums
is passed to the sum
function using the ...
syntax, which unpacks the slice elements as individual arguments.
5. Variadic Functions with Other Parameters
A variadic function can also have other parameters, but the variadic parameter must always come last in the function signature.
Example of a variadic function with other parameters:
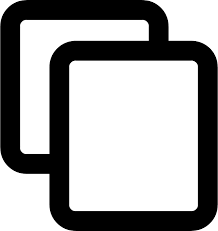
package main
import "fmt"
func greet(message string, names ...string) {
fmt.Println(message)
for _, name := range names {
fmt.Println("Hello, " + name)
}
}
func main() {
greet("Welcome", "Alice", "Bob", "Charlie")
}
Here, the greet
function takes a regular parameter message
and a variadic parameter names
. The regular parameter comes first, and the variadic parameter follows.
6. Limitations of Variadic Functions
While variadic functions are flexible and convenient, there are some limitations:
- Only One Variadic Parameter: A function can only have one variadic parameter, and it must be placed at the end of the function signature.
- Cannot Mix with Non-Variadic Parameters: You cannot have a variadic parameter before a non-variadic parameter in the function signature.
- Memory Usage: Variadic functions create a slice, so if you pass a large number of arguments, it can lead to additional memory overhead.
7. Conclusion
Variadic functions are a powerful feature in Go that allows you to define functions that can accept a variable number of arguments. This provides flexibility when you don't know in advance how many arguments will be passed to a function. By using variadic functions, you can write more concise and adaptable code, especially for functions that perform operations on a list or set of values.
Introduction to Arrays in Go
1. What is an Array in Go?
In Go, an array is a fixed-size, ordered collection of elements of the same type. The size of an array is specified when the array is declared and cannot be changed once it is created. Arrays are useful when you need to store a collection of elements that you know in advance will have a specific number of items.
Unlike slices (which are more commonly used in Go), arrays have a fixed size, and you cannot append or remove elements from them once they have been created. The size of an array is part of its type, so arrays of different sizes are considered different types in Go.
2. Declaring and Initializing Arrays
To declare an array in Go, you specify the type of the array elements and the number of elements in the array. The syntax is as follows:
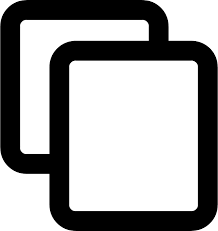
var arrayName [size]Type
Where size
is the number of elements in the array and Type
is the type of the elements.
Example of declaring an array:
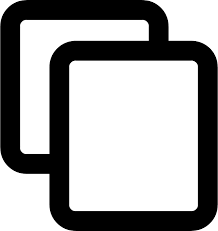
package main
import "fmt"
func main() {
var numbers [5]int // Declare an array of 5 integers
numbers[0] = 10 // Assign value to the first element
numbers[1] = 20 // Assign value to the second element
fmt.Println(numbers) // Output: [10 20 0 0 0]
}
In this example, we declare an array numbers
with 5 elements of type int
and assign values to the first two elements.
3. Initializing Arrays with Values
You can also initialize an array at the time of declaration by providing a list of values. Go will automatically determine the array's size based on the number of elements you provide:
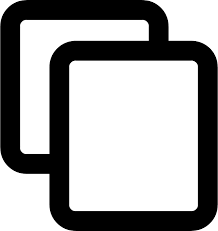
package main
import "fmt"
func main() {
numbers := [3]int{1, 2, 3} // Declare and initialize an array
fmt.Println(numbers) // Output: [1 2 3]
}
In this case, the array numbers
is initialized with three elements, and the size is automatically set to 3.
4. Arrays Are Fixed in Size
One important characteristic of arrays in Go is that their size is fixed and cannot be changed. Once an array is created, its size cannot be altered. If you need a collection that can change in size, you should use slices instead.
Example of trying to change the size of an array (this will result in a compile-time error):
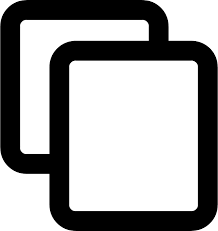
package main
import "fmt"
func main() {
var numbers [3]int
numbers = append(numbers, 4) // Error: cannot use append on arrays
fmt.Println(numbers)
}
5. Accessing Array Elements
You can access and modify elements of an array using the index. The indices of Go arrays start at 0, meaning the first element has an index of 0, the second has an index of 1, and so on.
Example of accessing array elements:
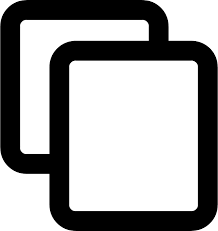
package main
import "fmt"
func main() {
numbers := [3]int{10, 20, 30}
fmt.Println(numbers[0]) // Output: 10 (first element)
fmt.Println(numbers[1]) // Output: 20 (second element)
fmt.Println(numbers[2]) // Output: 30 (third element)
}
6. Length of an Array
You can find the length of an array using the len()
function. The length of an array is the number of elements it contains.
Example of finding the length of an array:
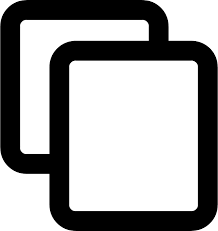
package main
import "fmt"
func main() {
numbers := [4]int{10, 20, 30, 40}
fmt.Println(len(numbers)) // Output: 4 (number of elements in the array)
}
7. Arrays Are Value Types
In Go, arrays are value types, which means that when you assign an array to another array, a copy of the original array is created. Modifying the copy does not affect the original array.
Example of arrays being copied:
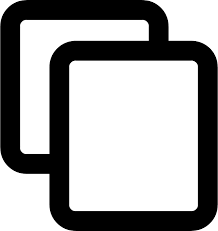
package main
import "fmt"
func main() {
arr1 := [3]int{1, 2, 3}
arr2 := arr1 // Copy arr1 to arr2
arr2[0] = 100 // Modify arr2
fmt.Println(arr1) // Output: [1 2 3] (original array is unchanged)
fmt.Println(arr2) // Output: [100 2 3] (modified copy)
}
In this example, when we assign arr1
to arr2
, a copy of the array is made. Changes to arr2
do not affect arr1
.
8. Multidimensional Arrays
Go also supports multidimensional arrays, where you can have arrays of arrays. The syntax for a two-dimensional array is as follows:
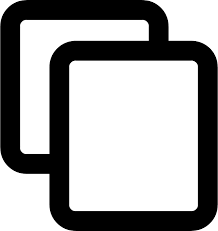
var arrayName [rowSize][columnSize]Type
Example of a two-dimensional array:
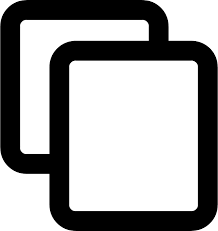
package main
import "fmt"
func main() {
var matrix [2][2]int
matrix[0][0] = 1
matrix[0][1] = 2
matrix[1][0] = 3
matrix[1][1] = 4
fmt.Println(matrix) // Output: [[1 2] [3 4]]
}
In this example, we declare a two-dimensional array matrix
with 2 rows and 2 columns and initialize it with values.
9. Conclusion
Arrays in Go are fixed-size collections of elements of the same type. While they provide a useful way to store a collection of items, their fixed size limits their flexibility. For more dynamic behavior, Go encourages the use of slices, which are more versatile. Understanding how arrays work in Go is crucial, as they form the foundation of more complex data structures and algorithms.
Declaring and Initializing Arrays in Go
1. Declaring an Array in Go
In Go, arrays are declared by specifying the type of elements they will hold and the number of elements (size) they will contain. The syntax for declaring an array is:
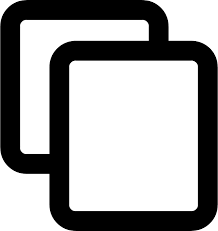
var arrayName [size]Type
Where:
- arrayName: The name of the array.
- size: The number of elements the array will hold.
- Type: The type of elements the array will hold (e.g., int, string, etc.).
Example of declaring an array:
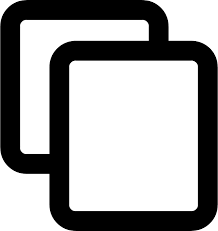
package main
import "fmt"
func main() {
var numbers [5]int // Declare an array of 5 integers
fmt.Println(numbers) // Output: [0 0 0 0 0] (default value of int is 0)
}
In this example, we declare an array numbers
that can hold 5 integers. Since we haven't initialized the array, all elements are set to the default value for integers (0).
2. Initializing Arrays with Values
Arrays can be initialized with specific values at the time of declaration. Go will automatically determine the size of the array based on the number of values provided.
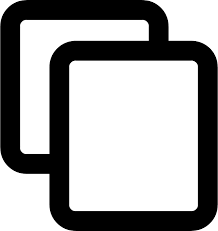
package main
import "fmt"
func main() {
numbers := [3]int{1, 2, 3} // Declare and initialize an array with 3 integers
fmt.Println(numbers) // Output: [1 2 3]
}
In this example, we declare and initialize the array numbers
with 3 integer values. The size of the array is automatically set to 3.
3. Initializing Arrays with Default Values
If you want to initialize an array with a default value for all elements, you can use the var
keyword with the array type, and Go will automatically assign the default value for the given type (e.g., 0 for integers, empty string for strings, etc.).
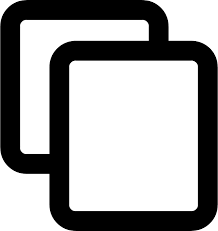
package main
import "fmt"
func main() {
var numbers [4]int // Declare an array of 4 integers
fmt.Println(numbers) // Output: [0 0 0 0] (default value for int)
}
Here, we declare an array numbers
with 4 elements, but we haven't assigned any values. Therefore, all elements are initialized to their default value (0 for integers).
4. Using the Short Declaration Operator
Go allows you to use the short declaration operator (: =
) to declare and initialize an array in a more concise way. The size of the array is determined by the number of elements provided in the initialization.
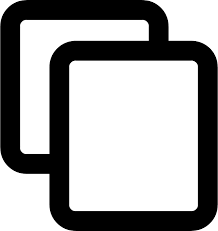
package main
import "fmt"
func main() {
numbers := [3]int{10, 20, 30} // Short declaration of an array with 3 elements
fmt.Println(numbers) // Output: [10 20 30]
}
In this example, we use the short declaration to declare and initialize the array numbers
with 3 elements. The array's size is automatically set to 3 based on the number of elements.
5. Declaring Arrays with Specific Indexes
You can also initialize arrays by explicitly specifying the index of each element. When you provide an index, the size of the array is automatically adjusted to accommodate the largest index.
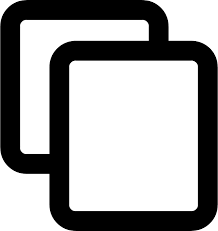
package main
import "fmt"
func main() {
numbers := [5]int{0: 10, 3: 20} // Explicitly setting values at specific indexes
fmt.Println(numbers) // Output: [10 0 0 20 0]
}
Here, we explicitly set the value at index 0 to 10 and the value at index 3 to 20. The other indices (1, 2, and 4) are set to their default value (0).
6. Multi-dimensional Arrays
Go also supports multi-dimensional arrays. A two-dimensional array, for example, is an array of arrays. The syntax for declaring a two-dimensional array is:
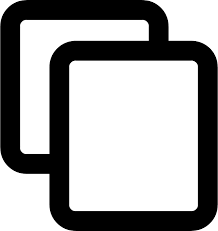
var arrayName [rowSize][columnSize]Type
Example of declaring and initializing a two-dimensional array:
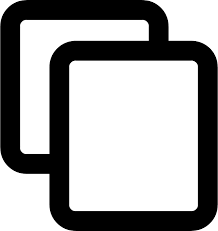
package main
import "fmt"
func main() {
var matrix [2][2]int // Declare a 2x2 integer array
matrix[0][0] = 1
matrix[0][1] = 2
matrix[1][0] = 3
matrix[1][1] = 4
fmt.Println(matrix) // Output: [[1 2] [3 4]]
}
In this example, we declare a 2x2 matrix and manually assign values to each element. The array is a collection of rows, each containing a set of columns.
7. Conclusion
In Go, arrays are fixed-size collections of elements of the same type. You can declare and initialize arrays with specific values or use default values. For flexible and resizable collections, Go encourages the use of slices. Understanding how to declare and initialize arrays is important when working with collections of data in Go.
Working with Slices in Go
1. Introduction to Slices
In Go, slices are more flexible and powerful than arrays. While arrays have a fixed size, slices allow for dynamic resizing and are more commonly used in Go programs. A slice is essentially a reference to a segment of an array and provides a way to work with portions of arrays without copying the data.
2. Declaring and Initializing Slices
Slices can be declared and initialized in several ways. The most common way is using the make
function or by slicing an existing array.
Using the make
function
The make
function is used to create a slice with a specified length and capacity. The syntax is:
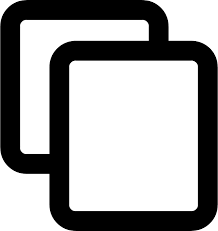
make([]Type, length, capacity)
Where:
- Type: The type of the elements in the slice.
- length: The number of elements in the slice.
- capacity: The capacity of the slice (optional, defaults to the length).
Example of creating a slice using make
:
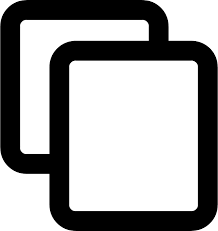
package main
import "fmt"
func main() {
numbers := make([]int, 5) // Create a slice of length 5
fmt.Println(numbers) // Output: [0 0 0 0 0] (default value for int is 0)
}
Using an Array
Slices can also be created by slicing an existing array or another slice:
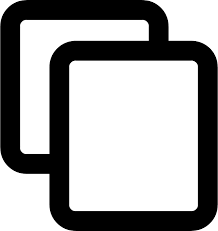
package main
import "fmt"
func main() {
arr := [5]int{1, 2, 3, 4, 5}
slice := arr[1:4] // Slice the array from index 1 to 3
fmt.Println(slice) // Output: [2 3 4]
}
In this example, we create a slice from the array arr
starting at index 1 and ending at index 3 (not including index 4).
3. Slice Length and Capacity
Every slice has two important properties: length and capacity.
- Length: The number of elements in the slice.
- Capacity: The total number of elements the slice can hold without allocating more memory. It starts as the length and grows as needed.
You can get the length and capacity of a slice using the len()
and cap()
functions:
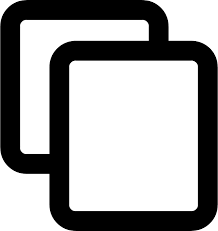
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
fmt.Println("Length:", len(numbers)) // Output: Length: 5
fmt.Println("Capacity:", cap(numbers)) // Output: Capacity: 5
}
In this example, the slice numbers
has both a length and capacity of 5.
4. Appending to a Slice
One of the most common operations with slices is appending new elements. Go provides the append()
function to add elements to the end of a slice. The append()
function automatically increases the capacity of the slice if needed.
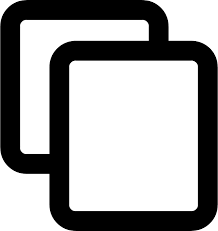
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3}
numbers = append(numbers, 4, 5) // Append two elements to the slice
fmt.Println(numbers) // Output: [1 2 3 4 5]
}
The append()
function returns a new slice with the added elements. The original slice is not modified directly.
5. Slicing a Slice
You can slice an existing slice just like you would slice an array. The syntax is:
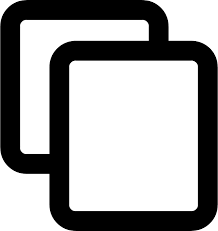
slice[start:end]
Where:
- start: The index at which to start the slice (inclusive).
- end: The index at which to end the slice (exclusive).
Example of slicing a slice:
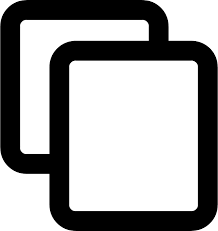
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
subSlice := numbers[1:4] // Slice the slice from index 1 to 3
fmt.Println(subSlice) // Output: [2 3 4]
}
6. Modifying a Slice
Since slices are references to arrays, modifying a slice will modify the underlying array. Here’s an example:
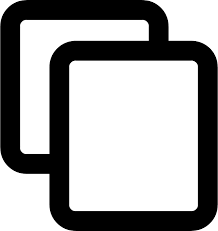
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4}
slice := numbers[1:3] // Slice the slice
slice[0] = 10 // Modify the first element of the slice
fmt.Println(numbers) // Output: [1 10 3 4]
fmt.Println(slice) // Output: [10 3]
}
In this example, we modify the first element of the slice, which also changes the underlying array.
7. Multi-dimensional Slices
Just like arrays, slices can also be multi-dimensional. A two-dimensional slice can be declared and initialized as follows:
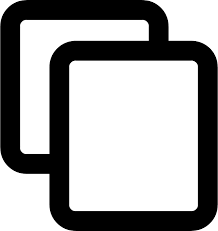
package main
import "fmt"
func main() {
matrix := [][]int{
{1, 2, 3},
{4, 5, 6},
{7, 8, 9},
}
fmt.Println(matrix) // Output: [[1 2 3] [4 5 6] [7 8 9]]
}
Here, matrix
is a slice of slices, representing a 2D array.
8. Conclusion
Slices are a fundamental and powerful feature of Go. They allow for efficient and dynamic manipulation of collections of data. Understanding how to declare, initialize, append, and manipulate slices is crucial for working with collections in Go. Unlike arrays, slices offer more flexibility, making them the preferred choice for most applications in Go programming.
Difference Between Arrays and Slices in Go
1. Introduction
In Go, both arrays and slices are used to store collections of data. While they share some similarities, there are significant differences between the two. Understanding these differences is crucial for choosing the appropriate data structure for your use case. Here’s a comparison of arrays and slices in Go:
2. Array Characteristics
Arrays in Go are fixed-size collections of elements of the same type. Once an array is defined with a specific size, it cannot be resized.
- Fixed Size: The size of an array must be specified at the time of its declaration and cannot be changed later.
- Value Type: Arrays are value types in Go. When you assign one array to another or pass an array to a function, a copy of the entire array is made.
- Memory Allocation: Arrays allocate memory for all of their elements upfront, even if not all elements are used.
Example of declaring an array:
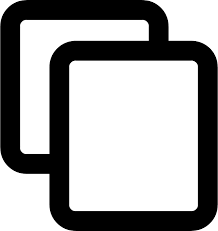
package main
import "fmt"
func main() {
var arr [5]int // Declare an array of 5 integers
fmt.Println(arr) // Output: [0 0 0 0 0] (default values)
}
3. Slice Characteristics
Slices, on the other hand, are more flexible and dynamic. They can be resized and are more commonly used in Go programs.
- Dynamic Size: Slices can grow or shrink dynamically during program execution. They don’t require a fixed size to be specified.
- Reference Type: Slices are reference types. When you assign one slice to another or pass a slice to a function, both refer to the same underlying array, and no copy is made.
- Memory Allocation: Slices use a smaller amount of memory initially and can allocate more as needed when elements are added.
Example of declaring and initializing a slice:
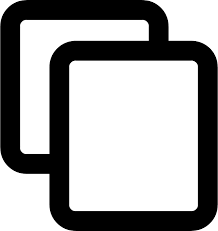
package main
import "fmt"
func main() {
slice := []int{1, 2, 3, 4, 5} // Declare and initialize a slice
fmt.Println(slice) // Output: [1 2 3 4 5]
}
4. Key Differences Between Arrays and Slices
Aspect | Array | Slice |
---|---|---|
Size | Fixed and must be specified at declaration | Dynamic size, can grow or shrink |
Type | Value type (copy made when assigned) | Reference type (no copy made when assigned) |
Memory Allocation | Memory is allocated for all elements upfront | Memory is allocated dynamically, and may change as elements are added or removed |
Use Case | Best for fixed-size collections where the size is known in advance | Best for dynamically-sized collections |
5. Examples to Illustrate the Differences
Array Example
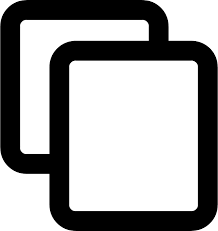
package main
import "fmt"
func main() {
arr1 := [3]int{1, 2, 3} // Declaring an array
arr2 := arr1 // Copying the array
arr2[0] = 10
fmt.Println(arr1) // Output: [1 2 3] (original array is unchanged)
fmt.Println(arr2) // Output: [10 2 3] (modified copy)
}
Slice Example
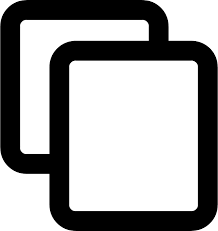
package main
import "fmt"
func main() {
slice1 := []int{1, 2, 3} // Declaring a slice
slice2 := slice1 // Slices refer to the same underlying array
slice2[0] = 10
fmt.Println(slice1) // Output: [10 2 3] (original slice is modified)
fmt.Println(slice2) // Output: [10 2 3] (both slices point to the same array)
}
6. When to Use Arrays vs Slices
Arrays are used when:
- The size of the collection is known ahead of time and does not change.
- Memory efficiency is important, as arrays allocate memory for all elements upfront.
Slices are used when:
- The size of the collection can change during program execution.
- More flexibility and dynamic resizing is needed.
- Memory usage is optimized as slices can grow and shrink as needed without reallocating the entire collection.
7. Conclusion
While arrays and slices in Go may seem similar, they have key differences that make slices the more commonly used data structure. Arrays are useful for fixed-size collections, while slices offer greater flexibility with dynamic sizing. When working with collections in Go, slices are often the preferred choice due to their versatility and ease of use.
Resizing Slices in Go
1. Introduction
In Go, slices are dynamic in nature, and their size can change during execution. Resizing slices involves creating a new slice with a larger or smaller capacity than the original. Go provides built-in functions to resize slices, making it easy to add or remove elements as needed.
2. Expanding a Slice
Expanding a slice means increasing its size by adding more elements. This can be done by using the append()
function, which adds elements to the end of the slice.
Syntax:
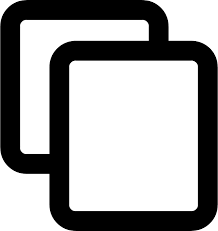
newSlice := append(slice, element1, element2, ...)
The append()
function automatically resizes the slice if necessary and returns a new slice with the added elements.
Example of expanding a slice:
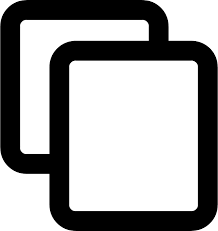
package main
import "fmt"
func main() {
slice := []int{1, 2, 3}
slice = append(slice, 4, 5) // Expanding the slice by adding 4 and 5
fmt.Println(slice) // Output: [1 2 3 4 5]
}
3. Resizing a Slice by Removing Elements
You can resize a slice by removing elements. This can be done by slicing the original slice and excluding the unwanted elements.
Syntax for removing elements:
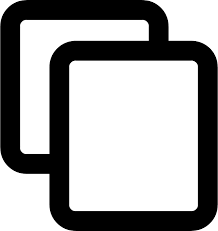
newSlice := slice[:n] // Removes elements from index n onwards
newSlice := slice[m:] // Removes elements from index 0 to m-1
In the first case, the slice will include elements from index 0 to n-1
, and in the second case, it will include elements from index m
to the end of the slice.
Example of removing elements from a slice:
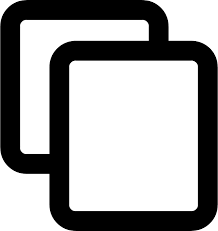
package main
import "fmt"
func main() {
slice := []int{1, 2, 3, 4, 5}
slice = slice[:3] // Removing elements starting from index 3
fmt.Println(slice) // Output: [1 2 3]
}
4. Using the copy()
Function for Resizing
The copy()
function in Go allows you to copy elements from one slice to another. This can be useful when you need to resize a slice manually.
Syntax:
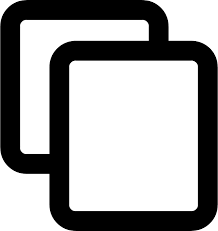
copy(destination, source)
This will copy elements from the source slice to the destination slice. The destination slice must be large enough to accommodate the source slice, or it will copy as much data as it can fit.
Example of resizing a slice using copy()
:
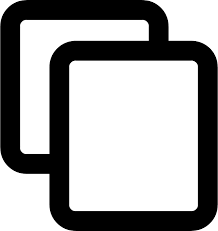
package main
import "fmt"
func main() {
slice := []int{1, 2, 3, 4}
newSlice := make([]int, len(slice) + 2) // Create a new slice with extra capacity
copy(newSlice, slice) // Copy elements from the original slice to the new one
newSlice = append(newSlice, 5, 6) // Add elements at the end of the new slice
fmt.Println(newSlice) // Output: [1 2 3 4 5 6]
}
5. Memory Considerations When Resizing Slices
When you expand a slice using append()
, Go may need to allocate new memory if the slice’s capacity is exceeded. Go will automatically increase the capacity by approximately double, which helps minimize the number of memory allocations. However, resizing a slice frequently can lead to performance overhead due to memory reallocation.
If you know the final size of the slice in advance, you can preallocate memory for the slice using the make()
function, which allows you to avoid multiple allocations during resizing.
Example of preallocating memory for a slice:
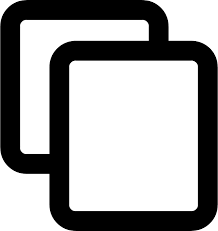
package main
import "fmt"
func main() {
slice := make([]int, 0, 10) // Create a slice with an initial capacity of 10
slice = append(slice, 1, 2, 3) // Adds elements without reallocating memory
fmt.Println(slice) // Output: [1 2 3]
}
6. Conclusion
Resizing slices in Go is a straightforward process, primarily using the append()
function. Slices offer a flexible and efficient way to manage collections of data, allowing you to grow or shrink them dynamically. While resizing slices can involve reallocation of memory, Go optimizes this process to minimize performance issues. By understanding how to resize slices and using the make()
function for preallocation, you can efficiently manage memory and improve the performance of your Go applications.
Slicing an Array in Go
1. Introduction
In Go, arrays are fixed in size, meaning their length is determined when they are created. However, Go provides the ability to slice an array, which allows you to work with a portion of the array without copying the entire array. Slices are more flexible than arrays and are widely used in Go for working with collections of data.
2. Slicing Syntax
The syntax for slicing an array in Go is:
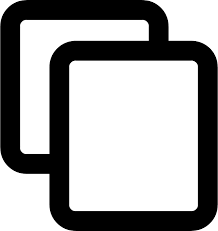
slice := array[startIndex:endIndex]
Here, startIndex
is the index from where the slice starts (inclusive), and endIndex
is the index where the slice ends (exclusive). If no startIndex
or endIndex
is provided, Go will use default values:
- If
startIndex
is omitted, it defaults to 0 (the beginning of the array). - If
endIndex
is omitted, it defaults to the length of the array (the end of the array).
Example of slicing an array:
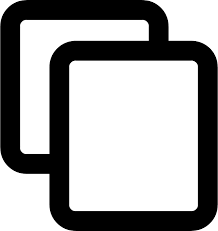
package main
import "fmt"
func main() {
array := [5]int{1, 2, 3, 4, 5}
slice := array[1:4] // Slicing from index 1 to 4 (excluding 4)
fmt.Println(slice) // Output: [2 3 4]
}
3. Slicing with Default Values
You can omit the startIndex
or endIndex
to get a slice starting from the beginning or going up to the end of the array.
Example of slicing with default values:
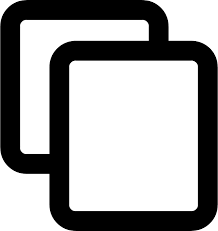
package main
import "fmt"
func main() {
array := [5]int{1, 2, 3, 4, 5}
slice1 := array[:3] // Slicing from the beginning to index 3 (excluding 3)
slice2 := array[2:] // Slicing from index 2 to the end of the array
fmt.Println(slice1) // Output: [1 2 3]
fmt.Println(slice2) // Output: [3 4 5]
}
4. Slicing Beyond Array Boundaries
When slicing an array, if the endIndex
exceeds the array length, Go will automatically adjust it to the array's length. It will not result in an error.
Example of slicing beyond the array boundaries:
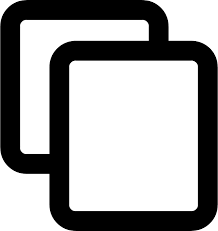
package main
import "fmt"
func main() {
array := [5]int{1, 2, 3, 4, 5}
slice := array[2:10] // endIndex exceeds array length but is automatically adjusted
fmt.Println(slice) // Output: [3 4 5]
}
5. Slicing Arrays of Different Types
Go allows you to slice arrays of any type, whether it's an array of integers, strings, or other types.
Example of slicing an array of strings:
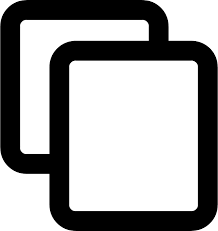
package main
import "fmt"
func main() {
array := [4]string{"apple", "banana", "cherry", "date"}
slice := array[1:3] // Slicing from index 1 to 3 (excluding 3)
fmt.Println(slice) // Output: [banana cherry]
}
6. Slices Are References to Arrays
When you slice an array, you're not creating a new copy of the array. Instead, the slice refers to the same underlying array. This means that modifying the elements of a slice will also modify the corresponding elements in the original array.
Example of modifying the original array through a slice:
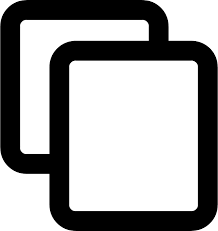
package main
import "fmt"
func main() {
array := [5]int{1, 2, 3, 4, 5}
slice := array[1:4] // Slicing from index 1 to 4
slice[0] = 100 // Modifying the slice
fmt.Println(array) // Output: [1 100 3 4 5]
}
7. Conclusion
Slicing an array in Go is a powerful feature that enables working with subsets of data without duplicating memory. Slices provide flexibility by allowing dynamic resizing, and their behavior is optimized for performance. Understanding how to slice arrays and the implications of slices as references to the original array is crucial for effective Go programming.
Defining Structs in Go
1. Introduction
In Go, a struct is a composite data type that groups together variables (fields) under a single name. Each field in a struct can be of a different type. Structs are commonly used to represent real-world entities and allow you to model complex data structures.
2. Defining a Struct
To define a struct, you use the type
keyword followed by the name of the struct and its fields. Each field in the struct has a name and a data type. The syntax for defining a struct is:
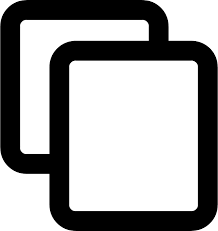
type StructName struct {
Field1 Type1
Field2 Type2
// more fields...
}
Example of defining a simple struct:
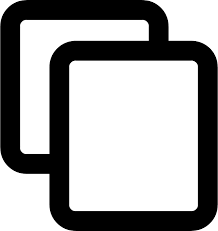
package main
import "fmt"
// Defining a struct called 'Person'
type Person struct {
Name string
Age int
}
func main() {
// Creating an instance of the 'Person' struct
p := Person{Name: "Alice", Age: 30}
fmt.Println(p) // Output: {Alice 30}
}
3. Struct Field Names and Types
Struct fields must have names, and the names must begin with an uppercase letter if they are to be accessed outside the package. Additionally, each field is associated with a data type, which can be any valid Go type, including other structs, arrays, or slices.
4. Instantiating a Struct
You can instantiate a struct in the following ways:
- Using field names: You can specify the values for each field using the field names.
- Using unnamed fields: If the fields in a struct are in the same order and have public types (starting with uppercase letters), you can initialize the struct without using field names.
Examples of struct instantiation:
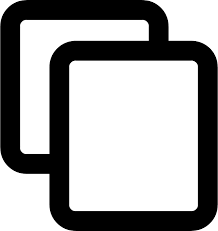
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
// Instantiating using field names
p1 := Person{Name: "Alice", Age: 30}
fmt.Println(p1) // Output: {Alice 30}
// Instantiating without field names
p2 := Person{"Bob", 25}
fmt.Println(p2) // Output: {Bob 25}
}
5. Anonymous Structs
In Go, you can also define anonymous structs, which are structs without a defined name. These are typically used when you need a quick, one-time-use struct.
Example of an anonymous struct:
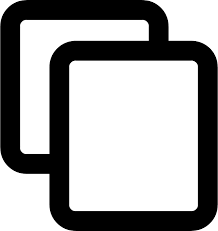
package main
import "fmt"
func main() {
// Defining and instantiating an anonymous struct
p := struct {
Name string
Age int
}{"Charlie", 22}
fmt.Println(p) // Output: {Charlie 22}
}
6. Accessing Struct Fields
You can access the fields of a struct using the dot (.
) notation. For example, to access the Name
field of the Person
struct, you would use p.Name
.
Example of accessing struct fields:
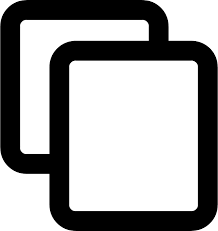
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "Dave", Age: 40}
fmt.Println("Name:", p.Name) // Output: Name: Dave
fmt.Println("Age:", p.Age) // Output: Age: 40
}
7. Structs and Pointers
Structs can also be used with pointers. When you pass a struct by reference, you can modify its fields directly. To create a pointer to a struct, use the &
operator.
Example of using structs with pointers:
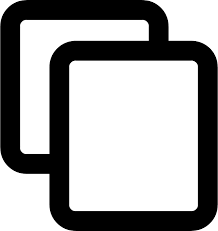
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := &Person{Name: "Eve", Age: 29} // Creating a pointer to the struct
p.Age = 30 // Modifying the field through the pointer
fmt.Println(*p) // Output: {Eve 30}
}
8. Conclusion
Structs are a powerful feature of Go that allow you to group related data together in a single entity. By using structs, you can model complex data structures and better organize your code. Understanding how to define, instantiate, and work with structs is crucial for building Go applications that are both efficient and maintainable.
Accessing Struct Members
1. Introduction
In Go, struct members (or fields) can be accessed using the dot (.) notation. This allows you to retrieve or modify the values stored in the struct fields. Understanding how to access and modify struct members is essential for working with structs effectively in Go.
2. Accessing Struct Members
To access a struct member, you use the struct instance followed by a dot and the field name. If the field is public (starting with an uppercase letter), it can be accessed from outside the struct's package. If the field is private (starting with a lowercase letter), it can only be accessed within the same package.
Example of accessing struct members:
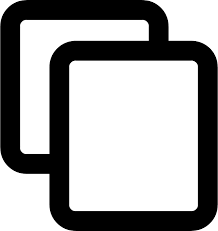
package main
import "fmt"
// Defining a struct called 'Person'
type Person struct {
Name string
Age int
}
func main() {
// Creating an instance of the 'Person' struct
p := Person{Name: "John", Age: 35}
// Accessing struct fields
fmt.Println("Name:", p.Name) // Output: Name: John
fmt.Println("Age:", p.Age) // Output: Age: 35
}
3. Modifying Struct Members
You can also modify struct members by accessing them using the dot notation. If the struct is a pointer, you can modify the fields directly. If it's a value type, you need to pass the struct by reference to modify the members.
Example of modifying struct members:
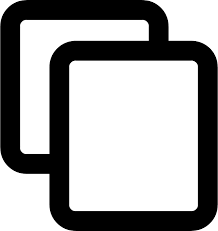
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "Alice", Age: 28}
// Modifying struct fields
p.Name = "Bob"
p.Age = 32
fmt.Println("Updated Person:", p) // Output: Updated Person: {Bob 32}
}
4. Accessing Struct Members Using Pointers
If you have a pointer to a struct, you can access its members directly using the dot notation, even though you are using a pointer. The Go compiler automatically dereferences the pointer for you in this case.
Example of accessing struct members using pointers:
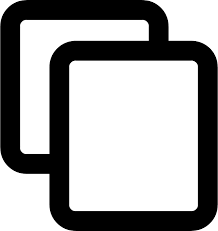
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
// Creating a pointer to a struct
p := &Person{Name: "Charlie", Age: 25}
// Accessing struct fields using the pointer
fmt.Println("Name:", p.Name) // Output: Name: Charlie
fmt.Println("Age:", p.Age) // Output: Age: 25
// Modifying struct fields using the pointer
p.Name = "David"
p.Age = 26
fmt.Println("Updated Person:", *p) // Output: Updated Person: {David 26}
}
5. Accessing Private Struct Members
Fields in Go structs that start with a lowercase letter are private to the package in which the struct is defined. You cannot access private fields directly from outside the package. To expose these fields, you would need to create getter and setter methods.
Example of accessing private struct members:
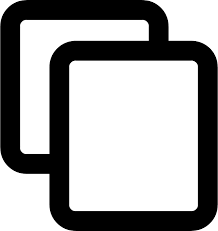
package main
import "fmt"
// Defining a struct with private fields
type Person struct {
name string
age int
}
// Getter method for the private 'name' field
func (p Person) GetName() string {
return p.name
}
// Setter method for the private 'name' field
func (p *Person) SetName(name string) {
p.name = name
}
func main() {
p := Person{age: 30}
// Using setter to modify the private field
p.SetName("Eve")
// Using getter to access the private field
fmt.Println("Name:", p.GetName()) // Output: Name: Eve
fmt.Println("Age:", p.age) // Output: Age: 30
}
6. Conclusion
Accessing struct members in Go is straightforward using the dot notation. You can access both public and private fields, modify their values, and work with structs using pointers. Understanding how to access and manage struct fields is key to effectively using structs in Go to model complex data.
Struct Methods and Functions
1. Introduction
In Go, you can define methods on structs. A method is a function that is associated with a specific type. Methods allow you to define behavior for your structs, enabling you to encapsulate functionality alongside your data. A function can be made into a method by associating it with a struct type via a receiver.
2. Defining Methods on Structs
To define a method for a struct, you need to specify a receiver. The receiver is the instance of the struct that the method operates on. It is specified in the method declaration and can be either a value receiver or a pointer receiver.
Example of defining a method on a struct:
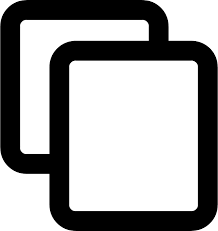
package main
import "fmt"
// Defining a struct called 'Person'
type Person struct {
Name string
Age int
}
// Defining a method on 'Person' type
// This is a value receiver
func (p Person) Greet() {
fmt.Printf("Hello, my name is %s and I am %d years old.\n", p.Name, p.Age)
}
func main() {
// Creating an instance of 'Person'
p := Person{Name: "Alice", Age: 30}
// Calling the method on the struct instance
p.Greet() // Output: Hello, my name is Alice and I am 30 years old.
}
3. Value Receiver vs Pointer Receiver
In Go, methods can be defined using either a value receiver or a pointer receiver. The choice of receiver determines how the method operates on the struct.
- Value Receiver: The method works with a copy of the struct. Changes made to the struct inside the method do not affect the original struct.
- Pointer Receiver: The method works with the original struct itself, allowing modifications to the struct inside the method.
Example with value receiver:
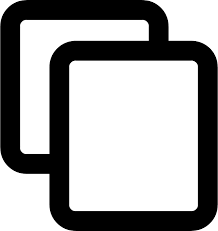
package main
import "fmt"
// Defining a struct called 'Person'
type Person struct {
Name string
Age int
}
// Method with a value receiver
func (p Person) HaveBirthday() {
p.Age++
fmt.Printf("Happy Birthday! You are now %d years old.\n", p.Age)
}
func main() {
p := Person{Name: "John", Age: 40}
p.HaveBirthday() // Output: Happy Birthday! You are now 41 years old.
fmt.Println(p.Age) // Output: 40 (Age is not modified because of value receiver)
}
Example with pointer receiver:
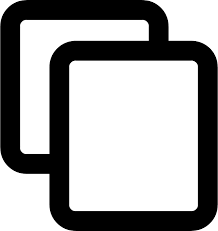
package main
import "fmt"
type Person struct {
Name string
Age int
}
// Method with a pointer receiver
func (p *Person) HaveBirthday() {
p.Age++
fmt.Printf("Happy Birthday! You are now %d years old.\n", p.Age)
}
func main() {
p := Person{Name: "John", Age: 40}
p.HaveBirthday() // Output: Happy Birthday! You are now 41 years old.
fmt.Println(p.Age) // Output: 41 (Age is modified because of pointer receiver)
}
4. Methods vs Functions
Methods are functions that are associated with a specific type, whereas functions are standalone and not tied to any type. The main difference is that methods have a receiver, which is the instance of the type the method operates on, while functions do not.
Example of a function (not associated with a struct):
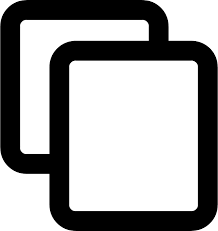
package main
import "fmt"
// Function with no receiver
func Greet(name string) {
fmt.Printf("Hello, %s!\n", name)
}
func main() {
Greet("Alice") // Output: Hello, Alice!
}
5. Conclusion
Struct methods in Go allow you to associate behavior with data, making your program more object-oriented. You can define methods with value receivers or pointer receivers depending on whether you want to modify the struct. Understanding the difference between methods and functions, as well as how to use receivers, is crucial for writing clean and efficient Go code.
Anonymous Structs
1. Introduction to Anonymous Structs
An anonymous struct in Go is a struct that is defined without a name. You define an anonymous struct directly when you create an instance of it, without needing to explicitly declare the struct type beforehand. Anonymous structs are useful when you need a quick, one-off data structure that doesn’t require a separate type definition.
2. Declaring and Using Anonymous Structs
Anonymous structs are commonly used in situations where you need a simple data structure to hold related fields temporarily. They are defined inline when creating an instance, and you can access their fields just like regular structs.
Example of declaring and using an anonymous struct:
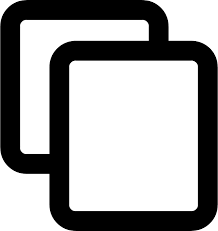
package main
import "fmt"
func main() {
// Creating an anonymous struct with fields directly in the instance
person := struct {
Name string
Age int
}{
Name: "Alice",
Age: 30,
}
// Accessing fields of the anonymous struct
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
}
3. Anonymous Structs in Arrays and Slices
Anonymous structs can also be used in arrays and slices. This allows you to store multiple instances of anonymous structs in a collection.
Example with an anonymous struct in a slice:
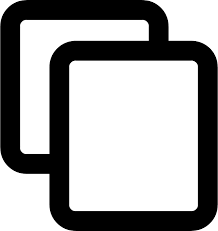
package main
import "fmt"
func main() {
// Creating a slice of anonymous structs
people := []struct {
Name string
Age int
}{
{Name: "Alice", Age: 30},
{Name: "Bob", Age: 25},
{Name: "Charlie", Age: 35},
}
// Looping through the slice and printing the fields
for _, person := range people {
fmt.Printf("Name: %s, Age: %d\n", person.Name, person.Age)
}
}
4. Advantages of Using Anonymous Structs
- Simplicity: You don’t need to create a separate type definition when you only need a struct temporarily.
- Conciseness: Saves space and avoids cluttering your code with unnecessary type definitions, especially for small, one-time use structures.
- Flexibility: Useful for quick data storage or when you are working with temporary data structures in functions or arrays.
5. Disadvantages of Using Anonymous Structs
- Reusability: Anonymous structs are not reusable. If you need the same structure in multiple places, you’ll have to duplicate the definition each time.
- Readability: Overuse of anonymous structs can make your code harder to understand because the structure is defined inline and lacks a descriptive name.
6. Conclusion
Anonymous structs provide a convenient way to define temporary data structures without the need for named types. They are particularly useful for quick, one-off data storage, but their use should be balanced to avoid readability and maintainability issues. For more complex or reusable structures, it’s better to define a named struct type instead.
Pointer to Structs
1. Introduction to Pointer to Structs
In Go, a pointer to a struct is a variable that holds the memory address of a struct rather than the struct itself. Pointers allow you to pass large structures efficiently to functions and methods without copying the entire struct. Using pointers to structs allows you to modify the original struct directly, as opposed to working with a copy.
2. Declaring and Using Pointers to Structs
A pointer to a struct is declared using the `&` operator, which gives the memory address of a struct instance. To access fields via a pointer, you can use the `->` operator (in C/C++) or the `.` operator (in Go), which automatically dereferences the pointer.
Example of declaring and using a pointer to a struct:
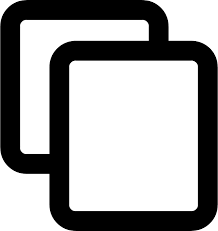
package main
import "fmt"
// Defining a struct type
type Person struct {
Name string
Age int
}
func main() {
// Creating an instance of the struct
person := Person{Name: "Alice", Age: 30}
// Declaring a pointer to the struct using the & operator
personPointer := &person
// Accessing struct fields using the pointer
fmt.Println("Name:", personPointer.Name)
fmt.Println("Age:", personPointer.Age)
// Modifying struct fields via the pointer
personPointer.Age = 31
fmt.Println("Updated Age:", personPointer.Age)
}
3. Passing a Pointer to a Function
When you pass a pointer to a function, you can modify the original struct data. This is more efficient than passing a large struct by value, as it avoids creating a copy of the struct.
Example of passing a pointer to a function:
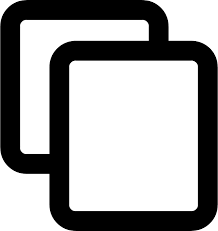
package main
import "fmt"
type Person struct {
Name string
Age int
}
// Function that modifies the struct via a pointer
func updateAge(person *Person) {
person.Age += 1 // Modifies the original struct
fmt.Println("Updated Age inside function:", person.Age)
}
func main() {
person := Person{Name: "Alice", Age: 30}
// Passing a pointer to the function
updateAge(&person)
// The original struct is modified
fmt.Println("Updated Age outside function:", person.Age)
}
4. Struct Methods with Pointers
In Go, methods can be defined on both value receivers and pointer receivers. When you define a method on a pointer receiver, you can modify the struct directly inside the method. This is useful when you want to change the state of the struct within the method.
Example of using pointer receivers in struct methods:
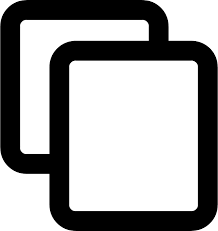
package main
import "fmt"
type Person struct {
Name string
Age int
}
// Method with a pointer receiver
func (p *Person) celebrateBirthday() {
p.Age++ // Modifies the original struct
fmt.Println("Happy Birthday!", p.Name, "is now", p.Age, "years old.")
}
func main() {
person := Person{Name: "Alice", Age: 30}
// Calling the method with a pointer to the struct
person.celebrateBirthday()
// The original struct is modified
fmt.Println("New Age after Birthday:", person.Age)
}
5. Advantages of Using Pointers to Structs
- Efficiency: Passing pointers to structs avoids copying large data structures, making your program more efficient in terms of memory and performance.
- Modifying Original Data: Pointers allow you to directly modify the fields of a struct, making it easier to change the data in functions or methods.
- Memory Management: With pointers, you have control over memory allocation and can avoid unnecessary memory duplication.
6. Disadvantages of Using Pointers to Structs
- Complexity: Working with pointers can add complexity to your code, especially for beginners. It requires careful memory management to avoid errors like dereferencing nil pointers.
- Potential for Bugs: Improper use of pointers can lead to bugs, such as accidental data modification or dereferencing invalid memory locations.
7. Conclusion
Pointers to structs are a powerful feature in Go that provide efficiency and flexibility. They allow you to pass and modify large data structures without duplicating them, making your programs more memory-efficient. However, pointers should be used carefully, as they introduce complexity and can lead to potential bugs if not handled properly. Understanding how and when to use pointers will help you write better, more efficient Go programs.
Introduction to Pointers
1. What is a Pointer in Go?
A pointer in Go is a variable that stores the memory address of another variable. Rather than holding the value of the data directly, a pointer holds the address where the data is located in memory. This allows you to efficiently pass large structures or variables to functions without copying the data itself, and also modify the original data from within functions.
2. Declaring Pointers
In Go, pointers are declared using the `*` symbol. To declare a pointer, you specify the type of data the pointer will point to, followed by the `*` symbol. The pointer will then hold the memory address of a variable of that type.
Example of declaring a pointer:
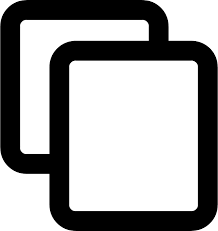
var ptr *int
Here, `ptr` is a pointer to an integer, meaning it will hold the memory address of an integer variable.
3. Assigning Values to Pointers
To assign a value to a pointer, you use the `&` operator, which gives you the memory address of a variable. This is called the "address of" operator.
Example of assigning a value to a pointer:
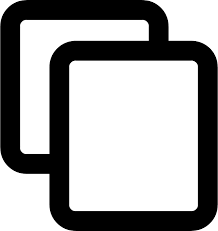
var num int = 10
var ptr *int = &num // ptr now holds the memory address of num
4. Dereferencing Pointers
Dereferencing a pointer means accessing the value stored at the memory address the pointer is holding. You can dereference a pointer using the `*` operator, which gives you the value stored at the address the pointer points to.
Example of dereferencing a pointer:
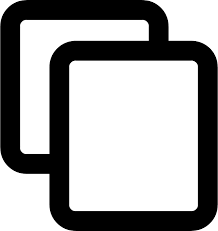
fmt.Println(*ptr) // Prints the value of num, which is 10
5. Pointers and Memory Management
Go automatically handles memory management through garbage collection, which means you don't need to manually allocate or deallocate memory. However, understanding pointers is important because they allow efficient memory usage and manipulation of data structures.
6. Example: Using Pointers
Here’s an example to demonstrate how pointers work in Go:
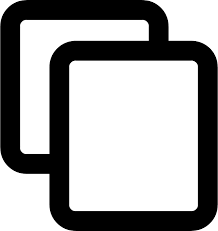
package main
import "fmt"
func main() {
var num int = 42
var ptr *int = &num // Pointer to the address of num
fmt.Println("Value of num:", num) // Prints: 42
fmt.Println("Address of num:", &num) // Prints: memory address of num
fmt.Println("Value pointed to by ptr:", *ptr) // Dereferencing ptr, prints: 42
*ptr = 58 // Modifying the value at the memory address using the pointer
fmt.Println("Updated value of num:", num) // Prints: 58
}
7. Why Use Pointers?
Pointers are used for several reasons in Go:
- Efficiency: Passing large structures or data by pointer avoids unnecessary copying, improving performance.
- Mutability: Pointers allow functions to modify the original value of a variable, rather than working with a copy.
- Memory Management: By using pointers, Go helps manage memory more efficiently, especially with large data structures.
8. Conclusion
Pointers are an important concept in Go that allow you to work with memory addresses directly. They enable efficient data manipulation and are fundamental to functions that modify variables directly. Understanding pointers is essential for writing optimized and memory-efficient Go programs.
Working with Pointers
1. Introduction to Working with Pointers
In Go, working with pointers allows you to directly manipulate memory locations, which is useful for modifying data outside of a function, improving efficiency, and managing large data structures. Understanding how to work with pointers can significantly enhance your ability to write optimized and memory-efficient code.
2. Declaring and Assigning Pointers
To declare a pointer, you use the `*` symbol to specify the type of data the pointer will refer to. To assign a pointer, you use the `&` operator to obtain the memory address of a variable.
Example of declaring and assigning a pointer:
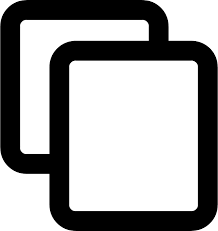
var x int = 10
var ptr *int = &x // ptr holds the address of x
3. Dereferencing Pointers
Dereferencing a pointer means accessing the value stored at the memory address that the pointer holds. You can dereference a pointer using the `*` operator.
Example of dereferencing a pointer:
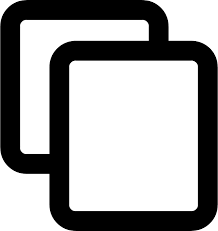
fmt.Println(*ptr) // Dereferencing ptr to print the value of x, which is 10
4. Modifying Values Through Pointers
When you use pointers, you can modify the value of the variable the pointer is pointing to. By dereferencing the pointer, you can access and modify the value at the memory location.
Example of modifying values through pointers:
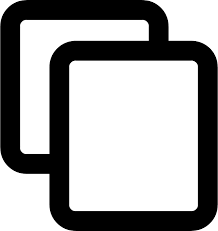
*ptr = 20 // Modify the value of x through ptr
fmt.Println(x) // Prints: 20
5. Passing Pointers to Functions
One of the most common use cases for pointers is passing them to functions. This allows the function to modify the original value of a variable rather than working on a copy of it. You can pass pointers as arguments to functions by using the `*` symbol in the function declaration.
Example of passing pointers to functions:
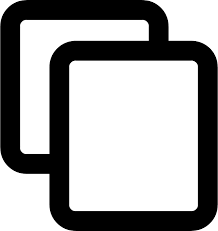
package main
import "fmt"
func updateValue(ptr *int) {
*ptr = 30 // Dereferencing ptr to modify the value
}
func main() {
var num int = 10
fmt.Println("Before:", num) // Prints: 10
updateValue(&num) // Pass the address of num to the function
fmt.Println("After:", num) // Prints: 30
}
6. Pointer Arithmetic in Go
Go does not allow pointer arithmetic like in languages such as C or C++. This restriction is in place to prevent pointer manipulation errors and improve safety. Instead, Go provides safe ways to work with slices, arrays, and other data structures.
7. Pointers to Structs
In Go, you can use pointers to structs to modify the fields of the struct directly. This is particularly useful when working with large structs, as it allows you to avoid copying the entire structure each time it is passed to a function.
Example of using pointers to structs:
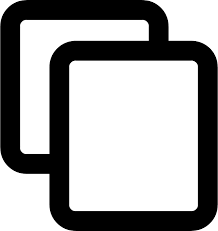
type Person struct {
name string
age int
}
func updatePerson(p *Person) {
p.name = "John" // Modify the name field through the pointer
p.age = 28 // Modify the age field through the pointer
}
func main() {
person := Person{name: "Alice", age: 25}
fmt.Println("Before update:", person) // Prints: {Alice 25}
updatePerson(&person) // Pass pointer to struct
fmt.Println("After update:", person) // Prints: {John 28}
}
8. Creating Pointers to Arrays and Slices
You can also create pointers to arrays and slices in Go. This allows you to modify the array or slice directly without copying it. However, remember that arrays in Go are fixed in size, while slices are dynamically sized.
Example of creating pointers to arrays:
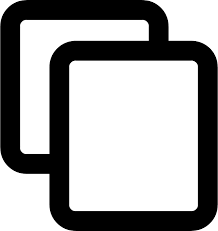
var arr [3]int = [3]int{1, 2, 3}
var ptr *[3]int = &arr // Pointer to an array
9. Pointer Nil and Safety
In Go, pointers can be `nil` by default when they are declared but not initialized. Dereferencing a `nil` pointer will cause a runtime panic. It’s important to check if a pointer is `nil` before dereferencing it to avoid errors.
Example of checking for a `nil` pointer:
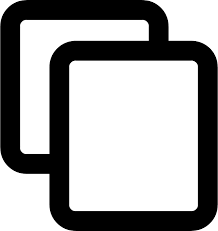
var ptr *int
if ptr != nil {
fmt.Println(*ptr)
} else {
fmt.Println("Pointer is nil")
}
10. Conclusion
Working with pointers in Go allows you to efficiently manage memory and modify variables outside the scope of functions. Understanding how to declare, assign, dereference, and pass pointers to functions is essential for writing optimized and memory-efficient programs. Go’s pointer system is designed with safety in mind, eliminating many potential errors found in other languages that allow pointer arithmetic.
Dereferencing Pointers
1. What is Dereferencing?
Dereferencing a pointer in Go means accessing the value that the pointer is pointing to. When you have a pointer, it stores the memory address of a variable, and dereferencing that pointer gives you access to the value stored at that address.
In Go, the `*` operator is used for both declaring a pointer and dereferencing it. When you declare a pointer, the `*` symbol indicates the type that the pointer will point to. When you use the `*` symbol with a pointer variable, it accesses the value stored at the memory address the pointer holds, i.e., dereferencing the pointer.
2. Example of Dereferencing a Pointer
Here’s a simple example to demonstrate dereferencing a pointer:
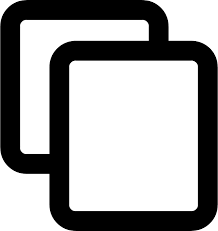
package main
import "fmt"
func main() {
var x int = 10
var ptr *int = &x // ptr holds the address of x
fmt.Println("Value of x:", x) // Prints: 10
fmt.Println("Address of x:", &x) // Prints: memory address of x
fmt.Println("Value pointed to by ptr:", *ptr) // Dereferencing ptr prints: 10
}
3. How Dereferencing Works
When you declare a pointer and assign it the address of a variable, it points to that variable in memory. To access or modify the value stored at that address, you dereference the pointer using the `*` operator. This allows you to work with the value directly rather than the memory address.
Example of modifying a variable through a pointer:
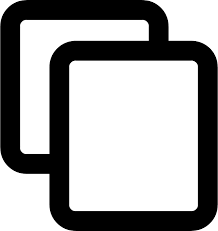
package main
import "fmt"
func main() {
var x int = 5
var ptr *int = &x // ptr points to the address of x
fmt.Println("Before:", x) // Prints: Before: 5
*ptr = 20 // Dereferencing ptr to modify the value of x
fmt.Println("After:", x) // Prints: After: 20
}
4. Dereferencing Pointers in Functions
Dereferencing pointers is often used when passing pointers to functions. By dereferencing the pointer inside a function, you can modify the value of the original variable that the pointer refers to.
Example of dereferencing pointers in a function:
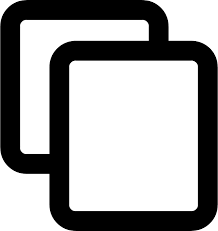
package main
import "fmt"
func updateValue(ptr *int) {
*ptr = 50 // Dereferencing ptr to update the value of the variable
}
func main() {
var num int = 10
fmt.Println("Before update:", num) // Prints: 10
updateValue(&num) // Passing the address of num
fmt.Println("After update:", num) // Prints: 50
}
5. Dereferencing Nil Pointers
If a pointer is `nil`, dereferencing it will cause a runtime panic. It’s important to check if a pointer is `nil` before dereferencing it to avoid errors.
Example of checking for `nil` pointers before dereferencing:
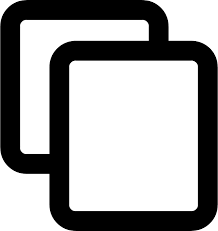
package main
import "fmt"
func main() {
var ptr *int
if ptr != nil {
fmt.Println(*ptr)
} else {
fmt.Println("Pointer is nil")
}
}
6. Conclusion
Dereferencing pointers in Go allows you to access or modify the value of variables indirectly by working with their memory addresses. It’s an essential concept when working with pointers to manipulate data efficiently. However, always ensure that the pointer is not `nil` before dereferencing it to avoid runtime errors.
Pointers and Structs
1. What Are Pointers and Structs?
In Go, pointers are used to store the memory addresses of variables. A struct, on the other hand, is a composite data type that groups together variables (fields) of different types under one name. Structs are commonly used to model real-world entities in Go programs.
Pointers to structs allow you to reference the struct’s memory address, making it possible to modify the contents of the struct without passing the entire struct around. This is particularly useful for efficiency and to avoid unnecessary memory allocation.
2. Declaring Pointers to Structs
You can declare a pointer to a struct by using the `&` operator to get the memory address of a struct variable.
Here’s an example of declaring a pointer to a struct:
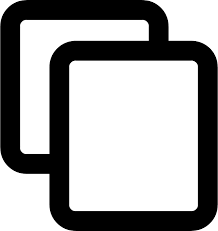
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "John", Age: 30}
ptr := &p // Pointer to struct
fmt.Println("Pointer to struct:", ptr) // Prints the memory address of p
fmt.Println("Name through pointer:", ptr.Name) // Accessing struct fields through the pointer
}
3. Modifying Struct Fields Through Pointers
When you have a pointer to a struct, you can modify the struct's fields directly by dereferencing the pointer or using the pointer syntax to access fields.
Here’s an example of modifying a struct field through a pointer:
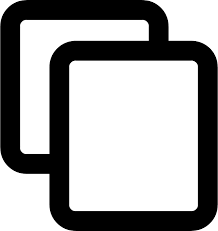
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "John", Age: 30}
ptr := &p // Pointer to struct
fmt.Println("Before:", ptr.Name) // Prints: John
ptr.Name = "Jane" // Modifying struct field through pointer
fmt.Println("After:", ptr.Name) // Prints: Jane
}
4. Using Pointers to Structs in Functions
When passing structs to functions, using a pointer allows the function to modify the original struct. Without a pointer, Go passes structs by value, meaning that the function works with a copy of the struct.
Here’s how you can pass a pointer to a struct to a function:
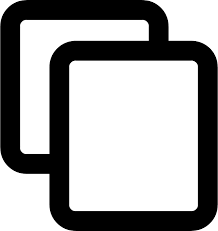
package main
import "fmt"
type Person struct {
Name string
Age int
}
func updateAge(p *Person) {
p.Age = 35 // Modify struct field via pointer
}
func main() {
p := Person{Name: "John", Age: 30}
fmt.Println("Before:", p.Age) // Prints: 30
updateAge(&p) // Passing the address of p
fmt.Println("After:", p.Age) // Prints: 35
}
5. Using Pointers with Anonymous Structs
It’s also possible to use pointers with anonymous structs. Anonymous structs are structs that are defined without a named type. You can create an instance of an anonymous struct and pass a pointer to it.
Example with an anonymous struct:
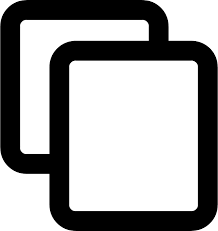
package main
import "fmt"
func main() {
// Anonymous struct
p := &struct {
Name string
Age int
}{
Name: "John",
Age: 30,
}
fmt.Println("Name:", p.Name) // Prints: John
p.Name = "Jane" // Modify the Name field
fmt.Println("Updated Name:", p.Name) // Prints: Jane
}
6. Conclusion
Pointers to structs are a powerful feature in Go. They allow you to work efficiently with struct data, modify struct fields, and pass structs to functions without making unnecessary copies. Understanding how to use pointers with structs can significantly improve the performance of your Go applications, especially when dealing with large or complex data structures.
Pointers to Functions
1. What Are Pointers to Functions?
In Go, functions are first-class citizens, meaning you can assign functions to variables, pass them as arguments, and even return them from other functions. A pointer to a function is a reference to a function’s memory address, allowing you to invoke the function indirectly via the pointer.
Using pointers to functions can be helpful when you need to pass functions around dynamically or store them for later execution, such as in event-driven programming or callback mechanisms.
2. Declaring and Assigning Pointers to Functions
To declare a pointer to a function, you need to specify the function’s type, including its parameter types and return type. You can then assign the function to the pointer and use the pointer to invoke the function.
Here’s an example of declaring and assigning a pointer to a function:
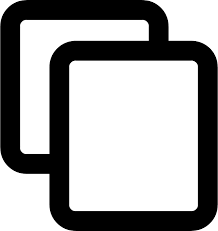
package main
import "fmt"
// Function that takes two integers and returns their sum
func add(a, b int) int {
return a + b
}
func main() {
// Declare a pointer to the add function
var funcPtr func(int, int) int
// Assign the add function to the pointer
funcPtr = add
// Use the function pointer to call the function
result := funcPtr(3, 4)
fmt.Println("Sum:", result) // Prints: Sum: 7
}
3. Passing Function Pointers as Arguments
You can pass a pointer to a function as an argument to another function. This is useful for creating flexible code, as the function behavior can change depending on the function pointer passed.
Here’s an example of passing a function pointer to another function:
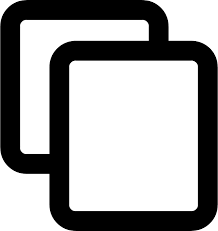
package main
import "fmt"
// Function that takes two integers and returns their sum
func add(a, b int) int {
return a + b
}
// Function that accepts a function pointer and uses it
func compute(a, b int, operation func(int, int) int) int {
return operation(a, b)
}
func main() {
// Use the compute function with a pointer to the add function
result := compute(3, 4, add)
fmt.Println("Sum via function pointer:", result) // Prints: Sum via function pointer: 7
}
4. Returning Function Pointers from Functions
You can also return a function pointer from another function, which can be useful for creating function factories or handlers that are selected based on conditions.
Here’s an example of returning a function pointer:
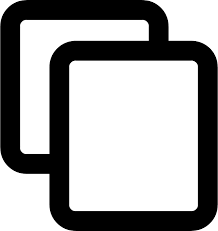
package main
import "fmt"
// Function that returns another function based on a condition
func getOperation(op string) func(int, int) int {
if op == "add" {
return func(a, b int) int {
return a + b
}
}
return func(a, b int) int { // Default subtraction
return a - b
}
}
func main() {
// Get a function pointer for addition
addFunc := getOperation("add")
result := addFunc(3, 4)
fmt.Println("Sum:", result) // Prints: Sum: 7
// Get a function pointer for subtraction
subFunc := getOperation("subtract")
result2 := subFunc(10, 4)
fmt.Println("Difference:", result2) // Prints: Difference: 6
}
5. Conclusion
Pointers to functions enable dynamic function invocation, adding a layer of flexibility to Go programs. By storing function references, you can pass functions around, modify their behavior, and create more modular and reusable code. Whether you're using function pointers for callbacks, event handling, or implementing strategies, understanding this concept will make your Go programming more versatile and powerful.
Go’s Garbage Collection
1. What is Garbage Collection in Go?
Garbage collection in Go is the automatic process of identifying and reclaiming memory that is no longer in use by the program. This process helps to free up resources, preventing memory leaks and optimizing memory management without requiring explicit memory handling by the programmer.
Go uses a concurrent garbage collector (GC) that operates in the background, allowing the application to continue execution while memory management tasks are being performed. This reduces the likelihood of performance bottlenecks during memory cleanup.
2. How Garbage Collection Works in Go?
Go’s garbage collector is designed to minimize pause times and maintain the performance of applications. It uses a mark-and-sweep algorithm in the following way:
- Mark Phase: The garbage collector identifies all live objects that are still in use by the program. It starts from root objects (such as global variables and stacks) and recursively marks all reachable objects.
- Sweep Phase: After marking, the garbage collector sweeps through the heap memory and deallocates memory occupied by unreachable objects, making it available for future use.
Go’s garbage collector is concurrent, meaning it can run in parallel with the program’s execution. This reduces the impact on application performance while the garbage collection process is running.
3. The Role of the Heap and Stack in Garbage Collection
In Go, memory is allocated on either the heap or the stack. Go’s garbage collector primarily manages heap memory, where long-lived objects are stored. Stack memory, which holds short-lived variables, is automatically cleaned up when the function returns, without requiring garbage collection.
4. The Impact of Garbage Collection on Performance
While garbage collection helps avoid memory leaks, it can also have performance implications, particularly during long-running or memory-intensive applications. Go’s garbage collector is designed to minimize pause times, but in some cases, it can still cause latency or performance degradation.
