What is Selenium?
Selenium is an open-source framework used to automate web browser interactions. It allows developers and testers to simulate real user actions such as clicking, typing, and navigating across web pages, making it an essential tool for functional and regression testing of web applications.
History of Selenium
Selenium was originally developed by Jason Huggins in 2004 as a JavaScript library to automate repetitive testing tasks. Over time, it evolved into a robust suite of tools, including Selenium IDE, Selenium WebDriver, and Selenium Grid, which support multiple programming languages, browsers, and operating systems.
Features of Selenium
Below are the key features that make Selenium a popular choice for web automation testing:
Feature | Description |
---|---|
Cross-Browser Compatibility | Selenium supports testing on all major browsers, including Chrome, Firefox, Safari, and Edge. |
Multi-Language Support | Selenium allows you to write test scripts in multiple programming languages like Java, Python, C#, Ruby, and JavaScript. |
Platform Independence | With Selenium, you can execute your tests on various operating systems such as Windows, macOS, and Linux. |
Integration with Other Tools | Selenium integrates seamlessly with CI/CD tools like Jenkins and testing frameworks like TestNG and JUnit. |
Setting Up Selenium
To start using Selenium, follow these steps:
- Download and install the required browser driver (e.g., ChromeDriver for Chrome).
- Install the Selenium library for your preferred programming language. For Python, you can run
pip install selenium
. - Set up your development environment and import the Selenium library into your project.
Code Example: Automating a Web Search
Here’s a simple example of using Selenium with Python to automate a Google search:
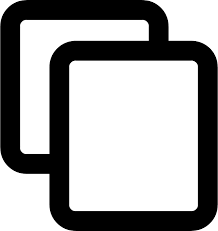
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Set up the WebDriver for Chrome
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
# Open Google
driver.get("https://www.google.com")
# Locate the search box, enter a query, and press Enter
search_box = driver.find_element("name", "q")
search_box.send_keys("What is Selenium?")
search_box.send_keys(Keys.RETURN)
# Close the browser
driver.quit()
Diagram: Selenium Workflow
The diagram below illustrates the workflow of Selenium WebDriver:

This diagram shows how Selenium interacts with the browser driver and the browser itself to automate web testing tasks.
History and Evolution of Selenium
Selenium has undergone significant evolution since its inception, growing from a simple JavaScript library into a comprehensive suite of tools for web automation. Its journey reflects its adaptability and the growing demand for automated testing in web development.
Origin of Selenium
Selenium was initially developed in 2004 by Jason Huggins while working at ThoughtWorks. It was designed as an internal tool to automate repetitive testing tasks for web applications. Originally named "JavaScriptTestRunner," it was later renamed Selenium.
Milestones in Selenium's Development
Key milestones in Selenium’s history include:
- 2004: Launch of the first version of Selenium, focused on automating browser-based testing using JavaScript.
- 2007: Introduction of Selenium Remote Control (RC), allowing test scripts to be written in multiple programming languages.
- 2008: Simon Stewart created WebDriver, which offered better performance and browser compatibility than Selenium RC.
- 2009: Selenium WebDriver and Selenium RC merged to form Selenium 2, combining the best features of both tools.
- 2016: Selenium 3 was released, focusing on stability and deprecating Selenium RC.
- 2021: Selenium 4 introduced significant updates, including W3C WebDriver standardization and support for modern web capabilities.
Components of Selenium
Selenium evolved into a suite of tools, each serving a specific purpose in web automation:
Component | Description |
---|---|
Selenium IDE | A browser extension for recording and playback of simple test scripts without programming. |
Selenium WebDriver | A tool for creating robust, browser-based automation scripts that support multiple programming languages. |
Selenium Grid | A tool for running tests in parallel on multiple machines and browsers, improving efficiency. |
Impact of Selenium on Testing
Selenium has revolutionized web testing by providing a free, open-source platform that integrates with modern tools and supports diverse programming languages and operating systems. It has become the de facto standard for web automation, widely used by companies and developers worldwide.
Code Example: Simple Selenium Test
Here’s a basic test using Selenium WebDriver to verify the title of a webpage:
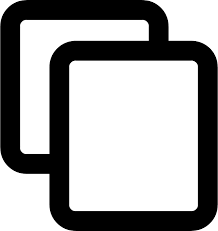
from selenium import webdriver
# Set up the WebDriver for Chrome
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
# Open a website
driver.get("https://www.example.com")
# Verify the title of the page
assert "Example Domain" in driver.title
# Close the browser
driver.quit()
Components of Selenium Suite
The Selenium suite consists of three core components, each designed to address different aspects of web automation. These components work together to provide a comprehensive solution for automated testing of web applications.
Selenium IDE
Selenium IDE (Integrated Development Environment) is a browser extension that simplifies test creation and execution. It is ideal for beginners and quick prototyping.
- Features:
- Record and Playback: Easily record actions performed on the browser and replay them as tests.
- Script Export: Convert recorded tests into programming languages like Java, Python, or C# for advanced use.
- Test Debugging: Includes debugging tools and breakpoints for refining test scripts.
- Best For: Quick test creation without coding.
Example: Recording a login test to verify successful authentication.
Selenium WebDriver
Selenium WebDriver is the most powerful and flexible component of the Selenium suite. It allows developers to create robust test scripts that interact directly with browsers.
- Features:
- Multi-Browser Support: Compatible with Chrome, Firefox, Safari, Edge, and more.
- Language Support: Write tests in Java, Python, Ruby, C#, JavaScript, and other programming languages.
- Advanced Controls: Perform complex actions like drag-and-drop, scrolling, and keyboard inputs.
- Best For: Complex and scalable test automation projects.
Example: Writing a Python script to interact with a search bar and validate search results.
Selenium Grid
Selenium Grid is designed for running tests in parallel across multiple browsers and environments, greatly improving test efficiency and coverage.
- Features:
- Parallel Testing: Execute multiple tests simultaneously on different machines and browsers.
- Hub-Node Architecture: Centralized control (Hub) with distributed execution (Nodes).
- Scalability: Supports large-scale testing across diverse environments.
- Best For: Cross-browser and cross-platform testing.
Example: Setting up a Selenium Grid to run tests on Chrome, Firefox, and Safari simultaneously.
Comparison of Selenium Components
Component | Purpose | Use Case |
---|---|---|
Selenium IDE | Record and playback tool for creating simple test cases. | Quick prototyping and beginner-friendly automation. |
Selenium WebDriver | Programmatic control of browsers for advanced automation. | Scalable and complex test scripts for production environments. |
Selenium Grid | Run tests in parallel on multiple browsers and environments. | Cross-platform and cross-browser compatibility testing. |
Code Example: Using Selenium WebDriver
Here’s a Python example using Selenium WebDriver to perform a Google search:
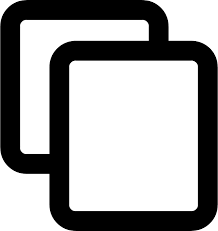
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Set up WebDriver for Chrome
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
# Open Google
driver.get("https://www.google.com")
# Find the search bar and perform a search
search_bar = driver.find_element("name", "q")
search_bar.send_keys("Selenium testing")
search_bar.send_keys(Keys.RETURN)
# Verify the title contains the search term
assert "Selenium testing" in driver.title
# Close the browser
driver.quit()
Selenium vs Other Automation Tools
Selenium is a popular choice for web automation, but it's essential to understand how it compares with other automation tools in terms of features, capabilities, and use cases. Below is a detailed comparison.
1. Selenium vs QTP/UFT (Unified Functional Testing)
Aspect | Selenium | QTP/UFT |
---|---|---|
Platform Support | Supports multiple platforms (Windows, macOS, Linux). | Primarily Windows-based. |
Browser Support | Supports all major browsers (Chrome, Firefox, Safari, Edge, etc.). | Limited browser support compared to Selenium. |
Programming Language | Supports multiple languages (Java, Python, C#, JavaScript, etc.). | Uses VBScript as its scripting language. |
Cost | Open source and free. | Commercial tool with licensing costs. |
Community Support | Large and active open-source community. | Limited community support; relies on vendor support. |
2. Selenium vs TestComplete
Aspect | Selenium | TestComplete |
---|---|---|
Ease of Use | Requires programming knowledge to build automation scripts. | Features a user-friendly interface with record-and-playback functionality. |
Cross-Browser Testing | Supports all major browsers. | Supports all major browsers, but setup is easier than Selenium. |
Cost | Free and open source. | Commercial tool with licensing fees. |
Integration | Integrates with tools like TestNG, JUnit, and CI/CD pipelines. | Integrates with other SmartBear tools and CI/CD systems. |
3. Selenium vs Cypress
Aspect | Selenium | Cypress |
---|---|---|
Language Support | Supports multiple languages (Java, Python, C#, JavaScript, etc.). | Built exclusively for JavaScript (Node.js). |
Test Speed | Depends on browser and environment setup. | Faster execution due to in-browser test execution. |
Testing Scope | Supports end-to-end, UI, and cross-browser testing. | Primarily focused on end-to-end testing for modern web apps. |
Community | Large and well-established community. | Growing community but smaller compared to Selenium. |
4. Selenium vs Puppeteer
Aspect | Selenium | Puppeteer |
---|---|---|
Browser Support | Supports multiple browsers. | Primarily supports Chrome and Chromium. |
Language Support | Multi-language support (Java, Python, C#, etc.). | JavaScript and TypeScript only. |
Automation Scope | Comprehensive web testing capabilities. | Best for headless browser automation and scraping. |
Community | Long-established community with extensive resources. | Smaller but growing community focused on modern web apps. |
Conclusion
The choice of an automation tool depends on your project requirements, budget, and technical expertise. Selenium remains a top choice for web automation due to its flexibility, browser support, and active community, but alternatives like Cypress, Puppeteer, and TestComplete offer unique advantages for specific scenarios.
Installing Selenium: Setting Up the Environment
Before starting with Selenium, you need to set up your development environment. Selenium supports multiple programming languages such as Python, Java, C#, and JavaScript. Below are the steps to install Selenium for Python and Java, two of the most widely used languages for automation testing.
1. Setting Up Selenium with Python
Follow the steps below to install Selenium and set up the environment for Python:
- Install Python:
- Download the Python installer from the official Python website.
- Run the installer and check the box "Add Python to PATH" during installation.
- Verify the installation by running
python --version
in the command line or terminal.
- Install Selenium:
- Open your terminal or command prompt and run the following command:
pip install selenium
- Open your terminal or command prompt and run the following command:
- Download a WebDriver:
- Choose the WebDriver for your browser (e.g., ChromeDriver for Chrome).
- Download the driver from the browser's official website and ensure it's compatible with your browser version.
- Add the WebDriver executable to your system PATH or specify its location in your script.
Python Code Example
A simple Python script to launch a browser using Selenium:
from selenium import webdriver
# Specify the path to the WebDriver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
driver.get("https://www.google.com")
print("Page Title:", driver.title)
driver.quit()
2. Setting Up Selenium with Java
Follow these steps to install and set up Selenium for Java:
- Install Java:
- Download and install the JDK (Java Development Kit) from the official website.
- Set up the JAVA_HOME environment variable and add the JDK's
bin
folder to your system PATH. - Verify the installation by running
java --version
andjavac --version
.
- Set Up a Build Tool:
- Use a build tool like Maven or Gradle to manage dependencies. For example, with Maven, add the following dependency to your
pom.xml
file:<dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.10.0</version> </dependency>
- Use a build tool like Maven or Gradle to manage dependencies. For example, with Maven, add the following dependency to your
- Download a WebDriver:
- Download the WebDriver executable (e.g., ChromeDriver) for your browser.
- Add the WebDriver to your system PATH or specify its location in your code.
Java Code Example
A simple Java program to launch a browser using Selenium:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumExample {
public static void main(String[] args) {
// Specify the path to the WebDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Launch the browser
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com");
// Print the title of the page
System.out.println("Page Title: " + driver.getTitle());
// Close the browser
driver.quit();
}
}
3. Common Notes for Other Languages
The steps for setting up Selenium with other languages like C# or JavaScript are similar. Install the language-specific bindings or libraries, configure the WebDriver, and write your test scripts in your preferred programming language.
Conclusion
Setting up Selenium is straightforward if you follow the steps for your chosen programming language. Once set up, you can start automating web applications and performing browser-based testing with ease.
Understanding Browser Drivers
Browser drivers play a crucial role in Selenium automation testing. They act as a bridge between the Selenium WebDriver and the browser, translating Selenium commands into browser-specific actions. Let's explore the key browser drivers and their roles in automation testing.
1. What Are Browser Drivers?
Browser drivers are executables provided by browser vendors to enable Selenium WebDriver to communicate with the browser. Each browser has its own dedicated driver, designed to support specific versions of the browser.
2. Popular Browser Drivers
Below are some widely used browser drivers:
Browser Driver | Description | Download Link |
---|---|---|
ChromeDriver | Supports automation for Google Chrome. It is frequently updated to support the latest Chrome versions. | Download ChromeDriver |
GeckoDriver | Enables automation for Mozilla Firefox. Required for Selenium WebDriver to interact with Firefox. | Download GeckoDriver |
EdgeDriver | Specifically for automating Microsoft Edge browser (Chromium-based). | Download EdgeDriver |
SafariDriver | Built into the Safari browser on macOS. No additional driver is required. | Built-in with Safari |
OperaDriver | Used for automating Opera browser, based on Chromium. | Download OperaDriver |
3. How Browser Drivers Work
The flow of communication between Selenium WebDriver and a browser via its driver is as follows:
- Selenium WebDriver sends commands to the browser driver.
- The browser driver translates these commands into browser-specific actions using the browser’s native support.
- The browser executes the actions and sends the results back to the driver, which then relays them to Selenium WebDriver.
Diagram: Selenium Communication Flow

This diagram shows how Selenium WebDriver communicates with the browser through the browser driver.
4. Setting Up Browser Drivers
To use a browser driver, follow these steps:
- Download the driver executable for your browser from its official website.
- Ensure the driver version matches your browser version to avoid compatibility issues.
- Add the driver to your system PATH or specify its location in your script.
Code Example: Using ChromeDriver with Python
from selenium import webdriver
# Specify the path to the ChromeDriver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
driver.get("https://www.google.com")
print("Page Title:", driver.title)
driver.quit()
Code Example: Using GeckoDriver with Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class GeckoDriverExample {
public static void main(String[] args) {
// Specify the path to the GeckoDriver
System.setProperty("webdriver.gecko.driver", "path/to/geckodriver");
// Launch Firefox
WebDriver driver = new FirefoxDriver();
driver.get("https://www.google.com");
// Print the title of the page
System.out.println("Page Title: " + driver.getTitle());
// Close the browser
driver.quit();
}
}
5. Key Notes
- Always use the browser driver version compatible with your browser.
- Keep your browser and driver updated for the best performance and compatibility.
- For Safari, ensure "Allow Remote Automation" is enabled in the browser's Developer menu.
Conclusion
Understanding browser drivers is essential for successful Selenium automation testing. By correctly setting up and managing browser drivers, you can ensure smooth communication between Selenium WebDriver and the browser, enabling effective test execution.
Basics of Web Automation
Web automation is the process of using tools and frameworks to automate repetitive tasks on web applications. It involves interacting with web elements like buttons, forms, and links to mimic user actions such as clicking, typing, and navigating.
1. Why Web Automation?
Web automation is essential for:
- Reducing manual effort in testing or repetitive tasks.
- Improving the accuracy and reliability of test cases.
- Saving time and resources in software development cycles.
- Performing data scraping and content extraction efficiently.
2. Key Components of Web Automation
Effective web automation relies on the following components:
Component | Description |
---|---|
Web Automation Tool | A software or framework like Selenium, Puppeteer, or Cypress used to automate tasks on web applications. |
Test Scripts | Scripts written in programming languages like Python, Java, or JavaScript to define the actions to be automated. |
Browser Drivers | Intermediaries like ChromeDriver or GeckoDriver that facilitate communication between the automation tool and the web browser. |
Web Elements | The HTML elements (e.g., buttons, input fields) that the automation interacts with. |
3. Common Actions in Web Automation
Web automation typically involves performing these actions:
- Opening a webpage.
- Locating web elements using selectors like ID, class, or XPath.
- Performing actions like clicking, typing, selecting, or scrolling.
- Validating the output or behavior of the application.
4. Tools for Web Automation
Popular tools for web automation include:
- Selenium: Open-source framework supporting multiple browsers and languages.
- Puppeteer: Node.js library for automating headless Chrome browsers.
- Cypress: End-to-end testing framework designed for modern web applications.
- Playwright: Framework for automating multiple browsers with a single API.
5. Code Example: Automating a Google Search with Selenium (Python)
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open Google
driver.get("https://www.google.com")
# Find the search bar and enter a query
search_box = driver.find_element("name", "q")
search_box.send_keys("Web automation basics")
search_box.send_keys(Keys.RETURN)
# Print the page title
print("Page Title:", driver.title)
# Close the browser
driver.quit()
6. Code Example: Automating Form Submission with Selenium (Java)
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class FormAutomation {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Open a demo form page
driver.get("https://example.com/form");
// Fill out the form
WebElement nameField = driver.findElement(By.id("name"));
nameField.sendKeys("John Doe");
WebElement emailField = driver.findElement(By.id("email"));
emailField.sendKeys("john.doe@example.com");
WebElement submitButton = driver.findElement(By.id("submit"));
submitButton.click();
// Print confirmation message
System.out.println("Form submitted successfully!");
driver.quit();
}
}
7. Best Practices for Web Automation
- Use explicit waits to handle dynamic loading of elements.
- Write reusable and modular test scripts.
- Use appropriate selectors for locating web elements (e.g., ID is faster and more reliable).
- Keep tools and browser drivers updated to ensure compatibility.
Conclusion
Web automation simplifies repetitive tasks and enhances productivity in testing and development. With a clear understanding of tools, browsers, and automation techniques, you can effectively automate tasks and ensure robust application performance.
Configuring Selenium with Different Programming Languages
Selenium is a widely used framework for automating web browsers. It supports multiple programming languages, allowing developers to write automation scripts in their preferred language. Below are the steps and code examples for configuring Selenium with Python, Java, and JavaScript.
1. Why Use Selenium?
Selenium is a powerful tool for automating web browsers. It is commonly used for:
- Automating repetitive tasks.
- Performing web scraping.
- Running automated tests for web applications.
- Validating UI/UX by simulating user actions.
2. Setting Up Selenium with Python
To use Selenium with Python, you need to install the Selenium package and a WebDriver (e.g., ChromeDriver).
pip install selenium
Here's a simple example of automating a Google search:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open Google
driver.get("https://www.google.com")
# Find the search bar and enter a query
search_box = driver.find_element("name", "q")
search_box.send_keys("Selenium automation")
search_box.send_keys(Keys.RETURN)
# Print the page title
print("Page Title:", driver.title)
# Close the browser
driver.quit()
3. Setting Up Selenium with Java
For Java, you will need to include the Selenium WebDriver dependency in your project (via Maven or Gradle).
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
Here's a code example for submitting a form with Selenium in Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class FormAutomation {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Open a demo form page
driver.get("https://example.com/form");
// Fill out the form
WebElement nameField = driver.findElement(By.id("name"));
nameField.sendKeys("John Doe");
WebElement emailField = driver.findElement(By.id("email"));
emailField.sendKeys("john.doe@example.com");
WebElement submitButton = driver.findElement(By.id("submit"));
submitButton.click();
// Print confirmation message
System.out.println("Form submitted successfully!");
driver.quit();
}
}
4. Setting Up Selenium with JavaScript (Node.js)
To use Selenium with JavaScript, install the Selenium WebDriver package for Node.js using npm:
npm install selenium-webdriver
Here's an example of automating a Google search in JavaScript:
const {Builder, By, Key} = require('selenium-webdriver');
(async function googleSearch() {
let driver = await new Builder().forBrowser('chrome').build();
try {
// Open Google
await driver.get('https://www.google.com');
// Find the search box and enter a query
let searchBox = await driver.findElement(By.name('q'));
await searchBox.sendKeys('Selenium automation', Key.RETURN);
// Print the page title
let title = await driver.getTitle();
console.log('Page Title:', title);
} finally {
await driver.quit();
}
})();
5. Best Practices for Using Selenium
- Always use explicit waits to handle dynamic web elements.
- Keep your WebDriver and browser drivers up-to-date.
- Write modular and reusable automation scripts.
- Use proper exception handling to deal with errors effectively.
Conclusion
Selenium offers flexibility by supporting multiple programming languages. By configuring Selenium for Python, Java, or JavaScript, developers can automate repetitive tasks and improve the efficiency of their testing and web scraping workflows.
Setting Up the First Automation Script
Creating your first automation script with Selenium is an essential step in understanding how web automation works. This section will guide you through setting up the environment and writing the first automation script in Python, Java, and JavaScript.
1. Prerequisites
Before you begin, ensure you have the following installed on your system:
- Selenium WebDriver: The core framework for automating browser interactions.
- Browser Driver: A driver like ChromeDriver for Chrome or GeckoDriver for Firefox to enable communication between Selenium and your browser.
- Programming Language: Python, Java, or JavaScript depending on your preference.
2. Install Selenium
Install Selenium using the package manager for your chosen language:
For Python
pip install selenium
For Java
Add the following dependency in your Maven project:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
For JavaScript
npm install selenium-webdriver
3. Write the First Automation Script
Now that Selenium is installed, let’s write a simple script that opens a website and performs an action, such as searching for a term in Google.
Python Example
This Python script opens Google and performs a search:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open Google
driver.get("https://www.google.com")
# Find the search bar and enter a query
search_box = driver.find_element("name", "q")
search_box.send_keys("Selenium tutorial")
search_box.send_keys(Keys.RETURN)
# Print the page title
print("Page Title:", driver.title)
# Close the browser
driver.quit()
Java Example
This Java code opens Google and performs a search:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class GoogleSearch {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Open Google
driver.get("https://www.google.com");
// Find the search box and enter a query
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("Selenium tutorial");
searchBox.submit();
// Print the page title
System.out.println("Page Title: " + driver.getTitle());
driver.quit();
}
}
JavaScript (Node.js) Example
This JavaScript code opens Google and performs a search:
const {Builder, By, Key} = require('selenium-webdriver');
(async function searchGoogle() {
let driver = await new Builder().forBrowser('chrome').build();
try {
// Open Google
await driver.get('https://www.google.com');
// Find the search box and enter a query
let searchBox = await driver.findElement(By.name('q'));
await searchBox.sendKeys('Selenium tutorial', Key.RETURN);
// Print the page title
let title = await driver.getTitle();
console.log('Page Title:', title);
} finally {
await driver.quit();
}
})();
4. Running the Script
To run your script:
- For Python, run the script with the command:
python script_name.py
. - For Java, compile and run your Java program using your IDE or command line.
- For JavaScript, run the script using Node.js:
node script_name.js
.
5. Troubleshooting Tips
- Ensure the browser driver path is set correctly in your script.
- Check that the browser version matches the version of the driver.
- If the script fails to locate an element, ensure that the element is present and accessible.
Conclusion
Your first automation script is a great starting point for automating tasks and performing testing in web applications. Once you’re comfortable with simple automation, you can expand to more complex scenarios, including form submissions, handling pop-ups, and interacting with dynamic content.
Locating Web Elements Using Locators
In Selenium, you can locate web elements using different types of locators. Understanding how to use these locators is crucial for interacting with elements on a webpage. Below are the most commonly used locators in Selenium: ID, Name, Class Name, Tag Name, Link Text, Partial Link Text, CSS Selector, and XPath.
1. Locating Elements by ID
The ID locator is one of the most reliable ways to locate an element, as IDs are expected to be unique within a page. Here’s how to find an element by its ID:
from selenium import webdriver
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the website
driver.get("https://example.com")
# Locate the element by ID
element = driver.find_element("id", "element_id")
element.click()
driver.quit()
2. Locating Elements by Name
You can also locate elements using the name
attribute. This is useful when the ID is not available but the element has a name attribute.
# Locate element by name
element = driver.find_element("name", "element_name")
element.send_keys("Some text")
3. Locating Elements by Class Name
The class name locator allows you to locate elements based on their class attribute. It’s often used when multiple elements share the same class.
# Locate element by class name
element = driver.find_element("class name", "element_class")
element.click()
4. Locating Elements by Tag Name
Tag name locators are used to locate elements based on the HTML tag name (e.g., div
, input
, a
). This is helpful when you want to interact with all elements of a specific type.
# Locate element by tag name
element = driver.find_element("tag name", "a")
element.click()
5. Locating Elements by Link Text
If you want to locate links (anchor tags) based on their visible text, you can use the link text locator. It is particularly useful when dealing with navigation links.
# Locate element by link text
element = driver.find_element("link text", "Click Here")
element.click()
6. Locating Elements by Partial Link Text
Partial link text allows you to find links by matching only part of the link text. This is useful when the link text is long or dynamic.
# Locate element by partial link text
element = driver.find_element("partial link text", "Click")
element.click()
7. Locating Elements by CSS Selector
CSS selectors are very powerful and flexible locators. You can use them to target elements based on a variety of attributes and even nested elements.
# Locate element by CSS selector
element = driver.find_element("css selector", "button.submit-btn")
element.click()
8. Locating Elements by XPath
XPath is a query language used for selecting nodes from an XML document. In Selenium, XPath can be used to locate elements in HTML documents. It is highly flexible and can be used to find elements based on almost any condition.
# Locate element by XPath
element = driver.find_element("xpath", "//button[@id='submit']")
element.click()
9. Best Practices for Locators
- Always prefer ID locators when possible, as they are the most reliable.
- Class name is useful for locating multiple elements but may be less unique.
- XPath and CSS selectors provide more flexibility but should be used carefully to avoid slow performance.
- Use link text and partial link text for clickable links where the text is unique.
Conclusion
Locators are the foundation of interacting with web elements in Selenium. By understanding the different types of locators, you can effectively find and interact with elements on a webpage. Select the appropriate locator based on the situation to make your automation scripts more efficient and reliable.
Opening a Browser and Navigating to a URL
In Selenium, opening a browser and navigating to a URL is the first step in automating web interactions. Selenium WebDriver allows you to launch a browser, navigate to a specified URL, and interact with the web page. Below is a guide on how to set up and use Selenium to open a browser and visit a website.
1. Setting Up Selenium WebDriver
Before you can open a browser, you need to set up Selenium WebDriver and download the appropriate browser driver (e.g., ChromeDriver for Chrome). Ensure that you have the necessary browser installed on your system.
2. Open a Browser (Chrome in this Example)
To open a browser, you'll first need to instantiate a WebDriver object. Here’s how to do it for Google Chrome:
from selenium import webdriver
# Set up the driver for Chrome
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a website (e.g., Google)
driver.get("https://www.google.com")
# Close the browser after some time
driver.quit()
In the above example, we used webdriver.Chrome()
to open the Chrome browser. The get()
method is used to navigate to a specified URL.
3. Opening Other Browsers
To open a different browser, you can use the respective driver for that browser (e.g., Firefox, Edge). Here’s how to open a Firefox browser:
from selenium import webdriver
# Set up the driver for Firefox
driver = webdriver.Firefox(executable_path="path/to/geckodriver")
# Open a website (e.g., Google)
driver.get("https://www.google.com")
# Close the browser after some time
driver.quit()
4. Navigating to a URL
Once the browser is open, you can navigate to any URL using the get()
method. This method will make the browser load the specified page.
# Navigate to a specific URL
driver.get("https://www.example.com")
The get()
method will take the browser to the URL you provide. You can use this method to open any web page for automation or testing.
5. Verifying Navigation
After navigating to a URL, you may want to verify that the page has loaded successfully. You can check the page title using the title
property of the driver:
# Verify the page title
print("Page Title:", driver.title)
This will print the title of the page that has been loaded in the browser, which can be used for validation in your automation scripts.
6. Handling Timeouts and Waiting for Page Load
If the page takes time to load, you may encounter issues where the script tries to interact with elements before they are available. To handle this, you can use WebDriver waits to ensure that the page has loaded before performing actions.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Wait for a specific element to be visible before proceeding
wait = WebDriverWait(driver, 10)
element = wait.until(EC.visibility_of_element_located((By.ID, "element_id")))
7. Closing the Browser
After performing the necessary tasks, you should close the browser to free up system resources. You can do this using the quit()
method, which closes the browser completely and ends the WebDriver session.
# Close the browser
driver.quit()
Conclusion
Opening a browser and navigating to a URL is the foundation of web automation in Selenium. With just a few lines of code, you can launch a browser, load a webpage, and prepare it for further interactions. By using Selenium's WebDriver and its various methods, you can automate web browsing tasks effectively and efficiently.
Managing Browser Windows and Tabs
In Selenium, managing browser windows and tabs is essential when dealing with multiple windows or tabs during automation. You can switch between them, close or open new ones, and perform actions in each as needed. This section covers how to handle browser windows and tabs in Selenium WebDriver.
1. Understanding Browser Windows and Tabs
When using a web browser, each page you open is typically loaded in a new tab or window. Selenium allows you to interact with these windows or tabs by switching between them. Each window or tab in Selenium is identified by a unique window handle.
2. Getting the Current Window Handle
To perform actions on the current window, you need to get the window handle. The window handle is a unique identifier for the window/tab that is currently in focus.
# Get the current window handle
current_window_handle = driver.current_window_handle
print("Current Window Handle:", current_window_handle)
3. Opening a New Window or Tab
To open a new window or tab, you can use JavaScript to trigger the opening of a new window. Selenium will automatically switch to the newly opened tab or window once it’s created.
# Open a new window or tab using JavaScript
driver.execute_script("window.open('https://www.example.com');")
4. Switching Between Windows or Tabs
After opening multiple windows or tabs, you can switch between them using their window handles. Selenium provides the window_handles
property, which returns a list of all open window handles. You can then switch to the desired window using the switch_to.window()
method.
# Get all window handles
all_window_handles = driver.window_handles
print("All Window Handles:", all_window_handles)
# Switch to the new window (assuming it's the second one)
driver.switch_to.window(all_window_handles[1])
# Perform actions in the new window
driver.get("https://www.example.com")
5. Closing a Window or Tab
Once you have finished interacting with a window or tab, you can close it using the close()
method. However, closing the current window will leave Selenium focused on the last open window. If you have multiple windows open, you may need to switch to the desired window before closing it.
# Close the current window
driver.close()
# Switch back to the original window
driver.switch_to.window(all_window_handles[0])
6. Handling Multiple Windows and Tabs
When working with multiple windows or tabs, it’s important to ensure that your automation script properly handles the switching and closing of windows. You can loop through window handles to interact with each window or tab:
# Loop through all window handles
for handle in all_window_handles:
driver.switch_to.window(handle)
print("Window Title:", driver.title)
# Perform actions in the window, if needed
# driver.close() # Uncomment to close specific windows
7. Switching Back to the Original Window
Once you've completed actions in a new window or tab, you can switch back to the original window by using the saved window handle.
# Switch back to the original window
driver.switch_to.window(current_window_handle)
8. Best Practices for Managing Windows and Tabs
- Always keep track of the original window handle so you can easily switch back after interacting with other windows.
- Close windows that are no longer needed to keep your test environment clean and efficient.
- Ensure that your script handles the switching of windows properly, especially when dealing with pop-ups or new tabs.
Conclusion
Managing browser windows and tabs is a crucial aspect of automating tasks that involve multiple pages. Selenium WebDriver provides the necessary tools to open, switch between, and close windows or tabs with ease. By understanding and using window handles effectively, you can create robust and flexible automation scripts.
Handling Browser Back, Forward, and Refresh Commands
Selenium WebDriver allows you to simulate browser navigation commands, such as going back, going forward, or refreshing the page. These commands are useful when you need to automate tasks that involve navigating through a website's history or reloading a page to get the latest content.
1. Understanding Browser Navigation
When interacting with a web page, the browser keeps track of the page history. Selenium provides commands to simulate actions like going back to the previous page, moving forward to the next page, or refreshing the current page. These actions help automate workflows that require navigation through different pages in a browser session.
2. Using the Browser Back Command
The back()
method allows you to simulate clicking the browser's back button, which navigates to the previous page in the history.
# Go back to the previous page
driver.back()
After calling back()
, the WebDriver will navigate back to the most recent page in the browser's history, simulating the action of the back button in the browser.
3. Using the Browser Forward Command
The forward()
method allows you to simulate clicking the browser's forward button, which navigates to the next page in the browser’s history if you have previously gone back.
# Go forward to the next page
driver.forward()
After calling forward()
, Selenium will navigate to the next page in the browser's history, if applicable.
4. Using the Browser Refresh Command
The refresh()
method allows you to reload the current page. This can be helpful when you need to refresh the content on the page or ensure that you're working with the most up-to-date version of the page.
# Refresh the current page
driver.refresh()
After calling refresh()
, Selenium will reload the current page, simulating the browser's refresh button.
5. Example: Navigating Through Pages
Here’s an example that demonstrates how to use the back, forward, and refresh commands in a typical web navigation scenario:
# Open the first page
driver.get("https://www.example1.com")
# Navigate to another page
driver.get("https://www.example2.com")
# Use the back command to go to the previous page
driver.back()
# Use the forward command to go to the next page
driver.forward()
# Refresh the current page
driver.refresh()
6. Best Practices for Browser Navigation
- Ensure that the page has loaded completely before using the back, forward, or refresh commands to avoid unexpected behavior.
- Use explicit waits when performing navigation to ensure that elements are available before performing actions like back, forward, or refresh.
- Test the navigation commands in the context of your specific use case. For example,
back()
andforward()
may not work as expected if the browser history is not populated correctly.
7. Conclusion
Handling browser navigation is a crucial part of automating web tasks. Selenium WebDriver makes it easy to simulate browser actions such as going back, going forward, and refreshing the page. These commands are essential for testing workflows that involve navigating through multiple pages or reloading content on the same page. By using these commands effectively, you can enhance your automation scripts and ensure smooth navigation through web applications.
Managing Cookies in Selenium
Cookies are small pieces of data stored by the browser that are used to maintain session information across web requests. Selenium WebDriver provides methods to manage cookies, allowing you to interact with cookies in your automated tests. You can add, delete, and retrieve cookies during your test execution.
1. Understanding Cookies in Web Automation
Cookies are essential for maintaining user sessions, tracking user activity, and storing preferences. In Selenium, you can manage cookies to simulate user behavior, test session persistence, or handle authentication without manual login.
2. Adding a Cookie
You can add a new cookie to the browser using the add_cookie()
method. This method requires a dictionary object containing the cookie's name, value, and other optional attributes such as domain, path, and expiration date.
# Add a new cookie to the browser
cookie = {
'name': 'username',
'value': 'test_user',
'domain': 'example.com',
'path': '/',
}
driver.add_cookie(cookie)
In this example, a cookie named 'username' is added with the value 'test_user'. The cookie is set for the domain 'example.com' and the root path ('/').
3. Getting a Cookie by Name
To retrieve the value of a specific cookie, you can use the get_cookie()
method and provide the cookie's name. This will return the cookie's details if it exists.
# Get a cookie by name
cookie = driver.get_cookie('username')
print(cookie)
The get_cookie()
method returns a dictionary containing the cookie's name, value, domain, and other attributes.
4. Getting All Cookies
If you want to retrieve all cookies stored in the browser, you can use the get_cookies()
method. This returns a list of dictionaries, each representing a cookie.
# Get all cookies
cookies = driver.get_cookies()
for cookie in cookies:
print(cookie)
The get_cookies()
method returns a list of dictionaries, where each dictionary represents a cookie with its attributes like name, value, domain, etc.
5. Deleting a Cookie
You can delete a specific cookie using the delete_cookie()
method by passing the cookie's name. If you want to delete all cookies, you can use the delete_all_cookies()
method.
# Delete a specific cookie by name
driver.delete_cookie('username')
# Delete all cookies
driver.delete_all_cookies()
In the example above, the cookie named 'username' is deleted. The second method deletes all cookies stored in the browser.
6. Example: Managing Cookies for Session Persistence
Here's an example of how you can add and manage cookies to maintain session persistence across different browser sessions:
# Open a website
driver.get("https://www.example.com")
# Add a cookie to maintain session
cookie = {'name': 'session_id', 'value': 'abc123'}
driver.add_cookie(cookie)
# Refresh the page to preserve session
driver.refresh()
# Retrieve and print the session cookie
session_cookie = driver.get_cookie('session_id')
print("Session Cookie:", session_cookie)
In this example, a cookie named 'session_id' is added to simulate a persistent session. After refreshing the page, the session cookie is retrieved and printed.
7. Best Practices for Managing Cookies
- Always ensure that the website domain and path match the cookie's domain and path when adding cookies.
- Use cookies for session management and authentication to avoid the need for manual logins during testing.
- Be cautious when deleting all cookies, as this may affect other parts of the test that rely on cookies for session management.
8. Conclusion
Managing cookies in Selenium WebDriver is a powerful technique for handling session management, authentication, and simulating user behavior. By understanding how to add, retrieve, and delete cookies, you can create more robust and efficient automation scripts that interact with web applications in a more realistic way.
Taking Screenshots with Selenium
Selenium WebDriver allows you to capture screenshots of web pages during test execution. This feature is particularly useful for debugging, visual verification, and generating reports. You can take screenshots in various formats (e.g., PNG, JPEG) and save them to a local directory for later analysis.
1. Why Take Screenshots in Automation?
Taking screenshots can help you:
- Verify the visual correctness of a page or element.
- Capture the state of a page during test failures for debugging.
- Generate visual reports for test execution logs.
- Assist in creating documentation for automated testing processes.
2. Capturing a Screenshot
Selenium provides the get_screenshot_as_file()
method to capture a screenshot and save it to a specified file. The file will be saved in the format you specify (typically PNG).
# Capture a screenshot and save it as a file
driver.get("https://www.example.com")
driver.get_screenshot_as_file("screenshot.png")
In this example, the screenshot of the webpage is captured and saved as 'screenshot.png' in the current working directory.
3. Capturing Screenshot as Base64 String
You can also capture a screenshot as a Base64-encoded string, which is useful if you want to embed the image directly into HTML, reports, or logs without saving it as an external file.
# Capture screenshot as Base64 string
screenshot_base64 = driver.get_screenshot_as_base64()
print(screenshot_base64)
The get_screenshot_as_base64()
method returns the screenshot as a Base64-encoded string, which can then be embedded wherever needed.
4. Capturing Screenshot as Bytes
If you prefer to work with the image in raw byte format (e.g., to send it over a network), you can capture the screenshot as bytes.
# Capture screenshot as raw bytes
screenshot_bytes = driver.get_screenshot_as_png()
with open("screenshot_bytes.png", "wb") as file:
file.write(screenshot_bytes)
In this example, the screenshot is captured as raw PNG bytes and written to a file named 'screenshot_bytes.png'.
5. Example: Taking a Screenshot on Test Failure
It is common to take screenshots when a test fails to capture the state of the application at the time of failure. Here's an example of how to automatically take a screenshot if a test fails:
from selenium import webdriver
import unittest
class TestExample(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome(executable_path="path/to/chromedriver")
def test_screenshot_on_failure(self):
try:
self.driver.get("https://www.example.com")
# Simulate a failure
self.assertEqual(1, 2)
except Exception as e:
# Capture screenshot on failure
self.driver.get_screenshot_as_file("failure_screenshot.png")
print("Test failed. Screenshot taken.")
raise e
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
In this example, the test_screenshot_on_failure()
method captures a screenshot if the test fails (simulated with an assertion failure). The screenshot is saved as 'failure_screenshot.png'.
6. Best Practices for Taking Screenshots
- Use descriptive filenames for screenshots to easily identify them later (e.g., include the test name and timestamp).
- Store screenshots in a dedicated directory (e.g., 'screenshots/') to keep your project organized.
- Take screenshots at key stages in your test, such as before and after an interaction or when a failure occurs.
- Use screenshots as part of your debugging and reporting process to visually demonstrate test results.
7. Conclusion
Taking screenshots during automated test execution in Selenium is a valuable tool for visual verification and debugging. By capturing screenshots at key moments, such as test failures or critical user interactions, you can gain insights into the state of your application and improve the quality of your tests. With simple methods like get_screenshot_as_file()
, get_screenshot_as_base64()
, and get_screenshot_as_png()
, Selenium makes it easy to integrate screenshot capture into your test scripts.
Types of Locators and Their Use Cases
In Selenium WebDriver, locators are used to find and interact with web elements on a page. There are various types of locators, each with specific use cases depending on the structure and attributes of the page. Choosing the right locator is crucial for ensuring the reliability and efficiency of your automation scripts.
1. ID Locator
The ID locator is one of the most commonly used locators in Selenium. It is fast and reliable because IDs are unique for each element on the page.
- Use case: When the element has a unique ID attribute, the ID locator is the best choice for locating elements.
- Example: Locating an element with an ID of 'submit-button'.
# Locating an element by ID
element = driver.find_element_by_id("submit-button")
2. Name Locator
The Name locator is used to locate an element by its 'name' attribute. It is useful when the element does not have a unique ID but has a name attribute.
- Use case: When the element has a name attribute that can uniquely identify it on the page.
- Example: Locating an input field with a name of 'username'.
# Locating an element by Name
element = driver.find_element_by_name("username")
3. Class Name Locator
The Class Name locator is used to find elements by their class attribute. While class names are often used for styling, they can also be used to locate elements, especially when multiple elements share the same class.
- Use case: When the element has a class attribute and you want to interact with all elements with the same class.
- Example: Locating a button with the class 'btn-primary'.
# Locating an element by Class Name
element = driver.find_element_by_class_name("btn-primary")
4. Tag Name Locator
The Tag Name locator is used to locate elements by their HTML tag name. This locator is useful for finding all elements of a specific tag, such as all input
elements or all div
elements.
- Use case: When you need to find all elements of a certain tag on the page.
- Example: Locating all
input
elements on the page.
# Locating an element by Tag Name
elements = driver.find_elements_by_tag_name("input")
5. Link Text Locator
The Link Text locator is used specifically to locate anchor
(<a>
) elements by their text content. It is ideal for finding links with specific labels.
- Use case: When you need to find a link by its exact text.
- Example: Locating a link with the text 'Home'.
# Locating a link by Link Text
element = driver.find_element_by_link_text("Home")
6. Partial Link Text Locator
The Partial Link Text locator is used to locate anchor
elements by a portion of their link text. This is useful when the link text is dynamic or too long to specify fully.
- Use case: When you want to locate a link using a part of the link text.
- Example: Locating a link with partial text 'Home' when the full text is 'Go to Home Page'.
# Locating a link by Partial Link Text
element = driver.find_element_by_partial_link_text("Home")
7. CSS Selector Locator
The CSS Selector locator is a powerful locator that allows you to locate elements using CSS selectors. It supports complex queries, including combinations of element attributes, classes, IDs, and even hierarchical structure.
- Use case: When you need to locate elements based on specific attributes or hierarchical relationships.
- Example: Locating a
div
with a class of 'container' and an ID of 'main'.
# Locating an element by CSS Selector
element = driver.find_element_by_css_selector("div#main.container")
8. XPath Locator
The XPath locator is a versatile locator that allows you to locate elements using XML path syntax. XPath can traverse both the DOM structure and element attributes, making it suitable for complex queries.
- Use case: When you need to locate elements based on complex attributes or structural hierarchy.
- Example: Locating an element with a specific attribute value or position in the DOM.
# Locating an element by XPath
element = driver.find_element_by_xpath("//div[@id='main']/span")
9. Best Practices for Locating Elements
- Use IDs whenever possible, as they are unique and faster for WebDriver to find.
- Use CSS Selectors for complex queries and when combining multiple attributes.
- Use XPath for hierarchical element location and when dealing with dynamic or complex elements.
- Use Link Text or Partial Link Text for locating links with clear and consistent text.
- Use Class Name for elements with common styling, but ensure it’s unique enough to avoid ambiguity.
10. Conclusion
Understanding the different types of locators in Selenium WebDriver is essential for effective web automation. Each locator has its specific use cases depending on the structure and attributes of the web elements on the page. By selecting the right locator for the task at hand, you can improve the reliability, performance, and maintainability of your Selenium scripts.
Writing Effective XPath Expressions
XPath is a powerful language used to navigate through elements and attributes in an XML document. In Selenium, XPath is used to locate elements on a webpage using their XML structure. Writing effective XPath expressions is crucial for locating elements reliably, especially when dealing with dynamic content.
1. Basics of XPath
XPath is a path expression that allows you to traverse the XML document’s tree structure. It can locate elements by their attributes, text content, or position in the document structure. XPath expressions typically begin with a double slash //
to search the entire document or a single slash /
to search from the root element.
- Syntax:
//tagname[@attribute='value']
- Example: To locate an element with the ID "login-button", use
//button[@id='login-button']
.
2. Absolute vs. Relative XPath
XPath expressions can be absolute or relative:
- Absolute XPath: Starts from the root element and uses the full path to the target element. It is prone to breaking if the structure of the page changes.
- Relative XPath: Starts from any element and can be more flexible. It is preferred in most cases because it is less likely to break with changes in the page structure.
# Absolute XPath
element = driver.find_element_by_xpath("/html/body/div[1]/div/button")
# Relative XPath
element = driver.find_element_by_xpath("//button[@id='login-button']")
3. Using Attributes in XPath
XPath allows you to locate elements using any attribute, such as id
, name
, class
, href
, etc. The syntax for using attributes is //tagname[@attribute='value']
.
- Example: Locating an element by its
id
attribute://input[@id='username']
. - Example: Locating an element by its
class
attribute://div[@class='login-form']
.
# Locating an element by ID
element = driver.find_element_by_xpath("//input[@id='username']")
# Locating an element by Class Name
element = driver.find_element_by_xpath("//div[@class='login-form']")
4. Using Text in XPath
XPath can also locate elements based on their visible text using the text()
function. This is particularly useful for buttons, links, and other elements with human-readable text.
