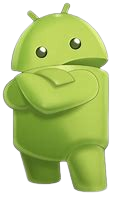
Overview of Android
1. What is Android?
Android is an open-source operating system developed by Google, designed primarily for touchscreen mobile devices such as smartphones and tablets. It is based on the Linux kernel and provides a rich application framework that allows developers to build innovative apps and games.
2. Key Features of Android
- Open Source: Android is an open-source platform, enabling developers and manufacturers to customize and enhance the system.
- Extensive App Ecosystem: With access to the Google Play Store, Android devices can run millions of apps across various categories.
- Multi-Tasking: Android supports running multiple apps simultaneously, offering seamless user experiences.
- Customizable UI: Users can customize the home screen, widgets, and other interface elements to suit their preferences.
3. Android Architecture
The Android platform is built on a layered architecture consisting of the following components:
- Linux Kernel: Provides low-level system services like memory and process management.
- Libraries: A set of C/C++ libraries used by various components of the Android system.
- Android Runtime: Includes the ART (Android Runtime) for running apps and a set of core libraries.
- Application Framework: Provides high-level building blocks like Activities, Services, and Content Providers.
- Applications: The top layer where user-installed apps and system apps reside.
4. Development Environment
To develop Android applications, you need to set up an integrated development environment (IDE) like Android Studio, which is the official IDE provided by Google. Android Studio offers tools for designing, coding, testing, and debugging Android apps.
5. Android Versions
Android has undergone numerous updates since its launch in 2008. Each version introduces new features and improvements. Here are some notable versions:
- Android 1.0: The first public release in 2008.
- Android 4.0 (Ice Cream Sandwich): Introduced a unified UI for phones and tablets.
- Android 5.0 (Lollipop): Introduced Material Design and ART runtime.
- Android 10: Added dark mode and gesture navigation.
- Android 13: The latest version (as of 2024), focusing on privacy and enhanced user experience.
6. Conclusion
Android's flexibility, extensive ecosystem, and continuous evolution make it one of the most popular operating systems worldwide. A solid understanding of Android's features, architecture, and development tools is essential for building robust and user-friendly mobile applications. In the next section, we'll explore Java/Kotlin basics for Android development to start building apps.
Tools Required: Android Studio, SDK, Emulator
1. Android Studio
Android Studio is the official Integrated Development Environment (IDE) for Android development. It provides a comprehensive set of tools to build, test, and debug Android applications. Key features include:
- Code Editor: A powerful editor with features like code completion, refactoring, and syntax highlighting.
- Layout Editor: A drag-and-drop editor to design XML layouts visually.
- Debugging Tools: Tools to analyze and debug applications.
- Build System: Based on Gradle, it automates and manages the build process.
You can download Android Studio from the official website.
2. Android SDK
The Android Software Development Kit (SDK) is a collection of tools, libraries, and APIs required to build Android applications. The SDK includes:
- Platform Tools: Tools like ADB (Android Debug Bridge) and Fastboot for interacting with Android devices.
- Build Tools: Tools for compiling and packaging applications.
- APIs: Libraries for accessing Android system features like cameras, sensors, and notifications.
The SDK Manager in Android Studio allows you to download and manage the necessary SDK components.
3. Android Emulator
The Android Emulator is a virtual device that simulates an Android environment on your computer. It allows you to test apps without needing a physical device. Key features include:
- Device Configurations: Simulate different screen sizes, resolutions, and device types.
- API Level Testing: Test apps on various Android versions.
- Advanced Features: Simulate GPS, network conditions, and device sensors.
To set up an emulator, use the AVD Manager in Android Studio to create and configure virtual devices.
4. Conclusion
Android Studio, the Android SDK, and the Emulator are essential tools for developing and testing Android applications. Setting up these tools properly will ensure a smooth development experience. In the next section, we’ll explore the basics of setting up your first Android project.
First Android App
1. Setting Up Your First Project
To create your first Android app, follow these steps:
- Open Android Studio and click on New Project.
- Choose a template, such as Empty Activity, and click Next.
- Configure your project by providing the following details:
- Name: The name of your app (e.g., MyFirstApp).
- Package Name: A unique identifier for your app (e.g., com.example.myfirstapp).
- Save Location: The directory where your project will be stored.
- Language: Choose either Java or Kotlin.
- API Level: Select the minimum API level your app will support.
- Click Finish to create your project.
2. Understanding the Project Structure
Android Studio generates the following key files and directories for your project:
- Manifest File:
AndroidManifest.xml
, which contains app metadata such as permissions and activities. - Java/Kotlin Code: The
java
orkotlin
folder contains the app’s source code. - Resources: The
res
folder stores XML layouts, drawable resources, and string values. - Gradle Scripts: Configuration files for building and managing dependencies.
3. Designing the User Interface
To design the user interface (UI) of your app:
- Open the
activity_main.xml
file in the res/layout folder. - Use the drag-and-drop features of the Layout Editor to add UI components like buttons, text fields, and labels.
- Modify the properties of each component using the Attributes panel or directly in XML code.
- Save your changes to update the layout.
4. Writing Code
To add functionality to your app, edit the MainActivity.java
or MainActivity.kt
file:
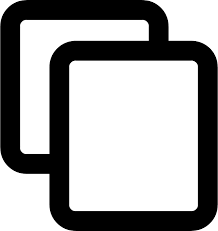
// Example Java code for a Button click event
Button myButton = findViewById(R.id.my_button);
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "Button clicked!", Toast.LENGTH_SHORT).show();
}
});
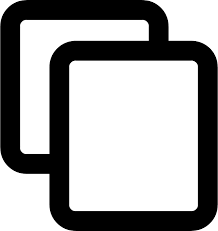
// Example Kotlin code for a Button click event
val myButton: Button = findViewById(R.id.my_button)
myButton.setOnClickListener {
Toast.makeText(this, "Button clicked!", Toast.LENGTH_SHORT).show()
}
5. Running Your App
To test your app:
- Connect a physical Android device or set up an emulator in Android Studio.
- Click the Run button or press
Shift + F10
. - Select your device or emulator as the target and wait for the app to launch.
You should see your app running on the device or emulator!
6. Conclusion
Congratulations! You’ve built and run your first Android app. This is your first step into Android development. In the next sections, we’ll explore advanced topics like UI components, navigation, and integrating APIs.
Installing Android Studio
1. System Requirements
Before installing Android Studio, ensure that your system meets the following requirements:
- Operating System: Windows, macOS, or Linux.
- RAM: Minimum 8 GB (16 GB recommended).
- Disk Space: Minimum 4 GB of free disk space.
- Java Development Kit (JDK): Android Studio includes the required JDK, so no separate installation is needed.
2. Downloading Android Studio
Follow these steps to download Android Studio:
- Go to the official Android Studio download page.
- Click on the Download Android Studio button.
- Review and accept the terms and conditions.
- Download the appropriate installer for your operating system.
3. Installing Android Studio
After downloading the installer, follow these steps to install Android Studio:
- Run the installer file.
- Follow the on-screen instructions to complete the installation. You may need to choose the installation location and components to install.
- Once the installation is complete, launch Android Studio.
4. Setting Up the Environment
When you open Android Studio for the first time, you will need to set up the development environment:
- Choose whether to import previous settings or start fresh.
- Follow the Setup Wizard to download the necessary SDK components and tools.
- Select the default or custom settings as per your requirements.
The Setup Wizard will configure the environment and ensure that all necessary components are installed.
5. Verifying the Installation
To verify that Android Studio has been installed correctly:
- Open Android Studio.
- Click on File > New > New Project.
- Create a test project and run it on an emulator or connected device.
If the project builds and runs successfully, your installation is complete!
6. Conclusion
With Android Studio installed, you are now ready to start developing Android apps. In the next sections, we’ll guide you through creating your first app and understanding the basics of Android development.
Setting Up the Android SDK
1. What is the Android SDK?
The Android Software Development Kit (SDK) is a set of tools that allows developers to build, test, and debug Android applications. It includes essential components like libraries, build tools, and the Android Emulator.
2. Installing the Android SDK
When you install Android Studio, the Android SDK is installed automatically. Follow these steps to verify and set it up:
- Open Android Studio.
- Go to File > Settings (on Windows/Linux) or Android Studio > Preferences (on macOS).
- Navigate to Appearance & Behavior > System Settings > Android SDK.
- Ensure the required SDK components are installed:
- SDK Platforms: Select the Android version(s) you want to develop for.
- SDK Tools: Ensure tools like the Android Emulator, SDK Build-Tools, and Google Play Services are checked.
- Click Apply to download and install any missing components.
3. Configuring the SDK
To configure the Android SDK for your development environment:
- Check the SDK location in the Android SDK settings. The default path is:
- Windows:
C:\Users\YourUsername\AppData\Local\Android\Sdk
- macOS:
/Users/YourUsername/Library/Android/sdk
- Linux:
/home/YourUsername/Android/Sdk
- Windows:
- Ensure that the path to the SDK is correctly set in your system's environment variables (optional for command-line tools).
4. Updating the SDK
To keep your SDK up to date:
- Open Android Studio.
- Go to File > Settings > Android SDK.
- In the SDK Platforms and SDK Tools tabs, check for updates and select the components you want to update.
- Click Apply to install the updates.
5. Verifying the SDK Installation
To verify that the SDK is set up correctly:
- Create a new project in Android Studio.
- Build and run the project on an emulator or connected Android device.
- If the app runs successfully, the SDK is configured correctly.
6. Conclusion
The Android SDK is an essential part of Android development. With the SDK set up, you're ready to start building and testing Android applications. In the next sections, we'll explore creating your first app and understanding the basics of Android development.
Creating a New Project
1. Opening Android Studio
To start creating a new Android project, follow these steps:
- Open Android Studio on your computer.
- On the welcome screen, click on New Project.
2. Configuring Your New Project
When you create a new project, you need to configure the basic settings:
- Application Name: Enter the name of your app (e.g., "MyFirstApp").
- Company Domain: Enter your domain or use a placeholder (e.g., "example.com").
- Project Location: Choose the directory where the project will be saved.
- Click Next to proceed.
3. Selecting the Target Device
In the next step, specify the devices your app will support:
- Phone and Tablet: Choose the minimum API level your app will support.
- Optionally, select additional device categories, such as Wear OS or Android TV, if your app targets them.
- Click Next to continue.
4. Choosing a Template
Android Studio provides pre-built templates to help you get started:
- Select a template for your project, such as Empty Activity, Basic Activity, or Navigation Drawer Activity.
- For your first project, choose Empty Activity.
- Click Next to proceed.
5. Configuring Activity Settings
Customize the activity settings for your app:
- Activity Name: Enter the name of the main activity (e.g., "MainActivity").
- Layout Name: Specify the layout file for the activity (e.g., "activity_main").
- Keep the Language as either Java or Kotlin, depending on your preference.
- Ensure the Generate Layout File and Backwards Compatibility (AppCompat) options are checked.
- Click Finish to create the project.
6. Exploring Your Project
Once the project is created:
- The Project Explorer shows your app's files and structure.
- The activity_main.xml file is where you design your app's UI.
- The MainActivity.java or MainActivity.kt file contains the logic for your app.
7. Running Your Project
To test your app:
- Click the green Run button in the toolbar.
- Select an emulator or connected Android device to run your app.
- Wait for the app to build and launch.
If everything is set up correctly, your app will display on the emulator or device.
8. Conclusion
You’ve successfully created and run your first Android project! This is the starting point for building and testing your Android applications. In the next sections, we’ll dive deeper into designing user interfaces and adding functionality to your app.
Navigating the Android Studio IDE
1. Introduction to the Android Studio Interface
Android Studio is a powerful Integrated Development Environment (IDE) designed for Android development. Understanding its interface will help you navigate and work more efficiently.
2. Key Components of the IDE
- Project Explorer: Displays the structure of your project, including files and resources. You can find it on the left side of the IDE.
- Code Editor: The main area where you write and edit your app’s code.
- Toolbar: Provides quick access to common actions like running the app, debugging, and version control.
- Navigation Bar: Located just above the Code Editor, it helps you quickly switch between files and directories.
- Run and Debug Panels: At the bottom, these panels display logs, errors, and debugging information when you run or debug your app.
- Tool Windows: Various panels like the Logcat, Terminal, and Build Output can be accessed via tabs at the edges of the IDE.
3. Common Views in the Project Explorer
The Project Explorer can display your project in several ways:
- Android View: Simplifies your project structure by showing only relevant files.
- Project View: Displays the complete file structure as it exists on the disk.
- Packages View: Organizes your files by package names.
- Problems View: Highlights issues or warnings in your project.
4. Using the Code Editor
The Code Editor is where most of your development work happens. Key features include:
- Syntax Highlighting: Makes your code more readable by color-coding elements.
- Code Suggestions: Provides auto-completion and error corrections.
- Quick Navigation: Use shortcuts like
Ctrl + Click
(orCmd + Click
on macOS) to navigate to definitions. - Lint Warnings: Highlights potential issues in your code with suggestions for fixes.
5. Tool Windows
Android Studio provides several tool windows to help with development:
- Logcat: Displays system messages, including errors and debugging logs.
- Terminal: A built-in command-line interface for running commands.
- Build Output: Shows the results of your build process, including errors and warnings.
- Resource Manager: Helps you manage app resources like images and layouts.
6. Customizing the IDE
You can customize Android Studio to suit your workflow:
- Change Themes: Go to File > Settings > Appearance & Behavior > Theme to switch between light and dark modes.
- Customize Shortcuts: Modify keyboard shortcuts under File > Settings > Keymap.
- Enable Plugins: Add functionality by installing plugins from File > Settings > Plugins.
7. Running and Debugging Your App
The toolbar at the top of the IDE provides quick access to running and debugging your app. Use the following buttons:
- Run: Builds and runs your app on a connected device or emulator.
- Debug: Launches your app with debugging tools enabled.
- Stop: Stops a running app.