To optimize the garbage collection process, Go offers the following techniques:
- Reducing Allocations: Minimize unnecessary memory allocations by reusing objects and managing memory efficiently.
- Using Object Pooling: Use object pools to reuse objects and avoid frequent allocations and deallocations.
- Controlling Garbage Collection Frequency: The garbage collector runs automatically, but you can influence its behavior using the
GODEBUG
environment variable to control the GC’s frequency and behavior.
5. Tuning Go’s Garbage Collector
Go provides environment variables that allow you to tune garbage collection behavior. For example:
- GOGC: This variable controls the garbage collection target percentage. The default is 100, meaning the garbage collector will run when the heap size doubles. Setting it to a lower value can make GC run more frequently, while a higher value reduces its frequency.
- GODEBUG: This variable can be used to enable debugging for the garbage collector, helping identify performance issues and track memory usage.
6. Example of Garbage Collection in Go
Here’s an example demonstrating how Go’s garbage collector works:
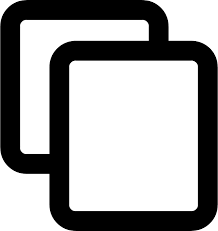
package main
import "fmt"
// Example of allocating memory and triggering garbage collection
func main() {
// Allocate memory for large objects
largeArray := make([]int, 1000000)
// Use the allocated memory to simulate work
largeArray[0] = 1
largeArray[999999] = 2
fmt.Println("Memory allocated.")
// At this point, the largeArray is no longer in use
// The garbage collector will eventually free this memory
// Simulate some work
for i := 0; i < 100000; i++ {
fmt.Println(i)
}
// The largeArray will be garbage collected once it is no longer in scope
}
7. Conclusion
Go’s garbage collector is a powerful tool that helps manage memory automatically, reducing the need for manual memory management and preventing memory leaks. By understanding how the garbage collector works and tuning its behavior, developers can optimize their Go applications for better performance, especially in memory-intensive applications.
What are Maps in Go?
1. Introduction to Maps in Go
Maps in Go are unordered collections of key-value pairs, where each key is unique, and is associated with a specific value. Maps are built-in data structures in Go that provide efficient lookups, inserts, and deletions of data, making them an essential part of many Go programs.
Maps are similar to dictionaries in Python or hash tables in other languages. They allow you to store data and retrieve it quickly using a key, which is hashed to provide an efficient lookup mechanism.
2. Declaring and Initializing Maps
You can declare and initialize maps in Go in several ways:
- Using the
make
function: You can create a map using the built-inmake
function. - Using map literals: You can also initialize maps using map literals.
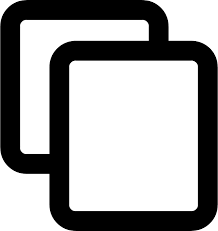
m := make(map[string]int)
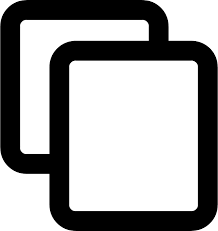
m := map[string]int{"apple": 5, "banana": 3}
3. Adding and Accessing Elements in a Map
To add elements to a map, you use the key as an index to assign a value:
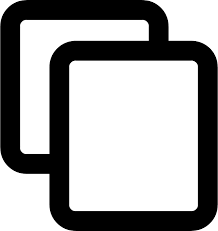
m["orange"] = 10
To access an element, you simply use the key:
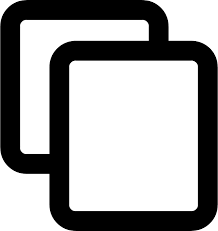
value := m["apple"]
If the key does not exist in the map, it returns the zero value for the map's value type (e.g., 0 for integers, "" for strings).
4. Deleting Elements from a Map
To delete a key-value pair from a map, use the delete
function:
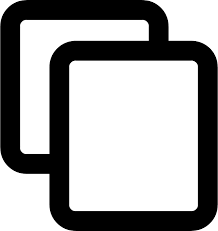
delete(m, "banana")
This removes the key "banana" and its associated value from the map.
5. Checking if a Key Exists in a Map
When accessing a map, you can also check if a key exists by using the two-value assignment form. The second value returned is a boolean indicating whether the key was found:
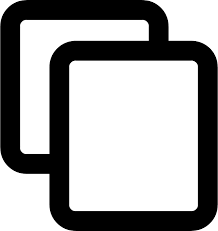
value, ok := m["orange"]
if ok {
fmt.Println("Found:", value)
} else {
fmt.Println("Key not found")
}
6. Iterating Over a Map
You can iterate over a map using a for
loop:
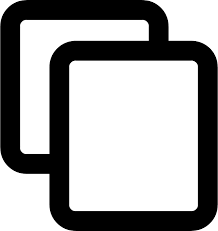
for key, value := range m {
fmt.Println(key, value)
}
This loop will print each key-value pair in the map.
7. Maps and Memory Management
Maps in Go are dynamically sized, meaning they automatically resize as elements are added or removed. However, since maps are reference types, they are allocated on the heap, and the garbage collector is responsible for cleaning up unused maps when they go out of scope.
8. Example of Using Maps in Go
Here’s an example demonstrating the use of maps in Go:
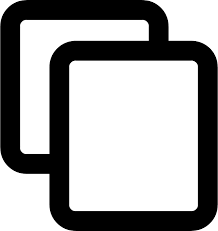
package main
import "fmt"
func main() {
// Declaring and initializing a map
m := map[string]int{"apple": 5, "banana": 3, "orange": 10}
// Adding an item to the map
m["grape"] = 7
// Accessing an item
fmt.Println("Apple count:", m["apple"])
// Checking if a key exists
value, ok := m["banana"]
if ok {
fmt.Println("Banana count:", value)
} else {
fmt.Println("Banana not found")
}
// Deleting an item
delete(m, "orange")
// Iterating over the map
for key, value := range m {
fmt.Println(key, value)
}
}
9. Conclusion
Maps are a powerful data structure in Go, providing efficient key-value pair storage and retrieval. By understanding how to declare, initialize, access, and manipulate maps, you can handle large amounts of data efficiently in your Go programs.
Declaring and Initializing Maps in Go
1. Declaring a Map
In Go, a map is a built-in data type that associates keys with values. To declare a map, you use the make
function or a map literal. Here are two common ways to declare a map:
- Using the
make
function: Themake
function creates a map with the specified key and value types. - Using a map literal: A map can also be initialized directly using a map literal, which allows you to specify initial key-value pairs.
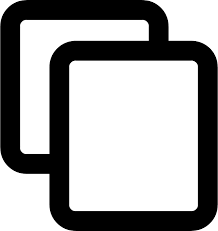
m := make(map[string]int)
In this example, the map m
is declared with keys of type string
and values of type int
.
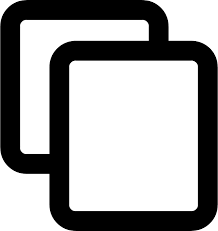
m := map[string]int{"apple": 5, "banana": 3}
This creates a map with two key-value pairs: "apple" maps to 5, and "banana" maps to 3.
2. Initializing a Map with make
Function
You can initialize an empty map with make
, and later add key-value pairs:
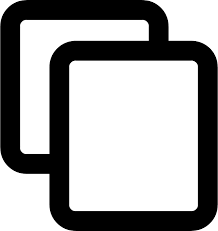
m := make(map[string]int)
After declaring the map, you can add elements like this:
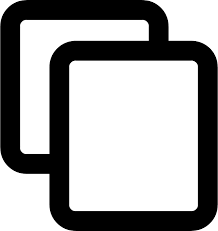
m["apple"] = 5
m["banana"] = 3
In this example, we first create an empty map and then add key-value pairs for "apple" and "banana".
3. Initializing a Map with a Map Literal
A map can also be initialized with values directly using a map literal:
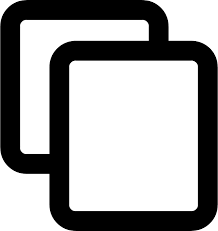
m := map[string]int{"apple": 5, "banana": 3, "cherry": 8}
This is a more concise way to declare and initialize a map with predefined key-value pairs.
4. Example of Declaring and Initializing Maps
Here is an example that demonstrates both declaring and initializing maps:
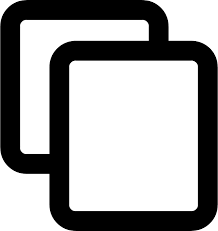
package main
import "fmt"
func main() {
// Declaring and initializing a map with the make function
m1 := make(map[string]int)
m1["apple"] = 5
m1["banana"] = 3
// Declaring and initializing a map with a map literal
m2 := map[string]int{"orange": 10, "grape": 7}
fmt.Println("Map 1:", m1)
fmt.Println("Map 2:", m2)
}
This code declares two maps: m1
using make
and m2
using a map literal. After initializing the maps with key-value pairs, it prints both maps.
5. Conclusion
In Go, maps can be declared and initialized using either the make
function or map literals. The make
function is useful when you want to initialize an empty map and add elements later, while map literals provide a more concise way to initialize a map with predefined key-value pairs.
Accessing and Modifying Map Elements in Go
1. Accessing Map Elements
To access the value associated with a specific key in a map, you use the key within square brackets []
. Here's how you can access map elements:
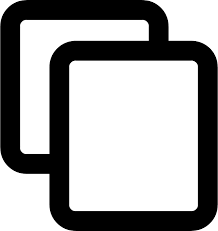
m := map[string]int{"apple": 5, "banana": 3}
value := m["apple"]
fmt.Println(value) // Output: 5
In this example, the value of the key "apple"
is retrieved and stored in the variable value
.
2. Checking if a Key Exists in a Map
When accessing an element, you can check if the key exists in the map using the second return value:
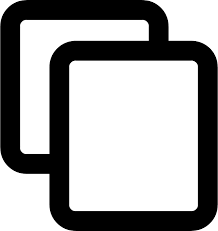
value, exists := m["apple"]
if exists {
fmt.Println("Apple exists:", value)
} else {
fmt.Println("Apple does not exist")
}
The exists
variable will be true
if the key is found, and false
if the key is not in the map. This is useful to avoid accessing non-existent keys.
3. Modifying Map Elements
You can modify the value associated with an existing key by simply assigning a new value to that key:
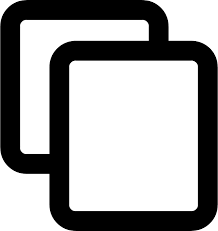
m["apple"] = 10
fmt.Println(m["apple"]) // Output: 10
In this example, the value of the key "apple"
is updated to 10
.
4. Adding New Elements to a Map
If the key doesn't already exist in the map, assigning a value to a new key will add that key-value pair to the map:
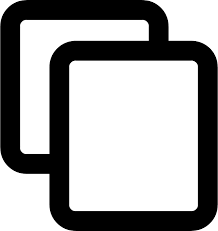
m["cherry"] = 7
fmt.Println(m["cherry"]) // Output: 7
In this example, a new key "cherry"
with the value 7
is added to the map.
5. Example of Accessing and Modifying Map Elements
Here is a complete example of accessing and modifying map elements:
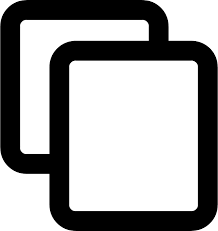
package main
import "fmt"
func main() {
// Declaring and initializing a map
m := map[string]int{"apple": 5, "banana": 3}
// Accessing a map element
value := m["apple"]
fmt.Println("Apple value:", value)
// Modifying an existing element
m["banana"] = 8
fmt.Println("Modified Banana value:", m["banana"])
// Adding a new element
m["cherry"] = 7
fmt.Println("New Cherry value:", m["cherry"])
// Checking if a key exists
value, exists := m["grape"]
if exists {
fmt.Println("Grape value:", value)
} else {
fmt.Println("Grape does not exist")
}
}
In this code, we declare a map, access elements, modify an existing element, and add a new element. We also check if a key exists in the map.
6. Conclusion
Accessing and modifying map elements in Go is straightforward. You can access values by providing the key, modify existing elements by reassigning the key, and add new key-value pairs by simply assigning a value to a new key. Additionally, you can check if a key exists in the map using the second return value to avoid errors when accessing non-existent keys.
Iterating Over Maps in Go
1. Introduction to Iterating Over Maps
In Go, you can iterate over a map using the for
loop with the range
keyword. This allows you to access both the keys and values of the map.
2. Basic Iteration Using range
To iterate over a map, you can use the range
keyword in a for
loop. The loop returns two values: the key and the value. Here's an example:
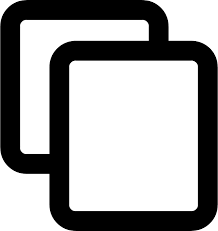
m := map[string]int{"apple": 5, "banana": 3, "cherry": 7}
for key, value := range m {
fmt.Println(key, value)
}
In this example, the loop will iterate through all the key-value pairs in the map m
and print each key and its corresponding value.
3. Iterating Over Keys Only
If you are only interested in the keys of the map, you can omit the value variable in the range
clause:
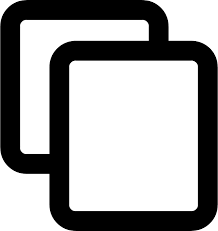
for key := range m {
fmt.Println(key)
}
In this case, the loop will only print the keys from the map, ignoring the values.
4. Iterating Over Values Only
Similarly, if you only want to access the values and not the keys, you can omit the key variable in the range
clause:
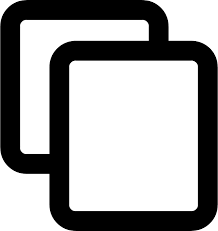
for _, value := range m {
fmt.Println(value)
}
The underscore _
is used to ignore the key, allowing you to access only the values.
5. Example of Iterating Over a Map
Here is a complete example that iterates over a map and prints both the keys and values:
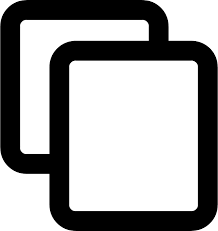
package main
import "fmt"
func main() {
// Declaring and initializing a map
m := map[string]int{"apple": 5, "banana": 3, "cherry": 7}
// Iterating over the map and printing key-value pairs
for key, value := range m {
fmt.Println(key, value)
}
// Iterating over the map and printing only keys
fmt.Println("Keys:")
for key := range m {
fmt.Println(key)
}
// Iterating over the map and printing only values
fmt.Println("Values:")
for _, value := range m {
fmt.Println(value)
}
}
In this code, we first iterate over the map to print both keys and values. Then, we iterate to print only the keys and later only the values.
6. Conclusion
Iterating over maps in Go is a simple and efficient process using the range
keyword. You can access both keys and values, or just the keys or values, depending on your needs. This makes working with maps in Go very flexible and easy to handle.
Deleting Elements from a Map in Go
1. Introduction to Deleting Elements
In Go, you can delete elements from a map using the delete
function. This function removes a key-value pair from the map.
2. Syntax of the delete
Function
The delete
function takes two arguments: the map and the key of the element you want to remove. Here's the syntax:
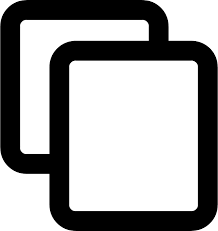
delete(map, key)
Where map
is the map from which you want to remove an element, and key
is the key of the element to be deleted.
3. Example of Deleting an Element
Here is an example that demonstrates how to delete an element from a map:
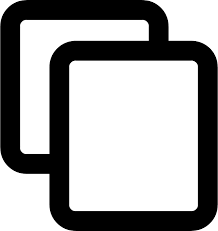
m := map[string]int{"apple": 5, "banana": 3, "cherry": 7}
delete(m, "banana")
fmt.Println(m)
In this example, the key-value pair with the key "banana"
is deleted from the map m
, and the map is printed without the "banana" entry.
4. Deleting an Element from an Empty Map
If you try to delete a key from a map that doesn't exist, Go will silently do nothing. There will be no error or panic:
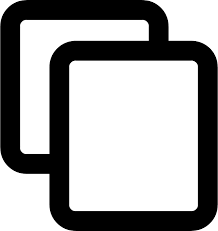
delete(m, "orange") // No error, does nothing if the key is not present
5. Example of Deleting an Element and Checking the Map
In this example, we delete a key from a map and then check if the key still exists in the map:
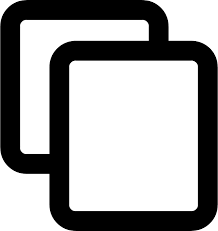
package main
import "fmt"
func main() {
// Declaring and initializing a map
m := map[string]int{"apple": 5, "banana": 3, "cherry": 7}
// Deleting an element
delete(m, "banana")
// Checking if the key exists after deletion
value, exists := m["banana"]
fmt.Println("Value:", value) // Should print 0 (default value for int)
fmt.Println("Exists:", exists) // Should print false
// Printing the map after deletion
fmt.Println(m)
}
In the code, we delete the key "banana"
and then check if it still exists in the map using the second return value of the map lookup, which indicates whether the key is present.
6. Conclusion
Deleting elements from a map in Go is a straightforward process using the delete
function. It does not raise an error if the key is not found, allowing you to safely remove elements without worrying about the existence of the key. After deletion, the key will no longer exist in the map.
Maps with Structs in Go
1. Introduction to Maps with Structs
In Go, you can use maps to store struct values as the elements. A map allows you to associate a key with a struct, making it easy to access and modify complex data structures.
2. Declaring a Map with Structs
To declare a map with structs, first define the struct type, then declare the map with the struct type as the value. Here’s how you can declare a map where the key is a string, and the value is a struct:
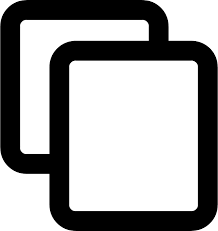
type Person struct {
Name string
Age int
}
m := map[string]Person{
"john": Person{"John", 30},
"mary": Person{"Mary", 25},
}
3. Accessing and Modifying Map Elements with Structs
You can access and modify elements in the map just like you would with primitive types. Here is an example of how to access and modify a struct in the map:
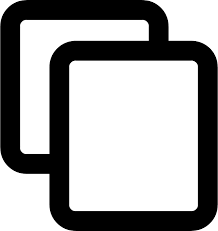
m := map[string]Person{
"john": Person{"John", 30},
"mary": Person{"Mary", 25},
}
// Accessing a struct value
fmt.Println(m["john"].Name) // Output: John
// Modifying a struct value
m["john"] = Person{"John", 31}
fmt.Println(m["john"].Age) // Output: 31
4. Example: Using Structs in Maps
Here’s a complete example where we use a map to store information about people and their ages:
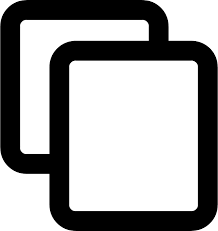
package main
import "fmt"
// Defining a struct
type Person struct {
Name string
Age int
}
func main() {
// Declaring a map with structs as values
people := map[string]Person{
"alice": Person{"Alice", 28},
"bob": Person{"Bob", 35},
}
// Accessing a value from the map
fmt.Println(people["alice"].Name) // Output: Alice
fmt.Println(people["alice"].Age) // Output: 28
// Modifying a struct value in the map
people["bob"] = Person{"Bob", 36}
fmt.Println(people["bob"].Age) // Output: 36
}
5. Iterating Over Maps with Structs
You can also iterate over a map with struct values just like with any other map. Here's an example:
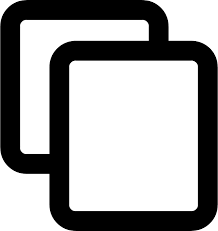
package main
import "fmt"
// Defining a struct
type Person struct {
Name string
Age int
}
func main() {
// Declaring a map with structs as values
people := map[string]Person{
"alice": Person{"Alice", 28},
"bob": Person{"Bob", 35},
}
// Iterating over the map
for key, person := range people {
fmt.Println(key, ":", person.Name, "-", person.Age)
}
}
This will print:
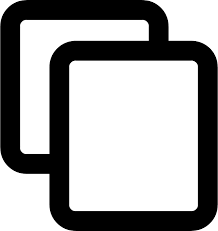
alice : Alice - 28
bob : Bob - 35
6. Conclusion
Maps with structs in Go are powerful data structures that allow you to associate complex data types with keys. This enables you to model real-world entities and their relationships efficiently. Accessing, modifying, and iterating over map elements with struct values is simple and intuitive in Go.
Understanding Interfaces in Go
1. Introduction to Interfaces
In Go, an interface is a type that specifies a set of method signatures (behavior) but does not provide the implementation of those methods. A type is said to implement an interface if it provides concrete implementations of the methods declared by the interface. This allows Go to achieve polymorphism and enables decoupling in Go programs.
2. Declaring an Interface
In Go, interfaces are declared using the interface
keyword, followed by the method signatures that types must implement. Here’s a simple example of an interface:
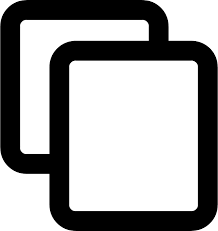
type Speaker interface {
Speak() string
}
This Speaker
interface declares a method called Speak()
, which returns a string. Any type that implements this method is considered to implement the Speaker
interface.
3. Implementing an Interface
In Go, you don’t explicitly declare that a type implements an interface. If a type provides the methods declared by the interface, it automatically satisfies that interface. Here’s how you can implement the Speaker
interface:
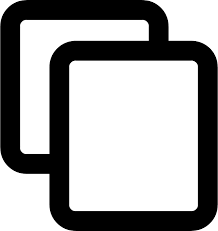
type Person struct {
Name string
}
func (p Person) Speak() string {
return "Hello, my name is " + p.Name
}
func main() {
var s Speaker = Person{Name: "Alice"}
fmt.Println(s.Speak()) // Output: Hello, my name is Alice
}
4. Empty Interface
The empty interface interface{}
is a special case in Go. It represents an interface with zero methods, meaning that any type satisfies the empty interface. This is commonly used when you don’t know the type of value you’re dealing with, such as in a function that accepts any type:
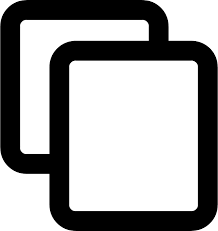
func PrintAnything(i interface{}) {
fmt.Println(i)
}
func main() {
PrintAnything(42) // Output: 42
PrintAnything("Hello, Go!") // Output: Hello, Go!
}
5. Type Assertion
Type assertion is used to extract the underlying value of an interface. You can assert the concrete type of an interface using the syntax i.(T)
, where i
is the interface and T
is the expected concrete type. If the assertion is successful, it returns the value; otherwise, it panics. You can also use the second return value to safely check if the assertion is successful:
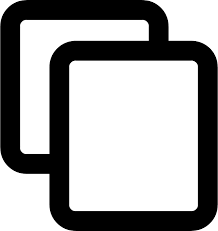
var i interface{} = 42
// Type assertion
v := i.(int)
fmt.Println(v) // Output: 42
// Safe type assertion
v, ok := i.(string)
if ok {
fmt.Println(v)
} else {
fmt.Println("Not a string") // Output: Not a string
}
6. Polymorphism with Interfaces
Interfaces in Go allow polymorphism, meaning that you can treat different types uniformly if they implement the same interface. Here’s an example:
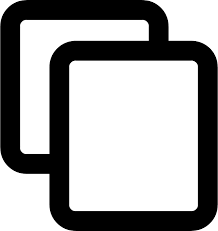
type Speaker interface {
Speak() string
}
type Dog struct {
Name string
}
func (d Dog) Speak() string {
return d.Name + " says Woof!"
}
type Cat struct {
Name string
}
func (c Cat) Speak() string {
return c.Name + " says Meow!"
}
func Introduce(s Speaker) {
fmt.Println(s.Speak())
}
func main() {
d := Dog{Name: "Rex"}
c := Cat{Name: "Whiskers"}
Introduce(d) // Output: Rex says Woof!
Introduce(c) // Output: Whiskers says Meow!
}
7. Conclusion
Interfaces in Go are a powerful feature that enables polymorphism and decoupling in your programs. By specifying method sets without defining how the methods are implemented, interfaces allow Go to work with different types in a uniform manner. Understanding how interfaces work helps you design flexible and maintainable Go programs.
Defining Interfaces in Go
1. Introduction to Interfaces
In Go, an interface is a type that defines a set of method signatures but does not implement them. Types that implement all the methods declared by an interface are said to implement that interface. Go’s interfaces provide a way to achieve polymorphism, allowing you to write functions and methods that can operate on different types that share common behavior.
2. Declaring an Interface
To define an interface in Go, you use the interface
keyword followed by a set of methods that the types must implement. The methods in an interface are only declared, not defined. A type is said to implement an interface if it provides concrete implementations for all the methods declared by that interface.
Here’s an example of defining an interface:
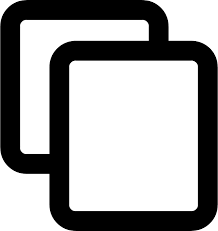
type Speaker interface {
Speak() string
}
In this example, the Speaker
interface declares a method Speak()
that returns a string. Any type that implements this method will be considered to implement the Speaker
interface.
3. Implementing an Interface
In Go, you don't explicitly declare that a type implements an interface. If a type provides the methods declared in an interface, it automatically satisfies that interface. Here's an example:
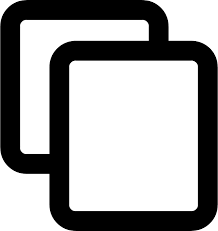
type Person struct {
Name string
}
func (p Person) Speak() string {
return "Hello, my name is " + p.Name
}
func main() {
var s Speaker = Person{Name: "Alice"}
fmt.Println(s.Speak()) // Output: Hello, my name is Alice
}
In this example, the Person
type has a Speak()
method, so it automatically satisfies the Speaker
interface. We don’t need to explicitly declare that Person
implements the Speaker
interface.
4. Empty Interface
An empty interface, represented as interface{}
, is a special kind of interface that doesn’t define any methods. In Go, any type implements the empty interface because all types implement zero methods. The empty interface is often used when you don’t know the type of the value you are working with, allowing you to accept any type.
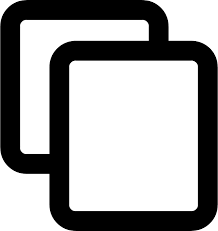
func PrintAnything(i interface{}) {
fmt.Println(i)
}
func main() {
PrintAnything(42) // Output: 42
PrintAnything("Hello, Go!") // Output: Hello, Go!
}
5. Conclusion
Defining interfaces in Go is straightforward and allows you to design flexible and reusable code. By declaring method sets in interfaces, you can create functions and methods that operate on different types, enabling polymorphism. Understanding interfaces is key to writing clean, modular, and extensible Go programs.
Implementing Interfaces in Go
1. What Does It Mean to Implement an Interface?
In Go, a type implements an interface by providing concrete methods that match the method signatures declared in the interface. Unlike many other languages, Go does not require you to explicitly declare that a type implements an interface. If the methods match, the type automatically implements the interface.
2. Example of Implementing an Interface
Here’s an example where we define an interface Speaker
and implement it using a Person
type:
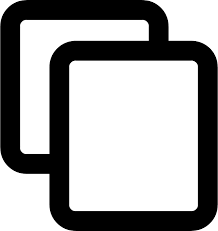
type Speaker interface {
Speak() string
}
type Person struct {
Name string
}
func (p Person) Speak() string {
return "Hello, my name is " + p.Name
}
func main() {
var s Speaker = Person{Name: "Alice"}
fmt.Println(s.Speak()) // Output: Hello, my name is Alice
}
In this example, the Person
type automatically implements the Speaker
interface because it provides a Speak()
method that matches the method signature in the interface.
3. Implementing Multiple Interfaces
A type can implement multiple interfaces by providing methods that match multiple method sets. Here's an example where a type implements both the Speaker
and another interface Greeter
:
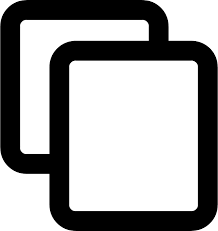
type Greeter interface {
Greet() string
}
type Person struct {
Name string
}
func (p Person) Speak() string {
return "Hello, my name is " + p.Name
}
func (p Person) Greet() string {
return "Good morning, " + p.Name
}
func main() {
var s Speaker = Person{Name: "Alice"}
var g Greeter = Person{Name: "Alice"}
fmt.Println(s.Speak()) // Output: Hello, my name is Alice
fmt.Println(g.Greet()) // Output: Good morning, Alice
}
In this case, Person
implements both Speaker
and Greeter
interfaces by providing the required methods.
4. Using Pointer Receivers to Implement Interfaces
When you define methods using pointer receivers, only pointer types can implement the interface. This is useful if you want to modify the state of the receiver object. Here’s an example:
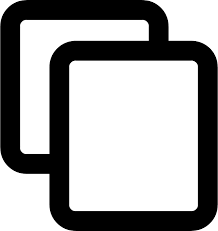
type Speaker interface {
Speak() string
}
type Person struct {
Name string
}
func (p *Person) Speak() string {
return "Hello, my name is " + p.Name
}
func main() {
p := &Person{Name: "Alice"}
var s Speaker = p
fmt.Println(s.Speak()) // Output: Hello, my name is Alice
}
In this case, the Speak
method is defined with a pointer receiver *Person
, so only a pointer to the Person
type can implement the Speaker
interface.
5. Interface Satisfaction at Compile Time
In Go, interface satisfaction is determined at compile time, not runtime. The compiler ensures that a type implements an interface by checking whether the type provides all the methods declared in the interface. If a type does not implement the interface, the code will fail to compile.
6. Conclusion
Implementing interfaces in Go is simple and powerful. A type automatically implements an interface if it provides the required methods, making Go’s interface system flexible and intuitive. By implementing multiple interfaces, using pointer receivers, and leveraging Go's compile-time checks, you can create clean, modular, and reusable code.
Empty Interfaces and Type Assertions in Go
1. What is an Empty Interface?
In Go, an empty interface is an interface that has no methods. It is often used when you want to accept any type. Every type in Go automatically satisfies the empty interface because all types have zero or more methods, so they implicitly implement the empty interface. The empty interface is denoted as interface{}
.
2. When to Use Empty Interfaces?
Empty interfaces are useful when you want to store values of different types in a collection or when you need to create a function that works with any type. For example, a function that takes an empty interface as a parameter can handle any data type.
3. Example of Using an Empty Interface
Here's an example where we use an empty interface to store different data types in a slice:
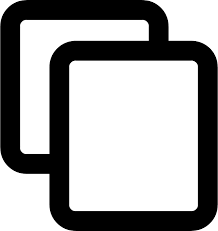
func printAny(value interface{}) {
fmt.Println(value)
}
func main() {
printAny(42) // Output: 42
printAny("Hello, Go!") // Output: Hello, Go!
printAny(3.14) // Output: 3.14
}
In this example, the printAny
function accepts a value of any type because it takes an empty interface as its parameter. It can print integers, strings, and floats.
4. Type Assertions
Type assertion is a way to retrieve the concrete value from an interface. It allows you to check if the interface contains a specific type and access that value. The syntax for type assertion is value := x.(T)
, where x
is the interface, and T
is the type you're asserting.
5. Example of Type Assertions
Here’s an example of how you can use type assertions with an empty interface to convert an interface value back to its original type:
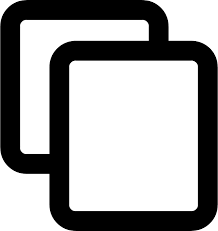
func main() {
var x interface{} = "Hello, Go!"
// Type assertion to convert the interface to a string
str, ok := x.(string)
if ok {
fmt.Println("String value:", str) // Output: String value: Hello, Go!
} else {
fmt.Println("Not a string")
}
}
In this example, we use type assertion to check if the value stored in the interface x
is of type string
. If the assertion is successful, the value is stored in str
, and we print it. The variable ok
indicates whether the type assertion was successful.
6. Using Type Assertions with Different Types
You can also perform type assertions with other types, such as structs, integers, or custom types. Here's an example:
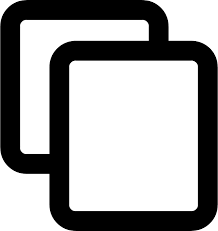
type Person struct {
Name string
}
func main() {
var x interface{} = Person{Name: "Alice"}
// Type assertion to convert the interface to a Person type
p, ok := x.(Person)
if ok {
fmt.Println("Person name:", p.Name) // Output: Person name: Alice
} else {
fmt.Println("Not a Person")
}
}
In this case, the type assertion checks if the interface x
contains a Person
type. If successful, it extracts the value and prints the Name
field.
7. Handling Failed Type Assertions
If the type assertion fails, Go will panic if you're not using the second return value that indicates success. To handle a failed assertion safely, always use the , ok
idiom to check if the assertion was successful:
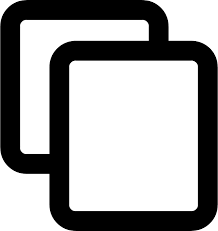
func main() {
var x interface{} = 42
// Unsafe type assertion
// y := x.(string) // This would cause a panic
// Safe type assertion with ok
y, ok := x.(string)
if !ok {
fmt.Println("Type assertion failed!")
} else {
fmt.Println("String value:", y)
}
}
In this example, the type assertion would fail because x
holds an int
, not a string
. By using , ok
, we can safely handle the failure and avoid a runtime panic.
8. Conclusion
Empty interfaces and type assertions provide powerful tools for handling dynamic types and interacting with unknown data in Go. By using empty interfaces, you can write functions and structures that work with any type, while type assertions allow you to retrieve and convert values from interfaces to their concrete types when needed.
Type Switches in Go
1. What is a Type Switch?
A type switch in Go is a special kind of switch statement that operates on types instead of values. It allows you to compare the type of an interface variable against different types, and execute different code based on the type of the value it holds. Type switches are useful when you want to handle multiple types stored in an empty interface (interface{}
) in a more structured way.
2. Syntax of Type Switch
The syntax of a type switch is similar to a regular switch statement, but instead of matching values, it matches types. Here’s the basic structure of a type switch:
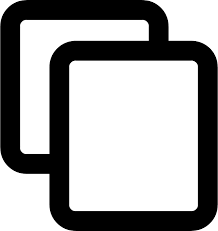
switch v := x.(type) {
case T1:
// Handle type T1
case T2:
// Handle type T2
default:
// Handle the default case if no types match
}
In this syntax, x
is the interface variable, and T1
, T2
, etc., are the types you're comparing x
against. The variable v
will hold the value of x
if the case matches.
3. Example of a Type Switch
Let's look at an example where we use a type switch to handle different types stored in an interface:
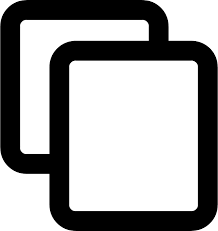
func printType(v interface{}) {
switch v := v.(type) {
case int:
fmt.Println("Integer:", v)
case string:
fmt.Println("String:", v)
case bool:
fmt.Println("Boolean:", v)
default:
fmt.Println("Unknown type")
}
}
func main() {
printType(42) // Output: Integer: 42
printType("Hello") // Output: String: Hello
printType(true) // Output: Boolean: true
printType(3.14) // Output: Unknown type
}
In this example, the printType
function accepts an empty interface, and based on the type of the value passed, it prints a message. If the type is not one of the cases (int, string, bool), the default
case is executed.
4. Type Switch with Multiple Cases
You can also check for multiple types in a type switch by handling more than one case in a single line. Here’s an example:
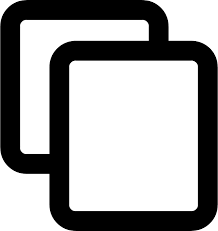
func printType(v interface{}) {
switch v := v.(type) {
case int, float64:
fmt.Println("Number:", v)
case string:
fmt.Println("String:", v)
default:
fmt.Println("Unknown type")
}
}
func main() {
printType(42) // Output: Number: 42
printType(3.14) // Output: Number: 3.14
printType("Hello") // Output: String: Hello
printType(true) // Output: Unknown type
}
In this example, both int
and float64
types are handled in the same case, allowing you to group similar types together.
5. Storing the Value of a Matched Type
When a type switch matches a case, you can store the value of the matching type in a new variable. This variable will have the type of the matched case. Here’s an example:
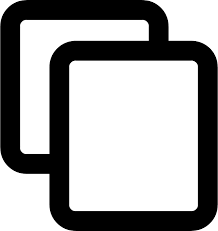
func describe(v interface{}) {
switch v := v.(type) {
case int:
fmt.Printf("Integer: %d\n", v)
case string:
fmt.Printf("String: %s\n", v)
case bool:
fmt.Printf("Boolean: %t\n", v)
default:
fmt.Printf("Unknown type\n")
}
}
func main() {
describe(42) // Output: Integer: 42
describe("Go") // Output: String: Go
describe(true) // Output: Boolean: true
describe(3.14) // Output: Unknown type
}
In this example, the type switch matches the type of v
and then prints the corresponding value. The value of v
is automatically assigned to the new variable in the matched case.
6. Conclusion
Type switches are a powerful feature in Go that allow you to handle different types stored in an interface. They provide a cleaner and more structured way to work with unknown types compared to using multiple type assertions. By leveraging type switches, you can write flexible and reusable code that handles a variety of types efficiently.
Introduction to Goroutines
1. What are Goroutines?
Goroutines are lightweight threads of execution in the Go programming language. They are managed by the Go runtime and allow concurrent execution of functions or methods. Goroutines are a fundamental part of Go's concurrency model, enabling developers to write efficient and concurrent programs that can handle multiple tasks simultaneously.
2. Creating Goroutines
Creating a goroutine is straightforward. You can launch a goroutine by prefixing a function call with the go
keyword. The function will then execute concurrently in a separate goroutine, allowing other parts of your program to continue executing without waiting for it to complete.
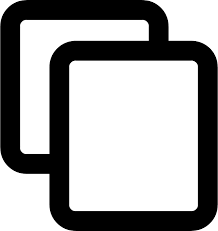
go functionName()
For example, to run a function myFunction()
concurrently, you would do:
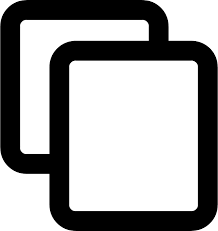
go myFunction()
3. Example of Goroutines
Here is a simple example demonstrating the usage of goroutines:
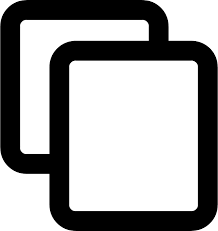
package main
import (
"fmt"
"time"
)
func sayHello() {
fmt.Println("Hello, World!")
}
func main() {
go sayHello() // Launching the goroutine
time.Sleep(time.Second) // Wait for the goroutine to finish
fmt.Println("Main function completed.")
}
In this example, the function sayHello
is launched as a goroutine in the main
function. To ensure that the main function waits for the goroutine to finish before exiting, we use time.Sleep
to pause for a second.
4. Goroutines and Concurrency
Goroutines allow for concurrent execution of tasks. Unlike traditional threads, goroutines are lightweight and share the same address space, making them less expensive in terms of memory and system resources. The Go scheduler manages the execution of goroutines, ensuring that they are run concurrently, often on multiple CPU cores.
5. Goroutines in Action: Concurrent Execution
Below is an example where multiple goroutines run concurrently:
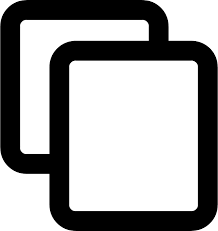
package main
import (
"fmt"
"time"
)
func task(name string) {
fmt.Println(name, "is starting")
time.Sleep(time.Second)
fmt.Println(name, "is completed")
}
func main() {
go task("Task 1")
go task("Task 2")
go task("Task 3")
time.Sleep(3 * time.Second) // Wait for all goroutines to complete
fmt.Println("All tasks completed.")
}
In this example, three goroutines are launched to run the task
function concurrently. The time.Sleep
in the main
function ensures that the program doesn't exit before all the goroutines have finished executing.
6. Goroutines and the Go Scheduler
The Go runtime has a scheduler that decides when and how goroutines should run. Goroutines are multiplexed onto a smaller number of operating system threads. The Go scheduler ensures that goroutines are executed efficiently, and it can switch between them at runtime, allowing multiple goroutines to make progress even on a limited number of processor cores.
7. Goroutines vs Traditional Threads
Goroutines are more lightweight and efficient than traditional threads. Some key differences are:
- Memory Efficiency: Goroutines use far less memory than traditional threads, making them more suitable for programs that need to handle thousands or even millions of concurrent tasks.
- Management: The Go runtime automatically schedules and manages goroutines, unlike traditional threads that are managed by the operating system.
- Scalability: Goroutines scale better with the number of cores compared to threads, thanks to the Go scheduler.
8. Conclusion
Goroutines are a powerful feature of the Go language, enabling developers to write concurrent programs easily and efficiently. With their lightweight nature, goroutines make it possible to execute tasks concurrently without the overhead of traditional threads. Whether building high-performance web servers, processing data in parallel, or handling multiple network connections, goroutines offer a simple and effective way to manage concurrency in Go.
Channels in Go
1. What are Channels?
In Go, channels are used to communicate between goroutines, allowing them to synchronize and exchange data. Channels provide a way for goroutines to send and receive values, facilitating concurrent programming. Channels are a powerful feature in Go that helps achieve synchronization and communication without the need for explicit locks or condition variables.
2. Declaring Channels
Channels are declared using the chan
keyword followed by the data type that the channel will carry. A channel can be created using the make
function.
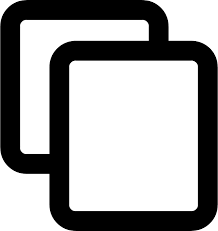
ch := make(chan int)
This creates a channel ch
that will carry int
values. You can also create buffered channels that can hold a specified number of values before blocking the sending operation.
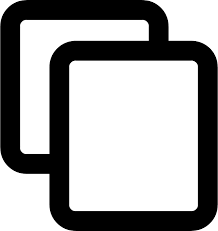
ch := make(chan int, 2) // Buffered channel with capacity of 2
3. Sending and Receiving Values Through Channels
To send a value into a channel, use the <-
operator:
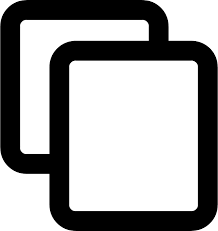
ch <- 42 // Send the value 42 to the channel
To receive a value from a channel, also use the <-
operator:
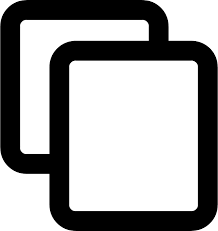
value := <-ch // Receive a value from the channel
4. Example: Sending and Receiving Values
Here is a simple example of sending and receiving values using channels:
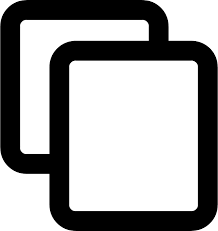
package main
import "fmt"
func main() {
ch := make(chan int)
// Sending a value to the channel
go func() {
ch <- 42
}()
// Receiving the value from the channel
value := <-ch
fmt.Println("Received:", value)
}
In this example, the value 42
is sent to the channel ch
inside a goroutine, and the main function receives it and prints it.
5. Buffered Channels
A buffered channel allows sending values into the channel without blocking until the buffer is full. The capacity of the buffered channel is defined when the channel is created.
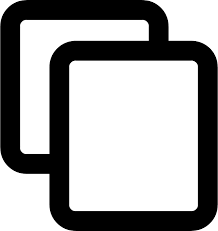
ch := make(chan int, 2)
In this case, the channel ch
can hold up to 2 values before the sending operation blocks. The receiving goroutine will unblock the sending goroutine as it consumes values from the channel.
6. Example: Buffered Channels
Here is an example demonstrating buffered channels:
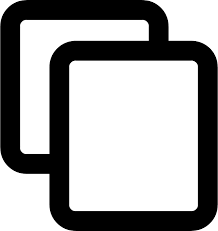
package main
import "fmt"
func main() {
ch := make(chan int, 2)
// Sending two values to the buffered channel
ch <- 1
ch <- 2
// Receiving values from the buffered channel
fmt.Println(<-ch) // Prints: 1
fmt.Println(<-ch) // Prints: 2
}
In this example, two values are sent to the buffered channel ch
. The channel can hold both values without blocking. The values are then received and printed in the main function.
7. Closing Channels
Once you no longer need a channel, you can close it using the close
function. Closing a channel indicates that no more values will be sent on it. A closed channel can still be read from, but attempting to send a value to a closed channel will cause a runtime panic.
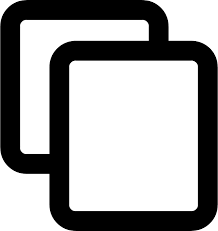
close(ch)
8. Example: Closing a Channel
Here is an example that demonstrates how to close a channel:
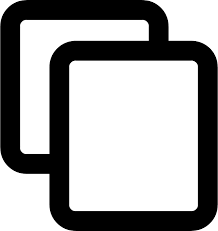
package main
import "fmt"
func main() {
ch := make(chan int)
// Sending values to the channel
go func() {
ch <- 1
ch <- 2
close(ch) // Closing the channel
}()
// Receiving values from the channel
for value := range ch {
fmt.Println(value)
}
}
In this example, the values 1
and 2
are sent to the channel ch
, and the channel is closed after that. The range
keyword is used to iterate over the values received from the channel until it is closed.
9. Select Statement with Channels
The select
statement allows you to wait on multiple channel operations. It is similar to a switch
statement but for channels. The select
statement blocks until one of its cases can execute, which is useful for handling multiple channel operations concurrently.
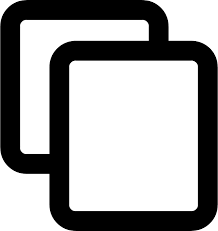
select {
case msg := <-ch1:
fmt.Println("Received from ch1:", msg)
case msg := <-ch2:
fmt.Println("Received from ch2:", msg)
}
10. Example: Using Select with Channels
Here is an example that demonstrates how to use select
with multiple channels:
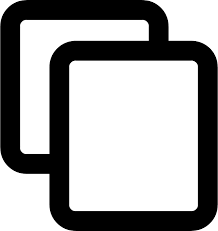
package main
import "fmt"
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
ch1 <- "Hello from ch1"
}()
go func() {
ch2 <- "Hello from ch2"
}()
select {
case msg1 := <-ch1:
fmt.Println(msg1)
case msg2 := <-ch2:
fmt.Println(msg2)
}
}
In this example, the select
statement waits for either ch1
or ch2
to receive a message. When a message is received, it prints the message and exits the select
block.
11. Conclusion
Channels in Go are a powerful tool for synchronizing and communicating between goroutines. They allow goroutines to send and receive values, enabling concurrent programming in a safe and efficient manner. With buffered channels, closing channels, and using the select
statement, Go offers a comprehensive concurrency model that makes it easy to write concurrent programs.
Select Statement for Channel Communication
1. What is the Select Statement?
The select
statement in Go is a powerful concurrency control feature that allows you to wait on multiple channel operations. It enables you to choose which channel to receive from or send to based on which one is ready, making it an essential tool for handling multiple channel operations concurrently.
2. How Does the Select Statement Work?
The select
statement is similar to a switch
statement, but it operates on channels. It blocks until one of its cases can execute. If multiple channels are ready, one case is selected at random. If no channels are ready, the select
statement will block until one becomes available.
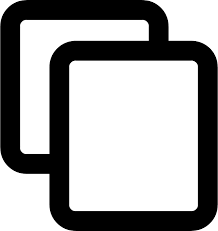
select {
case msg1 := <-ch1:
fmt.Println("Received from ch1:", msg1)
case msg2 := <-ch2:
fmt.Println("Received from ch2:", msg2)
default:
fmt.Println("No channels are ready")
}
3. Components of a Select Statement
- Channel operations: You can wait for a channel to send or receive data by using the
<-
operator inside the case statement. - Default case: If none of the channels are ready, the
default
case will execute. This is optional, but it allows you to avoid blocking indefinitely.
4. Example: Using Select for Channel Communication
Here’s an example that demonstrates how to use the select
statement to receive messages from multiple channels:
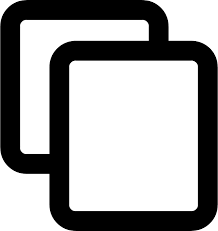
package main
import "fmt"
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
ch1 <- "Message from ch1"
}()
go func() {
ch2 <- "Message from ch2"
}()
select {
case msg1 := <-ch1:
fmt.Println(msg1)
case msg2 := <-ch2:
fmt.Println(msg2)
}
}
In this example, the select
statement waits for either ch1
or ch2
to receive a message. When a message is received, it prints the message and exits the select
block.
5. Select with Multiple Channels
The select
statement can handle multiple channels at the same time. This is useful in programs where you need to wait for multiple events to happen concurrently, such as when waiting for messages from multiple goroutines.
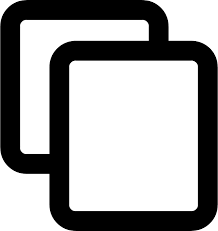
package main
import "fmt"
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
ch1 <- "First message from ch1"
}()
go func() {
ch2 <- "First message from ch2"
}()
select {
case msg1 := <-ch1:
fmt.Println("Received:", msg1)
case msg2 := <-ch2:
fmt.Println("Received:", msg2)
}
go func() {
ch1 <- "Second message from ch1"
}()
go func() {
ch2 <- "Second message from ch2"
}()
select {
case msg1 := <-ch1:
fmt.Println("Received:", msg1)
case msg2 := <-ch2:
fmt.Println("Received:", msg2)
}
}
This program demonstrates using the select
statement with multiple channels. The first select
block waits for messages from ch1
or ch2
, and the second block waits again for messages from the same channels.
6. Handling Timeouts with Select
You can use the select
statement to implement timeouts by creating a time.After
channel, which sends a value after a specified duration. If the timeout occurs before any channel operation completes, the select
statement will execute the default
case or handle the timeout case accordingly.
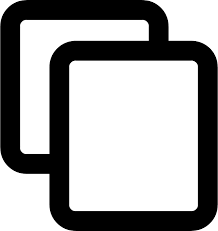
package main
import (
"fmt"
"time"
)
func main() {
ch1 := make(chan string)
go func() {
time.Sleep(2 * time.Second)
ch1 <- "Message from ch1"
}()
select {
case msg := <-ch1:
fmt.Println("Received:", msg)
case <-time.After(1 * time.Second):
fmt.Println("Timeout occurred")
}
}
In this example, the select
statement waits for a message from ch1
, but if the message does not arrive within 1 second, the timeout case is triggered, printing "Timeout occurred".
7. Select with Default Case
The default
case is executed if no channel is ready for communication. This can be useful if you want to perform a non-blocking operation or handle situations where no channels are ready.
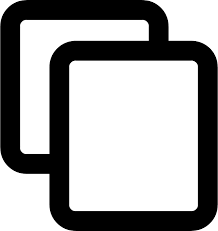
select {
case msg := <-ch1:
fmt.Println("Received:", msg)
case msg := <-ch2:
fmt.Println("Received:", msg)
default:
fmt.Println("No channels ready")
}
In this example, if neither ch1
nor ch2
is ready, the default
case will execute, and the message "No channels ready" will be printed.
8. Conclusion
The select
statement is an essential feature in Go for handling multiple channel operations concurrently. It allows you to wait on several channels, handle timeouts, and manage non-blocking operations efficiently. By using select
with multiple channels, you can write concurrent programs that are both readable and effective in terms of resource management.
Buffered and Unbuffered Channels
1. What are Channels in Go?
Channels are the conduits through which goroutines communicate with one another in Go. They allow the safe exchange of data between goroutines, ensuring that the data being passed remains synchronized and thread-safe. Channels can be either unbuffered or buffered, each with distinct behaviors and use cases.
2. Unbuffered Channels
An unbuffered channel is a channel with no capacity to hold any data. When you send data to an unbuffered channel, the sending goroutine is blocked until another goroutine is ready to receive that data. Similarly, a receiving goroutine is blocked until there is data to receive from the channel.
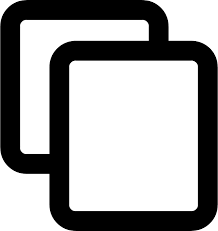
package main
import "fmt"
func main() {
ch := make(chan string)
// Sending data to an unbuffered channel
go func() {
ch <- "Hello, Go!"
}()
// Receiving data from an unbuffered channel
msg := <-ch
fmt.Println(msg)
}
In this example, the sending goroutine will block until the receiving goroutine is ready to receive the data from the unbuffered channel. Unbuffered channels are often used when synchronization between goroutines is required.
3. Buffered Channels
A buffered channel, on the other hand, has a capacity to hold a specified number of values. When you send data to a buffered channel, the sending goroutine does not block until the channel is full. Similarly, a receiving goroutine does not block until the channel is empty. This allows for more flexibility in communication between goroutines, as they can continue executing until the channel reaches its buffer limit.
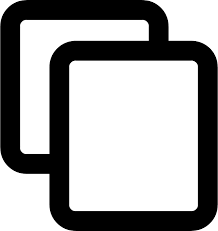
package main
import "fmt"
func main() {
ch := make(chan string, 2) // Buffered channel with a capacity of 2
// Sending data to a buffered channel
ch <- "Hello"
ch <- "Go"
// Receiving data from a buffered channel
fmt.Println(<-ch)
fmt.Println(<-ch)
}
In this example, the buffered channel can hold two values before the sending goroutine is blocked. The receiving goroutine can retrieve data from the channel without blocking until it is empty.
4. Key Differences Between Buffered and Unbuffered Channels
- Blocking Behavior: An unbuffered channel blocks the sender and receiver until both are ready. A buffered channel allows the sender to send data without blocking until the channel is full, and the receiver can receive data without blocking until the channel is empty.
- Use Cases: Unbuffered channels are useful when you need to synchronize the execution of goroutines. Buffered channels are helpful when you want to allow some degree of flexibility in the timing of goroutine execution and avoid blocking unnecessarily.
- Capacity: Unbuffered channels have a capacity of 0, while buffered channels have a user-defined capacity that allows them to hold multiple values.
5. Practical Example: Using Buffered and Unbuffered Channels
Let’s compare how unbuffered and buffered channels behave in the same scenario:
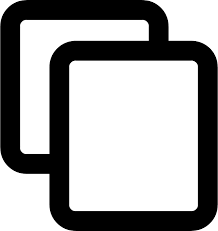
package main
import "fmt"
func main() {
// Unbuffered Channel Example
ch1 := make(chan string)
go func() {
fmt.Println("Sending data to unbuffered channel")
ch1 <- "Unbuffered channel data"
}()
fmt.Println("Receiving data from unbuffered channel")
fmt.Println(<-ch1)
// Buffered Channel Example
ch2 := make(chan string, 2) // Buffered channel with capacity 2
go func() {
fmt.Println("Sending data to buffered channel")
ch2 <- "Buffered channel data 1"
ch2 <- "Buffered channel data 2"
}()
fmt.Println("Receiving data from buffered channel")
fmt.Println(<-ch2)
fmt.Println(<-ch2)
}
In this example, the unbuffered channel causes the sending goroutine to block until the main goroutine is ready to receive the data. In contrast, the buffered channel allows the sending goroutine to send two pieces of data without blocking, since the channel can hold up to two values.
6. When to Use Buffered or Unbuffered Channels
- Use Unbuffered Channels: When you need strict synchronization between goroutines, such as when the sender and receiver must operate in a coordinated manner.
- Use Buffered Channels: When you want to allow some flexibility in goroutine communication, such as when the sender and receiver do not have to be synchronized exactly, or when you want to reduce blocking by allowing multiple values to be sent and received without waiting for the other side.
7. Conclusion
Both buffered and unbuffered channels are essential tools in Go for managing concurrency and communication between goroutines. The choice between using a buffered or unbuffered channel depends on the requirements of your specific use case. Unbuffered channels are ideal for strict synchronization, while buffered channels offer more flexibility and can reduce unnecessary blocking.
Synchronization with WaitGroups
1. What is a WaitGroup?
A WaitGroup in Go is used to wait for a collection of goroutines to finish executing. It is part of the sync
package and provides a mechanism for coordinating multiple goroutines by blocking until all goroutines have completed their tasks.
2. How Does a WaitGroup Work?
A WaitGroup has three key methods:
Add
: Increments the counter of the WaitGroup by the specified value. It is used to specify how many goroutines to wait for.Done
: Decrements the counter by 1. Each goroutine calls this method when it finishes.Wait
: Blocks until the counter becomes zero, indicating that all goroutines have finished executing.
3. Example: Using WaitGroup to Synchronize Goroutines
Here’s an example demonstrating the use of a WaitGroup to wait for multiple goroutines to complete:
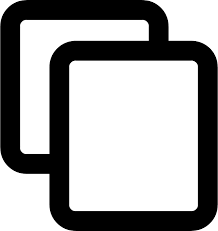
package main
import (
"fmt"
"sync"
"time"
)
func printMessage(msg string, wg *sync.WaitGroup) {
defer wg.Done() // Decrement the counter when the goroutine finishes
fmt.Println(msg)
time.Sleep(1 * time.Second) // Simulate work
}
func main() {
var wg sync.WaitGroup
// Add 3 to the WaitGroup counter as we have 3 goroutines
wg.Add(3)
go printMessage("Goroutine 1", &wg)
go printMessage("Goroutine 2", &wg)
go printMessage("Goroutine 3", &wg)
// Wait for all goroutines to finish
wg.Wait()
fmt.Println("All goroutines have completed.")
}
In this example, we create a WaitGroup
and add 3 to its counter to account for the 3 goroutines. Each goroutine calls Done
when it finishes, and the main function waits for all goroutines to complete by calling Wait
.
4. Important Points to Remember
- Calling
Add
before launching goroutines: TheAdd
method must be called before the goroutines are started so that the WaitGroup knows how many goroutines to wait for. - Defer
Done
: It’s common to usedefer
when calling theDone
method to ensure it gets called when the goroutine finishes, even if an error occurs. - Don’t call
Wait
in multiple goroutines: Only the main goroutine should callWait
to avoid deadlocks.
5. Example with Error Handling
Here’s an example that demonstrates error handling with goroutines and WaitGroups:
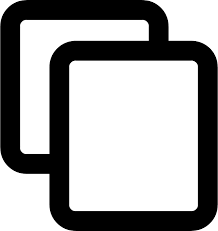
package main
import (
"fmt"
"sync"
"time"
"errors"
)
func processTask(id int, wg *sync.WaitGroup, ch chan error) {
defer wg.Done()
// Simulate work
time.Sleep(time.Second)
if id%2 == 0 {
ch <- nil // No error for even ID
} else {
ch <- errors.New("error occurred")
}
}
func main() {
var wg sync.WaitGroup
ch := make(chan error, 3) // Buffered channel to collect errors
wg.Add(3)
// Start goroutines
for i := 1; i <= 3; i++ {
go processTask(i, &wg, ch)
}
wg.Wait() // Wait for all goroutines to finish
// Check for errors
close(ch)
for err := range ch {
if err != nil {
fmt.Println("Task failed:", err)
} else {
fmt.Println("Task completed successfully")
}
}
fmt.Println("All tasks finished.")
}
This example demonstrates how to use a buffered channel to collect errors from multiple goroutines and handle them after all goroutines are done.
6. Summary
The WaitGroup is a useful tool for synchronizing multiple goroutines and ensuring that they all complete their work before the program proceeds. By using the Add
, Done
, and Wait
methods, you can easily coordinate the execution of concurrent tasks in Go.
Mutexes and Locks in Go
1. Introduction to Mutexes
A mutex (short for mutual exclusion) is a synchronization primitive that is used to ensure that only one goroutine can access a shared resource at a time. In Go, mutexes are provided by the sync
package. Mutexes are useful when you want to protect data that is accessed by multiple goroutines.
2. Declaring a Mutex
A mutex is declared using the sync.Mutex
type. It can be locked and unlocked using the Lock()
and Unlock()
methods respectively.
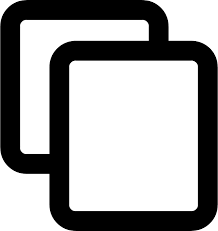
var mu sync.Mutex
3. Locking and Unlocking a Mutex
To ensure that only one goroutine can access a critical section, you need to lock the mutex before accessing the shared resource and unlock it afterward.
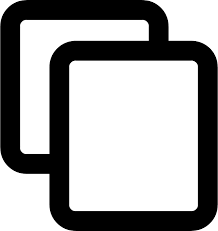
mu.Lock()
// critical section - access shared resource
mu.Unlock()
The Lock()
method blocks until the mutex is available, and the Unlock()
method releases the mutex, allowing other goroutines to lock it.
4. Example of Mutex Usage
Here’s an example demonstrating how mutexes work in Go:
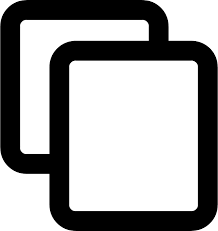
package main
import (
"fmt"
"sync"
)
var mu sync.Mutex
var counter int
func increment() {
mu.Lock()
counter++
mu.Unlock()
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
defer wg.Done()
increment()
}()
}
wg.Wait()
fmt.Println("Counter:", counter)
}
In this example, we use a mutex to protect access to the counter
variable so that no two goroutines can modify it at the same time.
5. Deadlocks and Avoiding Them
A deadlock occurs when two or more goroutines are waiting on each other to release locks, creating a situation where they cannot proceed. To avoid deadlocks:
- Always lock resources in the same order.
- Use the
defer
statement to ensure that mutexes are unlocked even in case of an error. - Use
sync.RWMutex
for read-heavy data access.
6. Read-Write Mutexes
Go also provides sync.RWMutex
, which allows multiple goroutines to read the data simultaneously, but only one goroutine can write to the data at a time. This can improve performance when reads are more frequent than writes.
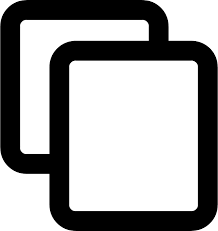
var rwmu sync.RWMutex
To lock for reading, use rwmu.RLock()
, and to lock for writing, use rwmu.Lock()
. Unlocking is done using rwmu.RUnlock()
or rwmu.Unlock()
.
7. Conclusion
Mutexes and locks are essential tools for managing concurrency and ensuring that shared resources are accessed safely in a multithreaded Go program. By using mutexes, you can prevent race conditions and ensure that your program behaves as expected in a concurrent environment.
Go’s Approach to Error Handling
1. Introduction to Error Handling in Go
In Go, error handling is done explicitly, and it is considered a core part of the language's design. Instead of using exceptions like other languages, Go uses error values that are returned from functions to indicate failure or success. This approach encourages writing code that handles errors at the point of occurrence, making it easy to manage and debug issues.
2. Returning Errors in Functions
Go functions commonly return an error value as the last return value. If the function encounters an error, it will return a non-nil error. If everything is successful, the error will be nil. Here's an example:
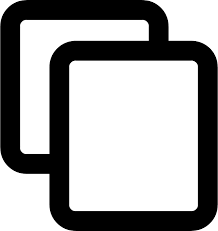
func divide(a, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
In this example, the function `divide` returns an error if `b` is zero.
3. Handling Errors in Go
When calling a function that returns an error, Go encourages handling the error immediately. It’s common to check if an error is returned and take appropriate action, such as logging it or terminating the function. Here's an example of error handling:
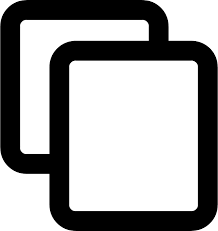
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Result:", result)
If an error occurs (like division by zero), the code prints the error message and terminates further execution. If the operation succeeds, it prints the result.
4. Custom Error Types
Go allows developers to create custom error types by implementing the `Error` method. This helps in adding more context to the error, such as additional details or error codes. A custom error type might look like this:
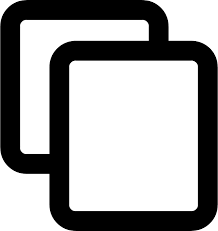
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Code %d: %s", e.Code, e.Message)
}
In this example, the custom error type `MyError` implements the `Error` method, which makes it compatible with Go's error handling system.
5. Using the errors Package
Go provides the `errors` package, which includes functions like `errors.New()` and `fmt.Errorf()`. These functions are used to create simple or formatted error messages. For example:
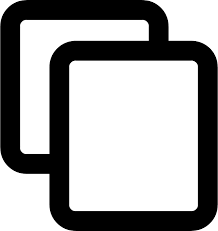
err := errors.New("something went wrong")
Or, you can use `fmt.Errorf` to create formatted error messages:
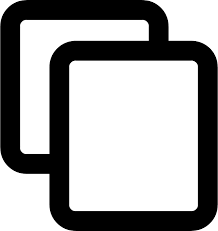
err := fmt.Errorf("failed to open file: %v", err)
6. Dealing with Panic and Recover
Go uses `panic` and `recover` for handling unexpected errors that are not meant to be caught during normal error handling. A `panic` stops the normal flow of the program and starts unwinding the stack. `recover` can be used to regain control of the program after a panic has occurred.
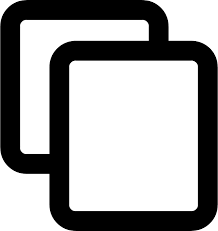
func safeDivide(a, b int) (int, error) {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}()
if b == 0 {
panic("division by zero")
}
return a / b, nil
}
In this example, `defer` is used to ensure that the function `recover` will run in case of a panic, allowing the program to handle unexpected errors gracefully instead of crashing.
7. Conclusion
Go’s error handling is simple yet powerful. By returning error values from functions and handling them explicitly, Go encourages developers to write code that deals with errors at the point of occurrence. Custom error types and the `panic` and `recover` mechanism provide additional flexibility for managing and responding to errors. This approach makes Go a robust language for building reliable and maintainable applications.
Returning Errors in Functions
1. Introduction to Error Handling in Go
In Go, error handling is done explicitly using the error
type. Unlike other languages that use exceptions, Go functions typically return an error value along with the result of the function. This makes error handling more predictable and forces the programmer to handle errors explicitly.
2. The Error Type in Go
In Go, an error is an interface type with a single method:
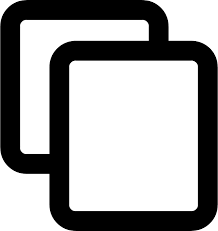
type error interface {
Error() string
}
Any type that implements this method can be treated as an error, allowing you to use custom error types to provide more detailed error messages.
3. Syntax for Returning Errors
Go functions commonly return an error value as the last return value. If there is no error, the function returns nil
for the error type. Here's the basic syntax:
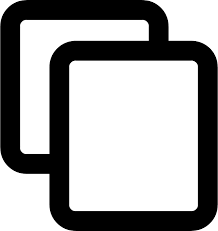
func someFunction() (resultType, error) {
// Function implementation
return result, nil // no error
// Or
return result, errors.New("something went wrong") // with error
}
4. Example: Returning Errors in a Function
Here's an example of a function that returns an error when something goes wrong:
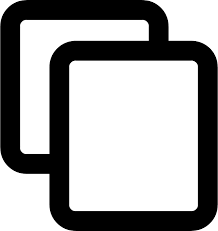
package main
import (
"errors"
"fmt"
)
func divide(a, b int) (int, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the divide
function returns an error if division by zero is attempted. Otherwise, it returns the result and nil
for the error.