- Example: Locating a link with the text 'Login':
//a[text()='Login']
. - Example: Locating a button with the text 'Submit':
//button[text()='Submit']
.
# Locating an element by text
element = driver.find_element_by_xpath("//a[text()='Login']")
5. Using Partial Text in XPath
If you don’t want to match the full text of an element, you can use the contains()
function to match part of the text.
- Example: Locating a link that contains the text 'Log':
//a[contains(text(), 'Log')]
. - Example: Locating a button that contains the word 'Sub':
//button[contains(text(), 'Sub')]
.
# Locating an element by partial text
element = driver.find_element_by_xpath("//a[contains(text(), 'Log')]")
6. Using Wildcards in XPath
XPath supports the use of wildcards to match elements without specifying exact tag names, attributes, or values.
- Wildcard for element names:
*
matches any element. For example,//input[@type='text']
can be written as//input[@type='*']
to match any input element with a type attribute. - Wildcard for attributes:
@*
matches any attribute of an element.
# Using wildcard for elements
element = driver.find_element_by_xpath("//input[@type='*']")
# Using wildcard for attributes
element = driver.find_element_by_xpath("//div[@*='container']")
7. Using Hierarchical XPath
XPath allows for navigating the DOM tree by selecting parent and child elements. This is useful when you need to locate an element relative to another element.
- Example: Locating a button inside a div with class 'login-form':
//div[@class='login-form']/button
. - Example: Locating an input field inside a form:
//form//input[@name='username']
.
# Navigating through parent-child hierarchy
element = driver.find_element_by_xpath("//div[@class='login-form']/button")
# Locating an input inside a form
element = driver.find_element_by_xpath("//form//input[@name='username']")
8. Using AND/OR Operators in XPath
XPath allows for logical operations like AND
and OR
to combine multiple conditions in a single expression.
- AND Operator: Used when both conditions must be true. Example:
//input[@type='text' and @name='username']
. - OR Operator: Used when at least one condition must be true. Example:
//input[@name='username' or @name='email']
.
# Using AND condition
element = driver.find_element_by_xpath("//input[@type='text' and @name='username']")
# Using OR condition
element = driver.find_element_by_xpath("//input[@name='username' or @name='email']")
9. Best Practices for Writing XPath Expressions
- Prefer using relative XPath over absolute XPath for better flexibility and resilience.
- Use unique attributes like
id
andname
whenever possible to make XPath more reliable. - Avoid using XPath with too many conditions or deep hierarchies, as this can make the expression brittle and hard to maintain.
- Use
contains()
for partial text matches, especially when dealing with dynamic content. - Test XPath expressions in the browser’s developer tools (e.g., Chrome DevTools) before using them in your scripts.
10. Conclusion
XPath is an essential tool in Selenium for locating elements with precision. By mastering XPath, you can write more flexible, reliable, and maintainable automation scripts. Understanding how to write effective XPath expressions is key to navigating complex web pages and interacting with elements efficiently.
CSS Selectors vs XPath: Pros and Cons
Both CSS selectors and XPath are powerful methods for locating elements in Selenium. However, they each have their strengths and weaknesses. Understanding their differences and when to use each can significantly improve the efficiency and reliability of your automation scripts.
1. What is a CSS Selector?
A CSS selector is a pattern used to select elements based on their attributes, such as id
, class
, name
, type
, and more. CSS selectors are typically used in web development for styling elements, but in Selenium, they are also used to locate elements.
2. What is XPath?
XPath is a query language used for selecting nodes in XML documents. In Selenium, XPath allows you to locate elements based on their structure, attributes, text content, and position within the HTML DOM. XPath expressions are more flexible than CSS selectors and can navigate through the DOM tree.
3. Pros and Cons of CSS Selectors
CSS selectors are widely used in Selenium for their simplicity and speed. Below are the advantages and disadvantages of using CSS selectors:
- Pros of CSS Selectors:
- Faster Execution: CSS selectors are generally faster than XPath. This is because modern browsers are optimized for CSS, making it quicker to parse and locate elements.
- Readable and Concise: CSS selectors are often more concise and easier to read compared to XPath expressions. Simple selectors like
#id
and.class
are straightforward. - Supports All HTML Elements: CSS selectors can be used to locate any HTML element based on attributes, such as
id
,class
,name
, etc. - Widely Used in Web Development: Since CSS selectors are a standard part of web development, they are familiar to many developers.
- Cons of CSS Selectors:
- Limited Flexibility: CSS selectors cannot navigate up the DOM tree (e.g., selecting a parent element or traversing siblings). This makes them less flexible for complex DOM structures.
- Cannot Locate Text: CSS selectors cannot locate elements based on their text content, unlike XPath, which can match elements based on text.
- Not Ideal for Complex Queries: While CSS selectors work well for simple queries, they are not as powerful as XPath for more complex queries that require navigating through the DOM tree.
4. Pros and Cons of XPath
XPath is a more powerful and flexible option, especially for complex scenarios. Below are the advantages and disadvantages of using XPath:
- Pros of XPath:
- Flexibility: XPath can navigate both up and down the DOM tree, allowing you to select elements based on their relationship with other elements. This makes it more versatile for complex queries.
- Supports Text Matching: XPath can locate elements based on their text content using the
text()
function. For example,//button[text()='Submit']
will locate a button with the text 'Submit'. - Advanced Queries: XPath allows for more advanced queries, such as using predicates to filter elements based on multiple conditions (e.g.,
//input[@type='text' and @name='username']
). - Can Handle Dynamic Content: XPath is often more effective in locating elements in dynamic content, especially when elements have changing attributes or structure.
- Cons of XPath:
- Slower Execution: XPath is generally slower than CSS selectors, especially when using complex expressions or traversing the DOM tree. This can impact test execution time.
- Complex Syntax: XPath expressions can be more verbose and harder to read, especially for beginners. More advanced XPath expressions can become difficult to maintain.
- Browser Inconsistencies: XPath might not behave the same across all browsers. Although modern browsers support XPath, there can be slight variations in behavior.
5. When to Use CSS Selectors
CSS selectors are ideal when:
- You need a simple and efficient way to locate elements based on attributes like
id
,class
, orname
. - You are working with a simple, static web page structure that doesn’t require navigating through complex relationships between elements.
- You prioritize faster execution and need to optimize test performance.
6. When to Use XPath
XPath is preferred when:
- You need to locate elements based on their text content.
- You need to traverse both up and down the DOM tree to find elements relative to other elements.
- You are working with a complex or dynamic page structure where elements’ attributes might change.
- You require advanced queries with multiple conditions or predicates.
7. Performance Comparison
In terms of performance, CSS selectors generally outperform XPath. This is because CSS selectors are more directly supported by browsers, while XPath requires additional processing. However, for complex queries or when navigating the DOM tree is necessary, XPath may still be the better choice despite the performance tradeoff.
8. Conclusion
Both CSS selectors and XPath have their place in Selenium automation. While CSS selectors are simpler and faster for straightforward element locators, XPath offers greater flexibility and power for more complex queries. Choosing between CSS selectors and XPath depends on the requirements of your automation tasks, the complexity of the DOM, and the performance considerations for your tests.
Handling Dynamic Elements (IDs, Classes)
Dynamic elements refer to elements on a web page whose attributes (such as IDs, classes, or text) change every time the page is loaded or refreshed. These changes can be caused by factors like session IDs, timestamps, or dynamically generated content. Handling dynamic elements is crucial in Selenium to ensure that your test scripts remain stable and reliable even when element attributes change.
1. Understanding Dynamic Elements
Dynamic elements are commonly found in web applications that use JavaScript to load or update content without reloading the entire page. These elements may have attributes like id
, class
, or name
that change with each page load. For example, an element's id
may include a timestamp or unique session ID, making it difficult to locate the element consistently using traditional locators.
2. Challenges with Dynamic Elements
Locating dynamic elements can be challenging because their attributes may change each time the page reloads. If your test script relies on static locators (like an exact id
or class
), it may fail when these attributes change. Common issues include:
- Flaky Tests: Tests may fail if they cannot find the element because the locator is no longer valid.
- Longer Execution Time: Additional logic may be required to handle dynamic elements, leading to longer test execution times.
- Maintenance Overhead: Dynamic locators require frequent updates to the test script as element attributes change.
3. Strategies for Handling Dynamic Elements
To handle dynamic elements effectively in Selenium, you can use several strategies that focus on locating elements reliably even when their attributes change.
3.1 Using Partial Matching for IDs and Classes
If the dynamic part of the id
or class
attribute is predictable, you can use partial matching. Selenium supports both partial matching for attributes using contains()
, starts-with()
, or ends-with()
functions in XPath.
# XPath example for partial matching of an ID
driver.findElement(By.xpath("//*[contains(@id, 'dynamic-prefix')]"));
# XPath example for partial matching of a class
driver.findElement(By.xpath("//*[contains(@class, 'dynamic-class')]"));
In the above examples, the test script will locate an element whose id
or class
contains the specified text. This allows for flexibility when the dynamic part changes (e.g., timestamps or session IDs).
3.2 Using CSS Selectors with Partial Matching
CSS selectors also support partial matching for dynamic attributes. You can use the *
operator to find elements whose attributes contain a specific substring.
# CSS selector example for partial matching of an ID
driver.findElement(By.cssSelector("[id*='dynamic-prefix']"));
# CSS selector example for partial matching of a class
driver.findElement(By.cssSelector("[class*='dynamic-class']"));
This method is similar to XPath's partial matching but uses CSS syntax, which can be more concise and easier to read.
3.3 Using Regular Expressions (XPath)
For more complex matching patterns, you can use regular expressions with XPath to match elements whose attributes follow a specific pattern. Regular expressions can be especially useful when the dynamic part of the element's attribute has a known structure.
# XPath example using regular expression for ID
driver.findElement(By.xpath("//*[matches(@id, '^dynamic-\\d{4}$')]"));
This XPath expression matches any element whose id
starts with dynamic-
followed by exactly four digits. Regular expressions provide powerful matching capabilities for handling complex dynamic elements.
3.4 Using Relative XPath to Locate Parent or Sibling Elements
In some cases, the dynamic elements may have a stable relationship with other elements on the page. Instead of relying on the dynamic attributes of the element itself, you can locate a parent, sibling, or nearby element with a static attribute and then navigate to the dynamic element relative to that stable element.
# XPath example using parent element
driver.findElement(By.xpath("//div[@id='static-div']//input[@id='dynamic-input']"));
# XPath example using sibling element
driver.findElement(By.xpath("//button[text()='Submit']//following-sibling::input[@id='dynamic-input']"));
This approach helps when the dynamic element is positioned relative to a stable element that does not change.
3.5 Waits for Dynamic Elements
When dealing with dynamic elements that may take some time to load or change, it is essential to use explicit waits. Selenium provides the WebDriverWait
class to wait until a condition is met, such as the visibility of an element. This helps ensure that the element is ready for interaction before proceeding with further actions.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamic-id")));
element.click();
By using waits, you can reduce the likelihood of encountering errors when working with dynamic elements that are still loading or changing.
4. Tips for Handling Dynamic Elements
- Use Stable Locators: Whenever possible, use locators that are less likely to change, such as
name
,id
, orclass
that do not depend on dynamic content. - Combine Multiple Locators: When an element's ID or class is dynamic, consider combining multiple attributes or using a combination of XPath and CSS selectors to create a more reliable locator.
- Use Waits Effectively: Always ensure that dynamic elements have fully loaded or changed before interacting with them to avoid timing issues.
5. Conclusion
Handling dynamic elements requires more advanced techniques than static elements, but it is essential for building reliable automation scripts. By using strategies like partial matching, regular expressions, relative XPath, and waits, you can create more robust and maintainable Selenium scripts. Proper handling of dynamic elements helps ensure that your tests remain stable and reliable even in the face of changing content on the web page.
Finding Multiple Elements (find_elements)
In Selenium, finding multiple elements is essential when automating tasks that involve interacting with multiple elements on a webpage, such as links, buttons, or form fields. The find_elements
method allows you to locate and interact with a collection of elements that match a specific locator.
1. What is find_elements
?
The find_elements
method in Selenium is used to locate multiple elements on a webpage that match a given locator. Unlike find_element
, which returns a single element, find_elements
returns a list (or array) of all matching elements. If no elements are found, it returns an empty list.
The syntax for using find_elements
is:
# Python example
elements = driver.find_elements(By.tagName("button"))
In this example, find_elements
will return a list of all <button>
elements on the page.
2. Common Use Cases for find_elements
find_elements
is commonly used in the following scenarios:
- Handling Lists of Elements: When you need to interact with multiple elements like links, buttons, or checkboxes,
find_elements
allows you to retrieve all matching elements. - Scraping Data: If you need to extract data from multiple elements, such as all links or all product names on an e-commerce website,
find_elements
helps you collect them. - Performing Bulk Actions: If your automation script needs to perform an action on every element in a list, such as clicking a series of buttons,
find_elements
allows you to loop through and execute actions on each element.
3. Syntax for Using find_elements
To use find_elements
, you need to provide the locator type and the value for the locator, just as you would with find_element
. Common locator strategies include By.id
, By.className
, By.name
, By.xpath
, and By.cssSelector
.
3.1 Python Example - Finding Multiple Elements by Tag Name
# Find all button elements on the page
buttons = driver.find_elements(By.tagName("button"))
# Iterate over the list of buttons and print their text
for button in buttons:
print(button.text)
button.click()
In this example, all buttons on the page are located using the find_elements
method with the By.tagName
locator. The script then iterates through the list of buttons and prints the text of each button before clicking it.
3.2 Java Example - Finding Multiple Elements by Class Name
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.List;
public class MultipleElementsExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Find all elements with the class name 'product'
List products = driver.findElements(By.className("product"));
// Iterate through the list and print the text of each product
for (WebElement product : products) {
System.out.println(product.getText());
}
driver.quit();
}
}
In the Java example, the script finds all elements with the class
name "product" and iterates through them to print the text of each product.
3.3 JavaScript Example - Finding Multiple Elements by CSS Selector
const elements = driver.findElements(By.cssSelector(".product-item"));
elements.forEach(element => {
console.log(element.getText());
});
This JavaScript example demonstrates how to use findElements
with a cssSelector
to find all elements with the "product-item" class. It then logs the text of each element to the console.
4. Working with the List of Elements
The list returned by find_elements
is a collection of WebElement objects. You can interact with these elements just like any other Selenium element. Common actions include:
- Clicking: You can perform actions such as clicking on each element in the list.
for button in buttons:
button.click()
getText()
method in Java or text
in Python.
for button in buttons:
print(button.text)
href
for links or value
for input fields.
for link in links:
print(link.get_attribute("href"))
5. Handling Empty or Non-Existent Lists
If no elements are found, find_elements
will return an empty list. This allows you to safely check if elements exist without throwing an exception. For example, you can check if the list is empty before performing actions:
buttons = driver.find_elements(By.tagName("button"))
if buttons:
for button in buttons:
button.click()
else:
print("No buttons found.")
By checking if the list is empty, you can ensure that your script does not fail unnecessarily when no elements are found.
6. Best Practices for Using find_elements
- Use Efficient Locators: Use the most efficient locator strategy (e.g.,
By.id
orBy.className
) to avoid performance issues when retrieving many elements. - Handle Empty Lists Gracefully: Always check if the list is empty before interacting with the elements to prevent errors in your script.
- Use Explicit Waits: Use waits to ensure that the elements are present on the page before interacting with them. This helps avoid issues with timing and loading delays.
7. Conclusion
Finding multiple elements in Selenium is a powerful way to interact with many elements on a webpage. By using the find_elements
method, you can locate and perform actions on multiple elements efficiently. Handling the returned list and using best practices for locating and interacting with elements ensures that your scripts are robust, stable, and maintainable.
Interacting with Text Boxes and Buttons
In Selenium, interacting with text boxes and buttons is a common task when automating form submissions, login processes, or other actions that require user input. Selenium provides various methods to interact with these elements, such as sending keystrokes to text boxes and clicking on buttons.
1. Interacting with Text Boxes
Text boxes are input fields where users enter data. To automate filling out forms, you can use Selenium to locate these text boxes and send text input to them.
1.1 Python Example - Entering Text into a Text Box
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com/form")
# Locate the text box and enter text
text_box = driver.find_element(By.id("username"))
text_box.send_keys("myUsername")
# Close the browser
driver.quit()
In this example, we locate the text box with the ID username
and send the text "myUsername" using the send_keys
method.
1.2 Java Example - Entering Text into a Text Box
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class EnterTextExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/form");
// Locate the text box and enter text
WebElement textBox = driver.findElement(By.id("username"));
textBox.sendKeys("myUsername");
driver.quit();
}
}
This Java example shows how to locate a text box by ID and send the text "myUsername" to it using the sendKeys
method.
1.3 JavaScript Example - Entering Text into a Text Box
const { Builder, By } = require('selenium-webdriver');
(async function enterText() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://example.com/form');
// Locate the text box and enter text
let textBox = await driver.findElement(By.id('username'));
await textBox.sendKeys('myUsername');
await driver.quit();
})();
This JavaScript example demonstrates how to use Selenium WebDriver to enter text into a text box by locating it with the ID "username" and sending the text "myUsername".
2. Interacting with Buttons
Buttons are elements that trigger actions when clicked. To automate button clicks, you can locate the button and use the click
method in Selenium.
2.1 Python Example - Clicking a Button
# Locate the button and click it
button = driver.find_element(By.id("submit"))
button.click()
In this Python example, we locate a button with the ID submit
and click it using the click
method.
2.2 Java Example - Clicking a Button
WebElement button = driver.findElement(By.id("submit"));
button.click();
The Java example locates a button with the ID submit
and clicks it using the click
method.
2.3 JavaScript Example - Clicking a Button
let button = await driver.findElement(By.id('submit'));
await button.click();
The JavaScript example demonstrates how to locate and click a button with the ID submit
using the click
method.
3. Clearing Text Boxes
If you need to clear a text box before entering new text, you can use the clear
method. This is helpful when automating form submissions where you need to reset input fields.
3.1 Python Example - Clearing a Text Box
# Locate the text box and clear any existing text
text_box.clear()
# Enter new text into the text box
text_box.send_keys("newUsername")
In this Python example, the text box is cleared first using the clear
method, and then new text ("newUsername") is entered.
3.2 Java Example - Clearing a Text Box
textBox.clear();
textBox.sendKeys("newUsername");
This Java example clears the text box before entering new text ("newUsername").
3.3 JavaScript Example - Clearing a Text Box
await textBox.clear();
await textBox.sendKeys('newUsername');
In this JavaScript example, the text box is cleared using the clear
method, followed by entering the new text ("newUsername").
4. Best Practices for Interacting with Text Boxes and Buttons
- Use Explicit Waits: Always use explicit waits when interacting with text boxes or buttons to ensure they are ready for interaction (e.g., visible, clickable).
- Clear Text Boxes Before Input: If the text box contains pre-filled data or you need to reset it, use the
clear
method before sending new text. - Check Button Availability: Ensure the button is enabled before attempting to click it. This prevents errors if the button is disabled or hidden.
- Handle Popups: If a button triggers a popup or a new page, ensure that the script waits for the popup to appear or the new page to load.
5. Conclusion
Interacting with text boxes and buttons is a fundamental part of automating web forms and actions. With Selenium, you can easily send text inputs, clear text boxes, and click buttons to simulate user interactions. By following best practices, you can make your scripts more reliable and effective.
Selecting Items from Drop-Down Menus
In many web applications, drop-down menus are used to allow users to select an option from a list of pre-defined values. Selenium provides a Select
class to interact with drop-down menus and select items based on their visible text, index, or value.
1. Interacting with Drop-Down Menus
To interact with a drop-down menu, first, you need to locate the <select>
element, and then you can use the Select
class to select options.
1.1 Python Example - Selecting an Item by Visible Text
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import Select
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com/form")
# Locate the drop-down menu and create a Select object
drop_down = driver.find_element(By.id("dropdown"))
select = Select(drop_down)
# Select an option by visible text
select.select_by_visible_text("Option 1")
# Close the browser
driver.quit()
In this Python example, the drop-down menu is located using its ID dropdown
, and an option is selected using select_by_visible_text
with the text "Option 1".
1.2 Java Example - Selecting an Item by Visible Text
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
public class SelectDropdownExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/form");
// Locate the drop-down menu
WebElement dropDown = driver.findElement(By.id("dropdown"));
// Create a Select object
Select select = new Select(dropDown);
// Select an option by visible text
select.selectByVisibleText("Option 1");
driver.quit();
}
}
This Java example locates the drop-down menu using its ID dropdown
and selects an option by its visible text using the selectByVisibleText
method.
1.3 JavaScript Example - Selecting an Item by Visible Text
const { Builder, By, until } = require('selenium-webdriver');
const { Select } = require('selenium-webdriver/lib/select');
(async function selectDropdown() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://example.com/form');
// Locate the drop-down menu
let dropDown = await driver.findElement(By.id('dropdown'));
// Create a Select object
let select = new Select(dropDown);
// Select an option by visible text
await select.selectByVisibleText('Option 1');
await driver.quit();
})();
The JavaScript example demonstrates how to select an option from a drop-down menu by visible text using the selectByVisibleText
method in Selenium WebDriver.
2. Alternative Methods to Select Items
In addition to selecting items by visible text, you can also select them by their value attribute or index in the list.
2.1 Python Example - Selecting an Item by Value
# Select an option by its value attribute
select.select_by_value("option_value")
This Python example demonstrates selecting an option by its value attribute. Replace option_value
with the actual value attribute of the option you want to select.
2.2 Java Example - Selecting an Item by Value
select.selectByValue("option_value");
In this Java example, the selectByValue
method is used to select an option based on its value attribute.
2.3 JavaScript Example - Selecting an Item by Value
await select.selectByValue('option_value');
The JavaScript example shows how to select an item by its value attribute using the selectByValue
method.
2.4 Python Example - Selecting an Item by Index
# Select an option by index (0-based)
select.select_by_index(1) # Selects the second option
This Python example demonstrates how to select an option based on its index in the drop-down list.
2.5 Java Example - Selecting an Item by Index
select.selectByIndex(1); // Selects the second option
The Java example shows how to select an item by index, where the index is 0-based.
2.6 JavaScript Example - Selecting an Item by Index
await select.selectByIndex(1); // Selects the second option
The JavaScript example demonstrates selecting an option by its index in the drop-down menu.
3. Handling Multiple Selections
Some drop-down menus allow multiple selections. To interact with these, you can use the is_multiple
property to check if the drop-down allows multiple selections, and use methods like select_by_visible_text
multiple times or select_all
to select multiple options.
3.1 Python Example - Selecting Multiple Options
# Check if the drop-down allows multiple selections
if select.is_multiple:
select.select_by_visible_text("Option 1")
select.select_by_visible_text("Option 2")
This Python example checks if the drop-down allows multiple selections, and if so, selects multiple options using the select_by_visible_text
method.
3.2 Java Example - Selecting Multiple Options
if(select.isMultiple()) {
select.selectByVisibleText("Option 1");
select.selectByVisibleText("Option 2");
}
The Java example demonstrates handling multiple selection drop-downs by checking the isMultiple
property and selecting multiple options.
4. Best Practices for Handling Drop-Down Menus
- Use Explicit Waits: Always use explicit waits to ensure the drop-down menu is present and interactable before making selections.
- Handle Dynamic Drop-Downs: If the drop-down options are dynamic (e.g., loaded via AJAX), ensure the options are fully loaded before interacting with them.
- Check for Multiple Selections: If you need to select multiple options, verify that the drop-down allows multiple selections by using the
is_multiple
property.
5. Conclusion
Interacting with drop-down menus is a common task in web automation. Selenium’s Select
class provides methods to select options by visible text, value, or index. By following best practices, you can ensure that your automation scripts interact with drop-down menus reliably and efficiently.
Handling Checkboxes and Radio Buttons
Checkboxes and radio buttons are commonly used form elements in web applications. Selenium provides methods to interact with these elements, allowing you to check, uncheck, or select specific options based on user interaction.
1. Interacting with Checkboxes
Checkboxes are used to allow users to select or deselect options. In Selenium, you can use methods like click()
to select or deselect a checkbox, and the is_selected()
method to check if a checkbox is checked or unchecked.
1.1 Python Example - Checking a Checkbox
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com/form")
# Locate the checkbox
checkbox = driver.find_element(By.id("checkbox"))
# Check the checkbox if it is not already checked
if not checkbox.is_selected():
checkbox.click()
# Close the browser
driver.quit()
This Python example demonstrates how to check a checkbox only if it is not already selected. The is_selected()
method is used to check the current state of the checkbox, and the click()
method is used to select it.
1.2 Java Example - Checking a Checkbox
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CheckboxExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/form");
// Locate the checkbox
WebElement checkbox = driver.findElement(By.id("checkbox"));
// Check the checkbox if it is not already checked
if (!checkbox.isSelected()) {
checkbox.click();
}
driver.quit();
}
}
This Java example shows how to check a checkbox using the isSelected()
and click()
methods.
1.3 JavaScript Example - Checking a Checkbox
const { Builder, By } = require('selenium-webdriver');
(async function checkCheckbox() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://example.com/form');
// Locate the checkbox
let checkbox = await driver.findElement(By.id('checkbox'));
// Check the checkbox if it is not already checked
let isChecked = await checkbox.isSelected();
if (!isChecked) {
await checkbox.click();
}
await driver.quit();
})();
The JavaScript example demonstrates how to check a checkbox using the isSelected()
and click()
methods in Selenium WebDriver.
2. Interacting with Radio Buttons
Radio buttons are used when only one option from a group of options should be selected. Selenium allows you to select a radio button by using the click()
method. If the radio button is already selected, no action will be performed.
2.1 Python Example - Selecting a Radio Button
# Locate the radio button
radio_button = driver.find_element(By.id("radio_button"))
# Select the radio button if it is not already selected
if not radio_button.is_selected():
radio_button.click()
This Python example demonstrates how to select a radio button only if it is not already selected, using is_selected()
and click()
.
2.2 Java Example - Selecting a Radio Button
WebElement radioButton = driver.findElement(By.id("radio_button"));
// Select the radio button if it is not already selected
if (!radioButton.isSelected()) {
radioButton.click();
}
This Java example demonstrates how to select a radio button using the isSelected()
and click()
methods.
2.3 JavaScript Example - Selecting a Radio Button
let radioButton = await driver.findElement(By.id('radio_button'));
// Select the radio button if it is not already selected
let isRadioSelected = await radioButton.isSelected();
if (!isRadioSelected) {
await radioButton.click();
}
The JavaScript example demonstrates how to select a radio button by checking its current state with isSelected()
and selecting it with click()
.
3. Best Practices for Handling Checkboxes and Radio Buttons
- Check Current State: Always check whether the checkbox or radio button is already in the desired state before interacting with it to avoid unnecessary clicks.
- Wait for Elements to Load: Ensure the checkbox or radio button is visible and interactable by using explicit waits before performing any action.
- Clear Selection (Checkboxes): If you need to uncheck a checkbox, use the
click()
method again to deselect it. - Radio Buttons: Radio buttons are mutually exclusive, meaning only one option can be selected at a time. Ensure that you select only the correct radio button for the scenario.
4. Conclusion
Handling checkboxes and radio buttons is a fundamental task when automating web forms. Selenium provides simple methods to interact with these elements, whether it’s checking/unchecking a checkbox or selecting a radio button. By following best practices, you can ensure that your automation scripts are robust and reliable when interacting with form elements.
Working with Date Pickers and Calendars
Date pickers and calendars are essential UI elements in web applications, enabling users to select dates from a calendar widget. Automating interactions with these elements can be tricky, but Selenium provides methods to select dates and handle calendar interactions smoothly.
1. Understanding Date Pickers
Date pickers are often implemented as input fields with an attached calendar popup. The user can either type the date directly or select it from the calendar. In Selenium, interacting with date pickers typically involves selecting specific date elements or sending keyboard input.
2. Interacting with Date Pickers in Selenium
To work with date pickers, you can either send the date directly as a string to the input field or interact with the calendar widget to select a specific date. Here’s how you can handle both approaches:
2.1 Python Example - Sending a Date to the Input Field
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com/form")
# Locate the date picker input field
date_picker = driver.find_element(By.id("date_picker"))
# Send the desired date to the input field
date_picker.send_keys("2025-01-24")
# Close the browser
driver.quit()
This Python example demonstrates how to interact with a date picker by sending a date string directly to the input field using the send_keys()
method.
2.2 Java Example - Sending a Date to the Input Field
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class DatePickerExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/form");
// Locate the date picker input field
WebElement datePicker = driver.findElement(By.id("date_picker"));
// Send the desired date to the input field
datePicker.sendKeys("2025-01-24");
driver.quit();
}
}
This Java example demonstrates how to interact with a date picker by sending a date string directly to the input field using the sendKeys()
method.
2.3 JavaScript Example - Sending a Date to the Input Field
const { Builder, By } = require('selenium-webdriver');
(async function sendDate() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://example.com/form');
// Locate the date picker input field
let datePicker = await driver.findElement(By.id('date_picker'));
// Send the desired date to the input field
await datePicker.sendKeys('2025-01-24');
await driver.quit();
})();
The JavaScript example demonstrates how to send a date to the input field using the sendKeys()
method in Selenium WebDriver.
3. Interacting with the Calendar Widget
If the date picker involves a calendar widget, you may need to interact with the individual date elements. You can locate the calendar and use Selenium to select the required date by clicking on the appropriate date element.
3.1 Python Example - Selecting a Date from the Calendar
# Locate the calendar icon and click it to open the calendar
calendar_icon = driver.find_element(By.id("calendar_icon"))
calendar_icon.click()
# Select a specific date from the calendar
date = driver.find_element(By.xpath("//td[@data-date='2025-01-24']"))
date.click()
This Python example demonstrates how to open a calendar widget and select a specific date by locating the date element using XPath and clicking on it.
3.2 Java Example - Selecting a Date from the Calendar
WebElement calendarIcon = driver.findElement(By.id("calendar_icon"));
calendarIcon.click();
// Locate and select the specific date from the calendar
WebElement date = driver.findElement(By.xpath("//td[@data-date='2025-01-24']"));
date.click();
This Java example demonstrates how to open a calendar widget and select a specific date by locating the date element using XPath.
3.3 JavaScript Example - Selecting a Date from the Calendar
let calendarIcon = await driver.findElement(By.id('calendar_icon'));
await calendarIcon.click();
// Locate and select the specific date from the calendar
let date = await driver.findElement(By.xpath("//td[@data-date='2025-01-24']"));
await date.click();
This JavaScript example demonstrates how to open a calendar widget and select a specific date by clicking on the appropriate date element using XPath.
4. Best Practices for Handling Date Pickers and Calendars
- Use Explicit Waits: Ensure that the date picker or calendar element is fully loaded and clickable before interacting with it. Use WebDriver waits to handle dynamic loading.
- Verify Date Format: When sending a date to an input field, ensure that the format matches the expected format (e.g., YYYY-MM-DD) to avoid errors.
- Consider Localization: Some date pickers may display dates in different formats based on the locale. Take this into account when automating.
- Handle Calendar Widgets: Interacting with calendar widgets may require clicking on specific date cells. Use appropriate locators (e.g., XPath, CSS selectors) to pinpoint the date element.
5. Conclusion
Working with date pickers and calendars can be challenging due to their dynamic nature. However, with the right techniques in Selenium, you can automate date selection with ease. Whether you are sending dates directly to input fields or interacting with calendar widgets, understanding how to handle these elements will enhance the reliability of your automation scripts.
Automating File Uploads and Downloads
Automating file uploads and downloads is a common requirement in testing web applications. Selenium provides ways to handle file input elements to automate these processes effectively. However, handling file downloads might require additional browser settings or manual configurations.
1. Automating File Uploads
To automate file uploads, you need to interact with file input elements (``) on a web page. Selenium allows you to send the file path directly to these input fields to upload files without any manual intervention.
1.1 Python Example - Uploading a File
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage with the file upload form
driver.get("https://example.com/upload")
# Locate the file input element
file_input = driver.find_element(By.id("file_input"))
# Send the file path to the input element
file_input.send_keys("C:/path/to/your/file.txt")
# Submit the form or trigger the upload
submit_button = driver.find_element(By.id("submit_button"))
submit_button.click()
# Close the browser
driver.quit()
In this Python example, the file input element is located using its ID, and the file path is sent to the element using the send_keys()
method. After the file is selected, the form is submitted.
1.2 Java Example - Uploading a File
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class FileUploadExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/upload");
// Locate the file input element
WebElement fileInput = driver.findElement(By.id("file_input"));
// Send the file path to the input element
fileInput.sendKeys("C:/path/to/your/file.txt");
// Submit the form or trigger the upload
WebElement submitButton = driver.findElement(By.id("submit_button"));
submitButton.click();
driver.quit();
}
}
This Java example demonstrates the same file upload process, where the file path is sent to the file input field and the form is submitted after the file is chosen.
1.3 JavaScript Example - Uploading a File
const { Builder, By } = require('selenium-webdriver');
(async function uploadFile() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://example.com/upload');
// Locate the file input element
let fileInput = await driver.findElement(By.id('file_input'));
// Send the file path to the input element
await fileInput.sendKeys('C:/path/to/your/file.txt');
// Submit the form or trigger the upload
let submitButton = await driver.findElement(By.id('submit_button'));
await submitButton.click();
await driver.quit();
})();
The JavaScript example follows a similar approach, sending the file path to the file input field and triggering the upload via the submit button.
2. Automating File Downloads
Automating file downloads is more complex compared to uploads. Selenium cannot directly interact with the "Save As" dialog or browser file download prompts. To automate downloads, you need to adjust browser preferences or use tools like AutoIT (for Windows) or Robot class in Java.
2.1 Python Example - Handling File Downloads
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
import os
# Set up browser preferences to auto-download files to a specific folder
options = webdriver.ChromeOptions()
download_dir = "C:/path/to/download/folder"
prefs = {"download.default_directory": download_dir}
options.add_experimental_option("prefs", prefs)
# Set up the driver with options
driver = webdriver.Chrome(executable_path="path/to/chromedriver", options=options)
# Open the webpage with the download link
driver.get("https://example.com/download")
# Locate and click the download link or button
download_button = driver.find_element(By.id("download_button"))
download_button.click()
# Wait for the file to be downloaded
time.sleep(5) # Wait time may vary depending on file size
# Verify the file has been downloaded
if os.path.exists(os.path.join(download_dir, "file.txt")):
print("File downloaded successfully!")
# Close the browser
driver.quit()
In this Python example, the browser’s download preferences are set to automatically save files to a specific directory. After clicking the download button, the script waits for the file to download and then verifies if the file is present in the target folder.
2.2 Java Example - Handling File Downloads
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.io.File;
import java.util.concurrent.TimeUnit;
public class FileDownloadExample {
public static void main(String[] args) throws InterruptedException {
// Set up ChromeOptions to automatically download files
ChromeOptions options = new ChromeOptions();
String downloadDir = "C:/path/to/download/folder";
options.addArguments("download.default_directory=" + downloadDir);
WebDriver driver = new ChromeDriver(options);
driver.get("https://example.com/download");
// Locate and click the download link
driver.findElement(By.id("download_button")).click();
// Wait for the file to download
TimeUnit.SECONDS.sleep(5); // Adjust the wait time as necessary
// Verify the file has been downloaded
File file = new File(downloadDir + "/file.txt");
if (file.exists()) {
System.out.println("File downloaded successfully!");
}
driver.quit();
}
}
This Java example sets the download preferences and waits for the file to download before verifying the download location.
2.3 JavaScript Example - Handling File Downloads
const { Builder, By } = require('selenium-webdriver');
const fs = require('fs');
const path = require('path');
(async function downloadFile() {
let downloadDir = 'C:/path/to/download/folder';
// Set up browser preferences for automatic download
let options = new (require('selenium-webdriver/chrome').Options)();
options.setUserPreferences({'download.default_directory': downloadDir});
let driver = await new Builder().forBrowser('chrome').setChromeOptions(options).build();
await driver.get('https://example.com/download');
// Locate and click the download link
let downloadButton = await driver.findElement(By.id('download_button'));
await downloadButton.click();
// Wait for the file to be downloaded
await new Promise(resolve => setTimeout(resolve, 5000)); // Adjust as necessary
// Verify the file has been downloaded
if (fs.existsSync(path.join(downloadDir, 'file.txt'))) {
console.log('File downloaded successfully!');
}
await driver.quit();
})();
This JavaScript example sets up download preferences and waits for the file to download before verifying the file’s presence in the specified directory.
3. Best Practices for Automating File Uploads and Downloads
- Use Correct File Paths: Ensure the file paths are correct and valid, whether for uploading or downloading files, to avoid errors.
- Automate Browser Preferences: Set browser preferences for file downloads to avoid the "Save As" dialogs and automate the download process.
- Handle Wait Times: Include wait times to ensure files are completely uploaded or downloaded before proceeding with further actions in the script.
- Test File Types: Ensure that the uploaded files are of the correct type and the downloaded files match the expected formats.
4. Conclusion
Automating file uploads and downloads in Selenium can be easily achieved with the right techniques. By interacting with file input elements for uploads and adjusting browser preferences for downloads, you can ensure smooth automation of these tasks. Always ensure that the file paths and browser settings are correct to avoid errors and improve the reliability of your scripts.
Implicit Waits vs Explicit Waits
In Selenium, waits are essential to handle elements that load dynamically, such as those that appear after a certain delay. Selenium provides two primary types of waits: Implicit Waits and Explicit Waits. Both waits are used to manage synchronization issues in automation scripts, but they function differently and have distinct use cases.
1. Implicit Wait
Implicit Wait is used to define a general wait time for the entire duration of the script. It tells Selenium to wait for a certain period before throwing an exception if the element is not immediately available. Once set, the implicit wait is applied to all elements in the script.
1.1 How Implicit Wait Works
When an element is not immediately found, Selenium will wait for the specified duration before throwing a NoSuchElementException
. If the element appears before the timeout, Selenium proceeds with the next operation.
1.2 Python Example - Using Implicit Wait
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Apply Implicit Wait of 10 seconds
driver.implicitly_wait(10)
# Open the webpage
driver.get("https://example.com")
# Locate the element
element = driver.find_element(By.id("element_id"))
# Interact with the element
element.click()
# Close the browser
driver.quit()
In this Python example, an implicit wait of 10 seconds is applied using the implicitly_wait()
method. Selenium will wait up to 10 seconds for the element to appear before interacting with it.
1.3 Java Example - Using Implicit Wait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ImplicitWaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Apply Implicit Wait of 10 seconds
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://example.com");
// Locate the element
driver.findElement(By.id("element_id")).click();
driver.quit();
}
}
This Java example demonstrates how to set an implicit wait of 10 seconds using manage().timeouts().implicitlyWait()
, which applies to all elements in the session.
2. Explicit Wait
Explicit Wait is used to define a specific wait condition for a particular element. Unlike implicit waits, explicit waits allow you to wait for specific conditions to be met, such as an element becoming visible, clickable, or present, before performing an action.
2.1 How Explicit Wait Works
Explicit waits are more flexible than implicit waits. You can specify conditions, such as waiting for an element to be visible or clickable, using the WebDriverWait
class combined with ExpectedConditions
in Selenium. Once the condition is met, the script continues; otherwise, it will throw a timeout exception.
2.2 Python Example - Using Explicit Wait
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com")
# Define Explicit Wait for an element to be clickable
wait = WebDriverWait(driver, 10)
element = wait.until(EC.element_to_be_clickable((By.id, "element_id")))
# Interact with the element
element.click()
# Close the browser
driver.quit()
This Python example demonstrates how to use WebDriverWait
to wait for a specific element to be clickable. The script waits up to 10 seconds for the element to be clickable before performing the click action.
2.3 Java Example - Using Explicit Wait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class ExplicitWaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Define Explicit Wait for an element to be clickable
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.elementToBeClickable(By.id("element_id"))).click();
driver.quit();
}
}
In this Java example, we use WebDriverWait
combined with ExpectedConditions
to explicitly wait for an element to be clickable before performing the click action.
3. Key Differences Between Implicit Wait and Explicit Wait
Aspect | Implicit Wait | Explicit Wait |
---|---|---|
Scope | Applied globally to all elements in the script. | Applied to specific elements and conditions. |
Wait Type | Waits for a fixed time (default for all elements). | Waits until a specific condition is met (e.g., element visibility, clickability). |
Flexibility | Less flexible, as it applies to all elements. | Highly flexible, as it can be customized for different conditions. |
Performance | May slow down tests if set for a long period. | More efficient, as it only waits for specific conditions. |
Timeout | Occurs after a fixed time if the element is not found. | Occurs after a condition timeout is met. |
4. Best Practices for Using Waits
- Use Explicit Waits for Specific Conditions: Prefer using explicit waits for elements where you need to wait for a specific condition, such as visibility or clickability.
- Use Implicit Waits for General Waits: Implicit waits are best for general waiting, but avoid setting a long implicit wait time, as it could lead to unnecessary delays.
- Don’t Mix Implicit and Explicit Waits: Mixing waits can lead to unpredictable behavior. It’s best to stick to one type of wait for consistency.
- Set Reasonable Timeout Durations: Set timeouts appropriately based on the expected load time of elements on your web page.
5. Conclusion
Both implicit and explicit waits are essential tools in Selenium for handling dynamic web elements. Implicit waits are useful for general synchronization, while explicit waits give you more control over waiting for specific conditions. By understanding the differences and best practices for each, you can write more efficient and reliable Selenium scripts.
WebDriverWait Class for Advanced Waits
The WebDriverWait
class in Selenium is used for advanced waits, providing more control over the conditions that need to be met before proceeding with actions. It is specifically designed for handling dynamic elements that may take time to appear, become clickable, or meet other conditions. By leveraging this class, you can implement more precise waits, ensuring that your automation scripts handle dynamic web elements efficiently.
1. What is WebDriverWait?
The WebDriverWait
class is used in conjunction with ExpectedConditions
to wait for specific conditions to be true before interacting with elements. Unlike implicit waits, which apply globally to all elements, the WebDriverWait
class allows you to specify an explicit condition for a specific element.
2. Syntax of WebDriverWait
The basic syntax of the WebDriverWait
class is as follows:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.elementToBeClickable(By.id("element_id")));
In this syntax, we create a new WebDriverWait
instance, passing the driver and the maximum wait time. Then, we use the until()
method with an expected condition, such as waiting for an element to be clickable.
3. Common ExpectedConditions with WebDriverWait
WebDriverWait works in combination with various ExpectedConditions
to wait for certain states of web elements. Some common expected conditions include:
- elementToBeClickable: Waits for an element to be visible and enabled so that it can be clicked.
- visibilityOfElementLocated: Waits for an element to be visible on the page.
- presenceOfElementLocated: Waits for an element to be present in the DOM.
- textToBePresentInElement: Waits for specific text to appear in an element.
- alertIsPresent: Waits for an alert box to appear.
- invisibilityOfElementLocated: Waits for an element to be invisible on the page.
4. Python Example - Using WebDriverWait
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open the webpage
driver.get("https://example.com")
# Create WebDriverWait instance
wait = WebDriverWait(driver, 10)
# Wait until the element is clickable
element = wait.until(EC.element_to_be_clickable((By.id, "element_id")))
# Interact with the element
element.click()
# Close the browser
driver.quit()
This Python example demonstrates how to use WebDriverWait
to wait for an element to be clickable before performing the click action.
5. Java Example - Using WebDriverWait
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class WebDriverWaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Create WebDriverWait instance
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// Wait until the element is clickable
wait.until(ExpectedConditions.elementToBeClickable(By.id("element_id"))).click();
driver.quit();
}
}
This Java example shows how to use WebDriverWait
with ExpectedConditions
to wait for an element to be clickable.
6. Wait for Multiple Conditions
In some cases, you might want to wait for multiple conditions to be met. You can combine multiple conditions using logical operators or by chaining waits. For example, you might wait for an element to be visible and clickable at the same time.
wait.until(EC.and_(
EC.visibility_of_element_located((By.id, "element_id")),
EC.element_to_be_clickable((By.id, "element_id"))
))
In this example, the script waits for an element to be both visible and clickable using the and_
operator in Python.
7. Handling Timeout Exceptions
When using WebDriverWait
, if the condition is not met within the specified time, a TimeoutException
will be thrown. To handle this exception, you can use a try-except block in Python or a try-catch block in Java to catch the exception and handle it gracefully.
Python Example - Handling TimeoutException
from selenium.common.exceptions import TimeoutException
try:
element = wait.until(EC.element_to_be_clickable((By.id, "element_id")))
element.click()
except TimeoutException:
print("The element was not clickable within the given time.")
finally:
driver.quit()
This Python example demonstrates how to handle a TimeoutException
if the element is not clickable within the specified time.
Java Example - Handling TimeoutException
import org.openqa.selenium.TimeoutException;
try {
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.elementToBeClickable(By.id("element_id"))).click();
} catch (TimeoutException e) {
System.out.println("The element was not clickable within the given time.");
} finally {
driver.quit();
}
This Java example shows how to handle the TimeoutException
using a try-catch block.
8. Best Practices for Using WebDriverWait
- Use Specific Conditions: Always use the most specific condition, such as
elementToBeClickable
orvisibilityOfElementLocated
, to avoid unnecessary waits. - Avoid Long Wait Times: Set reasonable wait times to avoid slowing down your tests. Too long waits can lead to inefficient test execution.
