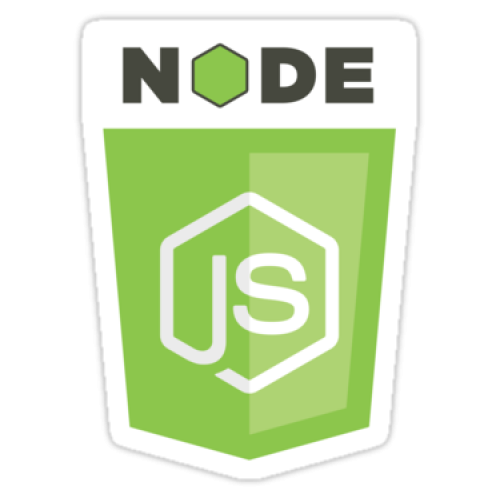
What is Node.js?
Node.js is an open-source, cross-platform, JavaScript runtime environment that allows developers to build scalable and high-performance applications. Built on Chrome's V8 JavaScript engine, Node.js enables JavaScript to be executed on the server side, outside the browser, making it a powerful tool for backend development.
History of Node.js
Node.js was created in 2009 by Ryan Dahl with the goal of enabling event-driven, non-blocking I/O operations. It revolutionized server-side programming by allowing developers to use JavaScript for both client-side and server-side development, creating a unified development environment. Today, Node.js is widely used for web applications, APIs, microservices, and real-time applications.
Node.js Features
Below are the key features that make Node.js a popular choice among developers:
Feature | Description |
---|---|
Non-blocking I/O | Node.js uses an asynchronous, event-driven architecture, which allows it to handle multiple tasks efficiently without blocking the execution thread. |
Single-threaded Model | Node.js operates on a single-threaded event loop, which makes it lightweight and efficient for handling concurrent operations. |
Cross-platform | Node.js is platform-independent and can run on Windows, macOS, and Linux, making it accessible to a wide range of developers. |
Rich Ecosystem | The Node.js ecosystem is powered by npm (Node Package Manager), which provides access to thousands of reusable libraries and modules for rapid development. |
Setting Up Node.js
Before developing with Node.js, you need to set it up on your system. Follow the steps below to install Node.js:
- Download the Node.js installer from the official website.
- Run the installer and follow the setup instructions. Ensure you add Node.js to your PATH during installation.
- Verify the installation by opening your terminal or command line and running
node --version
.
Code Example: Hello World
Let’s write a simple Node.js program that outputs the text "Hello, World!" to the console:
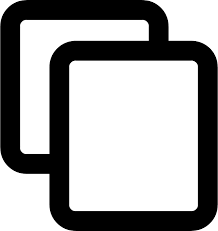
// Node.js program to print Hello World
console.log("Hello, World!");
Diagram: Node.js Runtime Flow
The following diagram explains the execution flow of a Node.js program:

In this diagram, you can see how Node.js processes requests using its event-driven architecture and single-threaded event loop.
Features and Advantages of Node.js
Node.js stands out as a powerful runtime environment for building fast, scalable, and efficient applications. Below are the key features and advantages that make Node.js an excellent choice for developers and organizations alike:
Key Features of Node.js
Feature | Description |
---|---|
Asynchronous and Event-driven | Node.js uses a non-blocking, event-driven architecture that enables handling multiple requests simultaneously without blocking the execution thread. |
Fast Execution | Built on Chrome's V8 JavaScript engine, Node.js compiles JavaScript into machine code, ensuring fast execution and performance. |
Single-threaded Model | Node.js operates on a single-threaded event loop, handling multiple concurrent requests efficiently, which makes it lightweight and scalable. |
Cross-platform | Node.js can run on multiple platforms, including Windows, macOS, and Linux, enabling developers to create applications that work seamlessly across different systems. |
Rich Ecosystem | Node.js has a vast ecosystem powered by npm (Node Package Manager), offering access to thousands of reusable libraries and modules for rapid application development. |
Advantages of Node.js
Node.js offers several advantages that make it a preferred choice for modern application development:
- Scalability: The event-driven architecture and non-blocking I/O make Node.js ideal for building scalable, real-time applications such as chat apps and online gaming platforms.
- High Performance: With its efficient single-threaded model and V8 engine, Node.js delivers excellent performance, even under heavy workloads.
- Unified Development: Node.js allows developers to use JavaScript on both the client and server sides, reducing the need to switch between languages and improving development speed.
- Community Support: The Node.js community is large and active, providing abundant resources, tutorials, and modules for developers of all skill levels.
- Real-time Applications: Node.js excels at building real-time applications like collaborative tools, live chat, and streaming services due to its WebSocket and event-driven capabilities.
- Cost-effective: Its lightweight nature and ability to handle high traffic with fewer resources make Node.js a cost-effective solution for startups and large enterprises alike.
Code Example: Event-driven Nature
The following example demonstrates how Node.js handles asynchronous, event-driven programming:
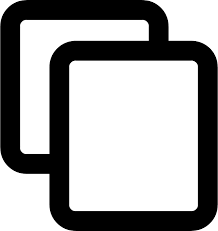
// Example: Event-driven nature in Node.js
const fs = require('fs');
// Asynchronous file reading
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
console.log('This message is logged first, before the file content is read!');
Use Cases of Node.js
Node.js is particularly well-suited for the following types of applications:
- Real-time chat applications
- Streaming services
- RESTful APIs and microservices
- Single-page applications (SPAs)
- IoT (Internet of Things) solutions
- Collaborative tools like online document editors
Setting Up Node.js on Your System
Before you can start developing applications with Node.js, you need to install and configure it on your system. Follow the steps below to set up Node.js and ensure everything is working correctly:
Download and Install Node.js
Node.js can be installed on various operating systems like Windows, macOS, and Linux. Here’s how to get started:
- Visit the official Node.js website.
- Download the Long-Term Support (LTS) version for stability or the Current version for the latest features.
- Run the installer and follow the on-screen instructions to complete the installation.
- Ensure the option to install npm (Node Package Manager) is selected during the installation process.
Verify Installation
Once Node.js is installed, you can verify it by checking the installed versions of Node.js and npm:
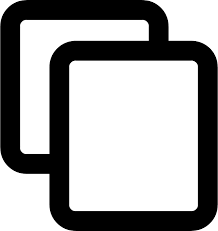
// Check Node.js version
node --version
// Check npm version
npm --version
If you see the version numbers displayed, Node.js and npm are successfully installed.
Setup a Basic Node.js Project
To start using Node.js, create a basic project by following these steps:
- Create a new directory for your project:
mkdir my-node-app cd my-node-app
- Initialize a new Node.js project by running:
npm init -y
This command generates a
package.json
file, which stores metadata about your project and its dependencies. - Create a simple JavaScript file, such as
app.js
, and add the following code:// app.js console.log('Hello, Node.js!');
- Run your Node.js script:
node app.js
Install and Use a Package
Node.js comes with npm, allowing you to install third-party packages. For example, to install the popular lodash
library:
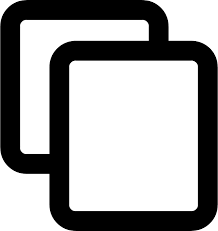
npm install lodash
To use the installed package in your project:
// app.js
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const reversedNumbers = _.reverse(numbers.slice());
console.log('Reversed Numbers:', reversedNumbers);
Keeping Node.js and npm Updated
It’s essential to keep Node.js and npm up-to-date to access the latest features and security updates:
- Update npm:
npm install -g npm@latest
- Update Node.js:
Download the latest version from the official website or use a version manager like
nvm
(Node Version Manager).
Node.js vs Traditional Web Servers
Node.js introduces a modern approach to handling web applications compared to traditional web servers like Apache or IIS. Below is a detailed comparison highlighting the differences and advantages of Node.js over traditional web servers:
Comparison Table
Aspect | Node.js | Traditional Web Servers |
---|---|---|
Architecture | Non-blocking, event-driven architecture allows handling multiple requests concurrently without creating separate threads for each connection. | Blocking, thread-based architecture where each request spawns a new thread, leading to higher resource usage under heavy traffic. |
Performance | High performance due to its asynchronous nature and use of the V8 JavaScript engine. | Performance can degrade with high concurrency as each thread consumes significant memory and CPU resources. |
Language | Uses JavaScript, making it easier for developers familiar with frontend development to work on the backend. | Uses various languages like PHP, Python, or Java, which require separate expertise from frontend languages. |
Scalability | Highly scalable due to its ability to handle concurrent requests with a single thread using asynchronous I/O. | Scalability can be challenging as adding more threads to handle requests increases resource consumption. |
Real-time Applications | Ideal for real-time applications like chat apps and collaborative tools due to WebSockets and event-driven architecture. | Not optimized for real-time applications as handling persistent connections can strain thread-based systems. |
Installation and Setup | Lightweight and straightforward setup. The server is part of the application, which gives more control. | Requires additional configuration and setup, often with external modules or plugins for specific functionalities. |
Community and Ecosystem | Vast ecosystem with npm offering numerous libraries and tools for rapid development. | Rich ecosystem for each language, but integration between tools can sometimes be complex. |
Advantages of Node.js Over Traditional Web Servers
- Asynchronous Processing: Node.js can handle thousands of concurrent connections without the overhead of thread management.
- Unified Development Language: Developers can use JavaScript for both frontend and backend, reducing the learning curve and improving collaboration.
- Real-time Capability: Built-in support for WebSockets makes Node.js perfect for real-time applications like gaming or messaging platforms.
- Lightweight: Node.js is lightweight and efficient, making it ideal for microservices and small-footprint applications.
- Scalability: Built with scalability in mind, Node.js supports both horizontal and vertical scaling.
Code Example: Handling Requests in Node.js
Below is an example of a simple HTTP server in Node.js:
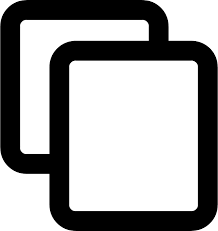
// Import the http module
const http = require('http');
// Create an HTTP server
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, this is a Node.js server!');
});
// Start the server on port 3000
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Conclusion
While traditional web servers have been reliable for years, Node.js offers a modern, scalable, and efficient alternative that is especially suited for real-time, high-performance web applications. Its event-driven architecture and JavaScript-based ecosystem make it a popular choice among developers.
Node.js Architecture (Event Loop and Non-blocking I/O)
Node.js operates on a unique architecture that makes it highly efficient for handling concurrent tasks. It uses an event-driven, non-blocking I/O model that allows it to handle multiple operations simultaneously without creating multiple threads.
Key Components of Node.js Architecture
- Single-threaded Event Loop: Node.js is single-threaded, meaning it processes all tasks on a single thread. However, it can handle multiple requests simultaneously using the event loop mechanism.
- Non-blocking I/O: I/O operations (e.g., reading files, making API calls) in Node.js are non-blocking, allowing the server to continue executing code without waiting for the operation to complete.
- V8 Engine: Node.js uses Google’s V8 engine to execute JavaScript code, ensuring fast and efficient performance.
- Libuv Library: Libuv handles the event loop and provides support for asynchronous operations like file systems, DNS, and networking.
How the Event Loop Works
The event loop is the core of Node.js. It allows Node.js to perform non-blocking I/O operations by offloading tasks to the system's kernel or background threads. The loop continuously checks for new events and processes them. Here’s how it works:
- Node.js receives incoming requests or events.
- Each request is added to an event queue.
- The event loop picks up tasks from the queue and executes callbacks associated with them.
- Long-running operations are delegated to worker threads or the system kernel, allowing the event loop to process other tasks.
Diagram: Node.js Event Loop
The diagram below illustrates the Node.js event loop:

This diagram shows how the event loop handles incoming events, delegates tasks, and processes callbacks.
Advantages of Event Loop and Non-blocking I/O
- High Concurrency: Handles thousands of simultaneous requests efficiently.
- Scalability: Ideal for scalable network applications like chat servers and APIs.
- Efficient Resource Utilization: Uses a single thread, reducing memory and CPU usage compared to thread-based architectures.
- Real-time Applications: Perfect for real-time use cases like live streaming and gaming.
Code Example: Non-blocking I/O in Node.js
Here’s a simple example demonstrating non-blocking file reading in Node.js:
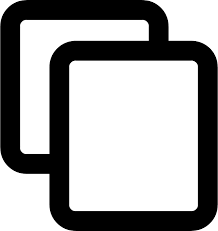
// Import the fs module
const fs = require('fs');
// Asynchronous (non-blocking) file read
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
console.log('This message is logged while the file is being read.');
Conclusion
Node.js's event-driven, non-blocking architecture makes it a powerful tool for building efficient, real-time, and scalable applications. Understanding how the event loop works is crucial for leveraging Node.js to its fullest potential.
How Node.js Works with Asynchronous Programming
Asynchronous programming is a key feature of Node.js that allows the server to handle multiple tasks concurrently without blocking the execution of other tasks. Instead of waiting for a task to complete before starting another one, Node.js continues executing the program while waiting for the task to finish. This approach is ideal for I/O-bound operations such as reading from or writing to files, making database queries, and handling HTTP requests.
The Event Loop
The event loop is at the heart of asynchronous programming in Node.js. It is responsible for executing asynchronous code, handling events, and managing the execution of callbacks. When an asynchronous task is initiated, Node.js does not wait for it to complete. Instead, it moves on to the next task in the queue, and once the initial task finishes, the corresponding callback function is triggered to handle the result.
Callback Functions
In asynchronous programming, callbacks are functions that are passed as arguments to other functions. These callbacks are executed once the asynchronous task completes. For example, in Node.js, when reading a file, you don't have to wait for the file to finish reading. Instead, you pass a callback function, which is called once the file is read.
Example: Asynchronous File Reading
Let’s look at an example where we read a file asynchronously using Node.js:
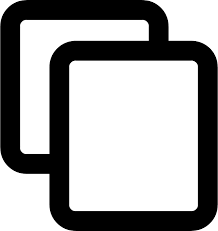
const fs = require('fs');
// Asynchronous file reading
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
console.log("File reading initiated...");
In this example:
fs.readFile
is an asynchronous function that reads a file without blocking the program.- The callback function is executed once the file is successfully read (or if an error occurs).
- Notice that the message "File reading initiated..." is logged first, even though the file has not been read yet. This shows how the event loop handles other tasks while waiting for asynchronous operations to complete.
Non-Blocking I/O
Node.js uses non-blocking I/O to handle multiple operations at once. This means that when an I/O operation is initiated, such as reading a file or making an HTTP request, Node.js doesn’t wait for the operation to finish before moving on to the next task. This improves performance, especially when handling large numbers of I/O operations, as the application can process multiple requests simultaneously without being delayed by slow operations.
Example: Asynchronous HTTP Request
Let’s consider an example where we make an asynchronous HTTP request using the http
module:
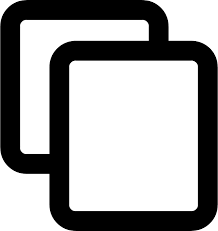
const http = require('http');
// Asynchronous HTTP request
http.get('http://example.com', (res) => {
let data = '';
// A chunk of data has been received.
res.on('data', (chunk) => {
data += chunk;
});
// The whole response has been received.
res.on('end', () => {
console.log("Response received:", data);
});
});
console.log("HTTP request sent...");
In this example:
- The HTTP request is made asynchronously, and the program continues executing while waiting for the response.
- The
data
event is triggered whenever a chunk of data is received, and theend
event is triggered once the entire response is received. - Just like before, the message "HTTP request sent..." is logged first, showing the non-blocking nature of the operation.
Promises and Async/Await
In addition to callbacks, Node.js also supports Promises and the async/await syntax for handling asynchronous operations. Promises represent the eventual result of an asynchronous operation, and async/await provides a more readable way to work with Promises by avoiding callback hell.
Code Example: Promises
Here’s an example using Promises to handle asynchronous operations:
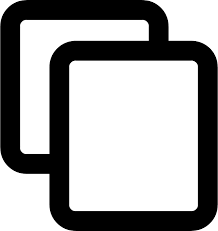
const fs = require('fs').promises;
// Asynchronous file reading with Promises
fs.readFile('example.txt', 'utf8')
.then(data => {
console.log("File content:", data);
})
.catch(err => {
console.error("Error reading file:", err);
});
console.log("File reading initiated...");
Diagram: Node.js Event Loop with Asynchronous Tasks
The following diagram shows how Node.js handles asynchronous tasks using the event loop:

In this diagram, you can see how the event loop processes asynchronous tasks, handling multiple operations concurrently without blocking the execution of the program.
Working with Modules (CommonJS vs ES Modules)
In Node.js, modules allow you to break down your code into reusable parts, making it easier to maintain and organize. Node.js supports two primary module systems: CommonJS (the default) and ES Modules (introduced in ES6). Both systems allow you to import and export code between files, but they have different syntax and behaviors.
CommonJS Modules
CommonJS is the original module system used by Node.js. It uses the require()
function to import modules and the module.exports
object to export functionality. This system is synchronous and works well in the context of server-side applications where modules are loaded during runtime.
Example: CommonJS Syntax
Here’s an example of how to use CommonJS modules:
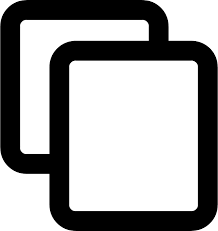
// math.js (module file)
function add(a, b) {
return a + b;
}
module.exports = { add };
// app.js (main file)
const math = require('./math');
console.log(math.add(2, 3)); // Output: 5
In this example:
- In
math.js
, theadd
function is exported usingmodule.exports
. - In
app.js
, the module is imported usingrequire('./math')
and theadd
function is accessed.
ES Modules (ECMAScript Modules)
ES Modules (ESM) is the modern standard for working with modules in JavaScript. It is part of ES6 and provides a more flexible and declarative way to import and export code using the import
and export
keywords. ES Modules are asynchronous and work well in both the browser and Node.js environments.
Example: ES Module Syntax
Here’s an example of how to use ES Modules:
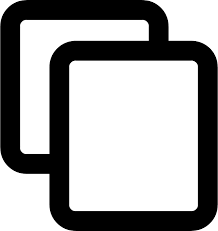
// math.mjs (module file)
export function add(a, b) {
return a + b;
}
// app.mjs (main file)
import { add } from './math.mjs';
console.log(add(2, 3)); // Output: 5
In this example:
- In
math.mjs
, theadd
function is exported using theexport
keyword. - In
app.mjs
, the module is imported using theimport
statement and theadd
function is accessed.
Differences Between CommonJS and ES Modules
Feature | CommonJS | ES Modules |
---|---|---|
Syntax | require() and module.exports |
import and export |
Loading | Synchronous | Asynchronous |
File Extension | No specific file extension (commonly .js ) |
Requires .mjs or setting "type": "module" in package.json |
Default Export | module.exports |
export default |
Compatibility | Primarily used in Node.js | Used in both Node.js and the browser |
Working with ES Modules in Node.js
To use ES Modules in Node.js, you need to either:
- Use the
.mjs
file extension for module files, or - Set the
"type": "module"
field in yourpackage.json
file.
Example: Using ES Modules with package.json
If you want to use ES Modules with the .js
extension in Node.js, you'll need to add the following to your package.json
:
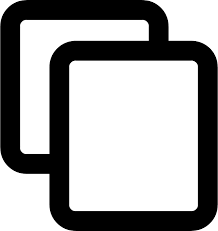
{
"type": "module"
}
Once this is done, you can use the import
and export
syntax in your JavaScript files with the .js
extension.
When to Use Each Module System
- Use CommonJS if you are working with legacy code or need to maintain compatibility with existing Node.js applications.
- Use ES Modules for modern JavaScript development, especially if you're working on projects that target both the browser and Node.js, or if you're using newer JavaScript features.
Conclusion
Both CommonJS and ES Modules have their uses in Node.js, and the choice between them depends on your project requirements and compatibility needs. While CommonJS is still the default in Node.js, ES Modules are the future of JavaScript, offering a more modern and flexible approach to handling modules.
Global Objects in Node.js (__dirname, __filename)
In Node.js, global objects are built-in variables and functions that are available throughout the entire Node.js application. Two commonly used global objects are __dirname
and __filename
. These objects provide essential information about the current working directory and the filename of the currently executing script, which can be helpful when working with paths and file operations.
__dirname
__dirname
is a global variable in Node.js that contains the absolute path to the directory of the currently executing script. It is especially useful when working with file paths, as it helps resolve the full path of files relative to the script’s location.
Example: Using __dirname
Here’s an example of how to use __dirname
to get the current directory of a script:
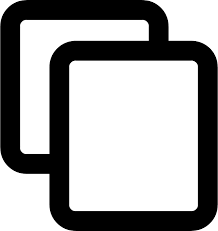
// script.js
console.log(__dirname);
When you run this code, it will output the absolute path to the directory where script.js
is located. For example:
/Users/username/projects/myapp
__filename
__filename
is a global variable that contains the absolute path to the currently executing script file, including the file name. This can be useful when you need to know the exact file being executed in the application.
Example: Using __filename
Here’s an example of how to use __filename
to get the full path of the current script file:
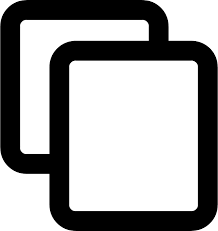
// script.js
console.log(__filename);
When you run this code, it will output the absolute path to the file, including the file name. For example:
/Users/username/projects/myapp/script.js
Using __dirname
and __filename
with File Operations
Both __dirname
and __filename
are commonly used when working with the fs
(File System) module. They help build absolute paths for reading, writing, or working with files in the application.
Example: Reading a File with __dirname
Here’s an example of how you can use __dirname
to read a file located in the same directory as the script:
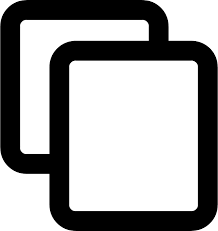
const fs = require('fs');
const path = require('path');
// Reading a file using __dirname
const filePath = path.join(__dirname, 'example.txt');
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
In this example:
path.join(__dirname, 'example.txt')
is used to build the absolute path to theexample.txt
file, ensuring the file is found relative to the current script.fs.readFile
then reads the file asynchronously using the absolute path.
Difference Between __dirname
and __filename
Global Object | What It Represents | Example Output |
---|---|---|
__dirname |
Path to the directory containing the currently executing script. | /Users/username/projects/myapp |
__filename |
Full path to the currently executing script file, including the file name. | /Users/username/projects/myapp/script.js |
When to Use __dirname
and __filename
- Use
__dirname
when you need the path of the directory containing the current file, such as when constructing file paths or working with directories. - Use
__filename
when you need the full path of the current file, including the file name, for tasks such as logging or debugging.
Conclusion
__dirname
and __filename
are useful global objects in Node.js that provide important information about the current script’s location. By using these objects, you can build absolute paths, work with files, and gain a deeper understanding of your application’s structure.
Built-in Modules (fs, http, os, path, etc.)
Node.js comes with a wide range of built-in modules that provide essential functionality, such as handling file systems, creating HTTP servers, working with operating system-related tasks, and manipulating file paths. These modules are part of the Node.js runtime and can be used without needing to install any additional packages. Some of the most commonly used built-in modules are fs
, http
, os
, and path
.
1. fs
(File System Module)
The fs
module provides an API for interacting with the file system. It allows you to read, write, update, delete, and manipulate files and directories on your system.
Example: Reading a File with fs
Here’s an example of how to use the fs
module to read a file asynchronously:
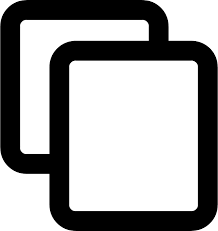
const fs = require('fs');
// Reading a file asynchronously
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
In this example, the fs.readFile
method is used to read the contents of the example.txt
file asynchronously. The callback function handles any errors and logs the file content if successful.
2. http
(HTTP Module)
The http
module provides utilities for creating HTTP servers and making HTTP requests. It is essential for building web applications and APIs in Node.js.
Example: Creating a Simple HTTP Server
Here’s an example of how to create a simple HTTP server with the http
module:
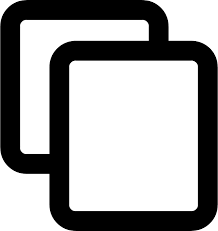
const http = require('http');
// Creating an HTTP server
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!');
});
// Listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code creates an HTTP server that listens on port 3000 and responds with "Hello, World!" for every request. The http.createServer
method is used to create the server, while the server.listen
method makes the server listen on a specified port.
3. os
(Operating System Module)
The os
module provides information about the operating system on which the Node.js process is running. It allows you to gather details like system architecture, hostname, platform, memory usage, and more.
Example: Getting System Information with os
Here’s an example of how to use the os
module to get information about the system:
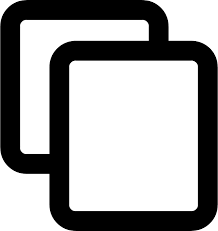
const os = require('os');
// Getting the system's hostname
console.log("Hostname:", os.hostname());
// Getting the system's platform
console.log("Platform:", os.platform());
// Getting the system's total memory
console.log("Total memory:", os.totalmem(), "bytes");
This example uses the os.hostname()
, os.platform()
, and os.totalmem()
methods to get the hostname, platform, and total memory of the operating system, respectively.
4. path
(Path Module)
The path
module provides utilities for working with file and directory paths. It allows you to join, resolve, normalize, and manipulate file paths in a cross-platform manner.
Example: Joining Paths with path
Here’s an example of how to use the path
module to join file paths:
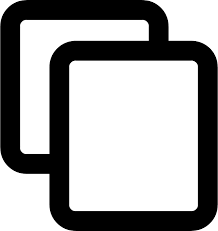
const path = require('path');
// Joining paths
const filePath = path.join(__dirname, 'folder', 'example.txt');
console.log("Full file path:", filePath);
In this example, the path.join
method is used to join the current directory (__dirname
), a folder name, and a file name into a full file path. This ensures that the path is correctly constructed for different operating systems.
5. Other Built-in Modules
Node.js offers many other built-in modules, each serving different purposes. Some of the notable ones include:
crypto
: Provides cryptographic functionality, such as hashing, encryption, and digital signatures.url
: Provides utilities for working with URLs, including parsing and resolving URLs.stream
: Provides an API for working with streaming data.querystring
: Provides utilities for working with query string parameters in URLs.events
: Provides the EventEmitter class for handling events in Node.js applications.
Conclusion
Built-in modules in Node.js provide a wide range of functionality that helps developers build efficient, scalable, and feature-rich applications. From file system manipulation with fs
, to creating HTTP servers with http
, working with the operating system with os
, and managing file paths with path
, these modules simplify common tasks and help you focus on building your application logic.
Understanding require()
and import
Statements
In Node.js, there are two primary ways to include external modules and files into your project: require()
and import
. Both serve the same purpose — allowing you to access the functionality provided by other modules — but they have different syntax and usage patterns. The require()
statement is part of the CommonJS module system, which was the original module system used in Node.js, whereas import
is part of the modern ES Modules (ESM) system, which is now supported in Node.js.
1. The require()
Statement (CommonJS)
The require()
function is used to load CommonJS modules. It's a synchronous operation and is widely used in Node.js for including built-in modules, third-party libraries, and custom modules.
Example: Using require()
to Import a Module
Here’s an example of using require()
to import a built-in module in Node.js:
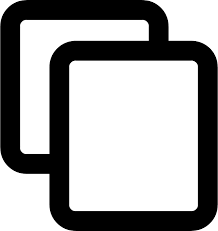
const fs = require('fs');
// Reading a file using fs module
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
In this example, the fs
module is imported using the require()
statement, and we use it to read the contents of a file asynchronously.
2. The import
Statement (ES Modules)
The import
statement is part of the ES Modules (ESM) system and allows you to import modules in a more flexible and standardized way. It is asynchronous and is now natively supported in Node.js, though it requires the use of the .mjs
file extension or the "type": "module"
field in the package.json
file to enable ESM.
Example: Using import
to Import a Module
Here’s an example of how to use the import
statement to import a built-in module in Node.js:
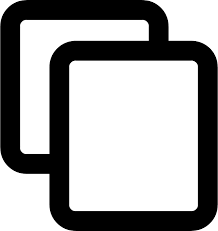
import fs from 'fs';
// Reading a file using fs module (ES Modules version)
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
This example shows how to use the import
statement to import the fs
module. Notice the syntax change, where the module is imported using the import
keyword followed by the module name. The rest of the usage remains the same.
3. Key Differences Between require()
and import
Feature | require() (CommonJS) |
import (ES Modules) |
---|---|---|
Syntax | const module = require('module'); |
import module from 'module'; |
File Extension | Supports .js , .json , .node |
Typically used with .mjs or .js with "type": "module" in package.json |
Asynchronous | Synchronous | Asynchronous |
Module Loading | CommonJS module system (eager loading) | ES Module system (lazy loading) |
Export Syntax | module.exports or exports |
export default or export { ... } |
4. Converting Between require()
and import
If you are working with a project that uses CommonJS modules but want to switch to ES Modules, you can refactor the code to use the import
syntax. However, some older libraries or packages may still rely on require()
, so you may need to combine both approaches in your project.
Example: Exporting a Module
Here’s an example of how to export a module using both CommonJS and ES Module syntax:
Using CommonJS
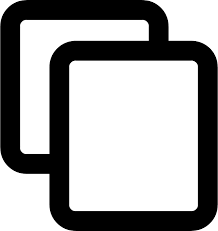
// Exporting using CommonJS
module.exports = function greet(name) {
return `Hello, ${name}!`;
};
Using ES Modules
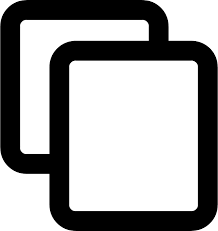
// Exporting using ES Modules
export default function greet(name) {
return `Hello, ${name}!`;
}
Conclusion
Both require()
and import
are essential for including external modules in your Node.js projects. While require()
is the older CommonJS method, import
offers a more modern and flexible approach, especially when working with ES Modules. As Node.js continues to evolve, the import
statement is expected to become the preferred method for importing modules, although require()
remains widely used in existing Node.js applications.
Package Management with npm
and yarn
In the Node.js ecosystem, managing dependencies and packages is crucial for development. Two of the most popular tools for handling package management are npm
(Node Package Manager) and yarn
. Both are used for installing, updating, and managing project dependencies, but they have some differences in terms of performance, features, and workflows. This section will explore both tools and how to use them effectively in your Node.js projects.
1. Introduction to npm
npm
is the default package manager for Node.js and comes installed with Node when you download it. It is widely used for managing dependencies, running scripts, and publishing packages to the npm registry. npm
is based on a command-line interface (CLI) that allows you to manage your project’s libraries and packages.
Installing Packages with npm
To install a package using npm
, use the following command:
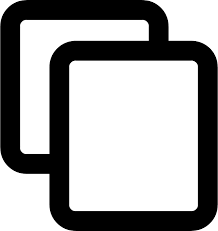
npm install
This command will install a package and add it to your node_modules
folder. To install all dependencies listed in your package.json
file, you can run:
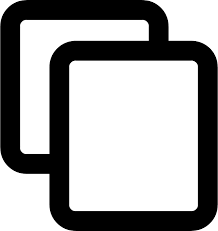
npm install
Saving Dependencies
By default, npm install
will add the package to your node_modules
folder but will not save it to the package.json
file. If you want to save it as a dependency, use the --save
flag (this is automatic in recent versions of npm):
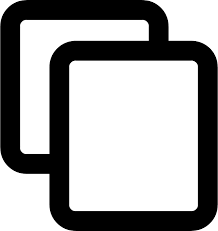
npm install --save
2. Introduction to yarn
yarn
is an alternative package manager developed by Facebook, primarily to address some of the shortcomings of npm
in terms of speed, reliability, and security. yarn
is also a command-line interface tool that helps you manage project dependencies, but it offers additional features like deterministic dependency resolution and offline capabilities.
Installing Packages with yarn
To install a package using yarn
, you can use the following command:
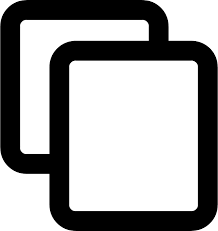
yarn add
This command will install the specified package and add it to your node_modules
folder, as well as to your package.json
file. To install all dependencies listed in your package.json
file, simply run:
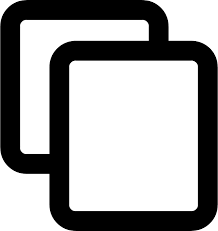
yarn install
Offline Mode
One of the major benefits of yarn
is its ability to cache packages locally. Once a package has been installed, it will be available for offline installations. This can significantly speed up subsequent installations.
3. Key Differences Between npm
and yarn
Feature | npm |
yarn |
---|---|---|
Speed | Generally slower due to a more complex dependency resolution process | Faster due to parallel downloads and offline caching |
Lockfile | Uses package-lock.json to lock dependencies |
Uses yarn.lock for deterministic dependency resolution |
Offline Support | No offline support by default | Supports offline installations after the first install |
Package Installation | Runs installations sequentially | Runs installations in parallel for faster performance |
Security | Basic security features | Includes integrity checks for packages for better security |
4. Managing Dependencies
Installing Specific Versions of Packages
Both npm
and yarn
allow you to install specific versions of a package:
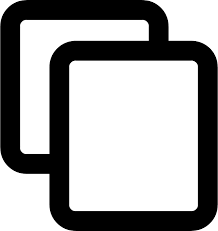
npm install @
yarn add @
For example, to install version 1.2.3 of a package, you would run:
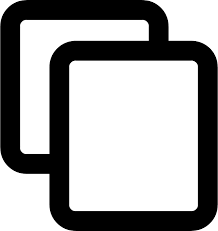
npm install @1.2.3
yarn add @1.2.3
Removing Packages
To remove a package using npm
or yarn
, you can use:
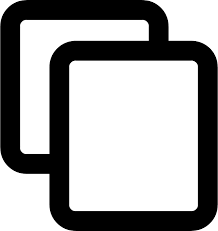
npm uninstall
yarn remove
5. Conclusion
Both npm
and yarn
are excellent tools for managing dependencies in Node.js projects. While npm
is the default and widely used package manager, yarn
offers certain features like speed and offline support that may be beneficial for larger projects. Ultimately, the choice between npm
and yarn
depends on your specific needs and preferences.
Creating Your Own Modules in Node.js
In Node.js, you can create your own modules to encapsulate functionality and share it across different parts of your application. Modules help in organizing code, improving maintainability, and promoting code reusability. Node.js provides a built-in module system, allowing you to easily export and import modules into other files.
1. Introduction to Modules
A module in Node.js is simply a JavaScript file that encapsulates a particular functionality or set of functionalities. Modules can be either core modules (like fs
or http
), third-party modules (installed via npm
), or custom modules created by the developer.
2. Creating a Simple Module
To create your own module, follow these steps:
Step 1: Create a JavaScript File for the Module
In your project folder, create a new JavaScript file that will act as your module. For example, create a file named math.js
:
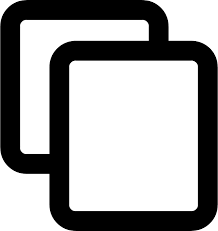
// math.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
// Export the functions so they can be used in other files
module.exports = {
add,
subtract
};
In this example, we define two functions, add
and subtract
, and use module.exports
to export them. The module.exports
object is what makes the functions available to other files that require this module.
Step 2: Import and Use the Module in Another File
Now that we have created our module, we can import and use it in another file. Create a new file, for example, app.js
, and use require()
to import the module:
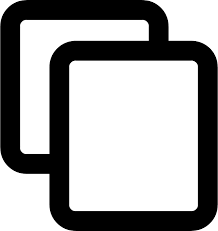
// app.js
const math = require('./math');
console.log(math.add(5, 3)); // Output: 8
console.log(math.subtract(9, 4)); // Output: 5
In this example, we use require('./math')
to import the math.js
module. We can now access the add
and subtract
functions from the math
object and use them as needed.
3. Exporting in Different Ways
Node.js offers various ways to export and import modules. The most common methods are:
Using module.exports
As seen in the previous example, module.exports
is used to export a single object, function, or value:
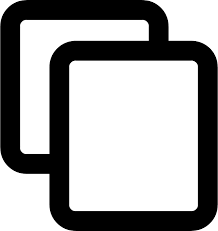
// Exporting a function
module.exports = function(a, b) {
return a + b;
};
Using exports
Object
You can also export multiple items using the exports
object. This is essentially a shorthand for module.exports
:
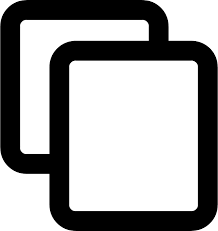
// math.js
exports.add = function(a, b) {
return a + b;
};
exports.subtract = function(a, b) {
return a - b;
};
In this example, we directly attach functions to the exports
object, making them accessible in the same way as with module.exports
.
4. Using ES6 Module Syntax (with import
and export
)
Node.js has added support for ECMAScript modules (ESM) in recent versions. This allows you to use the import
and export
statements, which are commonly used in frontend JavaScript development. To use ESM in Node.js, you need to specify the "type": "module"
in your package.json
file:
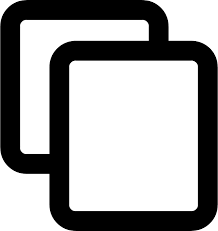
// package.json
{
"type": "module"
}
Now you can use import
and export
in your modules:
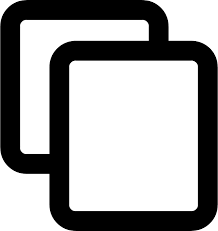
// math.mjs
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
To import the module in another file, use the import
statement:
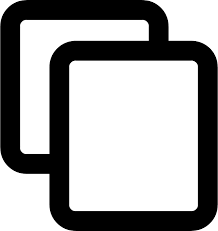
// app.mjs
import { add, subtract } from './math.mjs';
console.log(add(5, 3)); // Output: 8
console.log(subtract(9, 4)); // Output: 5
Note that with ES6 modules, you need to use the .mjs
extension for module files, or you must enable the "type": "module" setting in your package.json
file to use the import
and export
statements in .js
files.
