JavaScript Basics: Understanding the Foundation
1. What is JavaScript?
JavaScript is a versatile, high-level programming language commonly used to create interactive and dynamic web pages. It is a core technology of the web, alongside HTML and CSS, and plays a crucial role in enhancing user experiences by enabling features such as animations, form validations, and real-time content updates.
2. Key Features of JavaScript
- Client-Side Execution: JavaScript runs directly in the browser, making it ideal for creating responsive and interactive user interfaces.
- Event-Driven Programming: JavaScript can respond to user interactions like clicks, mouse movements, and keystrokes, allowing for dynamic updates to the web page.
- Platform Independence: JavaScript is supported by all modern web browsers, making it a universal choice for web development.
- Versatility: JavaScript is not limited to client-side scripting. With environments like Node.js, it can also be used for server-side programming.
- Extensibility: JavaScript can be extended with libraries and frameworks such as React, Angular, and Vue.js to create advanced applications.
3. Common Uses of JavaScript
- Interactive Web Elements: Enhance user experience with features like dropdown menus, modal dialogs, and sliders.
- Form Validation: Validate user input in forms before sending data to the server.
- Dynamic Content: Update web page content without requiring a full reload, using technologies like AJAX or Fetch API.
- Game Development: Create browser-based games with libraries such as Phaser.js.
- Data Visualization: Build interactive charts and graphs using libraries like D3.js or Chart.js.
4. How JavaScript Works
JavaScript code is executed by the browser's JavaScript engine. Here's a simplified overview of its workflow:
- Load the Script: JavaScript code is loaded in the browser through a
<script>
tag in the HTML file. - Interpret the Code: The browser's JavaScript engine interprets the code line by line.
- Execute the Code: The engine executes the code, enabling dynamic interactions and updates on the web page.
5. Examples of JavaScript in Action
- Image Carousel: Use JavaScript to create an automatic or clickable image slider.
- Interactive Forms: Provide real-time feedback to users, such as displaying error messages for incorrect input.
- Single Page Applications (SPAs): Build seamless web applications where navigation does not require full page reloads.
6. Conclusion
JavaScript is a fundamental language for web development, offering powerful capabilities to enhance user experiences. Its versatility and wide adoption make it an essential skill for developers. By leveraging JavaScript’s features, you can create highly interactive, dynamic, and responsive web applications.
Features of JavaScript
1. Lightweight and Interpreted
JavaScript is a lightweight scripting language that is interpreted by the browser's JavaScript engine. This allows for quick execution without the need for compilation.
2. Dynamic Typing
JavaScript uses dynamic typing, meaning variables are not bound to a specific data type. This provides flexibility in writing code but requires careful handling to avoid type-related errors.
3. Cross-Platform Compatibility
JavaScript runs on any device with a modern web browser, making it a cross-platform language that works seamlessly across different operating systems and devices.
4. Event-Driven Programming
JavaScript supports event-driven programming, enabling developers to create responsive and interactive web applications by responding to user actions such as clicks, keystrokes, and mouse movements.
5. Versatility
JavaScript is versatile and can be used for both client-side and server-side development. With frameworks like Node.js, developers can build complete applications using JavaScript alone.
6. Object-Oriented Capabilities
JavaScript supports object-oriented programming (OOP), allowing developers to use concepts like inheritance, encapsulation, and polymorphism to organize and manage complex codebases.
7. Rich Libraries and Frameworks
JavaScript has a vast ecosystem of libraries and frameworks such as React, Angular, and Vue.js that simplify the development of complex applications and enhance productivity.
8. Asynchronous Programming
With features like promises, async/await, and callbacks, JavaScript efficiently handles asynchronous tasks, such as fetching data from servers or interacting with APIs, without blocking the main thread.
9. High Performance
Modern JavaScript engines, such as Google's V8, optimize code execution, making JavaScript capable of handling performance-intensive tasks efficiently.
10. Integrations
JavaScript integrates seamlessly with HTML and CSS, making it an essential part of web development. It also works well with APIs, databases, and other technologies to build robust applications.
11. Security Features
JavaScript includes built-in security features, such as Content Security Policy (CSP) and techniques to prevent Cross-Site Scripting (XSS) attacks, ensuring safer web applications.
12. Community Support
JavaScript has a vast and active community of developers worldwide, providing extensive resources, tutorials, and tools for learning and troubleshooting.
Conclusion
The features of JavaScript make it a powerful and widely-used language for web development. Its flexibility, scalability, and rich ecosystem enable developers to create interactive and efficient web applications, making it an indispensable tool in modern development.
JavaScript vs. Other Programming Languages
1. JavaScript vs. Java
Despite their similar names, JavaScript and Java are distinct languages with different use cases:
- JavaScript: A lightweight, interpreted scripting language primarily used for web development. It runs in browsers and is dynamic and loosely typed.
- Java: A statically typed, compiled programming language used for building robust, platform-independent applications. It is commonly used for enterprise-level backend systems, mobile apps (via Android), and desktop applications.
2. JavaScript vs. Python
Both JavaScript and Python are high-level languages, but they differ significantly:
- JavaScript: Best suited for web development, providing dynamic interactivity on websites. It excels in asynchronous programming and has extensive frontend and backend frameworks like React and Node.js.
- Python: A versatile language with a simple syntax, popular for data science, machine learning, web development (Django, Flask), and scripting. It is better suited for computationally intensive tasks.
3. JavaScript vs. C++
JavaScript and C++ are vastly different in purpose and design:
- JavaScript: A high-level, interpreted language designed for web development. It is easy to learn and doesn't require manual memory management.
- C++: A low-level, compiled language offering fine-grained control over memory and hardware. It is used for system programming, game development, and performance-critical applications.
4. JavaScript vs. PHP
JavaScript and PHP are often compared in the context of web development:
- JavaScript: Primarily used for frontend and, with Node.js, for backend development. It allows developers to work on both client-side and server-side with a single language.
- PHP: A server-side scripting language specifically designed for web development. It is widely used for building dynamic websites and powering content management systems like WordPress.
5. JavaScript vs. Ruby
JavaScript and Ruby share some similarities but are used in different domains:
- JavaScript: Known for its versatility in building interactive web applications and its extensive ecosystem of libraries and frameworks.
- Ruby: A dynamic, object-oriented scripting language popular for web development, especially with the Ruby on Rails framework. It prioritizes simplicity and developer productivity.
6. JavaScript vs. Go
JavaScript and Go (Golang) serve different purposes and audiences:
- JavaScript: The dominant language for web development, with a focus on interactivity and asynchronous programming.
- Go: A statically typed, compiled language created by Google. It is designed for simplicity, concurrency, and scalability, making it ideal for building server-side applications and microservices.
7. JavaScript vs. Swift
JavaScript and Swift target different platforms:
- JavaScript: Runs in browsers or on servers with Node.js. It is platform-agnostic and widely used for web applications.
- Swift: A statically typed, compiled language developed by Apple for building iOS, macOS, watchOS, and tvOS applications. It is optimized for Apple's ecosystem.
Conclusion
JavaScript stands out for its unparalleled role in web development, offering flexibility for both frontend and backend tasks. While other languages like Python, Java, and C++ excel in specific domains, JavaScript's ubiquity and ecosystem make it an essential tool for modern developers.
How Browsers Interpret JavaScript
1. The Role of JavaScript in Browsers
Browsers use JavaScript to make web pages interactive and dynamic. While HTML defines the structure and CSS handles styling, JavaScript adds behavior, enabling features like form validation, animations, and event handling.
2. JavaScript Engines
Browsers have built-in JavaScript engines to execute JavaScript code. Examples include:
- V8: Used in Google Chrome and Node.js.
- SpiderMonkey: Used in Mozilla Firefox.
- JavaScriptCore (Nitro): Used in Apple Safari.
- Chakra: Used in older versions of Microsoft Edge.
These engines parse, compile, and execute JavaScript code efficiently, often optimizing it for better performance.
3. Steps in JavaScript Execution
When a browser encounters JavaScript code, it follows these steps:
- Parsing: The engine reads the JavaScript code and converts it into an Abstract Syntax Tree (AST), representing the code's structure.
- Compilation: The AST is compiled into machine code. Modern engines use Just-In-Time (JIT) compilation to optimize performance dynamically.
- Execution: The engine executes the machine code, interacting with the browser's APIs for DOM manipulation, event handling, and more.
4. JavaScript in the DOM
JavaScript interacts with the Document Object Model (DOM) to modify web pages in real-time:
- Accessing Elements: Methods like
document.getElementById
orquerySelector
are used to select elements. - Manipulating Content: You can change text, attributes, or styles of elements dynamically.
- Event Handling: JavaScript listens for user interactions like clicks, keypresses, or form submissions and responds accordingly.
5. Asynchronous JavaScript
Browsers handle asynchronous JavaScript using mechanisms like:
- Event Loop: Ensures non-blocking execution by managing tasks, microtasks, and rendering.
- Promises and Async/Await: Simplifies handling of asynchronous operations like fetching data from APIs.
- Web APIs: Browser-provided APIs like
setTimeout
, Fetch API, and DOM events allow asynchronous functionality.
6. Optimization Techniques
Modern browsers optimize JavaScript execution through:
- Lazy Compilation: Compiling only the necessary parts of the code.
- Inline Caching: Speeding up property access by caching type information.
- Garbage Collection: Automatically reclaiming memory by removing unused objects.
7. Limitations of JavaScript in Browsers
While JavaScript is powerful, it has limitations in browsers:
- It runs in a single-threaded environment, though Web Workers can handle multi-threading.
- Execution is sandboxed for security, restricting access to the file system and other sensitive resources.
Conclusion
Browsers interpret JavaScript through sophisticated engines, enabling dynamic and interactive web experiences. Understanding how JavaScript works in browsers helps developers write efficient, optimized, and secure code.
Writing Your First JavaScript Program
1. Setting Up Your Environment
To write and execute JavaScript, you need the following:
- A Text Editor: Use editors like Visual Studio Code, Sublime Text, or any text editor of your choice.
- A Web Browser: Modern browsers like Google Chrome, Firefox, or Edge come with built-in JavaScript engines to run your code.
2. Writing JavaScript Inline in HTML
To begin, create a simple HTML file and include JavaScript using the <script>
tag:
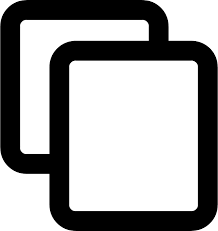
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>First JavaScript Program</title>
</head>
<body>
<h1>Welcome to JavaScript!</h1>
<script>
alert('Hello, World!');
</script>
</body>
</html>
Save this file as index.html
and open it in a browser. You’ll see an alert box with the message “Hello, World!”
3. Writing External JavaScript
For better organization, you can write JavaScript in an external file and link it to your HTML:
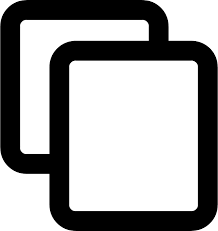
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>External JavaScript</title>
<script src="script.js"></script>
</head>
<body>
<h1>Welcome to External JavaScript!</h1>
</body>
</html>
Then, create a file named script.js
in the same directory and add this code:
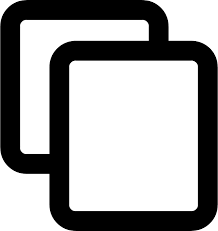
console.log('Hello from an external file!');
Open the HTML file in a browser and check the console (press F12 or Ctrl+Shift+I) to see the message.
4. Basic JavaScript Syntax
Here are some basic concepts for your first JavaScript program:
- Variables: Use
let
,const
, orvar
to declare variables. Example:let message = 'Hello, JavaScript!'; console.log(message);
- Functions: Write reusable blocks of code:
function greet() { console.log('Welcome to JavaScript programming!'); } greet();
- Events: Add interactivity by responding to user actions:
<button onclick="alert('Button clicked!')">Click Me</button>
5. Running JavaScript in the Browser Console
You can also test JavaScript directly in your browser's developer console:
- Open your browser and press F12 or Ctrl+Shift+I.
- Navigate to the “Console” tab.
- Type
console.log('Hello from the console!');
and press Enter. You’ll see the output in the console.
Conclusion
Writing your first JavaScript program is straightforward. Start with simple scripts and gradually explore more advanced features as you become comfortable. JavaScript is a versatile language, and mastering it opens up endless possibilities for web development.
JavaScript Syntax and Statements
1. JavaScript Syntax Basics
JavaScript syntax refers to the set of rules that define a correctly structured JavaScript program. It includes the following elements:
- Case Sensitivity: JavaScript is case-sensitive, meaning
myVar
andmyvar
would be treated as different variables. - Semicolons: Statements in JavaScript are usually terminated with a semicolon (
;
), although it is optional in most cases due to automatic semicolon insertion (ASI). - Whitespace: Whitespace such as spaces, tabs, and new lines are generally ignored, except when it affects the meaning of the code (e.g., within strings or variable names).
- Comments: Use comments to explain your code. There are two types of comments in JavaScript:
- Single-line comment:
// This is a comment
- Multi-line comment:
/* This is a multi-line comment */
- Single-line comment:
2. JavaScript Statements
In JavaScript, a statement is a complete unit of execution. It can be a variable declaration, a function call, a condition, or a loop. Some common types of statements include:
- Variable Declaration: Assigning a value to a variable:
let name = 'Alice';
- Assignment Statement: Assigning a new value to an existing variable:
name = 'Bob';
- Function Call: Invoking a function:
console.log('Hello, World!');
- Conditional Statement: Making decisions in code:
if (name === 'Alice') { console.log('Hello, Alice!'); } else { console.log('Hello, Stranger!'); }
- Loop Statement: Repeating a set of instructions:
for (let i = 0; i < 5; i++) { console.log(i); }
3. JavaScript Expressions
In JavaScript, an expression is any valid unit of code that resolves to a value. Examples of expressions include:
- Arithmetic Expressions: Performing mathematical operations:
let result = 10 + 5;
- String Expressions: Concatenating strings:
let greeting = 'Hello, ' + 'World!';
- Boolean Expressions: Testing conditions:
let isAdult = age >= 18;
4. JavaScript Blocks and Indentation
JavaScript code is often organized into blocks, which are groups of statements enclosed by curly braces ({}
). Proper indentation helps make the code more readable, though it’s not strictly required for execution:
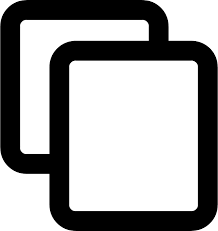
if (age >= 18) {
console.log('You are an adult.');
} else {
console.log('You are not an adult.');
}
5. JavaScript Keywords
JavaScript has reserved keywords that cannot be used as variable names. Some common keywords include:
let
,const
,var
: Used to declare variables.if
,else
,switch
: Used in conditional statements.for
,while
,do
: Used in loop statements.return
: Used to return a value from a function.function
: Used to declare functions.
6. JavaScript Block Scope and Global Scope
JavaScript has two types of variable scopes:
- Global Scope: Variables declared outside of functions are available throughout the program.
- Block Scope: Variables declared inside a block (like a loop or an if statement) are only accessible within that block when using
let
orconst
.
Conclusion
JavaScript syntax and statements define the rules for writing meaningful programs. By understanding and following these rules, you can write clean, efficient, and functional JavaScript code. Always remember to use proper indentation and naming conventions to enhance code readability and maintainability.
Variables and Constants (var, let, const)
1. Introduction to Variables
In JavaScript, variables are containers for storing data values. You can declare variables using the var
, let
, or const
keywords. The choice of keyword determines the scope and mutability of the variable.
2. The var
Keyword
The var
keyword is the oldest way to declare variables in JavaScript. However, it has some issues related to scope and hoisting:
- Function Scope: Variables declared with
var
are scoped to the nearest function or globally if declared outside a function. - Hoisting: Variables declared using
var
are hoisted to the top of their scope, meaning they are accessible before the declaration, but they are undefined until initialization.
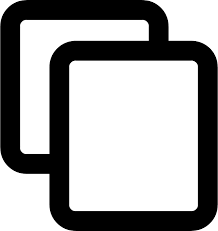
var name = 'Alice';
Example of hoisting:
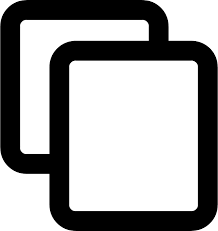
console.log(age); // undefined
var age = 25;
3. The let
Keyword
The let
keyword was introduced in ECMAScript 6 (ES6) to address the issues with var
. It provides block-level scope, which is more predictable and safer to use in modern JavaScript:
- Block Scope: Variables declared with
let
are scoped to the nearest enclosing block, such as within a loop or an if statement. - No Hoisting: Variables declared with
let
are not hoisted. You cannot access them before declaration.
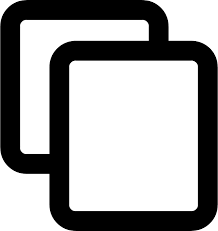
let age = 25;
Example of block scope:
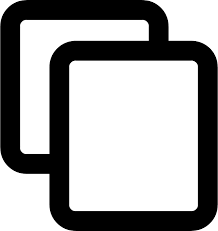
if (true) {
let name = 'Bob';
console.log(name); // Bob
}
console.log(name); // ReferenceError: name is not defined
4. The const
Keyword
The const
keyword is used to declare variables whose value cannot be reassigned. Like let
, const
also has block-level scope:
- Block Scope: Variables declared with
const
are scoped to the nearest enclosing block. - Immutability: The value assigned to a
const
variable cannot be reassigned. However, if the value is an object or array, the contents of the object or array can still be modified (but the reference itself cannot change).
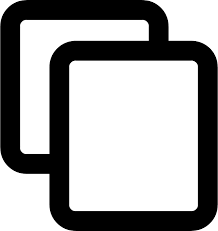
const pi = 3.14;
Example of immutability:
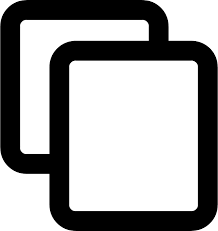
const person = { name: 'Alice', age: 25 };
person.age = 26; // This is allowed
person = {}; // TypeError: Assignment to constant variable
5. Choosing Between var, let, and const
When declaring variables, choose the appropriate keyword based on the intended use:
- Use
const
: When you don't need to reassign the variable after initialization (e.g., constants or values that shouldn't change). - Use
let
: When the variable's value needs to change, and you want block-scoping for better control over the variable's lifecycle. - Avoid
var
: It’s generally recommended to avoid usingvar
in modern JavaScript, as it has unpredictable behavior due to function scoping and hoisting.
6. Example of Using Variables and Constants
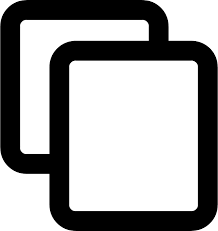
let temperature = 30; // Variable declared with let
if (temperature > 25) {
const message = 'It\'s a hot day!'; // Block-scoped constant
console.log(message); // It's a hot day!
}
// console.log(message); // ReferenceError: message is not defined
7. Conclusion
Understanding the differences between var
, let
, and const
is crucial for writing cleaner, more predictable JavaScript code. By using let
and const
appropriately, you can avoid common pitfalls such as variable hoisting and unexpected reassignment, making your code more maintainable and easier to understand.
Arithmetic Operators
1. Introduction to Arithmetic Operators
Arithmetic operators are used to perform mathematical operations on numbers. JavaScript provides a set of basic arithmetic operators that allow you to carry out operations such as addition, subtraction, multiplication, division, and more. These operators are essential for performing calculations and manipulating numeric data in JavaScript programs.
2. List of Arithmetic Operators
Here are the common arithmetic operators available in JavaScript:
- Addition (+): Adds two operands together. Example:
let sum = 10 + 5; // Result: 15
- Subtraction (-): Subtracts the right operand from the left operand. Example:
let difference = 10 - 5; // Result: 5
- Multiplication (*): Multiplies two operands. Example:
let product = 10 * 5; // Result: 50
- Division (/): Divides the left operand by the right operand. Example:
let quotient = 10 / 5; // Result: 2
- Modulus (%): Returns the remainder of a division operation. Example:
let remainder = 10 % 3; // Result: 1
- Exponentiation (**): Performs exponentiation (raises the base to the power of the exponent). Example:
let power = 2 ** 3; // Result: 8
3. Examples of Arithmetic Operators
Here are some examples of how to use arithmetic operators in JavaScript:
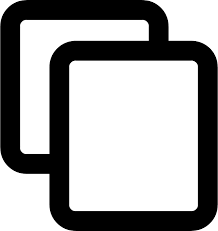
let a = 10;
let b = 5;
let addition = a + b; // 15
let subtraction = a - b; // 5
let multiplication = a * b; // 50
let division = a / b; // 2
let modulus = a % b; // 0
let exponentiation = a ** b; // 100000
4. Operator Precedence
In JavaScript, like in many programming languages, operators follow a specific order of precedence, which determines the order in which operations are performed. Arithmetic operators have the following precedence:
- Exponentiation (
**
) has the highest precedence. - Multiplication (
*
), division (/
), and modulus (%
) are evaluated next. - Addition (
+
) and subtraction (-
) are evaluated last.
For example:
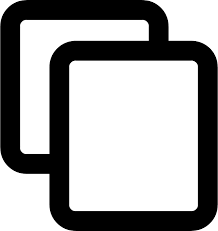
let result = 2 + 3 * 4; // Result: 14 (multiplication happens before addition)
If you want to change the order of operations, you can use parentheses:
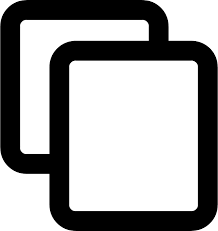
let result = (2 + 3) * 4; // Result: 20 (addition happens before multiplication)
5. Increment and Decrement Operators
In addition to basic arithmetic operators, JavaScript provides increment (++
) and decrement (--
) operators that are used to increase or decrease a variable's value by 1.
- Increment (
++
): Increases a variable's value by 1. Example:let x = 10; x++; // Result: 11
- Decrement (
--
): Decreases a variable's value by 1. Example:let x = 10; x--; // Result: 9
6. Conclusion
Arithmetic operators in JavaScript allow you to perform basic mathematical operations like addition, subtraction, multiplication, division, and modulus. These operators are essential for working with numeric values and performing calculations in your JavaScript programs. Understanding operator precedence and using increment and decrement operators effectively will help you write more efficient and accurate code.
Comparison and Logical Operators
1. Introduction to Comparison and Logical Operators
Comparison and logical operators are used to compare values and perform logical operations in JavaScript. Comparison operators are used to compare values and return a boolean result (true or false), while logical operators are used to combine multiple conditions.
2. Comparison Operators
Comparison operators are used to compare two values. They return a boolean value indicating whether the comparison is true or false.
- Equal to (==): Compares two values for equality, ignoring their data type. Example:
5 == '5' // true
- Strict equal (===): Compares two values for equality, considering both the value and the data type. Example:
5 === '5' // false
- Not equal to (!=): Compares two values for inequality, ignoring their data type. Example:
5 != '5' // false
- Strict not equal to (!==): Compares two values for inequality, considering both the value and the data type. Example:
5 !== '5' // true
- Greater than (>): Checks if the left value is greater than the right value. Example:
10 > 5 // true
- Greater than or equal to (>=): Checks if the left value is greater than or equal to the right value. Example:
10 >= 5 // true
- Less than (<): Checks if the left value is less than the right value. Example:
5 < 10 // true
- Less than or equal to (<=): Checks if the left value is less than or equal to the right value. Example:
5 <= 10 // true
3. Logical Operators
Logical operators are used to perform logical operations on boolean values and return a boolean result.
- Logical AND (&&): Returns true if both operands are true. Example:
true && false // false
- Logical OR (||): Returns true if at least one of the operands is true. Example:
true || false // true
- Logical NOT (!): Inverts the boolean value of the operand. Example:
!true // false
4. Combining Comparison and Logical Operators
You can combine comparison and logical operators to create complex conditions.
For example:
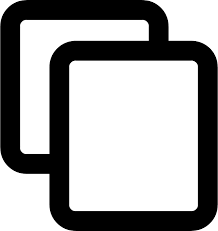
let x = 10;
let y = 5;
if (x > y && x !== 5) {
console.log("x is greater than y and not equal to 5");
}
In this example, both conditions are true, so the block of code will execute.
5. Short-circuit Evaluation
Logical operators like &&
and ||
use short-circuit evaluation, which means they evaluate the operands from left to right and stop as soon as the result is determined.
- AND (&&): If the first operand is false, the second operand is not evaluated because the result will always be false.
- OR (||): If the first operand is true, the second operand is not evaluated because the result will always be true.
For example:
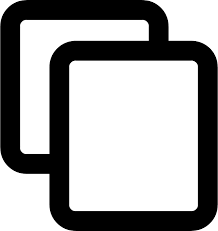
let a = false;
let b = true;
let result = a && b; // result is false (b is not evaluated)
6. Conclusion
Comparison and logical operators are fundamental tools in JavaScript for comparing values and combining conditions. By using comparison operators, you can check for equality, inequality, and relative values, while logical operators allow you to combine multiple conditions effectively. Understanding how to use these operators is key to writing complex conditional statements and controlling the flow of your JavaScript programs.
Assignment Operators
1. Introduction to Assignment Operators
Assignment operators are used to assign values to variables. In JavaScript, the assignment operator is the equals sign (=
), but there are also shorthand assignment operators that combine assignment with other operations.
2. Basic Assignment Operator (=
)
The basic assignment operator is used to assign a value to a variable. The syntax is:
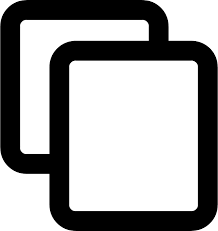
variable = value;
For example:
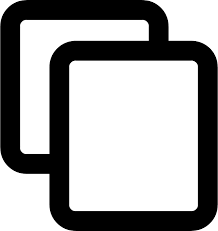
let x = 10;
In this case, the value 10
is assigned to the variable x
.
3. Compound Assignment Operators
JavaScript also provides shorthand assignment operators that combine an arithmetic operation with assignment. These operators are used to perform an operation and assign the result to the same variable.
- Addition assignment (
+=
): Adds a value to a variable and assigns the result back to that variable. - Subtraction assignment (
-=
): Subtracts a value from a variable and assigns the result back to that variable. - Multiplication assignment (
*=
): Multiplies a variable by a value and assigns the result back to that variable. - Division assignment (
/=
): Divides a variable by a value and assigns the result back to that variable. - Remainder assignment (
%=
): Assigns the remainder of dividing the variable by a value. - Exponentiation assignment (
**=
): Raises a variable to the power of a value and assigns the result back to that variable.
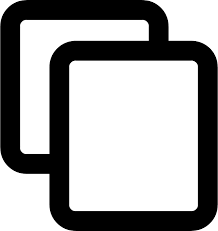
x += 5; // equivalent to x = x + 5;
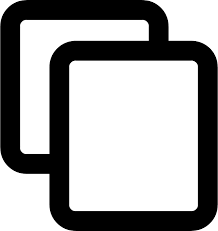
x -= 5; // equivalent to x = x - 5;
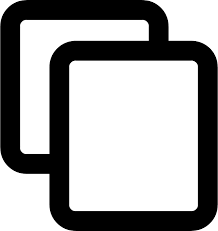
x *= 5; // equivalent to x = x * 5;
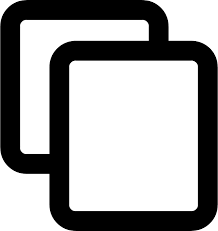
x /= 5; // equivalent to x = x / 5;
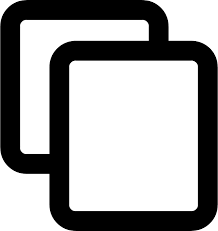
x %= 5; // equivalent to x = x % 5;
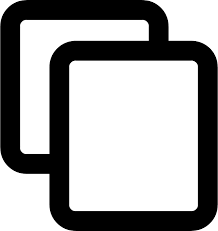
x **= 5; // equivalent to x = x ** 5;
4. Example of Compound Assignment
Here's an example using compound assignment operators:
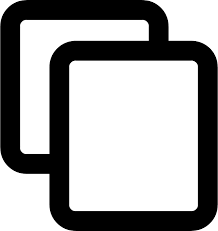
let x = 10;
x += 5; // x is now 15
x *= 2; // x is now 30
x -= 10; // x is now 20
5. Destructuring Assignment
Destructuring assignment allows you to extract values from arrays or objects and assign them to variables in a concise way. It is a feature introduced in ES6 (ECMAScript 2015).
Array Destructuring
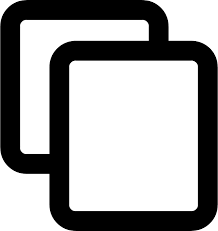
let [a, b] = [1, 2];
console.log(a); // 1
console.log(b); // 2
Object Destructuring
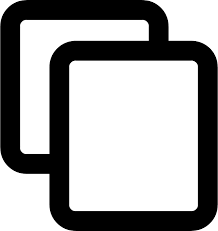
let {name, age} = {name: 'John', age: 30};
console.log(name); // John
console.log(age); // 30
6. Conclusion
Assignment operators are an essential part of JavaScript, enabling you to assign values to variables and perform operations on them in a concise manner. The basic assignment operator (=
) is often used, but compound assignment operators like +=
, *=
, and others provide shortcuts for common arithmetic operations. Destructuring assignment offers a more powerful and concise way to assign values from arrays and objects to variables.
Ternary Operator
1. Introduction to the Ternary Operator
The ternary operator is a shorthand for an if-else
statement. It is the only operator in JavaScript that takes three operands. The syntax of the ternary operator is:
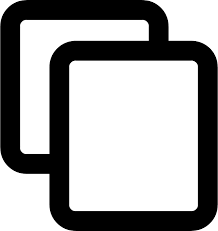
condition ? expression1 : expression2;
If the condition evaluates to true
, expression1
is executed. If the condition evaluates to false
, expression2
is executed.
2. Syntax of the Ternary Operator
Here is the basic structure of the ternary operator:
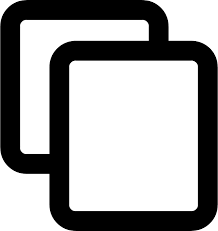
condition ? value_if_true : value_if_false;
3. Example of the Ternary Operator
The following example demonstrates how the ternary operator works:
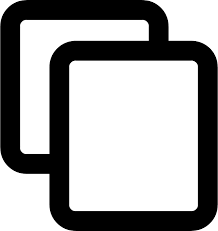
let age = 18;
let message = (age >= 18) ? "Adult" : "Minor";
console.log(message); // Output: Adult
In this case, since the condition age >= 18
is true
, the value "Adult"
is assigned to the message
variable.
4. Using Ternary Operator for Multiple Conditions
You can also chain multiple ternary operators to check for multiple conditions. Here's an example:
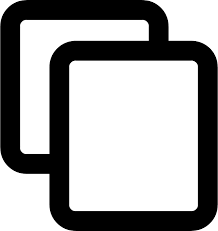
let age = 16;
let message = (age >= 18) ? "Adult" : (age >= 13) ? "Teenager" : "Child";
console.log(message); // Output: Teenager
In this example, the ternary operator checks if the age is greater than or equal to 18, then if it is greater than or equal to 13, and finally returns "Child" if none of the conditions are met.
5. Benefits of Using the Ternary Operator
- Concise Code: The ternary operator allows you to express conditional logic in a single line, making the code more concise.
- Improved Readability: It can improve readability when the condition is simple and when used for simple assignments.
6. Caveats of Using the Ternary Operator
While the ternary operator can make your code more concise, using it excessively or for complex conditions can decrease readability. It's important to use it appropriately in situations where the conditional logic is simple.
7. Conclusion
The ternary operator is a powerful and concise way to handle conditional logic in JavaScript. It allows you to evaluate expressions and assign values in a single line. However, for more complex conditions or logic, it is often better to use a traditional if-else
statement for clarity.
If, Else If, and Else Statements
1. Introduction to Conditional Statements
Conditional statements in JavaScript allow you to make decisions in your code. Based on certain conditions, you can control the flow of your program. The most common conditional statements are if
, else if
, and else
.
2. The if
Statement
The if
statement evaluates a condition. If the condition is true
, the code inside the block will be executed. Here's the basic syntax:
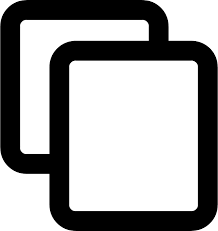
if (condition) {
// code to be executed if the condition is true
}
Example:
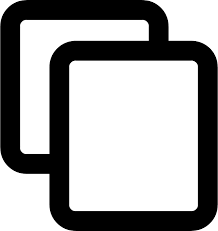
let age = 20;
if (age >= 18) {
console.log("You are an adult.");
}
In this example, if the age
is 18 or more, the message "You are an adult." will be logged to the console.
3. The else if
Statement
If you have multiple conditions to check, you can use the else if
statement after an if
statement. It allows you to check additional conditions if the initial condition is false
.
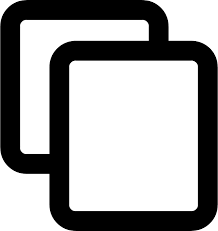
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
}
Example:
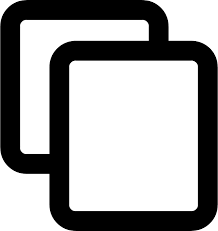
let age = 16;
if (age >= 18) {
console.log("You are an adult.");
} else if (age >= 13) {
console.log("You are a teenager.");
}
In this case, if the age
is 16, the message "You are a teenager." will be logged.
4. The else
Statement
The else
statement allows you to execute code when none of the preceding if
or else if
conditions are true
. It's a catch-all option for handling cases when all other conditions fail.
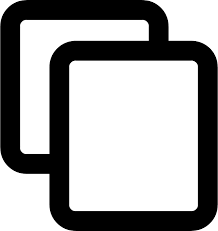
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
} else {
// code if none of the conditions are true
}
Example:
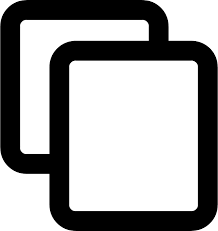
let age = 10;
if (age >= 18) {
console.log("You are an adult.");
} else if (age >= 13) {
console.log("You are a teenager.");
} else {
console.log("You are a child.");
}
In this example, the message "You are a child." will be logged because none of the other conditions are true.
5. Multiple Conditions with Logical Operators
You can combine multiple conditions in a single if
, else if
, or else
statement using logical operators like &&
(AND) and ||
(OR). Here's an example using both:
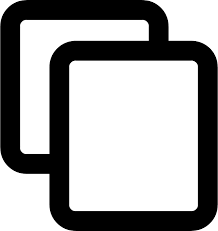
let age = 20;
let isStudent = true;
if (age >= 18 && isStudent) {
console.log("You are an adult student.");
} else if (age >= 18) {
console.log("You are an adult, but not a student.");
} else {
console.log("You are a minor.");
}
In this case, the message "You are an adult student." will be logged because both conditions are true
.
6. Conclusion
The if
, else if
, and else
statements are fundamental for controlling the flow of your program based on conditions. By using these statements effectively, you can create more dynamic and interactive JavaScript applications.
Switch Statement
1. Introduction to the switch
Statement
The switch
statement is used to perform one out of many possible actions based on the value of a variable or expression. It is often used as an alternative to multiple if
statements when there are many conditions to check.
2. Syntax of the switch
Statement
The basic syntax of the switch
statement is as follows:
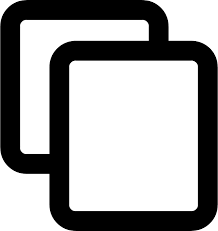
switch (expression) {
case value1:
// code block to be executed if expression === value1
break;
case value2:
// code block to be executed if expression === value2
break;
default:
// code block to be executed if none of the cases match
}
The expression
is evaluated once, and its value is compared with each case
value. If a match is found, the corresponding block of code is executed. The break
statement is used to terminate the switch
block once a case is matched. If no cases match, the default
case is executed.
3. Example of Using the switch
Statement
Here’s an example of how to use the switch
statement to check the value of a variable:
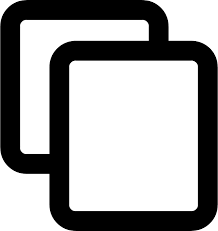
let day = 3;
switch (day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
case 4:
console.log("Thursday");
break;
case 5:
console.log("Friday");
break;
case 6:
console.log("Saturday");
break;
case 7:
console.log("Sunday");
break;
default:
console.log("Invalid day");
}
In this example, the message "Wednesday" will be logged because the value of the day
variable is 3.
4. Using the switch
Statement with Multiple Cases
You can group multiple cases together when the behavior for those cases is the same. This is called "fall-through." Here's an example:
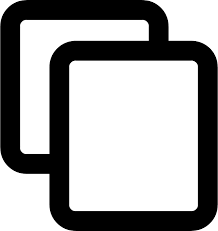
let fruit = "apple";
switch (fruit) {
case "apple":
case "banana":
case "cherry":
console.log("This is a fruit.");
break;
default:
console.log("This is not a fruit.");
}
In this case, if the fruit
is either "apple," "banana," or "cherry," the message "This is a fruit." will be logged.
5. The default
Case
The default
case is optional, but it’s useful for catching any values that don’t match any of the specified cases. It’s a good practice to include a default
case at the end to handle unexpected values.
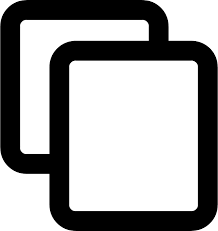
let color = "blue";
switch (color) {
case "red":
console.log("The color is red.");
break;
case "green":
console.log("The color is green.");
break;
default:
console.log("Unknown color.");
}
In this example, since the value of color
is "blue," the message "Unknown color." will be logged because it does not match any of the specified cases.
6. Conclusion
The switch
statement is a powerful tool for handling multiple conditions in a more readable way than using multiple if
statements. It's particularly useful when you have a fixed set of possible values for an expression, such as checking for days of the week, menu options, or status codes.
Loops (for, while, do-while)
1. Introduction to Loops in JavaScript
Loops are used to execute a block of code repeatedly as long as a specified condition is true. JavaScript provides several types of loops, including the for
, while
, and do-while
loops. Each loop has its own specific use case depending on the condition you want to check and how often you want the loop to run.
2. The for
Loop
The for
loop is generally used when the number of iterations is known. It consists of three parts: initialization, condition, and increment/decrement.
Syntax of the for
loop:
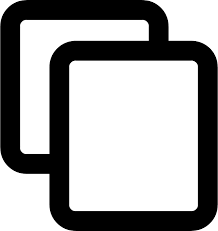
for (initialization; condition; increment/decrement) {
// code to be executed
}
Here’s an example of a for
loop that prints numbers from 1 to 5:
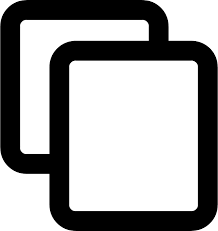
for (let i = 1; i <= 5; i++) {
console.log(i);
}
This loop starts by initializing i
to 1. The condition checks if i
is less than or equal to 5. After each iteration, i
is incremented by 1. The loop will stop once the condition is no longer true.
3. The while
Loop
The while
loop is used when you want the loop to continue as long as a specified condition is true. It checks the condition before each iteration.
Syntax of the while
loop:
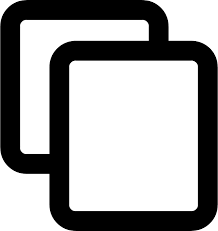
while (condition) {
// code to be executed
}
Example of a while
loop that prints numbers from 1 to 5:
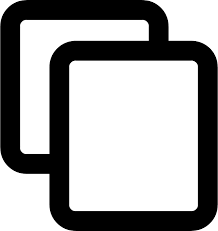
let i = 1;
while (i <= 5) {
console.log(i);
i++;
}
In this example, the condition i <= 5
is checked before each iteration. If the condition is true, the code inside the loop is executed. The loop continues until the condition becomes false.
4. The do-while
Loop
The do-while
loop is similar to the while
loop, except that it checks the condition after executing the code block, ensuring that the code is executed at least once, even if the condition is false.
Syntax of the do-while
loop:
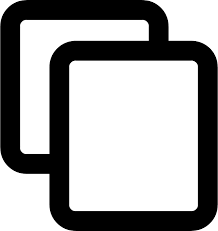
do {
// code to be executed
} while (condition);
Example of a do-while
loop that prints numbers from 1 to 5:
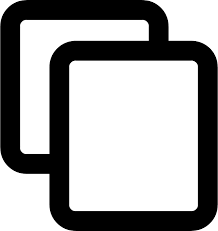
let i = 1;
do {
console.log(i);
i++;
} while (i <= 5);
In this example, the code block will execute at least once. After the first iteration, the condition i <= 5
is checked. If it's still true, the loop continues.
5. Choosing the Right Loop
Choose the type of loop based on the situation:
for
loop: Use when the number of iterations is known in advance or when you're iterating over arrays or collections.while
loop: Use when the number of iterations is not known, and you need to repeat an operation until a certain condition is met.do-while
loop: Use when you want to ensure that the code inside the loop runs at least once before checking the condition.
6. Conclusion
JavaScript provides three main types of loops: for
, while
, and do-while
. Each loop has its use case, and choosing the right loop depends on the specific requirements of your task. Understanding how to use these loops effectively is key to writing efficient and clean code.
Break and Continue
1. Introduction to Break and Continue
In JavaScript, break
and continue
are control flow statements used inside loops to alter the flow of execution. They help in controlling the iteration process based on specific conditions.
2. The break
Statement
The break
statement is used to exit or "break" out of a loop or a switch statement prematurely. Once the break
statement is executed, the program continues executing the code that follows the loop or switch statement.
Syntax of the break
statement:
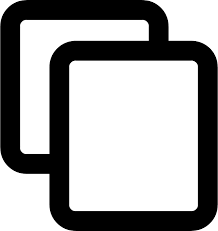
break;
Example of using break
inside a for
loop:
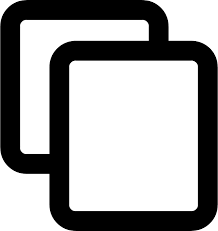
for (let i = 1; i <= 10; i++) {
if (i === 5) {
break; // Exit the loop when i equals 5
}
console.log(i);
}
In this example, the loop will print numbers from 1 to 4, and then it will break when i
equals 5, so the loop will terminate early.
3. The continue
Statement
The continue
statement is used to skip the current iteration of a loop and move to the next iteration. It doesn't terminate the loop entirely but skips the code that follows it for the current loop iteration.
Syntax of the continue
statement:
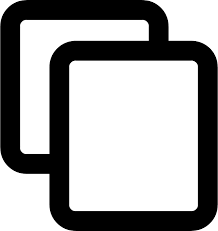
continue;
Example of using continue
inside a for
loop:
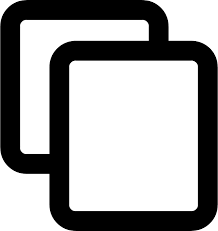
for (let i = 1; i <= 10; i++) {
if (i === 5) {
continue; // Skip the current iteration when i equals 5
}
console.log(i);
}
In this example, the loop will print numbers from 1 to 10, except for 5, as the continue
statement skips that iteration when i
equals 5.
4. Using break
and continue
in while
and do-while
Loops
Both break
and continue
can also be used in while
and do-while
loops, with similar behavior as in the for
loop:
Example of using break
in a while
loop:
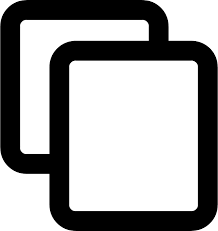
let i = 1;
while (i <= 10) {
if (i === 5) {
break; // Exit the loop when i equals 5
}
console.log(i);
i++;
}
Example of using continue
in a do-while
loop:
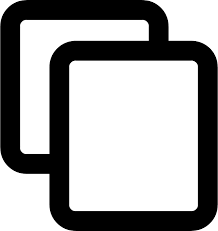
let i = 1;
do {
if (i === 5) {
i++;
continue; // Skip the iteration when i equals 5
}
console.log(i);
i++;
} while (i <= 10);
5. Best Practices for Using break
and continue
- Use
break
to exit loops early when a condition is met, especially when you no longer need to continue iterating once a specific condition is true. - Use
continue
when you need to skip a single iteration in the loop, but continue looping for the rest of the iterations. - Avoid overuse of
break
andcontinue
in complex loops, as it can make the code harder to read and maintain. Try to keep the loop logic simple and clear.
6. Conclusion
The break
and continue
statements provide useful ways to control the flow of loops in JavaScript. While break
exits the loop immediately, continue
skips the current iteration and proceeds with the next one. Using them effectively can help you manage loop execution based on specific conditions.
Declaring and Calling Functions
1. Introduction to Functions
In JavaScript, a function is a block of code designed to perform a particular task. Functions allow you to group reusable code that can be executed multiple times. You can declare a function and then call it when needed.
2. Declaring a Function
To declare a function, you use the function
keyword followed by the function name, parentheses for parameters, and a block of code inside curly braces.
Syntax for declaring a function:
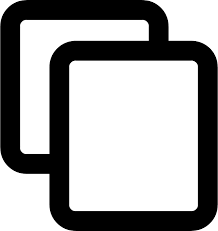
function functionName(parameters) {
// function body
// code to be executed
}
Example of declaring a simple function:
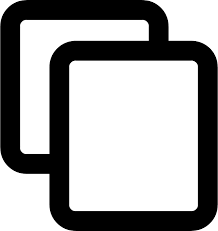
function greet() {
console.log('Hello, world!');
}
This declares a function named greet
that, when called, will log "Hello, world!" to the console.
3. Calling a Function
Once a function is declared, you can call it by using its name followed by parentheses. If the function takes parameters, you pass the values inside the parentheses.
Syntax for calling a function:
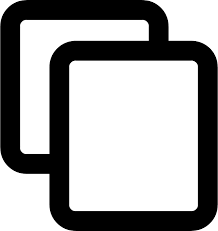
functionName(arguments);
Example of calling the greet
function:
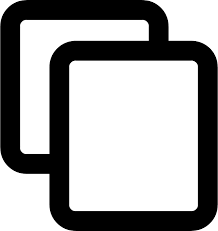
greet(); // Output: 'Hello, world!'
This will execute the function and print "Hello, world!" to the console.
4. Functions with Parameters
Functions can accept parameters, which are values passed into the function when it is called. These parameters are used inside the function body to perform calculations or other operations.
Syntax for declaring a function with parameters:
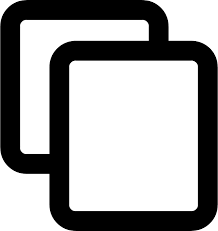
function functionName(parameter1, parameter2) {
// function body
// code to use parameters
}
Example of declaring a function with parameters:
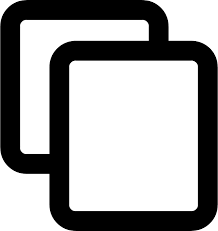
function greet(name) {
console.log('Hello, ' + name + '!');
}
Now, when calling the function, you pass an argument for the name
parameter:
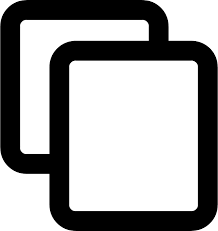
greet('Alice'); // Output: 'Hello, Alice!'
In this example, the function greet
accepts a name
parameter and logs a personalized greeting message to the console.
5. Functions with Return Values
Functions can also return values. You use the return
keyword to return a value from a function. This allows you to use the function's result in other parts of your code.
Syntax for returning a value from a function:
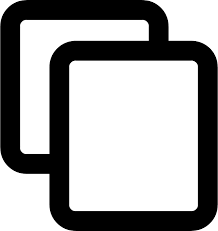
function functionName() {
return value;
}
Example of a function that returns a value:
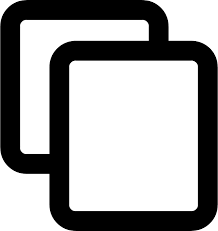
function add(a, b) {
return a + b;
}
Calling the add
function and storing the result:
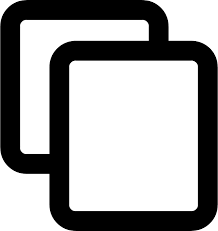
let result = add(3, 4);
console.log(result); // Output: 7
In this example, the function add
returns the sum of a
and b
, and we store the result in the result
variable.
6. Function Expressions
Functions can also be assigned to variables. This is known as a function expression. A function expression allows you to create functions dynamically at runtime.
Syntax for a function expression:
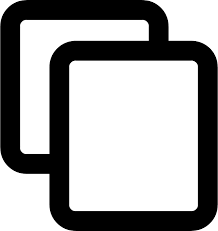
const functionName = function() {
// function body
};
Example of a function expression:
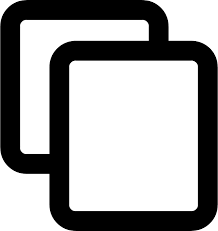
const greet = function(name) {
console.log('Hello, ' + name + '!');
};
Now, you can call the function using the variable name:
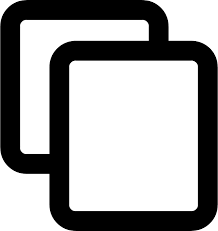
greet('Bob'); // Output: 'Hello, Bob!'
7. Arrow Functions
Arrow functions are a shorter syntax for writing functions in JavaScript. They are especially useful for anonymous functions and function expressions.
Syntax for an arrow function:
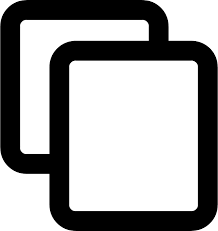
const functionName = (parameters) => {
// function body
};
Example of an arrow function:
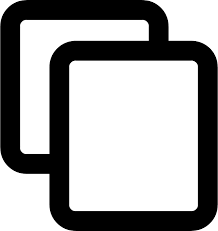
const add = (a, b) => a + b;
Calling the arrow function:
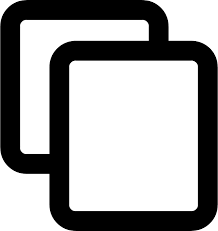
console.log(add(5, 6)); // Output: 11
8. Conclusion
In JavaScript, functions are essential building blocks that allow you to organize and reuse code efficiently. Functions can be declared in different ways, and they can accept parameters and return values. Understanding how to declare and call functions is key to becoming proficient in JavaScript programming.
Function Expressions
1. Introduction to Function Expressions
A function expression is a way of creating a function by assigning it to a variable. Unlike function declarations, function expressions are not hoisted, meaning the function cannot be called before it is defined. Function expressions are useful when you want to pass functions as arguments or return them from other functions.