- Don’t Overuse WebDriverWait: While
WebDriverWait
is essential, it should be used sparingly and only for situations where elements take time to load or become ready. - Combine Conditions When Necessary: Use logical operators to combine multiple conditions if necessary to wait for multiple events (e.g., visibility and clickability).
9. Conclusion
The WebDriverWait
class is a powerful tool in Selenium for handling dynamic elements and waiting for specific conditions to be met. By combining it with various ExpectedConditions
, you can create precise waits that ensure your automation scripts interact with elements only when they are ready. This leads to more stable, efficient, and reliable automation scripts.
Fluent Waits: Handling Dynamic Web Pages
Fluent Waits in Selenium are an advanced form of waiting that allows you to specify both the frequency with which the condition is checked and the maximum amount of time to wait for a condition to be met. This makes Fluent Waits ideal for handling dynamic web pages where elements may appear, change, or disappear at unpredictable times. Fluent Waits help you avoid unnecessary delays while ensuring your automation script can interact with elements that are not immediately available.
1. What is Fluent Wait?
A Fluent Wait is a type of explicit wait that provides more control over waiting for conditions to be met. It allows you to define:
- The maximum amount of time to wait.
- The polling interval, which is how often the condition is checked.
- The option to ignore specific exceptions (like
NoSuchElementException
) during the wait time.
This gives you greater flexibility than the standard WebDriverWait
class.
2. Syntax of Fluent Wait
The basic syntax of Fluent Wait is as follows:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.FluentWait;
import java.time.Duration;
import java.util.function.Function;
public class FluentWaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
FluentWait wait = new FluentWait(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(new Function() {
public WebElement apply(WebDriver driver) {
return driver.findElement(By.id("dynamicElement"));
}
});
element.click();
driver.quit();
}
}
In this example, the Fluent Wait checks for the presence of an element with ID dynamicElement
every 5 seconds. If it doesn't find the element within 30 seconds, it throws a TimeoutException
.
3. Key Components of Fluent Wait
- withTimeout: Defines the maximum time to wait for the condition to be true.
- pollingEvery: Defines how often the condition is checked during the wait time.
- ignoring: Specifies which exceptions should be ignored during the wait. This is useful to avoid exceptions like
NoSuchElementException
when elements are temporarily unavailable.
4. Python Example - Using Fluent Wait
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.ui import FluentWait
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
driver.get("https://example.com")
# Fluent wait setup
wait = WebDriverWait(driver, 30, 5)
wait.until(lambda driver: driver.find_element(By.id("dynamicElement")))
# Interact with the element
element = driver.find_element(By.id("dynamicElement"))
element.click()
# Close the browser
driver.quit()
This Python example demonstrates how to use Fluent Wait in Selenium. It waits for an element with the ID dynamicElement
to become available for a maximum of 30 seconds, checking every 5 seconds.
5. Advantages of Fluent Waits
- Customizable Polling: Fluent Waits allow you to specify how frequently the condition should be checked, which is useful for handling dynamic web elements that may appear or disappear intermittently.
- Exception Handling: You can ignore specific exceptions (e.g.,
NoSuchElementException
) during the wait period, making the automation more resilient to temporary issues. - Precise Timing: Fluent Waits provide more flexibility in controlling how long the script waits for specific conditions to be true, reducing the risk of unnecessary delays.
6. Use Cases for Fluent Waits
Fluent Waits are particularly useful in the following scenarios:- Dynamic Elements: When elements take unpredictable amounts of time to appear, disappear, or change state (e.g., animations, loading spinners, or AJAX calls).
- Handling Popups and Alerts: When popups or alerts may appear at varying times, Fluent Waits can be used to wait for them without causing test failures.
- Elements that Appear After Delay: When elements are loaded or updated after a delay (like data in a table or search results), Fluent Waits can ensure the elements are available before interacting with them.
7. Handling Multiple Dynamic Elements
Fluent Waits can be combined with other Selenium commands to handle multiple dynamic elements effectively. For example, you can wait for a list of elements to appear and then interact with them one by one.
List elements = wait.until(new Function>() {
public List apply(WebDriver driver) {
return driver.findElements(By.className("dynamicElements"));
}
});
for (WebElement element : elements) {
element.click();
}
This example waits for a list of elements with the class dynamicElements
to be present, then interacts with each element individually.
8. Best Practices for Fluent Waits
- Use with Dynamic Elements: Fluent Waits are ideal for handling elements that load dynamically or change states frequently.
- Set Reasonable Timeout and Polling Intervals: Ensure that the maximum wait time and polling frequency are optimized for your application to avoid unnecessary delays.
- Ignore Specific Exceptions: Use the
ignoring
method to avoid exceptions that may occur while waiting for elements to become available. - Use for Complex Wait Scenarios: If your application has complex dynamic behaviors, Fluent Waits offer more flexibility than simple implicit or explicit waits.
9. Conclusion
Fluent Waits offer a highly customizable waiting mechanism that can be particularly useful for handling dynamic elements in web applications. By specifying the polling interval and ignoring certain exceptions, Fluent Waits allow you to fine-tune your automation scripts for reliability and efficiency. They are especially useful in scenarios where elements appear or disappear unpredictably, allowing your scripts to interact with them at the right time.
Best Practices for Managing Timing Issues
In web automation, timing issues are one of the most common challenges that can lead to unreliable test results. These issues typically arise when elements take longer than expected to load or become interactive. Managing these timing issues effectively is crucial for creating stable and efficient automation scripts. This section covers best practices for handling timing issues in Selenium automation.
1. Understanding Timing Issues
Timing issues occur when Selenium tries to interact with an element before it is available on the page. This can happen because of slow page loads, delayed AJAX requests, or animations. If the element is not yet loaded or ready for interaction, Selenium might throw exceptions like:
NoSuchElementException
: The element was not found.ElementNotInteractableException
: The element was found, but it was not interactable (e.g., not visible or disabled).TimeoutException
: The operation timed out before the condition was satisfied.
2. Use Implicit Waits Wisely
Implicit waits are a simple way to handle timing issues by instructing Selenium to wait for a specified amount of time when trying to find an element. However, they are not ideal for every situation, as they apply globally to all elements and can introduce unnecessary delays.
- When to Use: Implicit waits are best suited for situations where you want to set a default wait time for finding elements, especially in applications with consistent load times.
- Best Practice: Keep the wait time reasonable (e.g., 10–15 seconds). Too long of an implicit wait can slow down your tests.
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
3. Leverage Explicit Waits for Specific Conditions
Explicit waits allow you to define specific conditions to wait for, giving you more control over your script. You can wait for elements to be visible, clickable, or have any other state that your script depends on before interacting with them. This is much more efficient than using implicit waits.
- When to Use: Use explicit waits when you need to wait for specific conditions, such as an element becoming visible or clickable.
- Best Practice: Always use
WebDriverWait
withExpectedConditions
to avoid unnecessary waits and improve test reliability.
WebDriverWait wait = new WebDriverWait(driver, 20);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
4. Combine Explicit Waits with Fluent Waits for Dynamic Elements
Fluent waits provide more flexibility than explicit waits by allowing you to define how often the condition should be checked and which exceptions to ignore. This is especially useful for handling dynamic elements that may not be immediately available or may change frequently.
- When to Use: Fluent waits are ideal for scenarios where elements appear or change after a delay, such as in AJAX-heavy applications or when interacting with popups.
- Best Practice: Use Fluent Waits with a polling interval to check for elements at regular intervals, ensuring that the element becomes available without waiting unnecessarily long.
FluentWait wait = new FluentWait(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(new Function() {
public WebElement apply(WebDriver driver) {
return driver.findElement(By.id("dynamicElement"));
}
});
5. Use JavaScript Executor for Complex Scenarios
In some cases, standard waits may not be sufficient to handle timing issues, especially for actions like scrolling, waiting for animations, or handling elements that are loaded asynchronously using JavaScript. In such cases, you can use JavaScript Executor to execute JavaScript commands directly in the browser.
- When to Use: Use JavaScript Executor for complex scenarios like waiting for page load, scrolling elements into view, or interacting with elements that require JavaScript execution.
- Best Practice: Always use JavaScript Executor cautiously, as it can bypass the standard Selenium interactions and may lead to less stable tests if overused.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0,500);"); // Scroll down by 500px
6. Handle Page Load Timing with PageLoadStrategy
Page load timing is another common issue in Selenium tests. By default, Selenium waits for the entire page to load before continuing. However, in some cases, waiting for the full page to load might not be necessary. You can configure the page load strategy to optimize wait times.
- When to Use: Use page load strategies to control how Selenium waits for page loads. For example, you might want to wait until the DOM is loaded but not necessarily wait for all resources (like images) to finish loading.
- Best Practice: Use the
PageLoadStrategy
property to configure the page load behavior based on your test needs.
ChromeOptions options = new ChromeOptions();
options.setPageLoadStrategy(PageLoadStrategy.EAGER); // Wait until DOM is loaded
WebDriver driver = new ChromeDriver(options);
7. Monitor and Log Timing Issues
Logging timing issues can help you analyze and diagnose problems during test execution. By logging the timestamps of important events or steps, you can identify where delays are occurring in your automation scripts.
- When to Use: Log timing issues when you notice intermittent failures or unpredictable delays during test execution.
- Best Practice: Use logging frameworks (e.g.,
Log4j
,SLF4J
) to capture and analyze timing-related issues efficiently.
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
Logger logger = LogManager.getLogger(YourClass.class);
long startTime = System.currentTimeMillis();
// Perform some action
long endTime = System.currentTimeMillis();
logger.info("Time taken: " + (endTime - startTime) + " ms");
8. Best Practices for Managing Timing Issues
- Use Explicit Waits for Critical Elements: Explicit waits are more effective than implicit waits when you need to wait for specific conditions (e.g., visibility, clickability).
- Optimize Wait Times: Avoid waiting for unnecessarily long periods. Use the shortest time that works for your application.
- Use JavaScript Executor for Complex Interactions: For actions that require JavaScript execution (e.g., scrolling, handling dynamic content), use JavaScript Executor.
- Consider Page Load Strategies: Adjust the page load strategy to optimize the time Selenium waits for page loads based on your needs.
- Log and Monitor Timing: Use logging to capture and monitor timing issues to diagnose and optimize your tests.
9. Conclusion
Timing issues are a common challenge in Selenium automation, but by following these best practices, you can ensure that your tests are stable and reliable. By using the right combination of waits, monitoring page load strategies, and handling dynamic content efficiently, you can significantly reduce the impact of timing issues in your Selenium tests.
Handling JavaScript Alerts (Accept, Dismiss, Get Text)
JavaScript alerts, confirms, and prompts are common in web applications to notify users or ask for their input. Selenium provides straightforward methods to interact with these pop-ups. This section explains how to handle JavaScript alerts, including accepting, dismissing, and retrieving text from alerts.
1. Understanding JavaScript Alerts
JavaScript alerts are pop-up windows that appear on the browser, usually to convey information or ask the user for confirmation. Selenium allows you to interact with these alerts through the Alert
interface. There are three types of JavaScript pop-ups:
- Alert: A simple message box with an OK button.
- Confirm: A pop-up that asks for confirmation with OK and Cancel buttons.
- Prompt: A pop-up that asks for user input with a text field and OK/Cancel buttons.
2. Handling Alert Boxes (Accept)
Alert boxes are the simplest type of JavaScript pop-up. Selenium provides the accept()
method to click the OK button on the alert box.
- When to Use: Use this method when you want to close an alert by accepting it (clicking the OK button).
- Best Practice: Always handle alerts before interacting with any other elements, as they may block the page and cause test failures.
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Trigger an alert (for demonstration)
driver.findElement(By.id("alertButton")).click();
// Switch to the alert
Alert alert = driver.switchTo().alert();
// Accept the alert
alert.accept();
driver.quit();
3. Handling Confirm Boxes (Dismiss)
Confirm boxes are similar to alert boxes, but they have two buttons: OK and Cancel. Use the dismiss()
method to click the Cancel button.
- When to Use: Use
dismiss()
when you want to cancel the action triggered by the pop-up, such as closing a confirmation dialog without accepting it. - Best Practice: Always check the context of the confirm dialog. If you are testing user interactions, ensure that both positive and negative outcomes are tested.
driver.get("https://example.com");
// Trigger a confirm box (for demonstration)
driver.findElement(By.id("confirmButton")).click();
// Switch to the confirm box
Alert confirmAlert = driver.switchTo().alert();
// Dismiss the confirm box (click Cancel)
confirmAlert.dismiss();
driver.quit();
4. Retrieving Text from Alerts (Get Text)
Sometimes, you may need to retrieve the message or text from an alert to verify its content. The getText()
method allows you to capture the message displayed in the alert.
- When to Use: Use
getText()
to capture the message from an alert or confirm box for validation or verification during testing. - Best Practice: Always assert the alert message to verify the correctness of the alert content in your tests.
driver.get("https://example.com");
// Trigger an alert (for demonstration)
driver.findElement(By.id("alertButton")).click();
// Switch to the alert
Alert alert = driver.switchTo().alert();
// Get the alert text
String alertText = alert.getText();
System.out.println("Alert Text: " + alertText);
// Accept the alert
alert.accept();
driver.quit();
5. Handling Prompt Boxes (Send Keys)
Prompt boxes are a type of alert where the user is asked to provide input. You can use the sendKeys()
method to enter text into a prompt and then accept or dismiss it as needed.
- When to Use: Use
sendKeys()
to provide input to a prompt box before accepting or dismissing it. - Best Practice: Always validate the input value to ensure your test is interacting with the prompt correctly.
driver.get("https://example.com");
// Trigger a prompt box (for demonstration)
driver.findElement(By.id("promptButton")).click();
// Switch to the prompt box
Alert promptAlert = driver.switchTo().alert();
// Enter text into the prompt
promptAlert.sendKeys("Test input");
// Accept the prompt
promptAlert.accept();
driver.quit();
6. Handling Multiple Alerts
When dealing with multiple alerts or pop-ups, you can switch between them using switchTo().alert()
each time. Be sure to handle each alert in sequence to avoid missing any important interactions.
- Best Practice: For complex workflows, ensure each alert is handled in the correct order and that your test waits appropriately for each pop-up.
driver.get("https://example.com");
// Trigger the first alert
driver.findElement(By.id("alertButton1")).click();
Alert alert1 = driver.switchTo().alert();
alert1.accept();
// Trigger the second alert
driver.findElement(By.id("alertButton2")).click();
Alert alert2 = driver.switchTo().alert();
alert2.accept();
driver.quit();
7. Best Practices for Handling Alerts in Selenium
- Always Wait for Alerts: Use explicit waits to ensure that the alert is present before trying to interact with it, preventing timing issues.
- Use Contextual Handling: For different types of alerts (alert, confirm, prompt), use the correct method to interact with them (e.g.,
accept()
,dismiss()
,sendKeys()
). - Test for Correct Alert Text: Always validate the text of the alert to ensure the correct message is displayed.
- Handle Multiple Alerts Sequentially: If multiple alerts appear, handle each in sequence to avoid missing any alert.
8. Conclusion
Handling JavaScript alerts is essential for automating tasks on web applications that use pop-ups for notifications, confirmations, or user input. By using the appropriate methods to accept, dismiss, and retrieve text from alerts, you can ensure that your tests run smoothly and reliably. Always follow best practices to avoid common issues such as timing problems or unhandled pop-ups.
Automating Browser Pop-Ups and Modal Dialogs
Browser pop-ups and modal dialogs are commonly used in web applications for displaying messages, capturing user input, or confirming actions. Selenium provides a way to handle these types of pop-ups and modals effectively. This section explains how to automate interactions with both browser pop-ups and modal dialogs, focusing on the differences and approaches for each.
1. Understanding Browser Pop-Ups and Modal Dialogs
Pop-ups and modal dialogs are windows that appear over the current page to capture user interaction. Pop-ups are typically browser-generated windows (e.g., alerts, confirms, prompts), while modal dialogs are part of the webpage itself, often triggered by JavaScript or user actions. Here’s a breakdown:
- Browser Pop-Ups: These are external windows generated by the browser (e.g., alerts, confirms, prompts). They require switching to the pop-up window to interact with it.
- Modal Dialogs: These are usually part of the web page and appear as overlaid content (e.g., login forms, notifications, or confirmation boxes). They don’t require switching windows but interacting with the HTML elements inside the modal.
2. Automating Browser Pop-Ups
Browser pop-ups are often handled using the Alert
interface in Selenium. These alerts can be of various types, such as alert boxes, confirm boxes, and prompt boxes. Selenium provides methods to accept, dismiss, or retrieve text from these pop-ups.
Steps to Handle Browser Pop-Ups:
- Switch to the alert using
switchTo().alert()
. - Interact with the alert using methods like
accept()
,dismiss()
, orgetText()
. - For prompt alerts, use
sendKeys()
to provide input before accepting or dismissing.
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Trigger an alert (for demonstration)
driver.findElement(By.id("alertButton")).click();
// Switch to the alert
Alert alert = driver.switchTo().alert();
// Accept the alert
alert.accept();
driver.quit();
3. Automating Modal Dialogs
Modal dialogs are part of the webpage and typically require interacting with elements inside the modal. These modals can include forms, confirmation messages, or custom HTML elements that need to be handled directly through Selenium WebDriver interactions.
- When to Use: Modal dialogs are used when you want to automate interactions with elements inside a modal, such as filling out forms or clicking buttons.
- Best Practice: Always wait for the modal dialog to be visible before interacting with it to avoid timing issues.
Steps to Handle Modal Dialogs:
- Locate the modal using appropriate locators (e.g.,
By.id
,By.className
, orBy.cssSelector
). - Interact with elements inside the modal (e.g., input fields, buttons) using WebDriver methods like
sendKeys()
andclick()
. - If necessary, close the modal by interacting with the close button or using
Esc
key events.
driver.get("https://example.com");
// Trigger a modal (for demonstration)
driver.findElement(By.id("modalButton")).click();
// Wait for the modal to be visible
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("modal")));
// Interact with elements inside the modal
driver.findElement(By.id("inputField")).sendKeys("Test input");
driver.findElement(By.id("submitButton")).click();
// Close the modal (if a close button exists)
driver.findElement(By.id("closeModalButton")).click();
driver.quit();
4. Handling Modal Dialogs with Dynamic Content
Some modal dialogs may load content dynamically or change based on user interactions. In such cases, it’s important to wait for the modal content to load before interacting with it. You can use explicit waits to handle these scenarios effectively.
- When to Use: Use explicit waits if the modal content is loaded asynchronously (e.g., after an AJAX call).
- Best Practice: Use
WebDriverWait
andExpectedConditions
to wait for elements inside the modal to be available before interacting with them.
driver.get("https://example.com");
// Trigger a dynamic modal (for demonstration)
driver.findElement(By.id("dynamicModalButton")).click();
// Wait for the modal content to load
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicModalContent")));
// Interact with the modal content
driver.findElement(By.id("inputField")).sendKeys("Dynamic content test");
driver.findElement(By.id("submitButton")).click();
driver.quit();
5. Switching Between Multiple Pop-Ups and Modals
If there are multiple pop-ups or modal dialogs, you can switch between them using WebDriver’s switchTo().window()
method for browser windows or switchTo().alert()
for alerts. For modals, locate each modal individually and interact with them as needed.
driver.get("https://example.com");
// Trigger multiple modals or pop-ups (for demonstration)
driver.findElement(By.id("firstModalButton")).click();
driver.findElement(By.id("secondModalButton")).click();
// Switch between modals or alerts and interact
driver.findElement(By.id("firstModalInput")).sendKeys("First modal");
driver.findElement(By.id("secondModalInput")).sendKeys("Second modal");
driver.quit();
6. Best Practices for Automating Browser Pop-Ups and Modal Dialogs
- Wait for Modals or Pop-Ups: Always wait for the pop-up or modal to be visible before interacting with it to avoid timing issues and ensure your tests are reliable.
- Handle Modals Sequentially: If there are multiple modals or pop-ups, handle them in the order in which they appear to avoid missing any interaction.
- Close Modals Properly: If necessary, close modals by clicking the close button or using keyboard actions (e.g.,
Esc
key). - Test for Modal Visibility: Always assert that a modal or pop-up is visible before interacting with its content to ensure proper synchronization.
7. Conclusion
Automating interactions with browser pop-ups and modal dialogs is an essential part of web application testing. By understanding the differences between browser pop-ups and modal dialogs and using the appropriate Selenium methods to handle them, you can ensure that your tests are effective and accurate. Always incorporate best practices, such as waiting for elements to be visible and handling multiple pop-ups sequentially, to avoid common issues in test automation.
File Upload Dialogs and Authentication Pop-Ups
File upload dialogs and authentication pop-ups are common in web applications and often require special handling in test automation scripts. Selenium provides ways to interact with these elements, though there are some challenges because of the nature of the dialogs and their interaction with the operating system. In this section, we'll explore how to automate file uploads and interact with authentication pop-ups using Selenium.
1. Understanding File Upload Dialogs
File upload dialogs are prompted when a user selects a file input field (e.g., <input type="file">
) on a webpage. These dialogs are part of the operating system and cannot be directly interacted with using Selenium. However, there are various approaches to automate file uploads in Selenium:
- Using the File Input Field: The most common approach is to interact with the file input field directly by sending the file path to it.
- Using AutoIT or Robot Class: For complex file upload dialogs (e.g., native OS file dialogs), tools like AutoIT (Windows) or the Robot class (Java) can simulate keypresses and mouse events.
2. Automating File Uploads Using Selenium
The easiest way to automate file uploads with Selenium is by interacting with the <input type="file">
element directly. You can send the file path using the sendKeys()
method to upload a file without opening the native file dialog.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/upload");
WebElement uploadElement = driver.findElement(By.id("uploadFileInput"));
uploadElement.sendKeys("C:\\path\\to\\file.txt");
// Submit the form (if necessary)
driver.findElement(By.id("submitButton")).click();
driver.quit();
In this example, the file path is provided directly to the file input field, allowing the file to be uploaded automatically.
3. Handling Authentication Pop-Ups
Authentication pop-ups are browser-level pop-ups that prompt users for credentials (username and password) when accessing protected resources. Selenium does not have direct support for interacting with these pop-ups because they are outside the HTML DOM and are generated by the browser itself.
There are a few methods to handle authentication pop-ups in Selenium:
- Basic Authentication in the URL: One way to handle authentication pop-ups is by passing the credentials directly in the URL in the format
http://username:password@domain.com
. - Using AutoIT or Robot Class: Another approach is to use AutoIT or the Robot class to simulate keyboard input to enter the username and password.
- Browser Options (for Chrome and Firefox): You can configure the browser to automatically bypass authentication pop-ups using browser capabilities.
4. Automating Authentication Pop-Ups Using URL
If the website uses basic HTTP authentication, you can bypass the authentication pop-up by embedding the credentials directly into the URL. This approach works for both HTTP and HTTPS sites:
WebDriver driver = new ChromeDriver();
driver.get("https://username:password@yourdomain.com");
driver.quit();
This method works well for basic authentication but will not work for other types of authentication pop-ups, such as those implemented with JavaScript or custom authentication forms.
5. Using AutoIT or Robot Class for Authentication Pop-Ups
If the authentication pop-up is not a basic authentication dialog but a browser-generated window, you can use external tools like AutoIT (for Windows) or Java's Robot class to simulate user input. These tools allow you to interact with the native pop-up dialog and enter the username and password programmatically.
Example using Robot Class in Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import java.awt.Robot;
import java.awt.event.KeyEvent;
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Trigger the authentication pop-up
driver.get("https://example.com/protected");
// Use Robot class to input credentials into the pop-up
Robot robot = new Robot();
robot.keyPress(KeyEvent.VK_TAB); // Focus on username field
robot.keyRelease(KeyEvent.VK_TAB);
robot.keyPress(KeyEvent.VK_A); // Type username
robot.keyRelease(KeyEvent.VK_A);
robot.keyPress(KeyEvent.VK_C); // Type password
robot.keyRelease(KeyEvent.VK_C);
robot.keyPress(KeyEvent.VK_ENTER); // Submit
robot.keyRelease(KeyEvent.VK_ENTER);
driver.quit();
With this example, the Robot class simulates key presses to enter the username and password and then submits the login form.
6. Using Browser Options to Handle Authentication
For browsers like Chrome and Firefox, you can configure the browser to bypass authentication dialogs by setting the credentials in the browser's options or preferences. This method is helpful for automating tests that involve basic authentication.
Example for Chrome:
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
ChromeOptions options = new ChromeOptions();
options.addArguments("user-agent=Mozilla/5.0");
options.addArguments("headless");
// Set up the credentials in the URL
String credentials = "username:password";
String url = "https://example.com/protected";
WebDriver driver = new ChromeDriver(options);
driver.get("http://" + credentials + "@" + url);
driver.quit();
7. Best Practices for Handling File Uploads and Authentication Pop-Ups
- Use Direct Input for File Uploads: Always try to interact with the file upload input field directly using
sendKeys()
for simplicity and reliability. - Use External Tools for Complex Dialogs: For complex file dialogs or authentication pop-ups, use tools like AutoIT or the Robot class to simulate user actions.
- Handle Authentication Pop-Ups Securely: Avoid hardcoding credentials in the test scripts. Use environment variables or secure storage for sensitive information.
- Test Across Different Browsers: Make sure to test your automation scripts across different browsers since handling authentication pop-ups may differ.
8. Conclusion
File upload dialogs and authentication pop-ups are common but tricky elements to automate. By leveraging the appropriate methods for interacting with file input fields, using external tools for native OS dialogs, and handling authentication pop-ups effectively, you can ensure that your Selenium automation scripts are robust and reliable. Always follow best practices for managing sensitive data and interacting with browser-specific pop-ups to make your tests more efficient and secure.
Switching Between Windows and Frames
In Selenium, switching between different windows and frames is a crucial part of automating interactions with web applications that involve multiple browser windows or iframes. Selenium provides various methods to handle and switch between windows and frames. This section will guide you through the process of switching between windows and frames in Selenium.
1. Understanding Windows and Frames
In web applications, you may encounter multiple windows or frames. These could be pop-up windows, new tabs, or embedded elements within a web page (iframes). Selenium allows you to switch between these elements to interact with them. Understanding how to handle these scenarios is essential for effective test automation.
2. Switching Between Browser Windows
When a new window or tab opens, Selenium provides methods to switch between the currently active window and other open windows. You can interact with the windows by switching to their respective window handles.
Window Handle: Each open window or tab has a unique identifier called a window handle. Selenium provides methods to get the window handle and switch to a different one.
Steps to Switch Between Windows:
- Get the window handle of the current window using
getWindowHandle()
. - Get the handles of all open windows using
getWindowHandles()
. - Switch to a window handle using
switchTo().window()
.
3. Example: Switching Between Windows (Java)
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.Set;
WebDriver driver = new ChromeDriver();
// Open the main page
driver.get("https://example.com");
// Click a link that opens a new window
driver.findElement(By.linkText("Open New Window")).click();
// Get the window handles
Set windowHandles = driver.getWindowHandles();
String mainWindow = driver.getWindowHandle(); // Store main window handle
// Switch to the new window
for (String handle : windowHandles) {
if (!handle.equals(mainWindow)) {
driver.switchTo().window(handle);
break;
}
}
// Perform actions in the new window
WebElement newWindowElement = driver.findElement(By.id("newWindowElement"));
newWindowElement.click();
// Switch back to the main window
driver.switchTo().window(mainWindow);
driver.quit();
In the above example, we open a new window, switch to it, perform an action, and then switch back to the main window.
4. Switching Between Frames (iFrames)
Frames (or iframes) are HTML elements used to embed another document within the current web page. Selenium provides methods to switch between frames to interact with elements inside them.
Steps to Switch Between Frames:
- Switch to a frame using
switchTo().frame()
and specify the frame by its index, name, or WebElement. - Once done with the frame, switch back to the main content using
switchTo().defaultContent()
.
5. Example: Switching Between Frames (Java)
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/iframePage");
// Switch to the frame by index
driver.switchTo().frame(0);
// Interact with elements inside the frame
WebElement frameElement = driver.findElement(By.id("frameElement"));
frameElement.click();
// Switch back to the main content
driver.switchTo().defaultContent();
driver.quit();
In this example, we switch to an iframe by its index and interact with an element inside the frame. Afterward, we switch back to the main content.
6. Switching Between Nested Frames
When frames are nested within other frames, you need to switch to the parent frame first and then to the nested frame. You can achieve this by using multiple switchTo().frame()
calls.
Example: Switching Between Nested Frames (Java)
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/nestedFrames");
// Switch to the first parent frame
driver.switchTo().frame("parentFrame");
// Switch to the child frame
driver.switchTo().frame("childFrame");
// Interact with elements in the nested frame
WebElement nestedElement = driver.findElement(By.id("nestedElement"));
nestedElement.click();
// Switch back to the main content
driver.switchTo().defaultContent();
driver.quit();
In this example, we switch to a parent frame, then to a nested child frame, interact with an element, and return to the main content.
7. Switching to Frames Using WebElement
Instead of using an index or name to switch to a frame, you can also switch using a WebElement. This is useful when the frame is identified dynamically.
Example: Switching to a Frame Using WebElement (Java)
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/iframePage");
WebElement frameElement = driver.findElement(By.id("iframeId"));
driver.switchTo().frame(frameElement);
// Interact with elements inside the frame
WebElement frameElementInside = driver.findElement(By.id("elementInsideFrame"));
frameElementInside.click();
// Switch back to the main content
driver.switchTo().defaultContent();
driver.quit();
Here, we locate the iframe element and switch to it using switchTo().frame()
with a WebElement reference.
8. Best Practices for Switching Between Windows and Frames
- Always store the main window handle: Before switching to a new window, store the main window handle so you can easily return to it later.
- Switch back to the main content: After interacting with elements inside an iframe, make sure to switch back to the main content using
switchTo().defaultContent()
. - Handle multiple windows carefully: When switching between multiple windows, make sure to handle them in the correct order, especially when the windows are opened in quick succession.
- Use indexes cautiously for frames: When switching to frames by index, ensure the index corresponds to the correct frame, as the frame order may change.
9. Conclusion
Switching between windows and frames is an essential skill in Selenium test automation. By understanding how to use window handles and frame switching methods, you can interact with multiple windows and embedded documents effectively. Whether dealing with simple windows or nested frames, these techniques are key to automating complex web applications.
Automating Mouse Actions (Hover, Drag and Drop, Right Click)
In Selenium, automating mouse actions like hovering over elements, performing drag-and-drop operations, and right-clicking (context menu) are common tasks for interacting with dynamic web elements. These actions can be easily handled using the Actions
class in Selenium. This section will demonstrate how to automate various mouse actions in Selenium.
1. The Actions Class in Selenium
The Actions
class in Selenium provides various methods to simulate mouse actions, such as moving the mouse, clicking, dragging, and more. It is essential for handling complex user interactions like hover, drag-and-drop, and right-click actions.
Example: Creating an Actions Object
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Your actions go here
driver.quit();
In the above code, we first create an instance of the Actions
class to perform mouse actions.
2. Hovering Over an Element (Mouse Hover)
Hovering the mouse over an element (e.g., showing a dropdown menu on hover) is done using the moveToElement()
method of the Actions
class. This simulates the action of moving the mouse to a specific element on the page.
Example: Hovering Over an Element (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Find the element to hover over
WebElement hoverElement = driver.findElement(By.id("hoverButton"));
// Perform hover action
actions.moveToElement(hoverElement).perform();
driver.quit();
In this example, we hover over an element identified by its ID. The perform()
method executes the action.
3. Performing Drag and Drop
Drag and drop actions are common in web applications, such as rearranging items in a list or moving elements between containers. You can perform drag-and-drop operations using the dragAndDrop()
method of the Actions
class.
Example: Dragging and Dropping an Element (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/dragAndDrop");
// Find the element to drag
WebElement sourceElement = driver.findElement(By.id("dragElement"));
// Find the target element
WebElement targetElement = driver.findElement(By.id("dropTarget"));
// Perform the drag and drop action
actions.dragAndDrop(sourceElement, targetElement).perform();
driver.quit();
In this example, we drag an element identified by its ID and drop it onto another target element. The dragAndDrop()
method makes it easy to automate such interactions.
4. Right-Clicking (Context Menu)
Right-clicking on an element (context menu) is another important action. You can simulate a right-click using the contextClick()
method of the Actions
class.
Example: Right Clicking on an Element (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/contextMenu");
// Find the element to right-click on
WebElement rightClickElement = driver.findElement(By.id("rightClickElement"));
// Perform the right-click action (context click)
actions.contextClick(rightClickElement).perform();
driver.quit();
In this example, we right-click on an element to trigger the context menu. The contextClick()
method simulates the right-click action.
5. Combining Multiple Actions
Sometimes, you may need to perform multiple mouse actions in sequence. You can chain actions together using the Actions
class. Once all actions are chained, the perform()
method executes them in sequence.
Example: Combining Hover and Click (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Find the element to hover over
WebElement hoverElement = driver.findElement(By.id("hoverButton"));
// Find the element to click after hover
WebElement clickElement = driver.findElement(By.id("clickButton"));
// Perform hover and click in sequence
actions.moveToElement(hoverElement).moveToElement(clickElement).click().perform();
driver.quit();
In this example, we hover over one element and then click on another element. The actions are chained together and executed in order.
6. Other Mouse Actions in Selenium
- Double Click: Use
doubleClick()
to perform a double-click on an element. - Click and Hold: Use
clickAndHold()
to simulate holding down the mouse button on an element. - Release: Use
release()
to release a mouse button after clicking and holding.
Example: Double Click (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/doubleClick");
// Find the element to double-click
WebElement doubleClickElement = driver.findElement(By.id("doubleClickButton"));
// Perform double click
actions.doubleClick(doubleClickElement).perform();
driver.quit();
Example: Click and Hold (Java)
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/clickAndHold");
// Find the element to click and hold
WebElement clickAndHoldElement = driver.findElement(By.id("dragElement"));
// Perform click and hold
actions.clickAndHold(clickAndHoldElement).perform();
// Release the mouse button
actions.release().perform();
driver.quit();
7. Best Practices for Automating Mouse Actions
- Use
moveToElement()
cautiously: When hovering over elements, make sure that the element is visible and not covered by other elements. - Handle drag-and-drop carefully: Ensure that the source and target elements are interactable during drag-and-drop operations.
- Test in multiple browsers: Different browsers may have slight differences in handling mouse actions, so it’s essential to test across multiple browsers.
- Use explicit waits: Ensure that elements are ready for interaction before performing mouse actions, especially in dynamic web pages.
8. Conclusion
Automating mouse actions such as hover, drag-and-drop, and right-clicking is a critical part of interacting with dynamic web elements. Selenium provides the Actions
class to handle these actions seamlessly. By using the methods provided by the Actions
class, you can simulate complex user interactions and automate tasks efficiently.
Working with the Actions Class
The Actions
class in Selenium provides a way to perform complex user interactions, such as mouse movements, keyboard actions, drag and drop, hover, and more. It allows you to chain multiple actions together to simulate real-world user behavior in a web application.
1. Introduction to the Actions Class
The Actions
class is part of the Selenium WebDriver library, and it is used to perform advanced user actions. This class provides methods for handling mouse actions, keyboard input, and other complex interactions.
Example: Creating an Actions Object
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Your actions go here
driver.quit();
In the above example, we create an instance of the Actions
class, which can then be used to perform multiple actions on web elements.
2. Common Actions Using the Actions Class
Here are some of the most commonly used methods of the Actions
class:
- moveToElement(): Moves the mouse pointer to a specific element on the page.
- click(): Clicks on an element.
- doubleClick(): Double-clicks on an element.
- contextClick(): Right-clicks (context menu) on an element.
- clickAndHold(): Clicks and holds the mouse on an element.
- release(): Releases the mouse button after clicking and holding.
- dragAndDrop(): Drags an element and drops it onto another element.
- sendKeys(): Sends keyboard input to an element.
3. Example: Moving the Mouse to an Element (Hover)
One of the most common actions is moving the mouse to an element (i.e., hovering over an element). The following example demonstrates how to perform a hover action:
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Find the element to hover over
WebElement hoverElement = driver.findElement(By.id("hoverButton"));
// Perform hover action
actions.moveToElement(hoverElement).perform();
driver.quit();
In this example, we use the moveToElement()
method to hover over a button (identified by its ID). The perform()
method triggers the action.
4. Example: Clicking an Element
Clicking on an element is a fundamental action. Below is an example of clicking on a button using the click()
method:
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Find the element to click
WebElement clickElement = driver.findElement(By.id("clickButton"));
// Perform the click action
actions.click(clickElement).perform();
driver.quit();
In this case, we find the element with the ID clickButton
and click on it using the click()
method.
5. Example: Drag and Drop
Drag-and-drop actions allow users to move elements from one location to another. Below is an example of how to perform a drag-and-drop operation using the dragAndDrop()
method:
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/dragAndDrop");
// Find the element to drag
WebElement sourceElement = driver.findElement(By.id("dragElement"));
// Find the target element
WebElement targetElement = driver.findElement(By.id("dropTarget"));
// Perform the drag and drop action
actions.dragAndDrop(sourceElement, targetElement).perform();
driver.quit();
The dragAndDrop()
method makes it simple to drag one element and drop it onto another.
6. Example: Right-Click (Context Menu)
Sometimes, you need to perform a right-click (context-click) action. This can be done using the contextClick()
method:
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/contextMenu");
// Find the element to right-click on
WebElement rightClickElement = driver.findElement(By.id("rightClickElement"));
// Perform right-click action
actions.contextClick(rightClickElement).perform();
driver.quit();
In this case, we right-click on an element identified by the ID rightClickElement
.
7. Chaining Multiple Actions Together
The Actions
class also allows you to chain multiple actions together. This is useful when you need to perform a sequence of actions in a single command.
Example: Hover and Click in Sequence
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com");
// Find elements to interact with
WebElement hoverElement = driver.findElement(By.id("hoverButton"));
WebElement clickElement = driver.findElement(By.id("clickButton"));
// Chain hover and click actions together
actions.moveToElement(hoverElement).moveToElement(clickElement).click().perform();
driver.quit();
In this example, we chain two actions: hover over one element and click another. The actions are executed sequentially when perform()
is called.
8. Keyboard Actions Using the Actions Class
In addition to mouse movements, you can also simulate keyboard actions such as typing, pressing keys, and releasing keys using the sendKeys()
method.
Example: Sending Keyboard Input
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/keyboardInput");
// Find the text box element
WebElement textBox = driver.findElement(By.id("textBox"));
// Send keyboard input (type text)
actions.sendKeys(textBox, "Hello, Selenium!").perform();
driver.quit();
In this example, we send the text "Hello, Selenium!" to a text box identified by its ID.
9. Best Practices for Using the Actions Class
- Use explicit waits: Ensure that elements are interactable before performing actions, especially for mouse movements and clicks.
- Chain actions: Use
Actions
chaining to create more complex and realistic user interactions. - Handle exceptions: Make sure to handle exceptions (e.g., element not found) when performing actions to avoid script failures.
- Use
moveToElement()
carefully: When hovering over elements, ensure that other elements don’t block the target element.
10. Conclusion
The Actions
class in Selenium is a powerful tool for simulating user interactions like mouse movements, clicks, and keyboard input. By using the various methods provided by the Actions
class, you can automate complex interactions on web applications and make your Selenium tests more robust and realistic.
Automating Keyboard Events (sendKeys and Key Actions)
In Selenium, automating keyboard events is an essential part of simulating user interaction with input fields, buttons, and other elements. Selenium provides the sendKeys()
method to send keystrokes to an element, and the Actions
class for more advanced keyboard actions like pressing and releasing specific keys.
1. Introduction to Keyboard Automation
Keyboard automation is helpful when interacting with elements such as text fields, password fields, search boxes, or even triggering key combinations (like Ctrl + C
or Ctrl + V
). Selenium provides two primary ways to simulate keyboard events:
- sendKeys(): Used for typing text directly into input fields and text areas.
- Key Actions (Actions class): Used for more complex keyboard actions such as pressing or releasing specific keys.
2. Using sendKeys() to Send Text Input
The sendKeys()
method is commonly used to type text into input fields. It simulates typing by sending keystrokes one by one to the element.
Example: Typing into a Text Box
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
// Find the username field
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys("myUsername");
// Find the password field
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys("myPassword");
driver.quit();
In this example, we send the username and password to their respective fields using sendKeys()
.
3. Using sendKeys() for Special Keys
In addition to regular text, you can also send special keys like Enter
, Tab
, Backspace
, Esc
, and others using the sendKeys()
method.
- Enter:
Keys.RETURN
orKeys.ENTER
- Tab:
Keys.TAB
- Backspace:
Keys.BACK_SPACE
- Escape:
Keys.ESCAPE
Example: Sending Special Keys
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
// Find the username field and type the username
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys("myUsername");
// Press the "Tab" key to move to the password field
usernameField.sendKeys(Keys.TAB);
// Type the password
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys("myPassword");
// Press "Enter" to submit the form
passwordField.sendKeys(Keys.RETURN);
driver.quit();
In this example, we use Keys.TAB
to move to the next field and Keys.RETURN
to submit the form.
4. Using the Actions Class for Key Actions
The Actions
class in Selenium allows you to perform more complex keyboard actions such as pressing and releasing specific keys. This is useful for actions like key combinations (Ctrl + A
, Ctrl + C
, etc.) or pressing keys in a specific order.
Example: Pressing a Key Combination (Ctrl + A)
import org.openqa.selenium.Keys;
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/textbox");
// Find the text box element
WebElement textBox = driver.findElement(By.id("textbox"));
// Press "Ctrl + A" to select all text
actions.keyDown(Keys.CONTROL).sendKeys("a").keyUp(Keys.CONTROL).perform();
driver.quit();
In this example, we use keyDown()
to simulate pressing the Ctrl
key, followed by the letter a
, and then keyUp()
to release the Ctrl
key.
Example: Pressing the "Shift" Key
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/shiftKey");
// Find the input field
WebElement inputField = driver.findElement(By.id("inputField"));
// Hold down the "Shift" key and type text
actions.keyDown(Keys.SHIFT).sendKeys("hello").keyUp(Keys.SHIFT).perform();
driver.quit();
This example shows how to simulate holding down the Shift
key while typing.
5. Handling Keyboard Events with KeyUp and KeyDown
In addition to sendKeys()
, the Actions
class allows you to simulate the pressing and releasing of keys individually using the keyDown()
and keyUp()
methods. These methods are especially useful for simulating key combinations.
Example: Holding and Releasing Keys
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
driver.get("https://example.com/keyboard");
// Find the text box element
WebElement textBox = driver.findElement(By.id("textbox"));
// Hold the "Shift" key, type "selenium", and release the "Shift" key
actions.keyDown(Keys.SHIFT).sendKeys("selenium").keyUp(Keys.SHIFT).perform();
driver.quit();
In this example, we simulate typing the word "selenium" with the "Shift" key held down, resulting in uppercase letters.
6. Best Practices for Automating Keyboard Events
- Use Explicit Waits: Always ensure the element is interactable before sending keystrokes.
- Avoid Hardcoding Keys: Use constants like
Keys.RETURN
orKeys.ENTER
instead of manually typing keys. - Chaining Actions: Use the
Actions
class to chain multiple key actions together for more complex scenarios. - Handle Special Keys: Make sure to correctly handle special keys like
Tab
orEnter
for smooth form submission and navigation.
7. Conclusion
Automating keyboard events in Selenium is a powerful way to simulate user interaction with web applications. Whether you're sending text with sendKeys()
or using the Actions
class for more advanced key combinations, mastering keyboard automation helps you create more realistic and effective automated tests.
Simulating Scrolling and Page Navigation
In Selenium, simulating scrolling and navigating through pages is essential for interacting with elements that are not in the visible area of the browser. This is especially useful for testing infinite scrolling, navigating through long pages, or ensuring elements are in view before interacting with them.
1. Introduction to Scrolling and Navigation
Web pages often contain more content than can be displayed on a single screen, so scrolling is necessary to access additional content. Selenium allows simulating scroll actions, either by scrolling to specific locations on the page or by using keyboard actions. Additionally, Selenium provides methods for navigating forward and backward through pages, mimicking real-world browsing behavior.
2. Scrolling Using JavaScript Executor
To simulate scrolling in a webpage, one of the most effective methods is to use JavaScript execution. Selenium’s JavascriptExecutor
interface allows you to execute JavaScript code directly in the browser.
Example: Scrolling to the Bottom of the Page
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Scroll to the bottom of the page using JavaScript Executor
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollTo(0, document.body.scrollHeight);");
driver.quit();
In this example, we use the window.scrollTo()
method to scroll to the bottom of the page.
Example: Scrolling to a Specific Element
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Find the element to scroll to
WebElement element = driver.findElement(By.id("targetElement"));
// Scroll to the specific element
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].scrollIntoView(true);", element);
driver.quit();
In this example, we use the scrollIntoView()
method to scroll to the specific element on the page.
3. Scrolling Using Keys (Page Up, Page Down)
Another way to simulate scrolling is by using the keyboard keys PAGE_DOWN
and PAGE_UP
. You can send these key events using the sendKeys()
method or the Actions
class.
Example: Scrolling Down Using PAGE_DOWN Key
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Use the PAGE_DOWN key to scroll down
WebElement body = driver.findElement(By.tagName("body"));
body.sendKeys(Keys.PAGE_DOWN);
driver.quit();
This example demonstrates how to simulate a scroll down by sending the PAGE_DOWN
key to the body of the webpage.
4. Scrolling by Pixel Value
You can also scroll by a specific number of pixels to control the scroll position. This can be done using the JavaScript Executor as well.
Example: Scrolling by Pixels
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Scroll down by 500 pixels
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0, 500);");
driver.quit();
In this example, the page scrolls down by 500 pixels vertically using the window.scrollBy()
method.