5. Common Error Handling Patterns
In Go, error handling typically follows some common patterns:
- Check for Errors Immediately: After calling a function that returns an error, check the error value right away and handle it.
- Return Errors from Helper Functions: If a helper function encounters an error, it should propagate the error back to the caller for handling.
- Use Custom Error Types: For more complex error handling, you can create custom error types that provide more details about the error.
6. Example: Using Custom Error Types
Custom error types in Go can provide additional information beyond the basic error message. Here’s an example:
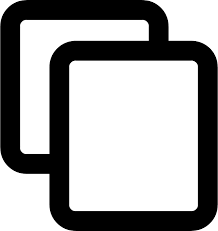
package main
import (
"fmt"
)
type DivideByZeroError struct {
message string
}
func (e *DivideByZeroError) Error() string {
return e.message
}
func divide(a, b int) (int, error) {
if b == 0 {
return 0, &DivideByZeroError{"cannot divide by zero"}
}
return a / b, nil
}
func main() {
_, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
}
}
In this example, the DivideByZeroError
is a custom error type that implements the Error()
method, providing a specific error message for division by zero.
7. Best Practices for Error Handling
- Return early: Handle errors immediately after a function call to keep the code clean and readable.
- Use
nil
for no errors: Always returnnil
when there is no error to signify success. - Be descriptive with error messages: Provide clear and meaningful error messages to make debugging easier.
- Use custom error types for complex errors: If necessary, create custom error types to carry more information about the error.
8. Conclusion
Returning errors in Go functions is a fundamental part of the language's error-handling philosophy. By explicitly checking for errors and using the error
type, Go encourages more predictable and robust error management in your programs. Following best practices ensures that your code is easier to maintain and debug.
Custom Error Types in Go
1. Introduction to Custom Error Types
In Go, errors are typically represented using the built-in error
type, which is an interface with a single method Error() string
. However, for more complex situations, you may want to create custom error types that carry additional information, such as error codes, context, or other relevant details. Custom error types allow for more meaningful error messages and better error handling in your Go programs.
2. Defining a Custom Error Type
To define a custom error type, you create a struct that includes the relevant fields. Then, you implement the Error()
method on that struct. Here's how you can define a basic custom error type:
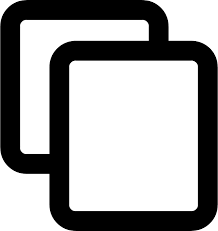
package main
import "fmt"
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error Code %d: %s", e.Code, e.Message)
}
func main() {
err := &MyError{Code: 404, Message: "Resource not found"}
fmt.Println(err)
}
In this example, MyError
is a custom error type that has two fields: Code
and Message
. The Error()
method returns a formatted string that includes the error code and message.
3. Returning Custom Errors from Functions
You can return your custom error types from functions just like regular errors. Here's an example:
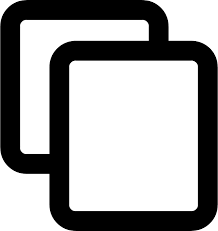
package main
import (
"errors"
"fmt"
)
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error Code %d: %s", e.Code, e.Message)
}
func doSomething() error {
return &MyError{Code: 500, Message: "Internal server error"}
}
func main() {
err := doSomething()
if err != nil {
fmt.Println("Error:", err)
}
}
In this example, the doSomething
function returns a custom error MyError
. The main function checks for the error and prints it if it exists.
4. Working with Custom Error Types
Once you have a custom error type, you can use it to provide additional context about errors in your application. For example, you can define methods that allow you to retrieve specific information from the custom error:
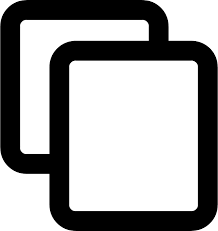
package main
import (
"fmt"
)
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error Code %d: %s", e.Code, e.Message)
}
func (e *MyError) GetCode() int {
return e.Code
}
func (e *MyError) GetMessage() string {
return e.Message
}
func main() {
err := &MyError{Code: 404, Message: "Page not found"}
if err != nil {
fmt.Println("Error Code:", err.GetCode())
fmt.Println("Error Message:", err.GetMessage())
}
}
In this example, the custom error type MyError
has additional methods GetCode()
and GetMessage()
that allow you to retrieve the error code and message separately.
5. Using Custom Error Types in Multiple Layers of an Application
Custom error types are especially useful in larger applications where errors need to be passed through multiple layers of a program. They allow you to propagate errors with context, which helps in debugging and understanding the flow of errors through your system. For instance, you can use custom error types to differentiate between errors that occur at different layers, like database errors, network errors, or business logic errors.
6. Example: Propagating Custom Errors
Here’s how you can propagate custom errors through multiple layers of an application:
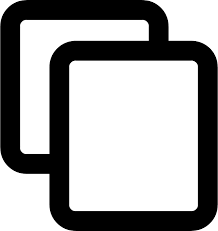
package main
import (
"fmt"
)
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error Code %d: %s", e.Code, e.Message)
}
func layerOne() error {
return &MyError{Code: 400, Message: "Bad Request"}
}
func layerTwo() error {
err := layerOne()
if err != nil {
return &MyError{Code: 500, Message: "Error in Layer Two: " + err.Error()}
}
return nil
}
func main() {
err := layerTwo()
if err != nil {
fmt.Println("Error:", err)
}
}
In this example, the error from layerOne
is propagated to layerTwo
, where it’s wrapped in a new MyError
to provide additional context.
7. Best Practices for Custom Error Types
- Use Custom Errors for Context: Create custom error types when you need to add context to your errors, such as error codes or extra details.
- Return Errors, Not Panics: Avoid using panics for recoverable errors. Instead, return errors that can be handled gracefully.
- Implement the
Unwrap()
Method for Wrapping Errors: In Go 1.13 and later, you can implement theUnwrap()
method to wrap errors, allowing you to chain multiple errors together. - Provide Meaningful Error Messages: When implementing
Error()
, ensure that the error message is descriptive and helpful for debugging.
8. Conclusion
Custom error types in Go provide a powerful way to add more context and detail to error messages. By using custom error types, you can improve error handling and make your Go programs more maintainable and easier to debug. By following best practices, you can create robust error handling strategies that scale with your application.
Using the errors Package in Go
1. Introduction to the errors Package
Go provides a standard library package called errors
to work with error handling. The errors
package allows you to create simple error values, as well as to wrap errors with additional context. This package is essential for effective error handling in Go programs, especially when you want to provide more detailed information about the cause of an error without losing the original error.
2. Creating Simple Errors with the errors Package
The errors.New()
function is used to create a basic error with a string message. Here's an example:
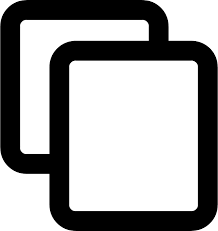
package main
import (
"errors"
"fmt"
)
func main() {
err := errors.New("This is a simple error")
fmt.Println(err)
}
In this example, the errors.New()
function creates a simple error with the message "This is a simple error," and then it is printed to the console.
3. Wrapping Errors with the errors Package
In Go 1.13 and later, the errors
package provides the fmt.Errorf()
function to wrap errors with additional context. This is useful when you want to add more information to an error while preserving the original error.
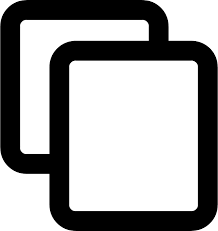
package main
import (
"errors"
"fmt"
)
func doSomething() error {
return errors.New("Something went wrong")
}
func main() {
err := doSomething()
if err != nil {
wrappedErr := fmt.Errorf("Wrapped error: %w", err)
fmt.Println(wrappedErr)
}
}
In this example, the error returned by the doSomething()
function is wrapped with additional context using fmt.Errorf()
and the %w
verb. The wrapped error is then printed to the console.
4. Unwrapping Errors
Starting with Go 1.13, you can unwrap an error to retrieve the original error using the errors.Unwrap()
function or the %w
verb in the fmt.Errorf()
function. This is useful for error inspection and handling.
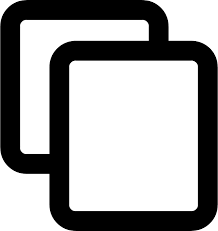
package main
import (
"errors"
"fmt"
)
func main() {
originalErr := errors.New("Original error")
wrappedErr := fmt.Errorf("Wrapped error: %w", originalErr)
// Unwrapping the error
unwrappedErr := errors.Unwrap(wrappedErr)
fmt.Println("Unwrapped error:", unwrappedErr)
}
In this example, the error is unwrapped using errors.Unwrap()
, and the original error is printed to the console.
5. Checking for Specific Errors
In some cases, you may want to check if an error matches a specific error value. The errors.Is()
function can be used to check whether an error is equal to a particular target error.
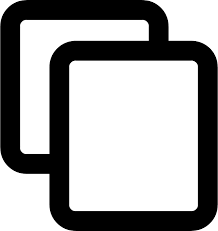
package main
import (
"errors"
"fmt"
)
var ErrNotFound = errors.New("Not found")
func doSomething() error {
return ErrNotFound
}
func main() {
err := doSomething()
if errors.Is(err, ErrNotFound) {
fmt.Println("Error: Item not found")
}
}
In this example, the errors.Is()
function checks if the error returned by the doSomething()
function is equal to the ErrNotFound
error, and prints an appropriate message.
6. Using errors in Custom Error Types
The errors
package can also be used to implement custom error types. You can use the fmt.Errorf()
function to wrap and propagate custom errors with additional context.
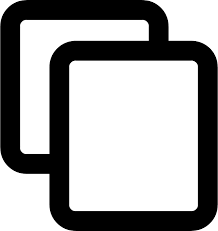
package main
import (
"errors"
"fmt"
)
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error Code %d: %s", e.Code, e.Message)
}
func main() {
myErr := &MyError{Code: 404, Message: "Resource not found"}
wrappedErr := fmt.Errorf("Wrapped custom error: %w", myErr)
if errors.Is(wrappedErr, myErr) {
fmt.Println("Custom error is wrapped:", wrappedErr)
}
}
In this example, a custom error type MyError
is created, and then it's wrapped using fmt.Errorf()
with the %w
verb. The errors.Is()
function is used to check if the wrapped error matches the original custom error.
7. Best Practices for Using the errors Package
- Use
errors.New()
for Simple Errors: For simple errors, useerrors.New()
to create lightweight error messages. - Wrap Errors with
fmt.Errorf()
: When adding context to an existing error, usefmt.Errorf()
with the%w
verb to preserve the original error. - Check for Specific Errors with
errors.Is()
: Useerrors.Is()
to check if an error is of a specific type or matches a particular value. - Unwrap Errors When Necessary: Use
errors.Unwrap()
to retrieve the original error from a wrapped error when needed. - Use Custom Errors for More Detail: For more complex errors, consider defining your custom error types and wrapping them with additional context.
8. Conclusion
The errors
package in Go provides essential tools for creating, wrapping, and checking errors. By using errors.New()
, fmt.Errorf()
, and errors.Is()
, you can handle errors effectively and add meaningful context to them. Wrapping and unwrapping errors is a powerful feature that helps you track the flow of errors in your application, making it easier to debug and maintain your code.
Dealing with Panic and Recover in Go
1. Introduction to Panic and Recover
In Go, panic
and recover
are mechanisms used for handling exceptional situations. A panic typically occurs when a program encounters an unexpected condition, and recover allows you to regain control after a panic occurs. These mechanisms are useful in situations where an error is so severe that the program cannot continue, but you want to prevent the program from crashing completely.
2. What is Panic?
panic
is used to abort the normal execution of a function and start unwinding the stack. When a panic occurs, the program will stop executing the current function and begin executing deferred functions in the current goroutine, unwinding the call stack until the program exits.
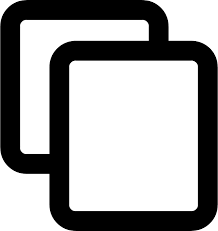
package main
import "fmt"
func triggerPanic() {
panic("Something went wrong!")
}
func main() {
fmt.Println("Before panic")
triggerPanic()
fmt.Println("After panic") // This will not be printed
}
In this example, the triggerPanic()
function causes a panic with the message "Something went wrong!" After the panic, the program stops executing, and the message "After panic" is never printed.
3. What is Recover?
recover
is used to regain control of a panicking goroutine. It can only be called inside a deferred function. If the current goroutine is panicking, recover will stop the panic and return the value passed to panic()
. If the current goroutine is not panicking, recover will return nil
.
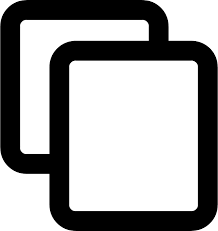
package main
import "fmt"
func recoverFromPanic() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}
func triggerPanic() {
defer recoverFromPanic()
panic("Something went wrong!")
}
func main() {
fmt.Println("Before panic")
triggerPanic()
fmt.Println("After panic") // This will be printed due to recover
}
In this example, the panic is caught by the deferred recoverFromPanic()
function, which prints the panic message and allows the program to continue executing after the panic.
4. Using Panic and Recover for Error Handling
While Go’s standard approach to error handling is through explicit error returns, panic and recover can be used for handling situations that are unexpected and should not occur under normal circumstances. For example, panic can be used when invalid arguments are passed to a function that the program cannot recover from, while recover can allow you to clean up or log the error before the program terminates.
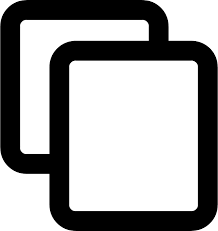
package main
import "fmt"
func divide(a, b int) int {
if b == 0 {
panic("division by zero")
}
return a / b
}
func handleDivision() {
defer recoverFromPanic()
fmt.Println(divide(10, 0)) // This will cause panic
}
func main() {
fmt.Println("Before division")
handleDivision()
fmt.Println("After division") // This will be printed due to recover
}
In this example, the divide()
function panics when division by zero occurs. The panic is caught by the deferred recoverFromPanic()
function, allowing the program to continue running after the panic.
5. Best Practices for Using Panic and Recover
- Avoid Overuse of Panic: Panic should be reserved for critical errors that cannot be handled by normal error handling mechanisms, such as internal inconsistencies or irrecoverable runtime errors.
- Use Recover for Cleanup: Use
recover
in deferred functions to clean up resources or log critical information before the program exits, but avoid using it to control normal program flow. - Do Not Rely on Panic for Normal Error Handling: For most error handling, you should return an error value from functions instead of using panic. Panic should not be used in place of regular error handling logic.
- Limit the Scope of Panic: Use panic in a controlled way and avoid allowing panics to propagate outside of specific areas where recovery is possible.
6. Example: Using Panic and Recover to Handle Multiple Errors
Sometimes, multiple errors may occur in a program, and panics may be used in combination with recover to handle each situation. This is a simple example of handling multiple errors in a sequence.
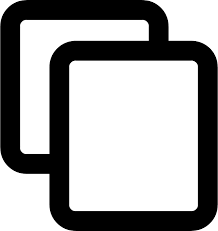
package main
import "fmt"
func firstError() {
panic("First error occurred")
}
func secondError() {
panic("Second error occurred")
}
func handleErrors() {
defer recoverFromPanic()
firstError()
secondError() // This will not be executed
}
func main() {
fmt.Println("Before handling errors")
handleErrors()
fmt.Println("After handling errors") // This will be printed due to recover
}
In this example, the first panic is recovered from, but the second one is not reached because the program has already panicked. This shows how the panic and recover pattern can be used to handle complex error scenarios.
7. Conclusion
Go provides the panic
and recover
mechanisms for dealing with critical errors that require immediate attention and prevention of program crashes. While panic can be used to stop execution in response to severe issues, recover allows you to regain control and continue execution. It’s important to use these mechanisms judiciously and to prefer error handling through explicit error returns in most cases. By following best practices, you can effectively manage unexpected situations in your Go programs.
Introduction to the Go Standard Library
1. What is the Go Standard Library?
The Go Standard Library is a collection of pre-written code that provides essential functions and utilities for Go programs. It includes packages for handling I/O, strings, networking, data serialization, concurrency, and much more. The Go Standard Library is designed to make it easier for developers to write efficient, cross-platform applications without needing to rely on third-party libraries for basic functionality.
2. Key Features of the Go Standard Library
- Comprehensive Coverage: The Go Standard Library covers a wide range of functionality, including networking, file handling, cryptography, HTTP, and more.
- Cross-Platform Support: Code written using the Go Standard Library is portable across different operating systems and architectures, making Go a great choice for building cross-platform applications.
- Optimized for Performance: The standard library packages are optimized for performance, helping you build efficient applications with minimal effort.
- Concurrency Support: Go’s concurrency model, known as goroutines, is supported by the standard library, with packages like
sync
andnet/http
facilitating concurrent programming.
3. Commonly Used Go Standard Library Packages
Below are some of the most commonly used packages in the Go Standard Library:
- fmt: Provides formatting and I/O functionality, such as printing to the console and formatting strings.
- net/http: Implements HTTP client and server functionality, useful for building web servers and making HTTP requests.
- os: Provides functions for interacting with the operating system, such as working with files, directories, and environment variables.
- strings: Offers functions for string manipulation, such as searching, splitting, and replacing substrings.
- encoding/json: Allows for encoding and decoding JSON data, commonly used for web APIs and data serialization.
- time: Provides functionality for working with dates, times, and durations.
- math: Includes basic mathematical functions such as trigonometric, logarithmic, and rounding operations.
- sync: Provides primitives for synchronizing goroutines, such as mutexes and wait groups.
4. Using the Go Standard Library
To use a package from the Go Standard Library, you simply import it into your program. Here’s an example of how to use the fmt
package to print a message to the console:
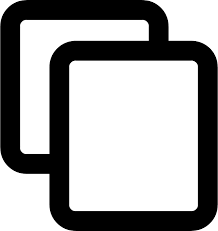
package main
import "fmt"
func main() {
fmt.Println("Hello, Go Standard Library!")
}
In this example, the fmt
package is imported, and the fmt.Println()
function is used to print the string "Hello, Go Standard Library!" to the console.
5. Example: Using the net/http Package
The net/http
package is used to build HTTP servers and make HTTP requests. Here’s an example of how to create a simple web server that responds with "Hello, World!" to HTTP requests:
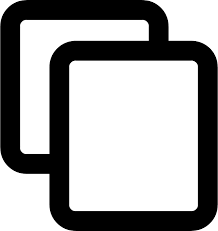
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
In this example, the program sets up an HTTP server that listens on port 8080 and responds with "Hello, World!" to any incoming requests.
6. Best Practices When Using the Go Standard Library
- Read the Documentation: The Go Standard Library is well-documented, and it’s important to refer to the official documentation when using packages to understand their functionality and usage patterns.
- Leverage Built-In Functionality: The standard library provides a wide range of tools, so before reaching for third-party libraries, check if the functionality you need is already included in the Go Standard Library.
- Understand Package Interdependencies: Some packages depend on other packages. Understanding how packages interact can help you write cleaner and more efficient code.
- Stay Up to Date: Keep track of updates to the Go Standard Library, as it evolves over time. New features and improvements are frequently added in new Go releases.
7. Conclusion
The Go Standard Library is one of the language’s greatest strengths, offering a rich set of packages for common tasks, from file handling to networking and concurrency. By leveraging the standard library, you can write powerful and efficient Go programs without needing to rely on external dependencies for basic functionality. Whether you're building a web server, performing data serialization, or working with concurrency, the Go Standard Library has you covered.
Commonly Used Packages: fmt, os, io, strconv
1. fmt Package
The fmt
package in Go provides functions for formatted I/O, such as printing output to the console or reading input from the user. It is one of the most commonly used packages in Go, allowing developers to format and display text in various ways.
2. Key Functions in the fmt Package
- fmt.Println(): Prints the arguments to the console, followed by a newline. Example:
fmt.Println("Hello, World!")
- fmt.Printf(): Prints formatted output to the console. It allows for placeholders and variable formatting. Example:
fmt.Printf("Hello, %s!", "Go")
- fmt.Sprintf(): Formats and returns a string rather than printing it. Example:
message := fmt.Sprintf("Hello, %s!", "Go")
3. os Package
The os
package provides functions for interacting with the operating system. You can use it to manipulate files, directories, environment variables, and more. This package is essential for handling system-level operations in Go.
4. Key Functions in the os Package
- os.Open(): Opens a file for reading. Example:
file, err := os.Open("file.txt")
- os.Create(): Creates a new file or opens an existing one for writing. Example:
file, err := os.Create("newfile.txt")
- os.Getenv(): Retrieves the value of an environment variable. Example:
value := os.Getenv("HOME")
- os.Exit(): Exits the program with a specified status code. Example:
os.Exit(1)
5. io Package
The io
package provides basic interfaces for I/O operations, such as reading from and writing to files, network connections, and more. It’s commonly used for handling streams of data.
6. Key Functions in the io Package
- io.Read(): Reads data from an I/O source, returning the number of bytes read and any error encountered. Example:
n, err := io.Read(file, buffer)
- io.Write(): Writes data to an I/O destination. Example:
n, err := io.Write(file, buffer)
- io.Copy(): Copies data from a source to a destination. It is often used to copy data between files or network connections. Example:
n, err := io.Copy(destFile, srcFile)
7. strconv Package
The strconv
package is used for converting between strings and basic data types such as integers, floats, and booleans. This package is especially useful for handling user input or serializing data.
8. Key Functions in the strconv Package
- strconv.Itoa(): Converts an integer to a string. Example:
str := strconv.Itoa(123)
- strconv.Atoi(): Converts a string to an integer. Example:
num, err := strconv.Atoi("123")
- strconv.ParseBool(): Converts a string to a boolean. Example:
value, err := strconv.ParseBool("true")
- strconv.FormatFloat(): Converts a floating-point number to a string with specified format. Example:
str := strconv.FormatFloat(3.14, 'f', -1, 64)
9. Example: Using fmt, os, io, and strconv Together
Here’s an example that demonstrates how to use the fmt
, os
, io
, and strconv
packages together:
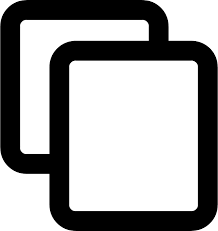
package main
import (
"fmt"
"os"
"io"
"strconv"
)
func main() {
// Using fmt to output a message
fmt.Println("Enter a number:")
// Using os to read input from the user
var input string
fmt.Scanln(&input)
// Using strconv to convert the input to an integer
num, err := strconv.Atoi(input)
if err != nil {
fmt.Println("Error:", err)
os.Exit(1)
}
// Using io to write the result to a file
file, err := os.Create("output.txt")
if err != nil {
fmt.Println("Error creating file:", err)
os.Exit(1)
}
defer file.Close()
result := "The number is: " + strconv.Itoa(num)
io.WriteString(file, result)
fmt.Println(result)
}
This program prompts the user to enter a number, converts the string input to an integer, and writes the result to a file using the fmt
, os
, io
, and strconv
packages.
10. Conclusion
The fmt
, os
, io
, and strconv
packages are essential building blocks for Go programs. They provide simple and effective ways to handle input/output operations, string conversions, and system-level tasks. Mastering these packages will help you write efficient and robust Go programs that interact seamlessly with users and the operating system.
Working with Files in Go
1. Introduction to File Handling in Go
In Go, file handling is done using the os
and io
packages. These packages provide various functions to open, read, write, and close files. Understanding how to work with files is essential for many applications, such as logging, data storage, and configuration management.
2. Opening Files
To open a file in Go, you use the os.Open()
or os.Create()
functions. The os.Open()
function is used to open an existing file for reading, while os.Create()
is used to create a new file or truncate an existing one.
3. Key Functions for Opening Files
- os.Open(): Opens a file for reading. If the file does not exist, an error is returned. Example:
file, err := os.Open("file.txt")
- os.Create(): Creates a new file or truncates an existing file for writing. Example:
file, err := os.Create("newfile.txt")
4. Reading from Files
To read from a file, you can use the ioutil.ReadFile()
function (deprecated, but still widely used) or os.Open()
and the Read()
method for more control.
5. Key Functions for Reading Files
- ioutil.ReadFile(): Reads the entire contents of a file into memory. Example:
content, err := ioutil.ReadFile("file.txt")
- os.Open() and Read(): Opens a file and reads it byte-by-byte. Example:
file, err := os.Open("file.txt") if err != nil { log.Fatal(err) } defer file.Close() buffer := make([]byte, 1024) n, err := file.Read(buffer) fmt.Println(string(buffer[:n]))
6. Writing to Files
To write to a file in Go, you can use the os.Create()
or os.OpenFile()
functions. These allow you to write data to a file or append to an existing file.
7. Key Functions for Writing Files
- os.Create(): Creates a new file (or truncates an existing one) and writes to it. Example:
file, err := os.Create("output.txt") if err != nil { log.Fatal(err) } defer file.Close() file.WriteString("Hello, Go!")
- os.OpenFile(): Opens a file for reading and writing. You can specify the file flags, such as
os.O_APPEND
to append to a file. Example:file, err := os.OpenFile("output.txt", os.O_APPEND|os.O_WRONLY, 0644) if err != nil { log.Fatal(err) } defer file.Close() file.WriteString("Appending text")
8. Closing Files
It’s important to close a file after you are done working with it to release resources. You can do this using the defer
keyword to ensure the file is closed when the function exits.
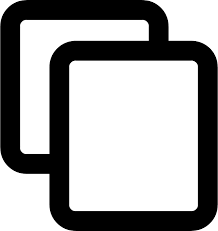
defer file.Close()
9. Example: Working with Files in Go
Here’s an example that demonstrates how to open, read, write, and close a file in Go:
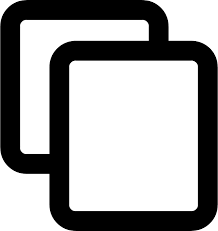
package main
import (
"fmt"
"os"
"log"
)
func main() {
// Create a new file and write to it
file, err := os.Create("example.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
_, err = file.WriteString("Hello, Go File Handling!")
if err != nil {
log.Fatal(err)
}
// Open the file for reading
file, err = os.Open("example.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
// Read the file content
content := make([]byte, 1024)
n, err := file.Read(content)
if err != nil {
log.Fatal(err)
}
fmt.Println("File Content:", string(content[:n]))
}
This program creates a file, writes to it, reads its content, and then prints the content to the console.
10. Handling Errors in File Operations
File operations may fail due to various reasons such as insufficient permissions, file not found, or errors while reading/writing. Always handle errors to ensure the program behaves correctly.
11. Conclusion
Working with files in Go is straightforward with the use of the os
and io
packages. Whether you are reading from or writing to files, Go provides simple and effective functions to handle these tasks efficiently. Remember to always close files after you're done and properly handle errors to make your file operations robust.
Regular Expressions in Go
1. Introduction to Regular Expressions
Regular expressions (regex) are patterns used to match character combinations in strings. Go provides built-in support for regular expressions through the regexp
package. Regular expressions are widely used for tasks such as validating inputs, searching and replacing text, and extracting information from strings.
2. The regexp
Package
The Go regexp
package allows you to compile and use regular expressions. It provides functions to match, find, and replace strings based on regular expression patterns.
3. Key Functions in the regexp
Package
- regexp.Compile(): Compiles a regular expression into a
Regexp
object that can be used for matching. Example:re, err := regexp.Compile("a[bcd]e")
- regexp.MustCompile(): Similar to
Compile()
, but panics if the regular expression is invalid. Example:re := regexp.MustCompile("a[bcd]e")
- regexp.MatchString(): Checks if a regular expression matches a string. Example:
match := regexp.MatchString("abc", "abcdef")
- Regexp.FindString(): Returns the first match of the regular expression in the string. Example:
match := re.FindString("abcdef")
- Regexp.FindAllString(): Returns all matches of the regular expression in the string. Example:
matches := re.FindAllString("abcdef abc", -1)
- Regexp.ReplaceAllString(): Replaces all occurrences of the regular expression in the string. Example:
result := re.ReplaceAllString("abcdef abc", "xyz")
4. Regular Expression Syntax
Go's regular expressions follow the syntax of Perl regular expressions. Here are some common components:
- Dot (.): Matches any single character except a newline. Example:
a.b
matches "acb", "axb", etc. - Character Classes: Defines a set of characters to match. Example:
[abc]
matches "a", "b", or "c". - Predefined Character Classes: Some common character classes are:
\d:
Matches any digit (0-9).\w:
Matches any word character (alphanumeric + underscore).\s:
Matches any whitespace character (space, tab, newline).\D:
Matches any non-digit character.
- Quantifiers: Defines how many times a character or group of characters should appear:
*:
Matches 0 or more occurrences.+:
Matches 1 or more occurrences.?:
Matches 0 or 1 occurrence.{n,m}:
Matches between n and m occurrences.
- Anchors: Specifies the position where the match should occur:
^:
Matches the beginning of the string.$:
Matches the end of the string.
5. Example: Matching Email Addresses
Here’s an example of using a regular expression to validate email addresses:
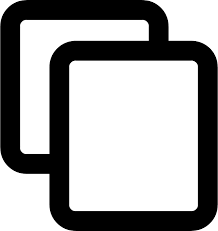
package main
import (
"fmt"
"regexp"
)
func main() {
email := "test@example.com"
re := regexp.MustCompile(`^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$`)
if re.MatchString(email) {
fmt.Println("Valid email address")
} else {
fmt.Println("Invalid email address")
}
}
This program uses a regular expression to check if the given email address is valid.
6. Example: Extracting Data with Groups
Regular expressions in Go support capturing groups. Here’s an example that extracts information from a string:
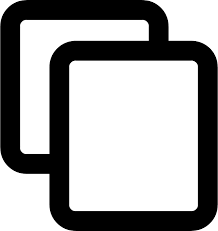
package main
import (
"fmt"
"regexp"
)
func main() {
text := "Order number: 12345, Date: 2025-01-16"
re := regexp.MustCompile(`Order number: (\d+), Date: (\d{4}-\d{2}-\d{2})`)
matches := re.FindStringSubmatch(text)
if len(matches) > 0 {
fmt.Println("Order number:", matches[1])
fmt.Println("Date:", matches[2])
}
}
This example uses a regular expression with capture groups to extract the order number and date from a string.
7. Example: Replacing Text
You can use regular expressions to replace parts of a string. Here's an example:
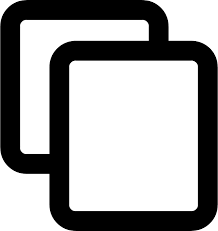
package main
import (
"fmt"
"regexp"
)
func main() {
text := "Hello, World!"
re := regexp.MustCompile(`World`)
result := re.ReplaceAllString(text, "Go")
fmt.Println(result)
}
This program replaces the word "World" with "Go", resulting in the output "Hello, Go!".
8. Error Handling with Regular Expressions
When working with regular expressions, it's important to handle errors properly. The regexp.Compile()
and regexp.MustCompile()
functions return an error if the regular expression is invalid. It's good practice to check for errors when using Compile()
.
9. Performance Considerations
Regular expressions are powerful but can be computationally expensive. When working with large datasets or complex patterns, it's important to consider performance. Avoid using very complex regular expressions in performance-critical parts of your application.
10. Conclusion
Regular expressions are a powerful tool for string manipulation in Go. The regexp
package provides a simple interface to compile, match, and manipulate strings using regular expressions. Understanding how to use regular expressions efficiently can save you a lot of time when working with text-based data.
JSON Handling in Go
1. Introduction to JSON in Go
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. Go provides built-in support for encoding and decoding JSON data through the encoding/json
package.
2. The encoding/json
Package
The encoding/json
package in Go provides functions for parsing JSON data into Go data structures and for encoding Go data structures into JSON format. The key functions include:
- json.Marshal(): Converts a Go value to JSON. Example:
json.Marshal(value)
- json.Unmarshal(): Converts JSON into a Go value. Example:
json.Unmarshal(data, &value)
- json.NewEncoder(): Creates a new encoder that writes JSON to an
io.Writer
. - json.NewDecoder(): Creates a new decoder that reads JSON from an
io.Reader
.
3. Marshaling (Encoding) Go Data to JSON
Marshaling is the process of converting a Go data structure into JSON format. You can use the json.Marshal()
function to achieve this. Here's an example:
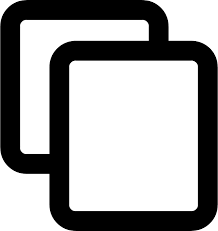
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
person := Person{"John Doe", 30}
jsonData, err := json.Marshal(person)
if err != nil {
fmt.Println("Error marshaling JSON:", err)
}
fmt.Println(string(jsonData))
}
In this example, the Person
struct is marshaled into a JSON string. The struct fields are tagged with JSON tags to specify how they should be named in the JSON output.
4. Unmarshaling (Decoding) JSON to Go Data
Unmarshaling is the process of converting JSON data into Go data structures. You can use the json.Unmarshal()
function to decode JSON into a Go value. Here's an example:
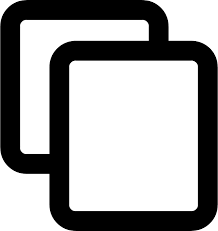
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
jsonData := `{"name":"John Doe", "age":30}`
var person Person
err := json.Unmarshal([]byte(jsonData), &person)
if err != nil {
fmt.Println("Error unmarshaling JSON:", err)
}
fmt.Println(person)
}
In this example, the JSON data is unmarshaled into a Person
struct. The struct fields are tagged to map the JSON keys to Go struct fields.
5. Handling JSON Arrays
Go can also handle JSON arrays. You can unmarshal a JSON array into a slice or array in Go. Here's an example:
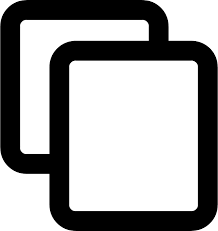
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
jsonData := `[{"name":"John Doe", "age":30}, {"name":"Jane Smith", "age":25}]`
var people []Person
err := json.Unmarshal([]byte(jsonData), &people)
if err != nil {
fmt.Println("Error unmarshaling JSON:", err)
}
fmt.Println(people)
}
This example unmarshals a JSON array into a slice of Person
structs. Each element in the JSON array corresponds to a Person
object.
6. JSON Tags in Go Structs
In Go, JSON tags are used to specify the names of JSON fields that correspond to struct fields. The tags are written in the form of json:"fieldname"
. You can also use tags to control other aspects of JSON encoding and decoding, such as omitting fields that are empty or renaming fields. Example:
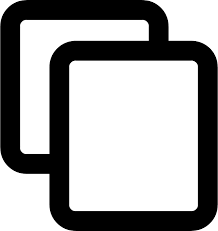
type Person struct {
Name string `json:"name"`
Age int `json:"age,omitempty"`
}
In this example, the omitempty
tag ensures that the Age
field is omitted from the JSON output if it is zero (the default value for integers in Go).
7. Working with Nested JSON
You can unmarshal and marshal nested JSON objects by using structs that contain other structs. Here's an example:
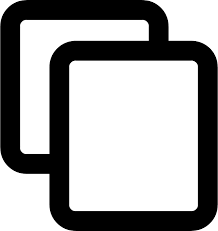
package main
import (
"encoding/json"
"fmt"
)
type Address struct {
Street string `json:"street"`
City string `json:"city"`
}
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Address Address `json:"address"`
}
func main() {
jsonData := `{"name":"John Doe", "age":30, "address":{"street":"123 Main St", "city":"New York"}}`
var person Person
err := json.Unmarshal([]byte(jsonData), &person)
if err != nil {
fmt.Println("Error unmarshaling JSON:", err)
}
fmt.Println(person)
}
This example shows how to unmarshal JSON data with nested objects (the address
field) into nested Go structs.
8. Encoding JSON with json.NewEncoder()
The json.NewEncoder()
function creates a new encoder that writes JSON to an io.Writer
. Here's an example of writing JSON to a file:
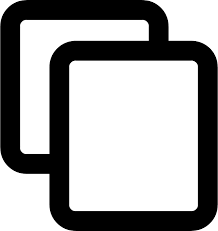
package main
import (
"encoding/json"
"fmt"
"os"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
person := Person{"John Doe", 30}
file, err := os.Create("person.json")
if err != nil {
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
encoder := json.NewEncoder(file)
err = encoder.Encode(person)
if err != nil {
fmt.Println("Error encoding JSON:", err)
}
}
This example writes the Person
struct to a file named person.json
in JSON format using the json.NewEncoder()
method.
9. Decoding JSON with json.NewDecoder()
The json.NewDecoder()
function creates a new decoder that reads JSON from an io.Reader
. Here's an example of reading JSON from a file:
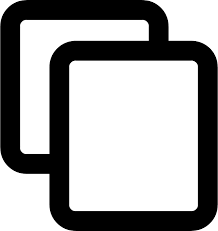
package main
import (
"encoding/json"
"fmt"
"os"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
file, err := os.Open("person.json")
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
var person Person
decoder := json.NewDecoder(file)
err = decoder.Decode(&person)
if err != nil {
fmt.Println("Error decoding JSON:", err)
}
fmt.Println(person)
}
This example reads JSON data from a file and unmarshals it into a Go struct using json.NewDecoder()
.
10. Conclusion
JSON handling in Go is straightforward thanks to the encoding/json
package. Go's support for marshaling and unmarshaling data makes it easy to work with JSON, whether you're encoding Go objects into JSON format or decoding JSON data into Go structs. By understanding how to use the key functions and features of this package, you can effectively manage JSON data in your Go applications.
Date and Time in Go
1. Introduction to Date and Time in Go
Go provides powerful tools for working with date and time through the time
package. This package allows you to manipulate and format dates and times, as well as perform operations such as parsing, formatting, and time comparison.
2. The time
Package
The time
package in Go includes a variety of functions and types for working with dates, times, and durations. Some of the key components of this package include:
- time.Time: The primary type for representing dates and times in Go.
- time.Duration: Represents the difference between two times, such as the number of seconds or nanoseconds.
- time.Now(): Returns the current local time.
- time.Parse(): Parses a string representing a time in a specific format.
- time.Format(): Formats a time into a string representation.
- time.Sleep(): Pauses the execution of the program for a specified duration.
3. Getting the Current Time
To get the current local time, you can use the time.Now()
function, which returns the current date and time as a time.Time
object. Here's an example:
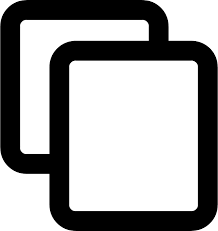
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
fmt.Println("Current time:", currentTime)
}
This code will print the current date and time in the local time zone.
4. Formatting Time
Go uses a unique way of formatting time. Instead of a common format string like "yyyy-mm-dd", Go uses a reference time: Mon Jan 2 15:04:05 2006
. You can format a time.Time
value by passing this reference time to the time.Format()
function. Here's an example:
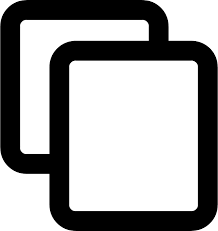
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("02-01-2006 03:04:05 PM")
fmt.Println("Formatted time:", formattedTime)
}
In this example, the current time is formatted in the format DD-MM-YYYY HH:mm:ss AM/PM
.
5. Parsing Time from a String
You can also parse time from a string using the time.Parse()
function. This function requires you to specify the layout format of the string. Here's an example:
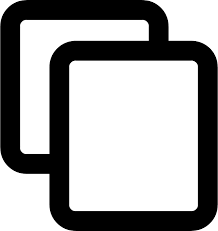
package main
import (
"fmt"
"time"
)
func main() {
timeString := "25-12-2025 10:30:00"
layout := "02-01-2006 15:04:05"
parsedTime, err := time.Parse(layout, timeString)
if err != nil {
fmt.Println("Error parsing time:", err)
return
}
fmt.Println("Parsed time:", parsedTime)
}
This code parses the string "25-12-2025 10:30:00"
into a time.Time
object using the layout DD-MM-YYYY HH:mm:ss
.
6. Calculating Durations
You can calculate the difference between two times using the time.Sub()
method, which returns a time.Duration
value. Here's an example:
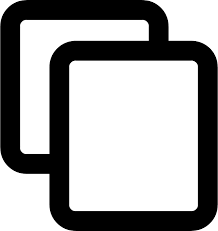
package main
import (
"fmt"
"time"
)
func main() {
startTime := time.Now()
time.Sleep(2 * time.Second)
endTime := time.Now()
duration := endTime.Sub(startTime)
fmt.Println("Duration:", duration)
}
This code calculates the duration between two time.Time
values and prints it. The program sleeps for 2 seconds between the two times, so the duration will be approximately 2 seconds.
7. Working with Time Zones
Go allows you to work with time zones using the time.Location
type. You can set the time zone when formatting or parsing times. Here's an example of working with time zones:
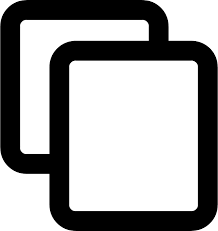
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
location, err := time.LoadLocation("America/New_York")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
newYorkTime := currentTime.In(location)
fmt.Println("Time in New York:", newYorkTime)
}
This example loads the time zone for New York and converts the current time to that time zone.
8. Sleeping and Waiting with Time
You can use the time.Sleep()
function to pause the execution of the program for a specific duration. It accepts a time.Duration
value. Here's an example:
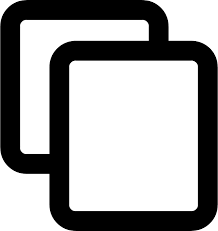
package main
import (
"fmt"
"time"
)
func main() {
fmt.Println("Starting sleep...")
time.Sleep(3 * time.Second)
fmt.Println("Woke up after 3 seconds.")
}
This code pauses the program for 3 seconds before printing a message.
9. Handling Timers and Tickers
Go provides timers and tickers for scheduling actions based on time intervals. A time.Timer
waits for a specific duration before sending a signal, and a time.Ticker
repeatedly sends a signal at specified intervals. Here's an example using a time.Ticker
:
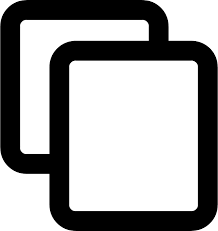
package main
import (
"fmt"
"time"
)
func main() {
ticker := time.NewTicker(2 * time.Second)
defer ticker.Stop()
for i := 0; i < 5; i++ {
<-ticker.C
fmt.Println("Tick", i+1)
}
}
This code uses a ticker to print a message every 2 seconds for 5 iterations.
10. Time Comparisons
You can compare time.Time
values using methods such as Before()
, After()
, and Equal()
. Here's an example:
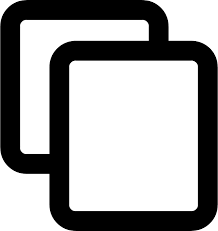
package main
import (
"fmt"
"time"
)
func main() {
time1 := time.Now()
time2 := time1.Add(1 * time.Hour)
fmt.Println("Is time1 before time2?", time1.Before(time2))
fmt.Println("Is time1 after time2?", time1.After(time2))
fmt.Println("Is time1 equal to time2?", time1.Equal(time2))
}
This example compares two time.Time
values and checks if one is before, after, or equal to the other.
11. Conclusion
The time
package in Go provides a rich set of functions and types for working with date and time. Understanding how to format, parse, manipulate, and compare times is essential for many applications. With the ability to handle time zones, durations, timers, and tickers, Go offers a robust toolkit for handling time-related tasks in your programs.
Introduction to Go Modules
1. What are Go Modules?
Go Modules are a way to manage dependencies in Go projects. Introduced in Go 1.11, they provide a more reliable and easier way to manage third-party libraries and dependencies compared to the older GOPATH-based workflow. Go Modules allow you to specify the exact versions of dependencies used in your project, ensuring that the project is reproducible and consistent across different environments.
2. Why Use Go Modules?
Go Modules solve several issues that were present in the older GOPATH model:
- Versioning: You can specify the exact versions of dependencies, avoiding conflicts and ensuring consistency across different environments.
- Compatibility: Go Modules work outside of the GOPATH, allowing for better project organization.
- Dependency management: Go Modules handle transitive dependencies automatically, removing the need to manually manage them.
- Clean project structure: Your Go code can be organized as a module, making it easier to build and maintain.
3. Enabling Go Modules
Go Modules are enabled by default in Go 1.13 and later. If you are using an older version of Go, you may need to upgrade to Go 1.11 or higher. To start using Go Modules, you must initialize a module in your project directory.
To initialize a Go module, run the following command in the root of your project:
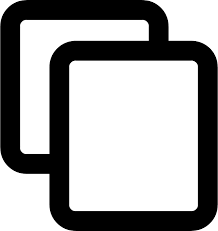
go mod init
This command creates a go.mod
file that tracks your module's dependencies.
4. The go.mod
File
The go.mod
file is the heart of a Go module. It contains metadata about the module, including its name and the versions of dependencies used. A typical go.mod
file looks like this:
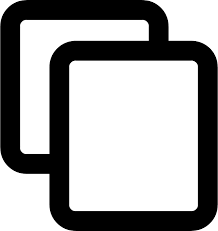
module github.com/username/project
go 1.18
require (
github.com/gin-gonic/gin v1.7.4
github.com/sirupsen/logrus v1.8.1
)
In this file:
- module: Specifies the module's name (usually the repository URL).
- go: Specifies the Go version used in the module.
- require: Lists the dependencies and their respective versions.
5. Managing Dependencies with Go Modules
Once you've initialized a Go module, you can start adding dependencies to your project. To add a dependency, simply import it into your Go code and run the following command:
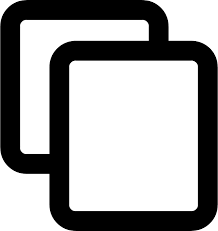
go get
This command will download the dependency and add it to the go.mod
file. For example, to add the popular web framework Gin
, you would run:
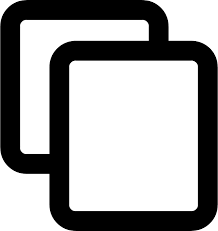
go get github.com/gin-gonic/gin
6. Updating Dependencies
To update a dependency to the latest version, you can use the following command:
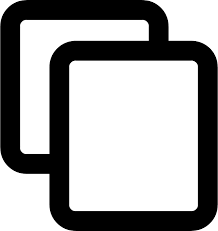
go get -u
For example, to update the Gin
framework, run:
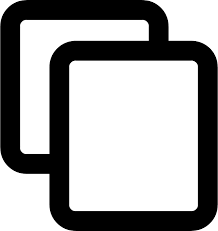
go get -u github.com/gin-gonic/gin
This will update the dependency to the latest version compatible with your Go version.
7. Go Module Proxy and Caching
Go Modules leverage a proxy to cache dependencies. By default, Go uses the Go Proxy to download and cache modules. This ensures that dependencies are available even if the upstream repository goes down.
You can configure Go Modules to use a different proxy or disable it entirely by setting the GO_PROXY
environment variable:
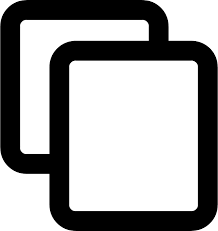
export GO_PROXY=https://proxy.golang.org
Additionally, Go caches downloaded modules in the $GOPATH/pkg/mod
directory for faster access during subsequent builds.
8. Go.sum File
The go.sum
file is automatically generated when you run commands like go mod tidy
. This file contains cryptographic hashes of all the modules your project depends on, ensuring that the exact same versions are used every time your project is built. It helps to verify the integrity of dependencies and prevents tampering.
9. Tidying Up Dependencies
As your project evolves, you may add or remove dependencies. The go mod tidy
command is used to clean up the go.mod
and go.sum
files by removing any dependencies that are no longer required:
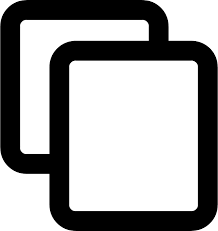
go mod tidy
This command will remove any unused dependencies and ensure that the go.mod
and go.sum
files are in sync with the actual code.
10. Versioning Dependencies
Go Modules allow you to specify exact versions for your dependencies. When adding or updating dependencies, you can specify a version number or commit hash. For example, to install a specific version of a package, you can run:
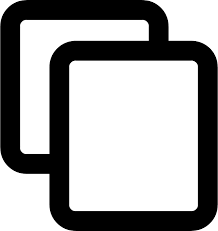
go get github.com/gin-gonic/gin@v1.7.4
This will install version 1.7.4 of the Gin
framework.
11. Removing Dependencies
If you no longer need a dependency, you can remove it from your project by simply deleting the import statement from your code. After that, run:
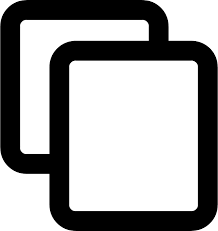
go mod tidy
This will remove the dependency from the go.mod
and go.sum
files.
12. Conclusion
Go Modules provide a powerful and flexible way to manage dependencies in Go projects. They make it easier to work with third-party libraries, handle versioning, and ensure that your projects are reproducible and maintainable. By using Go Modules, you can simplify your workflow and focus on writing Go code instead of managing dependencies.
Creating and Using Go Modules
1. What are Go Modules?
Go Modules are a way to manage dependencies in Go projects. Go Modules were introduced in Go 1.11 to address the limitations of the GOPATH-based dependency management. They allow for versioned dependencies, making it easier to build and manage projects with external libraries.
2. Enabling Go Modules
In Go 1.11 and later, Go Modules are enabled by default when you are outside of the GOPATH. You don't need to make any specific configuration changes to enable Go Modules. However, if you are still working in a GOPATH environment, you can explicitly enable Go Modules by setting the environment variable:
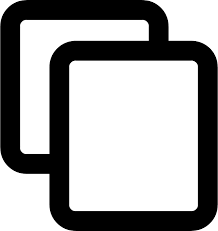
export GO111MODULE=on
With Go 1.13 and later, Go Modules are enabled by default, even if you're inside the GOPATH.
3. Initializing a Go Module
To create a Go module, you need to initialize the module in your project directory. Navigate to your project folder and run:
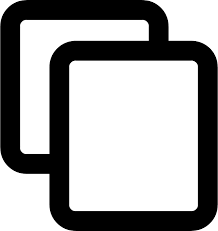
go mod init
This command creates a new go.mod
file in your project directory, which contains metadata about the module, including the module's name and its dependencies.
For example, if you're creating a Go module for a project called "myapp," you would run:
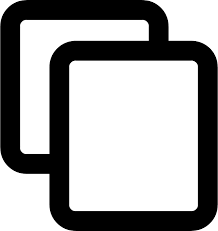
go mod init github.com/username/myapp
4. The go.mod
File
The go.mod
file is the configuration file for Go Modules. It records the module’s dependencies and provides information about the Go version your project is using. A basic go.mod
file might look like this:
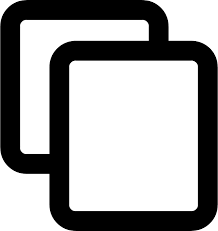
module github.com/username/myapp
go 1.18
require (
github.com/gin-gonic/gin v1.7.4
github.com/sirupsen/logrus v1.8.1
)
In this file:
- module: Specifies the name of your module, which is typically the repository URL.
- go: Specifies the version of Go you are using for the module.
- require: Lists the dependencies your module requires, along with their versions.
5. Adding Dependencies
To add a dependency to your Go module, import the package into your Go code and then use the go get
command. The go get
command downloads the dependency and updates the go.mod
file automatically.
For example, to add the Gin
web framework to your project, run:
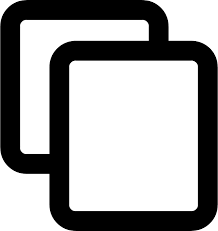
go get github.com/gin-gonic/gin
This command fetches the latest version of the Gin framework and adds it as a dependency to your project.
6. Updating Dependencies
You can update your dependencies to the latest available versions with the following command:
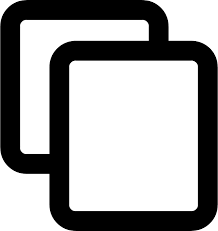
go get -u
For example, to update the Gin web framework to the latest version, run:
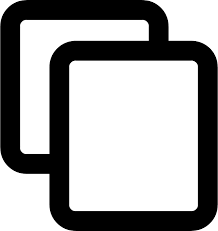
go get -u github.com/gin-gonic/gin
To update all dependencies at once, use:
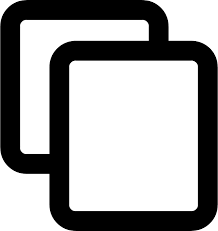
go get -u ./...
7. Removing Dependencies
If you no longer need a dependency, remove the import statement from your code. Then, run the following command to clean up your go.mod
and go.sum
files:
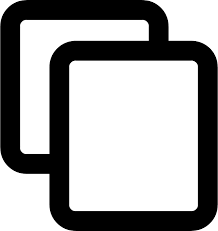
go mod tidy
This will remove any dependencies that are no longer needed and clean up the module files.
8. The go.sum
File
The go.sum
file contains cryptographic checksums for the dependencies used in your module. It ensures the integrity of the modules, preventing tampering. The file is automatically updated when you add or update dependencies.
You should include the go.sum
file in your version control system (e.g., Git) to ensure that others working on the project can verify the exact versions of dependencies used in the build.
9. Versioning in Go Modules
Go Modules allow you to specify exact versions of dependencies. You can specify a version when adding a dependency using @
to indicate a specific version or commit hash.
For example, to install a specific version of a package, run:
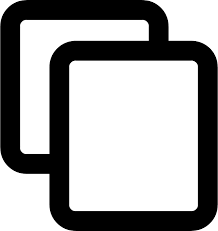
go get github.com/gin-gonic/gin@v1.7.4
This will install version v1.7.4
of the Gin framework.
10. Go Module Proxy
By default, Go Modules use the Go Proxy to download modules. The Go Proxy caches dependencies to improve performance and reliability. If necessary, you can configure Go Modules to use a different proxy or disable it entirely by setting the GO_PROXY
environment variable:
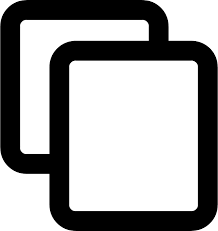
export GO_PROXY=https://proxy.golang.org
To disable the proxy, run:
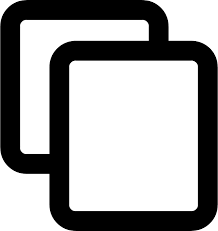
export GO_PROXY=direct
11. Go Module Commands
Here are some common Go Module commands:
- go mod init
: Initializes a new Go module in the current directory. - go get
: Adds a new dependency to the module. - go mod tidy: Removes unused dependencies and tidies the
go.mod
andgo.sum
files. - go mod vendor: Creates a
vendor
directory with the dependencies for your project. - go list -m all: Lists all modules and their dependencies used in your project.
12. Conclusion
Go Modules simplify dependency management and help you maintain a reproducible and consistent build environment. By using Go Modules, you can easily add, update, and remove dependencies, and handle versioning in your Go projects. The go.mod
and go.sum
files make it easy to track and manage dependencies, improving the reliability and maintainability of your Go code.
Dependency Management with Go Modules
1. What is Dependency Management?
Dependency management refers to the process of handling the libraries or packages that your Go project relies on. Go Modules offer a robust solution for managing these dependencies in a way that ensures consistency, versioning, and reproducibility across different environments and builds.
2. The Role of Go Modules in Dependency Management
Go Modules provide an easy-to-use system for tracking dependencies, specifying their versions, and ensuring that the correct versions are used in your project. By using Go Modules, developers can manage external libraries without worrying about conflicts or changes in the codebase of those libraries.
3. Understanding the go.mod
File
The go.mod
file is the heart of dependency management in Go Modules. It contains metadata about the module, including the module name and a list of dependencies with their specific versions. The go.mod
file is typically located in the root of your project.
A simple go.mod
file might look like this:
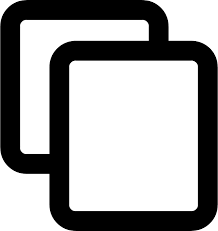
module github.com/username/myapp
go 1.18
require (
github.com/gin-gonic/gin v1.7.4
github.com/sirupsen/logrus v1.8.1
)
In this file:
- module: Specifies the name of your module, typically the repository URL.
- go: The Go version used in the project.
- require: A list of dependencies and their corresponding versions.
4. Adding Dependencies
To add a dependency to your Go project, you can use the go get
command. This command downloads the dependency and updates the go.mod
file with the new package and version.
For example, to add the Gin
web framework to your project, run:
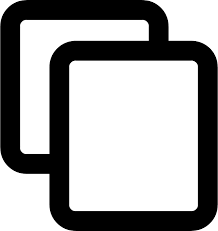
go get github.com/gin-gonic/gin
This command will automatically add Gin
and its version to the go.mod
file.
5. Managing Dependency Versions
Go Modules help you manage dependency versions. You can specify a specific version of a package by appending the version to the dependency name:
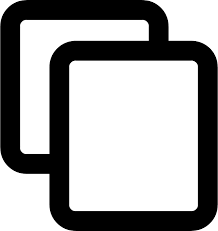
go get github.com/gin-gonic/gin@v1.7.4
You can also use semantic versioning, which includes major, minor, and patch versions:
- Major version: Significant changes that may break compatibility.
- Minor version: Backward-compatible changes with new features.
- Patch version: Backward-compatible fixes for bugs or issues.
If you want to update all dependencies to their latest compatible versions, you can use the following command:
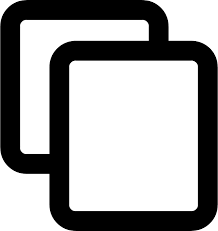
go get -u ./...
6. Removing Unused Dependencies
When you no longer use a dependency, you can remove it from your code. After that, run the go mod tidy
command to automatically clean up the go.mod
and go.sum
files:
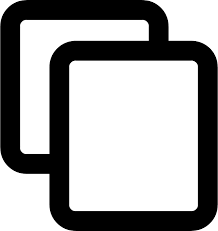
go mod tidy
This command removes any dependencies that are no longer required and ensures that your module files are up-to-date.
7. The go.sum
File
The go.sum
file is automatically generated and updated when adding or updating dependencies. It contains cryptographic checksums for each dependency used by your module, ensuring the integrity and security of the dependencies. The go.sum
file helps prevent malicious modifications to packages and verifies that the dependencies are the correct versions.
It is important to include the go.sum
file in your version control system to guarantee the consistency of your dependencies across different environments.
8. Handling Dependency Conflicts
Dependency conflicts arise when two different packages require different versions of the same dependency. Go Modules resolve conflicts by choosing the version that satisfies all constraints, which is typically the latest compatible version. However, if needed, you can specify the version of a dependency explicitly to avoid conflicts:
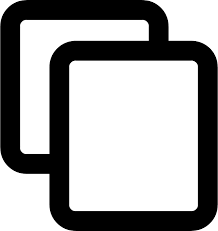
go get github.com/gin-gonic/gin@v1.7.4
If conflicts persist, consider reviewing the conflicting dependencies and adjusting versions accordingly, or use tools like go mod graph
to visualize the dependency tree.
9. Vendor Directory
Go Modules support the use of a vendor
directory, where all the dependencies are stored locally. This is useful for projects that need to ensure that dependencies are available even when the network is not accessible or when working in an isolated environment. To create a vendor
directory, use:
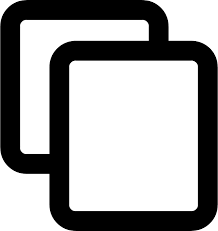
go mod vendor
This command copies all the dependencies listed in your go.mod
file to a local vendor
directory.
10. Using Private Repositories
Go Modules support private repositories. To access private modules, you may need to configure your environment to authenticate using a private Git repository. Use the GOPRIVATE
environment variable to specify the private repositories:
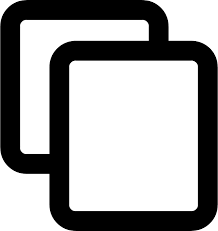
export GOPRIVATE=github.com/private/*
You may also need to configure authentication with your Git host to fetch private modules.
11. Dependency Management Best Practices
Here are some best practices for managing dependencies with Go Modules:
- Use Go Modules for all projects: It’s recommended to use Go Modules even for small projects to benefit from versioning and reproducibility.
- Keep dependencies up-to-date: Regularly update your dependencies to take advantage of bug fixes, security patches, and new features.
- Minimize direct dependencies: Use indirect dependencies judiciously and avoid unnecessary dependencies to reduce potential conflicts and vulnerabilities.
- Use semantic versioning: Follow semantic versioning for your own modules to make it clear when changes will break backward compatibility.
- Review the
go.mod
file: Periodically check thego.mod
andgo.sum
files for any vulnerabilities or unwanted dependencies.
12. Conclusion
Dependency management with Go Modules significantly streamlines the process of managing and versioning external libraries in your Go projects. By using the go.mod
and go.sum
files, you can ensure the consistency, security, and reproducibility of your project’s dependencies. Regularly updating and tidying up your dependencies can help maintain a clean and efficient project.
Versioning in Go Modules
1. What is Versioning in Go Modules?
Versioning in Go Modules allows you to track and specify which versions of dependencies your Go project uses. Go Modules make it easy to manage dependencies and ensure that your project uses the correct version of a library, which helps avoid compatibility issues and maintain stability across different environments.
2. Semantic Versioning (SemVer)
Go Modules follow Semantic Versioning (SemVer), a versioning scheme that uses three numbers: major, minor, and patch.
- Major version: Significant changes that may break backward compatibility.
- Minor version: Backward-compatible changes that introduce new features.
- Patch version: Backward-compatible fixes for bugs or issues.
A version number might look like v1.2.3
, where:
- 1 is the major version.
- 2 is the minor version.
- 3 is the patch version.
3. Specifying Versions in the go.mod
File
To manage dependencies, Go Modules uses the go.mod
file, where you can specify the exact version of a dependency. For example, to use a specific version of a dependency, you can define it in the go.mod
file like this:
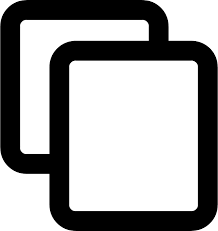
require github.com/gin-gonic/gin v1.7.4
This tells Go to use version v1.7.4
of the gin-gonic/gin
package.
4. Updating Dependencies
You can update dependencies to a newer version using the go get
command. This updates the version in your go.mod
file, and downloads the new version:
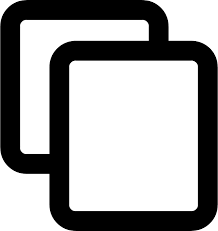
go get github.com/gin-gonic/gin@v1.8.0
This command updates the dependency to version v1.8.0
and modifies the go.mod
file accordingly.
5. Downgrading Dependencies
If you need to downgrade to an earlier version of a dependency, you can specify the desired version with the go get
command:
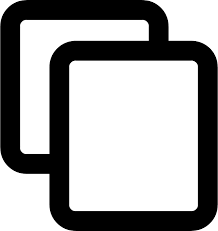
go get github.com/gin-gonic/gin@v1.6.0
This command downgrades the gin
dependency to version v1.6.0
and updates the go.mod
file.
6. Understanding Version Constraints
Go Modules supports version constraints, which allow you to specify compatible versions using operators such as:
- ^ (caret): Allows updates that do not change the major version.
- ~ (tilde): Allows updates that do not change the minor version.
- >=, <=, <, >: Specifies an exact range of allowed versions.
- vX.Y.Z: Exact version specification.
For example, to allow any version greater than or equal to v1.7.0
but less than v2.0.0
, you would use:
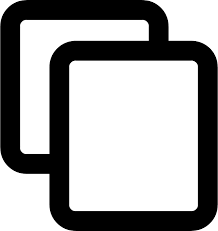
require github.com/gin-gonic/gin v1.7.0
This ensures your project uses a compatible version without moving to a breaking change in a major version.
7. The go.mod
Versioning Commands
Go provides several commands for managing versioning in Go Modules:
- go mod tidy: Removes unused dependencies and ensures that the
go.mod
andgo.sum
files are clean and up-to-date. - go mod verify: Verifies that the dependencies in your
go.mod
file are consistent and have not been tampered with. - go mod graph: Displays the dependency graph of your project, showing how the dependencies are interconnected.
- go list -m all: Lists all modules, including indirect dependencies, that are required by your project.
8. Handling Pre-release Versions
In Go Modules, you can specify pre-release versions (such as alpha, beta, or RC versions) by including the pre-release tag in the version. For example, to use a pre-release version like v1.7.0-beta
, you would use:
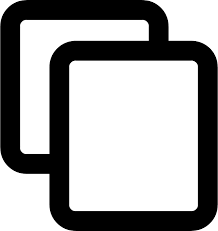
go get github.com/gin-gonic/gin@v1.7.0-beta
Pre-release versions can help test new features and updates before they become stable.
9. Using Go Modules with Git Tags
Go Modules use Git tags to track versions. A Git tag like v1.7.0
corresponds to a version in the go.mod
file. When you release a new version of your project, you should create a Git tag to indicate the release version:
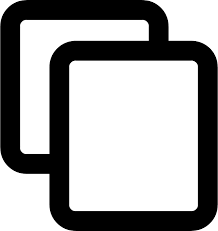
git tag v1.7.0
Once the tag is pushed to the repository, Go can automatically fetch the appropriate version of your module using the tag.
10. Versioning Best Practices
Here are some best practices for versioning your Go Modules:
- Use Semantic Versioning: Follow SemVer for backward compatibility and clear communication about changes to your library.
- Tag Releases: Always tag releases in your Git repository to keep track of versions, making it easier to fetch and use specific versions.
- Update Dependencies Regularly: Keep your dependencies up-to-date to benefit from bug fixes, security patches, and new features.
- Avoid Breaking Changes: If possible, avoid making breaking changes in a minor or patch version. If breaking changes are necessary, increment the major version number.
11. Conclusion
Versioning in Go Modules helps ensure that the dependencies used in your Go project are stable, secure, and compatible. By following Semantic Versioning, you can easily manage dependencies, track changes, and avoid compatibility issues. Using tools like go get
and go mod
commands makes it simple to update, manage, and specify versions for your project’s dependencies.
Introduction to Web Development in Go
1. What is Web Development in Go?
Web development in Go refers to building web applications and services using the Go programming language. Go is a statically typed, compiled language developed by Google that has gained popularity for its simplicity, speed, and efficiency. It is particularly well-suited for creating scalable and high-performance web applications, making it a popular choice for backend development.
2. Why Use Go for Web Development?
Go provides several benefits for web development:
- Performance: Go’s compiled nature ensures that web applications built with Go are fast and efficient, capable of handling high concurrency and heavy traffic.
- Concurrency: Go's goroutines and channels make it easy to handle multiple tasks concurrently, ideal for handling numerous web requests simultaneously.
- Simplicity: Go is known for its simplicity and ease of use, making it easier for developers to build and maintain web applications.
- Scalability: Go is designed for building scalable applications, making it perfect for large-scale web applications that need to handle growing user bases.
- Standard Library: Go’s extensive standard library includes packages for web development, such as
net/http
, making it simple to build web applications without the need for external frameworks.