5. Conclusion
Creating your own modules in Node.js is a powerful way to keep your code organized, reusable, and maintainable. By using module.exports
or exports
, you can easily share your code across different files. You can also take advantage of ES6 module syntax to write cleaner and more modern JavaScript code. Whether you choose CommonJS or ES modules, Node.js provides flexible ways to structure your applications with custom modules.
Semantic Versioning and Package Updates
Semantic Versioning (SemVer) is a versioning scheme for software that helps developers understand the compatibility and scope of changes between different versions of a package. By following SemVer, package maintainers can communicate the nature of updates, and users can decide whether to upgrade based on the potential impact of changes. This section will explain SemVer and how it relates to updating packages in your Node.js project.
1. What is Semantic Versioning?
Semantic Versioning is a versioning system that consists of three parts, separated by dots, in the format MAJOR.MINOR.PATCH
. Each part of the version number indicates a different level of changes made to the software:
- MAJOR version: Incremented when there are incompatible API changes, meaning that upgrading may break backward compatibility.
- MINOR version: Incremented when new features are added in a backward-compatible manner. This means the new version introduces new functionality, but existing functionality remains intact.
- PATCH version: Incremented when backward-compatible bug fixes are introduced. These are typically updates that resolve issues without affecting the functionality of existing features.
2. Semantic Versioning Example
Here’s an example to illustrate how versions are incremented:
Version | Change Description |
---|---|
1.0.0 |
Initial release with basic functionality. |
1.1.0 |
New feature added while maintaining backward compatibility. |
1.1.1 |
Bug fix for an issue without affecting existing features. |
2.0.0 |
Breaking change introduced, incompatible API changes. |
In this example:
- The release of
1.1.0
adds a new feature but doesn't break existing functionality. - The release of
1.1.1
is a patch that fixes a bug without introducing any new features or breaking changes. - The release of
2.0.0
introduces a breaking change, meaning it’s no longer compatible with the previous version.
3. How Semantic Versioning Relates to Node.js Packages
When you manage packages in a Node.js project, understanding how semantic versioning works is crucial for ensuring compatibility and stability. When you install a package using npm
or yarn
, the version of the package is defined by the semantic version number. The version specified in the package.json
file can be defined with different ranges to control which versions of a package your project depends on.
Version Ranges in package.json
In your package.json
file, the dependencies section contains the versions of packages your project uses. You can specify version ranges that allow for flexibility in the versions installed. Here are some common ways to specify version ranges:
Version Range | Meaning |
---|---|
^1.2.3 |
Allow any version that is compatible with 1.2.3 , meaning it can install any version with the same major version (e.g., 1.3.0 , 1.9.9 , but not 2.0.0 ). |
~1.2.3 |
Allow any version that is compatible with 1.2.3 at the patch level, meaning it can install versions like 1.2.4 or 1.2.9 , but not 1.3.0 . |
1.2.3 |
Only allow exactly version 1.2.3 to be installed. |
* |
Allow any version to be installed. |
4. Updating Packages with npm and Yarn
Keeping your project dependencies up to date is essential for security, performance, and new feature access. Both npm
and yarn
offer commands for updating packages:
Updating Packages with npm
To update a package in your project, use the following command:
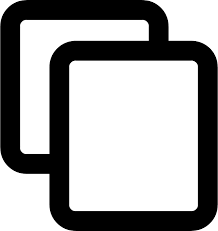
npm update
This will install the latest version of the package that satisfies the version range specified in your package.json
file. If you want to update a specific package to the latest version, you can run:
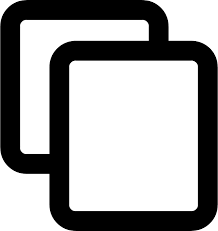
npm install @latest
Updating Packages with Yarn
To update a package using Yarn, you can use the following command:
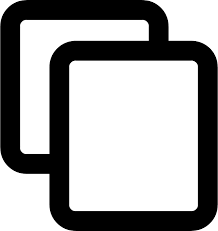
yarn upgrade
Just like with npm, if you want to upgrade to the latest version, you can use:
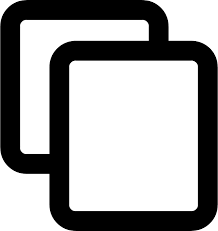
yarn upgrade @latest
5. Considerations for Updating Packages
Before updating packages, especially when dealing with major version upgrades, it’s essential to consider:
- Breaking Changes: Major version updates may introduce breaking changes. Always check the package's changelog to understand any changes that could break your code.
- Compatibility: Ensure that your other dependencies are compatible with the updated package versions.
- Testing: After updating packages, thoroughly test your application to ensure that everything works as expected.
6. Conclusion
Semantic Versioning is a powerful system that helps developers manage package updates in a predictable way. By understanding how version numbers work and using the right version ranges, you can maintain a stable and compatible set of dependencies for your Node.js project. Regularly updating your packages ensures that your application stays up-to-date with the latest features, bug fixes, and security patches.
Reading and Writing Files in Node.js
Node.js provides a built-in fs
(file system) module to interact with files on the server. With the fs
module, you can perform a variety of file operations, such as reading, writing, updating, and deleting files. In this section, we will explore how to read from and write to files using the fs
module in Node.js.
1. Importing the fs
Module
To use file system operations in Node.js, you first need to import the fs
module. You can do this using the require()
function:
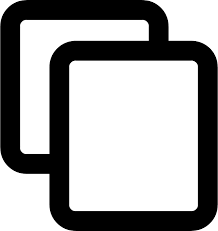
const fs = require('fs');
2. Reading Files
Node.js provides multiple methods for reading files. You can choose between asynchronous and synchronous methods based on your needs.
Asynchronous Reading
The fs.readFile()
method reads the contents of a file asynchronously. This means that the program will not be blocked while the file is being read.
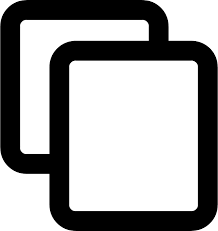
// Asynchronously reading a file
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File contents:", data);
});
In the above example, example.txt
is read asynchronously, and the callback function is executed once the file reading operation is complete. If there is an error, it is logged; otherwise, the file contents are printed to the console.
Synchronous Reading
If you need to read a file synchronously, use the fs.readFileSync()
method. However, note that this will block the execution of your program while the file is being read.
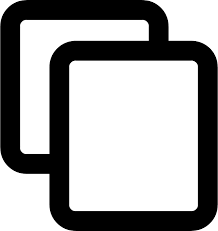
// Synchronously reading a file
try {
const data = fs.readFileSync('example.txt', 'utf8');
console.log("File contents:", data);
} catch (err) {
console.error("Error reading file:", err);
}
3. Writing Files
Node.js also provides ways to write to files using the fs.writeFile()
and fs.appendFile()
methods. These methods allow you to create new files, overwrite existing files, or append data to existing files.
Asynchronous Writing
The fs.writeFile()
method is used to asynchronously write data to a file. If the file does not exist, it will be created; if it exists, the file will be overwritten with the new data.
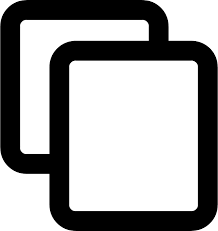
// Asynchronously writing data to a file
const content = "Hello, this is new content!";
fs.writeFile('output.txt', content, (err) => {
if (err) {
console.error("Error writing file:", err);
return;
}
console.log("File has been written successfully!");
});
In this example, output.txt
will be created (or overwritten if it already exists) with the specified content.
Append Data to File
If you want to append data to an existing file instead of overwriting it, you can use the fs.appendFile()
method:
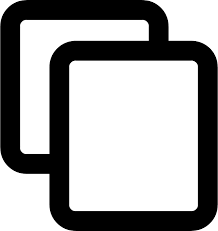
// Appending data to a file
const additionalContent = "\nThis is appended content!";
fs.appendFile('output.txt', additionalContent, (err) => {
if (err) {
console.error("Error appending to file:", err);
return;
}
console.log("Data has been appended to the file!");
});
Synchronous Writing
For synchronous file writing, you can use fs.writeFileSync()
:
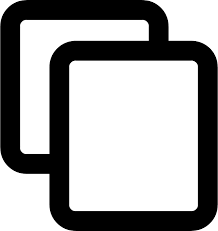
// Synchronously writing data to a file
const contentSync = "This is the content for synchronous write!";
try {
fs.writeFileSync('output-sync.txt', contentSync);
console.log("File has been written successfully!");
} catch (err) {
console.error("Error writing file:", err);
}
4. File Operations Overview
Here’s a summary of common file operations using the fs
module:
Method | Description |
---|---|
fs.readFile() |
Asynchronously reads the contents of a file. |
fs.readFileSync() |
Synchronously reads the contents of a file. |
fs.writeFile() |
Asynchronously writes data to a file, overwriting existing content. |
fs.writeFileSync() |
Synchronously writes data to a file, overwriting existing content. |
fs.appendFile() |
Asynchronously appends data to a file. |
fs.appendFileSync() |
Synchronously appends data to a file. |
fs.unlink() |
Asynchronously deletes a file. |
fs.unlinkSync() |
Synchronously deletes a file. |
5. Conclusion
Node.js provides a powerful set of file system APIs through the fs
module. Depending on your needs, you can choose between asynchronous or synchronous methods for reading and writing files. Asynchronous methods are non-blocking and preferred in most cases, while synchronous methods are more suitable for simple scripts or when blocking the event loop is not a concern.
Creating and Deleting Files and Directories
Node.js provides the fs
module to create and delete files and directories. These operations are essential for file system management and can be done using both asynchronous and synchronous methods. This section will guide you through how to create and delete files and directories in Node.js.
1. Importing the fs
Module
To use file system operations in Node.js, you need to import the fs
module:
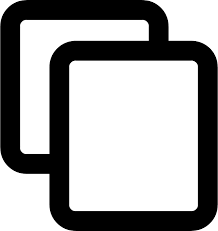
const fs = require('fs');
2. Creating Files
You can create files using the fs.writeFile()
or fs.open()
methods. If the file does not exist, it will be created; if it exists, the content will be overwritten (in the case of fs.writeFile()
).
Creating Files Asynchronously
The fs.writeFile()
method creates a file asynchronously. If the file already exists, it overwrites the content.
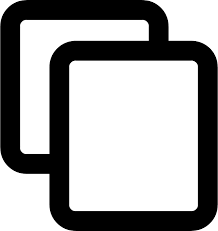
// Creating a file asynchronously
const content = "This is the content of the new file!";
fs.writeFile('newfile.txt', content, (err) => {
if (err) {
console.error("Error creating file:", err);
return;
}
console.log("File created successfully!");
});
In this example, if newfile.txt
does not exist, it will be created with the specified content. If it exists, the content will be overwritten.
Creating Files Synchronously
You can also create files synchronously using the fs.writeFileSync()
method:
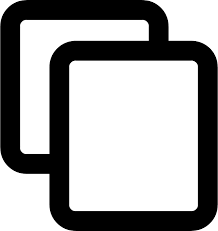
// Creating a file synchronously
const contentSync = "This is the content for the new file!";
try {
fs.writeFileSync('newfile-sync.txt', contentSync);
console.log("File created successfully!");
} catch (err) {
console.error("Error creating file:", err);
}
3. Creating Directories
To create directories, you can use the fs.mkdir()
or fs.mkdirSync()
methods. These methods create a new directory at the specified path.
Creating Directories Asynchronously
The fs.mkdir()
method creates a directory asynchronously. If the directory already exists, an error will be thrown unless you specify the { recursive: true }
option.
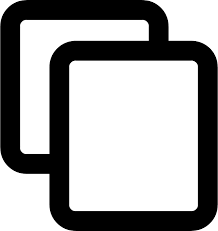
// Creating a directory asynchronously
fs.mkdir('newdir', { recursive: true }, (err) => {
if (err) {
console.error("Error creating directory:", err);
return;
}
console.log("Directory created successfully!");
});
Creating Directories Synchronously
You can also create directories synchronously using the fs.mkdirSync()
method:
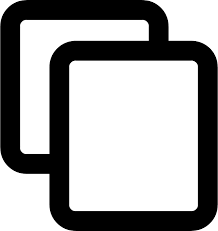
// Creating a directory synchronously
try {
fs.mkdirSync('newdir-sync', { recursive: true });
console.log("Directory created successfully!");
} catch (err) {
console.error("Error creating directory:", err);
}
4. Deleting Files
Node.js provides the fs.unlink()
or fs.unlinkSync()
method to delete files. These methods remove the specified file from the file system.
Deleting Files Asynchronously
The fs.unlink()
method deletes a file asynchronously:
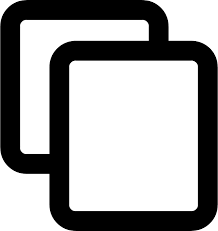
// Deleting a file asynchronously
fs.unlink('newfile.txt', (err) => {
if (err) {
console.error("Error deleting file:", err);
return;
}
console.log("File deleted successfully!");
});
Deleting Files Synchronously
You can also delete files synchronously using the fs.unlinkSync()
method:
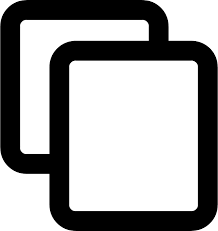
// Deleting a file synchronously
try {
fs.unlinkSync('newfile-sync.txt');
console.log("File deleted successfully!");
} catch (err) {
console.error("Error deleting file:", err);
}
5. Deleting Directories
To remove directories, you can use the fs.rmdir()
or fs.rmdirSync()
methods. These methods remove the directory at the specified path.
Deleting Directories Asynchronously
The fs.rmdir()
method deletes an empty directory asynchronously:
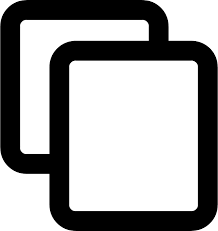
// Deleting a directory asynchronously
fs.rmdir('newdir', (err) => {
if (err) {
console.error("Error deleting directory:", err);
return;
}
console.log("Directory deleted successfully!");
});
Deleting Directories Synchronously
You can also delete directories synchronously using the fs.rmdirSync()
method:
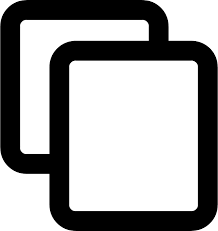
// Deleting a directory synchronously
try {
fs.rmdirSync('newdir-sync');
console.log("Directory deleted successfully!");
} catch (err) {
console.error("Error deleting directory:", err);
}
6. Directory Deletion with Contents
If you want to remove a directory that contains files or other directories, you must first remove the contents before deleting the directory. You can use the fs.rmdirSync()
method recursively or use the fs.rm()
method with the { recursive: true }
option.
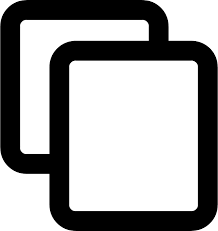
// Deleting a directory with contents
fs.rm('newdir', { recursive: true, force: true }, (err) => {
if (err) {
console.error("Error deleting directory with contents:", err);
return;
}
console.log("Directory and its contents deleted successfully!");
});
7. Conclusion
Node.js provides powerful file system operations via the fs
module, allowing you to create and delete both files and directories. You can choose between asynchronous and synchronous methods depending on your needs. Asynchronous methods are generally preferred because they allow the program to remain non-blocking, while synchronous methods may be useful in simple scripts or specific scenarios where blocking the event loop is acceptable.
Working with Streams and Buffers
In Node.js, streams and buffers are essential for handling large amounts of data efficiently. Streams allow you to read or write data in a continuous flow, while buffers store binary data in memory. These concepts are crucial for handling tasks like reading large files, real-time data processing, or interacting with databases and APIs.
1. Introduction to Streams
Streams are objects that allow you to read or write data in chunks. Node.js provides several types of streams, including:
- Readable Streams: Used for reading data.
- Writable Streams: Used for writing data.
- Duplex Streams: Can be both readable and writable.
- Transform Streams: A type of duplex stream that modifies the data as it is written and read.
2. Creating and Using Readable Streams
Readable streams allow you to read data from a source. You can use the fs.createReadStream()
method to read files as streams. For example, reading a file line by line:
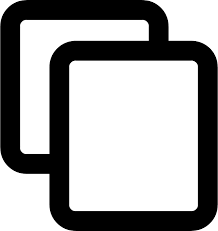
// Reading a file using a readable stream
const fs = require('fs');
const readableStream = fs.createReadStream('largeFile.txt', 'utf8');
readableStream.on('data', (chunk) => {
console.log("Received chunk:", chunk);
});
readableStream.on('end', () => {
console.log("File reading completed.");
});
readableStream.on('error', (err) => {
console.error("Error reading file:", err);
});
In this example, data is read from largeFile.txt
in chunks. The 'data' event is emitted when a chunk of data is available, and the 'end' event signals the completion of the read operation.
3. Creating and Using Writable Streams
Writable streams are used for writing data to a destination. You can use the fs.createWriteStream()
method to write data to a file. Here's an example:
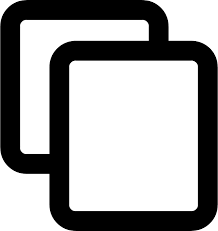
// Writing data to a file using a writable stream
const writableStream = fs.createWriteStream('output.txt');
writableStream.write('Hello, Stream!', 'utf8');
writableStream.end(); // Ends the stream
writableStream.on('finish', () => {
console.log("File writing completed.");
});
writableStream.on('error', (err) => {
console.error("Error writing file:", err);
});
In this example, data is written to output.txt
using a writable stream. The 'finish' event signals the end of the write operation.
4. Piping Streams
Streams in Node.js can be piped to connect a readable stream to a writable stream. This allows data from the readable stream to flow directly into the writable stream. The pipe()
method is used for this:
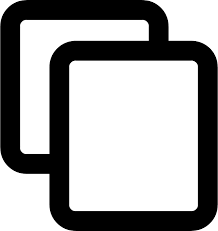
// Piping a readable stream to a writable stream
const readableStream = fs.createReadStream('largeFile.txt');
const writableStream = fs.createWriteStream('output.txt');
readableStream.pipe(writableStream);
writableStream.on('finish', () => {
console.log("File has been copied successfully.");
});
In this example, data is read from largeFile.txt
and written directly to output.txt
using the pipe()
method, making the process efficient and straightforward.
5. Introduction to Buffers
A buffer is a chunk of memory allocated to store binary data. Buffers in Node.js are used to handle raw binary data directly, such as when interacting with binary files, streams, or network protocols. Buffers are especially useful when working with binary data that doesn’t need to be interpreted as a string.
6. Creating Buffers
You can create a buffer by allocating memory or from an existing data source like an array or string. Here are some examples:
Creating an Empty Buffer
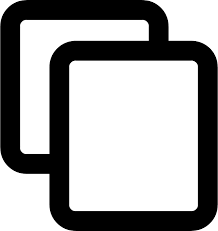
// Creating an empty buffer of size 10
const buffer = Buffer.alloc(10);
console.log(buffer);
Creating a Buffer from an Array
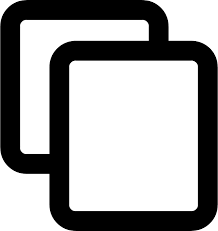
// Creating a buffer from an array
const bufferFromArray = Buffer.from([1, 2, 3, 4, 5]);
console.log(bufferFromArray);
Creating a Buffer from a String
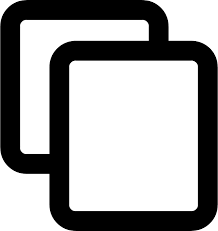
// Creating a buffer from a string
const bufferFromString = Buffer.from('Hello, Buffer!');
console.log(bufferFromString);
7. Manipulating Buffers
Once a buffer is created, you can access and manipulate its contents. Buffers are similar to arrays, but they represent raw binary data. Here's how to read and write to a buffer:
Reading from a Buffer
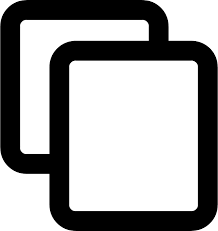
// Reading from a buffer
const buffer = Buffer.from('Hello, Buffer!');
console.log(buffer.toString('utf8')); // Converts buffer to string
Writing to a Buffer
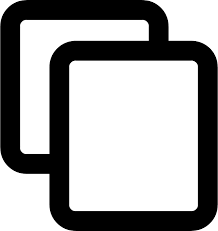
// Writing to a buffer
buffer.write('New Data');
console.log(buffer.toString());
8. Conclusion
Streams and buffers are fundamental in Node.js for working with large or binary data. Streams enable efficient data reading and writing, while buffers provide a way to handle raw binary data. By leveraging streams and buffers, you can build scalable and performant applications in Node.js.
File Upload Handling
In Node.js, handling file uploads is a common requirement for many applications such as social media platforms, document storage, and content management systems. To handle file uploads efficiently, you need to use middleware that facilitates the parsing and storage of uploaded files. One of the most popular libraries for file upload handling in Node.js is multer.
1. Introduction to Multer
Multer is a middleware for handling multipart/form-data, which is used for uploading files. It provides easy configuration options for file storage, file size limits, and file type validation.
2. Installing Multer
To get started with Multer, first install the package using npm:
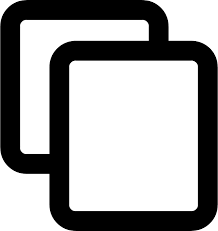
// Install multer using npm
npm install multer
3. Basic Configuration and Handling File Upload
Here’s how you can set up Multer to handle file uploads in your Node.js application:
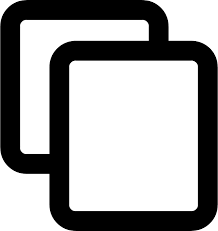
// Importing necessary modules
const express = require('express');
const multer = require('multer');
const path = require('path');
// Initialize express app
const app = express();
// Set up storage engine and configure file destination
const storage = multer.diskStorage({
destination: (req, file, cb) => {
cb(null, './uploads'); // Directory to store uploaded files
},
filename: (req, file, cb) => {
cb(null, Date.now() + path.extname(file.originalname)); // Naming convention for uploaded files
}
});
// Set up multer upload middleware
const upload = multer({ storage: storage });
// Define upload route
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded.');
}
res.send(`File uploaded successfully: ${req.file.filename}`);
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, the multer.diskStorage()
method is used to define the destination and filename for uploaded files. The upload.single('file')
middleware is used to handle a single file upload with the field name 'file'. The uploaded file is stored in the uploads
directory, and the file is renamed using the current timestamp to avoid conflicts.
4. Handling Multiple File Uploads
To handle multiple file uploads in a single request, you can use the upload.array()
middleware. Here’s an example of how to allow multiple files to be uploaded:
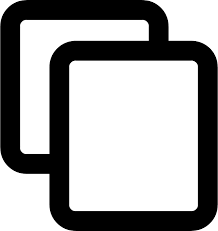
// Handling multiple file uploads
app.post('/upload-multiple', upload.array('files', 5), (req, res) => {
if (!req.files) {
return res.status(400).send('No files uploaded.');
}
const fileNames = req.files.map(file => file.filename);
res.send(`Files uploaded successfully: ${fileNames.join(', ')}`);
});
In this example, upload.array('files', 5)
allows a maximum of 5 files to be uploaded at once. The uploaded files are then stored in the uploads
directory, and their filenames are returned in the response.
5. File Validation
Multer also provides an option to validate files based on file size and file type. You can set limits and filters to ensure only valid files are uploaded. Here’s an example of how to limit the file size and restrict the file types to images:
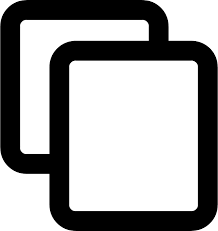
// Set up multer with file validation
const uploadWithValidation = multer({
storage: storage,
limits: { fileSize: 2 * 1024 * 1024 }, // Limit file size to 2 MB
fileFilter: (req, file, cb) => {
const fileTypes = /jpeg|jpg|png|gif/;
const extname = fileTypes.test(path.extname(file.originalname).toLowerCase());
const mimetype = fileTypes.test(file.mimetype);
if (extname && mimetype) {
return cb(null, true);
} else {
return cb(new Error('Only image files are allowed.'));
}
}
});
// Route for file upload with validation
app.post('/upload-validated', uploadWithValidation.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded or invalid file type.');
}
res.send(`File uploaded successfully: ${req.file.filename}`);
});
In this example, the limits
property restricts the uploaded file size to 2 MB, and the fileFilter
property ensures that only image files with the extensions jpeg
, jpg
, png
, or gif
can be uploaded. If a file is invalid, an error is thrown.
6. Handling Errors
When working with file uploads, it’s important to handle errors gracefully. Multer provides error handling via a try-catch
block or by listening for the error
event:
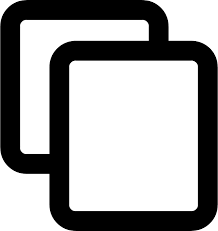
// Error handling for file upload
app.post('/upload-with-error-handling', upload.single('file'), (req, res) => {
try {
if (!req.file) {
throw new Error('No file uploaded.');
}
res.send(`File uploaded successfully: ${req.file.filename}`);
} catch (err) {
res.status(400).send(`Error: ${err.message}`);
}
});
In this example, if an error occurs during the file upload (e.g., no file is uploaded or an invalid file is uploaded), a custom error message is sent as the response.
7. Conclusion
Handling file uploads in Node.js with Multer is straightforward and flexible. You can configure it for single or multiple file uploads, apply file validation, and handle errors gracefully. By using Multer, you can ensure that file uploads are managed efficiently in your application.
Creating an HTTP Server with Node.js
Node.js provides a built-in module called http that allows you to create an HTTP server to handle requests and responses. Using the http module, you can build simple web servers to serve static files, handle API requests, or even build a full-fledged web application.
1. Introduction to the HTTP Module
The http
module in Node.js allows you to create an HTTP server that listens for incoming requests and sends responses. This module provides methods to handle request URLs, headers, and status codes, enabling you to build your server's functionality.
2. Setting Up a Basic HTTP Server
Let’s start by creating a basic HTTP server that listens on port 3000 and responds to requests with "Hello, World!".
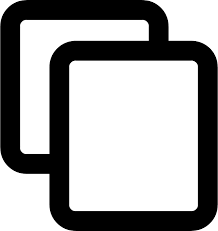
// Importing the http module
const http = require('http');
// Creating the server
const server = http.createServer((req, res) => {
// Setting the response header
res.writeHead(200, { 'Content-Type': 'text/plain' });
// Sending the response
res.end('Hello, World!\n');
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we use http.createServer()
to create a server instance. The server listens for incoming requests and responds by sending a "Hello, World!" message. The res.writeHead()
method sets the HTTP status code (200 OK) and the content type for the response. Finally, the server listens on port 3000 with the server.listen()
method.
3. Handling Different Request Methods
HTTP servers often need to handle different types of requests, such as GET
, POST
, and others. In the following example, we’ll handle GET
and POST
requests differently:
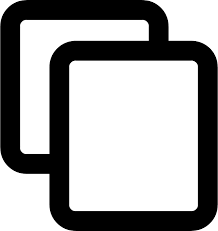
// Creating the server with different methods handling
const server = http.createServer((req, res) => {
if (req.method === 'GET') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('This is a GET request\n');
} else if (req.method === 'POST') {
let body = '';
req.on('data', chunk => {
body += chunk;
});
req.on('end', () => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end(`Received POST data: ${body}`);
});
} else {
res.writeHead(405, { 'Content-Type': 'text/plain' });
res.end('Method Not Allowed\n');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we check the req.method
property to determine the type of request. If it’s a GET
request, we send a message indicating it’s a GET request. If it’s a POST
request, we collect the data sent with the request, and send it back as the response. If the method is neither GET nor POST, we respond with a "Method Not Allowed" message.
4. Handling Query Strings and URL Parameters
Often, HTTP requests contain query parameters or URL parameters. To handle these, we can use the built-in url module in Node.js to parse the request URL. Here’s an example:
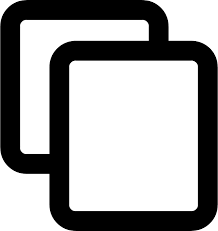
// Importing required modules
const http = require('http');
const url = require('url');
// Creating the server
const server = http.createServer((req, res) => {
const parsedUrl = url.parse(req.url, true);
const query = parsedUrl.query;
res.writeHead(200, { 'Content-Type': 'text/plain' });
if (parsedUrl.pathname === '/greet' && query.name) {
res.end(`Hello, ${query.name}!\n`);
} else {
res.end('Please provide a valid name in the query string.\n');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we use the url.parse()
method to parse the request URL. We extract the query parameters using the query
property, and if the name
parameter is provided, we greet the user. If no name is provided, we ask for a valid name.
5. Sending JSON Responses
It’s common in APIs to send responses in JSON format. Here’s how you can send a JSON response from your HTTP server:
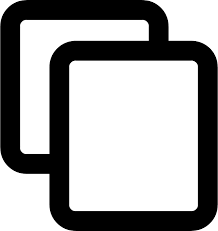
// Creating the server to send JSON responses
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'application/json' });
const responseObject = {
message: 'Welcome to the Node.js API',
status: 'success'
};
res.end(JSON.stringify(responseObject));
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we set the Content-Type
header to application/json
, and then use JSON.stringify()
to convert an object into a JSON string before sending it in the response.
6. Conclusion
Creating an HTTP server in Node.js is a simple and powerful way to handle HTTP requests. By using the http module, you can build servers that handle various HTTP methods, query strings, and JSON responses. This provides the foundation for building APIs, handling web requests, and creating dynamic web applications.
Handling GET, POST, PUT, and DELETE Requests
In Node.js, handling different HTTP methods is essential for building RESTful APIs and web applications. The four most common HTTP methods are GET, POST, PUT, and DELETE. These methods are used to request, create, update, and delete resources on a server, respectively.
1. Introduction to HTTP Methods
Each HTTP method corresponds to a specific action on the server. Here’s a brief overview:
- GET: Retrieves data from the server.
- POST: Sends data to the server to create a new resource.
- PUT: Updates an existing resource on the server.
- DELETE: Deletes a resource from the server.
2. Handling GET Requests
The GET
method is used to fetch data from the server. Typically, GET requests are used to retrieve resources such as web pages, images, or JSON data.
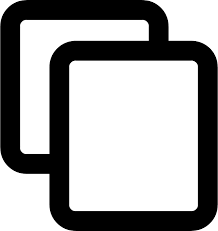
// Importing the http module
const http = require('http');
// Creating the server
const server = http.createServer((req, res) => {
if (req.method === 'GET' && req.url === '/users') {
res.writeHead(200, { 'Content-Type': 'application/json' });
const users = [{ id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Doe' }];
res.end(JSON.stringify(users));
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we handle GET requests to the /users
URL. The server sends a JSON response containing a list of users. If the URL is not found, the server returns a 404 error.
3. Handling POST Requests
The POST
method is used to send data to the server, typically to create a new resource. In this example, we’ll handle a POST request to create a new user:
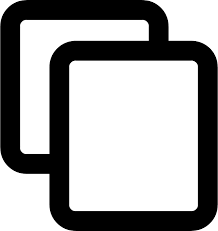
// Creating the server to handle POST requests
const server = http.createServer((req, res) => {
if (req.method === 'POST' && req.url === '/users') {
let body = '';
req.on('data', chunk => {
body += chunk;
});
req.on('end', () => {
const user = JSON.parse(body);
res.writeHead(201, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User created', user }));
});
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Here, we handle POST requests to the /users
URL. The server collects the data sent with the request, parses it, and returns a message confirming the creation of the new user.
4. Handling PUT Requests
The PUT
method is used to update an existing resource. In this example, we’ll handle a PUT request to update an existing user:
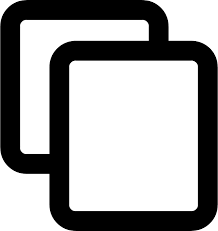
// Creating the server to handle PUT requests
const server = http.createServer((req, res) => {
if (req.method === 'PUT' && req.url === '/users/1') {
let body = '';
req.on('data', chunk => {
body += chunk;
});
req.on('end', () => {
const updatedUser = JSON.parse(body);
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User updated', updatedUser }));
});
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we handle PUT requests to update the user with an ID of 1
. The request body contains the updated user data, which the server parses and responds with a confirmation message.
5. Handling DELETE Requests
The DELETE
method is used to delete a resource. Here’s how to handle DELETE requests to remove a user:
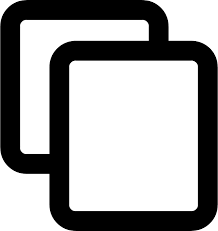
// Creating the server to handle DELETE requests
const server = http.createServer((req, res) => {
if (req.method === 'DELETE' && req.url === '/users/1') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User deleted' }));
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we handle DELETE requests to delete the user with an ID of 1
. If the user is found, the server responds with a message confirming the deletion.
6. Conclusion
Handling GET, POST, PUT, and DELETE requests in Node.js allows you to build robust RESTful APIs. By using these HTTP methods, you can create, read, update, and delete resources on your server. This is the foundation of interacting with data in web applications and APIs.
Building a Simple REST API with Node.js
Building a REST API using Node.js involves creating an HTTP server that listens for different HTTP methods (GET, POST, PUT, DELETE) and interacts with resources such as data stored in a database or an in-memory data structure. In this section, we will walk through the process of building a simple REST API using Node.js and the built-in http
module.
1. Setting Up the Server
The first step in building a REST API is to set up a server that listens for incoming HTTP requests. For simplicity, we will use the http
module in Node.js.
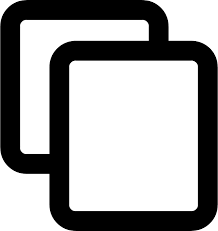
// Importing the http module
const http = require('http');
// Creating the server
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Welcome to the REST API' }));
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we create a basic HTTP server that listens on port 3000
and responds with a JSON object. This is our starting point for building a REST API.
2. Defining API Endpoints
Now that we have a basic server, let’s define a few endpoints for our REST API. We will handle GET
, POST
, and DELETE
requests for a simple resource—a list of users.
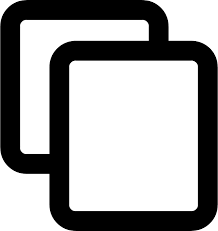
// Sample data
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
];
// Creating the server
const server = http.createServer((req, res) => {
// Handling GET requests
if (req.method === 'GET' && req.url === '/users') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify(users));
}
// Handling POST requests
else if (req.method === 'POST' && req.url === '/users') {
let body = '';
req.on('data', chunk => {
body += chunk;
});
req.on('end', () => {
const newUser = JSON.parse(body);
users.push(newUser);
res.writeHead(201, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User created', newUser }));
});
}
// Handling DELETE requests
else if (req.method === 'DELETE' && req.url.startsWith('/users/')) {
const id = parseInt(req.url.split('/')[2]);
const userIndex = users.findIndex(user => user.id === id);
if (userIndex !== -1) {
users.splice(userIndex, 1);
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User deleted' }));
} else {
res.writeHead(404, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'User not found' }));
}
}
else {
res.writeHead(404, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Not Found' }));
}
});
// Server listening on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we define three endpoints:
GET /users
: Retrieves a list of users.POST /users
: Adds a new user to the list.DELETE /users/:id
: Deletes a user by their ID.
When a GET
request is made to /users
, the server responds with a list of users. A POST
request to the same endpoint adds a new user. A DELETE
request removes a user by their ID from the list.
3. Testing the API
Once the server is running, you can test the API using tools like Postman, cURL, or the browser (for GET requests). Below are examples of how to interact with the API:
- GET /users: Retrieves the list of users.
- POST /users: Adds a new user. Use the following JSON format for the request body:
{ "id": 3, "name": "Alice Johnson" }
- DELETE /users/1: Deletes the user with ID 1.
4. Conclusion
In this section, we have built a simple REST API using Node.js and the http
module. We’ve defined basic CRUD operations—Create, Read, Update, and Delete—using the GET
, POST
, and DELETE
HTTP methods. This API can be expanded further by adding additional functionality, such as authentication, data validation, and more advanced error handling.
Using HTTPS for Secure Connections in Node.js
HTTPS (HyperText Transfer Protocol Secure) is the secure version of HTTP, which encrypts the data exchanged between the client and the server using SSL/TLS protocols. In a Node.js application, enabling HTTPS ensures that the data transmitted between the server and users is encrypted, preventing unauthorized access or tampering. In this section, we will demonstrate how to set up an HTTPS server in Node.js.
1. Prerequisites
Before setting up an HTTPS server, you need an SSL/TLS certificate. You can obtain a free certificate from Let's Encrypt or purchase a certificate from a Certificate Authority (CA). For the development environment, you can use self-signed certificates.
2. Setting Up the HTTPS Server
To use HTTPS in a Node.js application, you need to import the https
module and provide SSL certificate files (public and private keys). Below is a sample code to set up an HTTPS server:
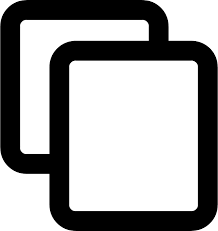
// Import required modules
const https = require('https');
const fs = require('fs');
// Load SSL certificate files
const options = {
key: fs.readFileSync('path/to/your/private-key.pem'),
cert: fs.readFileSync('path/to/your/certificate.pem')
};
// Create the HTTPS server
const server = https.createServer(options, (req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, HTTPS world!');
});
// Start the server on port 443
server.listen(443, () => {
console.log('HTTPS server running on https://localhost:443');
});
In this example, we create an HTTPS server using the https.createServer()
method. We specify the path to the private key and certificate files in the options
object. The server listens on port 443
, which is the default port for HTTPS connections.