8. Conclusion
Android Studio provides a comprehensive set of tools for Android development. Familiarizing yourself with its interface and features will make your development process smoother and more efficient. In the next section, we’ll dive into designing user interfaces for your Android app.
Activities
1. What Are Activities?
An Activity is a crucial component of an Android app that provides the user interface (UI) for interaction. Each screen in an Android app is typically associated with an activity. Activities serve as the entry point for user interaction and manage the lifecycle of the screen.
2. Anatomy of an Activity
An activity consists of two main components:
- Java/Kotlin Class: Defines the behavior and logic of the activity.
- XML Layout File: Specifies the UI design for the activity.
3. Declaring an Activity
To create an activity, you need to declare it in the AndroidManifest.xml
file:
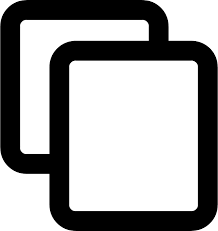
<application>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
The intent-filter
above makes MainActivity
the entry point of the app.
4. Activity Lifecycle
Every activity has a lifecycle that is managed by the Android operating system. Key lifecycle methods include:
- onCreate(): Called when the activity is first created. Use it to initialize resources.
- onStart(): Called when the activity becomes visible to the user.
- onResume(): Called when the user interacts with the activity.
- onPause(): Called when the activity goes into the background but is still partially visible.
- onStop(): Called when the activity is no longer visible to the user.
- onDestroy(): Called before the activity is destroyed.
Here’s a diagram of the activity lifecycle:

5. Navigating Between Activities
To switch between activities, use Intents. Here’s an example:
Java:
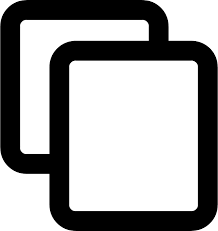
Intent intent = new Intent(this, SecondActivity.class);
startActivity(intent);
Kotlin:
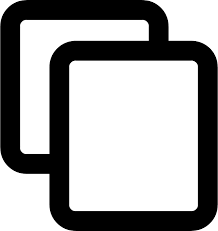
val intent = Intent(this, SecondActivity::class.java)
startActivity(intent)
6. Passing Data Between Activities
You can pass data between activities using Intent
extras:
Java:
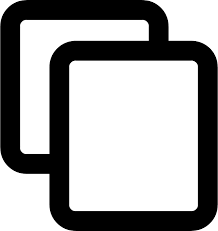
Intent intent = new Intent(this, SecondActivity.class);
intent.putExtra("KEY", "Value");
startActivity(intent);
Kotlin:
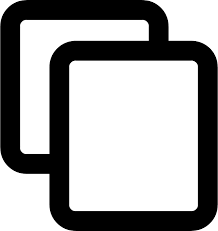
val intent = Intent(this, SecondActivity::class.java)
intent.putExtra("KEY", "Value")
startActivity(intent)
In the target activity, retrieve the data:
Java:
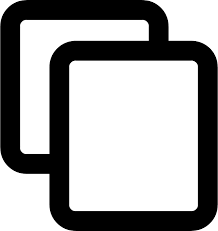
String value = getIntent().getStringExtra("KEY");
Kotlin:
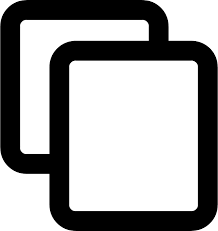
val value = intent.getStringExtra("KEY")
7. Conclusion
Activities are the backbone of Android app development. By understanding their lifecycle, navigating between them, and passing data effectively, you can build dynamic and interactive applications. In the next section, we’ll explore fragments, which allow for more modular and flexible UI design.
Services
1. What Are Services?
A Service in Android is a component that performs long-running operations in the background without a user interface. Services are used for tasks such as playing music, downloading files, or handling network transactions while the user interacts with other components of the app.
2. Types of Services
There are three types of services in Android:
- Foreground Service: Continues running in the foreground and displays a notification. For example, a music player.
- Background Service: Runs in the background without user interaction. For example, syncing data.
- Bound Service: Allows components to bind to it and interact with it, such as an activity interacting with a service for real-time updates.
3. Declaring a Service
To use a service, declare it in the AndroidManifest.xml
file:
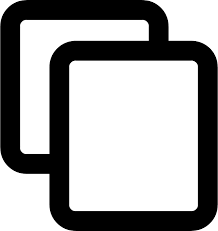
<application>
<service android:name=".MyService" />
</application>
4. Creating a Service
A service is created by extending the Service
class and overriding its methods. Here’s an example:
Java:
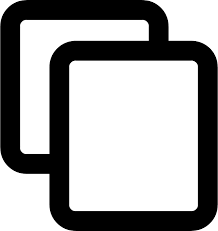
public class MyService extends Service {
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// Code to perform task
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
return null; // No binding
}
@Override
public void onDestroy() {
super.onDestroy();
// Cleanup resources
}
}
Kotlin:
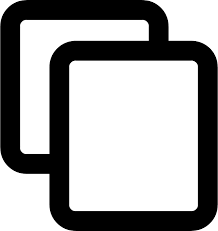
class MyService : Service() {
override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
// Code to perform task
return START_STICKY
}
override fun onBind(intent: Intent?): IBinder? {
return null // No binding
}
override fun onDestroy() {
super.onDestroy()
// Cleanup resources
}
}
5. Starting and Stopping a Service
You can start a service using the startService()
method:
Java:
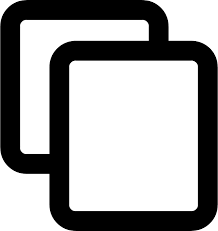
Intent intent = new Intent(this, MyService.class);
startService(intent);
Kotlin:
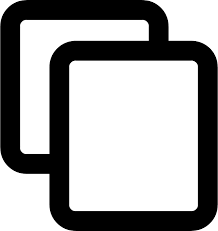
val intent = Intent(this, MyService::class.java)
startService(intent)
To stop the service, use stopService()
:
Java:
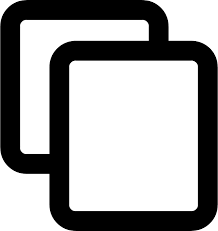
Intent intent = new Intent(this, MyService.class);
stopService(intent);
Kotlin:
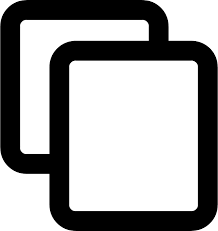
val intent = Intent(this, MyService::class.java)
stopService(intent)
6. Service Lifecycle
The lifecycle of a service involves the following key methods:
- onCreate(): Called when the service is first created.
- onStartCommand(): Called when the service is started using
startService()
. - onDestroy(): Called when the service is stopped or destroyed.
7. Foreground Services
Foreground services run with higher priority and display a notification to inform the user. To create a foreground service:
Kotlin Example:
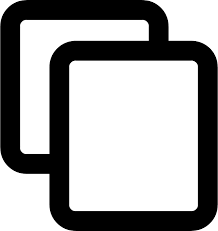
val notification = NotificationCompat.Builder(this, "CHANNEL_ID")
.setContentTitle("Foreground Service")
.setContentText("Running...")
.setSmallIcon(R.drawable.ic_notification)
.build()
startForeground(1, notification)
8. Conclusion
Services are essential for performing background tasks in Android apps. Understanding how to create and manage services, including their lifecycle and types, will help you build efficient and responsive applications. In the next section, we’ll explore Broadcast Receivers to handle system-wide events and communication.
Broadcast Receivers
1. What Are Broadcast Receivers?
A Broadcast Receiver in Android is a component that listens for and responds to system-wide broadcast events or intents. These events can be system-generated (e.g., battery low, network change) or app-specific (e.g., custom broadcasts).
2. Types of Broadcasts
Broadcasts in Android are categorized into two types:
- System Broadcasts: These are sent by the Android system, such as
android.intent.action.BATTERY_LOW
orandroid.net.conn.CONNECTIVITY_CHANGE
. - Custom Broadcasts: These are created and sent by applications to communicate with other components or apps.
3. Declaring a Broadcast Receiver
Broadcast Receivers can be declared in two ways:
- Statically: Declare the receiver in the
AndroidManifest.xml
file. - Dynamically: Register the receiver programmatically at runtime.
4. Creating a Broadcast Receiver
To create a broadcast receiver, extend the BroadcastReceiver
class and override the onReceive()
method:
Java:
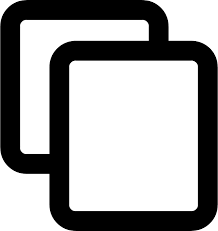
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (Intent.ACTION_BATTERY_LOW.equals(action)) {
// Handle battery low event
}
}
}
Kotlin:
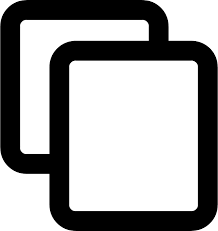
class MyReceiver : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
val action = intent.action
if (Intent.ACTION_BATTERY_LOW == action) {
// Handle battery low event
}
}
}
5. Registering a Broadcast Receiver
Broadcast receivers can be registered either statically or dynamically:
Static Registration:
Declare the receiver in the AndroidManifest.xml
file:
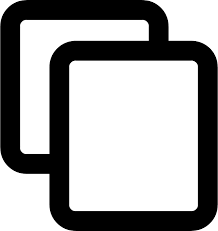
<application>
<receiver android:name=".MyReceiver">
<intent-filter>
<action android:name="android.intent.action.BATTERY_LOW" />
</intent-filter>
</receiver>
</application>
Dynamic Registration:
Register the receiver programmatically in your activity or service:
Java:
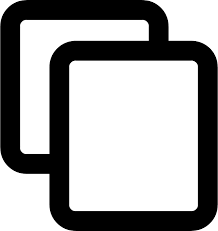
MyReceiver receiver = new MyReceiver();
IntentFilter filter = new IntentFilter(Intent.ACTION_BATTERY_LOW);
registerReceiver(receiver, filter);
Kotlin:
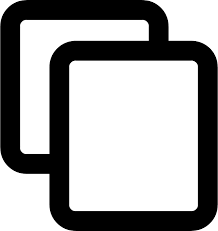
val receiver = MyReceiver()
val filter = IntentFilter(Intent.ACTION_BATTERY_LOW)
registerReceiver(receiver, filter)
6. Sending Custom Broadcasts
To send a custom broadcast, use the sendBroadcast()
method:
Java:
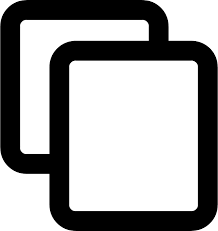
Intent intent = new Intent("com.example.MY_CUSTOM_ACTION");
sendBroadcast(intent);
Kotlin:
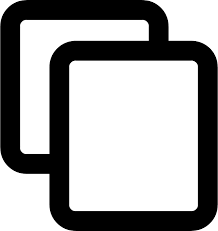
val intent = Intent("com.example.MY_CUSTOM_ACTION")
sendBroadcast(intent)
7. Best Practices
- Unregister dynamically registered receivers when they are no longer needed (e.g., in
onDestroy()
). - Avoid using sticky broadcasts as they are deprecated.
- Use
LocalBroadcastManager
for intra-app communication to improve security and performance.
8. Conclusion
Broadcast Receivers are a powerful tool for handling system-wide and custom events in Android. By understanding how to create, register, and use them effectively, you can build apps that respond to various events and improve user experience. In the next section, we’ll explore Content Providers for sharing data between apps.
Content Providers
1. What Are Content Providers?
A Content Provider in Android is a component that allows data to be shared between different applications. They provide a standardized interface for accessing and modifying data stored in one app and allow other apps to read and write to it, if permitted. Content Providers are particularly useful for sharing data like contacts, media, or app-specific data.
2. When to Use a Content Provider
Content Providers are typically used when you need to share data between apps or when an app needs to access data from another app. For example, you may want to access contacts, calendar events, or media files stored by other apps.
3. Content Provider URI
Content Providers use a URI (Uniform Resource Identifier) to access the data. These URIs are structured in the form of:
content:///
The authority
is typically the app package name or a unique identifier for the provider, and the path
is the specific data or table you want to access.
4. Creating a Content Provider
To create a Content Provider, you must extend the ContentProvider
class and implement its required methods like onCreate()
, query()
, insert()
, update()
, and delete()
.
Java:
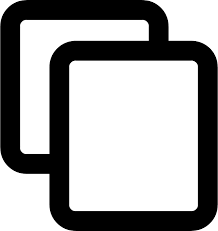
public class MyContentProvider extends ContentProvider {
@Override
public boolean onCreate() {
// Initialize your content provider here
return true;
}
@Override
public Cursor query(Uri uri, String[] projection, String selection, String[] selectionArgs, String sortOrder) {
// Query data from your database
return null;
}
@Override
public Uri insert(Uri uri, ContentValues values) {
// Insert new data
return null;
}
@Override
public int update(Uri uri, ContentValues values, String selection, String[] selectionArgs) {
// Update existing data
return 0;
}
@Override
public int delete(Uri uri, String selection, String[] selectionArgs) {
// Delete data
return 0;
}
@Override
public String getType(Uri uri) {
// Return MIME type
return null;
}
}
Kotlin:
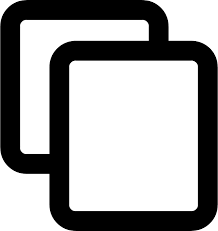
class MyContentProvider : ContentProvider() {
override fun onCreate(): Boolean {
// Initialize your content provider here
return true
}
override fun query(
uri: Uri, projection: Array?, selection: String?,
selectionArgs: Array?, sortOrder: String?