3. Setting Up a Basic Web Server in Go
To create a basic web server in Go, you can use the built-in net/http
package. Here’s a simple example of a web server that responds with "Hello, World!" to any HTTP request:
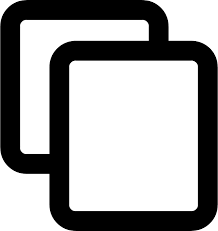
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
In this example:
http.HandleFunc("/", handler)
associates the root URL ("/") with thehandler
function.http.ListenAndServe(":8080", nil)
starts the server on port 8080.
To run the server, save the code to a file (e.g., main.go
) and run the following command:
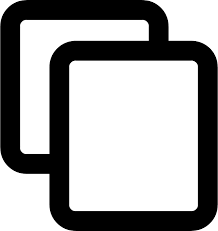
go run main.go
Visit http://localhost:8080
in your browser, and you should see "Hello, World!" displayed.
4. Handling HTTP Requests and Responses
Go’s net/http
package makes it easy to handle HTTP requests and responses. You can define functions to process different HTTP methods (GET, POST, PUT, DELETE, etc.), manage query parameters, and return responses in various formats (JSON, HTML, etc.).
Example: Handling GET and POST Requests
Here’s an example of handling both GET and POST requests:
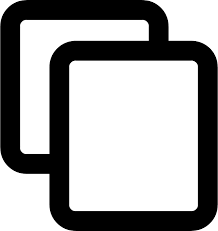
package main
import (
"fmt"
"net/http"
)
func getHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "GET request received")
}
func postHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "POST request received")
}
func main() {
http.HandleFunc("/get", getHandler)
http.HandleFunc("/post", postHandler)
http.ListenAndServe(":8080", nil)
}
In this example, /get
handles GET requests, and /post
handles POST requests. You can test these by sending different types of requests using a tool like Postman or cURL.
5. Routing in Go Web Development
Routing refers to mapping URLs to specific handler functions. The Go net/http
package provides basic routing capabilities, but for more advanced routing (e.g., URL parameters), you can use third-party routing libraries such as Gorilla Mux or Gin.
Example: Using Gorilla Mux
Here's how to use the Gorilla Mux router for handling URL parameters:
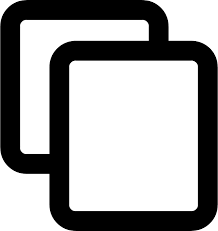
package main
import (
"fmt"
"github.com/gorilla/mux"
"net/http"
)
func handleRequest(w http.ResponseWriter, r *http.Request) {
vars := mux.Vars(r)
name := vars["name"]
fmt.Fprintf(w, "Hello, %s!", name)
}
func main() {
r := mux.NewRouter()
r.HandleFunc("/hello/{name}", handleRequest)
http.ListenAndServe(":8080", r)
}
In this example, the URL /hello/{name}
accepts a dynamic parameter name
, which is extracted and used in the response.
6. Structuring Web Applications in Go
For larger web applications, it’s important to structure your code effectively. A common approach is to organize the project into packages for routing, handlers, and models. Here's an example of a simple project structure:
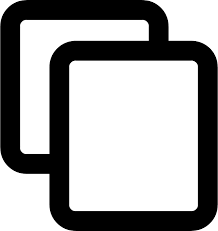
myapp/
├── main.go
├── handlers/
│ └── handler.go
└── models/
└── user.go
This structure keeps the code modular and easier to maintain. The main.go
file sets up the web server, while the handlers/
package contains the request handler functions, and the models/
package contains the application data models.
7. Handling JSON in Go Web Development
In web development, it’s common to interact with JSON data. Go makes it easy to encode and decode JSON using the encoding/json
package. Here’s an example of handling JSON in a web application:
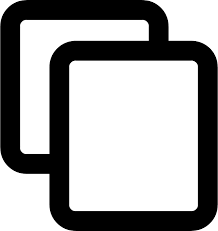
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Message struct {
Text string `json:"text"`
}
func handler(w http.ResponseWriter, r *http.Request) {
message := Message{Text: "Hello, World!"}
json.NewEncoder(w).Encode(message)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sends a JSON response with the message “Hello, World!” when you visit the root URL.
8. Conclusion
Go is a powerful and efficient language for web development, offering simplicity, speed, and scalability. With its built-in net/http
package and support for third-party libraries, Go is ideal for building modern web applications. Whether you are building small APIs or large-scale web services, Go provides the tools you need to create high-performance web applications with ease.
Handling HTTP Requests and Responses in Go
1. Introduction to HTTP Requests and Responses
In Go, handling HTTP requests and responses is done using the net/http
package. This package provides functions to process incoming HTTP requests and send corresponding HTTP responses. Web servers in Go can handle various HTTP methods such as GET, POST, PUT, DELETE, etc., and return responses in different formats like HTML, JSON, or plain text.
2. Creating a Simple Web Server to Handle HTTP Requests
To handle HTTP requests, you first need to create an HTTP server. Here's an example of a basic Go web server that handles a GET request:
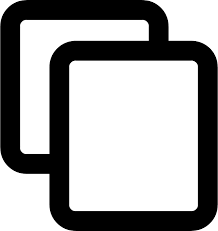
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sets up a simple web server that listens on port 8080 and responds with "Hello, World!" for any incoming request to the root URL (/
).
3. Handling Different HTTP Methods
Go allows you to handle different HTTP methods like GET, POST, PUT, DELETE, etc. In the net/http
package, you can use the http.HandleFunc
function to specify which handler to call for each method. Here's an example of handling GET and POST requests:
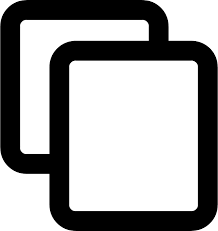
package main
import (
"fmt"
"net/http"
)
func getHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "GET request received")
}
func postHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "POST request received")
}
func main() {
http.HandleFunc("/get", getHandler)
http.HandleFunc("/post", postHandler)
http.ListenAndServe(":8080", nil)
}
In this example, the server handles GET requests at /get
and POST requests at /post
.
4. Reading Request Data
When handling requests, you often need to read the data sent by the client. This can include query parameters, form data, and JSON payloads. The net/http
package provides methods to retrieve these values.
Example: Reading Query Parameters
Query parameters are part of the URL and can be extracted using the r.URL.Query()
method:
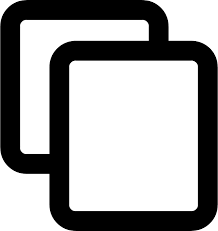
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
name := r.URL.Query().Get("name")
fmt.Fprintf(w, "Hello, %s!", name)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
In this example, the web server expects a query parameter name
in the URL. For example, http://localhost:8080/?name=John
would return "Hello, John!".
Example: Reading Form Data
For POST requests with form data, you can use r.ParseForm()
to parse the data:
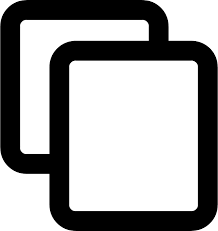
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
r.ParseForm()
name := r.FormValue("name")
fmt.Fprintf(w, "Hello, %s!", name)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example expects a form field named name
to be sent in a POST request, such as in an HTML form.
Example: Reading JSON Data
If the client sends JSON data, you can decode it using the encoding/json
package:
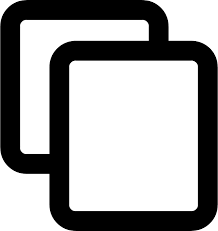
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Message struct {
Text string `json:"text"`
}
func handler(w http.ResponseWriter, r *http.Request) {
var msg Message
decoder := json.NewDecoder(r.Body)
err := decoder.Decode(&msg)
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
fmt.Fprintf(w, "Received message: %s", msg.Text)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example expects the client to send a JSON object with a field named text
. The server decodes the JSON and responds with the received message.
5. Sending HTTP Responses
Once you've processed the request, you can send a response back to the client. The http.ResponseWriter
interface is used to write the response. You can set the response status code, headers, and body.
Example: Sending a Plain Text Response
To send a plain text response, simply use the fmt.Fprintln
function:
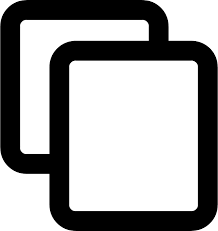
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "text/plain")
fmt.Fprintln(w, "This is a plain text response")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sends a plain text response with the header Content-Type: text/plain
.
Example: Sending a JSON Response
To send a JSON response, you can use the json.NewEncoder
function:
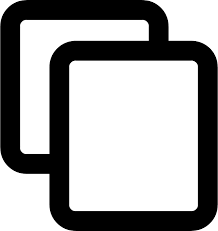
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Response struct {
Message string `json:"message"`
}
func handler(w http.ResponseWriter, r *http.Request) {
response := Response{Message: "Hello, World!"}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(response)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sends a JSON response with the Content-Type: application/json
header and a JSON body that contains the message "Hello, World!".
6. Setting Response Status Codes
You can set the HTTP status code for the response using the w.WriteHeader
method. If you don't set a status code, the default is 200 OK.
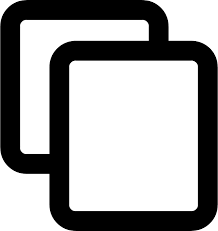
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
w.WriteHeader(http.StatusNotFound)
fmt.Fprintln(w, "Page not found")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sets the status code to 404 (Not Found) and sends a message to the client.
7. Handling Errors in HTTP Requests
When handling HTTP requests, it's important to handle errors appropriately. You can use the http.Error
function to return error messages with specific status codes.
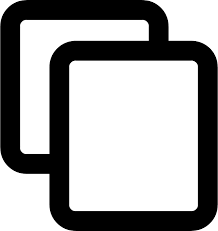
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
if r.Method != http.MethodGet {
http.Error(w, "Method not allowed", http.StatusMethodNotAllowed)
return
}
fmt.Fprintln(w, "GET request received")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example returns a 405 Method Not Allowed error if the client sends a request with any method other than GET.
8. Conclusion
Handling HTTP requests and responses is a fundamental part of web development in Go. By using the net/http
package, you can easily process incoming requests, extract data, and send appropriate responses back to the client. With Go’s simplicity and performance, building web applications becomes a streamlined process.
Creating a Simple Web Server in Go
1. Introduction to Web Servers in Go
Go provides a built-in net/http
package that makes it easy to create a simple web server. This package can handle incoming HTTP requests, route them to appropriate handlers, and send responses back to clients. A basic Go web server can handle multiple HTTP methods and serve static or dynamic content.
2. Setting Up the Web Server
To create a simple web server in Go, you need to use http.ListenAndServe
to listen for incoming HTTP requests on a specified port and route them to handlers. Here’s an example of the most basic Go web server:
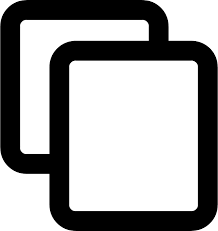
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
})
http.ListenAndServe(":8080", nil)
}
This example creates a web server that listens on port 8080. When you visit http://localhost:8080/
, the server responds with "Hello, World!"
3. Handling Requests with Handlers
In Go, you define handlers to handle specific HTTP requests. A handler is a function that takes an http.ResponseWriter
and an http.Request
as parameters. These handlers are registered using http.HandleFunc
, which associates a URL path with the handler function.
Example: Basic Request Handling
Here’s an example of a basic handler that responds to a GET request:
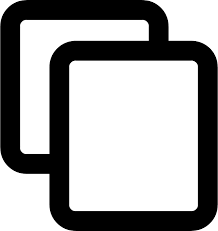
package main
import (
"fmt"
"net/http"
)
func greetHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Welcome to the Go web server!")
}
func main() {
http.HandleFunc("/greet", greetHandler)
http.ListenAndServe(":8080", nil)
}
In this example, the server listens on port 8080 and responds with "Welcome to the Go web server!" when you visit http://localhost:8080/greet
.
4. Handling Different HTTP Methods
Go allows you to handle different HTTP methods (GET, POST, PUT, DELETE, etc.) in your server. You can inspect the http.Request
object to check the HTTP method and route the request accordingly.
Example: Handling GET and POST Requests
This example demonstrates how to handle both GET and POST requests:
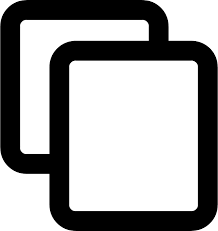
package main
import (
"fmt"
"net/http"
)
func handleGet(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "GET request received")
}
func handlePost(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "POST request received")
}
func main() {
http.HandleFunc("/get", handleGet)
http.HandleFunc("/post", handlePost)
http.ListenAndServe(":8080", nil)
}
Now, the server will respond with "GET request received" for GET requests at /get
and "POST request received" for POST requests at /post
.
5. Sending HTTP Responses
Once your web server handles a request, you can send a response back to the client using the http.ResponseWriter
. You can set status codes, headers, and the body of the response.
Example: Sending a Plain Text Response
Here’s an example of sending a plain text response:
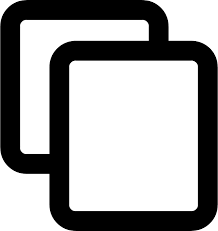
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "text/plain")
fmt.Fprintln(w, "This is a simple response")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example will send "This is a simple response" as plain text when you visit http://localhost:8080/
.
Example: Sending a JSON Response
To send a JSON response, you need to set the response header to application/json
and encode the response into JSON format:
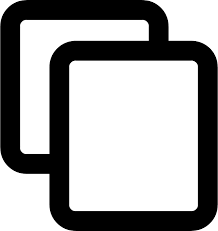
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Response struct {
Message string `json:"message"`
}
func handler(w http.ResponseWriter, r *http.Request) {
response := Response{Message: "Hello, World!"}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(response)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example sends a JSON object with a message to the client when you visit http://localhost:8080/
.
6. Customizing Responses
You can also set the response status code explicitly using w.WriteHeader
. For example, to send a 404 Not Found status code:
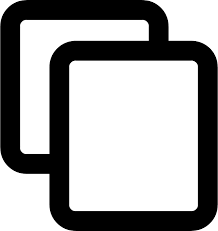
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
w.WriteHeader(http.StatusNotFound)
fmt.Fprintln(w, "Page Not Found")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This example will send a 404 status code along with the message "Page Not Found" when the root URL is visited.
7. Conclusion
Creating a simple web server in Go is straightforward, thanks to the net/http
package. By using http.HandleFunc
to define your handlers and http.ListenAndServe
to start the server, you can quickly set up a server to handle various HTTP requests. With Go’s simplicity and performance, it’s a great choice for creating fast and reliable web servers.
Working with Templates in Go
1. Introduction to Templates in Go
In Go, templates allow you to separate the logic of your program from the presentation of data. The text/template
and html/template
packages provide functionality to execute templates, making it easy to generate dynamic content for web pages or other outputs.
2. Basic Template Syntax
Go templates use a simple syntax with placeholders for dynamic content. Variables are enclosed in {{}}
and can be replaced with actual values during template execution. Here's an example of a basic template:
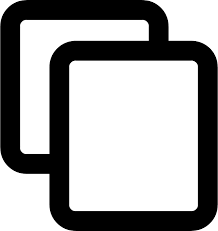
package main
import (
"fmt"
"text/template"
"os"
)
func main() {
tmpl, err := template.New("example").Parse("Hello, {{.}}!")
if err != nil {
fmt.Println("Error parsing template:", err)
return
}
tmpl.Execute(os.Stdout, "World")
}
This example defines a simple template that inserts the value "World" where {{.}}
is placed, resulting in the output "Hello, World!"
3. Using Variables in Templates
You can pass variables to a template using the Execute
method. The data passed can be anything from a simple string to complex structures. Go templates automatically convert Go data types into their string representation for output.
Example: Using Variables
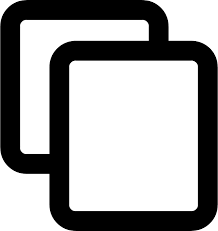
package main
import (
"fmt"
"text/template"
"os"
)
func main() {
tmpl, err := template.New("greeting").Parse("Hello, {{.Name}}!")
if err != nil {
fmt.Println("Error parsing template:", err)
return
}
data := struct {
Name string
}{
Name: "Alice",
}
tmpl.Execute(os.Stdout, data)
}
This example uses a struct to define the data passed to the template. The template prints "Hello, Alice!" when executed.
4. Control Structures in Templates
Go templates support control structures like conditionals and loops for more advanced rendering.
Example: If Statements
You can use {{if}}
to render content based on a condition:
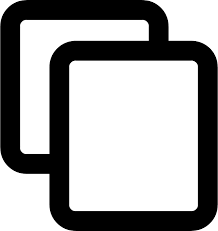
package main
import (
"fmt"
"text/template"
"os"
)
func main() {
tmpl, err := template.New("conditional").Parse(`
{{if .IsAdmin}}
Welcome, Admin!
{{else}}
Welcome, User!
{{end}}
`)
if err != nil {
fmt.Println("Error parsing template:", err)
return
}
data := struct {
IsAdmin bool
}{
IsAdmin: true,
}
tmpl.Execute(os.Stdout, data)
}
If the IsAdmin
field is true, the template outputs "Welcome, Admin!", otherwise it prints "Welcome, User!".
Example: Loops
To iterate over a collection of items, you can use the {{range}}
control structure:
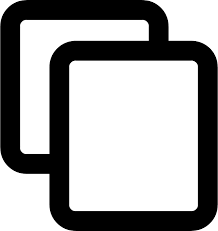
package main
import (
"fmt"
"text/template"
"os"
)
func main() {
tmpl, err := template.New("loop").Parse(`
Items:
{{range .Items}}
- {{.}}
{{end}}
`)
if err != nil {
fmt.Println("Error parsing template:", err)
return
}
data := struct {
Items []string
}{
Items: []string{"Apple", "Banana", "Cherry"},
}
tmpl.Execute(os.Stdout, data)
}
This template will iterate over the Items
slice and print each item with a bullet point.
5. Using HTML Templates
Go provides a special html/template
package that automatically escapes HTML to prevent cross-site scripting (XSS) attacks. This package is ideal for web applications.
Example: Rendering HTML
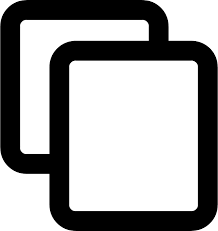
package main
import (
"fmt"
"html/template"
"os"
)
func main() {
tmpl, err := template.New("htmlExample").Parse("{{.}}
")
if err != nil {
fmt.Println("Error parsing template:", err)
return
}
tmpl.Execute(os.Stdout, "Hello, Go!")
}
This example renders an HTML header with the text "Hello, Go!" safely.
6. Template Files
Instead of embedding templates directly into the Go code, you can load templates from external files. Go provides the ParseFiles
and ParseGlob
methods for this.
Example: Loading Template from File
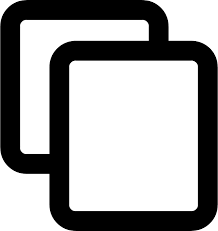
package main
import (
"fmt"
"text/template"
"os"
)
func main() {
tmpl, err := template.ParseFiles("template.txt")
if err != nil {
fmt.Println("Error parsing template file:", err)
return
}
tmpl.Execute(os.Stdout, "Hello from file!")
}
This example loads a template from the file template.txt
and renders it with the data "Hello from file!".
7. Conclusion
Working with templates in Go is a powerful way to generate dynamic content for your applications. Whether you're creating web pages, generating configuration files, or formatting text output, Go templates make it easy to separate your logic from presentation. By using control structures like conditionals and loops, you can create complex templates for your needs, while the html/template
package ensures safe HTML rendering.
Building a RESTful API in Go
1. Introduction to RESTful APIs
A RESTful API (Representational State Transfer) is an architectural style that allows communication between client and server over HTTP. It is commonly used for web applications to enable different systems to interact with each other. In Go, building a RESTful API involves setting up HTTP routes, handling requests, and sending responses in a structured format like JSON.
2. Setting Up the Go Project
Before you start building your API, ensure that Go is installed on your system. You can create a new directory for your project and initialize it as a Go module:
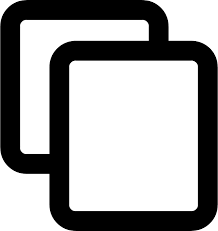
mkdir my-api
cd my-api
go mod init my-api
Next, you can create a Go file, such as main.go
, where the API logic will reside.
3. Creating Basic HTTP Routes
Go provides the net/http
package to create HTTP servers and handle requests. You can define routes to handle GET, POST, PUT, and DELETE requests. Here's a basic example of setting up a simple HTTP server with a GET route:
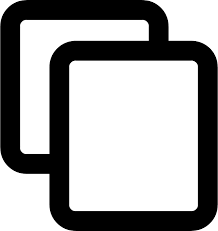
package main
import (
"fmt"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", helloHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
This code sets up a basic server that listens on port 8080. When a GET request is made to the root URL (/
), it responds with "Hello, World!".
4. Handling JSON Requests and Responses
In a RESTful API, data is typically sent and received in JSON format. You can use the encoding/json
package in Go to parse incoming JSON data and send JSON responses. Here's an example of a POST route that accepts JSON data:
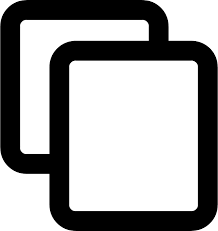
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func createPersonHandler(w http.ResponseWriter, r *http.Request) {
var p Person
err := json.NewDecoder(r.Body).Decode(&p)
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
response := fmt.Sprintf("Received: %s, Age: %d", p.Name, p.Age)
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(map[string]string{"message": response})
}
func main() {
http.HandleFunc("/person", createPersonHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, the createPersonHandler
function reads JSON data from the request body, decodes it into a Person
struct, and sends a response back in JSON format.
5. Structuring the API with Multiple Routes
As your API grows, you may want to structure it into multiple routes and endpoints. This can be done by creating separate handler functions for different routes. Here's an example with multiple routes:
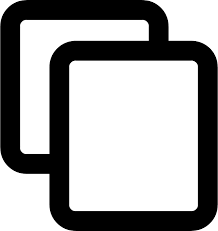
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func getPersonHandler(w http.ResponseWriter, r *http.Request) {
p := Person{Name: "Alice", Age: 30}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(p)
}
func createPersonHandler(w http.ResponseWriter, r *http.Request) {
var p Person
err := json.NewDecoder(r.Body).Decode(&p)
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
response := fmt.Sprintf("Created: %s, Age: %d", p.Name, p.Age)
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(map[string]string{"message": response})
}
func main() {
http.HandleFunc("/person", getPersonHandler)
http.HandleFunc("/create", createPersonHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, two routes are defined: /person
to retrieve a hardcoded person, and /create
to create a new person using a POST request.
6. Error Handling in RESTful API
When building APIs, it's essential to handle errors properly. You should return appropriate HTTP status codes and messages for successful and failed operations. For example:
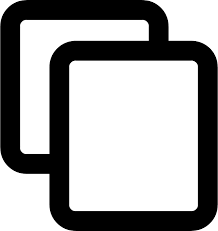
package main
import (
"encoding/json"
"fmt"
"net/http"
)
func errorHandler(w http.ResponseWriter, r *http.Request) {
http.Error(w, "Something went wrong", http.StatusInternalServerError)
}
func main() {
http.HandleFunc("/error", errorHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
This example demonstrates how to use the http.Error
function to send an error message with a 500 Internal Server Error status.
7. Conclusion
Building a RESTful API in Go is straightforward, thanks to the simplicity and flexibility of the net/http
package. By following best practices such as handling JSON data, structuring your API into multiple routes, and managing errors correctly, you can create efficient and maintainable APIs in Go. Whether you're building a simple API or a more complex system, Go provides the tools and functionality to get the job done efficiently.
Handling Form Data in Go
1. Introduction to Form Handling
In web development, forms are used to collect user input, such as text, selections, and file uploads. Go provides the net/http
package, which allows you to handle form submissions by parsing data from HTML forms submitted via the POST method. In this section, we will explore how to handle form data, including both simple form fields and file uploads, in Go.
2. Basic Form Handling
For basic form handling, the most common form fields are input fields, checkboxes, and radio buttons. When a user submits a form, the data is sent to the server, where it can be processed. Here's an example of handling a simple form that collects a user's name and email:
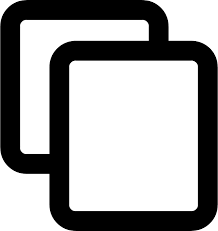
package main
import (
"fmt"
"net/http"
)
func formHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPost {
name := r.FormValue("name")
email := r.FormValue("email")
fmt.Fprintf(w, "Received Name: %s, Email: %s", name, email)
} else {
http.ServeFile(w, r, "form.html")
}
}
func main() {
http.HandleFunc("/form", formHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, the formHandler
function handles the form submission. If the request method is POST
, it retrieves the form values using r.FormValue()
and prints them to the response. If the method is not POST (such as when the form is first loaded), it serves the form HTML file to the user.
3. HTML Form Example
Here is the HTML form that goes with the Go handler above:
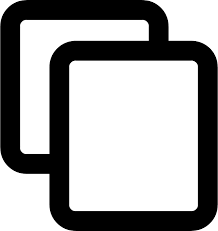
<html>
<body>
<form method="POST" action="/form">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
This form sends a POST request to the server at the /form
route with the user's name and email as form data.
4. Handling Multipart Form Data (File Uploads)
In addition to simple form data, Go can also handle multipart form data, which is used when uploading files. Here's an example of handling a file upload:
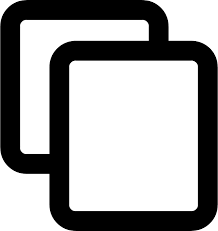
package main
import (
"fmt"
"net/http"
"os"
)
func uploadHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPost {
err := r.ParseMultipartForm(10 << 20) // Limit file size to 10MB
if err != nil {
fmt.Fprintf(w, "Error parsing form: %s", err)
return
}
file, _, err := r.FormFile("file")
if err != nil {
fmt.Fprintf(w, "Error retrieving file: %s", err)
return
}
defer file.Close()
outFile, err := os.Create("uploaded_file")
if err != nil {
fmt.Fprintf(w, "Error creating file: %s", err)
return
}
defer outFile.Close()
_, err = file.Copy(outFile)
if err != nil {
fmt.Fprintf(w, "Error saving file: %s", err)
return
}
fmt.Fprintf(w, "File uploaded successfully!")
} else {
http.ServeFile(w, r, "upload_form.html")
}
}
func main() {
http.HandleFunc("/upload", uploadHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, the uploadHandler
function handles file uploads. It uses r.ParseMultipartForm()
to parse the form data, which includes the uploaded file. The file is retrieved using r.FormFile()
, and it is saved to the server using os.Create()
.
5. HTML Form Example for File Upload
Here is the HTML form for the file upload example:
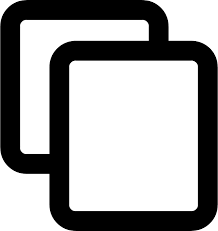
<html>
<body>
<form method="POST" action="/upload" enctype="multipart/form-data">
<label for="file">Choose a file:</label>
<input type="file" id="file" name="file"><br><br>
<input type="submit" value="Upload">
</form>
</body>
</html>
This form includes an enctype="multipart/form-data"
attribute, which is necessary for uploading files. The file is sent as part of the form data when the form is submitted via POST.
6. Validating Form Data
When handling form data, it's important to validate the input to ensure it meets the expected format and requirements. For example, if you expect a valid email address, you can use regular expressions to check its format:
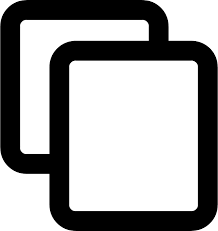
package main
import (
"fmt"
"net/http"
"regexp"
)
func formHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPost {
email := r.FormValue("email")
validEmailRegex := `^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$`
match, _ := regexp.MatchString(validEmailRegex, email)
if !match {
http.Error(w, "Invalid email format", http.StatusBadRequest)
return
}
fmt.Fprintf(w, "Received valid email: %s", email)
} else {
http.ServeFile(w, r, "form.html")
}
}
func main() {
http.HandleFunc("/form", formHandler)
fmt.Println("Server is running on port 8080...")
http.ListenAndServe(":8080", nil)
}
This example validates the email field using a regular expression. If the email format is invalid, it returns a 400 Bad Request error with an appropriate message.
7. Conclusion
Handling form data in Go is straightforward with the net/http
package. By using r.FormValue()
for simple inputs and r.FormFile()
for file uploads, you can efficiently process form submissions. Additionally, validating form data and handling errors ensures that your application receives and processes input correctly. Whether you're handling basic form fields or complex file uploads, Go provides the tools to build robust web applications.
Writing Unit Tests in Go
1. Introduction to Unit Testing
Unit testing is a crucial part of software development that ensures your code behaves as expected. In Go, unit tests are written using the testing
package, which provides a framework to write test functions and run them automatically. Unit tests help catch bugs early, improve code maintainability, and provide documentation for how the code should behave.
2. Writing a Basic Test Function
To write a unit test in Go, you need to create a function that starts with the word Test
and takes a pointer to testing.T
as an argument. Here's an example of a simple test function that checks if the sum of two numbers is correct:
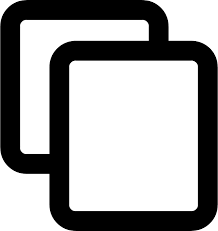
package main
import "testing"
// Function to add two numbers
func Add(a, b int) int {
return a + b
}
// Test function for Add()
func TestAdd(t *testing.T) {
got := Add(2, 3)
want := 5
if got != want {
t.Errorf("Add(2, 3) = %d; want %d", got, want)
}
}
In this example, the TestAdd
function checks if the Add
function correctly sums 2 and 3. The t.Errorf()
method is used to report an error if the result does not match the expected value.
3. Running Unit Tests
Once you've written your test functions, you can run them using the go test
command. The Go testing tool will automatically find all test functions in the current package and execute them.
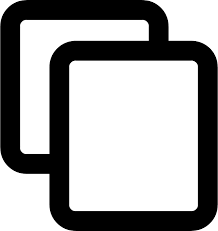
go test
If the tests pass, you will see output like:
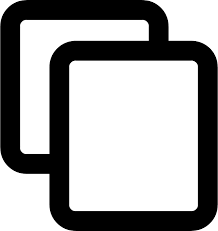
PASS
ok ./ 0.003s
If a test fails, you'll see a message detailing which test failed and why.
4. Table-Driven Tests
In Go, a common pattern for writing multiple tests for a single function is the table-driven test. This allows you to write several test cases in a single test function by iterating over a table of test inputs and expected outputs. Here's an example of using table-driven tests for the Add
function:
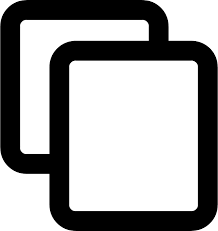
package main
import "testing"
func TestAdd(t *testing.T) {
tests := []struct {
a, b, want int
}{
{2, 3, 5},
{1, 1, 2},
{0, 0, 0},
{-1, -1, -2},
{-2, 3, 1},
}
for _, tt := range tests {
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
got := Add(tt.a, tt.b)
if got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
In this example, we define a slice of test cases where each test case is a struct with inputs a
and b
, and the expected result want
. We then loop over these test cases and run each one using t.Run()
, which allows individual reporting for each test case.
5. Testing Edge Cases
When writing tests, it is important to cover edge cases, such as handling empty inputs, negative numbers, or large values. For example, testing the behavior of the Add
function with negative numbers or zero can help ensure that the function works correctly in all situations.
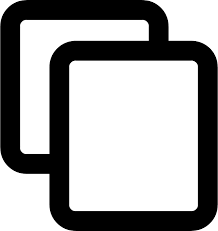
func TestAddWithEdgeCases(t *testing.T) {
tests := []struct {
a, b, want int
}{
{0, 0, 0}, // Edge case with zero
{-1, -1, -2}, // Edge case with negative numbers
{1000, 5000, 6000}, // Edge case with large numbers
}
for _, tt := range tests {
got := Add(tt.a, tt.b)
if got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
}
}
Testing edge cases ensures that your function can handle a wide range of possible inputs, making your code more robust.
6. Mocking Dependencies in Tests
In some cases, your functions may depend on external resources, such as databases or APIs. In such cases, you might want to mock those dependencies to isolate the unit being tested. While Go does not have a built-in mocking framework, you can use interfaces and create mock implementations for testing purposes.
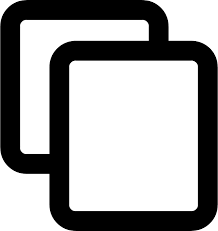
package main
import (
"testing"
)
// Database interface
type Database interface {
GetData(id int) string
}
// Function that depends on Database
func FetchData(db Database, id int) string {
return db.GetData(id)
}
// Mock implementation of Database
type MockDatabase struct{}
func (m *MockDatabase) GetData(id int) string {
if id == 1 {
return "Mock Data"
}
return "Unknown"
}
func TestFetchData(t *testing.T) {
mockDB := &MockDatabase{}
got := FetchData(mockDB, 1)
want := "Mock Data"
if got != want {
t.Errorf("FetchData() = %s; want %s", got, want)
}
}
In this example, we define a Database
interface and a mock implementation, MockDatabase
, which returns predefined values. The FetchData
function is then tested with the mock implementation instead of a real database.
7. Test Coverage
Test coverage is a measure of how much of your code is tested by your unit tests. Go provides a way to check test coverage using the go test -cover
command. This command shows the percentage of your code that is covered by tests.
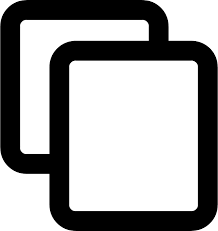
go test -cover
If you want to see a more detailed report of coverage for each function, you can use the go test -coverprofile
option to generate a coverage profile and analyze it with tools like go tool cover
.
8. Conclusion
Writing unit tests in Go is simple and effective thanks to the built-in testing
package. By writing tests for your functions, you can ensure that your code behaves as expected and prevent bugs from appearing in production. Using table-driven tests, mocking dependencies, and checking test coverage are important techniques that help improve the quality and reliability of your code.
Using the Testing Package in Go
1. Introduction to the Testing Package
The testing
package in Go provides the necessary tools for writing unit tests. It allows you to define test functions, check for expected values, and report errors when tests fail. Unit testing helps ensure that your code behaves as expected and aids in identifying bugs early in the development process.
2. Writing Test Functions
To write a test function in Go, the function must start with the prefix Test
and accept a pointer to a testing.T
type as its argument. The testing.T
type provides methods to report errors and failures during tests.
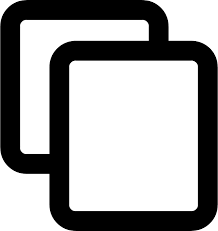
package main
import "testing"
// Function to be tested
func Add(a, b int) int {
return a + b
}
// Test function
func TestAdd(t *testing.T) {
got := Add(2, 3)
want := 5
if got != want {
t.Errorf("Add(2, 3) = %d; want %d", got, want)
}
}
In this example, the TestAdd
function tests the Add
function. If the result of Add(2, 3)
does not match the expected result of 5
, the test will fail and output an error message using t.Errorf
.
3. Running Tests
Once you've written your test functions, you can run them using the go test
command. The Go testing tool will automatically detect and run all functions that begin with Test
in the current package.
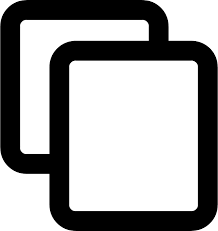
go test
If all tests pass, you will see a message indicating that the tests were successful:
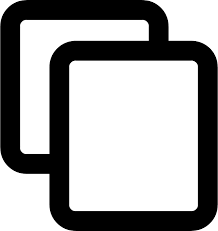
PASS
ok ./ 0.001s
If a test fails, the output will include details about the failure:
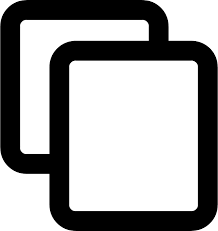
--- FAIL: TestAdd (0.00s)
main_test.go:10: Add(2, 3) = 6; want 5
FAIL
exit status 1
FAIL ./ 0.002s
4. Testing Multiple Cases with Table-Driven Tests
Table-driven tests are a popular pattern in Go for testing multiple cases within a single function. This is done by defining a table of test cases (often a slice of structs) and iterating over them to execute each test case in turn.
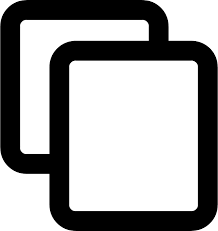
package main
import "testing"
func TestAdd(t *testing.T) {
tests := []struct {
a, b, want int
}{
{2, 3, 5},
{1, 1, 2},
{0, 0, 0},
{-1, -1, -2},
{-2, 3, 1},
}
for _, tt := range tests {
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
got := Add(tt.a, tt.b)
if got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
In the above example, we define a slice of test cases, and then run each test case using t.Run
. The benefit of this approach is that it provides clear and structured reporting for each individual test case.
5. Using Subtests with t.Run()
Subtests are useful when you want to group multiple assertions within a single test function. The t.Run
method allows you to define subtests, which are run separately but report results as part of the parent test.
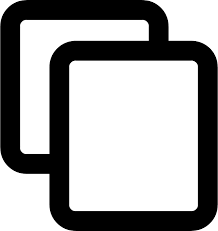
package main
import "testing"
func TestAdd(t *testing.T) {
tests := []struct {
a, b, want int
}{
{2, 3, 5},
{1, 1, 2},
{0, 0, 0},
}
for _, tt := range tests {
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
got := Add(tt.a, tt.b)
if got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
Here, t.Run
is used to create subtests for each test case, which makes the output more organized and easier to read when multiple cases are tested within a single test function.
6. Benchmarking in Go Tests
Go also supports benchmarking, allowing you to measure the performance of a function. Benchmark functions are similar to test functions but have the prefix Benchmark
and take a pointer to testing.B
as an argument.
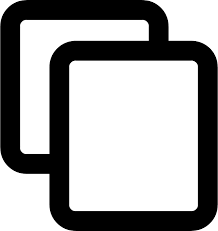
package main
import "testing"
// Benchmark function for Add
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(2, 3)
}
}
In this example, the BenchmarkAdd
function benchmarks the Add
function by calling it b.N
times. The b.N
value is automatically adjusted by the testing framework to run the benchmark multiple times to get a more accurate result. To run benchmarks, use the go test -bench
command:
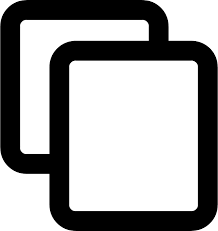
go test -bench .
7. Test Coverage in Go
Test coverage is a measure of how much of your code is tested by your unit tests. Go provides an option to check the test coverage of your code using the go test -cover
command. This command will show the percentage of your code that is covered by tests.
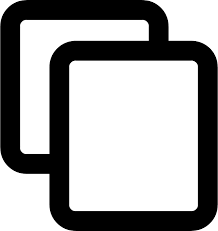
go test -cover
If you want more detailed coverage information, you can generate a coverage profile with the following command:
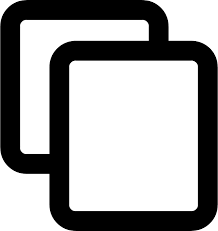
go test -coverprofile=coverage.out
Then, you can visualize the results with:
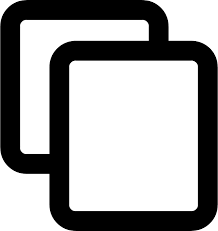
go tool cover -html=coverage.out
8. Handling Errors in Tests
When writing tests, it's important to handle errors correctly. If an error occurs during the test, you should use t.Errorf
or t.Fatal
to report the error. The difference is that t.Fatal
will stop the test immediately, whereas t.Errorf
allows the test to continue running.
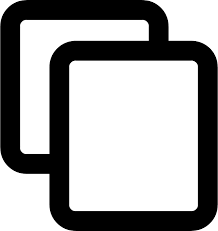
package main
import "testing"
func TestAddWithError(t *testing.T) {
got := Add(2, 3)
want := 6
if got != want {
t.Errorf("Add(2, 3) = %d; want %d", got, want)
}
}
If the test fails, the output will show an error message indicating the failure, and the test will continue running.
9. Conclusion
The testing
package in Go is a powerful tool for writing unit tests, benchmarking, and measuring test coverage. By using the various features of the package, such as subtests, table-driven tests, and benchmarks, you can write comprehensive and efficient tests for your code, helping you to maintain high-quality, bug-free software.
Table-Driven Tests in Go
1. Introduction to Table-Driven Tests
Table-driven tests are a popular pattern in Go for testing multiple cases within a single function. This approach improves code readability and maintainability by organizing test cases in a structured manner. Instead of writing separate assertions for each test case, you define a table (usually a slice of structs) and iterate over it to run all test cases in a loop.
2. Why Use Table-Driven Tests?
Table-driven tests provide several benefits:
- They make it easy to test multiple scenarios with minimal duplication.
- They improve test clarity by grouping related tests together.
- They reduce the chance of errors by centralizing test case data in one place.
3. Writing a Simple Table-Driven Test
To implement a table-driven test, define a slice of test cases (usually structs with input and expected output), and then loop through the table to execute each test case. In the loop, use t.Run
to run subtests for each case to get more detailed test output.
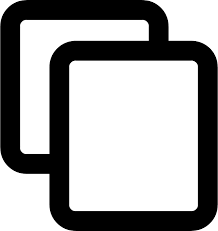
package main
import "testing"
// Function to be tested
func Add(a, b int) int {
return a + b
}
// Table-Driven Test Function
func TestAdd(t *testing.T) {
tests := []struct {
a, b, want int
}{
{2, 3, 5},
{1, 1, 2},
{0, 0, 0},
{-1, -1, -2},
{-2, 3, 1},
}
// Iterating over the test cases
for _, tt := range tests {
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
got := Add(tt.a, tt.b)
if got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
In the example above, the TestAdd
function is a table-driven test that tests the Add
function for multiple input combinations. The test cases are grouped in a slice of structs, and each case is executed by iterating over the slice using a loop. The results are presented clearly, and any mismatches between the actual and expected values are reported using t.Errorf
.
4. Using Subtests with t.Run()
Each iteration of the table-driven test runs a subtest using t.Run
. This allows you to isolate each test case within its own subtest, making it easier to identify which specific case has failed.
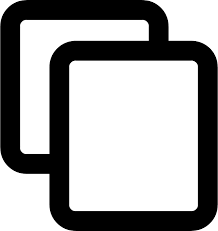
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
// Test logic here
})
By using t.Run
, you get detailed output for each test case, and Go handles the test results for each subtest separately. This is particularly useful when you have multiple test cases with varying inputs and expected outputs.
5. Example with More Complex Test Cases
Table-driven tests are not limited to simple functions. You can use this pattern to test more complex functions, including those that involve error handling or multiple return values. Here's an example of testing a function that handles multiple error scenarios:
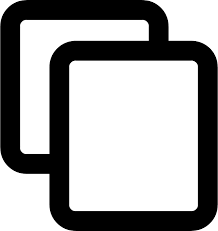
package main
import (
"errors"
"testing"
)
// Function to be tested
func Divide(a, b int) (int, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
// Table-Driven Test Function
func TestDivide(t *testing.T) {
tests := []struct {
a, b int
want int
wantErr bool
}{
{6, 3, 2, false},
{6, 0, 0, true},
{10, 2, 5, false},
{5, 2, 2, false},
}
for _, tt := range tests {
t.Run(fmt.Sprintf("%d/%d", tt.a, tt.b), func(t *testing.T) {
got, err := Divide(tt.a, tt.b)
if (err != nil) != tt.wantErr {
t.Errorf("Divide(%d, %d) error = %v, wantErr %v", tt.a, tt.b, err, tt.wantErr)
return
}
if got != tt.want {
t.Errorf("Divide(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
In this example, the TestDivide
function tests the Divide
function with multiple cases, including a division by zero. The test checks both the returned value and whether the error occurred as expected, demonstrating how table-driven tests can handle more complex logic.
6. Benefits of Table-Driven Tests
Using table-driven tests in Go provides several advantages:
- Scalability: New test cases can be easily added by extending the test table with new rows.
- Readability: Grouping similar tests together in a table structure makes the tests easier to understand and maintain.
- Reduced Code Duplication: You can test multiple cases with minimal code duplication, leading to cleaner and more concise test files.
7. Handling Edge Cases with Table-Driven Tests
Table-driven tests are well-suited for testing edge cases, such as boundary conditions, errors, and unexpected inputs. For example, you can test a function with a large number, zero, or negative values without writing separate test functions for each case.
8. Conclusion
Table-driven tests are a powerful and flexible pattern in Go that help you write clean, readable, and maintainable tests. By defining test cases in a table and iterating over them, you can test a wide variety of scenarios with minimal code duplication. This approach is highly recommended for testing functions with multiple input combinations or complex logic.
Benchmarking Code Performance in Go
1. Introduction to Benchmarking
Benchmarking is the practice of measuring the performance of your code, such as execution time, memory usage, and other resource consumption. In Go, benchmarking is an essential tool for identifying bottlenecks and optimizing code. Go's testing framework includes built-in support for benchmarking, allowing you to measure the performance of specific functions or code blocks.
2. The Importance of Benchmarking
Benchmarking helps developers ensure that their code meets performance requirements. By measuring the time taken by specific functions or algorithms, you can:
- Identify performance bottlenecks in your code.
- Compare the impact of code changes on performance.
- Optimize critical paths and reduce latency.
- Make informed decisions about algorithms and data structures.
3. Writing a Benchmark Test in Go
To write a benchmark test in Go, you can use the testing.B
type, which is available in the testing
package. Benchmark functions must start with the prefix Benchmark
and take a pointer to testing.B
as an argument. Inside the benchmark function, use b.N
to run the test multiple times and get accurate results.
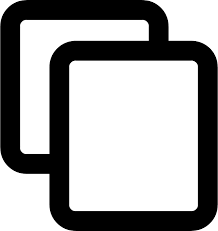
package main
import (
"testing"
)
// Function to be benchmarked
func Add(a, b int) int {
return a + b
}
// Benchmark Function
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(2, 3)
}
}
In the example above, the BenchmarkAdd
function benchmarks the Add
function by calling it b.N
times. The b.N
value is automatically adjusted by Go's testing framework to ensure that the benchmark runs a sufficient number of times for accurate measurement.
4. Running Benchmark Tests
To run a benchmark test, use the following command in your terminal:
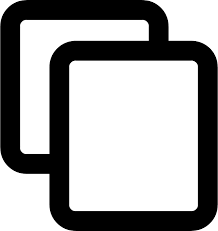
go test -bench .
This command runs all benchmarks in the current package. You can also specify a specific benchmark function to run by using:
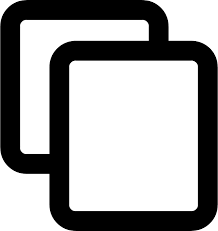
go test -bench BenchmarkAdd
By default, Go runs the benchmark multiple times and reports the results, including the average time taken for each operation. The results are displayed in nanoseconds per operation, providing insight into the performance of your code.
5. Example Benchmarking a Sorting Function
Let's benchmark a sorting function to see how Go handles performance measurement. In this example, we'll benchmark the sort.Sort
function:
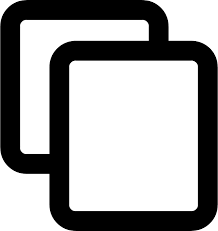
package main
import (
"sort"
"testing"
)
// Benchmark Sorting Function
func BenchmarkSort(b *testing.B) {
data := []int{5, 3, 8, 6, 2, 7, 1, 4}
b.ResetTimer() // Reset the timer to exclude setup time
for i := 0; i < b.N; i++ {
sort.Sort(sort.IntSlice(data))
}
}
In this benchmark, we sort an array of integers multiple times. The b.ResetTimer()
function is used to reset the timer before the loop to exclude the setup time (such as creating the array) from the benchmark results.
6. Understanding Benchmark Output
When running benchmarks, Go provides output that includes:
- Benchmark name: The name of the benchmark function being tested.
- Number of iterations: The number of times the benchmark was executed (controlled by
b.N
). - Duration: The time taken to execute the benchmark, displayed in nanoseconds per operation.
Example output:
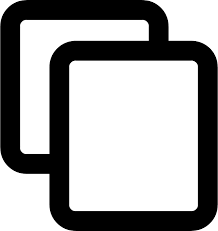
BenchmarkAdd-8 50000000 3.2 ns/op
In this example, the benchmark ran 50 million times, and each iteration took 3.2 nanoseconds to complete.
7. Comparing Benchmark Results
You can compare benchmark results over time to assess performance improvements or regressions. For example, you can measure the performance of an algorithm before and after making optimizations and compare the results to see if the changes resulted in better performance.
To ensure consistency, you should run benchmarks multiple times and analyze the average results. Go automatically handles multiple iterations and calculates the average time for each benchmark.
8. Benchmarking Memory Allocations
In addition to measuring execution time, Go also allows you to benchmark memory allocations using the b.ReportAllocs()
method. This is useful for identifying functions that allocate memory excessively, leading to memory pressure and performance degradation.
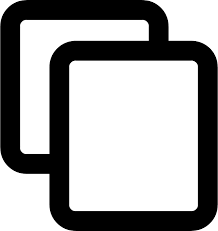
func BenchmarkAddWithAlloc(b *testing.B) {
b.ReportAllocs()
for i := 0; i < b.N; i++ {
_ = Add(2, 3)
}
}
When running this benchmark, Go will report the number of allocations and the average memory usage per operation, allowing you to track the memory efficiency of your code.
9. Best Practices for Benchmarking
To ensure accurate and meaningful benchmark results, consider the following best practices:
- Minimize setup time: Use
b.ResetTimer()
to avoid including setup time in your benchmark results. - Run benchmarks multiple times: Run your benchmarks several times to account for variability and get more reliable results.
- Test in a consistent environment: Ensure that your benchmarks run in a stable environment, with minimal system load and interference from other processes.
- Use real-world data: Benchmark with data that closely resembles the input your code will handle in production for more realistic results.
10. Conclusion
Benchmarking is an essential tool for optimizing code performance in Go. By using Go's built-in benchmarking framework, you can measure the execution time, memory usage, and other performance metrics of your code. This allows you to identify bottlenecks, make informed decisions about optimizations, and ensure that your code performs as expected under different conditions.
Code Coverage in Go
1. Introduction to Code Coverage
Code coverage is a measure of how much of your source code is executed during testing. It helps you identify untested parts of your codebase and ensures that your tests cover a significant portion of your application. In Go, code coverage can be easily measured using the built-in testing framework, which provides tools to generate coverage reports and analyze your test suite’s effectiveness.
2. The Importance of Code Coverage
Having high code coverage means that a large portion of your code is being tested, reducing the likelihood of bugs and regressions in your application. Code coverage helps:
- Ensure that your tests cover critical paths and edge cases.
- Identify code that is not tested, which could potentially lead to bugs.
- Improve the reliability and maintainability of your codebase.
3. Enabling Code Coverage in Go Tests
In Go, you can enable code coverage when running tests using the go test
command with the -cover
flag. This generates coverage information that you can analyze further. Here's an example of how to run tests with code coverage enabled:
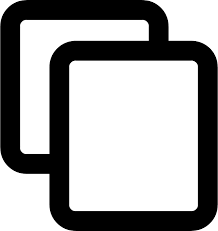
go test -cover
This will run all the tests in the current package and generate a coverage report showing the percentage of code covered by tests.
4. Interpreting the Code Coverage Output
The output of the go test -cover
command shows the percentage of code covered by your tests:
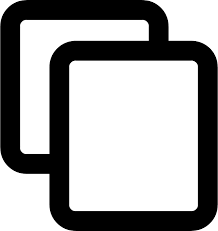
PASS
coverage: 80.0% of statements
ok ./mypackage 0.012s
In this example, 80% of the code in the package is covered by tests. The output also indicates whether the tests passed or failed and how long the tests took to run.
5. Viewing Detailed Coverage Information
For more detailed coverage information, you can generate an HTML report. This can be done with the following command:
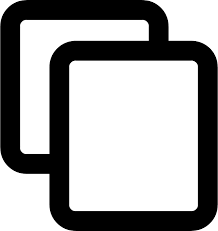
go test -coverprofile=coverage.out
go tool cover -html=coverage.out
The first command generates a coverage profile file (coverage.out
), and the second command generates an HTML report that allows you to see which lines of code were executed during tests. The HTML report highlights covered lines in green and uncovered lines in red, making it easy to spot untested code.
6. Example of Generating Coverage Reports
To see the coverage report in your browser, run:
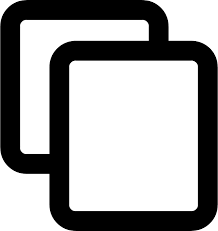
go test -coverprofile=coverage.out
go tool cover -html=coverage.out
This will open the coverage report in your default web browser, displaying detailed coverage for each file in your package.
7. Code Coverage for Specific Tests
You can also run code coverage for specific tests or benchmarks using the -run
flag to filter which tests are run. For example:
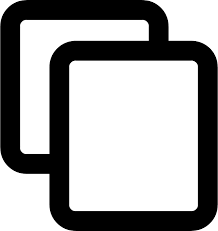
go test -cover -run TestFunctionName
This command runs only the tests that match the name of the function TestFunctionName
and generates a coverage report for those tests.
8. Code Coverage and Continuous Integration
Code coverage is especially useful in continuous integration (CI) environments. By integrating code coverage into your CI pipeline, you can ensure that new changes do not reduce the amount of code covered by tests. Many CI tools, such as Jenkins and GitHub Actions, support running tests with code coverage and reporting the results as part of the build process.
9. Code Coverage and Test Quality
While high code coverage is important, it does not guarantee that your tests are of high quality. Code coverage only measures whether code is executed during tests, not whether the tests validate the correctness of the code. It's essential to write meaningful tests that verify the behavior of your application. Striving for 100% coverage is not always necessary, but you should focus on covering critical paths and edge cases.
10. Excluding Code from Coverage
There are situations where you may want to exclude certain code from the coverage report, such as test-specific code or unreachable code. You can use the // coverage:ignore
directive in your Go code to exclude specific functions or lines from coverage measurement:
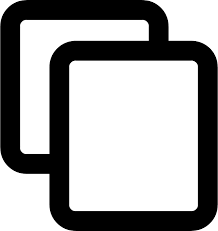
// coverage:ignore
func SomeFunctionThatShouldNotBeCovered() {
// This code will be excluded from coverage reports
}
This directive ensures that the specified code is not counted towards the overall coverage percentage.
11. Tools for Visualizing Code Coverage
In addition to the built-in tools provided by Go, there are third-party tools available for visualizing and reporting on code coverage in Go projects:
- Codecov: A popular tool for visualizing and reporting code coverage on GitHub repositories. It integrates easily with CI tools and provides detailed reports.
- Coveralls: Another popular service for tracking and reporting code coverage in your GitHub projects. It provides visual coverage reports and integrates with various CI systems.
12. Best Practices for Code Coverage
To make the most of code coverage in Go, follow these best practices:
- Prioritize critical code: Focus your tests on the most important functions and code paths in your application.
- Avoid testing trivial code: Do not spend time writing tests for trivial functions like getters and setters unless they involve critical logic.
- Write meaningful tests: Ensure that your tests validate the correctness of your code, not just that it executes.
- Monitor code coverage over time: Track code coverage trends in your CI pipeline to ensure that coverage is maintained or improved as your codebase evolves.
13. Conclusion
Code coverage is a valuable tool for ensuring the quality and reliability of your Go applications. By using Go's built-in coverage tools and integrating them into your development process, you can identify untested code, improve test effectiveness, and ensure that your application behaves as expected. However, it's essential to remember that code coverage is just one part of the testing process, and writing meaningful tests should always be the primary goal.
Mocking Dependencies in Tests
1. Introduction to Mocking in Tests
Mocking is a technique used in unit testing to simulate the behavior of complex dependencies within a system. By replacing real dependencies with mock objects, you can isolate the code being tested and focus on verifying its functionality. This is especially useful when testing code that interacts with external services, databases, or other components that are difficult or time-consuming to test directly.
2. Why Mocking is Useful
Mocking helps in several scenarios:
- Isolation: Ensure that tests focus only on the code under test, not on external dependencies.
- Control: Mimic different behaviors of dependencies, such as returning specific values or simulating errors.
- Speed: Mocking external services or databases can speed up tests by avoiding slow or costly operations.
- Reliability: Mocking removes dependencies on external systems, ensuring tests are consistent and repeatable.
3. Mocking in Go
In Go, mocking can be achieved using libraries like golang/mock or testify which provide tools for creating mock objects and verifying interactions in tests.
4. Creating Mocks with golang/mock
To create mocks using the golang/mock library, you first need to install the library and generate mock code for the interfaces you want to mock. Below are the steps:
4.1 Installing golang/mock
Use the following command to install golang/mock
:
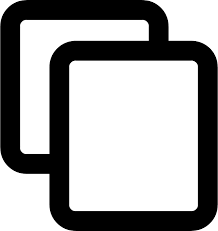
go get github.com/golang/mock/gomock
4.2 Generating Mocks
Once you have installed gomock
, you can generate mocks for your interfaces using the mockgen
tool. For example, if you have an interface Database
in a file database.go
, you can generate a mock like this:
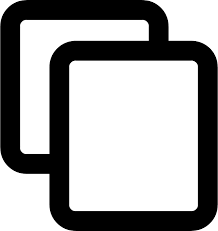
mockgen -source=database.go -destination=mock_database.go -package=mock
This command generates a mock implementation of the Database
interface in the mock_database.go
file in the mock
package.
5. Writing Tests with Mocks
Once you have generated your mocks, you can use them in your tests to simulate interactions with dependencies. Here’s an example of how to write a unit test using mocks:
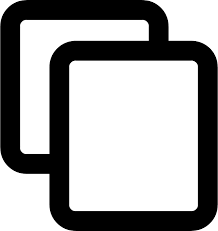
package main
import (
"testing"
"github.com/golang/mock/gomock"
"github.com/stretchr/testify/assert"
)
func TestDatabaseConnection(t *testing.T) {
// Create a mock controller
ctrl := gomock.NewController(t)
defer ctrl.Finish()
// Create a mock object for the Database interface
mockDB := NewMockDatabase(ctrl)
// Define the expected behavior of the mock
mockDB.EXPECT().Connect().Return(nil).Times(1)
// Call the function under test
err := mockDB.Connect()
// Assert the result
assert.Nil(t, err)
}
In this example, we use the mock to simulate a successful connection to the database. The EXPECT
method defines the behavior of the mock (in this case, returning nil
when the Connect
method is called), and the test then verifies that the connection function behaves as expected.
6. Mocking External HTTP Requests
When testing code that makes HTTP requests, you can mock the HTTP client to avoid actually making network calls. You can mock the http.Client
to simulate various responses. Here’s an example:
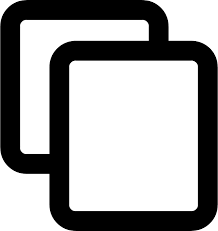
package main
import (
"testing"
"net/http"
"github.com/stretchr/testify/mock"
)
// Mock HTTP client
type MockHTTPClient struct {
mock.Mock
}
func (m *MockHTTPClient) Do(req *http.Request) (*http.Response, error) {
args := m.Called(req)
return args.Get(0).(*http.Response), args.Error(1)
}
func TestHTTPRequest(t *testing.T) {
mockClient := new(MockHTTPClient)
// Define mock response
mockResp := &http.Response{
StatusCode: 200,
}
mockClient.On("Do", mock.Anything).Return(mockResp, nil)
// Call the function under test
resp, err := mockClient.Do(&http.Request{})
// Assert the response and error
assert.NoError(t, err)
assert.Equal(t, 200, resp.StatusCode)
}
In this example, the MockHTTPClient
is used to mock the Do
method, which simulates the behavior of making an HTTP request. The mock returns a predefined response with a status code of 200
.
7. Mocking Databases
When writing tests for database interactions, you can mock the database client to avoid hitting a real database. Below is an example of mocking a database call:
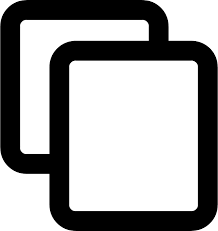
package main
import (
"testing"
"github.com/stretchr/testify/mock"
)
// Mock database client
type MockDatabaseClient struct {
mock.Mock
}
func (m *MockDatabaseClient) GetUser(id int) (User, error) {
args := m.Called(id)
return args.Get(0).(User), args.Error(1)
}
func TestGetUser(t *testing.T) {
mockDB := new(MockDatabaseClient)
// Define mock behavior
mockDB.On("GetUser", 1).Return(User{Name: "John Doe"}, nil)
// Call the function under test
user, err := mockDB.GetUser(1)
// Assert the result
assert.NoError(t, err)
assert.Equal(t, "John Doe", user.Name)
}
In this example, the mock database client is used to simulate a database call for fetching a user. The On
method is used to define the expected parameters and the return values of the GetUser
method.
8. Verifying Mock Interactions
After a test is run, you can verify that the expected interactions with the mocks occurred using the AssertExpectations
method:
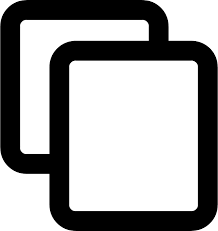
mockDB.AssertExpectations(t)
This will check that all expected calls were made to the mock objects and that they were called with the correct arguments. If any expectations were not met, the test will fail.
9. Best Practices for Mocking
Here are some best practices for mocking dependencies in your tests:
- Mock only dependencies: Avoid mocking the code you are testing. Focus on mocking only the external dependencies, such as APIs or databases.
- Keep mocks simple: Only mock the methods or behavior that are necessary for the test.
- Verify mock interactions: Always verify that the expected interactions with the mock objects occurred.
- Avoid overusing mocks: Use mocks where appropriate, but try to avoid excessive mocking, which could make tests harder to understand.
10. Conclusion
Mocking dependencies in tests allows you to isolate the code under test, making it easier to verify its behavior without relying on external systems. Libraries like golang/mock
and testify
provide powerful tools for mocking, verifying interactions, and controlling the behavior of dependencies in Go. By following best practices and using mocks effectively, you can improve the reliability and maintainability of your tests.
Introduction to Generics in Go
1. What Are Generics?
Generics in Go allow you to write functions, data structures, and algorithms that can operate on different types without sacrificing type safety. Before generics, Go developers often had to use interfaces and type assertions to handle multiple data types in a flexible way. With the introduction of generics in Go 1.18, you can now write code that works with any type while maintaining type safety and performance.
2. Why Generics Are Useful
Generics make it easier to write reusable and type-safe code that can handle a variety of types. This improves code flexibility and reduces redundancy. Some benefits of generics include:
- Code Reusability: Write functions and data structures that work with multiple types without duplicating code.
- Type Safety: Generics ensure type safety by enforcing that the correct types are used at compile time.
- Performance: Generics allow you to write flexible code without the runtime overhead of using interfaces and type assertions.
3. How Generics Work in Go
Generics in Go are implemented using type parameters. These parameters are placeholders for types and are defined in square brackets. The type parameter is used in place of an actual type and can be replaced with any valid type when the function or data structure is used.
4. Syntax for Generics in Go
The general syntax for defining a generic function or type is as follows:
4.1 Generic Functions
A generic function is a function that accepts type parameters. The type parameters are defined within square brackets before the function signature:
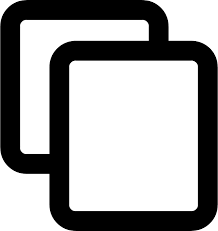
func FunctionName[T any](param T) T { ... }
In this example, T
is the type parameter, and any
is used as a constraint that allows any type.
4.2 Generic Types
A generic type is a type that can accept type parameters. For example:
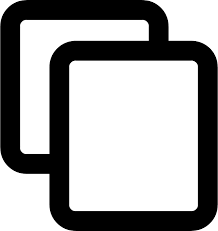
type MyType[T any] struct { value T }
Here, MyType
is a generic type that accepts a type parameter T
and stores a value of that type.
5. Working with Generics in Go
Let's look at some examples of using generics in Go.
5.1 Generic Function Example
Here's a simple example of a generic function that swaps the values of two variables:
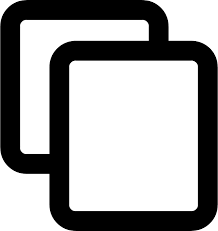
package main
import "fmt"
func Swap[T any](a, b T) (T, T) {
return b, a
}
func main() {
x, y := 1, 2
x, y = Swap(x, y)
fmt.Println(x, y) // Output: 2 1
}
In this example, Swap
is a generic function that swaps the values of a
and b
, and T
can be any type.
5.2 Generic Type Example
Next, let's see how to define and use a generic type. Here's an example of a generic stack data structure:
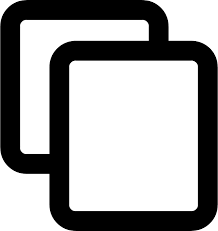
package main
import "fmt"
type Stack[T any] struct {
items []T
}
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
func (s *Stack[T]) Pop() T {
if len(s.items) == 0 {
var zero T
return zero
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item
}
func main() {
stack := Stack[int]{}
stack.Push(10)
stack.Push(20)
fmt.Println(stack.Pop()) // Output: 20
fmt.Println(stack.Pop()) // Output: 10
}
In this example, Stack
is a generic type that can hold items of any type. The Push
and Pop
methods operate on items of type T
.
6. Constraints on Type Parameters
Go allows you to specify constraints on type parameters. This restricts the types that can be used with a generic function or type. The constraint can be a specific type or an interface.
6.1 Using the any
Constraint
The any
keyword is used to specify that the type parameter can be any type:
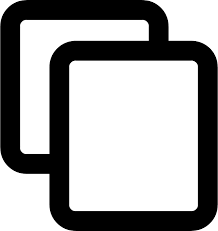
func Print[T any](value T) { fmt.Println(value) }
6.2 Using Custom Constraints
You can also define custom constraints using interfaces:
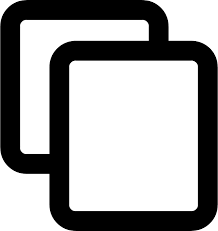
type Number interface {
int | float64
}
func Add[T Number](a, b T) T {
return a + b
}
In this example, the Number
interface is defined as a constraint, restricting T
to be either int
or float64
.
7. Type Inference and Generics
When calling a generic function, Go can automatically infer the type of the type parameter based on the arguments passed to the function. You don't need to explicitly specify the type parameter if the compiler can infer it:
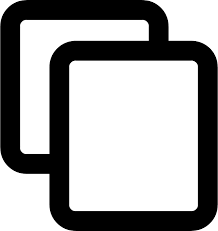
result := Swap(1, 2)
In this case, Go will infer that T
is of type int
based on the values passed to the Swap
function.
8. Limitations of Generics in Go
While generics provide a lot of flexibility, there are some limitations to keep in mind:
- No Type Reflection: Go does not support full type reflection with generics. You cannot directly inspect the type of a type parameter at runtime.
- Complexity: Generics can introduce complexity to your code, especially when combined with constraints and type inference.
- Compatibility: Generics are only available in Go 1.18 and later, so older versions of Go will not support them.
9. Conclusion
Generics in Go provide a powerful way to write reusable, type-safe code. With generics, you can create functions and data structures that work with any type, improving the flexibility and maintainability of your code. By using type parameters and constraints, you can ensure that your code remains type-safe while still allowing for a wide range of types. While generics have some limitations, they are a valuable tool for Go developers looking to write more flexible and reusable code.