3. Redirecting HTTP to HTTPS
It is a good practice to ensure that all traffic is served over HTTPS. You can redirect HTTP traffic to HTTPS by setting up an HTTP server that automatically redirects requests to the HTTPS version of your site.
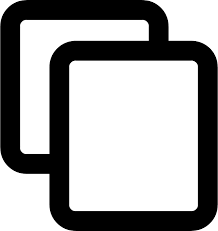
// Import the http module for HTTP server
const http = require('http');
// HTTP server that redirects to HTTPS
const redirectServer = http.createServer((req, res) => {
res.writeHead(301, { 'Location': 'https://' + req.headers['host'] + req.url });
res.end();
});
// Redirect HTTP traffic to HTTPS
redirectServer.listen(80, () => {
console.log('HTTP server running on http://localhost:80 (redirecting to HTTPS)');
});
This HTTP server listens on port 80
and redirects all requests to the same URL with the https
protocol.
4. Testing the HTTPS Server
Once the server is running, you can test the HTTPS server by navigating to https://localhost
in your browser. If you are using self-signed certificates, your browser may show a warning because the certificate is not trusted by a recognized Certificate Authority. In production, you should use a certificate from a trusted CA to avoid this warning.
5. Conclusion
In this section, we demonstrated how to set up a secure HTTPS server in Node.js. By enabling HTTPS, you ensure that all communications between the client and server are encrypted and secure. Additionally, we set up an HTTP server to automatically redirect traffic to HTTPS, ensuring that users always access the secure version of your site. Make sure to obtain a valid SSL/TLS certificate for production environments to ensure trustworthiness and security.
Making HTTP Requests with `http` and `https` Modules in Node.js
Node.js provides built-in modules such as http
and https
to make HTTP and HTTPS requests. These modules allow you to send requests to external APIs, fetch data from websites, and interact with remote servers. In this section, we will learn how to make HTTP requests using both the http
and https
modules in Node.js.
1. Making a Simple HTTP Request
To make an HTTP request, you can use the http.request()
method from the http
module. Below is a basic example of how to make a GET request to a public API:
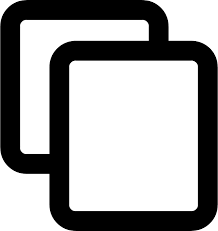
// Import the http module
const http = require('http');
// Making a GET request to a public API
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/todos/1',
method: 'GET'
};
const req = http.request(options, (res) => {
let data = '';
// Collect data chunks
res.on('data', (chunk) => {
data += chunk;
});
// When the response is complete, parse and display the data
res.on('end', () => {
console.log('Response:', JSON.parse(data));
});
});
// Handle any errors
req.on('error', (error) => {
console.error('Request error:', error);
});
// End the request
req.end();
In this example, we use http.request()
to send a GET request to jsonplaceholder.typicode.com
, which is a free API for testing and prototyping. We specify the request method, the hostname, and the path of the resource we want to fetch. The response is collected in chunks and printed to the console once the request is complete.
2. Making an HTTPS Request
To make an HTTPS request, you can use the https.request()
method from the https
module. The process is similar to using the http
module, but you must ensure that the URL uses the https
protocol.
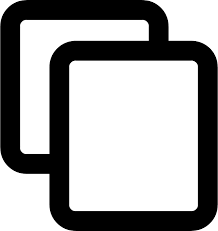
// Import the https module
const https = require('https');
// Making a GET request to a secure API
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/todos/1',
method: 'GET'
};
const req = https.request(options, (res) => {
let data = '';
// Collect data chunks
res.on('data', (chunk) => {
data += chunk;
});
// When the response is complete, parse and display the data
res.on('end', () => {
console.log('Response:', JSON.parse(data));
});
});
// Handle any errors
req.on('error', (error) => {
console.error('Request error:', error);
});
// End the request
req.end();
In this example, we use https.request()
to send a GET request to the same API. The key difference is that we import the https
module instead of http
. Otherwise, the process for sending the request and processing the response is identical.
3. Handling Query Parameters
If you need to include query parameters in your HTTP or HTTPS request, you can append them to the path
field in the options object. Below is an example of how to make a GET request with query parameters:
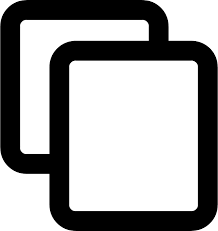
// Making a GET request with query parameters
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/todos?userId=1', // Adding query parameter
method: 'GET'
};
const req = https.request(options, (res) => {
let data = '';
// Collect data chunks
res.on('data', (chunk) => {
data += chunk;
});
// When the response is complete, parse and display the data
res.on('end', () => {
console.log('Response:', JSON.parse(data));
});
});
// Handle any errors
req.on('error', (error) => {
console.error('Request error:', error);
});
// End the request
req.end();
In this example, the query parameter userId=1
is appended to the API path. The server will use the query parameter to filter the results.
4. Sending Data with POST Requests
To send data in a POST request, you need to include the request body. You can use the req.write()
method to send the data and req.end()
to indicate the end of the request.
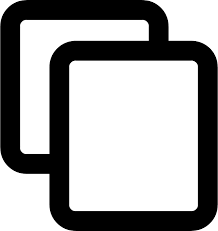
// Making a POST request with data
const data = JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1
});
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/posts',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': Buffer.byteLength(data)
}
};
const req = https.request(options, (res) => {
let responseData = '';
res.on('data', (chunk) => {
responseData += chunk;
});
res.on('end', () => {
console.log('Response:', JSON.parse(responseData));
});
});
req.on('error', (error) => {
console.error('Request error:', error);
});
// Write data to the request body
req.write(data);
// End the request
req.end();
In this example, we send a POST request to create a new post using the JSONPlaceholder API. The data is stringified and sent as the request body. The Content-Type
header is set to application/json
, and the length of the data is specified using the Content-Length
header.
5. Conclusion
In this section, we have explored how to make HTTP and HTTPS requests in Node.js using the http
and https
modules. We covered making simple GET requests, handling query parameters, sending POST requests with data, and handling responses. These capabilities allow you to communicate with external servers and APIs, enabling your Node.js applications to interact with remote resources.
Introduction to Express.js
Express.js is a fast, unopinionated, and minimalist web framework for Node.js that simplifies the process of building web applications and APIs. It is one of the most popular frameworks in the Node.js ecosystem due to its simplicity, flexibility, and robust features. Express provides a set of powerful tools to handle HTTP requests, middleware, routing, and more, making it a great choice for both small and large-scale applications.
1. Why Use Express.js?
Node.js provides the basic functionality to create HTTP servers, but building a full-featured web application from scratch can be cumbersome. Express.js is designed to make development easier by adding a layer of abstraction on top of Node.js. Some of the key benefits of using Express.js include:
- Simplified Routing: Express offers a clean and concise way to define routes for handling different HTTP methods (GET, POST, PUT, DELETE, etc.) and request paths.
- Middleware Support: Express allows you to define middleware functions that can be used to process requests before they are passed to route handlers.
- Template Engines: Express supports various template engines for rendering dynamic HTML views, such as EJS, Pug, and Handlebars.
- Extensibility: You can easily extend Express with additional third-party middleware to enhance functionality, such as handling file uploads, authentication, and more.
2. Setting Up Express.js
Before you can use Express.js in your application, you need to install it using npm (Node Package Manager). Here’s how to set up an Express.js project:
- Initialize a new Node.js project by running the following command in your terminal:
npm init -y
- Install Express.js as a dependency by running:
npm install express
- Create a new JavaScript file (e.g.,
app.js
) and import Express.js in your code:const express = require('express');
- Set up a simple Express server:
// Import express const express = require('express'); // Create an express app const app = express(); // Define a basic route app.get('/', (req, res) => { res.send('Hello, Express!'); }); // Start the server on port 3000 app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); });
This code sets up a basic Express server that listens on port 3000 and responds with "Hello, Express!" when you navigate to the root URL. You can test it by visiting http://localhost:3000
in your browser.
3. Express.js Routing
One of the core features of Express.js is routing. Routing allows you to define endpoints that handle requests with specific HTTP methods (GET, POST, PUT, DELETE) and URLs. Here’s an example of how to define multiple routes in an Express app:
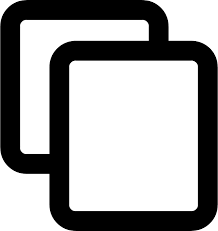
// Import express
const express = require('express');
const app = express();
// Define routes for different HTTP methods
app.get('/', (req, res) => {
res.send('GET request to the homepage');
});
app.post('/', (req, res) => {
res.send('POST request to the homepage');
});
app.put('/update', (req, res) => {
res.send('PUT request to the /update endpoint');
});
app.delete('/delete', (req, res) => {
res.send('DELETE request to the /delete endpoint');
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, we define routes for different HTTP methods on different paths. Express handles the requests based on the HTTP method and the route specified. For instance, when a GET request is made to the root URL, the first route will respond, and when a POST request is made, the second route will respond.
4. Middleware in Express.js
Middleware functions are functions that have access to the request, response, and the next function in the application’s request-response cycle. Middleware can be used to perform tasks like logging, authentication, and data validation. Here’s an example of how to use middleware in Express.js:
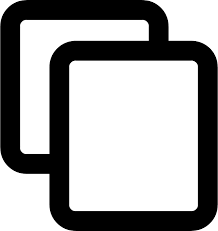
// Import express
const express = require('express');
const app = express();
// Define a middleware function
const logRequest = (req, res, next) => {
console.log(`${req.method} request made to ${req.url}`);
next(); // Pass control to the next middleware or route handler
};
// Use the middleware globally
app.use(logRequest);
// Define routes
app.get('/', (req, res) => {
res.send('Hello, Express with Middleware!');
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, the logRequest
middleware logs the HTTP method and URL of each incoming request. The next()
function is called to pass control to the next middleware or route handler.
5. Conclusion
Express.js is a powerful and flexible framework for building web applications and APIs in Node.js. It simplifies routing, handles middleware, and allows you to scale applications easily with its extensibility. In this section, we have covered the basics of setting up an Express app, defining routes, using middleware, and creating a simple server. Express.js is an excellent choice for developers who want to quickly build robust web applications with Node.js.
Setting Up Express.js
Setting up Express.js is a straightforward process. It involves installing the required dependencies, creating a basic Express application, and running a simple server. This section will guide you through the steps of setting up Express.js in your Node.js project.
1. Initialize a Node.js Project
Before setting up Express.js, you need to have a Node.js project. If you haven’t done so already, initialize your Node.js project by running the following command in your terminal or command prompt:
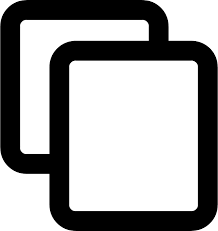
npm init -y
This command will generate a package.json
file with default values. The -y
flag automatically answers "yes" to all prompts during initialization, allowing you to quickly set up the project.
2. Install Express.js
After initializing the project, you need to install Express.js. To install Express, run the following command:
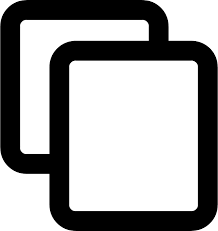
npm install express
This will add Express.js as a dependency in your package.json
file and install it in the node_modules
directory.
3. Create an Express Application
Now that Express.js is installed, you can create your Express application. Create a new JavaScript file, such as app.js
, in the root of your project directory, and set up a basic Express server:
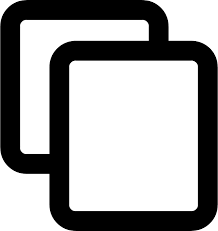
// Import Express
const express = require('express');
// Create an Express application
const app = express();
// Define a basic route
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this code:
express()
creates an instance of the Express application.app.get()
defines a route that handles GET requests to the root URL (/
).app.listen()
starts the server on port 3000 and logs a message indicating the server is running.
4. Run the Express Server
To run the server, open your terminal and navigate to the project directory where app.js
is located. Then, run the following command:
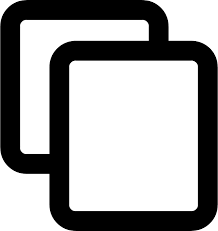
node app.js
This command will start the server, and you should see the message Server is running on http://localhost:3000
in your terminal.
5. Access the Application
Once the server is running, open a browser and navigate to http://localhost:3000
. You should see the message "Hello, Express!" displayed in your browser.
6. Basic Directory Structure
When you create an Express application, you can structure your project in a way that makes it scalable and maintainable. A simple directory structure for a basic Express app could look like this:
my-express-app/
├── node_modules/ # Contains installed dependencies
├── package.json # Project metadata and dependencies
├── app.js # Main application file
└── package-lock.json # Automatically generated for any operations where npm modifies the node_modules directory
As your project grows, you can add additional folders for routes, controllers, views, and other necessary components.
7. Conclusion
Setting up an Express.js application is simple and quick. By following the steps outlined above, you’ve created a basic Express server that handles HTTP requests. As you continue developing with Express, you can add routes, middleware, and views to extend your app’s functionality.
Routing in Express.js
Routing in Express.js is a way to define how an application responds to client requests for specific URLs (or paths) using various HTTP methods (GET, POST, PUT, DELETE). Express makes routing simple and intuitive, allowing you to define routes for different request types and URL paths.
1. Basic Routing
In Express, routing is defined by using methods like app.get()
, app.post()
, app.put()
, and app.delete()
, among others. Each method corresponds to a specific HTTP request method, and you can associate these methods with a path (URL) and a callback function that handles the request.
Here is a simple example of defining a route for a GET request:
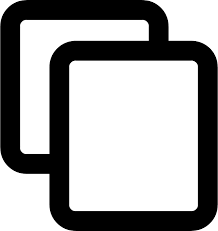
// Import Express
const express = require('express');
const app = express();
// Define a route for GET requests to the root path
app.get('/', (req, res) => {
res.send('Welcome to Express Routing!');
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, when a GET request is made to /
(the root URL), the server responds with the message "Welcome to Express Routing!".
2. Route Parameters
Express allows you to capture values from the URL using route parameters. These parameters can be used to pass dynamic values to your route handlers.
For example, you can define a route that captures a userId
parameter from the URL:
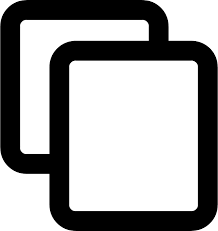
// Define a route with a dynamic parameter
app.get('/user/:userId', (req, res) => {
const userId = req.params.userId;
res.send(`User ID: ${userId}`);
});
In this case, if a user navigates to /user/123
, the response will be "User ID: 123". The value of userId
is captured from the URL using req.params.userId
.
3. Query Parameters
In addition to route parameters, you can also use query parameters to pass additional data. Query parameters are included in the URL after a question mark (?
) and are separated by an ampersand (&
) if there are multiple parameters.
For example, consider the following route that accepts a search
query parameter:
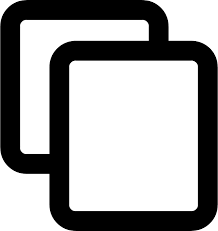
// Define a route that accepts query parameters
app.get('/search', (req, res) => {
const searchQuery = req.query.search;
res.send(`Search Results for: ${searchQuery}`);
});
If a user navigates to /search?search=express
, the response will be "Search Results for: express". You can access the query parameter using req.query.search
.
4. Handling Multiple Routes
Express allows you to define multiple routes for different HTTP methods and URL paths. You can handle routes for the same path but different methods (GET, POST, PUT, DELETE), or define completely different paths to handle different requests.
For example, you can define GET and POST routes for the same URL:
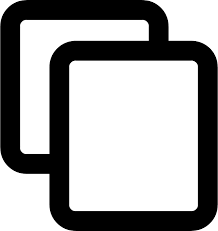
// Define a GET route for the /login path
app.get('/login', (req, res) => {
res.send('Login page');
});
// Define a POST route for the /login path
app.post('/login', (req, res) => {
res.send('Logging in...');
});
In this case, when a user sends a GET request to /login
, they will receive the response "Login page". When a POST request is made to the same path, the response will be "Logging in...".
5. Route Handlers with Multiple Callbacks
Express allows you to define multiple callback functions for a single route. These functions will be executed in the order they are defined. This is useful for implementing middleware or performing multiple actions on a single route.
Here is an example of using multiple route handlers:
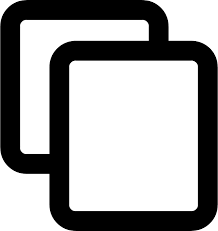
// Define a route with multiple callback functions
app.get('/profile', (req, res, next) => {
console.log('First callback - Logging request');
next(); // Pass control to the next callback
}, (req, res) => {
res.send('User Profile Page');
});
In this example, the first callback logs the request to the console, and the second callback sends the "User Profile Page" response to the client. The next()
function is used to pass control from one callback to the next.
6. Express Router
For larger applications, you may want to organize your routes into separate modules. Express provides a Router
object to help with this. The Router
allows you to group related routes together and use them in your main application.
Here’s how you can use the Express Router
:
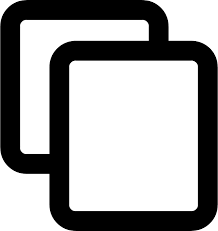
// Import express and create an app
const express = require('express');
const app = express();
// Create a router for user-related routes
const userRouter = express.Router();
// Define a route for getting user details
userRouter.get('/:userId', (req, res) => {
res.send(`User ID: ${req.params.userId}`);
});
// Mount the router on the /users path
app.use('/users', userRouter);
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, we create a new router for user-related routes and mount it on the /users
path. This allows us to group all user-related routes in one place, keeping the code organized and maintainable.
7. Conclusion
Routing in Express.js is powerful and flexible. You can easily define routes for different HTTP methods, handle dynamic URL parameters, and organize your routes into separate modules for larger applications. Express makes it simple to handle routing, allowing you to focus on building your application’s functionality.
Middleware in Express.js
Middleware in Express.js is a function that gets executed during the lifecycle of a request to the server. It sits in the request-response cycle and has access to the request object (req
), the response object (res
), and the next
function in the application’s request-response cycle. Middleware functions can modify the request and response objects, end the request-response cycle, or call the next middleware function in the stack.
1. What is Middleware?
Middleware functions are used to perform tasks such as logging requests, authenticating users, validating data, handling errors, and more. They are executed in the order they are defined in the application. Middleware can be applied globally (for all routes) or locally (for specific routes).
2. Types of Middleware
There are several types of middleware in Express.js:
- Application-level middleware: These middleware functions are bound to an instance of the Express application using
app.use()
orapp.METHOD()
(whereMETHOD
is a specific HTTP method likeget
,post
, etc.). - Router-level middleware: These are middleware functions that apply to a specific router.
- Error-handling middleware: These middleware functions handle errors that occur during request processing.
- Built-in middleware: Express comes with built-in middleware functions like
express.json()
,express.static()
, etc.
3. Basic Middleware Example
Here’s an example of a basic middleware function that logs the request method and URL for every incoming request:
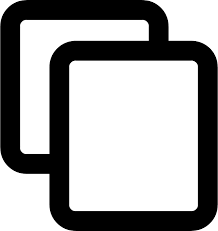
// Import Express
const express = require('express');
const app = express();
// Basic middleware function
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next(); // Pass control to the next middleware
});
// Define a simple route
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this example, the middleware logs the HTTP method (e.g., GET, POST) and the URL of every incoming request. The next()
function is called to pass control to the next middleware or route handler.
4. Application-Level Middleware
Application-level middleware is used for the entire application. It is defined using app.use()
, and it applies to all routes in the application unless specified otherwise.
For example, you can use middleware to serve static files or to parse incoming request bodies:
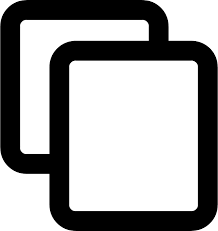
// Serve static files from the 'public' directory
app.use(express.static('public'));
// Parse incoming JSON request bodies
app.use(express.json());
The first line of code serves static files from the public
directory, and the second line parses incoming request bodies that are in JSON format.
5. Route-Level Middleware
Route-level middleware functions are middleware that apply only to specific routes. They are used when you need middleware for specific endpoints rather than globally for the entire application.
Here’s an example of using route-level middleware to log requests for a specific route:
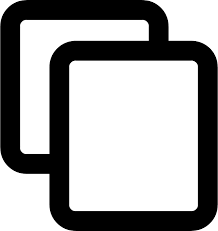
// Define route-level middleware for the '/user' path
app.get('/user', (req, res, next) => {
console.log('Request to /user');
next(); // Pass control to the next middleware
}, (req, res) => {
res.send('User Profile');
});
In this example, the middleware function logs a message whenever the /user
route is accessed. The second callback sends a response with the user profile page.
6. Error-Handling Middleware
Error-handling middleware functions are defined with four arguments: err
, req
, res
, and next
. These middleware functions can be used to catch and handle errors that occur during request processing.
Here’s an example of an error-handling middleware function that handles errors globally:
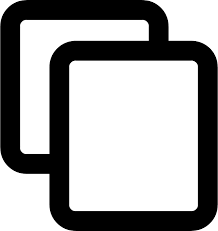
// Global error-handling middleware
app.use((err, req, res, next) => {
console.error(err.stack); // Log the error stack
res.status(500).send('Something went wrong!'); // Send error response
});
In this example, the error-handling middleware logs the error stack and sends a 500 status code response to the client with the message "Something went wrong!". This middleware will catch any errors that occur in the application.
7. Built-In Middleware in Express
Express provides several built-in middleware functions that make it easier to handle common tasks, such as parsing request bodies and serving static files. Some of the most commonly used built-in middleware include:
express.static()
: Serves static files (e.g., images, stylesheets, JavaScript files) from a specified directory.express.json()
: Parses incoming requests with JSON payloads.express.urlencoded()
: Parses incoming requests with URL-encoded payloads (e.g., form submissions).
8. Chaining Middleware Functions
You can chain multiple middleware functions together. They will execute in the order they are defined, one after the other, and each function can modify the request and response objects as needed.
Here’s an example of chaining multiple middleware functions:
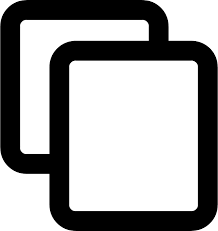
// Chaining middleware functions
app.use((req, res, next) => {
console.log('First middleware');
next(); // Pass control to the next middleware
});
app.use((req, res, next) => {
console.log('Second middleware');
next(); // Pass control to the next middleware
});
app.get('/', (req, res) => {
res.send('Chained Middleware Example');
});
In this example, the first middleware logs a message, then passes control to the second middleware, which also logs a message before sending the response.
9. Conclusion
Middleware in Express.js is a powerful concept that allows you to handle various tasks during the request-response cycle. By using middleware, you can easily add functionality like logging, authentication, error handling, and request parsing, making your Express applications more modular and maintainable.
Building RESTful APIs with Express.js
RESTful APIs (Representational State Transfer) are a popular architectural style used to build web services that are lightweight, maintainable, and scalable. Express.js, a web framework for Node.js, makes it easy to build RESTful APIs by providing simple methods for handling HTTP requests and routing.
1. What is a RESTful API?
A RESTful API is an interface that allows communication between systems using HTTP methods (GET, POST, PUT, DELETE) to access and manipulate resources. These resources are typically represented in formats like JSON or XML.
RESTful APIs follow a set of principles that include stateless communication, resource-based URLs, and the use of standard HTTP methods for CRUD (Create, Read, Update, Delete) operations:
- GET: Retrieve data from the server.
- POST: Send data to the server to create a new resource.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
2. Setting Up Express.js for Building APIs
To build a RESTful API with Express.js, start by setting up an Express application. Here are the basic steps:
npm init
to create a new Node.js project.npm install express
to install the Express.js framework.- Set up the basic structure of the API, including routes to handle the different HTTP methods.
3. Creating a Simple RESTful API
Let’s create a simple RESTful API for managing a list of users. We'll implement the four main CRUD operations: GET, POST, PUT, and DELETE.
Here’s how the basic setup might look:
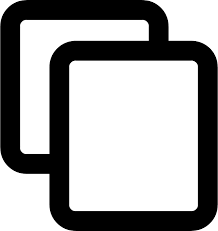
// Import Express and initialize the app
const express = require('express');
const app = express();
app.use(express.json()); // Middleware to parse JSON requests
// In-memory data storage for users
let users = [
{ id: 1, name: 'John Doe', email: 'johndoe@example.com' },
{ id: 2, name: 'Jane Doe', email: 'janedoe@example.com' }
];
// GET: Retrieve all users
app.get('/users', (req, res) => {
res.status(200).json(users);
});
// GET: Retrieve a single user by ID
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.status(200).json(user);
});
// POST: Create a new user
app.post('/users', (req, res) => {
const user = {
id: users.length + 1,
name: req.body.name,
email: req.body.email
};
users.push(user);
res.status(201).json(user);
});
// PUT: Update an existing user
app.put('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
user.name = req.body.name;
user.email = req.body.email;
res.status(200).json(user);
});
// DELETE: Remove a user
app.delete('/users/:id', (req, res) => {
const userIndex = users.findIndex(u => u.id === parseInt(req.params.id));
if (userIndex === -1) return res.status(404).send('User not found');
const deletedUser = users.splice(userIndex, 1);
res.status(200).json(deletedUser);
});
// Start the server
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
In this example:
- GET /users retrieves a list of all users.
- GET /users/:id retrieves a single user based on their ID.
- POST /users creates a new user by accepting data in the request body.
- PUT /users/:id updates an existing user based on their ID and the data provided in the request body.
- DELETE /users/:id deletes a user based on their ID.
4. Using HTTP Status Codes
In RESTful APIs, it is important to use appropriate HTTP status codes in the response to indicate the result of the operation:
- 200 OK: The request was successful (GET, PUT, DELETE).
- 201 Created: The resource was successfully created (POST).
- 204 No Content: The request was successful, but there is no content to return (DELETE).
- 404 Not Found: The resource was not found.
- 400 Bad Request: The request was malformed or missing required parameters.
- 500 Internal Server Error: The server encountered an error while processing the request.
5. Organizing Routes with Express Router
As your API grows, you may want to organize routes into separate files for better structure. You can use the Router
feature in Express to break up your routes into smaller modules. For example:
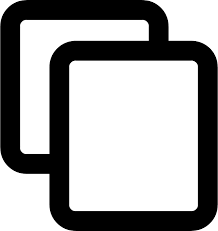
// users.js (routes/users.js)
const express = require('express');
const router = express.Router();
let users = [
{ id: 1, name: 'John Doe', email: 'johndoe@example.com' },
{ id: 2, name: 'Jane Doe', email: 'janedoe@example.com' }
];
// Routes for users
router.get('/', (req, res) => {
res.status(200).json(users);
});
router.get('/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.status(200).json(user);
});
router.post('/', (req, res) => {
const user = {
id: users.length + 1,
name: req.body.name,
email: req.body.email
};
users.push(user);
res.status(201).json(user);
});
module.exports = router;
// server.js (main server file)
const express = require('express');
const app = express();
const usersRouter = require('./routes/users');
app.use(express.json());
app.use('/users', usersRouter); // Use the users routes
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Here, the routes for user-related operations are moved to a separate file routes/users.js
. In the main server file, we import and use them with app.use('/users', usersRouter);
to make the routes available at the /users
endpoint.
6. Conclusion
Building RESTful APIs with Express.js is simple and efficient. By defining routes and using HTTP methods, you can quickly create a scalable and maintainable API. Express.js provides easy-to-use tools for handling requests, parsing data, and organizing routes, making it an ideal choice for building APIs.
Connecting to MongoDB with Mongoose
MongoDB is a popular NoSQL database that stores data in a flexible, JSON-like format, making it an excellent choice for applications that require scalability and high performance. Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js, which provides a straightforward way to interact with MongoDB databases using schemas, models, and built-in validation features.
1. What is Mongoose?
Mongoose is a powerful library that allows you to define models for MongoDB documents with a schema, providing an abstraction layer over MongoDB’s native operations. It simplifies tasks such as validation, type casting, query building, and business logic hooks.
2. Installing Mongoose
To begin using Mongoose, you first need to install it in your Node.js project. Run the following command to install Mongoose via npm:
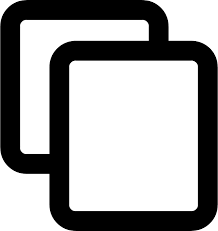
npm install mongoose
3. Connecting to MongoDB
Once Mongoose is installed, you can connect to your MongoDB database. The connection string will depend on whether you're using a local MongoDB server or a cloud-based service like MongoDB Atlas.
Here’s how you can connect to MongoDB using Mongoose:
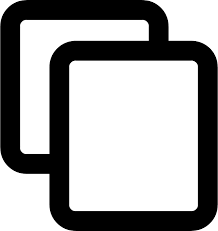
const mongoose = require('mongoose');
// Replace with your MongoDB connection string
const dbURI = 'mongodb://localhost:27017/mydatabase';
mongoose.connect(dbURI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => {
console.log('Connected to MongoDB');
})
.catch((err) => {
console.log('Error connecting to MongoDB:', err);
});
In this example, we are using mongoose.connect()
to establish a connection to the MongoDB instance. The useNewUrlParser
and useUnifiedTopology
options are required to avoid deprecation warnings.
4. Creating a Mongoose Schema and Model
Once connected to the database, you can define a schema to structure the documents in your MongoDB collection. A schema defines the shape and validation rules for your data.
Here’s how to create a schema and model in Mongoose:
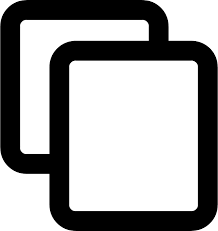
// Define a schema for a User
const userSchema = new mongoose.Schema({
name: {
type: String,
required: true
},
email: {
type: String,
required: true,
unique: true
},
age: {
type: Number,
min: 18,
max: 100
}
});
// Create a model based on the schema
const User = mongoose.model('User', userSchema);
// Example of creating a new user
const newUser = new User({
name: 'John Doe',
email: 'johndoe@example.com',
age: 30
});
newUser.save()
.then((user) => {
console.log('User saved:', user);
})
.catch((err) => {
console.log('Error saving user:', err);
});
In this example:
- We defined a
userSchema
to represent the structure of a user document, including fields for name, email, and age. - We created a model called
User
based on the schema. - We created a new user instance and saved it to the database using the
save()
method.
5. Querying Data from MongoDB
Once you have a model, you can query MongoDB to retrieve, update, and delete data. Mongoose provides several methods for querying data such as find()
, findOne()
, findById()
, and more.
Here’s an example of querying all users from the database:
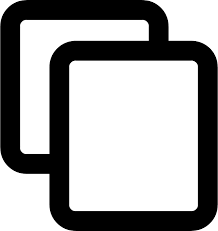
User.find()
.then((users) => {
console.log('All users:', users);
})
.catch((err) => {
console.log('Error fetching users:', err);
});
In this example, we use User.find()
to retrieve all user documents from the database.
6. Updating Documents in MongoDB
To update a document, you can use methods such as findByIdAndUpdate()
or updateOne()
.
Here’s an example of updating a user’s age:
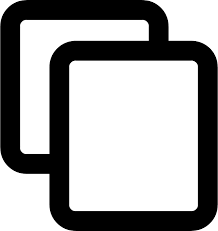
User.findByIdAndUpdate('user-id', { age: 35 }, { new: true })
.then((updatedUser) => {
console.log('Updated user:', updatedUser);
})
.catch((err) => {
console.log('Error updating user:', err);
});
In this example, we are updating a user’s age based on their _id
and returning the updated document with the new: true
option.
7. Deleting Documents in MongoDB
To delete documents, you can use methods like findByIdAndDelete()
or deleteOne()
.
Here’s an example of deleting a user by their ID:
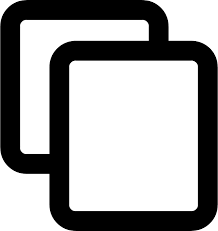
User.findByIdAndDelete('user-id')
.then(() => {
console.log('User deleted');
})
.catch((err) => {
console.log('Error deleting user:', err);
});
8. Conclusion
Connecting to MongoDB using Mongoose simplifies interacting with a MongoDB database by providing a structured approach to define schemas and models. Mongoose also offers powerful querying capabilities, validation, and middleware support, making it a great choice for building Node.js applications that need to store and retrieve data from MongoDB.
CRUD Operations with MongoDB
CRUD stands for Create, Read, Update, and Delete, which are the four basic operations of persistent storage. In MongoDB, these operations can be performed using the MongoDB shell or through a Node.js application with Mongoose. In this section, we’ll walk through performing each of these CRUD operations using Mongoose, an ODM (Object Data Modeling) library for MongoDB.
1. Create Operation
The Create operation allows you to add new documents to a MongoDB collection. In Mongoose, you can create a new instance of a model and save it to the database using the save()
method.
Here’s an example of how to create a new document in a MongoDB collection:
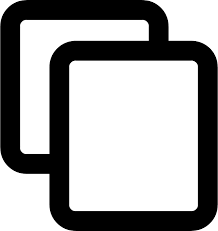
const mongoose = require('mongoose');
// Define a schema
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
// Create a model from the schema
const User = mongoose.model('User', userSchema);
// Create a new user document
const newUser = new User({
name: 'Jane Doe',
email: 'jane.doe@example.com',
age: 25
});
// Save the new user to the database
newUser.save()
.then((user) => {
console.log('New user created:', user);
})
.catch((err) => {
console.log('Error creating user:', err);
});
In this example, we define a userSchema
and create a new user document, which is then saved to the MongoDB database using the save()
method.
2. Read Operation
The Read operation allows you to retrieve data from the MongoDB database. Mongoose provides several methods for querying data, such as find()
, findOne()
, and findById()
.
Here’s an example of how to find all users in the database:
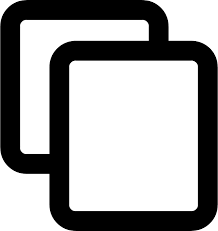
User.find() // Find all users
.then((users) => {
console.log('All users:', users);
})
.catch((err) => {
console.log('Error fetching users:', err);
});
You can also filter results by providing conditions inside the find()
method. For example, to find users older than 30:
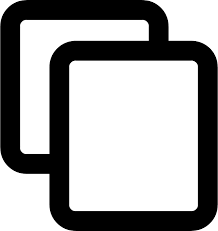
User.find({ age: { $gt: 30 } })
.then((users) => {
console.log('Users older than 30:', users);
})
.catch((err) => {
console.log('Error fetching users:', err);
});
3. Update Operation
The Update operation allows you to modify existing documents in the database. Mongoose provides several methods for updating documents, such as findByIdAndUpdate()
, updateOne()
, and updateMany()
.
Here’s an example of how to update a user’s age by their ID:
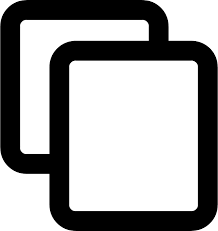
User.findByIdAndUpdate('user-id', { age: 28 }, { new: true })
.then((updatedUser) => {
console.log('Updated user:', updatedUser);
})
.catch((err) => {
console.log('Error updating user:', err);
});
In this example, the new: true
option ensures that the updated document is returned, rather than the original one.
4. Delete Operation
The Delete operation allows you to remove documents from the MongoDB database. Mongoose provides methods like findByIdAndDelete()
and deleteOne()
to delete documents.
Here’s an example of how to delete a user by their ID:
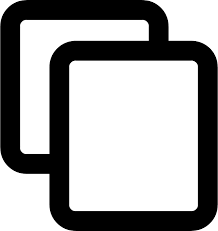
User.findByIdAndDelete('user-id')
.then(() => {
console.log('User deleted');
})
.catch((err) => {
console.log('Error deleting user:', err);
});
In this example, we use findByIdAndDelete()
to remove a user from the database by their _id
.
5. Conclusion
CRUD operations are the foundation of interacting with a database, and Mongoose simplifies these operations for MongoDB. Whether you're creating, reading, updating, or deleting documents, Mongoose provides powerful and easy-to-use methods to handle your database interactions efficiently. By understanding these basic CRUD operations, you can start building powerful and dynamic applications that interact with MongoDB.
Using MySQL with Node.js
MySQL is one of the most popular relational database management systems, and integrating it with Node.js allows you to interact with your database using SQL queries. In this section, we will explore how to connect MySQL with Node.js, perform basic CRUD operations, and handle connections effectively.
1. Setting Up MySQL with Node.js
To use MySQL in your Node.js application, you need to install the MySQL package. The most commonly used package is mysql2>, which provides both a promise-based API and a callback-based API for interacting with MySQL.
To install the MySQL package, run the following command in your project directory:
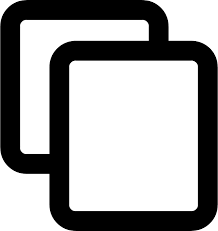
npm install mysql2
Once the package is installed, you can set up the MySQL connection in your Node.js application.
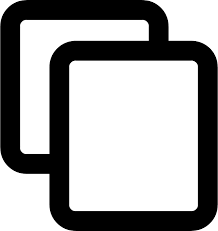
const mysql = require('mysql2');
// Create a connection to the database
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'my_database'
});
// Connect to the MySQL server
connection.connect((err) => {
if (err) {
console.error('Error connecting to the database:', err.stack);
return;
}
console.log('Connected to the MySQL database');
});
In this example, we create a connection to the MySQL database using the mysql.createConnection()
method, specifying the host, user, password, and database name. The connection.connect()
method is used to establish the connection to the MySQL server.