5. Navigating Forward and Backward
Selenium provides methods for simulating forward and backward navigation through pages, which is useful for testing web applications with multiple pages or ensuring proper history navigation.
Example: Navigating Backward and Forward
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Navigate to another page
driver.get("https://example.com/page2");
// Navigate backward to the previous page
driver.navigate().back();
// Navigate forward to the next page
driver.navigate().forward();
driver.quit();
In this example, we use navigate().back()
to go back to the previous page, and navigate().forward()
to go forward to the next page.
6. Refreshing the Page
Sometimes, you may need to refresh the page to simulate reloading or testing how the page behaves after a refresh. Selenium provides a simple method to reload the page.
Example: Refreshing the Page
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Refresh the page
driver.navigate().refresh();
driver.quit();
This example demonstrates how to refresh the current page using the navigate().refresh()
method.
7. Best Practices for Scrolling and Navigation
- Use JavaScript Executor Sparingly: While JavaScript-based scrolling is powerful, it can sometimes bypass normal browser behavior. Use this method when other options are not practical.
- Test on Real Devices: If possible, test scrolling and page navigation on real devices or real browsers to ensure compatibility.
- Use Page Navigation for Multi-Page Tests: Always test backward, forward, and refresh actions in web applications with multi-page navigation to ensure history works correctly.
- Consider Dynamic Content: For dynamic content or infinite scrolling, ensure you have mechanisms like waits to handle the loading of new content before interacting with it.
8. Conclusion
Simulating scrolling and page navigation is crucial for interacting with long web pages or testing multi-page web applications. By using JavaScript or keyboard keys, you can easily scroll through content or navigate through multiple pages, ensuring your automated tests reflect real user behavior.
Handling AJAX Calls in Selenium
AJAX (Asynchronous JavaScript and XML) calls are widely used in modern web applications to fetch data dynamically without refreshing the entire page. These calls can make testing more challenging because they may complete after the page has loaded, requiring Selenium to wait for the AJAX request to finish before interacting with the page.
1. What is AJAX?
AJAX enables web pages to send data to a web server and receive data from the server asynchronously, without refreshing the page. This allows dynamic content updates without disrupting the user experience. However, it can also cause issues for automation, as the page may appear to be fully loaded while AJAX requests are still processing in the background.
2. Challenges of Handling AJAX in Selenium
When automating with Selenium, one of the main challenges is to ensure that the AJAX request has completed before interacting with the elements that depend on it. If you attempt to interact with an element before the AJAX call finishes, it may lead to errors or unexpected behavior.
3. Ways to Handle AJAX Calls in Selenium
There are several strategies to handle AJAX calls in Selenium:
- Implicit Waits: Waits for a specified amount of time for an element to appear on the page. However, it may not always be suitable for AJAX calls.
- Explicit Waits: Waits for a specific condition to be true, like an element becoming visible or enabled. This is often the most reliable method when waiting for AJAX requests.
- Fluent Waits: A more flexible form of waiting that allows you to specify the frequency with which the condition is checked, and the maximum time to wait.
- JavaScript Executor: Use JavaScript to check if the AJAX call is complete by checking the status of the XMLHttpRequest object.
4. Using Explicit Waits for Handling AJAX
Explicit waits are highly effective when dealing with AJAX calls. Selenium’s WebDriverWait
class allows waiting until a specific condition is met (e.g., an element is visible, clickable, etc.). This is especially useful when AJAX calls load or update content on the page.
Example: Waiting for an Element to Be Visible After an AJAX Call
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/ajax-page");
// Wait for the element to be visible after AJAX call
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("ajaxElement")));
element.click();
driver.quit();
This example uses an explicit wait to wait for an element with the ID ajaxElement
to be visible after an AJAX call completes.
5. Using JavaScript Executor to Detect AJAX Completion
Another approach is to use JavaScript to determine if the AJAX request is still in progress. This can be done by checking the ready state of the XMLHttpRequest object.
Example: Using JavaScript Executor to Wait for AJAX Completion
JavascriptExecutor js = (JavascriptExecutor) driver;
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// Wait until AJAX call is completed
wait.until(driver -> ((JavascriptExecutor) driver).executeScript("return jQuery.active == 0"));
driver.quit();
This example checks if there are any active AJAX requests by using the jQuery.active
property. When the value is 0, it means there are no active AJAX requests.
6. Fluent Waits for AJAX Handling
Fluent waits are similar to explicit waits, but they allow more control over the polling frequency. Fluent waits are useful when you need to wait for an AJAX request while checking for certain conditions periodically.
Example: Using Fluent Wait to Handle AJAX
FluentWait fluentWait = new FluentWait(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
fluentWait.until(ExpectedConditions.visibilityOfElementLocated(By.id("ajaxElement")));
driver.quit();
In this example, a fluent wait checks every 500 milliseconds to see if the element with the ID ajaxElement
is visible, up to a maximum of 10 seconds.
7. Best Practices for Handling AJAX in Selenium
- Use Explicit Waits: Explicit waits are usually the most reliable and flexible solution for handling AJAX. Always prefer them over implicit waits when working with dynamic content.
- Ensure Correct Synchronization: Make sure your test scripts are synchronized with the page's state. Never assume an element is available before the AJAX call is completed.
- Handle Timeouts Gracefully: When using waits, always consider scenarios where the AJAX call takes longer than expected. Implement appropriate error handling for such cases.
- Optimize JavaScript Checks: If using JavaScript to track AJAX calls, ensure that the JavaScript code is optimized and runs efficiently, especially for dynamic websites with multiple AJAX requests.
8. Conclusion
Handling AJAX calls in Selenium can be tricky, but with the right techniques, such as explicit waits and JavaScript execution, you can effectively synchronize your tests with dynamic content. Always ensure that your tests wait for the necessary elements to load before interacting with them to avoid errors and flaky tests.
Waiting for Elements to Load Dynamically
In modern web applications, elements may load asynchronously or dynamically, especially when dealing with AJAX calls, JavaScript rendering, or partial page updates. Selenium provides several mechanisms to handle these dynamic elements, ensuring that tests wait for elements to be fully loaded before interacting with them.
1. What Does It Mean to Wait for Elements to Load Dynamically?
When interacting with web applications, dynamic elements are those that are not present at the initial page load but appear after some time due to actions like AJAX calls, JavaScript execution, or DOM updates. For example, data displayed after a user clicks a button or scrolls down the page. Handling such elements is crucial to prevent Selenium from interacting with elements that are not yet available, which could lead to errors or flaky tests.
2. Challenges of Waiting for Dynamic Elements
Dynamic elements often load at unpredictable times, depending on the network speed, server response, or user interaction. This unpredictability requires synchronization in your automation scripts to ensure that Selenium waits for elements to be ready before interacting with them. If an element is attempted to be accessed before it’s available, it can result in an exception, like NoSuchElementException
.
3. Solutions for Waiting for Dynamic Elements
There are several strategies to handle dynamically loaded elements in Selenium:
- Implicit Waits: Automatically applies a wait for all elements in the script. However, it is less flexible when dealing with specific dynamic elements.
- Explicit Waits: Allows for waiting for a specific condition (e.g., element visibility, element to be clickable) to be true before continuing with the test.
- Fluent Waits: A more advanced option that provides the ability to customize polling intervals and ignore specific exceptions during the wait process.
4. Using Explicit Waits for Dynamic Elements
Explicit waits are the most commonly used approach for waiting for dynamic elements. It allows you to wait for specific conditions, such as an element becoming visible, clickable, or present in the DOM, before performing an action on it.
Example: Waiting for an Element to Become Visible
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/dynamic-content");
// Wait for the element to become visible after dynamic loading
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
element.click();
driver.quit();
In this example, the script waits for an element with the ID dynamicElement
to become visible before clicking it. This ensures the element is ready for interaction after dynamic loading.
5. Using Fluent Waits for Dynamic Elements
Fluent waits provide more granular control over the wait process. You can specify the maximum wait time, polling interval, and which exceptions to ignore during the wait. Fluent waits are beneficial when dealing with frequent changes in dynamic content, such as elements that load intermittently or with delays.
Example: Using Fluent Wait to Wait for an Element
FluentWait fluentWait = new FluentWait(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
// Wait until the element is visible
fluentWait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
driver.quit();
In this example, the fluent wait checks every 500 milliseconds to see if the element with the ID dynamicElement
is visible, up to a maximum of 10 seconds.
6. Using JavaScript Executor to Wait for Dynamic Elements
Sometimes, dynamic elements may not directly trigger standard WebDriver waits, especially when the page uses custom JavaScript for content rendering. In such cases, you can use the JavaScript Executor to check if the element is available or if the page is ready.
Example: Using JavaScript Executor to Wait for Element Visibility
JavascriptExecutor js = (JavascriptExecutor) driver;
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// Wait until the element is visible using JavaScript
wait.until(driver -> ((JavascriptExecutor) driver)
.executeScript("return document.getElementById('dynamicElement') !== null"));
driver.quit();
This example uses JavaScript to check if an element with the ID dynamicElement
exists in the DOM and waits until it becomes available.
7. Best Practices for Waiting for Dynamic Elements
- Use Explicit Waits for Specific Conditions: Explicit waits are the most reliable method to wait for dynamic elements. Use them when waiting for conditions like visibility, presence, or clickability.
- Use Fluent Waits for Polling: Fluent waits are ideal for cases where you need to check the element multiple times with a custom interval. This is useful when working with elements that load intermittently.
- Avoid Overusing Implicit Waits: While implicit waits can be helpful, they may not be the best solution for waiting for dynamic elements. Explicit and fluent waits offer more control and reliability.
- Handle Exceptions Gracefully: Ensure that your scripts handle timeouts and exceptions gracefully, especially in the case of elements that might load slowly or intermittently.
- Check for Element State: Always ensure that the element you're interacting with is not only visible but also in an interactable state (e.g., not disabled or hidden).
8. Conclusion
Waiting for elements to load dynamically is a critical aspect of Selenium automation. By leveraging explicit waits, fluent waits, or JavaScript execution, you can ensure your tests are synchronized with the page's state and avoid errors related to dynamic content. Adopting best practices for handling dynamic elements will lead to more stable, reliable tests and improve the accuracy of your automation scripts.
Automating Infinite Scrolling Pages
Infinite scrolling is a popular design pattern used in modern web applications, where content loads dynamically as the user scrolls down the page. This presents a challenge for automation tools like Selenium, as the page does not have a fixed end or pagination element to interact with. Automating interactions with infinite scrolling pages requires techniques to simulate scrolling actions and wait for new content to load.
1. What is Infinite Scrolling?
Infinite scrolling is a user interface pattern where new content automatically loads and displays as the user scrolls down the page. It eliminates the need for pagination and provides a seamless browsing experience. However, for automated tests, it requires special handling as the content keeps loading dynamically when the user reaches the bottom of the page.
2. Challenges of Automating Infinite Scrolling
Automating interactions with infinite scrolling pages can be tricky because:
- There’s no predefined endpoint where the page ends, making it difficult to know when to stop scrolling.
- New content loads as the user scrolls, so the script must ensure that the page has finished loading before interacting with new elements.
- Traditional waiting techniques may not always work since content is continuously appended to the page.
3. Strategy for Automating Infinite Scrolling
To automate infinite scrolling pages effectively, you can simulate scroll actions and check for the appearance of new content after each scroll. The general approach is:
- Scroll down the page: Simulate user scrolling to the bottom of the page.
- Wait for new content: Wait for new elements or content to load after scrolling.
- Repeat scrolling: Continue scrolling until the desired amount of content is loaded or a certain condition is met (e.g., no new content appears).
4. Example: Automating Infinite Scrolling with Selenium
The following example demonstrates how to automate scrolling on a page with infinite scrolling using Selenium WebDriver in Java. The script will scroll down the page until all content is loaded or a maximum number of scrolls is reached.
Example: Scrolling to Load Content
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
import java.util.List;
public class InfiniteScrollAutomation {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/infinite-scroll");
JavascriptExecutor js = (JavascriptExecutor) driver;
int previousHeight = ((Long) js.executeScript("return document.body.scrollHeight")).intValue();
while (true) {
// Scroll to the bottom of the page
js.executeScript("window.scrollTo(0, document.body.scrollHeight);");
// Wait for new content to load
try {
Thread.sleep(2000); // Wait for 2 seconds for content to load
} catch (InterruptedException e) {
e.printStackTrace();
}
// Check the height of the page after scrolling
int newHeight = ((Long) js.executeScript("return document.body.scrollHeight")).intValue();
// If the height has not changed, we've reached the end of the content
if (newHeight == previousHeight) {
break;
}
previousHeight = newHeight;
}
// Interact with the loaded content
List items = driver.findElements(By.className("item"));
for (WebElement item : items) {
System.out.println(item.getText());
}
driver.quit();
}
}
In this example:
- The script scrolls to the bottom of the page using JavaScript.
- After each scroll, it waits for new content to load by checking the page height.
- If the page height does not change after scrolling, the script terminates, indicating that all content has been loaded.
5. Using WebDriverWait for Dynamic Content Loading
When automating infinite scrolling, it is important to wait for new content to load after each scroll. You can use WebDriverWait
along with appropriate expected conditions like visibilityOfAllElements
to wait for elements to appear before interacting with them.
Example: Using WebDriverWait to Wait for New Items
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.By;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.util.List;
public class InfiniteScrollWithWait {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/infinite-scroll");
JavascriptExecutor js = (JavascriptExecutor) driver;
WebDriverWait wait = new WebDriverWait(driver, 10);
int previousHeight = ((Long) js.executeScript("return document.body.scrollHeight")).intValue();
while (true) {
// Scroll to the bottom of the page
js.executeScript("window.scrollTo(0, document.body.scrollHeight);");
// Wait for new items to load
wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("item")));
// Check if new content has loaded
int newHeight = ((Long) js.executeScript("return document.body.scrollHeight")).intValue();
if (newHeight == previousHeight) {
break;
}
previousHeight = newHeight;
}
// Interact with the loaded items
List items = driver.findElements(By.className("item"));
for (WebElement item : items) {
System.out.println(item.getText());
}
driver.quit();
}
}
This example combines scrolling with a WebDriverWait
to wait for new elements to load after each scroll.
6. Best Practices for Automating Infinite Scrolling
- Use JavaScript for Scrolling: JavaScript is the most reliable method to simulate scrolling in Selenium, as it directly manipulates the browser’s scroll position.
- Handle Dynamic Content Waits: Always wait for elements to load after scrolling before interacting with them. Use WebDriverWait with appropriate conditions like visibility or presence of elements.
- Limit the Number of Scrolls: Avoid scrolling indefinitely. Set a maximum number of scrolls or stop when the content stops loading.
- Optimize Wait Times: Adjust wait times based on the speed of content loading. Shorter waits may be necessary for fast-loading pages, while longer waits may be needed for slower content loading.
- Monitor Performance: Infinite scrolling can significantly impact page performance. Consider testing the page load time and script execution time to ensure efficient automation.
7. Conclusion
Automating infinite scrolling pages requires simulating user scrolling actions and waiting for new content to load. By using JavaScript for scrolling, WebDriverWait for dynamic content, and best practices for efficient wait times, you can effectively automate testing on pages with infinite scrolling. This approach ensures that your tests wait for all content to load before interacting with elements, making your automation scripts more reliable and robust.
Interacting with Shadow DOM Elements
The Shadow DOM is a web standard that allows developers to encapsulate part of a webpage’s DOM tree, enabling the creation of reusable components with their own isolated scope. Elements inside the Shadow DOM are not accessible by conventional methods like standard CSS selectors or XPath. Interacting with Shadow DOM elements in Selenium requires special handling to penetrate the shadow boundary and interact with the encapsulated elements.
1. What is the Shadow DOM?
The Shadow DOM is a part of the Web Components specification, which allows developers to create custom, reusable components with encapsulated HTML, CSS, and JavaScript. The Shadow DOM provides style and behavior encapsulation, preventing external styles from affecting the component and vice versa. Web elements in the Shadow DOM are not directly accessible from the main DOM, making it challenging to interact with them using standard Selenium methods.
2. Challenges of Interacting with Shadow DOM Elements
Due to the isolation of the Shadow DOM, interacting with elements inside it requires special techniques:
- Not directly accessible: The elements inside the Shadow DOM are not part of the main DOM, making them inaccessible by standard locators like
By.id
,By.name
, or even XPath. - Multiple Shadow DOMs: A single page can contain multiple Shadow DOMs or nested Shadow DOMs, which increases the complexity of automation.
- Custom selectors: Standard CSS selectors and XPath won't work inside the Shadow DOM; Selenium requires a method to enter the Shadow DOM context.
3. Strategy for Interacting with Shadow DOM Elements
To interact with elements inside the Shadow DOM, you need to first access the shadow root and then interact with elements inside that context. The main strategy involves:
- Accessing the Shadow DOM: Use JavaScript execution to access the shadow root of an element that hosts the Shadow DOM.
- Finding elements inside the Shadow DOM: Once inside the shadow root, locate elements using standard selectors like
By.cssSelector
orBy.xpath
.
4. Example: Interacting with Shadow DOM Elements in Selenium (Java)
Below is an example that demonstrates how to interact with elements inside the Shadow DOM using Java and Selenium. The example shows how to access the shadow root and interact with a button inside the shadow DOM.
Example: Accessing and Clicking a Shadow DOM Button
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
public class ShadowDomAutomation {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Locate the shadow host element
WebElement shadowHost = driver.findElement(By.cssSelector("#shadow-host"));
// Access the shadow root using JavaScriptExecutor
JavascriptExecutor js = (JavascriptExecutor) driver;
WebElement shadowRoot = (WebElement) js.executeScript("return arguments[0].shadowRoot", shadowHost);
// Interact with elements inside the Shadow DOM
WebElement button = shadowRoot.findElement(By.cssSelector("button#shadow-button"));
button.click();
// Verify action
System.out.println("Button clicked inside Shadow DOM.");
driver.quit();
}
}
In this example:
- The
shadowHost
is located using a standard CSS selector. - JavaScript is executed to access the shadow root using
arguments[0].shadowRoot
. - The button inside the Shadow DOM is located using
findElement
on the shadow root and clicked.
5. Handling Nested Shadow DOMs
If the page contains nested Shadow DOMs, you need to access each shadow root sequentially. The process involves first accessing the outer shadow root, then locating the host of the nested shadow DOM, and repeating the process until you reach the desired element.
Example: Accessing Nested Shadow DOMs
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
public class NestedShadowDomAutomation {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Locate the outer shadow host element
WebElement outerShadowHost = driver.findElement(By.cssSelector("#outer-shadow-host"));
// Access the outer shadow root
JavascriptExecutor js = (JavascriptExecutor) driver;
WebElement outerShadowRoot = (WebElement) js.executeScript("return arguments[0].shadowRoot", outerShadowHost);
// Locate the inner shadow host element within the outer shadow root
WebElement innerShadowHost = outerShadowRoot.findElement(By.cssSelector("#inner-shadow-host"));
// Access the inner shadow root
WebElement innerShadowRoot = (WebElement) js.executeScript("return arguments[0].shadowRoot", innerShadowHost);
// Interact with elements inside the inner shadow root
WebElement button = innerShadowRoot.findElement(By.cssSelector("button#inner-shadow-button"));
button.click();
// Verify action
System.out.println("Button clicked inside nested Shadow DOM.");
driver.quit();
}
}
In this example:
- The outer shadow DOM is accessed first, followed by the nested shadow DOM.
- The button inside the nested shadow DOM is then clicked.
6. Best Practices for Interacting with Shadow DOM Elements
- Use JavaScriptExecutor: To access the Shadow DOM, you must use JavaScript execution to retrieve the shadow root.
- Handle Nested Shadow DOMs: If the page contains nested Shadow DOMs, ensure that you access each shadow root sequentially using
JavascriptExecutor
. - Use CSS Selectors: Once inside the Shadow DOM, you can use standard CSS selectors to locate elements. XPath expressions typically do not work inside the Shadow DOM.
- Be Mindful of Shadow DOM Changes: The Shadow DOM is dynamic, and its content may change. Ensure your script waits for elements to load before interacting with them.
7. Conclusion
Interacting with elements inside the Shadow DOM requires accessing the shadow root using JavaScript and then finding elements inside it. This process can be extended to handle nested Shadow DOMs. By following the correct approach and using best practices, you can effectively automate interactions with Shadow DOM elements in Selenium, even when they are encapsulated within custom components.
What is Selenium Grid?
Selenium Grid is a tool that allows you to run your Selenium WebDriver scripts in parallel across multiple machines and environments. It is designed to support cross-browser and cross-platform testing, which helps speed up the testing process by distributing test execution across different systems simultaneously. Selenium Grid facilitates the execution of tests on different browsers, operating systems, and devices, improving the efficiency of the testing process, particularly for large test suites.
1. Key Components of Selenium Grid
Selenium Grid consists of two main components:
- Hub: The central server that receives test requests and distributes them to the available nodes. The hub acts as the "brain" of the grid, managing the execution of tests on different machines.
- Node: The machines that are connected to the hub and execute the test scripts. Each node can run tests on different browsers and operating systems, allowing for cross-browser and cross-platform testing.
2. How Does Selenium Grid Work?
Selenium Grid works by setting up a hub and connecting multiple nodes to it. When a test is triggered, the hub receives the request and sends it to an available node that meets the test’s requirements (such as browser type, version, and operating system).
The test is then executed on the node, and the results are sent back to the hub. The hub acts as a coordinator, ensuring that the tests are executed on the appropriate machines and browsers.
3. Advantages of Using Selenium Grid
- Parallel Test Execution: Selenium Grid allows the execution of tests on multiple machines at the same time, significantly reducing the time required to run a large test suite.
- Cross-Browser Testing: Selenium Grid supports multiple browsers, including Chrome, Firefox, Safari, and Internet Explorer, enabling cross-browser testing with minimal configuration.
- Cross-Platform Testing: Selenium Grid supports different operating systems such as Windows, macOS, and Linux, which is essential for testing applications on various platforms.
- Scalability: Selenium Grid can be scaled easily by adding more nodes to handle more tests concurrently, making it suitable for large-scale testing environments.
- Efficiency: By distributing tests across multiple machines, Selenium Grid helps reduce the overall time taken for testing, improving the efficiency of the testing process.
4. Setting Up Selenium Grid
To set up Selenium Grid, you need to create a hub and connect one or more nodes to it. Below is a basic guide to setting up Selenium Grid:
Step 1: Start the Hub
To start the hub, run the following command in the terminal:
java -jar selenium-server-standalone.jar -role hub
This will start the hub and make it listen for requests from nodes.
Step 2: Start the Nodes
To start a node, run the following command on the machine that you want to use as a node:
java -jar selenium-server-standalone.jar -role node -hub http://:4444/grid/register
Replace <hub-ip>
with the IP address of the machine running the hub. The node will now be connected to the hub and ready to accept test requests.
5. Example: Running Tests on Selenium Grid
Once the hub and nodes are set up, you can run your tests on the grid. Below is an example in Java to run tests on Selenium Grid:
import org.openqa.selenium.Capabilities;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class SeleniumGridExample {
public static void main(String[] args) throws Exception {
// Set up desired capabilities for the browser
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName("chrome");
// Provide the hub URL
URL hubUrl = new URL("http://:4444/wd/hub");
// Initialize the WebDriver
WebDriver driver = new RemoteWebDriver(hubUrl, capabilities);
// Open the URL and interact with the page
driver.get("https://www.example.com");
System.out.println("Page title: " + driver.getTitle());
// Close the browser
driver.quit();
}
}
In this example:
- The test is configured to run on a Chrome browser.
- The
RemoteWebDriver
is used to send the test request to the Selenium Grid hub. - The hub will forward the request to an available node with the appropriate browser (in this case, Chrome).
- The test is executed on the node, and the results are sent back to the hub.
6. Best Practices for Using Selenium Grid
- Proper Configuration: Ensure that the hub and nodes are configured correctly with the necessary browser versions and operating systems.
- Monitor Node Health: Regularly monitor the health of nodes to ensure they are available for test execution and not overloaded.
- Use Desired Capabilities: Make use of
DesiredCapabilities
to specify the browser and version you want to run tests on, ensuring that tests are executed on the correct nodes. - Optimize Test Execution: Write tests that can be executed in parallel across multiple nodes to maximize the benefits of Selenium Grid.
- Scale as Needed: If your test suite grows, scale the number of nodes to distribute the load and speed up test execution.
7. Conclusion
Selenium Grid is a powerful tool for parallel test execution, enabling cross-browser and cross-platform testing. By using a hub to coordinate the execution of tests across multiple nodes, Selenium Grid helps reduce test execution time, improve efficiency, and scale testing efforts. Setting up and configuring Selenium Grid properly allows for effective and scalable automated testing of web applications across multiple environments.
Setting Up Selenium Grid for Parallel Testing
Selenium Grid allows you to execute tests in parallel across multiple machines and different environments (browsers, operating systems). This significantly reduces the time required for testing large test suites and accelerates the feedback loop for development teams. Setting up Selenium Grid for parallel testing involves configuring a hub and multiple nodes to run tests concurrently.
1. Prerequisites for Setting Up Selenium Grid
Before setting up Selenium Grid for parallel testing, ensure you have the following prerequisites:
- Selenium Server: Download the latest version of the Selenium Server (selenium-server-standalone.jar) from the official website.
- Java: Ensure you have Java installed on the hub and node machines. You can check the version by running
java -version
. - Browsers: Install the necessary browsers (Chrome, Firefox, etc.) on the machines where you’ll be running the nodes.
- Browser Drivers: Download and install the appropriate browser drivers (e.g., ChromeDriver, GeckoDriver) on the nodes.
2. Step-by-Step Guide to Setting Up Selenium Grid
Step 1: Start the Hub
The hub is the central component that receives test requests and distributes them to the available nodes. To start the hub:
java -jar selenium-server-standalone.jar -role hub
The hub will start listening on port 4444> by default. You can access the hub's status by navigating to
http://localhost:4444/grid/console
in your browser.
Step 2: Start the Nodes
Nodes are machines that execute the tests. You can set up multiple nodes on different machines or on the same machine with different browser configurations. To start a node and connect it to the hub, run the following command on each node machine:
java -jar selenium-server-standalone.jar -role node -hub http://:4444/grid/register
Replace <hub-ip>
with the IP address or hostname of the machine running the hub. Once the node is connected to the hub, it will be available for test execution.
Step 3: Configure Desired Capabilities
When running tests on Selenium Grid, you need to configure the desired capabilities for the test, specifying which browser and OS to use. For example, to run tests on Chrome, you can set the desired capabilities as follows:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class SeleniumGridTest {
public static void main(String[] args) throws Exception {
// Set the desired capabilities
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName("chrome");
// Set the hub URL
URL hubURL = new URL("http://:4444/wd/hub");
// Initialize the RemoteWebDriver
WebDriver driver = new RemoteWebDriver(hubURL, capabilities);
// Run a simple test
driver.get("https://www.example.com");
System.out.println("Page Title: " + driver.getTitle());
driver.quit();
}
}
In this example, the test will run on the Chrome browser on one of the available nodes connected to the hub.
3. Running Parallel Tests with Selenium Grid
Once the hub and nodes are set up, you can run tests in parallel across multiple nodes. Here’s an example of how to run parallel tests using TestNG, a popular testing framework:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.testng.annotations.Test;
import java.net.URL;
public class ParallelSeleniumGridTest {
@Test
public void testChrome() throws Exception {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName("chrome");
WebDriver driver = new RemoteWebDriver(new URL("http://:4444/wd/hub"), capabilities);
driver.get("https://www.example.com");
System.out.println("Chrome Test - Page Title: " + driver.getTitle());
driver.quit();
}
@Test
public void testFirefox() throws Exception {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName("firefox");
WebDriver driver = new RemoteWebDriver(new URL("http://:4444/wd/hub"), capabilities);
driver.get("https://www.example.com");
System.out.println("Firefox Test - Page Title: " + driver.getTitle());
driver.quit();
}
}
In this example, TestNG will run two tests in parallel, one on Chrome and the other on Firefox, across the available nodes connected to the hub.
4. Scaling Selenium Grid
To scale Selenium Grid and handle a larger number of tests, you can add more nodes to the grid. You can run the nodes on different physical or virtual machines, or you can run multiple nodes on the same machine with different configurations (e.g., different browsers or versions).
You can also configure the hub to distribute the tests dynamically based on available resources, ensuring that the test execution load is balanced across the nodes.
5. Best Practices for Parallel Testing with Selenium Grid
- Use Multiple Browsers and OS Environments: Test your application across different browsers and operating systems to ensure cross-browser compatibility.
- Optimize Your Test Suite: Split large test suites into smaller test cases and run them in parallel to reduce execution time.
- Monitor Node Health: Regularly monitor the health and availability of nodes to ensure they are ready to execute tests.
- Handle Test Failures Gracefully: Implement retry mechanisms in case tests fail due to node unavailability or other issues.
- Use Grid Logging and Reporting: Enable logging and reporting to keep track of test results and debug failures efficiently.
6. Conclusion
Setting up Selenium Grid for parallel testing allows you to run tests efficiently across multiple browsers, operating systems, and machines. By distributing the load and running tests in parallel, you can drastically reduce test execution time and improve the speed of your testing process. With the right setup and best practices, Selenium Grid becomes a powerful tool for scaling your test automation efforts.
Running Tests on Multiple Browsers and Devices
Running tests on multiple browsers and devices ensures your application behaves consistently across different environments. In Selenium, you can run tests on various browsers such as Chrome, Firefox, Safari, and Internet Explorer. Additionally, with tools like BrowserStack or Sauce Labs, you can run tests on real devices and different versions of browsers. This section will guide you through setting up Selenium tests for multiple browsers and devices.
1. Why Run Tests on Multiple Browsers and Devices?
Testing across multiple browsers and devices is important because:
- Cross-Browser Compatibility: Ensures the application works correctly on all popular browsers like Chrome, Firefox, Safari, and Internet Explorer.
- Mobile Responsiveness: Verifies that your application provides a seamless experience on mobile devices and tablets.
- Wide Audience Coverage: Your users may be using different browsers and devices, so testing on multiple platforms is crucial to ensure everyone has a good experience.
2. Running Tests on Multiple Browsers Locally
To run tests on multiple browsers, Selenium WebDriver supports browser-specific drivers. You need to set up the appropriate WebDriver for each browser and run the tests sequentially or in parallel. Below is an example of running tests on Chrome, Firefox, and Safari using Selenium WebDriver:
Example: Running Tests on Chrome, Firefox, and Safari (Java)
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.safari.SafariDriver;
import org.openqa.selenium.WebDriver;
public class MultiBrowserTest {
public static void main(String[] args) {
// Chrome test
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver chromeDriver = new ChromeDriver();
chromeDriver.get("https://www.example.com");
System.out.println("Chrome Browser Title: " + chromeDriver.getTitle());
chromeDriver.quit();
// Firefox test
System.setProperty("webdriver.gecko.driver", "path/to/geckodriver");
WebDriver firefoxDriver = new FirefoxDriver();
firefoxDriver.get("https://www.example.com");
System.out.println("Firefox Browser Title: " + firefoxDriver.getTitle());
firefoxDriver.quit();
// Safari test
WebDriver safariDriver = new SafariDriver();
safariDriver.get("https://www.example.com");
System.out.println("Safari Browser Title: " + safariDriver.getTitle());
safariDriver.quit();
}
}
In this example, the test will run sequentially on three different browsers: Chrome, Firefox, and Safari. You’ll need to set the path to the respective WebDriver executables.
3. Running Tests on Mobile Devices
For running tests on mobile devices, Selenium alone is not enough. You will need to use additional tools such as Appium or cloud-based services like BrowserStack or Sauce Labs to run tests on real devices or emulators/simulators.
Using Appium for Mobile Testing
Appium is an open-source tool that allows you to automate mobile apps on Android and iOS devices. It uses Selenium WebDriver to interact with the mobile app’s UI elements, making it possible to run tests on both native and hybrid mobile apps.
Here's an example of using Appium with Selenium for mobile automation:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import io.appium.java_client.android.AndroidDriver;
import java.net.URL;
public class MobileTest {
public static void main(String[] args) throws Exception {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("deviceName", "Android Emulator");
capabilities.setCapability("appPackage", "com.android.chrome");
capabilities.setCapability("appActivity", "com.google.android.apps.chrome.Main");
WebDriver driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities);
driver.get("https://www.example.com");
System.out.println("Mobile Browser Title: " + driver.getTitle());
driver.quit();
}
}
In this example, the Appium driver is used to automate a mobile browser (Chrome on Android) and navigate to a URL. You can also set up similar capabilities for iOS devices.
4. Using Cloud-Based Services for Device and Browser Testing
Cloud-based services like BrowserStack and Sauce Labs allow you to run tests on a wide range of real devices and browsers without the need to set up infrastructure. These services provide access to a cloud grid of browsers and devices, making it easier to scale and run tests in parallel across multiple environments.
Example: Running Tests on Multiple Browsers Using BrowserStack (Java)
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class BrowserStackTest {
public static void main(String[] args) throws Exception {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("browserName", "chrome");
caps.setCapability("os", "Windows");
caps.setCapability("os_version", "10");
caps.setCapability("browserstack.user", "YOUR_USERNAME");
caps.setCapability("browserstack.key", "YOUR_ACCESS_KEY");
WebDriver driver = new RemoteWebDriver(new URL("https://hub-cloud.browserstack.com/wd/hub"), caps);
driver.get("https://www.example.com");
System.out.println("BrowserStack Chrome Browser Title: " + driver.getTitle());
driver.quit();
}
}
In this example, we set the capabilities for the BrowserStack cloud service and use the RemoteWebDriver to run the test on a Chrome browser in a Windows 10 environment. Replace YOUR_USERNAME
and YOUR_ACCESS_KEY
with your BrowserStack credentials.
5. Running Tests in Parallel on Multiple Browsers
Running tests in parallel on multiple browsers is possible with frameworks like TestNG or JUnit. You can configure the test suite to run multiple tests concurrently on different browsers by specifying the browser to be used for each test. Here’s an example using TestNG for running tests in parallel on different browsers:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class ParallelTests {
@Test
public void testChrome() {
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
System.out.println("Chrome Browser Title: " + driver.getTitle());
driver.quit();
}
@Test
public void testFirefox() {
WebDriver driver = new FirefoxDriver();
driver.get("https://www.example.com");
System.out.println("Firefox Browser Title: " + driver.getTitle());
driver.quit();
}
}
TestNG will run both tests in parallel, using Chrome and Firefox, respectively. You can configure it further for more browsers or devices.
6. Best Practices for Cross-Browser and Cross-Device Testing
- Prioritize Popular Browsers: Test on browsers that are most commonly used by your target audience (e.g., Chrome, Firefox, Safari, Edge).
- Use Real Devices for Mobile Testing: While emulators and simulators are convenient, testing on real devices ensures more accurate results.
- Automate Responsiveness: Use tools like Selenium to automate testing of the responsiveness of your website or mobile app.
- Leverage Cloud Services: Use services like BrowserStack or Sauce Labs to test across a wide range of devices and browsers without managing the infrastructure.
- Handle Browser-Specific Issues: Be aware of browser-specific quirks (e.g., CSS rendering differences) and write tests that account for these variations.
7. Conclusion
Running tests on multiple browsers and devices is essential for ensuring a consistent user experience across various platforms. Selenium, combined with tools like Appium, BrowserStack, and Sauce Labs, provides a robust solution for cross-browser and cross-device testing. By leveraging these tools and best practices, you can ensure your application works flawlessly on all major browsers and devices.
Understanding the Hub-Node Architecture
Selenium Grid uses a hub-node architecture to distribute tests across multiple machines and browsers. This setup allows you to scale your tests across multiple environments (browsers, operating systems, devices) and run them in parallel. Understanding how the hub-node architecture works will help you set up Selenium Grid for distributed test execution.
1. What is Selenium Grid?
Selenium Grid is a tool used to run automated Selenium tests on multiple machines in parallel. It consists of a central hub and several nodes that communicate with it. The hub is responsible for managing the test execution and distributing tests to the available nodes based on the browser and platform configurations.
Components of Selenium Grid:
- Hub: The central point that manages all the test requests. It is responsible for routing the tests to the appropriate node based on the desired capabilities (browser, OS, etc.).
- Node: A machine that registers itself with the hub and offers specific browser configurations (e.g., Chrome, Firefox, etc.) for test execution.
2. Hub-Node Architecture
The architecture of Selenium Grid follows the hub-node structure, which allows you to distribute tests across multiple machines. The hub is the central server that directs the incoming test requests to the nodes. The nodes are the machines that actually run the tests on different browsers and platforms.
Hub:
The hub is a central server that acts as a dispatcher. It listens for incoming test requests and forwards them to an available node that matches the desired browser and platform. It is responsible for managing the overall test execution. The hub can be run on a single machine, and multiple nodes can be connected to this hub.
Node:
A node is a machine that runs one or more browsers and executes the tests sent by the hub. Each node registers itself with the hub, specifying the browser(s) and operating system(s) it supports. A node can run tests in parallel on different browsers and platforms, depending on how many browser instances it can handle.
3. Setting Up Selenium Grid
To set up Selenium Grid, you need to configure both the hub and the nodes. Below are the steps involved in setting up Selenium Grid:
Step 1: Starting the Hub
To start the hub, open a terminal or command prompt and run the following command:
java -jar selenium-server-standalone-x.xx.x.jar -role hub
This command starts the Selenium Grid hub on the local machine. The hub will start listening for incoming test requests on port 4444 by default (http://localhost:4444).
Step 2: Starting a Node
To start a node and connect it to the hub, use the following command:
java -jar selenium-server-standalone-x.xx.x.jar -role node -hub http://localhost:4444/grid/register
This command starts the node and registers it with the hub. You can configure the node to support specific browsers and operating systems by providing additional parameters.
Step 3: Configuring the Node
When launching a node, you can configure it to specify which browsers and platforms it will support. Here's an example of starting a node with Chrome and Firefox support on a Windows machine:
java -jar selenium-server-standalone-x.xx.x.jar -role node -hub http://localhost:4444/grid/register -browser browserName=chrome,maxInstances=5 -browser browserName=firefox,maxInstances=5
The above command configures the node to run both Chrome and Firefox browsers with a maximum of 5 instances each. You can add additional configurations for other browsers and platforms as needed.
4. How the Hub-Node Architecture Works
The hub-node architecture operates in the following manner:
- Test Request: A test script running on the client machine sends a request to the hub, specifying the desired browser and platform (e.g., Chrome on Windows).
- Hub Routes the Request: The hub checks which node is capable of handling the test based on the requested browser and platform. If a suitable node is found, the test request is forwarded to that node.
- Test Execution on the Node: The node receives the test request and executes the test using the specified browser and platform configuration.
- Result Return: Once the test is complete, the node sends the results back to the hub, which then returns the results to the client.
5. Advantages of the Hub-Node Architecture
- Distributed Test Execution: Allows you to run tests on multiple machines simultaneously, reducing test execution time.
- Scalability: You can easily add more nodes to scale the testing environment as needed.
- Cross-Browser Testing: Supports testing on various browsers and platforms, ensuring compatibility across different environments.
- Parallel Test Execution: Enables running multiple tests in parallel on different nodes, improving efficiency and reducing overall test time.
6. Troubleshooting Hub-Node Architecture
If you encounter issues with the hub-node architecture, here are some troubleshooting tips:
- Node Not Registering with Hub: Ensure the node’s URL and hub’s URL are correctly specified. Check the network connectivity between the node and the hub.
- Browser Not Found on Node: Ensure the correct browser drivers (e.g., ChromeDriver, GeckoDriver) are installed on the node machine.
- Test Timeout: If tests are timing out, ensure that the node has sufficient resources (memory, CPU) to handle multiple tests concurrently.
7. Conclusion
The hub-node architecture in Selenium Grid is a powerful way to distribute your tests across multiple browsers and platforms, enabling parallel execution and scaling your testing environment. By understanding how the hub and nodes interact, you can set up an efficient testing infrastructure that supports cross-browser, cross-platform, and parallel testing capabilities.
Using Docker with Selenium Grid
Using Docker with Selenium Grid allows you to easily set up and manage Selenium Grid infrastructure in a containerized environment. This setup simplifies the process of scaling Selenium Grid by eliminating the need for manual installation and configuration of the hub and nodes on individual machines.
1. What is Docker?
Docker is a platform that allows you to develop, ship, and run applications in lightweight, portable containers. It provides a consistent environment for your applications, making it easier to deploy them across different systems without worrying about compatibility issues.
2. Benefits of Using Docker with Selenium Grid
- Easy Setup: Docker simplifies the setup of Selenium Grid by providing pre-configured Docker images for the hub and nodes.
- Scalability: Docker containers can be easily scaled to meet the testing requirements by launching more containers as nodes.
- Isolation: Each node runs in its own container, ensuring a clean and isolated environment for different browsers or operating systems.
- Portability: Docker containers can be run on any system that supports Docker, making it easy to replicate environments across machines.
3. Setting Up Selenium Grid with Docker
Setting up Selenium Grid with Docker involves using Docker Compose to orchestrate the hub and node containers. Docker Compose allows you to define and run multi-container Docker applications with a simple YAML configuration file.
Step 1: Install Docker and Docker Compose
Before you can set up Selenium Grid with Docker, you need to install Docker and Docker Compose on your machine.
Step 2: Create a Docker Compose File
Create a `docker-compose.yml` file to define the services (hub and nodes) you want to run. Below is an example of a basic `docker-compose.yml` file for Selenium Grid using Docker:
version: '3'
services:
selenium-hub:
image: selenium/hub:latest
container_name: selenium-hub
ports:
- "4444:4444"
environment:
- HUB_HOST=selenium-hub
- HUB_PORT=4444
selenium-node-chrome:
image: selenium/node-chrome:latest
container_name: selenium-node-chrome
depends_on:
- selenium-hub
environment:
- HUB_HOST=selenium-hub
- HUB_PORT=4444
volumes:
- /dev/shm:/dev/shm
selenium-node-firefox:
image: selenium/node-firefox:latest
container_name: selenium-node-firefox
depends_on:
- selenium-hub
environment:
- HUB_HOST=selenium-hub
- HUB_PORT=4444
volumes:
- /dev/shm:/dev/shm
In this example, we have defined three services:
- selenium-hub: The central hub that manages test execution.
- selenium-node-chrome: A node that runs Chrome tests.
- selenium-node-firefox: A node that runs Firefox tests.
Step 3: Start Selenium Grid with Docker Compose
Once you have created the `docker-compose.yml` file, navigate to the directory where it is located and run the following command to start the Selenium Grid:
docker-compose up -d
This command will start the hub and nodes as Docker containers in detached mode (`-d`). The Selenium Grid will be accessible on http://localhost:4444
.
Step 4: Access Selenium Grid
You can access the Selenium Grid console at http://localhost:4444
to view the registered nodes and monitor the test execution.
4. Scaling Selenium Grid with Docker
To scale your Selenium Grid and add more nodes, you simply need to modify the `docker-compose.yml` file and increase the number of node instances.
Example: Adding More Nodes
selenium-node-chrome-2:
image: selenium/node-chrome:latest
container_name: selenium-node-chrome-2
depends_on:
- selenium-hub
environment:
- HUB_HOST=selenium-hub
- HUB_PORT=4444
volumes:
- /dev/shm:/dev/shm
After updating the `docker-compose.yml` file, run the following command to apply the changes:
docker-compose up -d --scale selenium-node-chrome=2
This will add another instance of the Chrome node to the grid, allowing you to run more tests in parallel.
5. Stopping and Removing Containers
To stop and remove the containers, use the following command:
docker-compose down
This will stop all running containers and remove them, cleaning up your Selenium Grid environment.
6. Managing Browser Versions
With Docker, you can easily manage different versions of browsers by specifying the version in the image tag. For example, to run a specific version of Chrome on the node, you can specify the version like this:
selenium-node-chrome:
image: selenium/node-chrome:91.0
container_name: selenium-node-chrome
depends_on:
- selenium-hub
environment:
- HUB_HOST=selenium-hub
- HUB_PORT=4444
volumes:
- /dev/shm:/dev/shm
7. Conclusion
Using Docker with Selenium Grid simplifies the setup process and provides a scalable, isolated environment for running Selenium tests. By leveraging Docker Compose, you can easily configure and manage the Selenium Grid, add more nodes, and scale up your testing infrastructure. This approach enhances the flexibility and scalability of automated testing in a containerized environment.
Understanding Automation Frameworks
An automation framework is a structured set of guidelines, tools, and practices designed to simplify and improve the efficiency of automated testing. Frameworks provide a foundation for writing, organizing, and executing test scripts, ensuring consistency, scalability, and maintainability of the test automation process.
1. What is an Automation Framework?
An automation framework is not a tool but a set of protocols and guidelines that allow testers to create and manage test scripts efficiently. It integrates tools, libraries, and best practices to ensure smooth execution of automation tasks.
Key characteristics of an automation framework include:
- Modularity: Test cases are broken down into reusable components.
- Scalability: Supports adding new test cases without significant code changes.
- Maintainability: Promotes easy updates and debugging of test scripts.
- Reporting: Provides detailed insights into test execution results.
2. Why Use an Automation Framework?
Automation frameworks streamline the testing process and provide several advantages:
- Efficiency: Reduces redundancy in test scripts by reusing components.
- Consistency: Enforces a uniform approach to writing and executing tests.
- Reduced Maintenance: Ensures that changes in the application or test cases require minimal updates.
- Integration: Easily integrates with CI/CD pipelines and other tools.
- Collaboration: Provides a standardized structure that makes it easier for teams to collaborate.