): Cursor? {
// Query data from your database
return null
}
override fun insert(uri: Uri, values: ContentValues?): Uri? {
// Insert new data
return null
}
override fun update(uri: Uri, values: ContentValues?, selection: String?, selectionArgs: Array?): Int {
// Update existing data
return 0
}
override fun delete(uri: Uri, selection: String?, selectionArgs: Array?): Int {
// Delete data
return 0
}
override fun getType(uri: Uri): String? {
// Return MIME type
return null
}
}
5. Registering a Content Provider
Once you have created your content provider, it needs to be registered in the AndroidManifest.xml
file:
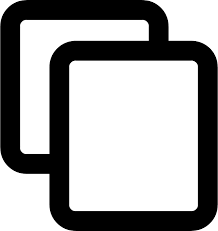
<application>
<provider
android:name=".MyContentProvider"
android:authorities="com.example.mycontentprovider"
android:exported="true" />
</application>
The authorities
attribute is crucial as it defines the URI of your content provider, allowing other apps to access it.
6. Accessing a Content Provider
Once your content provider is registered, other apps can access it by using a ContentResolver
and querying the URI:
Java:
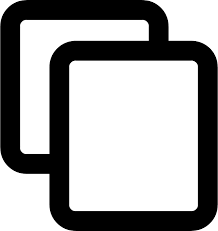
ContentResolver resolver = getContentResolver();
Uri uri = Uri.parse("content://com.example.mycontentprovider/data");
Cursor cursor = resolver.query(uri, null, null, null, null);
Kotlin:
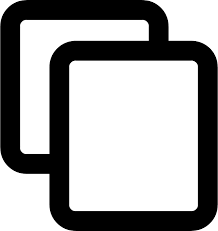
val resolver = contentResolver
val uri = Uri.parse("content://com.example.mycontentprovider/data")
val cursor = resolver.query(uri, null, null, null, null)
7. Permissions and Security
When using content providers, make sure to set the necessary permissions. If your content provider exposes sensitive data, you can restrict access using permissions defined in the AndroidManifest.xml
file:
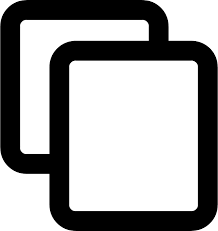
8. Best Practices
- Limit data exposure to only the necessary parts of your app’s data.
- Use
ContentProvider
to share data with other apps, and make sure to handle concurrency and data consistency. - Implement proper access control and permissions to ensure data security.
9. Conclusion
Content Providers are a powerful mechanism for sharing data between apps in Android. By understanding how to create, register, and securely access them, you can build more robust and data-driven Android apps. In the next section, we’ll explore the Android permissions model to ensure secure access to sensitive data in your applications.
Linear Layout
1. What is Linear Layout?
A LinearLayout is a view group in Android that arranges its child elements in a single row or column. The child elements can be laid out either horizontally or vertically based on the layout's orientation
attribute. It is one of the most commonly used layouts in Android development for simple layouts.
2. Linear Layout Orientation
The orientation
property of a LinearLayout determines whether the child elements are arranged horizontally or vertically. By default, it is set to vertical.
- vertical: Arranges the child elements in a vertical column.
- horizontal: Arranges the child elements in a horizontal row.
3. Example of Vertical Linear Layout
Here’s an example of a vertical LinearLayout where child elements are arranged one below the other:
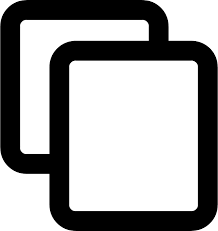
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
4. Example of Horizontal Linear Layout
Here’s an example of a horizontal LinearLayout where child elements are arranged side by side:
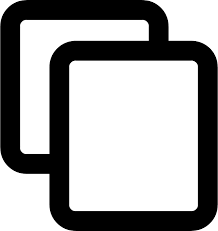
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
5. Weight in Linear Layout
The weight
attribute is used to allocate space between the child elements. When you use the android:layout_weight
attribute, the available space is divided between the child elements based on their respective weight. This is particularly useful for creating flexible layouts.
Example:
In the example below, we use the android:layout_weight
attribute to distribute the available space equally between two buttons:
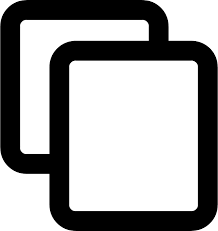
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button 1" />
<Button
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button 2" />
</LinearLayout>
Both buttons will take up equal space within the horizontal LinearLayout. The android:layout_width
is set to 0dp
to allow the layout weight to distribute the space.
6. Padding and Margins
Padding and margins can be used in LinearLayout to control the spacing between the layout and its content, as well as between child elements. Padding is the space inside the layout, while margins are the space outside the layout.
Example with Padding and Margin:
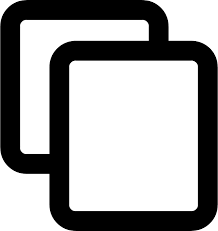
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp"
android:layout_margin="8dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!" />
</LinearLayout>
7. Nested LinearLayouts
In complex layouts, you might need to nest multiple LinearLayouts inside one another to create a more elaborate structure. This allows you to have a combination of horizontal and vertical orientations within the same layout.
Example of Nested LinearLayouts:
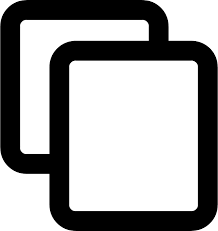
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Title" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2" />
</LinearLayout>
</LinearLayout>
8. Conclusion
The LinearLayout is a versatile and simple layout manager in Android. It is helpful when you need to align elements in a row or column. By using attributes like orientation, layout_weight, padding, and margin, you can create flexible and responsive layouts for your Android apps. In the next section, we will explore other layout managers like RelativeLayout and ConstraintLayout for more complex UI designs.
Relative Layout
1. What is Relative Layout?
A RelativeLayout is a type of layout in Android where the position of each child element is specified relative to other elements within the layout. It allows you to position elements in relation to one another, such as above, below, to the left, or to the right of other elements, making it more flexible than LinearLayout in terms of layout design.
2. Key Attributes of Relative Layout
In a RelativeLayout, you use various layout parameters to define how views are positioned relative to each other. Some of the most important attributes include:
- android:layout_alignParentTop: Aligns the view to the top of the parent.
- android:layout_alignParentBottom: Aligns the view to the bottom of the parent.
- android:layout_alignParentLeft: Aligns the view to the left of the parent.
- android:layout_alignParentRight: Aligns the view to the right of the parent.
- android:layout_centerInParent: Centers the view within the parent layout.
- android:layout_toLeftOf: Positions the view to the left of another specified view.
- android:layout_toRightOf: Positions the view to the right of another specified view.
- android:layout_below: Positions the view below another specified view.
- android:layout_above: Positions the view above another specified view.
3. Example of Relative Layout
Here is a simple example of a RelativeLayout where a TextView is aligned to the top of the parent and a Button is positioned below the TextView:
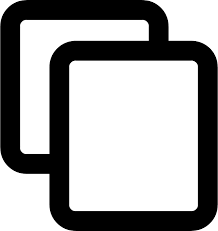
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
android:layout_below="@id/textView"
android:layout_centerHorizontal="true" />
</RelativeLayout>
4. Using Layout Relative to Other Views
In RelativeLayout, elements can be positioned relative to other views using their unique id
. For example, the Button in the above code is positioned below the TextView using the android:layout_below
attribute and referencing the TextView's ID (@id/textView).
5. Example: Aligning Multiple Views
Here is an example where multiple views are aligned relative to each other:
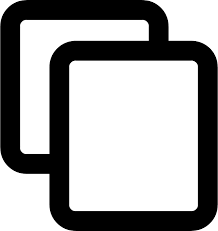
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Title"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start"
android:layout_below="@id/textView"
android:layout_alignParentLeft="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Exit"
android:layout_below="@id/textView"
android:layout_alignParentRight="true" />
</RelativeLayout>
6. Centering Views in Relative Layout
You can easily center views within a RelativeLayout using the android:layout_centerInParent
attribute. For example, to center a button both vertically and horizontally within the layout, you can use the following code:
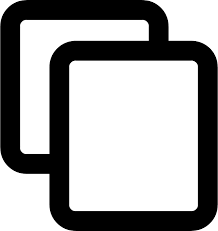
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Centered Button"
android:layout_centerInParent="true" />
7. Nested RelativeLayouts
Just like with other layouts, you can nest RelativeLayouts inside one another to create complex UI structures. This allows you to combine different layout strategies as needed to achieve the desired result.
Example of Nested RelativeLayouts:
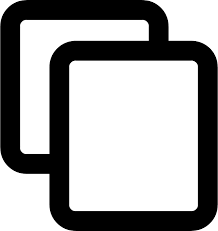
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Outer Layout"
android:layout_centerHorizontal="true"
android:layout_alignParentTop="true" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/textView">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Nested Button"
android:layout_centerInParent="true" />
</RelativeLayout>
</RelativeLayout>
8. Conclusion
RelativeLayout is a powerful layout manager in Android, enabling flexible positioning of views relative to one another. By using layout attributes such as android:layout_below
, android:layout_alignParentTop
, and android:layout_centerInParent
, you can easily create responsive layouts. In the next section, we will dive into other advanced layouts like ConstraintLayout for even more control over UI design.
Constraint Layout
1. What is ConstraintLayout?
ConstraintLayout is a flexible and powerful layout manager in Android that allows you to create complex layouts with a flat view hierarchy. Unlike other layouts such as LinearLayout and RelativeLayout, which rely on nested views, ConstraintLayout enables you to position and size views in a flat, more efficient structure. Views can be positioned relative to other views or parent containers using constraints.
2. Advantages of ConstraintLayout
- Flat View Hierarchy: Reduces the need for nested layouts, which can improve performance.
- Flexible Constraints: Views can be constrained to other views or the parent layout, allowing for complex layouts with fewer nested elements.
- Responsive Design: Easily adaptable to different screen sizes and orientations.
- Improved Performance: By avoiding deep view hierarchies, ConstraintLayout improves the rendering performance of your UI.
3. Key Attributes of ConstraintLayout
In ConstraintLayout, each view is positioned based on constraints relative to other views or the parent layout. Some common attributes used in ConstraintLayout are:
- app:layout_constraintTop_toTopOf: Constrains the top edge of the view to the top edge of another view or the parent.
- app:layout_constraintBottom_toBottomOf: Constrains the bottom edge of the view to the bottom edge of another view or the parent.
- app:layout_constraintStart_toStartOf: Constrains the start edge (left in LTR languages) of the view to the start edge of another view or the parent.
- app:layout_constraintEnd_toEndOf: Constrains the end edge (right in LTR languages) of the view to the end edge of another view or the parent.
- app:layout_constraintHorizontal_bias: Positions the view horizontally between its start and end constraints, with a bias value (0.0 for start, 1.0 for end).
- app:layout_constraintVertical_bias: Positions the view vertically between its top and bottom constraints, with a bias value (0.0 for top, 1.0 for bottom).
4. Example of ConstraintLayout
Here is a simple example of a ConstraintLayout where a TextView is constrained to the top and center of the parent layout, and a Button is constrained below the TextView:
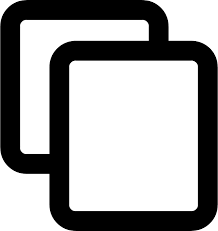
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, ConstraintLayout!"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
app:layout_constraintTop_toBottomOf="@id/textView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5" />
</androidx.constraintlayout.widget.ConstraintLayout>
5. Positioning Views Using Constraints
In the above example, the TextView is constrained to the top and horizontal center of the parent layout. The Button is constrained below the TextView and centered horizontally within the parent layout. The app:layout_constraintHorizontal_bias
attribute is used to control horizontal positioning between the start and end edges.
6. Guidelines in ConstraintLayout
Guidelines are invisible reference lines that help position views relative to them. They are useful for creating consistent layouts across different screen sizes. You can create horizontal or vertical guidelines and constrain views to them.
Example of Using a Guideline:
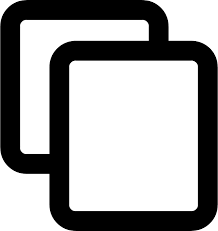
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline"
android:layout_width="wrap_content"
android:layout_height="match_parent"
app:layout_constraintGuidePercent="0.5"
android:orientation="vertical" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
app:layout_constraintStart_toStartOf="@id/guideline"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
7. Chains in ConstraintLayout
A chain is a group of views that are connected in a specific way to form a flexible structure. You can create horizontal or vertical chains, and within a chain, views can be arranged in various ways like spread, packed, or weighted.
Example of a Horizontal Chain:
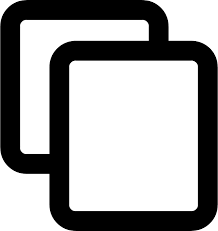
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
app:layout_constraintEnd_toStartOf="@id/button2"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
app:layout_constraintEnd_toStartOf="@id/button3"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 3"
app:layout_constraintStart_toEndOf="@id/button2"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
8. Conclusion
ConstraintLayout is an advanced, flexible, and powerful layout tool that helps create efficient and responsive user interfaces for Android apps. It allows you to position, size, and align views with great precision using constraints, guidelines, and chains. In the next section, we’ll explore advanced topics like handling different screen sizes and using custom views within ConstraintLayout.
Grid Layout
1. What is GridLayout?
GridLayout is a type of layout manager in Android that allows you to arrange views in a two-dimensional grid. It makes it easy to create complex layouts with rows and columns, similar to a table. GridLayout is ideal for applications that need to display content in a grid-like structure, such as calculators, photo galleries, or grid-based forms.
2. Advantages of GridLayout
- Two-Dimensional Layout: Allows for organizing views in rows and columns.
- Flexible: Views can span across multiple rows or columns, providing flexibility in creating complex layouts.
- Efficient: Reduces the need for nested layouts, which can improve performance.
- Easy to Align: Align views both horizontally and vertically with ease.
3. Key Attributes of GridLayout
GridLayout uses the following key attributes to manage its layout:
- android:rowCount: Sets the number of rows in the grid.
- android:columnCount: Sets the number of columns in the grid.
- android:orientation: Defines the orientation of the layout (either horizontal or vertical).
- android:layout_rowSpan: Defines how many rows a particular view should span.
- android:layout_columnSpan: Defines how many columns a particular view should span.