2. Performing CRUD Operations
2.1. Create Operation
To insert data into a MySQL table, you can use the INSERT INTO
SQL statement. Here's how you can insert a new record into a table using Node.js and MySQL:
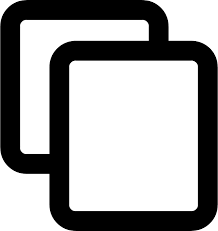
const query = 'INSERT INTO users (name, email) VALUES (?, ?)';
const values = ['John Doe', 'john.doe@example.com'];
connection.query(query, values, (err, results) => {
if (err) {
console.log('Error inserting data:', err);
return;
}
console.log('Inserted row ID:', results.insertId);
});
In this example, we use placeholders (?, ?)
in the query to safely insert the name
and email
values into the users
table. The query
method executes the query, and the results
object contains information about the insertion, such as the inserted row ID.
2.2. Read Operation
To retrieve data from a MySQL table, you can use the SELECT
SQL statement. Here's an example of how to query all users from the database:
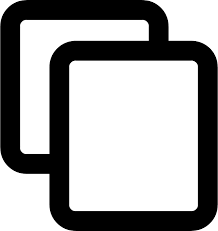
connection.query('SELECT * FROM users', (err, results) => {
if (err) {
console.log('Error fetching data:', err);
return;
}
console.log('All users:', results);
});
The query retrieves all rows from the users
table, and the results are returned in the results
array. You can process this array to display or manipulate the data.
2.3. Update Operation
To update existing data in a MySQL table, you can use the UPDATE
SQL statement. Here's an example of how to update a user's email address:
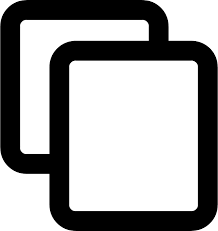
const updateQuery = 'UPDATE users SET email = ? WHERE id = ?';
const updateValues = ['new.email@example.com', 1];
connection.query(updateQuery, updateValues, (err, results) => {
if (err) {
console.log('Error updating data:', err);
return;
}
console.log('Rows affected:', results.affectedRows);
});
In this example, we update the email of the user with an ID of 1 in the users
table. The affectedRows
property in the results
object indicates how many rows were updated.
2.4. Delete Operation
To delete a record from a MySQL table, you can use the DELETE
SQL statement. Here's how to delete a user by their ID:
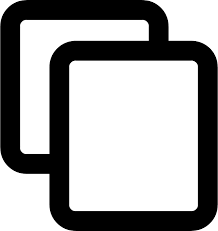
const deleteQuery = 'DELETE FROM users WHERE id = ?';
const deleteValues = [1];
connection.query(deleteQuery, deleteValues, (err, results) => {
if (err) {
console.log('Error deleting data:', err);
return;
}
console.log('Rows deleted:', results.affectedRows);
});
In this example, we delete the user with an ID of 1 from the users
table. The affectedRows
property indicates how many rows were deleted.
3. Closing the Connection
Once you are done working with the MySQL database, it is important to close the connection to free up resources. You can close the connection using the connection.end()
method:
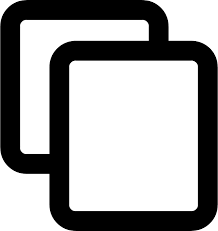
connection.end((err) => {
if (err) {
console.error('Error closing connection:', err.stack);
return;
}
console.log('Connection closed');
});
4. Conclusion
By following the steps outlined above, you can easily integrate MySQL with Node.js and perform basic CRUD operations. The mysql2
package provides a simple and efficient way to interact with MySQL databases in your Node.js applications, and it offers both callback and promise-based APIs to cater to different development styles.
PostgreSQL and Sequelize ORM
PostgreSQL is a powerful, open-source relational database management system. Sequelize is an Object Relational Mapping (ORM) library for Node.js that supports multiple databases, including PostgreSQL. Sequelize makes it easier to interact with PostgreSQL by allowing developers to use JavaScript objects instead of raw SQL queries. In this section, we will explore how to set up PostgreSQL with Sequelize and perform common database operations.
1. Setting Up PostgreSQL with Sequelize
To use PostgreSQL with Sequelize, you first need to install both PostgreSQL and the Sequelize library. Sequelize also requires a PostgreSQL driver, which can be installed via npm.
Start by installing the required packages:
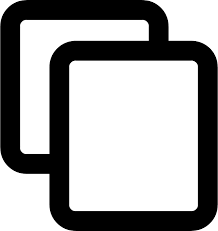
npm install sequelize pg pg-hstore
Here, pg
is the PostgreSQL client for Node.js, and pg-hstore
is a module for handling the storage of JSON data in PostgreSQL.
Once the packages are installed, you can set up the Sequelize instance and connect to your PostgreSQL database:
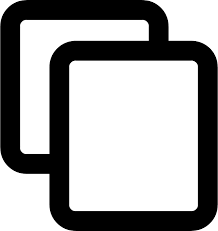
const { Sequelize } = require('sequelize');
// Create a new Sequelize instance and connect to the PostgreSQL database
const sequelize = new Sequelize('postgres://user:password@localhost:5432/mydatabase', {
dialect: 'postgres',
logging: false, // Disable logging for production
});
// Test the connection
sequelize.authenticate()
.then(() => {
console.log('Connection established successfully.');
})
.catch(err => {
console.error('Unable to connect to the database:', err);
});
In this example, we use the connection string format postgres://user:password@localhost:5432/mydatabase
to connect to the database. The sequelize.authenticate()
method checks if the connection is successful.
2. Defining Models with Sequelize
In Sequelize, models represent the tables in your database. You can define models as JavaScript classes, and Sequelize will map them to the corresponding database tables.
Here is an example of how to define a User
model:
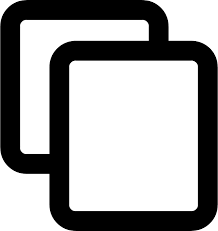
const { DataTypes } = require('sequelize');
// Define the User model
const User = sequelize.define('User', {
name: {
type: DataTypes.STRING,
allowNull: false,
},
email: {
type: DataTypes.STRING,
unique: true,
allowNull: false,
},
}, {
tableName: 'users', // Specify the table name (optional)
timestamps: true, // Enable timestamps (createdAt, updatedAt)
});
// Sync the model with the database
User.sync()
.then(() => {
console.log('User model synchronized with the database');
})
.catch(err => {
console.error('Error syncing model:', err);
});
In this example, we define a User
model with two fields: name
and email
. The model is then synchronized with the database using the sync()
method, which creates the corresponding table if it does not already exist.
3. Performing CRUD Operations with Sequelize
3.1. Create Operation
To create a new user in the database, you can use the create()
method:
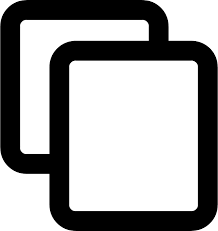
User.create({
name: 'John Doe',
email: 'john.doe@example.com',
})
.then(user => {
console.log('User created:', user);
})
.catch(err => {
console.error('Error creating user:', err);
});
The create()
method inserts a new record into the users
table using the data provided in the method arguments.
3.2. Read Operation
To retrieve users from the database, you can use methods like findAll()
and findOne()
. Here's how to fetch all users:
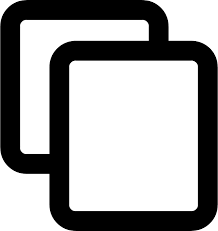
User.findAll()
.then(users => {
console.log('All users:', users);
})
.catch(err => {
console.error('Error fetching users:', err);
});
The findAll()
method retrieves all users from the users
table and returns them as an array of user objects.
3.3. Update Operation
To update an existing user, you can use the update()
method:
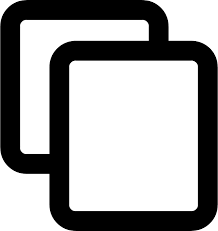
User.update(
{ email: 'new.email@example.com' }, // Values to update
{ where: { id: 1 } } // Condition for the update
)
.then(() => {
console.log('User updated');
})
.catch(err => {
console.error('Error updating user:', err);
});
The update()
method takes two arguments: an object representing the fields to update, and a condition to specify which records to update.
3.4. Delete Operation
To delete a user from the database, you can use the destroy()
method:
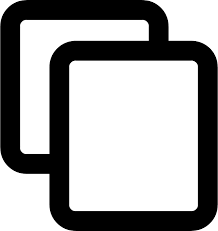
User.destroy({
where: { id: 1 }
})
.then(() => {
console.log('User deleted');
})
.catch(err => {
console.error('Error deleting user:', err);
});
The destroy()
method deletes records that match the specified condition (in this case, users with id = 1
).
4. Closing the Connection
Once you're done with database operations, it's a good practice to close the connection to the PostgreSQL database:
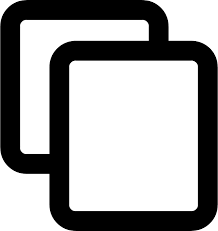
sequelize.close()
.then(() => {
console.log('Connection closed');
})
.catch(err => {
console.error('Error closing connection:', err);
});
5. Conclusion
Sequelize provides a powerful and easy-to-use ORM for interacting with PostgreSQL in Node.js applications. It allows you to perform CRUD operations with minimal code and eliminates the need to write raw SQL queries. By defining models and using Sequelize methods, you can efficiently interact with your PostgreSQL database in a more structured and object-oriented way.
Redis for Caching and Session Management
Redis is a powerful, in-memory data store that can be used as a cache, message broker, and more. It offers fast data retrieval and is ideal for caching frequently accessed data and managing sessions in web applications. In this section, we will explore how to use Redis for caching and session management in a Node.js environment.
1. Setting Up Redis with Node.js
To use Redis with Node.js, you need to install the Redis server and the ioredis
or redis
package. ioredis
is a popular Redis client for Node.js that supports cluster mode and automatic reconnection.
Start by installing the Redis server and the Redis client for Node.js:
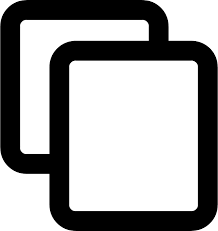
npm install ioredis
Next, ensure that Redis is running on your local machine or on a Redis server by following the installation instructions on the Redis website.
Once Redis is installed, you can connect to it using the ioredis
client:
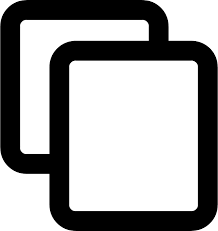
const Redis = require('ioredis');
// Create a Redis client and connect to the Redis server
const redis = new Redis({
host: 'localhost',
port: 6379, // Default Redis port
password: 'your_password', // Optional, if using a password
});
// Test the connection
redis.ping()
.then(response => {
console.log('Redis connection successful:', response);
})
.catch(err => {
console.error('Error connecting to Redis:', err);
});
In this example, we create a Redis client by providing the host, port, and an optional password. The ping()
method is used to check if the connection is successful.
2. Caching Data with Redis
Redis can be used to store frequently accessed data in memory, allowing for faster retrieval. To cache data in Redis, use the set()
method to store data and the get()
method to retrieve it.
Here’s an example of how to cache data in Redis:
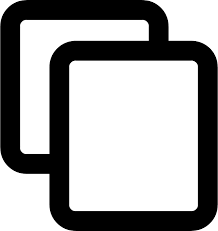
// Caching data in Redis
redis.set('user:1000', JSON.stringify({ name: 'John Doe', age: 30 }))
.then(() => {
console.log('User data cached');
})
.catch(err => {
console.error('Error caching data:', err);
});
// Retrieving cached data from Redis
redis.get('user:1000')
.then(data => {
if (data) {
console.log('Cached data:', JSON.parse(data));
} else {
console.log('Cache miss');
}
})
.catch(err => {
console.error('Error retrieving data:', err);
});
In this example, we cache the user data as a JSON string under the key user:1000
using the set()
method. To retrieve the cached data, we use the get()
method. If the data is found, it is parsed from the JSON string and logged to the console.
3. Expiring Cached Data
In Redis, you can set an expiration time for cached data, so it automatically expires after a certain period. This is useful for caching data that is likely to change over time, such as API responses or user session data.
To set an expiration time for a cached key, you can use the setex()
method, which takes the key, expiration time in seconds, and the value:
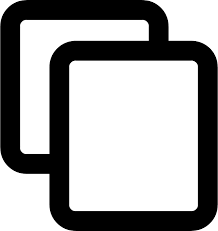
// Caching data with expiration time (10 seconds)
redis.setex('user:1000', 10, JSON.stringify({ name: 'John Doe', age: 30 }))
.then(() => {
console.log('User data cached with expiration');
})
.catch(err => {
console.error('Error caching data with expiration:', err);
});
In this example, the cached data for user:1000
will expire after 10 seconds. After the expiration, attempting to retrieve the key will result in a cache miss.
4. Session Management with Redis
Redis is commonly used for managing user sessions in web applications. By storing session data in Redis, you can easily scale your application while ensuring that session data is persistent and accessible across multiple servers.
Here’s an example of how to use Redis for session management in a Node.js app with the express-session
package:
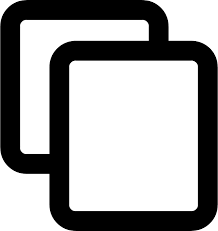
const express = require('express');
const session = require('express-session');
const RedisStore = require('connect-redis')(session);
const redis = new Redis();
// Create an Express app
const app = express();
// Set up session middleware with Redis
app.use(session({
store: new RedisStore({ client: redis }),
secret: 'your_session_secret',
resave: false,
saveUninitialized: false,
cookie: { secure: false, maxAge: 60000 }, // Session expiration time
}));
// Route to set a session value
app.get('/set-session', (req, res) => {
req.session.user = { name: 'John Doe', age: 30 };
res.send('Session data set');
});
// Route to get session data
app.get('/get-session', (req, res) => {
if (req.session.user) {
res.json(req.session.user);
} else {
res.send('No session data available');
}
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, we use the express-session
middleware to set up Redis as the session store. The connect-redis
package allows Redis to store session data. We create two routes: one to set the session data and another to retrieve it.
5. Conclusion
Redis is a versatile tool for improving the performance of your application by caching frequently accessed data and managing user sessions. Its speed and efficiency make it ideal for reducing database load, improving response times, and maintaining session persistence in a distributed environment. By integrating Redis with Node.js, you can significantly optimize your application's performance and scalability.
Handling Errors in Node.js Applications
Error handling is a crucial aspect of building robust and reliable Node.js applications. Proper error management ensures that your application can gracefully recover from unexpected situations and provide meaningful feedback to users. This section covers various techniques for handling errors in Node.js.
1. Types of Errors in Node.js
Errors in Node.js can generally be categorized into the following types:
- Operational Errors: These are predictable errors that occur during runtime, such as file not found, invalid input, or database connection failures.
- Programming Errors: These are bugs in your code, such as syntax errors, reference errors, or type errors.
- System Errors: These occur due to system-level issues, such as memory exhaustion or unavailable resources.
2. Using try...catch for Synchronous Code
The try...catch
statement is used to handle errors in synchronous code. Any exceptions thrown within the try
block will be caught in the catch
block:
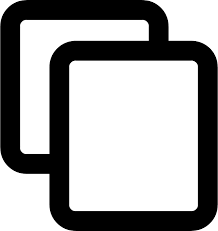
try {
const data = JSON.parse('{"invalidJson}');
} catch (err) {
console.error('Error parsing JSON:', err.message);
}
In this example, the invalid JSON string causes an exception, which is caught and handled in the catch
block.
3. Handling Errors in Asynchronous Code
Asynchronous code in Node.js, such as callbacks and promises, requires special attention for error handling. Here are some common approaches:
Using Callbacks
In callback-based functions, errors are typically passed as the first argument of the callback:
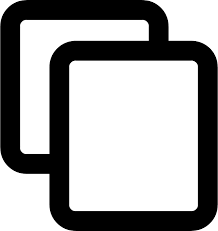
const fs = require('fs');
fs.readFile('nonexistent.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err.message);
return;
}
console.log('File content:', data);
});
In this example, the error is passed to the callback function, where it is handled appropriately.
Using Promises
In promise-based code, you can use .catch()
to handle errors:
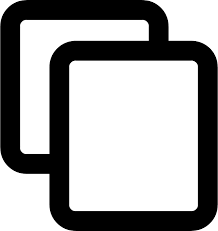
const fs = require('fs').promises;
fs.readFile('nonexistent.txt', 'utf8')
.then(data => {
console.log('File content:', data);
})
.catch(err => {
console.error('Error reading file:', err.message);
});
Using async/await
For async/await
syntax, use try...catch
for error handling:
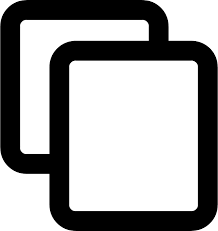
const fs = require('fs').promises;
async function readFile() {
try {
const data = await fs.readFile('nonexistent.txt', 'utf8');
console.log('File content:', data);
} catch (err) {
console.error('Error reading file:', err.message);
}
}
readFile();
4. Centralized Error Handling
Centralized error handling in an application improves maintainability and ensures consistent error responses. In an Express.js application, you can use middleware for centralized error handling:
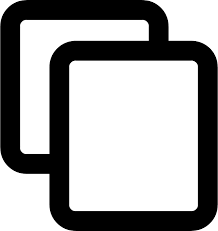
const express = require('express');
const app = express();
// Route handler
app.get('/', (req, res) => {
throw new Error('Something went wrong!');
});
// Error-handling middleware
app.use((err, req, res, next) => {
console.error('Error:', err.message);
res.status(500).json({ error: err.message });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, any error thrown in the route handler is caught by the error-handling middleware, which sends a consistent error response to the client.
5. Best Practices for Error Handling
- Always validate user input to prevent invalid data from causing errors.
- Use proper HTTP status codes in error responses, such as 400 for bad requests or 500 for server errors.
- Log errors using a logging library like
winston
orpino
to monitor and debug issues in production. - Avoid leaking sensitive information in error messages. Use generic messages for users and detailed logs for developers.
- Handle unhandled promise rejections and uncaught exceptions using process event handlers:
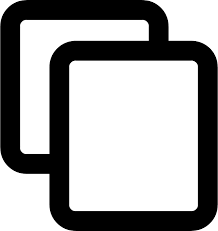
// Handle unhandled promise rejections
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection:', reason);
});
// Handle uncaught exceptions
process.on('uncaughtException', err => {
console.error('Uncaught Exception:', err.message);
process.exit(1); // Exit the process
});
6. Conclusion
By implementing proper error-handling techniques, you can make your Node.js applications more robust and user-friendly. Whether you’re working with synchronous code, asynchronous promises, or complex web applications, following best practices for error handling ensures better application stability and maintainability.
Using the try-catch and async/await Patterns
The try-catch
block combined with async/await
is a powerful pattern for handling errors in asynchronous code in Node.js. It allows developers to write asynchronous code in a synchronous style while effectively managing errors.
1. Introduction to try-catch
The try
block contains the code that might throw an error, while the catch
block handles the error if it occurs. This pattern ensures that your application can recover gracefully from exceptions.
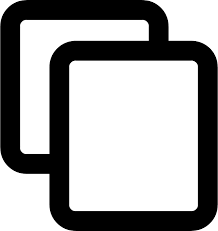
try {
// Code that may throw an error
const result = JSON.parse('{"invalidJson}');
} catch (err) {
console.error('Error:', err.message);
}
In this example, the invalid JSON string inside the try
block throws an error, which is caught and logged in the catch
block.
2. Using async/await with try-catch
When working with asynchronous functions, async/await
allows you to write cleaner and more readable code compared to traditional callbacks or promises. By wrapping await
expressions in a try-catch
block, you can handle errors effectively.
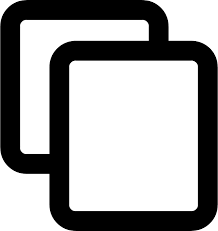
const fs = require('fs').promises;
async function readFile(filePath) {
try {
const data = await fs.readFile(filePath, 'utf8');
console.log('File content:', data);
} catch (err) {
console.error('Error reading file:', err.message);
}
}
readFile('example.txt');
Here, the readFile
function reads a file asynchronously. If an error occurs, such as the file not being found, it is caught and logged in the catch
block.
3. Handling Multiple Async Operations
When dealing with multiple asynchronous operations, you can use nested or sequential try-catch
blocks:
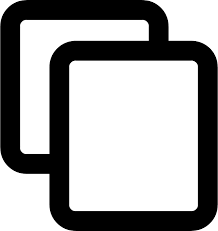
const fs = require('fs').promises;
async function processFiles() {
try {
const file1 = await fs.readFile('file1.txt', 'utf8');
console.log('File 1 content:', file1);
try {
const file2 = await fs.readFile('file2.txt', 'utf8');
console.log('File 2 content:', file2);
} catch (err) {
console.error('Error reading file 2:', err.message);
}
} catch (err) {
console.error('Error reading file 1:', err.message);
}
}
processFiles();
In this example, separate try-catch
blocks are used to handle errors for each file independently.
4. Combining async/await with Centralized Error Handling
You can centralize error handling by wrapping your asynchronous routes or functions in a higher-order function:
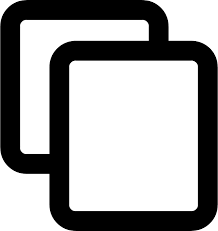
const express = require('express');
const fs = require('fs').promises;
const app = express();
// Higher-order function for error handling
const asyncHandler = fn => (req, res, next) => {
Promise.resolve(fn(req, res, next)).catch(next);
};
// Route with centralized error handling
app.get('/file', asyncHandler(async (req, res) => {
const data = await fs.readFile('example.txt', 'utf8');
res.send(data);
}));
// Error-handling middleware
app.use((err, req, res, next) => {
console.error('Error:', err.message);
res.status(500).json({ error: 'Internal Server Error' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Here, the asyncHandler
function wraps each route to catch errors and forward them to the centralized error-handling middleware.
5. Best Practices
- Always use
try-catch
to handle errors in asynchronous functions. - Centralize error handling where possible to reduce repetitive code.
- Log errors for monitoring and debugging purposes using tools like
winston
orpino
. - Validate user input to prevent predictable runtime errors.
- Handle unhandled promise rejections globally using
process.on
:
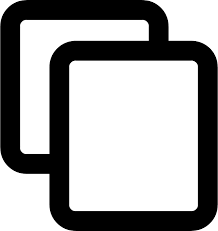
// Handle unhandled promise rejections
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection at:', promise, 'reason:', reason);
});
6. Conclusion
The try-catch
pattern combined with async/await
offers a clean and effective way to handle errors in asynchronous Node.js applications. By following best practices, you can ensure your application remains robust and user-friendly even in the face of unexpected errors.
Debugging Node.js Applications with console
and node inspect
Debugging is an essential part of application development. Node.js provides several tools to help developers identify and fix bugs effectively. Two commonly used methods are console
debugging and the node inspect
debugger.
1. Debugging with console
The console
object in Node.js is a simple yet powerful way to debug code. Using methods like console.log
, console.error
, and console.table
, you can print data to the console for inspection.
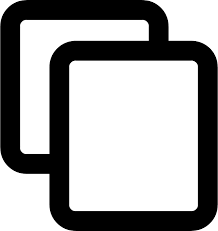
const fs = require('fs');
function readFile(filePath) {
console.log('Reading file:', filePath); // Log the file path
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err); // Log the error
return;
}
console.log('File content:', data); // Log the file content
});
}
readFile('example.txt');
In this example, console.log
and console.error
are used to log file operations and errors.
Additional console
Methods
console.warn(message)
- Prints a warning message.console.table(data)
- Displays tabular data in a readable format.console.time(label)
andconsole.timeEnd(label)
- Measures the time taken by a block of code.
2. Debugging with node inspect
The node inspect
command provides an interactive debugger for Node.js. It allows you to set breakpoints, inspect variables, and step through code execution.
Step-by-Step Guide
- Run your script with the
inspect
flag: - Start debugging: You will enter the debugger prompt (
debug>
). - Use Debugger Commands:
n
- Step to the next line.c
- Continue execution until the next breakpoint.repl
- Open a Read-Eval-Print Loop to inspect variables.watch(expression)
- Watch specific expressions or variables.
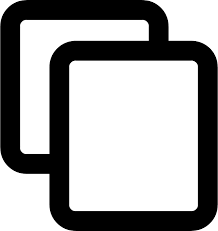
node inspect app.js
Example
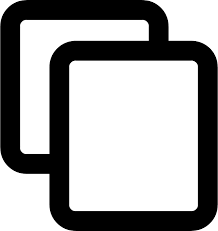
// app.js
const fs = require('fs');
function readFile(filePath) {
debugger; // Set a breakpoint
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error('Error:', err);
return;
}
console.log('Content:', data);
});
}
readFile('example.txt');
In this example, the debugger
statement sets a breakpoint. Run the script with node inspect app.js
to start debugging.
Using Chrome DevTools
- Run your script with the
--inspect
flag: - Open Chrome and navigate to
chrome://inspect
. - Click "Open dedicated DevTools for Node" to start debugging.
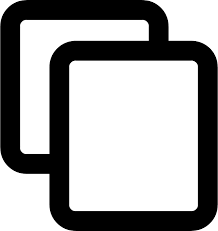
node --inspect app.js
3. Best Practices
- Use
console.log
sparingly to avoid cluttering the output. - Prefer structured logging tools like
winston
orpino
for production applications. - Set appropriate breakpoints in critical code sections for efficient debugging.
- Monitor unhandled errors with
process.on('uncaughtException')
orprocess.on('unhandledRejection')
.
4. Conclusion
Debugging Node.js applications is seamless with tools like console
for simple debugging and node inspect
for more advanced scenarios. Integrate these methods into your workflow to quickly identify and resolve issues in your code.
Implementing JWT Authentication
JSON Web Tokens (JWT) are a secure way to handle authentication and information exchange between clients and servers. JWTs are compact, URL-safe, and digitally signed to ensure data integrity.
1. What is JWT?
A JWT is a token that contains three parts:
- Header: Contains metadata about the token, such as the signing algorithm.
- Payload: Contains the claims (data) being transmitted.
- Signature: Ensures the token's integrity and authenticity.
A typical JWT looks like this:
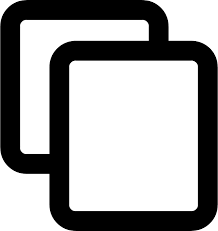
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VySWQiOjEsImlhdCI6MTYyMzUwMzIwMH0.4pA9TJSJ9aJ3L0a9M4U8Rgv9XH8hfO3XzJ9Q6jJ1WZs
2. Installing Required Packages
To implement JWT in Node.js, you need the following packages:
jsonwebtoken:
For creating and verifying tokens.bcrypt:
For hashing passwords.express:
For handling HTTP requests.
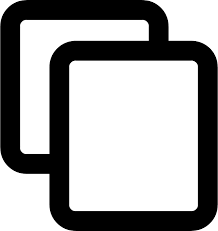
npm install jsonwebtoken bcrypt express
3. Setting Up JWT Authentication
Below is an example implementation of JWT authentication in a Node.js application.
Step 1: User Registration
Hash the user's password and store it securely in the database.
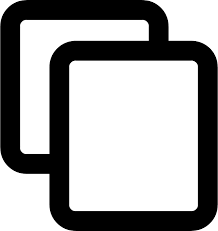
const bcrypt = require('bcrypt');
const users = []; // Temporary user storage
function registerUser(username, password) {
const hashedPassword = bcrypt.hashSync(password, 10); // Hash the password
users.push({ username, password: hashedPassword });
console.log('User registered:', username);
}
registerUser('testuser', 'password123');
Step 2: User Login and Token Generation
Validate user credentials and generate a JWT for authenticated users.
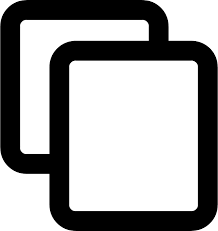
const jwt = require('jsonwebtoken');
function loginUser(username, password) {
const user = users.find(u => u.username === username);
if (!user || !bcrypt.compareSync(password, user.password)) {
return console.error('Invalid credentials');
}
const token = jwt.sign({ username: user.username }, 'secretKey', { expiresIn: '1h' });
console.log('Token:', token);
}
loginUser('testuser', 'password123');
Step 3: Protect Routes with Middleware
Use middleware to verify the JWT for protected routes.
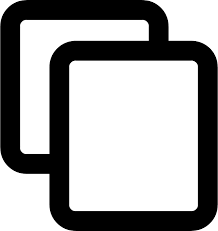
const express = require('express');
const app = express();
function authenticateToken(req, res, next) {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.status(401).send('Access denied');
jwt.verify(token, 'secretKey', (err, user) => {
if (err) return res.status(403).send('Invalid token');
req.user = user;
next();
});
}
app.get('/protected', authenticateToken, (req, res) => {
res.send('This is a protected route');
});
app.listen(3000, () => console.log('Server running on port 3000'));
4. Best Practices
- Use strong, random secret keys and store them securely (e.g., environment variables).
- Set an appropriate expiration time for tokens to enhance security.
- Use HTTPS to protect tokens in transit.
- Refresh tokens periodically to mitigate risks of token theft.
5. Conclusion
JWT authentication is a robust and scalable way to secure your Node.js applications. By integrating JWT with proper security practices, you can protect your application and enhance user experience.
OAuth2 and Third-party Login Integration
OAuth2 is a widely used authorization protocol that allows users to log in to applications using their existing accounts on third-party platforms like Google, Facebook, or GitHub. It eliminates the need for users to remember additional passwords and simplifies authentication.
1. What is OAuth2?
OAuth2 (Open Authorization 2.0) is a framework that allows a client application to access resources on behalf of a user without exposing their credentials. OAuth2 involves the following components:
- Client: The application requesting access (e.g., your web app).
- Authorization Server: The service that authenticates the user and provides access tokens (e.g., Google, Facebook).
- Resource Server: The server that stores user data and is accessed using the access token.
- Access Token: A token used to access the protected resources on behalf of the user.
2. Setting Up OAuth2 with Google
We will demonstrate how to integrate Google OAuth2 login into a Node.js application using the passport-google-oauth20
strategy with the help of the passport.js
Step 1: Install Required Packages
Install the necessary packages:
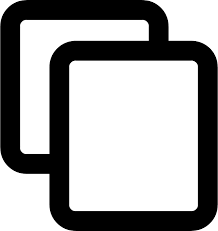
npm install express passport passport-google-oauth20 express-session
Step 2: Configure Google Developer Console
Before integrating Google OAuth, you need to create a project in the Google Developer Console and obtain your Client ID and Client Secret.
- Go to the Google Developer Console.
- Create a new project.
- Navigate to APIs & Services > Credentials and create OAuth 2.0 credentials.
- Set the redirect URI to
http://localhost:3000/auth/google/callback
(you can adjust this based on your app's domain). - Note the generated Client ID and Client Secret.
Step 3: Implement Google OAuth2 Login
Now, implement the OAuth2 login flow in your Node.js application:
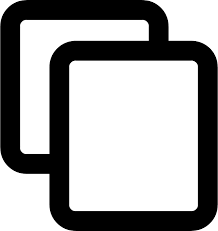
const express = require('express');
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth20').Strategy;
const session = require('express-session');
const app = express();
// Set up session
app.use(session({ secret: 'secret', resave: false, saveUninitialized: true }));
// Initialize Passport
app.use(passport.initialize());
app.use(passport.session());
// Configure Passport Google OAuth
passport.use(new GoogleStrategy({
clientID: 'YOUR_GOOGLE_CLIENT_ID',
clientSecret: 'YOUR_GOOGLE_CLIENT_SECRET',
callbackURL: 'http://localhost:3000/auth/google/callback'
}, (accessToken, refreshToken, profile, done) => {
return done(null, profile);
}));
// Serialize user info into session
passport.serializeUser((user, done) => {
done(null, user);
});
// Deserialize user info from session
passport.deserializeUser((user, done) => {
done(null, user);
});
// Route to start OAuth login
app.get('/auth/google', passport.authenticate('google', { scope: ['profile', 'email'] }));
// Callback route after user authenticates with Google
app.get('/auth/google/callback', passport.authenticate('google', { failureRedirect: '/' }), (req, res) => {
res.send('You are logged in with Google!');
});
// Route to display user info
app.get('/profile', (req, res) => {
if (!req.isAuthenticated()) {
return res.redirect('/');
}
res.send(`Hello ${req.user.displayName}`);
});
// Route to log out
app.get('/logout', (req, res) => {
req.logout((err) => {
res.redirect('/');
});
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
3. Flow of the OAuth2 Login
Here’s how the OAuth2 login process works:
- The user clicks the "Login with Google" button on your site (which triggers the
/auth/google
route). - They are redirected to Google’s OAuth2 consent screen to authorize the application.
- Once authorized, Google redirects the user back to your
/auth/google/callback
route with an access token. - The application uses the token to fetch user data and logs the user in, storing their profile in the session.
4. Best Practices
- Store sensitive OAuth credentials (Client ID, Secret) in environment variables for security.
- Use HTTPS in production to protect tokens and user data during the authentication flow.
- Implement token expiration and refresh mechanisms to ensure long-term user access.
- Handle errors appropriately, including failed login attempts or missing required permissions.
5. Conclusion
OAuth2 allows you to offer a seamless third-party login experience for users on your Node.js app. By integrating OAuth2 with Google or other providers, you can quickly authenticate users while maintaining security. Always ensure that sensitive data is protected and handle authentication errors gracefully.
Preventing Security Vulnerabilities (XSS, CSRF)
Security vulnerabilities like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF) are common threats in web applications that can lead to data theft, unauthorized actions, or loss of user trust. Understanding these vulnerabilities and how to prevent them is crucial for building secure applications.
1. Cross-Site Scripting (XSS)
XSS occurs when an attacker injects malicious scripts into web pages viewed by other users. These scripts can execute on the client side, stealing sensitive data like cookies, session tokens, or login credentials.
Types of XSS
- Reflected XSS: Malicious scripts are reflected off the web server and executed in the user's browser.
- Stored XSS: Malicious scripts are stored on the web server (e.g., in a database) and executed whenever the affected page is viewed.
- DOM-based XSS: The vulnerability is in the client-side JavaScript that manipulates the DOM, allowing an attacker to inject malicious scripts.
Preventing XSS in Node.js
Here are some strategies to prevent XSS vulnerabilities in Node.js applications:
- Escape user input: Always escape any user-generated content before rendering it on the page. Use libraries like
validator
orsanitize-html
to clean input. - Use Content Security Policy (CSP): Implement CSP headers to restrict the sources from which scripts can be loaded, reducing the chance of malicious script execution.
- Sanitize HTML: Use libraries like
DOMPurify
to sanitize HTML content before rendering it, ensuring that no malicious script tags are injected. - HTTPOnly and Secure Cookies: Use
HTTPOnly
andSecure
flags for cookies to prevent JavaScript from accessing session cookies.
Code Example: Escaping User Input
The following example demonstrates how to escape user input using the escape-html
library:
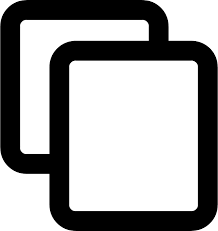
const escapeHtml = require('escape-html');
// User input
// Escape input to prevent XSS
const safeInput = escapeHtml(userInput);
console.log(safeInput); // <script>alert("XSS Attack!")</script>
2. Cross-Site Request Forgery (CSRF)
CSRF is an attack where a malicious actor tricks a user into making unwanted requests to a web application where the user is authenticated. This can lead to unauthorized actions, such as changing account settings, transferring funds, or deleting data.
How CSRF Works
In a CSRF attack, an attacker uses social engineering to trick a user into clicking on a link or loading an image that sends a request to an application that the user is logged into. Since the request contains the user's authentication token (e.g., session cookie), the application processes the request as if it was made by the legitimate user.
Preventing CSRF in Node.js
To prevent CSRF attacks, here are some recommendations:
- Use CSRF tokens: Generate a unique CSRF token for each form submission or AJAX request and validate it on the server side. Libraries like
csurf
can help implement CSRF protection. - SameSite Cookies: Set the
SameSite
attribute on cookies toStrict
orLax
to prevent cookies from being sent with cross-origin requests. - Double-Submit Cookie Pattern: In addition to CSRF tokens, use a double-submit technique where the CSRF token is sent both in the cookie and in the request body or headers, and both values must match.
Code Example: Implementing CSRF Protection
The following example shows how to use the csurf
middleware to protect against CSRF attacks:
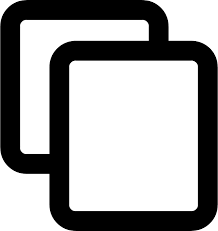
const express = require('express');
const csrf = require('csurf');
const cookieParser = require('cookie-parser');
const app = express();
// Setup middleware
app.use(cookieParser());
const csrfProtection = csrf({ cookie: true });
app.use(csrfProtection);
// Serve the form with a CSRF token
app.get('/form', (req, res) => {
res.send(`
`);
});
// Handle form submission
app.post('/submit', (req, res) => {
res.send('Form submitted successfully!');
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
3. Best Practices for Securing Node.js Applications
- Validate and sanitize input: Always sanitize user input to prevent injection attacks like XSS or SQL injection.
- Use HTTPS: Secure your application using HTTPS to protect data in transit.
- Implement proper authentication: Use secure authentication mechanisms like JWT and OAuth2.
- Regularly update dependencies: Ensure that your application and its dependencies are up to date to avoid known vulnerabilities.
- Monitor and log security events: Implement monitoring and logging for suspicious activity to detect and respond to threats quickly.