Defining Generic Functions and Types
1. What Are Generic Functions and Types?
Generics in Go allow you to write functions and types that can operate on values of any data type while maintaining type safety. Generic functions and types are defined with type parameters, which act as placeholders for actual types when the function or type is used. This enables you to write flexible, reusable code without sacrificing performance or safety.
2. Syntax for Defining Generic Functions
The syntax for defining a generic function in Go involves specifying type parameters within square brackets before the function signature. These type parameters represent placeholder types that can be replaced with actual types when the function is called.
2.1 Basic Generic Function Example
Here is an example of a basic generic function in Go that takes two parameters of the same type and returns their sum:
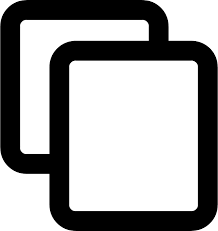
func Add[T any](a, b T) T {
return a + b
}
In this example, T
is the type parameter, and any
is the constraint that allows any type to be used for T
.
2.2 Using Constraints in Generic Functions
You can also specify constraints on the type parameter to restrict the types that can be passed to the function. For example, to create a function that adds two numbers, we can use a constraint like int
or float64>:
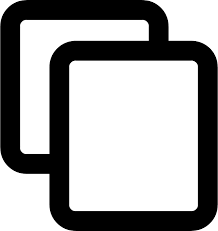
type Number interface {
int | float64
}
func Add[T Number](a, b T) T {
return a + b
}
Here, the Number
interface restricts the type parameter T
to only int
or float64
.
3. Syntax for Defining Generic Types
In addition to generic functions, Go also allows you to define generic types, such as structs and interfaces, that can hold values of any type. The syntax for defining a generic type is similar to that of generic functions, with the type parameter specified inside square brackets.
3.1 Generic Struct Example
Here’s an example of a generic struct that can hold a value of any type:
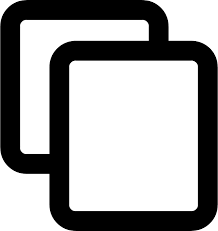
type Box[T any] struct {
value T
}
In this example, the Box
struct holds a value of type T
, which can be any type.
3.2 Generic Struct with Methods
Generic types can also have methods defined on them. For example, let's create a generic stack data structure with push and pop methods:
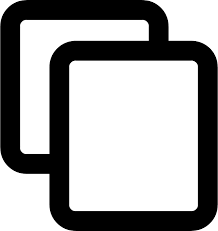
package main
import "fmt"
type Stack[T any] struct {
items []T
}
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
func (s *Stack[T]) Pop() T {
if len(s.items) == 0 {
var zero T
return zero
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item
}
func main() {
stack := Stack[int]{}
stack.Push(10)
stack.Push(20)
fmt.Println(stack.Pop()) // Output: 20
fmt.Println(stack.Pop()) // Output: 10
}
In this example, the Stack
struct is a generic type, and its methods Push
and Pop
work with any type T
.
4. Type Parameters and Constraints
In Go, type parameters can have constraints that limit the types that can be used with a generic function or type. Constraints are defined using interfaces, and they ensure that only types that satisfy the constraint can be used with the generic type or function.
4.1 Using the any
Constraint
The any
constraint allows any type to be used with a generic type or function. It’s the most flexible constraint available:
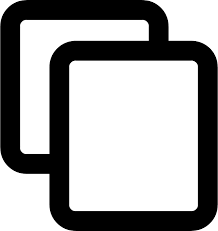
func Print[T any](value T) { fmt.Println(value) }
4.2 Using Custom Constraints
You can define custom constraints using interfaces. For example, to create a constraint that allows only numeric types, you could define an interface like this:
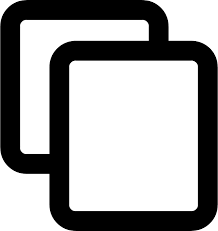
type Numeric interface {
int | float64
}
func Multiply[T Numeric](a, b T) T {
return a * b
}
In this example, the Numeric
interface restricts the type parameter T
to either int
or float64
.
5. Type Inference with Generics
Go has type inference, meaning you can omit the type parameter when calling a generic function or creating a generic type if Go can infer the type from the provided arguments. This makes the code more concise.
5.1 Type Inference in Function Calls
Go can infer the type parameter based on the arguments passed to the function:
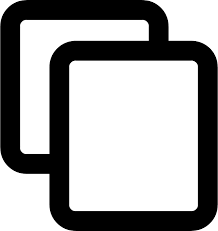
result := Add(3, 4)
In this case, Go infers that the type parameter T
is int
because both arguments are integers.
5.2 Type Inference in Type Initialization
Similarly, Go can infer the type when initializing a generic type like a struct:
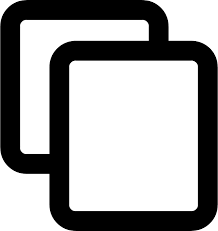
stack := Stack[int]{}
Here, Go infers that the type parameter T
is int
based on the initialization of the stack.
6. Conclusion
Defining generic functions and types in Go allows you to write flexible and reusable code while maintaining type safety. By using type parameters and constraints, you can specify exactly which types your functions and types can work with. Generics improve code reusability and reduce redundancy, making Go a more powerful language for developers who need to write generic code while avoiding the overhead of type assertions and interfaces.
Type Parameters in Go
1. Introduction to Type Parameters
Type parameters in Go allow you to create generic functions, types, and data structures. They are placeholders for types that can be defined when calling the function or creating an instance of the type. Type parameters provide flexibility by allowing a single function or type to work with multiple types while maintaining type safety and avoiding the need for type assertions.
2. Syntax for Type Parameters
In Go, type parameters are defined within square brackets [ ]
before the function or type signature. The type parameter can be any valid identifier, and it represents the type that will be passed to the function or type when used.
2.1 Defining Functions with Type Parameters
To define a generic function with type parameters, you specify the type parameter within square brackets [T any]
. Here, T
is the placeholder type and any
is the constraint, allowing any type to be used.
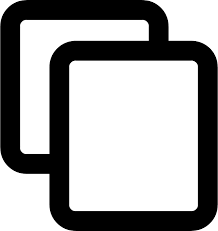
func Print[T any](value T) {
fmt.Println(value)
}
In this example, the Print
function works with any type T
because of the any
constraint.
2.2 Using Multiple Type Parameters
You can define multiple type parameters in a function or type by separating them with commas:
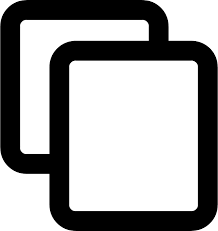
func Swap[T, U any](a T, b U) (U, T) {
return b, a
}
In this example, the Swap
function works with two type parameters, T
and U
, allowing it to swap values of different types.
3. Constraints on Type Parameters
Type parameters can have constraints, which specify which types are allowed to be passed to the function or type. Constraints are defined using interfaces, and they help enforce type safety by limiting the types that can be used.
3.1 Defining Type Constraints
You can define a custom constraint by creating an interface that restricts the allowed types. For example, you can create a constraint that limits the type parameter to numeric types:
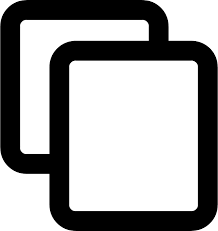
type Number interface {
int | float64
}
func Add[T Number](a, b T) T {
return a + b
}
Here, the constraint Number
ensures that the Add
function only works with int
or float64
types.
3.2 Using Built-in Constraints
Go provides some built-in constraints such as any
, which allows any type, and comparable
, which allows only types that can be compared with ==
and !=
operators. Here's an example of using the comparable
constraint:
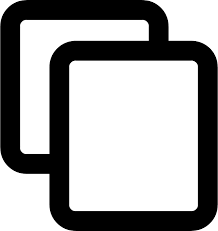
func Contains[T comparable](slice []T, value T) bool {
for _, item := range slice {
if item == value {
return true
}
}
return false
}
The constraint comparable
ensures that only types that support equality comparison can be used in the Contains
function.
4. Type Parameters in Data Structures
In addition to functions, type parameters can be used in Go data structures such as structs, slices, maps, and channels. This allows you to create generic data structures that work with any type while maintaining type safety.
4.1 Generic Struct Example
Here's an example of a generic stack data structure that uses a type parameter:
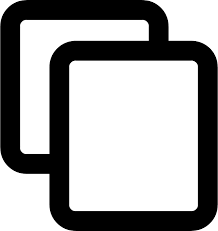
type Stack[T any] struct {
items []T
}
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
func (s *Stack[T]) Pop() T {
if len(s.items) == 0 {
var zero T
return zero
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item
}
In this example, the Stack
struct is a generic type that can hold items of any type T
.
4.2 Type Parameters in Maps
You can also use type parameters in maps. Here's an example:
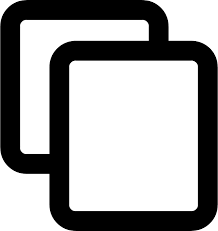
func CreateMap[K comparable, V any](keys []K, values []V) map[K]V {
result := make(map[K]V)
for i := 0; i < len(keys); i++ {
result[keys[i]] = values[i]
}
return result
}
In this example, the function CreateMap
takes two slices of type K
and V
and creates a map that associates keys of type K
with values of type V
.
5. Type Inference with Type Parameters
Go supports type inference with type parameters, meaning you can omit the type parameter when calling a generic function or creating an instance of a generic type, and Go will automatically infer the type based on the provided arguments.
5.1 Type Inference in Function Calls
Go can infer the type when calling a generic function:
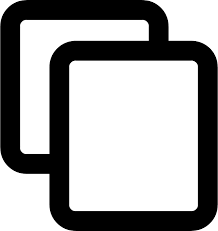
result := Add(3, 4)
In this case, Go infers that the type parameter T
is int
based on the arguments passed to the function.
5.2 Type Inference in Type Initialization
Similarly, Go can infer the type when initializing a generic type like a struct:
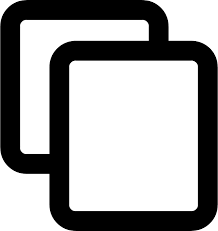
stack := Stack[int]{}
Here, Go infers that the type parameter T
is int
based on the initialization of the stack.
6. Conclusion
Type parameters in Go provide the ability to write flexible, reusable, and type-safe code. By allowing functions and data structures to work with any type, Go generics improve code reusability while maintaining type safety. Constraints on type parameters ensure that only valid types can be used, making Go a more powerful and efficient language for generic programming.
Using Constraints in Go Generics
1. Introduction to Constraints in Go Generics
Constraints in Go generics are used to restrict the types that can be used for type parameters. Constraints are defined using interfaces and allow you to enforce type safety while providing flexibility in the kinds of types that can be passed to generic functions and data structures. Constraints help ensure that only types that support certain operations can be used with generics.
2. Defining Constraints with Interfaces
In Go, constraints are defined using interfaces. A constraint is an interface that specifies the allowed types that a type parameter can take. By defining an interface with methods or types, you can restrict the valid types for a generic type or function.
2.1 Basic Example of a Constraint
Here’s an example where we define a constraint that restricts type T
to only int
and float64> types:
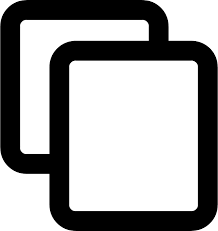
type Number interface {
int | float64
}
func Add[T Number](a, b T) T {
return a + b
}
In this example, the Number
constraint ensures that the Add
function can only work with int
or float64
types, ensuring type safety when performing arithmetic operations.
3. Using Constraints with Built-in Types
Go provides several built-in constraints that you can use in your generic functions and types. These constraints can simplify your code and make it easier to work with common use cases.
3.1 The any
Constraint
The any
constraint allows a type parameter to be any type, making it the most flexible option. It is equivalent to interface{}
, but more explicitly intended for generics.
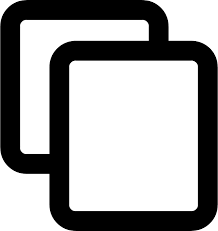
func Print[T any](value T) {
fmt.Println(value)
}
In this example, the Print
function can accept any type as its argument, as the type parameter T
is constrained by any
.
3.2 The comparable
Constraint
The comparable
constraint restricts the type to those that can be compared using the ==
and !=
operators. This can be useful when you need to compare the values of type parameters.
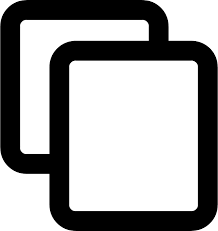
func Contains[T comparable](slice []T, value T) bool {
for _, item := range slice {
if item == value {
return true
}
}
return false
}
In this example, the Contains
function only works with types that can be compared using the equality operator, ensuring that the comparison operation is valid.
4. Defining Custom Constraints
You can define your own custom constraints by creating an interface that limits the types that can be used in a generic function or type. For example, you might want to restrict types to those that implement certain methods.
4.1 Custom Constraint Example
Here’s an example of a custom constraint that restricts the type parameter to types that implement the Stringer
interface:
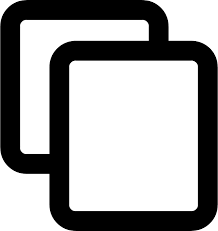
import "fmt"
type Stringer interface {
String() string
}
func PrintString[T Stringer](value T) {
fmt.Println(value.String())
}
In this example, the PrintString
function only accepts types that implement the Stringer
interface, ensuring that the value.String()
method can be called safely.
5. Using Multiple Constraints
Go allows you to apply multiple constraints to a type parameter, making your generic functions and types even more flexible. You can use multiple constraints by combining them with the &
operator.
5.1 Multiple Constraints Example
Here’s an example where we define a constraint that restricts the type parameter to types that are both comparable
and Stringer
:
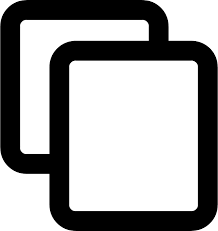
func PrintIfEqual[T comparable & Stringer](a, b T) {
if a == b {
fmt.Println(a.String())
}
}
In this example, the PrintIfEqual
function works with types that are both comparable and implement the Stringer
interface. This allows us to safely compare and print values.
6. Using Constraints in Data Structures
Constraints can also be used in Go data structures, such as slices, maps, and structs. This allows you to create more sophisticated data structures that enforce type restrictions while maintaining the flexibility of generics.
6.1 Generic Data Structure with Constraints
Here’s an example of a generic data structure (a stack) that enforces a constraint on the types it can store. The stack only accepts types that are comparable
:
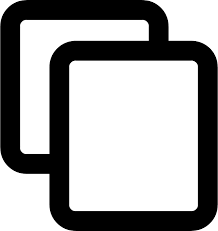
type Stack[T comparable] struct {
items []T
}
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
func (s *Stack[T]) Pop() T {
if len(s.items) == 0 {
var zero T
return zero
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item
}
In this example, the stack only accepts types that are comparable, ensuring that operations like equality checks are valid for the items in the stack.
7. Conclusion
Using constraints in Go generics allows you to control the types that can be passed to generic functions and types, providing both flexibility and type safety. By leveraging built-in constraints like any
and comparable
, as well as custom constraints, you can create powerful and reusable code. Constraints help enforce valid operations on types and ensure that your generics are used safely and effectively in a variety of scenarios.
Practical Use Cases for Generics
1. Introduction to Practical Use Cases
Generics in Go allow developers to write reusable, type-safe code. They enable the creation of functions, types, and data structures that can work with any data type, while still enforcing constraints on the types used. This flexibility makes generics highly useful for various real-world scenarios. In this section, we will explore several practical use cases for generics in Go.
2. Creating Reusable Data Structures
Generics are particularly useful for creating reusable data structures that work with different types. For example, you can implement generic data structures like stacks, queues, and linked lists that can store elements of any type.
2.1 Generic Stack Implementation
A stack is a fundamental data structure that operates on a "last in, first out" (LIFO) principle. By using generics, we can create a stack that works with any type:
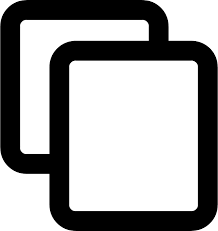
type Stack[T any] struct {
items []T
}
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
func (s *Stack[T]) Pop() T {
if len(s.items) == 0 {
var zero T
return zero
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item
}
This stack implementation can store elements of any type (e.g., int
, string
, etc.), making it highly reusable for different use cases.
3. Generic Sorting Algorithms
Generics can be used to write sorting algorithms that work with any data type that supports the comparison operators. This allows you to implement sorting functions that can sort slices of any type, provided that the type implements the appropriate comparison operations.
3.1 Generic Sorting Function
Here’s an example of a generic sorting function using the sort
package, which can sort any type that implements the comparable
constraint:
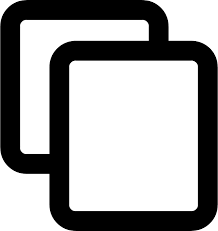
import "sort"
func Sort[T comparable](items []T) {
sort.Slice(items, func(i, j int) bool {
return items[i] < items[j]
})
}
This function sorts a slice of any type that is comparable, such as integers, floats, or strings.
4. Creating Generic Utility Functions
Generics allow you to create utility functions that can operate on different types. For example, you can write functions to check if an element exists in a slice, find the maximum value in a slice, or merge two slices together.
4.1 Generic Find Function
Here’s an example of a generic function that checks if an element exists in a slice:
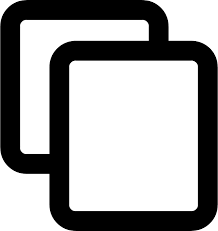
func Contains[T comparable](slice []T, value T) bool {
for _, item := range slice {
if item == value {
return true
}
}
return false
}
This function works with any type that is comparable and checks if the specified value exists in the slice.
5. Working with Collections
Generics are ideal for working with collections, such as slices, maps, and sets. You can create generic functions or types to perform common operations on collections without having to write type-specific code for each collection type.
5.1 Generic Map Filter Function
Here’s an example of a generic function that filters a map based on a condition. This function takes a map of any type and filters it based on the provided condition:
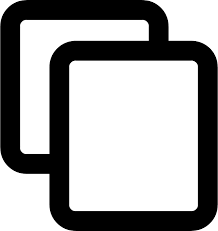
func FilterMap[K comparable, V any](m map[K]V, condition func(V) bool) map[K]V {
result := make(map[K]V)
for key, value := range m {
if condition(value) {
result[key] = value
}
}
return result
}
This function works with any map type and applies a filter condition to the values, returning a new map that contains only the elements that satisfy the condition.
6. Handling Multiple Types in Functions
Generics allow you to write functions that can handle multiple types while keeping the code type-safe. For instance, you can create a function that accepts different numeric types and performs arithmetic operations on them.
6.1 Generic Add Function for Numeric Types
Here’s an example of a generic function that adds two numbers. The function works with any numeric type (e.g., int
, float64
, etc.):
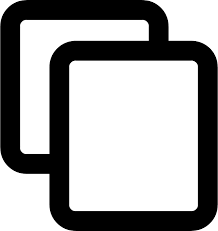
type Numeric interface {
int | float64
}
func Add[T Numeric](a, b T) T {
return a + b
}
This function can accept both int
and float64
types, allowing you to perform arithmetic operations on different numeric types.
7. Implementing Generic Algorithms
Generics make it easier to implement common algorithms that can work with different types of data. For example, you can write a generic search algorithm that works with different types of collections.
7.1 Generic Binary Search Algorithm
Here’s an example of a binary search algorithm implemented using generics:
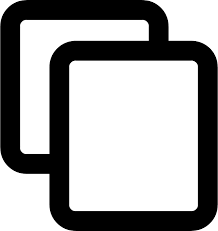
func BinarySearch[T comparable](items []T, target T) int {
low, high := 0, len(items)-1
for low <= high {
mid := low + (high-low)/2
if items[mid] == target {
return mid
}
if items[mid] < target {
low = mid + 1
} else {
high = mid - 1
}
}
return -1
}
This binary search function works with any type that is comparable, providing an efficient way to search for elements in sorted slices.
8. Conclusion
Generics in Go provide a powerful tool for writing reusable, type-safe code that can work with any data type while ensuring type safety. By using generics, you can create flexible and efficient functions and data structures that can handle a wide range of use cases, such as reusable data structures, sorting algorithms, utility functions, and more. With the ability to work with multiple types and apply constraints, generics enable Go developers to write more maintainable and scalable applications.
Introduction to Networking in Go
1. Overview of Networking in Go
Networking is a crucial aspect of modern software development, allowing applications to communicate over the internet or local networks. In Go, the net
package provides all the tools needed for building networked applications, from basic socket programming to creating robust server-client systems. This section introduces the fundamental concepts of networking in Go, including creating servers, clients, and handling network protocols.
2. The net
Package
The net
package is the foundation for networking in Go. It provides types and functions for working with IP addresses, URLs, and network protocols like TCP and UDP.
2.1 Commonly Used Types and Functions
net.Listen
: Creates a network listener, typically used for setting up a server.net.Dial
: Establishes a network connection to a remote address.net.Conn
: Represents a network connection, such as a TCP connection.net.IP
: Represents an IP address.net.URL
: Represents a URL for network communication.
3. Basic Networking Concepts
Before diving into Go's networking features, it's important to understand a few key concepts:
- IP Addressing: An IP address uniquely identifies a machine on a network.
- Ports: A port number identifies a specific service on a machine (e.g., HTTP runs on port 80).
- Protocols: Networking protocols define the rules for communication between devices (e.g., TCP, UDP, HTTP).
4. Creating a Simple TCP Server
One of the most common network protocols is TCP, which provides reliable, ordered, and error-checked delivery of a stream of data between applications. Here’s how to create a basic TCP server in Go:
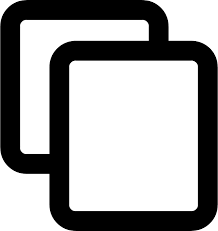
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Listen on TCP port 8080
listener, err := net.Listen("tcp", ":8080")
if err != nil {
fmt.Println("Error starting server:", err)
os.Exit(1)
}
defer listener.Close()
fmt.Println("Server is listening on port 8080...")
// Accept incoming connections
for {
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleConnection(conn)
}
}
func handleConnection(conn net.Conn) {
defer conn.Close()
fmt.Println("Connection established.")
// Read data from the connection
buffer := make([]byte, 1024)
for {
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading data:", err)
break
}
fmt.Println("Received:", string(buffer[:n]))
// Send a response
conn.Write([]byte("Message received"))
}
}
This server listens on port 8080 for incoming TCP connections. When a connection is established, it listens for data and responds with "Message received."
5. Creating a TCP Client
To connect to the TCP server, we can create a simple client that establishes a connection to the server and sends a message:
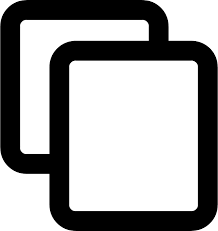
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Connect to the server at localhost:8080
conn, err := net.Dial("tcp", "localhost:8080")
if err != nil {
fmt.Println("Error connecting to server:", err)
os.Exit(1)
}
defer conn.Close()
// Send a message to the server
message := "Hello, Server!"
conn.Write([]byte(message))
// Read the server's response
buffer := make([]byte, 1024)
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading response:", err)
}
fmt.Println("Server response:", string(buffer[:n]))
}
This client connects to the server at localhost:8080
, sends a message, and prints the server’s response.
6. Working with UDP
UDP (User Datagram Protocol) is a connectionless protocol that is faster than TCP but does not guarantee message delivery or order. It is often used in real-time applications such as video streaming or online gaming.
6.1 Simple UDP Server
Here’s how you can create a simple UDP server in Go:
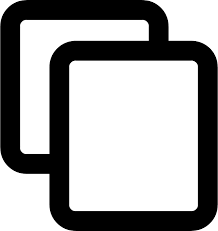
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Listen on UDP port 8080
udpAddress, err := net.ResolveUDPAddr("udp", ":8080")
if err != nil {
fmt.Println("Error resolving UDP address:", err)
os.Exit(1)
}
conn, err := net.ListenUDP("udp", udpAddress)
if err != nil {
fmt.Println("Error starting UDP server:", err)
os.Exit(1)
}
defer conn.Close()
fmt.Println("UDP server listening on port 8080...")
// Read and print incoming messages
buffer := make([]byte, 1024)
for {
n, addr, err := conn.ReadFromUDP(buffer)
if err != nil {
fmt.Println("Error reading from UDP:", err)
continue
}
fmt.Printf("Received %s from %s\n", string(buffer[:n]), addr)
}
}
This server listens for incoming UDP packets on port 8080 and prints the received message along with the sender's address.
6.2 Simple UDP Client
Here’s how you can create a simple UDP client to send a message to the server:
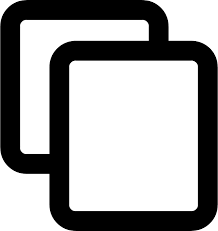
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Resolve server address
udpAddress, err := net.ResolveUDPAddr("udp", "localhost:8080")
if err != nil {
fmt.Println("Error resolving UDP address:", err)
os.Exit(1)
}
// Connect to the server
conn, err := net.DialUDP("udp", nil, udpAddress)
if err != nil {
fmt.Println("Error connecting to UDP server:", err)
os.Exit(1)
}
defer conn.Close()
// Send a message to the server
message := "Hello, UDP Server!"
conn.Write([]byte(message))
fmt.Println("Message sent to server")
}
This client sends a message to the UDP server at localhost:8080
.
7. Conclusion
Networking in Go is straightforward and powerful, thanks to the rich net
package. Whether you’re building a simple TCP server-client application or working with UDP for real-time communications, Go provides the tools needed to create robust networked applications. By understanding the basics of TCP and UDP, as well as how to handle sockets and connections, you can begin building scalable networked solutions in Go.
Working with Sockets in Go
1. Overview of Sockets
Sockets are endpoints for sending or receiving data across a computer network. In Go, the net
package provides support for creating and managing socket connections, both for client and server applications. Sockets are commonly used for implementing various communication protocols like TCP and UDP, which Go fully supports.
2. Types of Sockets
Go works with two main types of sockets:
- TCP Sockets: Reliable, connection-oriented sockets that ensure message delivery in order. These are commonly used for client-server applications.
- UDP Sockets: Connectionless, lightweight sockets that send data without guarantees, commonly used for real-time applications like gaming or video streaming.
3. Creating a TCP Server Socket
A TCP server socket listens for incoming client connections. Here’s how to create a simple TCP server socket in Go:
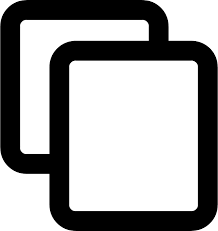
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Create a TCP server socket that listens on port 8080
listener, err := net.Listen("tcp", ":8080")
if err != nil {
fmt.Println("Error starting TCP server:", err)
os.Exit(1)
}
defer listener.Close()
fmt.Println("TCP server listening on port 8080...")
// Accept incoming client connections
for {
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleConnection(conn)
}
}
func handleConnection(conn net.Conn) {
defer conn.Close()
fmt.Println("Client connected:", conn.RemoteAddr())
// Read data from the client
buffer := make([]byte, 1024)
for {
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading from client:", err)
break
}
fmt.Println("Received:", string(buffer[:n]))
// Send a response to the client
conn.Write([]byte("Message received"))
}
}
This TCP server listens on port 8080, accepts incoming connections, and communicates with the client by reading and sending data through the socket.
4. Creating a TCP Client Socket
To connect to the TCP server, you can create a client socket using the net.Dial
function. Here’s an example of a simple TCP client:
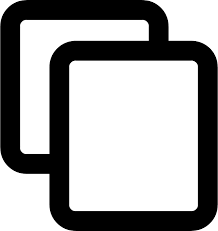
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Connect to the server at localhost:8080
conn, err := net.Dial("tcp", "localhost:8080")
if err != nil {
fmt.Println("Error connecting to TCP server:", err)
os.Exit(1)
}
defer conn.Close()
// Send a message to the server
message := "Hello, Server!"
conn.Write([]byte(message))
// Read the server's response
buffer := make([]byte, 1024)
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading from server:", err)
}
fmt.Println("Server response:", string(buffer[:n]))
}
This TCP client connects to a server at localhost:8080
, sends a message, and prints the server’s response received via the socket.
5. Creating a UDP Socket
UDP sockets work differently than TCP. They are connectionless, meaning there is no established connection between the client and server. Instead, data is sent as individual packets. Here’s how to create a simple UDP socket in Go:
5.1 UDP Server Socket
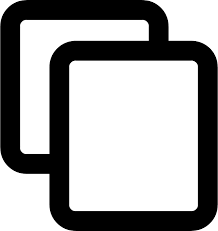
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Create a UDP server socket that listens on port 8080
udpAddr, err := net.ResolveUDPAddr("udp", ":8080")
if err != nil {
fmt.Println("Error resolving UDP address:", err)
os.Exit(1)
}
conn, err := net.ListenUDP("udp", udpAddr)
if err != nil {
fmt.Println("Error starting UDP server:", err)
os.Exit(1)
}
defer conn.Close()
fmt.Println("UDP server listening on port 8080...")
// Read incoming UDP packets
buffer := make([]byte, 1024)
for {
n, addr, err := conn.ReadFromUDP(buffer)
if err != nil {
fmt.Println("Error reading from UDP:", err)
continue
}
fmt.Printf("Received %s from %s\n", string(buffer[:n]), addr)
}
}
This server listens for incoming UDP packets on port 8080 and prints the message along with the sender’s address.
5.2 UDP Client Socket
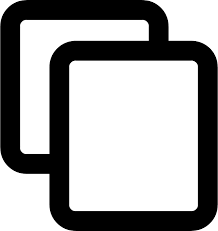
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Resolve the UDP server address
udpAddr, err := net.ResolveUDPAddr("udp", "localhost:8080")
if err != nil {
fmt.Println("Error resolving UDP address:", err)
os.Exit(1)
}
// Create a UDP client socket and send data to the server
conn, err := net.DialUDP("udp", nil, udpAddr)
if err != nil {
fmt.Println("Error connecting to UDP server:", err)
os.Exit(1)
}
defer conn.Close()
// Send a message to the server
message := "Hello, UDP Server!"
conn.Write([]byte(message))
fmt.Println("Message sent to UDP server")
}
This client sends a message to the UDP server at localhost:8080
.
6. Working with Unix Domain Sockets
Unix Domain Sockets (UDS) allow inter-process communication (IPC) on the same machine. These are commonly used in server applications to reduce overhead compared to networking over TCP/IP. Go provides support for Unix Domain Sockets through the net
package.
6.1 Example of a Unix Domain Socket Server
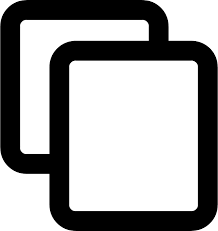
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Create a Unix domain socket server
socketPath := "/tmp/my_socket"
ln, err := net.Listen("unix", socketPath)
if err != nil {
fmt.Println("Error creating Unix domain socket:", err)
os.Exit(1)
}
defer ln.Close()
fmt.Println("Unix domain socket server listening on", socketPath)
// Accept incoming connections
for {
conn, err := ln.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleConnection(conn)
}
}
func handleConnection(conn net.Conn) {
defer conn.Close()
fmt.Println("Client connected:", conn.RemoteAddr())
// Read data from the client
buffer := make([]byte, 1024)
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading data from client:", err)
}
fmt.Println("Received:", string(buffer[:n]))
// Send a response to the client
conn.Write([]byte("Message received"))
}
This server listens on a Unix domain socket and handles incoming connections with a response message.
7. Conclusion
Working with sockets in Go is easy thanks to the powerful net
package, which allows you to create both TCP and UDP servers and clients. Whether you're building a simple network application or need more advanced features like Unix Domain Sockets, Go provides a great set of tools for networking. Understanding how to use sockets is an essential skill for developing networked applications, and Go’s simplicity and performance make it an ideal language for such tasks.
Creating a TCP Server and Client in Go
1. Overview of TCP Sockets
Transmission Control Protocol (TCP) is a connection-oriented, reliable protocol used to establish a connection between two devices on a network. In Go, you can build both a TCP server and client using the net
package. The server listens for incoming connections, while the client connects to the server to exchange data.
2. Steps for Creating a TCP Server in Go
A TCP server listens for incoming TCP connections from clients. Below is an example of how to create a basic TCP server in Go:
2.1 TCP Server Example
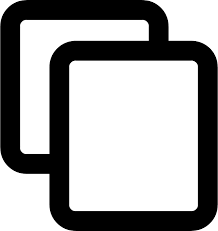
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Create a TCP server that listens on port 8080
listener, err := net.Listen("tcp", ":8080")
if err != nil {
fmt.Println("Error starting TCP server:", err)
os.Exit(1)
}
defer listener.Close()
fmt.Println("TCP server listening on port 8080...")
// Accept incoming client connections
for {
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleConnection(conn)
}
}
func handleConnection(conn net.Conn) {
defer conn.Close()
fmt.Println("Client connected:", conn.RemoteAddr())
// Read data from the client
buffer := make([]byte, 1024)
for {
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading from client:", err)
break
}
fmt.Println("Received:", string(buffer[:n]))
// Send a response to the client
conn.Write([]byte("Message received"))
}
}
This TCP server listens on port 8080. It accepts incoming client connections, reads the data sent by clients, and sends a response back to the client.
3. Steps for Creating a TCP Client in Go
A TCP client connects to a server and can send and receive data. Below is an example of how to create a basic TCP client in Go:
3.1 TCP Client Example
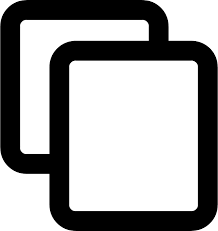
package main
import (
"fmt"
"net"
"os"
)
func main() {
// Connect to the server at localhost:8080
conn, err := net.Dial("tcp", "localhost:8080")
if err != nil {
fmt.Println("Error connecting to TCP server:", err)
os.Exit(1)
}
defer conn.Close()
// Send a message to the server
message := "Hello, Server!"
conn.Write([]byte(message))
// Read the server's response
buffer := make([]byte, 1024)
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading from server:", err)
}
fmt.Println("Server response:", string(buffer[:n]))
}
This TCP client connects to the server running on localhost:8080
, sends a message, and prints the server's response after receiving it.
4. How TCP Communication Works
In the examples above, the server listens for incoming connections, and the client connects to the server. The communication between the client and server happens in the following way:
- The server listens for connections on a specific port (in this case, port 8080).
- The client connects to the server using
net.Dial
, specifying the server's IP address and port. - Once a connection is established, the client sends data to the server using
Write
, and the server reads the data usingRead
. - The server processes the received data and responds back to the client.
- The communication can continue with the client sending more data and the server responding accordingly.
5. Handling Multiple Clients Concurrently
In the TCP server example, the server uses the go
keyword to handle each client connection in a separate goroutine. This ensures that the server can handle multiple clients concurrently. For each new client connection, the server spins off a new goroutine that calls the handleConnection
function, enabling it to interact with multiple clients at the same time.
6. Graceful Shutdown of Server
In a real-world application, you may want to gracefully shut down the server when it is no longer needed. You can do this by listening for system interrupts and closing the listener:
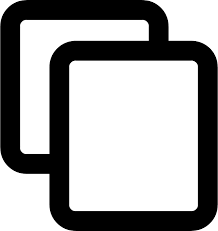
package main
import (
"fmt"
"net"
"os"
"os/signal"
"syscall"
)
func main() {
// Create a TCP server listener
listener, err := net.Listen("tcp", ":8080")
if err != nil {
fmt.Println("Error starting TCP server:", err)
os.Exit(1)
}
defer listener.Close()
fmt.Println("TCP server listening on port 8080...")
// Channel to listen for OS signals (e.g., termination signals)
signalChan := make(chan os.Signal, 1)
signal.Notify(signalChan, syscall.SIGINT, syscall.SIGTERM)
// Accept incoming connections in a separate goroutine
go func() {
for {
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleConnection(conn)
}
}()
// Wait for termination signal and gracefully shut down
<-signalChan
fmt.Println("\nShutting down server...")
}
In the modified server example, the server listens for termination signals (e.g., SIGINT or SIGTERM) and gracefully shuts down when such a signal is received. It also ensures that connections are handled in separate goroutines.
7. Conclusion
Creating a TCP server and client in Go is straightforward and efficient thanks to the net
package. The examples provided demonstrate how you can create a simple server that accepts client connections, processes data, and sends a response. Additionally, you can scale your server to handle multiple client connections concurrently using goroutines. By implementing proper error handling and graceful shutdown mechanisms, you can ensure the server operates reliably in real-world applications.
HTTP Client and Server in Go
1. Introduction to HTTP in Go
In Go, the net/http
package provides all the functionality needed to build both HTTP servers and clients. HTTP is a stateless protocol that allows clients (such as web browsers or other applications) to communicate with servers. You can create an HTTP server that listens for client requests and sends responses, and you can build an HTTP client that sends requests to a server and receives responses.
2. Creating an HTTP Server in Go
To create an HTTP server in Go, you can use the http.ListenAndServe
function to start the server, and http.HandleFunc
to define the routes (endpoints) that the server will handle. Here's an example:
2.1 HTTP Server Example
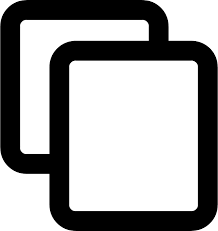
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
// Write a response to the client
fmt.Fprintf(w, "Hello, World!")
}
func main() {
// Define the route and associate it with the handler function
http.HandleFunc("/", handler)
// Start the HTTP server on port 8080
fmt.Println("Server is listening on port 8080...")
err := http.ListenAndServe(":8080", nil)
if err != nil {
fmt.Println("Error starting server:", err)
}
}
In this example, we define a handler function that sends a "Hello, World!" response to the client. We use http.HandleFunc
to map the route /
to the handler. The server is then started on port 8080 using http.ListenAndServe
.
3. Testing the HTTP Server
Once the server is running, you can test it by visiting http://localhost:8080
in your web browser or using a tool like curl
.
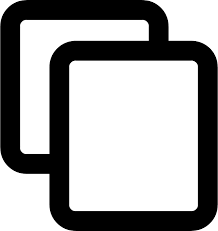
curl http://localhost:8080
This should display the message "Hello, World!" returned by the server.
4. Creating an HTTP Client in Go
To create an HTTP client in Go, you can use the http.Get
or http.Post
functions to send GET or POST requests to a server. You can also use http.NewRequest
to create more complex requests. Below is an example of creating a simple HTTP client that sends a GET request to the server:
4.1 HTTP Client Example
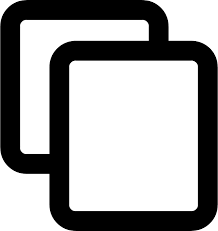
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
// Send a GET request to the server
resp, err := http.Get("http://localhost:8080")
if err != nil {
fmt.Println("Error making GET request:", err)
return
}
defer resp.Body.Close()
// Read and display the response body
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
fmt.Println("Response from server:", string(body))
}
In this example, the HTTP client sends a GET request to the server running on http://localhost:8080
and prints the server's response. The ioutil.ReadAll
function is used to read the response body.
5. Handling HTTP Methods
HTTP supports various methods such as GET, POST, PUT, DELETE, etc. In Go, you can handle different HTTP methods using the http.Request.Method
field in your handler function. Here's an example of handling both GET and POST requests:
5.1 Handling Different HTTP Methods
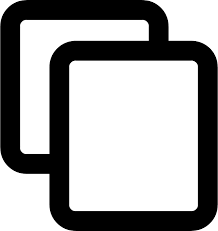
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
switch r.Method {
case "GET":
fmt.Fprintf(w, "This is a GET request")
case "POST":
fmt.Fprintf(w, "This is a POST request")
default:
http.Error(w, "Unsupported method", http.StatusMethodNotAllowed)
}
}
func main() {
http.HandleFunc("/", handler)
fmt.Println("Server is listening on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, the server checks the HTTP method of the incoming request and sends a different response based on whether the method is GET or POST.
6. HTTP Headers and Query Parameters
Both HTTP requests and responses can include headers and query parameters. You can access request headers using r.Header
and query parameters using r.URL.Query()
. Here’s an example:
6.1 Accessing Headers and Query Parameters
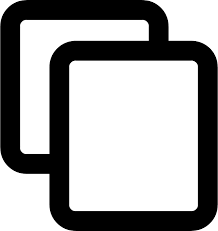
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
// Access query parameters
name := r.URL.Query().Get("name")
// Access headers
agent := r.Header.Get("User-Agent")
fmt.Fprintf(w, "Hello, %s! Your User-Agent is %s", name, agent)
}
func main() {
http.HandleFunc("/", handler)
fmt.Println("Server is listening on port 8080...")
http.ListenAndServe(":8080", nil)
}
In this example, the server retrieves the value of the name
query parameter and the User-Agent
header from the incoming request and sends a response including this information.
7. Sending POST Requests with JSON Data
To send a POST request with JSON data, you can use http.Post
or create a custom request with http.NewRequest
. Below is an example of sending JSON data in a POST request:
7.1 Sending JSON Data in POST Request
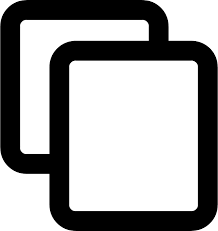
package main
import (
"bytes"
"encoding/json"
"fmt"
"net/http"
)
func main() {
// Create a map to hold the JSON data
data := map[string]string{"name": "John", "message": "Hello Server"}
// Marshal the map into JSON format
jsonData, err := json.Marshal(data)
if err != nil {
fmt.Println("Error marshalling JSON:", err)
return
}
// Send a POST request with JSON data
resp, err := http.Post("http://localhost:8080", "application/json", bytes.NewBuffer(jsonData))
if err != nil {
fmt.Println("Error making POST request:", err)
return
}
defer resp.Body.Close()
// Read and display the response
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
fmt.Println("Response from server:", string(body))
}
In this example, the client sends JSON data in the body of the POST request, and the server processes the request accordingly.
8. Conclusion
Creating an HTTP client and server in Go is easy and efficient with the net/http
package. You can define server routes, handle different HTTP methods, and send and receive data between clients and servers. This is the foundation for building web applications or services in Go. With Go's built-in concurrency and the simplicity of the HTTP package, you can build scalable and high-performance web applications.
Building a Web Scraper in Go
1. Introduction to Web Scraping
Web scraping is the process of extracting data from websites. It is often used to collect information from web pages for analysis or processing. In Go, web scraping can be achieved by sending HTTP requests to websites and parsing the HTML content of the pages. The net/http
and golang.org/x/net/html
packages in Go are commonly used for web scraping tasks.
2. Setting Up the Project
To get started, you'll need to install the necessary Go packages. For web scraping, the most commonly used package is x/net/html, which is a package for parsing HTML documents.
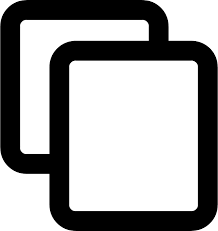
go get golang.org/x/net/html
Additionally, you may want to use an HTTP client package like net/http
to send requests to websites.
3. Sending HTTP Requests
Before scraping a website, you'll need to send an HTTP request to the target page to retrieve the HTML content. This can be done using the http.Get
function in Go. Here’s an example:
3.1 Sending an HTTP Request
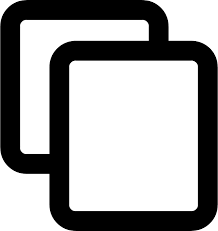
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
// Send a GET request to the target URL
resp, err := http.Get("https://example.com")
if err != nil {
fmt.Println("Error sending HTTP request:", err)
return
}
defer resp.Body.Close()
// Read the response body
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
// Print the HTML content of the page
fmt.Println(string(body))
}
In this example, a GET request is sent to https://example.com
, and the response body is printed to the console.
4. Parsing HTML Content
After retrieving the HTML content from the target website, you can parse it using the golang.org/x/net/html
package. This package provides a simple API to traverse and manipulate HTML documents. Below is an example of parsing the HTML content:
4.1 Parsing HTML Content
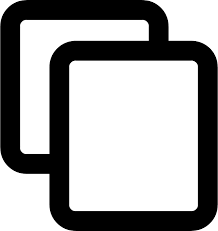
package main
import (
"fmt"
"net/http"
"golang.org/x/net/html"
"io/ioutil"
)
func main() {
// Send a GET request to the target URL
resp, err := http.Get("https://example.com")
if err != nil {
fmt.Println("Error sending HTTP request:", err)
return
}
defer resp.Body.Close()
// Read the response body
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
// Parse the HTML content
doc, err := html.Parse(string(body))
if err != nil {
fmt.Println("Error parsing HTML:", err)
return
}
// Traverse the HTML document and print the nodes
traverseHTML(doc)
}
func traverseHTML(n *html.Node) {
if n.Type == html.ElementNode {
fmt.Println("Element:", n.Data)
}
for c := n.FirstChild; c != nil; c = c.NextSibling {
traverseHTML(c)
}
}
In this example, the HTML document is parsed using the html.Parse
function, and the traverseHTML
function is used to iterate over the HTML nodes and print the names of the HTML elements.
5. Extracting Specific Data
Once the HTML content is parsed, you can extract specific elements or data from the page by searching for particular HTML tags or attributes. For example, if you want to extract all the <a>
tags (links) from a page, you can modify the traverseHTML
function:
5.1 Extracting Links from a Web Page
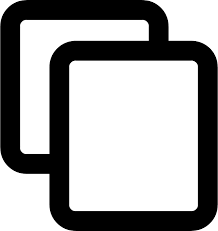
package main
import (
"fmt"
"net/http"
"golang.org/x/net/html"
"io/ioutil"
)
func main() {
// Send a GET request to the target URL
resp, err := http.Get("https://example.com")
if err != nil {
fmt.Println("Error sending HTTP request:", err)
return
}
defer resp.Body.Close()
// Read the response body
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
// Parse the HTML content
doc, err := html.Parse(string(body))
if err != nil {
fmt.Println("Error parsing HTML:", err)
return
}
// Extract and print all links from the page
extractLinks(doc)
}
func extractLinks(n *html.Node) {
if n.Type == html.ElementNode && n.Data == "a" {
// Look for the href attribute of tags
for _, attr := range n.Attr {
if attr.Key == "href" {
fmt.Println("Link:", attr.Val)
}
}
}
// Recursively traverse the HTML nodes
for c := n.FirstChild; c != nil; c = c.NextSibling {
extractLinks(c)
}
}
In this example, the extractLinks
function iterates through the parsed HTML nodes and checks for <a>
tags. If an <a>
tag has an href
attribute, the value of the attribute (the link) is printed to the console.
6. Handling Errors and Edge Cases
When building a web scraper, it’s essential to handle errors and edge cases such as network issues, invalid HTML, or missing data. You should always check for errors when sending requests, reading responses, or parsing HTML. Additionally, it’s important to ensure that your scraper is respectful of the website's robots.txt file, which specifies rules for web crawlers.
7. Throttling and Rate Limiting
To avoid overwhelming the server with too many requests in a short period, you should implement throttling or rate limiting in your web scraper. This can be achieved by introducing delays between requests or limiting the number of concurrent requests sent to the server. Go's goroutines and channels can be used to implement concurrency in your scraper while controlling the rate of requests.
7.1 Example of Throttling Requests
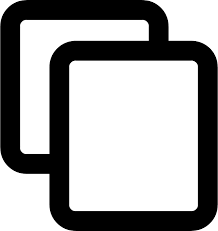
package main
import (
"fmt"
"net/http"
"time"
)
func main() {
// List of URLs to scrape
urls := []string{"https://example1.com", "https://example2.com", "https://example3.com"}
// Throttle the requests by introducing a delay
for _, url := range urls {
go scrape(url)
time.Sleep(1 * time.Second) // Delay between requests
}
// Wait for all requests to finish
time.Sleep(5 * time.Second)
}
func scrape(url string) {
// Send a GET request to the URL
resp, err := http.Get(url)
if err != nil {
fmt.Println("Error sending HTTP request:", err)
return
}
defer resp.Body.Close()
fmt.Println("Successfully scraped:", url)
}
In this example, we introduce a 1-second delay between each request using time.Sleep
to throttle the rate at which requests are sent.
8. Conclusion
Building a web scraper in Go is a straightforward process using the net/http
and golang.org/x/net/html
packages. With Go’s concurrency features, you can create efficient and scalable web scrapers. Remember to respect the website's robots.txt file, handle errors, and be mindful of rate limiting to avoid negatively affecting the target site.
Introduction to Cloud-Native Development with Go
1. What is Cloud-Native Development?
Cloud-native development refers to the practice of building and deploying applications that are designed to run in cloud environments. Cloud-native applications are typically broken down into smaller, manageable services (microservices) that can be independently developed, deployed, and scaled. These applications leverage cloud platforms' capabilities, such as scalability, high availability, and distributed systems.
Go, also known as Golang, is a statically typed, compiled programming language known for its simplicity, performance, and strong concurrency support. Go is well-suited for cloud-native development due to its speed, ease of use, and support for building scalable, distributed systems.
2. Key Concepts in Cloud-Native Development
Cloud-native development revolves around a few core principles:
- Microservices: Applications are broken down into small, independent services that can be developed, tested, and deployed individually.
- Containers: Software is packaged into containers (such as Docker) to ensure consistent deployment across environments.
- Orchestration: Managing containers and services using orchestration tools like Kubernetes for scaling and fault tolerance.
- Cloud Platforms: Cloud-native applications are often deployed on cloud platforms like AWS, Azure, and Google Cloud, which provide tools for managing services, storage, networking, and scaling.
3. Why Use Go for Cloud-Native Development?
Go is a popular choice for cloud-native development for several reasons:
- Performance: Go is a compiled language that generates efficient machine code, making it fast and lightweight, which is ideal for cloud applications that require high performance and low latency.
- Concurrency: Go's built-in concurrency model (goroutines and channels) makes it easy to build scalable applications that can handle multiple tasks simultaneously, an essential feature for cloud-native applications.
- Ease of Use: Go's simple syntax and comprehensive standard library make it easy to develop cloud-native applications quickly and maintain them over time.
- Microservices Support: Go is well-suited for building microservices, as it allows you to create independent, stateless services that can be deployed and scaled independently.
4. Building Cloud-Native Applications with Go
To begin building cloud-native applications with Go, you will need to focus on a few key aspects:
4.1 Creating a Simple Microservice
In cloud-native development, microservices are small, self-contained applications that perform specific tasks. Let's build a simple REST API using Go as a microservice that can be deployed in a cloud environment.
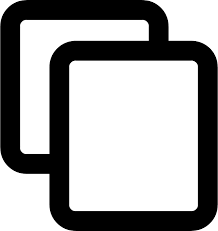
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type Message struct {
Message string `json:"message"`
}
func helloHandler(w http.ResponseWriter, r *http.Request) {
response := Message{Message: "Hello, Cloud-Native World!"}
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(response)
}
func main() {
http.HandleFunc("/hello", helloHandler)
fmt.Println("Listening on port 8080...")
http.ListenAndServe(":8080", nil)
}
This Go application defines a simple REST API with a single endpoint that returns a JSON response. This is a basic microservice that can be expanded to handle more complex tasks.
4.2 Containerizing the Go Application
To deploy our Go microservice to the cloud, we will containerize it using Docker. This ensures that the application runs consistently across different environments (local development, testing, production).
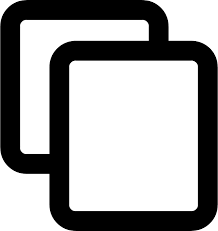
# Dockerfile
FROM golang:1.19-alpine
WORKDIR /app
COPY . .
RUN go mod tidy
RUN go build -o main .
CMD ["/app/main"]
This Dockerfile defines the steps to build and run the Go application inside a container. First, we use the official Go Docker image, copy the source code, run go mod tidy
to fetch dependencies, and then build the application. The container will run the built application using the CMD
instruction.
4.3 Building the Docker Image
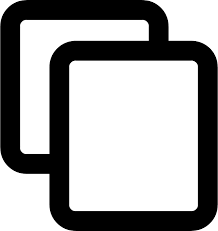
docker build -t go-microservice .
This command builds the Docker image for the Go application. Once the build is complete, we can run the application inside a container.
4.4 Running the Docker Container
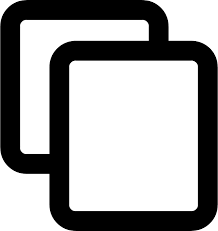
docker run -p 8080:8080 go-microservice
This command runs the container, mapping port 8080 on the host to port 8080 in the container. The application will be accessible at http://localhost:8080/hello
.
5. Cloud Deployment
Once our Go application is containerized, we can deploy it to a cloud platform like AWS, Google Cloud, or Azure. These platforms provide managed services for container orchestration, such as Amazon ECS, Google Kubernetes Engine (GKE), and Azure Kubernetes Service (AKS). Kubernetes is the most common orchestration platform used for cloud-native applications.
5.1 Deploying on Kubernetes
To deploy our Go microservice on Kubernetes, we need to define a Kubernetes deployment and service YAML file:
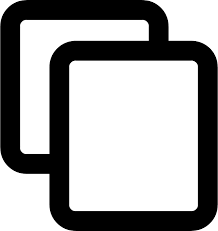
# deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: go-microservice
spec:
replicas: 2
selector:
matchLabels:
app: go-microservice
template:
metadata:
labels:
app: go-microservice
spec:
containers:
- name: go-microservice
image: go-microservice:latest
ports:
- containerPort: 8080
---
# service.yaml
apiVersion: v1
kind: Service
metadata:
name: go-microservice-service
spec:
selector:
app: go-microservice
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: LoadBalancer
This configuration defines a Kubernetes deployment with two replicas of the Go microservice and a service to expose the application externally via a LoadBalancer.
5.2 Applying Kubernetes Configurations
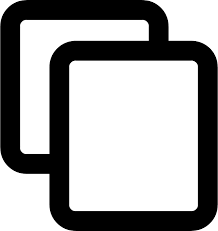
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
This command applies the Kubernetes configuration files to the Kubernetes cluster, creating a deployment and service for our Go microservice.
6. Monitoring and Scaling
After deploying a cloud-native application, monitoring and scaling become crucial. Cloud platforms provide monitoring tools such as CloudWatch on AWS, Stackdriver on Google Cloud, and Azure Monitor. These tools allow you to monitor the performance of your application and scale it based on traffic or load.
7. Conclusion
Cloud-native development with Go provides a powerful combination for building scalable, efficient, and highly available applications. By leveraging Go’s performance and concurrency model along with containerization and orchestration tools like Kubernetes, you can develop cloud-native applications that are ready to scale and meet the demands of modern cloud environments.
Using Go with AWS SDK
1. Introduction to AWS SDK for Go
The AWS SDK for Go allows you to interact with various AWS services, such as S3, EC2, DynamoDB, SQS, and more, directly from your Go applications. It provides a set of APIs for managing AWS resources, allowing you to create, read, update, and delete AWS services programmatically.
Using Go with the AWS SDK is highly beneficial due to Go's simplicity, performance, and support for concurrency, which makes it ideal for cloud-native applications that need to interact with AWS services.
2. Setting Up AWS SDK for Go
To get started with the AWS SDK for Go, you need to install the SDK and set up your AWS credentials.
2.1 Installing the AWS SDK for Go
Use the following command to install the AWS SDK for Go in your Go project:
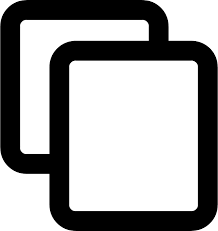
go get -u github.com/aws/aws-sdk-go
This command will install the latest version of the AWS SDK for Go into your Go project.
2.2 Setting Up AWS Credentials
To authenticate your Go application with AWS, you need to set up your AWS credentials. The AWS SDK for Go automatically looks for credentials in the following locations:
- Environment Variables: You can set AWS credentials using environment variables
AWS_ACCESS_KEY_ID
andAWS_SECRET_ACCESS_KEY
. - Shared Credentials File: The default credentials file is typically located at
~/.aws/credentials
. - IAM Role (for EC2 instances): If your Go application is running on an AWS EC2 instance, you can use IAM roles associated with the instance for authentication.
3. Using AWS SDK for Go with S3
One of the most common use cases for the AWS SDK for Go is interacting with Amazon S3 to store and retrieve files. Below is an example of how to upload and retrieve files from an S3 bucket using Go.
3.1 Example: Uploading a File to S3
To upload a file to an S3 bucket, you can use the PutObject
API provided by the AWS SDK for Go.
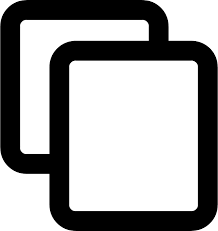
package main
import (
"bytes"
"fmt"
"log"
"os"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func uploadToS3(bucket string, key string, fileName string) error {
// Create a new session with AWS
sess, err := session.NewSession(&aws.Config{
Region: aws.String("us-east-1")},
)
if err != nil {
return err
}
// Open the file to upload
file, err := os.Open(fileName)
if err != nil {
return err
}
defer file.Close()
// Get file content
fileInfo, err := file.Stat()
if err != nil {
return err
}
fileContent := make([]byte, fileInfo.Size())
_, err = file.Read(fileContent)
if err != nil {
return err
}
// Create an S3 service client
s3Svc := s3.New(sess)
// Upload the file to S3
_, err = s3Svc.PutObject(&s3.PutObjectInput{
Bucket: aws.String(bucket),
Key: aws.String(key),
Body: bytes.NewReader(fileContent),
})
if err != nil {
return err
}
return nil
}
func main() {
err := uploadToS3("my-bucket", "my-file.txt", "file.txt")
if err != nil {
log.Fatalf("Failed to upload file: %v", err)
}
fmt.Println("File uploaded successfully to S3!")
}
This example demonstrates how to upload a file to an S3 bucket using the PutObject
API. The file is read, and then the content is uploaded to the specified bucket and key.
3.2 Example: Retrieving a File from S3
To retrieve a file from an S3 bucket, you can use the GetObject
API.
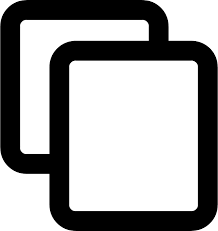
package main
import (
"fmt"
"io/ioutil"
"log"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func downloadFromS3(bucket string, key string) ([]byte, error) {
// Create a new session with AWS
sess, err := session.NewSession(&aws.Config{
Region: aws.String("us-east-1")},
)
if err != nil {
return nil, err
}
// Create an S3 service client
s3Svc := s3.New(sess)
// Get the object from S3
result, err := s3Svc.GetObject(&s3.GetObjectInput{
Bucket: aws.String(bucket),
Key: aws.String(key),
})
if err != nil {
return nil, err
}
defer result.Body.Close()
// Read the file content
fileContent, err := ioutil.ReadAll(result.Body)
if err != nil {
return nil, err
}
return fileContent, nil
}
func main() {
content, err := downloadFromS3("my-bucket", "my-file.txt")
if err != nil {
log.Fatalf("Failed to download file: %v", err)
}
fmt.Printf("File content: %s\n", content)
}
This code demonstrates how to download a file from an S3 bucket. The GetObject
API retrieves the file, and the content is printed to the console.
4. Working with Other AWS Services
The AWS SDK for Go supports a wide range of AWS services. Some other common services you might interact with include:
- EC2: Managing virtual machines (instances) in the AWS cloud.
- DynamoDB: Interacting with AWS's managed NoSQL database service.
- SQS: Using the Simple Queue Service for message queuing between distributed systems.
- Lambda: Executing serverless functions in the cloud.
Each of these services has its own specific API in the AWS SDK for Go, and you can interact with them similarly to how you interact with S3 (by creating a session, configuring the service, and calling the API). Refer to the AWS SDK for Go documentation for more details on using other services.
5. Conclusion
The AWS SDK for Go is a powerful tool for integrating AWS services into your Go applications. Whether you're working with storage, computing, or messaging services, the SDK provides all the necessary APIs for interacting with AWS resources. By leveraging Go's performance and the AWS SDK, you can build scalable, cloud-native applications that take full advantage of AWS's cloud services.
Building Microservices with Go
1. Introduction to Microservices Architecture
Microservices architecture is a design pattern where an application is divided into smaller, loosely coupled services, each responsible for a specific functionality. These services communicate with each other over the network, typically through APIs. The key advantages of microservices include scalability, flexibility in technology choice, fault tolerance, and easier maintenance.
Go is a great choice for building microservices due to its simplicity, concurrency support, and performance. It enables building lightweight and fast services that can be deployed independently.
2. Setting Up the Microservices Environment
To build microservices in Go, you need to set up a development environment that includes the following components:
- Go Programming Language: Make sure you have Go installed on your system. Download and install it from Go's official website.
- Docker: For containerization, ensuring that each microservice can run independently in a containerized environment.
- API Gateway (optional): A reverse proxy that routes incoming requests to the correct microservice.
- Message Broker (optional): For asynchronous communication between microservices. Examples include RabbitMQ or Kafka.
- Database: Each microservice should ideally have its own database to follow the principle of decentralized data management.
3. Creating a Simple Go Microservice
Let's start by creating a simple HTTP-based microservice using Go. This microservice will expose a REST API endpoint that returns a message when accessed.
3.1 Example: Simple Go Microservice
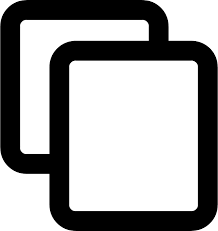
package main
import (
"fmt"
"log"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
w.WriteHeader(http.StatusOK)
fmt.Fprintf(w, "Hello from Go Microservice!")
}
func main() {
http.HandleFunc("/hello", helloHandler)
log.Println("Starting server on :8080")
if err := http.ListenAndServe(":8080", nil); err != nil {
log.Fatalf("Error starting server: %v", err)
}
}
This basic microservice listens on port 8080 and exposes a single `/hello` endpoint. When accessed, it responds with "Hello from Go Microservice!"
4. Structuring Microservices in Go
As you build more complex microservices, you need to think about structuring your project to keep code organized and maintainable. Here's an example of a typical Go microservice project structure:
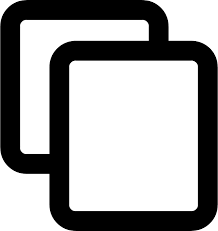
/my-microservice
|-- /cmd
| |-- /service
| |-- main.go
|-- /internal
| |-- /handlers
| |-- /models
| |-- /services
|-- /pkg
|-- /api
|-- Dockerfile
|-- go.mod
|-- go.sum
In this structure:
- /cmd: Contains the entry point to your application (e.g., main.go).
- /internal: Contains core application logic, such as handlers, models, and services.
- /pkg: Contains reusable libraries or utilities.
- /api: Contains the API definitions (e.g., Swagger files).
5. Inter-Service Communication in Microservices
Microservices often need to communicate with each other. There are two common ways to achieve this:
- HTTP-based communication: Services expose RESTful APIs, and other services interact with them over HTTP. Go’s standard
net/http
package provides everything you need to create HTTP clients and servers. - Message-based communication: Services can communicate asynchronously using a message broker like RabbitMQ, Kafka, or NATS. This is useful for decoupling services and handling tasks such as background jobs.
5.1 Example: HTTP Client in Go
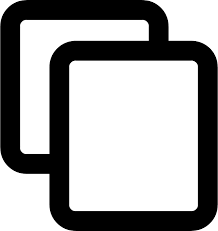
package main
import (
"fmt"
"log"
"net/http"
"io/ioutil"
)
func fetchData() {
resp, err := http.Get("http://localhost:8080/hello")
if err != nil {
log.Fatalf("Error fetching data: %v", err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
log.Fatalf("Error reading response: %v", err)
}
fmt.Println("Response from service:", string(body))
}
func main() {
fetchData()
}
This example shows how to make an HTTP GET request to another microservice running on localhost. It fetches the "Hello from Go Microservice!" message we defined earlier.
6. Containerizing Microservices with Docker
To deploy your Go microservice in a cloud environment or as part of a microservices architecture, you’ll likely want to containerize it using Docker. This ensures the service can run consistently across different environments.
6.1 Example: Dockerfile for a Go Microservice
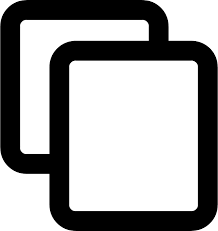
FROM golang:1.18-alpine AS build
WORKDIR /app
COPY . .
RUN go mod tidy
RUN go build -o my-microservice .
FROM alpine:latest
WORKDIR /root/
COPY --from=build /app/my-microservice .
CMD ["./my-microservice"]
This Dockerfile
creates a multi-stage build. First, it uses the official Go image to build the Go binary, then it copies the binary into a smaller Alpine Linux image to keep the final container lightweight.
7. Using API Gateway for Microservices
An API gateway is a common pattern in microservices architectures, which acts as a reverse proxy to route requests to the appropriate microservice. It can also handle other responsibilities like authentication, logging, and rate limiting.
Popular tools for API gateways include:
- NGINX: A powerful web server that can act as an API Gateway.
- Traefik: A dynamic reverse proxy designed for microservices environments.
8. Monitoring and Logging in Microservices
Effective monitoring and logging are crucial in a microservices architecture to ensure that each service is functioning as expected and to help debug issues. Some tools and techniques include:
- Prometheus and Grafana: For monitoring performance and visualizing metrics.
- ELK Stack: Elasticsearch, Logstash, and Kibana for centralized logging.
- Distributed Tracing: Tools like Jaeger or OpenTelemetry to trace requests across microservices.
9. Conclusion
Building microservices with Go allows you to take advantage of Go's simplicity, performance, and concurrency to create highly efficient and scalable services. By breaking down applications into smaller services, you gain flexibility, easier maintenance, and independent scalability. Docker, API Gateways, and monitoring tools help you deploy and manage these services in production.
Go and Docker Containers
1. Introduction to Docker Containers
Docker is a platform for developing, shipping, and running applications inside lightweight, portable containers. Containers package an application along with its dependencies, configuration, and runtime environment, ensuring it runs consistently across different environments.
Go and Docker work well together because Go applications are statically compiled, meaning they don’t rely on the host environment’s libraries. This makes containerization straightforward and efficient.
2. Why Use Docker with Go?
- Consistency: Docker ensures that your Go application runs consistently on any platform, be it your local machine, a staging server, or production.
- Isolation: Docker containers isolate your application from the host system and other services, preventing conflicts between dependencies.
- Portability: Docker containers are portable, meaning you can deploy your Go application to any cloud provider, on-premises system, or even on a developer’s machine.
- Scalability: Docker allows easy scaling of Go applications in a microservices environment, where each container can represent a separate service.
3. Setting Up Docker for Go Development
Before using Docker with Go, you need to have the following setup:
- Install Docker: Download and install Docker from Docker's official website.
- Go Environment: Make sure Go is installed on your system. You can download Go from Go's official website.
- Docker Compose (Optional): If you’re working with multiple services, Docker Compose can help you define and run multi-container Docker applications.
4. Creating a Go Application and Dockerizing It
To demonstrate how to use Docker with Go, let’s create a simple Go application and containerize it.
4.1 Example: Simple Go Web Application
This Go web application will serve a simple message when accessed via a web browser.