3. Types of Automation Frameworks
There are several types of automation frameworks, each with its unique approach to test automation:
- Linear Scripting Framework: A simple framework where test scripts are written sequentially for each test case. Best for small projects but lacks reusability.
- Modular Framework: Divides test cases into small, independent modules, improving reusability and maintainability.
- Data-Driven Framework: Uses external data sources (e.g., Excel, CSV, or databases) to drive test cases, allowing the same test script to run with different data sets.
- Keyword-Driven Framework: Uses keywords to represent actions, separating test logic from the automation code for better readability and reusability.
- Hybrid Framework: Combines the strengths of multiple frameworks (e.g., data-driven and keyword-driven) to provide a more flexible and scalable solution.
- Behavior-Driven Development (BDD) Framework: Focuses on collaboration between developers, testers, and business stakeholders using plain English syntax (e.g., Gherkin language with tools like Cucumber).
4. Components of an Automation Framework
An automation framework typically includes the following components:
- Test Scripts: Scripts that contain the steps for testing functionalities.
- Test Data: External files or databases containing input data and expected results.
- Object Repository: Centralized storage for UI elements, ensuring easy updates when changes occur.
- Driver Scripts: Orchestrates the execution of test scripts and integrates with the framework.
- Reporting: Generates detailed logs and test execution reports.
- Configuration Files: Stores environment settings, browser preferences, and other configurations.
5. Best Practices for Designing an Automation Framework
Follow these best practices to design an effective automation framework:
- Use a modular approach to improve reusability and maintainability of test scripts.
- Keep test data and test scripts separate to support data-driven testing.
- Leverage tools for reporting and logging to provide clear insights into test results.
- Ensure cross-browser and cross-platform compatibility for better test coverage.
- Integrate with CI/CD pipelines for continuous testing.
- Use version control systems (e.g., Git) to manage changes in test scripts.
6. Popular Tools for Building Automation Frameworks
Here are some popular tools that can be used to build and implement automation frameworks:
- Selenium: A widely used tool for web automation testing, supporting multiple frameworks.
- Appium: An open-source tool for automating mobile applications.
- Cucumber: A BDD tool that uses Gherkin language for writing test cases.
- TestNG: A testing framework inspired by JUnit, supporting parallel testing and data-driven testing.
- Robot Framework: A keyword-driven testing framework for both web and mobile applications.
7. Conclusion
Understanding automation frameworks is essential for creating efficient, maintainable, and scalable test automation solutions. By choosing the right framework and adhering to best practices, teams can achieve faster test execution, better collaboration, and improved software quality.
Types of Frameworks
Automation frameworks are structured sets of tools and guidelines that help testers create, execute, and manage test scripts efficiently. There are several types of automation frameworks, each catering to different testing needs and scenarios. Below, we explore four key types of frameworks: Data-Driven, Keyword-Driven, Hybrid, and Behavior-Driven Development (BDD).
1. Data-Driven Framework
A Data-Driven Framework focuses on separating test logic from test data. Test data is stored externally in files like Excel, CSV, or databases, and test scripts use this data to execute test cases. This approach allows the same test script to run multiple times with different data sets.
Key Features:
- Promotes reusability by decoupling test data from scripts.
- Supports large-scale testing with diverse data inputs.
- Reduces redundancy by using parameterized test cases.
Use Cases: Suitable for applications where input data varies significantly, such as form validation or e-commerce workflows.
Example:
<code> @Test(dataProvider = "loginData") public void testLogin(String username, String password) { // Test script using data from the provider } @DataProvider(name = "loginData") public Object[][] getData() { return new Object[][] { {"user1", "pass1"}, {"user2", "pass2"} }; } </code>
2. Keyword-Driven Framework
A Keyword-Driven Framework uses keywords to represent specific actions or operations in the application. Test cases are designed as a sequence of these keywords, making it easy for non-technical team members to contribute to test creation.
Key Features:
- Separates the test logic from the code base.
- Uses human-readable keywords for better clarity and collaboration.
- Enhances reusability by defining common actions as reusable keywords.
Use Cases: Suitable for teams with mixed technical expertise or applications with repetitive actions.
Example:
<code> Keyword: OpenBrowser, Argument: "https://example.com" Keyword: EnterText, Argument: "username", "testUser" Keyword: ClickButton, Argument: "Login" </code>
3. Hybrid Framework
A Hybrid Framework combines the best features of multiple frameworks, such as Data-Driven and Keyword-Driven, to create a flexible and robust testing solution. This approach addresses the limitations of individual frameworks while leveraging their strengths.
Key Features:
- Highly customizable to suit project needs.
- Combines the reusability of data-driven testing and the readability of keyword-driven testing.
- Scalable for complex applications and large test suites.
Use Cases: Ideal for complex projects requiring flexibility and diverse testing approaches.
Example:
<code> // Data-driven tests using keywords @Test(dataProvider = "testData") public void testActions(String action, String target, String value) { switch(action) { case "EnterText": // Code to enter text break; case "ClickButton": // Code to click a button break; } } </code>
4. Behavior-Driven Development (BDD) with Cucumber
Behavior-Driven Development (BDD) focuses on collaboration between developers, testers, and non-technical stakeholders. It uses plain English syntax (e.g., Gherkin language) to describe test scenarios, ensuring everyone can understand the requirements and test cases.
Key Features:
- Improves communication among team members with clear, human-readable test cases.
- Integrates seamlessly with tools like Cucumber, SpecFlow, or Behave.
- Supports test-driven development (TDD) by writing tests before development.
Use Cases: Best for projects where business stakeholders want to actively participate in test creation.
Example:
<code> Feature: Login functionality Scenario: Successful login with valid credentials Given I am on the login page When I enter valid username and password And I click on the login button Then I should see the dashboard </code>
Conclusion
Choosing the right framework depends on the project requirements, team expertise, and application complexity. Understanding these frameworks helps teams implement efficient and maintainable test automation solutions.
Setting Up a Simple Selenium Testing Framework
A Selenium testing framework organizes test scripts, ensures reusability, and simplifies test execution. Below, we outline the steps to create a simple, scalable framework using Selenium and TestNG in Java.
1. Prerequisites
Before setting up the framework, ensure the following tools are installed:
- Java Development Kit (JDK)
- Apache Maven (for dependency management)
- An Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
- Google Chrome and ChromeDriver
2. Create a Maven Project
Set up a Maven project in your preferred IDE. Add the following dependencies to the pom.xml
file for Selenium and TestNG:
<dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.1.3</version> </dependency> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency> </dependencies>
3. Directory Structure
Organize your project files as follows:
src/ main/ java/ utilities/ DriverManager.java pages/ LoginPage.java test/ java/ tests/ LoginTest.java resources/ testng.xml
4. Create a Driver Manager
The Driver Manager initializes and manages the WebDriver instance:
<code> package utilities; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class DriverManager { private static WebDriver driver; public static WebDriver getDriver() { if (driver == null) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); driver = new ChromeDriver(); driver.manage().window().maximize(); } return driver; } public static void quitDriver() { if (driver != null) { driver.quit(); driver = null; } } } </code>
5. Create a Page Object
Use the Page Object Model (POM) to represent web pages. For example, create a LoginPage
class:
<code> package pages; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; public class LoginPage { private WebDriver driver; private By usernameField = By.id("username"); private By passwordField = By.id("password"); private By loginButton = By.id("login"); public LoginPage(WebDriver driver) { this.driver = driver; } public void enterUsername(String username) { driver.findElement(usernameField).sendKeys(username); } public void enterPassword(String password) { driver.findElement(passwordField).sendKeys(password); } public void clickLogin() { driver.findElement(loginButton).click(); } } </code>
6. Write a Test Class
Create a test class using TestNG to execute the test scenario:
<code> package tests; import org.openqa.selenium.WebDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import pages.LoginPage; import utilities.DriverManager; public class LoginTest { private WebDriver driver; @BeforeMethod public void setUp() { driver = DriverManager.getDriver(); driver.get("https://example.com/login"); } @Test public void testValidLogin() { LoginPage loginPage = new LoginPage(driver); loginPage.enterUsername("testuser"); loginPage.enterPassword("password123"); loginPage.clickLogin(); // Add assertions to verify login } @AfterMethod public void tearDown() { DriverManager.quitDriver(); } } </code>
7. Create a TestNG XML File
Define the test suite and test classes in a testng.xml
file:
<?xml version="1.0" encoding="UTF-8"?> <suite name="TestSuite"> <test name="LoginTests"> <classes> <class name="tests.LoginTest" /> </classes> </test> </suite>
8. Run the Tests
Execute the tests by running the testng.xml
file or using Maven commands:
mvn test
Conclusion
This simple Selenium testing framework provides a foundation for scalable and maintainable test automation. You can extend it by adding reporting, logging, and more complex test scenarios.
Integrating Selenium with Testing Libraries (JUnit, TestNG, Pytest)
Testing libraries such as JUnit, TestNG, and Pytest are widely used with Selenium to streamline test case execution, reporting, and result validation. Below is an overview of how to integrate Selenium with these libraries in Java and Python:
1. Integration with JUnit
JUnit is a popular testing framework for Java applications. Below is an example of using Selenium with JUnit:
Setup
- Include the following dependencies in your
pom.xml
file:
<dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.1.3</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> </dependency> </dependencies>
Example Test
<code> import org.junit.After; import org.junit.Before; import org.junit.Test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class JUnitSeleniumTest { private WebDriver driver; @Before public void setUp() { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); driver = new ChromeDriver(); driver.manage().window().maximize(); } @Test public void testGoogleSearch() { driver.get("https://www.google.com"); assert driver.getTitle().contains("Google"); } @After public void tearDown() { if (driver != null) { driver.quit(); } } } </code>
2. Integration with TestNG
TestNG offers advanced features like parallel execution and parameterized tests, making it an excellent choice for Selenium.
Setup
Include the following dependency in your pom.xml
file:
<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency>
Example Test
<code> import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; public class TestNGSeleniumTest { private WebDriver driver; @BeforeMethod public void setUp() { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); driver = new ChromeDriver(); driver.manage().window().maximize(); } @Test public void testBingSearch() { driver.get("https://www.bing.com"); assert driver.getTitle().contains("Bing"); } @AfterMethod public void tearDown() { if (driver != null) { driver.quit(); } } } </code>
3. Integration with Pytest
In Python, Pytest is a powerful testing framework that works seamlessly with Selenium.
Setup
- Install the required dependencies using pip:
pip install selenium pytest
Example Test
<code> import pytest from selenium import webdriver @pytest.fixture def driver(): driver = webdriver.Chrome(executable_path="path/to/chromedriver") driver.maximize_window() yield driver driver.quit() def test_google_title(driver): driver.get("https://www.google.com") assert "Google" in driver.title </code>
Comparison of Testing Libraries
Feature | JUnit | TestNG | Pytest |
---|---|---|---|
Language | Java | Java | Python |
Parallel Execution | Limited | Supported | Supported (via plugins) |
Parameterization | Annotations | XML/Annotations | Fixture Parameters |
Ease of Use | Moderate | Advanced Features | Simple and Pythonic |
Conclusion
The integration of Selenium with JUnit, TestNG, or Pytest enhances the testing process, offering better structure, modularity, and scalability. Choose the library that best fits your project’s language and requirements.
Creating Test Suites with TestNG or JUnit
Test suites group related test cases to execute them collectively. They are essential for managing larger test scenarios efficiently. Below are steps and examples for creating test suites using TestNG and JUnit.
1. Creating Test Suites with TestNG
TestNG provides a flexible XML-based configuration for organizing and executing test suites.
Setup
- Include the following dependency in your
pom.xml
:
<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency>
Step 1: Create Test Classes
<code> // TestClass1.java import org.testng.annotations.Test; public class TestClass1 { @Test public void testMethod1() { System.out.println("TestClass1 - testMethod1"); } } // TestClass2.java import org.testng.annotations.Test; public class TestClass2 { @Test public void testMethod2() { System.out.println("TestClass2 - testMethod2"); } } </code>
Step 2: Create the TestNG Suite XML File
Define the suite structure in an XML file (e.g., testng-suite.xml
):
<?xml version="1.0" encoding="UTF-8"?> <suite name="Test Suite Example" verbose="1"> <test name="Example Test"> <classes> <class name="TestClass1" /> <class name="TestClass2" /> </classes> </test> </suite>
Step 3: Run the Test Suite
- Use your IDE or command line to run the suite.
- From the command line:
mvn test -DsuiteXmlFile=testng-suite.xml
2. Creating Test Suites with JUnit
JUnit uses annotations to define and execute test suites.
Setup
- Include the following dependency in your
pom.xml
:
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> </dependency>
Step 1: Create Test Classes
<code> // TestClass1.java import org.junit.Test; import static org.junit.Assert.assertTrue; public class TestClass1 { @Test public void testMethod1() { System.out.println("TestClass1 - testMethod1"); assertTrue(true); } } // TestClass2.java import org.junit.Test; import static org.junit.Assert.assertTrue; public class TestClass2 { @Test public void testMethod2() { System.out.println("TestClass2 - testMethod2"); assertTrue(true); } } </code>
Step 2: Create the Test Suite Class
Create a suite class annotated with @RunWith
and @Suite
:
<code> import org.junit.runner.RunWith; import org.junit.runners.Suite; @RunWith(Suite.class) @Suite.SuiteClasses({ TestClass1.class, TestClass2.class }) public class TestSuite { // This class remains empty. It is used only as a holder for the above annotations. } </code>
Step 3: Run the Test Suite
- Run the suite class directly from your IDE or command line:
- From the command line:
mvn test
Comparison of TestNG and JUnit for Test Suites
Feature | TestNG | JUnit |
---|---|---|
Configuration | XML-based | Annotation-based |
Parallel Testing | Supported | Limited |
Flexibility | More features (data-driven, groups, etc.) | Simpler |
Conclusion
Both TestNG and JUnit offer powerful features for creating test suites. Choose TestNG for complex projects requiring advanced features like parallel testing and parameterization, while JUnit suits simpler, annotation-driven test setups.
Generating Test Reports (Allure, Extent Reports)
Test reporting is essential for tracking and analyzing test execution results. Tools like Allure and Extent Reports provide user-friendly, visually appealing reports that simplify understanding the test outcomes.
1. Generating Test Reports with Allure
Allure is a flexible and popular reporting tool for Selenium and other test frameworks. It integrates seamlessly with TestNG, JUnit, and Pytest.
Setup
- Add the Allure dependencies to your
pom.xml
:
<dependency> <groupId>io.qameta.allure</groupId> <artifactId>allure-testng</artifactId> <version>2.20.1</version> </dependency> <dependency> <groupId>io.qameta.allure</groupId> <artifactId>allure-junit4</artifactId> <version>2.20.1</version> </dependency>
Step 1: Annotate Test Methods
Use Allure annotations to enhance the reports:
<code> import io.qameta.allure.Description; import io.qameta.allure.Step; import org.testng.annotations.Test; public class ExampleTest { @Test @Description("This is a sample test for Allure reporting.") public void sampleTest() { stepOne(); stepTwo(); } @Step("Step 1: Perform the first action") public void stepOne() { System.out.println("Executing step one"); } @Step("Step 2: Perform the second action") public void stepTwo() { System.out.println("Executing step two"); } } </code>
Step 2: Execute Tests and Generate Reports
- Run the tests in your IDE or via Maven.
- After execution, generate the Allure report:
mvn allure:serve
This command generates and serves the report on a local server.
Features
- Interactive HTML reports
- Detailed step-by-step logs
- Integration with CI tools like Jenkins
2. Generating Test Reports with Extent Reports
Extent Reports provides highly customizable and visually appealing reports with charts, filters, and logs.
Setup
- Add the Extent Reports dependency to your
pom.xml
:
<dependency> <groupId>com.aventstack</groupId> <artifactId>extentreports</artifactId> <version>5.0.9</version> </dependency>
Step 1: Configure Extent Reports in Your Code
Create an Extent Report instance and log test results:
<code> import com.aventstack.extentreports.ExtentReports; import com.aventstack.extentreports.ExtentTest; import com.aventstack.extentreports.reporter.ExtentHtmlReporter; import org.testng.annotations.AfterSuite; import org.testng.annotations.BeforeSuite; import org.testng.annotations.Test; public class ExtentReportExample { ExtentReports extent; ExtentTest test; @BeforeSuite public void setup() { ExtentHtmlReporter htmlReporter = new ExtentHtmlReporter("extent-report.html"); extent = new ExtentReports(); extent.attachReporter(htmlReporter); } @Test public void sampleTest() { test = extent.createTest("Sample Test", "This is a sample test for Extent Reports."); test.pass("Step 1 passed"); test.info("Execution details logged"); } @AfterSuite public void teardown() { extent.flush(); } } </code>
Step 2: Execute Tests and View the Report
- Run the test suite in your IDE or via Maven.
- Open the generated
extent-report.html
file in a browser to view the report.
Features
- Customizable HTML reports
- Charts and filters for detailed insights
- Integration with CI/CD pipelines
Comparison of Allure and Extent Reports
Feature | Allure | Extent Reports |
---|---|---|
Ease of Use | Simple and annotation-driven | Requires more configuration |
Visual Appeal | Moderate | Highly customizable and visually rich |
Integration | Works well with CI tools | Good support for CI/CD |
Conclusion
Both Allure and Extent Reports are excellent options for generating test reports. Allure is suited for developers looking for simplicity and quick setup, while Extent Reports is ideal for detailed and customizable reports.
Parallel Test Execution with TestNG
Parallel test execution in TestNG allows you to run multiple tests simultaneously, reducing the total execution time. This is particularly useful for large test suites and cross-browser testing.
1. Setting Up Parallel Test Execution
Parallel execution in TestNG can be achieved by configuring the testng.xml
file. You can set the parallel
attribute to execute tests, classes, or methods concurrently.
Step 1: Create Test Classes
Create multiple test classes for demonstration:
<code> // TestClass1.java import org.testng.annotations.Test; public class TestClass1 { @Test public void testMethod1() { System.out.println("Test Method 1 from TestClass1 is running on " + Thread.currentThread().getId()); } } // TestClass2.java import org.testng.annotations.Test; public class TestClass2 { @Test public void testMethod2() { System.out.println("Test Method 2 from TestClass2 is running on " + Thread.currentThread().getId()); } } </code>
Step 2: Configure testng.xml
Edit the testng.xml
file to enable parallel execution:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd"> <suite name="Parallel Execution Suite" parallel="classes" thread-count="2"> <test name="TestClass1"> <classes> <class name="TestClass1" /> </classes> </test> <test name="TestClass2"> <classes> <class name="TestClass2" /> </classes> </test> </suite>
Explanation:
parallel="classes"
: Runs test classes concurrently.thread-count="2"
: Defines the number of threads for parallel execution.
Step 3: Run the Suite
Execute the testng.xml
file in your IDE or via Maven. The output will show that tests are running in parallel on different threads.
2. Parallel Execution at the Method Level
To execute individual test methods in parallel, modify the parallel
attribute:
<suite name="Parallel Methods Suite" parallel="methods" thread-count="2"> <test name="Parallel Methods Test"> <classes> <class name="TestClass1" /> <class name="TestClass2" /> </classes> </test> </suite>
3. Parallel Execution at the Test Level
To execute test tags in parallel, use the parallel="tests"
attribute:
<suite name="Parallel Tests Suite" parallel="tests" thread-count="2"> <test name="Test1"> <classes> <class name="TestClass1" /> </classes> </test> <test name="Test2"> <classes> <class name="TestClass2" /> </classes> </test> </suite>
4. Managing Thread Safety
Since parallel execution involves multiple threads, ensure thread safety:
- Avoid using static variables for test data.
- Use thread-local storage for thread-specific data.
- Ensure test dependencies are isolated.
5. Benefits of Parallel Test Execution
- Reduces overall execution time for large test suites.
- Optimizes resource utilization for multi-core systems.
- Enables cross-browser and cross-platform testing.
6. Tips for Effective Parallel Testing
- Set an appropriate
thread-count
to avoid resource contention. - Use logging to track test execution across threads.
- Leverage cloud-based test platforms for cross-environment testing.
Conclusion
Parallel test execution in TestNG is a powerful feature that boosts efficiency in test automation. By properly configuring testng.xml
and ensuring thread safety, you can effectively run tests concurrently and achieve faster feedback on your test results.
Introduction to API Testing
API testing is a critical part of software testing that focuses on verifying the functionality, reliability, performance, and security of application programming interfaces (APIs). It ensures that APIs deliver the expected outcomes and meet business requirements.
1. What is an API?
An API (Application Programming Interface) is a set of rules and protocols that allows one application to communicate with another. APIs enable data exchange between systems, services, or applications, forming the backbone of modern software architecture.
Examples:
- REST APIs: Representational State Transfer APIs, widely used in web applications.
- SOAP APIs: Simple Object Access Protocol APIs, often used in enterprise solutions.
- GraphQL APIs: A query language for APIs, allowing clients to request only the data they need.
2. Why is API Testing Important?
APIs act as the intermediary between the user interface and the server. Testing APIs ensures:
- Data integrity between systems.
- Proper error handling and response codes.
- High performance under varying load conditions.
- Security against unauthorized access.
3. Types of API Testing
API testing covers various aspects of an API’s functionality:
- Functional Testing: Verifies that the API returns the expected responses for given inputs.
- Performance Testing: Assesses speed, scalability, and reliability under load.
- Security Testing: Ensures that the API is secure against threats like unauthorized access.
- Validation Testing: Confirms the API adheres to business requirements.
- Error Handling Testing: Checks how the API responds to invalid inputs.
4. Tools for API Testing
Several tools are available to facilitate API testing:
- Postman: A widely used tool for manual and automated API testing.
- SoapUI: Ideal for testing SOAP and REST APIs.
- REST Assured: A Java library for testing REST APIs.
- JMeter: Used for performance testing APIs.
- Katalon Studio: A comprehensive tool for API, web, and mobile testing.
5. Key Components of API Testing
When testing APIs, focus on the following:
- Request: The input sent to the API (e.g., GET, POST, PUT, DELETE).
- Response: The output received from the API, including headers, status codes, and the body.
- Headers: Metadata sent with requests/responses (e.g., Content-Type, Authorization).
- Authentication: Methods like API keys, OAuth, or JWT tokens to secure APIs.
6. Steps in API Testing
- Understand the API requirements and endpoints.
- Set up the testing environment.
- Formulate test cases for different scenarios.
- Execute the test cases using tools or scripts.
- Validate the response (status codes, data, headers).
- Log and report test results.
7. Best Practices for API Testing
- Use descriptive names for test cases to indicate their purpose.
- Test for edge cases, including invalid inputs and large payloads.
- Validate both positive and negative scenarios.
- Incorporate security testing to identify vulnerabilities.
- Automate repetitive test cases for efficiency.
8. Common Challenges in API Testing
- Understanding complex API documentation.
- Handling dynamic responses and data.
- Maintaining test scripts as APIs evolve.
- Ensuring comprehensive test coverage across all endpoints.
Conclusion
API testing is essential to ensure seamless communication between applications and the reliability of modern software systems. By using the right tools and following best practices, you can create a robust API testing strategy that ensures high-quality software delivery.
Simulating API Calls During Selenium Tests
In some scenarios, automating only the user interface (UI) with Selenium may not be sufficient to verify backend processes. Simulating API calls during Selenium tests can improve efficiency and provide deeper insights into application behavior.
1. Why Simulate API Calls in Selenium Tests?
- To verify backend functionality independently from the UI.
- To test scenarios that are difficult to reproduce through the UI.
- To reduce the overall execution time by bypassing the UI for certain operations.
- To validate the integration between the front end and back end.
2. Tools and Libraries for Simulating API Calls
- REST Assured: A Java library for testing RESTful APIs, commonly used alongside Selenium.
- Postman/Newman: For executing pre-configured API requests during Selenium tests.
- HTTP Clients: Libraries like Python’s
requests
, Node.js’saxios
, or Java’sHttpClient
.
3. Common Use Cases
- Preloading Data: Set up specific test data by making POST/PUT API calls before executing a Selenium test.
- Validating Backend Responses: Send API requests and verify responses directly.
- Mocking Unavailable APIs: Use simulated responses for APIs that are not yet implemented or unavailable.
4. Example: Simulating API Calls Using REST Assured (Java)
The following example demonstrates how to integrate REST Assured with Selenium to simulate API calls:
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumWithApiTest {
public static void main(String[] args) {
// API Call: Create test data
RestAssured.baseURI = "https://api.example.com";
Response response = RestAssured.given()
.header("Content-Type", "application/json")
.body("{ \"name\": \"Test User\", \"email\": \"test@example.com\" }")
.post("/users");
System.out.println("API Response: " + response.getBody().asString());
// Selenium Test: Verify UI behavior
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
// Perform UI actions to validate the API's effect
driver.quit();
}
}
5. Example: Simulating API Calls in Python with Requests
import requests
from selenium import webdriver
# API Call: Create test data
url = "https://api.example.com/users"
payload = { "name": "Test User", "email": "test@example.com" }
headers = { "Content-Type": "application/json" }
response = requests.post(url, json=payload, headers=headers)
print(f"API Response: {response.json()}")
# Selenium Test: Verify UI behavior
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
driver.get("https://example.com/login")
# Perform UI actions to validate the API's effect
driver.quit()
6. Mocking API Responses
When the actual API is unavailable, you can mock API responses using tools like:
- WireMock: For creating a local mock server to simulate API responses.
- Postman Mock Servers: For mocking API endpoints during development and testing.
7. Best Practices
- Keep API calls and Selenium tests modular to ensure maintainability.
- Log API requests and responses for debugging purposes.
- Handle API failures gracefully to avoid blocking the Selenium test execution.
- Use environment-specific configurations for API endpoints and credentials.
8. Challenges and Considerations
- Ensuring synchronization between API responses and UI changes.
- Mocking or stubbing APIs when working in isolated test environments.
- Handling rate limits or throttling for API calls during testing.
Conclusion
Simulating API calls during Selenium tests can significantly enhance your testing strategy by reducing dependencies on the UI and providing faster feedback on backend functionality. By leveraging tools like REST Assured, Python’s requests
, or mock servers, you can create robust and efficient test suites.
Using Tools Like REST Assured Alongside Selenium
REST Assured is a powerful Java library for testing and validating REST APIs. Combining it with Selenium allows testers to validate both backend APIs and frontend UI in a single test workflow. This approach ensures end-to-end testing of the application, covering both the logic layer and the user interface.
1. Why Use REST Assured with Selenium?
- Data Setup: Use API calls to prepare or manipulate test data before executing Selenium tests.
- Validation: Validate backend responses alongside UI actions to ensure data consistency.
- Efficiency: Reduce dependency on the UI by directly interacting with APIs where applicable.
2. Setting Up REST Assured
Follow these steps to set up REST Assured in your project:
Step 1: Add REST Assured to Your Project
Include REST Assured in your project's dependency management tool:
Maven
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>5.3.0</version>
</dependency>
Gradle
implementation 'io.rest-assured:rest-assured:5.3.0'
Step 2: Import REST Assured in Your Test Class
import io.restassured.RestAssured;
import io.restassured.response.Response;
import static io.restassured.RestAssured.*;
3. Integrating REST Assured with Selenium
Here’s how to integrate REST Assured with Selenium for comprehensive testing:
Example: Authenticate via API and Use in Selenium
Code Example
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
public class RestAssuredSeleniumIntegration {
public static void main(String[] args) {
// Step 1: API Call to Authenticate User
RestAssured.baseURI = "https://api.example.com";
Response response = given()
.header("Content-Type", "application/json")
.body("{ \"username\": \"testuser\", \"password\": \"testpass\" }")
.post("/login");
if (response.getStatusCode() == 200) {
String token = response.jsonPath().get("token");
System.out.println("Authentication Successful. Token: " + token);
// Step 2: Pass Token to Selenium WebDriver
System.setProperty("webdriver.chrome.driver", "path-to-chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://app.example.com");
((JavascriptExecutor) driver).executeScript(
"localStorage.setItem('authToken', '" + token + "');"
);
// Refresh the page to reflect logged-in state
driver.navigate().refresh();
// Perform UI validations
System.out.println("UI validations complete.");
driver.quit();
} else {
System.out.println("API Authentication Failed: " + response.getStatusLine());
}
}
}
Example: Pre-Fill Data Using API
- Use REST Assured to create test data, such as adding a user or setting up an order.
- Verify the data in the UI using Selenium.
Code Example
Response createOrderResponse = given()
.header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json")
.body("{ \"productId\": \"123\", \"quantity\": 2 }")
.post("/orders");
if (createOrderResponse.getStatusCode() == 201) {
System.out.println("Order created successfully.");
WebDriver driver = new ChromeDriver();
driver.get("https://app.example.com/orders");
// Selenium checks to verify the order appears in the UI
driver.quit();
}
4. Best Practices
- Modularize API and UI Logic: Keep API interactions in separate methods or classes.
- Validate Data: Use REST Assured to validate data consistency after performing UI actions.
- Handle API Failures: Implement retries and error handling for robust tests.
- Use Configurations: Store API base URLs, headers, and other configurations in a properties file for easy updates.
5. Challenges
- Ensuring API and UI states remain in sync during tests.
- Managing authentication tokens and session expiration efficiently.
- Handling dynamic data changes caused by concurrent API/UI operations.
Conclusion
Integrating REST Assured with Selenium enables testers to validate application logic comprehensively across layers. This combination enhances test coverage, ensures data consistency, and supports more efficient testing workflows.
Introduction to Continuous Integration (CI)
Continuous Integration (CI) is a software development practice where code changes are automatically built, tested, and integrated into the main branch multiple times a day. The goal of CI is to detect errors as soon as possible and improve the overall quality of software by automating the integration process.
1. Why Use Continuous Integration?
CI offers several benefits for software development teams:
- Faster Development: Developers can integrate code frequently, reducing integration problems and speeding up development cycles.
- Early Detection of Bugs: Automated tests run on each integration, helping to catch issues early in the development process.
- Improved Code Quality: With automated testing and frequent feedback, developers can maintain a higher standard of code quality.
- Reduced Manual Work: By automating testing and deployment processes, CI reduces manual intervention, freeing up resources for more important tasks.
2. Key Components of CI
A typical CI pipeline consists of the following components:
- Version Control System (VCS): Platforms like Git, SVN, or Mercurial are used to store and manage the source code, allowing teams to track changes and collaborate on development.
- Build Automation Tool: Tools like Maven, Gradle, or Ant are used to automate the build process, ensuring that the software is built consistently across different environments.
- Continuous Integration Server: Tools like Jenkins, GitLab CI, or CircleCI manage the CI pipeline, triggering builds and tests whenever new code is pushed to the repository.
- Automated Testing: Unit tests, integration tests, and UI tests are run automatically to ensure that new changes don’t break existing functionality.
- Artifact Repository: Once the build passes, the resulting binaries (e.g., JAR files, Docker images) are stored in an artifact repository like Nexus or Artifactory for deployment or distribution.
3. How Does Continuous Integration Work?
The typical workflow for CI involves several steps:
- Code Commit: Developers commit their changes to the version control system (VCS) frequently, usually several times a day.
- Build Triggered: The CI server detects the change and triggers an automated build process. This process compiles the code and runs unit tests to verify that the changes don’t break any functionality.
- Automated Testing: The CI server runs a suite of automated tests to validate that the new changes are working as expected.
- Feedback: If any issues are detected, the CI server provides feedback to the developer, allowing them to fix the issues before integration with the main codebase.
- Deployment (Optional): Once the code passes all tests, it can be automatically deployed to a staging or production environment for further testing or release.
4. Common Tools for Continuous Integration
There are several tools available for implementing CI in your development workflow:
- Jenkins: An open-source automation server popular for CI/CD pipelines, with a large number of plugins for integration with various tools and services.
- GitLab CI: A fully integrated CI/CD system built into GitLab, which offers built-in CI pipelines and deployment features.
- CircleCI: A cloud-based CI tool that integrates with GitHub and Bitbucket, offering fast and scalable builds.
- Travis CI: A CI service that integrates with GitHub repositories and provides an easy setup for automated builds and tests.
- Azure Pipelines: A cloud-based CI/CD service from Microsoft that integrates with Azure DevOps for automated builds and releases.
5. Example CI Pipeline
A basic CI pipeline might look like this:
stages:
- build
- test
- deploy
build:
stage: build
script:
- npm install
- npm run build
test:
stage: test
script:
- npm test
deploy:
stage: deploy
script:
- npm run deploy
In this example, the pipeline consists of three stages: build, test, and deploy. Each stage is defined with a set of commands to execute, and the pipeline will execute them in the specified order.
6. Best Practices for Continuous Integration
- Commit Frequently: Commit your changes often to avoid integration issues, and keep each commit small and focused on a single task.
- Automate Testing: Set up automated tests for unit tests, integration tests, and UI tests. Ensure all tests pass before integrating new changes.
- Use a Version Control System: Ensure all code changes are tracked in a version control system like Git to maintain a history of changes and support collaboration.
- Use Feature Branches: Use feature branches to work on new features or bug fixes and merge them into the main branch only after they’ve been tested and reviewed.
- Monitor Pipeline Health: Regularly monitor the CI pipeline to identify issues early and ensure it remains stable.
7. Challenges with Continuous Integration
- Initial Setup: Setting up CI infrastructure and configuring automated tests can take time and effort.
- Flaky Tests: Tests that intermittently fail can undermine the effectiveness of CI if not addressed.
- Handling Large Projects: As the project grows, managing the CI pipeline with multiple dependencies and a large number of tests can become challenging.
8. Conclusion
Continuous Integration is a crucial practice for modern software development teams. It helps ensure that the codebase is always in a deployable state by automating the process of building, testing, and integrating new changes. By implementing a CI pipeline, teams can improve the speed, quality, and reliability of their software.
Integrating Selenium with CI/CD Tools (Jenkins, GitHub Actions, CircleCI)
Continuous Integration and Continuous Deployment (CI/CD) tools help automate the process of building, testing, and deploying software. Integrating Selenium with CI/CD tools allows you to run automated Selenium tests as part of the build process, ensuring that your code is always tested and deployed efficiently. Here, we will explore how to integrate Selenium with three popular CI/CD tools: Jenkins, GitHub Actions, and CircleCI.
1. Why Integrate Selenium with CI/CD?
Integrating Selenium tests into your CI/CD pipeline offers several benefits:
- Automated Testing: Tests run automatically on every code commit, helping to catch errors early.
- Faster Feedback: Developers receive rapid feedback about the status of their code, improving development speed.
- Consistent Testing Environments: Selenium tests run in consistent environments, ensuring that test results are reliable.
- Improved Code Quality: Continuous testing helps maintain high-quality code by ensuring new changes do not break existing functionality.
2. Integrating Selenium with Jenkins
Jenkins is one of the most widely used CI/CD tools. Here's how you can integrate Selenium tests into Jenkins:
- Set Up Jenkins: Install Jenkins and set up a Jenkins job or pipeline for your project. You can use the Jenkins UI or a Jenkinsfile for pipeline configuration.
- Install Necessary Plugins: Install the necessary plugins, such as the JUnit or TestNG plugin to view test results, and the Git plugin to integrate with your version control system.
- Configure Selenium in Jenkins: Ensure that the machine where Jenkins is running has Selenium WebDriver and browser drivers installed (e.g., ChromeDriver, GeckoDriver). You can do this by installing the appropriate WebDriver binaries and adding them to the system’s PATH.
- Set Up Selenium Tests in the Pipeline: Add your Selenium test scripts to your project repository and configure Jenkins to run them as part of the build process. In your Jenkinsfile, you can define a test stage as follows:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install' // Build your project
}
}
stage('Test') {
steps {
sh 'mvn test' // Run your Selenium tests
}
}
stage('Deploy') {
steps {
sh 'mvn deploy' // Deploy the application
}
}
}
}
Jenkins will automatically run the Selenium tests during the 'Test' stage and provide feedback on whether the tests pass or fail.
3. Integrating Selenium with GitHub Actions
GitHub Actions is a powerful automation tool built into GitHub repositories, allowing you to automate workflows like building, testing, and deploying software. Here's how to integrate Selenium with GitHub Actions:
- Set Up GitHub Actions: Create a new file under the `.github/workflows` directory in your repository (e.g., `selenium-tests.yml`). This file will define your CI/CD pipeline.
- Install Dependencies: In the workflow file, use an appropriate action to set up Java, Node.js, or Python, depending on the language you are using for Selenium tests. If you're using Java, you could use:
name: Selenium Test Workflow
on:
push:
branches:
- main
jobs:
selenium-test:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Set up Java
uses: actions/setup-java@v2
with:
java-version: '11'
- name: Install dependencies
run: |
mvn install
- name: Run Selenium tests
run: |
mvn test
This YAML file defines a workflow that triggers on a push to the `main` branch. It installs the necessary dependencies and runs your Selenium tests using Maven.
4. Integrating Selenium with CircleCI
CircleCI is another popular CI/CD tool that can be used to automate testing and deployment. To integrate Selenium with CircleCI:
- Set Up CircleCI: Create a `.circleci/config.yml` file in your repository. This file defines the steps in your CI pipeline.
- Configure the CircleCI Pipeline: Define the pipeline steps to install dependencies, run tests, and deploy the application. Here's an example configuration for running Selenium tests:
version: 2.1
jobs:
selenium-tests:
docker:
- image: circleci/python:3.8
steps:
- checkout
- run:
name: Install Dependencies
command: |
pip install -r requirements.txt
- run:
name: Run Selenium Tests
command: |
python -m unittest discover tests/
workflows:
version: 2
test:
jobs:
- selenium-tests
This configuration runs the Selenium tests using Python and the `unittest` module. You can modify it based on the language and testing framework you use.
5. Best Practices for Integrating Selenium with CI/CD
- Run Tests on Every Commit: Set up your CI pipeline to run Selenium tests on every commit to ensure that new changes are continuously validated.
- Parallel Test Execution: Use parallel test execution to reduce the time taken by Selenium tests in your CI pipeline. Tools like Selenium Grid, Docker, or cloud-based services can help achieve this.
- Use Headless Browsers: For faster test execution, run tests in headless browsers (e.g., ChromeHeadless) instead of full browsers.
- Handle Test Failures Gracefully: Implement retries or notifications for failed tests, so you can quickly address issues and keep your pipeline running smoothly.
6. Conclusion
Integrating Selenium with CI/CD tools like Jenkins, GitHub Actions, and CircleCI ensures that your Selenium tests are automatically triggered as part of the development workflow. This integration enables faster feedback, better code quality, and more efficient testing cycles, allowing teams to maintain a high standard of software quality while accelerating the development process.
Automating Selenium Test Execution with Pipelines
Automating Selenium test execution through pipelines ensures that your tests are continuously run as part of the software development process. Integrating Selenium with pipelines such as Jenkins, GitHub Actions, and CircleCI allows you to automate the execution of tests on every code change, ensuring that the application remains bug-free and functional as new changes are made.
1. What is a Pipeline in CI/CD?
A pipeline in CI/CD (Continuous Integration/Continuous Deployment) is a set of automated steps that manage the software delivery process. These pipelines include stages such as building the code, running tests, and deploying the application. When integrated with Selenium, the pipeline can automatically trigger Selenium tests after the code is built, ensuring that the application is thoroughly tested before deployment.
2. Benefits of Automating Selenium Test Execution with Pipelines
- Early Bug Detection: Running tests on every code commit ensures bugs are detected early in the development cycle.
- Continuous Testing: Selenium tests are automatically executed as part of the CI/CD pipeline, ensuring that your application is always tested.
- Faster Feedback: Developers can quickly see whether their changes have broken the application or caused any issues.
- Reduced Manual Effort: Automating the test execution process reduces the need for manual intervention, ensuring more reliable and efficient testing.
3. Automating Selenium Tests with Jenkins Pipeline
Jenkins, a popular CI/CD tool, can be used to automate Selenium test execution by creating a Jenkins pipeline. Below is an example of how to set up Selenium tests in a Jenkins pipeline:
- Set Up Jenkins: Install Jenkins and create a new pipeline job.
- Configure the Pipeline: Add the following configuration to your `Jenkinsfile` to define the stages of the pipeline. This file can be placed in the root directory of your project.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install' // Build your project
}
}
stage('Test') {
steps {
sh 'mvn test' // Run your Selenium tests
}
}
stage('Deploy') {
steps {
sh 'mvn deploy' // Deploy the application
}
}
}
}
This Jenkins pipeline defines three stages: Build, Test, and Deploy. The test stage runs the Selenium tests using Maven. You can customize it based on your project’s requirements.
4. Automating Selenium Tests with GitHub Actions
GitHub Actions is another tool that can be used to automate Selenium tests. Below is an example of how to automate Selenium test execution using GitHub Actions:
- Set Up GitHub Actions: Create a `.github/workflows/selenium-tests.yml` file in your repository.
- Configure the Workflow: Define the steps in the workflow file to install dependencies, run tests, and deploy the application. Here's an example configuration:
name: Selenium Test Workflow
on:
push:
branches:
- main
jobs:
selenium-test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Java
uses: actions/setup-java@v2
with:
java-version: '11'
- name: Install dependencies
run: |
mvn install
- name: Run Selenium tests
run: |
mvn test
This GitHub Actions workflow is triggered when code is pushed to the `main` branch. It installs necessary dependencies and runs the Selenium tests using Maven.
5. Automating Selenium Tests with CircleCI
CircleCI also provides a simple way to automate Selenium test execution. Below is an example CircleCI configuration:
- Set Up CircleCI: Create a `.circleci/config.yml` file in your repository.
- Configure the Pipeline: Define the steps to install dependencies, run tests, and deploy the application. Here is an example configuration:
version: 2.1
jobs:
selenium-tests:
docker:
- image: circleci/python:3.8
steps:
- checkout
- run:
name: Install Dependencies
command: |
pip install -r requirements.txt
- run:
name: Run Selenium Tests
command: |
python -m unittest discover tests/
workflows:
version: 2
test:
jobs:
- selenium-tests
This configuration runs your Selenium tests using Python and the `unittest` module inside a Docker container. You can modify it based on your project needs.
6. Best Practices for Automating Selenium Test Execution with Pipelines
- Run Tests Frequently: Ensure that Selenium tests are run frequently (e.g., on every commit or push) to catch issues early.
- Parallel Test Execution: Use tools like Selenium Grid, Docker, or cloud-based services to run tests in parallel, reducing overall test execution time.
- Use Headless Browsers: For quicker test execution, use headless browsers (e.g., ChromeHeadless) instead of GUI-based browsers.
- Handle Test Failures Effectively: Implement test retries or notifications for failed tests to quickly address any issues and keep the pipeline flowing smoothly.
- Use Containers for Environment Consistency: Run Selenium tests inside containers (e.g., Docker) to ensure that all tests run in a consistent and isolated environment.
7. Conclusion
Automating Selenium test execution within CI/CD pipelines ensures that your application is continuously tested and validated. By integrating Selenium with tools like Jenkins, GitHub Actions, and CircleCI, you can streamline the testing process, reduce manual effort, and ensure faster, more reliable software delivery. Following best practices, such as running tests in parallel and using headless browsers, further optimizes the testing process and enhances the efficiency of your CI/CD pipeline.
Understanding Selenium's Limitations in Performance Testing
Selenium is a popular tool for automating web browsers and is widely used for functional testing. However, when it comes to performance testing, Selenium has certain limitations that can impact its effectiveness. While Selenium can simulate user interactions and validate functionality, it is not designed specifically for performance testing, such as load and stress testing. This section explores the key limitations of Selenium in performance testing and why other tools might be more suitable for this purpose.
1. Selenium is Primarily for Functional Testing
Selenium is built to test the functionality of web applications, not their performance. It allows you to automate user interactions like clicks, form submissions, and navigation, but it does not provide built-in features for testing how the application performs under load or stress. Performance testing involves measuring response times, throughput, and resource utilization, which is beyond Selenium's scope.
2. Selenium's Overhead in Simulating User Interactions
Selenium works by automating real browsers, meaning it opens a browser window, interacts with the DOM, and waits for responses. This overhead can significantly impact the accuracy of performance metrics. When using Selenium to simulate many concurrent users, the resource consumption of the browser (CPU, memory, and network) can cause inaccurate results, as it doesn't simulate how real users interact with the system in terms of load and scalability.
3. Lack of Load Testing Capabilities
For performance testing, you need to simulate a large number of virtual users interacting with the application simultaneously to measure how it performs under load. Selenium does not have built-in capabilities for simulating multiple concurrent users or for generating load. Other tools like Apache JMeter, Gatling, or LoadRunner are specifically designed to simulate high traffic and provide detailed load testing metrics.
4. Browser Resource Consumption
Selenium tests require real browsers, which means every test in a large-scale performance test scenario demands a full browser instance. This leads to high resource consumption, and running many simultaneous browser instances can overwhelm the system and cause the test to slow down. This resource usage is not ideal for performance testing, where thousands or even millions of virtual users need to be simulated.
5. No Native Support for Distributed Testing
In performance testing, especially load testing, it's often necessary to distribute the load across many machines to simulate a large number of virtual users. Selenium does not have native support for this type of distributed testing. Although Selenium Grid can run tests on multiple machines, it was designed for running functional tests in parallel, not for simulating large-scale load testing. Performance testing tools like Apache JMeter and Gatling have built-in support for distributed testing.
6. Limited Reporting and Metrics
Selenium does not provide detailed performance metrics like response times, throughput, or server resource utilization. While you can measure the time taken for a specific interaction using `System.currentTimeMillis()` or other manual timing methods, Selenium does not provide out-of-the-box tools for detailed performance analysis. For proper performance testing, specialized tools offer built-in reporting capabilities such as response times for each request, latency, and resource consumption.