4. Conclusion
Security vulnerabilities like XSS and CSRF pose significant threats to web applications, but with proper precautions, they can be prevented. By escaping user input, using CSRF tokens, and following security best practices, you can protect your Node.js application from these common attacks. Always stay informed about the latest security threats and keep your application secure.
Data Encryption and Hashing
Data encryption and hashing are vital techniques used to protect sensitive information in web applications. Encryption ensures that data is readable only by authorized parties, while hashing is used for securely storing passwords and verifying data integrity. These techniques are widely used in security protocols to prevent unauthorized access and ensure data confidentiality and integrity.
1. Data Encryption
Encryption is the process of converting readable data (plaintext) into an unreadable format (ciphertext) to prevent unauthorized access. Only authorized users with a decryption key can convert the data back to its original form.
Types of Encryption
- Symmetric Encryption: A single key is used for both encryption and decryption. The key must be kept secret. Common algorithms include
AES
(Advanced Encryption Standard). - Asymmetric Encryption: Uses a pair of keys: a public key for encryption and a private key for decryption.
RSA
is a commonly used asymmetric encryption algorithm.
How Encryption Works
Encryption algorithms take plaintext data and a key as input and produce ciphertext as output. Decryption algorithms reverse the process by using the appropriate key to convert ciphertext back to plaintext.
Encrypting Data in Node.js
Node.js provides the crypto
module, which is used to perform encryption and decryption operations. Below is an example of encrypting and decrypting data using symmetric encryption (AES):
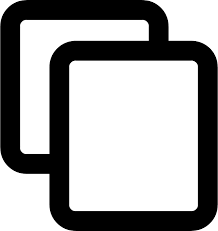
const crypto = require('crypto');
// Symmetric encryption (AES)
const algorithm = 'aes-256-cbc';
const secretKey = 'mySuperSecretKey123!';
const iv = crypto.randomBytes(16);
// Encrypt data
const encrypt = (data) => {
const cipher = crypto.createCipheriv(algorithm, Buffer.from(secretKey), iv);
let encrypted = cipher.update(data, 'utf8', 'hex');
encrypted += cipher.final('hex');
return { iv: iv.toString('hex'), encryptedData: encrypted };
};
// Decrypt data
const decrypt = (encryptedData, iv) => {
const decipher = crypto.createDecipheriv(algorithm, Buffer.from(secretKey), Buffer.from(iv, 'hex'));
let decrypted = decipher.update(encryptedData, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
};
// Example usage
const data = 'Sensitive Information';
const encrypted = encrypt(data);
console.log('Encrypted Data:', encrypted);
const decrypted = decrypt(encrypted.encryptedData, encrypted.iv);
console.log('Decrypted Data:', decrypted);
2. Data Hashing
Hashing is the process of converting data into a fixed-length value (hash) using a hashing algorithm. Unlike encryption, hashing is a one-way process, meaning that the original data cannot be recovered from the hash. Hashing is typically used for securely storing passwords or verifying data integrity.
Common Hashing Algorithms
- SHA-256: Part of the SHA-2 family of algorithms, SHA-256 produces a 256-bit hash and is widely used for securing data.
- MD5: A widely used but insecure hashing algorithm due to vulnerabilities in collision resistance. It should not be used for sensitive data.
- Bcrypt: A popular algorithm for hashing passwords, as it incorporates salting and multiple rounds to make brute-force attacks more difficult.
How Hashing Works
Hashing algorithms take an input (e.g., a password) and return a fixed-length hash value. When verifying the data (e.g., checking a password), the hash of the provided input is compared with the stored hash to determine if they match.
Hashing Passwords in Node.js
To securely hash passwords in Node.js, you can use libraries such as bcryptjs
or argon2
. Below is an example of how to hash and verify passwords using the bcryptjs
library:
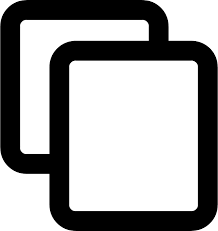
const bcrypt = require('bcryptjs');
// Hash a password
const hashPassword = async (password) => {
const salt = await bcrypt.genSalt(10); // Generate a salt
const hashedPassword = await bcrypt.hash(password, salt);
return hashedPassword;
};
// Verify a password
const verifyPassword = async (password, hashedPassword) => {
const match = await bcrypt.compare(password, hashedPassword);
return match;
};
// Example usage
const password = 'userPassword123';
const hashedPassword = await hashPassword(password);
console.log('Hashed Password:', hashedPassword);
const isMatch = await verifyPassword('userPassword123', hashedPassword);
console.log('Password Match:', isMatch);
3. Salting Hashes
Salting is the process of adding a random value (salt) to the data before hashing it. This prevents attackers from using precomputed hash databases (rainbow tables) to crack passwords. In the example above, the bcrypt.genSalt()
method generates a random salt for each password before hashing it.
4. Best Practices for Encryption and Hashing
- Use strong algorithms: Always use strong and well-tested encryption and hashing algorithms like
AES
for encryption andBcrypt
for password hashing. - Never store plain-text passwords: Always hash passwords before storing them in the database.
- Use unique salts for each hash: Salts should be unique for each password or piece of data being hashed to prevent rainbow table attacks.
- Secure your keys: Protect encryption keys by using secure storage solutions, such as environment variables or key management systems.
- Use encryption for sensitive data: Encrypt sensitive data before storing it, especially if it needs to be sent across the network.
5. Conclusion
Data encryption and hashing are essential techniques for securing sensitive information in modern web applications. While encryption ensures that data remains confidential, hashing is used for verifying data integrity and securely storing passwords. By following best practices and using strong algorithms, you can protect your application from data breaches and unauthorized access.
Introduction to WebSockets
WebSockets provide a full-duplex communication channel over a single, long-lived connection. Unlike traditional HTTP, where the client sends a request and waits for a response, WebSockets allow for bidirectional communication, enabling both the client and server to send and receive data in real-time.
What are WebSockets?
WebSockets are a protocol designed to enable two-way communication between a client (usually a web browser) and a server. Once established, the WebSocket connection remains open, allowing both parties to exchange data in real-time with minimal overhead. WebSockets are useful for applications that require frequent, real-time updates, such as chat applications, live notifications, gaming, or financial tickers.
How WebSockets Work
WebSockets work through a handshake process that starts as an HTTP request, but then the connection is upgraded to a WebSocket connection, allowing for continuous communication. Once the connection is established, the client and server can send messages back and forth without needing to re-establish the connection each time.
WebSocket Handshake
The WebSocket handshake is initiated by the client, which sends an HTTP request with an "Upgrade" header indicating that it wants to establish a WebSocket connection. If the server supports WebSockets, it responds with a status code of 101 (Switching Protocols), and the WebSocket connection is established.
Advantages of WebSockets
- Real-time Communication: WebSockets allow for low-latency, real-time communication between the client and server, making them ideal for interactive applications.
- Reduced Overhead: WebSockets minimize the overhead of repeatedly establishing HTTP connections, which can be costly for applications that require frequent updates.
- Bi-directional Communication: Both the client and server can send and receive data independently, enabling more interactive and dynamic user experiences.
- Persistent Connection: The WebSocket connection remains open, allowing for continuous data exchange without the need to re-establish connections.
WebSocket Use Cases
- Chat Applications: WebSockets are commonly used in real-time chat applications, enabling instant message delivery and notifications.
- Live Sports Updates: WebSockets allow users to receive live scores and statistics as events happen in real-time.
- Stock Price Tickers: Financial applications can use WebSockets to provide live stock price updates.
- Online Gaming: WebSockets allow real-time updates and communication between players in multiplayer games.
- Collaborative Tools: WebSockets are used for real-time collaboration, allowing multiple users to edit or view documents simultaneously.
Setting Up WebSockets in Node.js
To implement WebSockets in a Node.js application, you can use a package like ws
, which provides WebSocket server and client implementations. Below is an example of creating a WebSocket server and client in Node.js:
WebSocket Server Example (Node.js)
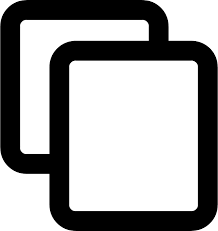
const WebSocket = require('ws');
// Create a WebSocket server on port 8080
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('A new client connected');
// Send a message to the client
ws.send('Welcome to the WebSocket server!');
// Handle incoming messages from the client
ws.on('message', (message) => {
console.log('Received message:', message);
ws.send(`You said: ${message}`);
});
// Handle client disconnection
ws.on('close', () => {
console.log('A client disconnected');
});
});
console.log('WebSocket server is running on ws://localhost:8080');
WebSocket Client Example (HTML + JavaScript)
The client-side WebSocket code can be implemented directly in the browser using JavaScript:
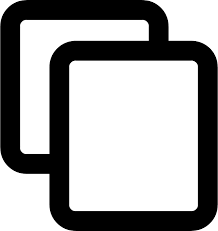
const socket = new WebSocket('ws://localhost:8080');
// Event listener for when the connection is established
socket.addEventListener('open', (event) => {
console.log('Connected to the WebSocket server');
socket.send('Hello, Server!');
});
// Event listener for when a message is received from the server
socket.addEventListener('message', (event) => {
console.log('Received from server:', event.data);
});
// Event listener for when the connection is closed
socket.addEventListener('close', () => {
console.log('Disconnected from the WebSocket server');
});
WebSocket Events
WebSocket connections trigger several events, which can be handled on both the client and server sides:
- open: Triggered when the WebSocket connection is successfully established.
- message: Triggered when a message is received from the other side of the connection.
- close: Triggered when the WebSocket connection is closed by either the client or the server.
- error: Triggered if an error occurs during communication.
Handling WebSocket Errors
WebSocket connections can fail due to network issues, server crashes, or protocol errors. It's important to handle WebSocket errors to ensure smooth communication and to retry the connection if necessary.
Example: Handling WebSocket Errors
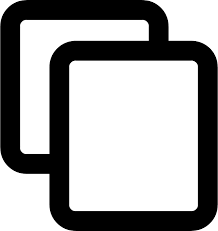
socket.addEventListener('error', (event) => {
console.error('WebSocket error:', event);
});
Conclusion
WebSockets provide an efficient way to enable real-time, bidirectional communication between clients and servers. They are widely used in applications that require instant data updates, such as chat apps, live notifications, and real-time gaming. By using WebSockets, developers can create more dynamic and interactive web applications with minimal latency.
Using Socket.io for Real-time Communication
Socket.io is a popular JavaScript library that enables real-time, bidirectional communication between web clients and servers. It is built on top of WebSockets and provides additional features such as automatic reconnection, broadcasting, and event handling, making it ideal for use cases like chat applications, notifications, and live updates.
What is Socket.io?
Socket.io is a framework that simplifies the process of implementing WebSockets in web applications. It provides an abstraction layer over the WebSocket protocol, allowing for easier communication and fallback to other technologies when WebSockets are not supported by the client’s browser. Socket.io supports both WebSocket and HTTP long-polling as transport mechanisms, ensuring that your application can work across all environments.
How Socket.io Works
Socket.io operates in two parts: the server-side component, which runs on the Node.js server, and the client-side component, which runs in the browser. The client connects to the server using a WebSocket connection or falls back to other HTTP-based protocols if WebSockets are not available. Once the connection is established, real-time communication can take place through emitting and receiving events.
Key Features of Socket.io
- Real-time Communication: Enables instantaneous, low-latency communication between clients and the server.
- Event-based Communication: Allows custom events to be emitted and received between the client and server.
- Auto-reconnection: Automatically reconnects clients if the connection is lost.
- Namespace Support: Lets you create multiple channels for communication between clients and servers.
- Room Support: Allows clients to join different rooms to receive specific messages.
- Fallback Mechanisms: Socket.io can fall back to HTTP long-polling for older browsers or when WebSockets are unavailable.
Setting Up Socket.io in Node.js
To start using Socket.io in a Node.js application, you need to install the socket.io
package on both the server and client sides. Below is an example of how to set up a simple real-time chat application using Socket.io in Node.js.
Step 1: Install Socket.io
First, install Socket.io in your Node.js project:
npm install socket.io
Step 2: Create a Socket.io Server
Next, create a basic Node.js server using the express
framework and integrate Socket.io:
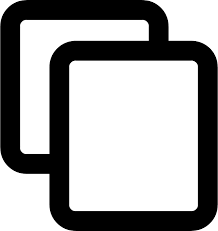
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
io.on('connection', (socket) => {
console.log('A user connected');
// Listen for a 'chat message' event from the client
socket.on('chat message', (msg) => {
console.log('Message received: ' + msg);
// Emit the 'chat message' event to all connected clients
io.emit('chat message', msg);
});
socket.on('disconnect', () => {
console.log('A user disconnected');
});
});
server.listen(3000, () => {
console.log('Socket.io server running on http://localhost:3000');
});
Step 3: Create the Client-side Code
The client-side code will establish a WebSocket connection to the server and send/receive messages. Create an index.html
file in the root directory of your project:
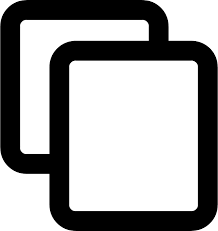
<!DOCTYPE html>
<html>
<head>
<title>Real-time Chat with Socket.io</title>
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io();
// Send chat message to the server
function sendMessage() {
var message = document.getElementById('message').value;
socket.emit('chat message', message);
document.getElementById('message').value = '';
}
// Listen for incoming chat messages
socket.on('chat message', function(msg){
var item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
</script>
</head>
<body>
<ul id="messages"></ul>
<input id="message" autocomplete="off"><button onclick="sendMessage()">Send</button>
</body>
</html>
Socket.io Events
Socket.io uses events to handle communication between the client and server. Below are some of the most commonly used events:
- connection: Triggered when a client successfully connects to the server.
- disconnect: Triggered when a client disconnects from the server.
- message: A custom event that can be used for sending messages between client and server.
- emit: Used to send data from the server to the clients.
- on: Used to listen for events on the client-side (e.g., when a message is received).
Socket.io Rooms
Socket.io allows you to group clients into "rooms", enabling targeted communication. Clients can join and leave rooms dynamically, and messages can be sent to specific rooms instead of broadcasting to all connected clients.
Example: Using Rooms
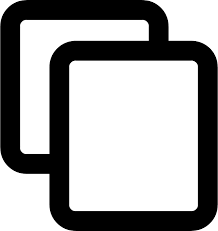
// Server-side code for using rooms
io.on('connection', (socket) => {
console.log('A user connected');
// Join a specific room
socket.on('join room', (room) => {
socket.join(room);
console.log(`User joined room: ${room}`);
});
// Emit a message to a specific room
socket.on('send room message', (room, msg) => {
io.to(room).emit('chat message', msg);
});
});
Handling Socket.io Errors
Socket.io includes error handling to ensure that any issues during communication are caught and managed gracefully. For example, if a client fails to connect, an error event can be emitted:
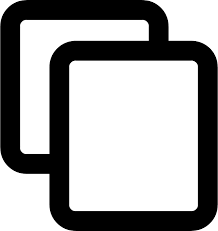
socket.on('connect_error', (err) => {
console.error('Connection error:', err);
});
Conclusion
Socket.io is a powerful tool for real-time communication in web applications. By abstracting WebSockets and providing robust features like automatic reconnection and room support, Socket.io makes it easier to build interactive, real-time applications. Whether you are building a chat app, live updates, or collaborative tools, Socket.io is a great choice for implementing real-time functionality.
Building a Chat Application
In this section, we will build a simple real-time chat application using Socket.io. This application will allow users to send and receive messages in real time. The user interface will be built with HTML and JavaScript, and we'll set up the server using Node.js and Express.
Features of the Chat Application
- Real-time Messaging: Users can send and receive messages instantly.
- Message History: The server stores the messages, and clients can retrieve them when they reconnect.
- Multiple Users: Multiple users can join the chat and exchange messages simultaneously.
Step-by-Step Guide
Let's break down the steps needed to build this chat application:
Step 1: Install Dependencies
First, install the necessary packages, including express
and socket.io
:
npm install express socket.io
Step 2: Create the Server
Next, create a basic Node.js server using Express and integrate Socket.io for real-time communication:
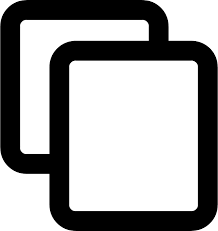
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
let messages = [];
// Serve static files (HTML, CSS, JS)
app.use(express.static('public'));
app.get('/', (req, res) => {
res.sendFile(__dirname + '/public/index.html');
});
// Real-time communication with Socket.io
io.on('connection', (socket) => {
console.log('A user connected');
// Send message history to the newly connected user
socket.emit('message history', messages);
// Listen for a 'chat message' event from the client
socket.on('chat message', (msg) => {
console.log('Message received: ' + msg);
messages.push(msg);
io.emit('chat message', msg); // Broadcast message to all users
});
// Handle disconnection
socket.on('disconnect', () => {
console.log('A user disconnected');
});
});
// Start the server
server.listen(3000, () => {
console.log('Chat app server running on http://localhost:3000');
});
Step 3: Create the Client-side Code
Now, create the HTML and JavaScript for the client-side. The client will connect to the server using Socket.io, send messages, and display incoming messages in real-time:
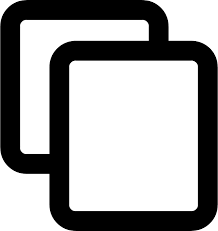
<!DOCTYPE html>
<html>
<head>
<title>Real-time Chat Application</title>
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io();
// Display message history on connection
socket.on('message history', function(messages) {
messages.forEach(function(msg) {
var item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
});
// Listen for incoming chat messages
socket.on('chat message', function(msg){
var item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
// Send chat message to the server
function sendMessage() {
var message = document.getElementById('message').value;
socket.emit('chat message', message);
document.getElementById('message').value = '';
}
</script>
</head>
<body>
<ul id="messages"></ul>
<input id="message" autocomplete="off"><button onclick="sendMessage()">Send</button>
</body>
</html>
How It Works
In this chat application:
- The server stores all sent messages in an array (
messages
), which is sent to the client when a new user connects. - The client uses Socket.io to listen for incoming messages and append them to the chat window in real-time.
- When a user sends a message, the client emits a
'chat message'
event to the server, which then broadcasts the message to all connected clients.
Improving the Chat Application
While the chat app we've built is simple, you can improve it by adding the following features:
- Authentication: Allow users to log in with a username or through OAuth.
- Private Messaging: Implement private rooms or direct messaging between users.
- Message Formatting: Use HTML or Markdown to allow for rich-text messages.
- Notifications: Add notifications when a new message is received or when a user joins or leaves the chat.
Conclusion
By following these steps, you've learned how to build a basic real-time chat application using Socket.io. This application demonstrates how to use WebSockets to enable live communication between clients and a Node.js server. Socket.io abstracts many of the complexities of WebSockets, making it easier to build interactive, real-time applications. You can extend this chat app with additional features to make it even more dynamic and useful.
Understanding and Managing the Event Loop
The event loop is one of the core concepts of Node.js, enabling asynchronous, non-blocking I/O operations. Node.js is built on a single-threaded event loop, meaning that it can handle multiple operations simultaneously without blocking execution. Understanding how the event loop works is crucial to building efficient Node.js applications.
What is the Event Loop?
The event loop is a mechanism that allows Node.js to perform non-blocking I/O operations. It does this by delegating operations such as reading files, querying databases, or making HTTP requests to the operating system. While these operations are being processed, Node.js continues executing other code without being blocked. Once the operation completes, the event loop returns the result and moves on to the next task.
How the Event Loop Works
The event loop works in several phases, processing different types of events and callbacks. The key phases are:
- Timer phase: Executes callbacks scheduled by
setTimeout()
andsetInterval()
. - I/O callbacks phase: Handles almost all callbacks, except for close callbacks, timers, and setImmediate callbacks.
- Idle, prepare phase: Used internally by Node.js for housekeeping.
- Poll phase: Retrieves new I/O events; executes I/O-related callbacks (except for timers and setImmediate).
- Check phase: Executes callbacks scheduled by
setImmediate()
. - Close callbacks phase: Executes close events, such as the
close
event on a TCP connection.
Event Loop Phases
Here's an overview of the event loop phases and their respective roles:
- Timer Phase: Executes functions scheduled by
setTimeout()
orsetInterval()
. These functions are executed after the specified time has passed. - Poll Phase: In this phase, Node.js checks for new events, such as I/O operations. It is responsible for executing I/O-related callbacks that are not timers or close callbacks.
- Check Phase: Handles callbacks from
setImmediate()
that are scheduled to run after the poll phase. - Close Callbacks Phase: This phase handles the cleanup of closed events, such as closing TCP connections or other resources.
Event Loop Example
Let's look at an example that demonstrates how the event loop works with asynchronous operations:
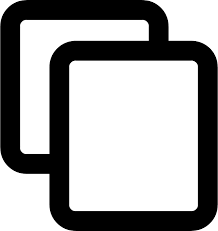
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
setImmediate(() => {
console.log('Immediate');
});
console.log('End');
Explanation:
The output of this code will be:
Start
End
Immediate
Timeout
This happens because:
- The
console.log('Start')
is executed first. - The
setTimeout()
callback is scheduled in the Timer phase, but since its delay is 0, it moves to the Poll phase, but thesetImmediate()
callback is executed first in the Check phase. - Finally, after the Check phase completes, the
setTimeout()
callback is executed in the Timers phase.
Managing the Event Loop
Node.js provides several mechanisms for managing the event loop, such as:
- setTimeout() and setInterval(): These functions are used to schedule callbacks to run after a specified time, which ensures that the event loop remains non-blocking.
- process.nextTick(): This function allows you to schedule a callback to be executed in the same phase of the event loop before any I/O operations.
- setImmediate(): This function schedules a callback to be executed immediately after the current event loop cycle, during the Check phase.
Best Practices for Event Loop Management
To ensure that your Node.js application runs efficiently, here are some best practices for managing the event loop:
- Avoid Blocking Operations: Use asynchronous APIs whenever possible to avoid blocking the event loop and affecting performance. For example, use
fs.readFile()
instead offs.readFileSync()
to prevent blocking. - Limit I/O Operations: Minimize the number of I/O operations that require waiting for external resources, such as file or network access, within the event loop.
- Use setImmediate() for Non-blocking Operations: Use
setImmediate()
to schedule operations that need to be executed after the event loop cycle, without blocking the current operations. - Monitor Event Loop Delays: Use tools like
clinic.js
ornode --inspect
to monitor the event loop's performance and diagnose any delays or blockages.
Conclusion
Understanding the event loop is essential for optimizing Node.js applications. By managing asynchronous operations and avoiding blocking calls, you can ensure that your application performs efficiently and responds to events in real-time. The event loop is the backbone of Node.js, enabling high-performance, non-blocking applications.
Using Clustering and Child Processes
Node.js is single-threaded by default, which means it runs on a single thread and can only use one core of the CPU. However, for applications that need to handle a large volume of requests, Node.js provides mechanisms such as clustering and child processes to take advantage of multi-core systems and improve performance.
What is Clustering?
Clustering in Node.js allows you to create multiple child processes (workers) that can share the same server port. Each worker runs on a separate core of the CPU, enabling your application to handle more requests concurrently. Node.js provides a cluster
module that makes it easy to create and manage worker processes.
How Clustering Works
The cluster
module is built on top of Node.js's child process module. It allows you to create child processes (workers) that share the same server port and distribute the incoming requests among them. The master process is responsible for spawning worker processes, while the worker processes handle requests independently.
Setting Up Clustering in Node.js
Here's how to implement clustering in a Node.js application:
Step 1: Import the Cluster Module
First, import the cluster
module and os
module to determine the number of CPU cores:
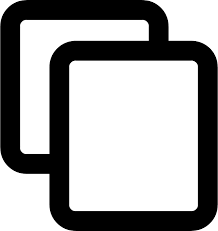
const cluster = require('cluster');
const http = require('http');
const os = require('os');
const numCPUs = os.cpus().length; // Get the number of CPU cores
Step 2: Create the Master and Worker Processes
Next, use the cluster module to create the master and worker processes. The master process will fork workers equal to the number of CPU cores:
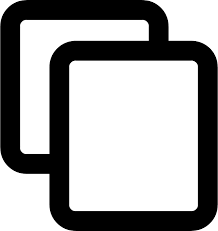
if (cluster.isMaster) {
// Fork workers
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
});
} else {
// Worker processes
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello from Worker ' + process.pid);
}).listen(8000);
}
Explanation:
- If the current process is the master process, it forks a number of workers equal to the number of CPU cores.
- Each worker creates an HTTP server that listens on port 8000.
- If a worker dies, the master process logs the event and can re-fork a new worker.
Benefits of Clustering
- Better Utilization of CPU Cores: Clustering allows Node.js to use all CPU cores, improving the performance and scalability of the application.
- Increased Throughput: With multiple workers handling requests in parallel, the application can handle more requests per second.
- Fault Tolerance: If a worker crashes, the master process can spawn a new one, ensuring that the application remains available.
What are Child Processes?
Child processes in Node.js allow you to spawn separate processes from the main application. These child processes can run independently and perform tasks like executing shell commands, processing data, or running other scripts. Node.js provides the child_process
module to create and manage child processes.
Using Child Processes in Node.js
Here's how to use child processes in Node.js to run an external command:
Step 1: Import the Child Process Module
First, import the child_process
module:
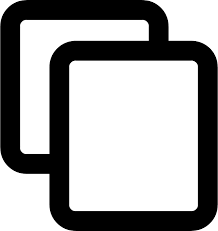
const { exec } = require('child_process');
Step 2: Run a Command
Use the exec
method to run a shell command:
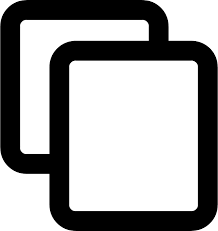
exec('ls -al', (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
if (stderr) {
console.error(`stderr: ${stderr}`);
return;
}
console.log(`stdout: ${stdout}`);
});
Explanation:
- The
exec
function runs thels -al
command, which lists the files and directories in the current directory. - The callback function handles any errors, standard output, and standard error that result from running the command.
Other Methods for Creating Child Processes
- spawn(): The
spawn
method is used for long-running processes or when you need to interact with the output of the process (i.e., streaming). Unlikeexec()
,spawn()
does not buffer the output. - fork(): The
fork()
method is a special case ofspawn()
used to spawn Node.js processes. It is commonly used in clustering scenarios where you need to spawn a new Node.js process.
Best Practices for Using Clustering and Child Processes
- Monitor Worker Processes: Always monitor the health of your worker processes and ensure that you can recover from failures by restarting workers.
- Use Clustering for Load Balancing: Leverage clustering to distribute requests across multiple workers, ensuring that your application scales efficiently.
- Limit Child Process Usage: Avoid spawning too many child processes, as it can lead to resource exhaustion and degrade performance.
Conclusion
Clustering and child processes are powerful tools for scaling Node.js applications. While Node.js is single-threaded by default, you can take advantage of multi-core systems by using clustering to distribute tasks across multiple workers. Additionally, child processes can be used for running external commands and handling long-running tasks. By understanding and implementing these techniques, you can build highly scalable and efficient Node.js applications.
Implementing Caching for Performance
Caching is a technique that stores copies of frequently accessed data in a temporary storage area (cache) to reduce the time and resources needed to fetch the data again. Implementing caching in your Node.js application can significantly improve performance by reducing database load, speeding up response times, and enhancing scalability.
What is Caching?
Caching involves storing data temporarily in a location that can be accessed much faster than the original source. For example, instead of querying a database every time a client requests data, you can store the result of the query in a cache. The next time the same data is requested, the cache will be checked first before querying the database.
Benefits of Caching
- Reduced Latency: Retrieving data from a cache is faster than querying the database or making external API calls, resulting in quicker response times.
- Reduced Load on Backend Systems: Caching reduces the number of database queries or external API calls, minimizing the load on your backend systems.
- Scalability: Caching allows your application to handle more requests without putting additional strain on the backend, improving scalability.
Types of Caching
- In-Memory Caching: Data is cached in the application's memory. This is the fastest type of caching but limited by the available memory.
- Distributed Caching: Data is cached across multiple machines or nodes. It provides scalability and redundancy, making it suitable for large applications.
- HTTP Caching: HTTP caching involves caching entire HTTP responses. It can be done using cache-control headers or reverse proxies.
Using Redis for Caching
Redis is a widely used, high-performance in-memory data store that can be used for caching. It supports various data types such as strings, hashes, lists, sets, and sorted sets, making it a versatile solution for caching data.
Setting Up Redis in Node.js
To use Redis in your Node.js application, you need to install the redis
client package:
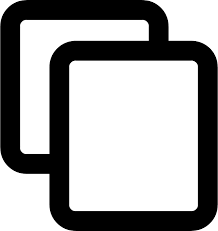
npm install redis
Step 1: Create a Redis Client
Next, create a Redis client in your application and connect it to the Redis server:
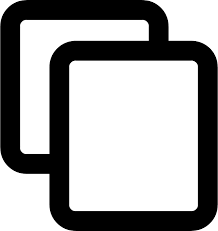
const redis = require('redis');
const client = redis.createClient(); // Connect to Redis server
client.on('connect', () => {
console.log('Connected to Redis');
});
Step 2: Setting and Getting Cached Data
You can set and retrieve cached data using the set
and get
methods:
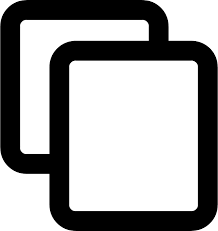
const cacheKey = 'user_data';
const userData = { name: 'John', age: 30 };
// Set data in the cache
client.set(cacheKey, JSON.stringify(userData), 'EX', 3600, (err, reply) => {
if (err) throw err;
console.log('Data cached:', reply);
});
// Get data from the cache
client.get(cacheKey, (err, reply) => {
if (err) throw err;
console.log('Cached data:', JSON.parse(reply));
});
Explanation:
- The
set
method stores data in Redis with an expiration time (in seconds) using theEX
option. In this example, the data expires after 3600 seconds (1 hour). - The
get
method retrieves data from the cache. If the data is not found, the callback will receive anull
value.
Cache Invalidation
Cache invalidation is the process of removing or updating cached data when the underlying data changes. If you don’t invalidate your cache properly, your application may serve outdated or stale data to users.
Strategies for Cache Invalidation
- Time-based Expiration: Set an expiration time for each cached entry. After the time expires, the cache is invalidated automatically.
- Manual Invalidation: Manually delete or update cached data when the underlying data changes. For example, after updating a user's information in the database, you can delete their cached data.
- Versioning: Use versioning to change the cache key whenever the data structure changes. This ensures that the old cache is bypassed.
Using Cache with HTTP Requests
In some cases, you might want to cache the results of HTTP requests to reduce the load on the server. One way to do this is by using HTTP cache control headers or reverse proxies like Varnish or Nginx.
Example: Caching HTTP Responses
In this example, we use Redis to cache HTTP responses for GET requests:
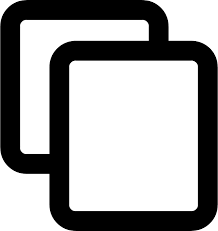
const express = require('express');
const redis = require('redis');
const client = redis.createClient();
const app = express();
app.get('/user/:id', (req, res) => {
const userId = req.params.id;
const cacheKey = `user_${userId}`;
// Check if data is cached
client.get(cacheKey, (err, cachedData) => {
if (err) throw err;
if (cachedData) {
// Return cached data
return res.json(JSON.parse(cachedData));
} else {
// Fetch data from database (simulated)
const user = { id: userId, name: 'John Doe' };
// Cache the data for 1 hour
client.setex(cacheKey, 3600, JSON.stringify(user));
// Return the fetched data
res.json(user);
}
});
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Explanation:
- When a client requests the user data, the server first checks if the data is cached in Redis.
- If the data is cached, it returns the cached data. Otherwise, it fetches the data from the database (simulated), caches it, and sends the response to the client.
Best Practices for Caching
- Cache Frequently Accessed Data: Cache data that is frequently requested and changes infrequently, such as user profiles or product details.
- Avoid Over-Caching: Don’t cache data that changes frequently or data that is unique to a specific user, as this can lead to outdated or irrelevant data being served.
- Use Expiration: Always set an expiration time for cached data to ensure that it remains fresh and that the cache doesn’t grow uncontrollably.
- Monitor Cache Performance: Keep track of cache hit/miss rates to ensure the caching strategy is effective and efficient.
Conclusion
Implementing caching in your Node.js application can significantly improve performance, reduce server load, and enhance scalability. Redis is a popular choice for caching, and it can be easily integrated into your Node.js applications. By using caching effectively, you can ensure that your application responds quickly to client requests while reducing the strain on your backend systems.
Load Balancing in Node.js
Load balancing is a technique used to distribute incoming network traffic across multiple servers to ensure no single server is overwhelmed. In Node.js applications, load balancing helps improve scalability, reliability, and performance. By spreading requests across multiple instances of the application, you can handle more traffic, avoid downtime, and ensure high availability.
Why Load Balancing is Important
When your Node.js application starts receiving a large number of requests, a single server may not be able to handle the load. Load balancing helps distribute the traffic across multiple servers or processes, preventing any one server from becoming a bottleneck. This ensures that users experience fast response times and the system remains operational even during high traffic periods.
Types of Load Balancing
- Round Robin: Requests are distributed evenly among servers in a circular order. This is the simplest method of load balancing.
- Least Connections: Requests are sent to the server with the least number of active connections. This method is useful when some requests are more resource-intensive than others.
- IP Hash: A hash of the client’s IP address is used to determine which server will handle the request. This method ensures that requests from the same client are always routed to the same server.
Implementing Load Balancing in Node.js
There are different ways to implement load balancing in Node.js. One common approach is using the cluster
module, which allows you to create multiple child processes that can share the same server port. Another approach is to use an external load balancer like NGINX
or HAProxy
to distribute traffic across multiple Node.js instances running on different servers.
Using the Cluster Module for Load Balancing
The cluster
module in Node.js allows you to create multiple worker processes that can handle requests concurrently, improving the scalability of your application. Each worker process runs on a separate core of the CPU, enabling better utilization of multi-core systems.
Setting Up the Cluster Module
Here is an example of how to set up load balancing using the cluster
module in a Node.js application:
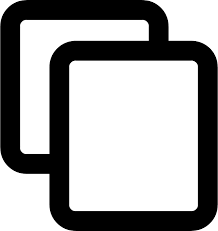
const cluster = require('cluster');
const http = require('http');
const os = require('os');
const numCPUs = os.cpus().length; // Get the number of CPU cores
if (cluster.isMaster) {
// Fork workers for each CPU core
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
});
} else {
// Worker processes have a HTTP server each
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, world!');
}).listen(8000);
}
Explanation:
- The
cluster.isMaster
condition checks if the current process is the master process. If it is, the master process forks worker processes equal to the number of CPU cores. - Each worker process runs an HTTP server and listens on the same port (8000). When a request comes in, one of the workers will handle it, depending on which worker is available.
- The
cluster.on('exit')
event is used to log when a worker process dies.
Using NGINX as a Load Balancer
Another popular approach for load balancing in Node.js applications is using NGINX, a high-performance web server and reverse proxy. NGINX can distribute incoming requests across multiple Node.js instances running on different servers or on the same server, providing better performance, redundancy, and fault tolerance.
Setting Up NGINX for Load Balancing
To use NGINX as a load balancer, you need to configure it to distribute requests among multiple Node.js instances. Here is an example configuration:
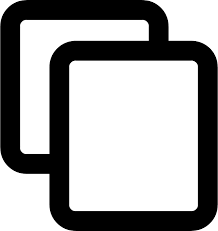
http {
upstream nodejs_servers {
server 127.0.0.1:3000;
server 127.0.0.1:3001;
server 127.0.0.1:3002;
}
server {
listen 80;
location / {
proxy_pass http://nodejs_servers;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
}
Explanation:
- The
upstream
directive defines a group of servers (in this case, three Node.js instances running on different ports). - The
proxy_pass
directive forwards incoming requests to one of the Node.js instances in thenodejs_servers
group. - NGINX will automatically distribute requests using a round-robin method by default, but this can be customized to use other methods like least connections.
Using HAProxy for Load Balancing
HAProxy is another popular load balancer that is often used to distribute traffic between multiple Node.js instances. It works similarly to NGINX and can be used to manage incoming requests to your application.
Setting Up HAProxy for Load Balancing
Here is an example configuration for HAProxy to load balance between multiple Node.js instances:
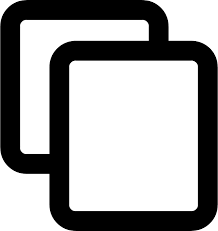
frontend http_front
bind *:80
default_backend http_back
backend http_back
balance roundrobin
server node1 127.0.0.1:3000 check
server node2 127.0.0.1:3001 check
server node3 127.0.0.1:3002 check
Explanation:
- The
frontend
section defines the entry point for incoming HTTP requests (port 80). All requests are forwarded to thehttp_back
backend. - The
backend
section defines the pool of servers that HAProxy will distribute requests to. Theroundrobin
method is used by default to distribute the requests evenly across the servers.
Monitoring and Scaling with Load Balancers
Once your application is running with a load balancer, it's important to monitor its performance and scale your infrastructure as needed. Most load balancers, including NGINX and HAProxy, provide tools for monitoring server health, traffic distribution, and overall performance. You can also automate scaling by adding more Node.js instances when traffic increases and removing them when traffic decreases.
Conclusion
Load balancing is essential for ensuring the scalability, reliability, and performance of Node.js applications. By using Node.js' cluster
module or external load balancers like NGINX or HAProxy, you can distribute traffic across multiple instances of your application and handle more requests without overloading your servers. Additionally, load balancers provide fault tolerance by redirecting traffic to healthy instances in case of failures, ensuring high availability for your application.