2. Syntax of Function Expressions
To create a function expression, simply assign a function to a variable. The syntax is as follows:
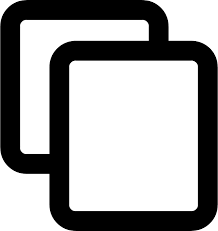
const functionName = function() {
// function body
};
Example of a basic function expression:
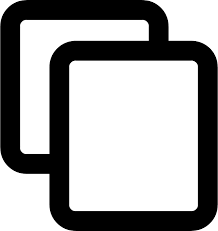
const greet = function() {
console.log('Hello, world!');
};
In this example, a function is assigned to the greet
variable, and the function logs 'Hello, world!' to the console when it is called.
3. Calling a Function Expression
Once a function is assigned to a variable, you can call it just like any other function, using the variable name followed by parentheses:
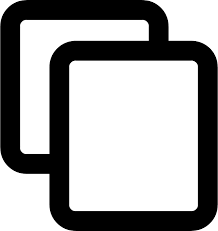
greet(); // Output: 'Hello, world!'
4. Anonymous Functions
Function expressions can also create anonymous functions, which are functions without a name. These are often used when the function is used only once or passed as an argument to another function.
Example of an anonymous function expression:
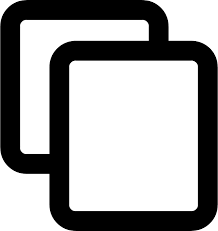
const sum = function(a, b) {
return a + b;
};
Here, we have an anonymous function that takes two parameters, a
and b
, and returns their sum. This function is assigned to the sum
variable.
5. Passing Function Expressions as Arguments
One of the key uses of function expressions is to pass them as arguments to other functions. This allows for more dynamic and flexible code. For example, you might pass a function expression as a callback to another function:
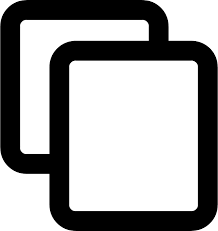
setTimeout(function() {
console.log('This is a delayed message.');
}, 2000);
In this example, an anonymous function expression is passed to setTimeout
, which will execute the function after a delay of 2000 milliseconds (2 seconds).
6. Returning Functions from Other Functions
Function expressions can also be returned from other functions. This is useful when you want to generate and return a function dynamically.
Example of returning a function from another function:
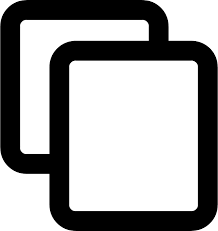
function createMultiplier(factor) {
return function(number) {
return number * factor;
};
}
In this example, createMultiplier
returns a function that multiplies its argument by a factor. When you call createMultiplier
, it returns a new function that you can call with a number:
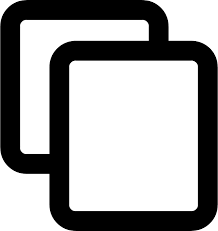
const multiplyByTwo = createMultiplier(2);
console.log(multiplyByTwo(5)); // Output: 10
7. Immediately Invoked Function Expressions (IIFE)
Another common type of function expression is the Immediately Invoked Function Expression (IIFE). These functions are executed immediately after they are defined, and they are often used to create a new scope for variables to avoid polluting the global scope.
Syntax for an IIFE:
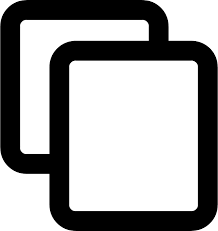
(function() {
// function body
})();
Example of an IIFE:
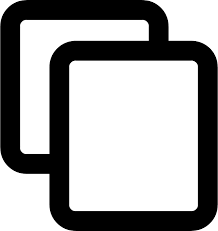
(function() {
console.log('I am an IIFE!');
})();
The function is wrapped in parentheses to convert it into an expression, followed by another set of parentheses to invoke it immediately.
8. Advantages of Function Expressions
- Flexibility: Function expressions can be assigned to variables, passed as arguments, and returned from other functions, allowing for more flexible code structures.
- Anonymous Functions: Function expressions are often used for anonymous functions that are not needed elsewhere in the code.
- Encapsulation: Using function expressions can help encapsulate functionality and reduce the risk of polluting the global scope.
9. Conclusion
Function expressions are a powerful feature in JavaScript that allows for more dynamic and flexible code. They enable anonymous functions, passing functions as arguments, and returning functions from other functions. By understanding function expressions, you can write more modular and maintainable JavaScript code.
Arrow Functions
1. Introduction to Arrow Functions
Arrow functions provide a shorter syntax for writing functions in JavaScript. They allow you to write functions more concisely and are often used for functions that are simple or anonymous. Arrow functions are defined using the =>
syntax, and they also behave differently from traditional functions in terms of how they handle the this
keyword.
2. Basic Syntax of Arrow Functions
The syntax for an arrow function is as follows:
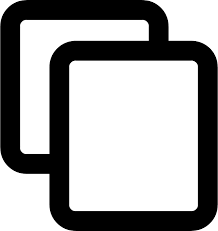
const functionName = (parameters) => {
// function body
};
Here, functionName
is the name of the function, and parameters
are the inputs that the function accepts. The function body contains the code to execute when the function is called.
3. Example of an Arrow Function
Here’s an example of a basic arrow function that adds two numbers:
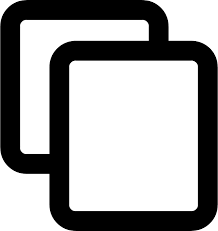
const add = (a, b) => {
return a + b;
};
In this example, the arrow function takes two parameters (a
and b
) and returns their sum.
4. Arrow Functions with Implicit Return
If the arrow function has a single expression in the body, you can omit the return
keyword and the curly braces. This is called an "implicit return." Here’s an example:
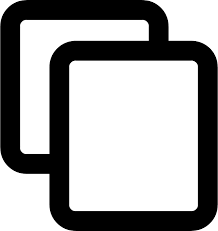
const add = (a, b) => a + b;
This is equivalent to the previous example but with a more concise syntax. The return statement is implicit, so you don’t need to explicitly use return
.
5. Arrow Functions with One Parameter
If the function only has one parameter, you can omit the parentheses around the parameter:
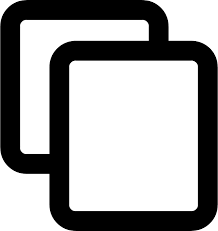
const square = x => x * x;
In this case, the function square
takes a single parameter x
and returns its square.
6. Arrow Functions with No Parameters
If the function has no parameters, you still need to use empty parentheses:
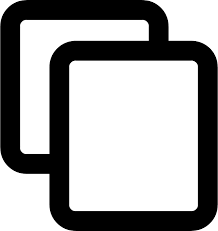
const greet = () => 'Hello, world!';
This function takes no parameters and returns the string 'Hello, world!'.
7. Arrow Functions and this
One of the key differences between arrow functions and traditional functions is how they handle the this
keyword. In a traditional function, this
refers to the object that the function is a method of. However, in arrow functions, this
is lexically bound, meaning it inherits this
from its surrounding context.
Here’s an example of how this
behaves in an arrow function:
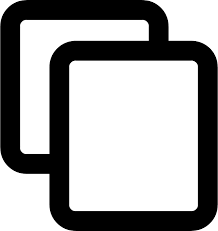
const obj = {
name: 'Arrow Function Example',
regularFunction: function() {
console.log(this.name); // 'Arrow Function Example'
},
arrowFunction: () => {
console.log(this.name); // undefined
}
};
obj.regularFunction(); // Output: 'Arrow Function Example'
obj.arrowFunction(); // Output: undefined
In the regularFunction
, this
refers to the obj
object. But in the arrowFunction
, this
is lexically bound and refers to the surrounding context (in this case, the global object or undefined
in strict mode).
8. Advantages of Arrow Functions
- Conciseness: Arrow functions provide a shorter syntax, making code more readable and concise.
- Lexical
this
Binding: Arrow functions do not bind their ownthis
, which can be helpful when working with callbacks or event handlers. - Implicit Return: Arrow functions allow for implicit returns, making code even more concise for simple expressions.
9. When to Use Arrow Functions
Arrow functions are best used for short, simple functions, especially when you want to avoid the complexities of this
binding. They are commonly used in callbacks, array methods like map
, filter
, and reduce
, and in concise operations.
10. Conclusion
Arrow functions are a powerful feature in JavaScript that offer a concise syntax and lexical this
binding. They are particularly useful for short functions and callbacks. By using arrow functions effectively, you can write cleaner and more maintainable code.
Parameters and Default Values
1. Introduction to Parameters and Default Values
In JavaScript, parameters are the variables that are passed into a function to provide input for the function to operate on. Default values can be assigned to parameters, allowing a function to use a predefined value when no argument is provided for that parameter. This feature makes functions more flexible and easier to work with in cases where certain parameters may be optional.
2. Function Parameters
When defining a function, you can specify parameters that will act as placeholders for the values passed into the function when it is called. Here is an example:
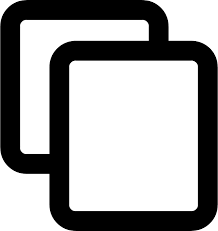
function greet(name) {
console.log('Hello, ' + name + '!');
}
In this example, name
is a parameter of the function greet
. When you call greet('Alice')
, the value 'Alice'
is passed to the name
parameter, and the function will log 'Hello, Alice!'
.
3. Default Values for Parameters
In JavaScript, you can assign default values to parameters by using the assignment operator (=
) in the function definition. If a parameter is not provided when calling the function, the default value will be used instead. Here is an example:
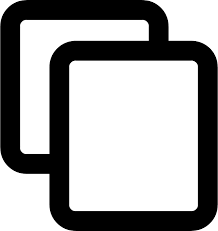
function greet(name = 'Guest') {
console.log('Hello, ' + name + '!');
}
In this example, the parameter name
has a default value of 'Guest'
. If no argument is provided when calling the function, the default value will be used:
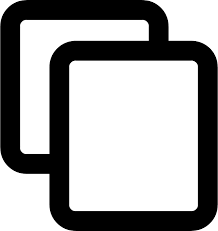
greet(); // Output: Hello, Guest!
4. Overriding Default Values
If you pass an argument to a parameter that has a default value, the argument will override the default value. For example:
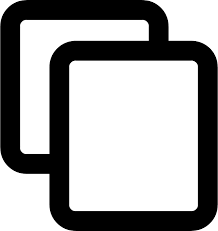
greet('Alice'); // Output: Hello, Alice!
In this case, the argument 'Alice'
overrides the default value 'Guest'
, and the function outputs 'Hello, Alice!'
.
5. Default Values for Multiple Parameters
You can assign default values to multiple parameters in a function:
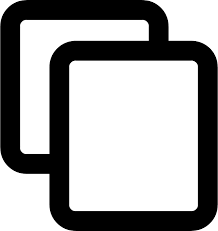
function greet(name = 'Guest', age = 25) {
console.log('Hello, ' + name + '. You are ' + age + ' years old.');
}
Here, both name
and age
have default values. If no arguments are provided, the function will use the default values:
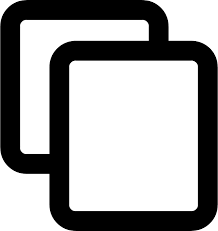
greet(); // Output: Hello, Guest. You are 25 years old.
If some arguments are provided, only the missing parameters will use the default values:
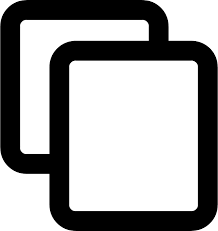
greet('Alice'); // Output: Hello, Alice. You are 25 years old.
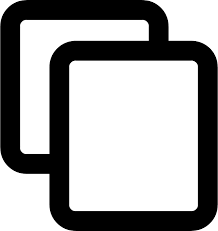
greet('Alice', 30); // Output: Hello, Alice. You are 30 years old.
6. Default Values and Expressions
Default values can also be expressions, not just static values. This allows you to calculate or evaluate a value before assigning it as the default:
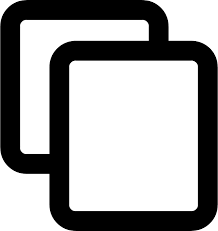
function greet(name = 'Guest', age = new Date().getFullYear() - 1990) {
console.log('Hello, ' + name + '. You are ' + age + ' years old.');
}
In this example, the age
parameter has a default value that calculates the age based on the current year (using new Date().getFullYear() - 1990
).
7. Rest Parameters and Default Values
In combination with rest parameters, you can assign default values for the entire set of arguments passed to a function. Rest parameters allow you to collect all remaining arguments into an array. Here is an example:
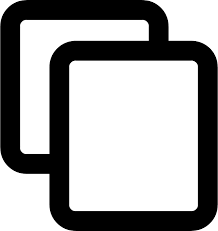
function greet(greeting = 'Hello', ...names) {
names.forEach(name => {
console.log(greeting + ', ' + name + '!');
});
}
In this case, greeting
has a default value of 'Hello'
, and the names
parameter collects all additional arguments passed to the function. You can call this function with multiple names:
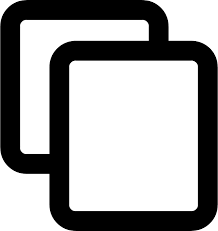
greet('Hi', 'Alice', 'Bob'); // Output: Hi, Alice! Hi, Bob!
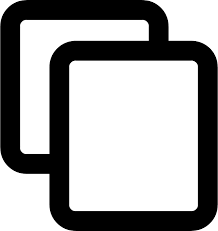
greet('Alice', 'Bob'); // Output: Hello, Alice! Hello, Bob!
8. Conclusion
Parameters and default values are important features in JavaScript that make functions more flexible and allow you to provide fallback values when arguments are not supplied. By using default values, you can ensure that your functions behave predictably, even when some values are missing or undefined.
IIFE (Immediately Invoked Function Expression)
1. Introduction to IIFE
An IIFE (Immediately Invoked Function Expression) is a function in JavaScript that is executed immediately after it is defined. It allows you to create a function and call it right away, without having to invoke it separately. IIFEs are commonly used to create isolated, self-contained code blocks that do not interfere with the surrounding code.
2. Syntax of an IIFE
The basic syntax of an IIFE involves wrapping the function expression in parentheses and then immediately invoking it by adding parentheses after the function definition:
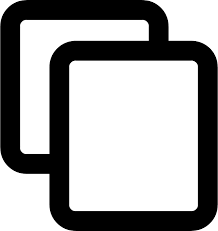
(function() {
console.log('I am an IIFE!');
})();
In this example, the function is defined and then immediately invoked. The parentheses around the function definition make it an expression, and the parentheses after the function definition invoke it right away.
3. Why Use IIFE?
IIFEs are useful for several reasons:
- Encapsulation: IIFEs help in encapsulating variables and functions within their scope, preventing them from leaking into the global scope and avoiding conflicts with other code.
- Preventing Global Namespace Pollution: IIFEs help avoid polluting the global scope by keeping the variables and functions defined within them private.
- Module Pattern: IIFEs are often used in the module pattern, where they can return an object or function that exposes only the necessary methods or properties, while keeping the internal details private.
4. Example of an IIFE
Here is an example of an IIFE where we define a variable and immediately log its value:
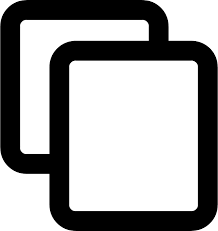
(function() {
var message = 'Hello, I am inside an IIFE!';
console.log(message);
})();
The variable message
is scoped to the function and will not be accessible outside of it. The output will be: Hello, I am inside an IIFE!
5. IIFE with Parameters
IIFEs can also accept parameters, allowing you to pass values to the function when it is invoked:
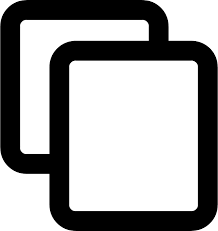
(function(name) {
console.log('Hello, ' + name + '!');
})('Alice');
In this example, we pass the argument 'Alice'
to the IIFE when it is invoked, and the function logs 'Hello, Alice!'
.
6. IIFE with Return Value
An IIFE can also return a value. This is useful when you want to execute some code and capture the result:
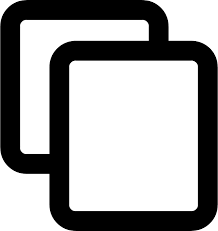
var result = (function() {
var sum = 5 + 3;
return sum;
})();
console.log(result); // Output: 8
In this example, the IIFE computes the sum of 5 and 3, and the result is captured in the result
variable.
7. IIFE with Arrow Function
You can also use arrow functions to define IIFEs, making the syntax more concise:
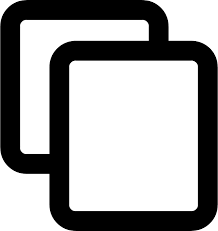
(() => {
console.log('I am an IIFE using an arrow function!');
})();
This example uses an arrow function to define and invoke the IIFE immediately. The syntax is more compact compared to the traditional function expression.
8. IIFE in JavaScript Modules
IIFEs are also commonly used in JavaScript modules, where they are used to encapsulate code and expose specific functionality while hiding internal details. Here is an example of using an IIFE to create a module:
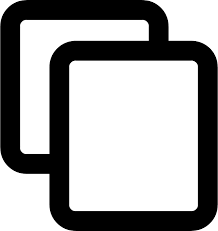
var counterModule = (function() {
var counter = 0;
return {
increment: function() {
counter++;
console.log(counter);
},
decrement: function() {
counter--;
console.log(counter);
}
};
})();
counterModule.increment(); // Output: 1
counterModule.increment(); // Output: 2
counterModule.decrement(); // Output: 1
In this example, the IIFE defines a counter module that exposes two methods: increment
and decrement
, while keeping the counter
variable private.
9. Conclusion
IIFE is a powerful concept in JavaScript that allows for immediate function execution and helps in encapsulating variables and logic. It is particularly useful in maintaining a clean global namespace and is frequently used in JavaScript modules and other advanced patterns. Understanding IIFEs is essential for writing modular and maintainable JavaScript code.
Global vs. Local Scope
1. Introduction to Scope
Scope in JavaScript refers to the region of the program where a variable can be accessed. There are two main types of scope in JavaScript: global scope and local scope. Understanding the difference between global and local scope is essential for managing variable accessibility and avoiding conflicts in your code.
2. Global Scope
Global scope refers to the outermost context in which variables and functions are defined. A variable declared in the global scope can be accessed from anywhere in the program, including inside functions.
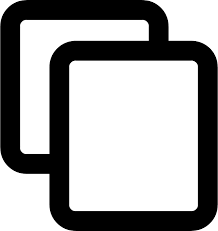
var globalVar = 'I am a global variable!';
function accessGlobal() {
console.log(globalVar); // Output: I am a global variable!
}
accessGlobal();
console.log(globalVar); // Output: I am a global variable!
In this example, the variable globalVar
is declared outside of any function, so it is accessible both inside the accessGlobal
function and outside of it. This makes it a global variable.
3. Local Scope
Local scope refers to the context within a function or block where variables are declared. A variable declared within a function or a block is only accessible within that function or block, and it is not available outside it.
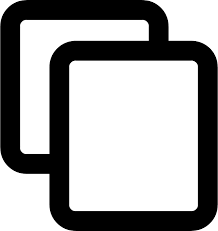
function localScopeExample() {
var localVar = 'I am a local variable!';
console.log(localVar); // Output: I am a local variable!
}
localScopeExample();
console.log(localVar); // Error: localVar is not defined
In this example, the variable localVar
is declared inside the function localScopeExample
, so it is only accessible within the function. Attempting to access it outside the function results in a reference error.
4. Function Scope
In JavaScript, function scope means that any variable declared with var
inside a function is scoped to that function and cannot be accessed outside of it. This is known as function-level scoping.
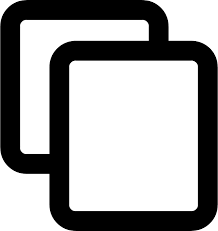
function functionScope() {
var functionVar = 'I am scoped to the function!';
console.log(functionVar); // Output: I am scoped to the function!
}
functionScope();
console.log(functionVar); // Error: functionVar is not defined
The variable functionVar
is declared with var
inside the functionScope
function, so it is only accessible within that function.
5. Block Scope (let, const)
In addition to function scope, JavaScript introduced block scope with the let
and const
keywords. Variables declared with let
or const
inside a block (such as inside an if
statement, loop, or any block enclosed by curly braces) are scoped to that block.
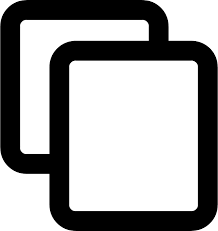
if (true) {
let blockVar = 'I am scoped to this block!';
console.log(blockVar); // Output: I am scoped to this block!
}
console.log(blockVar); // Error: blockVar is not defined
The variable blockVar
is declared inside the if
block using let
, so it is only accessible within that block. It is not available outside of it.
6. Lexical Scope
Lexical scope refers to how JavaScript determines the scope of variables based on where they are defined in the source code. This means that a function's scope is determined by its position in the code, not by where it is called from.
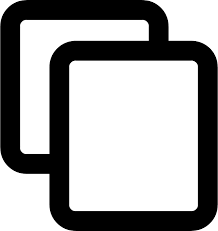
function outer() {
var outerVar = 'I am in the outer function!';
function inner() {
console.log(outerVar); // Output: I am in the outer function!
}
inner();
}
outer();
In this example, the inner
function has access to the variable outerVar
because of lexical scoping. The inner
function is defined within the outer
function, so it has access to variables declared in the outer function.
7. Global Scope and the Window Object
In the browser environment, variables declared in the global scope become properties of the window
object. This means that you can access global variables as properties of the window
object.
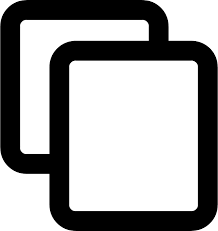
var globalWindowVar = 'I am a global variable!';
console.log(window.globalWindowVar); // Output: I am a global variable!
In this case, the global variable globalWindowVar
is accessible through the window
object in the browser.
8. Avoiding Global Scope Pollution
While it may seem convenient to declare variables in the global scope, it is generally considered good practice to avoid polluting the global scope. Global variables can lead to conflicts, especially when multiple scripts use the same variable names. To avoid this, you can use local scope as much as possible and declare variables inside functions or blocks, or use patterns like IIFEs (Immediately Invoked Function Expressions) to encapsulate your code.
9. Conclusion
Understanding the difference between global and local scope is essential for managing the accessibility and lifespan of variables in your JavaScript programs. By properly scoping your variables, you can avoid unintended side effects, conflicts, and issues with variable name collisions. Always strive to minimize the use of global variables and prefer local scope to ensure cleaner, more maintainable code.
Block Scope (let and const)
1. Introduction to Block Scope
In JavaScript, variables declared with let
and const
have block scope. This means that they are only accessible within the block, statement, or expression where they are defined. Block scope was introduced in ECMAScript 6 (ES6) to solve the limitations of the var
keyword, which is function-scoped, and to provide better control over variable accessibility within specific blocks of code.
2. Block Scope with let
The let
keyword allows you to declare variables with block scope. A variable declared with let
is only accessible within the block in which it is defined (e.g., inside a loop, if statement, or any other block surrounded by curly braces).
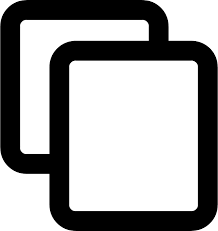
if (true) {
let blockVar = 'I am scoped to this block!';
console.log(blockVar); // Output: I am scoped to this block!
}
console.log(blockVar); // Error: blockVar is not defined
In this example, the variable blockVar
is declared inside the if
block using let
, so it is only accessible inside that block. Attempting to access it outside the block results in an error.
3. Block Scope with const
The const
keyword also provides block scope, but variables declared with const
must be assigned a value at the time of declaration, and they cannot be reassigned. Like let
, const
variables are only accessible within the block in which they are defined.
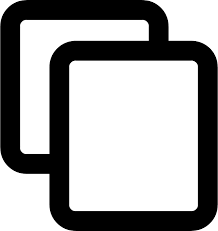
if (true) {
const blockConstVar = 'I am a constant scoped to this block!';
console.log(blockConstVar); // Output: I am a constant scoped to this block!
}
console.log(blockConstVar); // Error: blockConstVar is not defined
In this example, the blockConstVar
variable is declared inside the if
block using const
, so it is only accessible inside that block. Attempting to access it outside the block results in an error.
4. Reassigning Variables
With let
, you can reassign a value to the variable, but with const
, the variable cannot be reassigned once it is initialized.
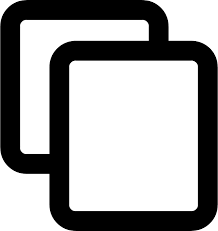
let x = 10;
x = 20; // Reassignment is allowed
console.log(x); // Output: 20
const y = 30;
y = 40; // Error: Assignment to constant variable
In this example, the value of the x
variable is reassigned successfully. However, attempting to reassign the value of y
, which is a constant, results in an error.
5. Block Scope in Loops
When using let
or const
inside loops, each iteration creates a new scope for the variable, making it safe to use in cases where you need the variable to be scoped to the iteration.
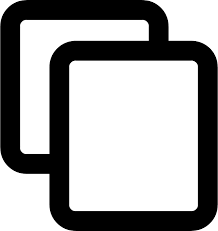
for (let i = 0; i < 3; i++) {
console.log(i); // Output: 0, 1, 2
}
console.log(i); // Error: i is not defined
In this example, the variable i
is scoped to the for
loop and is not accessible outside the loop.
6. Block Scope and Closures
Block-scoped variables are often used in closures, especially in loops, to preserve the correct value for each iteration. The use of let
or const
ensures that each iteration has its own separate value, preventing issues that occur when using var
in loops.
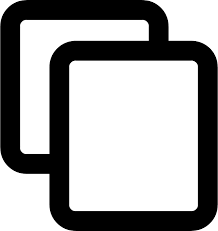
const arr = [];
for (let i = 0; i < 3; i++) {
arr.push(function() {
return i;
});
}
console.log(arr[0]()); // Output: 0
console.log(arr[1]()); // Output: 1
console.log(arr[2]()); // Output: 2
In this example, each function in the array arr
remembers the value of i
from its specific iteration. This is possible because i
is block-scoped using let
, and each iteration creates a new instance of the variable.
7. Temporal Dead Zone (TDZ)
Both let
and const
are hoisted to the top of their block, but they are not accessible before they are declared. This behavior is called the Temporal Dead Zone (TDZ). Trying to access a variable before it is declared will result in a reference error.
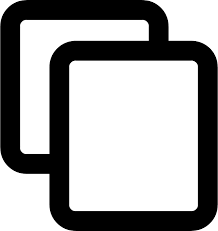
console.log(a); // Error: Cannot access 'a' before initialization
let a = 10;
In this example, accessing a
before its declaration results in an error, because it is within the TDZ.
8. Conclusion
The introduction of block-scoped variables let
and const
in JavaScript has made it easier to manage variable scope and avoid issues related to variable hoisting and unintended global scope pollution. Using let
and const
provides better control over variable visibility, especially in loops and closures, and helps to make your code more predictable and maintainable.
Hoisting
1. What is Hoisting?
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compile phase, before the code has been executed. This means that you can use variables and functions before they are declared in the code. However, only the declarations are hoisted, not the assignments.
2. Hoisting with var
When a variable is declared using var
, it is hoisted to the top of its function or global scope. The variable is initialized with undefined>, meaning that although the declaration is moved to the top, the assignment still happens where it is written in the code.
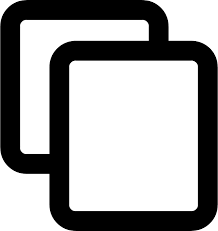
console.log(a); // Output: undefined
var a = 5;
console.log(a); // Output: 5
In this example, the var a
declaration is hoisted to the top, but the assignment happens at the line where it appears. Therefore, the first console.log(a)
outputs undefined>, and the second outputs
5>.
3. Hoisting with let and const
Variables declared with let
and const
are also hoisted, but they are not initialized with undefined
. Instead, they remain in a "temporal dead zone" (TDZ) from the start of the block until the point where they are declared. If you try to access them before the declaration, a ReferenceError
will occur.
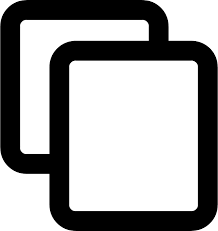
console.log(b); // Error: Cannot access 'b' before initialization
let b = 10;
In this case, attempting to access b
before its declaration results in a ReferenceError
, because let
variables are in the TDZ.
4. Hoisting with Functions
Function declarations are hoisted in their entirety, meaning both the function declaration and its definition are moved to the top of the scope. This allows you to call a function before it is declared.
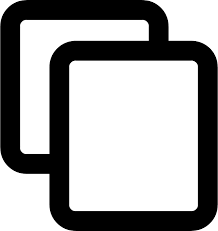
hello(); // Output: Hello, world!
function hello() {
console.log('Hello, world!');
}
In this example, the function hello()
is called before its declaration, but it works because the entire function is hoisted.
5. Function Expressions and Hoisting
Function expressions, however, are not hoisted in the same way as function declarations. Only the variable declaration (using var
, let
, or const
) is hoisted, not the function definition. If you try to call a function expression before its definition, a TypeError
will occur.
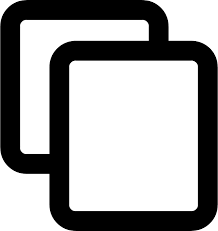
greet(); // Error: greet is not a function
var greet = function() {
console.log('Hello!');
};
In this case, the greet
variable is hoisted, but it is initially set to undefined>. The function expression is assigned to it only after the declaration line, so calling
greet()
before that results in a TypeError
.
6. How Hoisting Works in Loops
When using var
in loops, the variable is hoisted to the top of the loop's scope, which can cause unexpected behavior in certain cases. However, with let
and const
, each iteration creates a new block scope for the variable.
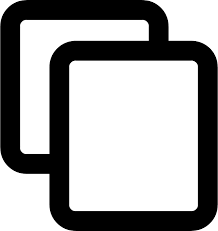
for (var i = 0; i < 3; i++) {
console.log(i); // Output: 0, 1, 2
}
console.log(i); // Output: 3 (i is hoisted outside the loop)
Here, the variable i
is hoisted with var>, so it is accessible outside the loop, which may not be the desired behavior. If you want to avoid this, you can use
let
or const
.
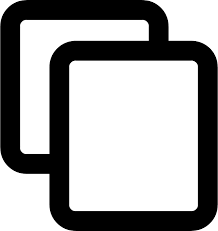
for (let j = 0; j < 3; j++) {
console.log(j); // Output: 0, 1, 2
}
console.log(j); // Error: j is not defined (because of block scope)
In this example, the variable j
is block-scoped using let
, so it is not accessible outside the loop.
7. Hoisting in Functions
In JavaScript, the behavior of hoisting is the same in functions. Function declarations are hoisted, while function expressions are not.
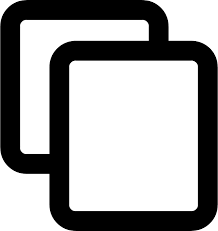
console.log(func()); // Output: Hello, world!
function func() {
return 'Hello, world!';
}
8. Conclusion
Hoisting is an important concept to understand in JavaScript. It allows variables and functions to be accessible before their declaration in the code. However, it is essential to be aware of the differences between var
, let
, and const
in terms of hoisting behavior, as well as the behavior of function declarations and function expressions. Understanding hoisting can help you write more predictable and error-free code.
Object Literals
1. What is an Object Literal?
An object literal is a way to define an object in JavaScript using a simple and concise syntax. Objects in JavaScript are collections of key-value pairs, where each key is a property name and the value can be any valid data type (e.g., string, number, array, function, etc.). Object literals are created using curly braces {}>, with key-value pairs separated by commas.
2. Creating an Object Literal
You can create an object literal by defining key-value pairs inside curly braces. Here's an example of an object literal:
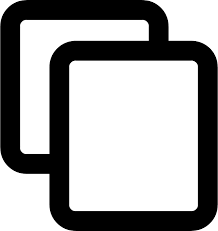
const person = {
name: 'John Doe',
age: 30,
greet: function() {
console.log('Hello, ' + this.name);
}
};
In this example, we have an object person> with three properties:
name
, age
, and greet
. The greet
property holds a function, which can be executed by calling person.greet()
.
3. Accessing Object Properties
You can access the properties of an object using either dot notation or bracket notation:
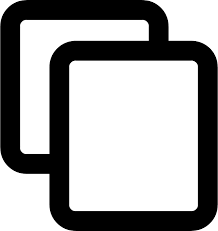
console.log(person.name); // Output: John Doe
console.log(person['age']); // Output: 30
Both dot notation and bracket notation can be used to retrieve values, but bracket notation is useful when the property name is dynamic or stored in a variable.
4. Modifying Object Properties
You can modify the properties of an object by assigning new values to them:
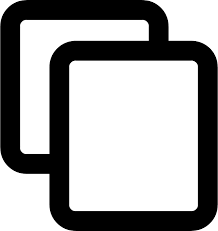
person.age = 31;
person.greet = function() {
console.log('Hi, ' + this.name);
};
In this example, we updated the age
property and changed the behavior of the greet
method.
5. Adding New Properties
New properties can be added to an object after it has been created:
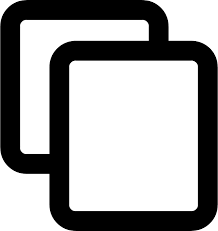
person.job = 'Developer';
console.log(person.job); // Output: Developer
Here, the new property job
is added to the person
object, and its value is 'Developer'
.
6. Removing Object Properties
To remove a property from an object, you can use the delete
operator:
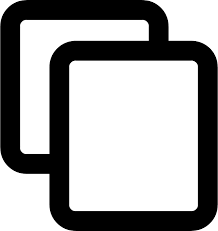
delete person.age;
console.log(person.age); // Output: undefined
The delete
operator removes the age
property from the person
object.
7. Object Shorthand Syntax (ES6)
In ES6, you can use shorthand syntax to define properties when the property name matches the variable name:
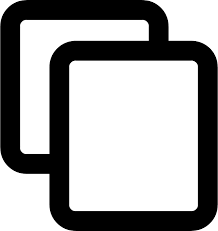
const name = 'Alice';
const age = 25;
const person = { name, age }; // Same as { name: name, age: age }
console.log(person); // Output: { name: 'Alice', age: 25 }
The shorthand syntax eliminates the need for repeating the variable name when defining object properties.
8. Computed Property Names
In JavaScript, you can also use computed property names, which allow you to define properties using expressions:
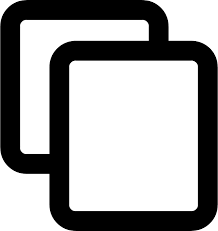
const propName = 'location';
const person = {
name: 'John',
[propName]: 'New York'
};
console.log(person.location); // Output: New York
In this example, the property name is computed dynamically using the value of the propName
variable.
9. Nested Objects
Objects can contain other objects as values, allowing you to create nested objects:
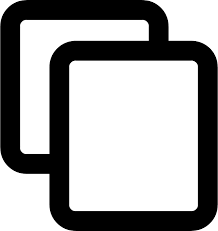
const person = {
name: 'John',
address: {
city: 'New York',
zip: '10001'
}
};
console.log(person.address.city); // Output: New York
Here, the address
property is itself an object with city
and zip
properties.
10. Conclusion
Object literals are a fundamental part of JavaScript and provide a simple way to create and manipulate objects. By using object literals, you can define properties, methods, and even nested objects. The flexibility of object literals makes them a powerful tool for structuring and organizing data in your JavaScript programs.
Accessing and Modifying Object Properties
1. Accessing Object Properties
In JavaScript, you can access object properties in two main ways: dot notation and bracket notation. These methods are used to retrieve the values of properties stored within an object.
Dot Notation
Dot notation is the most common way to access object properties. You simply use a dot (.) followed by the property name:
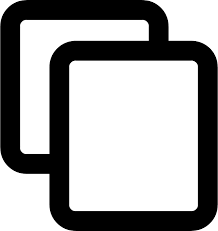
const person = {
name: 'John',
age: 30,
greet: function() {
console.log('Hello, ' + this.name);
}
};
console.log(person.name); // Output: John
Bracket Notation
Bracket notation allows you to access object properties using a string. It is useful when the property name is dynamic, contains spaces, or is a number:
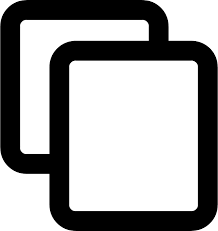
console.log(person['age']); // Output: 30
You can also use bracket notation to access properties with spaces in their names:
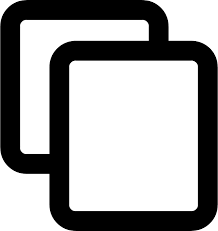
const car = {
'car model': 'Tesla',
year: 2021
};
console.log(car['car model']); // Output: Tesla
2. Modifying Object Properties
You can modify the properties of an object by assigning a new value to the property:
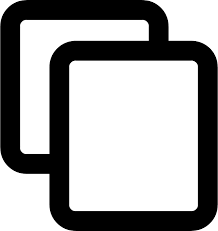
person.age = 31;
console.log(person.age); // Output: 31
Here, the age
property of the person
object is updated to 31
.
3. Adding New Properties
In JavaScript, you can also add new properties to an existing object:
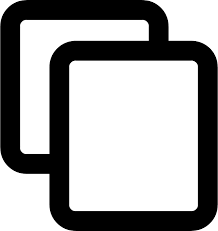
person.job = 'Developer';
console.log(person.job); // Output: Developer
In this example, the new job
property is added to the person
object with the value 'Developer'
.
4. Removing Object Properties
To remove a property from an object, you can use the delete
operator:
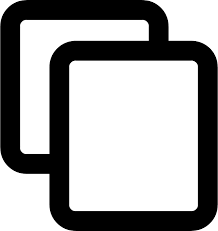
delete person.age;
console.log(person.age); // Output: undefined
The delete
operator removes the age
property from the person
object. After deletion, accessing the age
property returns undefined
.
5. Accessing Nested Object Properties
Objects can contain other objects as properties. To access nested object properties, you can chain dot notation or bracket notation:
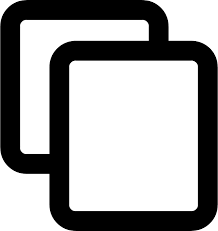
const person = {
name: 'John',
address: {
city: 'New York',
zip: '10001'
}
};
console.log(person.address.city); // Output: New York
In this example, the address
property of the person
object is itself an object. To access the city
property inside address
, we use person.address.city
.
6. Computed Property Names
JavaScript also allows computed property names, which means you can use expressions to define property names dynamically:
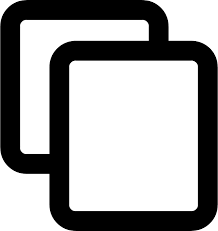
const propName = 'location';
const person = {
name: 'John',
[propName]: 'New York'
};
console.log(person.location); // Output: New York
In this example, the value of the propName
variable is used as the property name, and the property location
is dynamically added to the person
object.
7. Summary
To summarize:
- Use dot notation
object.property
to access or modify object properties when the property name is a valid identifier. - Use bracket notation
object['property']
to access or modify properties with spaces, special characters, or dynamic property names. - New properties can be added, and existing properties can be modified or removed.
- Nested object properties are accessed using dot or bracket notation.
- The
delete
operator removes properties from an object.
8. Conclusion
Accessing and modifying object properties is a fundamental concept in JavaScript. Understanding how to interact with objects using dot and bracket notation, along with adding and removing properties, will help you work more effectively with objects in your JavaScript programs.
Methods in Objects
1. What are Methods in Objects?
In JavaScript, methods are functions that are properties of objects. They are used to define behavior related to the object. A method is defined using function syntax and can be called on the object to perform actions or return values.
2. Defining Methods in Objects
Methods can be defined inside an object literal by assigning a function to a property:
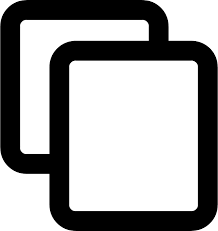
const person = {
name: 'John',
age: 30,
greet: function() {
console.log('Hello, ' + this.name);
}
};
person.greet(); // Output: Hello, John
In this example, the greet
method is a function assigned to the person
object. The method is called using person.greet()
.
3. Method Shorthand (ES6)
In ES6, you can define methods in objects using shorthand syntax, which is more concise:
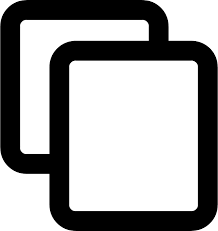
const person = {
name: 'John',
age: 30,
greet() {
console.log('Hello, ' + this.name);
}
};
person.greet(); // Output: Hello, John
In this example, the greet
method is defined without the function
keyword. This shorthand syntax is preferred in modern JavaScript.
4. Using this
Keyword in Methods
Inside an object method, the this
keyword refers to the object itself. It allows you to access other properties or methods of the same object:
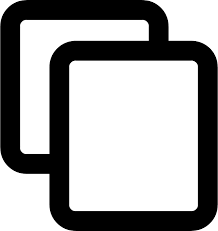
const person = {
name: 'John',
age: 30,
greet() {
console.log('Hello, ' + this.name);
},
birthday() {
this.age++;
}
};
person.greet(); // Output: Hello, John
person.birthday();
console.log(person.age); // Output: 31
In this example, the birthday
method increments the age
property of the person
object using this
.
5. Methods with Parameters
Methods can also accept parameters to make them more flexible. You can pass values into a method, and it can use those values in its logic:
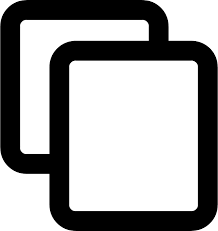
const person = {
name: 'John',
greet(message) {
console.log(message + ', ' + this.name);
}
};
person.greet('Good morning'); // Output: Good morning, John
Here, the greet
method takes a parameter message
and uses it in the output.
6. Returning Values from Methods
Methods can return values, just like regular functions. You can return any data type from a method, including strings, numbers, objects, or even other functions:
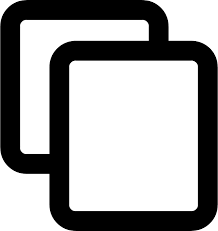
const person = {
name: 'John',
age: 30,
getDetails() {
return this.name + ' is ' + this.age + ' years old.';
}
};
console.log(person.getDetails()); // Output: John is 30 years old.
In this example, the getDetails
method returns a string containing the name
and age
properties of the person
object.
7. Methods in Nested Objects
Methods can also be defined inside nested objects. You can access and invoke methods within nested objects using dot notation:
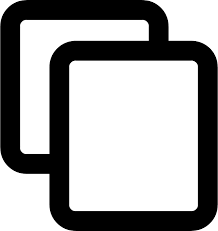
const person = {
name: 'John',
address: {
city: 'New York',
getCity() {
return this.city;
}
}
};
console.log(person.address.getCity()); // Output: New York
In this example, the getCity
method is inside the address
object, and it is called using person.address.getCity()
.
8. Summary
To summarize:
- Methods are functions that are properties of objects and are used to define behavior related to that object.
- You can define methods using traditional function syntax or shorthand method syntax (ES6).
- The
this
keyword is used inside methods to refer to the object itself. - Methods can accept parameters, return values, and be used to manipulate object properties.
- Nested objects can also have methods, which can be accessed through their respective parent objects.
9. Conclusion
Methods are an essential part of working with objects in JavaScript. They allow objects to perform actions and exhibit behaviors, making them more interactive and functional. Understanding how to define, call, and work with methods is crucial to mastering JavaScript objects.
The 'this' Keyword
1. What is the 'this' Keyword?
In JavaScript, the this
keyword refers to the context in which a function is executed. It allows you to access properties and methods of the object that owns the function. The value of this
is determined by how the function is called, and it can be different in various situations.
2. 'this' in Global Context
When you use this
in the global execution context (outside of any function or object), it refers to the global object. In browsers, the global object is typically the window
object:
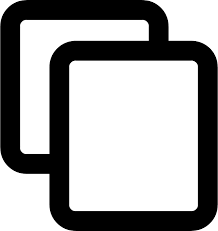
console.log(this); // In a browser, this refers to the 'window' object
In the example above, this
refers to the global window
object in a browser environment.
3. 'this' in Object Methods
When a function is called as a method of an object, this
refers to the object that the method is a part of:
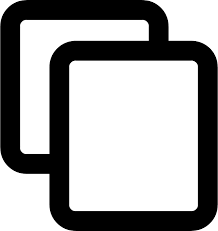
const person = {
name: 'John',
greet() {
console.log('Hello, ' + this.name);
}
};
person.greet(); // Output: Hello, John
In this example, this
refers to the person
object, allowing us to access the name
property within the method.
4. 'this' in Constructor Functions
In constructor functions, this
refers to the new object that is being created. Constructor functions are used to create instances of objects:
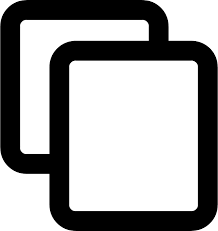
function Person(name, age) {
this.name = name;
this.age = age;
}
const john = new Person('John', 30);
console.log(john.name); // Output: John
console.log(john.age); // Output: 30
In this example, this
refers to the newly created john
object.
5. 'this' in Arrow Functions
Arrow functions behave differently from regular functions in terms of this
. In arrow functions, this
does not refer to the object that calls the function. Instead, it "inherits" the value of this
from the surrounding (lexical) context:
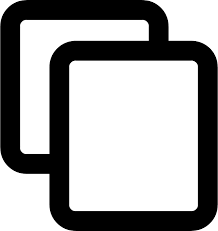
const person = {
name: 'John',
greet: () => {
console.log('Hello, ' + this.name);
}
};
person.greet(); // Output: Hello, undefined
Here, this
inside the arrow function refers to the global object, not the person
object, because arrow functions do not have their own this
context.
6. 'this' in Event Handlers
When you use this
in event handlers, it refers to the element that triggered the event:
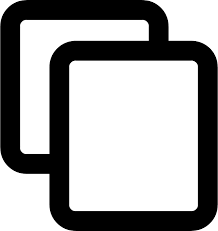
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
console.log(this); // Output: button element
});
In this example, this
refers to the button
element that was clicked.
7. Explicitly Setting 'this' with call
, apply
, and bind
You can explicitly set the value of this
using the call
, apply
, or bind
methods:
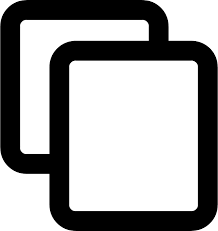
function greet() {
console.log('Hello, ' + this.name);
}
const person1 = { name: 'John' };
const person2 = { name: 'Jane' };
greet.call(person1); // Output: Hello, John
greet.apply(person2); // Output: Hello, Jane
The call
method immediately invokes the function with the specified this
value, while apply
works similarly but takes an array of arguments. bind
returns a new function with the specified this
value, which you can invoke later:
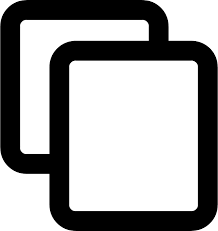
const boundGreet = greet.bind(person1);
boundGreet(); // Output: Hello, John
8. 'this' in Class Methods
In classes, this
refers to the instance of the class:
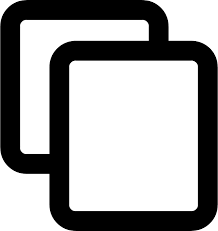
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log('Hello, ' + this.name);
}
}
const john = new Person('John', 30);
john.greet(); // Output: Hello, John
In this example, this
refers to the instance of the Person
class, which is john
.
9. Summary
To summarize:
this
refers to the object or context in which a function is executed.- In object methods,
this
refers to the object itself. - In constructor functions,
this
refers to the newly created instance. - Arrow functions do not have their own
this
and inherit it from the surrounding context. - In event handlers,
this
refers to the element that triggered the event. - You can explicitly set the value of
this
usingcall
,apply
, orbind
.
10. Conclusion
The this
keyword is a fundamental concept in JavaScript. Understanding how it works in different contexts is crucial for writing effective and predictable code. By mastering this
, you can handle object-oriented and functional programming with ease.
Declaring Arrays
1. What is an Array?
An array is a special variable in JavaScript that can hold multiple values at once. Arrays are used to store a collection of items, such as numbers, strings, objects, or even other arrays. Arrays are indexed, with the first element being at index 0.
2. Declaring an Array Using Array Literals
The most common way to declare an array in JavaScript is by using array literals, which are enclosed in square brackets []
. You can directly initialize the array with elements:
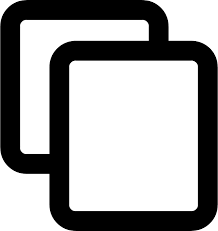
let fruits = ['Apple', 'Banana', 'Orange'];
In this example, the fruits
array contains three elements: 'Apple'
, 'Banana'
, and 'Orange'
.
3. Declaring an Array Using the new Array()
Constructor
You can also declare an array using the new Array()
constructor. This method creates an empty array or an array with a specified number of elements:
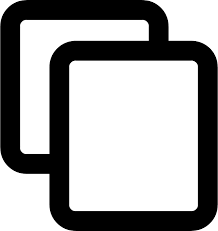
let numbers = new Array(5); // Creates an array with 5 empty slots
let colors = new Array('Red', 'Green', 'Blue'); // Creates an array with 3 elements
In the first example, the array numbers
will have 5 empty slots. In the second example, the colors
array is initialized with three colors.
4. Declaring an Array with Mixed Data Types
Arrays in JavaScript can hold elements of different data types. For example, you can mix strings, numbers, and other objects within a single array:
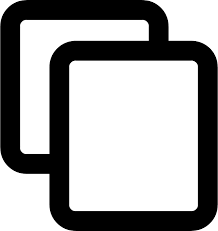
let mixedArray = [42, 'Hello', true, { name: 'John' }, [1, 2, 3]];
In this example, the mixedArray
contains a number, a string, a boolean, an object, and another array.
5. Accessing Array Elements
You can access the elements of an array using their index. JavaScript arrays are zero-indexed, meaning the first element has an index of 0:
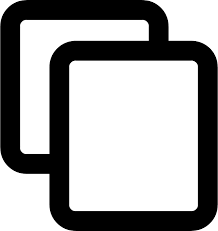
let fruits = ['Apple', 'Banana', 'Orange'];
console.log(fruits[0]); // Output: Apple
console.log(fruits[1]); // Output: Banana
In this example, fruits[0]
refers to the first element, 'Apple'
, and fruits[1]
refers to the second element, 'Banana'
.
6. Modifying Array Elements
You can modify an array element by accessing it through its index and assigning a new value:
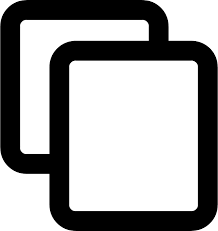
fruits[1] = 'Mango'; // Changes 'Banana' to 'Mango'
console.log(fruits[1]); // Output: Mango
In this example, fruits[1]
is updated to 'Mango'
.