7. Inability to Simulate Real-World Traffic Patterns
Performance testing requires simulating real-world traffic patterns, which can include various types of user behavior, different load variations, and network latencies. Selenium is limited in this area, as it mainly automates user interactions in a predefined, linear manner. Tools designed for performance testing, such as Apache JMeter, can simulate complex traffic patterns, including random think times, varying user load, and network conditions like latency and bandwidth throttling.
8. Alternative Tools for Performance Testing
While Selenium is excellent for functional testing, there are other tools designed specifically for performance testing that provide features that Selenium lacks. Some popular performance testing tools include:
- Apache JMeter: A widely used open-source tool designed for load testing and performance measurement. JMeter can simulate hundreds or even thousands of users, making it ideal for testing performance under load.
- Gatling: A powerful load testing tool that can simulate complex user behaviors and generate detailed performance reports.
- LoadRunner: A comprehensive tool by Micro Focus that supports performance testing for web applications, mobile applications, and other systems.
- BlazeMeter: A cloud-based performance testing platform based on JMeter, which allows you to easily simulate a large volume of traffic from different regions.
9. When to Use Selenium for Performance Testing
Despite its limitations, Selenium can still play a role in performance testing in certain scenarios, such as:
- Small-Scale Tests: If you only need to simulate a small number of users or want to test basic user interactions like page load times or response times for specific actions, Selenium can be useful.
- Integration with Other Tools: You can integrate Selenium with performance testing tools like Apache JMeter or Gatling. For example, you can use Selenium for automating browser interactions and JMeter to handle the load simulation and performance metrics.
10. Conclusion
While Selenium is a powerful tool for functional testing, it has several limitations when it comes to performance testing. Selenium is not designed to simulate large-scale load, measure detailed performance metrics, or distribute tests across multiple machines. For accurate and reliable performance testing, it is recommended to use specialized tools like Apache JMeter, Gatling, or LoadRunner. However, Selenium can still be a valuable part of the performance testing process when combined with other tools or used for small-scale performance tests.
Using Selenium for Basic Performance Monitoring
While Selenium is not typically used for performance testing, it can be leveraged for basic performance monitoring in specific scenarios. Selenium allows you to automate browser interactions, and when combined with basic timing and logging techniques, it can provide insights into the performance of certain web page elements. This section explores how to use Selenium for basic performance monitoring, including measuring page load times, element interaction times, and basic performance metrics.
1. Measuring Page Load Time
One of the simplest forms of performance monitoring in Selenium is measuring how long it takes for a page to load. By recording the time before and after navigating to a URL, you can get an idea of the page's load performance.
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Start timing the page load
start_time = time.time()
# Open a webpage
driver.get("https://www.example.com")
# Calculate the page load time
load_time = time.time() - start_time
print(f"Page Load Time: {load_time} seconds")
# Close the browser
driver.quit()
In this example, the time taken to load the page is measured using Python's built-in `time` module. This simple technique can be used to monitor how long it takes for a page to fully load in a browser.
2. Measuring Element Interaction Time
Selenium can also be used to measure how long it takes to interact with specific elements on a page. For example, you can measure the time it takes to click a button, fill out a form, or retrieve data from a web element.
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a webpage
driver.get("https://www.example.com")
# Start timing the button click interaction
start_time = time.time()
# Find a button and click it
button = driver.find_element_by_id("submit")
button.click()
# Calculate the interaction time
interaction_time = time.time() - start_time
print(f"Button Click Interaction Time: {interaction_time} seconds")
# Close the browser
driver.quit()
In this example, the time to click a button is measured. This basic interaction time can help you monitor how responsive elements are on your web application. You can extend this to other types of interactions, such as form submissions or retrieving data from tables.
3. Monitoring Resource Usage (CPU and Memory)
While Selenium itself does not provide built-in tools for monitoring system resources like CPU and memory usage, you can use external libraries or operating system commands to monitor these metrics while the Selenium tests are running. For example, you could use Python's `psutil` library to track CPU and memory usage during Selenium interactions.
import psutil
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Start monitoring CPU and memory usage
cpu_usage_before = psutil.cpu_percent()
memory_usage_before = psutil.virtual_memory().percent
# Open a webpage
driver.get("https://www.example.com")
# Wait for the page to load
time.sleep(2)
# Monitor resource usage after loading the page
cpu_usage_after = psutil.cpu_percent()
memory_usage_after = psutil.virtual_memory().percent
# Calculate the change in resource usage
cpu_usage_change = cpu_usage_after - cpu_usage_before
memory_usage_change = memory_usage_after - memory_usage_before
print(f"CPU Usage Change: {cpu_usage_change}%")
print(f"Memory Usage Change: {memory_usage_change}%")
# Close the browser
driver.quit()
In this example, the CPU and memory usage are monitored before and after opening a webpage. The change in resource usage can be indicative of how efficiently the browser is handling the page load.
4. Monitoring Response Time of Web Elements
Another form of basic performance monitoring is measuring how long it takes for specific web elements to appear or become interactable on the page. For example, you can use Selenium’s explicit waits to time how long it takes for an element to become visible or clickable after a page load.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver"))
# Open a webpage
driver.get("https://www.example.com")
# Start timing the element visibility
start_time = time.time()
# Wait for an element to become visible
element = WebDriverWait(driver, 10).until(
EC.visibility_of_element_located((By.ID, "submit"))
)
# Calculate the time taken for the element to become visible
visibility_time = time.time() - start_time
print(f"Element Visibility Time: {visibility_time} seconds")
# Close the browser
driver.quit()
In this example, the time it takes for the submit button to become visible is measured. You can use a similar approach to track how long it takes for other elements to load or become interactable on the page.
5. Limitations of Selenium in Performance Monitoring
While Selenium can provide basic insights into the performance of specific web interactions, it has several limitations when it comes to more comprehensive performance monitoring:
- Limited Scalability: Selenium can only simulate one user interacting with the browser at a time. It is not designed to simulate large-scale traffic or measure system performance under load.
- No Built-in Resource Monitoring: Selenium does not provide tools for monitoring server-side resources, network latency, or throughput.
- High Resource Consumption: Running Selenium tests in real browsers can consume significant system resources, which can interfere with the accuracy of performance metrics.
6. Conclusion
Although Selenium is primarily a functional testing tool, it can be used for basic performance monitoring. By measuring load times, interaction times, and monitoring resource usage, you can gain valuable insights into the performance of web pages and elements. However, for more comprehensive performance testing, it is recommended to use dedicated performance testing tools like Apache JMeter, LoadRunner, or Gatling, which are designed for handling large-scale load simulations and detailed performance analysis.
Using Selenium for Basic Performance Monitoring
While Selenium is not typically used for performance testing, it can be leveraged for basic performance monitoring in specific scenarios. Selenium allows you to automate browser interactions, and when combined with basic timing and logging techniques, it can provide insights into the performance of certain web page elements. This section explores how to use Selenium for basic performance monitoring, including measuring page load times, element interaction times, and basic performance metrics.
1. Measuring Page Load Time
One of the simplest forms of performance monitoring in Selenium is measuring how long it takes for a page to load. By recording the time before and after navigating to a URL, you can get an idea of the page's load performance.
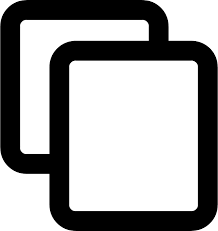
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Start timing the page load
start_time = time.time()
# Open a webpage
driver.get("https://www.example.com")
# Calculate the page load time
load_time = time.time() - start_time
print(f"Page Load Time: {load_time} seconds")
# Close the browser
driver.quit()
In this example, the time taken to load the page is measured using Python's built-in time
module. This simple technique can be used to monitor how long it takes for a page to fully load in a browser.
2. Measuring Element Interaction Time
Selenium can also be used to measure how long it takes to interact with specific elements on a page. For example, you can measure the time it takes to click a button, fill out a form, or retrieve data from a web element.
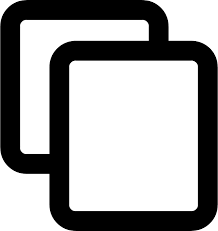
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a webpage
driver.get("https://www.example.com")
# Start timing the button click interaction
start_time = time.time()
# Find a button and click it
button = driver.find_element_by_id("submit")
button.click()
# Calculate the interaction time
interaction_time = time.time() - start_time
print(f"Button Click Interaction Time: {interaction_time} seconds")
# Close the browser
driver.quit()
In this example, the time to click a button is measured. This basic interaction time can help you monitor how responsive elements are on your web application. You can extend this to other types of interactions, such as form submissions or retrieving data from tables.
3. Monitoring Resource Usage (CPU and Memory)
While Selenium itself does not provide built-in tools for monitoring system resources like CPU and memory usage, you can use external libraries or operating system commands to monitor these metrics while the Selenium tests are running. For example, you could use Python's psutil
library to track CPU and memory usage during Selenium interactions.
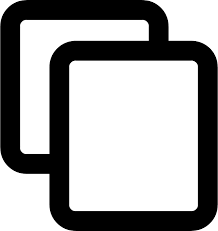
import psutil
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Start monitoring CPU and memory usage
cpu_usage_before = psutil.cpu_percent()
memory_usage_before = psutil.virtual_memory().percent
# Open a webpage
driver.get("https://www.example.com")
# Wait for the page to load
time.sleep(2)
# Monitor resource usage after loading the page
cpu_usage_after = psutil.cpu_percent()
memory_usage_after = psutil.virtual_memory().percent
# Calculate the change in resource usage
cpu_usage_change = cpu_usage_after - cpu_usage_before
memory_usage_change = memory_usage_after - memory_usage_before
print(f"CPU Usage Change: {cpu_usage_change}%")
print(f"Memory Usage Change: {memory_usage_change}%")
# Close the browser
driver.quit()
In this example, the CPU and memory usage are monitored before and after opening a webpage. The change in resource usage can be indicative of how efficiently the browser is handling the page load.
4. Monitoring Response Time of Web Elements
Another form of basic performance monitoring is measuring how long it takes for specific web elements to appear or become interactable on the page. For example, you can use Selenium’s explicit waits to time how long it takes for an element to become visible or clickable after a page load.
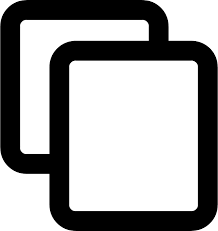
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver"))
# Open a webpage
driver.get("https://www.example.com")
# Start timing the element visibility
start_time = time.time()
# Wait for an element to become visible
element = WebDriverWait(driver, 10).until(
EC.visibility_of_element_located((By.ID, "submit"))
)
# Calculate the time taken for the element to become visible
visibility_time = time.time() - start_time
print(f"Element Visibility Time: {visibility_time} seconds")
# Close the browser
driver.quit()
In this example, the time it takes for the submit button to become visible is measured. You can use a similar approach to track how long it takes for other elements to load or become interactable on the page.
5. Limitations of Selenium in Performance Monitoring
While Selenium can provide basic insights into the performance of specific web interactions, it has several limitations when it comes to more comprehensive performance monitoring:
- Limited Scalability: Selenium can only simulate one user interacting with the browser at a time. It is not designed to simulate large-scale traffic or measure system performance under load.
- No Built-in Resource Monitoring: Selenium does not provide tools for monitoring server-side resources, network latency, or throughput.
- High Resource Consumption: Running Selenium tests in real browsers can consume significant system resources, which can interfere with the accuracy of performance metrics.
6. Conclusion
Although Selenium is primarily a functional testing tool, it can be used for basic performance monitoring. By measuring load times, interaction times, and monitoring resource usage, you can gain valuable insights into the performance of web pages and elements. However, for more comprehensive performance testing, it is recommended to use dedicated performance testing tools like Apache JMeter, LoadRunner, or Gatling, which are designed for handling large-scale load simulations and detailed performance analysis.
Integrating Selenium with Performance Tools (JMeter, Gatling)
Selenium is widely used for functional testing of web applications, but it can also be combined with performance testing tools like JMeter and Gatling for more comprehensive load and performance testing. By integrating Selenium with these tools, you can simulate real user interactions at scale and measure the performance of your web applications under load.
1. Integrating Selenium with JMeter
Apache JMeter is a popular open-source tool designed for load testing and performance testing of web applications. It can be integrated with Selenium to simulate real-world user interactions alongside performance monitoring. Here's how you can integrate Selenium with JMeter:
Steps to Integrate Selenium with JMeter
- Download and install JMeter from the official website.
- Install the Selenium WebDriver plugin for JMeter. This can be done by downloading the WebDriver plugin from the JMeter Plugins website and adding it to your JMeter's "lib/ext" folder.
- Create a new test plan in JMeter.
- Add a "Thread Group" to the test plan, which represents the number of virtual users.
- Add a "WebDriver Sampler" under the Thread Group, which will allow you to use Selenium scripts for browser automation.
- In the WebDriver Sampler, write your Selenium script (e.g., automating login, navigation, etc.) to simulate user interactions.
- Configure the JMeter settings for the number of threads (users) and ramp-up time.
- Run the test and analyze the performance metrics such as response time, throughput, and error rates.
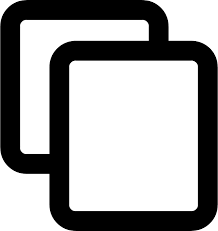
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
public class SeleniumJMeterExample {
public void runTest() {
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
// Perform actions like login or form submission
WebElement loginButton = driver.findElement(By.id("loginButton"));
loginButton.click();
// Add more actions as needed
driver.quit();
}
}
2. Integrating Selenium with Gatling
Gatling is another powerful tool for load testing and performance testing that can be integrated with Selenium for simulating user interactions. Unlike JMeter, Gatling is a high-performance tool that is particularly suited for testing applications under high load conditions. Here's how to integrate Selenium with Gatling:
Steps to Integrate Selenium with Gatling
- Download and install Gatling from the official website.
- Create a new project with Gatling using Maven or Gradle.
- Add the necessary dependencies for Selenium WebDriver in your Gatling project. For Maven, include the following in your
pom.xml
file: - Write your Selenium test script in a custom simulation class in Gatling.
- Use the
exec()
method in Gatling to simulate user interactions with Selenium. - Configure the number of virtual users and ramp-up time in Gatling's scenario definitions.
- Run the Gatling test and analyze the performance metrics generated by Gatling, such as response time, latency, and throughput.
org.seleniumhq.selenium
selenium-java
3.141.59
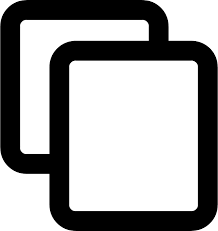
import io.gatling.core.Predef._
import io.gatling.selenium.Predef._
import org.openqa.selenium.WebDriver
import org.openqa.selenium.chrome.ChromeDriver
class SeleniumGatlingSimulation extends Simulation {
val driver: WebDriver = new ChromeDriver()
val scn = scenario("Selenium with Gatling")
.exec(
session => {
driver.get("https://www.example.com")
driver.findElementById("loginButton").click()
session
}
)
setUp(
scn.inject(atOnceUsers(10))
).protocols(
http.baseURL("https://www.example.com")
)
}
3. Benefits of Integrating Selenium with Performance Tools
- Realistic User Simulation: By using Selenium with JMeter or Gatling, you can simulate real user behavior (e.g., clicking buttons, filling forms) under load, which gives you a more realistic performance test scenario.
- Comprehensive Performance Metrics: Both JMeter and Gatling provide detailed performance metrics like response time, throughput, and error rates, which are essential for performance analysis.
- Scalability: Tools like JMeter and Gatling can handle a large number of virtual users, making them suitable for load and stress testing, which Selenium alone cannot handle at scale.
4. Limitations
- Resource Intensive: Combining Selenium with performance testing tools increases resource consumption, especially when running tests with a large number of virtual users.
- Complex Setup: Integrating Selenium with tools like JMeter or Gatling requires additional configuration and setup, which can be time-consuming, especially for beginners.
- Not Ideal for High-Load Simulations: While Selenium helps simulate real user interactions, it is not optimized for simulating heavy load at scale. Performance tools like JMeter and Gatling are better suited for that.
5. Conclusion
Integrating Selenium with performance tools like JMeter and Gatling allows you to simulate real user interactions under load, providing a more comprehensive view of your web application's performance. While these integrations can help you test the scalability and responsiveness of your application, it's important to keep in mind that Selenium is not designed to handle high-scale performance testing on its own. For large-scale load tests, it's better to rely on dedicated performance testing tools like JMeter and Gatling, with Selenium being used for simulating user behavior within those tests.
Common Selenium Errors and Fixes
Selenium is a powerful tool for web automation, but like any software, users may encounter errors during execution. Below are some common errors you might face while working with Selenium and their fixes.
1. SessionNotCreatedException: Session cannot be created
This error typically occurs when there is an issue with the browser or WebDriver version compatibility.
Fix:
- Ensure that the version of the WebDriver (e.g., ChromeDriver, GeckoDriver) matches the version of the browser you are using.
- Update both the browser and WebDriver to the latest versions to avoid compatibility issues.
- Check that the WebDriver path is correctly set up in your environment variables.
2. ElementNotFoundException: Unable to locate element
This error happens when Selenium is unable to find an element on the page based on the locator provided.
Fix:
- Ensure that the element exists on the page and is not hidden or inside an iframe.
- Use explicit waits to allow time for the element to appear if it's loaded dynamically (e.g., using
WebDriverWait
). - Double-check the locator strategy (e.g.,
id
,className
,XPath
) and make sure it is correct. - Use browser developer tools (e.g., inspect element) to verify the element's attributes and ensure the locator matches.
3. TimeoutException: Timed out after X seconds
This error occurs when Selenium is unable to complete an action within the specified time limit.
Fix:
- Increase the timeout duration using
WebDriverWait
to give the element more time to load. - Ensure the element is visible or clickable by checking if any popups or modals are obstructing the element.
- If you're working with dynamic content, ensure that the page is fully loaded before performing actions.
4. StaleElementReferenceException: Element is no longer attached to the DOM
This error occurs when an element is removed from the DOM or refreshed after it has been located by Selenium.
Fix:
- Re-locate the element before interacting with it again if the page has undergone a DOM update.
- Use
WebDriverWait
with expected conditions to wait for the element to be visible or clickable before interacting with it. - If an element is being reloaded dynamically, try waiting for the page to stabilize before interacting with elements.
5. NoSuchElementException: No such element
This error occurs when Selenium cannot find an element that matches the provided locator.
Fix:
- Verify the XPath or CSS selector used to locate the element is correct.
- Check if the element is visible or hidden behind other elements like modals or overlays.
- Use
findElements
instead offindElement
to check if the element exists before interacting with it.
6. WebDriverException: unknown error: cannot find Chrome binary
This error occurs when the Selenium WebDriver cannot find the browser binary (e.g., Chrome or Firefox) on your system.
Fix:
- Ensure that the browser is installed and the path to the browser is correctly set in your system environment variables.
- For Chrome, specify the path to the Chrome binary using
ChromeOptions
:
ChromeOptions options = new ChromeOptions();
options.setBinary("C:/path/to/chrome.exe");
WebDriver driver = new ChromeDriver(options); - If using a headless mode, ensure the headless configuration is set correctly.
7. InvalidArgumentException: Argument is invalid
This error can occur if an invalid argument is passed to a method, such as an incorrect URL format or invalid data.
Fix:
- Double-check the arguments passed to WebDriver methods, such as the URL or the value of input fields.
- Ensure that any URL passed to
driver.get()
starts withhttp://
orhttps://
. - Validate input parameters (e.g., form inputs, file paths) to ensure they are correctly formatted.
8. ElementClickInterceptedException: Element click intercepted
This occurs when an element is not clickable because another element (such as a popup or overlay) is covering it.
Fix:
- Use
WebDriverWait
withExpectedConditions.elementToBeClickable()
to wait for the element to become clickable. - Ensure that any overlays or modals are closed before attempting to click the element.
- If there is a fixed-position element (like a sticky header), use JavaScript to scroll the element into view using
arguments[0].scrollIntoView(true);
.
9. JavaScriptExecutorException: Failed to execute JavaScript
This error occurs when there is an issue while executing JavaScript in the browser through Selenium.
Fix:
- Ensure that the JavaScript code you are trying to execute is valid and error-free.
- Check that the element you are interacting with is accessible by JavaScript in the current page context.
- Verify that the WebDriver instance has been correctly initialized before executing JavaScript.
Conclusion
While working with Selenium, encountering errors is inevitable, but understanding the root causes and applying the appropriate fixes can greatly improve your automation process. Always ensure your environment is set up correctly, and use waits and proper locator strategies to handle dynamic and complex web pages effectively.
Debugging Techniques for Selenium Scripts
Debugging is an essential part of the software development process. When working with Selenium scripts, it’s important to have strategies in place to troubleshoot and identify issues. Below are some effective debugging techniques for Selenium scripts to help you identify and fix problems efficiently.
1. Use Explicit Waits
One of the most common issues in Selenium scripts is related to synchronization between the WebDriver and the web page. Elements may not be available immediately due to page load times or dynamic content. Using explicit waits can help resolve these issues.
How to Use:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Explicit waits tell Selenium to wait for an element to be visible, clickable, or present before proceeding with an action.
2. Take Screenshots on Failure
Taking screenshots during test execution can help you understand what went wrong when the script fails. Selenium provides functionality to take screenshots at any point during script execution.
How to Use:
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File("screenshot.png"));
This will capture a screenshot of the browser window and save it as "screenshot.png" on your local machine. You can take screenshots whenever an error occurs to capture the state of the application at that point in time.
3. Use Browser Developer Tools
Browser developer tools, such as Chrome DevTools, provide a wealth of information about the page's structure and behavior. You can use these tools to inspect elements, view network requests, and debug JavaScript errors.
How to Use:
- Right-click on a page element and choose
Inspect
to view the element’s properties and structure. - Use the
Console
tab to check for JavaScript errors or warnings that may affect your Selenium script. - The
Network
tab can help track network requests and responses to understand if the page is loading resources correctly.
Using developer tools alongside Selenium helps you identify and fix issues related to element loading or JavaScript execution.
4. Debug with Logging
Logging is an essential debugging technique that allows you to trace the execution flow of your Selenium scripts. You can log actions and errors to understand what is happening at each step of your script.
How to Use:
import java.util.logging.Logger;
Logger logger = Logger.getLogger("SeleniumDebug");
logger.info("Navigating to the website...");
driver.get("https://example.com");
Using logging helps you track the flow of execution, pinpoint failures, and understand the state of the application during the test. You can also log the values of variables to debug specific issues.
5. Use Debugger in IDE
Most Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse have built-in debuggers that allow you to step through your Selenium script line by line. This can help you pinpoint the exact line where the script fails and inspect the values of variables at that point.
How to Use:
- Set breakpoints in your code by clicking on the left margin next to a line of code in your IDE.
- Run the script in debug mode, which will stop at the breakpoints and allow you to step through the code.
- Inspect variables and the state of the application while stepping through the code.
Using the debugger in your IDE allows you to closely observe the flow of the script and identify bugs more efficiently.
6. Use Try-Catch Blocks
Try-catch blocks are useful for handling exceptions during script execution. By wrapping your test code in try-catch blocks, you can catch errors and handle them gracefully, making it easier to debug the issue and continue testing.
How to Use:
try {
WebElement element = driver.findElement(By.id("elementId"));
element.click();
} catch (NoSuchElementException e) {
System.out.println("Element not found: " + e.getMessage());
}
By using try-catch blocks, you can catch specific exceptions and print the relevant error messages, which can help you troubleshoot and identify issues quickly.
7. Check Browser and WebDriver Compatibility
Another common issue when debugging Selenium scripts is browser and WebDriver compatibility. Ensure that the WebDriver version you are using is compatible with the version of the browser installed on your system.
How to Fix:
- Update both your browser and the WebDriver to the latest versions to avoid compatibility issues.
- Verify that your WebDriver path is set correctly and that the right version is being used for the browser.
Incompatible versions of the browser and WebDriver can lead to issues such as session not being created or elements not being found.
8. Use Headless Mode for Faster Debugging
Running your Selenium tests in headless mode (without a UI) can speed up the debugging process. This is especially useful for running tests on continuous integration servers or in environments where a GUI is not available.
How to Use:
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless");
WebDriver driver = new ChromeDriver(options);
Headless mode helps you run tests faster and focuses on the test execution rather than the UI. However, it is essential to ensure that the elements behave the same way in headless mode as they do in a non-headless environment.
Conclusion
Debugging Selenium scripts effectively requires a combination of techniques, including using waits, logging, IDE debuggers, and browser developer tools. By implementing these strategies, you can identify issues faster, improve the reliability of your tests, and ensure the accuracy of your automation scripts.
Exception Handling in Selenium (TimeoutException, NoSuchElementException, etc.)
In Selenium, exceptions are common and can occur due to various reasons such as element not being found, timeouts, or browser issues. Proper exception handling is crucial for making your automation scripts more robust and reliable. This section will cover the most common exceptions in Selenium and how to handle them efficiently.
1. TimeoutException
The TimeoutException
occurs when an operation (such as locating an element or waiting for a condition) times out before completing. This typically happens when the page doesn't load within the specified time or an element is not found within the expected time.
How to Handle:
To handle a TimeoutException, you can use explicit waits to wait for an element to appear or a condition to be true before interacting with the element. This reduces the chances of timeouts by ensuring the element is ready for interaction.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
try {
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
element.click();
} catch (TimeoutException e) {
System.out.println("Element was not found within the timeout period: " + e.getMessage());
}
2. NoSuchElementException
The NoSuchElementException
occurs when Selenium can't find an element on the page. This can happen if the element is not present in the DOM or the locator is incorrect.
How to Handle:
To handle a NoSuchElementException, you should verify that the locator is correct and that the element exists in the DOM. You can also use try-catch
blocks to catch the exception and handle it gracefully.
try {
WebElement element = driver.findElement(By.id("elementId"));
element.click();
} catch (NoSuchElementException e) {
System.out.println("Element not found: " + e.getMessage());
}
3. StaleElementReferenceException
The StaleElementReferenceException
occurs when an element that was previously found is no longer attached to the DOM (the page has been refreshed, or the element has been removed or replaced). This can happen during dynamic page updates or AJAX calls.
How to Handle:
To handle this exception, you should re-locate the element before interacting with it again, as the reference to the element is no longer valid.
WebElement element = driver.findElement(By.id("elementId"));
try {
element.click();
} catch (StaleElementReferenceException e) {
element = driver.findElement(By.id("elementId")); // Re-locate the element
element.click();
}
4. ElementNotInteractableException
The ElementNotInteractableException
occurs when Selenium tries to interact with an element that is present in the DOM but cannot be interacted with, such as an element that is hidden or disabled.
How to Handle:
To handle this exception, you can first check if the element is visible and enabled before interacting with it. You can use isDisplayed()
and isEnabled()
methods to verify the element’s state.
WebElement element = driver.findElement(By.id("elementId"));
if (element.isDisplayed() && element.isEnabled()) {
element.click();
} else {
System.out.println("Element is not interactable");
}
5. NoSuchWindowException
The NoSuchWindowException
occurs when Selenium tries to switch to a window that does not exist. This can happen if the window has been closed or if the window handle is incorrect.
How to Handle:
To handle this exception, ensure that you are switching to the correct window handle and that the window you want to interact with is still open. Always check that the window handle exists before switching.
String currentWindow = driver.getWindowHandle();
try {
driver.switchTo().window("windowHandle");
// Perform actions on the new window
} catch (NoSuchWindowException e) {
System.out.println("Window not found: " + e.getMessage());
driver.switchTo().window(currentWindow); // Switch back to the original window
}
6. WebDriverException
The WebDriverException
is a generic exception that can occur for various reasons, such as browser or driver configuration issues, or if there is a problem with the WebDriver server.
How to Handle:
To handle WebDriverException, check if the WebDriver is properly configured and running, and verify that the browser and driver are compatible with each other. In case of an error, restart the WebDriver or reinitialize the browser session.
try {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
} catch (WebDriverException e) {
System.out.println("WebDriver error: " + e.getMessage());
// Handle the WebDriver issue or restart the driver
}
7. InvalidElementStateException
The InvalidElementStateException
occurs when an element is in an invalid state for the operation you are trying to perform. This can happen when trying to perform actions like clicking or typing on an element that is not in a valid state.
How to Handle:
To handle this exception, ensure that the element is enabled and in the right state before interacting with it. You can use the isEnabled()
and isSelected()
methods to verify the element’s state.
WebElement element = driver.findElement(By.id("elementId"));
if (element.isEnabled() && element.isSelected()) {
element.sendKeys("Text");
} else {
System.out.println("Element is not in a valid state for interaction.");
}
Conclusion
Exception handling in Selenium is crucial for building reliable and robust automation scripts. By understanding common exceptions like TimeoutException
, NoSuchElementException
, and StaleElementReferenceException
, and handling them effectively, you can ensure that your Selenium tests run smoothly and gracefully handle errors when they occur. Always use try-catch blocks and other strategies to mitigate the impact of these exceptions on your tests.
Running Selenium Tests in Headless Mode
Headless mode allows you to run Selenium tests without opening a graphical user interface (GUI) for the browser. This is particularly useful for running tests in environments where a display is not available, such as in continuous integration (CI) pipelines or when running tests on remote servers. Headless mode can also speed up tests since it eliminates the overhead of rendering the GUI.
What is Headless Mode?
Headless mode refers to running a browser without a visible GUI. While the browser’s functionality remains intact, it operates in the background without rendering the graphical interface. This mode is supported by browsers like Chrome, Firefox, and others, which provide headless versions of their regular browsers.
Benefits of Running Tests in Headless Mode
- Faster Execution: Since the browser doesn't need to render a GUI, tests can run faster, making it ideal for CI/CD environments.
- Less Resource Consumption: Without the need to display the UI, headless browsers consume fewer system resources, which is beneficial when running multiple tests or on limited hardware.
- Better for Automation: Headless mode is particularly useful for automating tests on remote servers or virtual machines that do not have a display attached.
How to Run Selenium Tests in Headless Mode
To run Selenium tests in headless mode, you need to configure the WebDriver to use a headless browser. Below are examples of setting up headless mode with popular browsers: Chrome and Firefox.
1. Running Tests in Headless Mode with Chrome
To run tests in headless mode with Chrome, you can use the ChromeOptions
class to set the headless argument.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class HeadlessTest {
public static void main(String[] args) {
// Set the path for ChromeDriver
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// Set Chrome options for headless mode
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless"); // Enable headless mode
options.addArguments("--disable-gpu"); // Disable GPU hardware acceleration (optional)
// Initialize WebDriver in headless mode
WebDriver driver = new ChromeDriver(options);
// Run your test case
driver.get("https://www.example.com");
System.out.println(driver.getTitle()); // Get title to verify the page loaded
// Close the browser after the test
driver.quit();
}
}
2. Running Tests in Headless Mode with Firefox
Similarly, to run tests in headless mode with Firefox, you can use the FirefoxOptions
class.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
public class HeadlessTest {
public static void main(String[] args) {
// Set the path for GeckoDriver
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Set Firefox options for headless mode
FirefoxOptions options = new FirefoxOptions();
options.addArguments("-headless"); // Enable headless mode
// Initialize WebDriver in headless mode
WebDriver driver = new FirefoxDriver(options);
// Run your test case
driver.get("https://www.example.com");
System.out.println(driver.getTitle()); // Get title to verify the page loaded
// Close the browser after the test
driver.quit();
}
}
Common Issues and Troubleshooting
While running tests in headless mode is convenient, there are a few common issues you may encounter:
- Element Visibility: Some elements may not be visible or interactable in headless mode, leading to issues when running tests. To resolve this, ensure that your test scripts interact with elements that are not dependent on a visible UI or add wait conditions to allow elements to load properly.
- Performance Differences: Headless browsers can sometimes behave differently from their full-browser counterparts. You may need to tweak your tests to account for these differences, especially if you rely on specific rendering behaviors or interactions.
- Browser-Specific Issues: Some features, such as video or animation, may not work the same in headless mode. Testing your application in a full browser environment may be necessary to catch these types of issues.
Conclusion
Running Selenium tests in headless mode is an effective way to speed up your tests and reduce resource consumption. It is especially useful for running tests on CI/CD servers, cloud environments, or virtual machines. By following the examples and best practices mentioned above, you can easily set up headless mode for Chrome and Firefox, and troubleshoot any common issues that may arise during testing.
Automating Captcha (Using External Services like OCR or AI)
Captcha is a security feature designed to differentiate between human users and automated bots by presenting challenges that are easy for humans to solve but difficult for machines. However, in some cases, you might need to automate captcha-solving for testing or other legitimate purposes. In this section, we explore how to automate captcha solving using external services like Optical Character Recognition (OCR) or Artificial Intelligence (AI).
Understanding Captcha Types
Captcha comes in different forms, each with unique challenges. The most common types include:
- Text-based Captcha: A distorted set of characters that the user must enter correctly.
- Image-based Captcha: A challenge that involves selecting specific images from a set of images.
- reCAPTCHA: A Google service that uses advanced risk analysis techniques and requires users to click a checkbox or solve an image puzzle.
- Invisible Captcha: A variation of reCAPTCHA that runs in the background and only triggers challenges when suspicious activity is detected.
Automating Captcha Solving Using OCR
Optical Character Recognition (OCR) can be used to automate the solving of text-based captchas. OCR technology analyzes images of text and converts them into machine-readable characters. Tools like Tesseract are commonly used to implement OCR in automation scripts.
Example: Solving a Text-based Captcha with Tesseract OCR
To automate captcha solving using Tesseract OCR, follow these steps:
- Capture the captcha image using Selenium.
- Use Tesseract to extract text from the image.
- Submit the extracted text to solve the captcha challenge.
from selenium import webdriver
from PIL import Image
import pytesseract
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a webpage with a captcha
driver.get("https://www.example.com/captcha")
# Capture the captcha image
captcha_image = driver.find_element_by_id("captcha_image")
captcha_image.screenshot("captcha.png")
# Use Tesseract to extract text from the image
captcha_text = pytesseract.image_to_string(Image.open("captcha.png"))
# Print the extracted captcha text
print("Captcha Text:", captcha_text)
# Find the input field and submit the extracted text
captcha_input = driver.find_element_by_id("captcha_input")
captcha_input.send_keys(captcha_text)
# Submit the form
captcha_input.submit()
# Wait for the page to load
time.sleep(3)
# Close the browser
driver.quit()
In this example, Selenium is used to capture the captcha image, and Tesseract OCR is employed to extract the text from the image. The extracted captcha text is then input into the form and submitted.
Automating Captcha Solving Using AI-based Services
For more complex captchas, such as image-based captchas or Google's reCAPTCHA, OCR may not be enough. In these cases, AI-based services like 2Captcha or Anti-Captcha can be used. These services employ human workers or AI models to solve captchas in real-time through an API.
Example: Using 2Captcha to Solve reCAPTCHA
2Captcha is an online service that allows you to automate solving of reCAPTCHAs and other captcha types. You can integrate 2Captcha with your Selenium tests as follows:
import requests
from selenium import webdriver
import time
# Set up the driver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open a webpage with reCAPTCHA
driver.get("https://www.example.com/recaptcha")
# Get the site key for reCAPTCHA
site_key = driver.find_element_by_class_name("g-recaptcha").get_attribute("data-sitekey")
# Use 2Captcha's API to solve reCAPTCHA
api_key = "your_2captcha_api_key"
url = f"http://2captcha.com/in.php?key={api_key}&method=userrecaptcha&googlekey={site_key}&pageurl={driver.current_url}"
response = requests.get(url)
captcha_id = response.text.split("|")[1]
# Get the solution from 2Captcha
solution_url = f"http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}"
solution_response = requests.get(solution_url)
captcha_solution = solution_response.text.split("|")[1]
# Use Selenium to input the captcha solution into the form
driver.execute_script("document.getElementById('g-recaptcha-response').innerHTML = arguments[0];", captcha_solution)
# Submit the form
submit_button = driver.find_element_by_id("submit_button")
submit_button.click()
# Wait for the page to load
time.sleep(3)
# Close the browser
driver.quit()
In this example, the 2Captcha API is used to solve Google's reCAPTCHA challenge. The solution is returned from the API and injected into the webpage using JavaScript. The form is then submitted using Selenium.
Considerations and Ethics
While automating captcha solving can be useful in testing scenarios, it's important to consider the ethical and legal implications. Many websites use captchas to protect against abuse and bots, and automating captcha solving may violate their terms of service. Always ensure that you are using captcha automation responsibly and only for legitimate purposes, such as testing or research.
Conclusion
Automating captcha solving can be achieved using OCR or AI-based services, depending on the complexity of the captcha. For basic text-based captchas, OCR tools like Tesseract can be used, while more advanced captchas like reCAPTCHA may require AI-based services like 2Captcha. Always be mindful of the ethical considerations when automating captcha solving, and ensure you're complying with the terms of service of the websites you're interacting with.
Testing Mobile Applications with Appium
Appium is an open-source, cross-platform automation tool that enables you to perform automated testing on mobile applications. It supports both Android and iOS platforms, making it an ideal solution for mobile app testing. Appium allows you to write tests using your preferred programming language, such as Java, Python, JavaScript, and C#, and interact with the mobile app's user interface (UI) to simulate user actions.
Setting Up Appium for Mobile Testing
Before you begin testing mobile applications with Appium, you need to set up the required components:
- Install Appium: You can install Appium globally using Node.js or use the Appium desktop client for GUI-based interaction. For Node.js installation, run the following command:
npm install -g appium
- Set up Android or iOS environment:
- For Android, ensure you have Android Studio installed along with the Android SDK.
- For iOS, you need Xcode and the necessary iOS simulators for testing.
- Install the Appium client for your programming language (e.g., Appium-Python-Client, Appium-Java-Client, etc.).
Appium Architecture
Appium has a client-server architecture consisting of the following components:
- Appium Server: The central server that receives requests from the client and communicates with the mobile device or emulator.
- Appium Clients: These are the test scripts written in various programming languages, such as Java, Python, or JavaScript, which interact with the Appium Server.
- Mobile Device: The physical mobile device or emulator/simulator where the mobile application is installed and tested.
Writing Tests Using Appium
Once the environment is set up, you can begin writing tests using Appium. Below is an example of a simple test written in Python that launches a mobile app and performs some actions:
from appium import webdriver
from time import sleep
# Set up the desired capabilities
desired_caps = {
"platformName": "Android",
"platformVersion": "11",
"deviceName": "Android Emulator",
"appPackage": "com.example.android",
"appActivity": ".MainActivity",
"automationName": "UiAutomator2",
"noReset": True
}
# Initialize the Appium driver
driver = webdriver.Remote('http://localhost:4723/wd/hub', desired_caps)
# Perform some actions on the mobile app
sleep(2)
driver.find_element_by_id("com.example.android:id/button1").click()
sleep(2)
driver.find_element_by_id("com.example.android:id/textview").send_keys("Hello Appium!")
# Close the app after the test
driver.quit()
In this example, we set up the desired capabilities, such as the platform name (Android), platform version, device name, and the app's package and activity. We then initialize the Appium driver, perform actions like clicking a button and typing into a text field, and finally close the app.
Locating Elements in Mobile Applications
In Appium, you can interact with mobile app elements using various locator strategies. Some common strategies include:
- ID:
driver.find_element_by_id("element_id")
- XPath:
driver.find_element_by_xpath("//android.widget.TextView[@text='Hello']")
- Class Name:
driver.find_element_by_class_name("android.widget.Button")
- Accessibility ID:
driver.find_element_by_accessibility_id("element_accessibility_id")
Running Tests on Real Devices and Emulators/Simulators
Appium allows you to run tests on both real devices and emulators/simulators:
- Emulators/Simulators: For Android, you can use Android Studio's AVD (Android Virtual Device) to create an emulator. For iOS, use Xcode's simulator.
- Real Devices: You can connect real Android or iOS devices via USB and run tests on them. Ensure that USB debugging is enabled on Android devices or that the devices are properly configured for iOS testing.
Appium Desired Capabilities
Desired capabilities are key-value pairs that provide information about the environment and app for testing. Some common desired capabilities include:
- platformName: The name of the mobile platform (e.g., "Android" or "iOS").
- platformVersion: The version of the platform (e.g., "11" for Android 11).
- deviceName: The name of the device or emulator (e.g., "Android Emulator").
- appPackage: The package name of the app (Android-specific).
- appActivity: The activity name of the app (Android-specific).
- automationName: The automation engine to use (e.g., "UiAutomator2" for Android).
Running Appium Tests in Parallel
Appium supports parallel test execution by allowing you to run tests on multiple devices simultaneously. To execute tests in parallel, you need to create multiple Appium server instances or use cloud-based testing services like Sauce Labs or BrowserStack that support parallel execution.
Conclusion
Appium is a powerful and flexible tool for automating mobile application testing across both Android and iOS platforms. With Appium, you can write tests in your preferred programming language, perform UI interactions, and run tests on both real devices and emulators/simulators. By integrating Appium into your testing workflow, you can ensure consistent app behavior and improve the overall quality of your mobile applications.
Automating Browser DevTools Protocol with Selenium
The Browser DevTools Protocol (BDP) is a set of APIs that allows you to interact with the internals of a web browser. It provides access to low-level browser features like network conditions, performance metrics, and DOM manipulations. Selenium, combined with the DevTools Protocol, allows you to control and automate these features for advanced browser testing and automation tasks.
What is the Browser DevTools Protocol?
The Browser DevTools Protocol is primarily used for interacting with Chrome and other Chromium-based browsers. It provides a set of commands to control the browser’s internals, such as:
- Intercepting network requests.
- Simulating network conditions (e.g., slow internet speeds).
- Manipulating the DOM directly.
- Accessing performance metrics like FPS and CPU usage.
- Capturing screenshots and videos of the page.
These capabilities make it an essential tool for performance testing and debugging web applications.
Setting Up Selenium with DevTools Protocol
Starting with Selenium 4, the DevTools Protocol is integrated into the Selenium WebDriver API, enabling you to take full advantage of its features. Here’s how to set it up:
- Install Selenium 4 or higher: Make sure you have the latest version of Selenium installed.
- Install a Chromium-based browser (e.g., Google Chrome or Microsoft Edge), as DevTools Protocol works with these browsers.
- Set up the WebDriver to interact with the DevTools Protocol.
Example: Using the DevTools Protocol with Selenium in Python
Here’s a basic example of how to interact with the DevTools Protocol in Selenium 4 using Python. The code demonstrates enabling DevTools, accessing network conditions, and simulating a slow 3G network:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.common.by import By
import time
# Set up Chrome options to use DevTools
chrome_options = Options()
chrome_options.add_argument("--remote-debugging-port=9222")
# Initialize the Chrome driver with DevTools enabled
driver = webdriver.Chrome(service=Service("path/to/chromedriver"), options=chrome_options)
# Access the DevTools interface
dev_tools = driver.execute_cdp_cmd("Network.enable", {})
# Simulate network conditions (e.g., slow 3G)
driver.execute_cdp_cmd("Network.emulateNetworkConditions", {
"offline": False,
"latency": 100, # 100ms latency
"downloadThroughput": 500 * 1024, # 500kbps
"uploadThroughput": 500 * 1024 # 500kbps
})
# Visit a website
driver.get("https://www.example.com")
# Perform some actions, such as waiting for elements to load
time.sleep(5)
# Take a screenshot to verify the page
driver.save_screenshot("screenshot.png")
# Close the browser
driver.quit()
This example demonstrates:
- Setting up Chrome to use the DevTools Protocol with the
--remote-debugging-port
argument. - Using the
execute_cdp_cmd
method to access network-related features of the DevTools Protocol, such asNetwork.enable
andNetwork.emulateNetworkConditions
. - Simulating slow network conditions by setting latency and throughput values.
- Capturing a screenshot after the page has loaded.
Common DevTools Protocol Commands
Here are some frequently used DevTools Protocol commands in Selenium:
- Network.enable: Enables network-related events like request interception and network conditions simulation.
- Network.emulateNetworkConditions: Simulates different network conditions (e.g., 3G, offline mode).
- Performance.enable: Enables performance-related events like page load times, FPS, and resource usage.
- DOM.enable: Enables DOM-related events, allowing you to interact with and manipulate the DOM.
- Page.captureScreenshot: Captures a screenshot of the current page.
Use Cases for Automating DevTools Protocol with Selenium
Integrating the DevTools Protocol with Selenium opens up a wide range of possibilities for advanced browser automation, including:
- Performance Testing: Track performance metrics like page load time, FPS, and CPU usage.
- Network Simulation: Test how your website behaves under different network conditions, such as slow 3G or offline mode.
- Intercepting and Modifying Network Requests: Mock API responses or test how your app behaves when a resource is not available.
- Automated Debugging: Capture logs, screenshots, and videos to troubleshoot issues in web applications.
Conclusion
Automating the Browser DevTools Protocol with Selenium opens up a new world of advanced testing and automation for web applications. By leveraging the DevTools Protocol, you can manipulate browser internals, test under different network conditions, and collect valuable performance data. This integration is especially useful for performance testing, network simulation, and debugging web applications.
Setting Up Selenium for Chrome, Firefox, Safari, and Edge
Setting up Selenium for different browsers involves installing the appropriate WebDriver for each browser and configuring it in your automation script. Below, we’ll cover the steps to set up Selenium for Chrome, Firefox, Safari, and Edge browsers.
Setting Up Selenium for Chrome
To use Selenium with Chrome, you need to install the ChromeDriver, which is a separate component that Selenium uses to interact with the Chrome browser. The steps are as follows:
- Install Selenium WebDriver via pip (if you haven’t already):
- Download ChromeDriver:
- Visit the official ChromeDriver download page.
- Download the version matching your Chrome browser version.