Logging in Node.js with Winston and Morgan
Logging is a crucial aspect of any application, as it helps you monitor and debug your code. In Node.js, two popular logging libraries are commonly used: Winston and Morgan. Winston is a versatile logging library that allows you to log messages to various transports (e.g., console, files, databases), while Morgan is an HTTP request logger middleware for Node.js that logs requests and responses in your application.
Why Logging is Important
Logging enables you to track application behavior, monitor performance, and diagnose issues in production environments. By logging important events and errors, you can gain insights into how your application is performing and identify potential problems.
Setting Up Winston in Node.js
Winston is a flexible and popular logging library for Node.js that supports different log levels and output formats. It allows you to log messages to multiple destinations such as files, databases, or external services.
Step 1: Install Winston
First, install Winston in your Node.js project:
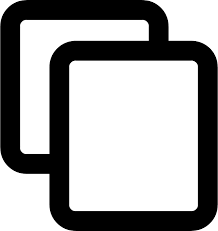
npm install winston
Step 2: Create a Logger with Winston
Now, you can create a logger using Winston. Below is an example of how to configure Winston to log messages to both the console and a log file:
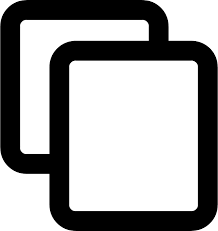
const winston = require('winston');
// Create a logger with multiple transports
const logger = winston.createLogger({
level: 'info', // Set default log level
format: winston.format.simple(), // Log format
transports: [
new winston.transports.Console(), // Log to console
new winston.transports.File({ filename: 'app.log' }) // Log to file
]
});
// Log messages with different severity levels
logger.info('This is an info message');
logger.warn('This is a warning message');
logger.error('This is an error message');
Explanation:
- The
winston.createLogger()
function is used to create a logger with specified configuration options. - The
level
option defines the default log level (e.g.,info
,warn
,error
, etc.). - The
transports
array defines where the log messages will be output. In this case, logs will be written to both the console and a file namedapp.log
. - The
logger.info()
,logger.warn()
, andlogger.error()
methods are used to log messages at different severity levels.
Setting Up Morgan for HTTP Request Logging
While Winston is great for logging general application events, Morgan is used specifically for logging HTTP requests and responses. It provides a simple middleware that you can use to log details of incoming HTTP requests in your application.
Step 1: Install Morgan
Install Morgan in your Node.js project:
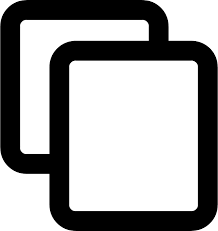
npm install morgan
Step 2: Use Morgan as Middleware
Now you can use Morgan as middleware in your Express application to log HTTP requests. Here is an example:
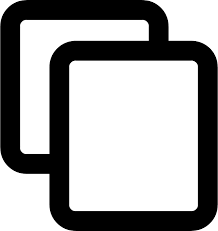
const express = require('express');
const morgan = require('morgan');
const app = express();
// Use Morgan to log HTTP requests
app.use(morgan('combined')); // 'combined' format is commonly used
// Example route
app.get('/', (req, res) => {
res.send('Hello World');
});
// Start the server
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Explanation:
- In this example,
morgan('combined')
logs detailed information about each HTTP request, including the IP address, request method, response status code, and more. - You can choose from several predefined formats (e.g.,
combined
,common
,dev
, etc.), or create a custom format usingmorgan.format()
. - The
app.use(morgan(...))
middleware logs every incoming HTTP request to the console or a file, depending on your configuration.
Combining Winston and Morgan
It’s common to use both Winston and Morgan in a Node.js application: Winston for general logging and Morgan for HTTP request logging. You can even configure Morgan to log to a file using Winston as the transport destination.
Example: Combining Winston and Morgan
In this example, we’ll configure both Winston and Morgan to log HTTP requests to a file using Winston:
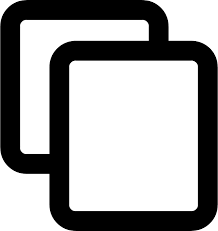
const express = require('express');
const winston = require('winston');
const morgan = require('morgan');
const app = express();
// Create a logger with Winston
const logger = winston.createLogger({
level: 'info',
format: winston.format.simple(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'app.log' })
]
});
// Create a Morgan middleware that logs HTTP requests using Winston
app.use(morgan('combined', {
stream: {
write: (message) => logger.info(message.trim()) // Log HTTP requests to Winston
}
}));
// Example route
app.get('/', (req, res) => {
res.send('Hello World');
});
// Start the server
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Explanation:
- We create a custom stream for Morgan that writes the HTTP request logs to Winston using
logger.info()
. - This setup ensures that both general logs and HTTP request logs are captured by Winston and written to the same log file or console.
Conclusion
Logging is an essential part of application development and maintenance. By using Winston for general logging and Morgan for HTTP request logging, you can ensure that your Node.js application has robust logging capabilities. You can log messages to various destinations, such as the console or files, and easily monitor and debug your application in production environments.
Using Linting Tools (ESLint)
Linting is the process of analyzing code for potential errors, code style issues, and best practice violations. In JavaScript, one of the most popular linting tools is ESLint. ESLint helps you maintain consistent code style, catch common bugs, and enforce coding standards in your project.
Why Use ESLint?
ESLint helps developers by:
- Finding and fixing problems: It can automatically detect syntax errors, potential bugs, and inconsistent formatting in your code.
- Enforcing coding style: You can set specific rules for code formatting, such as indentation, line length, and function definitions, to maintain consistency across your codebase.
- Improving code quality: By following best practices and identifying potential issues, ESLint can help improve the overall quality of your code.
Setting Up ESLint in Node.js
To start using ESLint in your Node.js project, follow these steps:
Step 1: Install ESLint
First, install ESLint as a development dependency in your project:
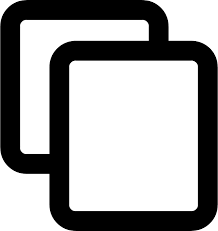
npm install eslint --save-dev
Step 2: Initialize ESLint Configuration
After installing ESLint, initialize it by running the following command:
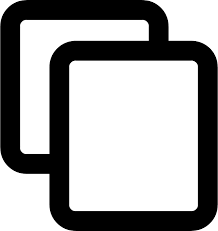
npx eslint --init
This will prompt you to answer a few questions about your coding style and environment, such as:
- What type of project are you working on? (Node.js, React, etc.)
- Do you want to use a popular style guide (e.g., Airbnb, Google)?
- What format do you want your configuration file in? (JavaScript, YAML, JSON)
After answering these questions, ESLint will generate a configuration file (e.g., .eslintrc.json
) in your project directory.
Step 3: Lint Your Code
Now that ESLint is configured, you can use it to lint your JavaScript files. Run the following command to lint a specific file or the entire project:
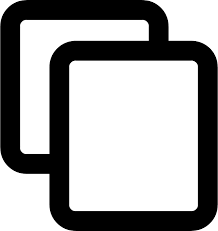
npx eslint yourfile.js
To lint all the files in your project, use:
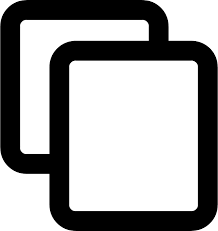
npx eslint .
Common ESLint Rules
ESLint allows you to enforce a wide range of rules to ensure code quality. Here are some common rules:
- no-console: Disallows the use of
console.log()
and other console methods. - indent: Enforces consistent indentation (e.g., spaces or tabs, and the number of spaces per indentation level).
- eqeqeq: Requires the use of strict equality (
===
) rather than loose equality (==
). - semi: Enforces the usage of semicolons at the end of statements.
- quotes: Enforces the use of single or double quotes for string literals.
Example: Custom ESLint Configuration
In your .eslintrc.json
file, you can customize rules like this:
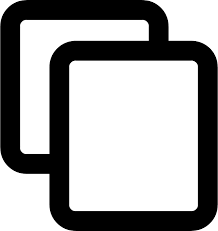
{
"env": {
"node": true,
"es2021": true
},
"extends": [
"eslint:recommended"
],
"rules": {
"no-console": "warn",
"indent": ["error", 2],
"semi": ["error", "always"],
"quotes": ["error", "single"]
}
}
Explanation:
- The
"env"
field specifies the environments your code is expected to run in (e.g., Node.js, ES2021). - The
"extends"
field allows you to use predefined configurations, such as ESLint's recommended settings. - The
"rules"
field customizes specific rules. In this example, theno-console
rule is set to"warn"
, and indentation is set to 2 spaces.
ESLint with Prettier
Prettier is an opinionated code formatter that helps you format your code consistently. You can integrate Prettier with ESLint to automatically format code while linting.
Step 1: Install Prettier
Install Prettier and the necessary ESLint plugin:
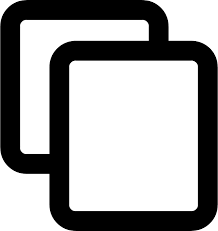
npm install --save-dev prettier eslint-plugin-prettier eslint-config-prettier
Step 2: Configure ESLint to Use Prettier
In your .eslintrc.json
file, add the following configuration to enable Prettier with ESLint:
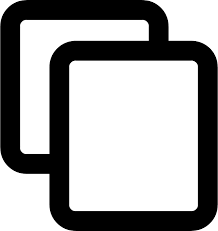
{
"extends": [
"eslint:recommended",
"plugin:prettier/recommended"
]
}
Explanation:
- The
plugin:prettier/recommended
configuration ensures that Prettier's formatting rules are applied and integrated into ESLint, preventing conflicts between the two. - Now, when you run ESLint, Prettier will automatically format your code according to its rules.
Running ESLint Automatically
To automate linting in your project, you can set up ESLint to run as part of your build process. For example, you can use npm scripts to run ESLint when you commit code to your repository:
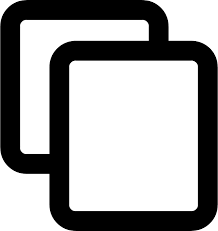
{
"scripts": {
"lint": "eslint .",
"lint:fix": "eslint . --fix"
}
}
Now, you can run npm run lint
to lint your code and npm run lint:fix
to automatically fix issues where possible.
Conclusion
ESLint is an essential tool for ensuring code quality and consistency in JavaScript projects. By using ESLint, you can catch bugs early, enforce coding standards, and maintain a clean and readable codebase. Pairing ESLint with Prettier further streamlines the development process by automatically formatting your code while enforcing best practices.
Writing Unit and Integration Tests
Writing tests is an essential part of software development. It ensures that your code behaves as expected and helps prevent bugs. In Node.js, you can write tests using various testing frameworks and libraries, such as Mocha, Chai, and Jest. This section will cover how to write unit tests and integration tests for your Node.js applications.
Unit Testing
Unit testing is the process of testing individual units or components of a software application in isolation. The goal of unit testing is to validate that each unit of the software performs as expected. In Node.js, Mocha and Jest are commonly used to write unit tests.
Setting Up Mocha and Chai for Unit Testing
To get started with Mocha and Chai, install them as development dependencies:
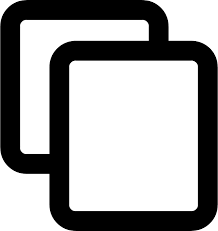
npm install --save-dev mocha chai
Writing a Simple Unit Test
Here’s an example of a simple unit test for a function that adds two numbers:
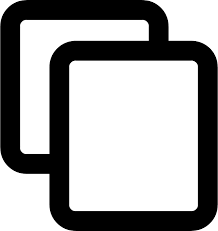
// add.js
function add(a, b) {
return a + b;
}
module.exports = add;
Now, create a test file test/add.test.js
to write the unit test:
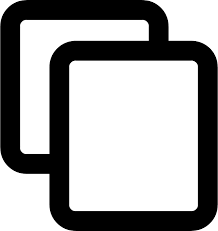
// test/add.test.js
const add = require('../add');
const { expect } = require('chai');
describe('add', () => {
it('should add two numbers correctly', () => {
const result = add(2, 3);
expect(result).to.equal(5);
});
});
To run the unit test, add a test script in your package.json
:
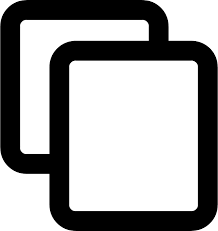
{
"scripts": {
"test": "mocha"
}
}
Now, you can run the test with the following command:
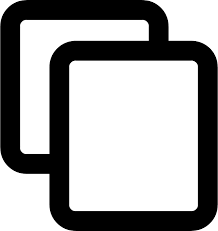
npm test
Integration Testing
Integration testing focuses on testing the interaction between different components or systems in your application. Unlike unit tests, integration tests evaluate how well the various parts of the system work together. In this section, we will demonstrate how to write integration tests for your API endpoints.
Setting Up Supertest for API Testing
Supertest is a popular library for testing HTTP APIs. It works well with Mocha and Chai for integration testing. Install Supertest as a development dependency:
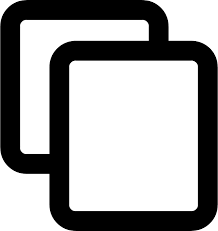
npm install --save-dev supertest
Writing an Integration Test for an API
Let’s write an integration test for a simple Express API. First, create a basic Express app in app.js
:
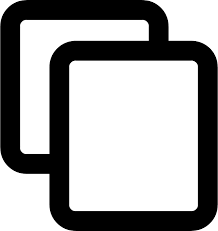
// app.js
const express = require('express');
const app = express();
app.get('/api/greet', (req, res) => {
res.json({ message: 'Hello, world!' });
});
module.exports = app;
Now, create an integration test in test/api.test.js
to test the /api/greet
endpoint:
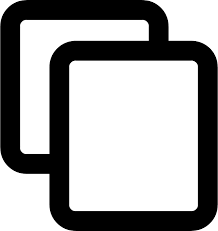
// test/api.test.js
const request = require('supertest');
const app = require('../app');
describe('GET /api/greet', () => {
it('should return a greeting message', async () => {
const res = await request(app).get('/api/greet');
expect(res.status).to.equal(200);
expect(res.body.message).to.equal('Hello, world!');
});
});
To run the integration tests, use the same npm test
command:
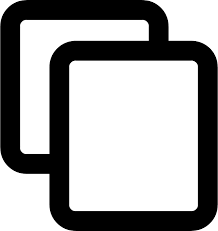
npm test
Best Practices for Writing Tests
Here are some best practices to follow when writing unit and integration tests:
- Keep tests isolated: Each unit test should test a single unit of functionality and should not rely on external systems or components.
- Write clear and descriptive tests: Ensure that your tests describe what is being tested and why, so others can understand the purpose of the test.
- Test edge cases: Make sure to test not only the typical use cases but also edge cases and error handling to ensure robustness.
- Run tests regularly: Include tests as part of your continuous integration (CI) pipeline to catch regressions early.
- Mock external dependencies: Use mocking frameworks like
sinon
to mock external API calls or database queries in unit tests.
Conclusion
Writing unit and integration tests is crucial for maintaining code quality and preventing bugs in your application. With frameworks like Mocha, Chai, and Supertest, testing in Node.js becomes manageable and efficient. By following best practices, you can ensure that your code is reliable and maintainable.
CI/CD with Node.js Projects
Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in modern software development, enabling teams to deliver code updates more frequently and reliably. In this section, we will explore how to set up CI/CD pipelines for Node.js projects using popular tools like GitHub Actions, Travis CI, and CircleCI.
What is CI/CD?
CI/CD stands for Continuous Integration and Continuous Deployment. CI is the practice of merging all developers' working copies to a shared codebase several times a day. CD automates the deployment process, ensuring that every change passes through automated tests and is deployed to the production environment automatically.
Setting Up CI/CD with GitHub Actions
GitHub Actions is a powerful automation tool integrated directly into GitHub. It allows you to define custom workflows for CI/CD, triggering actions based on specific events in your GitHub repository.
Step 1: Create a GitHub Actions Workflow
To get started with GitHub Actions, create a directory called .github/workflows
at the root of your repository. Inside that directory, create a YAML file (e.g., ci.yml
) to define your workflow.
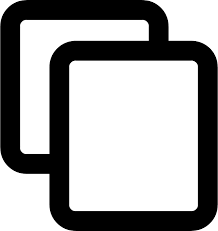
name: Node.js CI
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Build project
run: npm run build
- name: Deploy to production
if: github.ref == 'refs/heads/main'
run: npm run deploy
The ci.yml
file defines a simple CI pipeline that runs on every push or pull request to the main
branch. It checks out the code, sets up Node.js, installs dependencies, runs tests, builds the project, and deploys it to production if the push is to the main branch.
Step 2: Set Up Secrets for Deployment
To securely store secrets like API keys or deployment credentials, use GitHub Secrets. Go to your repository’s settings, navigate to the "Secrets" section, and add secrets such as DEPLOY_KEY
or API_TOKEN
. These will be available for use in your GitHub Actions workflow.
Setting Up CI/CD with Travis CI
Travis CI is another popular CI/CD service that integrates well with GitHub repositories. To use Travis CI, you must create a .travis.yml
file at the root of your repository.
Step 1: Create a .travis.yml File
Here is an example of a basic configuration for a Node.js project in .travis.yml
:
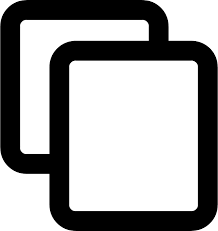
language: node_js
node_js:
- "14"
branches:
only:
- main
before_script:
- npm install
script:
- npm test
- npm run build
deploy:
provider: heroku
api_key:
secure:
app:
The .travis.yml
file defines the Node.js environment, specifies the main
branch, installs dependencies, runs tests, builds the project, and deploys it to Heroku using the secure API key stored in Travis CI’s environment variables.
Step 2: Set Up Deployment Credentials
In Travis CI, you can securely store sensitive data like your Heroku API key by adding it to the repository’s environment variables. Go to your Travis CI project settings and add the HEROKU_API_KEY
as a secret environment variable.
Setting Up CI/CD with CircleCI
CircleCI is another CI/CD service that can be integrated with GitHub repositories. It uses configuration files written in YAML format to define workflows.
Step 1: Create a .circleci/config.yml File
To configure CircleCI for your Node.js project, create a .circleci/config.yml
file in the root of your repository.
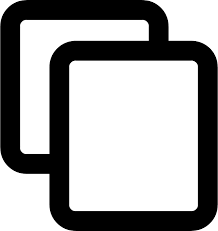
version: 2.1
jobs:
build:
docker:
- image: circleci/node:14
steps:
- checkout
- run:
name: Install dependencies
command: npm install
- run:
name: Run tests
command: npm test
- run:
name: Build project
command: npm run build
workflows:
version: 2
build_and_deploy:
jobs:
- build
filters:
branches:
only: main
This configuration defines a job called build
that runs in a Docker container using the CircleCI Node.js image. The job checks out the code, installs dependencies, runs tests, and builds the project. The workflow triggers only on changes to the main
branch.
Best Practices for CI/CD
- Automate tests: Always run tests as part of your CI pipeline to catch errors early.
- Use environment variables: Store sensitive information like API keys or credentials in environment variables, not in the code.
- Separate build and deploy steps: Keep your build and deployment steps separate to avoid deploying untested code.
- Monitor deployments: Set up monitoring to ensure that deployments are successful and that the application is functioning correctly.
- Use versioning: Tag your deployments to keep track of the changes in production.
Conclusion
CI/CD pipelines are essential for automating testing, building, and deploying your Node.js applications. GitHub Actions, Travis CI, and CircleCI are all great tools for setting up CI/CD workflows. By following best practices, you can ensure that your code is thoroughly tested and deployed to production efficiently and reliably.
Deploying Node.js Applications with Docker
Docker is a powerful platform that simplifies the process of deploying, managing, and scaling applications. With Docker, you can package your Node.js application along with its dependencies into a container, ensuring consistency across development, testing, and production environments. In this section, we will explore how to deploy a Node.js application using Docker.
What is Docker?
Docker is a containerization platform that allows you to package your application and its dependencies into a standardized unit called a container. Containers are lightweight, portable, and can run on any system that has Docker installed, regardless of the underlying environment.
Benefits of Docker for Node.js Applications
- Environment Consistency: Docker ensures that your Node.js application runs the same way in different environments (development, testing, production).
- Isolation: Docker containers isolate your application from the host system and other applications, preventing conflicts.
- Portability: Docker containers can be run on any machine with Docker installed, making it easy to move your Node.js application between environments.
- Scalability: Docker containers can be easily scaled up or down to handle increased traffic.
Setting Up Docker for a Node.js Application
To deploy a Node.js application with Docker, you need to create a Dockerfile
to define how your application will be built and run inside the container. Here are the steps to get started:
Step 1: Create a Dockerfile
In the root directory of your Node.js project, create a file named Dockerfile
. This file defines the instructions for building your Docker image. Here is an example of a simple Dockerfile for a Node.js application:
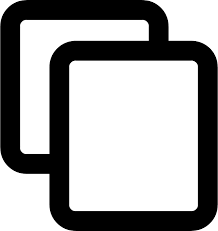
# Step 1: Use an official Node.js image as the base image
FROM node:14
# Step 2: Set the working directory in the container
WORKDIR /usr/src/app
# Step 3: Copy package.json and package-lock.json to the container
COPY package*.json ./
# Step 4: Install dependencies
RUN npm install
# Step 5: Copy the rest of the application files to the container
COPY . .
# Step 6: Expose the port that the app will run on
EXPOSE 3000
# Step 7: Define the command to run the application
CMD ["npm", "start"]
This Dockerfile performs the following steps:
- Uses the official Node.js image as the base.
- Sets the working directory to
/usr/src/app
inside the container. - Copies
package.json
andpackage-lock.json
to the container and installs dependencies. - Copies the rest of the application files to the container.
- Exposes port 3000 (or whichever port your app listens to) for communication with the outside world.
- Specifies the command to run the application using
npm start
.
Step 2: Build the Docker Image
Once you have defined your Dockerfile, you can build the Docker image. In the terminal, navigate to the directory containing the Dockerfile and run the following command:
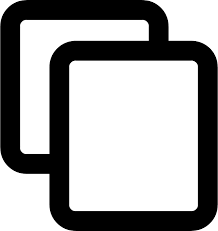
docker build -t my-node-app .
This command tells Docker to build an image named my-node-app
using the current directory (denoted by .
) as the build context. Docker will execute the instructions in the Dockerfile to create the image.
Step 3: Run the Docker Container
After building the Docker image, you can run your Node.js application inside a container. Use the following command to start the container:
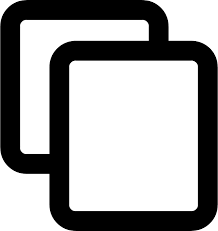
docker run -p 3000:3000 my-node-app
This command runs the my-node-app
image and maps port 3000 on your local machine to port 3000 inside the container. You can now access your Node.js application at http://localhost:3000
.
Deploying the Dockerized Application to the Cloud
Once your application is containerized, you can deploy it to various cloud platforms like AWS, Azure, or Google Cloud. The process involves pushing your Docker image to a container registry (like Docker Hub) and then pulling the image from the registry to run it on your cloud infrastructure.
Step 1: Push the Docker Image to Docker Hub
To push the Docker image to Docker Hub, you first need to log in to your Docker Hub account:
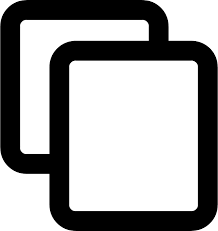
docker login
After logging in, tag your Docker image with your Docker Hub username and push it:
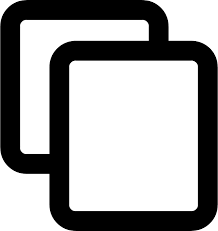
docker tag my-node-app /my-node-app
docker push /my-node-app
Step 2: Pull and Run the Image on a Cloud Server
On your cloud server (AWS, Azure, Google Cloud, etc.), install Docker, log in to Docker Hub, and pull the image:
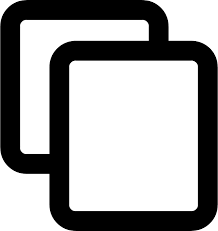
docker pull /my-node-app
docker run -p 80:3000 /my-node-app
This will pull the Docker image from Docker Hub and run the application on port 80, making it accessible to the public.
Scaling the Dockerized Application
Docker makes it easy to scale your Node.js application. You can create multiple containers and use a reverse proxy or load balancer to distribute traffic across them. For example, you can use docker-compose
to define and manage multi-container setups for more complex applications.
Example: Docker Compose
If your Node.js application uses multiple services (e.g., a database), you can define them in a docker-compose.yml
file:
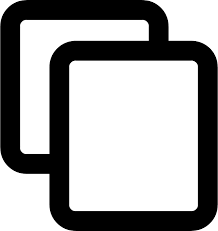
version: '3'
services:
web:
image: /my-node-app
ports:
- "3000:3000"
environment:
- NODE_ENV=production
db:
image: postgres:alpine
environment:
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
ports:
- "5432:5432"
This docker-compose.yml
file defines two services: the web
service (your Node.js application) and the db
service (a PostgreSQL database). You can then start both services with a single command:
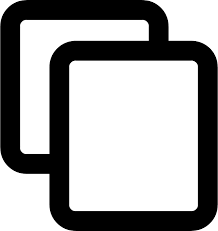
docker-compose up
Conclusion
Docker is an excellent tool for deploying Node.js applications. By containerizing your application, you can ensure consistency across environments, scale your application easily, and deploy it to cloud platforms with minimal effort. Docker also simplifies managing multi-service applications, making it a powerful tool for modern software development.
Deploying to AWS, Heroku, or DigitalOcean
Deploying your Node.js application to a cloud platform allows you to scale your app easily, ensure high availability, and reduce the burden of managing infrastructure. In this section, we will explore how to deploy your Node.js application to three popular cloud platforms: AWS, Heroku, and DigitalOcean.
1. Deploying Node.js to AWS
AWS (Amazon Web Services) provides a wide range of services for deploying and managing applications. To deploy a Node.js application to AWS, you typically use Amazon EC2 (Elastic Compute Cloud) instances or Elastic Beanstalk for easy deployment and scaling. Below, we'll go through the steps for both approaches.
Using EC2
EC2 is a virtual server that you can configure and manage directly. Here's how you can deploy your Node.js app to an EC2 instance:
- Set up an EC2 instance: Go to the AWS Console, navigate to EC2, and launch a new instance. Choose the
Amazon Linux 2
AMI orUbuntu
as the operating system. - SSH into the EC2 instance: Use SSH to connect to your EC2 instance from your local machine:
ssh -i your-key.pem ec2-user@your-ec2-public-dns
- Install Node.js: On the EC2 instance, install Node.js and npm:
sudo yum update -y sudo yum install -y nodejs
- Upload your app: You can use SCP or any other method to upload your Node.js application files to the EC2 instance.
- Install dependencies: On the EC2 instance, navigate to your app directory and run:
npm install
- Run the app: Start your Node.js application:
node app.js
- Configure security groups: In the AWS Console, ensure that the security group for the EC2 instance allows inbound traffic on the port your app is listening to (e.g., port 3000).
Using Elastic Beanstalk
Elastic Beanstalk is a managed service that automatically handles the deployment, scaling, and management of your Node.js application. Here's how to deploy with Elastic Beanstalk:
- Install AWS CLI: Install and configure the AWS CLI on your local machine.
- Create an Elastic Beanstalk environment: Use the following command to create an environment for your Node.js app:
eb init eb create my-node-app-env
- Deploy the app: Once the environment is created, deploy your Node.js app with:
eb deploy
- Access the app: After deployment, you can access your app via the URL provided by Elastic Beanstalk.
2. Deploying Node.js to Heroku
Heroku is a Platform-as-a-Service (PaaS) that simplifies the deployment process. It abstracts away much of the infrastructure management, making it ideal for quick deployments. Here's how to deploy your Node.js app to Heroku:
- Create a Heroku account: If you don't have one, sign up at Heroku.
- Install Heroku CLI: Download and install the Heroku CLI from the official website.
- Login to Heroku: Log in to your Heroku account using the command:
heroku login
- Create a Heroku app: Create a new app on Heroku:
heroku create my-node-app
- Deploy your app: Push your code to Heroku using Git:
git push heroku master
- Access the app: After deployment, Heroku will provide you with a URL to access your application.
3. Deploying Node.js to DigitalOcean
DigitalOcean is a cloud service provider that offers affordable virtual machines (called droplets). It's ideal for developers who want full control over their infrastructure. Here's how to deploy your Node.js app to a DigitalOcean droplet:
- Create a DigitalOcean account: Sign up at DigitalOcean.
- Create a Droplet: In the DigitalOcean dashboard, create a new droplet with a Linux distribution (e.g., Ubuntu).
- SSH into the Droplet: Use SSH to connect to your droplet:
ssh root@your-droplet-ip
- Install Node.js: On your droplet, install Node.js and npm:
sudo apt update sudo apt install nodejs npm
- Upload your app: Use SCP or Git to upload your Node.js app to the droplet.
- Install dependencies: Run
npm install
to install the app's dependencies. - Run the app: Start your app on the droplet:
node app.js
- Configure firewall: Ensure that the firewall allows inbound traffic on the port your app is listening to (e.g., port 3000).
Conclusion
Each cloud platform provides different benefits and trade-offs. AWS offers a wide variety of services and scalability, Heroku simplifies deployment with minimal setup, and DigitalOcean offers affordable and flexible virtual machines. Choose the platform that best suits your needs and deploy your Node.js application to the cloud to ensure it's scalable, secure, and always available.
Using PM2 for Process Management
PM2 is a popular process manager for Node.js applications. It helps manage the lifecycle of applications, making it easier to run, monitor, and keep your application alive forever. With PM2, you can easily deploy, monitor, and manage applications in a production environment, ensuring high availability and easy process management.
What is PM2?
PM2 is a production-grade process manager for Node.js applications. It is particularly useful for managing Node.js apps in production, as it offers features like automatic restarts, log management, and cluster mode, among others.
Installing PM2
To install PM2 globally, you can use npm. Run the following command in your terminal:
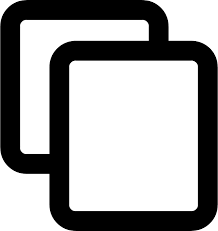
npm install -g pm2
Starting an Application with PM2
Once PM2 is installed, you can use it to start your Node.js application. To start your app, navigate to the directory containing your app.js
file and run the following command:
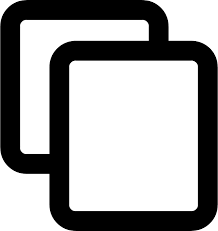
pm2 start app.js
PM2 will start your application and keep it running in the background. If your application crashes, PM2 will automatically restart it.
Running Multiple Instances with PM2
PM2 supports cluster mode, which allows you to run multiple instances of your application to take advantage of multi-core systems. To run your application in cluster mode, use the following command:
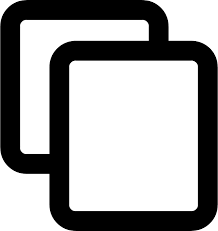
pm2 start app.js -i max
The -i max
option tells PM2 to start as many instances as the number of available CPU cores on your system. This helps in load balancing across multiple processes.
Managing Processes with PM2
PM2 provides several useful commands to manage and monitor your processes:
- List running processes: To view the list of applications being managed by PM2, use:
pm2 list
- Stop a process: To stop a specific process, use:
pm2 stop app.js
- Restart a process: To restart a process, use:
pm2 restart app.js
- Delete a process: To delete a process from PM2, use:
pm2 delete app.js
Monitoring Your Application with PM2
PM2 provides real-time monitoring of your application’s performance, including CPU and memory usage. To monitor your app, use:
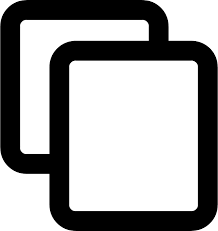
pm2 monit
This will open a real-time dashboard that shows various metrics of your running processes, helping you keep an eye on their performance.
Saving the PM2 Process List
If you want PM2 to automatically start your application when the server reboots, you can save the current process list with the following command:
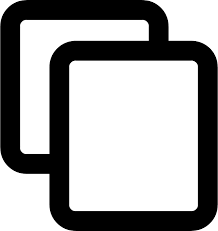
pm2 save
This will save your application’s current state, and you can restore it after a reboot by running:
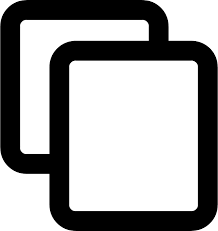
pm2 resurrect
Setting Up PM2 to Start on Boot
To ensure that PM2 automatically starts when the server boots, you can use the following command to generate a startup script for your system:
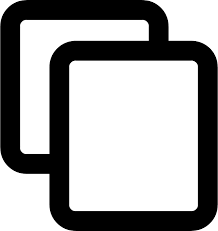
pm2 startup
This command will display instructions specific to your system (e.g., for Ubuntu, it generates a systemd service). Follow the instructions to complete the setup.
Log Management with PM2
PM2 also provides built-in logging functionality, making it easy to view the logs of your application. You can view the logs using:
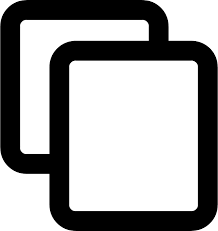
pm2 logs
If you want to view logs for a specific application, use:
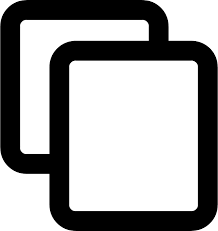
pm2 logs app.js
Conclusion
PM2 is an essential tool for managing Node.js applications in production. It simplifies the process of keeping your applications alive, provides real-time monitoring, and makes it easy to scale your app across multiple instances. With PM2, you can ensure that your application runs smoothly with minimal downtime and hassle.
Horizontal and Vertical Scaling of Node.js Apps
Scaling your Node.js application is crucial for handling increased traffic and ensuring high availability. There are two primary methods of scaling: horizontal scaling and vertical scaling. Both approaches have their use cases and benefits. Understanding the differences and how to implement them effectively is key to building a scalable Node.js application.
What is Vertical Scaling?
Vertical scaling (also known as "scaling up") involves increasing the resources (such as CPU, RAM, or storage) of a single server or instance to handle more load. It's the simplest way to scale an application, as it only requires upgrading the server's hardware or increasing the allocated resources in a cloud environment.
Advantages of Vertical Scaling
- Simple to Implement: Increasing the server's resources can often be done with minimal changes to your application.
- Cost-Effective for Small Applications: For smaller applications with moderate load, vertical scaling can be sufficient and more cost-effective.
- Less Complexity: Vertical scaling generally requires fewer changes to infrastructure and application architecture.
Limitations of Vertical Scaling
- Single Point of Failure: If the server goes down, the entire application becomes unavailable.
- Resource Limits: There are physical limits to how much you can scale a single server.
- Higher Costs Over Time: As your app grows, the cost of continually upgrading hardware may become inefficient.
What is Horizontal Scaling?
Horizontal scaling (also known as "scaling out") involves adding more instances (or servers) of your application to distribute the load. This approach helps ensure better availability and fault tolerance, as multiple instances can handle requests in parallel. Horizontal scaling is more suited to large, distributed systems and cloud environments.
Advantages of Horizontal Scaling
- Better Fault Tolerance: If one instance goes down, other instances can continue to serve traffic, ensuring high availability.
- Scalable with Traffic: You can easily add more servers to handle increased traffic.
- Cost-Effective for Large Applications: Horizontal scaling is often more cost-effective than continually upgrading hardware for vertical scaling.
Limitations of Horizontal Scaling
- Increased Complexity: Managing multiple instances requires sophisticated load balancing, distributed storage, and state synchronization across servers.
- Session Management: When scaling horizontally, managing user sessions across multiple instances can become tricky (use of sticky sessions or a shared session store like Redis is needed).
- Networking Overhead: More instances mean more network communication, which can introduce latency or additional complexity for managing traffic.
Implementing Vertical Scaling in Node.js
Vertical scaling in Node.js is relatively straightforward, as it typically involves upgrading your server’s resources. If you’re using cloud services like AWS, Google Cloud, or DigitalOcean, scaling vertically is often as simple as selecting a higher-tier instance type.
Here are a few steps to implement vertical scaling:
- Monitor your application’s resource usage (CPU, RAM, etc.) to identify performance bottlenecks.
- Upgrade the server’s hardware or increase the resource allocation (e.g., RAM or CPU) from your hosting provider.
- Ensure that your application can handle the increased resources efficiently, such as optimizing memory usage in Node.js.
Vertical scaling is particularly useful when your Node.js application requires more CPU or memory to handle large-scale computations, heavy data processing, or database queries.
Implementing Horizontal Scaling in Node.js
Horizontal scaling requires adding more instances of your application and balancing the load between them. This typically involves using a load balancer and ensuring your application is stateless or shares state across instances.
Step 1: Use a Load Balancer
A load balancer sits between clients and your application servers, distributing incoming traffic evenly across multiple instances. Popular load balancers include:
- NGINX: A high-performance web server and reverse proxy that can be configured to load balance HTTP requests.
- HAProxy: A widely used, open-source load balancer that supports TCP and HTTP load balancing.
- Cloud Load Balancers: Cloud providers like AWS and Google Cloud offer load balancing services that automatically distribute traffic across multiple instances.
Step 2: Use Sticky Sessions or Shared Session Store
When scaling horizontally, maintaining user sessions can be challenging because each new instance is stateless by default. There are two primary ways to handle sessions in a distributed environment:
- Sticky Sessions: This approach ensures that a client always connects to the same instance by assigning a session cookie. However, this solution limits scalability.
- Shared Session Store: A more scalable solution involves storing sessions in a shared data store like Redis or a database, so all instances can access the same session data.