7. Multi-Dimensional Arrays
Arrays in JavaScript can also be multi-dimensional, meaning they can contain other arrays as elements. These are often used for representing tables or matrices:
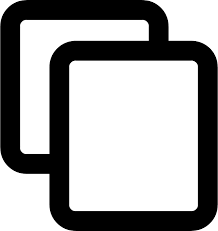
let matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
console.log(matrix[0][2]); // Output: 3
In this example, matrix[0][2]
refers to the third element of the first sub-array, which is 3.
8. Array Length
Every array in JavaScript has a length
property, which returns the number of elements in the array:
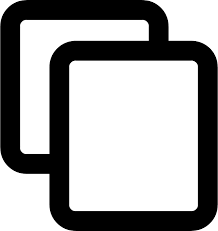
let fruits = ['Apple', 'Banana', 'Orange'];
console.log(fruits.length); // Output: 3
In this example, the length
property of the fruits
array returns 3, as there are three elements in the array.
9. Conclusion
In JavaScript, arrays are a powerful data structure that allows you to store and manage multiple values. You can declare arrays using array literals or the new Array()
constructor, and you can access, modify, and manipulate array elements efficiently. Understanding how to work with arrays is essential for handling collections of data in JavaScript.
Array Methods (push(), pop(), map(), filter())
1. The push()
Method
The push()
method is used to add one or more elements to the end of an array. It modifies the array in place and returns the new length of the array.
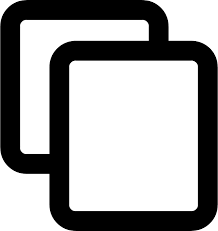
let fruits = ['Apple', 'Banana'];
fruits.push('Orange'); // Adds 'Orange' to the end of the array
console.log(fruits); // Output: ['Apple', 'Banana', 'Orange']
In this example, push()
adds 'Orange' to the end of the fruits
array.
2. The pop()
Method
The pop()
method is used to remove the last element from an array. It modifies the array in place and returns the element that was removed.
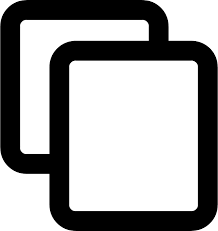
let fruits = ['Apple', 'Banana', 'Orange'];
let removedFruit = fruits.pop(); // Removes 'Orange'
console.log(fruits); // Output: ['Apple', 'Banana']
console.log(removedFruit); // Output: 'Orange'
In this example, pop()
removes 'Orange' from the fruits
array and assigns it to the removedFruit
variable.
3. The map()
Method
The map()
method creates a new array populated with the results of calling a provided function on every element in the array. This method does not modify the original array.
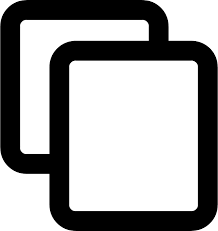
let numbers = [1, 2, 3, 4];
let doubledNumbers = numbers.map(num => num * 2); // Doubles each number
console.log(doubledNumbers); // Output: [2, 4, 6, 8]
In this example, map()
creates a new array where each number from the original numbers
array is doubled.
4. The filter()
Method
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. This method does not modify the original array.
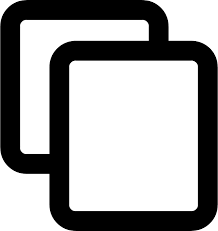
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(num => num % 2 === 0); // Filters even numbers
console.log(evenNumbers); // Output: [2, 4]
In this example, filter()
creates a new array that contains only the even numbers from the original numbers
array.
5. Conclusion
JavaScript arrays come with several built-in methods to manipulate and work with array data. The push()
and pop()
methods are used for adding and removing elements at the end of an array, while map()
and filter()
are used for creating new arrays based on existing ones. Understanding these array methods is essential for working with collections of data in JavaScript.
Iterating Over Arrays
1. Using a for
Loop
The traditional for
loop is one of the most common ways to iterate over an array. It gives you full control over the loop’s behavior and allows you to access each element by its index.
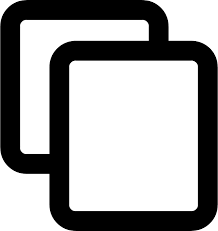
let fruits = ['Apple', 'Banana', 'Orange'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
// Output:
// Apple
// Banana
// Orange
In this example, we use a standard for
loop to iterate through the fruits
array and log each element to the console.
2. Using forEach()
The forEach()
method provides a more concise way to iterate over arrays. It executes a provided function once for each array element.
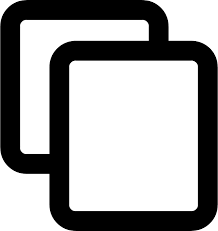
let fruits = ['Apple', 'Banana', 'Orange'];
fruits.forEach(fruit => {
console.log(fruit);
});
// Output:
// Apple
// Banana
// Orange
In this example, forEach()
is used to log each element in the fruits
array. It’s a cleaner and more readable alternative to the for
loop.
3. Using map()
Although map()
is typically used for creating new arrays, it can also be used to iterate over an array if you don’t need to modify the original array.
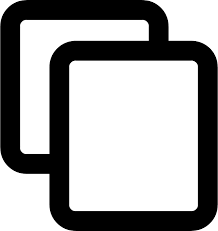
let fruits = ['Apple', 'Banana', 'Orange'];
fruits.map(fruit => {
console.log(fruit);
});
// Output:
// Apple
// Banana
// Orange
In this example, map()
is used to iterate over the fruits
array and log each element. Note that map()
returns a new array, but here we are simply using it for iteration.
4. Using for...of
Loop
The for...of
loop provides a simpler syntax for iterating over arrays (and other iterable objects) compared to the traditional for
loop. It automatically handles the iteration and gives you access to the array element directly.
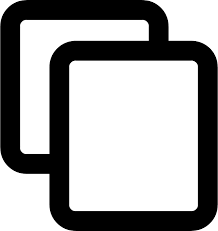
let fruits = ['Apple', 'Banana', 'Orange'];
for (let fruit of fruits) {
console.log(fruit);
}
// Output:
// Apple
// Banana
// Orange
In this example, the for...of
loop iterates over the fruits
array and directly assigns each element to the variable fruit
for logging.
5. Using for...in
Loop
The for...in
loop is generally used for iterating over object properties, but it can also be used to iterate over array indexes. However, it’s not recommended for arrays because it’s less reliable for array iteration compared to the other loops.
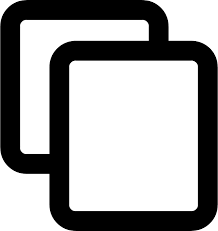
let fruits = ['Apple', 'Banana', 'Orange'];
for (let index in fruits) {
console.log(fruits[index]);
}
// Output:
// Apple
// Banana
// Orange
In this example, the for...in
loop iterates over the indices of the fruits
array and logs each element by accessing it using the index.
6. Conclusion
There are several ways to iterate over arrays in JavaScript, each with its use cases. The for
loop provides flexibility and control, while the forEach()
and map()
methods offer more concise and readable syntax for iteration. The for...of
loop is a great alternative to the traditional for
loop, and the for...in
loop should be used with caution when iterating over arrays. Understanding these iteration methods will help you choose the best one for your specific needs.
Multidimensional Arrays
1. What is a Multidimensional Array?
A multidimensional array is an array that contains other arrays as its elements. These arrays are nested, meaning you can have arrays within arrays, and each array can hold values or more arrays. The most common type of multidimensional array is the two-dimensional array, which can be thought of as a grid or matrix.
2. Declaring a Two-Dimensional Array
A two-dimensional array is an array of arrays. It can be declared by using nested arrays within an array.
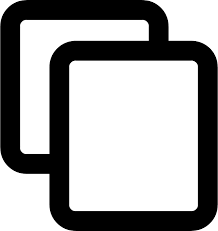
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
In this example, matrix
is a two-dimensional array with 3 rows and 3 columns, where each inner array represents a row.
3. Accessing Elements in a Two-Dimensional Array
You can access elements in a multidimensional array by specifying two indices: one for the row and one for the column.
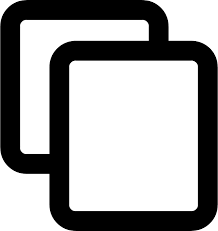
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[0][0]); // Output: 1
console.log(matrix[1][2]); // Output: 6
console.log(matrix[2][1]); // Output: 8
In this example, matrix[0][0]
accesses the element in the first row and first column, while matrix[1][2]
accesses the element in the second row and third column.
4. Iterating Over a Two-Dimensional Array
You can iterate over a two-dimensional array using nested loops. The outer loop iterates over the rows, and the inner loop iterates over the columns of each row.
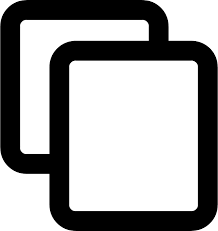
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j]);
}
}
// Output:
// 1 2 3 4 5 6 7 8 9
In this example, the outer loop iterates through each row, and the inner loop iterates through each element within that row.
5. Three-Dimensional Arrays
Multidimensional arrays can also have more than two dimensions. A three-dimensional array is an array of arrays of arrays. It can be visualized as a cube of values.
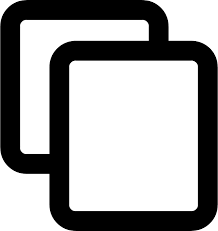
let cube = [
[
[1, 2], [3, 4]
],
[
[5, 6], [7, 8]
]
];
In this example, cube
is a three-dimensional array with 2 blocks, each containing 2 rows and 2 elements in each row.
6. Accessing Elements in a Three-Dimensional Array
Accessing elements in a three-dimensional array requires three indices: one for the block, one for the row, and one for the column.
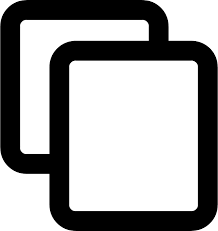
let cube = [
[
[1, 2], [3, 4]
],
[
[5, 6], [7, 8]
]
];
console.log(cube[0][1][1]); // Output: 4
console.log(cube[1][0][0]); // Output: 5
Here, cube[0][1][1]
accesses the element in the first block, second row, and second column, while cube[1][0][0]
accesses the element in the second block, first row, and first column.
7. Conclusion
Multidimensional arrays allow you to store data in a structured format with multiple dimensions, and they are useful for representing tables, grids, matrices, and more. While two-dimensional arrays are the most common, you can create arrays with more dimensions by nesting arrays within arrays. Iterating over multidimensional arrays requires using nested loops, and accessing the elements requires specifying multiple indices.
Common String Methods
1. String.length
The length
property returns the number of characters in a string, including spaces.
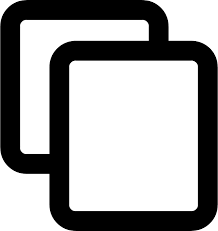
let str = "Hello World!";
console.log(str.length); // Output: 12
2. String.toUpperCase()
The toUpperCase()
method converts all characters in a string to uppercase.
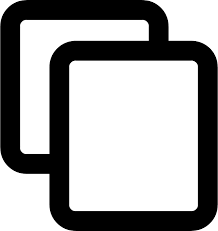
let str = "hello";
console.log(str.toUpperCase()); // Output: "HELLO"
3. String.toLowerCase()
The toLowerCase()
method converts all characters in a string to lowercase.
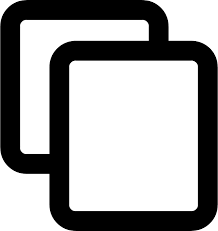
let str = "HELLO";
console.log(str.toLowerCase()); // Output: "hello"
4. String.indexOf()
The indexOf()
method returns the index of the first occurrence of a specified value in a string. It returns -1
if the value is not found.
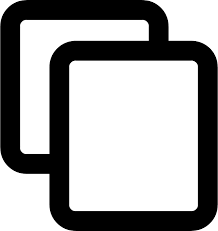
let str = "Hello World!";
console.log(str.indexOf("World")); // Output: 6
console.log(str.indexOf("world")); // Output: -1
5. String.slice()
The slice()
method extracts a part of a string and returns it as a new string. It takes two arguments: start index and end index (optional).
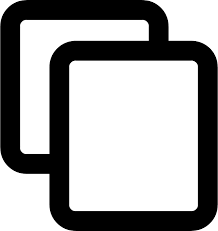
let str = "Hello World!";
console.log(str.slice(0, 5)); // Output: "Hello"
6. String.substring()
The substring()
method returns a part of the string between two specified indices. It’s similar to slice()
but does not accept negative indices.
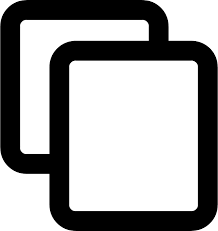
let str = "Hello World!";
console.log(str.substring(0, 5)); // Output: "Hello"
7. String.replace()
The replace()
method replaces a specified value with another value in a string. It only replaces the first occurrence unless you use a global regular expression.
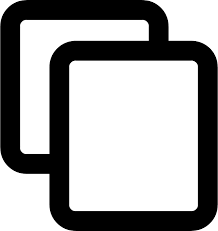
let str = "Hello World!";
console.log(str.replace("World", "JavaScript")); // Output: "Hello JavaScript!"
8. String.trim()
The trim()
method removes any leading and trailing whitespace characters from the string.
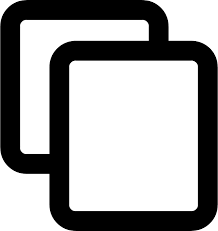
let str = " Hello World! ";
console.log(str.trim()); // Output: "Hello World!"
9. String.split()
The split()
method splits a string into an array of substrings based on a specified separator.
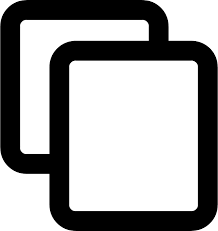
let str = "apple,banana,cherry";
console.log(str.split(",")); // Output: ["apple", "banana", "cherry"]
10. String.charAt()
The charAt()
method returns the character at a specific index in a string.
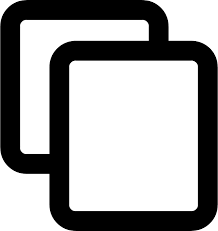
let str = "Hello";
console.log(str.charAt(1)); // Output: "e"
11. String.includes()
The includes()
method checks if a string contains a specified substring and returns true
if it does, or false
if it doesn’t.
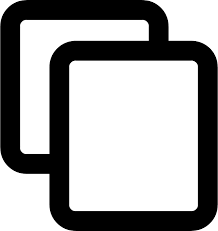
let str = "Hello World!";
console.log(str.includes("World")); // Output: true
console.log(str.includes("world")); // Output: false
12. String.repeat()
The repeat()
method repeats a string a specified number of times.
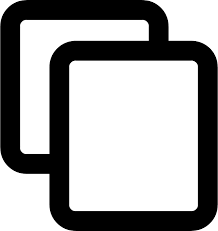
let str = "Hello ";
console.log(str.repeat(3)); // Output: "Hello Hello Hello "
13. String.startsWith() and String.endsWith()
The startsWith()
method checks if a string starts with a specified substring, while endsWith()
checks if it ends with a specified substring. Both methods return true
or false
.
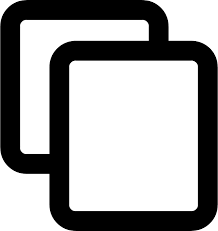
let str = "Hello World!";
console.log(str.startsWith("Hello")); // Output: true
console.log(str.endsWith("World!")); // Output: true
14. String.concat()
The concat()
method joins two or more strings and returns a new concatenated string.
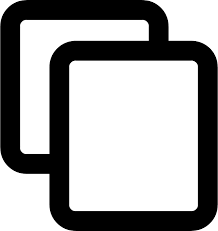
let str1 = "Hello";
let str2 = " World!";
console.log(str1.concat(str2)); // Output: "Hello World!"
15. String.match()
The match()
method retrieves the result of matching a string against a regular expression. It returns an array of matches or null
if no match is found.
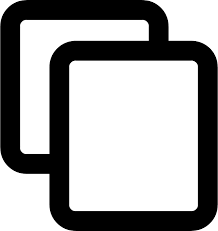
let str = "The quick brown fox jumps over the lazy dog";
console.log(str.match(/brown/)); // Output: ["brown"]
16. Conclusion
JavaScript provides a wide range of built-in string methods that make it easier to manipulate and interact with text data. These methods allow you to search, modify, and format strings efficiently, helping you handle common string operations with ease.
Template Literals
1. What are Template Literals?
Template literals are a feature in JavaScript that allows you to embed expressions inside string literals. They make it easier to work with strings, especially when you want to include variables or expressions inside a string without using concatenation.
2. Syntax of Template Literals
Template literals are defined using backticks (`
) instead of single or double quotes. Expressions inside the string are enclosed in ${}
.
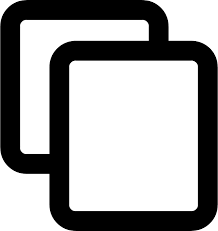
let name = "Alice";
let greeting = `Hello, ${name}!`;
console.log(greeting); // Output: "Hello, Alice!"
3. Multiline Strings
Template literals allow you to create strings that span multiple lines without the need for escape characters.
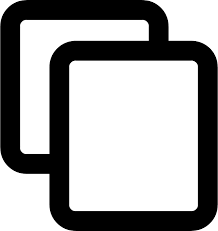
let message = `This is a
multiline string.`;
console.log(message);
// Output: "This is a
// multiline string."
4. Expression Interpolation
Template literals can include any valid JavaScript expression inside the ${}
syntax. This allows you to embed variables, calculations, function calls, and more.
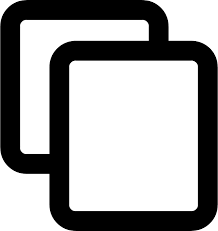
let a = 5;
let b = 10;
let sum = `The sum of ${a} and ${b} is ${a + b}.`;
console.log(sum); // Output: "The sum of 5 and 10 is 15."
5. Nesting Template Literals
You can also nest template literals inside one another for more complex string construction.
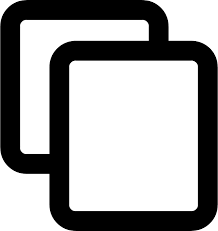
let name = "Bob";
let greeting = `Hello, ${`Mr. ${name}`}`;
console.log(greeting); // Output: "Hello, Mr. Bob"
6. Tagged Template Literals
Tagged template literals allow you to parse template literals with a function. This provides more flexibility for custom string formatting and manipulation.
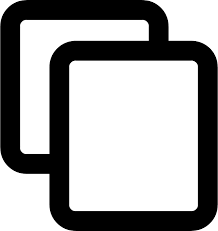
function tag(strings, ...values) {
let result = strings[0];
for (let i = 0; i < values.length; i++) {
result += values[i] + strings[i + 1];
}
return result.toUpperCase();
}
let name = "Alice";
let greeting = tag`Hello, ${name}!`;
console.log(greeting); // Output: "HELLO, ALICE!"
7. Advantages of Template Literals
- Improved Readability: Template literals make it easier to read and maintain strings that contain embedded expressions.
- Multiline Strings: You don’t need to manually add newline characters to create multiline strings.
- Expression Interpolation: You can embed expressions directly into strings without the need for concatenation.
- Tagged Templates: Tagged templates allow you to create custom string parsing functions.
8. Conclusion
Template literals are a powerful feature in JavaScript that simplify working with strings, making it easier to include expressions, create multiline strings, and define custom string formatting through tagged templates.
String Interpolation
1. What is String Interpolation?
String interpolation is the process of embedding expressions (such as variables, calculations, or function results) directly into a string. This allows you to create dynamic strings in a more readable and concise way, without the need for string concatenation.
2. String Interpolation with Template Literals
In JavaScript, string interpolation is achieved using template literals (backticks `
) and the ${}
syntax. The expressions inside the ${}
are evaluated and the result is inserted into the string.
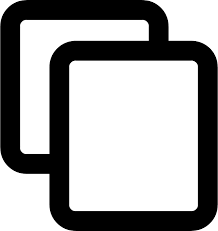
let name = "Alice";
let age = 25;
let introduction = `My name is ${name} and I am ${age} years old.`;
console.log(introduction); // Output: "My name is Alice and I am 25 years old."
3. String Interpolation with Expressions
String interpolation can include more than just variables. You can embed any valid JavaScript expression within the ${}
syntax, such as calculations or function calls.
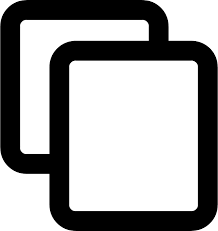
let a = 5;
let b = 10;
let result = `The sum of ${a} and ${b} is ${a + b}.`;
console.log(result); // Output: "The sum of 5 and 10 is 15."
4. Using Functions Inside Interpolation
You can also call functions inside string interpolation. This is helpful when you need to include processed or formatted data in your string.
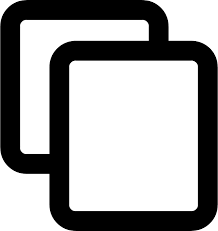
function greet(name) {
return `Hello, ${name}!`;
}
let name = "Bob";
let greeting = `The message is: ${greet(name)}`;
console.log(greeting); // Output: "The message is: Hello, Bob!"
5. Multiline Strings with Interpolation
String interpolation also allows for easy multiline strings by using template literals. You can embed expressions in these multiline strings just like any other template literal.
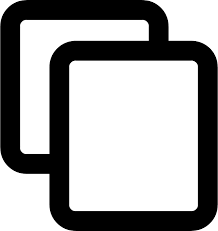
let user = "Charlie";
let message = `
Dear ${user},
Welcome to the platform!
Best regards,
Team`;
console.log(message);
// Output:
// "Dear Charlie,
// Welcome to the platform!
// Best regards,
// Team"
6. Advantages of String Interpolation
- Improved Readability: String interpolation makes your code more readable and less cluttered compared to traditional string concatenation.
- Fewer Errors: With string interpolation, you are less likely to make errors related to missing spaces or quotes between concatenated parts.
- Dynamic String Creation: It allows you to create dynamic strings that include expressions, function calls, and variable values in a clean and concise way.
7. Conclusion
String interpolation is a powerful and convenient feature in JavaScript that makes it easy to create strings with embedded expressions. By using template literals, JavaScript developers can improve code readability and reduce the need for cumbersome string concatenation.
Event Listeners (click, mouseover, etc.)
1. What is an Event Listener?
An event listener is a method in JavaScript that listens for a specific event (such as a click, mouseover, keypress, etc.) on a target element, and then executes a callback function when the event occurs. Event listeners are essential for handling user interactions in web applications.
2. Adding Event Listeners
You can add event listeners to elements using the addEventListener()
method. This method takes two parameters: the event type (such as "click", "mouseover", etc.) and the callback function to execute when the event occurs.
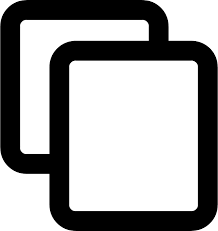
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button was clicked!");
});
3. Common Event Types
Here are some commonly used event types:
- click: Triggered when an element is clicked.
- mouseover: Triggered when the mouse pointer is moved over an element.
- mouseout: Triggered when the mouse pointer leaves an element.
- keydown: Triggered when a key is pressed down.
- keyup: Triggered when a key is released.
- submit: Triggered when a form is submitted.
- change: Triggered when an input or select element's value is changed.
4. Example: Click Event
This example shows how to listen for a click event on a button element:
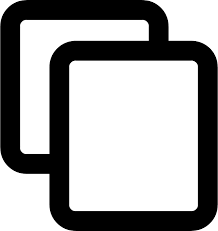
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
5. Example: Mouseover and Mouseout Events
In this example, we change the background color of a div when the mouse pointer enters and leaves the element:
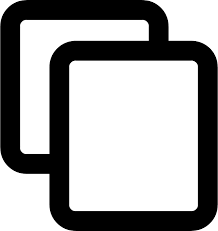
let box = document.getElementById("myBox");
box.addEventListener("mouseover", function() {
box.style.backgroundColor = "yellow";
});
box.addEventListener("mouseout", function() {
box.style.backgroundColor = "";
});
6. Removing Event Listeners
You can remove an event listener using the removeEventListener()
method. The event listener must be the same function that was originally added.
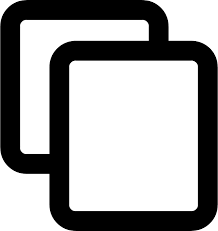
function handleClick() {
alert("Button clicked!");
}
let button = document.getElementById("myButton");
button.addEventListener("click", handleClick);
// To remove the event listener
button.removeEventListener("click", handleClick);
7. Anonymous Functions in Event Listeners
Instead of using a named function, you can also use an anonymous function directly within the event listener.
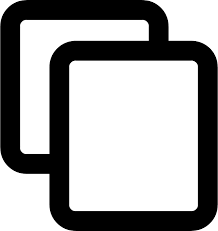
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked with an anonymous function!");
});
8. Event Propagation (Bubbling and Capturing)
In JavaScript, event propagation refers to the way events propagate through the DOM tree. There are two phases:
- Bubbling: The event starts from the target element and bubbles up to the root of the DOM.
- Capturing: The event starts from the root of the DOM and propagates down to the target element.
You can control event propagation using the stopPropagation()
method to prevent the event from bubbling or capturing further.
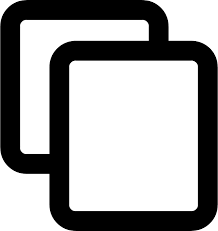
button.addEventListener("click", function(event) {
event.stopPropagation();
alert("Event propagation stopped!");
}, true);
9. Conclusion
Event listeners are an essential part of JavaScript for handling user interactions. By adding event listeners to elements, you can react to various events like clicks, mouseovers, and key presses. Additionally, you can control event propagation and remove event listeners when no longer needed.
Event Bubbling and Capturing
1. What is Event Propagation?
Event propagation refers to the way events travel through the DOM (Document Object Model) when an event is triggered on an element. The event can propagate in two different phases: bubbling and capturing. These phases determine the order in which event listeners are triggered in the DOM hierarchy.
2. Event Bubbling
Event bubbling is the default event propagation method in JavaScript. In this phase, the event starts from the target element (the element that triggered the event) and "bubbles" up through its ancestors in the DOM tree, triggering any event listeners attached to those elements along the way. The event will continue bubbling up until it reaches the root of the document.
For example, if you click on a button inside a div
, the click event will first be handled by the button, then by the parent div
, and so on until the event reaches the root of the document.
3. Event Capturing
Event capturing (also called "trickling") is the opposite of event bubbling. In this phase, the event starts at the root of the document and travels down to the target element, triggering any event listeners attached to the ancestors of the target element along the way.
Event capturing is not the default behavior, but it can be enabled by specifying the useCapture
parameter when attaching the event listener.
4. Bubbling vs Capturing
Here’s a comparison between event bubbling and event capturing:
- Bubbling: Event starts at the target element and bubbles up through the DOM.
- Capturing: Event starts at the root of the document and trickles down to the target element.
- Default Behavior: Bubbling is the default, while capturing must be explicitly enabled.
5. Example of Event Bubbling
The following example demonstrates event bubbling. The click event first triggers the button’s event listener, then the event listener on the parent div
is triggered:
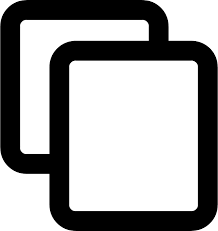
const button = document.querySelector("#myButton");
const div = document.querySelector("#myDiv");
button.addEventListener("click", function() {
alert("Button clicked!");
});
div.addEventListener("click", function() {
alert("Div clicked!");
});
When you click the button, the alert "Button clicked!" will appear first, followed by "Div clicked!" as the event bubbles up.
6. Example of Event Capturing
To enable event capturing, you must set the third argument of addEventListener
to true
. This allows the event to be captured during the capturing phase:
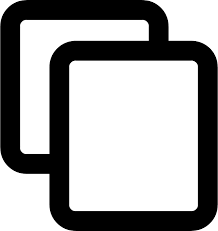
button.addEventListener("click", function() {
alert("Button clicked!");
}, true);
In this case, the event will be handled by the div
first, and then by the button
as the event trickles down.
7. Stopping Event Propagation
If you want to stop the event from propagating further (either during the capturing phase or bubbling phase), you can use the stopPropagation
method:
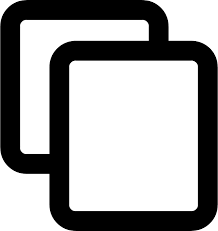
button.addEventListener("click", function(event) {
alert("Button clicked!");
event.stopPropagation();
});
In this example, the stopPropagation
method prevents the event from bubbling up to the parent div
.
8. Event Delegation
Event delegation takes advantage of event bubbling to efficiently manage events. Instead of attaching event listeners to each individual element, you can attach a single event listener to a parent element and let the event bubble up. This technique is useful for dynamically added elements.
For example, if you have a list of items that you want to listen for clicks on, you can attach a single click event listener to the ul
element:
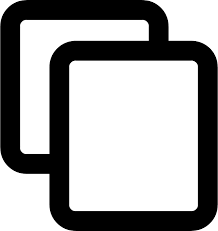
const list = document.querySelector("#myList");
list.addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
alert("List item clicked: " + event.target.textContent);
}
});
In this case, the event listener on the ul
element listens for click events on any li
element, even if they are added dynamically after the page loads.
9. Conclusion
Understanding event bubbling and capturing is essential for managing events efficiently in JavaScript. While bubbling is the default behavior, you can enable capturing when necessary. Using techniques like event delegation and controlling event propagation with stopPropagation
, you can create optimized and flexible event handling in your web applications.
Preventing Default Behavior
1. What is Default Behavior?
In JavaScript, default behavior refers to the standard action that a browser takes when an event occurs. For example, when a user clicks on a link, the default behavior is for the browser to navigate to the link’s URL. Similarly, when a user submits a form, the default behavior is for the browser to send the form data to the specified action URL.
2. Why Prevent Default Behavior?
In many cases, you may want to stop the browser from performing its default action in response to an event. This could be for a variety of reasons, such as handling form submissions with JavaScript, preventing links from navigating to a new page, or implementing custom behaviors for certain elements.
For example, you might want to handle a form submission with JavaScript instead of allowing the page to reload. Or, you may want to prevent a link from navigating to another page and instead perform some other action, such as opening a modal window.
3. Using event.preventDefault()
To prevent the default behavior of an event, you can use the preventDefault()
method on the event object. This method stops the browser from performing its default action associated with the event.
Here’s an example of preventing the default behavior of a form submission:
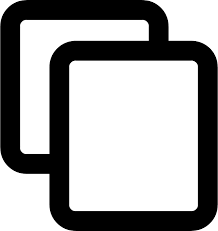
const form = document.querySelector("#myForm");
form.addEventListener("submit", function(event) {
event.preventDefault();
alert("Form submission prevented!");
});
In this example, the form will not be submitted to the server, and the page will not reload when the form is submitted. Instead, the alert message will be displayed.
4. Preventing Default Behavior on Links
You can also prevent the default behavior of anchor links (<a>
tags) to stop them from navigating to a new page. This is useful when you want to implement custom behaviors for links, such as opening a modal or loading content dynamically.
Here’s an example of preventing the default behavior of a link:
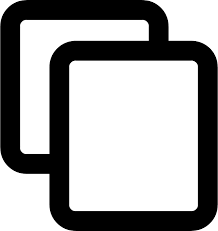
const link = document.querySelector("#myLink");
link.addEventListener("click", function(event) {
event.preventDefault();
alert("Link click prevented!");
});
In this example, when the link is clicked, the default navigation action is prevented, and the alert is shown instead.
5. Preventing Default Behavior on Other Events
In addition to form submissions and links, you can use preventDefault()
to stop other default behaviors, such as:
- Mouse events: Preventing the default context menu from opening when right-clicking on an element.
- Keyboard events: Preventing the default action when pressing certain keys, such as preventing the "Enter" key from submitting a form.
- Drag and drop events: Preventing the default behavior of dragging and dropping files or elements.
6. Example: Preventing the Default Context Menu
Sometimes, you may want to prevent the default right-click context menu from appearing. You can use preventDefault()
inside a "contextmenu" event listener:
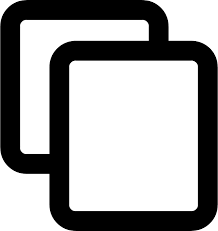
document.addEventListener("contextmenu", function(event) {
event.preventDefault();
alert("Right-click context menu prevented!");
});
In this case, when you right-click on the document, the default context menu won’t appear, and the alert will be triggered instead.
7. Example: Preventing Form Submission with Enter Key
You can prevent the default form submission behavior when the "Enter" key is pressed by listening to the "keydown" event and calling preventDefault()
:
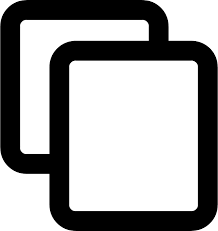
const form = document.querySelector("#myForm");
form.addEventListener("keydown", function(event) {
if (event.key === "Enter") {
event.preventDefault();
alert("Enter key pressed, form submission prevented!");
}
});
In this example, pressing the "Enter" key will not submit the form, and instead, the alert will be displayed.
8. Conclusion
Using preventDefault()
allows you to have more control over the behavior of your web application by preventing default actions that browsers perform automatically. This is especially useful when implementing custom interactions or handling forms, links, and other elements dynamically with JavaScript.
Selecting Elements (getElementById, querySelector)
1. What is Element Selection?
In JavaScript, selecting elements refers to the process of accessing HTML elements in the DOM (Document Object Model) so that you can manipulate or interact with them. JavaScript provides various methods to select elements, with getElementById
and querySelector
being two of the most commonly used methods.
2. getElementById()
The getElementById()
method is used to select an element by its unique ID. It returns a reference to the first element with the specified ID. This method is case-sensitive and can only return one element, as IDs should be unique within a document.
Here’s an example of how to use getElementById()
:
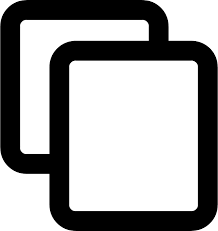
const element = document.getElementById("myElement");
In this example, getElementById
will select the element with the ID of "myElement" and store it in the element
variable.
3. Example: Changing Text Content Using getElementById()
Here’s an example of how to use getElementById()
to change the text content of an element:
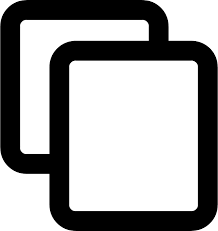
const element = document.getElementById("myElement");
element.textContent = "New Text Content";
In this example, the text content of the element with the ID "myElement" will be changed to "New Text Content".
4. querySelector()
The querySelector()
method is more versatile than getElementById()
. It allows you to select elements using any valid CSS selector (ID, class, attribute, etc.). It returns the first element that matches the specified selector. If no element is found, it returns null
.
Here’s an example of how to use querySelector()
to select an element by ID:
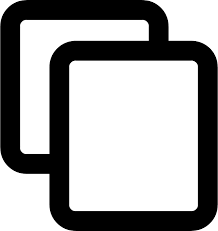
const element = document.querySelector("#myElement");
The querySelector()
method can also be used to select elements by other CSS selectors, such as:
class:
document.querySelector(".myClass")
attribute:
document.querySelector("[type='text']")
element type:
document.querySelector("div")
5. Example: Using querySelector() with Different Selectors
Here are some examples of using querySelector()
with different CSS selectors:
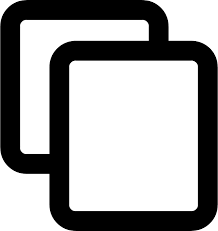
const classElement = document.querySelector(".myClass");
const inputElement = document.querySelector("[type='text']");
const divElement = document.querySelector("div");
In these examples, querySelector()
selects the first element with the class "myClass", the first text input element, and the first <div>
element, respectively.
6. Selecting Multiple Elements (querySelectorAll)
If you want to select all elements that match a certain selector, you can use querySelectorAll()
. This method returns a NodeList of all matching elements, which you can iterate over or manipulate.
Here’s an example of selecting all elements with a specific class:
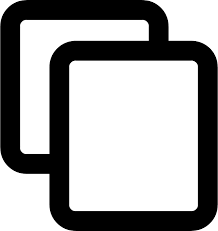
const elements = document.querySelectorAll(".myClass");
In this example, querySelectorAll()
returns a NodeList of all elements with the class "myClass". You can then loop through the NodeList to manipulate each element.
7. Example: Iterating Over Multiple Elements
Here’s an example of how to iterate over a NodeList returned by querySelectorAll()
:
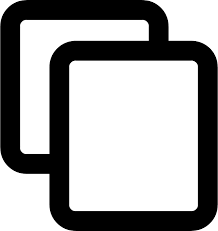
const elements = document.querySelectorAll(".myClass");
elements.forEach(function(element) {
element.textContent = "Updated Text";
});
In this example, the text content of each element with the class "myClass" is updated to "Updated Text".
8. Conclusion
Both getElementById()
and querySelector()
are powerful methods for selecting elements in the DOM. While getElementById()
is straightforward for selecting elements by ID, querySelector()
offers more flexibility by allowing you to use any valid CSS selector. Understanding both methods will help you efficiently interact with and manipulate elements in your web pages.
Adding and Removing Elements
1. Introduction to Adding and Removing Elements
In JavaScript, you can dynamically add, modify, or remove HTML elements from the DOM (Document Object Model) to create interactive and dynamic web pages. The process of adding and removing elements involves selecting the parent element and then using methods to insert or delete child elements.
2. Adding Elements
To add a new element to the DOM, you can use methods like createElement()
to create a new element, and appendChild()
, insertBefore()
, or append()
to insert it into the DOM.
3. Example: Adding an Element Using appendChild()
The appendChild()
method adds a new element as the last child of a specified parent element. Here’s an example:
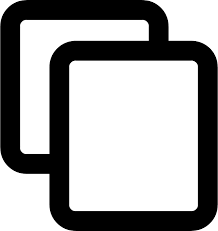
const newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph!";
const parentElement = document.getElementById("parent");
parentElement.appendChild(newElement);
In this example, a new <p>
element is created, its text content is set, and it is appended as the last child of the element with the ID "parent".
4. Example: Adding Multiple Elements Using append()
The append()
method allows you to append multiple nodes or strings to a parent element. Here’s an example of adding multiple elements:
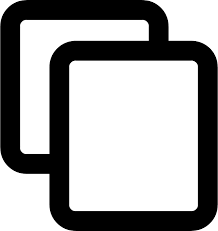
const newDiv = document.createElement("div");
newDiv.textContent = "This is a new div element!";
const newSpan = document.createElement("span");
newSpan.textContent = "This is a new span element!";
const parentElement = document.getElementById("parent");
parentElement.append(newDiv, newSpan);
In this example, both a <div>
and a <span>
element are created and appended to the parent element with the ID "parent".
5. Removing Elements
To remove an element from the DOM, you can use the removeChild()
method or the remove()
method.
6. Example: Removing an Element Using removeChild()
The removeChild()
method is used to remove a child element from its parent element. Here’s an example:
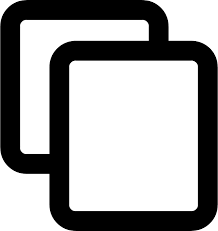
const parentElement = document.getElementById("parent");
const childElement = document.getElementById("child");
parentElement.removeChild(childElement);
In this example, the child element with the ID "child" is removed from its parent element with the ID "parent".
7. Example: Removing an Element Using remove()
The remove()
method can be used directly on the element you want to remove. Here’s an example:
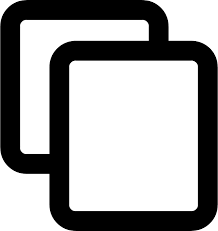
const elementToRemove = document.getElementById("child");
elementToRemove.remove();
In this example, the element with the ID "child" is removed from the DOM directly using the remove()
method.
8. Inserting Elements at Specific Positions
If you want to insert elements at a specific position (not just at the end), you can use the insertBefore()
method. This allows you to specify a reference node and insert the new element before it.
9. Example: Inserting an Element Using insertBefore()
Here’s an example of how to insert an element before another element:
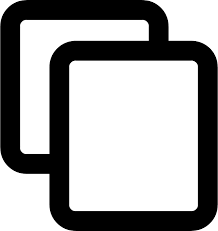
const newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph inserted before another element.";
const parentElement = document.getElementById("parent");
const referenceElement = document.getElementById("reference");
parentElement.insertBefore(newElement, referenceElement);
In this example, a new <p>
element is created and inserted before the element with the ID "reference" within the parent element with the ID "parent".
10. Conclusion
Adding and removing elements in JavaScript is a powerful way to interact with and manipulate the DOM. Whether you're adding new content, removing outdated elements, or inserting elements at specific positions, these methods give you full control over the structure of your web pages. By mastering these techniques, you can create dynamic and responsive websites that adapt to user interactions and other events.
Changing Attributes and Styles
1. Introduction to Changing Attributes and Styles
In JavaScript, you can dynamically modify the attributes and styles of HTML elements to change their appearance or behavior. Changing attributes like src
, href
, or class
can alter the functionality of an element, while changing styles can adjust its visual presentation without needing to modify the CSS stylesheet.
2. Changing Attributes
Attributes of an HTML element (like src
for images, href
for links, etc.) can be modified using JavaScript. You can use the setAttribute()
method to set new values for an attribute or the getAttribute()
method to retrieve its current value.
3. Example: Changing an Image Source Using setAttribute()
Let’s say you want to change the src
attribute of an image:
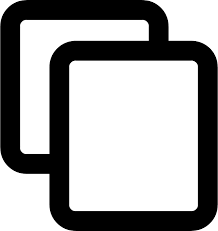
const image = document.getElementById("myImage");
image.setAttribute("src", "new-image.jpg");
In this example, the src
attribute of the image with the ID "myImage" is updated to point to a new image file, "new-image.jpg".
4. Example: Changing a Link's Destination Using setAttribute()
Similarly, you can change the destination URL of a link:
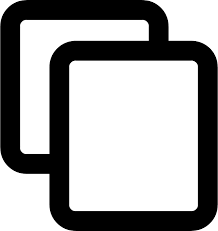
const link = document.getElementById("myLink");
link.setAttribute("href", "https://www.example.com");
In this example, the href
attribute of the link with the ID "myLink" is updated to redirect to "https://www.example.com".
5. Getting Attributes Using getAttribute()
You can also retrieve the value of an element’s attribute:
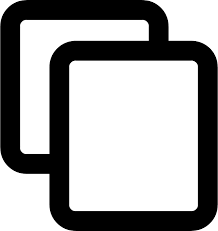
const imageSrc = image.getAttribute("src");
console.log(imageSrc); // Logs the current src of the image
This example retrieves the current src
attribute of the image and logs it to the console.
6. Changing Styles
JavaScript allows you to dynamically change the CSS styles of elements. You can modify the style
property of an element to change its inline CSS styles.
7. Example: Changing Background Color Using JavaScript
Here’s an example of changing the background color of an element:
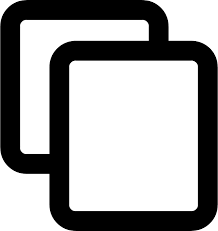
const element = document.getElementById("myElement");
element.style.backgroundColor = "lightblue";
In this example, the background color of the element with the ID "myElement" is changed to "lightblue".
8. Example: Changing Multiple Styles Using JavaScript
You can also modify multiple styles at once:
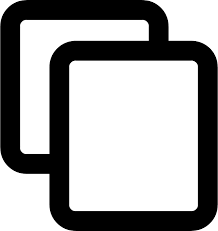
const element = document.getElementById("myElement");
element.style.width = "200px";
element.style.height = "100px";
element.style.border = "2px solid black";
This example changes the width, height, and border of the element with the ID "myElement".
9. Modifying CSS Classes
Instead of directly modifying individual styles, you can also modify CSS classes using JavaScript. The classList
property allows you to add, remove, or toggle CSS classes on an element.
10. Example: Adding a CSS Class Using classList
You can add a CSS class to an element dynamically:
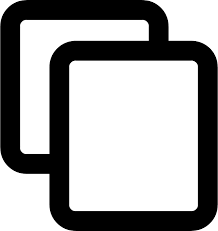
const element = document.getElementById("myElement");
element.classList.add("highlight");
In this example, the "highlight" class is added to the element with the ID "myElement", which can apply predefined styles from your CSS.
11. Example: Removing a CSS Class Using classList
Similarly, you can remove a CSS class from an element:
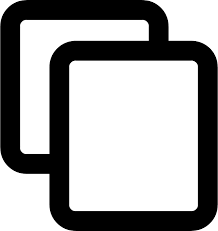
const element = document.getElementById("myElement");
element.classList.remove("highlight");
This example removes the "highlight" class from the element with the ID "myElement".
12. Example: Toggling a CSS Class Using classList
You can toggle a class on and off with the toggle()
method:
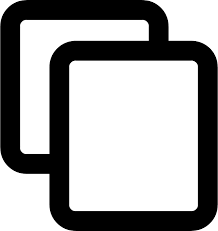
const element = document.getElementById("myElement");
element.classList.toggle("highlight");
This example will add the "highlight" class if it's not present, and remove it if it is already applied.
13. Conclusion
Changing attributes and styles dynamically with JavaScript allows for interactive web pages. Whether you're modifying elements' attributes, adjusting inline styles, or toggling CSS classes, JavaScript gives you the power to create dynamic, responsive user interfaces that can change based on user interaction or other events.
DOM Events
1. Introduction to DOM Events
DOM (Document Object Model) events are actions or occurrences that happen in the browser, such as when a user clicks a button, submits a form, or interacts with any element on a webpage. JavaScript allows you to listen for these events and execute functions in response. These events can be triggered by user actions, browser activities, or even by JavaScript code itself.
2. Types of DOM Events
There are many types of DOM events, including:
- Mouse Events: events triggered by mouse actions such as clicks, hover, and mouse movement (
click
,mouseover
,mouseout
, etc.) - Keyboard Events: events triggered by keypress actions (
keydown
,keyup
,keypress
) - Form Events: events triggered by form interactions such as submitting or changing a form input (
submit
,change
,focus
, etc.) - Window Events: events triggered by changes to the browser window or document, like resizing or scrolling (
resize
,scroll
) - Touch Events: events triggered by touch interactions on mobile devices (
touchstart
,touchend
, etc.)
3. Adding Event Listeners
In JavaScript, the most common way to handle events is by using the addEventListener()
method. This method attaches an event listener to an element, allowing you to specify the event type and the function to execute when the event occurs.
4. Example: Adding a Click Event Listener
Here’s an example of adding a click event listener to a button:
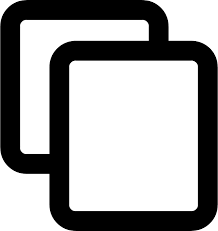
const button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
In this example, when the button with the ID "myButton" is clicked, an alert will pop up with the message "Button clicked!".
5. Example: Adding a Mouseover Event Listener
Here’s an example of adding a mouseover event listener to change the color of an element when the mouse hovers over it:
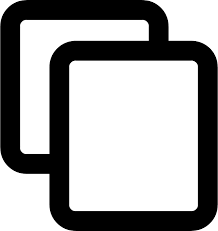
const element = document.getElementById("myElement");
element.addEventListener("mouseover", function() {
element.style.backgroundColor = "yellow";
});
In this example, the background color of the element with the ID "myElement" will change to yellow when the mouse hovers over it.
6. Event Handling with Anonymous Functions
You can also use anonymous functions (functions without a name) when adding event listeners:
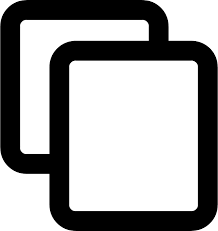
const button = document.getElementById("myButton");
button.addEventListener("click", function() {
console.log("Button was clicked!");
});
In this case, the anonymous function is executed when the button is clicked, logging the message "Button was clicked!" to the console.
7. Removing Event Listeners
If you no longer want to listen for an event, you can remove the event listener using the removeEventListener()
method:
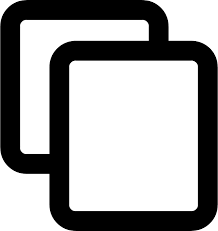
const button = document.getElementById("myButton");
function handleClick() {
alert("Button clicked!");
}
button.addEventListener("click", handleClick);
// To remove the event listener:
button.removeEventListener("click", handleClick);
In this example, the event listener is removed, so the "Button clicked!" alert will no longer appear when the button is clicked.
8. Event Propagation: Bubbling and Capturing
Event propagation refers to the way events are handled in the DOM tree. Events can propagate in two phases:
- Bubbling: The event starts from the target element and "bubbles" up to the root of the document.
- Capturing: The event starts from the root of the document and is captured by the target element.
By default, events bubble up the DOM tree, but you can control the phase during which the event is triggered by specifying an optional third argument when adding the event listener.
9. Example: Event Bubbling
In event bubbling, the event is first captured and handled by the innermost element, and then propagated outward to its parent elements:
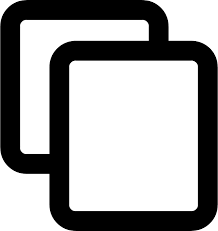
document.getElementById("parent").addEventListener("click", function() {
alert("Parent clicked!");
}, false);
document.getElementById("child").addEventListener("click", function() {
alert("Child clicked!");
}, false);
In this case, if the child element is clicked, both the child and the parent will trigger their respective event listeners in a bubbling fashion.
10. Example: Event Capturing
For event capturing, you can pass true
as the third argument to the addEventListener()
method:
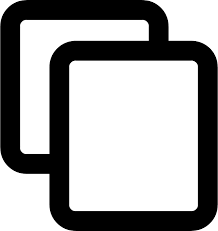
document.getElementById("parent").addEventListener("click", function() {
alert("Parent clicked!");
}, true);
document.getElementById("child").addEventListener("click", function() {
alert("Child clicked!");
}, true);
In this case, when the child element is clicked, the parent element’s event listener will be triggered first, followed by the child element’s event listener (capturing phase).
11. Event Object
The event object contains information about the event that occurred. You can use this object to get details about the event, such as the target element and the type of event.
12. Example: Accessing the Event Object
Here’s an example of accessing the event object in an event handler:
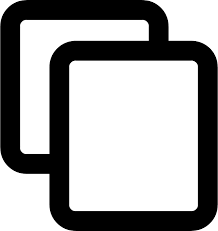
const button = document.getElementById("myButton");
button.addEventListener("click", function(event) {
console.log("Event type: " + event.type);
console.log("Event target: " + event.target);
});
In this example, when the button is clicked, the event type and the target element (button) will be logged to the console.
13. Conclusion
DOM events are a fundamental part of web development, enabling interactive and dynamic web pages. By using event listeners, JavaScript allows you to respond to user actions and create engaging user experiences. Understanding event propagation, the event object, and event handlers will help you effectively manage events in your web applications.
setTimeout and setInterval
1. Introduction
In JavaScript, setTimeout()
and setInterval()
are two built-in functions that allow you to execute code after a specified delay or at regular intervals, respectively. These functions are commonly used for timing events, animations, and handling delayed actions in web applications.