- android:layout_row: Specifies which row the view should be placed in.
- android:layout_column: Specifies which column the view should be placed in.
4. Example of GridLayout
Here is an example of a simple GridLayout with 2 rows and 2 columns. Each cell contains a Button:
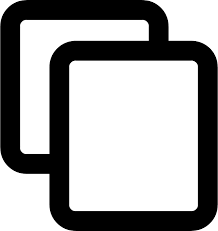
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="2"
android:rowCount="2">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="0" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="1" />
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="0" />
<Button
android:text="Button 4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="1" />
</GridLayout>
5. Spanning Cells in GridLayout
You can span a view across multiple rows or columns by using the android:layout_rowSpan and android:layout_columnSpan attributes.
Example of Spanning Views:
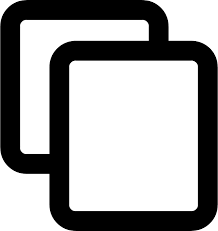
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="2"
android:rowCount="2">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="0"
android:layout_columnSpan="2" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="0" />
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="1" />
</GridLayout>
6. Aligning Views in GridLayout
You can align views within each cell of the grid by using the following attributes:
- android:gravity: Aligns the view within its own cell (e.g., center, start, end, top, bottom, etc.).
- android:layout_gravity: Aligns the view within the GridLayout container (e.g., center, top, left, etc.).
Example of View Alignment:
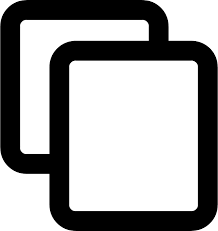
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="2"
android:rowCount="2">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="0"
android:gravity="center" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="1"
android:gravity="top|end" />
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="0"
android:layout_gravity="center" />
<Button
android:text="Button 4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="1"
android:layout_gravity="bottom|start" />
</GridLayout>
7. Conclusion
GridLayout is a great tool for creating flexible grid-based layouts that are easy to manage and modify. It allows you to arrange views in rows and columns, and offers powerful features like spanning and alignment to create complex user interfaces. In the next section, we will explore more advanced layout techniques and ways to handle different screen sizes effectively.
Table Layout
1. What is TableLayout?
TableLayout is a layout manager in Android that arranges its child views in rows and columns, similar to an HTML table. Unlike other layouts, TableLayout does not display borders or rows by default, but you can define them if needed. It’s useful for creating grid-based layouts where each row contains a set of views (such as forms, calculators, etc.) in a structured manner.
2. Advantages of TableLayout
- Grid-like Structure: Arranges views in rows and columns, making it easy to build structured UIs.
- Flexible: You can have uneven column sizes and different numbers of views in each row.
- Easy to Use: Simple syntax and layout compared to custom grids or other complex layouts.
- Alignment: Views are aligned in rows and columns, making the layout predictable and organized.
3. Key Attributes of TableLayout
TableLayout uses the following key attributes to manage its layout:
- android:stretchColumns: Defines which columns should stretch to fill the available space.
- android:shrinkColumns: Defines which columns should shrink to fit their content.
- android:collapseColumns: Defines which columns should be invisible.
4. Example of TableLayout
Here is an example of a simple TableLayout with 3 rows and 2 columns, where each cell contains a Button:
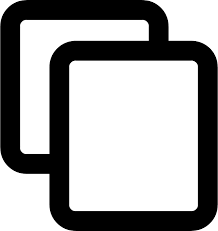
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical">
<TableRow>
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 4"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:text="Button 5"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 6"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
</TableLayout>
5. Spanning Cells in TableLayout
Just like with GridLayout, you can span a view across multiple rows or columns in TableLayout by using the android:layout_span attribute.
Example of Spanning Views:
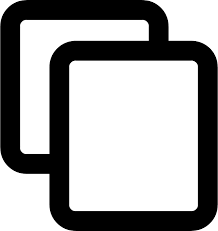
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical">
<TableRow>
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
<TableRow>
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_span="2" />
</TableRow>
<TableRow>
<Button
android:text="Button 5"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 6"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
</TableLayout>
6. Aligning Views in TableLayout
You can align views within each row of a TableLayout using the android:gravity attribute. This attribute allows you to control the alignment of child views within their parent cell (such as center, top, bottom, etc.).
Example of View Alignment:
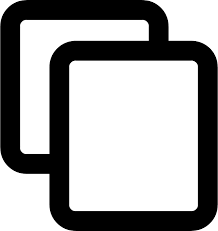
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical">
<TableRow android:gravity="center">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
<TableRow android:gravity="start">
<Button
android:text="Button 3"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 4"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
<TableRow android:gravity="end">
<Button
android:text="Button 5"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:text="Button 6"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableRow>
</TableLayout>
7. Conclusion
TableLayout is a simple and effective way to arrange views in rows and columns, providing a grid-like structure for your app's UI. It is a great choice for creating forms, grids, or any layout that requires a structured format. In the next section, we will dive into more advanced UI components and how to manage different screen sizes effectively in Android development.
Frame Layout
1. What is FrameLayout?
FrameLayout is a layout container in Android that is designed to display a single child view. It is useful when you need to stack multiple views on top of each other, where only one view is displayed at a time, or to create a placeholder for dynamic content. A typical use case for FrameLayout is in implementing container-like views, such as a fragment container or a background image with floating buttons.
2. Key Features of FrameLayout
- Simple Structure: FrameLayout can hold only one direct child, making it simple and lightweight.
- Layering Views: You can stack multiple child views within FrameLayout, and the views will be layered on top of each other.
- Positioning: The child views can be aligned using layout attributes like
android:layout_gravity
. - Useful for Dynamic UI: FrameLayout is commonly used for displaying dynamic content such as fragments or custom views.
3. Example of FrameLayout
This example demonstrates a simple FrameLayout with one child view (a Button) positioned at the center:
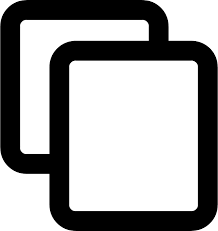
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:text="Click Me"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center" />
</FrameLayout>
4. Layering Multiple Views in FrameLayout
FrameLayout allows you to layer multiple views on top of each other. The child views will be stacked in the order they are declared in the XML. You can use the android:layout_gravity
attribute to control their alignment within the layout.
Example of Layering Views:
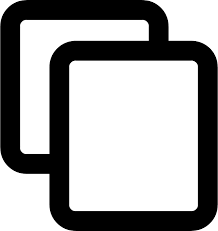
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:src="@drawable/background_image"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<Button
android:text="Click Me"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center" />
</FrameLayout>
5. Using FrameLayout for Fragments
FrameLayout is commonly used as a container for fragments in Android. You can dynamically replace or add fragments inside a FrameLayout during runtime using FragmentTransaction.
Example of Using FrameLayout for Fragments:
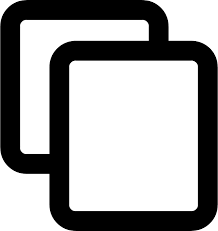
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent" />
In the Java/Kotlin code, you can dynamically add a fragment to this FrameLayout container like this:
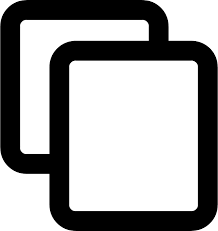
FragmentTransaction ft = getSupportFragmentManager().beginTransaction();
Fragment fragment = new MyFragment();
ft.replace(R.id.fragment_container, fragment);
ft.commit();
6. Layout Gravity in FrameLayout
The android:layout_gravity
attribute is used to position child views within a FrameLayout. It accepts values such as center
, top
, bottom
, left
, right
, fill
, etc.
Example of Using Layout Gravity:
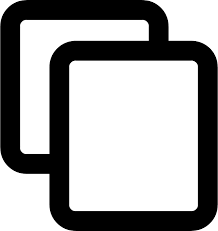
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:text="Top Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="top|center_horizontal" />
<Button
android:text="Bottom Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|center_horizontal" />
</FrameLayout>
7. Conclusion
FrameLayout is a simple but powerful layout container in Android that allows you to stack views on top of each other. It is ideal for use cases where you want to display a single view or multiple overlapping views. It’s commonly used in fragment transactions and dynamic UI elements. In the next section, we will explore other layout types to help you design more complex and responsive Android UIs.
TextView & EditText
1. TextView
TextView is a fundamental UI component in Android used to display text on the screen. It is often used to show static text, labels, titles, and other forms of content that do not require user interaction.
2. Key Features of TextView
- Displaying Text: TextView is used to display text of any size, color, or style.
- Text Styling: You can customize the font, size, and alignment of the text.
- Multi-line Support: TextView can display multiple lines of text and automatically wrap the text if it exceeds the width of the screen.
- TextView as a Link: TextView can also be used to display clickable links and handle user interactions like opening a URL.
3. Example of TextView
This example demonstrates a simple TextView with some formatted text:
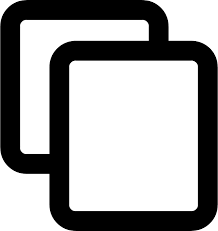
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!"
android:textSize="18sp"
android:textColor="#000000"
android:textStyle="bold"
android:gravity="center" />
4. EditText
EditText is a view used to allow users to input text. It is the basic input field in Android used for forms, search bars, text inputs, and other data collection purposes.
5. Key Features of EditText
- User Input: EditText allows users to type in text, and it supports different input types like text, numbers, passwords, etc.
- Text Editing: You can configure EditText to support features like multiline input, password fields, and custom input filters.
- Hint Text: You can set a hint text for EditText to guide the user on what type of input is expected.
- Text Masks: You can configure EditText to allow only certain types of characters, such as digits or email addresses, using input types and filters.
6. Example of EditText
This example demonstrates a simple EditText for user input:
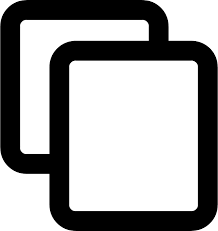
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter your name"
android:textSize="16sp"
android:inputType="textPersonName" />
7. Using TextView and EditText Together
TextView and EditText can be used together to create forms or interactive UI elements. For example, you might display a label with TextView and allow the user to input data using EditText.
Example of Using Both TextView and EditText:
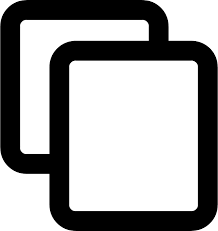
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/textLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enter your name:"
android:textSize="18sp"
android:textColor="#000000" />
<EditText
android:id="@+id/editTextName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Name"
android:textSize="16sp"
android:inputType="textPersonName" />
</LinearLayout>
8. Handling User Input from EditText
To retrieve user input from an EditText, you can use the getText()
method in your activity. Here’s how to get the text entered by the user:
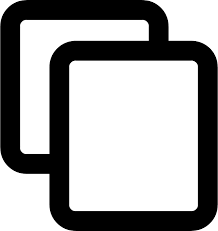
// Java code to get input from EditText
EditText editText = findViewById(R.id.editTextName);
String userInput = editText.getText().toString();
Similarly, in Kotlin:
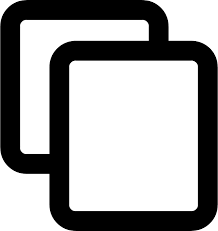
// Kotlin code to get input from EditText
val userInput = findViewById<EditText>(R.id.editTextName).text.toString()
9. Conclusion
TextView and EditText are essential UI components for displaying and collecting text in Android applications. While TextView is used for displaying static text, EditText allows users to input dynamic text. Both views can be easily customized for different input requirements, such as text size, color, and input type. In the next section, we will explore other UI components and layout techniques for creating interactive and responsive Android applications.
Button & ImageView
1. Button
Button is a user interface component that triggers actions when clicked by the user. It is used to execute tasks, navigate through screens, or submit data in Android applications.
2. Key Features of Button
- Click Event Handling: Buttons are primarily used to handle user clicks and perform actions.
- Customizable Appearance: You can customize the button's background, text color, text size, and more.
- Icons and Text: A button can display text, an icon, or both, depending on the design requirements.
- State Management: Buttons can have different states like pressed, focused, and normal, each of which can have different styles.
3. Example of Button
This example demonstrates a simple Button that displays text and triggers an action when clicked:
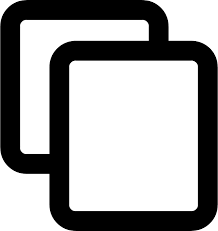
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
android:textSize="18sp"
android:background="#6200EE"
android:textColor="#FFFFFF" />
4. Handling Button Click Event
To handle the button click event, you can set an OnClickListener
in your activity or fragment:
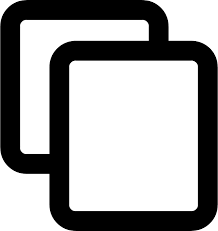
// Java code to handle button click
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Perform action when button is clicked
Toast.makeText(MainActivity.this, "Button clicked!", Toast.LENGTH_SHORT).show();
}
});
Similarly, in Kotlin:
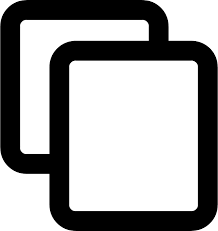
// Kotlin code to handle button click
val button: Button = findViewById(R.id.button)
button.setOnClickListener {
// Perform action when button is clicked
Toast.makeText(this, "Button clicked!", Toast.LENGTH_SHORT).show()
}
5. ImageView
ImageView is a UI component used to display images in Android applications. It is used for showing icons, pictures, and other graphical elements within your app’s interface.
6. Key Features of ImageView
- Image Display: ImageView allows you to display images in various formats such as PNG, JPEG, and GIF.
- Scalable Images: You can scale and adjust images to fit the screen size and layout, either by stretching or cropping.
- Clickable Images: ImageView can be used as a clickable element, such as a button or a link, by setting an
OnClickListener
.