Step 3: Cluster Your Node.js Application
Node.js is single-threaded, so it can only utilize one CPU core by default. To take advantage of multi-core systems, you can use Node.js’s cluster module to fork multiple processes (workers) that share the same server port.
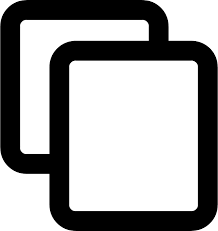
const cluster = require('cluster');
const http = require('http');
const os = require('os');
if (cluster.isMaster) {
const numCPUs = os.cpus().length;
// Fork workers
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
});
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!');
}).listen(8000);
}
Using the cluster
module, you can take advantage of all CPU cores and scale your app horizontally across multiple processes, improving performance and fault tolerance.
Scaling in the Cloud
When deploying to cloud platforms like AWS, Heroku, or Google Cloud, both vertical and horizontal scaling can be achieved with ease. Most cloud providers offer managed services and auto-scaling options to help scale your Node.js applications without much manual intervention.
AWS Auto Scaling
AWS provides an Auto Scaling feature that automatically adjusts the number of EC2 instances based on traffic. With Elastic Load Balancing (ELB), you can seamlessly distribute traffic across multiple instances. You can set policies to scale in or out based on CPU usage, memory, or other metrics.
Heroku Scaling
In Heroku, you can scale your application by adding more dynos (containers running your app). You can scale vertically by increasing the resources allocated to a dyno or horizontally by adding more dynos.
Conclusion
Scaling a Node.js application effectively involves understanding the differences between vertical and horizontal scaling and choosing the appropriate method based on your application's needs. While vertical scaling is easier to implement, horizontal scaling offers better fault tolerance, scalability, and high availability for large applications. By combining both approaches, you can build a robust, scalable Node.js application capable of handling high traffic loads efficiently.
Streams and Stream-Based Processing in Node.js
Streams are a powerful and efficient way to handle large amounts of data in Node.js. Rather than loading an entire dataset into memory, streams allow you to process data in chunks as it is read or written. This is particularly useful for handling I/O operations like reading files, making HTTP requests, or communicating over networks, without putting too much load on memory.
What are Streams?
In Node.js, a stream is an abstract interface for working with streaming data. Streams can be classified into four types based on how data flows:
- Readable Streams: These are streams from which data can be read. For example, reading data from a file or HTTP request body.
- Writable Streams: These streams allow you to write data. For example, writing data to a file or an HTTP response.
- Duplex Streams: These streams are both readable and writable. For example, a TCP socket allows both reading and writing data.
- Transform Streams: A special type of duplex stream where the data is modified as it is read and written. For example, a compression stream that compresses data as it’s written and decompresses it as it’s read.
Why Use Streams?
Streams provide significant benefits, especially when handling large amounts of data:
- Memory Efficiency: Streams allow you to process data without having to load the entire dataset into memory, which is useful for large files or data streams.
- Non-blocking: Streams are asynchronous, allowing your application to continue processing other tasks while the data is being read or written.
- Performance: Since data is processed in smaller chunks, streams can be faster and more efficient than loading and processing large amounts of data all at once.
Working with Streams in Node.js
Node.js provides a simple API for working with streams. Here are some common operations for using streams:
1. Reading from a Readable Stream
To read data from a stream, you can use the stream.Readable
API. The most common example is reading data from a file using the fs.createReadStream()
method.
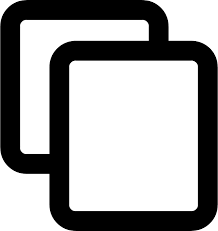
const fs = require('fs');
const readableStream = fs.createReadStream('largeFile.txt');
readableStream.on('data', (chunk) => {
console.log('Received chunk:', chunk);
});
readableStream.on('end', () => {
console.log('Finished reading the file');
});
In this example, the data
event is emitted whenever a chunk of data is available to read. The end
event signifies that the stream has been fully read.
2. Writing to a Writable Stream
Writable streams allow you to write data to a destination. For example, you can use fs.createWriteStream()
to write data to a file.
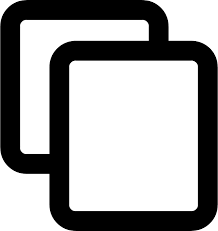
const fs = require('fs');
const writableStream = fs.createWriteStream('output.txt');
writableStream.write('Hello, this is a stream write example!\n');
writableStream.write('Streams are efficient for large data.\n');
writableStream.end(() => {
console.log('Finished writing to the file');
});
Here, data is written to the output.txt
file. The end
method is called when the writing is complete.
3. Piping Data Between Streams
One of the most powerful features of streams in Node.js is the ability to pipe data from one stream to another. This is commonly used to read from a readable stream and write to a writable stream, such as reading from a file and writing to another file.
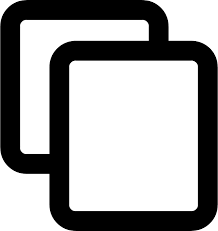
const fs = require('fs');
const readableStream = fs.createReadStream('input.txt');
const writableStream = fs.createWriteStream('output.txt');
readableStream.pipe(writableStream);
writableStream.on('finish', () => {
console.log('Data piped successfully!');
});
The pipe()
method automatically handles the flow of data from the readable stream to the writable stream, making it easier to manage the data flow.
4. Transforming Data with Transform Streams
Transform streams allow you to modify data as it is passed through. For example, you could use a transform stream to compress or encrypt data on the fly.
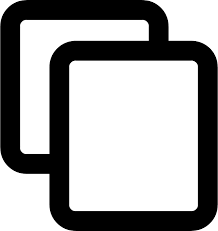
const { Transform } = require('stream');
const uppercaseStream = new Transform({
transform(chunk, encoding, callback) {
this.push(chunk.toString().toUpperCase());
callback();
}
});
process.stdin.pipe(uppercaseStream).pipe(process.stdout);
In this example, the transform stream converts all input data to uppercase before writing it to the standard output. The transform()
method is where the data transformation takes place.
Stream-Based Processing for Large Files
Streams are particularly useful when working with large files. Instead of loading the entire file into memory, streams allow you to process smaller chunks of the file at a time. This is ideal for scenarios like processing large log files or streaming video or audio content.
For example, you can use streams to process a large CSV file line by line, transforming or aggregating the data as it is read.
Handling Errors in Streams
Streams can encounter errors during their lifecycle. It is important to handle errors to prevent your application from crashing.
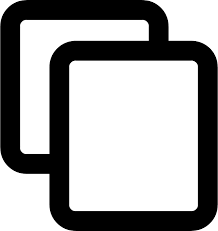
const fs = require('fs');
const readableStream = fs.createReadStream('largeFile.txt');
readableStream.on('data', (chunk) => {
console.log('Processing chunk:', chunk);
});
readableStream.on('error', (err) => {
console.error('Error reading the file:', err);
});
In this example, the error
event is used to handle any issues that arise while reading the file.
Conclusion
Streams are an essential feature of Node.js, offering an efficient and scalable way to handle large datasets and I/O-bound tasks. By processing data in chunks, streams minimize memory usage and provide non-blocking, asynchronous operations. Understanding how to use streams effectively can significantly enhance your ability to build performant applications, especially when dealing with large files or real-time data.
Building Command-Line Interfaces (CLIs) with Node.js
Command-Line Interfaces (CLIs) allow users to interact with an application via text-based commands. Building a CLI with Node.js is a great way to provide a simple interface for automating tasks, managing resources, or interacting with APIs directly from the terminal. Node.js provides tools and libraries to quickly build powerful CLIs that are efficient and easy to use.
Why Build CLIs with Node.js?
Node.js is an excellent choice for building CLIs because it is lightweight, fast, and runs on multiple platforms. With its non-blocking asynchronous nature, Node.js can handle tasks such as file manipulation, API requests, and database queries effectively. Additionally, Node.js has a rich ecosystem of libraries that make building CLIs faster and more flexible.
Setting Up a Simple CLI
To build a basic CLI, you can use the native process.argv
API or leverage a third-party module like yargs
or commander
to handle command-line arguments and options.
1. Using Process.argv
The process.argv
array provides access to the command-line arguments passed to your script. The first two elements are reserved, and the rest are the arguments you provide. Here's a simple example:
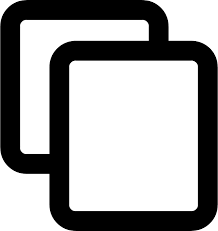
// cli.js
const args = process.argv.slice(2);
if (args.length > 0) {
console.log('Arguments:', args);
} else {
console.log('No arguments passed');
}
Run this script using the following command:
node cli.js hello world
This script will log the arguments passed to it. In this case, ['hello', 'world']
will be printed to the console.
2. Using Yargs for Argument Parsing
While process.argv
is useful for basic CLI functionality, libraries like yargs
provide a more user-friendly interface for parsing complex command-line arguments. Yargs can also generate help messages and handle flags and options.
First, install yargs
:
npm install yargs
Then, use it to enhance your CLI:
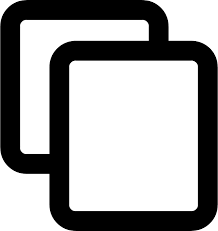
const yargs = require('yargs');
const argv = yargs
.command('greet ', 'Greet a person by name', (yargs) => {
yargs.positional('name', {
describe: 'Name of the person to greet',
type: 'string'
});
})
.option('uppercase', {
alias: 'u',
describe: 'Convert the greeting to uppercase',
type: 'boolean',
default: false
})
.help()
.argv;
if (argv._.includes('greet')) {
let greeting = `Hello, ${argv.name}!`;
if (argv.uppercase) {
greeting = greeting.toUpperCase();
}
console.log(greeting);
}
Now, you can run the following commands:
node cli.js greet John
To add the uppercase option:
node cli.js greet John --uppercase
This will greet John, and the second command will output the greeting in uppercase.
Advanced CLI Features
As you build more complex CLIs, you may want to integrate advanced features, such as:
1. Parsing Multiple Commands
CLIs can have multiple commands, each with its own options. Yargs allows you to define multiple commands, and each command can have its own parameters and behavior. Here's an example of a CLI with two commands: greet
and sum
:
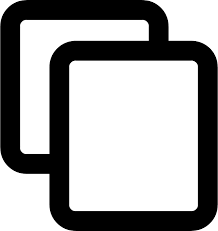
const yargs = require('yargs');
yargs
.command('greet ', 'Greet a person by name', (yargs) => {
yargs.positional('name', {
describe: 'Name of the person to greet',
type: 'string'
});
}, (argv) => {
console.log(`Hello, ${argv.name}!`);
})
.command('sum ', 'Calculate the sum of two numbers', (yargs) => {
yargs.positional('num1', {
describe: 'First number',
type: 'number'
});
yargs.positional('num2', {
describe: 'Second number',
type: 'number'
});
}, (argv) => {
const sum = argv.num1 + argv.num2;
console.log(`The sum is: ${sum}`);
})
.help()
.argv;
Run the commands like so:
node cli.js greet John
node cli.js sum 4 5
This CLI now supports both greet
and sum
commands, each with its own functionality.
2. Handling Asynchronous Operations
Sometimes, CLI operations involve asynchronous tasks, such as API calls, file operations, or database queries. Node.js allows you to handle asynchronous tasks with Promises
or async/await
.
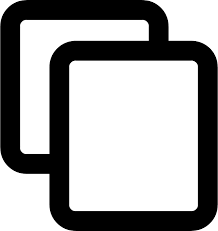
const yargs = require('yargs');
const fs = require('fs').promises;
yargs.command('read-file ', 'Read a file asynchronously', async (yargs) => {
yargs.positional('filename', {
describe: 'Name of the file to read',
type: 'string'
});
}, async (argv) => {
try {
const data = await fs.readFile(argv.filename, 'utf8');
console.log('File content:', data);
} catch (err) {
console.error('Error reading file:', err);
}
})
.help()
.argv;
In this example, the CLI reads a file asynchronously using async/await
and the fs.promises
API.
Distributing CLIs
Once your CLI is ready, you can distribute it as an executable tool. To do this:
- Make your script executable: Add a shebang line at the top of your CLI script to specify the Node.js interpreter. Example:
#!/usr/bin/env node
npm install -g
.commander
and inquirer
can enhance the CLI experience by adding features like interactivity and better argument parsing.Conclusion
Building a CLI with Node.js is a powerful way to automate tasks, interact with systems, and provide tools for end-users. By using libraries like yargs
, you can easily manage command-line arguments, handle multiple commands, and add advanced features like asynchronous operations. Whether you’re building a tool for personal use or distributing it to others, Node.js provides everything you need to create efficient and powerful command-line applications.
Implementing Worker Threads
Worker Threads in Node.js allow developers to perform CPU-intensive operations in parallel, without blocking the main thread. This is especially useful when building applications that require high-performance computing, such as data processing, image manipulation, or any application that involves time-consuming calculations. The Worker Threads module enables the creation of multiple threads that can run in the background, offloading computationally expensive tasks from the event loop.
Why Use Worker Threads?
Node.js is single-threaded by default, which means it can only execute one operation at a time. While Node.js handles I/O operations asynchronously, CPU-bound tasks can still block the event loop, leading to performance bottlenecks. Worker threads allow you to offload computationally expensive tasks to separate threads, enabling the main thread to continue handling I/O operations without interruption.
Setting Up Worker Threads
Worker threads are part of the worker_threads
module in Node.js. To implement worker threads, you need to import the module and create new worker instances.
1. Basic Worker Thread Example
To start using worker threads, you need to import the worker_threads
module. Here’s how to create a simple worker thread that performs a heavy task.
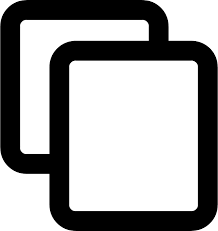
// main.js
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
// Main thread
console.log('Main thread starting worker...');
const worker = new Worker(__filename); // Spawn a new worker using the current script
worker.on('message', (message) => {
console.log('Received from worker:', message);
});
worker.on('error', (err) => {
console.error('Worker error:', err);
});
worker.on('exit', (code) => {
if (code !== 0) {
console.error(`Worker stopped with exit code ${code}`);
}
});
} else {
// Worker thread
console.log('Worker thread is running...');
parentPort.postMessage('Hello from worker!');
}
In this example:
isMainThread
is used to check if the current thread is the main thread.- If the current thread is the main thread, a new worker is created using
new Worker(__filename)
, which runs the same file in a separate thread. - The worker sends a message back to the main thread using
parentPort.postMessage()
.
2. Worker Threads with Data Processing
Worker threads are ideal for offloading data processing tasks. Here’s an example where a worker performs a computationally expensive operation, such as calculating a Fibonacci sequence:
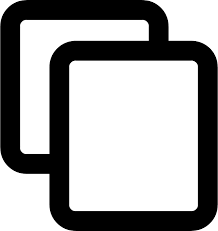
// main.js
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
// Main thread
const worker = new Worker('./worker.js');
worker.on('message', (result) => {
console.log('Fibonacci result:', result);
});
worker.on('error', (err) => {
console.error('Worker error:', err);
});
worker.on('exit', (code) => {
if (code !== 0) {
console.error(`Worker stopped with exit code ${code}`);
}
});
} else {
// worker.js
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
const result = fibonacci(40); // Compute Fibonacci for 40
parentPort.postMessage(result);
}
In this example, the main thread spawns a worker that calculates a Fibonacci number and sends the result back to the main thread. The Fibonacci function is computationally expensive, and using a worker thread ensures the main thread remains unblocked.
Handling Data Between Threads
Worker threads communicate with the main thread using the postMessage()
and on('message')
events. Data can be passed between threads in the form of messages. When passing large amounts of data, you should consider using SharedArrayBuffer
and Atomics
to minimize memory overhead.
Shared Memory Example
Shared memory allows multiple threads to access the same memory area. This is useful when you need to share data between the main thread and workers without copying it. Here's an example using SharedArrayBuffer
:
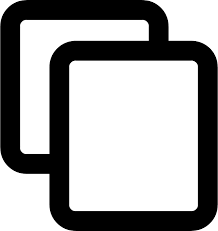
// main.js
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
const buffer = new SharedArrayBuffer(1024); // Create a shared memory buffer
const worker = new Worker('./worker.js', { workerData: buffer });
worker.on('message', () => {
console.log('Shared memory updated');
});
} else {
const buffer = workerData;
const uint32Array = new Uint32Array(buffer);
Atomics.add(uint32Array, 0, 1); // Increment the value in shared memory
parentPort.postMessage('Shared memory updated');
}
In this example, the main thread creates a SharedArrayBuffer
, which is shared with the worker. The worker updates the shared memory using Atomics.add()
to increment the value at index 0 of the shared array.
Handling Errors in Worker Threads
When working with worker threads, errors can occur in both the worker and the main thread. These errors can be handled using the error
event listener for workers, as shown in the previous examples. Additionally, workers can throw exceptions, which can be caught by the parent thread:
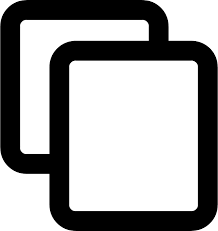
// worker.js
const { parentPort } = require('worker_threads');
try {
throw new Error('Something went wrong in the worker!');
} catch (err) {
parentPort.postMessage({ error: err.message });
}
In this example, the worker throws an error, and the main thread receives the error message in the message
event.
Worker Thread Performance Considerations
While worker threads provide parallel processing, there are some important performance considerations:
- Overhead: Creating and managing workers introduces some overhead. If the tasks are too small or quick, the overhead of managing workers may outweigh the benefits of parallelism.
- Inter-thread Communication: Passing large amounts of data between threads can be slow. Minimizing communication between threads can improve performance.
- Concurrency: Node.js’s worker threads allow for parallelism, but there’s a limit to how many workers can run simultaneously depending on system resources. It's important to manage the number of workers and distribute tasks appropriately.
Conclusion
Worker threads in Node.js enable you to offload CPU-intensive tasks to parallel threads, preventing the main thread from being blocked. By using worker threads, you can significantly improve the performance of your Node.js applications when handling computationally expensive operations. However, it's important to be mindful of the overhead and performance considerations when using workers. For complex applications that require parallelism, worker threads are a powerful tool for achieving high efficiency in Node.js.
Using Event Emitters
Event Emitters are a core feature in Node.js, providing a way to handle asynchronous events. Event-driven programming is central to Node.js, and the EventEmitter class in the events
module allows you to create custom events in your application. You can emit events and listen for them, enabling you to decouple different parts of your program and make it more modular and maintainable.
What is an Event Emitter?
An EventEmitter is an object that allows you to emit named events and attach listeners to those events. When an event is emitted, all listeners attached to that event are invoked. This model is especially useful for handling asynchronous operations like I/O, where you can emit events when certain tasks are completed, such as when a file is read or an HTTP request is finished.
Using the EventEmitter Class
To use EventEmitters in Node.js, you first need to import the events
module. Then, you can create an instance of the EventEmitter
class and use its methods to emit and listen for events.
1. Basic EventEmitter Example
Here is an example of creating a simple EventEmitter that emits an event and listens for it:
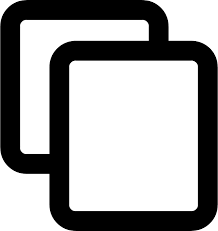
// eventEmitterExample.js
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
// Listener for the 'event' event
myEmitter.on('event', () => {
console.log('An event occurred!');
});
// Emitting the 'event'
myEmitter.emit('event');
In this example:
EventEmitter
is required from theevents
module.- A custom emitter class
MyEmitter
is created by extending theEventEmitter
class. - The
on()
method is used to register a listener for theevent
event. - The
emit()
method is used to trigger theevent
event, which calls the listener and logs a message.
2. Passing Arguments to Event Listeners
You can pass arguments to event listeners when emitting events. These arguments will be passed to the listener function:
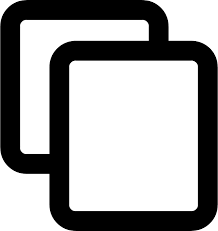
// eventEmitterWithArguments.js
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
// Listener for the 'greet' event
myEmitter.on('greet', (name) => {
console.log(`Hello, ${name}!`);
});
// Emitting the 'greet' event with an argument
myEmitter.emit('greet', 'Alice');
In this case, we emit the greet
event with an argument 'Alice'
, which is passed to the listener function and used in the log statement.
3. One-Time Event Listeners
You can also create a listener that is executed only once, by using the once()
method. After the listener is triggered, it will be removed automatically:
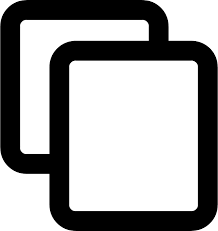
// eventEmitterOnce.js
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
// One-time listener for the 'onceEvent' event
myEmitter.once('onceEvent', () => {
console.log('This will run only once!');
});
// Emitting the 'onceEvent' event twice
myEmitter.emit('onceEvent');
myEmitter.emit('onceEvent'); // This will not trigger the listener again
In this example, the listener for the onceEvent
event is triggered only the first time the event is emitted. Subsequent calls to emit()
do not invoke the listener.
EventEmitter Methods
Here are some key methods provided by the EventEmitter
class:
on(eventName, listener)
: Adds a listener function to the specified event.once(eventName, listener)
: Adds a one-time listener function to the specified event.emit(eventName, [...args])
: Emits an event and passes arguments to the listeners.removeListener(eventName, listener)
: Removes a listener from an event.removeAllListeners(eventName)
: Removes all listeners for a specific event.listeners(eventName)
: Returns an array of listeners for the specified event.eventNames()
: Returns an array of all event names that have listeners.
4. Removing Event Listeners
If you want to remove a specific listener from an event, you can use the removeListener()
method:
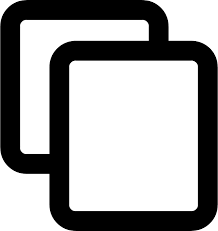
// eventEmitterRemoveListener.js
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
const greetListener = (name) => {
console.log(`Hello, ${name}!`);
};
// Adding a listener
myEmitter.on('greet', greetListener);
// Removing the listener
myEmitter.removeListener('greet', greetListener);
// Emitting the event (no listener will be triggered)
myEmitter.emit('greet', 'Alice');
In this example, we add a listener for the greet
event, then remove it using removeListener()
. When the event is emitted again, the listener is not triggered.
Handling Multiple Listeners for the Same Event
EventEmitter allows you to attach multiple listeners for the same event. All listeners will be called in the order they were added:
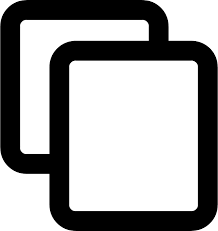
// eventEmitterMultipleListeners.js
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
// Adding multiple listeners for the same event
myEmitter.on('event', () => {
console.log('Listener 1');
});
myEmitter.on('event', () => {
console.log('Listener 2');
});
// Emitting the 'event'
myEmitter.emit('event');
In this example, both listeners for the event
event are called in the order they were added.
Event Emitters in Built-in Node.js Modules
Many built-in modules in Node.js, such as http
and fs
, are EventEmitters. This means they allow you to listen for events such as receiving an HTTP request or reading a file. Here’s an example using the http
module:
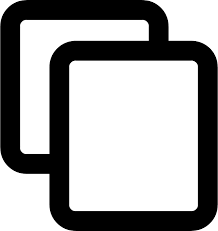
// httpServer.js
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.on('connection', () => {
console.log('A new connection was made!');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example, the http.createServer()
method returns an EventEmitter, and we listen for the connection
event to log when a new connection is made to the server.
Conclusion
Event Emitters are a powerful tool in Node.js for handling asynchronous events. By using custom events, you can decouple different parts of your application, making it more modular and easier to maintain. EventEmitters help in managing I/O-bound tasks, asynchronous operations, and even inter-process communication in a clean and efficient manner. Understanding how to work with Event Emitters is essential for building scalable and responsive Node.js applications.
Consuming REST APIs with Axios and node-fetch
When working with Node.js, it's common to interact with external REST APIs to fetch data or perform other actions. Two popular libraries for making HTTP requests in Node.js are axios
and node-fetch
. These libraries provide a simple interface for consuming REST APIs, handling JSON data, and dealing with asynchronous requests.
What is axios?
axios
is a promise-based HTTP client for both the browser and Node.js. It simplifies making HTTP requests, handling responses, and managing errors. axios supports features like request/response interception, automatic JSON parsing, and more.
What is node-fetch?
node-fetch
is a lightweight module that brings the native fetch
API to Node.js. It provides a simple and flexible way to make HTTP requests using the same syntax as the browser's fetch API. It's ideal for developers already familiar with the fetch API in the browser.
1. Installing axios and node-fetch
To get started, you need to install these libraries in your project:
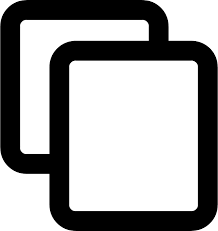
npm install axios
npm install node-fetch
2. Making GET Requests with axios
Let's start by using axios
to make a simple GET request to a REST API:
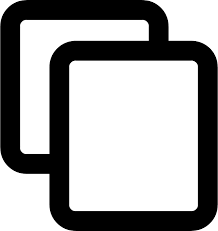
// axios-get.js
const axios = require('axios');
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log(response.data); // Logging the response data
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example:
axios.get(url)
is used to send a GET request to the specified URL.- The
then()
method handles a successful response, logging the data to the console. - The
catch()
method catches any errors during the request and logs them.
3. Making POST Requests with axios
You can also make POST requests with axios
. Here's an example of how to send data to an API:
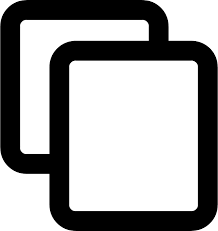
// axios-post.js
const axios = require('axios');
const postData = {
title: 'foo',
body: 'bar',
userId: 1
};
axios.post('https://jsonplaceholder.typicode.com/posts', postData)
.then(response => {
console.log(response.data); // Logging the response data
})
.catch(error => {
console.error('Error posting data:', error);
});
In this example:
axios.post(url, data)
sends a POST request to the specified URL with thepostData
object as the request body.- The response from the API is logged to the console.
4. Making GET Requests with node-fetch
Now, let's look at how you can use node-fetch
to make a GET request:
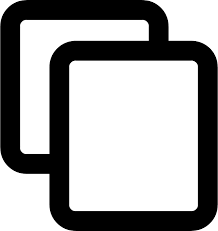
// node-fetch-get.js
const fetch = require('node-fetch');
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json()) // Parsing JSON response
.then(data => {
console.log(data); // Logging the data
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example:
fetch(url)
sends a GET request to the specified URL.response.json()
is used to parse the JSON response from the API.- The parsed data is logged to the console.
5. Making POST Requests with node-fetch
Similarly, you can use node-fetch
to send a POST request. Here's how:
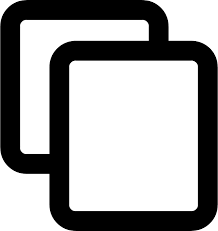
// node-fetch-post.js
const fetch = require('node-fetch');
const postData = {
title: 'foo',
body: 'bar',
userId: 1
};
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(postData)
})
.then(response => response.json())
.then(data => {
console.log(data); // Logging the response data
})
.catch(error => {
console.error('Error posting data:', error);
});
In this example:
fetch(url, options)
is used to send a POST request. Theoptions
object specifies the HTTP method, headers, and the request body.- The
body
is stringified usingJSON.stringify()
to ensure it's sent as a JSON object. - The response is parsed with
response.json()
, and the data is logged to the console.
6. Error Handling in axios and node-fetch
Both axios
and node-fetch
allow you to handle errors gracefully. Here's how:
axios
automatically rejects the promise if the response status is not in the 2xx range. You can catch these errors in thecatch()
block.node-fetch
does not automatically reject promises on HTTP errors. You need to check the response status and manually throw an error if necessary:
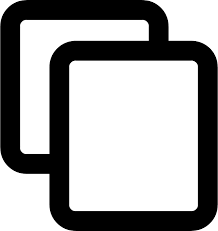
// node-fetch-error-handling.js
const fetch = require('node-fetch');
fetch('https://jsonplaceholder.typicode.com/invalid-url')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => {
console.error('Error:', error);
});
7. Choosing Between axios and node-fetch
Both axios
and node-fetch
are great tools for consuming REST APIs, but each has its own advantages:
axios
provides more built-in features such as automatic JSON parsing, request/response interceptors, and better error handling out-of-the-box.node-fetch
has a simpler API, and if you're already familiar with the browser'sfetch()
API, you may prefer it for consistency across environments.- If you need advanced features like retrying failed requests, handling timeouts, or setting global request defaults,
axios
is likely the better choice.
Conclusion
Consuming REST APIs is a common task in Node.js applications, and both axios
and node-fetch
provide excellent ways to handle HTTP requests. Whether you prefer the simple, native-like fetch
API with node-fetch
or the powerful and feature-rich axios
, both tools are reliable options for working with APIs in Node.js. The choice of library ultimately depends on your project's needs and your preferences for handling requests and responses.
Integrating with Payment Gateways (Stripe, PayPal)
Integrating a payment gateway into your Node.js application allows you to accept payments from your users. Two of the most popular and widely used payment gateways are Stripe and PayPal. Both offer APIs that allow developers to easily accept online payments and process transactions. In this section, we'll walk through the steps of integrating both Stripe and PayPal into your Node.js application.
1. Integrating Stripe
Stripe is a powerful payment gateway that allows you to accept credit card payments, set up subscriptions, and more. To get started with Stripe, you'll need to sign up for a Stripe account and obtain your API keys.
Steps to Integrate Stripe
- Sign up for a Stripe account at stripe.com and get your API keys (publishable and secret keys).
- Install the Stripe package using npm:
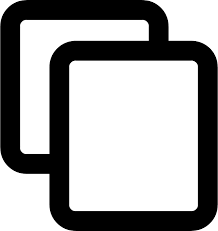
npm install stripe
Creating a Payment Intent with Stripe
To start accepting payments, you need to create a PaymentIntent on the server side. This object represents your intent to collect payment and includes information like the amount, currency, and a client secret that you will use on the client side to confirm the payment.
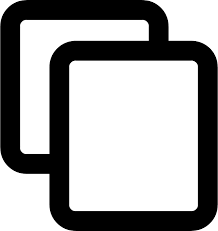
// stripe-payment.js
const express = require('express');
const stripe = require('stripe')('YOUR_STRIPE_SECRET_KEY');
const app = express();
const port = 3000;
app.use(express.json());
app.post('/create-payment-intent', async (req, res) => {
try {
const paymentIntent = await stripe.paymentIntents.create({
amount: 5000, // Amount in cents ($50.00)
currency: 'usd',
});
res.send({
clientSecret: paymentIntent.client_secret
});
} catch (error) {
console.error('Error creating payment intent:', error);
res.status(500).send('Error creating payment intent');
}
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
In this example:
- We set up an Express server and create a POST route to handle the payment intent creation.
- The
stripe.paymentIntents.create()
method creates a payment intent, and we send theclient_secret
back to the client to complete the payment.
Client-Side Integration
On the client side, you will use the Stripe.js library to handle the payment confirmation.
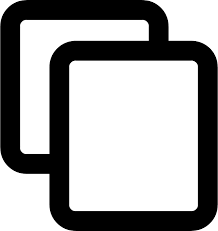
// stripe-client.js
const stripe = Stripe('YOUR_STRIPE_PUBLISHABLE_KEY'); // Your Stripe publishable key
const elements = stripe.elements();
const cardElement = elements.create('card');
cardElement.mount('#card-element');
const form = document.getElementById('payment-form');
form.addEventListener('submit', async (event) => {
event.preventDefault();
const { clientSecret } = await fetch('/create-payment-intent', {
method: 'POST',
}).then(r => r.json());
const { error, paymentIntent } = await stripe.confirmCardPayment(clientSecret, {
payment_method: {
card: cardElement,
}
});
if (error) {
console.error(error.message);
} else {
console.log('Payment successful:', paymentIntent);
}
});
In this example:
stripe.confirmCardPayment()
is used to confirm the payment on the client side using the receivedclientSecret
.- If the payment is successful, the payment intent's details are logged.
2. Integrating PayPal
PayPal is another widely used payment gateway. It offers simple integration with various checkout options, including Express Checkout and Subscription Billing. To get started, you'll need a PayPal account and access to the PayPal Developer Dashboard to generate your API credentials.
Steps to Integrate PayPal
- Sign up for a PayPal Developer account at developer.paypal.com and obtain your client ID and secret key.
- Install the PayPal Node.js SDK:
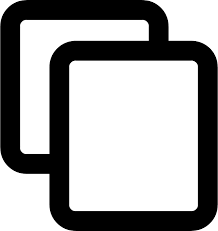
npm install @paypal/checkout-server-sdk
Creating a Payment with PayPal
To create a payment, you first need to set up a payment object that contains details about the transaction (e.g., amount, currency, etc.). After that, you create a payment and redirect the user to PayPal for approval.
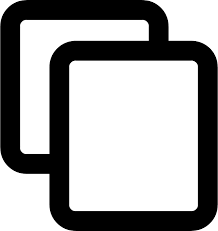
// paypal-payment.js
const express = require('express');
const paypal = require('@paypal/checkout-server-sdk');
const app = express();
const port = 3000;
const environment = new paypal.core.SandboxEnvironment('YOUR_CLIENT_ID', 'YOUR_CLIENT_SECRET');
const client = new paypal.core.PayPalHttpClient(environment);
app.use(express.json());
app.post('/create-payment', async (req, res) => {
const request = new paypal.orders.OrdersCreateRequest();
request.headers['prefer'] = 'return=representation';
request.requestBody({
intent: 'CAPTURE',
purchase_units: [{
amount: {
currency_code: 'USD',
value: '50.00'
}
}],
});
try {
const order = await client.execute(request);
res.send({
approval_url: order.result.links.find(link => link.rel === 'approve').href
});
} catch (error) {
console.error('Error creating PayPal order:', error);
res.status(500).send('Error creating PayPal order');
}
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
In this example:
- We create a PayPal order using the
paypal.orders.OrdersCreateRequest()
method. - After creating the order, we send the approval URL that the user can visit to approve the payment.
Client-Side Integration
On the client side, you use PayPal's JavaScript SDK to handle the approval and capture of the payment.
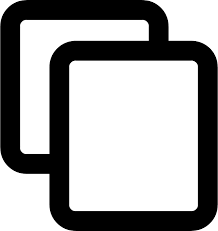
// paypal-client.js
paypal.Buttons({
createOrder: async (data, actions) => {
const response = await fetch('/create-payment', {
method: 'POST',
});
const { approval_url } = await response.json();
return actions.order.create({
purchase_units: [{
amount: {
currency_code: 'USD',
value: '50.00'
}
}],
});
},
onApprove: async (data, actions) => {
const capture = await actions.order.capture();
console.log('Payment successful:', capture);
},
onError: (err) => {
console.error('Error during payment:', err);
}
}).render('#paypal-button-container');
In this example:
paypal.Buttons()
is used to render the PayPal button on the client side.- The
createOrder
function calls the server to create an order and returns the approval URL. - The
onApprove
function captures the payment after the user approves it.
Conclusion
Integrating payment gateways like Stripe and PayPal into your Node.js application is essential for enabling users to make transactions. Both Stripe and PayPal offer flexible APIs and easy-to-use SDKs that simplify the payment process. Whether you're handling one-time payments or subscriptions, both gateways provide secure and reliable solutions for your Node.js application.
Introduction to Microservices with Node.js
Microservices architecture is an approach to software development where an application is divided into small, independently deployable services, each responsible for a specific business function. These services communicate with each other over well-defined APIs, allowing for more flexible and scalable systems. In this section, we’ll explore how to implement microservices using Node.js.
What is Microservices Architecture?
Microservices architecture is a method of designing software applications where each component (or service) is small, independent, and responsible for a specific task or domain within the system. Microservices are loosely coupled and can be developed, deployed, and scaled independently of each other.
Key Features of Microservices
- Independent Deployment: Each service can be deployed independently without affecting other parts of the system.
- Decentralized Data Management: Each service manages its own data, which is often stored in its own database.
- Technology Agnostic: Each microservice can be built with different technologies and programming languages.
- Resilience: Failure in one service does not affect the entire system, improving overall system reliability.
Why Use Microservices?
- Scalability: Microservices can be scaled independently based on demand, ensuring efficient use of resources.
- Flexibility: You can choose the most suitable technology stack for each service.
- Continuous Deployment: Changes to individual services can be deployed quickly without affecting other parts of the application.
- Improved Fault Isolation: Errors in one service do not bring down the entire system.
Building Microservices with Node.js
Node.js is a popular choice for building microservices because of its lightweight, event-driven architecture and non-blocking I/O, making it well-suited for building scalable and performant services. Here’s how you can start building a simple microservices-based application using Node.js:
Step 1: Setting Up the Project
Each microservice will be its own Node.js application. For this example, we’ll create two microservices—one for handling user data and another for handling product data. We will use Express.js to set up the basic server for each service.
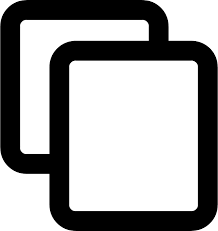
mkdir user-service product-service
cd user-service
npm init -y
npm install express
Step 2: Creating the User Service
In the user service, we will create a simple Express server that listens for requests related to user data. Here’s an example of setting up a basic API for the user service:
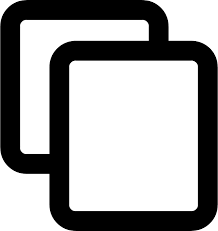
// user-service/index.js
const express = require('express');
const app = express();
const port = 3001;
app.get('/users', (req, res) => {
res.json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
]);
});
app.listen(port, () => {
console.log(`User Service listening at http://localhost:${port}`);
});
The user service exposes a simple endpoint /users
which returns a list of users in JSON format.