2. setTimeout
setTimeout()
is used to execute a function once after a specified delay (in milliseconds). After the delay, the function is called once, and the timer is cleared.
Syntax
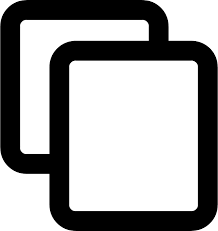
setTimeout(function, delay, [arguments]);
- function: The function to be executed after the delay.
- delay: The time in milliseconds (1000 ms = 1 second) after which the function will be executed.
- arguments: Optional parameters that will be passed to the function (if any).
Example: Using setTimeout
Here’s an example where a message is logged to the console after 2 seconds:
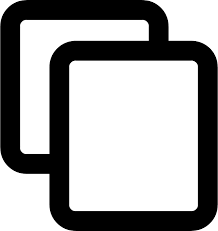
setTimeout(function() {
console.log("This message is displayed after 2 seconds.");
}, 2000);
In this example, the setTimeout()
function waits for 2000 milliseconds (2 seconds) before executing the provided function, which logs the message to the console.
3. Clearing a Timeout
If you want to cancel a timeout before it executes, you can use the clearTimeout()
method. This is useful when you want to stop the timer from executing the function if certain conditions change.
Syntax
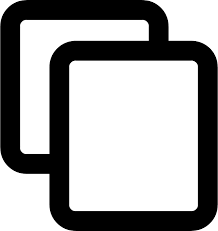
const timeoutId = setTimeout(function() {
console.log("This won't be logged.");
}, 3000);
clearTimeout(timeoutId);
In this example, the clearTimeout()
function is used to cancel the timeout, so the message will not be logged to the console.
4. setInterval
setInterval()
is used to repeatedly execute a function at specified intervals. The function will continue to execute at the given time interval until clearInterval()
is called to stop it.
Syntax
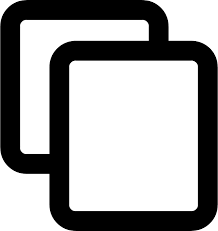
setInterval(function, interval, [arguments]);
- function: The function to be executed at regular intervals.
- interval: The time in milliseconds between each function call (1000 ms = 1 second).
- arguments: Optional parameters that will be passed to the function (if any).
Example: Using setInterval
Here’s an example where a message is logged to the console every 3 seconds:
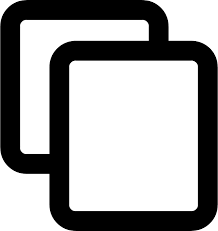
setInterval(function() {
console.log("This message is displayed every 3 seconds.");
}, 3000);
In this example, the setInterval()
function logs the message every 3000 milliseconds (3 seconds) until the interval is cleared.
5. Clearing an Interval
To stop an interval from continuing to execute, you can use the clearInterval()
function. You need to call setInterval()
and store its return value (the interval ID) in a variable, which is then used with clearInterval()
.
Syntax
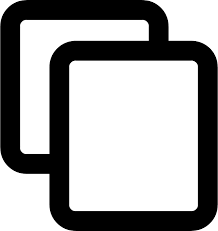
const intervalId = setInterval(function() {
console.log("This message will be logged repeatedly every 2 seconds.");
}, 2000);
clearInterval(intervalId);
In this example, the interval is cleared and will stop logging the message after the interval ID is passed to clearInterval()
.
6. Combining setTimeout and setInterval
Both setTimeout()
and setInterval()
are useful for controlling time-based actions in JavaScript. You can combine them to execute certain tasks with delays and intervals, providing more control over event handling and animations.
Example: Using setTimeout with setInterval
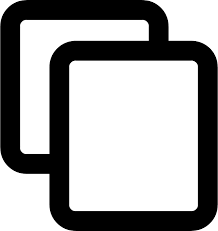
let count = 0;
const intervalId = setInterval(function() {
count++;
console.log("Interval count: " + count);
if (count === 5) {
clearInterval(intervalId);
console.log("Interval stopped.");
}
}, 1000);
setTimeout(function() {
console.log("This message is logged after 3 seconds.");
}, 3000);
In this example, setInterval()
logs the count every second, and after 3 seconds, setTimeout()
logs a message. Once the count reaches 5, the interval is cleared.
7. Conclusion
setTimeout()
and setInterval()
are powerful tools for handling timed actions and periodic tasks in JavaScript. By using these functions, you can create dynamic web applications that respond to time-based events, delay actions, or repeat tasks at regular intervals.
Clearing Timers
1. Introduction
In JavaScript, both setTimeout()
and setInterval()
functions return a unique identifier (timer ID) when called. This timer ID can be used to cancel or stop a timer before it executes or repeats. Clearing timers is useful when you want to stop an action that was scheduled to occur after a delay or at regular intervals.
2. Clearing a Timeout with clearTimeout()
clearTimeout()
is used to cancel a setTimeout()
before it has executed. When you call setTimeout()
, it returns a timer ID that you can use with clearTimeout()
to stop the timer.
Syntax
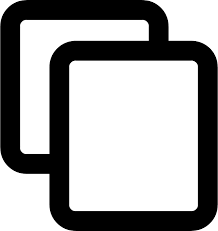
clearTimeout(timeoutId);
timeoutId: The ID returned by setTimeout()
that identifies the timeout to be cleared.
Example: Clearing a Timeout
The following code sets a timeout to log a message after 5 seconds, but the timeout is cleared before it executes:
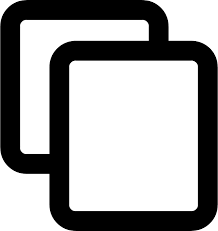
const timeoutId = setTimeout(function() {
console.log("This will not be logged.");
}, 5000);
// Clear the timeout before it executes
clearTimeout(timeoutId);
In this example, the timeout is scheduled to log a message after 5 seconds, but clearTimeout()
is called before the timer completes, so the message is never logged.
3. Clearing an Interval with clearInterval()
clearInterval()
is used to cancel a setInterval()
before it continues to execute at the specified intervals. Similar to setTimeout()
, setInterval()
returns a timer ID, which is used with clearInterval()
to stop the interval.
Syntax
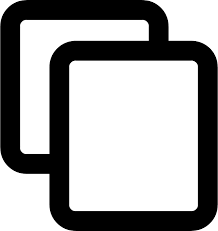
clearInterval(intervalId);
intervalId: The ID returned by setInterval()
that identifies the interval to be cleared.
Example: Clearing an Interval
The following example logs a message every 2 seconds, but the interval is cleared after 5 seconds:
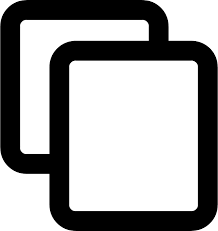
const intervalId = setInterval(function() {
console.log("This message is logged every 2 seconds.");
}, 2000);
// Clear the interval after 5 seconds
setTimeout(function() {
clearInterval(intervalId);
console.log("Interval cleared.");
}, 5000);
In this example, setInterval()
logs the message every 2 seconds, but after 5 seconds, clearInterval()
is called to stop the interval, and a message is logged indicating the interval was cleared.
4. When to Clear Timers
There are scenarios where you might need to clear timers:
- Preventing Unnecessary Actions: If a user performs an action (like clicking a button) that makes the timer unnecessary, you should clear it to prevent the action from executing.
- Stopping Animations: If you're using
setInterval()
for animations, you might want to stop the interval once the animation is complete. - Clearing Unused Timers: It's good practice to clear any timers when they are no longer needed, especially in long-running web applications, to avoid memory leaks.
5. Example: Using clearTimeout() and clearInterval() Together
In the following example, both clearTimeout()
and clearInterval()
are used to stop a timeout and interval:
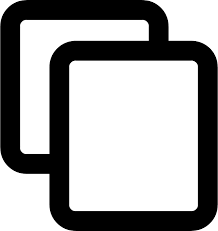
const timeoutId = setTimeout(function() {
console.log("This will not be logged due to clearTimeout.");
}, 4000);
const intervalId = setInterval(function() {
console.log("This will not be logged due to clearInterval.");
}, 1000);
// Clearing both timeout and interval
clearTimeout(timeoutId);
clearInterval(intervalId);
In this example, both the timeout and the interval are cleared before they have a chance to execute their respective functions, preventing any messages from being logged.
6. Conclusion
Clearing timers is essential for managing scheduled functions effectively in JavaScript. By using clearTimeout()
and clearInterval()
, you can ensure that unnecessary actions do not execute, optimize resource management, and prevent unexpected behavior in web applications.
try-catch-finally
1. Introduction
In JavaScript, try-catch-finally
is a mechanism used for handling exceptions (errors) in code. It allows you to attempt executing a block of code (try
), catch any errors that occur (catch
), and execute any code that must run regardless of whether an error occurred (finally
).
2. Syntax
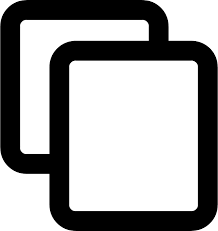
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
} finally {
// Code to run regardless of an error
}
3. Explanation of Each Part
- try: This block contains the code that may throw an error. If an error occurs, it is passed to the
catch
block. - catch: This block catches the error that occurred in the
try
block. It receives the error object, which can be used to identify or handle the error. - finally: This block is optional and contains code that will always be executed, regardless of whether an error was thrown or not. It is typically used for cleanup actions (e.g., closing resources, releasing memory).
4. Example: Basic try-catch-finally
The following example demonstrates a basic try-catch-finally
structure:
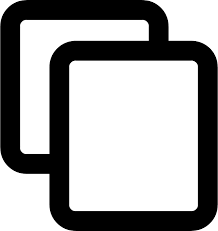
try {
let result = 10 / 0; // Division by zero (Infinity)
console.log(result);
} catch (error) {
console.error("An error occurred: " + error.message);
} finally {
console.log("This block is always executed.");
}
In this example, since division by zero does not throw an error but results in Infinity
, the catch
block is not executed. However, the finally
block will always run, printing the message "This block is always executed."
5. Example: Handling Errors
Here’s an example of using try-catch
to handle errors, such as when a function attempts to parse invalid JSON data:
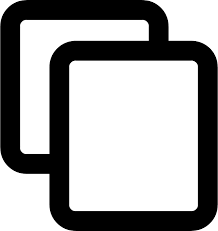
try {
let json = '{ "name": "John", age: 30 }'; // Invalid JSON (missing quotes around age)
let user = JSON.parse(json);
} catch (error) {
console.error("Invalid JSON: " + error.message);
} finally {
console.log("Attempted to parse JSON.");
}
In this case, the catch
block catches the error thrown by the JSON.parse()
method when it encounters invalid JSON syntax. The finally
block ensures that a message is logged regardless of whether the error occurred.
6. Example: Using Finally for Cleanup
The finally
block is commonly used for cleanup operations, such as closing a file or releasing a network resource:
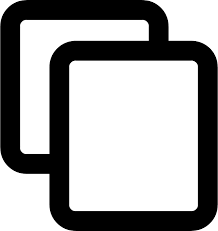
function fetchData() {
try {
console.log("Fetching data...");
throw new Error("Network failure");
} catch (error) {
console.error("An error occurred while fetching data: " + error.message);
} finally {
console.log("Closing connections and cleaning up resources.");
}
}
fetchData();
In this example, even if an error occurs while fetching the data (e.g., network failure), the finally
block ensures that cleanup is done.
7. Best Practices
- Use
try-catch
for expected errors: Usetry-catch
when you expect certain errors to occur, such as parsing JSON or reading a file. - Keep
try
blocks small: Limit the code inside thetry
block to the smallest possible code that might throw an error, which helps narrow down error sources. - Don’t overuse
try-catch
: Usetry-catch
sparingly, as it can be inefficient and make code harder to read. Prefer handling predictable cases with conditional logic when possible. - Use
finally
for cleanup: Use thefinally
block to handle cleanup tasks like closing files, releasing resources, or resetting variables.
8. Conclusion
The try-catch-finally
statement in JavaScript is a powerful way to handle errors gracefully and ensure that important cleanup tasks are always performed. By using this structure effectively, you can build more robust applications that gracefully handle unexpected scenarios and errors.
throw Keyword
1. Introduction
The throw
keyword in JavaScript is used to manually throw an exception (error). This allows developers to trigger an error when a specific condition is met, or when something unexpected occurs during the execution of the program. The throw
keyword can be used to create custom errors that can be caught and handled using try-catch
blocks.
2. Syntax
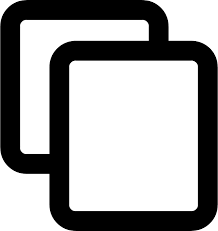
throw expression;
The expression can be any value, but typically it will be an Error
object or an instance of a custom error class.
3. Throwing Custom Errors
You can throw custom errors by creating a new Error
object or a subclass of Error
:
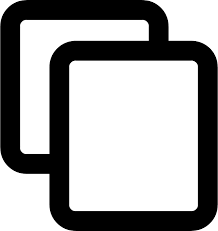
throw new Error("Something went wrong!");
This will throw a new error with the message "Something went wrong!" that can be caught in a try-catch
block.
4. Example: Throwing and Catching Errors
Here’s an example of how to throw and catch an error using throw
:
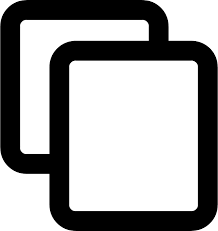
try {
throw new Error("Custom error triggered!");
} catch (error) {
console.error("Caught error: " + error.message);
}
In this example, the throw
keyword creates a custom error, and the catch
block catches the error and logs its message.
5. Example: Throwing Errors Based on Conditions
Errors can also be thrown conditionally depending on specific criteria. For example, if a function receives an invalid argument, it can throw an error:
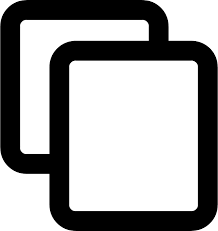
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero!");
}
return a / b;
}
try {
console.log(divide(10, 0));
} catch (error) {
console.error("Error: " + error.message);
}
In this example, the divide
function checks if the divisor is zero. If it is, the function throws an error, which is caught in the catch
block.
6. Throwing Custom Error Types
You can also throw custom error types by creating a subclass of the Error
class:
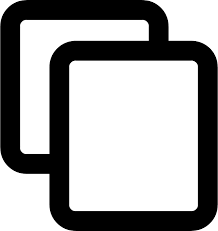
class ValidationError extends Error {
constructor(message) {
super(message);
this.name = "ValidationError";
}
}
try {
throw new ValidationError("Invalid input!");
} catch (error) {
if (error instanceof ValidationError) {
console.error("Caught validation error: " + error.message);
} else {
console.error("An unknown error occurred: " + error.message);
}
}
In this example, we create a custom error class called ValidationError
, which extends the built-in Error
class. This allows us to throw and handle specific types of errors.
7. Best Practices for Using throw
- Throw specific errors: When throwing errors, try to be as specific as possible about the error type and message to make it easier to debug.
- Use
throw
for critical errors: Usethrow
to handle errors that prevent the program from continuing in a meaningful way (e.g., invalid inputs, missing resources). - Provide helpful error messages: Include clear, descriptive messages when throwing errors so that developers know what went wrong.
- Handle errors with
try-catch
: Always usetry-catch
when throwing errors to ensure that the errors are handled gracefully and don’t crash the application.
8. Conclusion
The throw
keyword is a powerful tool in JavaScript for handling errors. By using throw
, you can create custom error messages, trigger exceptions when specific conditions are met, and improve the robustness of your programs by handling unexpected situations in a controlled way.
Custom Error Handling
1. Introduction
Custom error handling in JavaScript allows developers to create and manage their own error types, providing more detailed and specific error messages. By creating custom errors, you can define error conditions and handle them in a way that is tailored to your application’s needs, making debugging and maintenance easier.
2. Creating Custom Error Classes
To create custom error types, you can extend the built-in Error
class. This allows you to define unique error types that can be used to indicate specific problems in your application.
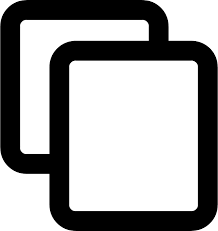
class CustomError extends Error {
constructor(message) {
super(message);
this.name = "CustomError"; // Custom error name
}
}
In this example, the CustomError
class extends the Error
class and adds a custom name
property to indicate that it's a "CustomError." You can also add additional properties or methods to the custom error class if needed.
3. Throwing Custom Errors
Once you have defined a custom error class, you can throw custom errors in your code when specific conditions are met:
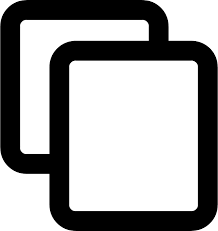
function checkNumber(num) {
if (num < 0) {
throw new CustomError("Negative numbers are not allowed!");
}
return num;
}
try {
console.log(checkNumber(-5));
} catch (error) {
console.error(error.name + ": " + error.message); // CustomError: Negative numbers are not allowed!
}
In this example, the checkNumber
function throws a CustomError
if the number is negative. The catch
block then logs the error's name and message.
4. Using Multiple Custom Error Types
You can create multiple custom error types to handle different types of errors more effectively. For example, you can define a ValidationError
and an AuthorizationError
:
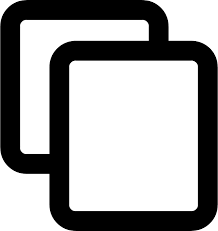
class ValidationError extends Error {
constructor(message) {
super(message);
this.name = "ValidationError";
}
}
class AuthorizationError extends Error {
constructor(message) {
super(message);
this.name = "AuthorizationError";
}
}
function login(user) {
if (!user) {
throw new ValidationError("User information is missing!");
}
if (!user.hasAccess) {
throw new AuthorizationError("User does not have access.");
}
return "Login successful!";
}
try {
console.log(login({ hasAccess: false }));
} catch (error) {
if (error instanceof ValidationError) {
console.error("Validation Error: " + error.message);
} else if (error instanceof AuthorizationError) {
console.error("Authorization Error: " + error.message);
} else {
console.error("General Error: " + error.message);
}
}
In this example, two custom error types—ValidationError
and AuthorizationError
—are used to differentiate between different error conditions in the login process. The instanceof
operator is used to check the type of the error in the catch
block.
5. Error Handling Best Practices
- Use descriptive error messages: Ensure your error messages provide meaningful information to the developer, such as what went wrong and why.
- Throw specific error types: Create multiple custom error types to handle different error conditions separately. This allows for more precise error handling and debugging.
- Include relevant data: When throwing custom errors, include any relevant data (such as input values or state) in the error object to assist with debugging.
- Use
try-catch
blocks effectively: Always handle errors thrown by custom error classes usingtry-catch
blocks to ensure your application doesn’t crash. - Don’t throw generic errors: Avoid throwing generic errors like the base
Error
class. Instead, create more specific custom errors for different situations.
6. Example: Handling Different Error Types with Custom Handlers
Here's an example of a more complex error-handling system where different error types are handled by different functions:
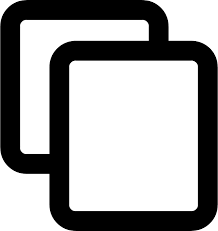
function handleValidationError(error) {
console.error("Validation Error: " + error.message);
}
function handleAuthorizationError(error) {
console.error("Authorization Error: " + error.message);
}
function handleError(error) {
if (error instanceof ValidationError) {
handleValidationError(error);
} else if (error instanceof AuthorizationError) {
handleAuthorizationError(error);
} else {
console.error("Unknown Error: " + error.message);
}
}
try {
throw new AuthorizationError("You do not have permission to access this resource.");
} catch (error) {
handleError(error);
}
In this example, different error-handling functions are used to handle different types of custom errors.
7. Conclusion
Custom error handling in JavaScript is an effective way to handle application-specific errors by creating custom error types. This approach allows you to handle errors more efficiently, provide clearer error messages, and improve the overall debugging process. By using custom error classes, you can make your code more maintainable and robust.
Creating and Using Promises
1. Introduction to Promises
A promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises allow you to handle asynchronous operations in a more manageable way compared to traditional callback functions.
2. Creating a Promise
To create a promise, you use the new Promise()
constructor, which takes a function known as the "executor." The executor function receives two arguments—resolve
and reject
—that are used to indicate the result of the asynchronous operation.
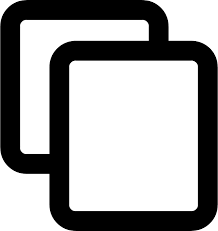
const myPromise = new Promise((resolve, reject) => {
const isSuccess = true;
if (isSuccess) {
resolve("Operation successful!");
} else {
reject("Operation failed!");
}
});
In this example, the promise will either be resolved with the message "Operation successful!" or rejected with "Operation failed!" depending on the value of isSuccess
.
3. Using Promises
Once a promise is created, you can use its then()
and catch()
methods to handle the resolution and rejection of the promise, respectively.
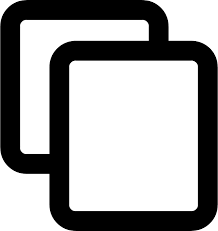
myPromise
.then(result => {
console.log(result); // Output: "Operation successful!"
})
.catch(error => {
console.error(error); // Output: "Operation failed!"
});
The then()
method is called when the promise is resolved, while the catch()
method is called when the promise is rejected.
4. Chaining Promises
Promises can be chained together. This is useful when you need to perform multiple asynchronous operations in sequence. Each then()
method returns a new promise, allowing you to chain further actions.
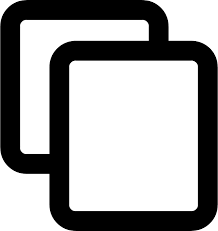
myPromise
.then(result => {
console.log(result);
return "Next operation";
})
.then(nextResult => {
console.log(nextResult); // Output: "Next operation"
})
.catch(error => {
console.error(error);
});
In this example, the result of the first operation is passed to the next then()
in the chain, allowing you to handle subsequent operations in a sequence.
5. Promise.all() and Promise.race()
JavaScript provides two useful methods for handling multiple promises:
- Promise.all(): Waits for all promises to be resolved (or for one to be rejected).
- Promise.race(): Resolves or rejects as soon as one of the promises is resolved or rejected.
Using Promise.all()
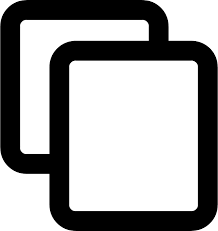
const promise1 = Promise.resolve("First operation");
const promise2 = Promise.resolve("Second operation");
const promise3 = new Promise((resolve, reject) => setTimeout(resolve, 1000, "Third operation"));
Promise.all([promise1, promise2, promise3])
.then(results => {
console.log(results); // Output: ["First operation", "Second operation", "Third operation"]
})
.catch(error => {
console.error(error);
});
Promise.all()
waits for all the promises in the array to resolve. If any promise is rejected, the catch()
block will be triggered.
Using Promise.race()
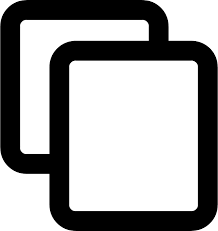
const promise4 = new Promise(resolve => setTimeout(resolve, 500, "Fast operation"));
const promise5 = new Promise(resolve => setTimeout(resolve, 1000, "Slow operation"));
Promise.race([promise4, promise5])
.then(result => {
console.log(result); // Output: "Fast operation"
})
.catch(error => {
console.error(error);
});
Promise.race()
resolves or rejects as soon as one of the promises resolves or rejects, whichever happens first.
6. Handling Errors with Promises
When working with promises, it’s important to handle errors appropriately. You can use the catch()
method to catch any errors that occur during the execution of promises.
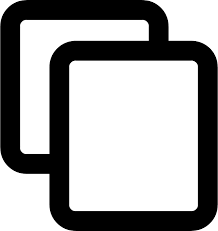
const failingPromise = new Promise((resolve, reject) => {
reject("Something went wrong!");
});
failingPromise
.then(result => {
console.log(result);
})
.catch(error => {
console.error("Error occurred: " + error); // Output: "Error occurred: Something went wrong!"
});
If a promise is rejected, the catch()
method will capture the error and handle it. It’s important to always handle errors to prevent uncaught exceptions.
7. Async/Await: Working with Promises in a Synchronous Style
Async/await is a modern way to handle promises in a more readable, synchronous-like manner, although it still works asynchronously under the hood. You can use async
functions to define asynchronous operations and await
to pause execution until the promise is resolved or rejected.
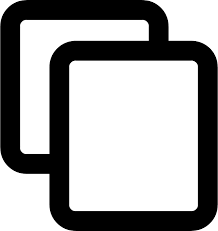
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data: " + error);
}
}
fetchData();
In this example, the await
keyword is used to wait for the promise to resolve, making the asynchronous code look more like synchronous code.
8. Conclusion
Promises provide a cleaner, more manageable way to handle asynchronous operations in JavaScript. By using promises, you can avoid callback hell and improve the readability and maintainability of your code. Promises also enable you to handle multiple asynchronous operations in sequence or parallel more effectively. Additionally, with async/await, handling promises becomes even simpler and more intuitive.
then(), catch(), and finally()
1. Introduction
In JavaScript, when working with promises, the then()
, catch()
, and finally()
methods allow you to handle the result of a promise, handle errors, and perform cleanup actions after the promise has completed, respectively.
2. the then()
Method
The then()
method is used to handle the result of a promise once it is resolved (fulfilled). It takes two arguments:
- onFulfilled: A function that gets called when the promise is resolved.
- onRejected (optional): A function that gets called when the promise is rejected.
In most cases, only the onFulfilled
function is provided, and the onRejected
function is handled via catch()
(explained below).
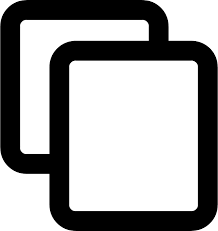
const promise = new Promise((resolve, reject) => {
const isSuccessful = true;
if (isSuccessful) {
resolve("Promise resolved successfully!");
} else {
reject("Promise rejected.");
}
});
promise
.then(result => {
console.log(result); // Output: "Promise resolved successfully!"
})
.catch(error => {
console.error(error);
});
3. the catch()
Method
The catch()
method is used to handle errors that occur during the execution of a promise. It is called when a promise is rejected. You can chain catch()
to handle errors after a then()
method.
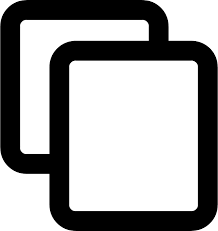
const promise = new Promise((resolve, reject) => {
const isError = true;
if (isError) {
reject("Something went wrong!");
} else {
resolve("All good!");
}
});
promise
.then(result => {
console.log(result); // This will not be executed.
})
.catch(error => {
console.error(error); // Output: "Something went wrong!"
});
4. the finally()
Method
The finally()
method is used to perform an action after the promise has been settled (i.e., either resolved or rejected). It is often used for cleanup tasks, like closing resources, regardless of whether the promise was successful or not.
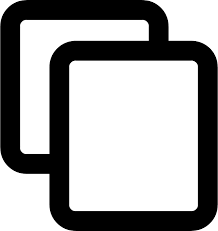
const promise = new Promise((resolve, reject) => {
const isSuccessful = true;
if (isSuccessful) {
resolve("Task completed.");
} else {
reject("Task failed.");
}
});
promise
.then(result => {
console.log(result); // Output: "Task completed."
})
.catch(error => {
console.error(error);
})
.finally(() => {
console.log("Promise has been settled."); // Always runs, regardless of resolve or reject.
});
Notice that the finally()
method always executes, regardless of the outcome of the promise (resolve or reject). It is useful for cleanup tasks that should always happen, regardless of success or failure.
5. Chaining then()
, catch()
, and finally()
All three methods can be chained together to handle multiple stages of a promise. This makes it easier to manage asynchronous operations while keeping the code clean and readable.
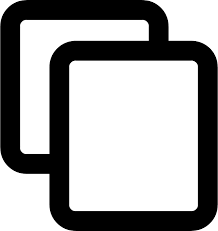
const myPromise = new Promise((resolve, reject) => {
const isSuccess = true;
if (isSuccess) {
resolve("Success!");
} else {
reject("Failure!");
}
});
myPromise
.then(result => {
console.log(result); // Output: "Success!"
return "Next step";
})
.then(nextStep => {
console.log(nextStep); // Output: "Next step"
})
.catch(error => {
console.error(error);
})
.finally(() => {
console.log("Promise settled."); // Output: "Promise settled."
});
In this example, the promise is resolved, and both then()
and finally()
are executed. The error handling with catch()
would only trigger if the promise was rejected.
6. Conclusion
The then()
, catch()
, and finally()
methods provide a powerful way to handle promises in JavaScript. With then()
, you can handle the resolved result of a promise. catch()
is used for handling rejections, and finally()
ensures cleanup actions are performed regardless of the outcome of the promise.
Promise Chaining
1. Introduction
Promise chaining allows you to chain multiple promises together, where each promise is dependent on the result of the previous one. The then()
method of a promise returns a new promise, which allows you to chain multiple then()
calls in sequence. This makes it easier to handle asynchronous operations in a more readable and manageable way.
2. Basic Example of Promise Chaining
In promise chaining, each then()
method returns a new promise. This allows subsequent then()
methods to be executed in the order they are called.
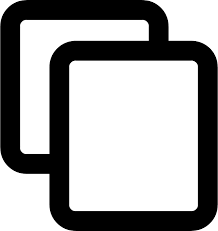
const firstPromise = new Promise((resolve, reject) => {
resolve("First step completed");
});
firstPromise
.then(result => {
console.log(result); // Output: "First step completed"
return "Second step completed";
})
.then(result => {
console.log(result); // Output: "Second step completed"
return "Third step completed";
})
.then(result => {
console.log(result); // Output: "Third step completed"
});
In this example, each then()
method takes the result from the previous one and passes it to the next one in the chain. Each step in the chain is executed in order.
3. Handling Errors in Chaining
In promise chaining, if any promise in the chain is rejected, the subsequent then()
methods will be skipped, and the control will jump directly to the nearest catch()
method. This makes it easier to handle errors globally for the entire chain.
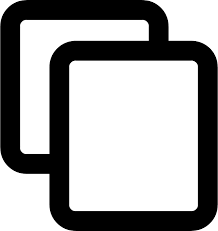
const promiseChain = new Promise((resolve, reject) => {
resolve("Step 1 completed");
});
promiseChain
.then(result => {
console.log(result); // Output: "Step 1 completed"
return "Step 2 completed";
})
.then(result => {
console.log(result); // Output: "Step 2 completed"
throw new Error("Something went wrong!");
})
.then(result => {
console.log(result); // This will not be executed
})
.catch(error => {
console.error(error); // Output: "Error: Something went wrong!"
});
In this example, an error is thrown in the second then()
, and the error is caught by the catch()
method.
4. Returning Promises in Chaining
Each then()
method can return a promise, which allows for asynchronous operations to be included in the chain. This way, you can chain promises that depend on asynchronous operations.
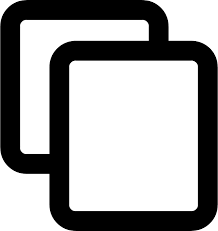
const promiseChain = new Promise((resolve, reject) => {
resolve("Starting promise chain");
});
promiseChain
.then(result => {
console.log(result); // Output: "Starting promise chain"
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Step 2 completed after 2 seconds");
}, 2000);
});
})
.then(result => {
console.log(result); // Output: "Step 2 completed after 2 seconds"
});
In this example, the second then()
returns a promise, causing the chain to wait for that promise to resolve before moving to the next step.
5. Chaining Promises with Multiple then()
Methods
Promise chaining makes it easy to handle multiple asynchronous operations in sequence. You can chain as many then()
methods as needed, and each then()
can return a new value or another promise.
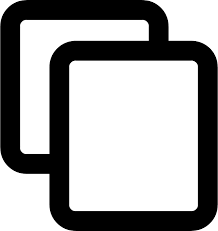
const promiseChain = new Promise((resolve, reject) => {
resolve("Initial step completed");
});
promiseChain
.then(result => {
console.log(result); // Output: "Initial step completed"
return "Step 2 completed";
})
.then(result => {
console.log(result); // Output: "Step 2 completed"
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Step 3 completed after 1 second");
}, 1000);
});
})
.then(result => {
console.log(result); // Output: "Step 3 completed after 1 second"
})
.catch(error => {
console.error(error);
});
6. Conclusion
Promise chaining is a powerful way to manage multiple asynchronous operations in a clean and efficient manner. By chaining promises together, you can ensure that each step in the process is executed in order. You can also easily handle errors with a single catch()
at the end of the chain.
Introduction to Async/Await
1. What is Async/Await?
Async/Await is a modern JavaScript feature that makes it easier to work with asynchronous code. It allows you to write asynchronous code that looks and behaves like synchronous code, improving readability and making code easier to maintain. async
and await
are built on top of promises and help you avoid deeply nested callbacks or promise chains.
2. The async
Keyword
The async
keyword is used to declare a function as asynchronous. When a function is declared with async
, it will always return a promise, even if you return a non-promise value. This allows you to use await
inside the function to pause execution until the promise resolves.
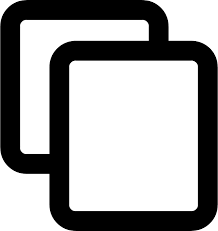
async function fetchData() {
return "Data fetched!";
}
fetchData().then(result => {
console.log(result); // Output: "Data fetched!"
});
In this example, fetchData
is an asynchronous function, and it returns a promise, which resolves with the string "Data fetched!".
3. The await
Keyword
The await
keyword can only be used inside an async
function. It pauses the execution of the function and waits for the promise to resolve. Once the promise resolves, the value is returned and execution continues from where it left off.
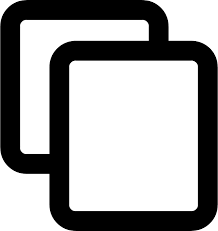
async function fetchData() {
let result = await new Promise(resolve => setTimeout(() => resolve("Data fetched after 2 seconds"), 2000));
console.log(result); // Output: "Data fetched after 2 seconds"
}
fetchData();
In this example, the function fetchData
is paused for 2 seconds before resolving the promise and logging the result. The use of await
ensures that the promise is resolved before continuing the function execution.
4. Handling Errors with Async/Await
Just like with promises, you can handle errors in async functions using try-catch
blocks. If any of the promises inside an async
function are rejected, the error can be caught and handled using catch
.
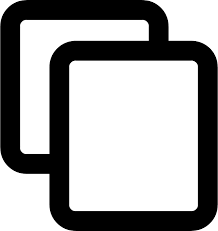
async function fetchData() {
try {
let result = await new Promise((resolve, reject) => setTimeout(() => reject("Error fetching data"), 2000));
console.log(result);
} catch (error) {
console.error(error); // Output: "Error fetching data"
}
}
fetchData();
In this example, the promise is rejected, and the error is caught by the catch
block, which logs the error message.
5. Async/Await with Multiple Promises
You can use await
with multiple promises as well. If you need to wait for multiple promises to resolve, you can use Promise.all()
or async
functions inside loops.
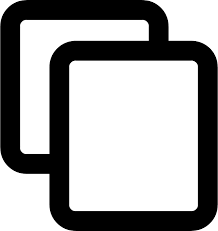
async function fetchData() {
let result1 = await new Promise(resolve => setTimeout(() => resolve("First data fetched"), 1000));
let result2 = await new Promise(resolve => setTimeout(() => resolve("Second data fetched"), 2000));
console.log(result1); // Output: "First data fetched"
console.log(result2); // Output: "Second data fetched"
}
fetchData();
In this example, the promises are awaited one by one, meaning they will resolve sequentially, and the second promise will wait for the first one to complete before executing.
6. Parallel Execution of Async Functions
If you want to run multiple async functions concurrently (in parallel) rather than sequentially, you can use Promise.all()
. This method accepts an array of promises and returns a single promise that resolves when all the promises are resolved.
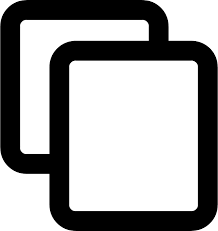
async function fetchData() {
let promise1 = new Promise(resolve => setTimeout(() => resolve("First data fetched"), 1000));
let promise2 = new Promise(resolve => setTimeout(() => resolve("Second data fetched"), 2000));
let [result1, result2] = await Promise.all([promise1, promise2]);
console.log(result1); // Output: "First data fetched"
console.log(result2); // Output: "Second data fetched"
}
fetchData();
In this example, both promises run concurrently, and Promise.all()
ensures that execution continues only after both promises have been resolved.
7. Conclusion
Async/Await provides a more readable and elegant way to handle asynchronous operations compared to traditional promise chains. It makes asynchronous code look more like synchronous code, improving clarity and reducing complexity. By using async
and await
, along with proper error handling using try-catch
, you can write cleaner and more maintainable asynchronous code.
Error Handling in Async Functions
1. Introduction to Error Handling in Async Functions
In async functions, errors are handled in a similar way to regular JavaScript promises. Using try-catch
blocks allows you to catch errors that occur during the execution of asynchronous code. Async functions return promises, so if a promise is rejected inside an async
function, it can be caught using catch
or inside a try-catch
block.
2. Using try-catch
for Error Handling
You can use a try-catch
block inside an async function to handle errors that occur during the asynchronous operation. If an error is thrown, the code inside the catch
block will be executed.
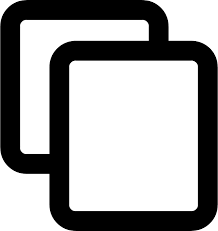
async function fetchData() {
try {
let result = await new Promise((resolve, reject) => {
setTimeout(() => reject("Error fetching data"), 2000);
});
console.log(result);
} catch (error) {
console.error("Caught an error:", error); // Output: Caught an error: Error fetching data
}
}
fetchData();
In this example, the promise is rejected, and the error is caught by the catch
block, which logs the error message.
3. Handling Specific Errors
You can also handle specific errors by checking the error message or type inside the catch
block. This can be useful when you want to handle different types of errors differently.
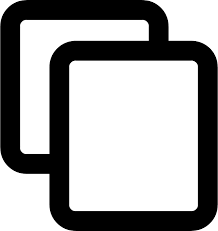
async function fetchData() {
try {
let result = await new Promise((resolve, reject) => {
setTimeout(() => reject("Network Error"), 2000);
});
console.log(result);
} catch (error) {
if (error === "Network Error") {
console.error("There was a network issue.");
} else {
console.error("An unknown error occurred:", error);
}
}
}
fetchData();
In this example, the error message is checked to determine if it's a "Network Error" and provides specific handling for that type of error.
4. Handling Multiple Errors
In cases where multiple asynchronous operations are happening, you can use multiple try-catch
blocks to handle errors in each operation independently.
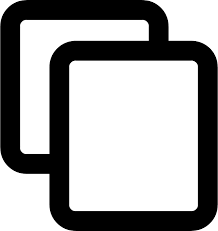
async function fetchData() {
try {
let result1 = await new Promise((resolve, reject) => {
setTimeout(() => resolve("First data fetched"), 1000);
});
console.log(result1); // Output: First data fetched
} catch (error) {
console.error("Error in first promise:", error);
}
try {
let result2 = await new Promise((resolve, reject) => {
setTimeout(() => reject("Error in second promise"), 2000);
});
console.log(result2);
} catch (error) {
console.error("Error in second promise:", error); // Output: Error in second promise: Error in second promise
}
}
fetchData();
Here, two separate promises are handled independently, and each has its own catch
block for error handling.
5. Rejection Handling Outside Async Functions
If you want to handle errors that occur outside of an async function, you can use the catch
method attached to the promise returned by the async function.
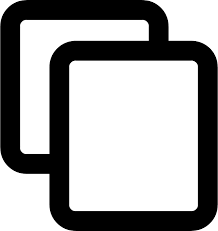
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => reject("Something went wrong!"), 2000);
});
}
fetchData().catch((error) => {
console.error("Caught outside the async function:", error); // Output: Caught outside the async function: Something went wrong!
});
In this example, the error is handled outside of the async
function by using the catch
method on the returned promise.
6. Handling Errors in Parallel Async Operations
When dealing with multiple promises running in parallel, you can use Promise.all()
for handling errors. If any of the promises are rejected, Promise.all()
will immediately reject with the first error encountered.
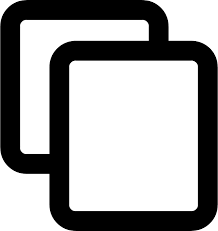
async function fetchData() {
let promise1 = new Promise((resolve, reject) => setTimeout(() => resolve("First data fetched"), 1000));
let promise2 = new Promise((resolve, reject) => setTimeout(() => reject("Error in second promise"), 2000));
try {
let results = await Promise.all([promise1, promise2]);
console.log(results);
} catch (error) {
console.error("Error in parallel promises:", error); // Output: Error in parallel promises: Error in second promise
}
}
fetchData();
In this example, since the second promise is rejected, the catch
block handles the rejection and logs the error.
7. Conclusion
Handling errors in async functions is easy with try-catch
blocks, and it works similarly to how you handle errors with promises. You can catch specific errors, manage multiple asynchronous operations independently, and even handle rejections outside async functions. Asynchronous code can now be written in a more structured, clear, and manageable way using async/await and proper error handling techniques.
Fetching Data from an API
1. Introduction to Fetching Data
Fetching data from an API (Application Programming Interface) is a common task in JavaScript. APIs allow your application to retrieve data from external servers or services. The fetch()
API is commonly used in modern JavaScript to make HTTP requests and handle responses. The fetch()
method returns a promise, which can be resolved to handle the response data.
2. Using fetch()
to Retrieve Data
The fetch()
function is used to make a request to a specified URL. It returns a promise that resolves to the Response
object. You can then parse the response as JSON or another format.
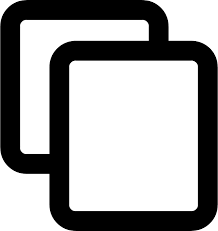
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json()) // Parse the JSON response
.then(data => console.log(data)) // Handle the data
.catch(error => console.error('Error fetching data:', error)); // Handle errors
In this example, the fetch request retrieves a list of posts from a public API, and the response is parsed as JSON.
3. Using async/await
with Fetch
You can also use async/await
syntax to simplify the code and handle the promise returned by the fetch()
function more elegantly.
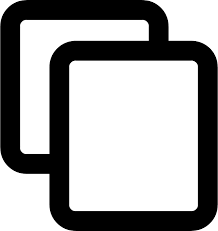
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
let data = await response.json(); // Parse the JSON response
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
In this example, we use await
to wait for the fetch request to complete, and then parse the response as JSON. The try-catch
block handles any errors that may occur during the fetch operation.
4. Handling Response Status
When fetching data, it's important to check the response status to ensure the request was successful. A common practice is to check if the response status is in the range of 200-299, which indicates a successful request.
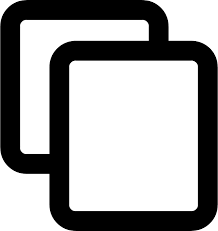
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Error: ' + response.status);
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Fetch error:', error);
}
}
fetchData();
In this example, we check the response.ok
property, which is true
if the HTTP status is in the 200 range. If it's not, we throw an error with the response status code.
5. Handling Different Content Types
APIs may return data in different formats, such as JSON, text, or HTML. You can use the response.json()
, response.text()
, or response.blob()
methods to handle different types of responses.
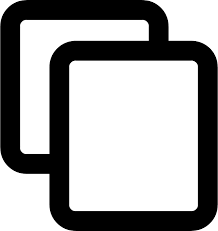
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
let data = await response.json(); // Parse JSON
console.log(data);
let textResponse = await fetch('https://example.com/text-file');
let textData = await textResponse.text(); // Parse text
console.log(textData);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
In this example, we handle both JSON and text responses from different requests.
6. Sending Data with Fetch (POST Requests)
You can also send data to an API using HTTP POST requests. You need to specify the method as 'POST'
and include a body with the data you want to send. The body is often serialized as JSON.
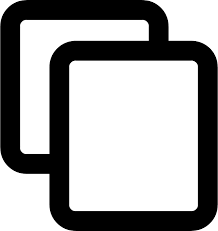
async function sendData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'New Post',
body: 'This is the content of the new post.',
userId: 1,
}),
});
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error sending data:', error);
}
}
sendData();
Here, we use a POST request to send JSON data to the API. The Content-Type
header is set to application/json
, and we stringify the body before sending it.
7. Handling Multiple API Calls
Sometimes you may need to make multiple API calls. You can use Promise.all()
to handle multiple fetch requests concurrently and wait for all of them to resolve.
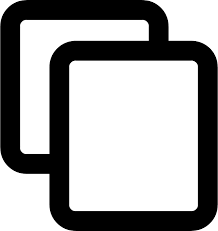
async function fetchMultipleData() {
try {
let [posts, users] = await Promise.all([
fetch('https://jsonplaceholder.typicode.com/posts').then(response => response.json()),
fetch('https://jsonplaceholder.typicode.com/users').then(response => response.json())
]);
console.log('Posts:', posts);
console.log('Users:', users);
} catch (error) {
console.error('Error:', error);
}
}
fetchMultipleData();
In this example, we use Promise.all()
to fetch both posts and users data concurrently. Once both promises resolve, we log the results.
8. Conclusion
Fetching data from an API is an essential skill in modern web development. With fetch()
and async/await
, you can easily retrieve and send data to APIs. Be sure to handle errors, check response status, and consider how to handle different types of responses. By using these techniques, you can build powerful applications that interact with external services.
Handling JSON Responses
1. Introduction to JSON
JSON (JavaScript Object Notation) is a lightweight data format used to store and exchange data between a server and a client. It's easy to read and write for humans, and easy to parse and generate for machines. In JavaScript, JSON is commonly used to represent data that comes from APIs.
2. Fetching and Parsing JSON Data
When you fetch data from an API, the response is usually in the form of a JSON string. To work with it in JavaScript, you need to parse the JSON string into a JavaScript object. The fetch()
API provides a method called response.json()
to parse the response as JSON.
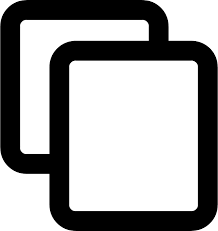
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json()) // Parse the response as JSON
.then(data => console.log(data)) // Handle the parsed data
.catch(error => console.error('Error:', error)); // Handle errors
In this example, we use response.json()
to parse the JSON response, and the resulting data is a JavaScript object that can be manipulated.
3. Using Async/Await with JSON Parsing
You can also use async/await
syntax for handling JSON responses, which makes the code cleaner and easier to read.
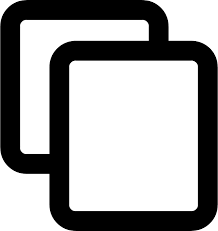
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
let data = await response.json(); // Parse JSON response
console.log(data);
} catch (error) {
console.error('Error:', error); // Handle errors
}
}
fetchData();
Here, we use await
to wait for the fetch request and the parsing of the response. The result is logged to the console.
4. Handling JSON with Errors
When parsing JSON, there is a possibility of errors if the response is not valid JSON. To handle such cases, you can use try-catch
blocks or check if the response is valid before parsing it.
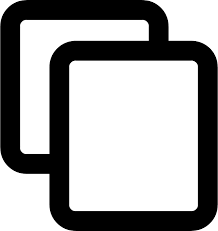
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error); // Handle errors
}
}
fetchData();
Here, we check the response.ok
property before calling response.json()
to ensure the response is successful. If the response is not ok, we throw an error.
5. Handling Large JSON Responses
For large JSON responses, it's important to parse the data efficiently. You can use methods like response.text()
to get the raw response and then try to parse it manually to handle the data in chunks if necessary. This can help prevent issues with large datasets.
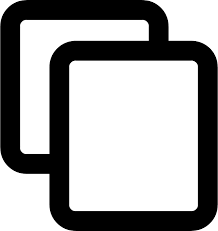
async function fetchLargeData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
let text = await response.text(); // Get response as text
let data = JSON.parse(text); // Manually parse JSON
console.log(data);
} catch (error) {
console.error('Error:', error); // Handle errors
}
}
fetchLargeData();
In this example, we use response.text()
to fetch the raw response as text, and then manually parse it using JSON.parse()
.
6. Handling Nested JSON Data
JSON responses can be nested, meaning the data returned can contain other objects or arrays. You can access nested data by using dot notation or bracket notation.
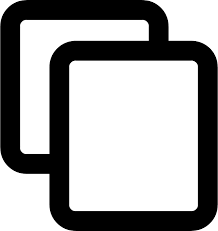
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/users');
let data = await response.json();
console.log(data[0].name); // Accessing a nested value (name of the first user)
} catch (error) {
console.error('Error:', error); // Handle errors
}
}
fetchData();
Here, we access the name of the first user in the array of users returned by the API. You can use similar syntax to access any nested values.
7. Working with JSON Arrays
JSON responses often include arrays of data. After parsing the JSON response, you can use array methods like map()
, filter()
, and forEach()
to work with the data.
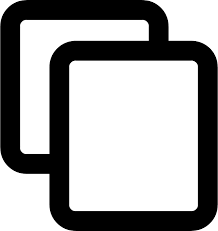
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
let posts = await response.json();
posts.forEach(post => console.log(post.title)); // Logging the title of each post
} catch (error) {
console.error('Error:', error); // Handle errors
}
}
fetchData();
In this example, we use forEach()
to iterate over the array of posts and log the title of each post.
8. Conclusion
Handling JSON responses is a crucial part of working with APIs in JavaScript. By understanding how to fetch, parse, and manipulate JSON data, you can build powerful applications that interact with external data sources. Always be mindful of error handling, especially when dealing with large or nested data.
Error Handling in Fetch
1. Introduction to Fetch Error Handling
When using the fetch()
API to make HTTP requests, it's important to handle errors properly. The fetch()
API itself only rejects promises on network failures or if there's an issue with the request. It doesn't reject on HTTP errors like 404 or 500. Therefore, you need to manually check the response status and handle errors accordingly.
2. Basic Error Handling with Fetch
The basic error handling in fetch()
involves using the catch()
method to catch network errors and checking the response status to handle HTTP errors.
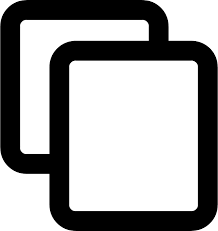
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON if the response is ok
})
.then(data => console.log(data)) // Handle the data
.catch(error => console.error('There was a problem with the fetch operation:', error)); // Handle errors
In the above example, we check if the response.ok
property is true. If it's false (e.g., for 404 or 500 errors), we throw an error, which will be caught by the catch()
method.
3. Handling HTTP Status Codes
It's often useful to handle specific HTTP status codes separately, such as 404 for "Not Found" or 500 for "Internal Server Error." You can use an if
or switch
statement to check for these codes and provide custom error messages.
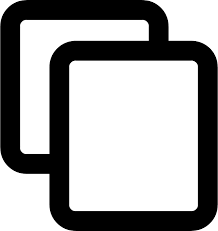
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
if (response.status === 404) {
throw new Error('Page not found (404)');
} else if (response.status === 500) {
throw new Error('Server error (500)');
} else if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Here, we check for specific status codes and throw different error messages based on the status code of the response.