7. Example of ImageView
This example demonstrates an ImageView that displays an image from the resources folder:
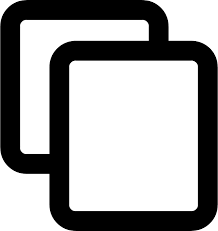
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/sample_image"
android:contentDescription="Sample Image" />
8. Adjusting Image Size
You can adjust the size of an image displayed in an ImageView using the layout_width
and layout_height
attributes:
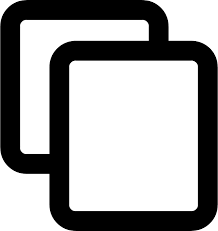
<ImageView
android:id="@+id/imageView"
android:layout_width="200dp"
android:layout_height="200dp"
android:src="@drawable/sample_image" />
9. ImageView with Click Event
Like buttons, ImageView can also trigger actions when clicked. You can set an OnClickListener
to handle the click event:
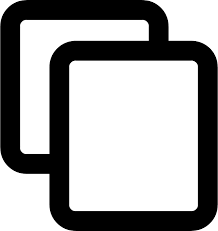
// Java code to handle image click
ImageView imageView = findViewById(R.id.imageView);
imageView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Perform action when image is clicked
Toast.makeText(MainActivity.this, "Image clicked!", Toast.LENGTH_SHORT).show();
}
});
Similarly, in Kotlin:
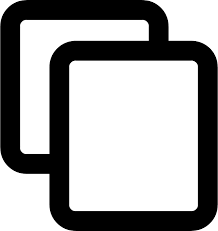
// Kotlin code to handle image click
val imageView: ImageView = findViewById(R.id.imageView)
imageView.setOnClickListener {
// Perform action when image is clicked
Toast.makeText(this, "Image clicked!", Toast.LENGTH_SHORT).show()
}
10. Conclusion
Button and ImageView are essential UI components for interacting with users in Android apps. Buttons allow users to trigger actions, while ImageView displays images and can be used interactively. Both components are highly customizable and can be styled to match the overall design of the app. In the next section, we will explore other UI elements and advanced topics in Android development.
ListView & RecyclerView
1. ListView
ListView is a view that displays a vertically-scrollable list of items. It's one of the most commonly used UI components for displaying a list of data in Android applications. ListView allows each item in the list to be clicked, and you can customize the layout of each item in the list.
2. Key Features of ListView
- Efficient Scrolling: ListView efficiently handles large datasets by recycling view items that are no longer visible.
- Item Clicks: ListView supports item click events, allowing users to interact with list items.
- Custom Layouts: You can define custom layouts for each item in the list by using an adapter.
3. Example of ListView
This example demonstrates how to create a ListView with a simple string array:
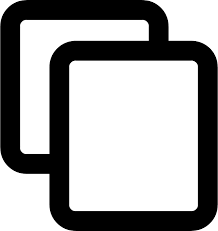
4. Adapter for ListView
To bind data to a ListView, you need to use an Adapter. Here's an example of using an ArrayAdapter:
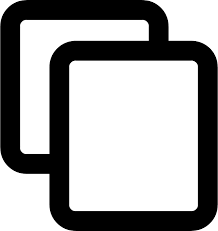
// Java code to set up ListView with ArrayAdapter
ListView listView = findViewById(R.id.listView);
String[] data = {"Item 1", "Item 2", "Item 3", "Item 4"};
ArrayAdapter adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, data);
listView.setAdapter(adapter);
5. Handling Item Clicks
To handle item clicks in ListView, set an OnItemClickListener
:
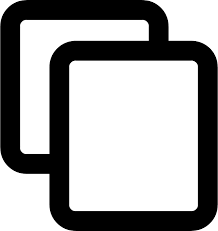
// Java code to handle item clicks
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView> parent, View view, int position, long id) {
// Handle the click event on the item
Toast.makeText(MainActivity.this, "Clicked: " + data[position], Toast.LENGTH_SHORT).show();
}
});
6. RecyclerView
RecyclerView is a more advanced and flexible version of ListView, introduced in Android Lollipop (API 21). RecyclerView provides better performance and more features, like support for different layouts (grid, staggered, etc.), item animations, and more.
7. Key Features of RecyclerView
- ViewHolder Pattern: RecyclerView uses the ViewHolder pattern to improve performance by avoiding frequent calls to
findViewById
. - Layouts: RecyclerView supports multiple layout types such as vertical lists, grids, and staggered grids.
- Item Animations: RecyclerView allows for advanced item animations, such as fade in/out and slide in/out.
8. Example of RecyclerView
This example demonstrates a basic implementation of RecyclerView with a simple item layout:
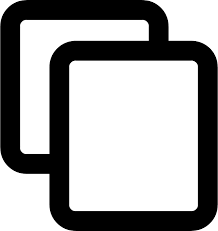
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
9. Adapter for RecyclerView
In RecyclerView, you need to create a custom adapter. Here's an example of a simple adapter:
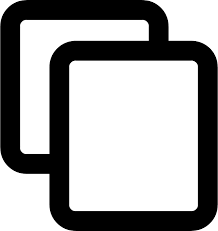
// Java code for RecyclerView Adapter
public class MyAdapter extends RecyclerView.Adapter {
private String[] data;
public MyAdapter(String[] data) {
this.data = data;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(android.R.layout.simple_list_item_1, parent, false);
return new MyViewHolder(view);
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.textView.setText(data[position]);
}
@Override
public int getItemCount() {
return data.length;
}
public class MyViewHolder extends RecyclerView.ViewHolder {
TextView textView;
public MyViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(android.R.id.text1);
}
}
}
10. Setting up RecyclerView
To use RecyclerView, you need to set up a LayoutManager
and an adapter:
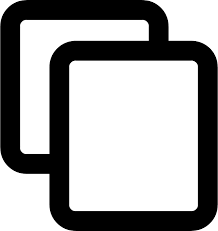
// Java code to set up RecyclerView
RecyclerView recyclerView = findViewById(R.id.recyclerView);
String[] data = {"Item 1", "Item 2", "Item 3", "Item 4"};
MyAdapter adapter = new MyAdapter(data);
recyclerView.setAdapter(adapter);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
11. Handling Item Clicks in RecyclerView
Item clicks in RecyclerView are handled using a click listener within the ViewHolder:
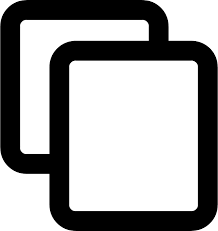
// Java code to handle item clicks in RecyclerView
public class MyAdapter extends RecyclerView.Adapter {
private String[] data;
private OnItemClickListener listener;
public MyAdapter(String[] data, OnItemClickListener listener) {
this.data = data;
this.listener = listener;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(android.R.layout.simple_list_item_1, parent, false);
return new MyViewHolder(view);
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.textView.setText(data[position]);
}
@Override
public int getItemCount() {
return data.length;
}
public class MyViewHolder extends RecyclerView.ViewHolder {
TextView textView;
public MyViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(android.R.id.text1);
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onItemClick(getAdapterPosition());
}
});
}
}
public interface OnItemClickListener {
void onItemClick(int position);
}
}
12. Conclusion
ListView is simple to use for small lists but lacks flexibility and performance optimizations for large data sets. RecyclerView is more advanced, offering better performance, flexibility, and custom layouts for large lists or complex views. Understanding both components and when to use them will help you build efficient and responsive Android applications.
Spinner, SeekBar & RatingBar
1. Spinner
Spinner is a widget that allows the user to select an item from a dropdown list. It is commonly used when there are multiple options and you want to save screen space.
2. Key Features of Spinner
- Dropdown Menu: Spinner displays options in a dropdown when clicked, allowing users to select one item.
- Customizable: You can customize the layout for each item in the dropdown list.
- Item Selection: Spinner allows you to handle item selection using an
OnItemSelectedListener
.
3. Example of Spinner
This example demonstrates how to use a Spinner with a simple array of options:
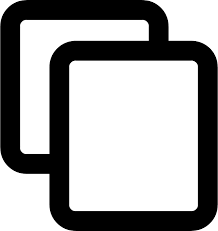
<Spinner
android:id="@+id/spinner"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
4. Adapter for Spinner
To populate the Spinner with data, you need to use an adapter. Here's an example of using an ArrayAdapter:
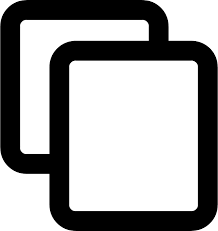
// Java code to set up Spinner with ArrayAdapter
Spinner spinner = findViewById(R.id.spinner);
String[] options = {"Option 1", "Option 2", "Option 3"};
ArrayAdapter adapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, options);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
spinner.setAdapter(adapter);
5. Handling Item Selection
To handle item selection in the Spinner, you need to set an OnItemSelectedListener
:
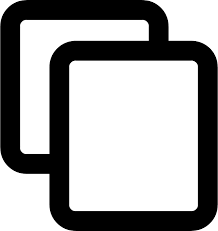
// Java code to handle item selection in Spinner
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView> parent, View view, int position, long id) {
// Handle selection
String selectedItem = options[position];
Toast.makeText(MainActivity.this, "Selected: " + selectedItem, Toast.LENGTH_SHORT).show();
}
@Override
public void onNothingSelected(AdapterView> parent) {
// Handle no selection
}
});
6. SeekBar
SeekBar is a widget that allows the user to select a value from a range, typically displayed as a horizontal slider. It is useful for adjusting values such as volume or brightness.
7. Key Features of SeekBar
- Range Selection: SeekBar allows the user to select a value within a predefined range.
- Progress Updates: SeekBar can show the current progress and update in real-time.
- Customizable: You can customize the look and feel of the SeekBar using attributes like color and size.
8. Example of SeekBar
This example demonstrates how to use SeekBar with progress tracking:
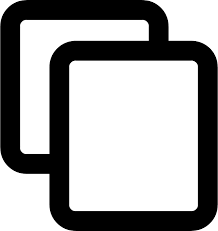
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="100"
android:progress="50" />
9. Handling SeekBar Progress
To track the progress of the SeekBar, you can set an OnSeekBarChangeListener
:
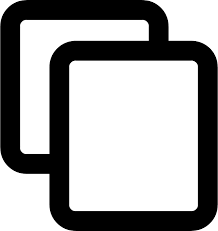
// Java code to handle SeekBar progress change
SeekBar seekBar = findViewById(R.id.seekBar);
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
// Handle progress change
Toast.makeText(MainActivity.this, "Progress: " + progress, Toast.LENGTH_SHORT).show();
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// Handle when touch starts
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// Handle when touch stops
}
});
10. RatingBar
RatingBar is a widget that allows the user to rate something using stars or other symbols. It’s commonly used in applications that require user ratings, such as review apps.
11. Key Features of RatingBar
- Star Ratings: RatingBar displays stars or custom icons to represent ratings.
- Customizable: You can customize the number of stars and the appearance of the RatingBar.
- Real-time Updates: RatingBar allows you to handle real-time changes as the user rates.
12. Example of RatingBar
This example demonstrates how to use RatingBar for user ratings:
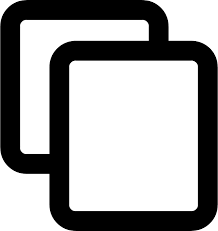
<RatingBar
android:id="@+id/ratingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="5"
android:rating="3"
android:stepSize="0.5" />
13. Handling RatingBar Changes
To track changes in the RatingBar, you can set an OnRatingBarChangeListener
:
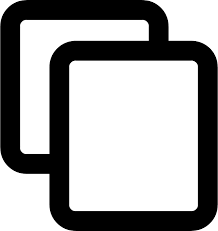
// Java code to handle RatingBar changes
RatingBar ratingBar = findViewById(R.id.ratingBar);
ratingBar.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) {
// Handle rating change
Toast.makeText(MainActivity.this, "Rating: " + rating, Toast.LENGTH_SHORT).show();
}
});
14. Conclusion
Spinner is ideal for presenting a list of options in a compact manner. SeekBar is useful for selecting values within a specified range, and RatingBar enables users to provide ratings with stars or other symbols. These widgets are essential for creating interactive and user-friendly Android applications.
Implicit and Explicit Intents
1. Implicit Intents
Implicit Intents are used when the specific component (activity, service, etc.) to handle the request is not specified. Instead, implicit intents allow the system to choose the best component based on the action, data, and category provided in the intent.
2. Key Features of Implicit Intents
- Action-based: You specify the action you want to perform (such as viewing, editing, or sending data) rather than the exact component to handle it.
- System Decision: The Android system decides which application or component should handle the intent, based on matching actions and categories.
- Data and Action: Implicit intents can contain data (such as a URL or file) and action (such as viewing or editing the data).
3. Example of Implicit Intent
This example demonstrates how to create an implicit intent to open a URL in a browser:
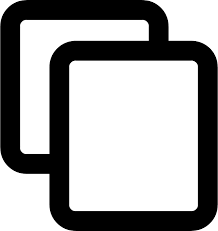
// Java code to open URL using Implicit Intent
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.example.com"));
startActivity(intent);
4. Handling Data in Implicit Intents
Implicit intents can also carry data. For example, you can send data (such as a contact's phone number) to another app for dialing:
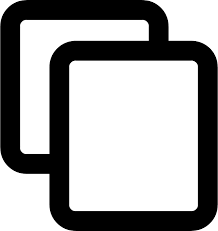
// Java code to dial a phone number using Implicit Intent
Intent intent = new Intent(Intent.ACTION_DIAL, Uri.parse("tel:1234567890"));
startActivity(intent);
5. Explicit Intents
Explicit Intents are used when you want to specify the exact component (such as a specific activity or service) that should handle the request. In this case, you directly provide the component's class name or package name.
6. Key Features of Explicit Intents
- Component-based: You specify the exact component (activity, service, etc.) that should be invoked.
- Direct Invocation: Explicit intents are useful when you want to start a specific activity within your app.
- Component Targeting: The target component is explicitly mentioned, so the system knows where to route the request.