- Set up the WebDriver in your script:
pip install selenium
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
# Set up Chrome options
chrome_options = Options()
chrome_options.add_argument("--headless") # Run in headless mode (optional)
# Provide the path to the ChromeDriver
driver = webdriver.Chrome(service=Service("/path/to/chromedriver"), options=chrome_options)
# Open a webpage
driver.get("https://www.example.com")
print(driver.title)
# Close the browser
driver.quit()
Setting Up Selenium for Firefox
For Firefox, you’ll need to install the GeckoDriver. The setup process is similar to Chrome:
- Install Selenium WebDriver if you haven’t already (as shown above).
- Download GeckoDriver:
- Visit the GeckoDriver GitHub page.
- Download the version matching your Firefox browser version.
- Set up the WebDriver in your script:
from selenium import webdriver
from selenium.webdriver.firefox.service import Service
from selenium.webdriver.firefox.options import Options
# Set up Firefox options
firefox_options = Options()
firefox_options.add_argument("--headless") # Run in headless mode (optional)
# Provide the path to the GeckoDriver
driver = webdriver.Firefox(service=Service("/path/to/geckodriver"), options=firefox_options)
# Open a webpage
driver.get("https://www.example.com")
print(driver.title)
# Close the browser
driver.quit()
Setting Up Selenium for Safari
Safari requires a built-in WebDriver called SafariDriver, which is included with macOS. However, you need to enable the WebDriver support in Safari:
- Open Safari and go to Preferences → Advanced → Show Develop menu in menu bar.
- In the Develop menu, enable Allow Remote Automation.
- Install Selenium WebDriver as shown above.
- Set up the WebDriver in your script:
from selenium import webdriver
# Set up Safari WebDriver (No need for a separate driver path)
driver = webdriver.Safari()
# Open a webpage
driver.get("https://www.example.com")
print(driver.title)
# Close the browser
driver.quit()
Setting Up Selenium for Edge
For Microsoft Edge, you need to install the Edge WebDriver. The setup process is quite similar to the other browsers:
- Install Selenium WebDriver if you haven’t already.
- Download Edge WebDriver:
- Visit the Edge WebDriver page.
- Download the version matching your Edge browser version.
- Set up the WebDriver in your script:
from selenium import webdriver
from selenium.webdriver.edge.service import Service
from selenium.webdriver.edge.options import Options
# Set up Edge options
edge_options = Options()
edge_options.add_argument("--headless") # Run in headless mode (optional)
# Provide the path to the Edge WebDriver
driver = webdriver.Edge(service=Service("/path/to/msedgedriver"), options=edge_options)
# Open a webpage
driver.get("https://www.example.com")
print(driver.title)
# Close the browser
driver.quit()
Conclusion
Now you have a setup for Selenium with Chrome, Firefox, Safari, and Edge browsers. Each of these browsers requires a specific WebDriver (ChromeDriver, GeckoDriver, SafariDriver, and EdgeDriver) to interact with the browser. By following the setup steps outlined here, you can automate browser actions, test web applications, and run scripts across multiple browsers with ease.
Cross-Browser Compatibility Issues and Solutions
Ensuring that your web application works seamlessly across different browsers is essential for providing a consistent user experience. However, cross-browser compatibility issues are common due to the varying ways browsers interpret HTML, CSS, and JavaScript. In this section, we’ll explore common compatibility issues and solutions to resolve them.
Common Cross-Browser Compatibility Issues
Here are some of the most common issues developers face when working with cross-browser compatibility:
- CSS Differences: Different browsers have slightly different CSS rendering engines, leading to inconsistencies in layout, fonts, and other styling elements.
- JavaScript Behavior: JavaScript support can vary between browsers, especially with newer features like ES6 or ECMAScript. Some older browsers may not fully support these features.
- HTML Element Rendering: Some HTML elements may not be rendered the same way across browsers. For example, form elements like input fields may look different in Chrome and Firefox.
- Browser-Specific Bugs: Browsers sometimes have unique bugs that cause rendering or functionality issues with specific elements, like flexbox or grid layouts.
- Vendor Prefixes: Some CSS properties require vendor-specific prefixes (e.g., `-webkit-`, `-moz-`) for certain browsers to function properly.
Solutions for Cross-Browser Compatibility
Here are some solutions to address the issues mentioned above:
1. Use CSS Resets
Different browsers come with their default styling for elements like margins, padding, and fonts. To ensure consistency across browsers, use a CSS reset or normalize file to reset or normalize the default styling. Popular examples include:
2. Use Feature Detection (Not Browser Detection)
Instead of detecting browsers, use feature detection to ensure that your code only runs if the browser supports the necessary functionality. Libraries like Modernizr can help with this:
3. Use Vendor Prefixes for CSS Properties
Some CSS properties require vendor prefixes for cross-browser compatibility. Use tools like Autoprefixer to automatically add the necessary prefixes during build time:
/* Example of vendor-prefixed CSS for flexbox */
.container {
display: -webkit-flex; /* Safari */
display: -ms-flexbox; /* IE 10 */
display: flex; /* Modern browsers */
}
4. Test with Browser Developer Tools
All major browsers come with developer tools that allow you to inspect and debug your web page. Use these tools to test layout, JavaScript, and network performance across different browsers. The following tools are commonly used:
- Chrome DevTools: Chrome provides an extensive set of developer tools accessible via the F12 or Ctrl + Shift + I keys.
- Firefox Developer Tools: Firefox also has a powerful set of developer tools accessible via the F12 or Ctrl + Shift + I keys.
- Safari Web Inspector: Safari allows inspection through the Web Inspector, which can be accessed by enabling Developer mode in Safari preferences.
- Edge DevTools: Microsoft Edge also provides an extensive developer toolset, available via the F12 key.
5. Use Cross-Browser Testing Tools
For testing how your website looks and functions across different browsers, consider using cross-browser testing tools like:
6. Polyfills for Older Browsers
If you need to support older browsers that don’t support newer HTML5 or CSS3 features, use polyfills. Polyfills are JavaScript libraries that implement missing web features in older browsers. For example, you can use:
7. Avoid Browser-Specific Hacks
Don’t rely on browser-specific hacks to fix issues in one browser, as this could break your website in others. Instead, focus on using standardized solutions and feature detection to ensure cross-browser compatibility.
Conclusion
Cross-browser compatibility is a crucial aspect of web development. By using CSS resets, vendor prefixes, feature detection, and the tools mentioned above, you can significantly reduce the likelihood of compatibility issues and ensure a consistent user experience across different browsers.
Running Cross-Browser Tests in Parallel
Running cross-browser tests in parallel is an effective way to speed up the testing process and ensure your application works correctly across multiple browsers simultaneously. With the right tools and setup, you can run tests on multiple browsers at once, reducing the overall testing time and improving efficiency.
Why Run Cross-Browser Tests in Parallel?
Running tests in parallel helps achieve the following benefits:
- Faster Test Execution: Instead of sequentially running tests on each browser, you can run them at the same time, reducing the total testing time.
- Improved Test Coverage: Parallel testing allows you to test your web application across different browsers and devices simultaneously, ensuring it works consistently in all environments.
- Cost Efficiency: Running parallel tests saves resources by reducing infrastructure and execution time required for testing.
Setting Up Parallel Testing with Selenium
To run Selenium tests in parallel, you can use tools like Selenium Grid or third-party services like Sauce Labs or BrowserStack. Below are steps to set up parallel testing using Selenium Grid and a local environment with multiple browsers.
1. Setting Up Selenium Grid
Selenium Grid allows you to run tests on multiple machines (remote or local) and different browsers at the same time. Here’s how you can set up Selenium Grid:
Step 1: Install Selenium Server Standalone
Download the latest Selenium Server Standalone jar file from the official Selenium website.
Step 2: Start the Selenium Hub
To start the Selenium Hub, run the following command in your terminal or command prompt:
java -jar selenium-server-standalone-x.xx.x.jar -role hub
This will start the Selenium Hub, which acts as the central point for managing test execution across multiple nodes.
Step 3: Start the Selenium Nodes
Next, you need to start Selenium Nodes on different machines or browsers. You can run the following command to start a node that will connect to the Hub:
java -jar selenium-server-standalone-x.xx.x.jar -role node -hub http://localhost:4444/grid/register
This will start a node that connects to the Hub and will be ready to accept test requests. You can start multiple nodes for different browsers (e.g., Chrome, Firefox, Safari, etc.).
Step 4: Configure Your Test to Run in Parallel
To run tests on multiple browsers in parallel, configure your test script to use WebDriver instances for different browsers. Here is an example in Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class ParallelTest {
@Test
public void testOnChrome() {
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
driver.quit();
}
@Test
public void testOnFirefox() {
WebDriver driver = new FirefoxDriver();
driver.get("http://example.com");
driver.quit();
}
}
The above test will run on Chrome and Firefox in parallel, using Selenium Grid to manage the execution.
2. Using Third-Party Services (BrowserStack or Sauce Labs)
If you don’t want to set up Selenium Grid yourself, you can use third-party services like BrowserStack or Sauce Labs. These platforms allow you to run Selenium tests in parallel across different browsers and devices without maintaining your own grid infrastructure.
Example Using BrowserStack
To run tests in parallel with BrowserStack, you need to use their API. Here is an example of how to configure Selenium with BrowserStack for parallel testing:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class ParallelBrowserStackTest {
public static void main(String[] args) throws Exception {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("browser", "Chrome");
caps.setCapability("browser_version", "latest");
caps.setCapability("os", "Windows");
caps.setCapability("os_version", "10");
caps.setCapability("name", "Parallel Test Example");
WebDriver driver = new RemoteWebDriver(new URL("https://:@hub-cloud.browserstack.com/wd/hub"), caps);
driver.get("http://example.com");
driver.quit();
}
}
In the above example, replace
and
with your BrowserStack credentials. With BrowserStack, you can run tests in parallel on multiple browsers and devices.
Best Practices for Running Tests in Parallel
- Organize Tests by Browser: Group your tests based on the browser or device you want to test. This helps to organize and scale parallel testing.
- Limit Resource Usage: Ensure that your system or cloud infrastructure has enough resources (like CPU and memory) to run tests in parallel without performance degradation.
- Use Test Suites: Use test suites to group and organize tests in a way that allows for efficient parallel execution.
- Handle Browser-Specific Tests: Some tests may fail on specific browsers due to browser-specific issues. Handle these cases separately to ensure that other tests run smoothly in parallel.
- Monitor Test Results: Use a central dashboard or reporting system to monitor the results of all parallel tests. This helps in tracking test status and identifying any failing tests quickly.
Conclusion
Running cross-browser tests in parallel is an efficient way to ensure your web application works consistently across different browsers while saving time and resources. Whether you're using Selenium Grid or third-party services like BrowserStack or Sauce Labs, parallel testing helps to improve your testing process and reduce the time to market.
Running Selenium Tests on Cloud Services (BrowserStack, Sauce Labs)
Running Selenium tests on cloud services like BrowserStack and Sauce Labs allows you to test your web application across different browsers and devices without the need to maintain your own infrastructure. These cloud services provide a wide range of browser and device combinations, helping you ensure cross-browser compatibility and improve the overall quality of your application.
Why Use Cloud Services for Selenium Testing?
- Access to Real Browsers and Devices: Cloud services offer access to a variety of real-world browsers and mobile devices, ensuring that your tests are performed in real environments.
- No Infrastructure Maintenance: Cloud testing eliminates the need for managing and maintaining testing infrastructure, saving time and resources.
- Parallel Testing: Cloud services allow you to run multiple tests in parallel, reducing testing time and accelerating the release cycle.
- Cross-Browser and Cross-Platform Testing: These services support a wide range of browsers and operating systems, allowing you to test your web applications across platforms you might not have access to locally.
Setting Up Selenium Tests on BrowserStack
BrowserStack is a popular cloud service that allows you to run Selenium tests on a variety of real browsers and devices. To run Selenium tests on BrowserStack, follow these steps:
Step 1: Create a BrowserStack Account
Sign up for a BrowserStack account at BrowserStack and obtain your username and access key from the dashboard.
Step 2: Install Required Dependencies
Make sure you have the latest version of Selenium WebDriver and the BrowserStack integration libraries installed. For example, with Java, you can install the BrowserStack dependencies via Maven:
com.browserstack
browserstack
3.1.0
Step 3: Create Test Script
Below is an example of how to set up Selenium WebDriver to run tests on BrowserStack:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class BrowserStackTest {
public static void main(String[] args) throws Exception {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("browser", "Chrome");
caps.setCapability("browser_version", "latest");
caps.setCapability("os", "Windows");
caps.setCapability("os_version", "10");
caps.setCapability("name", "BrowserStack Test");
WebDriver driver = new RemoteWebDriver(new URL("https://:@hub-cloud.browserstack.com/wd/hub"), caps);
driver.get("http://example.com");
System.out.println(driver.getTitle());
driver.quit();
}
}
In this example, replace
and
with your BrowserStack credentials. The test will run on Chrome in a Windows 10 environment.
Step 4: Run Tests in Parallel
BrowserStack allows you to run parallel tests by specifying the number of parallel sessions in your test configuration. This can be done through your BrowserStack dashboard or in the script itself using the BrowserStack API for parallel test execution.
Setting Up Selenium Tests on Sauce Labs
Sauce Labs is another popular cloud-based testing platform. It provides real-time browser testing, mobile testing, and automated test execution. Here's how to set up Selenium tests on Sauce Labs:
Step 1: Create a Sauce Labs Account
Sign up for Sauce Labs at Sauce Labs and obtain your username and access key.
Step 2: Install Required Dependencies
To use Sauce Labs with Selenium, you'll need the Sauce Labs WebDriver bindings. For example, with Java, you can include the following dependency in your Maven configuration:
com.saucelabs
saucelabs
1.0.0
Step 3: Create Test Script
The following example demonstrates how to configure a Selenium WebDriver test using Sauce Labs:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class SauceLabsTest {
public static void main(String[] args) throws Exception {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("browserName", "chrome");
caps.setCapability("platform", "Windows 10");
caps.setCapability("version", "latest");
WebDriver driver = new RemoteWebDriver(new URL("https://:@ondemand.saucelabs.com:443/wd/hub"), caps);
driver.get("http://example.com");
System.out.println(driver.getTitle());
driver.quit();
}
}
Replace
and
with your Sauce Labs credentials. This test will run on Chrome in Windows 10.
Step 4: Run Tests in Parallel
With Sauce Labs, you can specify the number of parallel tests you want to run via their dashboard or API. This helps you optimize test execution time by running multiple tests simultaneously on different browsers and platforms.
Best Practices for Cloud Testing
- Use Real Browsers and Devices: Ensure that you're testing on real devices and browsers to get accurate results. Both BrowserStack and Sauce Labs offer real-device cloud testing.
- Enable Parallel Execution: Take advantage of cloud services' parallel execution capabilities to speed up your testing process.
- Monitor Test Results: Use the dashboards provided by the cloud services to track the status of your tests, monitor performance, and debug failures.
- Optimize Test Configurations: Configure your tests to run in different environments, browsers, and devices to ensure broad compatibility.
Conclusion
Running Selenium tests on cloud services like BrowserStack and Sauce Labs is an efficient way to ensure your web application performs well across different browsers and devices. By leveraging parallel testing and real-device environments, you can improve the speed and accuracy of your testing process while saving on infrastructure costs.
Advantages of Cloud-Based Testing Platforms
Cloud-based testing platforms offer numerous benefits over traditional on-premise testing setups. These platforms allow teams to run tests on a wide range of devices, browsers, and operating systems without the need to maintain a large infrastructure. Below, we explore the key advantages of using cloud-based testing for your Selenium tests.
1. Access to a Wide Range of Devices and Browsers
Cloud-based testing platforms like BrowserStack, Sauce Labs, and others provide access to real browsers, devices, and operating systems. This allows you to test your application across multiple environments without the need to set up and maintain physical machines for each configuration. This broad compatibility ensures that your application works for all users, regardless of their device or browser choice.
2. No Need for Infrastructure Maintenance
Setting up a local testing infrastructure requires buying, configuring, and maintaining various devices, browsers, and operating systems. With cloud-based platforms, all of this is handled by the service provider. You can run tests without worrying about the overhead of maintaining test machines, which saves both time and resources.
3. Parallel Test Execution
Cloud-based testing platforms allow you to run multiple tests in parallel on different machines. This significantly reduces the amount of time needed to complete tests, especially when testing across different browsers and devices. Parallel execution speeds up the feedback loop, helping you catch issues early and deliver updates faster.
4. Scalability and Flexibility
Cloud services offer a scalable solution to your testing needs. Whether you need to run a few tests or hundreds of tests simultaneously, cloud platforms can easily scale up or down based on your requirements. You don’t need to invest in additional hardware or worry about capacity planning. This flexibility makes it easy to adapt to changing needs in your testing process.
5. Real-Time Collaboration
Cloud-based testing platforms provide real-time collaboration capabilities. Teams can view test results, share logs, and debug issues collaboratively, even if they are working remotely. This ensures that everyone on the team is on the same page and can quickly address any issues that arise during testing.
6. Access to Latest Browser Versions and Updates
Cloud-based platforms ensure that you always have access to the latest browser versions and updates. This is crucial for testing the latest features and ensuring that your application remains compatible with the newest browser releases. You don’t have to worry about manually updating your test environments or missing out on important updates.
7. Cost-Effective
Maintaining an on-premise testing infrastructure can be expensive, especially when considering the cost of hardware, software licenses, and maintenance. Cloud-based platforms eliminate these costs by offering pay-as-you-go pricing models. This makes them a more cost-effective solution, especially for smaller teams or startups that don’t have the budget for extensive testing infrastructure.
8. Improved Test Coverage
Cloud platforms allow you to test on a variety of devices and browsers that you might not have access to locally. This increases test coverage and ensures that your application is compatible with a larger audience. You can test on mobile devices, tablets, and desktops, ensuring that your web application delivers a seamless experience across all platforms.
9. Easy Integration with CI/CD Pipelines
Cloud-based testing platforms integrate easily with Continuous Integration (CI) and Continuous Deployment (CD) pipelines. This allows you to automatically run Selenium tests as part of your build process, ensuring that new features or bug fixes don’t introduce regressions. Integration with popular CI/CD tools like Jenkins, GitHub Actions, and GitLab CI makes it easy to incorporate automated testing into your development workflow.
10. Detailed Reporting and Analytics
Cloud platforms provide detailed reports and analytics, making it easy to track test results, identify trends, and pinpoint areas for improvement. These reports can include screenshots, videos of test sessions, logs, and performance metrics. This data is invaluable for debugging and optimizing your application.
Conclusion
Cloud-based testing platforms offer a range of advantages that make them an attractive choice for teams looking to streamline their testing process. From the ability to run tests across a wide variety of devices and browsers to cost savings and real-time collaboration, these platforms provide the flexibility and scalability needed to optimize your testing efforts and deliver high-quality applications faster.
Setting Up Cloud Tests with Selenium
Cloud-based testing allows you to run Selenium tests on real browsers and devices hosted in the cloud, providing access to a wide variety of configurations without the need for a local setup. Popular platforms like BrowserStack, Sauce Labs, and CrossBrowserTesting make it easy to run Selenium tests on different environments. This section outlines how to set up cloud tests using Selenium and these platforms.
1. Choosing a Cloud-Based Testing Platform
Before you begin setting up cloud tests, you need to select a cloud-based testing platform. Popular options include:
- BrowserStack: Provides access to real devices and browsers for manual and automated testing.
- Sauce Labs: Offers cross-browser testing in the cloud with real-time testing and parallel execution.
- CrossBrowserTesting: Another cloud-based platform offering a variety of devices and browsers for Selenium testing.
Each platform has its own setup process, but the general steps to integrate Selenium with cloud platforms are similar.
2. Creating an Account on a Cloud Platform
To get started with cloud testing, sign up for an account on the chosen platform. After signing up, you will typically receive an API key or credentials that are used to authenticate your tests on the cloud service.
3. Configuring Selenium WebDriver for Cloud Testing
Once you have your credentials, you will need to configure Selenium WebDriver to run tests on the cloud platform. Below is an example of how to set up Selenium with BrowserStack.
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
# BrowserStack credentials
username = "your_browserstack_username"
access_key = "your_browserstack_access_key"
# Desired capabilities for BrowserStack
desired_cap = {
'browser': 'Chrome',
'browser_version': 'latest',
'os': 'Windows',
'os_version': '10',
'name': 'Selenium Test',
'build': '1.0',
'project': 'Cloud Testing Project'
}
# URL for BrowserStack's Selenium hub
url = f"https://{username}:{access_key}@hub-cloud.browserstack.com/wd/hub"
# Initialize the WebDriver with the desired capabilities
driver = webdriver.Remote(
command_executor=url,
desired_capabilities=desired_cap
)
# Run your test case (example)
driver.get("https://www.example.com")
print(driver.title)
# Close the browser
driver.quit()
This example shows how to use Selenium with BrowserStack's cloud service. The desired capabilities define the browser, version, and operating system for the test. You can configure this for other platforms like Sauce Labs or CrossBrowserTesting by changing the URL and desired capabilities accordingly.
4. Running Tests in Parallel
Cloud platforms like BrowserStack and Sauce Labs allow you to run multiple Selenium tests in parallel. This is particularly useful when testing across multiple browsers and devices simultaneously, significantly speeding up test execution.
For parallel testing, you can use tools like TestNG (for Java) or pytest (for Python) to run multiple tests in parallel. These tools integrate easily with cloud-based platforms and allow for efficient parallel test execution.
5. Integrating with CI/CD Pipelines
Cloud-based testing platforms integrate seamlessly with Continuous Integration (CI) and Continuous Deployment (CD) tools, ensuring automated tests run every time code is pushed to the repository. Popular CI/CD tools like Jenkins, GitHub Actions, and GitLab CI can easily integrate with Selenium running on the cloud.
Here's an example of setting up cloud-based Selenium tests in a CI pipeline using GitHub Actions:
name: Selenium Tests on BrowserStack
on: [push]
jobs:
selenium-tests:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install selenium
- name: Run Selenium tests
run: |
python run_tests.py
env:
BROWSERSTACK_USERNAME: ${{ secrets.BROWSERSTACK_USERNAME }}
BROWSERSTACK_ACCESS_KEY: ${{ secrets.BROWSERSTACK_ACCESS_KEY }}
In this example, the GitHub Actions workflow installs the necessary dependencies, checks out the code, and runs the Selenium tests using the BrowserStack credentials stored in GitHub Secrets.
6. Viewing Results and Logs
After running the tests, cloud-based platforms provide detailed logs, screenshots, and videos of your Selenium tests. This makes it easy to debug issues, understand failures, and optimize your application. You can view these results directly on the cloud platform's dashboard.
7. Conclusion
Setting up cloud tests with Selenium is a straightforward process that can significantly improve your testing workflow. By using cloud-based testing platforms like BrowserStack, Sauce Labs, or CrossBrowserTesting, you can run tests on real devices, scale your tests in parallel, and easily integrate with CI/CD pipelines. This allows you to ensure that your web application works across different browsers and devices without the need to maintain a local infrastructure.
Writing Maintainable and Reusable Test Scripts
Writing maintainable and reusable test scripts is crucial for ensuring the long-term success and scalability of your automated testing efforts. As your application evolves, so should your test scripts. This section covers best practices for writing test scripts that are easy to maintain, update, and reuse across different test cases.
1. Keep Tests Simple and Focused
Each test script should focus on a single unit of functionality. This makes it easier to understand, maintain, and debug. When test scripts are too complex, it becomes difficult to pinpoint issues, and it can increase the maintenance overhead. Ideally, each test should verify one behavior or feature of your application.
2. Use Page Object Model (POM)
The Page Object Model (POM) is a design pattern that helps in creating maintainable and reusable Selenium scripts. It separates the test logic from the user interface (UI) elements, allowing you to create a model for each page or component in your application. This approach makes the test scripts more modular and easier to manage.
Here is an example of a Page Object Model in Python:
# page_object.py
from selenium.webdriver.common.by import By
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, "username")
self.password_field = (By.ID, "password")
self.login_button = (By.ID, "login")
def enter_username(self, username):
self.driver.find_element(*self.username_field).send_keys(username)
def enter_password(self, password):
self.driver.find_element(*self.password_field).send_keys(password)
def click_login(self):
self.driver.find_element(*self.login_button).click()
In this example, the LoginPage
class models the login page and provides methods to interact with the page elements. This allows the test scripts to focus on the logic and actions, while the page objects encapsulate the specifics of interacting with the UI.
3. Avoid Hard-Coding Values
Hard-coding values like URLs, usernames, and passwords directly in test scripts can lead to issues when those values change. Instead, externalize such values in configuration files or environment variables. This makes your scripts more flexible and easier to update.
For example, you can use a configuration file or environment variables for sensitive data:
import os
# Use environment variables for sensitive data
url = os.getenv('BASE_URL', 'https://defaulturl.com')
username = os.getenv('USER_NAME', 'defaultuser')
password = os.getenv('USER_PASSWORD', 'defaultpass')
# Accessing the values
print(f"URL: {url}, Username: {username}, Password: {password}")
This way, your test scripts are more flexible and secure by not exposing sensitive data directly within the code.
4. Use Assertions for Validation
Assertions are vital for confirming that your application behaves as expected. When writing reusable test scripts, ensure that assertions are not hard-coded but instead based on expected outcomes derived from the application’s state or context.
For example, in Python, you can use assert
statements to check page content:
# Example of an assertion
assert "Welcome" in driver.page_source
Using assertions makes it easy to validate conditions in your test scripts without repetitive code. This improves readability and helps ensure your tests cover all scenarios.
5. Modularize and Reuse Code
One of the core principles of maintainable and reusable test scripts is modularization. Break your test scripts down into smaller, reusable functions or methods. This avoids code duplication and makes it easier to update tests when the application changes.
For example, you can create utility functions for common tasks like login, data entry, and navigation:
def login(driver, username, password):
login_page = LoginPage(driver)
login_page.enter_username(username)
login_page.enter_password(password)
login_page.click_login()
def navigate_to_dashboard(driver):
driver.find_element(By.ID, "dashboard").click()
By modularizing common actions like login and navigation, you make the test scripts more concise and easier to maintain.
6. Use Descriptive Naming Conventions
Use clear and descriptive names for your test functions, variables, and classes. This will help others (or your future self) understand the purpose of the test without having to read through every line of code.
For example:
def test_login_with_valid_credentials(driver):
login(driver, "valid_user", "valid_password")
assert "Dashboard" in driver.page_source
def test_login_with_invalid_credentials(driver):
login(driver, "invalid_user", "invalid_password")
assert "Invalid credentials" in driver.page_source
Descriptive names like test_login_with_valid_credentials
make it clear what each test is verifying, reducing the time spent understanding the tests.
7. Leverage Test Data Files
Test data should be stored separately from the test logic, ideally in external files like CSV, JSON, or Excel sheets. This allows for easier updates to the test data without having to modify the test scripts themselves.
For example, you can use a CSV file to store login credentials:
import csv
# Reading test data from a CSV file
with open('test_data.csv', mode='r') as file:
reader = csv.reader(file)
for row in reader:
username, password = row
login(driver, username, password)
assert "Dashboard" in driver.page_source
By storing test data separately, you make it easier to reuse the data across multiple tests, improving maintainability.
8. Conclusion
By following these best practices for writing maintainable and reusable test scripts, you can ensure that your Selenium tests are scalable, easy to update, and simple to debug. The goal is to create modular, flexible test scripts that can be reused across different test cases and adapted to changes in the application with minimal effort.
Page Object Model (POM) Design Pattern
The Page Object Model (POM) is a design pattern used to create object-oriented classes that serve as an interface to interact with the elements of a web page. This pattern helps in creating maintainable, reusable, and scalable test scripts by separating the test logic from the UI elements. The POM design pattern is widely used in Selenium automation testing to improve the readability and maintainability of the test code.
1. Benefits of Using POM
Here are the key advantages of using the Page Object Model in Selenium:
- Separation of Concerns: By separating the UI interaction and test logic, the Page Object Model makes it easier to maintain both the UI and test scripts independently.
- Reusability: Page objects are reusable across multiple test cases, reducing code duplication and making the tests more modular.
- Scalability: As your test suite grows, POM allows you to scale efficiently by reusing page objects in different tests.
- Ease of Maintenance: If the UI elements change, you only need to update the page object class without affecting the test scripts that use it.
- Improved Readability: Tests become more readable because the test logic is separated from the UI actions, and the test scripts focus only on test execution.
2. Page Object Model Structure
The Page Object Model involves creating a separate class for each page of the application you are testing. Each class should represent a specific page and provide methods to interact with the elements on that page. These methods can perform actions (click, input, etc.) or return data (text, values, etc.) from the page.
A typical structure of a POM-based project might look like this:
src/
├── pages/
│ ├── HomePage.py
│ ├── LoginPage.py
│ └── DashboardPage.py
├── tests/
│ ├── test_login.py
│ └── test_dashboard.py
├── drivers/
│ └── webdriver.py
└── utilities/
└── config.py
3. Example of Page Object Model
Let’s look at an example of how to implement the Page Object Model using Selenium with Python:
# login_page.py (Page Object)
from selenium.webdriver.common.by import By
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, "username")
self.password_field = (By.ID, "password")
self.login_button = (By.ID, "login")
def enter_username(self, username):
self.driver.find_element(*self.username_field).send_keys(username)
def enter_password(self, password):
self.driver.find_element(*self.password_field).send_keys(password)
def click_login(self):
self.driver.find_element(*self.login_button).click()
# test_login.py (Test Script)
from selenium import webdriver
from login_page import LoginPage
def test_login():
driver = webdriver.Chrome()
driver.get("http://example.com/login")
login_page = LoginPage(driver)
login_page.enter_username("testuser")
login_page.enter_password("password123")
login_page.click_login()
assert "Dashboard" in driver.page_source
driver.quit()
In this example, the LoginPage
class represents the login page and provides methods to interact with the username field, password field, and login button. The test script uses these methods to perform actions and verify the login behavior.
4. Best Practices for Implementing POM
To implement the Page Object Model effectively, follow these best practices:
- Keep Page Object Classes Small: Each page object class should represent a single page or component. Avoid cramming too many actions into one class.
- Use Descriptive Names: Name your methods and variables in a descriptive manner to make the code more readable. For example,
enter_username
is more descriptive thaninput_data
. - Use Getters and Setters: For elements that require reading values or modifying them, use getter and setter methods for better encapsulation.
- Don’t Overload Page Objects: Avoid adding business logic or complex assertions in the page object classes. Keep them focused on interacting with the web elements.
5. Combining POM with Other Design Patterns
Although POM is a powerful design pattern, it can be combined with other design patterns to further enhance the maintainability and reusability of your test scripts. Some common combinations include:
- Factory Pattern: Use the Factory Pattern to create instances of page objects dynamically based on the test requirements.
- Singleton Pattern: Use the Singleton Pattern to ensure that only one instance of the WebDriver is created during the test execution, optimizing test speed.
- Data-Driven Testing: Combine POM with data-driven testing by parameterizing tests and feeding data from external sources (e.g., CSV, Excel).
6. Conclusion
The Page Object Model (POM) is an essential design pattern in Selenium that promotes maintainability, reusability, and scalability of test scripts. By following the principles of POM, you can create clear, modular, and easy-to-maintain test code that can handle changes in the application’s UI with minimal impact on your tests. Whether you're working on a small project or large-scale automation efforts, POM is a critical design pattern to ensure long-term success in Selenium-based testing.
Data-Driven Testing with External Files (CSV, Excel, JSON)
Data-driven testing is a testing methodology in which the test data is externalized from the test scripts and stored in external files such as CSV, Excel, or JSON. This approach allows you to execute the same test case multiple times with different sets of input data. In Selenium, data-driven testing can be achieved by reading test data from external files and using it to drive test execution. This helps in improving test coverage and makes the test scripts more maintainable and reusable.
1. Benefits of Data-Driven Testing
Data-driven testing provides numerous advantages, including:
- Reusability: The same test logic can be reused with different sets of input data, reducing the need for duplicating test scripts.
- Improved Test Coverage: By testing the same functionality with different data, you ensure a more comprehensive test coverage.
- Ease of Maintenance: Test data can be updated independently of test scripts, making it easier to modify test scenarios without changing the code.
- Separation of Test Logic and Test Data: Test data is kept separate from the test logic, making the tests more modular and easier to manage.
2. Types of External Files for Data-Driven Testing
There are several types of external files that you can use for data-driven testing:
- CSV (Comma-Separated Values): A simple text file format where each line of the file represents a test case, with data values separated by commas.
- Excel (XLSX): A spreadsheet file format that allows structured storage of test data with rows and columns.
- JSON (JavaScript Object Notation): A lightweight data format that is easy to read and write, commonly used for test data storage in a structured format.
3. Example: Data-Driven Testing with CSV File
In this example, we will use a CSV file to drive our Selenium tests. The CSV file will contain login credentials (username and password), which will be used in the test script.
CSV File Example (login_data.csv):
username,password
testuser1,password123
testuser2,password456
testuser3,password789
Selenium Test Script in Python:
import csv
from selenium import webdriver
from selenium.webdriver.common.by import By
# Function to read data from CSV
def read_csv(file_path):
data = []
with open(file_path, mode='r') as file:
csv_reader = csv.reader(file)
next(csv_reader) # Skip header
for row in csv_reader:
data.append(row)
return data
# Test function to perform login
def test_login(username, password):
driver = webdriver.Chrome()
driver.get("http://example.com/login")
driver.find_element(By.ID, "username").send_keys(username)
driver.find_element(By.ID, "password").send_keys(password)
driver.find_element(By.ID, "login").click()
# Add assertion to verify successful login
assert "Dashboard" in driver.page_source
driver.quit()
# Reading data from CSV and executing test for each set of credentials
login_data = read_csv('login_data.csv')
for data in login_data:
test_login(data[0], data[1])
In this script, the read_csv
function reads login credentials from the CSV file, and the test_login
function uses those credentials to log in and verify the login behavior.
4. Example: Data-Driven Testing with Excel File
In this example, we will use an Excel file to drive our Selenium tests. Python's openpyxl
library is used to read the data from the Excel file.
Excel File Example (login_data.xlsx):
| username | password |
|-----------|------------|
| testuser1 | password123|
| testuser2 | password456|
| testuser3 | password789|
Selenium Test Script in Python:
from openpyxl import load_workbook
from selenium import webdriver
from selenium.webdriver.common.by import By
# Function to read data from Excel file
def read_excel(file_path):
workbook = load_workbook(file_path)
sheet = workbook.active
data = []
for row in sheet.iter_rows(min_row=2, values_only=True): # Skip header row
data.append(row)
return data
# Test function to perform login
def test_login(username, password):
driver = webdriver.Chrome()
driver.get("http://example.com/login")
driver.find_element(By.ID, "username").send_keys(username)
driver.find_element(By.ID, "password").send_keys(password)
driver.find_element(By.ID, "login").click()
# Add assertion to verify successful login
assert "Dashboard" in driver.page_source
driver.quit()
# Reading data from Excel and executing test for each set of credentials
login_data = read_excel('login_data.xlsx')
for data in login_data:
test_login(data[0], data[1])
In this script, the read_excel
function reads login credentials from the Excel file, and the test_login
function uses those credentials to log in and verify the login behavior.
5. Example: Data-Driven Testing with JSON File
In this example, we will use a JSON file to drive our Selenium tests. JSON is a popular format for storing structured data.
JSON File Example (login_data.json):
[
{"username": "testuser1", "password": "password123"},
{"username": "testuser2", "password": "password456"},
{"username": "testuser3", "password": "password789"}
]
Selenium Test Script in Python:
import json
from selenium import webdriver
from selenium.webdriver.common.by import By
# Function to read data from JSON file
def read_json(file_path):
with open(file_path, 'r') as file:
data = json.load(file)
return data
# Test function to perform login
def test_login(username, password):
driver = webdriver.Chrome()
driver.get("http://example.com/login")
driver.find_element(By.ID, "username").send_keys(username)
driver.find_element(By.ID, "password").send_keys(password)
driver.find_element(By.ID, "login").click()
# Add assertion to verify successful login
assert "Dashboard" in driver.page_source
driver.quit()
# Reading data from JSON and executing test for each set of credentials
login_data = read_json('login_data.json')
for data in login_data:
test_login(data['username'], data['password'])
In this script, the read_json
function reads login credentials from the JSON file, and the test_login
function uses those credentials to log in and verify the login behavior.
6. Conclusion
Data-driven testing is a powerful technique for improving test coverage and reusability. By externalizing test data into files such as CSV, Excel, or JSON, you can execute the same tests with different data sets, making your tests more efficient and effective. Selenium, combined with data-driven testing, allows you to automate complex web applications with various input combinations, ensuring that your application functions correctly under different scenarios.
Automating Real-Time Web Applications (WebSocket Testing)
Real-time web applications, such as chat applications, online games, or live updates, rely on WebSockets to maintain an open connection between the client and the server. Unlike traditional HTTP requests, WebSockets allow for two-way communication, enabling real-time data transfer between the client and server. Automating the testing of these WebSocket-based applications involves simulating WebSocket connections and ensuring that messages are sent and received in real-time, which is crucial for testing the responsiveness and stability of the application.
1. Understanding WebSockets
WebSockets provide a full-duplex communication channel that operates over a single TCP connection. Once established, a WebSocket connection remains open, allowing both the client and server to send messages at any time. This is different from traditional HTTP requests, where the client sends a request to the server and waits for a response. WebSocket is commonly used in applications that require real-time data exchange, such as:
- Chat applications
- Live sports updates
- Stock market tracking
- Online multiplayer games
2. Challenges in WebSocket Testing
Testing WebSocket connections can be complex due to the real-time and continuous nature of communication. Some of the challenges include:
- Simulating multiple WebSocket connections: Testing a WebSocket server with multiple clients requires simulating concurrent connections and message exchanges.
- Verifying message delivery: Ensuring that messages are delivered to the correct clients in the right order can be challenging in real-time scenarios.
- Performance testing: Ensuring that the WebSocket connection can handle a large number of concurrent clients without performance degradation.
- Error handling: Ensuring that the WebSocket server handles errors (e.g., disconnections, timeouts) gracefully.
3. Tools for WebSocket Testing
There are several tools available for automating WebSocket testing. Some of the common tools include:
- Selenium with WebSocket Support: Selenium WebDriver does not have built-in WebSocket support, but it can be extended using third-party libraries or custom JavaScript to interact with WebSocket connections.
- WebSocket Clients: Tools like WebSocket.org Echo Test can be used to manually connect to a WebSocket server and monitor the communication.
- Browser Developer Tools: Browser dev tools (e.g., Chrome DevTools) allow you to inspect WebSocket messages and connections in real-time.
- JMeter: Apache JMeter has WebSocket plugins that allow you to automate WebSocket tests by simulating multiple WebSocket connections and exchanging messages.
- Artillery: A modern, powerful load testing tool that supports WebSocket testing for performance and scalability.
4. Example: Selenium Testing with WebSocket
While Selenium itself does not natively support WebSockets, you can use JavaScript to interact with WebSockets and integrate it within a Selenium test. Below is an example of how you might write a Selenium test using JavaScript and WebSocket:
Test Setup: WebSocket Server (Example in Node.js)
const WebSocket = require('ws');
// Create a simple WebSocket server
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('A client connected');
// Send a message to the client
ws.send('Welcome to the WebSocket server');
ws.on('message', function incoming(message) {
console.log('Received: %s', message);
});
});
Test Script: Selenium with WebSocket in Python
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
import json
# WebSocket client to interact with the server
from websocket import create_connection
# Function to test WebSocket communication
def test_websocket():
# WebSocket client connects to the server
ws = create_connection("ws://localhost:8080")
print("Connected to WebSocket server")
# Send message to server
ws.send("Hello WebSocket Server!")
# Receive response from server
response = ws.recv()
print(f"Received message: {response}")
# Perform assertions on the received message
assert response == "Welcome to the WebSocket server"
# Close WebSocket connection
ws.close()
# Setup Selenium WebDriver
driver = webdriver.Chrome()
driver.get("http://example.com") # Replace with your web application's URL
# Example of interacting with the WebSocket client
test_websocket()
# Verify WebSocket functionality in the browser
assert "WebSocket Test" in driver.title
# Close the browser
driver.quit()
In this example, we create a simple WebSocket server using Node.js and test the WebSocket connection using Python’s websocket-client
library. The test sends a message to the WebSocket server, receives a response, and verifies that the message matches the expected response.
5. Performance Testing WebSockets
To ensure that your WebSocket-based application can handle a large number of concurrent connections, it is important to perform performance testing. Tools like Artillery and JMeter can simulate multiple WebSocket connections and evaluate the system’s performance under load. For example, you can simulate thousands of concurrent WebSocket connections and measure how long it takes for the server to process and respond to messages.
Example: Artillery Load Test with WebSockets
config:
target: 'ws://localhost:8080'
phases:
- duration: 60
arrivalRate: 10 # Simulate 10 new connections every second
scenarios:
- engine: "ws"
flow:
- send: '{"action": "subscribe", "channel": "updates"}'
- think: 2
- send: '{"action": "unsubscribe", "channel": "updates"}'
- think: 3
This Artillery script simulates 10 new WebSocket connections per second for a duration of 60 seconds, sending subscribe and unsubscribe messages to the WebSocket server.
6. Conclusion
Automating real-time web applications that rely on WebSockets requires simulating WebSocket connections and verifying that messages are sent and received as expected. Using Selenium in combination with WebSocket clients, such as websocket-client
in Python or JavaScript-based libraries, allows you to automate WebSocket testing and ensure that your application handles real-time communication efficiently. For performance testing, tools like Artillery and JMeter can help you simulate large numbers of concurrent WebSocket connections to measure scalability and performance.
Using AI-Powered Tools with Selenium (Testim, Mabl)
AI-powered tools like Testim and Mabl are revolutionizing the way we approach test automation, enhancing Selenium-based testing with machine learning and intelligent automation. These tools leverage AI to improve test coverage, speed up test creation, and make testing more resilient to changes in the application. Integrating AI-powered tools with Selenium can enhance your testing process, making it faster and more accurate while reducing the manual effort needed to maintain tests.
1. Introduction to AI-Powered Testing Tools
AI-powered testing tools, such as Testim and Mabl, use machine learning algorithms to automatically generate, execute, and maintain tests. These tools can analyze the application's user interface (UI) and learn from the interactions to adapt tests based on the changes in the application. By leveraging AI, these tools can automatically detect UI changes, making test maintenance easier and more reliable compared to traditional Selenium tests.
2. Testim: AI-Powered Test Automation
Testim is an AI-powered test automation tool that integrates seamlessly with Selenium. It uses machine learning models to automatically create and maintain tests by learning from user interactions with the application. It can detect UI changes and adapt tests accordingly, which helps to minimize the impact of UI modifications on test stability.
Here’s how Testim enhances Selenium tests:
- AI-based Test Creation: Testim uses machine learning to create tests that are more resilient to changes in the UI.
- Test Maintenance: AI automatically adapts to changes in the UI, reducing the effort needed for test maintenance.
- Smart Locators: Testim's AI identifies the most reliable locators for elements in the application, improving test stability.
- Data-Driven Testing: Testim supports data-driven testing, allowing tests to run with different input data sets.
Testim Example: Integrating with Selenium
Testim integrates with Selenium WebDriver to run tests on the browser. Here’s a basic example of integrating a Testim test with Selenium:
// Example of integrating Testim with Selenium WebDriver
const { Builder } = require('selenium-webdriver');
const Testim = require('testim-api');
async function runTest() {
// Initialize WebDriver
let driver = await new Builder().forBrowser('chrome').build();
// Set up Testim project and run the test
const testimClient = new Testim.Client();
const test = await testimClient.runTest({ projectId: 'your_project_id', testId: 'your_test_id' });
// Running Selenium WebDriver tests
await driver.get('https://example.com');
let title = await driver.getTitle();
console.log('Page Title:', title);
// Close WebDriver after the test
await driver.quit();
}
runTest();
3. Mabl: AI-Powered Test Automation
Mabl is another AI-powered testing tool that focuses on automating functional and regression testing. It integrates with Selenium to enhance test coverage and maintainability. Mabl uses AI to analyze application behavior and continuously learn from interactions, making it easier to adapt tests to changes in the application.
Here’s how Mabl enhances Selenium testing:
- AI-Powered Test Creation: Mabl uses machine learning algorithms to create tests based on user interactions, reducing the need for manual test creation.
- Resilient Test Execution: Mabl automatically handles UI changes and adapts the test scripts accordingly, ensuring stable test execution.
- Integrated Insights: Mabl provides detailed insights and analytics about test failures, helping teams to identify issues faster.
- Cloud Integration: Mabl runs tests on the cloud, making it easier to execute tests at scale across different environments.
Mabl Example: Integrating with Selenium
While Mabl runs tests in the cloud, you can integrate it with Selenium tests for advanced scenarios. Here is an example of running Mabl tests alongside Selenium WebDriver:
// Example of integrating Mabl with Selenium WebDriver
const { Builder } = require('selenium-webdriver');
const mabl = require('mabl-node-sdk');
async function runTest() {
// Set up Mabl test
const mablTest = new mabl.Test({
apiKey: 'your_api_key',
environment: 'your_environment',
});
// Run the Mabl test
const result = await mablTest.runTest('your_test_id');
console.log('Mabl Test Result:', result);
// Set up Selenium WebDriver
let driver = await new Builder().forBrowser('chrome').build();
// Perform Selenium test actions
await driver.get('https://example.com');
let title = await driver.getTitle();
console.log('Page Title:', title);
// Close WebDriver after the test
await driver.quit();
}
runTest();
4. Benefits of Using AI-Powered Tools with Selenium
Integrating AI-powered tools like Testim and Mabl with Selenium brings several benefits to the table:
- Reduced Maintenance: AI automatically adapts to UI changes, reducing the need for manual updates to test scripts.