4. Handling Non-200 HTTP Status Codes
In some cases, you may want to handle all non-200 status codes uniformly. This approach can be useful for API calls where only a specific range of status codes (such as 2xx) represents success.
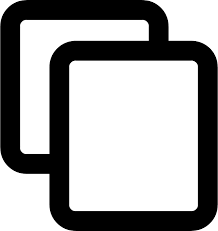
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
if (response.status >= 400) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Here, we check if the status code is 400 or higher (indicating an error) and throw an error with the status code.
5. Async/Await with Error Handling
When using async/await
syntax with fetch()
, you can handle errors by using a try-catch
block. This provides a more readable and synchronous-like way to handle asynchronous operations.
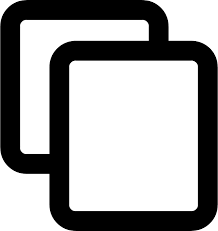
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error('There was a problem with the fetch operation:', error);
}
}
fetchData();
In this example, we use await
to wait for the fetch request and response.json()
. If there's an error, it will be caught by the catch()
block.
6. Handling Fetch Timeouts
Fetch doesn't provide a built-in timeout feature, but you can implement one using setTimeout()
to reject the fetch promise after a certain amount of time.
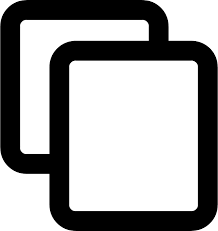
function fetchWithTimeout(url, options, timeout = 5000) {
return new Promise((resolve, reject) => {
const timer = setTimeout(() => reject(new Error('Request timeout')), timeout);
fetch(url, options)
.then(resolve)
.catch(reject)
.finally(() => clearTimeout(timer)); // Clear the timeout if the fetch is successful
});
}
fetchWithTimeout('https://jsonplaceholder.typicode.com/posts', { method: 'GET' })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In this example, we define a fetchWithTimeout()
function that rejects the fetch request if it takes longer than the specified timeout (default is 5 seconds). The setTimeout()
function creates a timer, and clearTimeout()
stops it if the fetch completes successfully.
7. Conclusion
Error handling is a critical part of making HTTP requests in JavaScript using the fetch()
API. Always check the response status and handle both network errors and HTTP status errors. Using async/await
with try-catch
offers a cleaner and more readable approach to error handling. Additionally, consider implementing timeouts for requests that may hang indefinitely.
let and const
1. Introduction to let and const
In JavaScript, let
and const
are two ways to declare variables that were introduced in ECMAScript 6 (ES6). Both are block-scoped, meaning they are only accessible within the block or statement where they are defined, unlike var
, which is function-scoped.
2. The let
Keyword
The let
keyword is used to declare variables that can be reassigned. It has block scope, which means the variable is only available within the block it is defined in (such as inside a loop or a conditional statement).
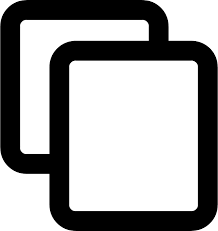
let x = 10;
x = 20; // Reassignable variable
In the example above, the variable x
is declared with let
and its value can be reassigned from 10 to 20.
3. The const
Keyword
The const
keyword is used to declare constants, meaning the value of the variable cannot be reassigned once it is set. However, this does not mean the value is immutable. If the constant holds an object or an array, the contents of the object or array can still be modified.
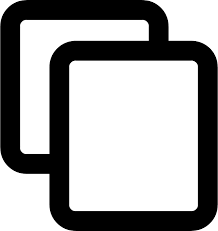
const y = 30;
// y = 40; // Error: Assignment to constant variable
const obj = { key: 'value' };
obj.key = 'new value'; // Allowed: modifying the contents of the object
In the above example, trying to reassign y
will result in an error. But modifying the properties of the object obj
is allowed.
4. Block Scope of let
and const
Both let
and const
have block scope, meaning they are only available within the block where they are declared. This is different from var
, which has function scope.
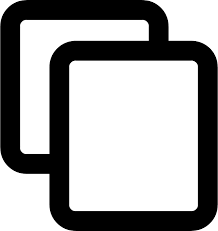
if (true) {
let a = 5;
const b = 10;
console.log(a, b); // 5 10
}
console.log(a); // Error: a is not defined
console.log(b); // Error: b is not defined
Here, a
and b
are only accessible within the if
block. Trying to access them outside the block will result in an error.
5. Temporal Dead Zone (TDZ)
Both let
and const
are affected by the "Temporal Dead Zone" (TDZ). The TDZ refers to the time between entering a scope and when the variable is declared and initialized. During this period, trying to access the variable will throw a ReferenceError.
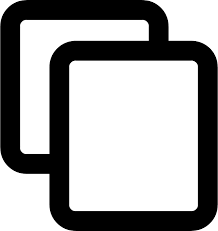
console.log(a); // Error: Cannot access 'a' before initialization
let a = 10;
In this example, the variable a
is in the TDZ until it is initialized. Trying to access it before the declaration results in an error.
6. Differences Between let
, const
, and var
While let
and const
are block-scoped, var
is function-scoped. Furthermore, var
allows reassigning a variable, even after it has been declared, while const
prevents reassignment.
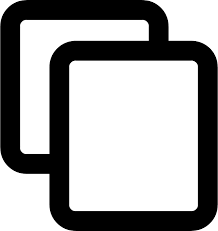
var x = 10;
x = 20; // Reassignable
let y = 30;
y = 40; // Reassignable
const z = 50;
// z = 60; // Error: Assignment to constant variable
7. Best Practices
- Use
const
by default for variables that will not be reassigned. - Use
let
only when you expect the variable's value to change. - Avoid using
var
unless you need function-scoped variables, aslet
andconst
are more predictable and less error-prone.
8. Conclusion
Both let
and const
offer better scoping rules than var
and help prevent common bugs related to variable hoisting and reassignment. It's recommended to use const
as the default for variables that should not change, and let
for variables that need to be reassigned.
Arrow Functions
1. Introduction to Arrow Functions
Arrow functions are a more concise way of writing functions in JavaScript, introduced in ECMAScript 6 (ES6). They provide a shorter syntax for writing function expressions and also have different behavior for the this
keyword compared to regular functions.
2. Syntax of Arrow Functions
Arrow functions have a simpler syntax compared to traditional function expressions. They do not require the function
keyword and are written using an arrow (=>
) between the parameters and the function body.
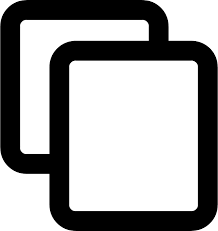
const add = (a, b) => a + b;
console.log(add(2, 3)); // Output: 5
In this example, the arrow function add
takes two parameters a
and b
, and returns their sum. The return keyword is implied for single-expression functions.
3. Single Parameter Arrow Functions
If the arrow function has only one parameter, you can omit the parentheses around the parameter.
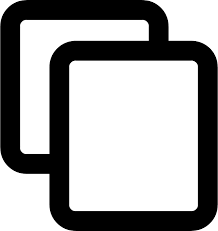
const square = x => x * x;
console.log(square(4)); // Output: 16
Here, the arrow function square
takes a single parameter x
and returns its square. The parentheses are not necessary when there is only one parameter.
4. No Parameters Arrow Functions
If an arrow function does not take any parameters, you still need to include empty parentheses.
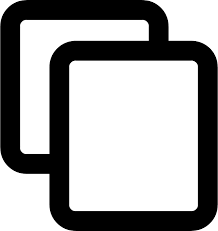
const sayHello = () => console.log('Hello!');
sayHello(); // Output: Hello!
In the above example, the arrow function sayHello
does not take any parameters but logs a message to the console.
5. Implicit Return
Arrow functions with a single expression automatically return the result of that expression. The return
keyword is not needed.
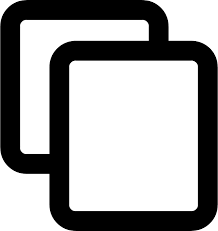
const multiply = (a, b) => a * b;
console.log(multiply(3, 4)); // Output: 12
In this example, the result of a * b
is returned automatically, without using the return
keyword.
6. Multi-line Arrow Functions
If the arrow function has more than one statement, you need to use curly braces and an explicit return
statement.
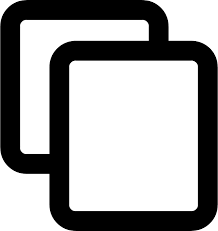
const addAndLog = (a, b) => {
const sum = a + b;
console.log(sum);
return sum;
};
addAndLog(2, 3); // Output: 5
Here, the arrow function addAndLog
has multiple statements, so we use curly braces to define the function body, and explicitly use return
to return the value.
7. Arrow Functions and this
One of the key differences between arrow functions and regular functions is how they handle the this
keyword. In regular functions, this
refers to the object that is calling the function. In arrow functions, this
refers to the surrounding context (the lexical scope) in which the function is defined, not where it is called.
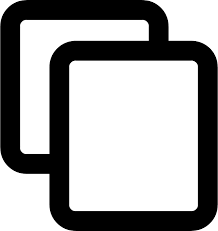
function Person(name) {
this.name = name;
setTimeout(function() {
console.log(this.name); // Undefined, because 'this' refers to the global context
}, 1000);
}
const person1 = new Person('Alice');
function PersonArrow(name) {
this.name = name;
setTimeout(() => {
console.log(this.name); // 'Alice', because 'this' refers to the PersonArrow context
}, 1000);
}
const person2 = new PersonArrow('Bob');
In the first example, the regular function inside the setTimeout
does not have access to the this
of the Person
object, resulting in undefined
this.name
. In the second example, the arrow function correctly captures the this
of the PersonArrow
constructor, and outputs the correct value.
8. When to Use Arrow Functions
- Use arrow functions for short function expressions, especially when you want a concise syntax.
- Use arrow functions when you need to preserve the lexical
this
context from the surrounding code (e.g., in event handlers or callbacks). - Avoid using arrow functions as methods inside objects, as they will not have their own
this
context.
9. Conclusion
Arrow functions provide a concise and more readable syntax for functions in JavaScript. They are especially useful for inline functions, callbacks, and handling this
in specific contexts. However, they are not suitable for every use case, particularly as methods within objects.
Template Literals
1. Introduction to Template Literals
Template literals are a feature introduced in ECMAScript 6 (ES6) that provide a more powerful way to work with strings in JavaScript. They allow for multi-line strings, string interpolation, and embedding expressions inside strings.
2. Syntax of Template Literals
Template literals are enclosed in backticks (\`
), rather than single or double quotes. This allows for more flexibility in creating strings.
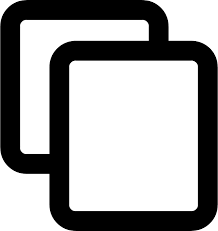
const greeting = `Hello, World!`;
console.log(greeting); // Output: Hello, World!
In this example, the string is enclosed in backticks instead of quotation marks.
3. String Interpolation
One of the key features of template literals is string interpolation. This allows you to embed variables or expressions directly into a string using the ${}
syntax.
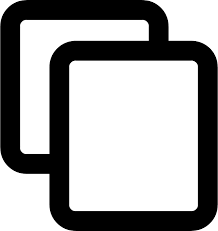
const name = 'Alice';
const age = 25;
const message = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(message); // Output: Hello, my name is Alice and I am 25 years old.
Here, the values of the name
and age
variables are inserted into the string using ${}
.
4. Multi-line Strings
Template literals can span multiple lines without the need for escape characters. This makes working with long strings or text easier and more readable.
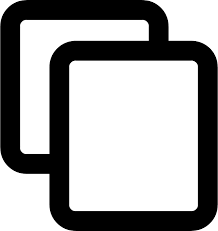
const message = `This is a string
that spans multiple
lines.`;
console.log(message); // Output: This is a string
// that spans multiple
// lines.
In this example, the string spans multiple lines, and the line breaks are preserved in the output.
5. Expression Embedding
You can embed JavaScript expressions inside template literals. This allows you to evaluate expressions and embed their results directly into the string.
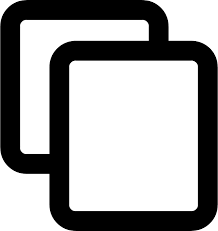
const a = 5;
const b = 10;
const result = `The sum of ${a} and ${b} is ${a + b}.`;
console.log(result); // Output: The sum of 5 and 10 is 15.
In this case, the expression a + b
is evaluated and its result is embedded in the string.
6. Nesting Template Literals
You can also nest template literals inside other template literals. This can be useful for creating complex strings or formatting.
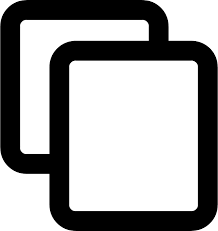
const name = 'Bob';
const greeting = `Hello, ${name}!
You have ${`3 new messages`} in your inbox.`;
console.log(greeting); // Output: Hello, Bob!
// You have 3 new messages in your inbox.
In this example, a nested template literal is used inside the main template literal.
7. Tagged Template Literals
Tagged template literals allow you to parse template literals with a function. The function can manipulate the string and expressions before returning the final result.
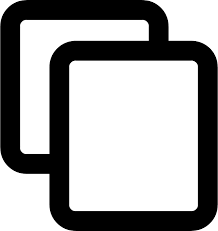
function tag(strings, ...values) {
let result = strings[0];
values.forEach((value, index) => {
result += value.toUpperCase() + strings[index + 1];
});
return result;
}
const name = 'Alice';
const message = tag`Hello, ${name}!`;
console.log(message); // Output: Hello, ALICE!
In this example, the tag
function processes the string and expression, transforming the value of name
to uppercase.
8. Use Cases for Template Literals
- Creating readable multi-line strings.
- Embedding variables and expressions inside strings without needing concatenation.
- Formatting strings dynamically based on conditions or calculations.
- Working with HTML templates in JavaScript (e.g., in front-end development).
9. Advantages of Template Literals
- Cleaner and more readable code, especially with multi-line strings.
- Easy and efficient string interpolation.
- Ability to embed expressions and manipulate string values dynamically.
10. Conclusion
Template literals are a powerful feature in JavaScript that make working with strings more flexible and concise. They allow for string interpolation, multi-line strings, and embedding expressions, making them a valuable tool for developers.
Destructuring Assignment
1. Introduction to Destructuring
Destructuring is a feature in JavaScript that allows you to unpack values from arrays or properties from objects into distinct variables. It provides a more concise and readable way of extracting data from complex structures.
2. Array Destructuring
Array destructuring allows you to assign elements from an array to variables in a more readable way.
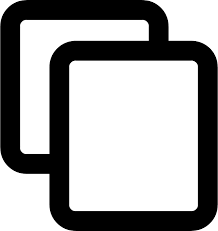
const arr = [1, 2, 3];
const [a, b, c] = arr;
console.log(a); // Output: 1
console.log(b); // Output: 2
console.log(c); // Output: 3
In this example, the elements of the array arr
are destructured into variables a
, b
, and c
.
3. Skipping Values in Arrays
You can skip over elements in an array during destructuring by leaving gaps in the assignment.
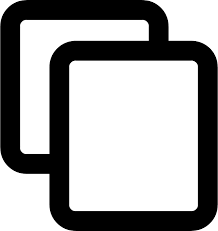
const arr = [1, 2, 3];
const [a, , c] = arr;
console.log(a); // Output: 1
console.log(c); // Output: 3
Here, we skip the second element by leaving an empty space between the commas.
4. Default Values in Array Destructuring
With destructuring, you can also set default values for variables in case the values from the array are undefined
.
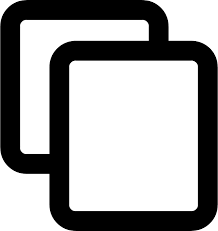
const arr = [1];
const [a, b = 2] = arr;
console.log(a); // Output: 1
console.log(b); // Output: 2
If a value is missing in the array, the default value is used instead.
5. Object Destructuring
Object destructuring allows you to extract values from an object and assign them to variables.
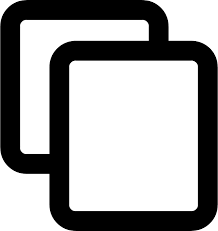
const person = { name: 'Alice', age: 25 };
const { name, age } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 25
In this example, the name
and age
properties of the person
object are destructured into variables.
6. Renaming Variables in Object Destructuring
If the variable name differs from the property name in the object, you can rename the variable during destructuring.
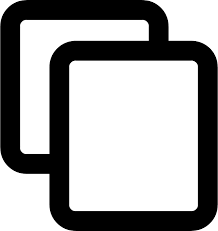
const person = { name: 'Alice', age: 25 };
const { name: fullName, age: yearsOld } = person;
console.log(fullName); // Output: Alice
console.log(yearsOld); // Output: 25
Here, the name
property is renamed to fullName
and the age
property is renamed to yearsOld
.
7. Nested Destructuring
Destructuring can also be used with nested objects or arrays, allowing you to extract values from deeper structures.
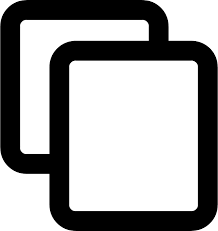
const person = { name: 'Alice', address: { city: 'New York', zip: '10001' } };
const { name, address: { city, zip } } = person;
console.log(name); // Output: Alice
console.log(city); // Output: New York
console.log(zip); // Output: 10001
In this example, we destructure the address
object within the person
object.
8. Destructuring with Function Parameters
You can also use destructuring in function parameters to directly unpack values from objects or arrays passed to the function.
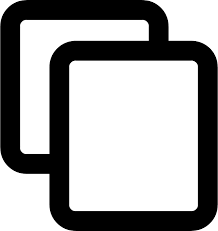
function greet({ name, age }) {
console.log(`Hello, my name is ${name} and I am ${age} years old.`);
}
const person = { name: 'Alice', age: 25 };
greet(person); // Output: Hello, my name is Alice and I am 25 years old.
Here, the function greet
accepts an object and destructures the name
and age
properties directly in the parameters.
9. Rest Syntax with Destructuring
With the rest syntax (...
), you can collect the remaining elements of an array or object into a new array or object.
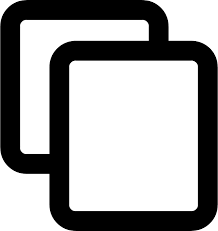
const arr = [1, 2, 3, 4];
const [first, ...rest] = arr;
console.log(first); // Output: 1
console.log(rest); // Output: [2, 3, 4]
In this example, the first element is assigned to first
, and the remaining elements are collected into the rest
array.
10. Advantages of Destructuring
- Cleaner and more readable code, especially when dealing with complex data structures.
- Allows for easy extraction of specific values from arrays and objects.
- Helps avoid repetitive code and makes it easier to work with functions that return multiple values.
- Can improve the maintainability of your code when dealing with nested data.
11. Conclusion
Destructuring is a powerful feature in JavaScript that simplifies working with arrays and objects. It allows for more concise, readable, and maintainable code by making it easier to unpack and assign values to variables.
Rest and Spread Operators
1. Introduction to Rest and Spread Operators
The rest and spread operators are two powerful features in JavaScript that allow for more flexible handling of arrays and objects. Both use the same syntax (...
), but they serve different purposes depending on the context.
2. The Rest Operator
The rest operator (...
) is used to collect multiple elements into a single variable. It is most commonly used in function parameters and array destructuring.
3. Rest in Function Parameters
The rest operator can be used in function parameters to collect all remaining arguments into a single array. It allows a function to accept an indefinite number of arguments.
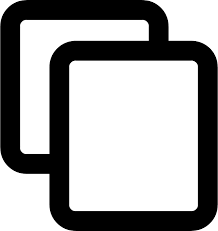
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4)); // Output: 10
In this example, the numbers
parameter collects all arguments passed to the sum
function into an array.
4. Rest in Array Destructuring
The rest operator can also be used in array destructuring to collect all the remaining elements into a new array.
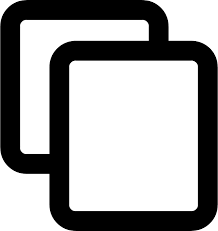
const arr = [1, 2, 3, 4, 5];
const [first, second, ...rest] = arr;
console.log(first); // Output: 1
console.log(second); // Output: 2
console.log(rest); // Output: [3, 4, 5]
In this example, first
and second
are assigned the first two elements of the array, and the rest of the elements are collected into the rest
array.
5. The Spread Operator
The spread operator (...
) is used to unpack elements from an array or object. It is often used to merge arrays or objects or to pass elements as individual arguments to a function.
6. Spread in Arrays
The spread operator can be used to unpack elements from an array, allowing you to easily combine arrays or create copies of arrays.
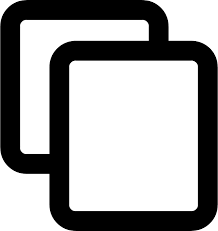
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, ...arr2];
console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
In this example, the elements of arr1
and arr2
are unpacked and combined into a new array combined
.
7. Spread in Objects
The spread operator can also be used to copy properties from one object into another, or to merge multiple objects.
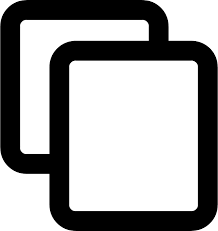
const obj1 = { name: 'Alice', age: 25 };
const obj2 = { city: 'New York', country: 'USA' };
const mergedObj = { ...obj1, ...obj2 };
console.log(mergedObj);
// Output: { name: 'Alice', age: 25, city: 'New York', country: 'USA' }
Here, we use the spread operator to merge the properties of obj1
and obj2
into a new object mergedObj
.
8. Spread in Function Arguments
The spread operator can be used to pass elements of an array as separate arguments to a function.
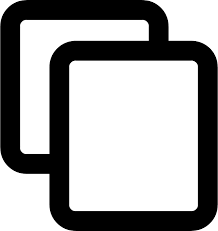
function greet(name, age) {
console.log(`Hello, my name is ${name} and I am ${age} years old.`);
}
const person = ['Alice', 25];
greet(...person); // Output: Hello, my name is Alice and I am 25 years old.
In this example, the spread operator unpacks the elements of the person
array and passes them as individual arguments to the greet
function.
9. Differences Between Rest and Spread
- The rest operator (
...
) is used to collect multiple elements into a single variable, while the spread operator (...
) is used to unpack elements from an array or object. - The rest operator is primarily used in function parameters and array/object destructuring, while the spread operator is used for copying/merging arrays and objects, and passing arguments to functions.
10. Advantages of Using Rest and Spread
- More concise and readable code for working with arrays and objects.
- Helps avoid repetitive code when merging or copying arrays/objects.
- Provides flexibility in function parameters, allowing functions to accept an indefinite number of arguments.
11. Conclusion
Both the rest and spread operators are essential tools in modern JavaScript. They make it easier to work with collections of data, creating cleaner, more readable, and maintainable code.
Classes and Inheritance
1. Introduction to Classes
In JavaScript, classes are a blueprint for creating objects. Classes allow for the creation of objects with shared properties and methods. They were introduced in ECMAScript 6 (ES6) as a more structured way to work with objects and provide a cleaner syntax for object-oriented programming (OOP).
2. Defining a Class
To define a class, use the class
keyword followed by the class name. Inside the class, you can define a constructor and methods.
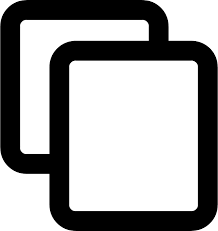
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
const person1 = new Person('Alice', 25);
person1.greet(); // Output: Hello, my name is Alice and I am 25 years old.
In this example, the Person
class has a constructor that initializes the name
and age
properties. The greet
method is defined to print a greeting message.
3. Constructor Method
The constructor
method is a special method for creating and initializing objects created within a class. It is called automatically when a new instance of the class is created.
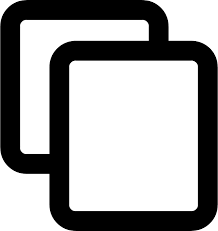
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
}
const car1 = new Car('Toyota', 'Corolla');
console.log(car1.make); // Output: Toyota
4. Methods in Classes
Methods in classes are similar to regular functions, but they belong to the class's prototype. They are invoked using an instance of the class.
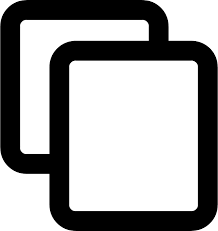
class Calculator {
add(a, b) {
return a + b;
}
subtract(a, b) {
return a - b;
}
}
const calc = new Calculator();
console.log(calc.add(5, 3)); // Output: 8
5. Inheritance in JavaScript
Inheritance is a core concept in object-oriented programming (OOP) that allows a class to inherit properties and methods from another class. In JavaScript, inheritance is achieved using the extends
keyword.
6. Extending a Class
You can create a subclass that inherits from a parent class using the extends
keyword. The subclass can then access and modify the methods and properties of the parent class.
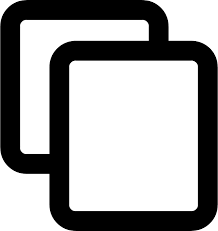
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Buddy');
dog.speak(); // Output: Buddy barks.
In this example, the Dog
class extends the Animal
class. The Dog
class overrides the speak
method to provide its own implementation.
7. Calling Superclass Methods
The super
keyword allows you to call methods or the constructor of a superclass from a subclass.
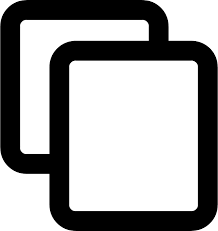
class Dog extends Animal {
constructor(name, breed) {
super(name); // Calls the constructor of the parent class (Animal)
this.breed = breed;
}
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Buddy', 'Golden Retriever');
console.log(dog.breed); // Output: Golden Retriever
dog.speak(); // Output: Buddy barks.
In this example, the super(name)
call invokes the constructor of the Animal
class to initialize the name
property of the Dog
class.
8. Instance and Static Methods
Methods can be either instance methods (which are invoked on instances of the class) or static methods (which are invoked on the class itself).
Instance Method
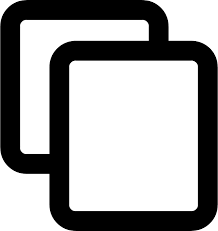
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
const person1 = new Person('Alice');
person1.greet(); // Output: Hello, my name is Alice
Static Method
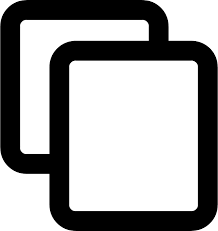
class MathOperations {
static multiply(a, b) {
return a * b;
}
}
console.log(MathOperations.multiply(2, 3)); // Output: 6
9. Conclusion
Classes and inheritance are powerful features in JavaScript that enable object-oriented programming. Classes provide a blueprint for creating objects and allow for easier management of shared properties and methods. Inheritance allows subclasses to extend and reuse functionality from parent classes, promoting code reuse and a more structured approach to programming.
Modules (import and export)
1. Introduction to JavaScript Modules
JavaScript modules allow you to break your code into smaller, reusable pieces, making it easier to maintain and organize. Modules enable better code organization and help avoid polluting the global namespace. With modules, you can import and export functionality between different files.
2. Exporting from a Module
To make a function, object, or variable available to other modules, you can export it using the export
keyword. There are two ways to export: named exports and default exports.
Named Exports
Named exports allow you to export multiple items from a module. You can export functions, variables, or classes by using the export
keyword before the declaration.
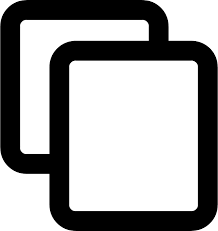
// math.js
export function add(a, b) {
return a + b;
}
export const pi = 3.14;
In this example, the add
function and the pi
constant are exported from the math.js
module using named exports.
Default Exports
A default export allows you to export a single value or function as the default export of the module. A module can only have one default export.
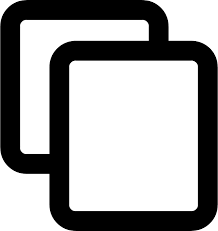
// calculator.js
export default function multiply(a, b) {
return a * b;
}
In this case, the multiply
function is the default export of the calculator.js
module. You can only have one default export per module.
3. Importing from a Module
To use the functionality from another module, you can import it into your current file using the import
keyword. You can import named exports or the default export.
Importing Named Exports
To import named exports, use curly braces {}> around the exported values.
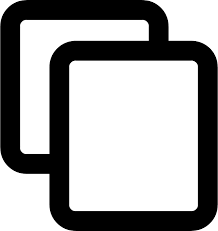
// app.js
import { add, pi } from './math.js';
console.log(add(2, 3)); // Output: 5
console.log(pi); // Output: 3.14
Here, we import the named exports add
and pi
from the math.js
module.
Importing the Default Export
To import the default export, you do not need curly braces. You can give it any name you like.
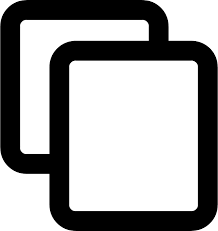
// app.js
import multiply from './calculator.js';
console.log(multiply(3, 4)); // Output: 12
In this case, we import the default export (the multiply
function) from the calculator.js
module.
Renaming Imports
You can also rename imported modules using the as
keyword.
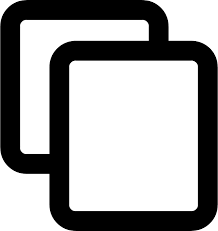
// app.js
import { add as addition, pi as piValue } from './math.js';
console.log(addition(2, 3)); // Output: 5
console.log(piValue); // Output: 3.14
In this example, we rename the add
function to addition
and the pi
constant to piValue
when importing.
4. Importing All Exports as an Object
If you want to import all the exports from a module as a single object, you can use the * as
syntax.
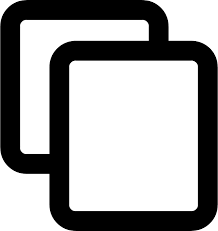
// app.js
import * as math from './math.js';
console.log(math.add(2, 3)); // Output: 5
console.log(math.pi); // Output: 3.14
In this case, we import everything from the math.js
module as an object named math>, and then access the exported values using
math.add
and math.pi
.
5. Dynamic Imports
Dynamic imports allow you to import modules at runtime using the import()
function. This can be useful when you want to load a module conditionally or on demand.
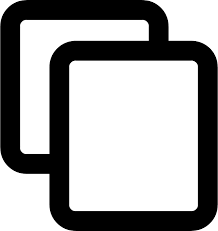
// app.js
const loadCalculator = async () => {
const { default: multiply } = await import('./calculator.js');
console.log(multiply(3, 4)); // Output: 12
}
loadCalculator();
In this example, we dynamically import the calculator.js
module when the loadCalculator
function is called.
6. Conclusion
JavaScript modules provide a clean and efficient way to organize and manage code. By using import
and export
, you can break your code into reusable pieces, improve maintainability, and avoid polluting the global namespace. Understanding how to work with modules is an essential skill for building scalable JavaScript applications.
Understanding Closures
1. What is a Closure?
A closure is a function that has access to its own scope, the scope of the outer function, and the global scope. Closures allow inner functions to continue to access variables from their outer scope even after the outer function has finished executing. This is a fundamental concept in JavaScript that is often used in situations like data encapsulation, callbacks, and event handlers.
2. How Closures Work
When a function is defined inside another function, it forms a closure. The inner function has access to the variables of its outer function, and even after the outer function finishes executing, the inner function can still access those variables.
Example 1: Basic Closure
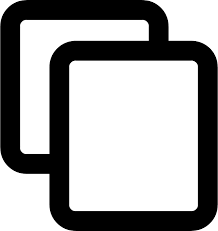
// Outer function
function outer() {
let outerVar = 'I am an outer variable';
// Inner function (closure)
function inner() {
console.log(outerVar); // Accesses outerVar
}
return inner; // Return the inner function
}
const closureFunction = outer();
closureFunction(); // Output: I am an outer variable
In this example, the inner()
function is a closure because it has access to the outerVar
variable from the outer outer()
function, even after outer()
finishes executing.
3. Lexical Scoping
Closures are possible because of lexical scoping. In JavaScript, a function's scope is determined by where it is defined, not where it is executed. This means that inner functions have access to variables from their surrounding scope, even if they are executed outside that scope.
Example 2: Lexical Scoping
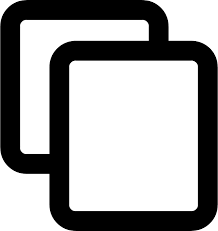
// Outer function
function outer() {
let outerVar = 'I am an outer variable';
// Inner function
function inner() {
console.log(outerVar); // Accesses outerVar from outer scope
}
inner(); // Output: I am an outer variable
}
outer();
In this case, the inner()
function is still able to access the outerVar
variable, even though it is executed inside the outer()
function.
4. Closures with Arguments
Closures can also access and remember arguments passed to the outer function.
Example 3: Closure with Arguments
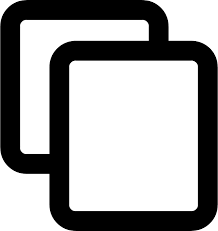
// Outer function
function outer(outerArg) {
// Inner function
return function inner(innerArg) {
console.log('Outer argument:', outerArg);
console.log('Inner argument:', innerArg);
};
}
const closureWithArgs = outer('Outer value');
closureWithArgs('Inner value');
// Output:
// Outer argument: Outer value
// Inner argument: Inner value
In this example, the inner function inner()
has access to both the argument passed to the outer function outer()
and the argument passed directly to the inner function.
5. Closures and Memory
Closures can sometimes result in memory issues, especially when large objects or arrays are captured by closures. Since closures retain references to variables from their outer scope, they can cause those variables to remain in memory even if they are no longer needed, leading to memory leaks. It's important to be aware of this when designing closures in performance-sensitive applications.
6. Practical Use Cases of Closures
Closures are commonly used in JavaScript for a variety of purposes:
- Data Encapsulation: Closures can be used to create private variables and methods, which are not accessible from outside the function.
- Event Handlers: Closures are often used to preserve the state in event handlers and callbacks.
- Currying: Closures can be used to create curried functions, allowing partial application of arguments.
- Callbacks and Promises: Closures are used in asynchronous programming to maintain the state of variables in callback functions and promises.
Example 4: Data Encapsulation with Closures
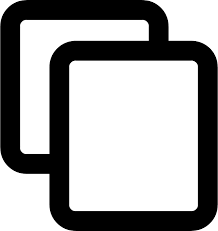
// Function that creates a private counter
function createCounter() {
let count = 0;
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
},
getCount: function() {
return count;
}
};
}
const counter = createCounter();
counter.increment(); // Output: 1
counter.increment(); // Output: 2
counter.decrement(); // Output: 1
console.log(counter.getCount()); // Output: 1
In this example, the count
variable is private to the createCounter()
function, and can only be modified or accessed using the methods defined in the returned object. This is a common use of closures to create private state.
7. Conclusion
Closures are a powerful feature of JavaScript that allow functions to remember and access variables from their outer scope, even after the outer function has finished executing. They are useful for many programming patterns, including data encapsulation, callbacks, and event handlers. Understanding closures is essential for writing efficient and maintainable JavaScript code.
Practical Examples
1. Function to Create Private Variables (Data Encapsulation)
One common use of closures is to create private variables that cannot be accessed from outside the function. This is useful for encapsulating data and creating modules with private state.
Example: Creating a Counter with Private State
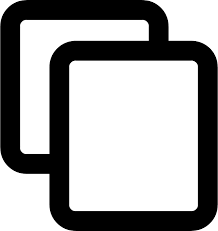
// Function to create a counter with private state
function createCounter() {
let count = 0; // Private variable
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
},
getCount: function() {
return count;
}
};
}
const counter = createCounter();
counter.increment(); // Output: 1
counter.increment(); // Output: 2
counter.decrement(); // Output: 1
console.log(counter.getCount()); // Output: 1
In this example, the count
variable is encapsulated within the closure and is not accessible from outside the function. The only way to modify or access it is through the methods increment()
, decrement()
, and getCount()
.
2. Implementing a Memoization Function
Memoization is a technique used to optimize functions by caching their results. Closures can be used to remember the results of previous function calls and return the cached result when the same inputs are encountered again.
Example: Fibonacci Sequence with Memoization
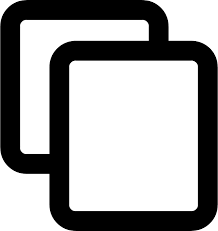
// Function that uses closures to memoize Fibonacci numbers
function memoizeFibonacci() {
const cache = {}; // Private cache
return function fib(n) {
if (n <= 1) return n;
if (cache[n]) return cache[n]; // Return cached result if available
cache[n] = fib(n - 1) + fib(n - 2); // Compute and cache the result
return cache[n];
};
}
const fibonacci = memoizeFibonacci();
console.log(fibonacci(10)); // Output: 55 (after computing)
console.log(fibonacci(10)); // Output: 55 (from cache)
In this example, the cache
object is encapsulated within the closure and is used to store previously computed Fibonacci numbers. This allows the function to return cached results instead of recalculating them each time.
3. Handling Asynchronous Operations
Closures are often used in asynchronous programming to maintain access to variables even after the outer function has finished executing. This is particularly useful for callbacks, event handlers, and promises.
Example: Using Closures in Event Handlers
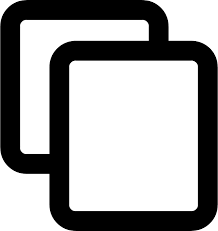
// Function to create a button with a click handler
function createButton(buttonId) {
let clicks = 0; // Private variable to track number of clicks
const button = document.getElementById(buttonId);
button.addEventListener('click', function() {
clicks++; // Accesses the clicks variable from the outer scope
console.log(`Button clicked ${clicks} times`);
});
}
createButton('myButton');
In this example, the click event handler is a closure that has access to the clicks
variable from the outer scope. Each time the button is clicked, the clicks
variable is incremented and logged to the console.
4. Currying Functions
Currying is a functional programming technique where a function is transformed into a sequence of functions that each take a single argument. Closures are used to create curried functions that allow for partial application of arguments.
Example: Curried Function for Multiplication
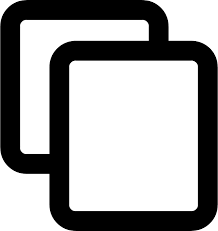
// Curried function to multiply numbers
function multiply(a) {
return function(b) {
return a * b;
};
}
const multiplyByTwo = multiply(2);
console.log(multiplyByTwo(5)); // Output: 10
console.log(multiplyByTwo(10)); // Output: 20
In this example, the multiply()
function returns a closure that remembers the value of a
(the first argument) and uses it when the second argument b
is provided. This is a simple form of currying that allows us to create a new function for multiplying by a specific number.
5. Using Closures with Promises
Closures are often used in promises to maintain access to variables from the outer scope, even in asynchronous code.
Example: Fetching Data and Using Closures
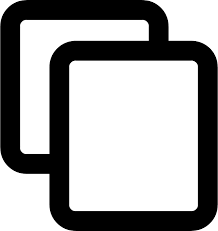
// Function to fetch data and log the result
function fetchData(url) {
let isLoading = true; // Private variable to track loading state
console.log('Loading data...');
fetch(url)
.then(response => response.json())
.then(data => {
isLoading = false; // Update loading state when data is fetched
console.log('Data received:', data);
})
.catch(error => {
isLoading = false;
console.log('Error fetching data:', error);
});
}
fetchData('https://api.example.com/data');
In this example, the isLoading
variable is captured by the closure inside the fetchData()
function. This allows us to track the loading state while the data is being fetched asynchronously.
6. Conclusion
Closures are a powerful feature in JavaScript that allow us to create private state, optimize performance with memoization, handle asynchronous operations, and implement functional programming techniques like currying. Understanding closures and their practical use cases is crucial for writing efficient and maintainable JavaScript code.
Callback Functions
A callback function is a function that is passed as an argument to another function and is executed after the completion of a task. Callback functions are commonly used for handling asynchronous operations like data fetching, event handling, and more.
1. Synchronous Callback Function
A synchronous callback function is executed immediately when it is passed to another function. It runs during the execution of the higher-order function.
Example: Basic Synchronous Callback
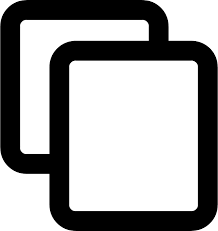
// Function that accepts a callback and executes it
function greet(name, callback) {
console.log('Hello, ' + name);
callback();
}
greet('Alice', function() {
console.log('This is a callback function.');
});
In this example, the greet
function accepts a callback
function that is invoked after the greeting message is logged to the console. The callback function runs synchronously after the main function execution.
2. Asynchronous Callback Function
Asynchronous callback functions are commonly used in tasks that take time to complete, such as API requests, file reading, or event handling. These functions are executed once the asynchronous operation completes.
Example: Asynchronous Callback with setTimeout
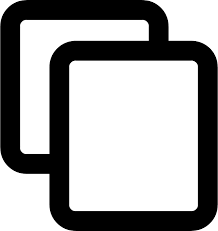
// Function with asynchronous callback
function fetchData(callback) {
setTimeout(function() {
console.log('Data fetched successfully.');
callback();
}, 2000); // Simulating an API request with a 2-second delay
}
fetchData(function() {
console.log('Callback executed after data fetch.');
});
In this example, the fetchData
function simulates a delay (like fetching data from an API) using setTimeout
. The callback function is executed after the data fetching process is complete.
3. Callback Functions in Array Methods
Callback functions are heavily used in array methods like forEach()
, map()
, and filter()
to process elements in an array.
Example: Using Callback in forEach
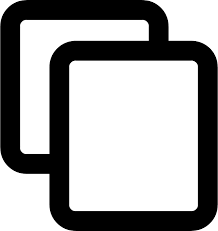
// Using a callback function with forEach
const numbers = [1, 2, 3, 4];
numbers.forEach(function(number) {
console.log(number * 2); // Callback function that doubles each number
});
In this example, the forEach
method takes a callback function that is called for each element in the numbers
array. The callback doubles each number and logs it to the console.
Example: Using Callback in map
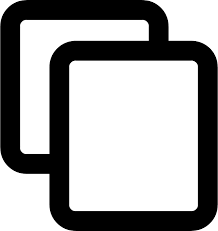
// Using a callback function with map
const numbers = [1, 2, 3, 4];
const doubledNumbers = numbers.map(function(number) {
return number * 2; // Callback function that returns a doubled value
});
console.log(doubledNumbers); // Output: [2, 4, 6, 8]
In this example, the map
method uses a callback to transform each element in the numbers
array by multiplying it by 2. The callback returns the transformed value, and the resulting array is logged to the console.
4. Callback Functions with Error Handling
Callback functions can also be used for error handling, especially when working with asynchronous tasks. The callback can accept an error as the first argument, which is a common pattern known as the error-first callback.
Example: Error-First Callback
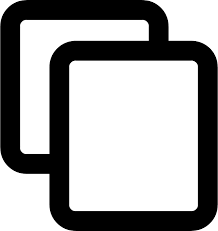
// Function to simulate an asynchronous operation with error handling
function fetchData(callback) {
setTimeout(function() {
const error = false;
if (error) {
callback('Error occurred while fetching data.');
} else {
callback(null, 'Data fetched successfully.');
}
}, 2000); // Simulating a 2-second delay
}
fetchData(function(error, data) {
if (error) {
console.log(error); // Error handling
} else {
console.log(data); // Success message
}
});
In this example, the fetchData
function uses an error-first callback. If an error occurs, the error message is passed as the first argument to the callback. Otherwise, the success message is passed as the second argument.
5. Advantages of Callback Functions
- Asynchronous Handling: Callbacks allow you to handle tasks asynchronously, preventing your program from blocking while waiting for an operation to complete.
- Code Reusability: By passing different callback functions, you can reuse the same higher-order function for various tasks.
- Flexible and Modular: Callbacks allow you to write more flexible and modular code, especially when combined with array methods or event handling.
6. Conclusion
Callback functions are an essential part of JavaScript, providing a powerful mechanism for handling asynchronous operations, error handling, and enabling functional programming patterns. Understanding and using callbacks effectively will help you write efficient, maintainable, and modular code.
Callback Hell and Solutions
Callback hell, sometimes referred to as pyramid of doom, occurs when multiple nested callback functions are used in a way that makes the code harder to read, maintain, and debug. As callbacks are nested inside each other, the code becomes more complex and indentation increases, leading to poor readability.
1. The Problem with Callback Hell
Callback hell typically happens when you have asynchronous functions that rely on each other, and you pass a callback function to handle the result of each function. When multiple asynchronous functions need to be executed in sequence, the callbacks are nested, resulting in deeply indented code.
Example: Callback Hell
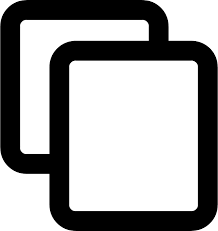
// Example of callback hell
function fetchData(callback) {
setTimeout(() => {
console.log('Data fetched');
callback();
}, 1000);
}
function processData(callback) {
setTimeout(() => {
console.log('Data processed');
callback();
}, 1000);
}
function saveData(callback) {
setTimeout(() => {
console.log('Data saved');
callback();
}, 1000);
}
fetchData(function() {
processData(function() {
saveData(function() {
console.log('All tasks completed');
});
});
});
In the example above, each function relies on the completion of the previous one, and callbacks are nested inside each other. This leads to deeply indented code, which is hard to follow and maintain. This is what we call callback hell.
2. Solutions to Avoid Callback Hell
There are several approaches to avoid callback hell, making your code more readable and manageable.2.1. Using Named Functions
One solution to avoid the pyramid of doom is to use named functions for the callbacks. This helps reduce the nesting and keeps the code more readable and maintainable.
Example: Using Named Functions
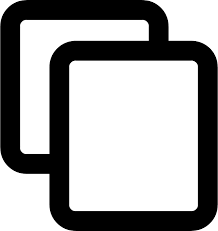
// Using named functions to avoid callback hell
function fetchData(callback) {
setTimeout(() => {
console.log('Data fetched');
callback();
}, 1000);
}
function processData(callback) {
setTimeout(() => {
console.log('Data processed');
callback();
}, 1000);
}
function saveData(callback) {
setTimeout(() => {
console.log('Data saved');
callback();
}, 1000);
}
function allTasksCompleted() {
console.log('All tasks completed');
}
fetchData(function() {
processData(function() {
saveData(allTasksCompleted);
});
});
In this example, the callback functions have been replaced with a named function allTasksCompleted
, which reduces the indentation and makes the code easier to understand.
2.2. Promises
One of the most popular solutions to callback hell is using Promises. Promises allow you to chain asynchronous operations and handle them in a more readable way, avoiding nested callbacks.
Example: Using Promises
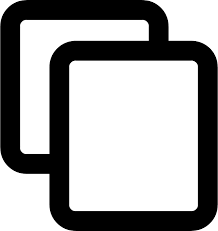
// Using Promises to avoid callback hell
function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Data fetched');
resolve();
}, 1000);
});
}
function processData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Data processed');
resolve();
}, 1000);
});
}
function saveData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Data saved');
resolve();
}, 1000);
});
}
fetchData()
.then(processData)
.then(saveData)
.then(() => {
console.log('All tasks completed');
})
.catch((error) => {
console.error('Error:', error);
});
In this solution, we use Promises
to handle the asynchronous operations. Each function returns a promise, and we chain them together using then()
. This eliminates the nested callbacks and makes the code much easier to read and maintain.
2.3. Async/Await
Another modern solution to deal with callback hell is using async/await. With async/await, asynchronous code looks and behaves more like synchronous code, making it even more readable and easier to manage than promises.
Example: Using Async/Await
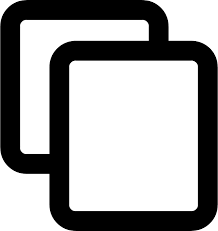
// Using async/await to avoid callback hell
async function performTasks() {
try {
await fetchData();
await processData();
await saveData();
console.log('All tasks completed');
} catch (error) {
console.error('Error:', error);
}
}
performTasks();
In this example, the async/await
syntax allows us to write asynchronous code that looks synchronous. Each asynchronous function is awaited before moving to the next one. This makes the code more concise and readable.
3. Conclusion
Callback hell is a common problem when dealing with nested asynchronous functions. However, there are various solutions to avoid it, such as using named functions, promises, and async/await syntax. These solutions make the code more readable, manageable, and easier to debug.
Event Handling with addEventListener
In JavaScript, event handling allows us to execute functions when certain user interactions occur, such as clicks, key presses, mouse movements, etc. One of the most common methods for handling events is by using the addEventListener
method.
1. What is addEventListener?
The addEventListener
method is used to attach an event handler to a specified element without overwriting any existing event handlers. This method allows multiple event listeners to be attached to the same element for the same event type.
Syntax:
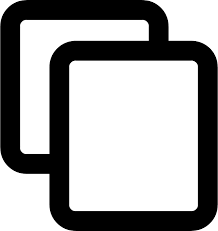
element.addEventListener(event, callback, useCapture);
- event: The name of the event (e.g.,
click
,mouseover
,keydown
). - callback: The function that will be executed when the event is triggered.
- useCapture (optional): A Boolean value that determines whether the event should be handled in the capturing phase or the bubbling phase. The default is
false
, which means the event will be handled in the bubbling phase.
2. Example: Handling a Click Event
Here is an example of using addEventListener
to handle a click event on a button.
Example Code:
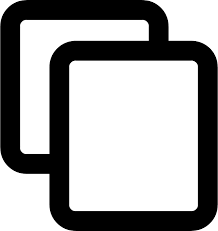
// HTML
<button id="myButton">Click Me</button>
// JavaScript
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button clicked!');
});
In this example, when the button is clicked, the callback function is executed, and an alert is displayed with the message "Button clicked!".
3. Using Event Listener with Multiple Elements
You can also attach an event listener to multiple elements. For example, to attach a click event to all buttons on a page, you can loop through the elements and add the event listener to each one.
Example Code:
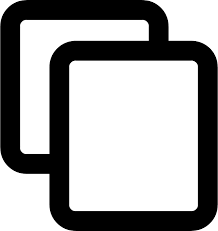
// HTML
<button>Button 1</button>
<button>Button 2</button>
<button>Button 3</button>
// JavaScript
const buttons = document.querySelectorAll('button');
buttons.forEach(button => {
button.addEventListener('click', function() {
alert('Button clicked!');
});
});
This code will attach the click event listener to all the buttons on the page. When any button is clicked, the alert will be triggered.
4. Removing Event Listeners
To remove an event listener, you can use the removeEventListener
method. The syntax is the same as addEventListener
, but it requires you to pass the same function reference that was used when adding the event listener.
Example Code:
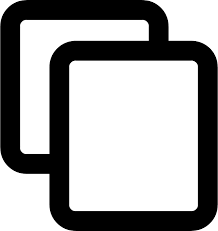
// HTML
<button id="myButton">Click Me</button>
// JavaScript
const button = document.getElementById('myButton');
function handleClick() {
alert('Button clicked!');
}
button.addEventListener('click', handleClick);
// Remove the event listener
button.removeEventListener('click', handleClick);
In this example, the handleClick
function is removed from the button after being added as an event listener, which prevents the event handler from being triggered when the button is clicked again.