7. Example of Explicit Intent
This example demonstrates how to create an explicit intent to start a specific activity in the same application:
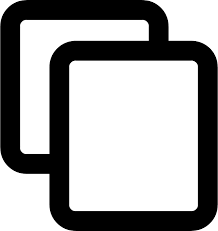
// Java code to start a specific activity using Explicit Intent
Intent intent = new Intent(this, SecondActivity.class);
startActivity(intent);
8. Passing Data with Explicit Intents
You can also pass data between activities using explicit intents. Here's how you can send data (e.g., a string) to another activity:
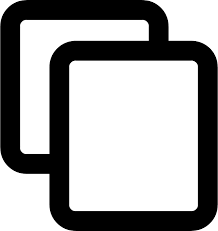
// Java code to pass data with Explicit Intent
Intent intent = new Intent(this, SecondActivity.class);
intent.putExtra("message", "Hello from MainActivity!");
startActivity(intent);
9. Receiving Data with Explicit Intents
In the receiving activity, you can retrieve the data passed using the getIntent()
method:
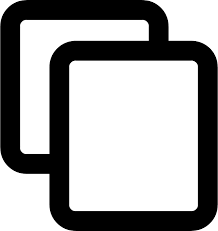
// Java code to receive data in SecondActivity
Intent intent = getIntent();
String message = intent.getStringExtra("message");
Toast.makeText(this, message, Toast.LENGTH_SHORT).show();
10. Difference Between Implicit and Explicit Intents
- Implicit Intents: Don't specify the target component, rely on the system to find a suitable component to handle the request based on the action and data.
- Explicit Intents: Specify the target component explicitly, typically used for starting activities or services within your own application.
11. Conclusion
Implicit Intents are ideal when you don't need to specify a particular component and want the system to choose the best one for the action. Explicit Intents are used when you want to specify a particular component in your application, offering more control over the flow of the app.
Passing Data Between Activities
1. Introduction
In Android, passing data between activities is a common task. You can pass data using Intents, which allow you to send values (such as strings, integers, or objects) from one activity to another.
2. Passing Data from the First Activity
To pass data from one activity to another, you can use the Intent
object with the putExtra()
method. You can send simple types like strings, integers, and booleans, or even more complex objects if they implement Parcelable
or Serializable
.
Example: Passing Data from MainActivity to SecondActivity
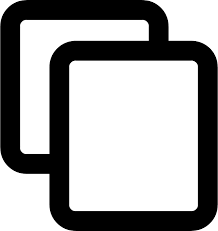
// Java code in MainActivity
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("username", "JohnDoe");
intent.putExtra("age", 25);
startActivity(intent);
3. Receiving Data in the Second Activity
In the receiving activity, you can retrieve the data using the getIntent()
method, followed by methods like getStringExtra()
or getIntExtra()
to access the data you passed.
Example: Receiving Data in SecondActivity
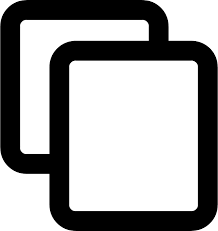
// Java code in SecondActivity
Intent intent = getIntent();
String username = intent.getStringExtra("username");
int age = intent.getIntExtra("age", -1); // Default value if not found
Toast.makeText(this, "Username: " + username + ", Age: " + age, Toast.LENGTH_SHORT).show();
4. Passing Different Data Types
You can pass various types of data using putExtra()
:
putExtra("key", "value")
– For strings.putExtra("key", intValue)
– For integers.putExtra("key", booleanValue)
– For booleans.putExtra("key", arrayList)
– For lists or arrays.putExtra("key", parcelableObject)
– For objects implementingParcelable
.
Example: Passing Different Data Types
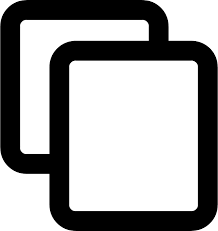
// Java code in MainActivity to pass various data types
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("username", "JaneDoe");
intent.putExtra("isRegistered", true);
intent.putExtra("balance", 100.50);
intent.putParcelableArrayListExtra("orders", orderList); // Passing a list of objects
startActivity(intent);
5. Handling Null Data
If the data passed is null, the methods like getStringExtra()
or getIntExtra()
will return null
or a default value (e.g., 0 or -1 for integers). Always check for null values before using the data received from the intent.
Example: Handling Null Data
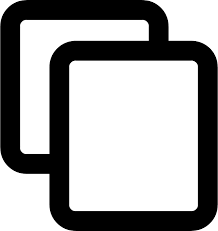
// Java code to handle null data in SecondActivity
String username = intent.getStringExtra("username");
if (username != null) {
Toast.makeText(this, "Hello, " + username, Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "No username provided", Toast.LENGTH_SHORT).show();
}
6. Passing Objects Between Activities
In Android, you can also pass objects between activities. To do this, the object must implement Parcelable
or Serializable
so that it can be properly serialized and deserialized.
Example: Passing a Parcelable Object
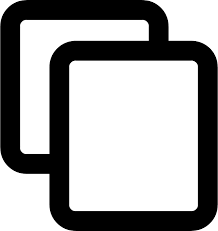
// Java code in MainActivity
MyObject myObject = new MyObject("John Doe", 30);
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("object", myObject); // Passing a Parcelable object
startActivity(intent);
Example: Defining the Parcelable Object
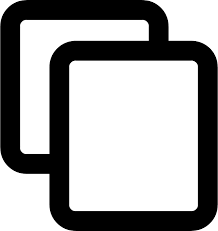
// Java code for MyObject class implementing Parcelable
public class MyObject implements Parcelable {
private String name;
private int age;
public MyObject(String name, int age) {
this.name = name;
this.age = age;
}
protected MyObject(Parcel in) {
name = in.readString();
age = in.readInt();
}
public static final Creator CREATOR = new Creator() {
@Override
public MyObject createFromParcel(Parcel in) {
return new MyObject(in);
}
@Override
public MyObject[] newArray(int size) {
return new MyObject[size];
}
};
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(name);
dest.writeInt(age);
}
@Override
public int describeContents() {
return 0;
}
}
7. Conclusion
Passing data between activities is a fundamental part of Android development. By using Intents and the putExtra()
and getExtra()
methods, you can easily share data of various types between different components of your app. Always be mindful of handling null data and consider using Parcelable
for passing objects efficiently.
Intent Filters and Broadcast Receivers
1. Introduction to Intent Filters and Broadcast Receivers
In Android, Intent Filters and Broadcast Receivers play a crucial role in enabling different components of an application to communicate with each other, as well as allowing apps to respond to system-wide events such as Wi-Fi changes, battery status, and more.
2. What is an Intent Filter?
An Intent Filter is a declaration in an Android app that specifies the types of intents that a component (such as an Activity, Service, or Broadcast Receiver) can respond to. Intent filters are declared in the AndroidManifest.xml
file and enable Android components to respond to specific actions, categories, and data types.
Example: Declaring an Intent Filter
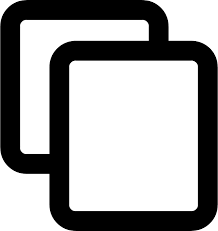
<activity android:name=".MainActivity">
<intent-filter>
</intent-filter>
</activity>
In this example, the activity MainActivity
will respond to any VIEW
action that matches the specified URL scheme (http://www.example.com
).
3. What is a Broadcast Receiver?
A Broadcast Receiver is a component that listens for and responds to broadcast messages from other applications or from the system. Broadcast Receivers allow your application to listen for system-wide events, such as when the device is charging, the Wi-Fi is turned on/off, or when a new SMS message is received.
Example: Creating a Broadcast Receiver
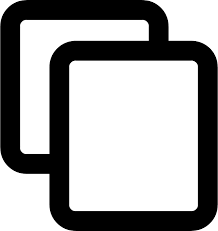
// Java code for a simple BroadcastReceiver
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (Intent.ACTION_AIRPLANE_MODE_CHANGED.equals(action)) {
boolean isAirplaneModeOn = intent.getBooleanExtra("state", false);
Toast.makeText(context, "Airplane Mode: " + isAirplaneModeOn, Toast.LENGTH_SHORT).show();
}
}
}
This BroadcastReceiver
listens for the ACTION_AIRPLANE_MODE_CHANGED
broadcast, indicating whether the airplane mode has been turned on or off.
4. Registering a Broadcast Receiver
Broadcast Receivers can be registered either statically (in the AndroidManifest.xml
) or dynamically (in Java/Kotlin code) depending on whether the receiver needs to listen to system-wide events at all times or only while the app is running.
Example: Static Registration in Manifest
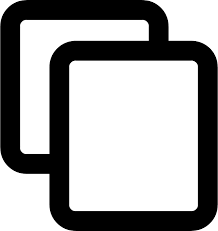
<receiver android:name=".MyReceiver">
<intent-filter>
</intent-filter>
</receiver>
Example: Dynamic Registration in Code
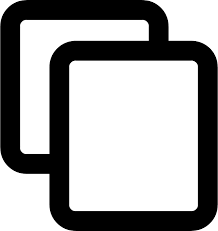
// Java code for dynamically registering a BroadcastReceiver
IntentFilter filter = new IntentFilter(Intent.ACTION_AIRPLANE_MODE_CHANGED);
MyReceiver receiver = new MyReceiver();
registerReceiver(receiver, filter);
In dynamic registration, you can register the receiver within an activity or service using registerReceiver()
. Don't forget to unregister it when you're done, using unregisterReceiver()
.
5. Sending a Broadcast
Apps can also send broadcasts to notify other apps about events. You can use the sendBroadcast()
method to send an implicit broadcast, or you can send a local broadcast using LocalBroadcastManager
for communication within your app.
Example: Sending a Broadcast
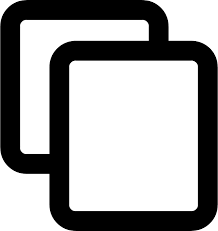
// Java code for sending a broadcast
Intent intent = new Intent("com.example.broadcast.MY_NOTIFICATION");
intent.putExtra("message", "Hello, this is a broadcast message!");
sendBroadcast(intent);
This code sends an implicit broadcast with a custom action com.example.broadcast.MY_NOTIFICATION
along with some data (the message).
6. Conclusion
Intent filters and Broadcast Receivers are powerful components in Android, enabling inter-application communication and responding to system-wide events. By using intent filters, you can specify which types of intents your components respond to, and by using broadcast receivers, you can listen for various system or application-wide broadcasts. Always be mindful of registering and unregistering your receivers to avoid memory leaks or unnecessary resource usage.
Activity Lifecycle: onCreate, onStart, onResume, etc.
1. Introduction to Activity Lifecycle
The Activity Lifecycle in Android defines the series of states an activity goes through from being created to being destroyed. Understanding the lifecycle helps developers manage an app’s memory, handle user interactions, and ensure smooth transitions between different screens. The key methods that correspond to these states are onCreate()
, onStart()
, onResume()
, onPause()
, onStop()
, and onDestroy()
.
2. Key Lifecycle Methods
Here are the most common lifecycle methods that Android calls as the activity transitions between states:
onCreate()
This method is called when the activity is first created. It's where you should initialize your activity, set up the user interface, and perform other one-time setup tasks. For example, you might inflate the layout and initialize variables here.
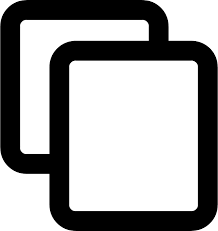
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialization code here
}
onStart()
This method is called when the activity is becoming visible to the user. You can use this method to start things that should only be running while the activity is visible, such as starting animations or initializing resources that are needed for the UI.
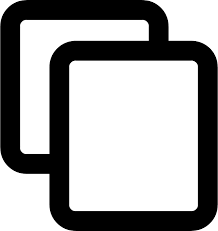
@Override
protected void onStart() {
super.onStart();
// Code to run when the activity becomes visible
}
onResume()
This method is called when the activity is in the foreground and interacting with the user. This is where you should resume any operations that were paused in onPause()
and start any continuous tasks, like playing music or starting a camera preview.
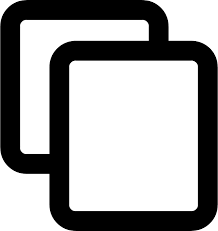
@Override
protected void onResume() {
super.onResume();
// Code to resume any ongoing tasks
}
onPause()
This method is called when the activity is no longer in the foreground. It’s used for saving data, pausing animations, or releasing resources that are only needed when the activity is visible. If the user is navigating to another activity or the app is about to go into the background, use this method to pause any ongoing tasks.
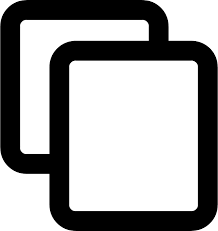
@Override
protected void onPause() {
super.onPause();
// Code to pause ongoing tasks, save data
}
onStop()
This method is called when the activity is no longer visible to the user. You should stop any background tasks that don’t need to run when the activity is in the background. This is a good place to release resources that are not needed until the activity is resumed.
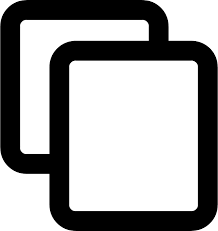
@Override
protected void onStop() {
super.onStop();
// Code to release resources, stop background tasks
}
onDestroy()
This method is called when the activity is about to be destroyed. It’s the final clean-up step where you should release any remaining resources and do any last-minute save operations if needed.
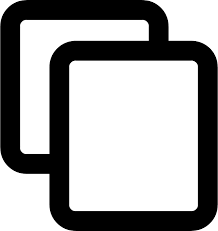
@Override
protected void onDestroy() {
super.onDestroy();
// Cleanup code
}
3. Full Activity Lifecycle
The activity lifecycle is not a simple one-way path. Activities can enter and exit states based on how the app is used, such as returning from a paused or stopped state when the user navigates back to the app. Here’s a breakdown of the entire cycle:
- onCreate() - When the activity is first created.
- onStart() - When the activity becomes visible.
- onResume() - When the activity is in the foreground and interacting with the user.