- Faster Test Creation: AI speeds up the test creation process by learning from interactions and generating reliable tests.
- Improved Test Stability: AI-powered tools can automatically detect and adjust to changes in the application’s UI, preventing flaky tests.
- Enhanced Test Coverage: These tools help ensure that a wide range of user interactions and edge cases are covered.
- Increased Collaboration: AI tools provide insights and analytics that can be shared across teams, leading to better collaboration and faster issue resolution.
5. Conclusion
AI-powered tools like Testim and Mabl significantly enhance Selenium test automation by automating test creation, improving test stability, and reducing maintenance efforts. By integrating these tools with Selenium, teams can achieve more reliable tests, faster test execution, and better coverage of real-time application changes. As the tools continue to evolve, they will further streamline the testing process, making it easier to test complex applications with higher efficiency and accuracy.
Automating a Login and Registration Form
Automating the testing of login and registration forms is a critical aspect of web application testing. By using Selenium, you can simulate user interactions, such as entering credentials, clicking buttons, and validating form submissions. This section will guide you through automating login and registration form tests using Selenium WebDriver.
1. Introduction to Login and Registration Automation
Login and registration forms are essential parts of most web applications, and ensuring their functionality is paramount. Automation of these forms helps verify that the forms are working as expected, and it can also help detect issues like validation errors, incorrect form submissions, and UI problems.
2. Steps to Automate Login and Registration Form
To automate the login and registration process, you need to simulate user actions like typing credentials, submitting the form, and verifying successful login or registration. Below are the steps involved in automating these forms using Selenium WebDriver:
- Launch the browser: Open the browser and navigate to the login/registration page.
- Locate form elements: Identify the input fields (e.g., username, password, email) and buttons (e.g., login, register) using locators like ID, name, or CSS selectors.
- Enter data: Input the required data into the form fields, such as username, password, and email address.
- Submit the form: Click the login or register button to submit the form.
- Verify the response: Check if the correct response is received, such as a successful login or registration confirmation, or an error message.
3. Automating the Login Form
Here is an example of automating a login form using Selenium WebDriver in Java:
// Import required packages
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class LoginFormAutomation {
public static void main(String[] args) {
// Set the path for the ChromeDriver
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// Initialize the WebDriver
WebDriver driver = new ChromeDriver();
// Open the login page
driver.get("https://example.com/login");
// Find and fill the username field
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys("testuser");
// Find and fill the password field
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys("password123");
// Find and click the login button
WebElement loginButton = driver.findElement(By.id("loginButton"));
loginButton.click();
// Wait for the login to complete and verify successful login
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.urlContains("dashboard"));
// Verify successful login by checking if the dashboard page is displayed
System.out.println("Login Successful: " + driver.getTitle().contains("Dashboard"));
// Close the browser
driver.quit();
}
}
4. Automating the Registration Form
Similarly, you can automate the registration form by filling out the necessary fields such as username, email, password, and confirming the password. Here’s an example of automating a registration form:
// Import required packages
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.support.ui.ExpectedConditions;
public class RegistrationFormAutomation {
public static void main(String[] args) {
// Set the path for the ChromeDriver
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// Initialize the WebDriver
WebDriver driver = new ChromeDriver();
// Open the registration page
driver.get("https://example.com/register");
// Find and fill the username field
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys("newuser");
// Find and fill the email field
WebElement emailField = driver.findElement(By.id("email"));
emailField.sendKeys("newuser@example.com");
// Find and fill the password field
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys("password123");
// Find and fill the confirm password field
WebElement confirmPasswordField = driver.findElement(By.id("confirmPassword"));
confirmPasswordField.sendKeys("password123");
// Find and click the register button
WebElement registerButton = driver.findElement(By.id("registerButton"));
registerButton.click();
// Wait for registration to complete and verify successful registration
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.urlContains("confirmation"));
// Verify successful registration by checking the confirmation page
System.out.println("Registration Successful: " + driver.getTitle().contains("Confirmation"));
// Close the browser
driver.quit();
}
}
5. Common Challenges and Solutions
When automating login and registration forms with Selenium, you may face several challenges. Below are some common issues and their solutions:
- Element Not Found: Ensure the locator (e.g., ID, name, or CSS selector) is correct and that the elements are visible on the page. Use explicit waits to ensure the elements are loaded before interacting with them.
- Captcha on Forms: Captchas are designed to prevent automation. You can use third-party services like 2Captcha or AntiCaptcha to bypass captchas in testing environments.
- Dynamic Forms: If the form changes dynamically (e.g., adding fields), use dynamic locators (like XPath with contains()) to interact with these elements.
- Form Validation: Verify that appropriate validation messages are displayed when incorrect data is entered, and ensure that successful submission redirects to the correct page.
6. Conclusion
Automating login and registration forms is a critical step in web application testing. By using Selenium WebDriver, you can simulate real user interactions, automate form submissions, and ensure that your application behaves as expected. Integrating proper validation, handling dynamic elements, and dealing with common challenges like captchas will make your test automation more robust and reliable.
End-to-End Testing of an E-Commerce Website
End-to-end (E2E) testing is a crucial part of ensuring that an e-commerce website works as expected across various user journeys. This testing simulates real-world usage by automating user interactions with the website, testing everything from product selection to payment processing. In this section, we will explore how to perform end-to-end testing of an e-commerce website using Selenium WebDriver.
1. Importance of End-to-End Testing for E-Commerce Websites
End-to-end testing is vital for e-commerce websites due to the complexity of user flows, including product browsing, adding to cart, checkout, payment, and order confirmation. E-commerce websites often have dynamic content and integrations with external services (payment gateways, shipping services, etc.), so it's essential to ensure that everything works seamlessly.
2. Key User Journeys to Test in E-Commerce Websites
When performing end-to-end testing on an e-commerce website, here are some critical user journeys you should automate:
- Product Search and Browsing: Ensure users can search for products, filter results, and navigate product categories.
- Product Details: Verify that clicking on a product shows the correct product details page with images, descriptions, pricing, and options.
- Adding to Cart: Ensure that the user can add items to the cart, view the cart, and update quantities.
- Checkout Process: Test the user journey through the checkout process, including entering shipping information, selecting payment methods, and reviewing the order.
- Payment Processing: Test the integration with payment gateways (you can use test credentials for payment gateways like PayPal or Stripe).
- Order Confirmation: Verify that after completing the payment, the user sees the order confirmation screen with accurate details.
3. Setting Up Selenium for E-Commerce Testing
Before automating the e-commerce website testing, you need to set up Selenium WebDriver and necessary dependencies for the browser you want to use (e.g., Chrome, Firefox).
4. Example: Automating an E-Commerce User Journey
Here’s an example of automating the product selection, adding to the cart, and completing checkout using Selenium WebDriver in Java:
// Import required packages
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class EcommerceEndToEndTest {
public static void main(String[] args) {
// Set the path for the ChromeDriver
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// Initialize the WebDriver
WebDriver driver = new ChromeDriver();
// Step 1: Open the e-commerce website
driver.get("https://example-ecommerce.com");
// Step 2: Search for a product
WebElement searchBox = driver.findElement(By.id("search"));
searchBox.sendKeys("Laptop");
WebElement searchButton = driver.findElement(By.id("searchButton"));
searchButton.click();
// Step 3: Select the first product from the search results
WebElement firstProduct = driver.findElement(By.cssSelector(".product-item:nth-child(1)"));
firstProduct.click();
// Step 4: Add the product to the cart
WebElement addToCartButton = driver.findElement(By.id("addToCart"));
addToCartButton.click();
// Step 5: Navigate to the cart and proceed to checkout
WebElement cartButton = driver.findElement(By.id("cartButton"));
cartButton.click();
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.elementToBeClickable(By.id("checkoutButton")));
// Step 6: Proceed with the checkout process
WebElement checkoutButton = driver.findElement(By.id("checkoutButton"));
checkoutButton.click();
// Step 7: Fill in shipping information
WebElement nameField = driver.findElement(By.id("name"));
nameField.sendKeys("John Doe");
WebElement addressField = driver.findElement(By.id("address"));
addressField.sendKeys("123 Main St");
WebElement paymentMethod = driver.findElement(By.id("paymentMethod"));
paymentMethod.click();
WebElement completeOrderButton = driver.findElement(By.id("completeOrder"));
completeOrderButton.click();
// Step 8: Verify order confirmation
wait.until(ExpectedConditions.urlContains("order-confirmation"));
System.out.println("Order placed successfully: " + driver.getTitle());
// Step 9: Close the browser
driver.quit();
}
}
5. Dealing with Common Issues in E-Commerce Testing
While automating e-commerce websites, you may encounter issues like:
- Dynamic Content: E-commerce websites often load content dynamically (e.g., images or product details). Use explicit waits to ensure the content is loaded before interacting with it.
- Payment Gateway Integration: Testing payment gateways with real transactions can be risky. Use sandbox/test environments provided by payment providers like PayPal or Stripe to simulate payments without real transactions.
- Authentication: If the website requires login, automate the login process or use pre-existing test accounts to avoid manual login during tests.
- Responsive Design: Test the website on multiple screen sizes to ensure it works well on both desktop and mobile devices. Use Selenium’s window resizing functionality or run tests on real devices using Appium or cloud-based services like BrowserStack or Sauce Labs.
6. Performance Considerations
End-to-end tests can sometimes be slow, especially when simulating multiple user journeys. Here are some performance considerations:
- Parallel Testing: Run tests in parallel across multiple browsers and devices to save time. Selenium Grid or cloud-based services like BrowserStack and Sauce Labs can help with parallel execution.
- Headless Browsers: Use headless browsers like ChromeHeadless or FirefoxHeadless to speed up the tests by avoiding the overhead of launching a graphical interface.
- Test Data Management: Use mock data or predefined test accounts to speed up tests by avoiding the need for real-time data entry or account creation.
7. Conclusion
End-to-end testing of an e-commerce website ensures that your users can successfully browse products, complete purchases, and receive confirmation. By automating these user journeys with Selenium WebDriver, you can reduce the time spent on manual testing, ensure a seamless shopping experience, and quickly identify issues that affect critical workflows like checkout and payment. Integrating parallel testing, headless browsers, and proper test data management can help optimize the performance of your e-commerce testing suite.
Testing Online Booking Systems (Flights, Hotels)
Online booking systems for flights and hotels are essential tools for travel companies, providing a convenient platform for customers to book services. Testing these booking systems ensures that the applications are functioning correctly, efficiently, and securely. In this section, we will explore how to automate the testing of online booking systems using Selenium WebDriver and Python.
1. Why Test Online Booking Systems?
Online booking systems are critical for businesses in the travel industry. Ensuring that these systems work correctly can help prevent issues like booking errors, payment failures, and poor user experiences. Automated testing can be used to:
- Ensure Functionality: Verify that users can successfully search, select, and book flights or hotels.
- Improve User Experience: Test the interface for ease of use, navigation, and performance.
- Ensure Security: Ensure that sensitive data like personal information and payment details are handled securely.
- Reduce Errors: Automate repetitive tasks to identify issues early in the development cycle and improve software quality.
2. Setting Up the Environment for Testing
Before automating the testing of booking systems, make sure you have the necessary tools installed:
- Python: Install Python from Python's official website.
- Selenium WebDriver: Install Selenium WebDriver using the command:
pip install selenium
. - WebDriver for Chrome: Download ChromeDriver or the driver for your preferred browser.
3. Automating Flight Booking Testing
Flight booking systems typically allow users to search for flights based on various criteria, select a flight, and complete the booking process. Below is an example of how to test a flight booking system using Selenium.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from time import sleep
# Setup WebDriver
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
# Step 1: Open the flight booking website
driver.get("https://www.example-flight-booking.com")
# Step 2: Enter flight search details
from_field = driver.find_element(By.id("from"))
from_field.send_keys("New York")
to_field = driver.find_element(By.id("to"))
to_field.send_keys("London")
date_field = driver.find_element(By.id("departure_date"))
date_field.send_keys("2025-06-01")
search_button = driver.find_element(By.id("search_button"))
search_button.click()
sleep(3) # Wait for results to load
# Step 3: Select a flight
select_flight = driver.find_element(By.xpath("//button[contains(text(), 'Select Flight')]"))
select_flight.click()
# Step 4: Enter passenger details and proceed to payment
passenger_name_field = driver.find_element(By.id("passenger_name"))
passenger_name_field.send_keys("John Doe")
payment_button = driver.find_element(By.id("payment_button"))
payment_button.click()
# Step 5: Wait for confirmation and close the browser
sleep(5)
driver.quit()
4. Automating Hotel Booking Testing
Hotel booking systems allow users to select dates, choose a hotel room, and confirm the booking. Below is an example of how to automate the testing of a hotel booking system using Selenium.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from time import sleep
# Setup WebDriver
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
# Step 1: Open the hotel booking website
driver.get("https://www.example-hotel-booking.com")
# Step 2: Enter hotel search details
location_field = driver.find_element(By.id("location"))
location_field.send_keys("Paris")
checkin_date_field = driver.find_element(By.id("checkin_date"))
checkin_date_field.send_keys("2025-07-01")
checkout_date_field = driver.find_element(By.id("checkout_date"))
checkout_date_field.send_keys("2025-07-10")
search_button = driver.find_element(By.id("search_button"))
search_button.click()
sleep(3) # Wait for results to load
# Step 3: Select a hotel
select_hotel = driver.find_element(By.xpath("//button[contains(text(), 'Select Hotel')]"))
select_hotel.click()
# Step 4: Enter guest details and proceed to payment
guest_name_field = driver.find_element(By.id("guest_name"))
guest_name_field.send_keys("Jane Doe")
payment_button = driver.find_element(By.id("payment_button"))
payment_button.click()
# Step 5: Wait for confirmation and close the browser
sleep(5)
driver.quit()
5. Handling Edge Cases and Validating Errors
When testing online booking systems, it's important to validate error messages, edge cases, and incorrect input handling:
- Invalid Dates: Test the system's response when users enter dates in the past or conflicting dates (e.g., checkout date before check-in date).
- Invalid Payment Information: Test scenarios where users provide incorrect payment details.
- Empty Fields: Ensure the system handles empty or incomplete form submissions gracefully, displaying appropriate error messages.
- Session Timeout: Test how the booking system responds when a session times out or the user is inactive for too long.
6. Performance Testing
Performance testing is crucial for online booking systems, especially during peak seasons when many users might access the system simultaneously. Tools like JMeter and Gatling can be integrated with Selenium to simulate high user loads and test the system’s behavior under stress.
7. Conclusion
Automating the testing of online booking systems for flights and hotels ensures that these platforms are functioning smoothly and providing users with a seamless experience. By using Selenium WebDriver, you can automate the testing of various booking scenarios, validate edge cases, and ensure that the system can handle real-world usage scenarios. Additionally, integrating with performance testing tools can help you evaluate the scalability and robustness of your booking system.
Automating Data Scraping Tasks with Selenium
Data scraping is a technique used to extract information from websites. It is widely used in various industries, including e-commerce, finance, and market research. Selenium, a powerful tool for web automation, can also be used for scraping dynamic content from websites. In this section, we will learn how to use Selenium to automate data scraping tasks.
1. Why Use Selenium for Data Scraping?
Many websites today use JavaScript to render content dynamically, making it difficult for traditional scraping tools like BeautifulSoup or Scrapy to capture all the necessary data. Selenium, being a browser automation tool, can interact with web pages just like a user, allowing it to handle JavaScript-rendered content effectively. Here are some reasons to use Selenium for scraping:
- Dynamic Content: Selenium can scrape websites that load content dynamically with JavaScript.
- Web Interactions: Selenium can simulate user actions like clicks, scrolls, and form submissions, which are often required to retrieve data from websites.
- Multiple Browsers: Selenium supports multiple browsers, such as Chrome, Firefox, and Safari, allowing flexibility in scraping tasks.
2. Setting Up the Environment
Before you start scraping data with Selenium, you need to set up your environment. Follow these steps:
- Install Python: If you don't have Python installed, download it from python.org.
- Install Selenium: You can install Selenium using pip by running the command:
pip install selenium
. - Install WebDriver: Download the WebDriver for your browser. For example, you can get ChromeDriver for Google Chrome.
3. Writing a Basic Selenium Data Scraping Script
Let’s write a simple Python script to scrape data from a webpage. This script will use Selenium to open a website, extract data, and print it to the console.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
import time
# Setup WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Step 1: Open the website
driver.get("https://example.com")
# Step 2: Wait for the page to load
time.sleep(3)
# Step 3: Locate the data (example: extracting titles of articles)
article_titles = driver.find_elements(By.CLASS_NAME, "article-title")
# Step 4: Extract and print the data
for title in article_titles:
print(title.text)
# Step 5: Close the browser
driver.quit()
4. Handling Dynamic Content
Many modern websites load content dynamically using JavaScript. Selenium can handle this by waiting for elements to load before scraping them. Here’s how you can wait for elements to appear on the page:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Setup WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Step 1: Open the website
driver.get("https://example.com")
# Step 2: Wait for the dynamic content to load
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.CLASS_NAME, "dynamic-content")))
# Step 3: Extract the data
dynamic_content = driver.find_element(By.CLASS_NAME, "dynamic-content")
print(dynamic_content.text)
# Step 4: Close the browser
driver.quit()
5. Handling Pagination
Many websites display content across multiple pages. Selenium can handle pagination by simulating clicks on the next page button and scraping data from each page. Here’s an example:
# Setup WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Step 1: Open the website
driver.get("https://example.com")
# Step 2: Scrape data from multiple pages
while True:
# Extract data from the current page
items = driver.find_elements(By.CLASS_NAME, "item")
for item in items:
print(item.text)
# Click the "Next" button to go to the next page
try:
next_button = driver.find_element(By.CLASS_NAME, "next-page")
next_button.click()
time.sleep(3) # Wait for the next page to load
except:
print("No more pages to scrape.")
break
# Step 3: Close the browser
driver.quit()
6. Scraping Data from Forms
Sometimes you need to scrape data from forms, such as search results or login forms. Selenium can automate filling out forms and submitting them to retrieve relevant data. Here’s an example of automating a search form submission:
# Setup WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Step 1: Open the search page
driver.get("https://example.com/search")
# Step 2: Locate the search input and submit a query
search_box = driver.find_element(By.NAME, "search")
search_box.send_keys("Selenium WebDriver")
search_box.send_keys(Keys.RETURN)
# Step 3: Wait for the results to load
time.sleep(3)
# Step 4: Extract and print the search results
results = driver.find_elements(By.CLASS_NAME, "search-result")
for result in results:
print(result.text)
# Step 5: Close the browser
driver.quit()
7. Storing Scraped Data
Once you've scraped the data, you can store it in various formats such as CSV, Excel, or JSON. Here’s an example of saving the scraped data to a CSV file:
import csv
# Setup WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Step 1: Open the website
driver.get("https://example.com")
# Step 2: Scrape data
items = driver.find_elements(By.CLASS_NAME, "item")
data = [item.text for item in items]
# Step 3: Save data to CSV
with open("scraped_data.csv", mode="w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["Item"])
for row in data:
writer.writerow([row])
# Step 4: Close the browser
driver.quit()
8. Conclusion
Automating data scraping tasks with Selenium allows you to efficiently collect data from dynamic websites. Selenium’s ability to simulate user interactions with a web page, combined with its support for JavaScript-rendered content, makes it a powerful tool for web scraping. Whether you're collecting product prices, news articles, or search results, Selenium can help you automate the process and save time.
Writing Clean and Maintainable Automation Code
Writing clean and maintainable automation code is essential for long-term success in test automation projects. Well-structured code is easier to debug, extend, and maintain, which improves the efficiency of the development and testing processes. In this section, we will discuss best practices for writing clean and maintainable test automation code.
1. Follow Coding Standards and Conventions
Adhering to coding standards and conventions helps maintain consistency across your automation scripts. Whether you are working individually or in a team, following a common set of rules makes the code easier to read and understand. Some basic guidelines include:
- Use Descriptive Names: Choose meaningful variable, function, and class names that convey the purpose of the code. For example, instead of naming a variable
temp
, useuserName
orloginButton
. - Indentation and Spacing: Use consistent indentation (e.g., 4 spaces per level) and include blank lines where appropriate to separate logical blocks of code.
- Commenting: Provide comments to explain complex logic, but avoid over-commenting. Focus on writing self-explanatory code.
2. Use Page Object Model (POM) Design Pattern
The Page Object Model (POM) is a design pattern that helps in creating maintainable and reusable automation code. In this model, each web page is represented by a class that contains methods for interacting with elements on that page. This approach helps decouple test scripts from page-specific details, making it easier to maintain and scale test cases.
# Page Object for Login Page
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = driver.find_element(By.ID, "username")
self.password_field = driver.find_element(By.ID, "password")
self.login_button = driver.find_element(By.ID, "loginBtn")
def enter_username(self, username):
self.username_field.send_keys(username)
def enter_password(self, password):
self.password_field.send_keys(password)
def click_login(self):
self.login_button.click()
# Test case using Page Object Model
def test_login():
driver = webdriver.Chrome()
login_page = LoginPage(driver)
login_page.enter_username("user")
login_page.enter_password("password")
login_page.click_login()
assert "Dashboard" in driver.title
3. Keep Tests Independent and Atomic
Tests should be independent of each other, meaning that the outcome of one test should not affect the execution of another. Each test case should focus on a single unit of functionality, also known as an atomic test. Keeping tests atomic has the following benefits:
- Isolation: Tests run independently, so failures are easier to identify and fix.
- Reusability: Smaller, focused tests can be reused in different test scenarios.
- Parallel Execution: Independent tests can be executed in parallel to speed up the testing process.
4. Handle Test Data Effectively
Managing test data effectively is crucial for clean and maintainable automation code. Test data should be externalized and managed in a structured format such as CSV, Excel, or JSON. Avoid hardcoding test data in the test scripts as it makes code difficult to update and maintain.
import json
# Reading test data from an external JSON file
def load_test_data(file_path):
with open(file_path, 'r') as file:
return json.load(file)
test_data = load_test_data("test_data.json")
# Test case using external data
def test_login_with_multiple_users():
for user in test_data["users"]:
driver = webdriver.Chrome()
login_page = LoginPage(driver)
login_page.enter_username(user["username"])
login_page.enter_password(user["password"])
login_page.click_login()
assert "Dashboard" in driver.title
driver.quit()
5. Use Assertions Effectively
Assertions are crucial to verify whether the automation tests produce the expected results. Be sure to use assertions effectively:
- Assert Specific Conditions: Ensure that your assertions check for specific conditions that reflect the desired state of the application.
- Fail Fast: If an assertion fails, terminate the test immediately to save time and resources.
- Group Assertions: Group related assertions together to improve test case readability and maintainability.
6. Optimize Test Execution Time
Efficient test execution is crucial for maintaining a fast and responsive test suite. Here are some tips to optimize test execution time:
- Minimize Browser Setup: Avoid launching and closing browsers in every test case. Use setup and teardown methods (e.g.,
setUp
andtearDown
) to reuse browsers across multiple tests. - Parallel Execution: Run tests in parallel to improve execution speed. Tools like Selenium Grid, Docker, or cloud-based services such as Sauce Labs and BrowserStack can help achieve this.
- Headless Browsers: When running tests in non-UI environments, use headless browsers to save on system resources.
7. Keep Your Code DRY (Don't Repeat Yourself)
The DRY principle encourages the reuse of code and avoids duplication. Repeated code makes automation scripts harder to maintain and increases the chances of errors. To follow the DRY principle:
- Use Functions and Methods: Group repetitive logic into reusable functions or methods to avoid duplication.
- Use Setup and Teardown Methods: Utilize setup and teardown methods to initialize and clean up resources, reducing code duplication across tests.
8. Log and Report Test Results
Logging and reporting are essential for understanding the outcome of automation tests. Proper logging helps track the flow of test execution and provides insights into failures. Some best practices include:
- Use Structured Logging: Use logging libraries to capture different levels of logs (e.g., info, debug, error) with timestamps.
- Generate Test Reports: Use tools like TestNG, JUnit, or Allure to generate detailed test reports after each test run.
9. Conclusion
Writing clean and maintainable automation code is essential for the long-term health of your testing framework. By following best practices like using design patterns, optimizing test execution, and externalizing test data, you can make your test automation scripts more robust, scalable, and easier to maintain. This not only makes your work more efficient but also improves the overall quality of your automated tests.
Using Git for Version Control in Selenium Projects
Version control is crucial for managing and tracking changes in your automation code. Git is a widely used version control system that helps developers collaborate, maintain code history, and manage different versions of their projects. This section explores how to use Git for version control in your Selenium projects.
1. Why Use Git in Selenium Projects?
Using Git in Selenium projects offers several benefits:
- Collaboration: Git enables multiple developers to work on the same project simultaneously, reducing conflicts and merging changes efficiently.
- Version History: Git tracks every change made to your project, allowing you to revert to previous versions if something goes wrong or identify when bugs were introduced.
- Branching and Merging: Git’s branching and merging features allow you to create separate branches for different features or bug fixes and merge them back to the main codebase when ready.
- Backup and Recovery: Git provides a reliable backup of your automation code that can be restored if needed.
2. Setting Up Git in a Selenium Project
To start using Git in your Selenium project, you need to initialize a Git repository and commit your automation code. Here's how you can do it:
- Initialize the Git Repository: In your project directory, run the following command to initialize a Git repository:
git init
- Track Your Files: Add your project files to the repository using the
git add
command:git add .
- Commit the Files: Commit the added files to Git with a descriptive message:
git commit -m "Initial commit of Selenium test automation code"
- Push to Remote Repository: If you have a remote repository (e.g., GitHub, GitLab, or Bitbucket), you can push your local repository to the remote server:
git remote add origin
git push -u origin master
3. Branching and Merging in Selenium Projects
When working on features or bug fixes, it's a good idea to create separate branches. This keeps the main branch (typically master
or main
) stable while allowing you to work on new changes without affecting the main codebase. Here’s how to manage branches in Git:
- Create a New Branch: To start working on a new feature or bug fix, create a new branch:
git checkout -b feature/new-login-test
- Switch Between Branches: To switch back to the main branch or any other existing branch:
git checkout main
- Merge Changes: Once the work on the branch is complete, you can merge it back into the main branch:
git checkout main git merge feature/new-login-test
- Push the Changes to Remote: After merging, push the changes to the remote repository:
git push origin main
4. Resolving Merge Conflicts
Merge conflicts may occur when two branches modify the same part of a file. Git will alert you to conflicts during the merge process. To resolve conflicts:
- Identify the Conflicted Files: Git will mark the conflicted files and show a message indicating where the conflict occurred.
- Manually Resolve the Conflict: Open the conflicted file and manually edit the conflicting sections. Git will mark the conflicting areas with special markers (e.g.,
<<<<<<<
,=======
,>>>>>>>`). Remove the markers and decide which changes to keep.
- Mark the Conflict as Resolved: After resolving the conflict, mark the file as resolved:
git add
- Commit the Merge: Once all conflicts are resolved, commit the merge:
git commit -m "Resolved merge conflict in login test"
5. Git Best Practices for Selenium Projects
Here are some best practices to follow when using Git in your Selenium projects:
- Commit Often: Commit your changes frequently with descriptive commit messages that explain the purpose of the changes.
- Use Feature Branches: Always create separate branches for new features, bug fixes, or tests. This keeps the main branch clean and stable.
- Review Pull Requests: If you're working in a team, use pull requests (PRs) for code review. This helps ensure that changes are peer-reviewed before they are merged into the main branch.
- Tag Releases: Use Git tags to mark important milestones or releases in your Selenium project. For example, you can tag a version when a set of tests is ready for production deployment.
- Maintain a .gitignore File: Use a
.gitignore
file to exclude unnecessary files (e.g., log files, temporary files, IDE configurations) from being tracked by Git.
6. Example: A Simple Selenium Project with Git
Here is an example of how a simple Selenium test project might look with Git:
my-selenium-project/
├── .git/
├── .gitignore
├── tests/
│ ├── login_test.py
│ └── checkout_test.py
├── page_objects/
│ ├── login_page.py
│ └── checkout_page.py
├── README.md
└── requirements.txt
In this example, the project is structured with separate folders for tests and page objects. The .gitignore
file ensures that unnecessary files are not tracked in the repository, and the requirements.txt
file lists the dependencies for the project.
7. Conclusion
Using Git for version control in your Selenium projects enhances collaboration, tracking, and project management. By following best practices such as branching, committing often, and using Git effectively, you can maintain a clean and organized codebase. Git also provides a safety net for recovering code and helps automate tasks such as continuous integration, making it an essential tool for any Selenium test automation project.
Organizing Tests for Scalability
As the size of your Selenium test suite grows, it becomes essential to organize and structure your tests in a way that allows for scalable execution, easy maintenance, and faster performance. This section will explore best practices for organizing Selenium tests to ensure that your test suite can handle large-scale applications efficiently.
1. Why Test Organization Matters?
Organizing tests effectively is crucial for large-scale testing environments where multiple teams may work on the same project. Proper test organization allows you to:
- Save Time: Quick identification of relevant tests allows for faster execution, reducing overall test execution time.
- Enhance Maintainability: A well-structured test suite is easier to maintain, update, and scale as the project evolves.
- Improve Collaboration: Clear and consistent organization enables better collaboration between team members, especially when different teams are responsible for different parts of the project.
- Scale Efficiently: Organized tests can be executed in parallel on multiple machines, allowing for faster feedback and improved scalability.
2. Key Strategies for Organizing Tests
Below are some important strategies you can use to organize your Selenium tests for scalability:
2.1. Use a Modular Test Framework
Breaking down your tests into smaller, reusable modules is key for scalability. This allows you to isolate functionality into test components, making it easier to update and maintain them. The Page Object Model (POM) pattern is a great way to organize tests into manageable modules:
- Page Objects: Create separate classes for different web pages or components of your application (e.g., login page, home page, etc.). This encapsulation makes tests easier to read and maintain.
- Reusable Functions: Create utility functions (e.g., for login, navigation) that can be reused across multiple tests to reduce code duplication and improve maintainability.
2.2. Separate Test Suites by Functionality
Organizing your test cases by functionality or feature is another effective way to scale your test suite. You can group tests based on different modules of your application, such as:
- Login Tests
- Checkout Tests
- Search Functionality Tests
- Performance Tests
This method ensures that you can run specific test suites based on the functionality being worked on, reducing the overall time spent executing unnecessary tests.
2.3. Implement Test Prioritization
Not all tests need to be executed every time. Test prioritization helps you decide which tests to run depending on the current development cycle or project stage. Common strategies include:
- Critical Path Tests: Always run tests that cover core functionality and must pass before deployment (e.g., login, payment). These tests should be prioritized for every build.
- Smoke Tests: A set of basic tests that verify the application is working before executing a more extensive suite.
- Regression Tests: Tests that ensure new changes do not break existing features. These tests can be executed periodically or on specific branches.
2.4. Use Parallel Test Execution
To scale your tests efficiently, run multiple tests in parallel across different browsers, devices, and environments. This allows you to execute tests faster and reduce the overall time required for test execution. Tools like Selenium Grid or cloud-based testing platforms (e.g., BrowserStack, Sauce Labs) help run tests in parallel on multiple machines:
- Selenium Grid: Set up a Selenium Grid to distribute tests across multiple nodes (browsers and devices). This helps in running tests concurrently, speeding up test execution.
- Cloud Testing: Leverage cloud-based testing platforms to run tests across multiple browsers and devices in parallel. This reduces infrastructure setup time and allows for testing in diverse environments.
2.5. Organize Test Data
Test data plays a crucial role in automated testing. For scalability, store and manage test data efficiently:
- External Data Files: Store test data in external files such as CSV, Excel, or JSON to separate it from test scripts. This allows you to manage and scale your tests without modifying the code.
- Data-Driven Testing: Implement data-driven testing to run the same test with different sets of data. This increases test coverage and helps test various scenarios with minimal effort.
3. Organizing Tests for Continuous Integration (CI)
Integrating your tests into a continuous integration (CI) pipeline is essential for scalability, allowing you to run tests automatically whenever changes are made to the codebase. Here are some best practices for integrating Selenium tests into CI:
- Automate Test Execution: Set up your CI pipeline (e.g., using Jenkins, GitLab CI, or Travis CI) to trigger Selenium tests for every code commit or pull request, ensuring that any new changes don’t break existing functionality.
- Run Tests on Multiple Environments: Configure your CI pipeline to execute tests across multiple browsers, devices, and operating systems to ensure cross-browser compatibility.
- Generate Reports: Use reporting tools like TestNG, Allure, or ExtentReports to generate detailed test execution reports, so you can quickly identify failures and resolve them.
- Manage Test Artifacts: Store test artifacts (logs, screenshots, videos) generated during test execution for easy access and troubleshooting.
4. Example Directory Structure
Here is an example of how you might organize the directory structure of a scalable Selenium test project:
my-selenium-project/
├── tests/
│ ├── login/
│ │ ├── login_test.py
│ │ └── login_page.py
│ ├── checkout/
│ │ ├── checkout_test.py
│ │ └── checkout_page.py
│ └── search/
│ ├── search_test.py
│ └── search_page.py
├── data/
│ ├── login_data.csv
│ └── checkout_data.json
├── reports/
│ ├── test_report.html
├── config/
│ ├── config.json
├── .gitignore
└── requirements.txt
In this structure, tests are organized by feature (e.g., login
, checkout
, search
), and test data is stored separately in the data
folder. The config
folder holds configuration files, and the reports
folder contains execution reports.
5. Conclusion
Organizing Selenium tests for scalability is essential for managing large test suites and improving the efficiency of test execution. By using modular frameworks, separating tests by functionality, prioritizing tests, running tests in parallel, and integrating tests into continuous integration pipelines, you can ensure that your Selenium tests scale efficiently and remain maintainable as your project grows.
Reducing Flakiness in Automation Tests
Flakiness in automation tests refers to tests that sometimes pass and sometimes fail, even though there is no change in the codebase. This can lead to unreliable test results and wasted time diagnosing false negatives or positives. Reducing flakiness is crucial for ensuring the reliability of your test suite and the quality of your software. This section explores causes of test flakiness and provides strategies to minimize it.
1. What Causes Flakiness in Automation Tests?
Flakiness can occur due to a variety of reasons, some of the most common include:
- Timing Issues: Automation scripts often rely on specific timing or delays between actions, leading to failures if the application is slower or faster than expected.
- Element Locators: Incorrect or unstable locators (e.g., dynamic IDs or class names) can cause tests to break when the DOM changes.
- Network Delays: Tests that depend on external resources or APIs may fail due to network or server issues.
- Environmental Differences: Variations in browser configurations, screen resolutions, or operating systems can cause tests to behave inconsistently.
- Unreliable External Services: Tests that rely on third-party services, such as authentication or payment gateways, may fail if those services experience downtime or delays.
2. Strategies to Reduce Flakiness
Here are several techniques you can use to reduce flakiness in your automation tests:
2.1. Implement Explicit Waits
Explicit waits are a powerful way to handle timing issues in Selenium tests. Instead of using hard waits like Thread.sleep()
, use WebDriverWait
to wait for specific elements to become interactable before performing actions. This ensures that elements are present and ready for interaction:
// Example of an explicit wait in Selenium (Java)
WebDriver driver = new ChromeDriver();
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("submitButton")));
element.click();
Using explicit waits helps ensure that Selenium interacts with elements only when they are ready, reducing the likelihood of race conditions and timing issues.
2.2. Use Stable Element Locators
Flaky tests often result from using unstable or dynamic element locators (e.g., auto-generated IDs or class names that change with each page load). Instead, use more stable locators like:
- CSS Selectors: Use CSS attributes that remain constant across page loads.
- XPath: Use XPath expressions based on text or other consistent attributes.
- Custom IDs: If possible, work with developers to use static and meaningful IDs or class names for elements.
By using stable and unique locators, you can reduce the chances of Selenium failing to find elements on the page.
2.3. Handle Dynamic Content Appropriately
Many modern web applications load content dynamically, which can cause test flakiness if elements are not fully loaded when your test interacts with them. Use Selenium's WebDriverWait
in combination with conditions like visibilityOf()
or presenceOfElementLocated()
to ensure that the element is fully loaded before interacting with it:
// Wait for an element to be visible
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
element.click();
2.4. Avoid Hard-Coding Wait Times
Hard-coding wait times, such as using Thread.sleep()
, can introduce unnecessary delays and lead to unreliable tests. Instead, use dynamic waits like WebDriverWait
to wait for specific conditions. This allows tests to adapt to varying conditions, such as slower network speeds or page load times.
2.5. Isolate Tests from External Dependencies
Tests that depend on external services, APIs, or third-party systems can become flaky due to issues like network latency or downtime. To minimize flakiness, consider:
- Mocking External Services: Use mock services or APIs to simulate external interactions. Tools like
WireMock
can help you mock API responses and isolate tests from external dependencies. - Using Stubs: Stub out services or database calls to ensure consistency in your tests.
By isolating tests from external systems, you can make them more reliable and easier to run consistently.
2.6. Use Headless Browser for Testing
Headless browsers (e.g., Chrome Headless, Firefox Headless) can help reduce environmental issues that might contribute to flakiness. Running tests in headless mode can also improve speed and reduce resource consumption, allowing for more consistent results in CI/CD pipelines:
// Set up headless Chrome browser
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless", "--disable-gpu");
WebDriver driver = new ChromeDriver(options);
Headless mode eliminates the need for a graphical interface, reducing the possibility of failures due to UI rendering issues.
2.7. Use Retry Logic for Temporary Failures
Some failures are transient and may be resolved by retrying the test. Implement retry logic in your tests to re-execute failed tests a limited number of times before marking them as failed. This can help mitigate issues caused by transient network problems or server delays:
// Example of retry logic using TestNG
@RetryAnalyzer(Retry.class)
@Test
public void testExample() {
Assert.assertEquals(driver.getTitle(), "Expected Title");
}
By adding retry logic, you can reduce false negatives caused by temporary issues.
2.8. Run Tests in Parallel
Running tests in parallel can reduce flaky behavior by spreading out the load across multiple environments and executing tests faster. Tools like Selenium Grid, Docker, or cloud services (e.g., BrowserStack, Sauce Labs) allow you to run tests in parallel across different browsers, devices, and configurations, ensuring that tests are more stable and consistent.
2.9. Monitor and Analyze Test Results
Regularly monitor test results to identify patterns in flaky tests. Tools like Jenkins, Allure, or TestNG can help generate detailed reports and logs, allowing you to identify root causes of failures. By analyzing these reports, you can identify recurring issues and fix them to improve test stability.
3. Conclusion
Reducing flakiness in Selenium tests is an ongoing process that requires careful attention to test design, synchronization, and external dependencies. By implementing strategies such as explicit waits, stable element locators, and retry logic, you can make your tests more reliable and prevent wasted time spent on false failures. Consistent monitoring and analysis of test results will also help you maintain a stable and efficient test suite.
Keeping Up-to-Date with Selenium Updates
Maintaining an up-to-date knowledge of Selenium is crucial for developers and testers to ensure their test automation is efficient, secure, and compatible with the latest features and fixes. Selenium evolves quickly, with new releases and improvements being made regularly. Staying current with updates allows you to leverage new functionality, fix bugs, and address security concerns. This section discusses the importance of staying updated with Selenium and strategies to keep your automation process current.
1. Why Stay Updated with Selenium?
Keeping your Selenium setup up-to-date ensures that:
- New Features: You can take advantage of the latest features and improvements that make your testing more efficient and easier to maintain.
- Bug Fixes: Regular updates help resolve known issues, ensuring that your tests run smoothly and reliably.
- Security Enhancements: Updates often include important security patches to protect your test automation from vulnerabilities.
- Compatibility: Keeping your Selenium version updated ensures compatibility with the latest browser versions and web technologies.
- Support: Older versions of Selenium may no longer be supported, meaning you could lose access to valuable community support and documentation.
2. How to Stay Updated with Selenium?
To stay informed about the latest releases and developments in Selenium, here are some useful strategies:
2.1. Follow Official Selenium Channels
The official Selenium channels are the most reliable sources of information regarding updates and news. These include:
- Official Selenium Website: Visit the official Selenium website for the latest news, release notes, and downloads. The website also contains detailed documentation for every Selenium release.
- GitHub Repository: Selenium’s GitHub repository is the primary source for the latest releases, bug fixes, and pull requests. You can follow the Selenium GitHub repository to stay informed about new releases and ongoing development.
- Mailing List: Subscribe to the Selenium Users mailing list for updates, announcements, and discussions from the Selenium community.
2.2. Watch for Release Notes
Release notes provide an overview of what's new, what's fixed, and what's changed in the latest version of Selenium. Every new release is accompanied by detailed release notes, which help you understand the improvements and updates made. These notes often include:
- New Features: Information on new functionality added to Selenium.
- Bug Fixes: A list of bugs that have been fixed in the latest version.
- Breaking Changes: Any changes that may break backward compatibility, along with recommended actions to migrate your code.
- Deprecations: Information about deprecated features and alternatives for future-proofing your scripts.
Always read the release notes before upgrading to a new version, especially for major releases, as they may include breaking changes or new configurations.
2.3. Participate in the Selenium Community
Engaging with the Selenium community is a great way to stay informed and learn about new developments, best practices, and upcoming features. Here are a few ways to participate:
- Forums and Discussion Groups: Join Selenium-focused forums like Selenium Forum where you can ask questions, share knowledge, and learn from other testers.
- Stack Overflow: Follow the Selenium tag on Stack Overflow to stay up-to-date with common issues, new features, and solutions provided by the community.
- Contribute to Selenium: If you have the skills, consider contributing to the development of Selenium itself by submitting bug reports, feature requests, or even pull requests. The Selenium GitHub repository welcomes contributions from the community.
2.4. Follow Selenium Blogs and Social Media
Many developers and testers share their experiences, insights, and tutorials related to Selenium on blogs and social media platforms. Some popular blogs and platforms to follow include:
- Selenium Blog: The official Selenium blog features updates, tutorials, and news about Selenium.
- Medium: Many developers write tutorials and share their experiences with Selenium on Medium, providing valuable insights on the latest trends and tools in automation testing.
- Twitter: Follow the official Selenium Twitter account for the latest updates, news, and announcements.
- LinkedIn: Join Selenium groups on LinkedIn or follow automation experts and influencers for discussions and updates on Selenium.
2.5. Use Dependency Management Tools
To manage Selenium dependencies efficiently in your projects, use dependency management tools like Maven (for Java), npm (for JavaScript), or pip (for Python). These tools can help you automatically check and update to the latest stable versions of Selenium:
org.seleniumhq.selenium
selenium-java
4.5.0
By using these tools, you can ensure your project always uses the latest stable version of Selenium without manually checking for updates.
3. Updating Selenium
To keep Selenium up-to-date, follow the steps appropriate for your programming environment:
3.1. Java (Maven/Gradle)
For Java projects, you can update Selenium via Maven or Gradle by modifying the version number in your dependency configuration and re-running the build process. For example, in Maven:
org.seleniumhq.selenium
selenium-java
4.5.0
3.2. JavaScript (npm)
For JavaScript projects, use the npm update
command to update Selenium WebDriver to the latest version.
npm update selenium-webdriver
3.3. Python (pip)
For Python projects, you can update Selenium using pip
by running:
pip install --upgrade selenium
4. Conclusion
By staying up-to-date with Selenium, you can ensure that your test automation remains efficient, secure, and compatible with modern browsers and frameworks. Regularly check for new releases, participate in the Selenium community, and use dependency management tools to simplify the process of keeping your environment current. With continuous updates and improvements, Selenium will continue to be a powerful tool for browser automation and testing.
Automating Social Media Posting (e.g., Facebook, Twitter)
Automating social media posting can save time and ensure consistent engagement with your audience. With the help of Selenium WebDriver, you can automate the process of posting content to social media platforms like Facebook and Twitter. In this section, we will explore how to automate the posting process for these platforms using Selenium and Python.
1. Why Automate Social Media Posting?
Automating social media posting can help businesses, marketers, and influencers schedule posts, maintain consistent branding, and improve productivity. By automating your posts, you can achieve:
2. Setting Up the Environment
Before automating social media posting, make sure you have the necessary tools installed:
pip install selenium
.3. Automating Posting on Facebook
To automate Facebook posting, you can use Selenium WebDriver to interact with Facebook's UI. However, Facebook API is preferred for most automation tasks. But for simple post automation, here's an example using Selenium.
4. Automating Posting on Twitter
To automate posting on Twitter, you can use Selenium WebDriver to interact with Twitter's UI. However, Twitter's API is more suited for automation, as it allows you to post tweets without needing to interact with the UI. Below is an example using Selenium.
5. Using APIs for Better Automation
While Selenium is a great tool for automating UI interactions, using the official APIs of platforms like Facebook and Twitter is a better and more reliable approach. Both platforms offer APIs for posting content programmatically.
6. Handling Captchas
Social media platforms like Facebook and Twitter may trigger captchas when they detect automated login attempts. To handle captchas, use third-party services like OCR (Optical Character Recognition) APIs or AI-based services like 2Captcha or AntiCaptcha to bypass these challenges.
7. Scheduling Posts
To schedule posts, you can integrate Selenium with scheduling tools like cron jobs (Linux) or use task schedulers on Windows to run your automation scripts at specific intervals.
8. Conclusion
Automating social media posts using Selenium can be a useful technique for businesses and individuals who want to maintain an active social media presence. While Selenium can be used to automate the UI interaction, it's recommended to use the official APIs of Facebook and Twitter for more reliable and scalable automation. Ensure to handle captchas and consider scheduling posts to make the process more efficient.