5. Event Object
When an event is triggered, an event object is passed to the callback function. This object contains information about the event, such as the type of event, the target element, and the state of the event (e.g., whether a key is being pressed or the mouse is moved).
Example Code:
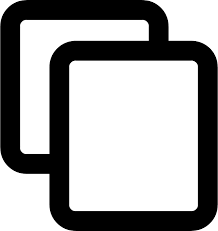
// HTML
<button id="myButton">Click Me</button>
// JavaScript
const button = document.getElementById('myButton');
button.addEventListener('click', function(event) {
console.log('Event Type:', event.type); // Output: 'click'
console.log('Event Target:', event.target); // Output: button element
});
In this example, the event object is passed to the callback function, and we log the type
of the event and the target
element, which is the button that was clicked.
6. Event Delegation
Event delegation is a technique where you attach a single event listener to a parent element rather than individual child elements. This technique is useful when you have many elements that need the same event handling, and it can also handle dynamically added elements.
Example Code:
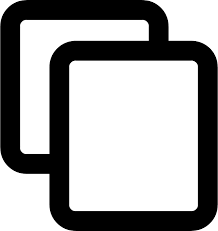
// HTML
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
// JavaScript
const list = document.getElementById('myList');
list.addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
alert('List item clicked: ' + event.target.textContent);
}
});
In this example, the click event listener is attached to the <ul>
element. When a <li>
item is clicked, the event listener checks if the target is an <li>
and displays an alert with the clicked item's text. This technique works for dynamically added list items as well.
7. Conclusion
The addEventListener
method is a powerful tool for handling events in JavaScript. It allows you to attach multiple event listeners to elements, handle various types of events, and take advantage of techniques like event delegation to manage events efficiently.
Delegation for Dynamic Content
Event delegation is a powerful technique in JavaScript that allows you to manage events for dynamically added content. Instead of attaching event listeners to individual elements, event delegation involves attaching a single event listener to a parent element, which can handle events for its child elements, including dynamically added ones.
1. What is Event Delegation?
Event delegation is the practice of using a single event listener on a parent element to handle events for multiple child elements. This is particularly useful when you have elements that are created dynamically, meaning they're not present in the DOM when the page is initially loaded, or when you're dealing with large numbers of similar elements.
Example of Event Delegation:
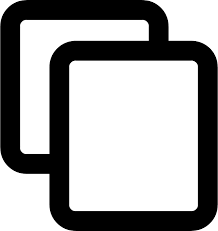
// HTML
<ul id="parentList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
// JavaScript
const parentList = document.getElementById('parentList');
parentList.addEventListener('click', function(event) {
// Check if the clicked element is an tag
if (event.target.tagName === 'LI') {
alert('Item clicked: ' + event.target.textContent);
}
});
In this example, the event listener is attached to the parent <ul>
element. When a user clicks on any of the child <li>
items, the event listener is triggered, and the appropriate action is taken. This approach works even if new <li>
items are dynamically added to the list later.
2. Why Use Event Delegation?
- Performance: Instead of attaching individual event listeners to each element (which can be inefficient), you attach one listener to the parent element, reducing memory usage and improving performance.
- Dynamic Content: Event delegation allows you to handle events for dynamically added elements without needing to re-attach event listeners each time new elements are created or added to the DOM.
- Cleaner Code: With event delegation, you avoid repeating code, making your scripts more maintainable and cleaner.
3. Example: Adding Dynamic Content
Let's see how event delegation works when dynamically adding elements to a list.
Example Code:
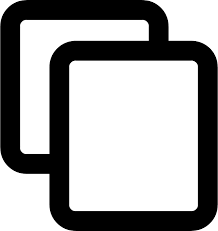
// HTML
<ul id="dynamicList">
<!-- Dynamic items will be added here -->
</ul>
<button id="addItemBtn">Add Item</button>
// JavaScript
const dynamicList = document.getElementById('dynamicList');
const addItemBtn = document.getElementById('addItemBtn');
addItemBtn.addEventListener('click', function() {
const newItem = document.createElement('li');
newItem.textContent = 'New Item';
dynamicList.appendChild(newItem);
});
dynamicList.addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
alert('Item clicked: ' + event.target.textContent);
}
});
In this example, each time the "Add Item" button is clicked, a new <li>
item is dynamically added to the <ul>
list. The event listener attached to the parent <ul>
element will handle the click events for both the originally existing and newly added <li>
items.
4. Benefits of Delegation for Dynamic Content
- No Need to Reattach Listeners: You don't have to reattach event listeners every time new elements are added to the DOM. The parent element will catch events for newly added child elements automatically.
- More Efficient: Instead of adding listeners to each element individually, you attach only one listener to the parent, which listens for events on all child elements.
- Handles Events for Elements Added After Page Load: As long as the parent element exists, you can capture events for elements that are dynamically created and added to the DOM.
5. Example: Handling Multiple Event Types
Event delegation can also be used for handling different types of events for dynamic content. For instance, you can listen for both click and mouseover events on dynamically added elements.
Example Code:
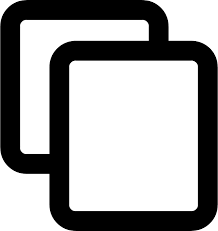
// HTML
<ul id="dynamicList">
<!-- Dynamic items will be added here -->
</ul>
<button id="addItemBtn">Add Item</button>
// JavaScript
const dynamicList = document.getElementById('dynamicList');
const addItemBtn = document.getElementById('addItemBtn');
addItemBtn.addEventListener('click', function() {
const newItem = document.createElement('li');
newItem.textContent = 'New Item';
dynamicList.appendChild(newItem);
});
dynamicList.addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
alert('Item clicked: ' + event.target.textContent);
}
});
dynamicList.addEventListener('mouseover', function(event) {
if (event.target.tagName === 'LI') {
event.target.style.backgroundColor = 'lightgray';
}
});
dynamicList.addEventListener('mouseout', function(event) {
if (event.target.tagName === 'LI') {
event.target.style.backgroundColor = '';
}
});
In this example, both click and mouseover events are delegated to the parent <ul>
element. When a user clicks or hovers over any <li>
item (including dynamically added ones), the corresponding actions are executed.
6. Conclusion
Event delegation is an essential technique for handling dynamic content in JavaScript. By attaching event listeners to parent elements rather than individual child elements, you can manage events more efficiently, improve performance, and handle events for dynamically added content without the need to reattach listeners.
JavaScript Classes
JavaScript classes provide a way to create objects and manage object-oriented programming (OOP) principles. Classes allow you to define objects with shared properties and methods, making it easier to work with similar objects and provide better structure for your code.
1. What is a Class?
A class in JavaScript is a blueprint for creating objects with shared properties and methods. It is a syntactical sugar over JavaScript's existing prototype-based inheritance, providing a simpler and cleaner way to define objects and their behavior.
Example of a Simple Class:
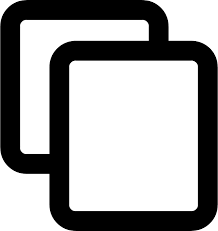
class Car {
constructor(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
displayInfo() {
console.log(`Car: ${this.year} ${this.make} ${this.model}`);
}
}
const car1 = new Car('Toyota', 'Camry', 2020);
car1.displayInfo(); // Output: Car: 2020 Toyota Camry
In this example, the class Car
defines a constructor to initialize the properties make
, model
, and year
. The method displayInfo()
is used to display the car's information.
2. Constructor Method
The constructor()
method is a special method for creating and initializing an object created with a class. It is automatically called when a new instance of the class is created.
Example of Constructor:
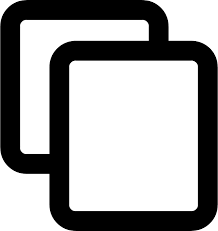
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
const person1 = new Person('Alice', 30);
person1.greet(); // Output: Hello, my name is Alice and I am 30 years old.
3. Methods in Classes
In addition to the constructor, you can also define methods within the class. These methods can be used to perform actions on the data within the object or provide functionality related to the object.
Example with Method:
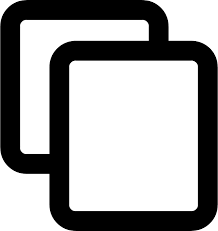
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
calculateArea() {
return this.width * this.height;
}
}
const rect = new Rectangle(10, 5);
console.log(rect.calculateArea()); // Output: 50
4. Inheritance and Subclasses
JavaScript allows you to create subclasses that extend a parent class. A subclass inherits the properties and methods of the parent class, and it can also define its own properties and methods.
Example of Inheritance:
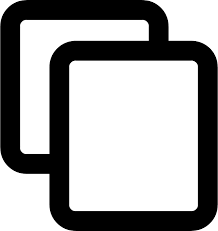
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name);
this.breed = breed;
}
speak() {
console.log(`${this.name} barks.`);
}
}
const dog1 = new Dog('Rex', 'German Shepherd');
dog1.speak(); // Output: Rex barks.
In this example, the class Dog
extends the Animal
class. It inherits the speak()
method from Animal
but overrides it to provide specific functionality for dogs.
5. Accessor and Mutator Methods (Getters and Setters)
JavaScript classes can include getter and setter methods to access and modify private properties. These methods allow you to encapsulate data and control how properties are accessed or modified.
Example of Getters and Setters:
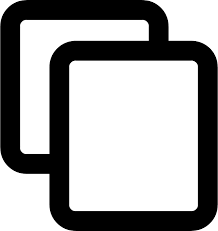
class Circle {
constructor(radius) {
this._radius = radius; // Private property with underscore
}
get radius() {
return this._radius;
}
set radius(value) {
if (value > 0) {
this._radius = value;
} else {
console.log('Radius must be positive.');
}
}
calculateArea() {
return Math.PI * this._radius * this._radius;
}
}
const circle = new Circle(5);
console.log(circle.calculateArea()); // Output: 78.53981633974483
circle.radius = -3; // Output: Radius must be positive.
circle.radius = 10; // No error
console.log(circle.calculateArea()); // Output: 314.1592653589793
6. Static Methods
Static methods are methods that belong to the class itself, rather than instances of the class. You can call static methods without creating an instance of the class.
Example of Static Method:
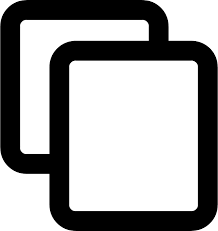
class MathUtility {
static add(a, b) {
return a + b;
}
static subtract(a, b) {
return a - b;
}
}
console.log(MathUtility.add(5, 3)); // Output: 8
console.log(MathUtility.subtract(5, 3)); // Output: 2
7. Conclusion
JavaScript classes provide a robust and organized way to work with objects and implement object-oriented programming principles. With classes, you can define objects, manage inheritance, and encapsulate logic in a more structured and reusable manner. Understanding how to use constructors, methods, inheritance, getters, setters, and static methods will greatly improve your ability to work with JavaScript in an object-oriented way.
Constructor Functions
Constructor functions in JavaScript are used to create objects. They work similarly to classes but are based on the function syntax. Constructor functions allow you to create multiple instances of similar objects with shared properties and methods.
1. What is a Constructor Function?
A constructor function is a function used to initialize an object. When a function is used as a constructor, it should be called using the new
keyword. This ensures that a new instance of the object is created.
Example of a Constructor Function:
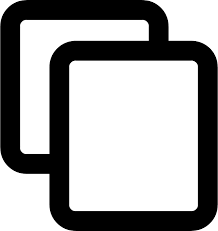
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
const car1 = new Car('Toyota', 'Corolla', 2022);
console.log(car1.make); // Output: Toyota
console.log(car1.model); // Output: Corolla
console.log(car1.year); // Output: 2022
In this example, the function Car
is acting as a constructor. It is used to create a new object with properties make
, model
, and year
.
2. Using the new
Keyword
The new
keyword is essential when calling a constructor function. It does the following:
- Creates a new empty object.
- Sets the
this
keyword to the new object. - Returns the new object unless explicitly returned from the constructor function.
Example of Creating an Object:
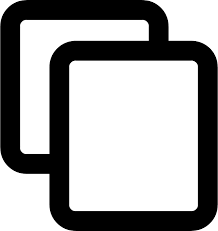
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person('Alice', 30);
console.log(person1.name); // Output: Alice
console.log(person1.age); // Output: 30
3. Adding Methods to Constructor Functions
Methods can also be added to constructor functions. These methods will be shared by all instances of the created object. You can add methods to the constructor function by attaching them to the prototype
of the constructor function.
Example of Adding Methods:
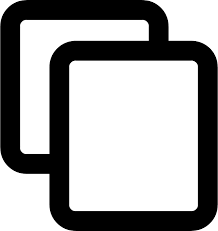
function Rectangle(width, height) {
this.width = width;
this.height = height;
}
Rectangle.prototype.calculateArea = function() {
return this.width * this.height;
};
const rect1 = new Rectangle(10, 5);
console.log(rect1.calculateArea()); // Output: 50
In this example, the method calculateArea
is added to the Rectangle
constructor function's prototype. This ensures that all Rectangle
instances share the same method.
4. Constructor Functions vs. Classes
Constructor functions and classes in JavaScript are similar, but they have some differences:
- Syntax: Constructor functions use the function declaration syntax, while classes use the
class
keyword. - Prototype: Methods in constructor functions are added using the
prototype
object. In classes, methods are defined directly within the class body. - Inheritance: Inheritance in constructor functions is achieved using
Object.create()
or theprototype
chain, while in classes, inheritance is achieved using theextends
keyword.
Example of Constructor Function and Class Comparison:
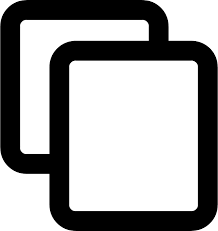
// Constructor Function Approach
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
// Class Approach
class AnimalClass {
constructor(name) {
this.name = name;
}
speak() {
console.log(this.name + ' makes a noise.');
}
}
const animal1 = new Animal('Dog');
animal1.speak(); // Output: Dog makes a noise.
const animal2 = new AnimalClass('Cat');
animal2.speak(); // Output: Cat makes a noise.
5. Constructor Functions for Inheritance
Constructor functions can be used for inheritance by calling the parent constructor function from the child constructor function using the call()
or apply()
method.
Example of Inheritance with Constructor Functions:
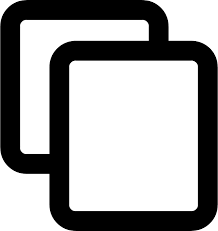
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
function Dog(name, breed) {
Animal.call(this, name); // Call the parent constructor
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
console.log(this.name + ' barks.');
};
const dog1 = new Dog('Rex', 'German Shepherd');
dog1.speak(); // Output: Rex makes a noise.
dog1.bark(); // Output: Rex barks.
6. Conclusion
Constructor functions are a core part of JavaScript's object-oriented programming features. They provide a way to create and initialize objects, and they can be extended with methods through prototypes. While the class syntax provides a more modern and easier-to-read approach, understanding constructor functions is essential for working with JavaScript's prototype-based inheritance and for writing efficient and reusable code.
Inheritance in JavaScript
Inheritance in JavaScript allows objects to inherit properties and methods from other objects. This is a fundamental concept in object-oriented programming (OOP) that enables code reuse and the creation of more complex systems by extending existing functionality.
1. What is Inheritance?
Inheritance allows one object to access properties and methods of another object. In JavaScript, inheritance is implemented using prototypes. All JavaScript objects have a prototype, and they inherit methods and properties from it.
Example of Inheritance:
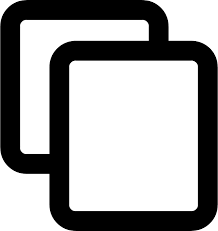
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
function Dog(name) {
Animal.call(this, name); // Call the parent constructor
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
const dog1 = new Dog('Rex');
dog1.speak(); // Output: Rex makes a noise.
In this example, the Dog
constructor function inherits from the Animal
constructor function, and it has access to the speak
method of Animal
.
2. Prototypical Inheritance
In JavaScript, inheritance is prototype-based. This means that every function and object in JavaScript has a prototype
property. When you create a new object, it inherits the properties and methods defined on the prototype of its constructor function.
Example of Prototypical Inheritance:
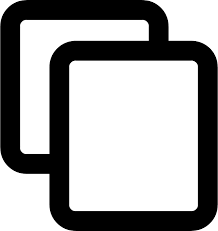
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
const animal1 = new Animal('Lion');
animal1.speak(); // Output: Lion makes a noise.
The animal1
object inherits the speak
method from the Animal
prototype.
3. Inheritance with the class
Syntax
JavaScript classes provide a more modern and simpler syntax for inheritance. Classes use the extends
keyword to create a child class that inherits from a parent class. The child class can access methods and properties of the parent class.
Example of Inheritance with Classes:
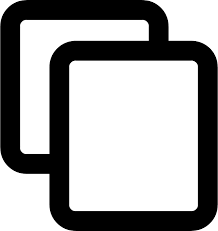
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(this.name + ' makes a noise.');
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name); // Call the parent constructor
this.breed = breed;
}
bark() {
console.log(this.name + ' barks.');
}
}
const dog1 = new Dog('Rex', 'German Shepherd');
dog1.speak(); // Output: Rex makes a noise.
dog1.bark(); // Output: Rex barks.
In this example, the Dog
class inherits from the Animal
class. The super()
method is used to call the constructor of the parent class.
4. Overriding Methods
In JavaScript, a child class can override methods inherited from the parent class. This allows for more specific functionality in the child class.
Example of Method Overriding:
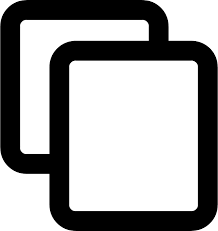
class Animal {
speak() {
console.log('Animal makes a noise.');
}
}
class Dog extends Animal {
speak() {
console.log('Dog barks.');
}
}
const animal1 = new Animal();
const dog1 = new Dog();
animal1.speak(); // Output: Animal makes a noise.
dog1.speak(); // Output: Dog barks.
In this example, the Dog
class overrides the speak
method from the Animal
class to provide its own implementation.
5. Inheriting Static Methods
Static methods are methods that are called on the class itself, rather than on instances of the class. These methods are not inherited by child classes. However, you can explicitly call a static method from a parent class in a child class.
Example of Static Method Inheritance:
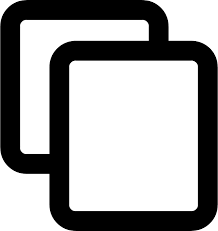
class Animal {
static type() {
console.log('This is an animal.');
}
}
class Dog extends Animal {}
Animal.type(); // Output: This is an animal.
Dog.type(); // Output: This is an animal.
In this example, both the Animal
and Dog
classes have access to the static type
method because it is inherited from the parent class.
6. Conclusion
Inheritance is a powerful feature in JavaScript that allows for the creation of more flexible and reusable code. It supports both prototypical inheritance and class-based inheritance. Understanding inheritance is crucial for writing clean, maintainable, and object-oriented code in JavaScript.
Prototypes and Prototype Chain
In JavaScript, prototypes are a mechanism for code reuse and inheritance. Every JavaScript object has a prototype, which is itself an object. The prototype chain allows for the inheritance of properties and methods from other objects, enabling a hierarchical structure for objects in JavaScript.
1. What is a Prototype?
Every JavaScript object has an internal property called [[Prototype]]
, which refers to another object (its prototype). The prototype provides default methods and properties that are available to all objects of a certain type. For example, arrays in JavaScript inherit methods like push()
and pop()
from their prototype, Array.prototype
.
Example of Prototypes:
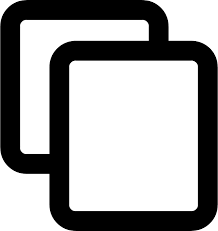
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
const animal1 = new Animal('Lion');
animal1.speak(); // Output: Lion makes a noise.
In this example, the speak
method is added to the prototype of the Animal
constructor function. All instances of Animal
can access this method.
2. Prototype Chain
The prototype chain is the series of prototypes that an object will traverse in search of a property or method. When a property or method is accessed on an object, JavaScript first checks if the property exists directly on the object itself. If not, it looks up the prototype chain.
Here's how the prototype chain works:
- When an object is created, it inherits properties and methods from its prototype.
- If a property is not found on the object, JavaScript looks for it on the object's prototype, and then on the prototype's prototype, and so on.
- This continues until JavaScript reaches the top of the prototype chain (the
Object.prototype
), at which point it stops.
Example of the Prototype Chain:
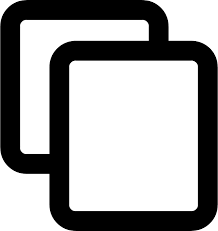
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
function Dog(name) {
Animal.call(this, name); // Call the parent constructor
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
const dog1 = new Dog('Rex');
dog1.speak(); // Output: Rex makes a noise.
In this example, the Dog
object inherits from the Animal
object. When the speak
method is called, JavaScript looks for it in the Dog.prototype
first. Since it doesn't find it there, it checks the prototype of Dog.prototype
, which is Animal.prototype
, and finds the method there.
3. Object.prototype
The Object.prototype
is the top-most object in the prototype chain. All objects in JavaScript (unless explicitly set otherwise) inherit from Object.prototype
. This means that methods like toString()
and hasOwnProperty()
are available on all objects.
Example of Object.prototype
:
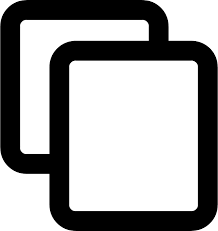
const obj = { name: 'John' };
console.log(obj.toString()); // Output: [object Object]
In this example, the toString
method is inherited from the Object.prototype
and is available on the obj
object.
4. Modifying Prototypes
You can modify an object's prototype by directly adding properties or methods to it. This allows for extending the functionality of built-in objects or custom objects.
Example of Modifying Prototypes:
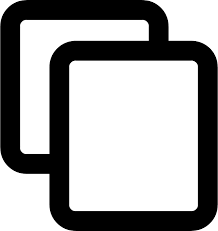
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
const animal1 = new Animal('Lion');
animal1.speak(); // Output: Lion makes a noise.
// Adding a new method to the Animal prototype
Animal.prototype.sleep = function() {
console.log(this.name + ' is sleeping.');
};
animal1.sleep(); // Output: Lion is sleeping.
In this example, we add a new method called sleep
to the prototype of Animal
, and all instances of Animal
can now use this method.
5. The instanceof
Operator
The instanceof
operator checks whether an object is an instance of a particular constructor function and whether the object is found in the prototype chain of that constructor.
Example of instanceof
:
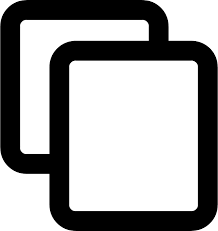
function Animal(name) {
this.name = name;
}
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
const dog1 = new Dog('Rex');
console.log(dog1 instanceof Dog); // Output: true
console.log(dog1 instanceof Animal); // Output: true
console.log(dog1 instanceof Object); // Output: true
In this example, dog1
is an instance of both Dog
and Animal
because Dog
inherits from Animal
via the prototype chain. It is also an instance of Object
, the top-most object.
6. Conclusion
Understanding prototypes and the prototype chain is crucial for working with inheritance in JavaScript. It helps in code reuse, extending objects, and creating hierarchical relationships between objects. JavaScript uses prototypes to implement object-oriented features such as inheritance and method sharing.
Introduction to Modules
In JavaScript, modules are a way to break up your code into smaller, reusable pieces. A module is a file that contains code that can be imported into other files. This allows for better organization, maintainability, and reusability of your code. With the introduction of ES6 (ECMAScript 2015), JavaScript now has a native module system built into the language.
1. What Are Modules?
Modules are JavaScript files that export functionality (such as variables, functions, or classes) and allow other files to import and use them. This modular approach helps to avoid polluting the global scope and makes the code more maintainable and modular.
Example of a Module:
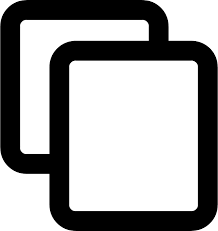
// math.js (Module)
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
In this example, the math.js
file exports two functions: add
and subtract
.
2. Importing Modules
Once a module is created, we can import its functionality into other JavaScript files using the import
keyword. You can import specific exports or import everything from a module.
Example of Importing a Module:
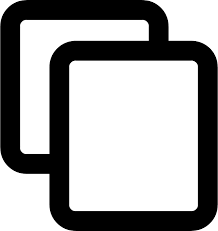
// main.js
import { add, subtract } from './math.js';
console.log(add(2, 3)); // Output: 5
console.log(subtract(5, 3)); // Output: 2
In this example, we import the add
and subtract
functions from the math.js
module and use them in the main.js
file.
3. Default Exports
In addition to named exports, a module can also have a default export. A default export is useful when a module only exports a single value, such as a function or an object.
Example of a Default Export:
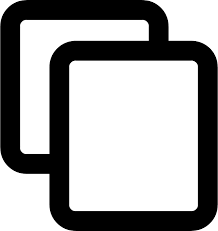
// math.js (Module)
export default function multiply(a, b) {
return a * b;
}
Example of Importing a Default Export:
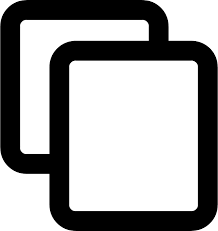
// main.js
import multiply from './math.js';
console.log(multiply(2, 3)); // Output: 6
In this example, we export the multiply
function as the default export from the math.js
module and import it in the main.js
file without using curly braces.
4. Importing All Exports
If you want to import all exports from a module, you can use the *
syntax to import everything as a single object. This is useful when you want to access multiple exports as properties of a single object.
Example of Importing All Exports:
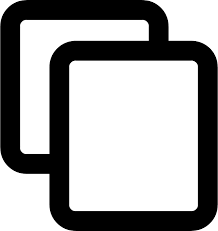
// main.js
import * as math from './math.js';
console.log(math.add(2, 3)); // Output: 5
console.log(math.subtract(5, 3)); // Output: 2
In this example, we import everything from the math.js
module into the math
object and access the add
and subtract
functions as properties of the math
object.
5. Modules in the Browser
Modules can also be used in the browser, but to enable the module system, the type="module"
attribute must be added to the <script>
tag in the HTML file.
Example of Using Modules in the Browser:
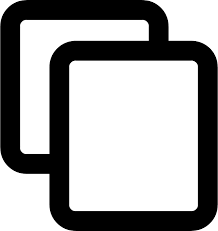
In this example, we load the main.js
file as a module in the browser.
6. Benefits of Using Modules
- Code Reusability: Modules allow you to reuse code across different parts of your application or even across different projects.
- Namespace Management: Modules help avoid polluting the global namespace by encapsulating variables and functions inside specific modules.
- Maintainability: Modules make your code more maintainable by organizing it into smaller, manageable files.
- Separation of Concerns: Modules allow you to separate different concerns of your application, such as data handling, UI rendering, or utility functions, into different modules.
7. Conclusion
Modules are an essential feature in modern JavaScript development. They allow for better organization, maintainability, and reusability of code. With the ES6 module system, it's easier than ever to structure your JavaScript code into smaller, more manageable pieces. By using the import
and export
keywords, you can organize your code and avoid cluttering the global namespace, making your code more efficient and scalable.
Using ES6 Modules
ES6 (ECMAScript 2015) introduced a native module system to JavaScript that allows you to organize your code into smaller, reusable pieces. With ES6 modules, you can export functions, objects, or values from one file and import them into another. This system makes your code more modular, cleaner, and easier to maintain.
1. Introduction to ES6 Modules
Before ES6, JavaScript didn’t have a native module system. Developers had to rely on libraries like CommonJS (used in Node.js) or AMD (Asynchronous Module Definition) to handle modules. ES6 introduced a standard way to define and use modules, which allows for better compatibility and interoperability across different JavaScript environments.
Key Features of ES6 Modules:
- Explicit Exports: You define what parts of your code should be available for other files to use using the
export
keyword. - Explicit Imports: You can import specific parts of a module into another file using the
import
keyword. - Strict Mode: ES6 modules are always in strict mode, which helps catch common coding errors.
- Static Structure: Imports and exports are evaluated statically, which enables optimizations like tree shaking.
2. Exporting from a Module
There are two main ways to export items from a module: named exports and default exports.
Named Exports:
In named exports, you can export multiple functions, variables, or objects from a module, and each export must be imported by its exact name in the importing module.
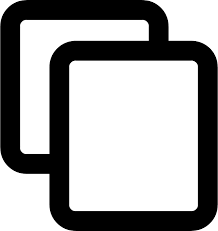
// math.js (Module with named exports)
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
Default Export:
If a module is exporting a single value or function, you can use the default export. The default export can be imported without curly braces, and you can choose any name when importing it.
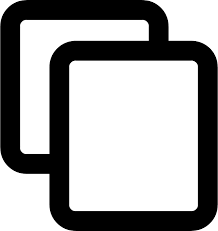
// math.js (Module with default export)
export default function multiply(a, b) {
return a * b;
}
3. Importing from a Module
Once you have exported functionality from a module, you can import it into another file using the import
keyword. You can import named exports, default exports, or everything from a module.
Importing Named Exports:
When importing named exports, you must use the exact name of the exported item inside curly braces.
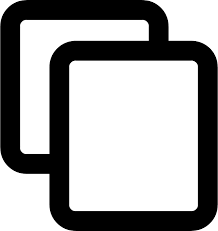
// main.js
import { add, subtract } from './math.js';
console.log(add(2, 3)); // Output: 5
console.log(subtract(5, 3)); // Output: 2
Importing a Default Export:
When importing a default export, you can name the imported value whatever you like.
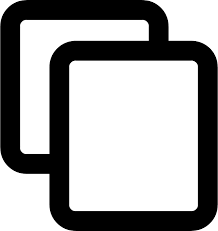
// main.js
import multiply from './math.js';
console.log(multiply(2, 3)); // Output: 6
Importing Everything from a Module:
You can import all exports from a module as a single object using the *
wildcard syntax.
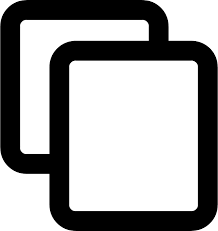
// main.js
import * as math from './math.js';
console.log(math.add(2, 3)); // Output: 5
console.log(math.subtract(5, 3)); // Output: 2
4. Modules in the Browser
In the browser, ES6 modules are supported, but you need to specify the type="module"
attribute when including the script in an HTML file. This allows the browser to recognize and properly handle the module syntax.
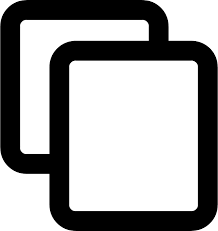
Using the type="module"
attribute ensures that the browser handles your JavaScript file as a module, allowing you to use import
and export
statements.
5. Benefits of Using ES6 Modules
- Encapsulation: Modules allow you to encapsulate functionality, keeping your code organized and preventing pollution of the global namespace.
- Reusability: By breaking your code into small, reusable modules, you can easily reuse code across different parts of your application or even across different projects.
- Better Maintainability: Modules improve code maintainability by organizing it into smaller, manageable files, making it easier to modify or extend.
- Optimizations: ES6 modules support static analysis, which allows for optimizations like tree shaking (removing unused code during bundling).
6. Conclusion
ES6 modules provide a powerful way to structure your JavaScript code. With import
and export
, you can write more modular, reusable, and maintainable code. The introduction of ES6 modules has made JavaScript development more efficient, and it’s now the standard for organizing JavaScript in modern applications.
Creating and Using RegExp
Regular Expressions (RegEx or RegExp) are a powerful tool for pattern matching and text manipulation in JavaScript. They allow you to search for, replace, or validate strings based on specific patterns, which can be very useful for tasks such as form validation, searching text in a document, and data extraction.
1. Introduction to Regular Expressions
A regular expression is a pattern that describes a set of strings. It can be used to check if a string contains a specified pattern, replace text, or extract specific parts of a string. Regular expressions are widely used in programming and can be implemented in JavaScript through the RegExp
object or by using regular expression literals.
Basic Structure of a Regular Expression:
- Literal characters: These are characters that match themselves (e.g.,
a
,1
,&
). - Metacharacters: Special characters that have a specific meaning in regular expressions (e.g.,
.
,^
,$
,[ ]
,?
). These characters are used to define patterns for matching.
2. Creating a Regular Expression
In JavaScript, you can create a regular expression either using a regular expression literal or the RegExp
constructor.
Using Regular Expression Literals:
A regular expression literal is a pattern enclosed between two forward slashes (/
) and can optionally include flags after the second slash.
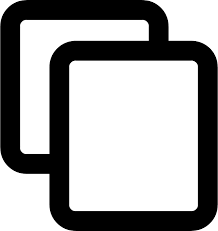
const regex = /hello/i; // 'i' flag makes the pattern case-insensitive
Using the RegExp Constructor:
You can also create a regular expression using the RegExp
constructor. This method is useful when the regular expression pattern or flags are stored in variables.
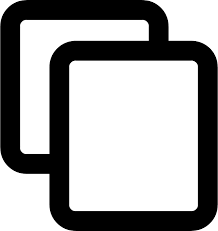
const regex = new RegExp('hello', 'i');
3. Regular Expression Flags
Regular expressions in JavaScript support several flags, which modify the behavior of the regular expression. The most common flags are:
- i: Makes the regular expression case-insensitive.
- g: Global search; finds all matches rather than stopping after the first match.
- m: Multiline search; allows
^
and$
to match the beginning and end of a line, not just the beginning and end of the string. - s: Allows
.
to match newline characters. - u: Enables full Unicode matching.
- d: Matches duplicate code points.
4. Using Regular Expressions for Matching
You can use regular expressions with several methods in JavaScript to perform pattern matching:
test() Method:
The test()
method tests whether a pattern matches a string. It returns true
if there is a match, and false
otherwise.
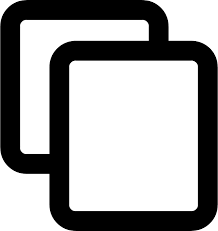
const regex = /hello/;
console.log(regex.test('hello world')); // Output: true
console.log(regex.test('goodbye world')); // Output: false
exec() Method:
The exec()
method executes a search for a match in a string. It returns an array of information about the match or null
if no match is found.
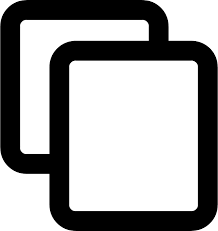
const regex = /hello/;
console.log(regex.exec('hello world')); // Output: ["hello"]
console.log(regex.exec('goodbye world')); // Output: null
5. Using Regular Expressions for Replacing Text
Regular expressions can be used with the replace()
method to replace matched text in a string.
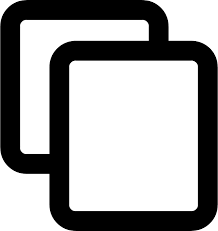
const str = 'Hello world';
const regex = /world/;
const newStr = str.replace(regex, 'JavaScript');
console.log(newStr); // Output: Hello JavaScript
6. Using Regular Expressions with String Methods
JavaScript offers several string methods that work well with regular expressions. Here are a few examples:match() Method:
The match()
method searches for a match in a string and returns an array of all matches.
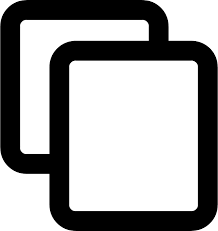
const str = 'The quick brown fox jumps over the lazy dog.';
const regex = /\b\w{5}\b/g; // Matches words with exactly 5 characters
console.log(str.match(regex)); // Output: ["quick", "brown"]
split() Method:
The split()
method divides a string into an array based on a regular expression pattern.
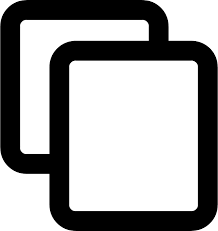
const str = 'apple,banana,cherry';
const regex = /,/;
console.log(str.split(regex)); // Output: ["apple", "banana", "cherry"]
7. Practical Examples
Here are some common use cases for regular expressions in JavaScript:
Validating an Email Address:
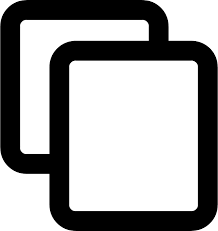
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
console.log(emailRegex.test('test@example.com')); // Output: true
console.log(emailRegex.test('invalid-email')); // Output: false
Extracting All Numbers from a String:
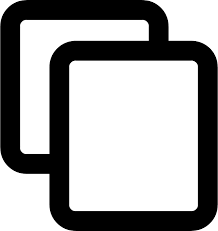
const str = 'I have 2 apples and 3 oranges.';
const regex = /\d+/g; // Matches one or more digits
console.log(str.match(regex)); // Output: ["2", "3"]
Replacing Multiple Spaces with a Single Space:
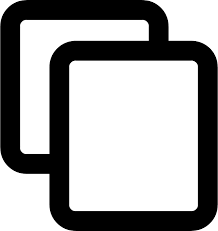
const str = 'This is a sentence with extra spaces.';
const regex = /\s+/g; // Matches one or more whitespace characters
console.log(str.replace(regex, ' ')); // Output: "This is a sentence with extra spaces."
8. Conclusion
Regular expressions are an incredibly powerful tool in JavaScript for pattern matching, text manipulation, and validation. By mastering regular expressions, you can perform complex text operations with just a few lines of code, making your JavaScript code cleaner and more efficient.
Common RegExp Patterns
Regular expressions (RegEx) provide a wide range of patterns that can be used to search, match, and manipulate strings. Below are some of the most commonly used RegEx patterns in JavaScript.
1. Literal Characters
Literal characters match themselves. For example, the pattern /hello/
matches the string "hello"
.
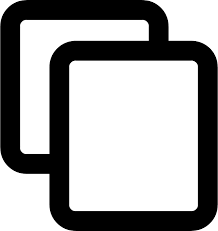
const regex = /hello/;
console.log(regex.test('hello world')); // Output: true
2. Dot (.) - Matches Any Character Except Newline
The dot .
matches any character except for a newline character. It is often used when you want to match any character in a given position of a string.
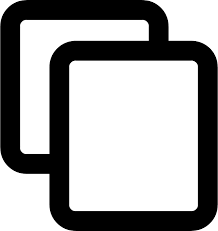
const regex = /h.llo/;
console.log(regex.test('hello')); // Output: true
console.log(regex.test('hallo')); // Output: true
console.log(regex.test('hxllo')); // Output: true
console.log(regex.test('h\nllo')); // Output: false
3. Metacharacters
Metacharacters are special characters that have a specific meaning in regular expressions. Some of the most common ones are:
- ^ - Matches the beginning of a string.
- $ - Matches the end of a string.
- \b - Matches a word boundary (spaces, punctuation, etc.).
- \d - Matches any digit (equivalent to [0-9]).
- \w - Matches any word character (alphanumeric or underscore, equivalent to [a-zA-Z0-9_]).
- \s - Matches any whitespace character (spaces, tabs, newlines).
- \D - Matches any non-digit character (equivalent to [^0-9]).
Example:
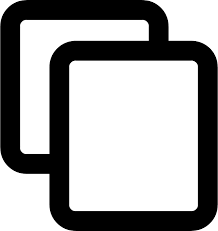
const regex = /^\d{3}-\d{2}-\d{4}$/;
console.log(regex.test('123-45-6789')); // Output: true
console.log(regex.test('123-456-789')); // Output: false
4. Character Classes
Character classes allow you to define a set of characters that can match a single character in the input string. Some common character classes include:
- [abc] - Matches any one of the characters
a
,b
, orc
. - [^abc] - Matches any character except
a
,b
, orc
. - [a-z] - Matches any lowercase letter from
a
toz
. - [A-Z] - Matches any uppercase letter from
A
toZ
. - [0-9] - Matches any digit from
0
to9
.
Example:
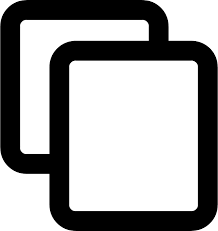
const regex = /[a-zA-Z]/;
console.log(regex.test('hello')); // Output: true
console.log(regex.test('12345')); // Output: false
5. Quantifiers
Quantifiers specify how many times an element in the regular expression should be matched:
- * - Matches 0 or more occurrences of the preceding character or group.
- + - Matches 1 or more occurrences of the preceding character or group.
- ? - Matches 0 or 1 occurrence of the preceding character or group.
- {n} - Matches exactly
n
occurrences of the preceding character or group. - {n,} - Matches
n
or more occurrences of the preceding character or group. - {n,m} - Matches between
n
andm
occurrences of the preceding character or group.
Example:
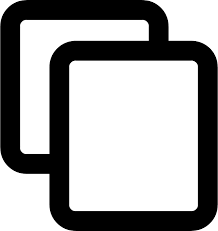
const regex = /\d{3}/;
console.log(regex.test('123')); // Output: true
console.log(regex.test('12')); // Output: false
console.log(regex.test('12345')); // Output: true
6. Grouping and Capturing
Parentheses ( )
are used to group parts of a regular expression. This allows you to apply quantifiers or other operations to multiple elements at once.
Example:
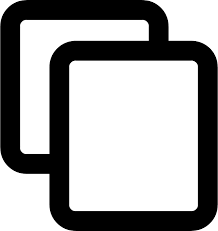
const regex = /(abc)+/;
console.log(regex.test('abcabc')); // Output: true
console.log(regex.test('abc')); // Output: true
7. Non-Capturing Groups
If you don’t need to capture the matched group, you can use a non-capturing group by adding ?:
inside the parentheses.
Example:
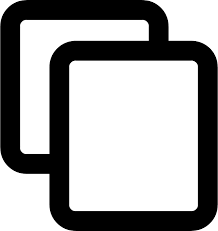
const regex = /(?:abc){2}/;
console.log(regex.test('abcabc')); // Output: true
8. Lookahead and Lookbehind
Lookahead and lookbehind are advanced regular expressions that allow you to match a pattern only if it is followed or preceded by another pattern.
- Positive Lookahead:
(?=pattern)
- Matches if the pattern is followed by the specified string. - Negative Lookahead:
(?!pattern)
- Matches if the pattern is not followed by the specified string. - Positive Lookbehind:
(?<=pattern)
- Matches if the pattern is preceded by the specified string. - Negative Lookbehind:
(? - Matches if the pattern is not preceded by the specified string.
Example:
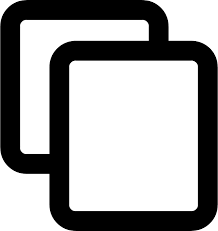
const regex = /(?<=\d{3})\d+/; // Matches digits only if preceded by 3 digits
console.log(regex.test('123456')); // Output: true
9. Matching Optional Characters
The question mark ?
is used to match a character or group that is optional (0 or 1 occurrence).
Example:
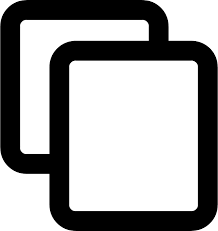
const regex = /colou?r/; // Matches "color" or "colour"
console.log(regex.test('color')); // Output: true
console.log(regex.test('colour')); // Output: true
10. Escape Characters
Some characters have special meaning in regular expressions. If you need to match one of these special characters, you must escape it using a backslash \
.
Example:
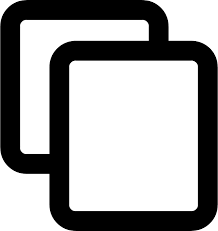
const regex = /\./; // Matches a literal dot
console.log(regex.test('hello.')); // Output: true
Conclusion
These are just a few of the most common regular expression patterns used in JavaScript. Regular expressions are extremely flexible and can be used to solve many text-processing tasks. With practice, you can create complex patterns to match almost any type of string.
Using localStorage and sessionStorage
The localStorage
and sessionStorage
objects provide a way to store data on the client side in a web browser. They are part of the Web Storage API and allow you to store data that persists even when the browser is closed (for localStorage
) or only for the duration of the page session (for sessionStorage
). Both are key-value storage systems.
1. localStorage
localStorage
stores data with no expiration time. The data remains stored even when the browser is closed and reopened. It is commonly used for storing preferences or user-specific data across different sessions.
Example:
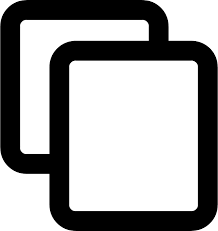
// Storing data in localStorage
localStorage.setItem('username', 'JohnDoe');
// Retrieving data from localStorage
const username = localStorage.getItem('username');
console.log(username); // Output: JohnDoe
// Removing data from localStorage
localStorage.removeItem('username');
// Clearing all data from localStorage
localStorage.clear();
Note: The data stored in localStorage
is accessible across tabs and windows in the same browser. However, it is not shared between different browsers or devices.
2. sessionStorage
sessionStorage
stores data for the duration of the page session. The data is only available as long as the browser tab is open. Once the tab or browser is closed, the data is lost.
Example:
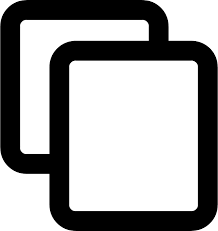
// Storing data in sessionStorage
sessionStorage.setItem('sessionData', 'Session Info');
// Retrieving data from sessionStorage
const sessionData = sessionStorage.getItem('sessionData');
console.log(sessionData); // Output: Session Info
// Removing data from sessionStorage
sessionStorage.removeItem('sessionData');
// Clearing all data from sessionStorage
sessionStorage.clear();
3. Differences Between localStorage and sessionStorage
- Persistence: Data in
localStorage
persists even after the browser is closed and reopened, while data insessionStorage
is cleared when the browser tab is closed. - Scope:
localStorage
is accessible across tabs and windows in the same browser, whereassessionStorage
is specific to the tab in which it was set. - Storage Limit: Both
localStorage
andsessionStorage
typically support a storage limit of around 5MB per origin (though this limit may vary depending on the browser).
4. Use Cases
- localStorage: Ideal for storing user preferences, settings, themes, or any data that you want to persist even after the user leaves your site and returns later.
- sessionStorage: Suitable for temporary data such as a shopping cart or form data that should only persist for the duration of the page session.
5. Example of Combining localStorage and sessionStorage
You can use both localStorage
and sessionStorage
in a single application to handle different types of data. For example, you might store user preferences in localStorage
and session-specific data (like a shopping cart) in sessionStorage
.
Example:
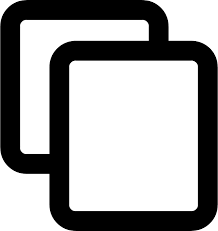
// Storing user preferences in localStorage
localStorage.setItem('theme', 'dark');
// Storing session-specific data in sessionStorage
sessionStorage.setItem('cart', JSON.stringify(['item1', 'item2']));
// Retrieving both
const theme = localStorage.getItem('theme');
const cart = JSON.parse(sessionStorage.getItem('cart'));
console.log(theme); // Output: dark
console.log(cart); // Output: ['item1', 'item2']
6. Security Considerations
While both localStorage
and sessionStorage
are convenient for storing client-side data, be aware of security concerns:
- Data Storage: Data stored in both
localStorage
andsessionStorage
is not encrypted. Avoid storing sensitive data such as passwords or credit card information in these storage systems. - Cross-Site Scripting (XSS): Be cautious of XSS attacks, as malicious scripts could potentially access data stored in
localStorage
orsessionStorage
if not properly sanitized.
7. Conclusion
localStorage
and sessionStorage
are useful tools for storing data on the client side. They are simple to use and provide a way to store data across sessions or just within a session. However, they should be used cautiously with sensitive information due to security concerns.
Storing and Retrieving Data
In JavaScript, we can store and retrieve data using various storage mechanisms. The most common methods are utilizing browser storage options such as localStorage
and sessionStorage
, as well as interacting with server-side databases via APIs. This section will focus on how to store and retrieve data using browser storage mechanisms.
1. Storing Data in localStorage
localStorage
allows you to store data persistently across different sessions in a user's browser. The data stored in localStorage
remains available even after the browser is closed and reopened.
Example:
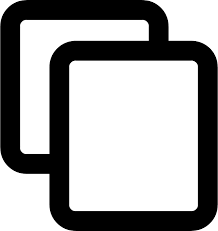
// Storing a simple key-value pair
localStorage.setItem('username', 'JohnDoe');
// Storing an object (must be converted to a string)
const user = { name: 'John', age: 30 };
localStorage.setItem('user', JSON.stringify(user));
2. Retrieving Data from localStorage
Once data is stored in localStorage
, it can be retrieved using the getItem()
method. To retrieve objects, you will need to parse the JSON string back into an object.
Example:
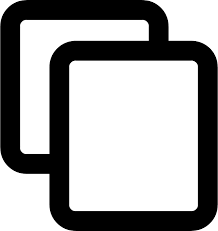
// Retrieving a simple value
const username = localStorage.getItem('username');
console.log(username); // Output: JohnDoe
// Retrieving an object
const user = JSON.parse(localStorage.getItem('user'));
console.log(user.name); // Output: John
console.log(user.age); // Output: 30
3. Storing Data in sessionStorage
sessionStorage
works similarly to localStorage
, but its data is cleared once the page session ends (i.e., when the browser tab is closed).
Example:
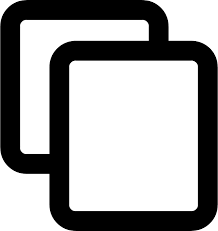
// Storing data in sessionStorage
sessionStorage.setItem('sessionKey', 'sessionValue');
// Storing an object (converted to a string)
const sessionData = { page: 'home', timestamp: Date.now() };
sessionStorage.setItem('sessionData', JSON.stringify(sessionData));
4. Retrieving Data from sessionStorage
Just like localStorage
, you can retrieve data from sessionStorage
using getItem()
and parse objects as needed.
Example:
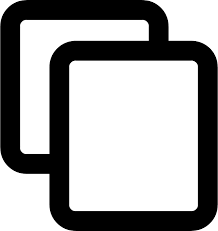
// Retrieving a simple value
const sessionKey = sessionStorage.getItem('sessionKey');
console.log(sessionKey); // Output: sessionValue
// Retrieving an object
const sessionData = JSON.parse(sessionStorage.getItem('sessionData'));
console.log(sessionData.page); // Output: home
console.log(sessionData.timestamp); // Output: [timestamp]
5. Removing Data from Storage
Both localStorage
and sessionStorage
provide methods to remove individual items or clear all stored data.
Example:
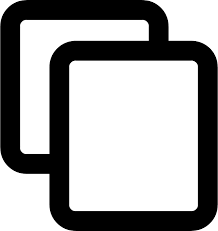
// Removing a single item
localStorage.removeItem('username');
sessionStorage.removeItem('sessionKey');
// Clearing all data
localStorage.clear();
sessionStorage.clear();
6. Practical Use Case Example: Storing User Preferences
Let's consider an example where you store and retrieve user preferences such as theme choice (light/dark) using localStorage
so that the user's preference is retained even after they close and reopen the browser.