- onPause() - When another activity is partially covering the current one or when the app is about to be paused.
- onStop() - When the activity is no longer visible.
- onRestart() - When the activity is brought back to the foreground from the stopped state.
- onDestroy() - When the activity is about to be destroyed.
4. Conclusion
Understanding the activity lifecycle is essential for building efficient and responsive Android applications. Each method in the activity lifecycle provides an opportunity to manage resources, handle user input, save data, and more. By properly handling these methods, you can create apps that provide a better user experience and use system resources effectively.
Managing Activity States
1. Introduction to Managing Activity States
In Android, managing activity states is crucial for providing a seamless user experience, especially when the app is switched between the foreground and background. The Android operating system calls specific lifecycle methods to manage the state of an activity, allowing the app to handle memory efficiently, save user data, and maintain its integrity when it's resumed. Understanding how to manage activity states, such as handling memory and saving state, helps you build more efficient Android applications.
2. Saving and Restoring Activity State
When an activity is paused or stopped (e.g., when the user navigates to another app or the system reclaims memory), Android may destroy the activity to free up resources. In such cases, it’s important to save the activity's state so that it can be restored when the activity is resumed or recreated.
onSaveInstanceState()
The onSaveInstanceState()
method is called before the activity is paused or stopped. It allows you to save temporary state information that will be needed when the activity is recreated. You can store data in the Bundle
object, which can hold primitive data types, strings, arrays, or other objects.
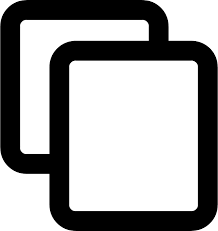
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("key", "value");
outState.putInt("score", 10);
}
onRestoreInstanceState()
The onRestoreInstanceState()
method is called after onStart()
when the activity is being restored from the saved state. It retrieves the state stored in the Bundle
object from onSaveInstanceState()
and restores the UI or variables accordingly.
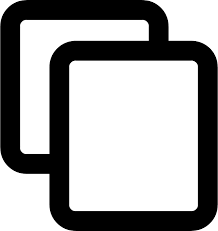
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
String value = savedInstanceState.getString("key");
int score = savedInstanceState.getInt("score");
}
3. Handling Configuration Changes
Configuration changes (such as device rotation, locale changes, or screen size adjustments) can cause Android to destroy and recreate the activity. By default, this leads to the activity being recreated, potentially losing the current state. To manage this, you can use onSaveInstanceState()
and onRestoreInstanceState()
or handle configuration changes manually.
Configuring Activity to Handle Changes
To prevent the activity from being recreated during configuration changes, you can specify in the AndroidManifest.xml
file that the activity should handle specific changes manually.
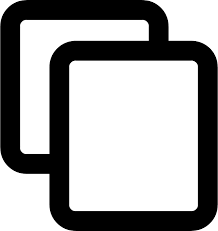
<activity android:name=".MainActivity"
android:configChanges="orientation|screenSize|keyboardHidden">
</activity>
The android:configChanges
attribute helps Android know which configuration changes should be handled by the activity itself instead of triggering a recreation.
4. Managing Activity Transitions
Activity transitions are important for creating smooth user experiences. When an activity is started or resumed, a transition animation can be shown to the user, and when an activity is paused or finished, you can also display an exit animation. Android provides built-in methods to manage these transitions.
Using Animations with Activities
Android offers several ways to customize activity transitions using the overridePendingTransition()
method. You can specify animations for the transition between activities, such as fading, sliding, or scaling effects.
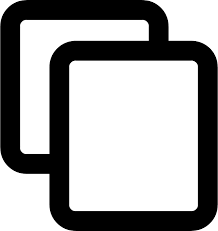
@Override
public void finish() {
super.finish();
overridePendingTransition(R.anim.slide_in_left, R.anim.slide_out_right);
}
5. Handling Background Activities
When an activity moves to the background, it may not be destroyed immediately by the system. The system may decide to keep it in memory for faster resumption. You should ensure that resources like network connections, database access, or sensors are released when the activity moves to the background to avoid excessive resource consumption. The onPause()
and onStop()
methods are used for releasing resources and saving data.
6. Conclusion
Managing activity states effectively is crucial for creating Android applications that are resource-efficient, responsive, and provide a smooth user experience. By understanding how to save and restore activity state, handle configuration changes, manage transitions, and handle background activities, you can prevent issues like data loss, slow performance, and unresponsive apps. Each method in the activity lifecycle provides an opportunity to optimize your app’s behavior and deliver a better experience for users.
Handling Configuration Changes
1. Introduction to Configuration Changes
In Android, configuration changes occur when there are modifications to the device’s configuration, such as screen orientation changes, language/locale changes, or screen size adjustments. By default, Android will destroy and recreate the activity when such changes happen, which can cause the loss of UI data or application state. Understanding how to handle configuration changes properly ensures that your app provides a consistent user experience across different device configurations.
2. Common Configuration Changes
Some of the most common configuration changes in Android include:
- Screen orientation change (portrait to landscape or vice versa)
- Screen size changes (e.g., switching from a small to a large screen)
- Language or locale change
- Keyboard availability (e.g., on-screen keyboard showing or hiding)
- Font size change
3. Default Behavior of Configuration Changes
By default, Android handles configuration changes by destroying and recreating the activity. This process can be costly in terms of performance and user experience if not handled properly. When an activity is recreated due to a configuration change, the system calls the onSaveInstanceState()
and onRestoreInstanceState()
methods, allowing the app to save and restore its state.
4. Handling Configuration Changes Manually
To prevent Android from destroying and recreating an activity during configuration changes, you can configure your activity to handle specific changes manually. This can be done in the AndroidManifest.xml
file using the android:configChanges
attribute.
Configuring Activities to Handle Configuration Changes
You can specify which configuration changes should not cause the activity to be recreated by listing them in the android:configChanges
attribute. When a configuration change occurs, the activity is notified via the onConfigurationChanged()
method, where you can handle the change manually.
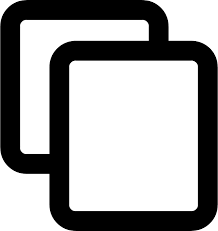
<activity android:name=".MainActivity"
android:configChanges="orientation|screenSize|keyboardHidden|locale">
</activity>
In the example above, the activity is configured to handle changes in orientation, screen size, keyboard visibility, and locale without being recreated.
Handling Configuration Changes in onConfigurationChanged()
If you choose to handle a configuration change manually, the system calls onConfigurationChanged()
when the change occurs. You can override this method to perform specific tasks related to the configuration change, such as updating the layout or changing resources dynamically.
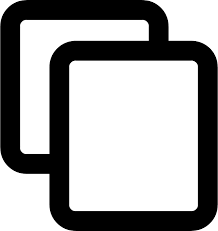
@Override
public void onConfigurationChanged(Configuration newConfig) {
super.onConfigurationChanged(newConfig);
if (newConfig.orientation == Configuration.ORIENTATION_LANDSCAPE) {
// Handle landscape orientation change
} else if (newConfig.orientation == Configuration.ORIENTATION_PORTRAIT) {
// Handle portrait orientation change
}
}
5. Best Practices for Handling Configuration Changes
While handling configuration changes manually can be useful, it’s important to carefully consider the user experience. The best practices include:
- Use
onSaveInstanceState()
andonRestoreInstanceState()
to save and restore activity state, especially for simple cases where data is lost during recreation. - Use
android:configChanges
only for changes that your app can handle properly without requiring a full activity restart. - When handling orientation changes, consider optimizing your UI for different screen sizes or orientations instead of simply adjusting the layout.
- Test the app extensively on different devices and configurations to ensure a smooth user experience across all scenarios.
6. Example: Handling Orientation Change
In the example below, the app handles orientation changes without restarting the activity. The onConfigurationChanged()
method is used to change the layout based on the current screen orientation.
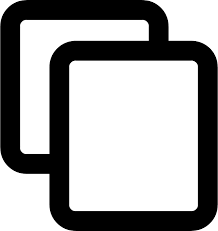
@Override
public void onConfigurationChanged(Configuration newConfig) {
super.onConfigurationChanged(newConfig);
if (newConfig.orientation == Configuration.ORIENTATION_LANDSCAPE) {
setContentView(R.layout.activity_landscape);
} else if (newConfig.orientation == Configuration.ORIENTATION_PORTRAIT) {
setContentView(R.layout.activity_portrait);
}
}
7. Conclusion
Handling configuration changes is an essential part of developing robust Android applications. By default, Android destroys and recreates activities during configuration changes, but developers can manage these changes manually to avoid unnecessary overhead. By properly using methods such as onConfigurationChanged()
, onSaveInstanceState()
, and onRestoreInstanceState()
, developers can ensure that their apps provide a consistent user experience across different device configurations.
Introduction to Fragments
1. What are Fragments?
Fragments are modular sections of an activity in Android. They represent a portion of the user interface (UI) in an activity, and they can be thought of as "sub-activities" that manage their own layout and behavior. Fragments allow for more flexible and reusable UI components, especially in devices with varying screen sizes, such as tablets and smartphones.
2. Why Use Fragments?
Fragments provide several advantages in Android development:
- UI Reusability: Fragments enable the reuse of UI components within different activities and layouts.
- Flexible UI Design: They are ideal for designing flexible and dynamic user interfaces, especially on devices with large screens like tablets.
- Modular Code: By separating UI and behavior into fragments, your code becomes more modular and easier to maintain.
- Dynamic Layouts: Fragments allow dynamic handling of layouts based on the available screen space or user interaction.
3. Fragment Lifecycle
Fragments have their own lifecycle, distinct from the activity lifecycle, but they are also tied to the activity that contains them. The fragment lifecycle includes methods such as onAttach()
, onCreateView()
, onActivityCreated()
, and others. These methods are used to manage the fragment's state and handle changes in the fragment's UI.
The fragment lifecycle is as follows:
- onAttach(): Called when the fragment is associated with its parent activity.
- onCreateView(): Called to inflate the fragment’s UI. This is where the fragment's layout is defined.
- onActivityCreated(): Called when the activity's
onCreate()
method has finished. - onStart(), onResume(): Called when the fragment is visible and interacting with the user.
- onPause(), onStop(): Called when the fragment is no longer interacting with the user.
- onDestroyView(): Called when the fragment’s view is being destroyed.
- onDetach(): Called when the fragment is no longer associated with its activity.
4. Creating a Fragment
To create a fragment, you need to define a class that extends Fragment
and override the required lifecycle methods. Here’s a simple example of creating a fragment that displays a text message:
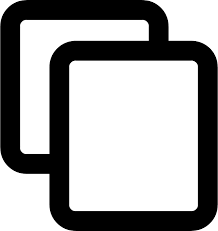
public class MyFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_my, container, false);
}
}
In the example above, the fragment’s UI is defined in the layout file fragment_my.xml
.
5. Adding a Fragment to an Activity
Fragments can be added to an activity either statically or dynamically.
Static Fragment
You can add a fragment statically by including it in the activity's layout file. Here’s an example of how to declare a fragment statically:
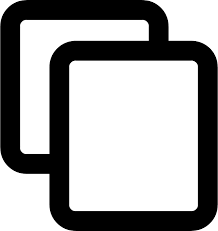
<fragment
android:id="@+id/myFragment"
android:name="com.example.myapp.MyFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
Dynamic Fragment
To add a fragment dynamically, use the FragmentTransaction
class in the activity. Here’s an example:
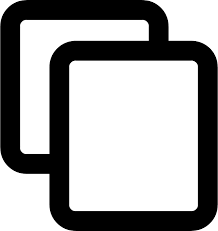
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, new MyFragment());
transaction.commit();
In this example, the fragment is dynamically added by replacing a container view with the new fragment.
6. Communication Between Fragments
Fragments can communicate with each other or with their parent activity. There are several ways to do this:
- Using a Shared ViewModel: Fragments can share a ViewModel to communicate and share data.
- Using the Parent Activity: A fragment can call methods in its parent activity to communicate with other fragments.
- Interface Callbacks: A fragment can define an interface and notify the activity to communicate with other fragments.
7. Example: Fragment Communication
Here’s an example of how fragments can communicate using an interface:
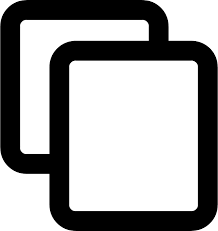
// Fragment 1: Defining an interface
public interface OnDataPass {
void onDataPass(String data);
}
// Fragment 1: Passing data to Fragment 2
public class Fragment1 extends Fragment {
OnDataPass dataPasser;
@Override
public void onAttach(Context context) {
super.onAttach(context);
dataPasser = (OnDataPass) context; // The parent activity must implement this interface
}
public void passData(String data) {
dataPasser.onDataPass(data); // Passing data to the activity
}
}
In this example, Fragment1 defines an interface to pass data to its parent activity, which will handle the communication to other fragments.
8. Conclusion
Fragments are powerful tools for building flexible and efficient UIs in Android. They allow for modular, reusable UI components and enable developers to design dynamic layouts that adapt to different screen sizes and orientations. Understanding fragments, their lifecycle, and how to manage their communication with activities and other fragments is crucial for creating modern Android apps.
Fragment Lifecycle
1. Introduction to Fragment Lifecycle
The fragment lifecycle refers to the various stages a fragment goes through from when it is created to when it is destroyed. Understanding the fragment lifecycle is essential for managing resources, handling UI updates, and ensuring efficient app performance. The fragment lifecycle methods are closely tied to the activity lifecycle, but fragments have their own independent set of lifecycle methods.
2. Fragment Lifecycle Methods
Here are the key fragment lifecycle methods:
- onAttach(): Called when the fragment is first attached to its activity. This is the first method in the lifecycle.
- onCreate(): Called when the fragment is created. This is where you initialize resources that will not depend on the fragment’s view.
- onCreateView(): Called to create the view hierarchy for the fragment. This is where you inflate the fragment’s layout.