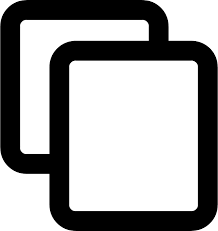
package main
import (
"fmt"
"log"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, Docker and Go!")
}
func main() {
http.HandleFunc("/", handler)
log.Println("Server is starting...")
if err := http.ListenAndServe(":8080", nil); err != nil {
log.Fatalf("Failed to start server: %v", err)
}
}
This Go web application listens on port 8080 and responds with "Hello, Docker and Go!" to all incoming HTTP requests.
4.2 Dockerizing the Go Application
Now, let's containerize this Go application using Docker. The first step is to create a Dockerfile
in the root of your Go project.
Dockerfile
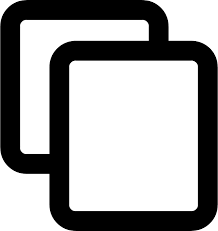
# Step 1: Use the official Go image to build the application
FROM golang:1.18-alpine AS build
WORKDIR /app
COPY . .
# Install dependencies
RUN go mod tidy
RUN go build -o my-go-app .
# Step 2: Use a smaller image to run the application
FROM alpine:latest
WORKDIR /root/
COPY --from=build /app/my-go-app .
# Expose port
EXPOSE 8080
CMD ["./my-go-app"]
This Dockerfile
has two stages:
- Build stage: The Go application is built using the official Go image.
- Runtime stage: The application is copied into a smaller Alpine Linux image to reduce the size of the final container.
4.3 Building the Docker Image
To build the Docker image, run the following command in the terminal:
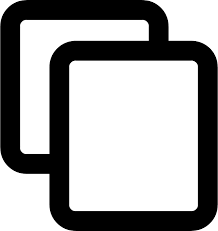
docker build -t my-go-app .
This command tells Docker to build the image using the current directory (.) as the context and tag it as my-go-app
.
4.4 Running the Docker Container
Once the image is built, you can run the container with the following command:
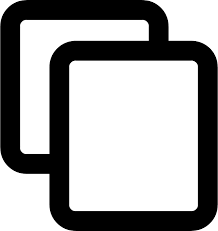
docker run -p 8080:8080 my-go-app
This runs the container and maps port 8080 on your local machine to port 8080 inside the container. You can now access your Go web application by navigating to http://localhost:8080 in your browser.
5. Docker Compose for Multi-Service Applications
If your Go application interacts with other services (such as a database or a cache), Docker Compose allows you to define and manage multi-container applications. Here's a basic example of how to set up Docker Compose for a Go application with a PostgreSQL database:
5.1 docker-compose.yml
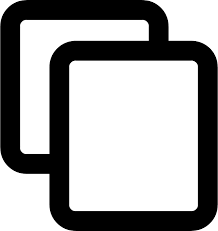
version: "3.8"
services:
web:
build: .
ports:
- "8080:8080"
depends_on:
- db
db:
image: postgres:alpine
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
ports:
- "5432:5432"
This docker-compose.yml
file defines two services:
- web: The Go application, which depends on the
db
service (PostgreSQL). - db: A PostgreSQL database running on the official PostgreSQL Alpine image.
To start the services, run:
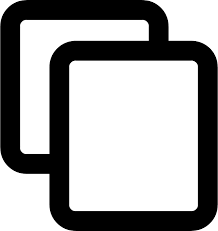
docker-compose up
6. Optimizing Docker Images for Go
While Docker makes it easy to containerize Go applications, there are several ways to optimize your Docker images:
- Multi-stage builds: As shown earlier, use multi-stage builds to create smaller, more efficient images by separating the build process and the runtime environment.
- Use minimal base images: For example, use
alpine
as the base image to reduce the size of your container. - Clean up after yourself: Remove unnecessary files (e.g., Go cache or test files) from the container after the build process.
7. Troubleshooting and Debugging Go Applications in Docker
When working with Go applications in Docker, common issues include:
- Port Mapping: Ensure that the correct ports are mapped between the container and the host machine.
- Missing Dependencies: Make sure all dependencies are correctly included in your Docker image, either via the
go mod
command or by copying the correct files into the container. - Logs: Use
docker logs
to view logs from a running container.
8. Conclusion
Docker simplifies the process of containerizing Go applications, making them easier to deploy, scale, and maintain. By using Docker's efficient containerization, you can ensure that your Go applications run consistently across different environments. Whether you're deploying a single Go service or a multi-service application, Docker provides a flexible and portable way to manage your services.
Go for Kubernetes Development
1. Introduction to Kubernetes
Kubernetes is an open-source platform that automates the deployment, scaling, and management of containerized applications. It manages clusters of containers, ensuring that applications run efficiently and reliably across cloud environments.
Go is the language used by Kubernetes itself, and it is often the preferred language for developing tools and applications that interact with Kubernetes. Learning Go for Kubernetes development allows you to extend Kubernetes, build operators, and create custom controllers.
2. Why Use Go for Kubernetes Development?
- Native Kubernetes Support: Kubernetes is written in Go, making it an ideal language for writing Kubernetes controllers, operators, and extensions.
- Strong Concurrency Model: Go’s goroutines and channels make it perfect for handling parallelism and concurrent tasks, a common requirement in Kubernetes development.
- Rich Ecosystem: Go provides a robust standard library and many third-party packages for interacting with Kubernetes APIs and managing clusters.
- Performance: Go is compiled to machine code, making it highly performant for cloud-native environments.
3. Setting Up Go for Kubernetes Development
Before you start developing with Go for Kubernetes, you need to set up your local development environment:
- Install Go: Download and install Go from Go's official website.
- Set Up Kubernetes: Install and configure Kubernetes on your machine using tools like K3s or Minikube.
- Install kubectl: Install the Kubernetes command-line tool,
kubectl
, to interact with Kubernetes clusters. - Install Docker: Docker is essential for building container images that Kubernetes will deploy.
4. Interacting with Kubernetes Using Go
Kubernetes provides a rich REST API that can be accessed programmatically to manage resources such as pods, services, deployments, and more. Go has a dedicated client library for interacting with Kubernetes, which is the client-go
package.
4.1 Setting Up client-go
To interact with Kubernetes from a Go application, you need to install the client-go
library:
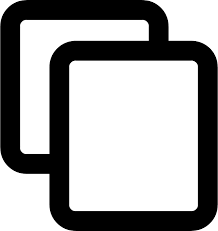
go get k8s.io/client-go@v0.23.0
You can also install other useful packages like apiextensions-apiserver
for handling custom resources and controller-runtime
for building controllers and operators.
4.2 Example: Get Kubernetes Pods with Go
Here is an example of using the client-go
package to retrieve a list of pods from a Kubernetes cluster:
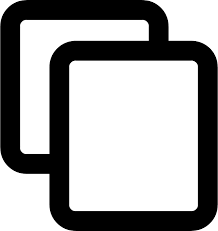
package main
import (
"context"
"fmt"
"log"
metav1 "k8s.io/apimachinery/pkg/apis/meta/v1"
"k8s.io/client-go/kubernetes"
"k8s.io/client-go/tools/clientcmd"
)
func main() {
// Load kubeconfig from default location (~/.kube/config)
kubeconfig := "/Users/yourname/.kube/config"
config, err := clientcmd.BuildConfigFromFlags("", kubeconfig)
if err != nil {
log.Fatalf("Failed to build config: %v", err)
}
// Create a Kubernetes client
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
log.Fatalf("Failed to create client: %v", err)
}
// Get a list of pods in the default namespace
pods, err := clientset.CoreV1().Pods("default").List(context.Background(), metav1.ListOptions{})
if err != nil {
log.Fatalf("Failed to list pods: %v", err)
}
// Print the names of the pods
for _, pod := range pods.Items {
fmt.Println(pod.Name)
}
}
This example demonstrates how to:
- Load the Kubernetes configuration from the kubeconfig file.
- Create a Kubernetes client using the
client-go
package. - Retrieve a list of pods in the "default" namespace and print their names.
5. Building Kubernetes Operators with Go
Operators are controllers in Kubernetes that extend its capabilities to manage custom resources. They are written in Go and use libraries like controller-runtime
to handle the business logic for managing resources.
5.1 What is a Kubernetes Operator?
An Operator is a custom Kubernetes controller that watches for changes to a custom resource and takes action based on those changes. For example, an Operator might automatically manage the deployment of a database, ensuring that backups are taken regularly or that the database is scaled up and down as needed.
5.2 Building a Simple Operator
To build an Operator, you can use the operator-sdk
tool, which simplifies the process of creating and managing Kubernetes Operators.
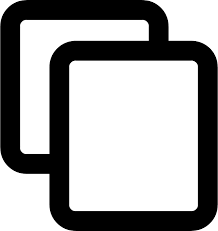
# Install the operator-sdk tool
go get github.com/operator-framework/operator-sdk
# Create a new operator project
operator-sdk init --domain mydomain.com --repo github.com/myusername/my-operator
The operator-sdk
generates the basic structure for your operator project, including scaffolding for your API, controller, and deployment files.
6. Kubernetes Custom Resources and CRDs in Go
Custom Resources (CRs) allow you to define your own Kubernetes objects, and Custom Resource Definitions (CRDs) define how those resources are validated and managed.
To create a CRD in Go, you need to define your resource’s schema and register it with the Kubernetes API server. Here’s a simple example of defining a custom resource and applying the CRD:
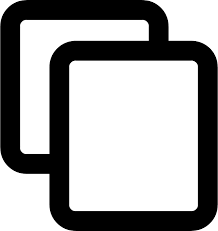
# Define the CRD for a "Database" resource
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: databases.mydomain.com
spec:
group: mydomain.com
names:
kind: Database
plural: databases
scope: Namespaced
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
storage:
type: string
Once the CRD is applied to the cluster, you can create and manage instances of the "Database" resource, which can be watched and handled by your custom operator.
7. Deploying Go Applications on Kubernetes
Once your Go application is ready, you can package it into a Docker container and deploy it to Kubernetes using kubectl
.
7.1 Example: Dockerfile for Go Application
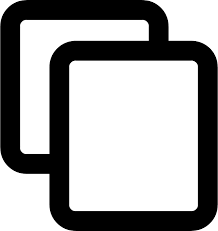
FROM golang:1.18-alpine AS build
WORKDIR /app
COPY . .
# Build the Go application
RUN go build -o my-go-app .
# Use a smaller image for the runtime
FROM alpine:latest
WORKDIR /root/
COPY --from=build /app/my-go-app .
EXPOSE 8080
CMD ["./my-go-app"]
This Dockerfile builds a Go application and deploys it in a minimal Alpine container.
7.2 Example: Kubernetes Deployment
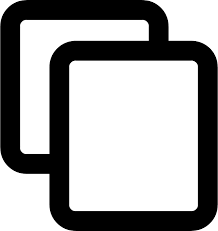
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-go-app
spec:
replicas: 3
selector:
matchLabels:
app: my-go-app
template:
metadata:
labels:
app: my-go-app
spec:
containers:
- name: my-go-app
image: myusername/my-go-app:latest
ports:
- containerPort: 8080
This Kubernetes deployment file specifies the Go application container, its replica count, and the port on which the application listens.
8. Troubleshooting Kubernetes Development with Go
When developing for Kubernetes using Go, you may encounter several challenges:
- API Errors: Ensure that your Go application correctly handles Kubernetes API responses and errors.
- Resource Management: Properly manage Kubernetes resources like CPU and memory limits when deploying your Go application.
- Log Aggregation: Use tools like Fluentd or Kubernetes logging to aggregate logs from your Go application deployed on Kubernetes.
9. Conclusion
Go is an excellent language for Kubernetes development due to its performance, concurrency model, and integration with Kubernetes tools. By using Go, you can build custom controllers, operators, and other Kubernetes extensions, making it easier to manage and scale containerized applications in Kubernetes environments.
Using Go for Data Processing
1. Introduction to Data Processing in Go
Data processing refers to the manipulation and transformation of data to extract useful information. Go is a powerful language for building efficient data processing pipelines due to its speed, concurrency model, and simplicity. It’s commonly used for tasks like data scraping, transformation, cleaning, aggregation, and analysis.
In this section, we’ll explore how to use Go for handling and processing large volumes of data, including reading from files, processing streams of data, and performing transformations efficiently.
2. Why Use Go for Data Processing?
- Performance: Go is a statically typed, compiled language that is optimized for performance, making it ideal for high-performance data processing.
- Concurrency: Go’s goroutines and channels make it easy to process data in parallel, which can significantly speed up the processing of large datasets.
- Ease of Use: Go’s simple syntax and standard library make it easy to work with data and build processing pipelines with minimal boilerplate code.
- Strong Ecosystem: Go has a rich ecosystem of libraries for working with various data sources, including files, databases, and APIs.
3. Reading and Writing Data in Go
Before processing data, you need to read it into your Go application. Go provides several ways to read data from files, including using the standard library os
, bufio
, and io
packages.
3.1 Reading Data from a File
Here is an example of reading text from a file in Go:
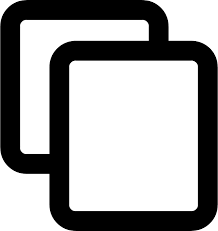
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
// Open the file
file, err := os.Open("data.txt")
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
// Create a scanner to read the file line by line
scanner := bufio.NewScanner(file)
for scanner.Scan() {
// Print each line of the file
fmt.Println(scanner.Text())
}
if err := scanner.Err(); err != nil {
fmt.Println("Error reading file:", err)
}
}
3.2 Writing Data to a File
Writing processed data back to a file can be done using the os
and bufio
packages:
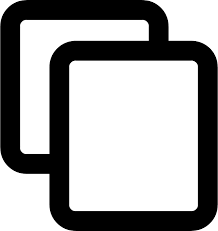
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
// Create a new file
file, err := os.Create("output.txt")
if err != nil {
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
// Write data to the file
writer := bufio.NewWriter(file)
_, err = writer.WriteString("Processed data\n")
if err != nil {
fmt.Println("Error writing to file:", err)
return
}
writer.Flush()
}
4. Processing Data in Parallel with Goroutines
Go’s goroutines and channels are powerful tools for handling concurrent tasks. You can use them to process data in parallel, which is especially useful for tasks that involve processing large datasets.
4.1 Example: Parallel Data Processing
In this example, we will use goroutines to process data concurrently:
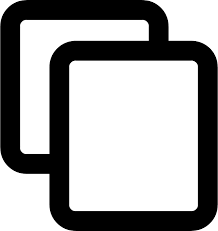
package main
import (
"fmt"
"sync"
)
func processData(data int, wg *sync.WaitGroup) {
defer wg.Done()
// Simulate processing
fmt.Printf("Processing data: %d\n", data)
}
func main() {
var wg sync.WaitGroup
dataList := []int{1, 2, 3, 4, 5}
// Process data in parallel
for _, data := range dataList {
wg.Add(1)
go processData(data, &wg)
}
// Wait for all goroutines to finish
wg.Wait()
}
In this example, we create a goroutine for each data item and use a sync.WaitGroup
to wait for all goroutines to finish before exiting the program.
5. Data Transformation in Go
Data transformation involves changing the format or structure of data. Go’s built-in functions and libraries make it easy to perform common transformations such as filtering, mapping, and reducing.
5.1 Example: Filtering Data
Here’s an example of filtering a list of integers in Go:
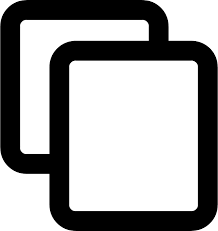
package main
import "fmt"
func filter(data []int, condition func(int) bool) []int {
var result []int
for _, item := range data {
if condition(item) {
result = append(result, item)
}
}
return result
}
func main() {
data := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
// Filter even numbers
filteredData := filter(data, func(x int) bool {
return x%2 == 0
})
fmt.Println("Filtered data:", filteredData)
}
In this example, we use a higher-order function filter
that takes a condition function to filter out even numbers from a list.
5.2 Example: Mapping Data
Mapping data involves applying a transformation to each element in a collection. Here is an example of mapping data in Go:
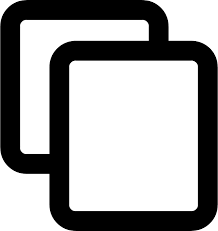
package main
import "fmt"
func mapData(data []int, transform func(int) int) []int {
var result []int
for _, item := range data {
result = append(result, transform(item))
}
return result
}
func main() {
data := []int{1, 2, 3, 4, 5}
// Double each number in the list
mappedData := mapData(data, func(x int) int {
return x * 2
})
fmt.Println("Mapped data:", mappedData)
}
This example demonstrates how to map over a slice of data and apply a transformation to each element, doubling the numbers in this case.
6. Working with Data Streams in Go
Data streams are continuous sequences of data that can be read in chunks. Go provides a variety of tools for working with streams, including the io.Reader
and io.Writer
interfaces. You can process data in chunks to handle large datasets efficiently without loading everything into memory.
6.1 Example: Reading Data Stream from a URL
Here’s an example of reading data from a URL using Go’s http
package:
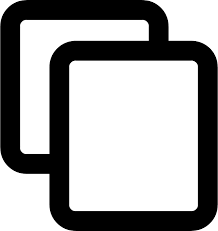
package main
import (
"fmt"
"io"
"net/http"
)
func main() {
// Make a GET request to fetch data from a URL
resp, err := http.Get("https://example.com/data")
if err != nil {
fmt.Println("Error fetching data:", err)
return
}
defer resp.Body.Close()
// Read data in chunks
buf := make([]byte, 1024) // Buffer size of 1 KB
for {
n, err := resp.Body.Read(buf)
if err == io.EOF {
break
}
if err != nil {
fmt.Println("Error reading data:", err)
return
}
fmt.Print(string(buf[:n])) // Print the chunk of data
}
}
In this example, we make an HTTP GET request to fetch data from a URL and process the response body in chunks using a buffer.
7. Conclusion
Go is a great choice for data processing due to its performance, concurrency model, and simplicity. Whether you're working with files, databases, streams, or APIs, Go provides a rich ecosystem of tools to handle data efficiently. By leveraging Go’s concurrency model with goroutines and channels, you can easily scale your data processing tasks and build high-performance pipelines for large datasets.
Integrating Go with Pandas and NumPy (via Go bindings)
1. Introduction to Go with Pandas and NumPy
Pandas and NumPy are two of the most widely used libraries in Python for data manipulation and numerical computations, respectively. While Go does not have native libraries for these tasks, integrating Go with Pandas and NumPy can be achieved using Go bindings for Python. This allows developers to leverage the power of Go for performance-critical tasks while still utilizing the rich functionality of Pandas and NumPy for data analysis and manipulation.
In this section, we will explore how to set up Go bindings for Pandas and NumPy, enabling you to use these libraries within Go applications for data processing and numerical computations.
2. Why Integrate Go with Pandas and NumPy?
- Performance: Go is a statically typed, compiled language known for its excellent performance, concurrency capabilities, and ease of use for large-scale applications. By integrating Go with Python’s powerful data libraries, you can speed up critical sections of your code while taking advantage of Go’s concurrency model.
- Ease of Use: Pandas and NumPy offer rich APIs for handling structured and numerical data, which can be cumbersome to replicate in Go. With bindings, you don’t need to reinvent the wheel and can access these libraries directly from Go.
- Parallel Processing: Go’s goroutines and Python’s data libraries can work in tandem to efficiently parallelize computations, making Go an ideal choice for handling large datasets while leveraging Python’s advanced data manipulation tools.
3. Setting Up Go Bindings for Pandas and NumPy
To integrate Go with Pandas and NumPy, you need to use the Go bindings for NumPy and the go-python3 package for interacting with Python in Go. These bindings allow you to call Python functions directly from Go.
3.1 Installing Go-Python3
You can install the go-python3
package to enable Go to interact with Python:
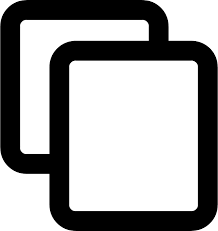
go get github.com/go-python/gopy
After installation, you can start writing Go code that calls Python functions using these bindings.
3.2 Installing NumPy and Pandas in Python
First, you need to ensure that NumPy and Pandas are installed in your Python environment:
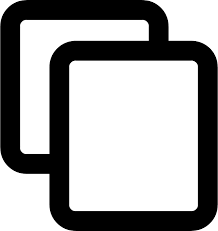
pip install numpy pandas
4. Example: Using NumPy in Go
Let’s start by demonstrating how to use NumPy (via Python bindings) in Go. We will perform a simple computation such as creating an array and computing the mean of the array.
4.1 Go Code to Call NumPy
Here’s an example Go program that uses NumPy to perform a basic operation:
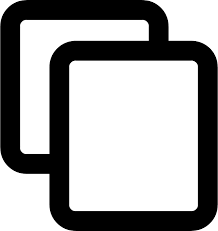
package main
import (
"fmt"
"log"
"github.com/go-python/gopy"
)
func main() {
// Initialize Python
err := gopy.Initialize()
if err != nil {
log.Fatal("Error initializing Python:", err)
}
defer gopy.Finalize()
// Import NumPy
np, err := gopy.Import("numpy")
if err != nil {
log.Fatal("Error importing NumPy:", err)
}
// Create a NumPy array
arr, err := np.Call("array", gopy.Args{gopy.NewList([]interface{}{1, 2, 3, 4, 5})})
if err != nil {
log.Fatal("Error creating NumPy array:", err)
}
// Compute the mean of the array
mean, err := np.Call("mean", gopy.Args{arr})
if err != nil {
log.Fatal("Error computing mean:", err)
}
// Print the result
fmt.Println("Mean of the array:", mean)
}
In this example, we initialize Python, import NumPy, create an array, and compute the mean using NumPy's mean
function. The result is printed in the Go program.
5. Example: Using Pandas in Go
Next, let’s see how we can use Pandas for data manipulation within Go. We will load a CSV file, compute the mean of a column, and filter the data.
5.1 Go Code to Call Pandas
Here’s a Go program that uses Pandas to load data from a CSV and perform operations:
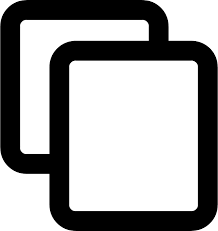
package main
import (
"fmt"
"log"
"github.com/go-python/gopy"
)
func main() {
// Initialize Python
err := gopy.Initialize()
if err != nil {
log.Fatal("Error initializing Python:", err)
}
defer gopy.Finalize()
// Import Pandas
pd, err := gopy.Import("pandas")
if err != nil {
log.Fatal("Error importing Pandas:", err)
}
// Load a CSV file into a DataFrame
df, err := pd.Call("read_csv", gopy.Args{"data.csv"})
if err != nil {
log.Fatal("Error loading CSV:", err)
}
// Compute the mean of a column
mean, err := pd.Call("mean", gopy.Args{df.GetItem("column_name")})
if err != nil {
log.Fatal("Error computing mean:", err)
}
// Print the result
fmt.Println("Mean of column_name:", mean)
}
In this example, we use Pandas to load a CSV file into a DataFrame, compute the mean of a specific column, and print the result. The CSV file should be located in the same directory as the Go program.
6. Performance Considerations
While integrating Go with Pandas and NumPy is convenient, there are some performance considerations:
- Python-Golang Overhead: Calling Python functions from Go introduces some overhead. For high-performance applications, this overhead may impact performance, so use this approach when the computational workload requires Pandas/NumPy functionality.
- Concurrency: Go’s goroutines can run concurrently with Python code, which allows you to process multiple data streams in parallel. However, keep in mind that concurrency in Go does not directly translate to Python’s multithreading or multiprocessing capabilities.
- Memory Management: When working with large datasets, ensure that you manage memory efficiently in both Go and Python, as memory usage can increase with large datasets.
7. Conclusion
Integrating Go with Pandas and NumPy via Go bindings allows you to use the strengths of both languages. You can leverage Go’s performance and concurrency for handling large datasets and use Python’s powerful data manipulation libraries for analysis and transformation. This integration enables the development of efficient, high-performance data processing pipelines that combine the best of both worlds.
Data Serialization with Go (JSON, Protobuf)
1. Introduction to Data Serialization
Data serialization is the process of converting data structures or objects into a format that can be easily stored, transmitted, or reconstructed later. In Go, the two most common methods of data serialization are JSON (JavaScript Object Notation) and Protobuf (Protocol Buffers). JSON is widely used for web APIs and data interchange, while Protobuf is a more compact and efficient format, commonly used for high-performance applications and communication between services.
In this section, we will explore how to serialize and deserialize data in Go using both JSON and Protobuf, comparing their use cases and benefits.
2. JSON Serialization in Go
JSON is a lightweight data-interchange format that is easy to read and write for humans and machines. Go provides a standard library package, encoding/json
, for working with JSON data. You can use it to convert Go structs into JSON and vice versa.
2.1 Encoding and Decoding JSON in Go
To encode data into JSON, you can use the json.Marshal()
function. To decode JSON into Go structs, you can use the json.Unmarshal()
function.
2.1.1 Example: Encoding and Decoding JSON
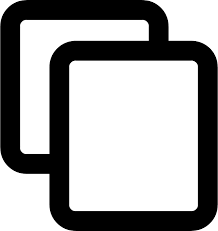
package main
import (
"encoding/json"
"fmt"
"log"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
// Create an instance of Person
p := Person{Name: "John Doe", Age: 30}
// Encode Person to JSON
jsonData, err := json.Marshal(p)
if err != nil {
log.Fatal("Error encoding JSON:", err)
}
fmt.Println("JSON:", string(jsonData))
// Decode JSON back into Person
var pDecoded Person
err = json.Unmarshal(jsonData, &pDecoded)
if err != nil {
log.Fatal("Error decoding JSON:", err)
}
fmt.Println("Decoded Person:", pDecoded)
}
In this example, we define a Person
struct, encode it into JSON using json.Marshal()
, and then decode it back into a struct using json.Unmarshal()
. The tags in the struct (e.g., json:"name"
) specify the JSON keys when encoding and decoding.
2.2 JSON with Nested Structures
Go handles nested structures in JSON seamlessly. You can define Go structs with nested structs, and encoding/json
will handle the serialization and deserialization accordingly.
2.2.1 Example: Nested Structures
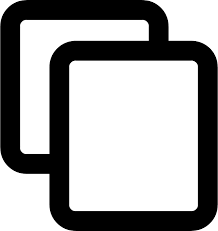
package main
import (
"encoding/json"
"fmt"
"log"
)
type Address struct {
Street string `json:"street"`
City string `json:"city"`
}
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Address Address `json:"address"`
}
func main() {
// Create an instance of Person with a nested Address
p := Person{Name: "John Doe", Age: 30, Address: Address{Street: "123 Main St", City: "Anytown"}}
// Encode Person to JSON
jsonData, err := json.Marshal(p)
if err != nil {
log.Fatal("Error encoding JSON:", err)
}
fmt.Println("JSON:", string(jsonData))
// Decode JSON back into Person
var pDecoded Person
err = json.Unmarshal(jsonData, &pDecoded)
if err != nil {
log.Fatal("Error decoding JSON:", err)
}
fmt.Println("Decoded Person:", pDecoded)
}
3. Protobuf Serialization in Go
Protocol Buffers (Protobuf) is a language-neutral, platform-neutral, extensible way of serializing structured data. Protobuf is known for its efficiency in both size and speed when compared to JSON. It is often used in RPC systems and high-performance distributed systems.
To work with Protobuf in Go, you need to use the google.golang.org/protobuf
package, which provides support for generating Go code from Protobuf definitions and serializing and deserializing Protobuf messages.
3.1 Defining Protobuf Messages
Protobuf messages are defined in a .proto file. A basic Protobuf message definition might look like this:
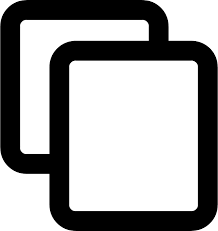
syntax = "proto3";
package main;
message Person {
string name = 1;
int32 age = 2;
}
In this example, we define a Person
message with two fields: name
and age
.
3.2 Generating Go Code from Protobuf
Once you’ve defined your Protobuf messages in a .proto file, you need to generate Go code using the protoc
compiler. You can install protoc
and the Go plugin with the following commands:
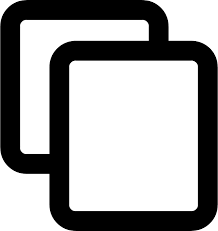
# Install the Protobuf compiler
brew install protobuf
# Install the Go plugin for Protobuf
go install google.golang.org/protobuf/cmd/protoc-gen-go@latest
Then, you can generate the Go code:
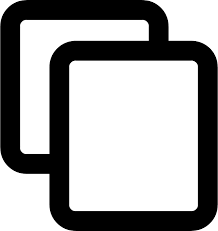
protoc --go_out=. person.proto
This will generate a person.pb.go
file containing Go structs and methods for your Protobuf messages.
3.3 Serializing and Deserializing Protobuf in Go
Once you’ve generated Go code from your Protobuf definitions, you can serialize and deserialize Protobuf messages using the proto.Marshal()
and proto.Unmarshal()
functions.
3.3.1 Example: Protobuf in Go
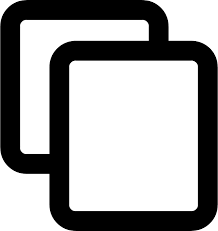
package main
import (
"fmt"
"log"
"google.golang.org/protobuf/proto"
)
type Person struct {
Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"`
Age int32 `protobuf:"varint,2,opt,name=age,proto3" json:"age,omitempty"`
}
func main() {
// Create an instance of Person
p := &Person{Name: "John Doe", Age: 30}
// Serialize Person to Protobuf
data, err := proto.Marshal(p)
if err != nil {
log.Fatal("Error serializing Protobuf:", err)
}
fmt.Println("Protobuf Data:", data)
// Deserialize Protobuf back into Person
var pDecoded Person
err = proto.Unmarshal(data, &pDecoded)
if err != nil {
log.Fatal("Error deserializing Protobuf:", err)
}
fmt.Println("Decoded Person:", pDecoded)
}
In this example, we create a Person
struct, serialize it into Protobuf format using proto.Marshal()
, and then deserialize it back into a struct using proto.Unmarshal()
.
4. Comparison of JSON and Protobuf
- JSON: Human-readable, flexible, and widely supported in web APIs. However, it is less efficient in terms of size and speed compared to Protobuf.
- Protobuf: Compact, faster, and more efficient for large-scale data transfer. It requires pre-compilation and is not human-readable, making it less suitable for debugging and direct inspection.
Choose JSON when you need easy interoperability and readability, and choose Protobuf when you require high performance and efficiency in data serialization.
5. Conclusion
Data serialization in Go can be achieved using multiple methods, with JSON and Protobuf being the most common. JSON is ideal for human-readable data exchange, while Protobuf excels in performance and compactness. By understanding and using these serialization techniques, you can efficiently handle data transfer and storage in Go applications, choosing the right method based on your specific use case.
Go and Machine Learning Libraries (Gorgonia)
1. Introduction to Gorgonia
Gorgonia is a powerful machine learning library for Go that facilitates the creation of neural networks and other machine learning models. It provides tools for tensor operations, graph-based computation, and automatic differentiation, making it suitable for a wide range of machine learning applications.
Gorgonia is often compared to frameworks like TensorFlow and PyTorch but is specifically tailored for Go developers. It allows you to define and evaluate mathematical expressions and train machine learning models directly within Go applications.
2. Installing Gorgonia
To get started with Gorgonia, install it using go get
:
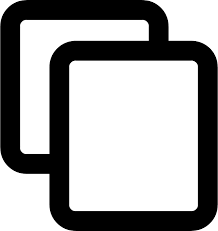
go get -u gorgonia.org/gorgonia
This command will download and install the Gorgonia library along with its dependencies.
3. Basic Concepts in Gorgonia
Before diving into examples, it’s essential to understand some of the core concepts in Gorgonia:
- Tensors: Multi-dimensional arrays used for data representation.
- Graphs: A computation graph represents mathematical operations and dependencies.
- Nodes: Elements of the computation graph that hold tensors or represent operations.
- Automatic Differentiation: Gorgonia supports computing gradients automatically, crucial for training machine learning models.
4. Example: Basic Tensor Operations
Let’s start with a simple example of creating tensors and performing basic operations:
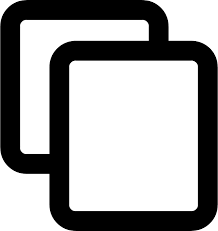
package main
import (
"fmt"
"log"
"gorgonia.org/gorgonia"
"gorgonia.org/tensor"
)
func main() {
// Create a computation graph
g := gorgonia.NewGraph()
// Define two tensors
a := gorgonia.NewTensor(g, tensor.Float32, 2, gorgonia.WithShape(2, 2), gorgonia.WithValue(tensor.New(tensor.WithBacking([]float32{1, 2, 3, 4}), tensor.WithShape(2, 2))))
b := gorgonia.NewTensor(g, tensor.Float32, 2, gorgonia.WithShape(2, 2), gorgonia.WithValue(tensor.New(tensor.WithBacking([]float32{5, 6, 7, 8}), tensor.WithShape(2, 2))))
// Perform matrix multiplication
c, err := gorgonia.Mul(a, b)
if err != nil {
log.Fatal(err)
}
// Create a VM to execute the graph
machine := gorgonia.NewTapeMachine(g)
defer machine.Close()
// Execute the graph
if err := machine.RunAll(); err != nil {
log.Fatal(err)
}
// Print the result
fmt.Println("Result:", c.Value())
}
In this example, we define two tensors and perform matrix multiplication using the Mul
function. The result is calculated by executing the computation graph.
5. Example: Building a Simple Neural Network
Next, we’ll build a simple neural network for binary classification using Gorgonia:
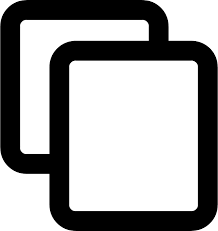
package main
import (
"fmt"
"log"
"gorgonia.org/gorgonia"
"gorgonia.org/tensor"
)
func main() {
// Create a computation graph
g := gorgonia.NewGraph()
// Define input and weights
x := gorgonia.NewMatrix(g, tensor.Float32, gorgonia.WithShape(2, 2), gorgonia.WithValue(tensor.New(tensor.WithBacking([]float32{1, 0, 0, 1}), tensor.WithShape(2, 2))))
w := gorgonia.NewMatrix(g, tensor.Float32, gorgonia.WithShape(2, 1), gorgonia.WithInit(gorgonia.GlorotU(1)))
// Compute output: y = sigmoid(x * w)
dot, err := gorgonia.Mul(x, w)
if err != nil {
log.Fatal(err)
}
y, err := gorgonia.Sigmoid(dot)
if err != nil {
log.Fatal(err)
}
// Define a target and loss function
target := gorgonia.NewMatrix(g, tensor.Float32, gorgonia.WithShape(2, 1), gorgonia.WithValue(tensor.New(tensor.WithBacking([]float32{1, 0}), tensor.WithShape(2, 1))))
loss := gorgonia.Must(gorgonia.Mean(gorgonia.Must(gorgonia.Square(gorgonia.Must(gorgonia.Sub(y, target))))))
// Compute gradients
if _, err := gorgonia.Grad(loss, w); err != nil {
log.Fatal(err)
}
// Create a VM and train the model
machine := gorgonia.NewTapeMachine(g)
defer machine.Close()
for i := 0; i < 100; i++ {
if err := machine.RunAll(); err != nil {
log.Fatal(err)
}
machine.Reset()
fmt.Printf("Iteration %d: Loss = %v\n", i+1, loss.Value())
}
}
This example demonstrates creating a simple neural network with input, weights, and a sigmoid activation function. The model is trained using gradient descent to minimize the loss function.
6. Advantages of Using Gorgonia
- Native support for Go, enabling seamless integration into Go applications.
- Powerful tensor operations and automatic differentiation.
- Flexibility to build custom machine learning models.
7. Limitations
- Smaller community and fewer prebuilt models compared to Python libraries like TensorFlow and PyTorch.
- Requires more effort to implement complex models.
8. Conclusion
Gorgonia is a robust library for implementing machine learning models in Go. Its native integration with Go makes it an excellent choice for developers looking to add machine learning capabilities to their Go applications. While it may not yet match the ecosystem of Python-based frameworks, it provides sufficient tools for building and training models in a Go environment.
Performing Statistical Analysis in Go
1. Introduction to Statistical Analysis in Go
Statistical analysis is a critical component of data science and analytics. With Go's focus on performance and scalability, it is well-suited for implementing statistical computations. Go offers several libraries to perform statistical analysis, such as gonum
, stats
, and goml
.
These libraries provide built-in functions for descriptive statistics, probability distributions, hypothesis testing, and more.
2. Popular Libraries for Statistical Analysis
Here are some popular Go libraries for statistical analysis:
- Gonum: A comprehensive library for numerical and scientific computations.
- Stats: A lightweight library offering basic statistical functions.
- GoML: A library for machine learning and statistics.
3. Example: Basic Descriptive Statistics
The stats
library simplifies common statistical operations. Install it using:
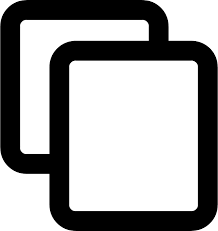
go get -u github.com/montanaflynn/stats
Here’s an example of computing mean, median, and standard deviation:
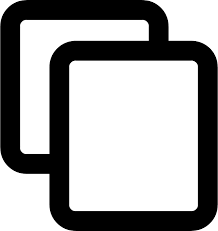
package main
import (
"fmt"
"log"
"github.com/montanaflynn/stats"
)
func main() {
// Sample data
data := stats.Float64Data{1.5, 2.3, 3.1, 4.7, 5.0}
// Calculate mean
mean, err := stats.Mean(data)
if err != nil {
log.Fatal(err)
}
fmt.Println("Mean:", mean)
// Calculate median
median, err := stats.Median(data)
if err != nil {
log.Fatal(err)
}
fmt.Println("Median:", median)
// Calculate standard deviation
stdDev, err := stats.StandardDeviation(data)
if err != nil {
log.Fatal(err)
}
fmt.Println("Standard Deviation:", stdDev)
}
4. Example: Probability Distributions with Gonum
The Gonum library supports various probability distributions. Install it using:
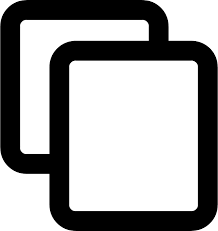
go get -u gonum.org/v1/gonum/stat
Here’s an example of generating random numbers from a normal distribution:
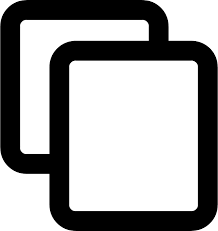
package main
import (
"fmt"
"math/rand"
"time"
"gonum.org/v1/gonum/stat/distuv"
)
func main() {
// Seed random number generator
rand.Seed(time.Now().UnixNano())
// Create a normal distribution with mean=0 and stdDev=1
normal := distuv.Normal{
Mu: 0,
Sigma: 1,
}
// Generate 5 random numbers
for i := 0; i < 5; i++ {
fmt.Println("Random Value:", normal.Rand())
}
// Calculate the probability density for x=1.0
probDensity := normal.Prob(1.0)
fmt.Println("Probability Density at x=1.0:", probDensity)
}
5. Example: Linear Regression
The Gonum library also supports linear regression:
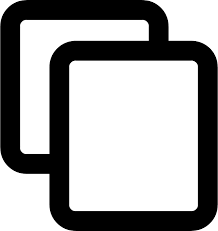
package main
import (
"fmt"
"gonum.org/v1/gonum/stat"
)
func main() {
// Data points
x := []float64{1, 2, 3, 4, 5}
y := []float64{2, 4, 5, 4, 5}
// Perform linear regression
alpha, beta := stat.LinearRegression(x, y, nil, false)
fmt.Printf("Linear Regression:\nIntercept (α): %v\nSlope (β): %v\n", alpha, beta)
}
6. Advanced Statistical Techniques
For more advanced techniques, such as hypothesis testing, ANOVA, or clustering, you can explore libraries like goml
or integrate Go with Python libraries using cgo
or go-python
.
7. Advantages of Statistical Analysis in Go
- High performance and efficient computation.
- Strong concurrency support for parallel processing.
- Ease of integration with other Go-based systems.
8. Limitations
- Smaller ecosystem compared to Python's statistics and machine learning libraries.
- Limited prebuilt solutions for specialized statistical methods.
9. Conclusion
Go provides powerful tools for performing statistical analysis, especially for applications requiring high performance and scalability. While its ecosystem is not as extensive as Python's, libraries like Gonum and Stats offer robust functionality for most statistical needs. With Go, you can seamlessly integrate statistical computations into larger systems, making it a valuable language for data analysis and processing.
Introduction to Database Handling in Go
1. Overview
Database handling is a critical part of building modern applications. Go provides robust support for interacting with databases through its standard library and third-party packages. The database/sql
package in Go serves as a generic interface for SQL databases, while libraries such as gorm
and sqlx
offer higher-level abstractions.
2. Popular Libraries for Database Handling
Here are some commonly used libraries for database handling in Go:
database/sql
: A standard library package for SQL database interactions.GORM
: An ORM (Object-Relational Mapper) for Go with powerful features and abstractions.sqlx
: An extension todatabase/sql
for simplifying queries and struct mapping.go-pg
: A PostgreSQL client and ORM for Go.
3. Installing Database Drivers
To interact with a specific database, you need to install its driver. Examples:
- MySQL:
go get -u github.com/go-sql-driver/mysql
- PostgreSQL:
go get -u github.com/lib/pq
- SQLite:
go get -u modernc.org/sqlite
4. Connecting to a Database
Using the database/sql
package, you can establish a connection to a database:
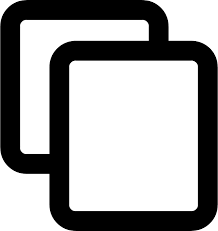
package main
import (
"database/sql"
"fmt"
"log"
_ "github.com/go-sql-driver/mysql" // Import MySQL driver
)
func main() {
// Define the data source name (DSN)
dsn := "username:password@tcp(127.0.0.1:3306)/dbname"
// Open a database connection
db, err := sql.Open("mysql", dsn)
if err != nil {
log.Fatal(err)
}
defer db.Close()
// Test the connection
err = db.Ping()
if err != nil {
log.Fatal(err)
}
fmt.Println("Connected to the database successfully!")
}
5. Executing Queries
Here’s how you can execute a query and fetch results:
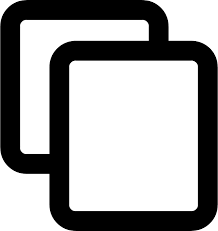
package main
import (
"database/sql"
"fmt"
"log"
_ "github.com/go-sql-driver/mysql"
)
func main() {
dsn := "username:password@tcp(127.0.0.1:3306)/dbname"
db, err := sql.Open("mysql", dsn)
if err != nil {
log.Fatal(err)
}
defer db.Close()
// Query the database
rows, err := db.Query("SELECT id, name FROM users")
if err != nil {
log.Fatal(err)
}
defer rows.Close()
// Iterate through the results
for rows.Next() {
var id int
var name string
err := rows.Scan(&id, &name)
if err != nil {
log.Fatal(err)
}
fmt.Printf("ID: %d, Name: %s\n", id, name)
}
}
6. Using GORM for Simplified Database Handling
GORM provides an ORM-like interface for interacting with databases. Install it using:
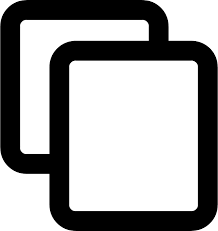
go get -u gorm.io/gorm
go get -u gorm.io/driver/mysql
Example with GORM:
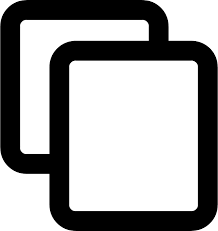
package main
import (
"fmt"
"log"
"gorm.io/driver/mysql"
"gorm.io/gorm"
)
type User struct {
ID uint `gorm:"primaryKey"`
Name string `gorm:"size:255"`
}
func main() {
dsn := "username:password@tcp(127.0.0.1:3306)/dbname"
db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{})
if err != nil {
log.Fatal(err)
}
// Auto-migrate schema
db.AutoMigrate(&User{})
// Create a new user
newUser := User{Name: "Alice"}
db.Create(&newUser)
// Fetch all users
var users []User
db.Find(&users)
for _, user := range users {
fmt.Printf("ID: %d, Name: %s\n", user.ID, user.Name)
}
}
7. Best Practices
- Use connection pooling to optimize performance.
- Close database connections using
defer
to prevent resource leaks. - Use prepared statements to prevent SQL injection.
- Log and handle errors effectively.
8. Conclusion
Go offers versatile tools for database handling, from the standard database/sql
package to advanced ORMs like GORM. Whether you're building a simple application or a complex data-driven system, Go provides the flexibility and performance needed to manage your database interactions effectively.
Connecting to MySQL and PostgreSQL in Go
1. Overview
Go provides robust support for connecting to SQL databases like MySQL and PostgreSQL using the database/sql
package and third-party drivers. This guide demonstrates how to connect to these databases, execute queries, and handle common tasks.
2. Prerequisites
- Go installed on your machine.
- A running instance of MySQL or PostgreSQL.
- Database credentials (username, password, host, port, database name).
3. Installing Drivers
Before connecting to a database, install the appropriate driver:
- MySQL:
go get -u github.com/go-sql-driver/mysql
- PostgreSQL:
go get -u github.com/lib/pq
4. Connecting to MySQL
Below is a sample program to connect to a MySQL database and execute a simple query:
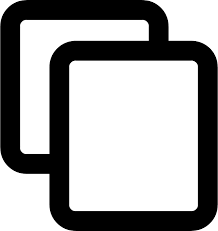
package main
import (
"database/sql"
"fmt"
"log"
_ "github.com/go-sql-driver/mysql" // Import MySQL driver
)
func main() {
// Define the data source name (DSN)
dsn := "username:password@tcp(127.0.0.1:3306)/dbname"
// Open a database connection
db, err := sql.Open("mysql", dsn)
if err != nil {
log.Fatalf("Error opening database: %v", err)
}
defer db.Close()
// Test the connection
err = db.Ping()
if err != nil {
log.Fatalf("Error pinging database: %v", err)
}
fmt.Println("Successfully connected to MySQL database!")
// Execute a query
query := "SELECT id, name FROM users"
rows, err := db.Query(query)
if err != nil {
log.Fatalf("Error executing query: %v", err)
}
defer rows.Close()
// Iterate through results
for rows.Next() {
var id int
var name string
err := rows.Scan(&id, &name)
if err != nil {
log.Fatalf("Error scanning row: %v", err)
}
fmt.Printf("ID: %d, Name: %s\n", id, name)
}
}
5. Connecting to PostgreSQL
Here’s a similar example for connecting to a PostgreSQL database:
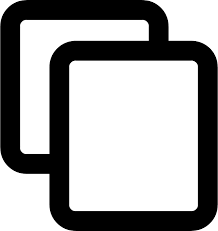
package main
import (
"database/sql"
"fmt"
"log"
_ "github.com/lib/pq" // Import PostgreSQL driver
)
func main() {
// Define the connection string
connStr := "postgres://username:password@localhost:5432/dbname?sslmode=disable"
// Open a database connection
db, err := sql.Open("postgres", connStr)
if err != nil {
log.Fatalf("Error opening database: %v", err)
}
defer db.Close()
// Test the connection
err = db.Ping()
if err != nil {
log.Fatalf("Error pinging database: %v", err)
}
fmt.Println("Successfully connected to PostgreSQL database!")
// Execute a query
query := "SELECT id, name FROM users"
rows, err := db.Query(query)
if err != nil {
log.Fatalf("Error executing query: %v", err)
}
defer rows.Close()
// Iterate through results
for rows.Next() {
var id int
var name string
err := rows.Scan(&id, &name)
if err != nil {
log.Fatalf("Error scanning row: %v", err)
}
fmt.Printf("ID: %d, Name: %s\n", id, name)
}
}
6. Common Tasks
- Connection String: Ensure the connection string format matches the database and driver documentation.
- Connection Pooling: Use
db.SetMaxOpenConns
anddb.SetMaxIdleConns
to manage connections efficiently. - Prepared Statements: Use
db.Prepare
for executing repeated queries securely. - Error Handling: Always check for errors and handle them appropriately.
7. Best Practices
- Use environment variables to store sensitive information such as database credentials.
- Close database connections with
defer
to avoid resource leaks. - Validate inputs to prevent SQL injection vulnerabilities.
- Log and monitor query execution times for performance optimization.
8. Conclusion
Connecting to MySQL and PostgreSQL in Go is straightforward with the database/sql
package and the respective drivers. By following best practices and leveraging Go's robust database support, you can build reliable and efficient applications that interact with your databases seamlessly.
Performing CRUD Operations in Go
1. Overview
CRUD operations (Create, Read, Update, Delete) are fundamental in building applications that interact with databases. In Go, these operations can be efficiently performed using the database/sql
package and appropriate database drivers (e.g., MySQL, PostgreSQL).
2. Prerequisites
- Go installed on your machine.
- A database instance (MySQL or PostgreSQL) with a table for demonstration.
- Basic knowledge of SQL queries.
3. Setting Up
First, install the database driver:
- MySQL:
go get -u github.com/go-sql-driver/mysql
- PostgreSQL:
go get -u github.com/lib/pq
Below, we use a sample table named users
:
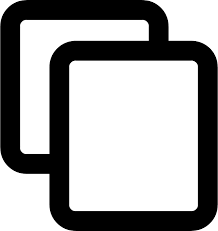
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100),
age INT
);
4. Connecting to the Database
Set up a connection to the database:
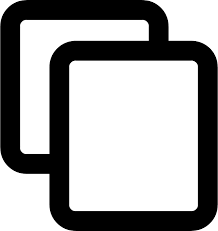
package main
import (
"database/sql"
"log"
_ "github.com/go-sql-driver/mysql" // For MySQL
// _ "github.com/lib/pq" // For PostgreSQL
)
func connectToDB() *sql.DB {
dsn := "username:password@tcp(127.0.0.1:3306)/dbname" // MySQL DSN
// dsn := "postgres://username:password@localhost:5432/dbname?sslmode=disable" // PostgreSQL DSN
db, err := sql.Open("mysql", dsn) // Use "postgres" for PostgreSQL
if err != nil {
log.Fatalf("Error connecting to database: %v", err)
}
return db
}
5. Create Operation
Insert a new record into the database:
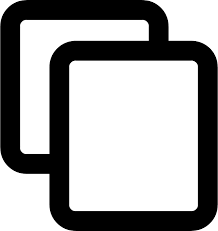
func createUser(db *sql.DB, name, email string, age int) {
query := "INSERT INTO users (name, email, age) VALUES (?, ?, ?)" // Use $1, $2, $3 for PostgreSQL
_, err := db.Exec(query, name, email, age)
if err != nil {
log.Fatalf("Error inserting user: %v", err)
}
log.Println("User created successfully!")
}
6. Read Operation
Retrieve records from the database:
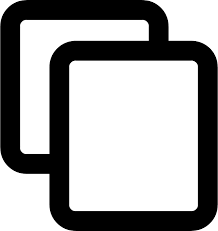
func getUsers(db *sql.DB) {
query := "SELECT id, name, email, age FROM users"
rows, err := db.Query(query)
if err != nil {
log.Fatalf("Error fetching users: %v", err)
}
defer rows.Close()
for rows.Next() {
var id int
var name, email string
var age int
err := rows.Scan(&id, &name, &email, &age)
if err != nil {
log.Fatalf("Error scanning row: %v", err)
}
log.Printf("ID: %d, Name: %s, Email: %s, Age: %d\n", id, name, email, age)
}
}
7. Update Operation
Modify an existing record:
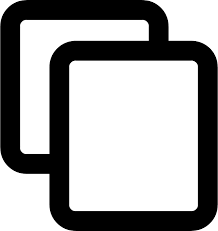
func updateUser(db *sql.DB, id int, newEmail string) {
query := "UPDATE users SET email = ? WHERE id = ?" // Use $1, $2 for PostgreSQL
_, err := db.Exec(query, newEmail, id)
if err != nil {
log.Fatalf("Error updating user: %v", err)
}
log.Println("User updated successfully!")
}
8. Delete Operation
Remove a record from the database:
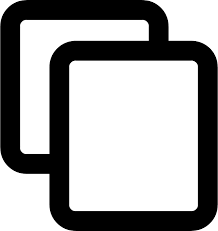
func deleteUser(db *sql.DB, id int) {
query := "DELETE FROM users WHERE id = ?" // Use $1 for PostgreSQL
_, err := db.Exec(query, id)
if err != nil {
log.Fatalf("Error deleting user: %v", err)
}
log.Println("User deleted successfully!")
}
9. Main Function
Combine these operations in the main
function:
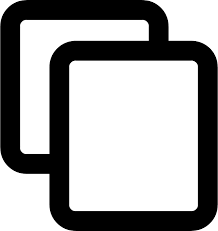
func main() {
db := connectToDB()
defer db.Close()
createUser(db, "John Doe", "john.doe@example.com", 30)
getUsers(db)
updateUser(db, 1, "john.new@example.com")
deleteUser(db, 1)
}
10. Best Practices
- Use prepared statements (
db.Prepare
) for dynamic queries to prevent SQL injection. - Handle database errors gracefully and log them for debugging.
- Close rows (
rows.Close()
) and statements (stmt.Close()
) to release resources. - Use transactions for operations that require atomicity.
- Leverage connection pooling (
db.SetMaxOpenConns
,db.SetMaxIdleConns
).
11. Conclusion
With the database/sql
package, performing CRUD operations in Go is straightforward and efficient. By following best practices, you can ensure reliable and secure database interactions in your applications.
Using ORM (Object-Relational Mapping) with Go (e.g., GORM)
1. Overview
Object-Relational Mapping (ORM) simplifies database interactions by mapping database tables to Go structs. GORM is a popular ORM library for Go that provides powerful tools for managing databases with less boilerplate code.
2. Prerequisites
- Go installed on your machine.
- A database instance (MySQL, PostgreSQL, etc.).
- Basic knowledge of Go and SQL.
3. Installing GORM
Install GORM and the respective database driver:
- For MySQL:
go get -u gorm.io/gorm gorm.io/driver/mysql
- For PostgreSQL:
go get -u gorm.io/gorm gorm.io/driver/postgres
4. Database Connection
Set up a database connection using GORM:
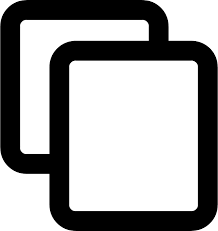
package main
import (
"log"
"gorm.io/driver/mysql" // Use "gorm.io/driver/postgres" for PostgreSQL
"gorm.io/gorm"
)
func connectToDB() *gorm.DB {
dsn := "username:password@tcp(127.0.0.1:3306)/dbname?charset=utf8mb4&parseTime=True&loc=Local" // MySQL DSN
// dsn := "host=localhost user=username password=password dbname=dbname port=5432 sslmode=disable TimeZone=Asia/Shanghai" // PostgreSQL DSN
db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) // Use postgres.Open(dsn) for PostgreSQL
if err != nil {
log.Fatalf("Error connecting to database: %v", err)
}
return db
}
5. Defining Models
Define a Go struct to represent a database table:
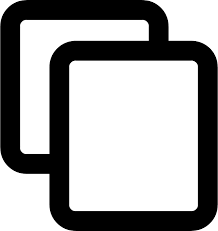
type User struct {
ID uint `gorm:"primaryKey"`
Name string `gorm:"size:100"`
Email string `gorm:"uniqueIndex;size:100"`
Age int
}
To auto-create the table, use GORM’s AutoMigrate
:
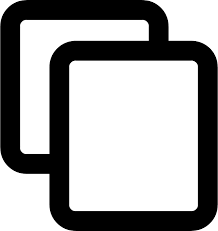
func autoMigrate(db *gorm.DB) {
err := db.AutoMigrate(&User{})
if err != nil {
log.Fatalf("Error in auto migration: %v", err)
}
}
6. Create Operation
Insert a new record using GORM:
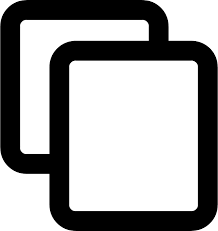
func createUser(db *gorm.DB, user User) {
result := db.Create(&user)
if result.Error != nil {
log.Fatalf("Error creating user: %v", result.Error)
}
log.Println("User created successfully!")
}
7. Read Operation
Retrieve records using GORM:
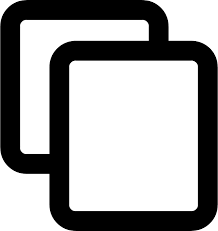
func getUsers(db *gorm.DB) {
var users []User
result := db.Find(&users)
if result.Error != nil {
log.Fatalf("Error fetching users: %v", result.Error)
}
for _, user := range users {
log.Printf("ID: %d, Name: %s, Email: %s, Age: %d\n", user.ID, user.Name, user.Email, user.Age)
}
}
8. Update Operation
Update an existing record:
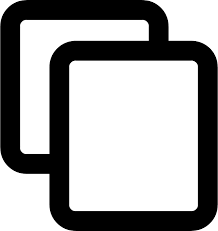
func updateUser(db *gorm.DB, id uint, newEmail string) {
result := db.Model(&User{}).Where("id = ?", id).Update("email", newEmail)
if result.Error != nil {
log.Fatalf("Error updating user: %v", result.Error)
}
log.Println("User updated successfully!")
}
9. Delete Operation
Delete a record from the database:
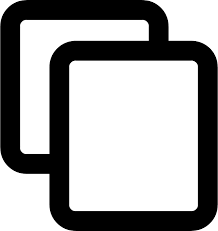
func deleteUser(db *gorm.DB, id uint) {
result := db.Delete(&User{}, id)
if result.Error != nil {
log.Fatalf("Error deleting user: %v", result.Error)
}
log.Println("User deleted successfully!")
}
10. Main Function
Integrate the operations in the main
function:
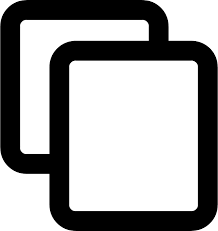
func main() {
db := connectToDB()
autoMigrate(db)
createUser(db, User{Name: "Alice", Email: "alice@example.com", Age: 25})
getUsers(db)
updateUser(db, 1, "alice.new@example.com")
deleteUser(db, 1)
}
11. Advantages of Using GORM
- Reduces boilerplate code for database operations.
- Supports multiple database types (MySQL, PostgreSQL, SQLite, etc.).
- Built-in features for relationships, migrations, and query building.
12. Best Practices
- Use GORM tags in struct definitions to specify constraints and indexes.
- Enable logging for debugging queries:
db.Logger = logger.Default.LogMode(logger.Info)
. - Handle transactions with
db.Transaction()
for atomicity. - Always check for errors in database operations.
13. Conclusion
GORM provides a powerful and user-friendly way to manage databases in Go. By leveraging its features, developers can build robust applications with minimal effort while maintaining flexibility and performance.
Transactions and Database Queries in Go
1. Overview
Transactions allow multiple database operations to be grouped together and executed as a single unit. If any operation in the transaction fails, all changes are rolled back, ensuring data integrity. Go provides robust support for transactions and queries using packages like database/sql
and ORMs like GORM.
2. Using Transactions with database/sql
Here's how to handle transactions using the database/sql
package:
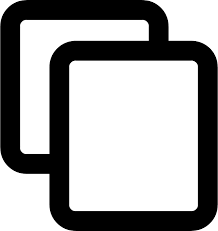
import (
"database/sql"
"log"
_ "github.com/go-sql-driver/mysql" // Replace with your database driver
)
func performTransaction(db *sql.DB) {
tx, err := db.Begin() // Start a transaction
if err != nil {
log.Fatalf("Error starting transaction: %v", err)
}
// Perform operations within the transaction
_, err = tx.Exec("INSERT INTO accounts (name, balance) VALUES (?, ?)", "Alice", 1000)
if err != nil {
tx.Rollback() // Rollback on error
log.Fatalf("Transaction failed: %v", err)
}
_, err = tx.Exec("UPDATE accounts SET balance = balance - ? WHERE name = ?", 500, "Alice")
if err != nil {
tx.Rollback() // Rollback on error
log.Fatalf("Transaction failed: %v", err)
}
// Commit the transaction
err = tx.Commit()
if err != nil {
log.Fatalf("Error committing transaction: %v", err)
}
log.Println("Transaction completed successfully!")
}
3. Using Transactions with GORM
GORM simplifies transaction handling with the Transaction
method:
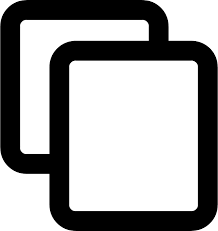
import (
"log"
"gorm.io/driver/mysql"
"gorm.io/gorm"
)
func performGORMTransaction(db *gorm.DB) {
err := db.Transaction(func(tx *gorm.DB) error {
// Insert operation
if err := tx.Create(&Account{Name: "Alice", Balance: 1000}).Error; err != nil {
return err // Rollback automatically
}
// Update operation
if err := tx.Model(&Account{}).Where("name = ?", "Alice").Update("balance", gorm.Expr("balance - ?", 500)).Error; err != nil {
return err // Rollback automatically
}
// Return nil to commit the transaction
return nil
})
if err != nil {
log.Fatalf("Transaction failed: %v", err)
} else {
log.Println("Transaction completed successfully!")
}
}
type Account struct {
ID uint
Name string
Balance float64
}
4. Writing Database Queries
In Go, you can write database queries using database/sql
or GORM.
4.1. Using database/sql
Write raw SQL queries to interact with the database:
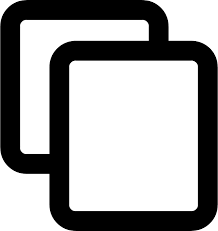
func fetchAccounts(db *sql.DB) {
rows, err := db.Query("SELECT id, name, balance FROM accounts")
if err != nil {
log.Fatalf("Error fetching accounts: %v", err)
}
defer rows.Close()
for rows.Next() {
var id int
var name string
var balance float64
if err := rows.Scan(&id, &name, &balance); err != nil {
log.Fatalf("Error scanning row: %v", err)
}
log.Printf("ID: %d, Name: %s, Balance: %.2f", id, name, balance)
}
}
4.2. Using GORM Queries
GORM provides methods for writing queries without raw SQL:
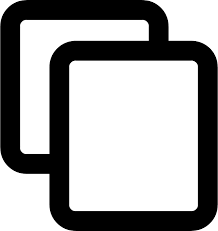
func fetchGORMAccounts(db *gorm.DB) {
var accounts []Account
result := db.Find(&accounts)
if result.Error != nil {
log.Fatalf("Error fetching accounts: %v", result.Error)
}
for _, account := range accounts {
log.Printf("ID: %d, Name: %s, Balance: %.2f", account.ID, account.Name, account.Balance)
}
}
5. Best Practices for Transactions and Queries
- Always handle errors during transactions and queries.
- Use transactions for operations that must be atomic (e.g., bank transfers).
- Close rows and statements to avoid resource leaks.
- Use parameterized queries to prevent SQL injection.
- Enable logging during development to debug database interactions.
6. Conclusion
Go provides powerful tools for managing database transactions and queries. Whether you use raw SQL with database/sql
or an ORM like GORM, understanding how to efficiently handle transactions and queries is essential for building reliable and robust applications.
Introduction to Go Security Best Practices
1. Overview
Security is a critical aspect of software development, and Go provides a variety of features and libraries to help developers build secure applications. By following best practices, you can minimize vulnerabilities and enhance the overall security of your application.
2. Input Validation
- Sanitize and Validate Inputs: Always validate user inputs to prevent injection attacks (e.g., SQL injection, command injection).
- Use Libraries: Use libraries like
validator
for input validation in Go.
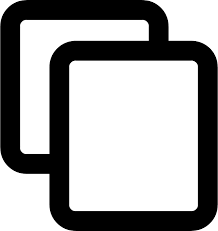
import "github.com/go-playground/validator/v10"
type User struct {
Email string `validate:"required,email"`
Age int `validate:"gte=18"`
}
func validateInput(user User) error {
validate := validator.New()
return validate.Struct(user)
}
3. Secure Data Storage
- Encrypt Sensitive Data: Use encryption libraries to secure sensitive data before storing it.
- Hash Passwords: Never store passwords in plain text. Use bcrypt for hashing.
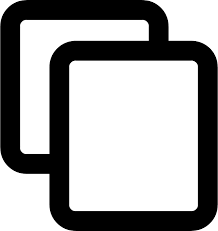
import (
"golang.org/x/crypto/bcrypt"
)
func hashPassword(password string) (string, error) {
hashed, err := bcrypt.GenerateFromPassword([]byte(password), bcrypt.DefaultCost)
return string(hashed), err
}
func checkPassword(hashedPassword, password string) error {
return bcrypt.CompareHashAndPassword([]byte(hashedPassword), []byte(password))
}
4. Avoid Hard-Coding Secrets
- Store sensitive information like API keys and database credentials in environment variables or secret management systems.
- Use packages like
os
or libraries likeviper
to manage configurations securely.
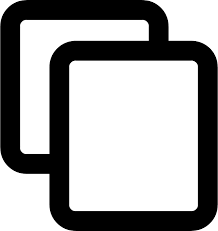
import "os"
func getDatabaseURL() string {
return os.Getenv("DATABASE_URL")
}
5. Secure Communications
- Use HTTPS: Encrypt communications using HTTPS with TLS certificates.
- Verify Certificates: Use libraries like
crypto/tls
to configure secure connections.
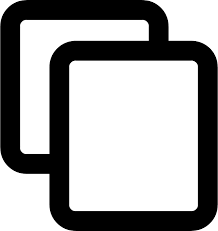
import (
"crypto/tls"
"net/http"
)
func createSecureClient() *http.Client {
tlsConfig := &tls.Config{
InsecureSkipVerify: false, // Ensure certificates are verified
}
transport := &http.Transport{TLSClientConfig: tlsConfig}
return &http.Client{Transport: transport}
}
6. Prevent Injection Attacks
- Use Parameterized Queries: Always use parameterized queries to prevent SQL injection.
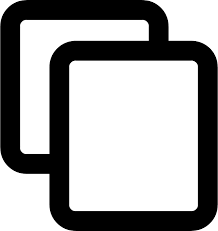
import "database/sql"
func fetchUserByID(db *sql.DB, id int) error {
query := "SELECT * FROM users WHERE id = ?"
row := db.QueryRow(query, id)
// Process row data...
return row.Err()
}
7. Secure Error Handling
- Avoid exposing sensitive information in error messages.
- Log errors securely and use proper logging libraries like
log
orzerolog
.
8. Use Static Analysis Tools
- Use tools like
gosec
to analyze your code for security vulnerabilities.
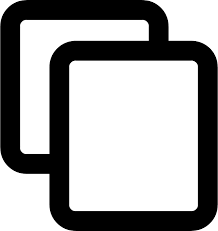
# Install gosec
go install github.com/securego/gosec/v2/cmd/gosec@latest
# Run gosec
gosec ./...
9. Implement Rate Limiting
Prevent abuse by implementing rate limiting for APIs. Libraries like golang.org/x/time/rate
can help with this.