Example:
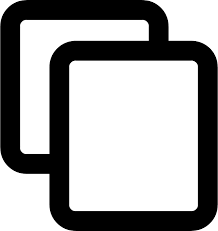
// Storing the user's theme preference
const theme = 'dark';
localStorage.setItem('theme', theme);
// Retrieving the user's theme preference
const storedTheme = localStorage.getItem('theme');
if (storedTheme) {
document.body.className = storedTheme; // Apply the theme to the page
} else {
document.body.className = 'light'; // Default theme
}
7. Security Considerations
When using localStorage
and sessionStorage
, keep the following security concerns in mind:
- Data stored in both
localStorage
andsessionStorage
is not encrypted, so avoid storing sensitive information like passwords or payment details. - Be cautious of Cross-Site Scripting (XSS) attacks, where malicious scripts might access stored data. Always sanitize input data and avoid injecting user data directly into the DOM without proper escaping.
8. Conclusion
Storing and retrieving data using localStorage
and sessionStorage
is a straightforward way to save client-side data. While localStorage
provides persistent storage, sessionStorage
is more suitable for temporary data that should be available only during a single session. Remember to handle sensitive data securely and avoid storing critical information in either of these storage options.
Parsing JSON Strings
In JavaScript, JSON (JavaScript Object Notation) is a popular format for exchanging data between a client and a server. JSON data is typically represented as a string, and in order to work with it in JavaScript, we need to parse the JSON string into a JavaScript object.
1. JSON.parse()
The JSON.parse()
method is used to parse a JSON string into a JavaScript object. It takes a valid JSON string and converts it into an object, array, or other valid JavaScript data type, depending on the structure of the JSON string.
Example:
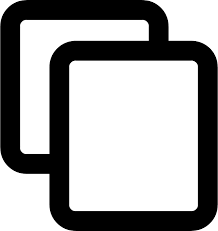
// A valid JSON string
const jsonString = '{"name": "John", "age": 30, "isEmployed": true}';
// Parsing the JSON string into an object
const parsedObject = JSON.parse(jsonString);
// Accessing the parsed object's properties
console.log(parsedObject.name); // Output: John
console.log(parsedObject.age); // Output: 30
console.log(parsedObject.isEmployed); // Output: true
2. Parsing JSON Arrays
JSON strings can also represent arrays. When parsing JSON arrays, the JSON.parse()
method converts the JSON array string into a JavaScript array.
Example:
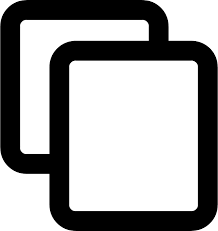
// A JSON array string
const jsonArrayString = '[1, 2, 3, 4, 5]';
// Parsing the JSON string into an array
const parsedArray = JSON.parse(jsonArrayString);
// Accessing elements of the parsed array
console.log(parsedArray[0]); // Output: 1
console.log(parsedArray[4]); // Output: 5
3. Handling Errors While Parsing
If the JSON string is not valid, the JSON.parse()
method will throw a SyntaxError
. To handle such errors, you should use a try-catch block.
Example:
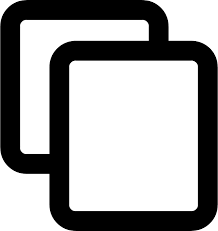
try {
// An invalid JSON string (missing quotes around the key)
const invalidJsonString = '{name: "John", age: 30}';
// Attempting to parse the invalid JSON string
const result = JSON.parse(invalidJsonString);
} catch (error) {
console.error('Error parsing JSON:', error.message); // Output: Error parsing JSON: Unexpected token n in JSON at position 1
}
4. Using JSON.parse() with a Reviver Function
The JSON.parse()
method also accepts an optional reviver
function. The reviver
function can be used to transform the data during the parsing process.
Example:
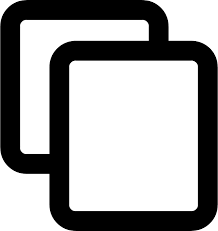
// A JSON string with date information
const jsonStringWithDate = '{"name": "John", "birthdate": "1990-01-01"}';
// Parsing the JSON string with a reviver function
const parsedData = JSON.parse(jsonStringWithDate, (key, value) => {
// If the key is "birthdate", convert the value into a Date object
if (key === 'birthdate') {
return new Date(value);
}
return value;
});
// Accessing the transformed data
console.log(parsedData.name); // Output: John
console.log(parsedData.birthdate instanceof Date); // Output: true
console.log(parsedData.birthdate); // Output: 1990-01-01T00:00:00.000Z
5. Common Parsing Issues
Here are some common issues that can arise while parsing JSON strings:
- Invalid JSON Syntax: JSON strings must be properly formatted with double quotes for keys and string values. For example,
{name: 'John'}
is invalid, but{"name": "John"}
is correct. - Unexpected Data Types: Ensure the data you're trying to parse follows the correct JSON format. For instance,
undefined
cannot be serialized into JSON. - Circular References: Circular references in objects cannot be serialized to JSON, and attempting to parse them will result in an error.
6. Conclusion
Parsing JSON strings is a fundamental part of working with data in JavaScript. By using the JSON.parse()
method, you can convert JSON-formatted strings into JavaScript objects or arrays. It’s important to handle errors gracefully and ensure that the JSON you're working with is properly formatted. With the optional reviver
function, you can even modify or transform the data during the parsing process.
Converting Objects to JSON
In JavaScript, it’s often necessary to convert JavaScript objects into a JSON (JavaScript Object Notation) string, especially when transmitting data over the network, such as sending data to a server via an HTTP request. The JSON.stringify()
method is used for this purpose.
1. JSON.stringify()
The JSON.stringify()
method takes a JavaScript object or array and converts it into a JSON-formatted string. This string can then be used for data exchange, storage, or transmission.
Example:
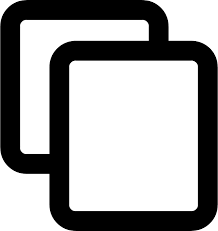
// A JavaScript object
const person = {
name: "John",
age: 30,
isEmployed: true
};
// Converting the object into a JSON string
const jsonString = JSON.stringify(person);
console.log(jsonString);
// Output: {"name":"John","age":30,"isEmployed":true}
2. Converting Arrays to JSON
Arrays in JavaScript can also be converted to JSON strings. The JSON.stringify()
method works similarly for arrays as it does for objects.
Example:
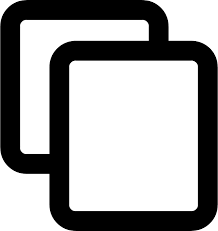
// A JavaScript array
const numbers = [1, 2, 3, 4, 5];
// Converting the array into a JSON string
const jsonArrayString = JSON.stringify(numbers);
console.log(jsonArrayString);
// Output: [1,2,3,4,5]
3. Converting Nested Objects to JSON
If your object contains nested objects or arrays, the JSON.stringify()
method recursively converts all nested properties as well.
Example:
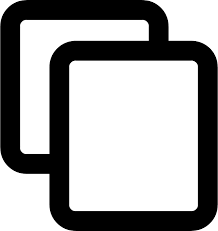
// A nested JavaScript object
const personWithAddress = {
name: "Alice",
age: 28,
address: {
street: "123 Main St",
city: "New York",
zip: "10001"
}
};
// Converting the nested object into a JSON string
const jsonStringWithAddress = JSON.stringify(personWithAddress);
console.log(jsonStringWithAddress);
// Output: {"name":"Alice","age":28,"address":{"street":"123 Main St","city":"New York","zip":"10001"}}
4. Using Replacer Function
The JSON.stringify()
method accepts an optional second argument called a "replacer." The replacer can be a function or an array that allows you to control which values should be included or excluded from the resulting JSON string.
Example of a Replacer Function:
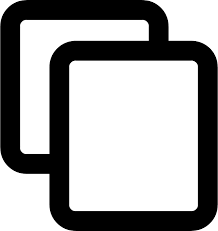
// A JavaScript object
const person = {
name: "John",
age: 30,
isEmployed: true,
password: "secret"
};
// A replacer function to exclude the "password" property
const replacer = (key, value) => {
if (key === "password") {
return undefined; // Exclude the "password" property
}
return value;
};
// Converting the object into a JSON string using the replacer function
const jsonStringWithoutPassword = JSON.stringify(person, replacer);
console.log(jsonStringWithoutPassword);
// Output: {"name":"John","age":30,"isEmployed":true}
Example of a Replacer Array:
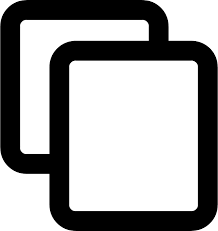
// A JavaScript object
const person = {
name: "John",
age: 30,
isEmployed: true,
password: "secret"
};
// A replacer array to include only the "name" and "age" properties
const replacerArray = ["name", "age"];
// Converting the object into a JSON string using the replacer array
const jsonStringWithReplacerArray = JSON.stringify(person, replacerArray);
console.log(jsonStringWithReplacerArray);
// Output: {"name":"John","age":30}
5. Handling Non-Serializable Values
Not all JavaScript values can be converted to JSON. For example, functions, undefined
, Symbol
, and circular references are not serializable.
- Functions: Functions will be excluded from the JSON string.
- undefined: If
undefined
is encountered in an object or array, it will be omitted in the final JSON string. - Symbols: Symbols are not included in the JSON string.
- Circular References: If there are circular references in an object,
JSON.stringify()
will throw an error.
Example of Excluding a Function:
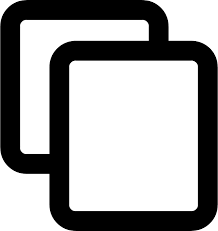
// A JavaScript object with a function
const person = {
name: "John",
greet: function() { return "Hello"; }
};
// Converting the object into a JSON string (the function is excluded)
const jsonString = JSON.stringify(person);
console.log(jsonString);
// Output: {"name":"John"} (the "greet" function is excluded)
6. Conclusion
Converting JavaScript objects to JSON strings using the JSON.stringify()
method is a common task when working with data exchange and storage. It’s important to be aware of non-serializable values and how to handle them with the optional replacer function. With JSON, you can easily convert complex JavaScript objects into a widely-used format for communication between systems.
Working with Date Objects
The Date
object in JavaScript is used to work with dates and times. It provides a variety of methods to create, manipulate, and format dates.
1. Creating a Date Object
You can create a new Date
object in several ways:
Creating a Date Object for the Current Date and Time:
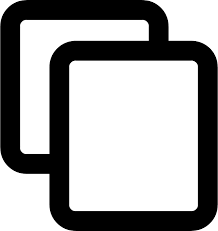
// Create a Date object with the current date and time
const currentDate = new Date();
console.log(currentDate);
// Output: Fri Jan 18 2025 12:30:00 GMT+0000 (UTC)
Creating a Date Object for a Specific Date:
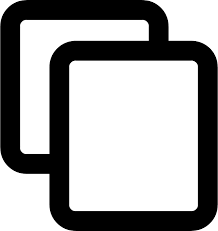
// Create a Date object for a specific date (Year, Month, Day, Hour, Minute, Second, Millisecond)
const specificDate = new Date(2025, 0, 18, 12, 30, 0); // January 18, 2025 at 12:30:00
console.log(specificDate);
// Output: Sat Jan 18 2025 12:30:00 GMT+0000 (UTC)
Creating a Date Object from a Date String:
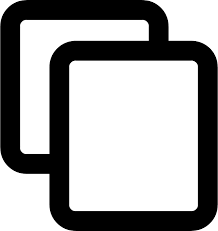
// Create a Date object from a date string
const dateString = new Date("2025-01-18T12:30:00Z");
console.log(dateString);
// Output: Sat Jan 18 2025 12:30:00 GMT+0000 (UTC)
2. Date Methods
Once you have a Date
object, you can use various methods to retrieve or manipulate date and time values.
Getting the Current Date and Time:
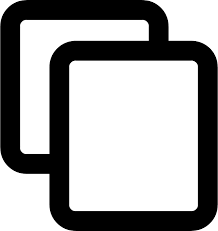
// Get the current date and time as a timestamp (milliseconds since Jan 1, 1970)
const timestamp = currentDate.getTime();
console.log(timestamp);
// Output: 1674045600000 (example timestamp)
// Get the current year, month, day, hours, minutes, seconds, and milliseconds
const year = currentDate.getFullYear();
const month = currentDate.getMonth(); // 0-11 (January is 0)
const day = currentDate.getDate();
const hours = currentDate.getHours();
const minutes = currentDate.getMinutes();
const seconds = currentDate.getSeconds();
const milliseconds = currentDate.getMilliseconds();
console.log(`Date: ${month + 1}/${day}/${year}, Time: ${hours}:${minutes}:${seconds}`);
Setting Date and Time:
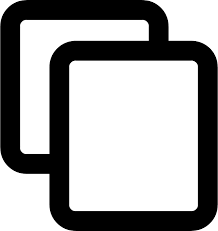
// Set the year, month, day, hours, minutes, seconds, and milliseconds
currentDate.setFullYear(2026);
currentDate.setMonth(5); // June (0-based)
currentDate.setDate(25);
currentDate.setHours(14);
currentDate.setMinutes(45);
currentDate.setSeconds(30);
console.log(currentDate);
// Output: Fri Jun 25 2026 14:45:30 GMT+0000 (UTC)
3. Date Formatting
JavaScript does not have built-in date formatting utilities, but the Date
object provides methods to convert the date to readable strings.
Using toLocaleDateString()
and toLocaleTimeString()
:
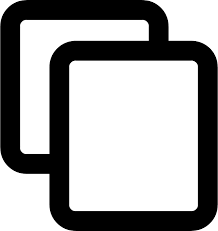
// Format the date and time using the browser's locale
const formattedDate = currentDate.toLocaleDateString("en-US");
const formattedTime = currentDate.toLocaleTimeString("en-US");
console.log(`Date: ${formattedDate}, Time: ${formattedTime}`);
// Output: Date: 1/18/2025, Time: 12:30:00 PM
Using toISOString()
to get an ISO 8601 string:
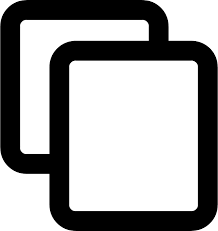
// Get the ISO string representation of the current date
const isoString = currentDate.toISOString();
console.log(isoString);
// Output: 2025-01-18T12:30:00.000Z
4. Working with Time Zones
JavaScript Date
objects are always in the local time zone of the user's browser. You can convert to different time zones using libraries like moment.js
or date-fns
, but by default, the date is represented in UTC when you use methods like toISOString()
or getUTCDate()
.
Getting UTC Date and Time:
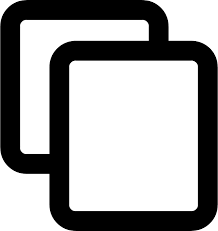
// Get UTC year, month, day, hours, minutes, seconds
const utcYear = currentDate.getUTCFullYear();
const utcMonth = currentDate.getUTCMonth();
const utcDay = currentDate.getUTCDate();
const utcHours = currentDate.getUTCHours();
const utcMinutes = currentDate.getUTCMinutes();
const utcSeconds = currentDate.getUTCSeconds();
console.log(`UTC Date: ${utcMonth + 1}/${utcDay}/${utcYear}, Time: ${utcHours}:${utcMinutes}:${utcSeconds}`);
5. Date Arithmetic
You can perform basic date arithmetic by manipulating timestamps (milliseconds). For example, you can calculate the difference between two dates, add days, subtract months, etc.
Calculating the Difference Between Two Dates:
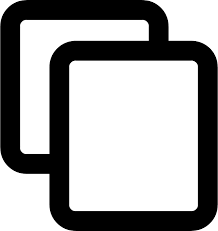
// Get two different date objects
const startDate = new Date("2025-01-01");
const endDate = new Date("2025-01-18");
// Get the difference in milliseconds
const differenceInMillis = endDate - startDate;
const differenceInDays = differenceInMillis / (1000 * 60 * 60 * 24);
console.log(`Difference: ${differenceInDays} days`);
// Output: Difference: 17 days
Adding Days to a Date:
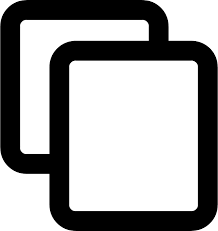
// Add 5 days to the current date
const futureDate = new Date(currentDate);
futureDate.setDate(currentDate.getDate() + 5);
console.log(futureDate);
// Output: (Current date + 5 days)
6. Conclusion
Working with Date
objects in JavaScript allows you to manipulate dates and times efficiently. While the built-in methods provide powerful functionality, for more advanced date manipulations or formatting, external libraries like moment.js
or date-fns
can be helpful.
Formatting and Manipulating Dates
In JavaScript, working with dates often involves formatting and manipulating them. The Date
object provides basic functionality, but sometimes you need more control over how dates are formatted or manipulated. Below, we explore different techniques for formatting and manipulating dates in JavaScript.
1. Formatting Dates
JavaScript provides several ways to format dates, either by using built-in methods or external libraries.
Using toLocaleDateString()
and toLocaleTimeString()
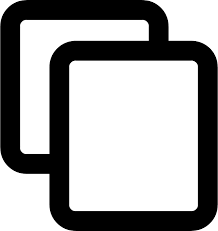
// Format the date and time according to the browser's locale
const date = new Date();
const formattedDate = date.toLocaleDateString('en-US'); // MM/DD/YYYY
const formattedTime = date.toLocaleTimeString('en-US'); // HH:MM:SS AM/PM
console.log(`Date: ${formattedDate}, Time: ${formattedTime}`);
// Output: Date: 1/18/2025, Time: 12:30:00 PM
Using toISOString()
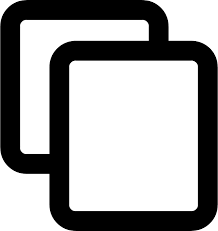
// Get an ISO 8601 formatted string (UTC)
const isoString = date.toISOString();
console.log(isoString);
// Output: 2025-01-18T12:30:00.000Z
Using Intl.DateTimeFormat()
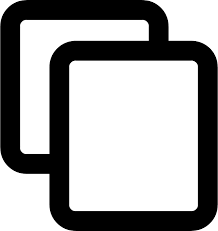
// Create a custom date formatter
const formatter = new Intl.DateTimeFormat('en-US', {
weekday: 'long',
year: 'numeric',
month: 'long',
day: 'numeric',
});
const formatted = formatter.format(date);
console.log(formatted);
// Output: Saturday, January 18, 2025
2. Manipulating Dates
Sometimes you need to manipulate a date, like adding or subtracting days, months, or years. Here are a few methods for manipulating dates.
Adding or Subtracting Days
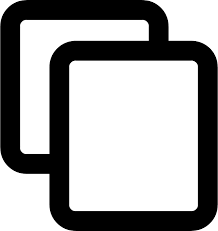
// Add 5 days to the current date
const currentDate = new Date();
currentDate.setDate(currentDate.getDate() + 5);
console.log(currentDate);
// Output: Date 5 days from current date
// Subtract 3 days from the current date
currentDate.setDate(currentDate.getDate() - 3);
console.log(currentDate);
// Output: Date 3 days before the adjusted date
Adding or Subtracting Months
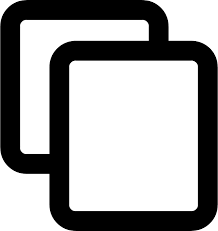
// Add 2 months to the current date
const futureDate = new Date();
futureDate.setMonth(futureDate.getMonth() + 2);
console.log(futureDate);
// Output: Date 2 months ahead
// Subtract 6 months from the current date
futureDate.setMonth(futureDate.getMonth() - 6);
console.log(futureDate);
// Output: Date 6 months behind
Adding or Subtracting Years
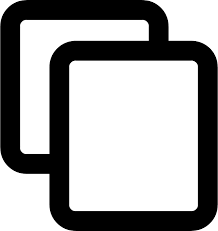
// Add 1 year to the current date
const nextYear = new Date();
nextYear.setFullYear(nextYear.getFullYear() + 1);
console.log(nextYear);
// Output: Date 1 year ahead
// Subtract 1 year from the current date
nextYear.setFullYear(nextYear.getFullYear() - 1);
console.log(nextYear);
// Output: Date 1 year before
3. Working with Timezones
JavaScript Date
objects are always in the local timezone of the user's machine. However, you can manipulate and format dates in different timezones using libraries like moment.js
or date-fns-tz
.
Getting UTC Date and Time
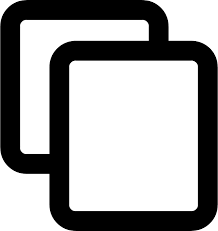
// Get the current date and time in UTC (Coordinated Universal Time)
const utcDate = new Date();
const utcYear = utcDate.getUTCFullYear();
const utcMonth = utcDate.getUTCMonth();
const utcDay = utcDate.getUTCDate();
const utcHours = utcDate.getUTCHours();
const utcMinutes = utcDate.getUTCMinutes();
const utcSeconds = utcDate.getUTCSeconds();
console.log(`UTC Date: ${utcMonth + 1}/${utcDay}/${utcYear}, Time: ${utcHours}:${utcMinutes}:${utcSeconds}`);
4. Date Arithmetic
In JavaScript, you can perform simple date arithmetic by manipulating timestamps (milliseconds). You can calculate the difference between two dates, or add or subtract time intervals (days, months, years).
Calculating the Difference Between Two Dates
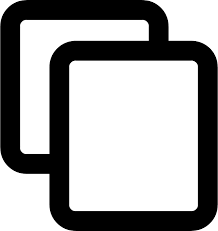
// Get two dates
const date1 = new Date("2025-01-01");
const date2 = new Date("2025-01-18");
// Calculate the difference in milliseconds
const diffInMillis = date2 - date1;
const diffInDays = diffInMillis / (1000 * 60 * 60 * 24); // Convert to days
console.log(`Difference: ${diffInDays} days`);
// Output: Difference: 17 days
Calculating Future or Past Dates
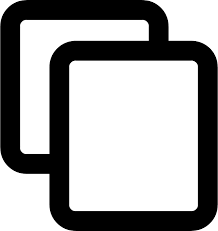
// Add 10 days to the current date
const futureDate = new Date();
futureDate.setDate(futureDate.getDate() + 10);
console.log(futureDate);
// Output: Date 10 days in the future
// Subtract 10 days from the current date
const pastDate = new Date();
pastDate.setDate(pastDate.getDate() - 10);
console.log(pastDate);
// Output: Date 10 days in the past
5. Working with Libraries for Advanced Date Manipulations
For more advanced date manipulations, you might want to use libraries like moment.js
or date-fns
, which provide a more powerful and consistent API for handling dates and times.
Using date-fns
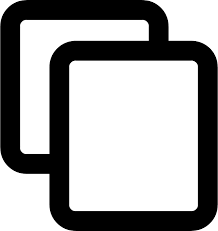
// Import the addDays function from date-fns
import { addDays } from 'date-fns';
// Add 5 days to the current date
const newDate = addDays(new Date(), 5);
console.log(newDate);
// Output: Date 5 days in the future
Using moment.js
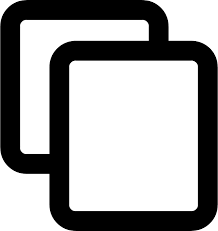
// Moment.js allows easy manipulation of dates
const moment = require('moment');
// Add 5 days to the current date
const futureDate = moment().add(5, 'days');
console.log(futureDate.format());
// Output: Date 5 days ahead
6. Conclusion
Formatting and manipulating dates in JavaScript is relatively simple with built-in methods, but for more advanced use cases, external libraries like moment.js
and date-fns
can offer additional functionality and a more intuitive syntax. Whether you need to format dates, perform arithmetic, or work with time zones, JavaScript provides the tools to help you manage and manipulate date and time values efficiently.
Code Readability
Code readability is the ease with which a human reader can understand the purpose, logic, and flow of the code. Writing readable code is crucial for collaboration, maintenance, and debugging. In this section, we will explore key principles for improving the readability of your code.
1. Use Meaningful Variable and Function Names
Choose descriptive and meaningful names for variables, functions, and classes. Avoid using short, cryptic names, and aim for clarity. A good name should describe the purpose of the element it represents.
Example: Bad Naming
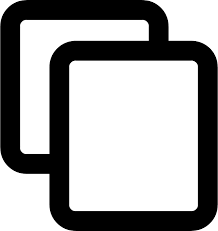
// Bad variable names
let a = 10;
let b = 20;
function c(x, y) {
return x + y;
}
console.log(c(a, b));
Example: Good Naming
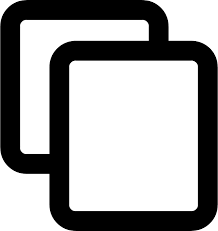
// Good variable and function names
let price = 10;
let tax = 20;
function calculateTotal(price, tax) {
return price + tax;
}
console.log(calculateTotal(price, tax));
2. Keep Functions Small and Focused
A function should do one thing and do it well. Break up large functions into smaller, focused ones. This makes your code easier to understand, test, and maintain.
Example: Large Function
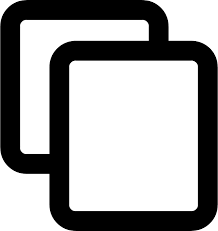
// Large function
function processOrder(order) {
// Validate order
if (!order.item || !order.quantity) {
console.error("Invalid order");
return;
}
// Calculate total
let total = order.quantity * order.item.price;
// Apply discount
if (order.discount) {
total -= order.discount;
}
// Print receipt
console.log(`Order processed: Total = $${total}`);
}
Example: Small, Focused Functions
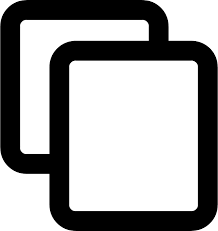
// Small, focused functions
function validateOrder(order) {
if (!order.item || !order.quantity) {
console.error("Invalid order");
return false;
}
return true;
}
function calculateTotal(order) {
return order.quantity * order.item.price;
}
function applyDiscount(total, discount) {
return discount ? total - discount : total;
}
function processOrder(order) {
if (!validateOrder(order)) return;
let total = calculateTotal(order);
total = applyDiscount(total, order.discount);
console.log(`Order processed: Total = $${total}`);
}
3. Use Consistent Indentation
Consistent indentation is key to making your code visually appealing and easy to follow. Standardize your indentation style (e.g., 2 spaces, 4 spaces, or tabs) and apply it consistently throughout your codebase.
Example: Inconsistent Indentation
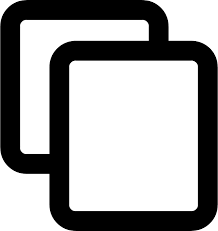
function example() {
let x = 10;
if (x > 5) {
console.log('Greater than 5');
}
}
Example: Consistent Indentation
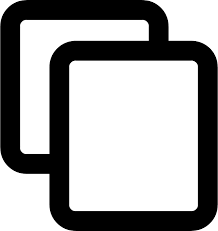
function example() {
let x = 10;
if (x > 5) {
console.log('Greater than 5');
}
}
4. Write Comments and Documentation
Use comments to explain the purpose of complex code or logic. Comments should be used to clarify "why" something is done, rather than "what" is being done, as the latter should be clear from the code itself.
Example: Bad Commenting
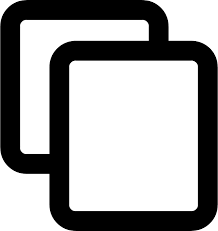
// Add 10 to x
x = x + 10;
Example: Good Commenting
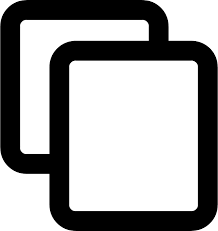
// Increase x by 10 to apply the discount
x = x + 10;
5. Avoid Nested Loops and Conditionals
Deeply nested loops and conditionals can make your code harder to read and maintain. Try to flatten the logic by using early returns, breaking down functions, or using helper functions.
Example: Nested Loops
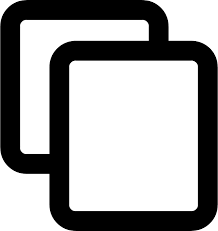
function checkItems(items) {
for (let i = 0; i < items.length; i++) {
for (let j = 0; j < items[i].reviews.length; j++) {
if (items[i].reviews[j].rating < 3) {
console.log("Low rating found");
return;
}
}
}
}
Example: Flattened Logic
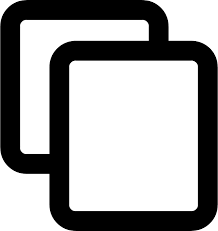
function checkItems(items) {
for (let i = 0; i < items.length; i++) {
if (hasLowRating(items[i])) {
console.log("Low rating found");
return;
}
}
}
function hasLowRating(item) {
for (let j = 0; j < item.reviews.length; j++) {
if (item.reviews[j].rating < 3) {
return true;
}
}
return false;
}
6. Use Descriptive Error Messages
When throwing errors, provide descriptive messages that help others (or your future self) understand what went wrong and where. Avoid generic error messages.
Example: Bad Error Message
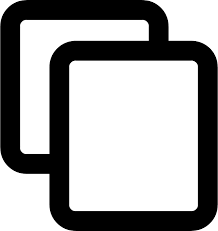
throw new Error("Something went wrong");
Example: Descriptive Error Message
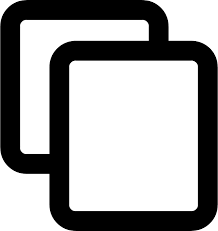
throw new Error("Failed to fetch user data: User ID is missing");
7. Maintain a Consistent Style Guide
Adopting a consistent coding style guide (e.g., Airbnb, Google, or StandardJS) ensures that your code remains uniform across different developers and projects. This makes it easier to read, understand, and modify code in a collaborative setting.
8. Conclusion
Writing readable code is not only about making your code look nice but also about making it easier for others to understand and maintain. By following best practices like using meaningful names, keeping functions small, and maintaining consistent indentation, you’ll improve the overall quality of your code and make collaboration more effective.
Avoiding Common Pitfalls
In JavaScript, there are several common pitfalls that can lead to bugs, performance issues, or hard-to-maintain code. Being aware of these pitfalls and knowing how to avoid them can greatly improve the quality and reliability of your code. In this section, we will explore some of the most common mistakes and how to avoid them.
1. Improper Use of the `this` Keyword
The `this` keyword in JavaScript can be tricky, as its value depends on the context in which it’s used. Common mistakes include misinterpreting the value of `this` inside functions, especially when they are used as callbacks or event handlers.
Example: `this` in Regular Functions
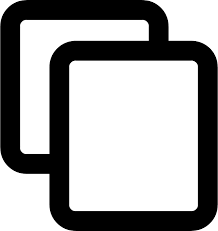
function showContext() {
console.log(this);
}
showContext(); // 'this' will refer to the global object or undefined in strict mode
Solution: Use Arrow Functions for Lexical `this`
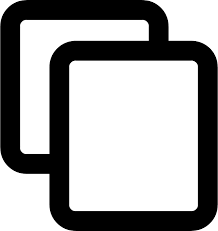
const showContext = () => {
console.log(this); // 'this' refers to the enclosing context
};
showContext();
2. Forgetting to Bind `this` in Event Handlers
When using methods in event handlers, `this` may not refer to the object you expect. Binding the function context to the correct object can solve this problem.
Example: Missing Bind
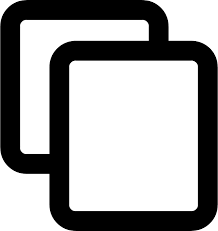
class Counter {
constructor() {
this.count = 0;
document.getElementById('increment').addEventListener('click', this.increment);
}
increment() {
this.count++;
console.log(this.count); // 'this' is undefined or refers to the DOM element
}
}
const counter = new Counter();
Solution: Bind `this`
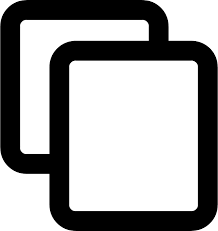
class Counter {
constructor() {
this.count = 0;
document.getElementById('increment').addEventListener('click', this.increment.bind(this));
}
increment() {
this.count++;
console.log(this.count); // 'this' correctly refers to the Counter instance
}
}
const counter = new Counter();
3. Using `==` Instead of `===` for Comparison
In JavaScript, the `==` operator performs type coercion, which can lead to unexpected results. Always use `===` to ensure strict comparison without type conversion.
Example: Using `==`
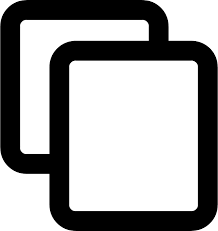
console.log(1 == '1'); // true, because the string '1' is coerced to a number
Solution: Use `===`
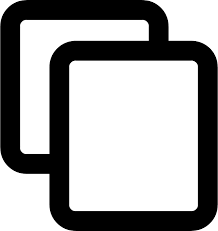
console.log(1 === '1'); // false, because the types are different
4. Modifying Objects or Arrays While Iterating
Modifying an object or array while iterating over it can lead to unexpected behavior or skipped elements. This is a common mistake when using loops.
Example: Modifying Array While Iterating
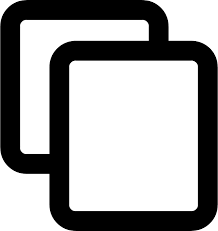
let arr = [1, 2, 3, 4, 5];
for (let i = 0; i < arr.length; i++) {
if (arr[i] === 3) {
arr.splice(i, 1); // Modifies the array while iterating
}
}
console.log(arr); // [1, 2, 4, 5], elements are skipped
Solution: Create a Copy or Use a Different Approach
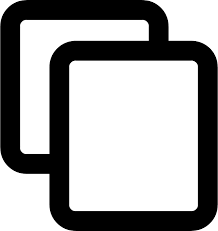
let arr = [1, 2, 3, 4, 5];
arr = arr.filter(item => item !== 3); // Use filter to remove items safely
console.log(arr); // [1, 2, 4, 5]
5. Not Handling Asynchronous Code Properly
Asynchronous code, such as using `setTimeout`, `Promises`, and `async/await`, can be tricky. Not handling asynchronous code properly can lead to issues like unhandled promises or race conditions.
Example: Not Handling Promises Properly
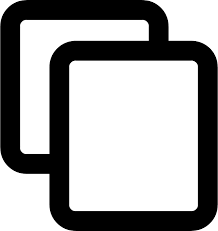
let fetchData = fetch('https://api.example.com/data');
console.log(fetchData); // Promise is logged, but data is not handled
Solution: Use `.then()` or `async/await` to Handle Promises
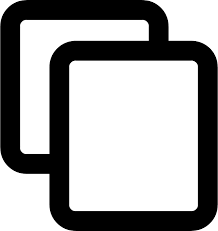
// Using .then()
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
// Using async/await
async function getData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.log(error);
}
}
getData();
6. Not Handling Edge Cases
Forgetting to handle edge cases in your code can lead to unexpected behavior, crashes, or incorrect results. Always consider edge cases and provide fallbacks or validations where necessary.
Example: Not Handling Edge Case
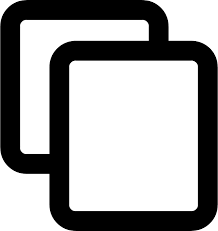
function divide(a, b) {
return a / b;
}
console.log(divide(10, 0)); // Infinity, no validation for division by zero
Solution: Handle Edge Case
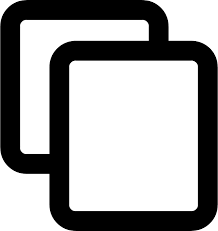
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
}
console.log(divide(10, 0)); // Throws error
7. Overusing Global Variables
Global variables can lead to unintended side effects, especially when working in larger applications. Limit the use of global variables and encapsulate your code in functions or modules when possible.
Example: Overusing Global Variables
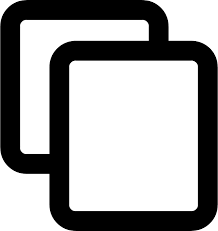
let globalVar = 10;
function updateGlobal() {
globalVar = 20; // Modifying a global variable
}
updateGlobal();
console.log(globalVar); // 20, unexpected side effect
Solution: Encapsulate in Functions
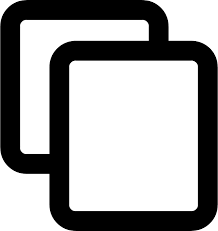
function createCounter() {
let count = 0;
return {
increment() {
count++;
console.log(count);
},
decrement() {
count--;
console.log(count);
}
};
}
const counter = createCounter();
counter.increment(); // 1
counter.increment(); // 2
8. Conclusion
By avoiding these common pitfalls and following best practices, you can write more reliable, maintainable, and efficient JavaScript code. Keep these lessons in mind to prevent bugs and performance issues, and always strive to write clean, understandable code.
Introduction to jQuery
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, and animation. jQuery is designed to make it much easier to use JavaScript on your website by providing a simpler API for things that would otherwise require long and complex code. In this section, we will introduce you to jQuery and some of its core features.
What is jQuery?
jQuery is a JavaScript library that makes it easier to interact with HTML elements, handle events, perform animations, and make AJAX requests. It was created in 2006 by John Resig, and it quickly became one of the most popular JavaScript libraries due to its simplicity and cross-browser compatibility. jQuery allows you to write less code to achieve more functionality, making it a valuable tool for web developers.
Getting Started with jQuery
To use jQuery, you need to include the jQuery library in your HTML document. You can either download the library and host it yourself or use a CDN (Content Delivery Network) to link to the library.
Example: Including jQuery via CDN
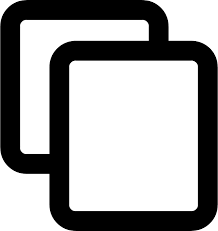
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once the jQuery library is included in your HTML file, you can start using jQuery functions within a <script> tag or an external JavaScript file.
Basic Syntax of jQuery
The basic syntax of jQuery consists of the following structure:
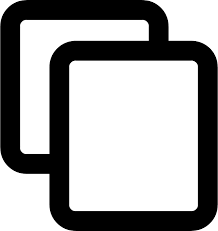
$(selector).action();
Here, selector is used to select an HTML element, and action refers to the jQuery action you want to perform on that element (e.g., changing its style, adding a click event, etc.).
Example: Selecting an Element and Changing Its Text
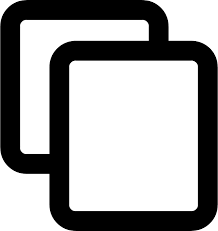
$(document).ready(function() {
$("button").click(function() {
$("p").text("Hello, jQuery!");
});
});
In this example, when the button is clicked, the text of the <p> element is changed to "Hello, jQuery!".
jQuery Selectors
jQuery selectors are used to select HTML elements and manipulate them. They are similar to CSS selectors, but with added functionality. The most common types of jQuery selectors are:
- Element Selector: Selects all elements of a specific type (e.g., <code>$( "p" )</code> selects all <p> elements).
- ID Selector: Selects an element with a specific ID (e.g., <code>$( "#myDiv" )</code> selects the element with ID "myDiv").
- Class Selector: Selects all elements with a specific class (e.g., <code>$( ".myClass" )</code> selects all elements with the class "myClass").
- Attribute Selector: Selects elements with a specific attribute (e.g., <code>$("[type='text']")</code> selects all <input> elements with the type "text").
Common jQuery Actions
jQuery makes it easy to perform a wide range of actions. Here are some of the most common ones:
- Manipulating HTML content: Use the <code>.html()</code> and <code>.text()</code> methods to get or set the HTML or text content of elements.
- Manipulating CSS: Use the <code>.css()</code> method to change the CSS styles of elements.
- Event Handling: Use methods like <code>.click()</code>, <code>.hover()</code>, and <code>.on()</code> to handle events on elements.
- Animation: Use methods like <code>.fadeIn()</code>, <code>.fadeOut()</code>, <code>.slideUp()</code>, and <code>.slideDown()</code> to create animations.
- AJAX Requests: Use the <code>$.ajax()</code> method to make asynchronous HTTP requests (AJAX) to fetch or send data from or to the server.
Example: jQuery Event Handling
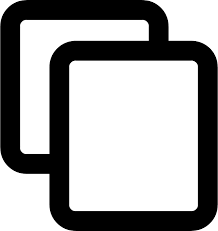
$(document).ready(function() {
$("button").click(function() {
$("p").toggle(); // Toggles the visibility of the <p> element
});
});
Advantages of jQuery
- Cross-browser compatibility: jQuery handles many browser inconsistencies, so you don’t have to worry about them when writing your code.
- Smaller code base: jQuery allows you to accomplish more tasks with less code compared to vanilla JavaScript.
- Rich set of features: jQuery provides powerful methods for DOM manipulation, animation, event handling, and AJAX.
- Large community and plugins: jQuery has a vast ecosystem of plugins that you can use to add functionality to your website.
When to Use jQuery
Although jQuery is a powerful library, modern JavaScript (ES6+) has introduced many features that overlap with jQuery. For example, with native JavaScript, you can easily select elements, manipulate the DOM, and handle events without the need for jQuery. Therefore, it's important to evaluate whether you need the extra functionality that jQuery provides or if native JavaScript will suffice for your project.
Conclusion
jQuery is a useful tool that can help simplify JavaScript tasks, particularly when working with older browsers or needing to accomplish tasks with less code. However, with the rise of modern JavaScript features and frameworks, using jQuery may not always be necessary in new projects. Still, understanding jQuery can be valuable when maintaining legacy code or working with existing libraries that depend on it.
Overview of Modern Frameworks (React, Vue.js, Angular)
Modern JavaScript frameworks like React, Vue.js, and Angular have become essential tools for building dynamic, single-page web applications. These frameworks provide developers with powerful tools for structuring and managing the complex interactions within a web application, making it easier to develop, maintain, and scale. In this section, we will give a brief overview of each of these frameworks, highlighting their key features and differences.
React
React is a JavaScript library for building user interfaces, developed and maintained by Facebook. It is widely used for creating single-page applications (SPAs) and allows developers to build reusable UI components. React uses a declarative approach, which means you describe what the UI should look like based on the state, and React takes care of updating the DOM when the state changes. One of the key features of React is its virtual DOM, which improves performance by minimizing direct manipulation of the actual DOM.
Key Features of React:
- Component-based architecture: React applications are built using reusable components that encapsulate both logic and UI.
- Virtual DOM: React uses a virtual DOM to optimize performance by reducing the number of direct DOM manipulations.
- Unidirectional data flow: Data flows in one direction, making it easier to manage state in large applications.
- JSX (JavaScript XML): JSX allows you to write HTML-like syntax in JavaScript, making it easier to define components.
Example: React Component
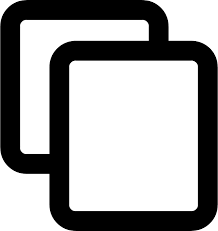
import React from 'react';
class App extends React.Component {
render() {
return <h1>Hello, React!</h1>
}
}
export default App;
Vue.js
Vue.js is a progressive JavaScript framework for building user interfaces. Unlike React, which is more focused on the view layer, Vue.js is a full-fledged framework that provides all the tools needed to build modern web applications. Vue.js is designed to be incrementally adoptable, meaning you can use it as a simple library to enhance specific parts of your application or scale up to a full-fledged SPA framework.
Key Features of Vue.js:
- Reactive data binding: Vue.js automatically updates the DOM whenever the underlying data changes.
- Component-based architecture: Like React, Vue.js uses a component-based approach to build applications.
- Directives: Vue.js provides special syntax (directives) like <code>v-bind</code> and <code>v-for</code> for DOM manipulation.
- Single-file components: Vue components are often written in a single file that includes HTML, CSS, and JavaScript.
Example: Vue Component
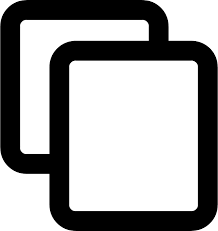
<template>
<h1>Hello, Vue.js!</h1>
</template>
<script>
export default {
name: 'App'
}
</script>
Angular
Angular is a comprehensive, full-featured JavaScript framework developed by Google. It is designed for building large-scale, complex web applications. Angular uses a component-based architecture and provides powerful tools for handling form validation, HTTP requests, routing, and more. It uses TypeScript as its primary language, which is a superset of JavaScript that adds static typing and other features to help developers write more maintainable code.
Key Features of Angular:
- Two-way data binding: Angular synchronizes the model and the view, making it easier to keep the UI in sync with data.
- Dependency injection: Angular provides a robust dependency injection system to manage dependencies and improve testability.
- RxJS (Reactive Extensions for JavaScript): Angular uses RxJS for handling asynchronous operations and events using observables.
- TypeScript: Angular is built with TypeScript, which provides static typing and object-oriented features.
Example: Angular Component
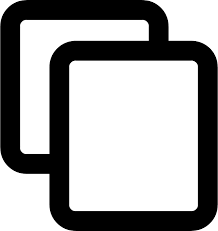
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<h1>Hello, Angular!</h1>',
})
export class AppComponent {}
Comparison of React, Vue.js, and Angular
While all three frameworks have their strengths, they differ in their approach and usage:
- React is best for building dynamic, reusable components with a focus on the view layer. It is ideal for projects where flexibility and performance are key.
- Vue.js is great for developers who want a framework that is easy to integrate into existing projects or for building full-fledged applications with minimal setup.
- Angular is suited for large-scale, enterprise-level applications that require a full-fledged framework with built-in tools for routing, state management, and HTTP requests.
Conclusion
React, Vue.js, and Angular each offer unique features and advantages, depending on the needs of your project. React is great for building UI components with flexibility, Vue.js is perfect for both small and large applications with its simplicity, and Angular is a robust framework suited for large-scale applications requiring a structured approach. As web development continues to evolve, understanding these frameworks will help you choose the right one for your specific use case.
Avoiding Memory Leaks
Memory leaks occur when a program or application consumes memory but fails to release it when it's no longer needed, leading to reduced performance or application crashes. In JavaScript, memory leaks can happen due to various reasons such as unattached event listeners, large unused data structures, or references to objects that are no longer necessary. In this section, we’ll cover common causes of memory leaks in JavaScript and how to avoid them to ensure optimal performance of your applications.
Common Causes of Memory Leaks
- Unremoved Event Listeners: If event listeners are attached to DOM elements but not removed when no longer needed, they can cause memory leaks by maintaining references to those DOM elements.
- Global Variables: Accidentally declaring variables globally can cause memory leaks because they remain in memory for the lifetime of the application.
- Detached DOM Elements: If elements are removed from the DOM but still referenced by JavaScript, they cannot be garbage collected, leading to memory leaks.
- Closures: While closures are powerful in JavaScript, they can also cause memory leaks if they hold references to large data structures or DOM elements that are no longer needed.
How to Avoid Memory Leaks
1. Remove Event Listeners
Always remove event listeners when they are no longer needed. For example, when using addEventListener
to add events, ensure that you call removeEventListener
when the event is no longer necessary.
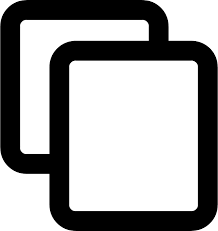
document.getElementById('myButton').addEventListener('click', myFunction);
// Later, remove the event listener to prevent memory leaks
document.getElementById('myButton').removeEventListener('click', myFunction);
2. Avoid Unnecessary Global Variables
Try to avoid declaring variables in the global scope. Use let
and const
to define variables within a block scope, and avoid attaching properties to the window
object unless absolutely necessary.
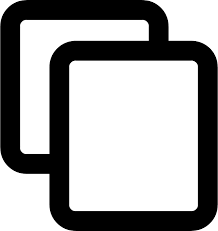
// Avoid creating global variables
var globalVar = 'This is a global variable'; // Can cause memory leaks
// Use block-scoped variables
let localVar = 'This is a local variable'; // Safer and more efficient
3. Clean Up Detached DOM Elements
When removing DOM elements, ensure that there are no remaining references to them in your JavaScript code. Detached DOM elements that are still referenced can’t be garbage collected, causing memory leaks.
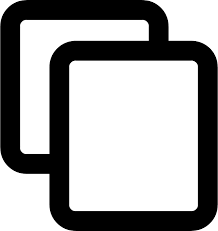
// Remove the element from the DOM
let element = document.getElementById('myDiv');
element.parentNode.removeChild(element);
// Ensure no references to the element remain
element = null;
4. Be Careful with Closures
Closures can hold onto variables even after the outer function has finished execution. Be mindful of closures that retain references to large objects or DOM elements and avoid creating circular references.
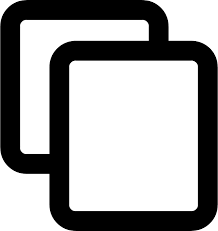
function createClosure() {
let largeData = new Array(1000000); // Simulating a large data structure
return function() {
// This closure still has access to 'largeData', even after the function has finished
console.log(largeData);
};
}
const myClosure = createClosure();
// When 'myClosure' is no longer needed, ensure it does not hold onto largeData
5. Use Weak References for Caching
Instead of using regular objects to store caches, you can use WeakMap
or WeakSet
, which allow objects to be garbage collected when they are no longer referenced.
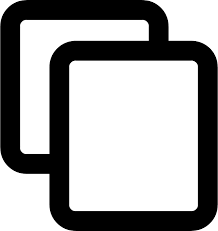
let myCache = new WeakMap();
let obj = {};
myCache.set(obj, 'some data');
// If 'obj' is no longer referenced, it will be garbage collected
Tools for Monitoring Memory Leaks
There are various tools and browser developer tools that can help you detect and monitor memory leaks in your application:
- Chrome DevTools: The Memory panel in Chrome DevTools allows you to take heap snapshots and track memory usage over time.
- Heap Profiler: Heap profiling tools can help you analyze memory allocation and identify objects that are using excessive memory.
- JSHint or ESLint: Static code analysis tools like JSHint or ESLint can help you catch potential memory leaks caused by global variables or unused references.
Conclusion
Memory leaks can severely impact the performance of your web applications, but they are often preventable with careful coding practices. By ensuring that event listeners are removed, avoiding global variables, cleaning up detached DOM elements, and being mindful of closures, you can significantly reduce the risk of memory leaks. Additionally, using tools like Chrome DevTools to monitor memory usage will help you identify and fix memory leaks early in your development process.
Efficient DOM Manipulation
DOM (Document Object Model) manipulation is a common task in JavaScript when working with web pages. However, inefficient DOM manipulation can lead to performance problems, especially in complex applications. Manipulating the DOM repeatedly can be slow, leading to jank and poor user experience. In this section, we will discuss strategies for efficient DOM manipulation to help you create faster and more responsive applications.
Why DOM Manipulation Can Be Slow
Each time you modify the DOM, the browser needs to recalculate styles, reflow the page layout, and repaint the affected elements. If these changes are made repeatedly or inefficiently, it can significantly affect performance.
Best Practices for Efficient DOM Manipulation
1. Minimize Reflows and Repaints
Reflows and repaints occur when the layout or appearance of the page needs to be recalculated. They are expensive operations, and performing them multiple times can degrade performance. To minimize reflows and repaints, try to:
- Batch DOM updates: Make multiple changes to the DOM in a single operation instead of doing it one by one.