- onActivityCreated(): Called when the activity’s
onCreate()
method has completed. At this point, the fragment’s view has been created. - onStart(): Called when the fragment becomes visible to the user, typically after
onCreateView()
. - onResume(): Called when the fragment is fully interactive and visible to the user.
- onPause(): Called when the fragment is no longer in the foreground but is still partially visible (e.g., when another fragment or activity comes into view).
- onStop(): Called when the fragment is no longer visible to the user.
- onDestroyView(): Called when the fragment’s view is being destroyed. This is where you should clean up any resources associated with the fragment’s view.
- onDestroy(): Called when the fragment is being removed or the activity is being destroyed. This method is used to clean up non-view-related resources.
- onDetach(): Called when the fragment is detached from its activity. This is the final lifecycle method.
3. Fragment Lifecycle Flow
The following sequence shows the general flow of a fragment’s lifecycle:
- onAttach() → onCreate() → onCreateView() → onActivityCreated() → onStart() → onResume()
- onPause() → onStop() → onDestroyView() → onDestroy() → onDetach()
These methods are called in a specific order as the fragment goes through its lifecycle stages. The exact sequence can vary based on how the fragment is added or removed from the activity.
4. Fragment Lifecycle Example
Here’s an example that demonstrates the typical usage of the fragment lifecycle methods:
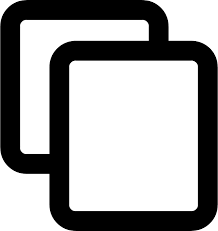
public class MyFragment extends Fragment {
@Override
public void onAttach(Context context) {
super.onAttach(context);
Log.d("Fragment", "onAttach() called");
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Log.d("Fragment", "onCreate() called");
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
Log.d("Fragment", "onCreateView() called");
return inflater.inflate(R.layout.fragment_my, container, false);
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
Log.d("Fragment", "onActivityCreated() called");
}
@Override
public void onStart() {
super.onStart();
Log.d("Fragment", "onStart() called");
}
@Override
public void onResume() {
super.onResume();
Log.d("Fragment", "onResume() called");
}
@Override
public void onPause() {
super.onPause();
Log.d("Fragment", "onPause() called");
}
@Override
public void onStop() {
super.onStop();
Log.d("Fragment", "onStop() called");
}
@Override
public void onDestroyView() {
super.onDestroyView();
Log.d("Fragment", "onDestroyView() called");
}
@Override
public void onDestroy() {
super.onDestroy();
Log.d("Fragment", "onDestroy() called");
}
@Override
public void onDetach() {
super.onDetach();
Log.d("Fragment", "onDetach() called");
}
}
In this example, each lifecycle method is overridden to log the corresponding event. You can see how the fragment goes through each stage of its lifecycle as the app runs.
5. Handling Fragment Lifecycle Changes
Managing fragment lifecycle changes is crucial, especially when dealing with configuration changes like screen rotations. You can use the onSaveInstanceState()
method to store the fragment’s state and restore it when the fragment is recreated. This is particularly important for maintaining the fragment's UI and state across activity or configuration changes.
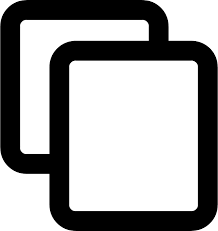
// Saving fragment state
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("key", "value"); // Save data to outState
}
// Restoring fragment state
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
if (savedInstanceState != null) {
String value = savedInstanceState.getString("key"); // Retrieve saved data
}
}
In this example, the fragment’s state is saved and restored during configuration changes, ensuring that the fragment behaves correctly when the activity is recreated.
6. Conclusion
Understanding the fragment lifecycle is essential for building efficient and responsive Android apps. By properly managing fragments and their lifecycle methods, you can ensure that your app behaves correctly when activities and fragments are created, paused, resumed, or destroyed. Fragment lifecycle methods give you the control you need to manage resources, UI updates, and state preservation effectively.
Dynamic Fragment Transactions
1. Introduction to Dynamic Fragment Transactions
Dynamic fragment transactions refer to the process of adding, removing, or replacing fragments dynamically at runtime. Unlike static fragments, which are defined in the XML layout file, dynamic fragments allow for more flexible and responsive UIs that can adapt based on user interaction or app state changes.
2. Fragment Transactions Overview
Fragment transactions are used to modify the fragment stack, allowing you to perform operations like adding, removing, or replacing fragments within an activity. These operations are performed using the FragmentTransaction
class.
3. Basic Steps of a Fragment Transaction
- Begin a Transaction: Start the transaction by calling
FragmentTransaction.beginTransaction()
. - Perform Operations: Use methods like
add()
,remove()
, orreplace()
to perform the desired fragment operation. - Commit the Transaction: Finally, call
commit()
to apply the transaction. Optionally, you can usecommitNow()
to apply immediately.
4. Example: Adding a Fragment Dynamically
Here's how you can add a fragment dynamically to your activity using a FragmentTransaction
:
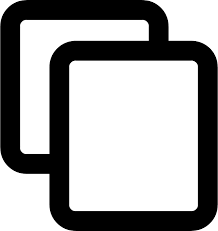
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = new MyFragment();
transaction.add(R.id.fragment_container, fragment); // Add the fragment to the container
transaction.addToBackStack(null); // Optional: Add the transaction to the back stack
transaction.commit(); // Commit the transaction to apply changes
In this example, we create a new instance of MyFragment
and add it to the container defined in the activity's layout. The transaction is then committed to apply the changes.
5. Example: Replacing a Fragment Dynamically
You can also replace an existing fragment with a new one by using the replace()
method:
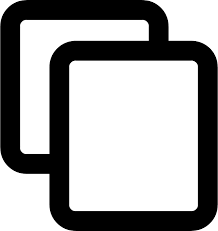
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment newFragment = new MyFragment();
transaction.replace(R.id.fragment_container, newFragment); // Replace the existing fragment
transaction.addToBackStack(null); // Optional: Add the transaction to the back stack
transaction.commit(); // Commit the transaction
In this case, the existing fragment in the R.id.fragment_container
is replaced with the new fragment.
6. Example: Removing a Fragment Dynamically
To remove a fragment, you can use the remove()
method:
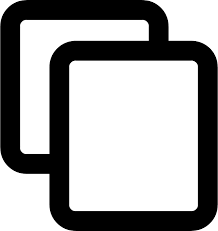
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = getSupportFragmentManager().findFragmentByTag("MyFragmentTag");
if (fragment != null) {
transaction.remove(fragment); // Remove the fragment from the container
transaction.commit(); // Commit the transaction
}
This code looks for a fragment by its tag and removes it from the UI.
7. Using the Fragment Back Stack
The fragment back stack allows you to add fragments to a stack so that you can navigate backward through fragment transactions. When a user presses the back button, the fragments are popped off the stack in reverse order.
To add a transaction to the back stack, call addToBackStack()
on the FragmentTransaction
instance:
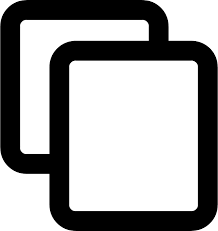
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, new MyFragment());
transaction.addToBackStack(null); // Add the transaction to the back stack
transaction.commit(); // Commit the transaction
When the user presses the back button, the previous fragment will be restored from the back stack.
8. Example: Pop a Fragment from the Back Stack
To pop a fragment from the back stack, use the popBackStack()
method:
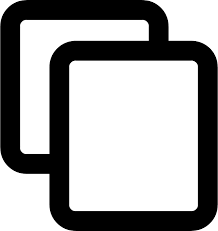
getSupportFragmentManager().popBackStack(); // Pop the top fragment off the back stack
This removes the top fragment from the back stack and restores the previous fragment.
9. Example: Handling Fragment Transactions with Animations
Fragment transactions can be enhanced with animations. You can define custom enter, exit, and pop animations like this:
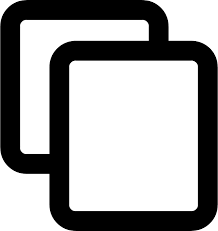
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.setCustomAnimations(R.anim.enter, R.anim.exit, R.anim.pop_enter, R.anim.pop_exit);
transaction.replace(R.id.fragment_container, new MyFragment());
transaction.addToBackStack(null);
transaction.commit();
In this example, we specify custom animations for entering and exiting fragments, as well as popping fragments from the back stack.
10. Conclusion
Dynamic fragment transactions provide a powerful way to modify the user interface during runtime, allowing for flexible and responsive designs. Understanding how to add, replace, remove, and manage fragments dynamically is essential for building modern Android applications. By using fragment transactions effectively, you can create a seamless user experience with easy navigation between different fragments in your app.
Using Fragments with Activities
1. Introduction to Using Fragments with Activities
Fragments allow you to build more flexible and modular user interfaces within an Android application. A fragment is a reusable UI component or behavior that can be embedded within an activity. Using fragments with activities enables you to create dynamic, multi-pane UIs that can adapt to different screen sizes, such as tablets and smartphones.
2. Adding a Fragment to an Activity
To use a fragment in an activity, you need to add it to the activity’s layout. This can be done statically (via XML) or dynamically (via code at runtime). Here's an example of adding a fragment statically:
In the activity's XML layout:
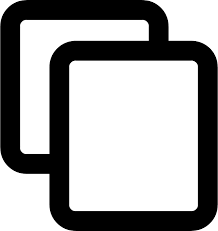
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent" />
In the activity’s Java code:
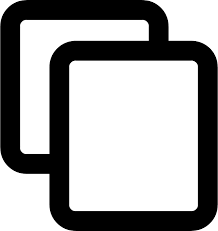
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = new MyFragment();
transaction.add(R.id.fragment_container, fragment); // Add fragment to activity
transaction.commit();
In this example, the fragment is added to a FrameLayout
container in the activity’s layout. The transaction is committed to display the fragment inside the activity.
3. Dynamically Adding a Fragment to an Activity
To add a fragment dynamically at runtime, use the FragmentTransaction
class. This allows you to add, replace, or remove fragments based on user actions or other conditions:
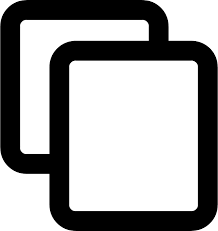
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = new MyFragment();
transaction.replace(R.id.fragment_container, fragment); // Replace fragment dynamically
transaction.addToBackStack(null); // Optional: Add to back stack
transaction.commit(); // Apply changes
The replace()
method is used to replace any existing fragment with a new one, and addToBackStack()
ensures that the user can navigate back to the previous fragment.
4. Communicating Between Activities and Fragments
Fragments can communicate with their parent activity and other fragments. Communication can be achieved using interfaces or directly manipulating fragment instances.
Using an Interface for Communication
To make a fragment communicate with its activity, define an interface in the fragment, and implement it in the activity. This allows the fragment to send data or events to the activity:
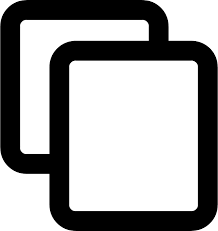
// Inside the Fragment
public class MyFragment extends Fragment {
public interface OnFragmentInteractionListener {
void onFragmentInteraction(String data);
}
private OnFragmentInteractionListener mListener;
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString() + " must implement OnFragmentInteractionListener");
}
}
// Trigger the event when needed
public void sendData(String data) {
if (mListener != null) {
mListener.onFragmentInteraction(data);
}
}
}
In the activity, implement the interface:
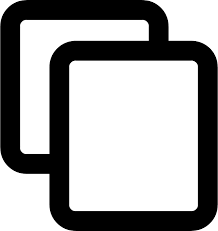
public class MainActivity extends AppCompatActivity implements MyFragment.OnFragmentInteractionListener {
@Override
public void onFragmentInteraction(String data) {
// Handle interaction from fragment
Toast.makeText(this, "Received data: " + data, Toast.LENGTH_SHORT).show();
}
}
This approach helps in decoupling the fragment and activity logic. The fragment sends data or events to the activity through the interface methods.
Direct Communication
Alternatively, you can directly access fragment instances from the activity and invoke methods. For example:
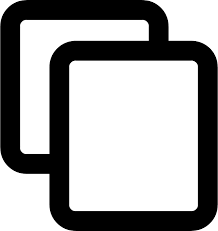
// Accessing fragment directly from activity
MyFragment fragment = (MyFragment) getSupportFragmentManager().findFragmentById(R.id.fragment_container);
fragment.sendData("Hello, Activity!");
However, this approach is less flexible compared to using interfaces, and it tightly couples the activity and fragment.
5. Removing a Fragment from an Activity
To remove a fragment from the activity, you can use the FragmentTransaction.remove()
method. Here's an example of removing a fragment dynamically:
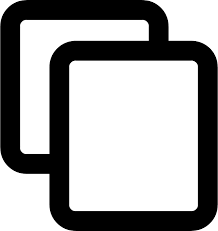
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
MyFragment fragment = (MyFragment) getSupportFragmentManager().findFragmentById(R.id.fragment_container);
if (fragment != null) {
transaction.remove(fragment); // Remove the fragment
transaction.commit(); // Commit the transaction
}
In this example, the fragment is removed from the container, and the transaction is committed to apply the changes.
6. Managing Fragments in the Back Stack
Using the back stack with fragments allows for better navigation within your app. When a fragment is added or replaced, you can choose to add the transaction to the back stack. This enables users to use the device’s back button to navigate back to previous fragments:
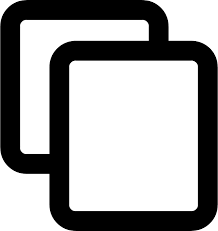
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, new MyFragment());
transaction.addToBackStack(null); // Add to back stack
transaction.commit();
This allows the fragment to be popped off the back stack when the user presses the back button, returning to the previous fragment.
7. Conclusion
Using fragments with activities is a powerful way to build flexible and reusable UI components in Android. Fragments can be added, removed, and replaced dynamically, allowing for an adaptive user experience. By managing communication between activities and fragments and using the back stack, you can create seamless navigation and interactions within your app.