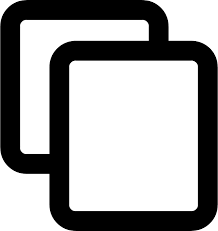
import (
"net/http"
"golang.org/x/time/rate"
)
var limiter = rate.NewLimiter(2, 5) // 2 requests per second, burst of 5
func rateLimitedHandler(w http.ResponseWriter, r *http.Request) {
if !limiter.Allow() {
http.Error(w, "Too Many Requests", http.StatusTooManyRequests)
return
}
w.Write([]byte("Request allowed"))
}
10. Conclusion
By following these best practices, you can build secure applications in Go. Regularly update dependencies, stay informed about new security vulnerabilities, and use tools to automate the detection of security issues.
Securing Web Applications with Go
1. Overview
Web applications are a common target for attackers, making security a top priority for developers. Go provides several tools and best practices to help secure web applications against common vulnerabilities such as XSS, CSRF, SQL injection, and more.
2. Secure HTTP Headers
- Set Security Headers: Use headers like
Content-Security-Policy
,X-Content-Type-Options
, andX-Frame-Options
to protect against attacks like clickjacking and MIME sniffing. - Use middleware to add these headers to every response.
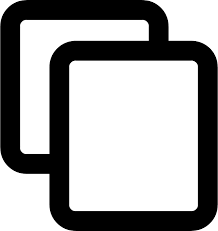
import "net/http"
func securityHeadersMiddleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Security-Policy", "default-src 'self'")
w.Header().Set("X-Content-Type-Options", "nosniff")
w.Header().Set("X-Frame-Options", "DENY")
next.ServeHTTP(w, r)
})
}
3. Preventing Cross-Site Scripting (XSS)
- Escape User Input: Use Go's
html/template
package to escape output automatically in templates. - Avoid including raw user input in JavaScript or HTML without proper encoding.
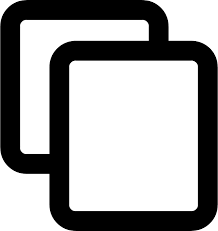
import "html/template"
var tmpl = template.Must(template.New("example").Parse(`
{{.Message}}
`))
func renderTemplate(w http.ResponseWriter, data interface{}) {
tmpl.Execute(w, data)
}
4. Preventing Cross-Site Request Forgery (CSRF)
- Use CSRF Tokens: Generate and validate CSRF tokens for form submissions.
- Use libraries like
gorilla/csrf
to simplify CSRF protection.
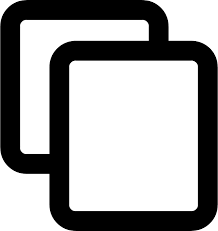
import (
"github.com/gorilla/csrf"
"github.com/gorilla/mux"
)
func main() {
r := mux.NewRouter()
csrfMiddleware := csrf.Protect([]byte("32-byte-long-auth-key"))
r.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Secure content"))
})
http.ListenAndServe(":8080", csrfMiddleware(r))
}
5. Authentication and Authorization
- Use Secure Authentication: Store hashed passwords using bcrypt and implement secure login mechanisms.
- Implement Role-Based Access Control (RBAC): Restrict access to resources based on user roles and permissions.
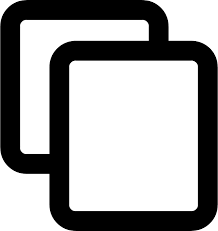
func checkUserRole(role string, allowedRoles []string) bool {
for _, r := range allowedRoles {
if r == role {
return true
}
}
return false
}
6. Preventing SQL Injection
Always use parameterized queries to prevent attackers from injecting malicious SQL code.
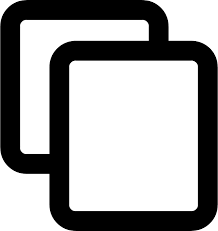
import "database/sql"
func getUser(db *sql.DB, username string) (*User, error) {
query := "SELECT id, name FROM users WHERE username = ?"
row := db.QueryRow(query, username)
var user User
err := row.Scan(&user.ID, &user.Name)
return &user, err
}
7. Secure Session Management
- Use secure session cookies with the
Secure
andHttpOnly
flags. - Use libraries like
gorilla/sessions
to manage sessions securely.
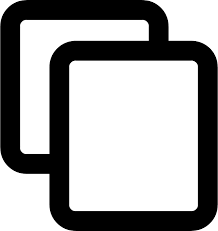
import "github.com/gorilla/sessions"
var store = sessions.NewCookieStore([]byte("your-secret-key"))
func setSession(w http.ResponseWriter, r *http.Request, key, value string) {
session, _ := store.Get(r, "session-name")
session.Values[key] = value
session.Save(r, w)
}
8. Rate Limiting
Prevent abuse by implementing rate limiting. This protects your application from brute force and DoS attacks.
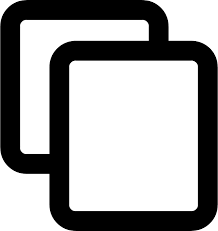
import (
"net/http"
"golang.org/x/time/rate"
)
var limiter = rate.NewLimiter(5, 10) // Allow 5 requests per second with a burst of 10
func rateLimitMiddleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
if !limiter.Allow() {
http.Error(w, "Too Many Requests", http.StatusTooManyRequests)
return
}
next.ServeHTTP(w, r)
})
}
9. Logging and Monitoring
- Log security events like failed login attempts and suspicious activities.
- Use structured logging libraries like
logrus
orzerolog
.
10. Conclusion
Securing web applications requires a proactive approach to mitigate risks and vulnerabilities. By implementing the practices discussed here, you can protect your Go web applications from common threats and ensure a secure experience for your users.
Using HTTPS in Go
1. Overview
HTTPS (HyperText Transfer Protocol Secure) is a secure version of HTTP, where the data is encrypted using SSL/TLS. In Go, setting up an HTTPS server requires obtaining an SSL/TLS certificate and configuring the server to handle encrypted connections. This is important for protecting sensitive data during transmission between the client and server.
2. Obtaining an SSL/TLS Certificate
- Use Let's Encrypt: A popular choice for obtaining a free SSL/TLS certificate. You can use tools like
certbot
to automate the process. - Self-Signed Certificates: For testing or development purposes, you can create a self-signed certificate using tools like
openssl
.
3. Setting Up a Basic HTTPS Server
In Go, you can use the http
package to serve HTTPS content by specifying the certificate and private key files.
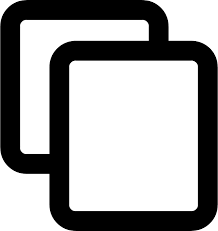
import (
"fmt"
"net/http"
"log"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, HTTPS World!")
}
func main() {
http.HandleFunc("/", handler)
err := http.ListenAndServeTLS(":443", "server.crt", "server.key", nil)
if err != nil {
log.Fatalf("ListenAndServeTLS failed: %s", err)
}
}
This sets up a simple HTTPS server on port 443, using the certificate file server.crt
and the private key file server.key
.
4. Testing the HTTPS Server
Once the server is running, you can test it by navigating to https://localhost
or using curl
:
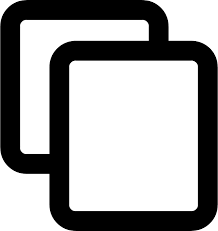
curl -k https://localhost
The -k
flag skips certificate validation (useful for self-signed certificates).
5. Redirect HTTP to HTTPS
To ensure that all HTTP requests are redirected to HTTPS, you can set up an HTTP-to-HTTPS redirect handler:
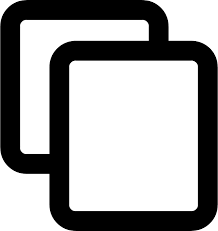
func redirectToHTTPS(w http.ResponseWriter, r *http.Request) {
http.Redirect(w, r, "https://"+r.Host+r.URL.String(), http.StatusMovedPermanently)
}
func main() {
go func() {
log.Fatal(http.ListenAndServe(":80", http.HandlerFunc(redirectToHTTPS)))
}()
http.HandleFunc("/", handler)
err := http.ListenAndServeTLS(":443", "server.crt", "server.key", nil)
if err != nil {
log.Fatalf("ListenAndServeTLS failed: %s", err)
}
}
This redirects all HTTP traffic to HTTPS, helping ensure secure communication.
6. Handling Insecure Connections (SSL/TLS Verification)
When working with HTTPS clients (e.g., making requests to external servers), you should handle SSL/TLS verification properly. Avoid disabling verification in production environments, but you can configure Go's HTTP client to skip verification during testing with self-signed certificates.
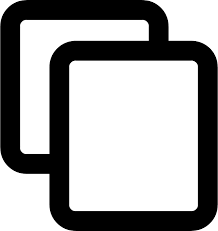
import (
"crypto/tls"
"net/http"
)
func createInsecureHTTPClient() *http.Client {
tr := &http.Transport{
TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
}
return &http.Client{Transport: tr}
}
Use the InsecureSkipVerify
option only for testing purposes with self-signed certificates.
7. HTTPS with Strong Cipher Suites
For better security, it's important to configure your server with strong TLS cipher suites. This can be done by setting the MinVersion
and CipherSuites
in the tls.Config
.
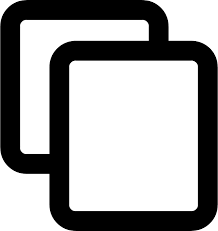
import (
"crypto/tls"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Hello, Secure World!"))
}
func main() {
tlsConfig := &tls.Config{
MinVersion: tls.VersionTLS13,
CipherSuites: []uint16{tls.TLS_AES_128_GCM_SHA256, tls.TLS_AES_256_GCM_SHA384},
}
server := &http.Server{
Addr: ":443",
Handler: http.HandlerFunc(handler),
TLSConfig: tlsConfig,
}
err := server.ListenAndServeTLS("server.crt", "server.key")
if err != nil {
log.Fatalf("ListenAndServeTLS failed: %s", err)
}
}
This configures the server to use TLS 1.3 and strong ciphers, providing better security for your HTTPS server.
8. Conclusion
Using HTTPS is an essential step in ensuring the security of web applications. Go provides an easy-to-implement solution for setting up HTTPS servers, whether for production or development. By following best practices like redirecting HTTP to HTTPS, using strong cipher suites, and handling SSL/TLS verification, you can significantly enhance the security of your Go-based web applications.
Protecting Against SQL Injection
1. Overview
SQL injection is a type of attack where malicious SQL code is inserted into an input field, allowing attackers to execute arbitrary SQL queries. This can lead to unauthorized access to or manipulation of the database. Protecting your application from SQL injection is crucial to avoid data breaches and maintain the integrity of your system.
2. Best Practices for Preventing SQL Injection
- Use Prepared Statements and Parameterized Queries: The most effective defense against SQL injection is using prepared statements with parameterized queries. This ensures that user input is treated as data, not executable code.
- Use Stored Procedures: Stored procedures are predefined SQL queries stored in the database. When used with proper parameterization, they reduce the risk of SQL injection.
- Validate and Sanitize Input: Always validate user inputs to ensure they match the expected data type and format. Additionally, sanitize inputs to remove any potentially dangerous characters.
- Limit Database Permissions: Ensure that the database user account used by your application has the minimum required privileges. This limits the impact of an SQL injection attack.
- Use ORM (Object-Relational Mapping) Libraries: ORMs, such as GORM in Go, can help protect against SQL injection by using parameterized queries and abstracting away raw SQL code.
- Use Web Application Firewalls (WAFs): WAFs can help detect and block SQL injection attempts before they reach your database.
3. Using Prepared Statements and Parameterized Queries in Go
In Go, you can use the database/sql
package to prevent SQL injection by using prepared statements. A prepared statement allows you to define a SQL query with placeholders for user inputs, and then bind values to these placeholders.
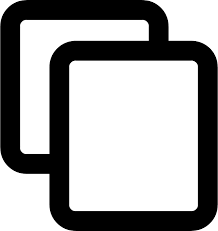
import (
"database/sql"
"fmt"
"log"
_ "github.com/lib/pq"
)
func main() {
// Establish database connection
db, err := sql.Open("postgres", "user=username dbname=mydb sslmode=disable")
if err != nil {
log.Fatal(err)
}
defer db.Close()
// Use prepared statement to prevent SQL injection
query := "SELECT name, age FROM users WHERE id = $1"
var name string
var age int
err = db.QueryRow(query, 1).Scan(&name, &age)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Name: %s, Age: %d\n", name, age)
}
In this example, the user input is safely passed as a parameter to the query, preventing any SQL injection attempts.
4. Example of Vulnerable Code
Here is an example of code that is vulnerable to SQL injection:
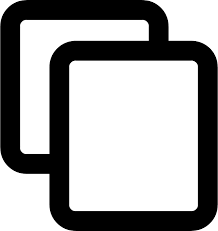
func vulnerableQuery(db *sql.DB, userInput string) {
query := "SELECT name, age FROM users WHERE name = '" + userInput + "'"
rows, err := db.Query(query)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
}
This code concatenates user input directly into the SQL query, making it vulnerable to SQL injection. An attacker could input something like ' OR 1=1 --
to bypass authentication or manipulate the query.
5. Using Stored Procedures
Stored procedures are a safer way to execute SQL queries, as they encapsulate the SQL logic in the database and prevent direct manipulation of the query by user input.
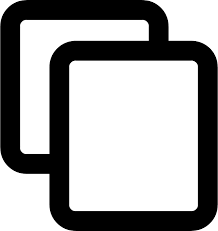
func getUserByID(db *sql.DB, userID int) {
// Call a stored procedure to fetch the user by ID
_, err := db.Exec("CALL GetUserByID($1)", userID)
if err != nil {
log.Fatal(err)
}
}
In this example, GetUserByID
is a stored procedure in the database that safely handles the query execution.
6. Validating and Sanitizing User Input
Even when using prepared statements, it's important to validate and sanitize user input to ensure that it conforms to the expected format. For example, if you expect an integer, make sure the input is numeric. You can use regular expressions or custom validation functions to achieve this.
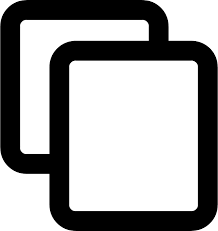
import "regexp"
func isValidEmail(email string) bool {
re := regexp.MustCompile(`^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$`)
return re.MatchString(email)
}
This function uses a regular expression to validate that the input is a valid email address. By validating input, you reduce the risk of malicious data being inserted into your queries.
7. Limiting Database Permissions
Make sure your application uses a database user with limited permissions. For example, if your application only needs to read data from the database, grant the user only read access. This minimizes the potential damage if an attacker successfully exploits an SQL injection vulnerability.
8. Conclusion
SQL injection is a critical security risk that can be mitigated by following best practices. Using prepared statements and parameterized queries, validating user input, and employing stored procedures are key techniques to protect your Go applications from SQL injection. Always prioritize security and follow these guidelines to secure your database interactions.
JWT Authentication in Go
1. Overview of JWT
JSON Web Tokens (JWT) are a widely used method for securely transmitting information between parties as a JSON object. JWTs can be used for authentication and authorization in web applications. They are compact, URL-safe, and can be digitally signed to ensure the integrity of the data. In Go, JWT is commonly used for implementing stateless authentication where the server does not store session data, but instead, the client sends a token for every request.
2. Structure of a JWT
A JWT consists of three parts:
- Header: Contains metadata about the token, such as the signing algorithm (e.g., HMAC SHA256 or RSA).
- Payload: Contains the claims or the actual data. Claims can be registered (standard claims), public, or private.
- Signature: A hash of the header and payload, signed using a secret or private key.
3. Installing JWT Package in Go
To work with JWTs in Go, we can use the popular "jwt-go" library. Install it using the following command:
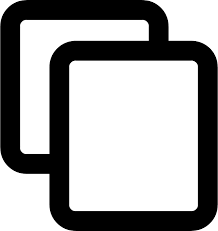
go get github.com/dgrijalva/jwt-go
4. Generating a JWT in Go
To generate a JWT, you need to create a signing key and define the claims (payload) that will be included in the token. Here's how to generate a JWT using the "jwt-go" package:
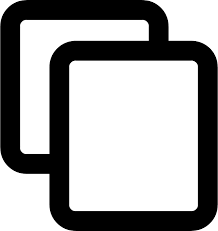
package main
import (
"fmt"
"log"
"time"
"github.com/dgrijalva/jwt-go"
)
var mySigningKey = []byte("secret")
func GenerateJWT() (string, error) {
// Create the JWT claims
claims := jwt.MapClaims{
"username": "user123",
"exp": time.Now().Add(time.Hour * 1).Unix(),
}
// Create the token
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims)
// Generate the signed token
signedToken, err := token.SignedString(mySigningKey)
if err != nil {
return "", err
}
return signedToken, nil
}
func main() {
token, err := GenerateJWT()
if err != nil {
log.Fatal(err)
}
fmt.Println("Generated Token:", token)
}
In this example, we create a JWT with a "username" claim and an expiration time of one hour. The token is signed using a secret key "secret".
5. Verifying a JWT in Go
When receiving a JWT from a client, you need to verify its authenticity and decode the claims. Here's an example of how to verify a JWT and read the claims:
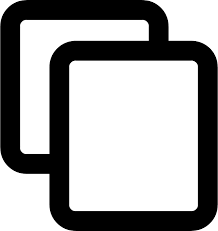
func VerifyJWT(tokenString string) (*jwt.Token, error) {
// Parse the JWT string and check for validity
token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) {
// Ensure the token's signing method is HMAC
if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok {
return nil, fmt.Errorf("Unexpected signing method: %v", token.Header["alg"])
}
return mySigningKey, nil
})
return token, err
}
func main() {
// Example token string (from client)
tokenString := ""
// Verify the token
token, err := VerifyJWT(tokenString)
if err != nil {
log.Fatal(err)
}
// Extract claims
claims, ok := token.Claims.(jwt.MapClaims)
if ok && token.Valid {
fmt.Println("Username:", claims["username"])
} else {
fmt.Println("Invalid token")
}
}
In this example, the token is first parsed and verified using the secret key. If the token is valid, the claims can be extracted and used.
6. Using JWT for Authentication in Go
In a typical scenario, a client sends a JWT in the HTTP Authorization header when making requests to a server. The server verifies the token, and if valid, grants access to protected resources.
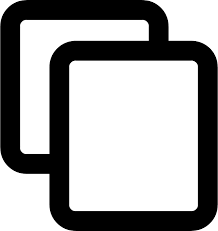
package main
import (
"fmt"
"log"
"net/http"
"strings"
"github.com/dgrijalva/jwt-go"
)
func Middleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
// Get token from Authorization header
tokenString := r.Header.Get("Authorization")
tokenString = strings.Replace(tokenString, "Bearer ", "", 1)
// Verify token
token, err := VerifyJWT(tokenString)
if err != nil || !token.Valid {
http.Error(w, "Unauthorized", http.StatusUnauthorized)
return
}
// Pass request to the next handler
next.ServeHTTP(w, r)
})
}
func ProtectedEndpoint(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "This is a protected endpoint")
}
func main() {
http.Handle("/protected", Middleware(http.HandlerFunc(ProtectedEndpoint)))
log.Fatal(http.ListenAndServe(":8080", nil))
}
In this example, we define a middleware that verifies the JWT token sent with a request. If the token is valid, the request is passed to the protected endpoint. Otherwise, the server responds with "Unauthorized".
7. Best Practices for JWT Authentication
- Use Strong Secrets: Always use a strong and unique secret key to sign your JWTs. Avoid using simple or predictable keys.
- Set Expiration Times: JWTs should have an expiration time to limit the window of attack. Use the "exp" claim to set the expiration time.
- Use HTTPS: Always use HTTPS to transmit JWTs to prevent them from being intercepted in transit.
- Handle Token Revocation: Since JWTs are stateless and don't require server-side sessions, implementing token revocation can be tricky. You can handle this with a blacklist or short expiration times.
- Validate Claims: Always validate JWT claims (e.g., "exp", "aud", "iss") to ensure that the token has not been tampered with and that it is still valid.
8. Conclusion
JWT authentication is a powerful and efficient way to implement stateless authentication in Go applications. By following best practices such as using strong signing keys, setting expiration times, and validating the JWT claims, you can securely manage user authentication and authorization in your Go applications.
Data Encryption and Decryption in Go
1. Overview of Data Encryption
Data encryption is the process of converting plaintext into an unreadable format (ciphertext) to protect it from unauthorized access. In Go, encryption and decryption can be implemented using various cryptographic algorithms such as AES, RSA, and more. Go’s standard library provides robust support for encryption, and third-party libraries can also be used for advanced encryption methods.
2. Symmetric vs. Asymmetric Encryption
There are two primary types of encryption:
- Symmetric Encryption: The same key is used for both encryption and decryption. The key must be kept secret between the sender and receiver. AES (Advanced Encryption Standard) is a popular symmetric encryption algorithm.
- Asymmetric Encryption: Uses a pair of keys, one for encryption (public key) and one for decryption (private key). RSA is a commonly used asymmetric encryption algorithm.
3. Installing the Go Crypto Package
Go provides a "crypto" package in its standard library that includes functions for hashing, encryption, and decryption. You don’t need to install any external packages for basic encryption tasks.
4. Symmetric Encryption with AES in Go
AES is a widely used symmetric encryption algorithm. It uses a secret key to encrypt and decrypt data. Here’s an example of encrypting and decrypting a message using AES:
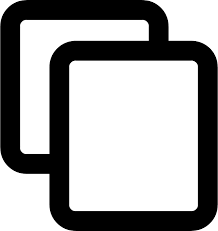
package main
import (
"crypto/aes"
"crypto/cipher"
"crypto/rand"
"fmt"
"io"
"log"
)
func encrypt(plaintext []byte, key []byte) ([]byte, error) {
block, err := aes.NewCipher(key)
if err != nil {
return nil, err
}
// IV (Initialization Vector) should be random for each encryption
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
return nil, err
}
stream := cipher.NewCFBEncrypter(block, iv)
stream.XORKeyStream(ciphertext[aes.BlockSize:], plaintext)
return ciphertext, nil
}
func decrypt(ciphertext []byte, key []byte) ([]byte, error) {
block, err := aes.NewCipher(key)
if err != nil {
return nil, err
}
// Extract IV from the beginning of the ciphertext
if len(ciphertext) < aes.BlockSize {
return nil, fmt.Errorf("ciphertext too short")
}
iv := ciphertext[:aes.BlockSize]
ciphertext = ciphertext[aes.BlockSize:]
stream := cipher.NewCFBDecrypter(block, iv)
stream.XORKeyStream(ciphertext, ciphertext)
return ciphertext, nil
}
func main() {
key := []byte("the32byteencryptionkey12345678") // 32-byte key for AES-256
plaintext := []byte("Hello, Go Encryption!")
// Encrypt the message
ciphertext, err := encrypt(plaintext, key)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Encrypted: %x\n", ciphertext)
// Decrypt the message
decryptedText, err := decrypt(ciphertext, key)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Decrypted: %s\n", decryptedText)
}
This example demonstrates how to encrypt and decrypt data using AES in CFB mode. The initialization vector (IV) ensures that the same plaintext will encrypt to different ciphertexts every time.
5. Asymmetric Encryption with RSA in Go
RSA is an asymmetric encryption algorithm that uses a pair of public and private keys. The public key encrypts data, and the private key is used to decrypt it. Here’s an example of how to encrypt and decrypt data using RSA:
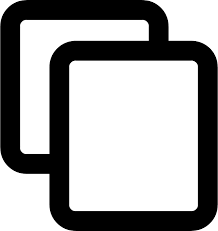
package main
import (
"crypto/rand"
"crypto/rsa"
"crypto/sha256"
"fmt"
"log"
)
func encryptWithRSA(plaintext []byte, pubkey *rsa.PublicKey) ([]byte, error) {
// Encrypt the message with the public key
ciphertext, err := rsa.EncryptOAEP(sha256.New(), rand.Reader, pubkey, plaintext, nil)
if err != nil {
return nil, err
}
return ciphertext, nil
}
func decryptWithRSA(ciphertext []byte, privkey *rsa.PrivateKey) ([]byte, error) {
// Decrypt the message with the private key
plaintext, err := rsa.DecryptOAEP(sha256.New(), rand.Reader, privkey, ciphertext, nil)
if err != nil {
return nil, err
}
return plaintext, nil
}
func main() {
// Generate RSA keys (public and private)
privkey, err := rsa.GenerateKey(rand.Reader, 2048)
if err != nil {
log.Fatal(err)
}
pubkey := &privkey.PublicKey
plaintext := []byte("Hello, RSA Encryption!")
// Encrypt the message with the public key
ciphertext, err := encryptWithRSA(plaintext, pubkey)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Encrypted: %x\n", ciphertext)
// Decrypt the message with the private key
decryptedText, err := decryptWithRSA(ciphertext, privkey)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Decrypted: %s\n", decryptedText)
}
This example demonstrates the RSA encryption and decryption process using RSA key pairs. The message is encrypted with the public key and decrypted with the private key.
6. Base64 Encoding and Decoding for Secure Data Transmission
Since encrypted data may contain non-printable characters, it is often encoded using Base64 for safe transmission over HTTP or storing in databases. Go provides a built-in "encoding/base64" package for encoding and decoding data:
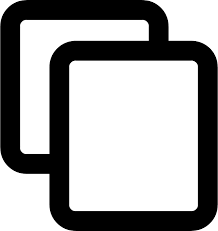
package main
import (
"encoding/base64"
"fmt"
)
func main() {
// Example data to encode
data := "Hello, secure world!"
// Encode data in Base64
encoded := base64.StdEncoding.EncodeToString([]byte(data))
fmt.Println("Encoded:", encoded)
// Decode data from Base64
decoded, err := base64.StdEncoding.DecodeString(encoded)
if err != nil {
fmt.Println("Error decoding:", err)
return
}
fmt.Println("Decoded:", string(decoded))
}
In this example, the "encoding/base64" package is used to encode and decode a string. This ensures that encrypted data can be safely transmitted as text.
7. Best Practices for Data Encryption
- Use Strong Keys: Always use sufficiently long and random keys to ensure the security of your encryption. For AES, use at least 256-bit keys.
- Choose the Right Algorithm: Select an encryption algorithm based on your specific needs. AES is typically used for fast, symmetric encryption, while RSA is used for secure key exchange.
- Handle Key Management Carefully: Protect your encryption keys from unauthorized access. Use secure storage solutions for storing private keys.
- Use IVs (Initialization Vectors): Always use a random IV for each encryption operation to ensure that the same plaintext results in different ciphertexts.
- Follow the Principle of Least Privilege: Only allow access to encrypted data or encryption keys by authorized entities.
8. Conclusion
Data encryption and decryption are vital aspects of ensuring the confidentiality and security of sensitive information. Go’s standard library provides powerful tools for implementing various encryption algorithms, including AES and RSA. By following best practices and using robust encryption methods, you can protect your data from unauthorized access.
Identifying Performance Bottlenecks in Go
1. Overview of Performance Bottlenecks
Performance bottlenecks occur when a part of your program consumes more resources (CPU, memory, etc.) than expected, slowing down the overall execution. Identifying and resolving these bottlenecks is crucial for improving the efficiency and scalability of your Go applications. In Go, you can diagnose performance issues using profiling, benchmarking, and monitoring tools provided by the standard library and third-party libraries.
2. Common Sources of Performance Bottlenecks
Performance bottlenecks can arise from various sources, including but not limited to:
- CPU-bound operations: Intensive computations or CPU-heavy algorithms can dominate the CPU and slow down the program.
- Memory management: Excessive memory allocation, memory leaks, or poor memory usage can lead to high memory consumption, causing the program to run slowly.
- Concurrency issues: Improper handling of goroutines, channels, and locks can lead to thread contention, deadlocks, or race conditions.
- I/O operations: Slow disk access, network operations, or database queries can become a bottleneck.
3. Profiling in Go
Go’s built-in profiling tools allow you to measure CPU usage, memory allocation, and goroutine activity. You can use these to find the parts of your application that are consuming the most resources.
3.1. CPU Profiling
CPU profiling allows you to see where most of your program’s CPU time is spent. The Go runtime supports CPU profiling through the "net/http/pprof" package.
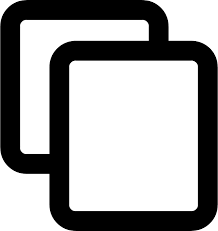
package main
import (
"fmt"
"net/http"
_ "net/http/pprof"
"log"
)
func heavyComputation() {
// Simulating a CPU-intensive operation
for i := 0; i < 1000000000; i++ {
_ = i * i
}
}
func main() {
// Start pprof HTTP server
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
// Simulate a heavy computation
heavyComputation()
fmt.Println("Computation finished!")
}
In this example, we use the "net/http/pprof" package to start a profiling server. You can run the program and access the profiling data by visiting `http://localhost:6060/debug/pprof/` in your browser. This allows you to visualize the CPU profile and pinpoint any performance issues related to CPU usage.
3.2. Memory Profiling
Memory profiling helps identify memory allocation issues such as memory leaks or excessive memory usage. You can capture memory profiles using the `pprof` package.
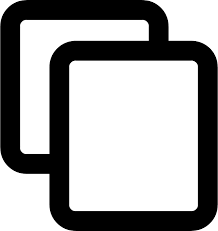
package main
import (
"fmt"
"net/http"
_ "net/http/pprof"
"log"
"runtime"
)
func memoryIntensive() {
// Allocate memory in a loop
for i := 0; i < 1000000; i++ {
_ = make([]byte, 1024*1024) // Allocate 1MB
}
}
func main() {
// Start pprof HTTP server
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
// Simulate memory intensive operation
memoryIntensive()
// Print memory stats
var mem runtime.MemStats
runtime.ReadMemStats(&mem)
fmt.Printf("Memory Allocated: %v MB\n", mem.Alloc/1024/1024)
}
This example allocates memory repeatedly, simulating a memory leak. You can inspect memory profiles and see if your program is allocating more memory than expected.
4. Benchmarking in Go
Benchmarking is another way to measure performance. Go supports benchmarking through the built-in testing
package, which allows you to write tests that measure how long certain code takes to execute.
4.1. Writing Benchmark Tests
Benchmark tests can be used to measure the execution time of specific functions or code blocks. Here's how you can write a benchmark in Go:
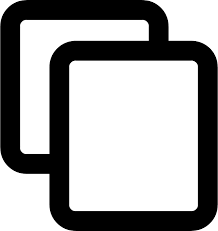
package main
import (
"testing"
)
func BenchmarkHeavyComputation(b *testing.B) {
for i := 0; i < b.N; i++ {
// Simulate a heavy computation
_ = i * i
}
}
To run the benchmark, execute the following command:
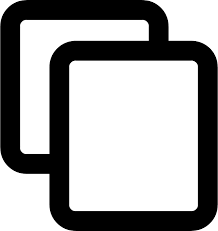
go test -bench .
This will run the benchmark and output the time it takes to execute the code inside the benchmark function. You can compare the performance of different implementations to find the most efficient one.
5. Identifying Goroutine and Channel Bottlenecks
Concurrency-related performance issues can often be detected by examining goroutine and channel activity. The Go runtime provides tools to analyze and profile goroutines.
5.1. Goroutine Profiling
In addition to CPU and memory profiling, you can also profile the number of goroutines in your program using the built-in pprof tool. An excessive number of goroutines or blocked goroutines can indicate performance bottlenecks.
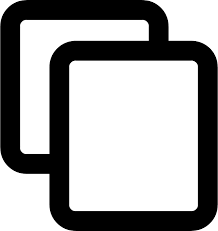
go tool pprof http://localhost:6060/debug/pprof/goroutine?debug=2
This command will give you a detailed view of the goroutine stack traces, helping you identify issues like deadlocks, excessive goroutines, or contention in channels.
6. Using Go’s Built-in Tools
In addition to profiling and benchmarking, Go provides other useful tools for performance analysis:
- pprof: For detailed CPU and memory profiling. Use the `go tool pprof` command to analyze your program’s performance.
- goroutines: Use the `go tool trace` command to visualize your program’s execution and identify performance bottlenecks in goroutines.
- trace: Capture detailed information about your program’s execution (e.g., goroutine lifetimes, blocking times, etc.).
7. Third-Party Profiling Tools
In addition to Go's built-in profiling tools, several third-party libraries and tools can help identify performance bottlenecks:
- GoTrace: A tool for visualizing Go program execution that can help identify concurrency-related bottlenecks.
- Delve: A debugger for Go that can be used to pause execution, inspect variables, and understand where bottlenecks are occurring.
- Prometheus and Grafana: For monitoring application performance and resource consumption over time, especially in production environments.
8. Best Practices for Optimizing Go Performance
- Optimize Algorithms: Always choose the most efficient algorithms for your use case. Optimize the big-O complexity of your algorithms.
- Use Goroutines Efficiently: Avoid creating too many goroutines. Use worker pools or other concurrency patterns to manage goroutines effectively.
- Avoid Memory Leaks: Ensure that you’re freeing memory properly and not holding on to unused resources.
- Minimize I/O Operations: Reduce the number of I/O operations, batch them if possible, or use non-blocking operations to prevent I/O from becoming a bottleneck.
- Profile Regularly: Regularly profile your application to identify new performance bottlenecks as your code evolves.
9. Conclusion
Identifying and resolving performance bottlenecks is a crucial step in optimizing Go applications. By utilizing Go's built-in profiling and benchmarking tools, as well as best practices for concurrent programming and algorithm optimization, you can ensure that your Go applications run efficiently and scale well in production.
Profiling Go Code with pprof
1. Introduction to pprof
The Go programming language provides a powerful tool called pprof
for profiling and analyzing the performance of Go applications. pprof helps you identify performance bottlenecks such as excessive CPU usage, memory consumption, and goroutine blocking. It provides detailed insights into where your program spends most of its time and resources, allowing you to optimize the code for better performance.
2. Enabling pprof in Your Go Application
To start using pprof, you need to import the net/http/pprof
package and set up an HTTP server to expose the pprof data. By doing so, you can collect profiling information while the application is running.
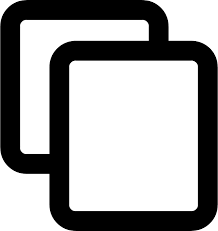
package main
import (
"fmt"
"net/http"
_ "net/http/pprof" // Import pprof package for profiling
"log"
)
func main() {
// Start pprof HTTP server
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
// Simulate some work
for i := 0; i < 100000000; i++ {
_ = i * i
}
fmt.Println("Application running with pprof enabled")
}
In this example, the net/http/pprof
package is imported anonymously to register its handlers with the HTTP server. Once the application is running, you can access profiling data at `http://localhost:6060/debug/pprof/`.
3. Accessing pprof Data
Once pprof is integrated into your application, you can access various types of profiling data through the following URLs:
- CPU profile: Captures CPU usage over a specified duration (default 30 seconds).
- Heap profile: Provides memory allocation information.
- Goroutine profile: Displays all active goroutines.
- Block profile: Shows where goroutines are blocked.
- Thread creation profile: Displays thread creation information.
These profiles provide insights into various aspects of your application’s performance, and you can analyze them using the Go tool or a web browser.
4. Analyzing CPU Profile
The CPU profile shows where your program is spending CPU time. To generate a CPU profile, visit /debug/pprof/cpu
. You can also capture a CPU profile using the Go tool:
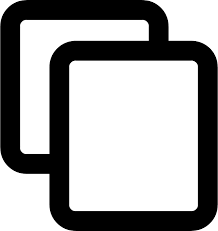
go tool pprof http://localhost:6060/debug/pprof/profile?seconds=30
This command will download the CPU profile as a file and open the pprof interactive tool for analysis. Here's how to use it:
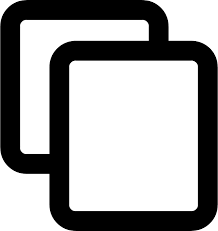
(pprof) top
The top
command shows the top functions consuming CPU time. You can also use list
to see the annotated source code, or web
to generate a graphical representation of the profile.
5. Analyzing Memory Profile
The memory (heap) profile helps you identify memory usage and possible memory leaks. To generate a heap profile, visit /debug/pprof/heap
. You can also capture it using the Go tool:
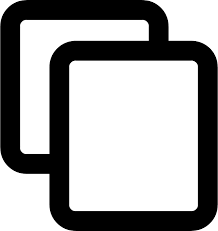
go tool pprof http://localhost:6060/debug/pprof/heap
Once you have the heap profile, you can use commands like top
, list
, or web
to analyze memory allocation patterns and identify areas of excessive memory usage.
6. Analyzing Goroutines
The goroutine profile helps you identify issues related to concurrency, such as excessive goroutines or deadlocks. To capture the goroutine profile, visit /debug/pprof/goroutine
. You can analyze the profile using:
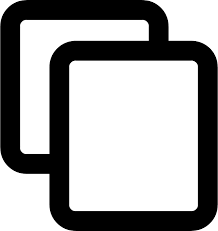
go tool pprof http://localhost:6060/debug/pprof/goroutine
This will show you the goroutines that are running and their stack traces, helping you spot any concurrency issues in your application.
7. Using pprof for Optimizing Code
After collecting the profiling data, use the following steps to optimize your code:
- CPU Profile: Look for hot spots in your application where CPU usage is high. Consider optimizing algorithms or reducing unnecessary computations.
- Memory Profile: Identify functions that allocate large amounts of memory. Optimize memory usage, reduce allocations, or consider using memory pools.
- Goroutine Profile: Look for excessive goroutines or blocked goroutines. Optimize concurrency and avoid unnecessary goroutines.
8. Visualizing pprof Data
In addition to using the command-line pprof
tool, you can visualize pprof data in a graphical format. Use the web
command to generate a `.svg` file, which can be opened in a web browser:
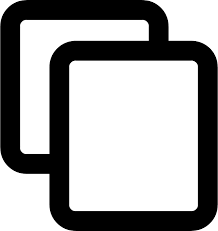
(pprof) web
This will generate a visual profile that you can analyze for performance bottlenecks.
9. Conclusion
Profiling your Go code using pprof is an essential step in identifying performance bottlenecks and optimizing your application. By leveraging the built-in pprof package and the powerful go tool pprof
, you can gain valuable insights into your program's performance and make informed decisions on how to improve it. Regular profiling should be part of the development process to ensure that your Go applications run efficiently at scale.
Memory Management and Optimization in Go
1. Introduction to Memory Management in Go
Go provides automatic memory management through garbage collection (GC), which helps manage memory allocation and deallocation efficiently. However, understanding how Go handles memory and optimizing memory usage is crucial for building performant applications, especially for large-scale systems.
2. Understanding Go's Garbage Collection
Garbage collection in Go is automatic and runs concurrently with the application. Go’s garbage collector (GC) is a mark-and-sweep collector that identifies objects no longer in use and reclaims their memory. The GC process happens in the background, but it can still impact performance if the application is memory-intensive or if object allocation is frequent.
Go uses a combination of techniques to minimize the impact of garbage collection, such as:
- Generational collection: Objects that survive multiple GC cycles are less likely to be collected again, improving efficiency.
- Concurrent collection: The garbage collector runs concurrently with the application, minimizing pause times.
3. Memory Allocation in Go
Go allocates memory for variables and objects using the built-in memory allocator. The memory is split into two areas:
- Stack: The stack is used for local variables and function call frames. It is relatively small and has automatic memory management. Variables on the stack are deallocated when the function they belong to returns.
- Heap: The heap is used for objects that need to persist beyond the scope of the function call. These objects are dynamically allocated and managed by the garbage collector.
4. Optimizing Memory Usage in Go
To optimize memory usage in Go, developers should focus on how memory is allocated and freed, as well as how the garbage collector interacts with the application. Here are some tips for optimizing memory management:
4.1 Minimize Memory Allocation
Excessive memory allocation can lead to high GC activity and poor performance. Avoid unnecessary allocations by:
- Reusing objects instead of creating new ones where possible.
- Using memory pools (e.g.,
sync.Pool
) to manage reusable objects. - Avoiding large allocations in tight loops.
4.2 Avoid Memory Leaks
Memory leaks can occur when objects are not properly de-referenced, causing them to remain in memory even when no longer needed. To avoid memory leaks:
- Ensure that unused objects are de-referenced explicitly.
- Use tools like the
pprof
package to analyze memory usage and detect leaks.
4.3 Use Value Semantics When Possible
Go’s default behavior for passing variables is by value, which means that when you pass a variable to a function, a copy is created. While this approach can increase memory usage when working with large data, it can be more efficient when dealing with small or immutable objects. Consider using value types (e.g., struct
) when appropriate, especially for small objects.
4.4 Optimize Memory Access Patterns
Efficient memory access patterns can improve the performance of your application. When working with large data sets, use the following strategies:
- Access memory in a localized and predictable manner to benefit from CPU caching.
- Use arrays or slices for sequential data access instead of hash maps when appropriate.
5. Using the sync.Pool
for Memory Optimization
Go provides the sync.Pool
type to manage reusable objects and reduce memory allocation overhead. The sync.Pool
is a concurrency-safe memory pool that can be used to store and retrieve objects that are frequently created and discarded.
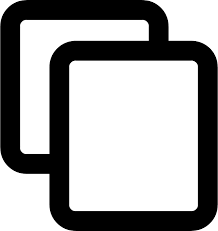
package main
import (
"fmt"
"sync"
)
var pool = sync.Pool{
New: func() interface{} {
return &MyType{}
},
}
type MyType struct {
ID int
}
func main() {
// Get an object from the pool
obj := pool.Get().(*MyType)
obj.ID = 42
fmt.Println("Object ID:", obj.ID)
// Put the object back into the pool
pool.Put(obj)
}
Using sync.Pool
helps minimize memory allocation and deallocation costs, especially for frequently used objects. It reduces the pressure on Go’s garbage collector and improves overall performance.
6. Memory Profiling and Analysis
Profiling your application’s memory usage is critical for identifying memory bottlenecks and opportunities for optimization. The pprof
package provides a way to analyze memory usage, including heap allocations and goroutine stack traces.
To capture a heap profile, visit /debug/pprof/heap
in your application, or use the go tool pprof
tool to collect and analyze the profile:
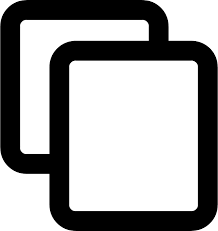
go tool pprof http://localhost:6060/debug/pprof/heap
This allows you to analyze memory allocations and identify where memory is being used inefficiently. You can use the top
and list
commands to view the most memory-consuming functions.
7. Conclusion
Memory management and optimization are crucial in Go for building efficient and scalable applications. By understanding Go’s garbage collection process, minimizing memory allocation, reusing objects, and using tools like sync.Pool
and pprof
, you can ensure that your application runs efficiently with minimal memory usage. Regular profiling and optimization will help maintain optimal performance as your application grows in complexity and scale.
Improving Concurrency Performance in Go
1. Introduction to Concurrency in Go
Go is known for its strong concurrency model, which is built around goroutines and channels. Concurrency allows you to perform multiple tasks simultaneously, improving the performance of your application. However, while concurrency can improve throughput and responsiveness, it can also introduce complexity and performance bottlenecks if not managed properly.
This section covers strategies to improve concurrency performance in Go, focusing on optimizing goroutine usage, minimizing contention, and utilizing Go’s concurrency primitives effectively.
2. Understanding Goroutines and Channels
Goroutines are lightweight threads managed by Go’s runtime, and channels are used for communication between goroutines. Properly managing these concurrency primitives is crucial for maximizing performance.
While goroutines are efficient in terms of memory and scheduling, excessive goroutines or poor synchronization can lead to contention or context-switching overhead.
3. Optimizing Goroutine Usage
Efficient use of goroutines is key to maximizing concurrency performance. Here are some strategies for optimizing goroutines:
3.1 Limit Goroutine Creation
Creating too many goroutines can lead to increased scheduling overhead and contention, reducing performance. Instead of spawning a new goroutine for every task, consider batching tasks and limiting the number of concurrent goroutines.
Use worker pools to control the number of goroutines. This allows you to process multiple tasks concurrently while limiting the number of goroutines.
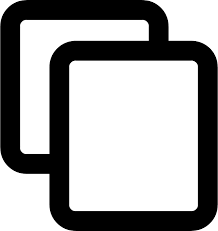
package main
import (
"fmt"
"sync"
)
func worker(wg *sync.WaitGroup, id int) {
defer wg.Done()
fmt.Printf("Worker %d started\n", id)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 10; i++ {
wg.Add(1)
go worker(&wg, i)
}
wg.Wait()
}
3.2 Use Worker Pools
A worker pool is a common concurrency pattern where a fixed number of workers (goroutines) process tasks from a queue. This helps to avoid overwhelming the system with too many goroutines and ensures efficient resource usage.
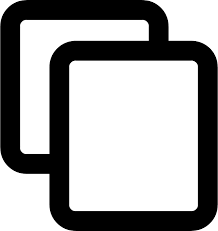
package main
import (
"fmt"
"sync"
)
func worker(id int, tasks <-chan int, wg *sync.WaitGroup) {
defer wg.Done()
for task := range tasks {
fmt.Printf("Worker %d processing task %d\n", id, task)
}
}
func main() {
var wg sync.WaitGroup
tasks := make(chan int, 10)
// Create worker pool with 3 workers
for i := 1; i <= 3; i++ {
wg.Add(1)
go worker(i, tasks, &wg)
}
// Add tasks to the channel
for i := 1; i <= 10; i++ {
tasks <- i
}
close(tasks)
wg.Wait()
}
3.3 Avoid Goroutine Leaks
Goroutine leaks occur when goroutines are started but never finished. This can lead to resource exhaustion and degraded performance. Always ensure that goroutines are properly synchronized and terminated when they are no longer needed.
Using sync.WaitGroup
or channels to signal completion is an effective way to avoid goroutine leaks.
4. Minimizing Contention
Contention occurs when multiple goroutines try to access shared resources simultaneously, leading to performance bottlenecks. Minimizing contention is key to improving concurrency performance. Here are some strategies for reducing contention:
4.1 Use Mutexes for Critical Sections
Mutexes are used to protect shared resources from concurrent access. However, excessive use of mutexes can lead to performance degradation due to locking overhead. Try to minimize the scope of critical sections and avoid holding locks for long periods.
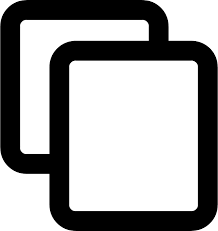
package main
import (
"fmt"
"sync"
)
var mu sync.Mutex
var counter int
func increment() {
mu.Lock()
counter++
mu.Unlock()
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 1000; i++ {
wg.Add(1)
go func() {
defer wg.Done()
increment()
}()
}
wg.Wait()
fmt.Println("Counter:", counter)
}
4.2 Use Atomic Operations
For simple integer or boolean operations, atomic operations can be used to avoid the overhead of mutexes. The sync/atomic
package provides operations that ensure safe manipulation of variables without the need for locks.
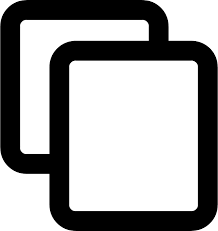
package main
import (
"fmt"
"sync"
"sync/atomic"
)
var counter int64
func increment() {
atomic.AddInt64(&counter, 1)
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 1000; i++ {
wg.Add(1)
go func() {
defer wg.Done()
increment()
}()
}
wg.Wait()
fmt.Println("Counter:", counter)
}
4.3 Reduce Lock Contention with Read/Write Mutexes
In some cases, you may have resources that are read frequently but modified infrequently. In such cases, using a sync.RWMutex
allows multiple readers to access the resource concurrently while ensuring exclusive access for writers.
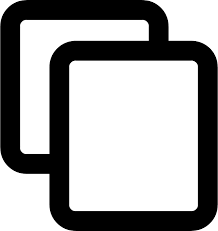
package main
import (
"fmt"
"sync"
)
var rwmu sync.RWMutex
var data int
func readData() int {
rwmu.RLock() // Shared lock for reading
defer rwmu.RUnlock()
return data
}
func writeData(value int) {
rwmu.Lock() // Exclusive lock for writing
defer rwmu.Unlock()
data = value
}
func main() {
var wg sync.WaitGroup
// Start multiple readers
for i := 0; i < 5; i++ {
wg.Add(1)
go func() {
defer wg.Done()
fmt.Println("Read Data:", readData())
}()
}
// Start a writer
wg.Add(1)
go func() {
defer wg.Done()
writeData(42)
}()
wg.Wait()
}
5. Profiling Concurrency Performance
Go provides the pprof
package to profile the performance of your application, including the analysis of goroutines and lock contention. Profiling can help identify bottlenecks and areas for optimization in your concurrency model.
You can capture goroutine and CPU profiles via HTTP endpoints, and analyze them with the go tool pprof
tool.
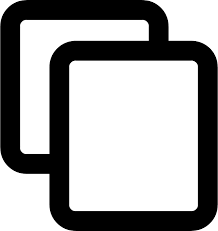
go tool pprof http://localhost:6060/debug/pprof/goroutine
go tool pprof http://localhost:6060/debug/pprof/heap
6. Conclusion
Improving concurrency performance in Go requires understanding how to use goroutines and channels efficiently, minimizing contention, and using tools like sync.Pool
and atomic operations to optimize synchronization. By profiling your application and identifying concurrency bottlenecks, you can fine-tune your application to handle high levels of concurrency with minimal overhead. With the right strategies, you can maximize the performance of your concurrent Go applications.
Effective Use of Goroutines and Channels in Go
1. Introduction to Goroutines and Channels
Goroutines and channels are the fundamental concurrency primitives in Go. Goroutines allow you to run functions concurrently, and channels allow goroutines to communicate and synchronize. When used effectively, they can make your Go programs highly concurrent and scalable.
However, improper use of these tools can lead to performance bottlenecks, race conditions, and difficulties in maintaining the program. This section will guide you through the best practices for using goroutines and channels effectively to achieve high-performance concurrency in Go.
2. Understanding Goroutines
Goroutines are lightweight threads managed by the Go runtime. They are efficient in terms of memory usage, and Go’s scheduler handles their execution in the background. Goroutines are ideal for performing tasks concurrently without the overhead of traditional threads.
To create a goroutine, you simply prefix a function call with the go
keyword. This will execute the function concurrently with the calling function.
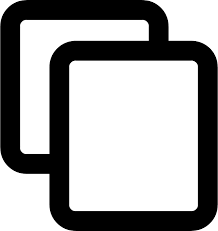
package main
import "fmt"
func sayHello() {
fmt.Println("Hello from goroutine!")
}
func main() {
go sayHello() // Start a goroutine
fmt.Println("Hello from main!")
}
Note that the sayHello
function will run concurrently with the main
function, so the output may vary depending on when the goroutine is scheduled to run.
3. Channels: Communication Between Goroutines
Channels provide a way for goroutines to communicate with each other. They allow you to send and receive data safely, ensuring synchronization between concurrent tasks.
To create a channel, use the make
function. Channels are typed, meaning you can specify the type of data they carry.
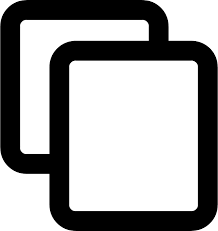
package main
import "fmt"
func sendData(ch chan int) {
ch <- 42 // Send data to the channel
}
func main() {
ch := make(chan int) // Create a channel
go sendData(ch) // Start a goroutine to send data
data := <-ch // Receive data from the channel
fmt.Println("Received:", data)
}
In this example, the sendData
goroutine sends an integer to the channel, and the main function receives it and prints the result.
4. Buffered Channels
By default, channels are unbuffered, meaning that the sender will be blocked until the receiver is ready to receive the data. Buffered channels, on the other hand, allow you to specify a capacity, and the sender can continue without waiting for the receiver if there is space in the channel.
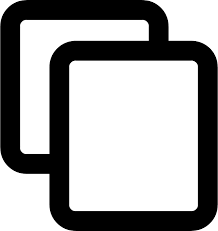
package main
import "fmt"
func main() {
ch := make(chan int, 2) // Create a buffered channel with capacity of 2
ch <- 1 // Send data to the channel
ch <- 2 // Send more data
// ch <- 3 // Would block since the channel is full
fmt.Println(<-ch) // Receive data from the channel
fmt.Println(<-ch) // Receive more data
}
Buffered channels are useful when you want to decouple the sending and receiving goroutines or when you need to avoid blocking the sender.
5. Closing Channels
Once a channel is no longer needed, it should be closed to signal that no more data will be sent through it. Closing a channel is important for goroutines that are receiving from the channel, as it allows them to exit gracefully when there is no more data to process.
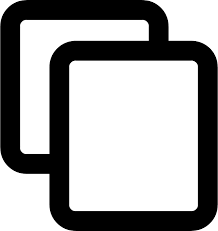
package main
import "fmt"
func sendData(ch chan int) {
for i := 0; i < 3; i++ {
ch <- i
}
close(ch) // Close the channel when done sending
}
func main() {
ch := make(chan int)
go sendData(ch)
for data := range ch { // Iterate over the channel until it's closed
fmt.Println("Received:", data)
}
}
In the above example, the main function receives from the channel until it is closed, signaling the end of data transmission.
6. Select Statement: Handling Multiple Channels
The select
statement allows a goroutine to wait on multiple channels simultaneously, making it easy to handle multiple concurrent operations. It works similarly to a switch
statement, but each case is a channel operation.
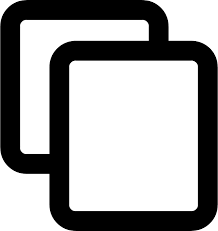
package main
import "fmt"
func main() {
ch1 := make(chan int)
ch2 := make(chan int)
go func() { ch1 <- 1 }()
go func() { ch2 <- 2 }()
select {
case data := <-ch1:
fmt.Println("Received from ch1:", data)
case data := <-ch2:
fmt.Println("Received from ch2:", data)
}
}
The select
statement allows the first channel to be received from as soon as it's ready, making it a powerful tool for handling multiple concurrent operations.
7. Synchronization with WaitGroups
When dealing with multiple goroutines that need to synchronize at the end, you can use a sync.WaitGroup
to wait for all goroutines to finish before proceeding.
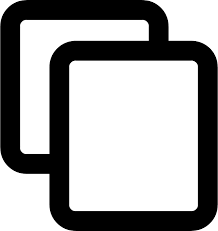
package main
import (
"fmt"
"sync"
)
func work(wg *sync.WaitGroup, id int) {
defer wg.Done() // Decrement counter when done
fmt.Printf("Worker %d started\n", id)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 3; i++ {
wg.Add(1) // Increment counter before starting goroutine
go work(&wg, i)
}
wg.Wait() // Wait for all goroutines to finish
fmt.Println("All workers finished")
}
In this example, the WaitGroup
ensures that the main function waits for all the worker goroutines to finish before it prints the final message.
8. Best Practices for Effective Use of Goroutines and Channels
- Limit the number of goroutines: Creating too many goroutines can overwhelm your system. Use worker pools to limit concurrency and avoid excessive context-switching.
- Use buffered channels for decoupling: Buffered channels allow you to decouple the sender and receiver, improving efficiency in certain cases.
- Gracefully close channels: Always close channels when you're done sending data to signal completion to receiving goroutines.
- Use
select
for handling multiple channels: Theselect
statement is an excellent way to handle multiple operations concurrently without blocking. - Ensure proper synchronization: Use
sync.WaitGroup
or other synchronization techniques to ensure that all goroutines finish their work before the program exits.
9. Conclusion
Goroutines and channels are powerful tools that can help you write highly concurrent and scalable applications in Go. By understanding their behavior, using them effectively, and following best practices, you can avoid common pitfalls and create efficient, maintainable concurrency in your applications.
Using Go for Automation Scripting
1. Introduction to Automation with Go
Go is a versatile programming language well-suited for automation scripting. Its simplicity, concurrency support, and rich standard library make it an ideal choice for creating scripts to automate repetitive tasks, manage system administration, interact with APIs, handle file manipulation, and much more.
In this section, we will explore how to use Go for creating automation scripts, highlighting key areas where Go can be particularly useful, along with some practical examples.
2. Why Use Go for Automation?
While languages like Python and Bash are commonly used for automation, Go offers several advantages:
- Performance: Go is a compiled language, making it faster than interpreted languages like Python and Bash.
- Concurrency: Go has built-in support for concurrency, making it easy to handle tasks that require parallelism, such as downloading files, handling multiple API requests, or processing data in parallel.
- Simplicity: Go's syntax is minimalistic, which allows you to write clean and maintainable scripts.
- Cross-platform: Go compiles to standalone binaries, meaning your automation scripts can run on any platform without the need for an interpreter.
3. Automating File Operations
One of the most common tasks in automation scripting is working with files. Go provides a comprehensive set of tools for file manipulation, including reading, writing, and deleting files.
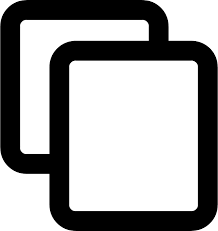
package main
import (
"fmt"
"io/ioutil"
"os"
)
func main() {
// Create a new file
file, err := os.Create("example.txt")
if err != nil {
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
// Write to the file
file.WriteString("Hello, Go!\n")
// Read the file
data, err := ioutil.ReadFile("example.txt")
if err != nil {
fmt.Println("Error reading file:", err)
return
}
fmt.Println("File content:", string(data))
// Delete the file
err = os.Remove("example.txt")
if err != nil {
fmt.Println("Error deleting file:", err)
return
}
fmt.Println("File deleted successfully!")
}
This script demonstrates basic file operations like creating, writing, reading, and deleting a file in Go. These types of tasks are commonly automated in system administration and maintenance scripts.
4. Automating API Interactions
Go's rich standard library includes the net/http
package, which makes it easy to interact with APIs, send HTTP requests, and process responses. This is useful for automating tasks like fetching data from web services, interacting with cloud platforms, or integrating with third-party APIs.
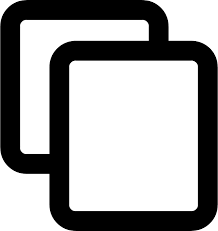
package main
import (
"fmt"
"io/ioutil"
"net/http"
)
func main() {
// Send an HTTP GET request
response, err := http.Get("https://api.example.com/data")
if err != nil {
fmt.Println("Error sending request:", err)
return
}
defer response.Body.Close()
// Read the response body
body, err := ioutil.ReadAll(response.Body)
if err != nil {
fmt.Println("Error reading response:", err)
return
}
fmt.Println("API Response:", string(body))
}
This simple example demonstrates how to send an HTTP GET request to an API and process the response. Go's built-in HTTP client makes it easy to integrate with RESTful APIs, which is a common task in automation scripts.
5. Concurrency in Automation Scripts
For tasks that involve multiple operations that can be performed in parallel (such as downloading files or processing multiple items), Go's goroutines and channels provide a powerful way to implement concurrency.
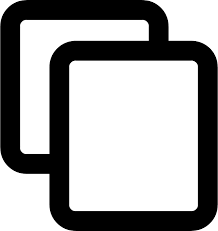
package main
import (
"fmt"
"time"
)
func fetchData(id int, ch chan string) {
fmt.Printf("Fetching data for task %d\n", id)
time.Sleep(time.Second) // Simulate network delay
ch <- fmt.Sprintf("Data for task %d", id)
}
func main() {
ch := make(chan string)
// Launch multiple goroutines
for i := 1; i <= 3; i++ {
go fetchData(i, ch)
}
// Collect results
for i := 1; i <= 3; i++ {
fmt.Println(<-ch)
}
}
In this example, multiple goroutines are launched to fetch data concurrently. The results are collected through a channel and printed once all tasks have completed. This demonstrates how Go's concurrency model can be leveraged to speed up tasks in automation scripts.
6. Automating System Administration Tasks
Go provides packages for interacting with the underlying operating system, making it suitable for automating system administration tasks like managing processes, interacting with the file system, and running shell commands.
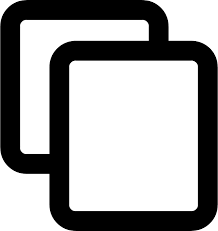
package main
import (
"fmt"
"os/exec"
)
func main() {
// Execute a shell command
out, err := exec.Command("ls", "-l").Output()
if err != nil {
fmt.Println("Error executing command:", err)
return
}
fmt.Println("Command output:", string(out))
}
This script runs a shell command using Go's exec
package and prints the output. You can extend this approach to automate more complex system tasks, such as managing services, scheduling jobs, or manipulating system configurations.
7. Automating Cloud Infrastructure Management
Go is a popular choice for cloud-native development, and many cloud service providers offer Go SDKs. For example, the AWS SDK for Go allows you to automate tasks like creating resources, managing services, and processing data in the cloud.
Automating cloud infrastructure management with Go can help you provision resources, configure services, monitor usage, and handle deployments programmatically.
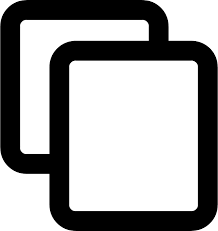
package main
import (
"fmt"
"log"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
// Create a new session
sess, err := session.NewSession(&aws.Config{
Region: aws.String("us-west-2")},
)
if err != nil {
log.Fatal("Failed to create session:", err)
}
// Create an S3 service client
svc := s3.New(sess)
// List buckets
result, err := svc.ListBuckets(nil)
if err != nil {
log.Fatal("Unable to list buckets:", err)
}
fmt.Println("Buckets:")
for _, bucket := range result.Buckets {
fmt.Println(*bucket.Name)
}
}
This example demonstrates how you can automate cloud infrastructure tasks like listing S3 buckets using the AWS SDK for Go. Similar SDKs exist for other cloud platforms like GCP and Azure.
8. Best Practices for Writing Automation Scripts in Go
- Modularize your code: Break your scripts into smaller, reusable functions to improve maintainability and readability.
- Handle errors gracefully: Always check for errors when interacting with files, network resources, or external services to avoid unexpected failures.
- Use concurrency where appropriate: Use goroutines and channels to parallelize tasks, especially for I/O-bound operations such as fetching data from multiple sources.
- Optimize for readability: While Go offers power and flexibility, make sure your automation scripts are easy to read and maintain by following Go's best practices for code style.
9. Conclusion
Go is an excellent choice for writing automation scripts. Its speed, concurrency model, and ease of use make it well-suited for tasks ranging from simple file manipulation to complex cloud infrastructure automation. By leveraging Go’s powerful standard library and concurrency features, you can create robust and efficient automation scripts to streamline your development and operations workflows.
Writing Go Scripts for Deployment and Monitoring
1. Introduction to Deployment and Monitoring with Go
Go is widely used for building scalable web applications, microservices, and cloud-native systems. It is also an excellent choice for writing scripts that can handle deployment and monitoring tasks. In this section, we will explore how Go can be used to automate deployment processes and monitor system performance or service health.
2. Why Use Go for Deployment and Monitoring?
Go offers several advantages that make it a strong choice for deployment and monitoring scripts:
- Speed: Go is a compiled language, which means that deployment scripts written in Go are often faster than those written in interpreted languages like Python or Bash.
- Cross-platform: Go can be compiled into static binaries that run on different operating systems without requiring additional runtime dependencies.
- Concurrency: Go's goroutines and channels make it easy to handle multiple tasks concurrently, which is especially useful for monitoring systems or managing multiple deployment steps in parallel.
- Cloud-Native Support: Go is commonly used in cloud-native environments and integrates well with cloud services, container orchestration platforms like Kubernetes, and infrastructure-as-code tools.
3. Writing Deployment Scripts in Go
Go can be used to automate different stages of the deployment pipeline, such as building and deploying applications to cloud platforms, managing containers, and performing post-deployment checks.
3.1 Automating Docker Container Deployment
Go can be used to automate the deployment of Docker containers. You can interact with Docker using the docker/docker
Go client package, which allows you to build, run, and manage Docker containers programmatically.
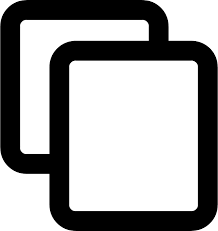
package main
import (
"fmt"
"log"
"github.com/docker/docker/api/types"
"github.com/docker/docker/client"
)
func main() {
// Create a new Docker client
cli, err := client.NewClientWithOpts(client.WithAPIVersionNegotiation())
if err != nil {
log.Fatal("Error creating Docker client:", err)
}
// List containers
containers, err := cli.ContainerList(context.Background(), types.ContainerListOptions{})
if err != nil {
log.Fatal("Error listing containers:", err)
}
fmt.Println("Running containers:")
for _, container := range containers {
fmt.Println(container.ID)
}
}
This example demonstrates using Go to interact with Docker and list currently running containers. Similar logic can be used to automate container builds, pushes, pulls, and deployments as part of a CI/CD pipeline.
3.2 Managing Cloud Deployments
If you're deploying to cloud services like AWS, GCP, or Azure, Go SDKs for these platforms allow you to automate infrastructure management and deployment tasks. For example, you can use the AWS SDK for Go to provision EC2 instances, deploy Lambda functions, or interact with S3 buckets.
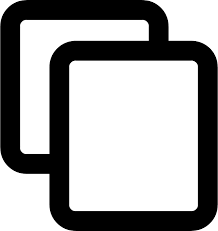
package main
import (
"fmt"
"log"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/ec2"
)
func main() {
// Create an AWS session
sess, err := session.NewSession(&aws.Config{
Region: aws.String("us-west-2")},
)
if err != nil {
log.Fatal("Error creating session:", err)
}
// Create EC2 service client
svc := ec2.New(sess)
// Describe EC2 instances
result, err := svc.DescribeInstances(nil)
if err != nil {
log.Fatal("Error describing instances:", err)
}
fmt.Println("EC2 Instances:")
for _, reservation := range result.Reservations {
for _, instance := range reservation.Instances {
fmt.Println(*instance.InstanceId)
}
}
}
This script demonstrates how to interact with AWS EC2 instances using Go. You can extend this to automate the deployment of AWS resources such as EC2 instances, RDS databases, or Lambda functions in your deployment pipeline.
4. Writing Monitoring Scripts in Go
Monitoring is a critical part of ensuring the health and performance of deployed applications. Go’s concurrency model and rich ecosystem of libraries make it easy to write monitoring scripts that can periodically check the status of applications, services, or infrastructure.
4.1 Monitoring HTTP Service Health
To monitor the health of a web service or API, you can write a Go script that periodically makes HTTP requests to check the service’s health endpoint (commonly known as a "health check") and logs the status.
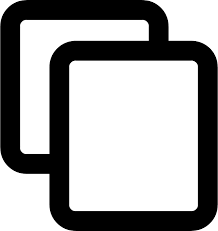
package main
import (
"fmt"
"net/http"
"time"
)
func checkHealth(url string) {
resp, err := http.Get(url)
if err != nil {
fmt.Println("Error checking health:", err)
return
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusOK {
fmt.Println("Service is healthy!")
} else {
fmt.Printf("Service returned status: %d\n", resp.StatusCode)
}
}
func main() {
for {
checkHealth("http://example.com/health")
time.Sleep(30 * time.Second)
}
}
This script checks the health of a service by sending an HTTP GET request to the health endpoint. If the service is healthy, it prints a success message. If not, it logs the returned status code. This type of script is useful for monitoring the availability of services or endpoints in your infrastructure.
4.2 Monitoring System Resource Usage
Go can also be used to monitor system resources such as CPU, memory, and disk usage. The github.com/shirou/gopsutil
package provides a cross-platform way to gather information about system performance.