- Modify elements off-screen first, then append them to the DOM to avoid unnecessary reflows.
- Read layout properties (e.g.,
offsetHeight
,offsetWidth
) before making changes to avoid triggering reflow.
Example: Batched DOM Updates
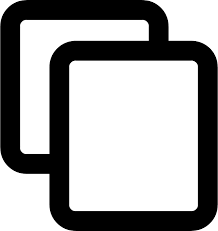
// Avoid frequent reflows by batching updates
const element = document.getElementById('myDiv');
// Bad approach (triggers reflow each time)
element.style.width = '200px';
element.style.height = '100px';
element.innerHTML = 'Hello World!';
// Good approach (batch the updates)
element.style.cssText = 'width: 200px; height: 100px;';
element.innerHTML = 'Hello World!';
2. Use DocumentFragments for Multiple Changes
A DocumentFragment
is a lightweight container for DOM elements that is not part of the live DOM. You can make changes to a fragment, and then append it all at once to the actual DOM. This reduces the number of reflows and repaints.
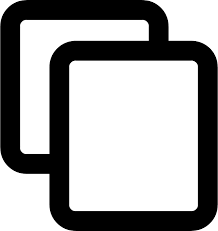
// Create a DocumentFragment
const fragment = document.createDocumentFragment();
const ul = document.getElementById('myList');
// Add items to the fragment
for (let i = 0; i < 100; i++) {
const li = document.createElement('li');
li.textContent = `Item ${i}`;
fragment.appendChild(li);
}
// Append the fragment to the DOM
ul.appendChild(fragment);
3. Use Event Delegation
Instead of attaching event listeners to every individual element, use event delegation. This approach involves adding a single event listener to a parent element and using event propagation to catch events on child elements. It improves performance, especially when dealing with a large number of elements.
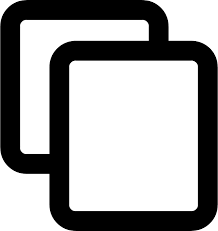
// Instead of attaching an event listener to each button, use event delegation
document.getElementById('parentDiv').addEventListener('click', function(event) {
if (event.target && event.target.matches('button')) {
alert('Button clicked!');
}
});
4. Avoid Using innerHTML
When Possible
While innerHTML
is quick for adding HTML content, it can be dangerous and inefficient. It can re-parse the entire HTML content and cause performance issues, especially when used frequently. Instead, consider using methods like createElement
, appendChild
, or textContent
for safer and more efficient DOM manipulation.
5. Limit DOM Queries
DOM queries like querySelector
or getElementById
can be slow if called repeatedly. If you need to access an element multiple times, it’s better to store the reference to that element in a variable.
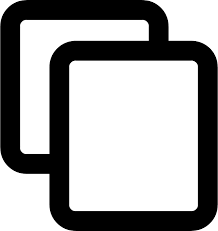
// Instead of querying the DOM multiple times
document.querySelector('#myDiv').style.color = 'red';
document.querySelector('#myDiv').style.fontSize = '16px';
// Store the element in a variable
const myDiv = document.querySelector('#myDiv');
myDiv.style.color = 'red';
myDiv.style.fontSize = '16px';
Additional Tips for Efficient DOM Manipulation
- Use CSS Transitions or Animations: Instead of manually manipulating styles with JavaScript, use CSS transitions or animations for smoother and more efficient visual effects.
- Limit DOM Traversal: Avoid unnecessary DOM traversal operations, such as traversing up and down the DOM tree, as they can be expensive.
- Use CSS Classes for Styling: Apply CSS classes to elements rather than directly manipulating the style properties through JavaScript. This ensures better separation of concerns and more efficient reflows.
Conclusion
Efficient DOM manipulation is essential for creating fast and responsive web applications. By minimizing reflows and repaints, using techniques like event delegation and DocumentFragment
, and avoiding inefficient methods like innerHTML
, you can ensure that your web pages perform well even with complex content. Following these best practices will result in a smoother user experience and better overall performance.
Content Security Policy (CSP)
Content Security Policy (CSP) is a security feature that helps prevent a variety of attacks, including Cross-Site Scripting (XSS) and data injection attacks. CSP allows web developers to define which dynamic resources are allowed to load and execute on their website. By enforcing this policy, CSP can significantly reduce the risk of malicious content being injected into your web applications.
What is CSP?
A Content Security Policy (CSP) is a set of rules defined by the server that determines which resources the browser is allowed to load for a particular web page. It is communicated via a HTTP header or a <meta>
tag and enables web developers to declare which domains can serve specific types of content, such as scripts, styles, images, etc.
By restricting where content can be loaded from, CSP helps prevent malicious content from external sources, which can be used to execute attacks like XSS. For example, if your site only loads scripts from trusted domains, CSP can block any third-party scripts from being executed.
How CSP Works
CSP works by specifying a policy for different types of resources (e.g., scripts, styles, images) that can be loaded by the browser. The policy is defined in the Content-Security-Policy
HTTP header or in a <meta>
tag within the HTML document.
Here’s a basic example of how CSP works:
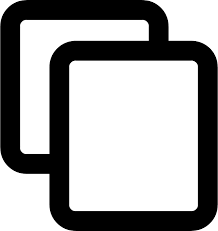
// Content-Security-Policy HTTP header
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted-cdn.com;
In this example, the policy allows content to be loaded from the same origin (denoted by 'self'
) and allows scripts to be loaded from https://trusted-cdn.com
.
CSP Directives
CSP directives specify the types of resources and where they can be loaded from. Here are some of the most common directives:
- default-src: Specifies the default source for all content types if no other directives match (e.g.,
default-src 'self'
allows only content from the same origin). - script-src: Specifies the allowed sources for JavaScript. It’s important to restrict this to trusted sources to prevent XSS attacks.
- style-src: Defines the allowed sources for CSS stylesheets.
- img-src: Specifies the allowed sources for images.
- connect-src: Defines the allowed sources for XMLHttpRequest, WebSocket, and EventSource connections.
- frame-src: Restricts the sources of frames (e.g., iframes) that can be embedded within your web page.
- child-src: Specifies valid sources for web workers and embedded content.
- font-src: Specifies the allowed sources for fonts.
- object-src: Specifies allowed sources for
<object>
,<embed>
, and<applet>
elements.
Example CSP Header
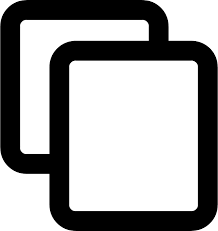
// A stricter Content-Security-Policy header example:
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted-cdn.com; style-src 'self'; img-src 'self' data:;
In this example, the policy restricts:
- All content to be loaded from the same origin (
'self'
). - Scripts to be loaded only from the same origin and
https://trusted-cdn.com
. - Styles to be loaded only from the same origin.
- Images to be loaded only from the same origin, and also allows images embedded as data URIs (
data:
).
Using CSP with <meta>
Tag
In addition to the HTTP header, you can also define a CSP policy using the <meta>
tag in your HTML. The <meta>
tag is useful for situations where you do not have control over the HTTP headers, such as when working with a static website.
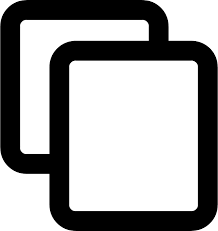
Reporting Violations
CSP provides a feature called reporting that allows you to receive notifications when a policy violation occurs. You can include a report-uri
or report-to
directive in your CSP header to define the URL where violation reports should be sent.
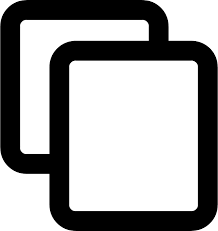
// Example of including a reporting URL
Content-Security-Policy: default-src 'self'; report-uri /csp-violation-report-endpoint;
When a violation occurs, the browser will send a JSON payload to the specified report URL.
Why Use CSP?
CSP is an essential tool for preventing security vulnerabilities like XSS and data injection attacks. By defining strict rules about where content can come from, CSP minimizes the risk of malicious scripts running on your website. Additionally, CSP helps reduce the impact of attacks by blocking the execution of unauthorized scripts, even if an attacker manages to inject them into your application.
Challenges and Considerations
While CSP is an effective security measure, it can also introduce challenges. For example:
- Compatibility issues: Some older browsers may not support CSP, and certain features may not work correctly in these browsers.
- False positives: If the policy is too strict, legitimate resources such as third-party scripts or analytics tools may be blocked.
- Maintainability: As your web application evolves, managing and updating the CSP policy can become complex, especially if you rely on third-party services.
Conclusion
Content Security Policy (CSP) is a powerful tool to protect your website from various attacks, especially Cross-Site Scripting (XSS). By defining strict rules about where resources can be loaded from, CSP helps prevent malicious code from executing in your users' browsers. However, it’s important to carefully configure and maintain your CSP policy to avoid compatibility issues and false positives. With the right approach, CSP can significantly improve the security of your web applications.
Singleton Pattern
The Singleton Pattern is a design pattern that ensures a class has only one instance and provides a global point of access to that instance. This pattern is used when you need to restrict the instantiation of a class to only one object, and that object should be used throughout the application.
What is the Singleton Pattern?
In software design, the Singleton Pattern is a creational pattern that limits the number of instances of a class to one. It is often used when a system needs a single shared resource, such as a database connection, logging system, or configuration manager, and it’s important to maintain a single instance of that resource throughout the application’s lifetime.
How the Singleton Pattern Works
The Singleton Pattern involves creating a class that has a static variable to hold the single instance. The class exposes a static method to access the instance. If the instance does not exist, the static method creates and returns the instance; otherwise, it returns the existing instance.
Example: Singleton Pattern in JavaScript
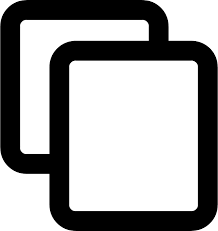
class Singleton {
constructor() {
if (!Singleton.instance) {
Singleton.instance = this; // Assign the instance if it doesn't exist
}
return Singleton.instance; // Return the existing instance
}
// Example method
getRandomNumber() {
return Math.random();
}
}
// Usage
const instance1 = new Singleton();
const instance2 = new Singleton();
console.log(instance1 === instance2); // true, both instances are the same
In this example, the Singleton
class ensures that only one instance of the class can be created. Every time a new instance is created, the constructor checks if an instance already exists, and if it does, it returns the existing instance. Otherwise, it creates and assigns the instance.
Advantages of the Singleton Pattern
- Global access point: The Singleton Pattern provides a global point of access to the instance, which is useful when you need to share data or resources across different parts of the application.
- Controlled access to resources: The pattern ensures that only one instance of a resource (e.g., a database connection or configuration) is created, which can help with resource management and efficiency.
- Lazy initialization: The instance is created only when it is needed, which can improve performance and reduce memory usage.
Disadvantages of the Singleton Pattern
- Global state: The Singleton Pattern introduces global state into your application, which can make it harder to maintain and test because the state can be modified from any part of the program.
- Difficulty in testing: Since the Singleton Pattern ensures there is only one instance, it can make unit testing difficult because it’s harder to mock or replace the instance for testing purposes.
- Potential for misuse: If not used properly, the Singleton Pattern can lead to tightly coupled code and over-reliance on global state, which can make your application harder to scale and maintain.
When to Use the Singleton Pattern
The Singleton Pattern is best used in situations where:
- Only one instance of a class is needed, such as a logging system, configuration manager, or database connection.
- The global state needs to be controlled and accessed consistently across different parts of the application.
- The resource needs to be lazily initialized to save on performance or memory.
Conclusion
The Singleton Pattern is a useful design pattern that ensures a class has only one instance throughout the application. It is particularly useful when managing shared resources or data. However, it’s important to use the pattern judiciously, as it can introduce global state into your application and make testing and maintenance more challenging. Understanding when and how to use the Singleton Pattern effectively can help improve the structure and efficiency of your application.
Factory Pattern
The Factory Pattern is a creational design pattern used to create objects in a way that allows for flexibility and extensibility. Instead of calling a constructor directly to create an object, a factory method is used to instantiate the object. This pattern is particularly useful when you have a superclass that defines a common interface, but the instantiation of the concrete class depends on different conditions or configurations.
What is the Factory Pattern?
The Factory Pattern provides a way to create objects without specifying the exact class of the object that will be created. The pattern involves creating a factory class or method that returns instances of different classes, based on the given input or conditions. This enables the creation of objects in a more flexible and abstract way, avoiding direct calls to constructors in client code.
How the Factory Pattern Works
The core idea behind the Factory Pattern is to define a method or class that can instantiate different objects based on input or configuration. Instead of directly calling the constructor of a class, the client code calls a factory method, which returns the appropriate object.
Example: Factory Pattern in JavaScript
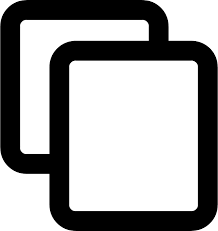
class Car {
drive() {
console.log("Driving a car");
}
}
class Truck {
drive() {
console.log("Driving a truck");
}
}
class VehicleFactory {
createVehicle(type) {
if (type === "car") {
return new Car();
} else if (type === "truck") {
return new Truck();
}
throw new Error("Vehicle type not supported");
}
}
// Usage
const factory = new VehicleFactory();
const vehicle1 = factory.createVehicle("car");
vehicle1.drive(); // Output: Driving a car
const vehicle2 = factory.createVehicle("truck");
vehicle2.drive(); // Output: Driving a truck
In this example, the VehicleFactory
class is responsible for creating instances of Car
and Truck
based on the input parameter type
. The client code calls the createVehicle
method, which returns the appropriate class instance.
Advantages of the Factory Pattern
- Decoupling: The Factory Pattern decouples the creation of an object from its usage. The client code doesn't need to know the exact class being instantiated, allowing for more flexibility.
- Extensibility: It is easy to extend the system to support new types of objects without modifying existing code. You only need to modify the factory method or class to add new types of objects.
- Centralized object creation: The creation of objects is centralized within the factory, making it easier to manage object creation logic and ensure consistency across the application.
Disadvantages of the Factory Pattern
- Complexity: Introducing a factory can add extra complexity to the code, especially if you need to manage multiple subclasses or if you have many different factory methods.
- Overhead: The Factory Pattern can introduce some overhead, as the factory class itself needs to be maintained and may require additional logic to instantiate objects.
When to Use the Factory Pattern
The Factory Pattern is useful when:
- You have a common interface or superclass, but need to instantiate different types of objects based on input or configuration.
- You want to decouple the client code from the specific classes that need to be instantiated.
- You anticipate the need for future extensions or modifications to the types of objects being created.
Conclusion
The Factory Pattern is a powerful creational pattern that allows for flexibility in object creation. It decouples the client code from the specifics of class instantiation, promoting maintainability and extensibility in your code. While it can add some complexity, the advantages of flexibility and centralization often outweigh the drawbacks, especially in larger applications where object creation logic is complex or subject to change.
Observer Pattern
The Observer Pattern is a behavioral design pattern that defines a one-to-many dependency relationship between objects. When one object (the subject) changes its state, all dependent objects (observers) are notified and updated automatically. This pattern is commonly used in situations where an object needs to notify multiple other objects about changes in its state without knowing who or what those objects are.
What is the Observer Pattern?
The Observer Pattern enables an object (called the subject) to notify a list of observers (or listeners) about any changes in its state. When the state of the subject changes, all observers that are subscribed to the subject receive an update. This pattern is widely used in implementing event handling systems, like in UI frameworks where user interactions need to trigger updates in other parts of the system.
How the Observer Pattern Works
The core idea behind the Observer Pattern is that the subject maintains a list of its observers and notifies them of any changes. Observers can subscribe to or unsubscribe from the subject, and the subject will handle notifying the observers when its state changes.
Example: Observer Pattern in JavaScript
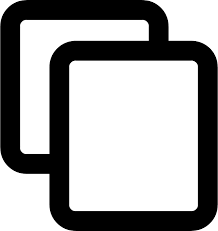
class Subject {
constructor() {
this.observers = [];
}
subscribe(observer) {
this.observers.push(observer);
}
unsubscribe(observer) {
this.observers = this.observers.filter(obs => obs !== observer);
}
notify() {
this.observers.forEach(observer => observer.update());
}
}
class Observer {
update() {
console.log("Observer has been notified!");
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.subscribe(observer1);
subject.subscribe(observer2);
subject.notify(); // Output: Observer has been notified! (twice)
In this example, the Subject
class maintains a list of its observers. The subscribe
method adds observers to the list, while the unsubscribe
method removes them. The notify
method calls the update
method on each observer, notifying them of changes in the subject's state.
Advantages of the Observer Pattern
- Loose coupling: The subject does not need to know the details of the observers, only that they implement the
update
method. This allows for loose coupling between the subject and the observers. - Dynamic relationships: Observers can be added or removed at runtime, making the system flexible and dynamic.
- Broadcast communication: The Observer Pattern is ideal for situations where one object needs to broadcast information to many others, like in event handling or messaging systems.
Disadvantages of the Observer Pattern
- Memory leaks: If observers are not properly removed from the subject’s list, they can stay in memory and lead to memory leaks, especially if they are no longer needed.
- Unnecessary updates: If the observers are not carefully managed, they may be notified of changes that they don’t need to handle, which could lead to performance issues.
When to Use the Observer Pattern
The Observer Pattern is useful when:
- You have a system where one object’s state changes need to be reflected in many other objects.
- You want to avoid tight coupling between objects, enabling more flexibility and extensibility in your system.
- You need an event-driven system or want to implement messaging or notification systems.
Conclusion
The Observer Pattern is a versatile pattern that makes it easy to create systems with dynamic relationships between objects. It helps decouple the objects that produce events from the ones that consume them, allowing for more maintainable and scalable code. While it can introduce complexity if observers are not managed properly, it remains a powerful tool for implementing event-driven and notification-based systems.
Understanding the Event Loop
The Event Loop is a fundamental concept in JavaScript that allows for asynchronous programming. It is the mechanism that enables JavaScript to perform non-blocking I/O operations despite being single-threaded. Understanding how the Event Loop works is crucial for writing efficient, non-blocking JavaScript code.
What is the Event Loop?
JavaScript is single-threaded, meaning it can only execute one operation at a time. However, it is capable of handling asynchronous operations like I/O, network requests, and timers without freezing the execution of other code. The Event Loop is responsible for managing this process by continuously checking if there are any tasks that need to be executed and if the call stack is empty. If the stack is empty and there are tasks in the queue, the Event Loop moves those tasks to the call stack for execution.
The Call Stack and Task Queue
The Event Loop works in conjunction with the call stack and the task queue.
- Call Stack: The call stack is where JavaScript stores function calls. It works in a Last In, First Out (LIFO) manner. When a function is called, it is added to the top of the stack. When the function finishes executing, it is removed from the stack.
- Task Queue: The task queue (or message queue) holds messages or tasks that are waiting to be processed. These tasks can be events, callbacks, or timers that need to be executed once the call stack is empty.
Example: Basic Event Loop
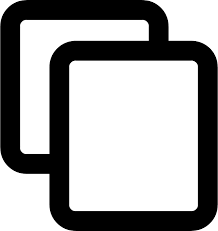
console.log("Start");
setTimeout(function() {
console.log("Inside Timeout");
}, 0);
console.log("End");
In this example, the output will be:
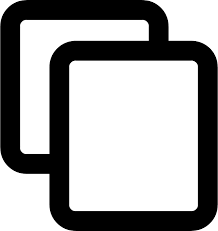
Start
End
Inside Timeout
Even though the setTimeout
has a delay of 0 milliseconds, the "Inside Timeout" log is executed after "End" because the setTimeout
callback is placed in the task queue and must wait until the call stack is empty before it is executed.
How the Event Loop Works
The basic flow of the Event Loop can be described as follows:
- JavaScript starts executing from the top of the script, adding function calls to the call stack.
- Asynchronous operations such as
setTimeout
,setInterval
, and Promises are handled by the Web APIs (in the browser) or Node.js APIs. - Once the asynchronous operation completes, the callback is placed into the task queue.
- The Event Loop continuously checks if the call stack is empty. If the stack is empty and there are tasks in the queue, the Event Loop moves one task from the queue to the stack for execution.
- This process repeats until there are no more tasks in the queue or the script finishes execution.
Microtasks and Macrotasks
The Event Loop has two types of task queues: the microtask queue and the macrotask queue.
- Microtasks are tasks that have higher priority and are executed after the currently executing script and before any other macrotasks. Promises and
MutationObserver
callbacks are examples of microtasks. - Macrotasks include events like
setTimeout
,setInterval
, and I/O operations.
Example: Microtasks vs. Macrotasks
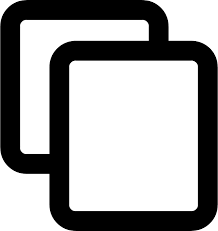
console.log("Start");
setTimeout(function() {
console.log("Inside Timeout");
}, 0);
Promise.resolve().then(function() {
console.log("Inside Promise");
});
console.log("End");
In this example, the output will be:
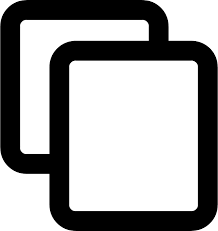
Start
End
Inside Promise
Inside Timeout
Even though the setTimeout
is set to 0 milliseconds, the Promise callback (a microtask) gets executed first because it has higher priority than the setTimeout
callback (a macrotask).
Visualizing the Event Loop
To help visualize how the Event Loop works, consider this simple diagram:
- The script starts and adds tasks to the call stack.
- Asynchronous operations are offloaded to the Web APIs or Node.js APIs.
- Once the asynchronous task completes, its callback is placed into the task queue.
- The Event Loop checks the call stack, and if it's empty, it moves tasks from the task queue to the call stack.
- Microtasks are processed before macrotasks.
Conclusion
The Event Loop is a crucial concept in JavaScript’s asynchronous execution model. Understanding how the Event Loop works allows you to write more efficient and non-blocking JavaScript code. It is essential for handling asynchronous code, avoiding blocking the main thread, and ensuring that your application remains responsive even with heavy I/O or long-running tasks.
Microtasks and Macrotasks
In JavaScript, there are two types of tasks that are processed by the Event Loop: microtasks and macrotasks. Understanding the difference between them is crucial for writing efficient and predictable asynchronous code.
What are Microtasks?
Microtasks are tasks that are executed after the current script has executed and before any other macrotasks. They are considered high-priority tasks and are processed in the same event loop cycle as the currently executing script. Microtasks include tasks like Promises and MutationObserver
callbacks.
Example of Microtasks
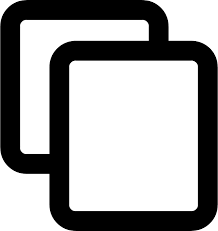
console.log("Start");
Promise.resolve().then(function() {
console.log("Inside Promise");
});
console.log("End");
In the above example, the output will be:
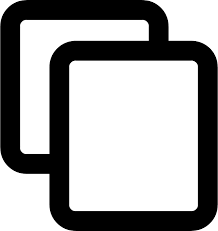
Start
End
Inside Promise
Even though "End" is logged after "Start," the "Inside Promise" log appears before "End" because the Promise is a microtask and gets executed after the current synchronous code (before the next macrotask).
What are Macrotasks?
Macrotasks are tasks that are queued to be executed after the current script and after all microtasks have been processed. Macrotasks include tasks like setTimeout
, setInterval
, and I/O operations. These tasks are added to the macrotask queue, and the event loop executes them after all microtasks in the microtask queue have been executed.
Example of Macrotasks
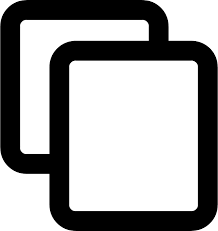
console.log("Start");
setTimeout(function() {
console.log("Inside Timeout");
}, 0);
console.log("End");
In the above example, the output will be:
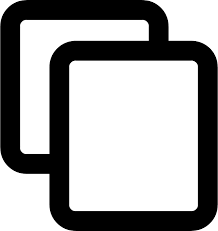
Start
End
Inside Timeout
Although the setTimeout
is set to 0 milliseconds, the "Inside Timeout" log appears after "End" because the setTimeout
callback is a macrotask and is placed in the macrotask queue, which is processed after the microtask queue is empty.
Microtasks vs. Macrotasks
The key differences between microtasks and macrotasks are:
- Priority: Microtasks have higher priority than macrotasks and are executed before them.
- Queue: Microtasks are placed in the microtask queue, while macrotasks are placed in the macrotask queue.
- Execution Order: Microtasks are processed after the current script finishes but before any macrotasks are processed.
Example: Comparing Microtasks and Macrotasks
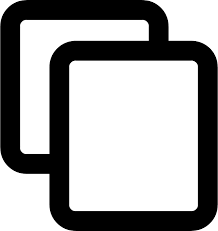
console.log("Start");
setTimeout(function() {
console.log("Inside Timeout");
}, 0);
Promise.resolve().then(function() {
console.log("Inside Promise");
});
console.log("End");
The output will be:
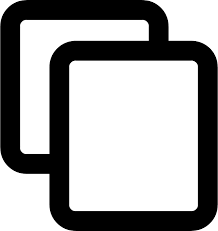
Start
End
Inside Promise
Inside Timeout
In this example, the microtask (the Promise callback) is executed first, followed by the macrotask (the setTimeout
callback), even though they are both scheduled with 0 milliseconds delay.
Why Does This Matter?
Understanding the order in which tasks are processed in the event loop can help you avoid unexpected behavior in your JavaScript code. For example, if you have a setTimeout
call inside a Promise, you can predict when the callback will be executed in relation to other asynchronous tasks.
Conclusion
Microtasks and macrotasks are both important concepts in JavaScript’s asynchronous execution model. Microtasks have higher priority and are executed before macrotasks, which allows for more immediate handling of asynchronous code. By understanding how the event loop handles microtasks and macrotasks, you can write more efficient and predictable JavaScript code.
Debouncing and Throttling
Debouncing and throttling are two techniques used to limit the rate at which a function is executed in response to an event. These techniques help improve performance, especially when dealing with events that can trigger a function multiple times in quick succession, such as scroll
, resize
, and keypress
events. By using debouncing and throttling, you can prevent unnecessary function calls and make your application more efficient.
What is Debouncing?
Debouncing is a technique used to ensure that a function is only executed after a certain amount of time has passed since the last event. If the event keeps firing repeatedly, the function will not be executed until a specified delay has passed without any new events. This is useful for limiting the number of times a function is triggered when the user is continuously interacting with the page (e.g., typing in a search box).
Example of Debouncing
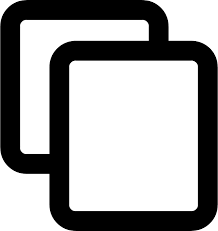
let timer;
function debounce(func, delay) {
return function(...args) {
clearTimeout(timer);
timer = setTimeout(() => func(...args), delay);
};
}
const searchInput = document.getElementById("search");
searchInput.addEventListener("input", debounce(function() {
console.log("Searching:", searchInput.value);
}, 500));
In this example, the search function is debounced, meaning it will only be triggered 500 milliseconds after the user stops typing. This prevents the function from being called on every keystroke.
What is Throttling?
Throttling is a technique used to limit the rate at which a function is executed. Unlike debouncing, which waits for a period of inactivity, throttling ensures that the function is called at regular intervals, no matter how many times the event is triggered. This is useful for events that fire continuously, like scrolling or resizing, where you want to limit the number of function calls over time.
Example of Throttling
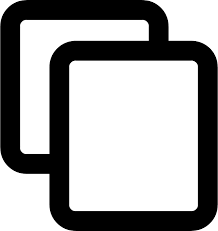
function throttle(func, limit) {
let lastFunc;
let lastTime;
return function(...args) {
const now = new Date().getTime();
if (!lastTime || now - lastTime >= limit) {
func(...args);
lastTime = now;
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(() => func(...args), limit - (now - lastTime));
}
};
}
const scrollHandler = throttle(function() {
console.log("Scrolling");
}, 1000);
window.addEventListener("scroll", scrollHandler);
In this example, the scroll handler function is throttled, meaning it will only run once every 1000 milliseconds, regardless of how fast the user scrolls.
Debouncing vs. Throttling
The key differences between debouncing and throttling are:
- Debouncing delays the execution of the function until a certain time has passed after the last event.
- Throttling ensures the function is executed at a regular interval, regardless of how many times the event is triggered.
When to Use Debouncing
Debouncing is ideal when you want to limit the frequency of function execution in response to events that are triggered in quick succession but where you only care about the final state. Common use cases for debouncing include:
- Input field validation (e.g., searching or auto-completion).
- Window resizing events.
- Button click events for repeated actions.
When to Use Throttling
Throttling is useful when you want to ensure a function is executed at regular intervals, even if the event is triggered continuously. Common use cases for throttling include:
- Scrolling events (e.g., infinite scrolling or lazy loading).
- Window resizing events.
- Mousemove or mousewheel events for animations.
Conclusion
Both debouncing and throttling are powerful techniques for optimizing performance and reducing the number of function calls in your JavaScript applications. Debouncing is best used when you want to wait for a period of inactivity before executing a function, while throttling is ideal for executing a function at consistent intervals. By choosing the right technique for the right situation, you can improve both the performance and user experience of your website or web application.
Unit Testing with Jest
Unit testing is a crucial practice in software development that helps ensure individual components of a program work as expected. Jest is a popular JavaScript testing framework that makes writing and running tests easier. It is commonly used for testing JavaScript code, especially in projects built with React. In this section, we will introduce Jest and how to use it for unit testing.
What is Jest?
Jest is a testing framework developed by Facebook, primarily for testing JavaScript applications. It provides a simple and powerful way to write tests for your code. Jest comes with built-in test runners, assertion libraries, and mocking capabilities, making it a complete testing solution. Its features include:
- Zero configuration: Jest works out of the box with minimal setup, making it easy to get started.
- Snapshot testing: Jest can capture and compare the output of components to ensure that they do not change unexpectedly.
- Mocking and spies: Jest allows you to mock functions, modules, and timers to isolate components for testing.
- Code coverage: Jest provides built-in code coverage reports to show how much of your code is tested.
Setting Up Jest
To get started with Jest, you need to install it in your project. You can install Jest using npm or yarn:
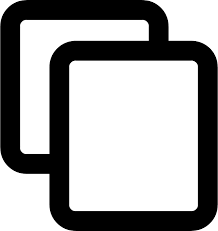
npm install --save-dev jest
Once installed, you can create a test file and write your first test. Jest will automatically look for test files with the extension .test.js
or .spec.js
.
Writing Your First Test
Let's start by writing a simple test. Suppose you have a function that adds two numbers:
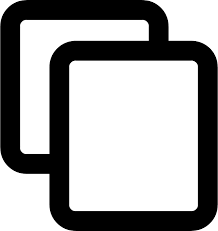
// add.js
function add(a, b) {
return a + b;
}
module.exports = add;
You can write a test for this function as follows:
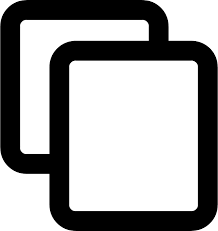
// add.test.js
const add = require('./add');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
In this test, we are using Jest's test
function to define a test case. The expect
function is used to make assertions about the result of calling add(1, 2)
. The toBe
matcher checks if the result is exactly equal to 3.
Running Tests
To run your tests, you can use the following npm command:
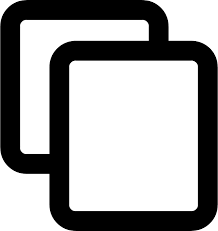
npx jest
Jest will find all test files in your project and run them. It will display the results in the terminal, showing which tests passed and which failed.
Common Jest Matchers
Jest provides a variety of matchers to assert different conditions in your tests. Some commonly used matchers include:
toBe(value)
: Checks if the value is exactly the same as the expected value (strict equality).toEqual(value)
: Checks if the value is deeply equal to the expected value (useful for objects and arrays).toBeTruthy()
: Checks if the value is truthy (i.e., notnull
,undefined
,false
,0
, or an empty string).toBeFalsy()
: Checks if the value is falsy (i.e.,null
,undefined
,false
,0
, or an empty string).toHaveLength(length)
: Checks if an array or string has a specific length.toContain(item)
: Checks if an array or string contains a specific item.
Mock Functions
Jest has powerful mocking capabilities that allow you to mock functions, modules, and timers. This is useful for isolating the unit of code you're testing and simulating different scenarios.
For example, you can mock a function like this:
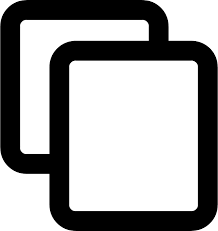
const mockFn = jest.fn();
mockFn.mockReturnValue(42);
test('mock function returns value', () => {
expect(mockFn()).toBe(42);
});
Snapshot Testing
Snapshot testing is a feature in Jest that allows you to capture the output of a component and compare it against a saved snapshot. If the output changes unexpectedly, Jest will notify you.
To use snapshot testing, you can write a test like this:
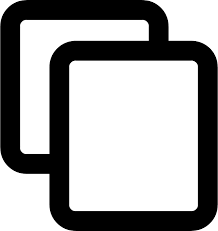
const button = ;
test('button snapshot', () => {
expect(button).toMatchSnapshot();
});
If the output of the button changes, Jest will alert you that the snapshot does not match, and you can review the changes.
Code Coverage
Jest provides built-in code coverage reports that show how much of your code is covered by tests. To generate a code coverage report, run the following command:
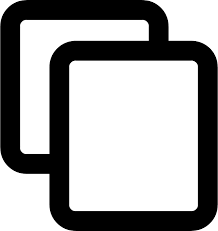
npx jest --coverage
This will generate a detailed report showing the percentage of code covered by tests for each file.
Conclusion
Jest is a powerful and easy-to-use testing framework for JavaScript. It provides a complete testing solution with minimal setup, making it ideal for unit testing JavaScript functions and applications. By writing tests with Jest, you can ensure your code is reliable and maintainable, and catch bugs early in the development process.
Introduction to Mocha and Chai
Mocha and Chai are two popular testing libraries in the JavaScript ecosystem. Mocha is a test framework that provides a test runner and various utilities to organize your tests, while Chai is an assertion library that allows you to write expressive tests with different assertion styles. Together, they provide a powerful, flexible, and easy-to-use solution for unit testing JavaScript code.
What is Mocha?
Mocha is a feature-rich JavaScript test framework that runs on Node.js and in the browser. It provides a simple API for organizing and running tests and supports asynchronous testing. Mocha allows you to write tests in a behavior-driven development (BDD), test-driven development (TDD), or other styles. Some key features of Mocha include:
- Test organization: Mocha allows you to group tests into suites using
describe
, and define individual tests usingit
. - Asynchronous testing: Mocha makes it easy to write asynchronous tests using callback functions or promises.
- Flexible reporting: Mocha supports different reporting styles, making it easy to integrate with other tools or display results in various formats.
- Hooks: Mocha provides hooks like
before
,after
,beforeEach
, andafterEach
to set up and tear down tests.
Setting Up Mocha
To use Mocha, you first need to install it in your project. You can do this with npm:
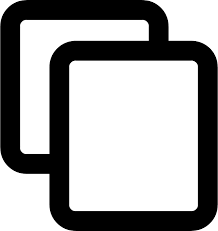
npm install --save-dev mocha
Once Mocha is installed, you can create a test file and start writing your first test.
Writing Your First Test with Mocha
Here's a simple Mocha test that adds two numbers:
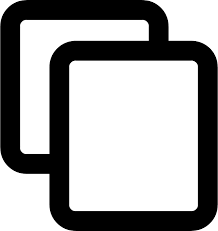
// math.js
function add(a, b) {
return a + b;
}
module.exports = add;
Now, let's write a test for the add
function using Mocha:
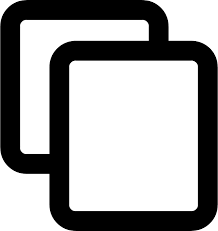
// test/math.test.js
const add = require('../math');
const assert = require('assert');
describe('Add Function', function() {
it('should add two numbers correctly', function() {
assert.equal(add(1, 2), 3);
});
});
In this example, we use describe
to define a test suite and it
to define a test case. The assert.equal
function is used to check if the result of add(1, 2)
is equal to 3.
Running Tests with Mocha
To run your Mocha tests, use the following command:
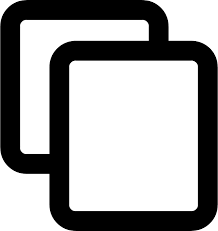
npx mocha
Mocha will automatically find all test files (with .test.js
or .spec.js
extensions) and execute them.
What is Chai?
Chai is an assertion library that works seamlessly with Mocha to make your tests more expressive. Chai allows you to write assertions using different styles, such as BDD (Behavior-Driven Development), TDD (Test-Driven Development), and others. Chai’s assertion methods help you check if your code behaves as expected.
Chai supports three assertion styles:
- Assert: A simple, traditional assertion style using methods like
assert.equal()
. - Expect: A more readable, natural language style, e.g.,
expect(result).to.equal(3)
. - Should: Another readable style, e.g.,
result.should.equal(3)
.
Setting Up Chai
To install Chai, run the following npm command:
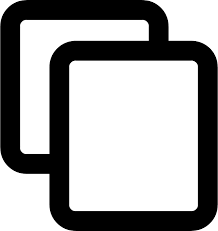
npm install --save-dev chai
Writing Tests with Chai
Here’s how to write a simple test using Chai's expect
style:
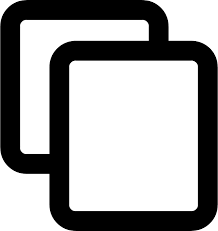
const chai = require('chai');
const expect = chai.expect;
const add = require('../math');
describe('Add Function', function() {
it('should add two numbers correctly', function() {
expect(add(1, 2)).to.equal(3);
});
});
Running Tests with Mocha and Chai
To run Mocha and Chai tests, you can simply use the same Mocha command as before:
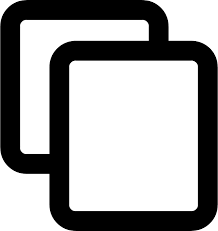
npx mocha
Mocha will run the tests, and Chai will handle the assertions.
Advantages of Mocha and Chai
- Flexibility: Mocha and Chai allow you to choose different assertion styles and customize your testing setup.
- Asynchronous support: Mocha makes it easy to test asynchronous code, and Chai works well with promises and async/await.
- Extensibility: Mocha supports plugins, and Chai has many useful extensions for more advanced assertions.
- Readable tests: With Chai’s BDD-style assertions, your tests can be written in a natural, human-readable way.
Conclusion
Mocha and Chai form a powerful combination for unit testing JavaScript applications. Mocha provides a flexible test runner, while Chai offers a variety of assertion styles to make your tests more readable. Together, they help you write tests that ensure the correctness and reliability of your code.
Building a To-Do List App
In this section, we will build a simple to-do list app that allows users to add, remove, and toggle tasks as completed. The app will use basic HTML, CSS, and JavaScript to manage the to-do list functionality.
Setting Up the HTML Structure
We’ll begin by creating a simple HTML structure for the to-do list app. The app will include an input field to add new tasks, a button to submit the task, and a list to display the tasks.
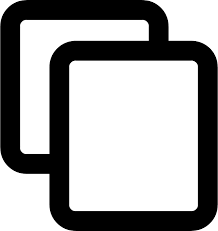
To-Do List App
To-Do List
This HTML includes:
- An input field to enter a new task.
- A button to add the task to the list.
- An unordered list to display the tasks.
Styling the To-Do List App with CSS
We’ll add some basic CSS to style the app, so it has a clean and user-friendly interface.
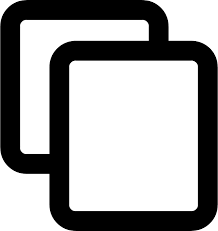
/* style.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
.todo-app {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
width: 300px;
text-align: center;
}
input[type="text"] {
width: 80%;
padding: 10px;
margin-bottom: 10px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc;
}
button {
padding: 10px 20px;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
#task-list {
list-style-type: none;
padding: 0;
}
#task-list li {
margin: 10px 0;
padding: 10px;
background-color: #f9f9f9;
border-radius: 5px;
display: flex;
justify-content: space-between;
align-items: center;
}
.completed {
text-decoration: line-through;
color: #888;
}
This CSS will style the to-do app with a clean layout, making it easy to see and interact with the tasks. It also includes a class for completed tasks, which will add a line-through effect and change the color.
Adding Functionality with JavaScript
Now, let’s add the JavaScript code to handle adding tasks, marking them as completed, and removing them. We’ll also use localStorage to save the tasks so that they persist even if the page is refreshed.
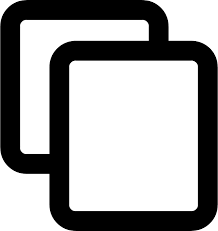
// app.js
document.addEventListener('DOMContentLoaded', () => {
const taskInput = document.getElementById('task-input');
const addTaskButton = document.getElementById('add-task');
const taskList = document.getElementById('task-list');
// Load tasks from localStorage
const loadTasks = () => {
const tasks = JSON.parse(localStorage.getItem('tasks')) || [];
taskList.innerHTML = '';
tasks.forEach(task => {
const li = document.createElement('li');
li.textContent = task.text;
if (task.completed) {
li.classList.add('completed');
}
const deleteButton = document.createElement('button');
deleteButton.textContent = 'Delete';
deleteButton.addEventListener('click', () => {
removeTask(task.text);
});
li.appendChild(deleteButton);
taskList.appendChild(li);
});
};
// Add a new task
const addTask = () => {
const taskText = taskInput.value.trim();
if (taskText) {
const tasks = JSON.parse(localStorage.getItem('tasks')) || [];
tasks.push({ text: taskText, completed: false });
localStorage.setItem('tasks', JSON.stringify(tasks));
loadTasks();
taskInput.value = '';
}
};
// Remove a task
const removeTask = (taskText) => {
let tasks = JSON.parse(localStorage.getItem('tasks')) || [];
tasks = tasks.filter(task => task.text !== taskText);
localStorage.setItem('tasks', JSON.stringify(tasks));
loadTasks();
};
// Toggle task completion
taskList.addEventListener('click', (event) => {
if (event.target.tagName === 'LI') {
const tasks = JSON.parse(localStorage.getItem('tasks')) || [];
const taskText = event.target.textContent.replace('Delete', '').trim();
const task = tasks.find(task => task.text === taskText);
if (task) {
task.completed = !task.completed;
localStorage.setItem('tasks', JSON.stringify(tasks));
loadTasks();
}
}
});
// Initialize the app
addTaskButton.addEventListener('click', addTask);
loadTasks();
});
This JavaScript code does the following:
- It listens for button clicks and adds tasks to the list and localStorage.
- It allows tasks to be marked as completed by clicking on them, which toggles the
completed
class. - It allows tasks to be deleted by clicking the "Delete" button next to each task.
- The tasks are saved in
localStorage
so that they persist across page reloads.
Conclusion
In this tutorial, we built a simple to-do list app that allows users to add, remove, and toggle tasks as completed. We used basic HTML, CSS, and JavaScript, and incorporated localStorage to persist tasks across page reloads. This app is a great starting point for building more advanced task management apps with additional features like task due dates, categorization, or sorting.
Simple Calculator
In this section, we will build a simple calculator app that can perform basic arithmetic operations such as addition, subtraction, multiplication, and division. The app will use HTML for the structure, CSS for styling, and JavaScript for the functionality.
Setting Up the HTML Structure
Let's start by creating the basic HTML layout for the calculator. It will have buttons for digits (0-9), operations (+, -, *, /), a display area for the result, and a button for clearing the display.
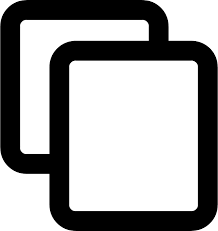
Simple Calculator
This HTML includes:
- An input field to display the result (disabled so the user can't type directly into it).
- Buttons for numbers (0-9), operators (+, -, *, /), clear (C), and equals (=).
Styling the Calculator with CSS
Next, let's add some basic CSS to style the calculator. We'll make it visually appealing and easy to use.
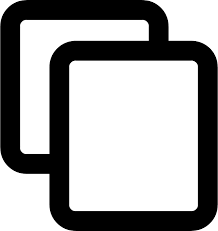
/* style.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.calculator {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
width: 250px;
}
input[type="text"] {
width: 100%;
padding: 10px;
font-size: 24px;
text-align: right;
border-radius: 5px;
border: 1px solid #ccc;
margin-bottom: 10px;
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
}
button {
padding: 20px;
font-size: 18px;
background-color: #f0f0f0;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #ddd;
}
button:active {
background-color: #ccc;
}
This CSS makes the calculator responsive and visually appealing. It uses a grid layout for the buttons and gives each button a hover effect for a better user experience.
Adding Functionality with JavaScript
Now, let's add JavaScript to handle the calculator’s functionality. This includes adding numbers to the display, performing arithmetic operations, and calculating the result when the equals button is clicked.
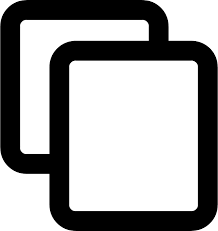
// app.js
document.addEventListener('DOMContentLoaded', () => {
const display = document.getElementById('display');
let currentInput = '';
let operator = '';
let previousInput = '';
// Button click event handler
const buttonClick = (value) => {
if (value === 'C') {
currentInput = '';
previousInput = '';
operator = '';
display.value = '';
} else if (value === '=') {
if (previousInput && operator && currentInput) {
currentInput = String(eval(previousInput + operator + currentInput));
display.value = currentInput;
previousInput = '';
operator = '';
}
} else if (['+', '-', '*', '/'].includes(value)) {
if (currentInput) {
if (previousInput) {
currentInput = String(eval(previousInput + operator + currentInput));
display.value = currentInput;
}
previousInput = currentInput;
operator = value;
currentInput = '';
}
} else {
currentInput += value;
display.value = currentInput;
}
};
// Attach event listeners to buttons
document.getElementById('btn-1').addEventListener('click', () => buttonClick('1'));
document.getElementById('btn-2').addEventListener('click', () => buttonClick('2'));
document.getElementById('btn-3').addEventListener('click', () => buttonClick('3'));
document.getElementById('btn-4').addEventListener('click', () => buttonClick('4'));
document.getElementById('btn-5').addEventListener('click', () => buttonClick('5'));
document.getElementById('btn-6').addEventListener('click', () => buttonClick('6'));
document.getElementById('btn-7').addEventListener('click', () => buttonClick('7'));
document.getElementById('btn-8').addEventListener('click', () => buttonClick('8'));
document.getElementById('btn-9').addEventListener('click', () => buttonClick('9'));
document.getElementById('btn-0').addEventListener('click', () => buttonClick('0'));
document.getElementById('btn-add').addEventListener('click', () => buttonClick('+'));
document.getElementById('btn-subtract').addEventListener('click', () => buttonClick('-'));
document.getElementById('btn-multiply').addEventListener('click', () => buttonClick('*'));
document.getElementById('btn-divide').addEventListener('click', () => buttonClick('/'));
document.getElementById('btn-clear').addEventListener('click', () => buttonClick('C'));
document.getElementById('btn-equal').addEventListener('click', () => buttonClick('='));
});
This JavaScript code does the following:
- Handles button clicks and updates the display with the corresponding value.
- Performs arithmetic operations using the
eval()
function when the equals button is clicked. - Clears the display when the clear button (C) is pressed.
- Handles operator buttons (+, -, *, /) to store the current input and operator for future calculations.
Conclusion
In this tutorial, we created a simple calculator that can handle basic arithmetic operations like addition, subtraction, multiplication, and division. We used HTML for the structure, CSS for styling, and JavaScript for the functionality. This app is a great example of building a simple interactive app using core web technologies.
Weather App Using APIs
In this section, we'll build a simple weather app that fetches current weather data from an external API. The app will take a city name as input and display the current weather conditions, including the temperature, humidity, and weather description.
Setting Up the HTML Structure
Let's start by creating the basic HTML layout for the weather app. It will include an input field to enter the city name, a button to fetch the weather data, and a section to display the weather information.
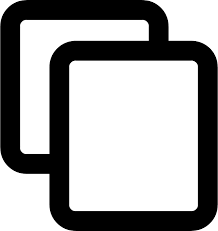
Weather App
Weather App
This HTML includes:
- An input field to enter the city name.
- A button to trigger the weather data fetch.
- A section to display the weather information, including the city name, temperature, weather description, and humidity.
Styling the Weather App with CSS
Next, let's add some basic CSS to style the app and make it more visually appealing.
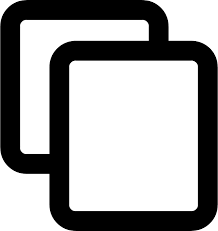
/* style.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.weather-app {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
width: 300px;
text-align: center;
}
input[type="text"] {
width: 80%;
padding: 10px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc;
margin-bottom: 10px;
}
button {
padding: 10px 20px;
font-size: 16px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
#weather-info {
margin-top: 20px;
}
h3 {
margin: 0;
font-size: 24px;
}
p {
font-size: 18px;
}
This CSS gives the weather app a clean and simple look, making it easy to use and visually appealing. It includes styles for the input field, button, and weather information section.
Fetching Weather Data Using an API
Now, let's add JavaScript to fetch weather data from an external weather API. We'll use the OpenWeatherMap API to get the current weather data. You will need to sign up for an API key from OpenWeatherMap to use this service.
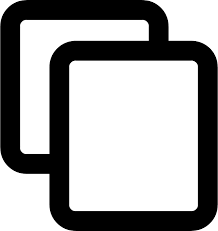
// app.js
document.getElementById('get-weather').addEventListener('click', function() {
const city = document.getElementById('city').value;
const apiKey = 'YOUR_API_KEY'; // Replace with your OpenWeatherMap API key
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`;
fetch(url)
.then(response => response.json())
.then(data => {
if (data.cod === '404') {
alert('City not found');
} else {
document.getElementById('city-name').textContent = data.name;
document.getElementById('temperature').textContent = `Temperature: ${data.main.temp}°C`;
document.getElementById('description').textContent = `Description: ${data.weather[0].description}`;
document.getElementById('humidity').textContent = `Humidity: ${data.main.humidity}%`;
}
})
.catch(error => console.error('Error fetching weather data:', error));
});
This JavaScript code does the following:
- Listens for a click event on the "Get Weather" button.
- Uses the
fetch()
API to get weather data from OpenWeatherMap, passing in the city name and API key. - Displays the weather data (city name, temperature, weather description, and humidity) in the
#weather-info
section. - Handles errors, such as when the city is not found, by showing an alert message.
Conclusion
In this tutorial, we built a weather app that fetches weather data from the OpenWeatherMap API. The app allows users to enter a city name, click a button to fetch the weather data, and display the current temperature, weather description, and humidity. This project demonstrates how to work with APIs, handle user input, and display dynamic content on the web.