Step 3: Creating the Product Service
Similarly, the product service will have its own API to manage product data. Here’s an example for the product service:
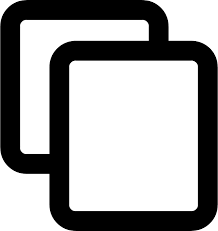
// product-service/index.js
const express = require('express');
const app = express();
const port = 3002;
app.get('/products', (req, res) => {
res.json([
{ id: 1, name: 'Laptop', price: 1000 },
{ id: 2, name: 'Smartphone', price: 500 }
]);
});
app.listen(port, () => {
console.log(`Product Service listening at http://localhost:${port}`);
});
The product service exposes a simple endpoint /products
which returns a list of products in JSON format.
Step 4: Communication Between Microservices
Microservices communicate with each other using HTTP requests or messaging systems like RabbitMQ or Kafka. In this example, we will use HTTP requests to allow one service to retrieve data from another service.
In the user service, for example, we could make an HTTP request to the product service to fetch product data:
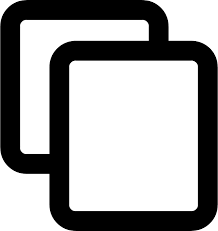
// user-service/index.js (Updated)
const express = require('express');
const axios = require('axios'); // Add axios for HTTP requests
const app = express();
const port = 3001;
app.get('/users', async (req, res) => {
const products = await axios.get('http://localhost:3002/products');
res.json({
users: [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
],
products: products.data
});
});
app.listen(port, () => {
console.log(`User Service listening at http://localhost:${port}`);
});
Now, the user service combines both user and product data into a single response when the /users
endpoint is called.
Best Practices for Microservices
- Keep Services Small and Focused: Each microservice should have a clear, focused responsibility.
- Use API Gateways: An API Gateway can help manage requests from clients and route them to the appropriate microservices.
- Design for Failure: Build resiliency into your services so that failures in one service do not bring down the entire system.
- Use Asynchronous Communication: Use event-driven and message queueing systems like RabbitMQ or Kafka to decouple services and handle communication asynchronously.
- Monitor and Log: Implement logging and monitoring tools to track the health of each service.
Conclusion
Microservices architecture is a powerful design pattern that enables scalable, flexible, and resilient applications. With Node.js, you can easily build microservices that are lightweight and efficient. By following the steps outlined in this section, you can start building microservices that communicate with each other and handle different business functions independently. As your application grows, adopting microservices will help you manage complexity, improve fault tolerance, and scale more easily.
Using AWS Lambda for Serverless Functions
AWS Lambda is a serverless computing service provided by Amazon Web Services (AWS) that allows you to run code without provisioning or managing servers. With Lambda, you can execute functions in response to events such as HTTP requests, database changes, file uploads, and more, without worrying about the underlying infrastructure. In this section, we’ll explore how to create and deploy serverless functions using AWS Lambda and integrate them with other AWS services.
What is AWS Lambda?
AWS Lambda is a serverless compute service that automatically manages the infrastructure required to run your code. You only pay for the compute time you consume, and there’s no need to manage servers or scaling. Lambda functions can be triggered by various AWS services or external events, making it ideal for a wide range of use cases such as data processing, real-time file processing, and API backends.
Key Features of AWS Lambda
- Serverless: No need to provision or manage servers; you focus on writing code and AWS Lambda takes care of the rest.
- Event-Driven: Lambda functions can be triggered by events such as HTTP requests, database updates, or file uploads.
- Scalability: Lambda scales automatically based on the number of events, handling a few requests or thousands without manual intervention.
- Pay-as-you-go: You only pay for the execution time of your functions, making Lambda cost-effective for variable workloads.
- Integrates with AWS Services: Lambda integrates seamlessly with other AWS services such as API Gateway, DynamoDB, S3, and more.
Creating a Simple AWS Lambda Function
To create a Lambda function, you need to write the function code, configure the event source (the trigger), and deploy it in the AWS Lambda console. Below is an example of setting up a simple Lambda function that responds to HTTP requests using AWS API Gateway.
Step 1: Write the Lambda Function
You can write a Lambda function in various languages, including Node.js, Python, and Java. Here’s an example of a basic Lambda function written in Node.js that returns a response to an HTTP request:
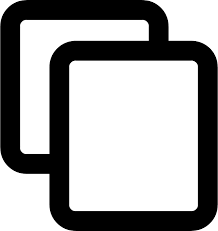
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify('Hello from AWS Lambda!'),
};
return response;
};
This Lambda function takes an event
object as input, processes it, and returns a response with a status code of 200 and a body containing a simple message.
Step 2: Deploy the Lambda Function
To deploy the Lambda function:
- Go to the AWS Lambda Console.
- Click on "Create function" and choose "Author from scratch".
- Specify a name for your function (e.g.,
helloLambda
) and choose the runtime (Node.js). - Paste the function code into the inline editor and click "Deploy".
Step 3: Set Up API Gateway
To trigger the Lambda function via HTTP requests, you need to set up an API Gateway:
- Go to the API Gateway Console.
- Create a new API and define a resource (e.g.,
/hello
) and a method (e.g., GET). - In the method configuration, select "Lambda Function" as the integration type and choose your Lambda function.
- Deploy the API to make it accessible via HTTP requests.
Now, when you make a GET request to the API Gateway endpoint (e.g., https://your-api-id.execute-api.us-east-1.amazonaws.com/hello
), AWS Lambda will invoke the function and return the response.
Using AWS Lambda with Other AWS Services
AWS Lambda can be integrated with various AWS services to create powerful serverless applications. Here are a few common use cases:
Lambda with S3
You can use Lambda to process files uploaded to an S3 bucket. For example, a Lambda function can be triggered when a new image is uploaded to S3, and it can resize the image or extract metadata from it.
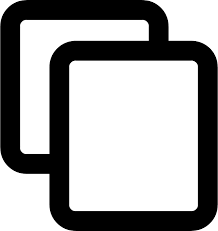
const AWS = require('aws-sdk');
const s3 = new AWS.S3();
exports.handler = async (event) => {
const bucket = event.Records[0].s3.bucket.name;
const key = decodeURIComponent(event.Records[0].s3.object.key.replace(/\+/g, ' '));
const params = {
Bucket: bucket,
Key: key,
};
try {
const data = await s3.getObject(params).promise();
console.log('File Content:', data.Body.toString());
} catch (err) {
console.log('Error getting object:', err);
}
};
Lambda with DynamoDB
You can use Lambda to automatically process changes to DynamoDB tables. For example, when a new item is added to a table, you can trigger a Lambda function to perform further actions such as sending an email or updating another service.
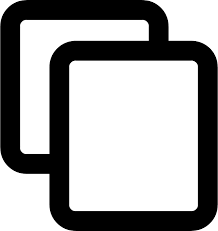
const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB();
exports.handler = async (event) => {
event.Records.forEach((record) => {
if (record.eventName == 'INSERT') {
console.log('New Item:', record.dynamodb.NewImage);
}
});
};
Best Practices for Using AWS Lambda
- Keep Functions Small and Focused: Each Lambda function should perform a single task to maintain simplicity and reusability.
- Use Environment Variables: Store configuration values like database credentials or API keys in environment variables to make your functions more flexible and secure.
- Handle Errors Gracefully: Always handle errors in your Lambda functions to ensure your application is resilient and can recover from unexpected situations.
- Optimize for Cold Starts: Cold starts happen when a Lambda function is invoked after being idle. Minimize cold start times by reducing the size of your deployment package and using lighter runtimes.
- Monitor Lambda Functions: Use AWS CloudWatch to monitor the performance and logs of your Lambda functions to troubleshoot and optimize them.
Conclusion
AWS Lambda is a powerful service that enables you to build scalable, event-driven applications without worrying about managing infrastructure. By writing small, focused functions and integrating them with other AWS services, you can create highly efficient serverless applications. AWS Lambda allows you to respond to events in real-time, making it ideal for a wide range of use cases like file processing, API backends, and real-time data processing.
Working with Serverless Framework
The Serverless Framework is an open-source tool that simplifies the deployment and management of serverless applications. It helps developers build applications that run on cloud services like AWS Lambda, Azure Functions, Google Cloud Functions, and more. By using the Serverless Framework, you can easily define your serverless architecture, deploy your functions, and manage resources with minimal configuration. In this section, we’ll explore how to work with the Serverless Framework to deploy serverless applications on AWS.
What is the Serverless Framework?
The Serverless Framework is a command-line tool that makes it easier to develop and deploy serverless functions. It abstracts away much of the complexity involved in serverless application development by providing a simple configuration file (serverless.yml) that defines the functions, events, and resources that your application needs. The framework supports multiple cloud providers, but we'll focus on using it with AWS Lambda in this section.
Key Features of the Serverless Framework
- Ease of Use: The Serverless Framework simplifies the process of defining, deploying, and managing serverless functions with minimal configuration.
- Multi-Cloud Support: It can be used with AWS, Azure, Google Cloud, and other cloud providers, enabling you to deploy serverless applications on different platforms.
- Infrastructure as Code: The serverless.yml configuration file defines your infrastructure and resources, making deployments reproducible and manageable.
- Plugins and Extensibility: The Serverless Framework supports plugins to extend functionality, enabling you to customize your serverless application development workflow.
- Automatic Scaling: Serverless functions automatically scale based on the volume of events, and the framework manages this for you.
Setting Up the Serverless Framework
Before you can start deploying your serverless applications, you need to install and configure the Serverless Framework. Below are the steps to get started:
Step 1: Install Serverless Framework
You need to have Node.js installed on your computer. Once Node.js is installed, you can install the Serverless Framework globally using npm:
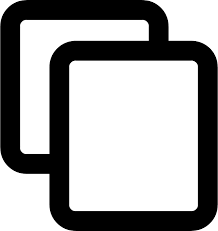
npm install -g serverless
After the installation is complete, you can verify that the Serverless Framework has been installed correctly by running:
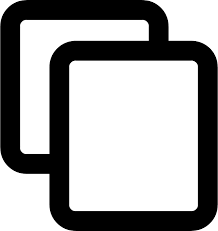
serverless --version
Step 2: Set Up AWS Credentials
The Serverless Framework requires AWS credentials to interact with AWS services. You can set up these credentials by configuring the AWS CLI:
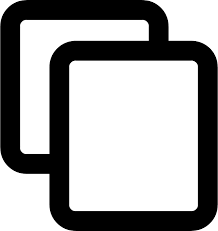
aws configure
Provide your AWS access key, secret key, and the AWS region where your application will be deployed. Alternatively, you can use environment variables or an IAM role to authenticate.
Creating a Serverless Application
Once you’ve set up the Serverless Framework, you can start creating your serverless applications. Below is an example of creating a simple serverless application with AWS Lambda and API Gateway:
Step 1: Create a Serverless Service
A service in the Serverless Framework is essentially a project or application. You can create a new service by running the following command:
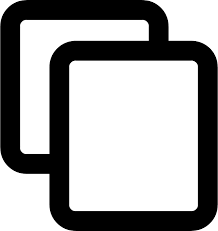
serverless create --template aws-nodejs --path my-service
This will create a new directory called my-service
with the basic structure for an AWS Lambda function written in Node.js.
Step 2: Define Your Lambda Function in serverless.yml
The serverless.yml
file is where you define your Lambda functions, events, and resources. Here’s an example configuration for a simple Lambda function that gets triggered by an HTTP request via API Gateway:
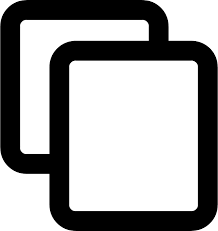
service: my-service
provider:
name: aws
runtime: nodejs14.x
region: us-east-1
functions:
hello:
handler: handler.hello
events:
- http:
path: hello
method: get
This configuration creates a service called my-service
, specifies the AWS provider and Node.js runtime, and defines a function called hello
that is triggered by an HTTP GET request at the /hello
path.
Step 3: Implement the Lambda Function
In the handler.js
file, you can implement your Lambda function. Here’s an example of a simple function that returns a JSON response:
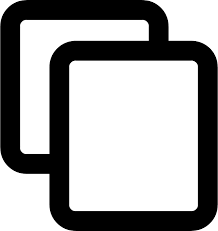
module.exports.hello = async (event) => {
return {
statusCode: 200,
body: JSON.stringify({ message: 'Hello from Serverless Framework!' }),
};
};
Step 4: Deploy the Application
Once you’ve defined your function and events, you can deploy your application to AWS using the following command:
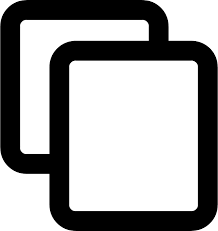
serverless deploy
The Serverless Framework will package your application, create the necessary AWS resources (such as Lambda functions, API Gateway, etc.), and deploy it to AWS. After the deployment is complete, it will provide an endpoint for you to invoke your Lambda function via HTTP.
Managing and Monitoring Serverless Applications
The Serverless Framework provides powerful tools for monitoring and managing your serverless applications:
Logs
You can view the logs of your Lambda function by running:
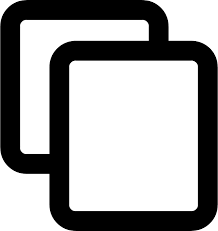
serverless logs -f hello -t
This will show the logs of the hello
function in real time.
Monitoring
The Serverless Framework integrates with AWS CloudWatch to monitor the performance of your Lambda functions. You can view metrics such as invocation count, duration, error count, and more.
Remove the Service
If you want to remove the serverless application from AWS, simply run:
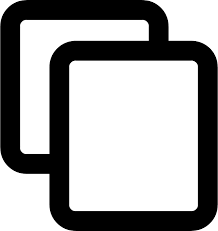
serverless remove
This will delete all the AWS resources created by the Serverless Framework for this service.
Best Practices for Using the Serverless Framework
- Use Environment Variables: Store sensitive data (like API keys or database credentials) in environment variables to keep them secure.
- Keep Functions Small: Keep your Lambda functions small and focused on a single task to improve maintainability and scalability.
- Use Layers: AWS Lambda layers allow you to share common code across multiple functions, improving reusability and reducing deployment package size.
- Use API Gateway Features: Take advantage of API Gateway features such as request validation, caching, and throttling to manage your API endpoints efficiently.
Conclusion
The Serverless Framework makes it easy to build and deploy serverless applications on AWS and other cloud platforms. With simple configuration files, automatic deployment, and the ability to manage and monitor your serverless functions, the Serverless Framework is a powerful tool for modern cloud-native application development. By following best practices and utilizing the features provided by the framework, you can build scalable, cost-effective applications without worrying about managing servers.
Building a Simple Blog with Express and MongoDB
In this tutorial, we will walk through the process of building a simple blog application using Express.js and MongoDB. Express is a web framework for Node.js, and MongoDB is a NoSQL database that stores data in a flexible, JSON-like format. Together, they provide an efficient way to create a full-stack application that allows users to create, read, update, and delete blog posts.
What You'll Learn
- Setting up an Express server
- Connecting to MongoDB using Mongoose
- Creating routes for blog posts (Create, Read, Update, Delete)
- Building views to display blog posts
Prerequisites
Before you start, make sure you have the following installed:
- Node.js and npm
- MongoDB (You can use a local instance or MongoDB Atlas for a cloud database)
- Basic knowledge of JavaScript, Node.js, and Express
Step 1: Setting Up the Project
Start by creating a new directory for the project and initialize a Node.js project:
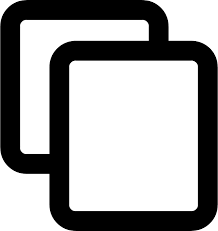
mkdir simple-blog
cd simple-blog
npm init -y
Install the necessary dependencies:
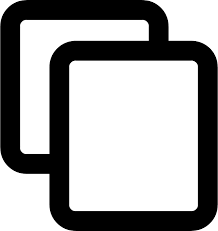
npm install express mongoose ejs body-parser
We are installing the following packages:
- express - Web framework for Node.js
- mongoose - ODM (Object Data Modeling) library for MongoDB
- ejs - Templating engine for rendering HTML views
- body-parser - Middleware to parse incoming request bodies
Step 2: Setting Up the Express Server
Create an app.js
file in the root directory of your project. This file will set up the Express server and configure routes for our blog:
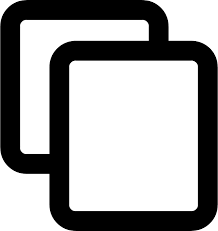
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const app = express();
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/simple-blog', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.log('Failed to connect to MongoDB', err));
// Middleware
app.use(bodyParser.urlencoded({ extended: true }));
app.set('view engine', 'ejs');
app.use(express.static('public'));
// Home route
app.get('/', (req, res) => {
res.render('index');
});
// Listen on port 3000
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Explanation of the code:
- mongoose.connect is used to connect to MongoDB. The database name is
simple-blog
. - body-parser.urlencoded middleware is used to parse incoming request bodies, particularly for forms.
- app.set('view engine', 'ejs') tells Express to use EJS for rendering views.
- app.use(express.static('public')) serves static files (like CSS or images) from the
public
directory. - The home route
/
renders theindex.ejs
view.
Step 3: Defining the Blog Post Model
Now, let’s define a BlogPost model using Mongoose. Create a folder called models
and inside it, create a file named blogPost.js
:
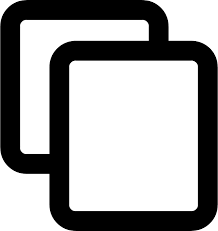
const mongoose = require('mongoose');
const blogPostSchema = new mongoose.Schema({
title: String,
content: String,
date: {
type: Date,
default: Date.now,
},
});
module.exports = mongoose.model('BlogPost', blogPostSchema);
The schema defines a blog post with a title, content, and date. The date field defaults to the current date and time when a new post is created.
Step 4: Creating Routes for Blog Posts
We will now create routes to handle creating, viewing, editing, and deleting blog posts. Update your app.js
file to include the following routes:
Create Route
This route will render a form where users can create new blog posts:
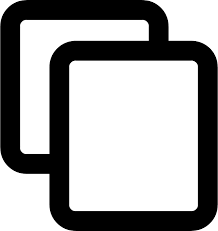
// Create Route
app.get('/new', (req, res) => {
res.render('new');
});
app.post('/new', (req, res) => {
const { title, content } = req.body;
const blogPost = new BlogPost({ title, content });
blogPost.save()
.then(() => res.redirect('/'))
.catch(err => console.log(err));
});
Read Route
This route will display all blog posts on the homepage:
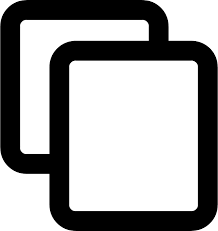
// Home Route (Display all blog posts)
app.get('/', (req, res) => {
BlogPost.find()
.then(posts => res.render('index', { posts }))
.catch(err => console.log(err));
});
Edit and Delete Routes
We can add routes for editing and deleting blog posts. These routes will be similar to the create route but will require the blog post ID as a parameter:
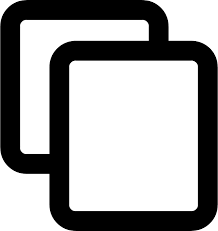
// Edit Route
app.get('/edit/:id', (req, res) => {
const { id } = req.params;
BlogPost.findById(id)
.then(post => res.render('edit', { post }))
.catch(err => console.log(err));
});
app.post('/edit/:id', (req, res) => {
const { id } = req.params;
const { title, content } = req.body;
BlogPost.findByIdAndUpdate(id, { title, content })
.then(() => res.redirect('/'))
.catch(err => console.log(err));
});
// Delete Route
app.post('/delete/:id', (req, res) => {
const { id } = req.params;
BlogPost.findByIdAndDelete(id)
.then(() => res.redirect('/'))
.catch(err => console.log(err));
});
Step 5: Creating Views for the Blog
Next, we’ll create views using EJS to display our blog posts. Create a views
folder and add the following files:
index.ejs
This file will display a list of all blog posts:
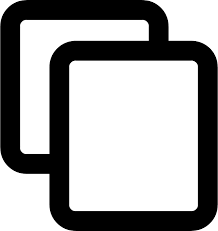
Simple Blog
Blog Posts
Create New Post
<% posts.forEach(post => { %>
-
<%= post.title %>
<%= post.content %>
<%= post.date.toLocaleString() %>
Edit
<% }) %>
new.ejs
This file will render a form to create new blog posts:
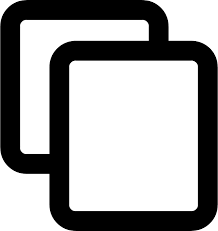
Create New Post
Create New Post
Step 6: Running the Application
Now that everything is set up, start the Express server by running:
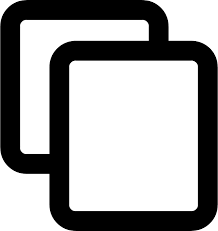
node app.js
Visit http://localhost:3000
in your browser to see the simple blog application in action. You can create, view, edit, and delete blog posts.
Conclusion
Congratulations! You've built a simple blog application using Express and MongoDB. This project introduces you to basic CRUD (Create, Read, Update, Delete) operations with MongoDB and how to structure an Express application. You can further improve this project by adding user authentication, comments, and more advanced features.
Creating a Task Manager Application
In this tutorial, we will build a simple task manager application using Node.js, Express, and MongoDB. This application will allow users to create, view, update, and delete tasks. You will learn how to work with a MongoDB database, manage tasks, and build a REST API to interact with the data.
What You'll Learn
- Setting up an Express server
- Connecting to MongoDB using Mongoose
- Creating routes for task management (CRUD operations)
- Building a simple API for managing tasks
Prerequisites
Before you start, make sure you have the following installed:
- Node.js and npm
- MongoDB (You can use a local instance or MongoDB Atlas for a cloud database)
- Basic knowledge of JavaScript, Node.js, and Express
Step 1: Setting Up the Project
Start by creating a new directory for the project and initializing a Node.js project:
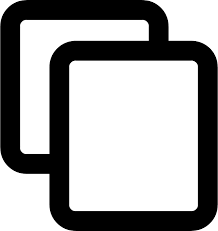
mkdir task-manager
cd task-manager
npm init -y
Install the necessary dependencies:
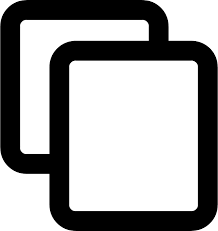
npm install express mongoose body-parser
We are installing the following packages:
- express - Web framework for Node.js
- mongoose - ODM (Object Data Modeling) library for MongoDB
- body-parser - Middleware to parse incoming request bodies
Step 2: Setting Up the Express Server
Create an app.js
file in the root directory of your project. This file will set up the Express server and configure routes for our task manager application:
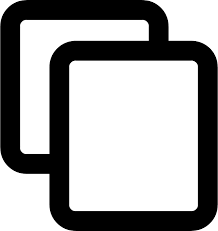
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const app = express();
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/task-manager', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.log('Failed to connect to MongoDB', err));
// Middleware
app.use(bodyParser.json());
app.use(express.static('public'));
// Listen on port 3000
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Explanation of the code:
- mongoose.connect is used to connect to MongoDB. The database name is
task-manager
. - body-parser.json middleware is used to parse incoming JSON request bodies (for the REST API).
- app.use(express.static('public')) serves static files (like CSS or images) from the
public
directory.
Step 3: Defining the Task Model
Now, let’s define a Task model using Mongoose. Create a folder called models
and inside it, create a file named task.js
:
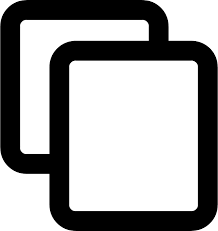
const mongoose = require('mongoose');
const taskSchema = new mongoose.Schema({
title: {
type: String,
required: true,
},
description: {
type: String,
required: true,
},
completed: {
type: Boolean,
default: false,
},
});
module.exports = mongoose.model('Task', taskSchema);
The schema defines a task with a title, description, and a completed field. The completed field defaults to false
when a new task is created.
Step 4: Creating the Routes for Managing Tasks
We will now create routes to handle creating, viewing, updating, and deleting tasks. Update your app.js
file to include the following routes:
Create Route
This route will allow users to create new tasks:
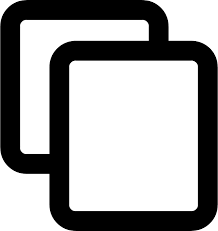
// Create Task Route
app.post('/tasks', (req, res) => {
const { title, description } = req.body;
const task = new Task({ title, description });
task.save()
.then(task => res.status(201).json(task))
.catch(err => res.status(400).json({ error: err.message }));
});
Read Route
This route will return all tasks in the system:
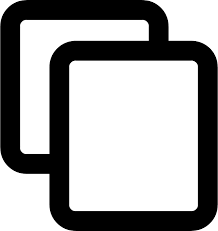
// Get All Tasks Route
app.get('/tasks', (req, res) => {
Task.find()
.then(tasks => res.json(tasks))
.catch(err => res.status(500).json({ error: err.message }));
});
Update Route
This route will allow users to update an existing task:
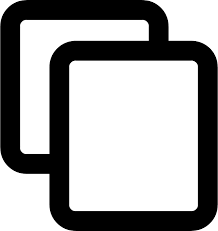
// Update Task Route
app.patch('/tasks/:id', (req, res) => {
const { id } = req.params;
const { title, description, completed } = req.body;
Task.findByIdAndUpdate(id, { title, description, completed }, { new: true })
.then(task => res.json(task))
.catch(err => res.status(400).json({ error: err.message }));
});
Delete Route
This route will allow users to delete a task by its ID:
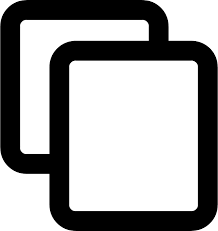
// Delete Task Route
app.delete('/tasks/:id', (req, res) => {
const { id } = req.params;
Task.findByIdAndDelete(id)
.then(() => res.status(204).send())
.catch(err => res.status(400).json({ error: err.message }));
});
Step 5: Running the Application
Now that everything is set up, start the Express server by running:
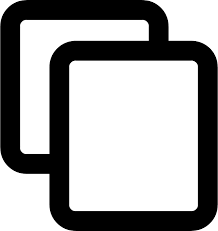
node app.js
Your Task Manager application is now running at http://localhost:3000
. You can interact with the API using tools like Postman or curl to create, read, update, and delete tasks.
Step 6: Testing the Application
Once your server is running, you can test your endpoints by sending HTTP requests:
- POST /tasks - Create a new task (Provide
title
anddescription
in the body of the request) - GET /tasks - Retrieve all tasks
- PATCH /tasks/:id - Update a task (Provide
title
,description
, andcompleted
status in the request body) - DELETE /tasks/:id - Delete a task by its ID
Conclusion
Congratulations! You've built a simple task manager application using Node.js, Express, and MongoDB. This project covers key concepts like working with MongoDB, building a REST API, and managing CRUD operations. You can extend this project by adding features like user authentication, task categories, and due dates.
Developing a URL Shortener
In this tutorial, we will build a simple URL shortener application using Node.js, Express, and MongoDB. The application will allow users to input long URLs and receive a shortened version. When users visit the shortened URL, they will be redirected to the original long URL. This project will help you understand how to work with databases and URL routing in Express.
What You'll Learn
- Setting up an Express server
- Connecting to MongoDB using Mongoose
- Generating short URLs
- Redirecting users to the original URL
Prerequisites
Before you start, make sure you have the following installed:
- Node.js and npm
- MongoDB (You can use a local instance or MongoDB Atlas for a cloud database)
- Basic knowledge of JavaScript, Node.js, and Express
Step 1: Setting Up the Project
Start by creating a new directory for the project and initializing a Node.js project:
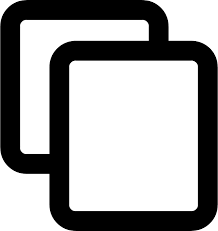
mkdir url-shortener
cd url-shortener
npm init -y
Install the necessary dependencies:
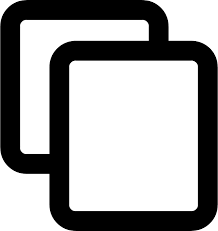
npm install express mongoose shortid
We are installing the following packages:
- express - Web framework for Node.js
- mongoose - ODM (Object Data Modeling) library for MongoDB
- shortid - A simple tool for generating short, unique IDs
Step 2: Setting Up the Express Server
Create an app.js
file in the root directory of your project. This file will set up the Express server and configure routes for our URL shortener application:
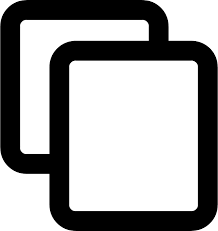
const express = require('express');
const mongoose = require('mongoose');
const shortid = require('shortid');
const app = express();
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/url-shortener', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.log('Failed to connect to MongoDB', err));
// Middleware
app.use(express.json());
// URL Schema
const urlSchema = new mongoose.Schema({
longUrl: {
type: String,
required: true,
},
shortUrl: {
type: String,
required: true,
unique: true,
}
});
const Url = mongoose.model('Url', urlSchema);
// Create URL shortener route
app.post('/shorten', async (req, res) => {
const { longUrl } = req.body;
const shortUrl = shortid.generate();
const url = new Url({ longUrl, shortUrl });
try {
await url.save();
res.json({ longUrl, shortUrl: `${req.protocol}://${req.get('host')}/${shortUrl}` });
} catch (err) {
res.status(400).json({ error: err.message });
}
});
// Redirect route
app.get('/:shortUrl', async (req, res) => {
const { shortUrl } = req.params;
const url = await Url.findOne({ shortUrl });
if (url) {
res.redirect(url.longUrl);
} else {
res.status(404).json({ error: 'Short URL not found' });
}
});
// Start server
app.listen(3000, () => {
console.log('URL shortener is running on port 3000');
});
Explanation of the code:
- mongoose.connect is used to connect to MongoDB. The database name is
url-shortener
. - express.json() middleware is used to parse incoming JSON request bodies.
- shortid.generate() generates a unique short ID for each URL.
- Url is a model that defines the
longUrl
andshortUrl
fields in the database. - The /shorten route handles creating the short URL and stores the mapping in MongoDB.
- The /:shortUrl route handles redirecting users when they visit the short URL.
Step 3: Running the Application
Now that everything is set up, start the Express server by running:
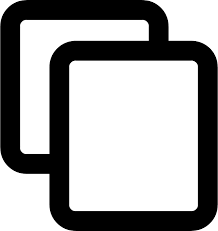
node app.js
Your URL shortener application is now running at http://localhost:3000
.
Step 4: Testing the Application
Once your server is running, you can test your endpoints by sending HTTP requests:
- POST /shorten - Create a new short URL. In the request body, provide the
longUrl
(e.g.,https://www.example.com
) - GET /:shortUrl - Visit the shortened URL to be redirected to the original long URL.
For example, if you POST https://www.example.com
to /shorten
, you will receive a response with a shortened URL like:
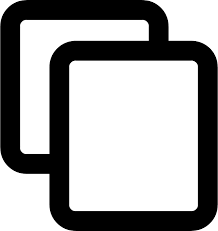
{
"longUrl": "https://www.example.com",
"shortUrl": "http://localhost:3000/abcd1234"
}
Now, if you visit http://localhost:3000/abcd1234
, you will be redirected to https://www.example.com
.
Step 5: Conclusion
Congratulations! You've built a simple URL shortener using Node.js, Express, and MongoDB. You've learned how to:
- Generate unique short URLs
- Store them in a MongoDB database
- Redirect users to the original long URLs
You can extend this project by adding features like URL expiration, user authentication, analytics to track how many times the shortened URLs are visited, or custom short URL aliases.
Building a Real-time To-do List
In this tutorial, we will build a real-time To-do list application using Node.js, Express, and Socket.io. This application will allow users to add, update, and delete tasks, and the changes will be reflected in real-time for all connected users. This project will teach you how to work with WebSockets and real-time communication in Node.js.
What You'll Learn
- Setting up an Express server
- Using Socket.io for real-time communication
- Handling CRUD operations (Create, Read, Update, Delete) on tasks
- Building a simple front-end with HTML and JavaScript
Prerequisites
Before you start, make sure you have the following installed:
- Node.js and npm
- Basic knowledge of JavaScript, Node.js, and Express
Step 1: Setting Up the Project
Start by creating a new directory for the project and initializing a Node.js project:
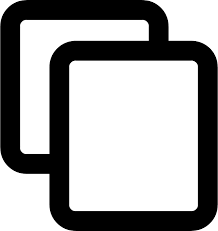
mkdir real-time-todo
cd real-time-todo
npm init -y
Install the necessary dependencies:
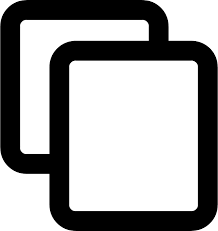
npm install express socket.io
We are installing the following packages:
- express - Web framework for Node.js
- socket.io - A library for real-time WebSocket communication
Step 2: Setting Up the Express Server and Socket.io
Create an app.js
file in the root directory of your project. This file will set up the Express server and configure Socket.io for real-time communication:
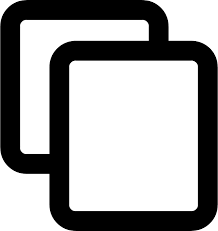
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
// Middleware
app.use(express.static('public'));
// In-memory store for tasks
let tasks = [];
// Socket.io event for a new connection
io.on('connection', (socket) => {
console.log('A user connected');
// Send the list of tasks to the new client
socket.emit('load-tasks', tasks);
// Add a new task
socket.on('add-task', (task) => {
tasks.push(task);
io.emit('task-added', task); // Broadcast the new task to all clients
});
// Delete a task
socket.on('delete-task', (taskId) => {
tasks = tasks.filter(task => task.id !== taskId);
io.emit('task-deleted', taskId); // Broadcast the deletion to all clients
});
// Disconnect event
socket.on('disconnect', () => {
console.log('A user disconnected');
});
});
// Start server
server.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Explanation of the code:
- http.createServer is used to create an HTTP server with Express.
- socketIo is initialized with the HTTP server to enable WebSocket communication.
- We define an in-memory store for tasks in the tasks array. This is where tasks will be stored temporarily while the app is running.
- The connection event listens for new WebSocket connections, and we use emit to send data to clients (e.g., sending the task list when a user connects).
- We have events for adding a task, deleting a task, and notifying all clients about changes in real-time using io.emit.
Step 3: Building the Front-end
Create a public
folder in the root directory of your project. Inside this folder, create an index.html
file for the front-end of the application:
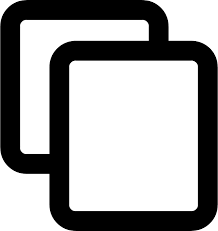
Real-time To-Do List
Real-time To-Do List
Explanation of the front-end code:
- The socket.io.js script is included to enable WebSocket communication with the server.
- When the "Add Task" button is clicked, a new task is created with a unique ID (based on the current timestamp) and emitted to the server using socket.emit('add-task', task).
- The socket.on('task-added') event listens for new tasks and dynamically adds them to the task list in the DOM.
- Each task has a "Delete" button. When clicked, it emits a delete-task event to the server, which removes the task.
- We also load existing tasks when the page is first loaded using the load-tasks event, and we listen for task deletions with task-deleted.
Step 4: Running the Application
Now that everything is set up, start the server:
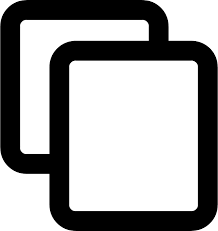
node app.js
Your real-time to-do list application is now running at http://localhost:3000
.
Step 5: Conclusion
Congratulations! You've built a real-time to-do list application using Node.js, Express, and Socket.io. You've learned how to:
- Set up real-time communication with Socket.io
- Handle CRUD operations on tasks
- Update the front-end in real-time for all users
You can extend this project by adding features like task persistence (using a database), user authentication, or even task categories or deadlines.
Working with Social Media APIs (Facebook, Twitter, Google)
Many applications integrate with social media platforms like Facebook, Twitter, and Google to enhance user experience by enabling features like authentication, data sharing, and more. In this section, we’ll look at how to integrate these social media APIs into your Node.js application for functionalities such as OAuth authentication, fetching user data, and posting content.
1. Facebook API Integration
The Facebook Graph API allows you to interact with Facebook's social graph, including features like user authentication, reading and posting user content, and interacting with pages and groups.
Steps to Integrate Facebook
fb
npm package:Authentication with Facebook
Facebook supports OAuth 2.0 for user authentication. You need to request an access token to interact with user data.
In this example:
2. Twitter API Integration
The Twitter API allows you to interact with user timelines, send tweets, and perform other actions. To use the Twitter API, you need to register your application on the Twitter Developer Console to obtain API keys and access tokens.
Steps to Integrate Twitter
twitter
npm package:Tweeting with Twitter API
Here’s an example of sending a tweet using the Twitter API in Node.js:
In this example:
statuses/update
endpoint is used to send a tweet.3. Google API Integration
The Google API offers a variety of services, such as authentication with Google OAuth, accessing Google Sheets, sending emails through Gmail, and much more. To get started, you need to create a project in the Google Cloud Console and enable the appropriate APIs.
Steps to Integrate Google
googleapis
Google OAuth Authentication
To authenticate users with Google, you can use the OAuth2 client from the Google APIs Node.js client library.
In this example:
Conclusion
Integrating social media APIs like Facebook, Twitter, and Google into your Node.js application can provide powerful capabilities, such as user authentication, sharing content, and accessing user data. By following the steps outlined for each platform, you can easily add social media functionality to your app and enhance your users' experience.