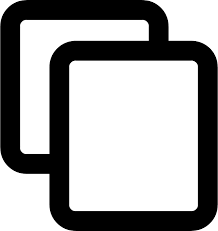
package main
import (
"fmt"
"log"
"github.com/shirou/gopsutil/cpu"
"github.com/shirou/gopsutil/mem"
)
func main() {
// Get CPU usage
cpuPercent, err := cpu.Percent(0, true)
if err != nil {
log.Fatal("Error getting CPU usage:", err)
}
fmt.Println("CPU Usage:", cpuPercent)
// Get memory usage
vmStat, err := mem.VirtualMemory()
if err != nil {
log.Fatal("Error getting memory usage:", err)
}
fmt.Println("Memory Usage:", vmStat.UsedPercent)
}
This script monitors CPU and memory usage using the gopsutil
package. It’s helpful for writing scripts that can alert you when system resources exceed certain thresholds or when performance issues occur.
5. Automating Deployment Rollbacks
In the event of a failed deployment, it’s important to have a mechanism for rolling back to a previous stable state. Go can help automate deployment rollback scripts, whether it's rolling back a Docker container, a cloud resource, or a database migration.
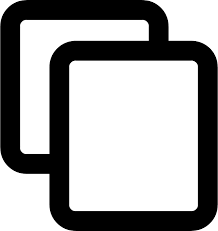
package main
import (
"fmt"
"log"
"os/exec"
)
func rollbackDeployment() {
// Example: Rollback a Docker container
cmd := exec.Command("docker", "rollback", "my-app")
err := cmd.Run()
if err != nil {
log.Fatal("Error during rollback:", err)
}
fmt.Println("Deployment rollback successful!")
}
func main() {
rollbackDeployment()
}
This script demonstrates how you can automate the rollback of a deployment using Docker. Similar rollback mechanisms can be implemented for cloud resources or database migrations in a Go script.
6. Conclusion
Go is an excellent language for writing deployment and monitoring scripts due to its speed, concurrency model, and ease of integration with cloud platforms and other services. By leveraging Go, you can automate deployment pipelines, monitor the health of your applications, and ensure the performance and stability of your infrastructure. Additionally, Go’s cross-platform capabilities and compiled nature make it a reliable choice for deploying scripts in production environments.
Integrating Go with CI/CD Pipelines
1. Introduction to CI/CD Pipelines
CI/CD (Continuous Integration/Continuous Deployment) is a key practice for modern software development. CI involves automatically testing and integrating code changes into a shared repository, while CD focuses on deploying those changes to production. Go is an ideal language for writing applications within CI/CD pipelines due to its speed, scalability, and ease of integration with various CI/CD tools.
2. Why Use Go in CI/CD Pipelines?
There are several reasons why Go is a great choice for CI/CD pipelines:
- Speed: Go compiles into a single binary, making it fast to deploy and run. This is particularly beneficial when automating build and test processes.
- Cross-Platform Support: Go binaries can run on different platforms without additional dependencies, which is useful when deploying in diverse environments like test, staging, and production.
- Concurrency: Go's goroutines and channels allow you to handle multiple tasks concurrently, such as running tests, building artifacts, and deploying services.
- Integration with Other Tools: Go can easily integrate with popular CI/CD tools like Jenkins, GitLab CI, GitHub Actions, and CircleCI, among others.
3. Setting Up Go in a CI/CD Pipeline
To integrate Go with a CI/CD pipeline, you'll need to configure the pipeline to run Go commands as part of the build and deployment process. Common tasks include:
- Running Go tests
- Building Go applications
- Deploying Go applications to servers or cloud platforms
Let's explore how to set up Go in different CI/CD tools.
3.1 Setting Up Go with GitHub Actions
GitHub Actions is a popular CI/CD service for automating workflows directly within GitHub repositories. Here's an example of a simple GitHub Actions workflow for building and testing a Go project:
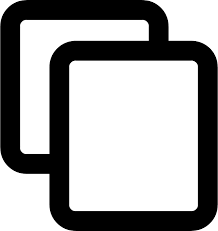
name: Go CI
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Go
uses: actions/setup-go@v2
with:
go-version: '1.18'
- name: Install dependencies
run: |
go mod tidy
- name: Run tests
run: |
go test ./...
- name: Build
run: |
go build -o myapp
This GitHub Actions workflow checks out the code, sets up the Go environment, installs dependencies, runs tests, and builds the application. The CI pipeline runs whenever there is a push or pull request to the main branch.
3.2 Setting Up Go with GitLab CI
GitLab CI is another popular CI/CD tool that integrates well with Go projects. Below is an example of a GitLab CI pipeline configuration for building and testing a Go project:
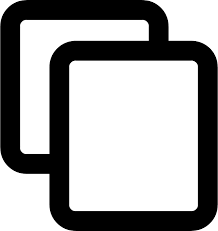
stages:
- build
- test
variables:
GO_VERSION: "1.18"
before_script:
- apt-get update -y
- apt-get install -y golang-go
build:
stage: build
script:
- go mod tidy
- go build -o myapp
test:
stage: test
script:
- go test ./...
This configuration defines two stages: build and test. It sets up the Go environment, installs dependencies, builds the Go binary, and runs tests in the test stage.
3.3 Setting Up Go with Jenkins
Jenkins is a widely used open-source CI/CD automation server. You can integrate Go into Jenkins using a Jenkins pipeline (Jenkinsfile). Here's an example of a Jenkins pipeline that builds and tests a Go application:
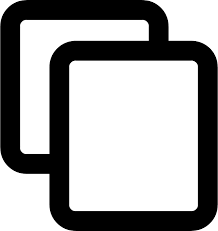
pipeline {
agent any
environment {
GO_VERSION = '1.18'
}
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-username/your-repository.git'
}
}
stage('Build') {
steps {
script {
sh 'go mod tidy'
sh 'go build -o myapp'
}
}
}
stage('Test') {
steps {
script {
sh 'go test ./...'
}
}
}
}
}
This Jenkins pipeline defines three stages: Checkout (retrieves the repository), Build (installs dependencies and builds the Go binary), and Test (runs the Go tests).
4. Automating Go Deployment in CI/CD Pipelines
Besides testing and building, CI/CD pipelines can also automate the deployment of Go applications. You can deploy Go applications to cloud platforms, Kubernetes, or other server environments as part of the pipeline.
4.1 Deploying Go Applications to Kubernetes
To deploy a Go application to Kubernetes, you can integrate kubectl commands in the pipeline to deploy the built Go application to a Kubernetes cluster.
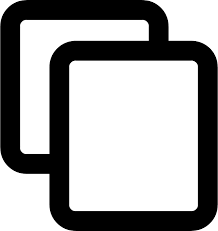
deploy:
stage: deploy
script:
- kubectl apply -f k8s/deployment.yaml
This GitLab CI pipeline step deploys the Go application to a Kubernetes cluster using kubectl. You can similarly automate deployments to AWS, GCP, Azure, or other cloud platforms by using their respective CLI tools in the pipeline.
4.2 Deploying Go Applications to AWS EC2
If you're deploying to an AWS EC2 instance, you can use the AWS CLI to copy the built Go binary to the EC2 instance and restart the service.
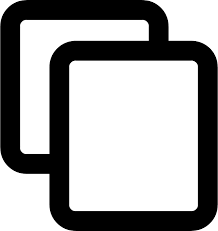
deploy:
stage: deploy
script:
- aws s3 cp myapp s3://my-bucket/myapp
- ssh ec2-user@my-ec2-instance 'cd /path/to/app && ./myapp'
In this pipeline, the Go binary is copied to an S3 bucket and then deployed to an EC2 instance via SSH.
5. Monitoring and Notifications in CI/CD Pipelines
CI/CD pipelines can also include steps for monitoring the deployment status and notifying team members of the results. For example, you can use Slack or email notifications to alert team members about successful builds, test failures, or deployment issues.
5.1 Slack Notifications in GitHub Actions
You can use the slackapi/slack-github-action
to send Slack notifications in GitHub Actions:
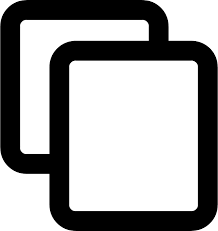
name: Go CI
on:
push:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Go
uses: actions/setup-go@v2
with:
go-version: '1.18'
- name: Run tests
run: |
go test ./...
- name: Notify Slack
uses: slackapi/slack-github-action@v1.0.0
with:
channel: '#build-notifications'
text: 'Go CI build completed!'
This example sends a Slack message after a Go build completes. You can similarly configure other notification systems to alert stakeholders of build, test, or deployment results.
6. Conclusion
Integrating Go into CI/CD pipelines enhances the automation and efficiency of build, test, and deployment processes. Whether you're using tools like GitHub Actions, GitLab CI, Jenkins, or others, Go's performance, ease of integration, and cross-platform capabilities make it an excellent choice for CI/CD workflows. With Go, you can streamline the development lifecycle, reduce manual errors, and increase the speed of deploying new features and fixes to production.
Writing Command-Line Tools in Go
1. Introduction to Command-Line Tools
Command-line tools (CLIs) are programs that are run from the terminal or command prompt. They are typically used for automating tasks, processing files, interacting with system resources, and managing configurations. Go is a great language for writing command-line tools due to its simplicity, speed, and ability to easily compile into a single binary that can be run on different platforms.
2. Why Use Go for Command-Line Tools?
Go is a popular choice for building command-line tools for several reasons:
- Fast Compilation: Go compiles directly to a single binary, which can be distributed and run on different platforms without dependencies.
- Cross-Platform: Go supports cross-compilation, allowing you to build tools for different operating systems from a single codebase.
- Easy to Learn: Go has a simple syntax, making it easy to pick up for building small to medium-sized utilities.
- Concurrency: Go’s built-in concurrency model (goroutines and channels) makes it well-suited for tools that need to manage multiple tasks simultaneously.
3. Setting Up a Command-Line Tool in Go
To create a command-line tool in Go, you need to structure your project, handle command-line arguments, and perform the necessary actions based on user input. Here’s an example of how to set up a simple CLI that accepts arguments and performs an action:
3.1 Hello World CLI
Let's start with a simple “Hello, World!” command-line tool:
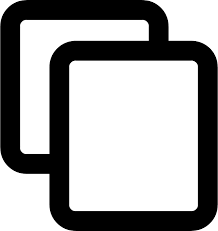
package main
import (
"fmt"
"os"
)
func main() {
if len(os.Args) > 1 {
fmt.Println("Hello,", os.Args[1])
} else {
fmt.Println("Hello, World!")
}
}
This tool prints "Hello, World!" by default. If you provide an argument when running the tool, it will greet the user by name. For example, running go run main.go John
will output "Hello, John".
3.2 Handling Multiple Commands with Cobra
For more complex CLIs, you can use libraries like Cobra to manage multiple commands and subcommands. Cobra provides an easy way to define flags, arguments, and subcommands in a clean and organized manner.
Here’s an example of using Cobra to create a simple CLI with a "greet" command:
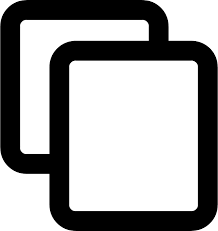
package main
import (
"fmt"
"os"
"github.com/spf13/cobra"
)
func main() {
var rootCmd = &cobra.Command{Use: "cli-tool"}
var greetCmd = &cobra.Command{
Use: "greet [name]",
Short: "Greets a user by name",
Args: cobra.MinimumNArgs(1),
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("Hello,", args[0])
},
}
rootCmd.AddCommand(greetCmd)
if err := rootCmd.Execute(); err != nil {
fmt.Println(err)
os.Exit(1)
}
}
This tool defines a "greet" command that accepts a name as an argument. You can run it with go run main.go greet John
, and it will output "Hello, John".
4. Working with Flags and Arguments
Command-line tools often need flags and arguments to customize their behavior. Flags are optional parameters that provide additional information or configuration to the tool. Arguments are usually required and specify the data that the tool should operate on.
4.1 Defining Flags
Flags can be defined using the flag
package or with third-party libraries like Cobra. Here’s an example of a tool that accepts a flag for verbosity:
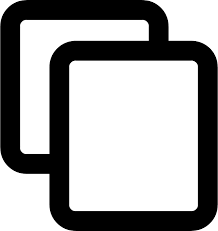
package main
import (
"fmt"
"flag"
)
func main() {
verbose := flag.Bool("verbose", false, "Enable verbose output")
flag.Parse()
if *verbose {
fmt.Println("Verbose mode is enabled")
} else {
fmt.Println("Verbose mode is disabled")
}
}
This code defines a --verbose
flag. Running the program with go run main.go --verbose
will enable verbose output.
4.2 Handling Arguments
Arguments can be processed using the os.Args
array (as shown earlier) or using libraries like Cobra for more advanced parsing. Here's an example that handles multiple arguments:
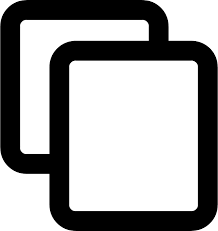
package main
import (
"fmt"
"os"
)
func main() {
if len(os.Args) < 3 {
fmt.Println("Usage: go run main.go ")
os.Exit(1)
}
name := os.Args[1]
age := os.Args[2]
fmt.Printf("Hello, %s. You are %s years old.\n", name, age)
}
This program expects two arguments: a name and an age. Running go run main.go John 30
will output "Hello, John. You are 30 years old."
5. Advanced CLI Features
Once you've created a basic CLI, you can add more advanced features such as environment variable parsing, configuration files, and logging. These features can make your tool more robust and user-friendly.
5.1 Reading from Configuration Files
Your CLI can read from configuration files (e.g., JSON, YAML, or TOML) to simplify the setup process. Here’s an example of reading a configuration file in Go:
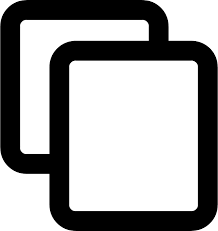
package main
import (
"fmt"
"os"
"encoding/json"
)
type Config struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
file, err := os.Open("config.json")
if err != nil {
fmt.Println("Error opening config file:", err)
os.Exit(1)
}
defer file.Close()
var config Config
decoder := json.NewDecoder(file)
if err := decoder.Decode(&config); err != nil {
fmt.Println("Error decoding config:", err)
os.Exit(1)
}
fmt.Printf("Hello, %s. You are %d years old.\n", config.Name, config.Age)
}
This CLI reads the configuration from a JSON file and prints a greeting based on the values in the file. You could expand this to support more complex configuration setups.
5.2 Logging
For better debugging and monitoring, it’s useful to log output from your CLI. You can use Go's built-in log
package for logging messages at different severity levels:
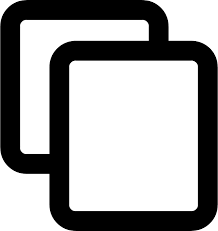
package main
import (
"fmt"
"log"
"os"
)
func main() {
log.SetOutput(os.Stdout)
log.Println("Starting the application")
if len(os.Args) < 2 {
log.Fatal("Missing required argument")
}
fmt.Println("Argument provided:", os.Args[1])
log.Println("Application finished successfully")
}
This example logs messages at various points, such as when the application starts, when an error occurs, and when the application finishes.
6. Packaging and Distributing Your Command-Line Tool
Once your CLI is ready, you may want to package it into a binary for distribution. Go makes this easy by allowing you to cross-compile for different platforms. For example, to build a binary for Linux, you can use:
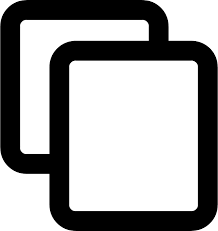
GOOS=linux GOARCH=amd64 go build -o mytool-linux
Similarly, you can build for Windows, macOS, or ARM architectures by adjusting the GOOS
and GOARCH
values.
7. Conclusion
Go is a powerful and efficient language for writing command-line tools. Whether you're building simple utilities or complex systems, Go’s ease of use, fast compilation, and cross-platform support make it an excellent choice for CLI development. By handling flags, arguments, logging, and configuration files, you can create robust and user-friendly tools that automate tasks and streamline workflows.
Writing Clean and Idiomatic Go Code
1. Introduction to Idiomatic Go
Writing clean and idiomatic Go code is essential for ensuring that your codebase is maintainable, readable, and efficient. Idiomatic Go refers to following the conventions and best practices that are commonly accepted by the Go community. It helps ensure that your code is consistent with the standard Go style, making it easier for others to understand, contribute to, and maintain.
2. Key Principles for Writing Clean Go Code
To write clean and idiomatic Go code, follow these key principles:
- Consistency: Stick to conventions and patterns commonly used in the Go community. This ensures your code is predictable and easy to understand.
- Readability: Write code that is easy to read and understand. Avoid unnecessary complexity and keep your code simple and clear.
- Efficiency: Use Go’s built-in features and libraries to write performant code. Avoid unnecessary allocations, and be mindful of concurrency and memory usage.
- Maintainability: Write code that is easy to maintain and extend. Keep functions small, and separate concerns into logical packages.
3. Go Code Style Guidelines
There are several style guidelines that are considered idiomatic for Go developers. Following these conventions ensures that your code looks familiar to others who read it, making collaboration easier.
3.1 Use Proper Naming Conventions
Go emphasizes simplicity and clarity in naming. Here are some naming conventions to follow:
- Function and variable names: Use short, descriptive names. Go uses camelCase for multi-word names, starting with a lowercase letter for unexported variables and functions, and with an uppercase letter for exported functions and variables.
- Packages: Package names should be short, lowercase, and descriptive of their contents. For example, the package
fmt
handles formatting, andhttp
handles HTTP requests. - Constants: Constants should be written in uppercase letters, with words separated by underscores, e.g.,
MAX_CONNECTIONS
.
3.2 Avoid Unnecessary Comments
While comments are important, avoid over-commenting your code. Your code should be self-explanatory, so use comments when necessary to explain why something is done, not what is done. It’s better to write clear and concise code than to rely on comments to explain complex logic.
3.3 Use Go’s Built-in Formatting
Go’s formatting tool, gofmt
, automatically formats your code according to Go’s official style guide. This helps ensure consistent indentation, spacing, and alignment across your codebase. You should run gofmt
on your code before committing it to version control.
3.4 Use Error Handling Properly
In Go, errors are handled explicitly. It is idiomatic to always check for errors after operations that can fail, such as opening a file or making an HTTP request. You should always handle errors promptly and appropriately, either by returning them or logging them.
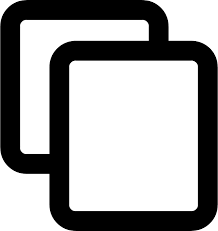
if err != nil {
log.Fatal(err)
}
4. Go Idioms for Clean Code
Go provides several idioms to help write clean and efficient code. These idioms are commonly used by experienced Go developers.
4.1 Defer for Cleanup
Go’s defer
statement is useful for cleanup, especially in functions that involve resource management (e.g., file handling, database connections). The deferred function will be executed when the surrounding function returns, ensuring that resources are released properly.
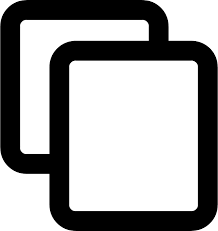
f, err := os.Open("file.txt")
if err != nil {
log.Fatal(err)
}
defer f.Close()
The defer f.Close()
line ensures that the file is closed when the function exits, even if an error occurs earlier in the function.
4.2 Use Interfaces for Flexibility
Interfaces in Go are a powerful feature that allows you to write flexible and reusable code. Instead of tightly coupling your code to specific types, define interfaces to abstract behavior and allow different types to implement them.
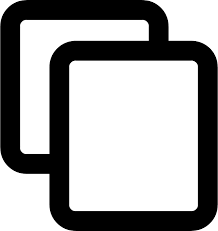
type Logger interface {
Log(message string)
}
type FileLogger struct {}
func (f *FileLogger) Log(message string) {
fmt.Println("File log:", message)
}
type ConsoleLogger struct {}
func (c *ConsoleLogger) Log(message string) {
fmt.Println("Console log:", message)
}
In this example, both FileLogger
and ConsoleLogger
implement the Logger
interface, allowing your code to work with either type interchangeably.
4.3 Avoid Global Variables
Global variables can make your code harder to maintain and test. They create hidden dependencies and can lead to unpredictable behavior. Where possible, prefer passing variables explicitly to functions or using dependency injection.
5. Writing Maintainable Code
In addition to adhering to Go’s idioms and style conventions, writing maintainable code involves structuring your codebase logically and organizing your packages effectively.
5.1 Keep Functions Small
Functions should be small and focused on a single task. If a function is doing too much, it can be hard to understand and maintain. Break down large functions into smaller, more manageable pieces.
5.2 Organize Code into Packages
Group related functions and types into separate packages. Each package should have a clear and well-defined responsibility. This makes your code easier to navigate and maintain in the long term.
5.3 Use Testing to Maintain Code Quality
Regularly write tests for your code to ensure its correctness and maintainability. Go’s built-in testing package makes it easy to write unit tests, and you can use tools like golint
and gocyclo
to analyze your code for potential issues.
6. Conclusion
Writing clean and idiomatic Go code is essential for producing reliable, maintainable, and efficient software. By following Go’s conventions for naming, error handling, and structuring code, and by adhering to best practices for writing clean code, you can ensure that your Go projects are easy to read, understand, and extend. Always aim for simplicity, consistency, and clarity in your code, and your Go code will be a joy to work with.
Go Code Formatting with gofmt
1. Introduction to Code Formatting in Go
In Go, code formatting plays a crucial role in ensuring that code is readable, consistent, and maintainable. Go's official code formatting tool, gofmt
, automatically formats Go source code according to a standard style guide, helping Go developers maintain uniformity in their codebase. Consistent formatting is essential, especially when collaborating with other developers or contributing to open-source projects.
2. The Importance of Consistent Code Formatting
Consistent code formatting makes the code easier to read and understand, reduces the chance of errors, and streamlines collaboration. Adhering to a common style guide eliminates debates over formatting preferences, allowing developers to focus on writing clean, effective code. The Go community has embraced gofmt
as the tool to enforce consistency across all Go projects.
3. What is gofmt?
gofmt
is the official Go formatting tool, which automatically formats Go source code files according to Go’s style rules. It ensures that all Go code in a project looks the same, regardless of the individual developer's preferences. gofmt
is included with the Go distribution, so you don't need to install any additional tools to use it.
4. How to Use gofmt
Using gofmt
is simple. You can run it from the command line or integrate it into your development environment.
4.1 Format a Single File
To format a single Go file, use the following command:
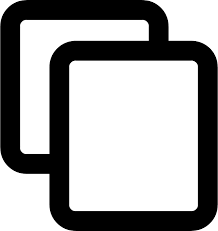
gofmt -w filename.go
The -w
flag tells gofmt
to overwrite the original file with the formatted version. If you omit the -w
flag, gofmt
will output the formatted code to the terminal instead of modifying the file.
4.2 Format All Go Files in a Directory
To format all Go files in the current directory (and subdirectories), run:
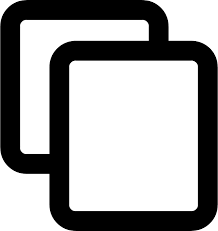
gofmt -w ./
4.3 Check for Formatting Differences
If you want to check whether a Go file is properly formatted without modifying it, you can use the -d
flag:
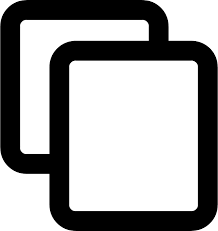
gofmt -d filename.go
This will display the differences between the original and the formatted code without changing the file.
5. Integrating gofmt into Your Workflow
To ensure that all code is consistently formatted, it’s a good idea to run gofmt
as part of your development workflow. Here are some ways to integrate gofmt
into your process:
5.1 Automate Formatting in Editors
Many Go-aware text editors and IDEs, such as Visual Studio Code, GoLand, and Vim, have built-in support for gofmt
or offer plugins that can automatically format code when you save a file. Enabling automatic formatting on save ensures that your code is always properly formatted without requiring manual intervention.
5.2 Include gofmt in Git Hooks
To ensure that all code committed to your repository is formatted correctly, you can use a Git pre-commit hook to run gofmt
before each commit. This ensures that code is consistently formatted across all contributors.
5.3 Use gofmt in Continuous Integration (CI)
Incorporate gofmt
into your CI pipeline to check if the codebase is consistently formatted. If the code is not properly formatted, the CI build can fail, prompting developers to fix the formatting before merging changes into the main branch.
6. Common gofmt Errors and Issues
Although gofmt
is very reliable, there are some common issues to be aware of:
- Unwanted Imports:
gofmt
will reorder your imports automatically, so it’s important to manually remove unused imports before running it. - Trailing Whitespace:
gofmt
will remove any unnecessary trailing whitespace in your files. - Formatting Conflicts: In cases where two developers have conflicting formatting preferences,
gofmt
will ensure that the final code adheres to Go’s official formatting style, resolving any conflicts.
7. Conclusion
Using gofmt
is an essential part of writing clean and idiomatic Go code. By ensuring that all Go code in a project follows a consistent formatting style, gofmt
helps eliminate debates over formatting preferences and makes the codebase more readable and maintainable. Make it a habit to run gofmt
on your code regularly, and integrate it into your workflow and CI pipeline to ensure that your Go code is always formatted according to the Go style guidelines.
Using Go Linters and Static Analysis Tools
1. Introduction to Linters and Static Analysis
In Go development, ensuring code quality goes beyond formatting. Linters and static analysis tools help identify potential issues, bugs, and areas for improvement before the code is executed. These tools analyze the source code without running it, identifying code smells, potential errors, and opportunities for optimization. Incorporating linters and static analysis into your workflow can significantly improve code quality and maintainability.
2. What Are Linters?
A linter is a tool that checks Go code for potential issues such as syntax errors, style violations, or suspicious constructs. It doesn’t execute the code but analyzes it to identify patterns or practices that might lead to bugs or inconsistencies. Linters help enforce coding standards and best practices, making code more readable, maintainable, and robust.
3. Popular Go Linters
Several linters are available for Go that can help you catch common mistakes and enforce coding standards. Some of the most popular Go linters include:
- golint: A simple Go linter that checks for style mistakes, such as improper naming conventions and missing comments.
- go vet: A tool that reports suspicious constructs, such as incorrect formatting or errors that could cause issues at runtime.
- gocyclo: This linter checks for complex functions and methods, helping to keep code simple and maintainable.
- staticcheck: A comprehensive static analysis tool that combines multiple linters, reporting potential bugs, code smells, and style violations.
4. How to Use Go Linters
To use a Go linter, you generally need to install it first. Below are some examples of how to install and use common linters:
4.1 Installing golint
To install golint, use the following command:
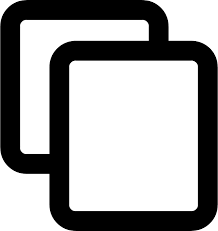
go install golang.org/x/lint/golint@latest
Once installed, you can run it on your Go project directory with:
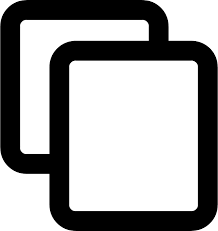
golint ./...
This will check all Go files in the current directory and its subdirectories for style violations.
4.2 Installing go vet
go vet
is part of the Go toolchain, so you don't need to install it separately. To run it, use the following command:
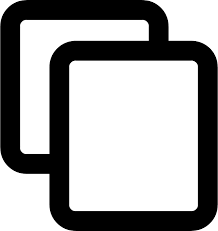
go vet ./...
This command will check your Go files for various potential issues, such as misuse of certain functions or incorrect format strings.
4.3 Installing staticcheck
To install staticcheck
, use the following command:
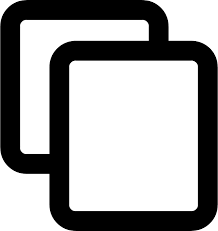
go install honnef.co/go/tools/cmd/staticcheck@latest
Then, run it with:
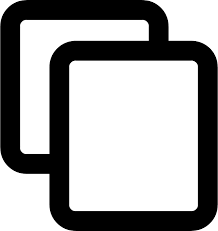
staticcheck ./...
This will run a comprehensive static analysis of your project, reporting common bugs, inefficiencies, and unnecessary complexity.
5. Integrating Linters into Your Development Workflow
To ensure that linters are used consistently throughout your project, integrate them into your development workflow:
5.1 Automating Linters in Editors
Many text editors and IDEs, like Visual Studio Code, GoLand, and Vim, offer plugins that integrate linters directly into the editor. These plugins can automatically run linters as you type, providing real-time feedback and highlighting issues before you even commit the code.
5.2 Using Linters in Pre-Commit Hooks
To ensure that code is linted before being committed, use Git pre-commit hooks. This guarantees that only code that passes the linting checks is allowed to be committed to the repository. For example, you can use a tool like pre-commit
to configure and automate the linter checks before commits:
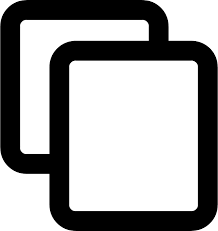
pre-commit install
Then, add configuration for the Go linters in the .pre-commit-config.yaml
file.
5.3 Integrating Linters in CI/CD Pipelines
Integrating linters into your CI/CD pipeline ensures that code is linted before being merged into the main branch. If the code doesn't pass the linter checks, the build can fail, preventing style violations or potential bugs from entering the codebase. Here's an example of how to integrate a linter into a CI pipeline using GitHub Actions:
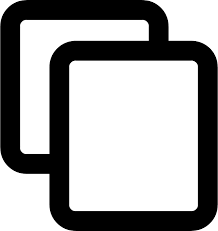
name: Go Linter
on:
pull_request:
paths:
- '**/*.go'
jobs:
lint:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Install golint
run: go install golang.org/x/lint/golint@latest
- name: Run golint
run: golint ./...
6. Static Analysis Tools
In addition to linters, static analysis tools provide a deeper analysis of your code, looking for potential bugs, inefficiencies, and other issues that linters may miss. These tools analyze your code's structure and logic, often identifying bugs that might only become apparent at runtime.
6.1 Using staticcheck
staticcheck
is a powerful static analysis tool that combines multiple checks, including common bugs, style violations, and performance issues. It integrates multiple linters into one, providing a comprehensive review of the codebase.
6.2 Other Static Analysis Tools
Some other static analysis tools that can be used alongside Go linters include:
- gosimple: Finds simple code patterns and suggests improvements.
- goconst: Detects repeated constants in Go code.
- errcheck: Identifies unchecked errors in Go code.
7. Benefits of Using Go Linters and Static Analysis
- Early Bug Detection: Linters help detect potential bugs early in the development cycle, making it easier to fix issues before they become critical.
- Enforcing Best Practices: Linters encourage consistent coding practices, improving the overall quality and maintainability of the codebase.
- Improved Code Readability: Static analysis tools help identify overly complex or inefficient code, encouraging developers to write cleaner, more readable code.
- Code Consistency: Linters help enforce a consistent style across the entire codebase, reducing disagreements over formatting and style.
8. Conclusion
Using Go linters and static analysis tools is an essential part of maintaining high-quality, maintainable, and bug-free code. Integrating these tools into your development workflow, either by automating them in your editor, using pre-commit hooks, or incorporating them into CI/CD pipelines, ensures that your code remains clean and consistent. As your codebase grows, these tools become invaluable in catching issues early and enforcing best practices across the team.
Code Organization and Project Structure
1. Introduction to Code Organization
Proper code organization is essential for building maintainable, scalable, and easy-to-understand Go projects. A well-structured project not only makes development easier but also ensures that other developers can understand and contribute to the project more effectively. A good project structure helps in organizing code logically, separating concerns, and ensuring that the application can scale smoothly as it grows.
2. Why Code Organization Matters
- Scalability: A well-organized project structure allows the application to scale with ease as new features are added.
- Maintainability: Code that is logically grouped and organized is easier to maintain, debug, and refactor.
- Collaboration: A consistent structure makes it easier for multiple developers to work on the same codebase without confusion.
- Testing: Proper organization facilitates unit testing, integration testing, and ensures that dependencies are handled correctly.
3. Common Go Project Structure
There is no one-size-fits-all structure for Go projects, but following a standard structure can help improve code quality and consistency. Below is a commonly used structure for Go projects:
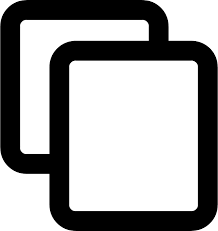
my-go-project/
├── cmd/ # Entry points for applications (main packages)
├── pkg/ # Library code (shared by applications)
├── internal/ # Private code (not importable by other projects)
├── api/ # API definitions and versions (optional)
├── web/ # Web server and frontend code (optional)
├── scripts/ # Helper scripts for builds, deployments, etc.
├── configs/ # Configuration files
├── test/ # Tests (integration and unit tests)
├── go.mod # Go module definition
├── go.sum # Go checksum file
3.1 cmd/
The cmd/
directory contains the entry point for different applications within the project. Each application should have its own directory under cmd/
with a main.go
file. This structure allows for multiple applications (e.g., a web server and a CLI tool) to coexist within the same codebase.
3.2 pkg/
The pkg/
directory is used for shared library code that is meant to be imported by other projects or applications. This directory can contain packages with reusable components that are not specific to one application. For example, a utility package for logging or database connection management can be placed here.
3.3 internal/
The internal/
directory is reserved for code that should not be imported by other projects. This can be used for implementation details or private packages that are meant to stay within the project. Go enforces this restriction by preventing external code from importing anything within the internal/
directory.
3.4 api/
The api/
directory contains API definitions, including protocol definitions, GraphQL schemas, or gRPC service definitions. This directory is useful for projects that expose an API for communication with other services or clients.
3.5 web/
The web/
directory is often used for the web server code and related frontend assets. This includes handlers, templates, CSS, JavaScript, and static files. If your project includes a web frontend, it’s common to put the server-related code in this directory.
3.6 scripts/
The scripts/
directory contains helper scripts for automation tasks like building the project, deploying it, or running tests. These scripts simplify the management of repetitive tasks like creating releases or setting up development environments.
3.7 configs/
The configs/
directory is where configuration files (such as JSON, YAML, or TOML files) are stored. These configuration files can be used by different components of the application to manage environment-specific settings, such as database credentials or API keys.
3.8 test/
The test/
directory contains test-specific code, such as integration tests, mocks, and test fixtures. This is where tests that require external resources, such as a database or a web service, can be placed.
4. Go Modules and Dependency Management
Go introduced modules with Go 1.11 to simplify dependency management. Every Go project should have a go.mod
file in its root directory, which defines the project's dependencies and versioning. The go.sum
file, generated automatically by Go, ensures that dependencies are fetched in a consistent and secure manner.
5. Structuring Large Go Projects
For larger Go projects, it’s important to structure code in a way that promotes separation of concerns, making it easier to scale, manage dependencies, and implement features. Here are some tips:
- Modularize your code: Split functionality into smaller modules or packages, which can be independently developed and tested. This helps with code organization, and code reuse, and allows teams to work in parallel.
- Separate business logic from infrastructure code: Keep business logic in separate packages from infrastructure concerns like database connections, API handlers, and service clients.
- Use interfaces to decouple components: Interfaces in Go allow you to decouple components and abstract away implementation details. This makes testing easier and promotes flexibility in your design.
- Stick to the Go idioms: Follow Go's conventions for file naming, package structure, and documentation. This ensures consistency across the codebase and makes it easier for other Go developers to contribute to the project.
6. Keeping Your Codebase Clean and Maintainable
A well-organized project structure is only part of maintaining a clean and maintainable codebase. You also need to establish good practices for code readability, test coverage, and documentation:
- Write clear and concise code: Avoid overly complex or convoluted code. Keep things simple and clear, so your code is easy to read and understand.
- Write comprehensive tests: Unit tests, integration tests, and end-to-end tests help ensure that your code works as expected and can handle edge cases.
- Document your code: Use Go's documentation tools like
godoc
to generate inline documentation for your packages, functions, and methods. This helps others understand how to use your code and reduces the need for long README files. - Refactor regularly: As your codebase grows, it’s important to refactor and reorganize code to keep it clean and maintainable. Don’t let technical debt accumulate over time.
7. Conclusion
Having a well-organized project structure is crucial for building scalable, maintainable, and efficient Go applications. By following a logical structure and adhering to Go's best practices, your project will be easier to manage, collaborate on, and extend in the future. Whether you’re building a small tool or a large enterprise application, ensuring that your code is well-organized will save time and effort in the long run.
Optimizing Go Code for Scalability and Maintainability
1. Introduction
Optimizing Go code for scalability and maintainability is crucial for creating applications that can handle growth in both traffic and complexity. Scalability ensures that your application can handle an increasing number of users or requests, while maintainability ensures that the code remains readable, testable, and easy to modify as your application evolves.
2. Key Principles for Optimizing Go Code
- Separation of Concerns: Organize the code into distinct modules or packages, each responsible for a specific functionality. This makes it easier to scale components independently and manage complexity.
- Code Reusability: Write functions, methods, and types that are reusable across different parts of the application. This reduces code duplication and simplifies maintenance.
- Efficient Memory Management: Go’s garbage collector handles memory management, but it's still important to minimize memory allocations and manage memory effectively, especially in high-performance applications.
- Concurrency: Leverage Go’s goroutines and channels for handling concurrency efficiently. Proper use of concurrency allows you to scale applications to handle thousands or even millions of simultaneous tasks.
- Testing and Documentation: Ensure that your code is thoroughly tested and well-documented. This helps with maintainability and makes it easier for other developers to understand the code and contribute to it.
3. Optimizing for Scalability
Scalability is the ability of your application to handle increased load, whether it's more users, data, or requests. Here are a few ways to optimize Go code for scalability:
3.1 Use Concurrency and Parallelism
Go's goroutines and channels make it easy to write concurrent code. By using goroutines to handle multiple tasks simultaneously, you can significantly improve the scalability of your application. Here's an example of using goroutines for concurrency:
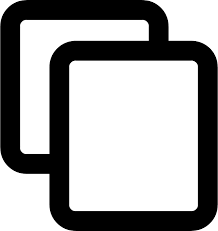
go func() {
// Simulate work in a goroutine
fmt.Println("This is a concurrent task")
}()
3.2 Load Balancing
In distributed systems, load balancing allows you to evenly distribute traffic across multiple servers. Go provides libraries such as net/http
that can be used to implement load balancing strategies for scaling web applications.
3.3 Use Caching for Frequently Accessed Data
In high-load applications, caching frequently accessed data can significantly improve performance and scalability. Consider using tools like Redis or Memcached to store data in memory, reducing the load on the database.
3.4 Efficient Database Queries
Optimize database queries to reduce load times and improve scalability. Use indexing, pagination, and batching techniques to handle large datasets efficiently. Avoid N+1 query problems by fetching related data in a single query.
4. Optimizing for Maintainability
Maintainability ensures that your code can be easily understood, modified, and extended by other developers. Here are some strategies to optimize Go code for maintainability:
4.1 Follow Go Code Conventions
Following Go's coding conventions (such as naming conventions, formatting, and file organization) helps make your code more readable and easier to maintain. This includes using gofmt
for formatting your code and adhering to the Go idioms.
4.2 Keep Functions Short and Focused
One of the key principles of clean code is that functions should do one thing and do it well. Keeping functions small and focused makes them easier to test and maintain. Functions that perform multiple tasks should be split into smaller, more manageable pieces.
4.3 Use Clear Naming Conventions
Descriptive names for variables, functions, and types help to communicate the purpose of the code. Avoid abbreviations and use meaningful names that provide clarity. For example, use getUserData()
instead of gud()
for function names.
4.4 Write Tests
Unit tests and integration tests help ensure that your code works as expected and prevent regressions when making changes. Writing tests makes it easier to maintain your codebase as it grows. Use Go’s testing
package to write tests that cover different use cases.
4.5 Modularize the Code
Group related functionality into packages or modules. This helps with code organization and makes it easier to manage dependencies. A well-organized codebase with clear boundaries between modules is easier to maintain and scale.
4.6 Use Interfaces for Flexibility
Interfaces in Go allow you to decouple components and make your code more flexible. Instead of tightly coupling your code to specific implementations, define interfaces that can be implemented by different types. This promotes easier testing and allows you to swap out implementations without breaking your code.
5. Practical Tips for Improving Scalability and Maintainability
- Refactor Regularly: Don’t wait for the code to become unmanageable. Refactor regularly to improve code quality and keep the codebase clean.
- Use Dependency Injection: This allows for easier testing and better separation of concerns. By injecting dependencies, you can avoid tightly coupling components together.
- Monitor Performance: Use Go's built-in profiling tools (e.g.,
pprof
) to monitor the performance of your application. Identify bottlenecks and optimize them to improve scalability. - Optimize Network Calls: Reduce the number of network calls and ensure that your application handles connections efficiently. Consider using connection pooling or persistent connections for HTTP clients.
- Leverage Goroutines Wisely: While goroutines are lightweight, creating too many goroutines in a short period of time can overwhelm your system. Make sure to use channels for proper synchronization and avoid resource exhaustion.
6. Conclusion
Optimizing Go code for scalability and maintainability is an ongoing process that requires careful consideration of design, performance, and code quality. By following best practices such as leveraging concurrency, maintaining clean code, and using Go-specific features like goroutines and channels, you can build applications that can scale with increasing demand while remaining easy to maintain and extend.
Building a To-Do List Application
1. Introduction
Building a To-Do List application is a great way to learn the basics of web development and application design. In this section, we will walk through how to build a simple To-Do List application in Go, focusing on how to structure the code, manage tasks, and persist them using a basic file storage or in-memory database.
2. Key Features of the To-Do List Application
- Adding Tasks: Allow users to add new tasks to the to-do list.
- Viewing Tasks: Display the list of tasks that have been added.
- Marking Tasks as Completed: Enable users to mark tasks as completed.
- Deleting Tasks: Allow users to delete tasks from the list.
- Task Persistence: Store tasks in a file or database so that they are not lost when the application is restarted.
3. Setting Up the Project
First, create a new Go project for the To-Do List application. You will need to set up a Go module and create the necessary files for the project.
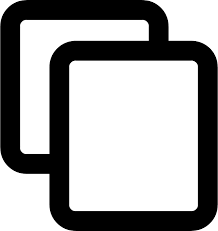
mkdir todo-app
cd todo-app
go mod init todo-app
touch main.go
4. Structuring the Code
The application will require a few basic components:
- Task Struct: A data structure to represent a task.
- Task Management Functions: Functions to add, delete, view, and update tasks.
- Storage Mechanism: A way to store the tasks (using a simple file or in-memory). For simplicity, we will use in-memory storage in this example.
- HTTP Handlers: To interact with the user through a web interface (optional for this example).
5. Task Struct
Define a Task
struct to store the task details:
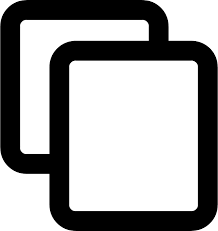
type Task struct {
ID int
Description string
Completed bool
}
6. Task Management Functions
Next, create functions to manage the tasks. These functions will allow users to add, delete, and update tasks.
6.1 Adding a Task
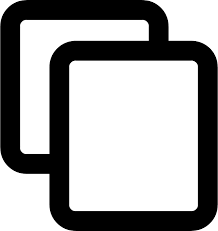
var tasks []Task
var nextID int
func addTask(description string) Task {
nextID++
task := Task{ID: nextID, Description: description, Completed: false}
tasks = append(tasks, task)
return task
}
6.2 Marking a Task as Completed
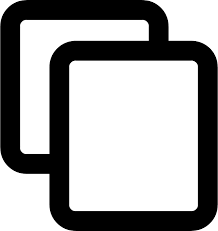
func markTaskCompleted(id int) bool {
for i, task := range tasks {
if task.ID == id {
tasks[i].Completed = true
return true
}
}
return false
}
6.3 Deleting a Task
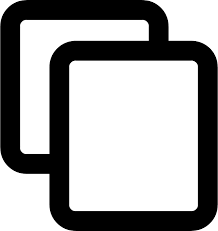
func deleteTask(id int) bool {
for i, task := range tasks {
if task.ID == id {
tasks = append(tasks[:i], tasks[i+1:]...)
return true
}
}
return false
}
7. Storing Tasks
For simplicity, we are storing tasks in memory. However, you can easily extend this to store tasks in a file or a database.
8. Building the Web Interface
Now, let’s set up a simple HTTP server to interact with the To-Do List application through the web.
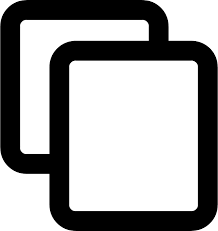
package main
import (
"fmt"
"net/http"
"html/template"
)
func main() {
http.HandleFunc("/", homeHandler)
http.ListenAndServe(":8080", nil)
}
func homeHandler(w http.ResponseWriter, r *http.Request) {
tmpl := template.Must(template.New("index").Parse(`
To-Do List
{{range .}}
- {{.Description}} - {{if .Completed}}Completed{{else}}Not Completed{{end}}
{{end}}
`))
tmpl.Execute(w, tasks)
}`
9. Handling Form Submissions
In the form, when the user adds a task, a POST request is sent to the server. We can handle this in the addTask
handler.
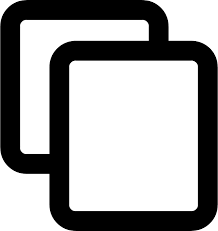
func addTaskHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPost {
taskDescription := r.FormValue("task")
task := addTask(taskDescription)
fmt.Fprintf(w, "Task Added: %v", task)
}
}
10. Running the Application
To run the application, use the following command:
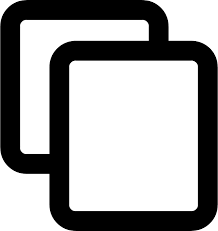
go run main.go
Your to-do list application will be accessible at http://localhost:8080
.
11. Conclusion
In this tutorial, we built a simple To-Do List application in Go, including task management functionality and an optional web interface. This basic application demonstrates how to structure Go code for managing simple tasks, handle form submissions, and serve an HTTP interface. You can further improve this application by adding more features such as task persistence (using files or databases) and more advanced user interactions.
Building a File Backup Utility
1. Introduction
Building a file backup utility is a practical use case for learning how to work with files, directories, and error handling in Go. In this section, we will walk through how to build a simple utility that allows users to back up files from one directory to another, either locally or to a remote server.
2. Key Features of the Backup Utility
- Source and Destination Directories: Allow users to specify a source directory containing files to be backed up and a destination directory where the backups will be stored.
- File Copying: Copy files from the source to the destination directory, preserving the original file structure.
- Overwriting Behavior: Allow users to specify whether existing files in the destination should be overwritten or not.
- Logging and Error Handling: Log the success or failure of each file backup and handle any errors that may arise during the copying process.
- Backup Scheduling (Optional): Implement a feature to schedule backups to run at specific intervals (e.g., using cron jobs or Go's time package).
3. Setting Up the Project
Create a new Go project for the file backup utility. Set up your Go module and create the necessary files:
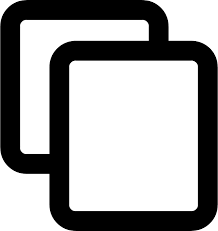
mkdir file-backup
cd file-backup
go mod init file-backup
touch main.go
4. Structuring the Code
The main components of the backup utility will include:
- Backup Function: A function to copy files from the source directory to the destination directory.
- Directory Traversal: Functions to traverse the source directory and identify files that need to be backed up.
- Error Handling: Logic to handle errors (e.g., file not found, permission issues) during the backup process.
- Logging: A simple logging mechanism to track the backup status of each file.
5. Directory Traversal
We'll begin by creating a function to traverse the source directory and get a list of files that need to be backed up.
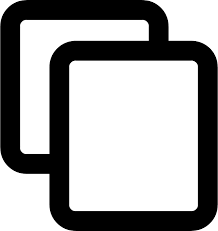
import (
"os"
"path/filepath"
)
func getFilesToBackup(sourceDir string) ([]string, error) {
var files []string
err := filepath.Walk(sourceDir, func(path string, info os.FileInfo, err error) error {
if err != nil {
return err
}
if !info.IsDir() {
files = append(files, path)
}
return nil
})
if err != nil {
return nil, err
}
return files, nil
}
6. Copying Files
Next, we'll create a function to copy each file from the source to the destination directory. We'll preserve the file structure in the destination.
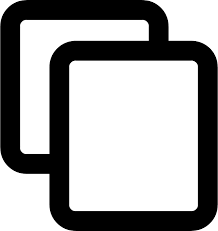
import (
"io"
"os"
"path/filepath"
)
func copyFile(sourcePath, destPath string) error {
sourceFile, err := os.Open(sourcePath)
if err != nil {
return err
}
defer sourceFile.Close()
destFile, err := os.Create(destPath)
if err != nil {
return err
}
defer destFile.Close()
_, err = io.Copy(destFile, sourceFile)
return err
}
7. Backup Function
Now, let's create the main backup function that will use the functions we created above to back up all files from the source to the destination directory.
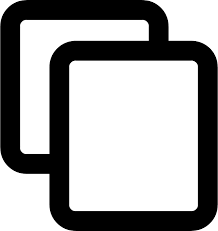
func backupFiles(sourceDir, destDir string) error {
files, err := getFilesToBackup(sourceDir)
if err != nil {
return err
}
for _, file := range files {
relativePath, err := filepath.Rel(sourceDir, file)
if err != nil {
return err
}
destPath := filepath.Join(destDir, relativePath)
destDirPath := filepath.Dir(destPath)
err = os.MkdirAll(destDirPath, os.ModePerm)
if err != nil {
return err
}
err = copyFile(file, destPath)
if err != nil {
return err
}
}
return nil
}
8. Logging the Backup Process
We can add basic logging to track the success or failure of each backup operation. For simplicity, we'll log the results to the console.
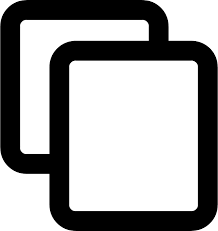
import (
"fmt"
)
func logBackupStatus(file string, err error) {
if err != nil {
fmt.Printf("Failed to backup %s: %v\n", file, err)
} else {
fmt.Printf("Successfully backed up %s\n", file)
}
}
9. Handling Command-Line Arguments
We can allow users to specify the source and destination directories via command-line arguments.
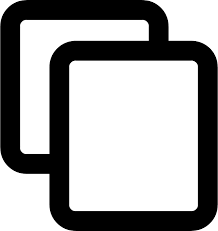
import (
"flag"
"log"
)
func main() {
sourceDir := flag.String("source", "", "Source directory to back up")
destDir := flag.String("dest", "", "Destination directory for backups")
flag.Parse()
if *sourceDir == "" || *destDir == "" {
log.Fatal("Source and destination directories are required")
}
err := backupFiles(*sourceDir, *destDir)
if err != nil {
log.Fatalf("Backup failed: %v", err)
}
fmt.Println("Backup completed successfully.")
}
10. Running the Backup Utility
Now that we have implemented the backup functionality, you can run the utility from the command line like this:
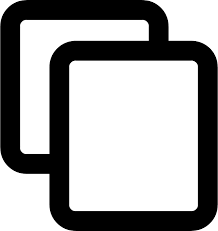
go run main.go -source=/path/to/source -dest=/path/to/destination
11. Optional Features
You can extend this backup utility with additional features, such as:
- Backup Scheduling: Use Go’s
time
package or a cron job to schedule backups at regular intervals. - File Compression: Compress files before backing them up to save storage space.
- Remote Backups: Use FTP, SFTP, or cloud storage APIs to back up files to remote servers or cloud storage providers like AWS S3 or Google Cloud Storage.
12. Conclusion
In this tutorial, we built a simple file backup utility in Go that allows users to back up files from one directory to another. We learned how to work with file I/O, traverse directories, copy files, and handle basic error logging. You can enhance this utility further by adding features such as file compression, remote backups, or backup scheduling for more advanced use cases.
Creating a Simple Chat Application in Go
1. Introduction
In this tutorial, we will create a simple chat application in Go that allows multiple users to communicate in real-time. We'll use Go's concurrency features, such as goroutines and channels, to handle multiple users and facilitate communication between them. The chat application will have both a server and client component, where the server will manage connections and clients will send and receive messages.
2. Key Features of the Chat Application
- Real-Time Communication: Users can send and receive messages instantly.
- Multiple Users: The server can handle multiple clients simultaneously.
- Broadcasting Messages: The server broadcasts messages sent by any user to all other connected users.
- Client-Server Architecture: The application will use a basic client-server architecture where clients connect to the server to chat.
3. Setting Up the Project
Create a new Go project for your chat application. Start by setting up the Go module and creating the necessary files:
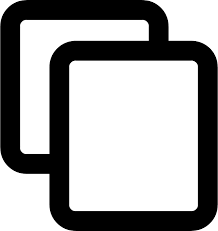
mkdir go-chat
cd go-chat
go mod init go-chat
touch main.go server.go client.go
4. Writing the Server Code
The server will accept incoming connections from clients, manage active clients, and broadcast messages to all connected clients.
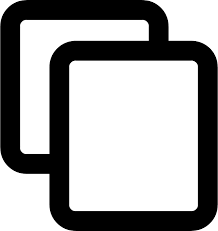
import (
"fmt"
"log"
"net"
"sync"
)
type Client struct {
conn net.Conn
name string
}
var clients = make(map[net.Conn]Client)
var mu sync.Mutex
func handleClient(conn net.Conn) {
defer conn.Close()
client := Client{conn: conn, name: conn.RemoteAddr().String()}
mu.Lock()
clients[conn] = client
mu.Unlock()
fmt.Printf("%s connected\n", client.name)
// Read and broadcast messages
for {
buffer := make([]byte, 1024)
length, err := conn.Read(buffer)
if err != nil {
fmt.Printf("%s disconnected\n", client.name)
mu.Lock()
delete(clients, conn)
mu.Unlock()
return
}
message := string(buffer[:length])
fmt.Printf("Message from %s: %s", client.name, message)
// Broadcast message to all clients
for _, c := range clients {
if c.conn != conn {
c.conn.Write([]byte(fmt.Sprintf("%s: %s", client.name, message)))
}
}
}
}
func startServer() {
server, err := net.Listen("tcp", ":8080")
if err != nil {
log.Fatalf("Error starting server: %v", err)
}
defer server.Close()
fmt.Println("Server started on port 8080")
for {
conn, err := server.Accept()
if err != nil {
fmt.Println("Error accepting connection:", err)
continue
}
go handleClient(conn)
}
}
func main() {
startServer()
}
5. Writing the Client Code
The client will connect to the server, send messages, and display messages received from other clients. We'll use a goroutine to read messages and display them concurrently.
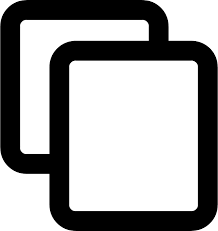
import (
"fmt"
"log"
"net"
"os"
"bufio"
)
func readMessages(conn net.Conn) {
for {
message, err := bufio.NewReader(conn).ReadString('\n')
if err != nil {
fmt.Println("Disconnected from server")
os.Exit(0)
}
fmt.Print(message)
}
}
func main() {
fmt.Print("Enter your name: ")
var name string
fmt.Scanln(&name)
conn, err := net.Dial("tcp", "localhost:8080")
if err != nil {
log.Fatal("Error connecting to server:", err)
}
defer conn.Close()
// Start reading messages from the server
go readMessages(conn)
fmt.Println("Connected to server. Type your messages below:")
// Send user input to the server
for {
reader := bufio.NewReader(os.Stdin)
message, _ := reader.ReadString('\n')
fmt.Fprintf(conn, message)
}
}
6. Running the Chat Application
Now that we have written the server and client code, it's time to run the chat application.
- Start the server by running the server code:
- Start the client by running the client code:
- Multiple clients can connect to the server and start chatting with each other. Open multiple terminal windows and run the client code in each to simulate multiple users.
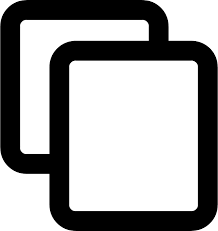
go run server.go
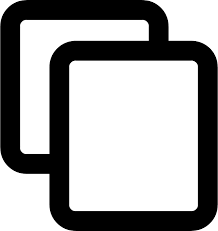
go run client.go
7. Enhancing the Chat Application
Here are some optional features to enhance the chat application:
- User Authentication: Add a simple login system where users need to authenticate before chatting.
- Private Messaging: Implement private messaging between users where a user can send a message to a specific user rather than broadcasting it to everyone.
- Chat Rooms: Create chat rooms where users can join different rooms and communicate with other users in the same room.
- Message History: Store and retrieve message history from a file or a database so users can view previous conversations.
- File Sharing: Allow users to send files to each other over the chat.
8. Conclusion
In this tutorial, we built a simple chat application in Go that supports real-time communication between multiple users. We used Go's concurrency features, such as goroutines and channels, to manage multiple clients and broadcast messages. You can enhance this application further by adding features such as private messaging, chat rooms, user authentication, and message history. This project demonstrates how to build networked applications in Go and is a great starting point for more complex systems.
Developing a Web Scraper in Go
1. Introduction
In this tutorial, we will develop a simple web scraper in Go. A web scraper is a tool that extracts data from websites. Scraping can be useful for collecting information like product prices, news articles, or any data available on the web. We'll use Go’s `net/http` package to send HTTP requests and the `github.com/PuerkitoBio/goquery` package to parse and extract data from HTML.
2. Installing Dependencies
Before starting, you will need to install the `goquery` package, which provides a jQuery-like interface for manipulating and extracting data from HTML documents.
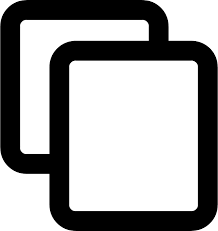
go get github.com/PuerkitoBio/goquery
3. Setting Up the Project
Create a new Go project for the web scraper and initialize the Go module:
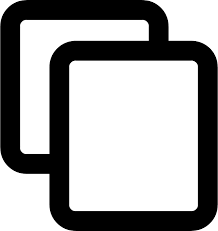
mkdir go-web-scraper
cd go-web-scraper
go mod init go-web-scraper
4. Writing the Web Scraper Code
Now, we will write a simple scraper that fetches the HTML content of a webpage and extracts specific data. Let's scrape headlines from a news website as an example.
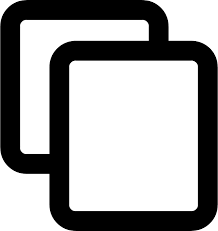
package main
import (
"fmt"
"log"
"net/http"
"github.com/PuerkitoBio/goquery"
)
func main() {
// URL of the page to scrape
url := "https://example.com/news"
// Send HTTP request to fetch the page content
res, err := http.Get(url)
if err != nil {
log.Fatal(err)
}
defer res.Body.Close()
// Check if the request was successful
if res.StatusCode != 200 {
log.Fatalf("Failed to fetch the page. Status code: %d\n", res.StatusCode)
}
// Use goquery to parse the HTML content
doc, err := goquery.NewDocumentFromReader(res.Body)
if err != nil {
log.Fatal(err)
}
// Extract and print the headlines
doc.Find(".headline").Each(func(index int, item *goquery.Selection) {
headline := item.Text()
fmt.Printf("Headline %d: %s\n", index+1, headline)
})
}
5. How the Code Works
- Sending the HTTP Request: We use the `http.Get` function to send a GET request to the URL of the page we want to scrape. If the request is successful, the response body is passed to `goquery` to parse the HTML.
- Parsing HTML with goquery: The `goquery.NewDocumentFromReader` function is used to parse the response body and create a `goquery.Document` object that allows us to navigate and manipulate the HTML content.
- Extracting Data: The `Find` method is used to select elements from the HTML document. In this case, we are looking for elements with the class `.headline`. The `Each` method iterates over each matched element, and we extract the text using the `Text` method.
6. Running the Web Scraper
Once the code is written, you can run the web scraper with the following command:
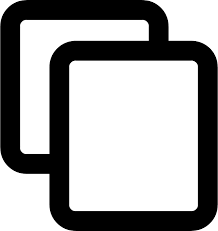
go run main.go
If the website has headlines with the class `.headline`, the scraper will print the headlines to the console. You can modify the CSS selector to extract other types of data from the page, such as links, images, or product information.
7. Handling Errors and Edge Cases
When building a web scraper, it's important to handle errors and edge cases, such as:
- HTTP Errors: Ensure the HTTP request is successful and handle various status codes (e.g., 404 Not Found, 500 Internal Server Error).
- Invalid HTML: The structure of HTML might change, so ensure your scraper can handle changes in the page layout or missing elements.
- Rate Limiting: Some websites may block or rate-limit scrapers. Add delays between requests to avoid overloading the server and respect the site's `robots.txt` file, which defines scraping rules.
8. Storing Scraped Data
Once you have scraped the data, you may want to store it in a file or a database. Here’s an example of how to write the scraped headlines to a CSV file:
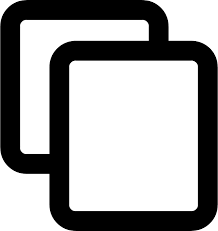
import (
"encoding/csv"
"os"
)
func saveToCSV(headlines []string) {
file, err := os.Create("headlines.csv")
if err != nil {
log.Fatal(err)
}
defer file.Close()
writer := csv.NewWriter(file)
defer writer.Flush()
for _, headline := range headlines {
writer.Write([]string{headline})
}
}
func main() {
// Scrape data as before
// Assume headlines are stored in a slice of strings
headlines := []string{"Headline 1", "Headline 2", "Headline 3"}
// Save scraped headlines to CSV
saveToCSV(headlines)
}
9. Conclusion
In this tutorial, we've learned how to build a simple web scraper in Go. We used the `net/http` package to send HTTP requests and the `goquery` package to parse HTML and extract data from websites. We also discussed handling errors, dealing with edge cases, and storing the scraped data in a CSV file. Web scraping can be a powerful tool for gathering data from websites, and Go’s performance and concurrency features make it an excellent choice for building fast and efficient scrapers.
Building a URL Shortener in Go
1. Introduction
A URL shortener is a service that converts long URLs into shorter, more user-friendly URLs, while still redirecting users to the original address. In this tutorial, we will build a simple URL shortener in Go. We'll use Go's net/http package to handle requests and a simple key-value store (in-memory or database) to map shortened URLs to their original counterparts.
2. Project Setup
Let's start by creating a new Go project for the URL shortener:
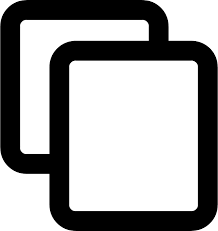
mkdir go-url-shortener
cd go-url-shortener
go mod init go-url-shortener
3. Defining the URL Mapping
For simplicity, we will store the URL mappings in-memory using a Go map. In a production environment, you would typically use a database for persistence, but this is a good start for building the basics.
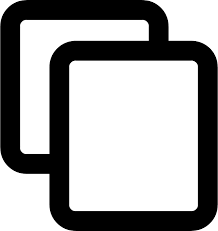
package main
import (
"fmt"
"log"
"net/http"
"math/rand"
"strings"
)
var urlMapping = make(map[string]string) // In-memory URL mapping
// Function to generate a random short URL key
func generateShortURL() string {
const charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
shortURL := make([]byte, 6) // Shortened URL will be 6 characters long
for i := range shortURL {
shortURL[i] = charset[rand.Intn(len(charset))]
}
return string(shortURL)
}
4. Creating the Shortening Handler
We'll create a handler function that takes the long URL, generates a unique short URL, and stores the mapping in the in-memory map. The short URL will be returned as the response.
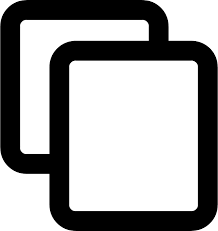
func shortenURLHandler(w http.ResponseWriter, r *http.Request) {
if r.Method != http.MethodPost {
http.Error(w, "Invalid request method", http.StatusMethodNotAllowed)
return
}
// Parse the long URL from the form data
r.ParseForm()
longURL := r.FormValue("longURL")
if longURL == "" {
http.Error(w, "Missing URL", http.StatusBadRequest)
return
}
// Generate a short URL and store the mapping
shortURL := generateShortURL()
urlMapping[shortURL] = longURL
// Return the short URL
fmt.Fprintf(w, "Shortened URL: http://localhost:8080/%s\n", shortURL)
}
5. Creating the Redirection Handler
Next, we need to create a handler that takes a short URL and redirects the user to the corresponding long URL.
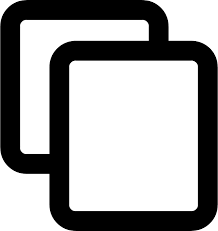
func redirectHandler(w http.ResponseWriter, r *http.Request) {
shortURL := strings.TrimPrefix(r.URL.Path, "/")
// Look up the long URL in the mapping
longURL, exists := urlMapping[shortURL]
if !exists {
http.Error(w, "URL not found", http.StatusNotFound)
return
}
// Redirect to the original URL
http.Redirect(w, r, longURL, http.StatusFound)
}
6. Setting Up the HTTP Server
Now, we can set up the HTTP server to handle both shortening and redirection requests. We will use the http.HandleFunc method to map the request paths to the appropriate handler functions.
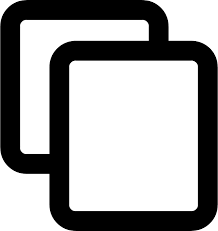
func main() {
http.HandleFunc("/shorten", shortenURLHandler) // Endpoint to shorten URLs
http.HandleFunc("/", redirectHandler) // Endpoint to redirect short URLs
fmt.Println("Starting server on http://localhost:8080")
log.Fatal(http.ListenAndServe(":8080", nil))
}
7. Running the URL Shortener
Once the code is complete, you can run the URL shortener with the following command:
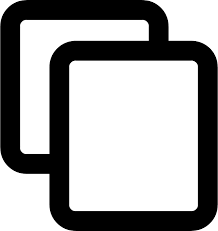
go run main.go
8. Testing the URL Shortener
To test the URL shortener, you can follow these steps:
- Send a POST request to
http://localhost:8080/shorten
with a long URL as form data. Example: - The response will contain a shortened URL, such as
http://localhost:8080/abc123
. - To test the redirection, visit the shortened URL in your browser, and you will be redirected to the original long URL.
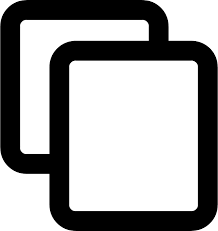
curl -X POST -d "longURL=https://www.example.com" http://localhost:8080/shorten
9. Handling Edge Cases
In a real-world application, you would need to handle various edge cases, such as:
- Duplicate short URLs: The `generateShortURL` function could produce the same short URL for different long URLs. To prevent this, you can check for duplicates before storing a new mapping.
- Invalid URLs: Ensure that the long URL provided is a valid URL by using a URL validation function.
- URL expiration: You could implement a mechanism to expire URLs after a certain period of time or a set number of uses.
10. Conclusion
In this tutorial, we've built a simple URL shortener in Go. We used Go's `net/http` package to create a basic HTTP server, handled URL shortening and redirection, and stored the URL mappings in memory. While this example is simplified for learning purposes, you can extend this application by adding features like URL expiration, custom short URLs, and persistence using a database like MySQL or Redis. Go’s simplicity and performance make it an excellent choice for building high-performance web applications like this URL shortener.