
Introduction to Postman
Postman is a popular API testing tool that simplifies the process of developing, testing, and interacting with APIs. It allows users to send requests, view responses, and automate testing for APIs. Initially created as a Chrome extension, Postman has grown into a full-fledged application that supports a wide range of API workflows.
History of Postman
Postman was launched in 2012 by Abhinav Asthana as a tool for testing APIs. It started as a Chrome extension and quickly gained popularity due to its ease of use and powerful features for testing REST APIs. Over time, it evolved into a standalone desktop application that supports REST, SOAP, GraphQL, and WebSocket protocols. Today, it is one of the most widely used API testing tools, with millions of users worldwide.
Postman Features
Below are the key features that make Postman a preferred choice for API developers and testers:
Feature | Description |
---|---|
API Request Sending | Postman allows users to send HTTP requests (GET, POST, PUT, DELETE, etc.) to test the behavior of APIs and view the returned responses. |
Tests and Automation | Postman supports writing automated tests using JavaScript, making it possible to verify API responses and automate API workflows. |
Environment Management | Postman allows users to manage environments, making it easy to switch between different API configurations for development, staging, and production environments. |
Mock Servers | Postman allows you to create mock servers to simulate API responses, which is useful for testing and prototyping APIs before they are fully developed. |
Setting Up Postman
To start using Postman, follow the steps below to install it on your system:
- Visit the Postman downloads page to download the appropriate version for your operating system.
- Once downloaded, run the installer and follow the on-screen instructions to install Postman on your machine.
- After installation, launch Postman, and you can start testing APIs immediately.
Code Example: Sending a GET Request
Let’s see how to send a GET request to an API using Postman:
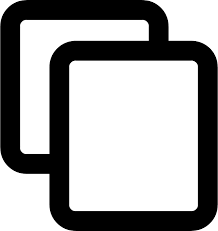
// Example of sending a GET request using Postman
pm.sendRequest('https://api.example.com/data', function (err, res) {
console.log(res);
});
Diagram: Postman Request Flow
The following diagram explains the flow of making an API request in Postman:

This diagram illustrates how Postman sends requests, processes responses, and allows testers to validate API behavior with built-in tests.
Features and Benefits of Using Postman
Postman is a comprehensive tool for API development and testing, providing several features and benefits that make it a preferred choice for developers and testers. Below, we explore some of the key features and the advantages they bring to API workflows.
Key Features of Postman
Postman offers a wide range of features that enhance the process of developing, testing, and maintaining APIs:
Feature | Description |
---|---|
API Request Builder | Postman’s intuitive request builder allows users to quickly create HTTP requests with different methods (GET, POST, PUT, DELETE) and configure headers, body, and parameters. |
Request History | Postman tracks all the API requests you send, allowing you to view, re-run, or modify previous requests directly from the History tab. |
Automated Testing | Postman supports writing automated tests in JavaScript, enabling users to validate API responses, check status codes, and ensure consistency in API behavior. |
Mock Servers | Postman allows you to create mock servers, which simulate API responses, making it easier to test API behavior before the actual backend is developed. |
Environment and Variable Management | Postman provides environment variables to manage different API configurations (development, staging, production), and allows easy switching between them during testing. |
Collaboration | Postman supports collaboration features like shared collections, workspace synchronization, and version control, making it easy for teams to work together on API projects. |
Benefits of Using Postman
Here are some of the key benefits that make Postman a standout tool for API development:
- Improved Productivity: With its user-friendly interface and powerful features like request history, automation, and debugging tools, Postman enables developers to work efficiently and quickly test APIs without writing complex scripts.
- Time-Saving Automation: Automated tests and pre-request scripts help in verifying responses, thus saving time and ensuring that APIs meet expected behavior without manual intervention.
- Collaboration & Sharing: Postman allows teams to collaborate seamlessly by sharing collections and environments, ensuring that everyone is on the same page when working on API projects.
- Simulating Real-World Scenarios: Mock servers help simulate API responses, which is particularly useful when the backend is not yet available or when developing front-end code with mock data.
- Cross-Platform Support: Postman works across different platforms (Windows, macOS, and Linux), making it easily accessible for teams using different operating systems.
- Rich API Testing Capabilities: Postman supports REST, SOAP, GraphQL, and WebSocket protocols, offering flexibility for testing a wide range of APIs.
Code Example: Automated Test in Postman
Here’s an example of a simple automated test written in Postman to check if an API returns a 200 status code:
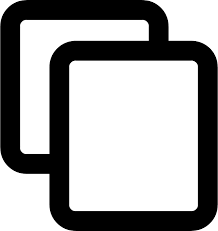
// Example of an automated test in Postman
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
Diagram: Postman API Testing Workflow
The following diagram shows the workflow of using Postman for API testing:

This diagram illustrates the flow of API requests, response validation, and automated testing in Postman, helping teams ensure that their APIs are working as expected before deployment.
Installing and Setting Up Postman
Postman is a powerful tool for API testing, and setting it up is straightforward. This section will guide you through the process of installing and configuring Postman on different operating systems.
System Requirements
Before installing Postman, ensure that your system meets the following requirements:
- Windows 7 or later
- macOS 10.10 or later
- Linux (Ubuntu, Debian, Fedora, or similar)
- At least 4 GB of RAM
Installation Steps
Follow the steps below for your respective operating system:
Windows
- Download the Postman installer from the official Postman website.
- Run the downloaded installer and follow the on-screen instructions.
- Once the installation is complete, launch Postman from the Start menu or desktop shortcut.
- On the first launch, Postman may prompt you to sign in or create an account. You can skip this step and use Postman as a guest or sign in for additional collaboration features.
macOS
- Download the Postman installer from the official Postman website.
- Open the downloaded .dmg file and drag the Postman app to the Applications folder.
- Open Postman from the Applications folder.
- On the first launch, Postman may prompt you to sign in or create an account. You can skip this step and use Postman as a guest or sign in for additional collaboration features.
Linux
- Open a terminal and run the following command to download the Postman .tar file:
wget https://dl.pstmn.io/download/latest/linux64 -O postman.tar.gz
- Extract the downloaded file:
tar -xvzf postman.tar.gz
- Move the extracted folder to the desired location, for example:
sudo mv Postman /opt
- Create a symbolic link to easily launch Postman:
sudo ln -s /opt/Postman/Postman /usr/bin/postman
- Launch Postman from the terminal by typing:
postman
Setting Up Postman for the First Time
Once Postman is installed, you can begin setting it up for your API testing:
- When you first launch Postman, you will be prompted to sign in. You can either sign in with a Postman account or use Postman as a guest.
- After logging in, you will be taken to the Postman workspace. Workspaces allow you to organize and collaborate on collections, environments, and other resources.
- If you are working on a team project, you can create a new workspace or join an existing one by clicking on the "Workspaces" icon on the left sidebar.
- You can now start creating API requests by clicking the "New" button at the top left and selecting "Request" from the dropdown menu.
Postman Configuration Options
Postman also allows you to configure various settings to personalize your experience:
- Theme: Choose between light or dark themes from the settings menu.
- Proxy Settings: Configure proxy settings for requests under the "Settings" tab.
- Keyboard Shortcuts: You can customize keyboard shortcuts to speed up your workflow.
- Sync: If you're signed in, your collections, environments, and other data will be synced across all devices that you use Postman on.
Verifying Installation
To verify that Postman is installed correctly, follow these steps:
- Open Postman and click the "New" button.
- Create a simple API request, for example, a GET request to
https://jsonplaceholder.typicode.com/todos/1
to retrieve a sample JSON response. - If the request is successful and you see the response in Postman, your installation is complete and functioning correctly.
Code Example: Simple GET Request in Postman
Here's an example of how to create a simple GET request in Postman to fetch data from an API:
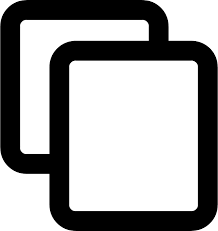
// Simple GET request in Postman
GET https://jsonplaceholder.typicode.com/todos/1
Diagram: Postman Installation Process
The following diagram illustrates the steps for installing and setting up Postman:

This diagram summarizes the process of downloading, installing, and configuring Postman for the first time.
Postman Web vs Desktop Application
Postman offers two main ways to use the tool: the Web version and the Desktop application. Both have similar core features, but there are key differences that might make one better suited for your workflow depending on your needs. In this section, we'll compare both versions to help you decide which one to use.
Postman Web
The Postman Web version allows you to access Postman directly through your browser. It's convenient for users who want to access Postman from any device without installing software. Here are some key features of the Postman Web version:
- Accessibility: Accessible from any device with an internet connection using a supported browser (Chrome, Firefox, etc.).
- No Installation Required: You don't need to install anything on your system. Simply sign in to your Postman account and start using it.
- Cloud Sync: Your data is automatically synced to the cloud, so you can access your collections, environments, and other data from any device.
- Limited Features: Some features available in the Desktop version, such as offline capabilities and performance optimizations, may not be available in the web version.
- Browser-Dependent: The performance and features are dependent on the browser you use.
Postman Desktop Application
The Postman Desktop application is a native application that you install on your computer. It offers a more robust and optimized experience, especially for heavy users. Here are some key features of the Postman Desktop application:
- Offline Capabilities: You can use Postman without an internet connection, making it ideal for working in environments where internet access is limited or unavailable.
- Faster Performance: The desktop version is generally faster than the web version, particularly when working with large collections or running complex tests.
- Native Integration: The desktop application integrates better with your system, offering features like native notifications, better memory management, and enhanced performance.
- Automatic Updates: Postman Desktop application automatically updates itself to the latest version, ensuring you always have the newest features and fixes.
- More Features: The desktop version includes all of Postman’s features, including advanced performance testing, local file handling, and better control over APIs and requests.
Comparison Table
Feature | Postman Web | Postman Desktop |
---|---|---|
Installation Required | No | Yes |
Offline Support | No | Yes |
Cloud Sync | Yes | Yes |
Performance | Depends on Browser | Optimized for Desktop |
Advanced Features | Limited | Full Feature Set |
Automatic Updates | No | Yes |
System Integration | Browser-Based | Native Integration |
Choosing the Right Version
The decision between using Postman Web or the Desktop application depends on your needs and working environment:
- If you need access to Postman from multiple devices or don’t want to worry about installing software, the Postman Web version might be the best option.
- If you're working on larger projects, need offline access, or require enhanced performance and features, the Postman Desktop application is likely the better choice.
Code Example: Using Postman Web and Desktop
Regardless of whether you're using the Web or Desktop version, the core functionality of Postman remains the same. Here's an example of how you can send a simple GET request to an API:
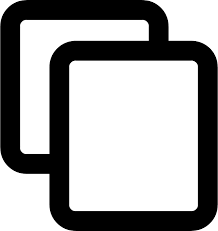
// Example GET request in Postman
GET https://jsonplaceholder.typicode.com/posts
Diagram: Postman Web vs Desktop Application
The following diagram compares the two versions of Postman in terms of features and performance:

This diagram summarizes the key differences between the Postman Web and Desktop applications, helping you make an informed decision on which version to choose.
Overview of Postman Interface
The Postman interface is designed to be intuitive and user-friendly, offering various features to facilitate API development and testing. Understanding the layout and key components of the interface is essential for effectively using Postman. In this section, we’ll walk you through the main sections of the Postman interface and their functionalities.
Key Components of the Postman Interface
The Postman interface consists of several key components that enable you to send requests, organize collections, manage environments, and analyze responses. Let’s explore these components:
- Request Builder: This is the main area where you build and send HTTP requests to an API. Here you can set the request method (GET, POST, PUT, etc.), enter the API endpoint URL, add headers, parameters, and body data, and send requests to test the API.
- Navigation Pane: Located on the left, the Navigation Pane allows you to organize and access your collections, environments, and history. It helps you manage your API projects and keep track of previous requests and responses.
- Response Section: The Response Section displays the response data from the API, including the status code, response body, headers, and other details. You can also inspect the response time and size here.
- Tabs: Postman allows you to open multiple tabs within the interface, so you can work on several requests simultaneously without losing your progress on others.
- Console: The Console is a log of all the requests and responses you’ve sent. It provides additional insights into your API calls and is useful for debugging and troubleshooting.
- Search Bar: The Search Bar helps you quickly find requests, collections, environments, and other items within the Postman interface. It’s particularly helpful when working with large projects.
Layout of the Postman Interface
The interface of Postman is divided into distinct sections, each serving a specific purpose. Below is a breakdown of these sections:
1. Request Builder Area
The Request Builder is the heart of Postman, where you compose HTTP requests. It includes several sub-sections:
- Method Selector: Choose the HTTP method (GET, POST, PUT, DELETE, etc.) for the request.
- URL Field: Enter the API endpoint URL for the request.
- Headers: Add custom headers to your request, such as authorization tokens or content-type headers.
- Body: If you're making a POST or PUT request, this is where you can specify the request body (e.g., JSON, form data, etc.).
- Send Button: Once you’ve configured your request, click the “Send” button to send the request to the API and view the response.
2. Response Section
The Response Section displays the results of your API call, including:
- Status Code: The HTTP status code returned by the API (e.g., 200 OK, 404 Not Found).
- Response Body: The body of the response, which can be in various formats such as JSON, XML, HTML, etc.
- Headers: The headers returned in the response, including content type, caching, and other metadata.
- Response Time: The time it took for the API to respond to the request.
- Response Size: The size of the response data, often displayed in KB or MB.
3. Navigation Pane
The Navigation Pane on the left side of the Postman interface is where you can:
- Collections: Organize your API requests into collections for better management. Collections are groups of related API requests that you can save, share, and reuse.
- Environments: Manage different environments (e.g., development, staging, production) that store variables such as API URLs, keys, and tokens.
- History: View the history of your previous requests. You can quickly re-send past requests without re-entering the information manually.
4. Tabs
Postman supports multiple tabs, allowing you to work on different API requests at the same time. Each tab is independent, so you can keep track of multiple requests and responses without losing any context.
5. Console
The Console is a powerful tool for debugging and troubleshooting. It logs all requests and responses, along with any errors or issues encountered during the request process. You can access the Console by clicking the “Console” button at the bottom of the interface.
Code Example: Basic Request in Postman
Here is an example of how to send a simple GET request using the Postman interface:
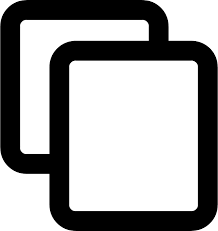
// Example GET request in Postman
GET https://jsonplaceholder.typicode.com/posts
Diagram: Postman Interface Layout
The diagram below shows the layout of the Postman interface, highlighting the key sections mentioned above:

This diagram provides a visual overview of the Postman interface, helping you navigate and utilize the tool effectively.
Creating and Sending Your First Request
In this section, you will learn how to create and send your first HTTP request using Postman. This is an essential step in working with APIs, as it allows you to interact with the API endpoints and view the responses.
Steps to Create a Request
Follow these simple steps to create and send your first request using Postman:
- Open Postman: Launch the Postman application on your computer.
- Create a New Request: Click the New button in the top left of the interface and select Request from the dropdown menu.
- Set the Request Method: In the Request Builder section, choose the HTTP method (e.g., GET, POST) for your request. For this example, select GET.
- Enter the Request URL: In the URL field, enter the endpoint you want to send the request to. For example, use the following placeholder API URL to retrieve a list of posts:
https://jsonplaceholder.typicode.com/posts
- Send the Request: Once you have set the request method and URL, click the Send button. Postman will send the request to the specified API endpoint.
- View the Response: After sending the request, Postman will display the response in the Response Section. You can inspect the status code, response body, headers, and other details.
Understanding the Response
Once the request is sent, you’ll see the response from the server. The response includes the following key information:
- Status Code: The HTTP status code returned by the server (e.g., 200 OK, 404 Not Found).
- Response Body: The content of the response. In this case, it will be a list of posts in JSON format.
- Headers: The headers returned by the API. Headers may include content type, authorization information, etc.
- Response Time: The time it took for the server to process and respond to the request.
Code Example: Making a Simple GET Request
Here's an example of how the GET request and response may look in Postman:
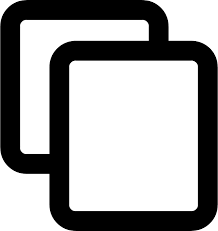
// Simple GET request in Postman
GET https://jsonplaceholder.typicode.com/posts
Diagram: Request and Response Flow
The diagram below illustrates the flow of creating and sending an HTTP request using Postman:

This diagram shows the process of making an API request, sending it to the server, and receiving the response.
GET, POST, PUT, DELETE, and Other HTTP Methods
HTTP methods define the action to be performed on a particular resource on the server. Postman allows you to send requests using various HTTP methods to interact with APIs. Each HTTP method serves a specific purpose when communicating with an API.
Common HTTP Methods
Here are the most commonly used HTTP methods:
- GET: The GET method retrieves data from the server. It is a read-only operation and does not alter the state of the resource.
- POST: The POST method sends data to the server to create a new resource. It is often used when submitting forms or sending data to the server.
- PUT: The PUT method updates an existing resource on the server. It typically requires the full resource data in the request body.
- DELETE: The DELETE method removes a specified resource from the server.
- PATCH: The PATCH method is used to update a resource partially. It only requires the changes to be sent, not the entire resource.
- HEAD: The HEAD method is similar to GET but only retrieves the headers of a resource, not the body.
- OPTIONS: The OPTIONS method returns the allowed HTTP methods for a specific resource, helping clients understand which actions are permissible.
Using HTTP Methods in Postman
In Postman, you can easily switch between different HTTP methods by selecting the appropriate option from the dropdown list in the request builder. Below are examples of how each HTTP method is used:
Example 1: GET Request
The GET method is used to request data from a specified resource. For example, to retrieve a list of posts from an API:
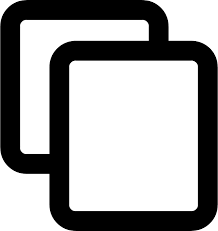
// GET request to fetch posts
GET https://jsonplaceholder.typicode.com/posts
Example 2: POST Request
The POST method is used to send data to the server to create a new resource. Here's an example of creating a new post:
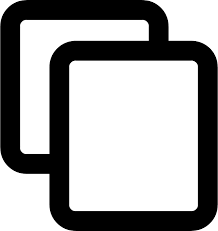
// POST request to create a new post
POST https://jsonplaceholder.typicode.com/posts
{
"title": "New Post",
"body": "This is the content of the new post.",
"userId": 1
}
Example 3: PUT Request
The PUT method is used to update an existing resource. Here is an example of updating a post:
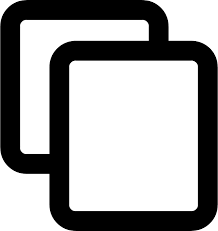
// PUT request to update a post
PUT https://jsonplaceholder.typicode.com/posts/1
{
"title": "Updated Post",
"body": "This content has been updated.",
"userId": 1
}
Example 4: DELETE Request
The DELETE method is used to delete a specified resource. For example, here’s how to delete a post:
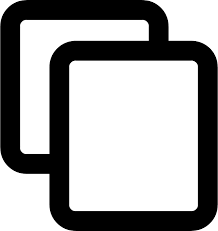
// DELETE request to remove a post
DELETE https://jsonplaceholder.typicode.com/posts/1
When to Use Each HTTP Method
Each HTTP method is designed for a specific purpose when interacting with APIs:
- GET: Use GET when you only need to retrieve data without making any changes to the resource.
- POST: Use POST when you need to create a new resource on the server, such as submitting a form or creating a new record.
- PUT: Use PUT when you need to update an existing resource completely with new data.
- DELETE: Use DELETE to remove a resource from the server.
- PATCH: Use PATCH to apply partial updates to a resource, especially when updating only specific attributes.
Code Example: HTTP Methods Summary
Here’s a quick summary of how the HTTP methods might look in Postman:
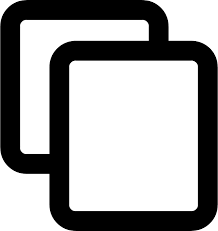
// Example of different HTTP methods in Postman
// GET request: Retrieve posts
GET https://jsonplaceholder.typicode.com/posts
// POST request: Create a new post
POST https://jsonplaceholder.typicode.com/posts
{
"title": "New Post",
"body": "Content of the post",
"userId": 1
}
// PUT request: Update an existing post
PUT https://jsonplaceholder.typicode.com/posts/1
{
"title": "Updated Post",
"body": "Updated content",
"userId": 1
}
// DELETE request: Delete a post
DELETE https://jsonplaceholder.typicode.com/posts/1
Diagram: HTTP Methods Overview
The diagram below shows a visual representation of the different HTTP methods and their typical uses:

This diagram helps to understand when each HTTP method should be used during API interactions.
Using Query Parameters, Headers, and Body
When sending requests to APIs, Postman allows you to add various components like query parameters, headers, and body content to customize your request. These elements play a critical role in defining how the request is handled and what data is sent to the server.
Query Parameters
Query parameters are key-value pairs that are appended to the URL to provide additional information to the server. These parameters are often used for filtering data, specifying page numbers, or passing other dynamic values.
In Postman, you can add query parameters easily in the "Params" tab under the request URL. For example, a request URL with query parameters might look like this:
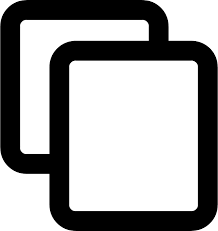
// Example URL with query parameters
GET https://jsonplaceholder.typicode.com/posts?userId=1&limit=10
In this case, the query parameters are userId and limit, which would filter the posts by a specific user and limit the number of posts returned.
Headers
Headers contain metadata about the request or response, such as authentication tokens, content type, or other information the server needs to know about the request. Common headers include Content-Type, Authorization, and Accept.
In Postman, you can add headers by switching to the "Headers" tab. Here’s an example of a request with headers:
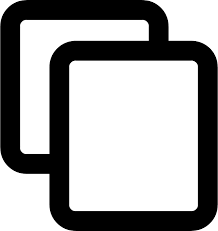
// Example of request headers
GET https://jsonplaceholder.typicode.com/posts
Headers:
Authorization: Bearer your_api_token
Content-Type: application/json
In this example, the Authorization header is used to send a Bearer token for authentication, and the Content-Type header specifies that the request body is in JSON format.
Request Body
The request body is used to send data to the server, typically for POST or PUT requests. The body can be sent in various formats, such as JSON, XML, or form data.
In Postman, you can specify the body content using the "Body" tab. Depending on the request type, you can choose different body formats such as raw, form-data, or x-www-form-urlencoded. Below is an example of sending JSON data in a POST request:
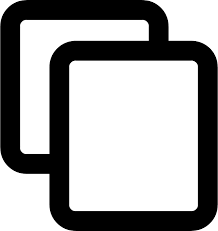
// Example of sending JSON data in the body
POST https://jsonplaceholder.typicode.com/posts
Body (raw, JSON):
{
"title": "New Post",
"body": "This is the content of the new post.",
"userId": 1
}
In this POST request, we send JSON data in the body to create a new post on the server. The data is sent in the raw format, and the Content-Type header indicates that the body contains JSON.
Using URL, Query Parameters, Headers, and Body Together
In many API requests, you will need to use query parameters, headers, and request bodies together to fully customize your request. Below is an example that combines all three elements:
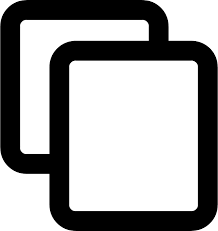
// Example with URL, query parameters, headers, and body
PUT https://jsonplaceholder.typicode.com/posts/1?userId=1
Headers:
Authorization: Bearer your_api_token
Content-Type: application/json
Body (raw, JSON):
{
"title": "Updated Post",
"body": "This content has been updated.",
"userId": 1
}
This PUT request updates an existing post with new data. The URL contains a query parameter for filtering, the headers provide authentication and content type, and the body contains the updated data.
Common Use Cases
Here are some common scenarios where query parameters, headers, and body content are used:
- Filtering Data: Use query parameters to filter results, such as specifying which user’s data to retrieve (e.g.,
?userId=1
). - Authentication: Use headers to include authentication tokens (e.g.,
Authorization: Bearer token
) to access protected resources. - Submitting Forms: Use the body to send form data in POST or PUT requests. This can include JSON, XML, or multipart form data.
Code Example: Query Parameters, Headers, and Body
Here’s an example that combines query parameters, headers, and body content in a single request:
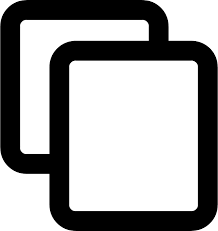
// GET request with query parameters, headers, and body
GET https://jsonplaceholder.typicode.com/posts?userId=1&limit=10
Headers:
Authorization: Bearer your_api_token
Accept: application/json
Body (raw, JSON):
{
"title": "New Post",
"body": "This is some content",
"userId": 1
}
Diagram: Request Components Overview
The following diagram illustrates the different components of an HTTP request, including the URL, query parameters, headers, and body:

This diagram helps to visualize how query parameters, headers, and request bodies work together in a typical HTTP request.
Creating and Managing Collections
Collections in Postman are a way to organize and group API requests. They allow you to save, share, and reuse your requests efficiently. Postman Collections are ideal for organizing test cases, documentation, and workflows for APIs.
What is a Collection?
A Postman Collection is a group of saved API requests that are organized in a folder structure. Collections can contain requests of different types (GET, POST, PUT, DELETE, etc.), and you can add variables, tests, and scripts to each request within the collection. Collections help you keep your API testing organized, making it easier to run tests and share them with your team.
Creating a New Collection
To create a new collection in Postman, follow these simple steps:
- Click on the Collections tab in the left sidebar.
- Click the New Collection button (a plus icon) at the top of the sidebar.
- Enter a name for your collection and optionally add a description.
- Click the Create button to save the collection.
Once you have created a collection, you can start adding requests to it.
Adding Requests to a Collection
After creating a collection, you can add requests to it by following these steps:
- Create a new request by selecting the New button in the top left corner.
- In the request window, configure the HTTP method and URL for your request.
- Click the Save button.
- In the "Save Request" dialog, select the collection you want to add the request to and give it a name.
- Click Save again to add the request to the selected collection.
Managing Collections
Once you have created a collection and added requests to it, you can manage and organize your collections. Here are some common management tasks:
- Reordering Requests: You can easily reorder requests within a collection by dragging and dropping them in the sidebar.
- Creating Folders: To keep your requests organized, you can create folders within collections. Right-click on the collection and select New Folder.
- Renaming and Deleting Requests: Right-click on a request to rename or delete it. You can also rename or delete a collection itself by right-clicking on the collection name.
- Adding Variables: You can add environment or global variables to your collection to make it more dynamic. These variables can be reused across multiple requests within the collection.
Running Collections
Postman allows you to run collections directly from the app. Running a collection can be useful for testing multiple requests sequentially, running tests, and validating your API workflows. To run a collection:
- Click on the collection name in the left sidebar to open it.
- Click the Run button at the top of the collection details page.
- The Postman Collection Runner window will open, allowing you to select options like the environment to use, the number of iterations, and data files (e.g., CSV or JSON).
- Click the Start Run button to execute the collection.
Sharing Collections
Postman provides an easy way to share your collections with teammates. You can share collections in the following ways:
- Public Link: You can generate a public link to share your collection with others. This link allows others to view and use the collection in their Postman app.
- Team Workspaces: If you're working in a team, you can share your collections within a Postman Workspace. All team members can access and collaborate on the collection in real time.
- Exporting: You can export collections as JSON files and share them with others. To export a collection, right-click on the collection and select Export, then choose the export format.
Code Example: Collection of Requests
Let’s say you have created a collection with three different requests: a GET request to fetch user details, a POST request to create a new user, and a PUT request to update user data. Here’s an example of how the requests might be organized in a collection:
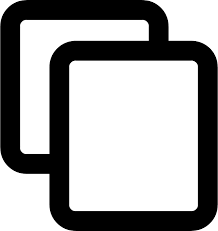
// GET request to fetch user details
GET https://jsonplaceholder.typicode.com/users/1
// POST request to create a new user
POST https://jsonplaceholder.typicode.com/users
Body (raw, JSON):
{
"name": "John Doe",
"email": "john@example.com"
}
// PUT request to update user details
PUT https://jsonplaceholder.typicode.com/users/1
Body (raw, JSON):
{
"name": "John Doe Updated",
"email": "john.updated@example.com"
}
In this example, the collection contains three requests. Each one has its own HTTP method, URL, and request body (for POST and PUT requests).
Diagram: Collection Structure
The following diagram illustrates the structure of a Postman collection, with requests organized into folders:

This diagram shows how you can organize requests within folders and manage your API testing workflows efficiently.
Adding Requests to Collections
Postman allows you to organize your API requests into collections. Once you have a collection, you can add individual requests to it. This helps you keep related requests together, making it easier to test and manage your API workflow.
Why Add Requests to Collections?
Adding requests to collections helps you organize your tests, manage APIs, and easily run groups of requests in sequence. It also helps with sharing your work with others by keeping all related requests grouped together.
Steps to Add Requests to a Collection
Follow these steps to add a new request to an existing collection:
- Click on the New button in the top left corner of the Postman app.
- Choose the type of request (GET, POST, PUT, DELETE, etc.) from the dropdown and fill in the request details like URL, headers, and body as needed.
- Once you've configured the request, click on the Save button located in the upper-right corner of the request tab.
- In the Save Request dialog, select the collection where you want to save the request. If you want to save it to a new collection, click Create New Collection.
- Give your request a name and (optionally) a description to help identify its purpose later.
- Click Save to add the request to the selected collection.
Adding Requests to an Existing Collection
If you already have a collection, you can easily add new requests to it. Follow these steps:
- Open the collection in the left sidebar by clicking on its name.
- Click the New Request button inside the collection.
- Fill in the request details such as HTTP method, URL, headers, and body.
- Click Save, and the request will be saved directly into the collection.
Managing Requests in Collections
Once requests are added to a collection, you can manage them effectively:
- Reordering Requests: You can drag and drop requests to reorder them within a collection.
- Creating Folders: Within a collection, you can create folders to further organize your requests. Right-click on the collection name and select New Folder to start organizing requests into subcategories.
- Editing Requests: To edit an existing request, click on the request, make your changes, and save it again. You can also rename requests or update descriptions to keep your collection organized.
- Deleting Requests: Right-click on a request within a collection and select Delete if you no longer need it.
Using Variables in Requests
Postman allows you to use variables in your requests to make them more flexible. You can add environment or global variables that will be replaced at runtime. Here's how you can use variables in your requests:
- In the request URL, headers, or body, you can use variables by wrapping them in
{{variableName}}
. - For example, you can set a variable for the base URL like
{{baseUrl}}/users
. When the request runs, the variable will be replaced with the actual value. - To define a variable, go to the Environment tab, add a new environment or select an existing one, and add key-value pairs for your variables.
Example: Adding a Request to a Collection
Here’s an example of how to add a GET request to a collection:
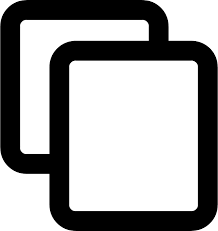
// GET request to fetch user details
GET https://jsonplaceholder.typicode.com/users/1
Once you've created this GET request and added it to your collection, you can run it along with other requests, share it, and include it in your test sequences.
Diagram: Request and Collection Structure
The following diagram illustrates how a request fits into a collection:

This diagram shows how requests are nested within collections and how you can organize your API testing workflow.
Grouping Requests and Using Folders
As your collection grows, it can become overwhelming to manage a large number of requests. Postman provides a solution by allowing you to group related requests into folders within your collections. This makes your workspace more organized, and it helps you easily navigate through requests.
Why Use Folders?
Folders allow you to organize your requests into categories or logical groupings, making it easier to manage and navigate through your test suites. For example, you can group requests by functionality, user stories, or any other logical structure that fits your testing needs. Some benefits of using folders include:
- Better Organization: Keeping related requests together helps with clarity and structure.
- Improved Navigation: Folders reduce the clutter in your workspace, making it easier to find the requests you need.
- Better Collaboration: When sharing collections, grouping requests in folders allows team members to focus on specific areas of the project.
How to Create Folders in Postman
Creating folders in Postman is simple. Follow the steps below:
- Open the collection where you want to add folders.
- Click on the three dots (More Options) next to the collection name in the left sidebar.
- Select New Folder from the dropdown menu.
- Give the folder a descriptive name, such as "User Authentication" or "API Endpoints".
- Click Create to add the folder to the collection.
Adding Requests to Folders
Once you've created a folder, you can easily add requests to it. Follow these steps to add a request to a folder:
- Click on a request and open it.
- Click on the Save button in the top-right corner of the request tab.
- In the Save Request dialog, choose the folder you want to add the request to.
- If you want to create a new folder within an existing collection, click on New Folder.
- Click Save to save the request to the selected folder.
Organizing Requests with Multiple Folders
As your collection grows, you might want to organize requests into multiple folders. You can create subfolders within folders, allowing for a more granular organization. Here's how you can create subfolders:
- Click on the folder where you want to create a subfolder.
- Click the three dots next to the folder name.
- Select New Folder to create a subfolder.
- Give the subfolder a meaningful name and click Create.
Example: Organizing API Endpoints
Let’s say you are testing a user management API. You can create folders like this:
- User Authentication: Contains requests for login, registration, and password reset.
- User Profile: Includes requests for viewing and updating user profiles.
- Admin Endpoints: Includes requests for admin-specific actions such as creating and deleting users.
Managing Folders
Once folders are created, you can:
- Reorder Folders: Drag and drop folders within your collection to reorder them as per your preference.
- Delete Folders: Right-click on a folder and select Delete if you no longer need it.
- Edit Folders: You can edit the folder name or description by right-clicking on the folder and selecting Edit.
Diagram: Folder Structure
The following diagram shows how requests can be grouped into folders within a collection:

This diagram illustrates how folders help you organize your requests and keep your collections well-structured.
Duplicating and Sharing Requests
In Postman, you can easily duplicate and share requests, making it convenient to reuse requests or collaborate with team members. Below, we’ll walk through how to duplicate a request and share it with others.
Why Duplicate Requests?
Duplicating requests is useful when you need to create variations of an existing request without altering the original. For example, if you have a GET request for fetching user data and you want to test it with different query parameters, duplicating the request allows you to make changes without modifying the original request.
- Speed up testing: Duplicating a request allows you to quickly create similar requests without having to rebuild them from scratch.
- Preserve original configurations: By duplicating a request, you ensure that the original configuration remains unchanged while you experiment with new variations.
How to Duplicate a Request in Postman
Follow these steps to duplicate a request:
- Open the request you want to duplicate.
- Click on the three dots (More Options) next to the request name in the request tab.
- Select Duplicate from the dropdown menu.
- A new tab with the duplicated request will appear, and you can modify it as needed.
Why Share Requests?
Sharing requests allows you to collaborate with your team members or share your work with others. You can share requests to ensure that everyone is testing the same API endpoints with the same configurations, making the testing process more efficient and consistent.
- Collaboration: Share requests with your colleagues to work on the same API tests in real-time.
- Consistency: Ensure everyone in the team is using the same request configurations, reducing errors and discrepancies.
How to Share a Request in Postman
Follow these steps to share a request with others:
- Open the request you want to share.
- Click on the Share button located on the top-right corner of the request tab.
- Choose the sharing method. You can:
- Share via Link: Share a link to the request. This method allows others to view and fork the request.
- Share to Workspace: Share the request to a Postman workspace, allowing team members in that workspace to access it.
- Export Request: Export the request as a file and share it manually. This is useful if you want to send it over email or through another communication channel.
- Click Send or Copy Link to share the request via the chosen method.
Sharing Requests in Team Workspaces
In Postman, you can also create or join team workspaces where you can share requests, collections, environments, and more with your team members. Sharing within a workspace ensures that all team members have access to the same requests and can collaborate effectively.
- Create a Workspace: Go to the Workspaces tab in Postman and click Create Workspace to create a new workspace for your team.
- Share Requests: Once the workspace is created, you can share requests by saving them to that workspace. Team members with access to the workspace can then view and modify the requests.
Example: Duplicating and Sharing a Test Request
Let’s say you have a GET request to fetch user details, and you want to test the same endpoint with different user IDs. You can duplicate the request and modify the user ID parameter each time. Then, you can share the collection with your team to ensure they are testing the same API endpoint with the same configurations.
Diagram: Duplicating and Sharing Requests
The following diagram illustrates how you can duplicate and share requests with others:

This diagram explains the process of duplicating a request and sharing it with team members via different methods.
Importing APIs from OpenAPI/Swagger
Postman allows you to import APIs from OpenAPI (formerly known as Swagger) specifications, making it easy to work with API definitions in a standardized format. OpenAPI/Swagger is a widely-used specification that describes REST APIs, and Postman supports importing these definitions to generate collections, requests, and more for easy testing and collaboration.
Why Import APIs from OpenAPI/Swagger?
Importing APIs from OpenAPI/Swagger offers several advantages:
- Standardized Format: OpenAPI and Swagger offer a clear, structured way to define APIs, making it easier to understand and work with APIs.
- Speed Up Testing: Importing API definitions directly into Postman eliminates the need to manually create requests and configurations, saving time during the testing phase.
- Consistency: By importing the API definition, you ensure that the requests, endpoints, and data structures are consistent with the official API documentation.
How to Import OpenAPI/Swagger Files in Postman
Follow these steps to import an OpenAPI/Swagger file into Postman:
- Click on the Import button in the top left corner of Postman.
- In the Import window, you can either drag and drop your OpenAPI/Swagger file or click on the Upload Files button to browse and select the file from your system.
- Select the file in JSON or YAML format (Swagger API specifications are often available in either of these formats). Postman also supports importing from URLs that point to a hosted OpenAPI or Swagger file.
- Once the file is imported, Postman will automatically generate a collection with the endpoints and methods defined in the API specification.
- You can now view the requests and their corresponding parameters, headers, body, and responses directly in Postman.
Importing OpenAPI/Swagger from a URL
If you have a URL that points to an OpenAPI/Swagger specification, you can import it directly into Postman:
- Click on the Import button in the top left corner of Postman.
- Select the Link tab in the Import window.
- Paste the URL of the OpenAPI/Swagger file into the provided field.
- Postman will fetch the file from the URL and generate the collection based on the API definition.
Working with Imported APIs
Once the OpenAPI/Swagger file is imported, you can begin using it in Postman:
- View Endpoints: All the defined API endpoints will appear in the generated collection, organized by categories or tags defined in the OpenAPI specification.
- Test API Requests: You can directly send requests to the API and view responses without needing to manually configure each request.
- Modify Requests: Customize requests as needed by adding or modifying query parameters, headers, body data, etc.
- Generate Documentation: Postman also allows you to generate and share documentation for the imported API, making collaboration with team members and external users easier.
Example: Importing an OpenAPI Specification
Let’s say you have an OpenAPI specification for a hypothetical e-commerce API available at https://example.com/api/openapi.json
. To import it into Postman:
- Click on the Import button in Postman.
- Go to the Link tab and paste the URL
https://example.com/api/openapi.json
. - Click Import, and Postman will generate a collection with all the API endpoints from the OpenAPI specification.
Diagram: Importing OpenAPI/Swagger API
The following diagram illustrates the flow of importing and utilizing OpenAPI/Swagger files in Postman:

The diagram shows how to import an API definition from a Swagger/OpenAPI file and start interacting with the API endpoints in Postman.
Sending Data in Request Body (form-data, x-www-form-urlencoded, raw, binary)
When sending requests in Postman, the request body can be used to send data to the server. Depending on the type of data you need to send, you can choose from several content types, such as form-data, x-www-form-urlencoded, raw, or binary.
1. Form-Data
form-data is typically used when submitting data through HTML forms, especially when the request includes files. It allows you to send data as key-value pairs, where each key represents the form field, and the value represents the input provided by the user.
In Postman, you can set the body to form-data when sending multipart/form-data requests. Here’s how you can use it:
- Select the Body tab in Postman.
- Choose the form-data option.
- Enter key-value pairs for the fields you want to send.
- If you need to upload a file, click the Select Files button and choose the file to include in the request.
This type of request is often used for file uploads or when sending data from an HTML form.
2. x-www-form-urlencoded
x-www-form-urlencoded is a format used to encode key-value pairs. It is similar to form-data, but the data is URL-encoded and sent as a single string. This format is commonly used for submitting form data in HTTP requests, especially for APIs that don’t require file uploads.
To send data with x-www-form-urlencoded in Postman:
- Select the Body tab in Postman.
- Choose the x-www-form-urlencoded option.
- Enter the key-value pairs as required by the API.
The data will be encoded in the URL query string format, making it suitable for APIs expecting form data in this format.
3. Raw
raw is used to send data as plain text or JSON, which is often required when interacting with RESTful APIs. This is useful for sending JSON, XML, or other custom data formats in the request body.
To send data as raw in Postman:
- Select the Body tab in Postman.
- Choose the raw option.
- In the dropdown next to the raw option, select the format (e.g., JSON, Text, XML) in which the data will be sent.
- Enter your raw request body. For example, if sending JSON, you could enter:
{
"name": "John Doe",
"email": "john.doe@example.com"
}
Raw data is typically used when your API requires JSON or XML payloads.
4. Binary
binary data is used for sending files in the request body. This is often used when you need to upload files such as images, videos, or documents to an API.
To send binary data in Postman:
- Select the Body tab in Postman.
- Choose the binary option.
- Click on the Select File button and choose the file you wish to upload.
Postman will send the file as binary data in the request body. This is essential for APIs that require file uploads.
Diagram: Request Body Formats
The following diagram explains the four different ways of sending data in the request body:

The diagram illustrates the differences between form-data, x-www-form-urlencoded, raw, and binary data formats.
Uploading Files via Postman
Uploading files through Postman is a straightforward process that allows you to send files to an API endpoint. This is especially useful when working with APIs that require file uploads, such as image or document uploading endpoints.
1. Setting Up the Request for File Upload
To upload a file using Postman, you need to send the file as part of the request body, typically as form-data or binary.
Using Form-Data for File Upload
form-data is used when you want to upload files along with other form data (e.g., name, description). In this case, the file is included as a key-value pair in the form data, where the key is the field name and the value is the file you want to upload.
Follow these steps to upload a file using form-data in Postman:
- Select the Body tab in Postman.
- Choose the form-data option.
- In the key column, enter the field name that the API expects for the file upload (e.g., file, image, etc.).
- In the value column, click on the Select Files button to browse and choose the file you want to upload.
- If needed, add other form fields by providing additional key-value pairs (e.g., name, description).
Postman will send the file as part of the form-data request to the server.
Using Binary for File Upload
For some APIs, you might need to send the file directly as binary data without any additional form fields. In this case, you can use the binary option to upload the file.
Follow these steps to upload a file as binary data in Postman:
- Select the Body tab in Postman.
- Choose the binary option.
- Click on the Select File button to browse and choose the file you wish to upload.
When you select the file, Postman will include it in the request body as raw binary data. This is ideal for APIs that expect files to be sent without any form fields.
2. Example File Upload
Let’s consider an example where you want to upload an image to a server using Postman. Below is how you would set up the request:
- Choose the POST method for the request.
- Enter the API endpoint URL (e.g.,
https://api.example.com/upload
). - In the Body tab, choose form-data.
- Enter file in the key column (or the field name expected by the API) and click Select Files to choose the image you want to upload.
- If the API requires additional fields (e.g., description), add them as key-value pairs.
- Click Send to upload the file to the server.
Postman will upload the image as part of the request body, and the server will process the uploaded file accordingly.
3. Handling File Upload Responses
After sending the file upload request, you’ll receive a response from the server. The response might include information about the uploaded file, such as a success message, file URL, or other metadata.
For example, a successful file upload might return a response like:
{
"success": true,
"message": "File uploaded successfully",
"fileUrl": "https://example.com/uploads/file.jpg"
}
You can inspect the response body to verify the success of the upload and retrieve any necessary information about the uploaded file.
4. Troubleshooting File Uploads
If you encounter issues while uploading files via Postman, consider the following troubleshooting tips:
- Check the API Documentation: Ensure you are using the correct field name for the file upload and that the server supports the type of file you are uploading.
- File Size Limits: Some APIs may have file size limits. Verify the file size and ensure it doesn’t exceed the maximum allowed by the API.
- Response Codes: Look for any error response codes (e.g.,
400
or413
) which may indicate issues like invalid file types or file size limits.
Diagram: File Upload Process
The following diagram illustrates the process of uploading a file via Postman:

This diagram shows the key steps in sending a file upload request and handling the server's response.
Adding API Keys, Bearer Tokens, and Basic Auth
When interacting with APIs, authentication is often required to ensure that only authorized users can access the data. Postman supports several authentication methods, such as API keys, bearer tokens, and basic authentication. In this section, we will explore how to add and use each of these authentication methods in Postman.
1. Adding API Keys
API keys are a common way of authenticating requests to an API. Many APIs require an API key to be sent as part of the request headers or query parameters.
Adding API Key as a Header
Follow these steps to add an API key to the request headers in Postman:
- Click on the Headers tab in Postman.
- In the Key column, enter the key name expected by the API (e.g., Authorization or x-api-key).
- In the Value column, enter the API key provided by the API service.
The API key will be included in the request headers, allowing you to authenticate your request.
Adding API Key as a Query Parameter
Alternatively, some APIs require the API key to be sent as a query parameter in the URL. Here’s how to add it:
- Click on the Params tab in Postman.
- In the Key column, enter the parameter name (e.g., api_key).
- In the Value column, enter your API key.
- Postman will automatically append the API key as a query parameter in the request URL.
2. Adding Bearer Tokens
Bearer tokens are commonly used in OAuth 2.0 authentication. The token is included in the request header to authenticate the request.
Adding Bearer Token to the Header
Follow these steps to add a bearer token to the request headers in Postman:
- Click on the Authorization tab in Postman.
- Select Bearer Token from the Type dropdown.
- In the Token field, enter the bearer token you received from the authentication service.
Once the bearer token is added, Postman will send it in the request header as Authorization: Bearer <token>.
3. Adding Basic Authentication
Basic Authentication requires sending a username and password encoded as a base64 string in the request header. Postman provides an easy way to add basic authentication to your requests.
Adding Basic Auth to the Request
Follow these steps to use basic authentication in Postman:
- Click on the Authorization tab in Postman.
- Select Basic Auth from the Type dropdown.
- Enter your Username and Password in the respective fields.
Postman will automatically encode the username and password into a base64 string and include it in the request header as Authorization: Basic <base64-encoded string>.
4. Example: Using API Key, Bearer Token, and Basic Auth
Let’s walk through an example where you might use each of these methods:
- API Key: You are accessing a weather API. You include the API key in the headers or as a query parameter.
- Bearer Token: You are accessing a protected resource using OAuth 2.0, so you include the bearer token in the request header.
- Basic Auth: You are accessing an internal API that uses basic authentication. You provide a username and password.
5. Troubleshooting Authentication Issues
If you encounter issues with authentication, consider the following:
- Incorrect API Key: Double-check the key and ensure that it's placed in the correct part of the request (header or query string).
- Expired Bearer Token: Bearer tokens often expire after a set period. Ensure that you are using a valid token.
- Basic Auth Errors: If you receive a 401 Unauthorized error, check that your username and password are correct and properly encoded.
Diagram: Authentication Process
The following diagram explains the authentication process using API keys, bearer tokens, and basic auth:

This diagram illustrates how the API key, bearer token, and basic authentication are sent in the request header and how they are used for authentication.
OAuth 2.0 Authorization in Postman
OAuth 2.0 is a popular authorization framework that allows third-party applications to securely access user data without exposing the user's credentials. Postman supports OAuth 2.0 for testing APIs that require user authorization via tokens. In this section, we will walk through the process of using OAuth 2.0 in Postman to authenticate API requests.
1. What is OAuth 2.0?
OAuth 2.0 is an authorization protocol that enables applications to act on behalf of a user by obtaining an access token. This access token is used to authenticate API requests. OAuth 2.0 is widely used in APIs for services like Google, Facebook, and GitHub.
2. Setting Up OAuth 2.0 in Postman
To set up OAuth 2.0 authentication in Postman, follow these steps:
- Click on the Authorization tab in your Postman request.
- In the Type dropdown, select OAuth 2.0.
- Click on the Get New Access Token button to initiate the OAuth 2.0 flow.
3. Configuring OAuth 2.0 Settings
After clicking Get New Access Token, a new modal will appear with the following fields to configure OAuth 2.0:
Field | Description |
---|---|
Auth URL | The URL where the user will be redirected to authenticate. This is provided by the API's OAuth provider. |
Access Token URL | The URL that Postman will use to exchange the authorization code for an access token after the user authorizes the request. |
Client ID | The unique identifier issued by the OAuth provider when you register your app (e.g., Google, Facebook). |
Client Secret | The secret issued by the OAuth provider, used to authenticate your application. Keep it confidential. |
Scope | The permissions that your application needs. Scopes are typically specified as a space-separated list (e.g., profile email). |
State | An optional parameter to maintain state between the request and callback. It helps prevent CSRF attacks. |
Client Authentication | Choose how the client authentication should be sent (e.g., Send as Basic Auth header). |
4. Obtaining the Access Token
Once you have configured the OAuth 2.0 settings, click on the Request Token button. This will initiate the OAuth 2.0 flow:
- Postman will redirect you to the OAuth provider's authorization page, where you can log in and authorize access.
- Once the user authorizes the request, the OAuth provider will redirect you back to Postman with the authorization code.
- Postman will automatically exchange the authorization code for an access token using the Access Token URL.
- If the process is successful, Postman will display the obtained access token in the Access Token field.
5. Using the Access Token
After obtaining the access token, Postman will automatically add the token to the request header as Authorization: Bearer <access_token> when you send the API request. This allows you to make authenticated requests to the API on behalf of the user.
6. Refreshing the Access Token
OAuth 2.0 access tokens may expire after a set period. If the OAuth provider supports it, Postman can automatically refresh the access token:
- In the OAuth 2.0 Settings, you can specify the Refresh Token URL.
- Once the access token expires, Postman will use the refresh token to obtain a new access token without requiring the user to log in again.
7. Example: OAuth 2.0 Flow
Let’s take an example of authenticating with Google’s OAuth 2.0 service:
- Client ID: YOUR_GOOGLE_CLIENT_ID
- Client Secret: YOUR_GOOGLE_CLIENT_SECRET
- Auth URL: https://accounts.google.com/o/oauth2/auth
- Access Token URL: https://oauth2.googleapis.com/token
- Scope: https://www.googleapis.com/auth/userinfo.email
Once you configure the OAuth details in Postman, click on Get New Access Token and follow the OAuth flow to authenticate and obtain the token.
8. Troubleshooting OAuth 2.0 Issues
If you encounter issues with the OAuth 2.0 flow, consider the following troubleshooting steps:
- Invalid Client ID or Secret: Ensure that the client ID and client secret are correct and have been properly registered with the OAuth provider.
- Incorrect Scopes: Verify that the scopes you requested match the permissions required by the API.
- Expired Access Token: If the access token has expired, use the refresh token to obtain a new token or reauthorize the user.
- Invalid Redirect URI: Ensure that the redirect URI used in Postman matches the one registered with the OAuth provider.
Diagram: OAuth 2.0 Flow
The following diagram shows the OAuth 2.0 authorization flow:

This diagram illustrates the step-by-step process of obtaining an access token through OAuth 2.0 and how it is used to authenticate API requests.
Digest and Hawk Authentication
In addition to standard OAuth 2.0 and Basic Auth, Postman also supports Digest and Hawk authentication methods. These authentication mechanisms are often used for securing API requests, especially in scenarios where sensitive data is transmitted or when additional security measures are required.
1. Digest Authentication
Digest Authentication is a more secure alternative to Basic Authentication. It involves hashing the credentials and using a challenge-response mechanism to verify the identity of the client. Unlike Basic Authentication, Digest Authentication does not send the password in plain text but instead sends a hashed value.
How Digest Authentication Works:
- The client sends a request to the server without authentication.
- The server responds with a 401 Unauthorized status code and a WWW-Authenticate header containing a nonce and other challenge parameters.
- The client then computes a hash of the credentials, including the nonce, and sends a response with the Authorization header containing the hash.
- If the server verifies the hash, it grants access to the client.
Setting Up Digest Authentication in Postman:
To use Digest Authentication in Postman:
- In the Postman request, select the Authorization tab.
- From the Type dropdown, choose Digest Auth.
- Fill in the required fields:
- Username: The username for authentication.
- Password: The password associated with the username.
- Realm: The authentication realm (provided by the server).
- Nonce: The nonce value received from the server's 401 response.
- URI: The URI being requested.
- Once the fields are populated, Postman will automatically handle the hashing and include the Authorization header in the request.
2. Hawk Authentication
Hawk Authentication is a protocol that is designed for providing security for HTTP-based services. It uses a combination of a shared secret and a timestamp to calculate a hash, which is sent as part of the request to authenticate the client.
How Hawk Authentication Works:
- The client and server share a secret key.
- The client sends a request with a Hawk Header that includes a timestamp, a nonce (random number), and a hash of the request body and other headers.
- The server verifies the hash using the shared secret and ensures that the timestamp is within an acceptable time range to avoid replay attacks.
- If the server verifies the authenticity of the request, the response is returned to the client.
Setting Up Hawk Authentication in Postman:
To set up Hawk Authentication in Postman:
- In the Postman request, select the Authorization tab.
- From the Type dropdown, choose Hawk.
- Fill in the required fields:
- Access ID: The unique identifier associated with the client (provided by the server).
- Key: The shared secret key used to authenticate the request.
- Algorithm: The algorithm used for hashing (e.g., SHA-256).
- Nonce: A unique value generated for each request, usually provided by the server or generated by the client.
- Timestamp: A timestamp indicating the time the request was made, used to prevent replay attacks.
- Once the fields are populated, Postman will automatically generate the necessary Hawk header and send the request with the appropriate authentication details.
3. Digest Authentication vs Hawk Authentication
While both Digest Authentication and Hawk Authentication provide secure methods of authenticating API requests, there are key differences:
Feature | Digest Authentication | Hawk Authentication |
---|---|---|
Security | Uses hashed credentials and challenge-response mechanism to authenticate. | Uses a shared secret key and a timestamp-based hash to secure the request. |
Implementation | Requires both client and server to support Digest Authentication. | Requires both client and server to support Hawk Authentication protocol. |
Use Case | Commonly used for APIs requiring authentication without sending passwords in plain text. | Ideal for securing HTTP services and protecting against replay attacks. |
4. Troubleshooting Digest and Hawk Authentication
If you encounter issues with Digest or Hawk Authentication, consider the following troubleshooting steps:
- Invalid Credentials: Double-check the username, password, and shared secret. Ensure they are correct.
- Invalid Nonce or Timestamp: Ensure that the nonce and timestamp are correctly generated and within an acceptable time range.
- Incorrect Hashing: Verify that the hashing algorithm used in Postman matches the one expected by the server.
- API Server Misconfiguration: Ensure that the server is properly configured to accept Digest or Hawk authentication requests.
Diagram: Digest and Hawk Authentication Flow
The following diagram illustrates the flow of both Digest and Hawk Authentication methods:

This diagram shows the step-by-step process of using Digest and Hawk authentication to securely authorize API requests.
Creating and Using Environment Variables
Environment Variables in Postman are a powerful way to make your API requests more flexible and dynamic. They allow you to store values that can be used across multiple requests, collections, and environments. This helps to manage different environments (like development, staging, production) and avoid hardcoding values in your requests.
1. What Are Environment Variables?
Environment Variables are placeholders for values that can be used throughout Postman. These values can be set for specific environments (such as development, testing, or production) and can be referenced in your requests to dynamically change the request behavior based on the environment. Examples of environment variables include API keys, server URLs, and authentication tokens.
2. Creating an Environment in Postman
To create an environment in Postman:
- Click on the Environment quick look icon (eye icon) in the top right corner of the Postman app.
- Click on Manage Environments to open the environment manager.
- Click on the Add button to create a new environment.
- Give your environment a name (e.g., "Development", "Staging", "Production").
- Under the environment, add variables by specifying their Name and Initial Value.
- Click Save to save the environment.
3. Adding Variables to an Environment
To add variables to an environment:
- In the environment manager, under the environment you just created, click Add under the "Variables" section.
- Enter a Name for the variable (e.g., "base_url", "auth_token").
- Enter the Initial Value for the variable (e.g., "https://api.example.com", "Bearer xyz123").
- If you want to change the value of the variable during the execution of your requests, you can also set the Current Value.
- Click Save to save the variable.
4. Using Environment Variables in Requests
Once you have created an environment and added variables, you can use them in your requests. To reference a variable in a request:
- In the Request URL, Headers, or Body section, use the variable by wrapping its name in double curly braces. For example, if you created a variable called base_url, you would reference it like this:
{{base_url}}
. - When you send the request, Postman will replace the variable with its Current Value>.
- For example, if your base_url variable's current value is
https://api.example.com
, the request URL might look like:{{base_url}}/users
, which Postman will resolve ashttps://api.example.com/users
.
5. Switching Between Environments
To switch between environments in Postman:
- Click on the Environment quick look icon (eye icon) in the top right corner of the Postman app.
- Select the environment you want to use from the dropdown.
- Once selected, all variables in that environment will be used in your requests.
6. Using Global Variables
Global variables are variables that are available across all environments and requests in Postman. These are useful for values that you want to be globally accessible, such as API keys or authentication tokens.
To create a global variable:
- Click on the Environment quick look icon (eye icon).
- Click on Globals in the dropdown.
- Click Add and enter the variable name and value.
- Click Save to save the global variable.
7. Using Variables in Pre-request Scripts and Tests
You can also use environment variables in Postman’s pre-request scripts and tests. To access variables in scripts:
- Use the
pm.environment.get('variable_name')
method to retrieve the value of an environment variable. - Use the
pm.environment.set('variable_name', value)
method to set the value of an environment variable dynamically during a request.
Example: Using Variables in Pre-request Script
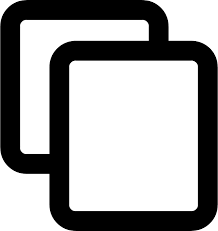
pm.environment.set("auth_token", "Bearer {{new_token}}");
8. Debugging and Inspecting Variables
To inspect the current values of the environment variables:
- Click on the Environment quick look icon (eye icon) at the top right.
- Review the Current Value of each variable.
- If the values are incorrect or outdated, you can modify them directly in the environment manager.
9. Exporting and Importing Environments
Postman allows you to export and import environments to share them with others or to back them up:
- In the environment manager, click the three dots next to the environment you want to export.
- Select Export to download the environment as a JSON file.
- To import an environment, click on Import in the environment manager and select the environment JSON file you wish to import.
10. Best Practices for Using Environment Variables
- Use meaningful names: Name your variables clearly (e.g.,
api_base_url
,auth_token
) to make them easy to understand. - Keep sensitive data secure: Avoid storing sensitive data like passwords in environment variables unless necessary, and consider encrypting values when possible.
- Reuse variables: Reuse environment variables across multiple requests to avoid redundancy and ensure consistency.
Diagram: Environment Variables Workflow
The following diagram shows how environment variables are used in Postman requests:

This diagram illustrates how Postman dynamically replaces variables in requests with their corresponding values from the selected environment.
Using Global Variables
Global variables in Postman are variables that are accessible across all requests, collections, and environments within Postman. They are helpful for storing values that you want to use universally, regardless of the environment, such as API keys, authentication tokens, or base URLs. Global variables are not tied to a specific environment, so they can be referenced in any request at any time.
1. What Are Global Variables?
Global variables are variables that you define in Postman and can be used across all your requests, collections, and environments. Unlike environment variables, which are specific to a particular environment (e.g., development, production), global variables are available globally and are ideal for values you need to use in multiple environments.
2. Creating Global Variables
To create global variables in Postman:
- Click on the Environment quick look icon (the eye icon) located at the top right corner of the Postman app.
- In the quick look window, click on the Globals button to open the global variables manager.
- Click on the Add button in the Globals section to create a new global variable.
- Give your variable a Name (e.g.,
api_key
,base_url
) and set an Initial Value (e.g.,12345
,https://api.example.com
). - If you want to modify the variable later, you can update its Current Value as needed.
- Click Save to store the global variable.
3. Using Global Variables in Requests
Once you have created global variables, you can use them in any request by referencing them in curly braces. To use a global variable in a request:
- In the Request URL, Headers, or Body section, reference the global variable by enclosing its name in double curly braces. For example:
{{api_key}}
. - Postman will automatically replace the placeholder
{{api_key}}
with its corresponding value when you send the request. If the global variable has a value of12345
, the request will use that value. - For example, if you defined a global variable called
base_url
with a value ofhttps://api.example.com
, and your request URL is{{base_url}}/users
, Postman will replace{{base_url}}
with the valuehttps://api.example.com
.
4. Updating Global Variables
You can update the value of global variables while working on your requests:
- Click the Environment quick look icon and then click Globals.
- Select the global variable you want to update, and modify the Current Value field.
- Click Save to apply the changes.
5. Accessing Global Variables in Scripts
You can use global variables in Postman’s Pre-request scripts and Tests. To access a global variable:
- Use the
pm.globals.get('variable_name')
method to retrieve the value of a global variable in scripts. - To set the value of a global variable dynamically, use
pm.globals.set('variable_name', value)
in your Pre-request script or Test.
Example: Using Global Variables in a Pre-request Script
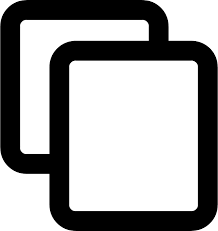
pm.globals.set("auth_token", "Bearer {{new_token}}");
6. Inspecting Global Variables
To inspect the current values of your global variables:
- Click on the Environment quick look icon (eye icon) located in the top right corner of the Postman app.
- Click on Globals to view the list of global variables and their current values.
- Make sure the values are correct, and update them if necessary.
7. Exporting and Importing Global Variables
If you want to share or back up your global variables, you can export and import them:
- Click on the three dots next to the global variables section in the Globals view and choose Export.
- This will download a JSON file containing your global variables.
- To import global variables, click on Import in the same section and select the downloaded JSON file.
8. Best Practices for Using Global Variables
- Use descriptive names: Name your global variables in a way that clearly indicates their purpose (e.g.,
base_url
,api_key
,auth_token
). - Keep sensitive data secure: Avoid storing sensitive data like passwords or tokens in global variables unless necessary. Consider encrypting sensitive data if possible.
- Reuse variables: Reuse global variables across requests, collections, and environments to ensure consistency and reduce redundancy.
- Document global variables: Keep track of the global variables you use in your collections, and document their usage and values to avoid confusion.
Diagram: Global Variables Workflow
The following diagram illustrates how global variables are used in Postman requests:

This diagram shows how global variables are accessed and replaced with actual values in requests and scripts.
Managing Multiple Environments (Production, Development, etc.)
In Postman, environments allow you to manage different sets of variables for different stages of the development lifecycle, such as Production, Development, or Testing. With environments, you can easily switch between different configurations of variables, such as API URLs or credentials, without manually changing each request.
1. What Are Environments in Postman?
An environment in Postman is a collection of variables that are specific to a particular context or stage of development. These variables can be used across all requests and collections within Postman. Common use cases for environments include setting different API base URLs for different stages (e.g., https://dev.api.com
for development and https://prod.api.com
for production) or storing authentication tokens for different environments.
2. Creating a New Environment
To create a new environment in Postman:
- Click on the Environment quick look icon (eye icon) in the top right corner of the Postman app.
- Click on the Manage Environments button.
- In the Environments modal, click on the Add button to create a new environment.
- Give your environment a name (e.g.,
Development
,Production
, etc.). - Add variables to your environment by entering the Name and Initial Value of each variable (e.g.,
base_url
,auth_token
). - Click Save to store the environment.
3. Switching Between Environments
Once you have created multiple environments, you can easily switch between them:
- Click on the Environment quick look icon (eye icon) in the top right corner.
- Select the environment you want to use from the drop-down list (e.g.,
Development
,Production
). - Postman will automatically switch to the selected environment and use the associated variables.
4. Using Environment Variables in Requests
Once your environment is selected, you can use the environment variables in your requests:
- In the Request URL, Headers, or Body, reference the environment variable by wrapping its name in double curly braces. For example:
{{base_url}}
. - When the request is sent, Postman will replace the variable
{{base_url}}
with its corresponding value from the selected environment.
5. Updating Environment Variables
To update the value of an environment variable:
- Click on the Environment quick look icon and select the environment.
- Click on the Manage Environments button.
- Select the environment you want to modify and update the Current Value of the variable.
- Click Save to apply the changes.
6. Using Environment Variables in Scripts
You can use environment variables in Postman’s Pre-request scripts and Tests. To access an environment variable:
- Use the
pm.environment.get('variable_name')
method to retrieve the value of a variable in your scripts. - To set the value of an environment variable programmatically, use
pm.environment.set('variable_name', value)
in your Pre-request script or Test.
Example: Using Environment Variables in a Pre-request Script
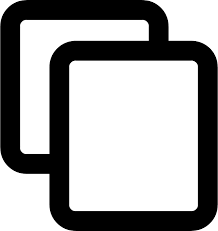
pm.environment.set("auth_token", "Bearer {{new_token}}");
7. Exporting and Importing Environments
If you want to share or back up your environment settings, you can export and import environments:
- Click on the three dots next to the environment you want to export and select Export.
- Choose the file format (JSON) and save the environment file to your local system.
- To import an environment, click on the Import button in the Manage Environments window and select the exported environment file.
8. Best Practices for Managing Multiple Environments
- Use meaningful names: Name your environments based on their purpose (e.g.,
Development
,Staging
,Production
) to make it clear which environment is being used. - Keep environment-specific data separate: Store values that change across environments (e.g., API URLs, authentication tokens) as environment variables.
- Avoid storing sensitive data: Be cautious about storing sensitive data like API keys and tokens in environments, especially when sharing environments.
- Use different environments for testing: If you're testing new features, create a separate environment to avoid accidentally affecting production data.
- Document environment variables: Keep track of the variables in each environment and document their purpose for easier collaboration.
9. Common Use Cases for Environments
Environments are especially useful for managing different configurations across various stages of the development lifecycle. Some common use cases include:
- API testing: Switch between different environments (e.g., Development, Production) to test APIs under different configurations.
- Authentication: Store different authentication tokens or API keys for different environments.
- API base URLs: Set different base URLs for the API depending on whether you're working with the Development, Staging, or Production environment.
Diagram: Environment Variables Workflow
The following diagram illustrates how environment variables are used in requests and tests:

This diagram shows how environment variables can be dynamically replaced in your requests based on the selected environment.
Collaborating in Shared Workspaces
Postman’s workspaces enable teams to collaborate and share resources such as collections, environments, and monitors. A workspace can be public or private, and it provides a centralized location where members can work together on API development, testing, and documentation. Shared workspaces are ideal for enhancing team collaboration and streamlining workflows across different teams or departments.
1. What is a Workspace in Postman?
A workspace in Postman is a collection of resources—such as collections, environments, and variables—that are shared among team members. It allows team members to collaborate on API development and testing in a centralized location, making it easy to manage and share API-related assets.
2. Types of Workspaces
- Personal Workspaces: These are private workspaces that only you have access to. They are useful for individual use and personal projects.
- Team Workspaces: These workspaces are shared with other team members. They are ideal for collaboration and team projects, where multiple people can work on the same resources.
- Public Workspaces: These are open to the public and can be accessed by anyone. Public workspaces are great for open-source projects or sharing resources with the broader Postman community.
3. Creating a New Workspace
To create a new workspace in Postman:
- Click on the Workspaces dropdown in the top left corner of Postman.
- Click on the Create Workspace button.
- Give your workspace a name, and optionally, add a description to provide context.
- Choose the visibility for the workspace: Personal, Team, or Public.
- Click Create Workspace to finish.
4. Inviting Members to a Shared Workspace
If you are working in a Team or Public workspace, you can invite others to collaborate with you:
- In the workspace, click the Invite button in the top right corner.
- Enter the email addresses of the people you wish to invite to the workspace.
- Choose whether the invitees should have Editor or Viewer access. Editors can make changes, while Viewers can only view the content.
- Click Send Invite.
5. Managing Access and Permissions
In a shared workspace, you can control who has access and what level of permissions they have:
- Admin: Admins have full control over the workspace and can modify settings, invite members, and manage permissions.
- Editor: Editors can add, modify, and delete resources within the workspace (e.g., collections, environments, etc.).
- Viewer: Viewers can only view resources and cannot make any changes within the workspace.
To manage permissions:
- In the workspace, click on the Manage Members button.
- Here you can see all current members and their roles.
- Click on the role to modify it or remove a member from the workspace.
6. Sharing Collections and Environments
In shared workspaces, you can share collections, environments, and other resources with your teammates. Here’s how you can share a collection or environment:
- Navigate to the collection or environment you want to share.
- Click on the three dots ... next to the resource.
- Click on Share.
- Select the workspace where you want to share the resource, or choose from the list of available workspaces.
- Click Share to confirm.
7. Collaborating on Collections
In a shared workspace, you and your team can work together on collections. Here's how you can collaborate on a collection:
- View Changes: You can see real-time updates made by other collaborators in the collection.
- Commenting: You can leave comments on specific requests in a collection to discuss changes or ask questions.
- Version Control: When working with teams, Postman automatically keeps track of changes made to collections. You can revert to previous versions if needed.
8. Using Postman Workspaces for API Documentation
Postman allows you to generate and share API documentation in a shared workspace. This feature is useful for teams to collaborate on API design and documentation:
- Click on the Collections tab in your workspace and select the collection you want to document.
- Click on the three dots ... next to the collection and select Generate Docs.
- Postman will generate a public URL where your API documentation can be viewed.
- Share the documentation link with your team for easy access and collaboration.
9. Notifications and Activity Feed
In a shared workspace, you can stay updated on all the changes made by other members through the Activity Feed and notifications:
- Activity Feed: The Activity Feed shows a timeline of all changes, comments, and updates within the workspace.
- Notifications: You will receive notifications when someone invites you to a workspace, shares resources with you, or when your attention is needed on a specific resource.
10. Best Practices for Collaborating in Shared Workspaces
- Set clear roles and permissions: Ensure that team members have the appropriate level of access (Admin, Editor, Viewer) to avoid conflicts.
- Communicate changes: Use comments and the Activity Feed to communicate changes and updates in the workspace.
- Version control: Regularly use version control to keep track of changes and avoid overwriting important data.
- Organize resources: Keep collections, environments, and other resources organized in folders to maintain a clean workspace.
11. Diagram: Collaborating in Postman Workspaces
Below is a diagram that illustrates the collaboration flow in shared workspaces:

This diagram shows how team members can share, edit, and collaborate on resources in a shared workspace.
Introduction to Pre-request Scripts
In Postman, pre-request scripts are executed before sending a request. They allow you to execute custom logic, modify request parameters, or prepare data before the request is sent to the server. Pre-request scripts are useful for tasks like setting dynamic variables, performing calculations, or even generating authentication tokens that are needed for the request.
1. What are Pre-request Scripts?
A pre-request script is a piece of JavaScript code that runs before a request is sent. It can be used to modify the request, set variables, or execute other logic that prepares the request for execution. Pre-request scripts are particularly useful for tasks like generating authentication tokens, creating dynamic values, or modifying headers before sending an API request.
2. Where to Write Pre-request Scripts
Pre-request scripts can be written in the Pre-request Script tab, which is available in the Postman request window. To access the pre-request script section:
- Open the request in Postman.
- Click on the Pre-request Script tab next to the Body, Params, and Headers tabs.
- Write your JavaScript code in the editor that appears in the Pre-request Script section.
3. Setting Variables in Pre-request Scripts
Pre-request scripts are commonly used for setting dynamic variables that are used later in the request. You can set variables using the pm.environment.set or pm.globals.set functions, depending on whether you want to use environment or global variables.
Example code for setting a variable:
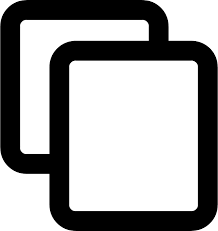
// Set an environment variable before sending the request
pm.environment.set("auth_token", "Bearer " + pm.variables.get("access_token"));
4. Using Dynamic Data in Pre-request Scripts
Pre-request scripts are also useful for generating dynamic data, such as timestamps, random values, or user-specific data. For example, you can generate a random string to use as a unique identifier or timestamp for your request.
Example code for generating dynamic data:
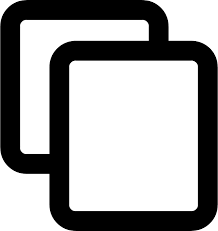
// Generate a random number and set it as a variable
const randomNumber = Math.floor(Math.random() * 1000);
pm.environment.set("random_id", randomNumber);
5. Using External Data in Pre-request Scripts
Pre-request scripts can also be used to fetch external data (for example, from a database or API) and use it in the request. This can be done by making an HTTP request within the pre-request script itself.
Example code for fetching external data:
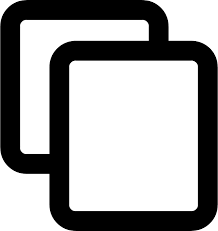
// Fetch data from an external API and use it in the request
pm.sendRequest("https://api.example.com/getData", function (err, res) {
if (err) {
console.log("Error fetching data:", err);
} else {
pm.environment.set("external_data", res.json().data);
}
});
6. Common Use Cases for Pre-request Scripts
Here are some common use cases for pre-request scripts:
- Setting Authentication Tokens: Pre-request scripts can be used to generate or retrieve authentication tokens dynamically, ensuring that the API request is authorized.
- Modifying Request Headers: You can modify request headers based on dynamic values, such as adding a timestamp or authentication token before sending the request.
- Generating Unique Identifiers: Pre-request scripts can generate unique IDs or session tokens for use in the request body or URL parameters.
- Performing Calculations: You can perform calculations, such as generating dates or random numbers, that are required for the API request.
7. Example: Using a Pre-request Script for OAuth 2.0 Authentication
Here’s an example of how to use a pre-request script to retrieve an OAuth 2.0 token before sending an API request:
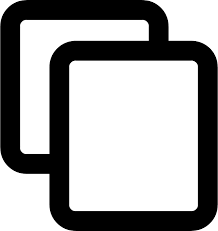
// Request the OAuth 2.0 token and set it as an environment variable
pm.sendRequest({
url: 'https://api.example.com/oauth2/token',
method: 'POST',
body: {
mode: 'formdata',
formdata: [
{ key: 'grant_type', value: 'client_credentials' },
{ key: 'client_id', value: 'your_client_id' },
{ key: 'client_secret', value: 'your_client_secret' }
]
}
}, function (err, res) {
if (err) {
console.log("OAuth token request failed:", err);
} else {
pm.environment.set("access_token", res.json().access_token);
}
});
8. Debugging Pre-request Scripts
If your pre-request script isn’t working as expected, you can debug it using console.log to output values to the Postman console. This can help you track the flow of data and identify issues in your script.
To view the console in Postman, click on the Console button at the bottom left of the Postman window.
9. Best Practices for Pre-request Scripts
- Keep it simple: Pre-request scripts should be simple and focused on the task at hand. Avoid complex logic that may slow down the request processing.
- Use comments: Add comments to your code to explain its purpose, especially when working with dynamic values or external data.
- Test thoroughly: Test your pre-request scripts in isolation to ensure they work as expected before integrating them into larger workflows.
10. Diagram: Pre-request Script Execution Flow
The following diagram illustrates the flow of a pre-request script:

This diagram shows how the pre-request script is executed before the actual request is sent, allowing you to modify the request as needed.
Using pm Object and JavaScript in Pre-request Scripts
Postman provides the pm object, which is a powerful tool for managing and manipulating request data in pre-request scripts. The pm object allows you to set and get variables, manage environments, handle responses, and perform a variety of other tasks within your pre-request scripts. In this section, we will explore how to use the pm object in combination with JavaScript to enhance the functionality of your pre-request scripts.
1. What is the pm Object?
The pm object is a special API provided by Postman to interact with requests, responses, and variables within Postman. It is available in pre-request scripts, tests, and other areas in Postman where you can run JavaScript code. The pm object is a convenient way to access and modify variables, send requests, validate responses, and more during the lifecycle of your API request.
2. Accessing pm Object in Pre-request Scripts
You can use the pm object directly in the pre-request script editor. Below are some key features and methods provided by the pm object.
Example: Accessing pm Object in Pre-request Script
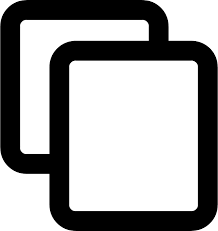
// Accessing pm object to set an environment variable
pm.environment.set("username", "JohnDoe");
// Accessing pm object to get an environment variable
var username = pm.environment.get("username");
console.log("Username: " + username);
3. Key pm Methods for Pre-request Scripts
Here are some commonly used methods of the pm object in pre-request scripts:
- pm.environment.set(variable, value): Sets an environment variable with the specified name and value.
- pm.environment.get(variable): Retrieves the value of an environment variable.
- pm.globals.set(variable, value): Sets a global variable with the specified name and value.
- pm.globals.get(variable): Retrieves the value of a global variable.
- pm.request.headers.add(headerObject): Adds a header to the request.
- pm.request.body.update(bodyObject): Updates the body of the request.
- pm.response: Used to access the response data after the request is made (though typically used in tests, it can be useful for chaining requests).
4. Using Variables in Pre-request Scripts
Variables are critical for making your requests dynamic. In Postman, you can use the pm object to manage environment, global, and collection variables. Variables can be set and retrieved in pre-request scripts to modify or generate data required for the request.
Example: Setting and Getting Variables in Pre-request Script
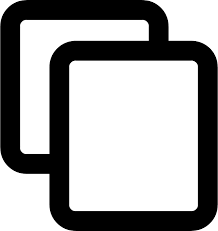
// Setting environment variables
pm.environment.set("auth_token", "Bearer " + pm.variables.get("access_token"));
// Getting environment variables
var authToken = pm.environment.get("auth_token");
console.log("Authorization token: " + authToken);
// Setting global variables
pm.globals.set("session_id", "12345");
// Getting global variables
var sessionId = pm.globals.get("session_id");
console.log("Session ID: " + sessionId);
5. Using JavaScript Functions with pm Object
In addition to the pm methods, you can also use JavaScript functions and logic to manipulate data before the request is sent. For example, you can generate dynamic values using JavaScript and use them in your request.
Example: Using JavaScript Functions to Generate Dynamic Data
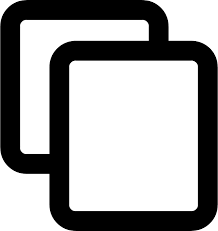
// Generate a random user ID and set it as an environment variable
var randomUserId = Math.floor(Math.random() * 10000);
pm.environment.set("user_id", randomUserId);
// Use the generated user ID in the request
console.log("Generated User ID: " + randomUserId);
6. Chaining Requests Using pm Object
In Postman, you can chain multiple requests together by using the pm object to pass data from one request to another. After sending a request, you can extract data from the response and use it in subsequent requests, making your tests or workflows more dynamic.
Example: Using Data from One Request in Another
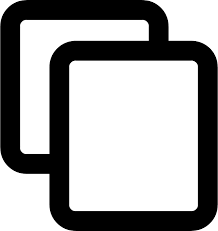
// Request 1: Fetch an access token
pm.sendRequest({
url: 'https://api.example.com/oauth2/token',
method: 'POST',
body: {
mode: 'formdata',
formdata: [
{ key: 'grant_type', value: 'client_credentials' },
{ key: 'client_id', value: 'your_client_id' },
{ key: 'client_secret', value: 'your_client_secret' }
]
}
}, function (err, res) {
if (err) {
console.log("Error fetching token:", err);
} else {
// Store the token as an environment variable
pm.environment.set("access_token", res.json().access_token);
// Use the token in subsequent requests
pm.sendRequest({
url: 'https://api.example.com/data',
method: 'GET',
headers: {
'Authorization': 'Bearer ' + res.json().access_token
}
}, function (err, res) {
if (err) {
console.log("Error fetching data:", err);
} else {
console.log("Data fetched:", res.json());
}
});
}
});
7. Logging and Debugging with pm Object
When working with the pm object in your pre-request scripts, it can be helpful to use logging for debugging. You can use console.log to print out values of variables, responses, or any other data you're working with.
Example: Debugging with console.log
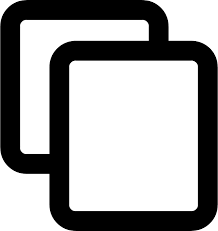
// Log environment variables
console.log("Auth Token: " + pm.environment.get("auth_token"));
console.log("User ID: " + pm.environment.get("user_id"));
8. Best Practices for Using pm Object in Pre-request Scripts
- Keep it simple: Pre-request scripts should be lightweight and focused. Avoid complex logic that might slow down the execution of your requests.
- Use descriptive variable names: When setting and getting variables, choose meaningful names that will make your scripts easier to understand and maintain.
- Test and debug: Use console.log to inspect values and troubleshoot issues in your scripts.
- Manage dependencies: If you are chaining requests, ensure the data flow between requests is clear and that each request has the required data.
9. Diagram: pm Object Usage in Pre-request Scripts
The following diagram illustrates the use of the pm object and JavaScript in a pre-request script:

This diagram shows the steps involved in using the pm object to manipulate data and send requests within Postman.
Writing Tests with Postman Scripts
Postman allows you to write tests for your API requests directly in the app. These tests are written using JavaScript, and you can use them to validate responses, check for specific conditions, or even compare response data with expected values. In this section, we will discuss how to write tests using Postman scripts, which can help you automate the process of validating your API endpoints and ensure they are functioning as expected.
1. What are Postman Scripts?
Postman scripts are written in JavaScript and allow you to define tests and pre-request actions for your API calls. There are two primary types of scripts in Postman:
- Pre-request Scripts: These are executed before the request is sent and are used to prepare the request or manipulate data.
- Tests: These are executed after the request is sent and are used to verify the response from the API.
In this section, we will focus on writing tests for validating API responses.
2. Writing Basic Tests in Postman
Postman provides a built-in set of assertion libraries that allow you to easily write tests for various API response attributes such as status codes, response time, headers, and body content. The tests are written in the "Tests" tab of the Postman request window.
Example: Writing a Basic Test
Here’s an example of a simple test to validate the status code and response body:
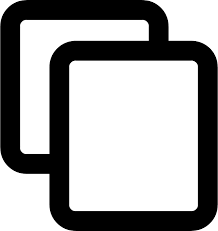
// Test the status code to be 200 (OK)
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Test the response body to contain a specific string
pm.test("Response body contains 'success'", function () {
pm.response.to.have.body("success");
});
3. Common Test Assertions
Postman provides a set of built-in assertions that you can use to test various aspects of your API response:
- pm.response.to.have.status(code): Verifies that the response status code matches the expected value.
- pm.response.to.have.body(content): Verifies that the response body contains the specified content.
- pm.response.to.have.header(name, value): Verifies that the response contains a header with the specified name and value.
- pm.response.to.have.jsonBody(json): Verifies that the response body is a valid JSON object and matches the expected structure.
- pm.response.to.have.statusCode(code): A shorthand for checking the status code.
- pm.response.to.have.property(name): Checks whether the response body contains a specific property.
Example: Using Common Assertions
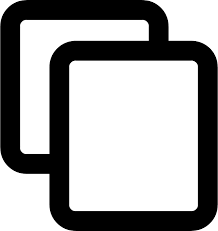
// Check that the response has a status code of 200
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Check that the response contains a 'Content-Type' header with value 'application/json'
pm.test("Response contains Content-Type header", function () {
pm.response.to.have.header("Content-Type", "application/json");
});
// Check that the response body contains a specific JSON property
pm.test("Response body contains 'userId' property", function () {
pm.response.to.have.property("userId");
});
4. Working with JSON Responses
Many APIs return responses in JSON format. Postman allows you to easily parse JSON data and perform tests on specific values within the response body.
Example: Parsing JSON Response
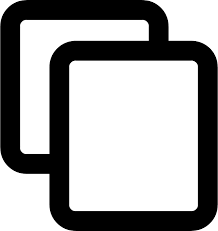
// Parse the JSON response body
var responseJson = pm.response.json();
// Test that the response contains a specific property in the JSON
pm.test("Response contains userId", function () {
pm.expect(responseJson).to.have.property("userId");
});
// Test that the userId is a number
pm.test("userId is a number", function () {
pm.expect(responseJson.userId).to.be.a("number");
});
5. Using Variables in Tests
Variables in Postman can be used to store dynamic values, and you can reference those variables in your tests. This is useful when you want to use data from one request in subsequent tests or when you need to validate responses against different sets of data.
Example: Using Variables in Tests
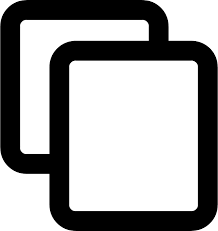
// Set a variable in the environment
pm.environment.set("expectedUserId", 12345);
// Use the variable in a test
pm.test("UserId is correct", function () {
var responseJson = pm.response.json();
pm.expect(responseJson.userId).to.eql(pm.environment.get("expectedUserId"));
});
6. Validating Response Time
Postman allows you to test the response time of your API. This can be useful to ensure that your API is not only returning the correct data but is also doing so within an acceptable performance threshold.
Example: Validating Response Time
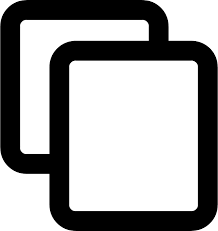
// Test that the response time is less than 2000ms (2 seconds)
pm.test("Response time is less than 2000ms", function () {
pm.expect(pm.response.responseTime).to.be.below(2000);
});
7. Writing Complex Tests
Postman tests can be used to create more complex validation rules. You can combine multiple assertions, check the response against expected values, and even perform conditional logic within your tests.
Example: Complex Test Scenario
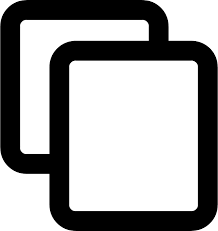
// Complex test that checks multiple conditions
pm.test("Valid user data", function () {
var responseJson = pm.response.json();
pm.expect(responseJson).to.have.property("userId");
pm.expect(responseJson.userId).to.be.a("number");
pm.expect(responseJson.name).to.be.a("string");
pm.expect(responseJson.email).to.match(/^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$/);
});
8. Running Tests Automatically
Once you’ve written your tests, you can run them automatically as part of a Postman Collection run. This allows you to execute multiple tests in sequence and get a report of the results.
- Run from Collection Runner: You can select multiple requests and execute them sequentially with all associated tests.
- Automated Testing with Newman: Newman is Postman’s command-line collection runner. You can run your Postman tests from the command line and integrate them with your continuous integration (CI) pipeline.
9. Diagram: Test Execution Flow
The following diagram illustrates the flow of test execution in Postman:

This diagram shows the steps from making the request to executing the tests and validating the response.
Basic Assertions (pm.expect) and Status Code Checks
In Postman, writing tests is an essential part of ensuring that your API is functioning as expected. One of the most common test types is checking the API response status code and validating specific conditions in the response. In this section, we will explore how to use the pm.expect
function to perform basic assertions and how to check the status code of the response.
1. What is pm.expect?
The pm.expect
function is used in Postman scripts to assert that certain conditions are met in the API response. It is part of Postman's built-in assertion library and allows for readable and expressive tests. The expect
function is based on the Chai Assertion Library, which provides a variety of methods to test different types of conditions in your API response.
2. Basic Assertions with pm.expect
You can use pm.expect
to write assertions for different response elements, such as status codes, headers, body content, and more. Below are some examples of basic assertions using pm.expect
:
Example: Checking the Status Code
One of the most common assertions is to check if the status code of the response is as expected. Here’s how you can check for a 200 OK status code:
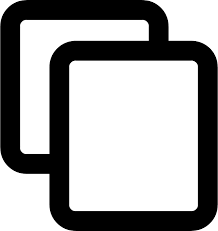
// Test the response status code to be 200 (OK)
pm.test("Status code is 200", function () {
pm.expect(pm.response.code).to.eql(200);
});
Example: Checking the Response Body
You can also use pm.expect
to check values in the response body. For instance, if you want to check that the response body contains a specific string, you can do the following:
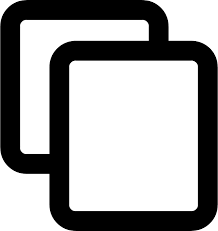
// Test that the response body contains the string "success"
pm.test("Response body contains 'success'", function () {
pm.expect(pm.response.text()).to.include("success");
});
3. Status Code Checks
Checking the status code is one of the most important tests you can perform. The status code indicates whether the request was successful and can be used to validate the behavior of your API endpoints. In Postman, you can easily check the response status code using either pm.response.code
or the shorthand pm.response.to.have.status(code)
.
Example: Checking Specific Status Codes
Let’s assume you want to test if the status code is either 200 (OK), 201 (Created), or 400 (Bad Request). Here's how you can do it:
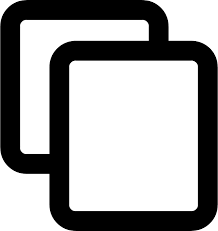
// Test that the status code is 200, 201, or 400
pm.test("Status code is 200, 201, or 400", function () {
pm.expect([200, 201, 400]).to.include(pm.response.code);
});
Example: Checking if the Status Code is Not 404
You can also check that the status code is not 404 (Not Found) using the not
operator in the assertion:
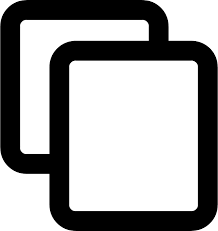
// Test that the status code is not 404
pm.test("Status code is not 404", function () {
pm.expect(pm.response.code).to.not.eql(404);
});
4. Combining Multiple Assertions
Postman allows you to combine multiple assertions in one test. You can check both the status code and the response body in a single test to ensure that the response is both valid and contains the expected content.
Example: Checking Status Code and Response Body Together
Here’s an example of combining multiple assertions in a single test:
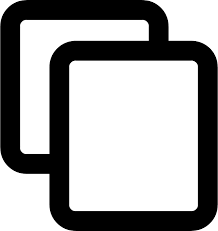
// Test that the status code is 200 and the response body contains "success"
pm.test("Status code is 200 and response body contains 'success'", function () {
pm.expect(pm.response.code).to.eql(200);
pm.expect(pm.response.text()).to.include("success");
});
5. Using pm.response.to.have.status for Simplicity
Postman also provides a shorthand syntax for checking the status code using pm.response.to.have.status
. This is more concise and can be used to improve readability in your tests.
Example: Shorthand Status Code Check
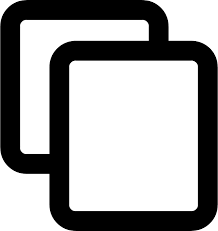
// Test that the status code is 200 (OK)
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
6. Handling Unexpected Status Codes
Sometimes, you may encounter unexpected status codes, such as 500 (Internal Server Error) or 401 (Unauthorized). You can write tests to check for those scenarios as well and ensure the API is returning the correct status in edge cases.
Example: Checking for 500 Status Code
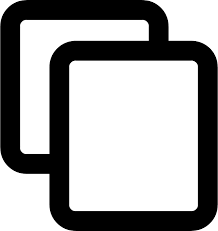
// Test that the status code is not 500 (Internal Server Error)
pm.test("Status code is not 500", function () {
pm.expect(pm.response.code).to.not.eql(500);
});
7. Diagram: Status Code and Assertion Flow
The following diagram illustrates the flow of the status code check and the assertion process in Postman:

This diagram shows how the status code is checked, followed by assertions to verify the validity and content of the response.
Writing Custom Tests for Response Validation
Writing custom tests in Postman allows you to validate specific conditions in the API response that may not be covered by basic assertions. Custom tests enable you to write more complex logic to ensure the API response meets the requirements of your application. In this section, we will explore how to write custom tests to validate various aspects of the response, such as data integrity, structure, and specific field values.
1. What Are Custom Tests?
Custom tests are user-defined scripts in Postman that allow you to validate the response from the API based on your unique testing needs. Using JavaScript and the Postman scripting environment, you can write tests to check the values of specific fields, validate response types, or verify the integrity of data returned by the API.
2. Writing a Custom Test for Response Body
One common use case for custom tests is validating the structure of the response body. You can write tests to ensure that the response contains specific fields or that the data is in the correct format.
Example: Checking if Response Body Contains Specific Fields
Let’s say you want to verify that the response body contains a field called userId
and that its value is a number. You can write a custom test like this:
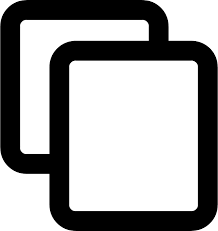
// Custom test to check if userId exists and is a number
pm.test("Response body contains userId as number", function () {
var jsonData = pm.response.json(); // Parse the JSON response
pm.expect(jsonData).to.have.property('userId'); // Check if userId exists
pm.expect(jsonData.userId).to.be.a('number'); // Check if userId is a number
});
3. Custom Tests for Nested JSON Responses
In many cases, the response body may contain nested JSON objects or arrays. You can write custom tests to check the values in these nested structures as well.
Example: Validating Nested Objects
Let’s say the response body contains a nested object address
, and you want to validate that the street
field exists within the address
object. Here’s how you could write a custom test:
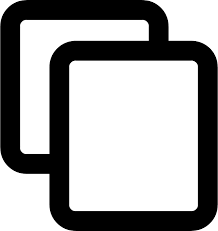
// Custom test to check if address.street exists in the response
pm.test("Address contains street", function () {
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.nested.property('address.street'); // Check if street exists in address object
});
4. Custom Tests for API Response Time
In addition to validating response content, you can also write custom tests to check the performance of your API. One common test is to ensure that the API response time is under a specified threshold.
Example: Checking API Response Time
This custom test checks that the response time is less than 500 milliseconds:
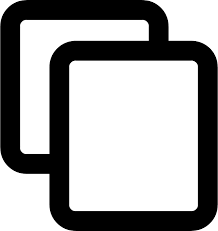
// Custom test to check if the response time is under 500ms
pm.test("Response time is less than 500ms", function () {
pm.expect(pm.response.responseTime).to.be.below(500); // Assert that response time is below 500ms
});
5. Custom Tests for Response Headers
Custom tests can also be written to validate the headers returned in the API response. This is particularly useful for checking security-related headers, content type, or custom headers that your API might return.
Example: Checking Content-Type Header
This custom test checks if the Content-Type
header is set to application/json
:
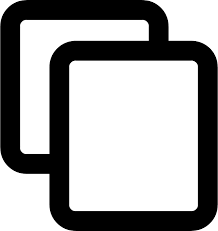
// Custom test to check if Content-Type is application/json
pm.test("Content-Type is application/json", function () {
pm.expect(pm.response.headers.get('Content-Type')).to.include('application/json');
});
6. Custom Tests for Status Codes and Response Validation
You can combine custom tests for status codes with the checks on the response body. For example, you might want to check that a 200 OK status is returned along with the correct response data.
Example: Validating Status Code and Response Data
This custom test verifies that the status code is 200 and that the response body contains a specific field:
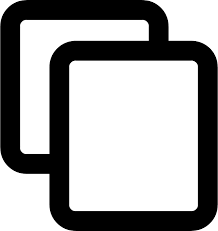
// Custom test for status code and response validation
pm.test("Status code is 200 and response contains userId", function () {
pm.expect(pm.response.code).to.eql(200); // Check if status code is 200
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.property('userId'); // Check if userId exists in the response
});
7. Handling Dynamic Data in Custom Tests
Sometimes you may want to test for dynamic data that changes with each request. In such cases, you can write custom tests that handle variables and store data for use in subsequent requests.
Example: Storing Data for Future Requests
In this custom test, we store a value from the API response (for example, a userId
) and use it in a subsequent request:
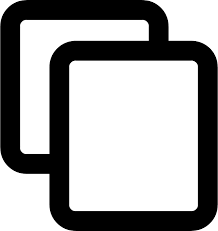
// Store userId from the response to be used in later requests
pm.test("Store userId for future requests", function () {
var jsonData = pm.response.json();
pm.environment.set("userId", jsonData.userId); // Store userId in the environment variable
});
8. Diagram: Custom Test Execution Flow
The following diagram demonstrates how custom tests are executed in Postman, from receiving the API response to validating specific elements within it:

This diagram illustrates the flow of a custom test, where you first validate the status code, then inspect the response body, and finally check dynamic data or response headers.
Checking JSON and XML Responses
In Postman, you can test both JSON and XML responses to ensure that the API returns the expected data. JSON (JavaScript Object Notation) is the most commonly used format for APIs, but XML (eXtensible Markup Language) is also widely used in certain legacy systems and web services. This section will guide you on how to check JSON and XML responses in Postman using custom tests.
1. Validating JSON Responses
Postman provides an easy way to validate JSON responses using the pm.response.json()
method. This method parses the response body into a JavaScript object, allowing you to access and validate specific fields in the response.
Example: Validating JSON Response Structure
Let’s say you are testing an API that returns user information in a JSON format. You may want to check if the response contains the expected fields, such as userId
, name
, and email
.
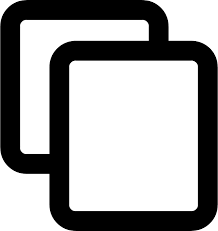
// Custom test to validate JSON response structure
pm.test("Response body contains userId, name, and email", function () {
var jsonData = pm.response.json(); // Parse the JSON response
pm.expect(jsonData).to.have.property('userId'); // Check if userId exists
pm.expect(jsonData).to.have.property('name'); // Check if name exists
pm.expect(jsonData).to.have.property('email'); // Check if email exists
});
2. Checking Values in JSON Responses
You can also validate specific values within a JSON response. For example, you might want to check that a status
field contains the value success
, or that the userId
is a valid integer.
Example: Validating Values in JSON Response
This test checks if the status
field is equal to success
and if the userId
is a number:
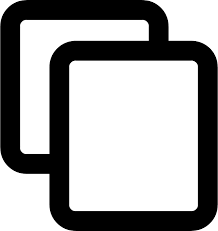
// Custom test to validate specific values in the JSON response
pm.test("Status is success and userId is a number", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.status).to.eql('success'); // Check if status is 'success'
pm.expect(jsonData.userId).to.be.a('number'); // Check if userId is a number
});
3. Validating XML Responses
Postman also allows you to validate XML responses. Unlike JSON, XML responses are structured with tags, and Postman provides a way to parse XML and extract data from it. You can use the pm.response.xml()
method to parse the XML response into an XML document object.
Example: Validating XML Response Structure
Let’s say you are working with an API that returns user information in XML format. You can write a custom test to check if the userId
, name
, and email
elements are present in the response.
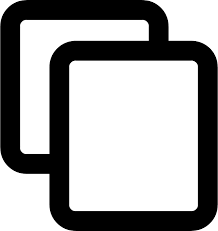
// Custom test to validate XML response structure
pm.test("Response body contains userId, name, and email in XML", function () {
var xmlData = pm.response.xml(); // Parse the XML response
pm.expect(xmlData).to.have.xpath('//userId'); // Check if userId exists
pm.expect(xmlData).to.have.xpath('//name'); // Check if name exists
pm.expect(xmlData).to.have.xpath('//email'); // Check if email exists
});
4. Checking Values in XML Responses
Just like JSON responses, you can also validate specific values within an XML response. For example, you may want to check if the status
element is success
, or if the userId
is a valid integer.
Example: Validating Values in XML Response
This test checks if the status
element equals success
and if the userId
element is a number:
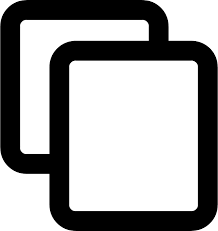
// Custom test to validate specific values in XML response
pm.test("Status is success and userId is a number in XML", function () {
var xmlData = pm.response.xml();
var status = xmlData.getElementsByTagName('status')[0].textContent; // Get status element
var userId = xmlData.getElementsByTagName('userId')[0].textContent; // Get userId element
pm.expect(status).to.eql('success'); // Check if status is 'success'
pm.expect(parseInt(userId)).to.be.a('number'); // Check if userId is a number
});
5. Handling JSON and XML in the Same Request
Sometimes, an API may return both JSON and XML responses, depending on the request headers or parameters. In such cases, you can add conditionals in your tests to handle both response formats correctly.
Example: Conditional Test for JSON or XML Response
This test checks if the response is either JSON or XML and then validates the appropriate fields:
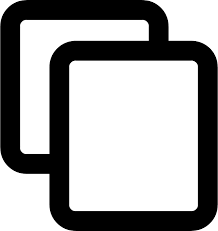
// Conditional test to handle JSON or XML response
pm.test("Response is JSON or XML and contains userId", function () {
var contentType = pm.response.headers.get('Content-Type');
if (contentType.includes('application/json')) {
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.property('userId'); // Check if userId exists in JSON response
} else if (contentType.includes('application/xml')) {
var xmlData = pm.response.xml();
pm.expect(xmlData).to.have.xpath('//userId'); // Check if userId exists in XML response
} else {
pm.test.fail('Response is neither JSON nor XML'); // Fail test if response is neither JSON nor XML
}
});
6. Diagram: JSON and XML Response Validation Flow
The following diagram illustrates the flow of checking JSON and XML responses in Postman, from parsing the response to validating specific fields and values:

This diagram shows how Postman parses the response based on the content type and validates the required fields in both JSON and XML formats.
Handling Dynamic Variables and Data in Tests
In API testing, you often need to work with dynamic data in your requests and responses. This data can vary from one request to another and may depend on the execution of previous requests in a collection. Postman provides powerful features for handling dynamic variables and data, making it easy to write tests that work with this kind of data.
1. Using Dynamic Variables in Requests
Postman allows you to use dynamic variables in both the request body and request parameters. These variables can represent random values, timestamps, or any other data that is generated dynamically.
Example: Using Dynamic Variables for Random Data
Postman offers predefined dynamic variables such as {{randomInt}}
, {{randomEmail}}
, and {{timestamp}}
to generate random data.
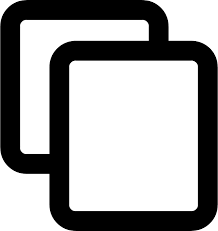
// Example request body using dynamic variables
{
"userId": "{{randomInt}}", // Generate a random integer for userId
"email": "{{randomEmail}}", // Generate a random email address
"timestamp": "{{timestamp}}" // Use current timestamp
}
2. Extracting Data from Responses
Often, you'll need to extract data from an API response to use in subsequent requests. Postman provides the pm.response
object, which allows you to extract values from the response body and store them in variables for later use.
Example: Extracting Data from JSON Response
Suppose an API returns a user ID in the JSON response, and you need to extract it to use in the next request. You can use the following script in the Tests
tab to achieve this:
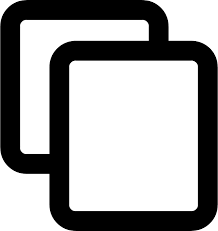
// Extract userId from the response and store it in an environment variable
var jsonData = pm.response.json();
pm.environment.set("userId", jsonData.userId); // Store userId as an environment variable
3. Using Extracted Data in Subsequent Requests
Once you’ve extracted data and stored it in variables, you can use those variables in subsequent requests. This enables you to create dynamic workflows where the data for each request is determined based on the response from previous requests.
Example: Using Extracted Data in a Subsequent Request
Here’s how you can use the userId
extracted from the previous response in a subsequent request:
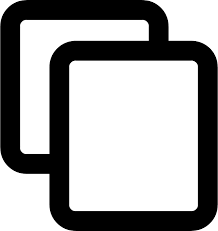
// Use the userId variable in the request body
{
"userId": "{{userId}}", // Use the extracted userId from the environment variable
"action": "updateProfile"
}
4. Data-Driven Testing with CSV and JSON Files
Postman also allows you to perform data-driven testing by using external data files like CSV and JSON. You can iterate through the data in these files, running multiple test cases with different sets of data.
Example: Data-Driven Testing with CSV File
Suppose you have a CSV file containing multiple sets of user credentials (username and password). You can use this file to run multiple tests with different user data.
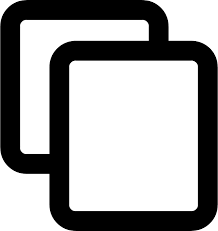
// Example of reading data from a CSV file and using it in tests
pm.test("Check user login with credentials from CSV", function () {
var username = data.username; // Get username from the CSV file
var password = data.password; // Get password from the CSV file
pm.expect(username).to.be.a('string'); // Validate username type
pm.expect(password).to.be.a('string'); // Validate password type
});
Example: Data-Driven Testing with JSON File
Similarly, you can use a JSON file for data-driven testing in a similar way:
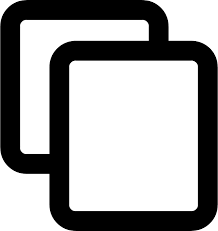
// Example of reading data from a JSON file and using it in tests
pm.test("Check user login with credentials from JSON", function () {
var userData = data[0]; // Get user data from the JSON file
var username = userData.username; // Get username
var password = userData.password; // Get password
pm.expect(username).to.be.a('string'); // Validate username type
pm.expect(password).to.be.a('string'); // Validate password type
});
5. Handling Dynamic Data Across Multiple Requests
In complex workflows, you may need to handle dynamic data across multiple requests. You can use environment or global variables to store and share data between requests within a collection. Postman allows you to chain requests together by passing data from one request to another.
Example: Chaining Multiple Requests with Dynamic Data
Here’s an example where you chain two requests together. The first request returns a session token, and the second request uses that token for authentication.
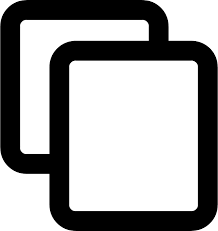
// First request: Obtain session token
pm.test("Extract session token from response", function () {
var jsonData = pm.response.json();
pm.environment.set("sessionToken", jsonData.token); // Store session token as environment variable
});
// Second request: Use session token for authentication
pm.test("Authenticate user with session token", function () {
var sessionToken = pm.environment.get("sessionToken"); // Retrieve session token
pm.expect(sessionToken).to.not.be.null; // Ensure token is not null
});
6. Diagram: Handling Dynamic Data in Postman
The following diagram illustrates how dynamic variables and extracted data flow through multiple requests in Postman:

This diagram shows how data is extracted, stored, and passed between requests to create dynamic workflows in Postman.
Chaining Requests with Scripts
Chaining requests in Postman allows you to create complex workflows where the output of one request is used as the input for the next request in the sequence. This is useful when you need to test APIs that rely on session data, authentication tokens, or dynamically generated values from previous requests.
1. What is Request Chaining?
Request chaining in Postman involves using data from one request's response to drive the next request in the sequence. This can be achieved by extracting values from the response body and storing them as variables (e.g., environment, global, or collection variables) that can be used in subsequent requests.
2. How to Chain Requests in Postman
In order to chain requests, you will generally follow these steps:
- Make the first request to retrieve data.
- Use
pm.response
to extract the required data from the response. - Store the extracted data in variables (environment, global, or collection variables).
- In the next request, reference these variables to use the extracted data.
Example: Chaining Requests to Authenticate and Access User Data
In the following example, we first authenticate a user by sending a POST request with their credentials. We extract the authentication token from the response, then use that token to send a GET request to retrieve user information.
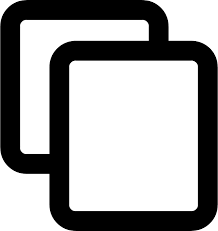
// Step 1: First request - Authenticate user and retrieve token
pm.test("Authentication successful", function () {
var jsonData = pm.response.json();
pm.environment.set("authToken", jsonData.token); // Store token in environment variable
});
// Step 2: Second request - Use the token for authentication in subsequent requests
pm.test("Authenticate user and access data", function () {
var token = pm.environment.get("authToken"); // Retrieve token from environment variable
pm.expect(token).to.not.be.null; // Ensure token is not null
});
3. Using Scripts to Automate Data Flow Between Requests
Postman scripts allow you to automate the extraction and use of dynamic data between requests. This is especially useful when testing APIs that require authentication or when performing multiple sequential API calls where the data generated by one request feeds into another.
Example: Chaining Requests with Dynamic Data
In this example, we send a POST request to create a new user and then use the created user’s ID in the next request to update the user information.
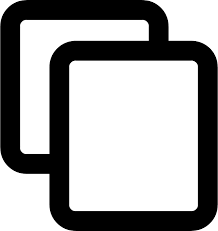
// Step 1: Create new user and extract userId
pm.test("User created", function () {
var jsonData = pm.response.json();
pm.environment.set("userId", jsonData.id); // Store userId in environment variable
});
// Step 2: Update user details using extracted userId
pm.test("Update user details", function () {
var userId = pm.environment.get("userId"); // Retrieve userId from environment variable
pm.expect(userId).to.not.be.null; // Ensure userId is not null
// Use userId in the request body or URL
var requestBody = {
"userId": userId,
"name": "Updated Name",
"email": "updated.email@example.com"
};
pm.request.body.raw = JSON.stringify(requestBody); // Update the body with new data
});
4. Chaining Requests with Dynamic URLs
Besides request bodies, you can also chain requests using dynamic URLs. This is useful when the URL of the next request depends on the response from the previous request.
Example: Chaining Requests with Dynamic URLs
In this case, we first send a GET request to retrieve a list of users, then use the ID of the first user to send a GET request to retrieve the user’s details.
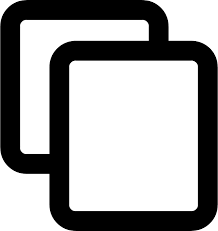
// Step 1: Get the list of users
pm.test("Retrieve list of users", function () {
var jsonData = pm.response.json();
pm.environment.set("firstUserId", jsonData[0].id); // Store the ID of the first user
});
// Step 2: Use the first user's ID to get user details
pm.test("Retrieve user details", function () {
var userId = pm.environment.get("firstUserId"); // Retrieve firstUserId from environment variable
var userDetailsUrl = "https://api.example.com/users/" + userId; // Construct dynamic URL
pm.sendRequest(userDetailsUrl, function (err, res) { // Send a GET request to the dynamic URL
pm.expect(res.status).to.equal(200); // Validate response status
var userDetails = res.json();
pm.expect(userDetails.id).to.equal(userId); // Validate user details
});
});
5. Handling Errors and Conditional Logic in Chained Requests
When chaining requests, you may need to handle errors or conditional logic based on the outcome of each request. Postman allows you to write logic in the tests section to check for specific conditions before proceeding with subsequent requests.
Example: Conditional Logic Based on Previous Request
This example demonstrates how you can stop the execution of further requests if a certain condition is met, such as if a previous request failed.
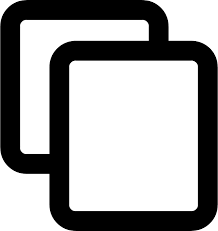
// Step 1: Check if the authentication request was successful
if (pm.response.code === 200) {
var jsonData = pm.response.json();
pm.environment.set("authToken", jsonData.token); // Store token if successful
// Step 2: Continue with further requests if the token is successfully retrieved
pm.test("Proceed with next request", function () {
var token = pm.environment.get("authToken");
pm.expect(token).to.not.be.null;
});
} else {
// If authentication failed, stop further requests
pm.test("Authentication failed, stopping further requests", function () {
pm.expect(false).to.be.true; // Fail the test and prevent further requests
});
}
6. Diagram: Chaining Requests in Postman
The following diagram illustrates how requests are chained together in Postman, where the output of one request serves as the input for the next request:

This diagram shows the flow of data between requests and how Postman can automate the process of chaining them together.
Automating API Testing with Postman
Automating API testing with Postman helps streamline the testing process, enabling faster and more efficient validation of APIs. With Postman’s built-in tools, you can automate the execution of your tests and run them on multiple environments or with different data sets, ensuring comprehensive test coverage.
1. What is API Test Automation?
API test automation involves running predefined tests on your API endpoints automatically. This process ensures that APIs are functional and meet the specified requirements without requiring manual intervention. Postman offers various features like Collections, Environments, and the Postman Runner to automate API testing workflows.
2. Using Postman Collections for Automation
Postman Collections serve as a container for your API requests. Once you group your requests into a collection, you can run them in a specific order with automated tests. Collections enable you to organize API tests and automate their execution through the Postman Collection Runner or Newman CLI.
Steps to Automate API Testing using Postman Collections
- Create a Collection and add requests that represent the API tests.
- Write Test Scripts for each request to validate the API's response.
- Use Environment Variables to run tests across multiple environments.
- Run the Collection Runner to execute tests automatically.
3. Postman Collection Runner
The Postman Collection Runner is a tool that allows you to run your entire collection of API requests in one go. You can execute your tests on multiple environments, run them with different data sets, and view test results in the same interface.
Steps to Use Postman Collection Runner
- Open the Postman application and navigate to your Collection.
- Click on the Runner button located in the top-right corner.
- Select the Environment for the test execution.
- Choose to run the collection for a specific number of iterations or using a data file.
- Click Start Run to begin automating the test execution.
Example: Running a Collection with Multiple Iterations
Here’s an example where we run the same collection multiple times with different sets of input data from a CSV file. This helps test your API with various input conditions.
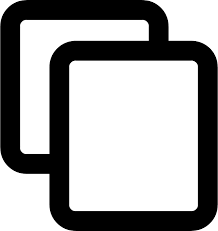
// Example data file (data.csv)
userId, email
1, user1@example.com
2, user2@example.com
3, user3@example.com
// Example test script for the API request
pm.test("Email is valid", function () {
var email = pm.request.body.email; // Get email from the request data
pm.expect(email).to.match(/^.+@.+\..+$/); // Test the validity of the email
});
In this example, the collection is run three times, once for each user in the CSV file. Each iteration tests the email validation for different users.
4. Newman: Running Collections from the Command Line
Newman is the command-line version of Postman, allowing you to run collections and automate tests in a CI/CD pipeline. You can install Newman globally and use it to execute your Postman collections from the terminal or integrate it into your build process.
Steps to Run Postman Collections Using Newman
- Install Newman by running the following command in your terminal:
npm install -g newman
- Export your collection from Postman.
- Run the collection with Newman using this command:
newman run path/to/your/collection.json
- View the results in your terminal or generate a report in HTML or JSON format.
Example: Running a Collection with Newman
Here’s an example of running a collection with Newman and generating an HTML report:
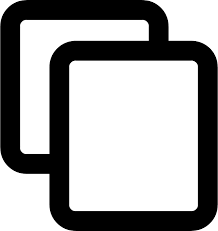
newman run my_collection.json -r html --reporter-html-export report.html
This command runs your collection and generates an HTML report named report.html
.
5. Scheduling Automated Tests
You can schedule automated tests to run at specific intervals using Postman Monitors. Monitors allow you to run your tests at regular intervals (e.g., hourly, daily) and receive reports on the results. This feature helps keep track of your API’s health and performance over time.
Steps to Set Up Postman Monitors
- Navigate to your Collection in Postman.
- Click on the Monitor button located in the top-right corner.
- Set the frequency for the monitor (e.g., hourly, daily).
- Choose the environment to run the monitor in.
- Click Create Monitor to schedule the tests.
6. Benefits of Automating API Testing with Postman
- Consistency: Automated tests run the same way every time, ensuring consistency across test executions.
- Efficiency: Automated tests save time compared to manual testing, allowing for faster feedback and more frequent testing.
- Comprehensive Testing: Running tests on different environments and with different data sets ensures a comprehensive validation of your API.
- Integration with CI/CD: Postman tests can be integrated into your CI/CD pipeline using Newman, helping to automate testing during the build and deployment process.
- Monitoring API Health: Postman Monitors allow you to keep track of your API’s performance and availability over time.
7. Diagram: Automated API Testing Workflow
The following diagram illustrates the workflow of automated API testing using Postman, from creating collections and writing tests to running them with the Postman Collection Runner or Newman:

This diagram helps visualize the process of automating API tests and ensuring that your API works as expected in various scenarios.
Running Collections with Postman Runner
Postman Runner allows you to run collections of API requests in a specific sequence, making it easier to automate and validate multiple requests without manually triggering them. This tool is ideal for running tests, simulating workflows, and validating the behavior of an entire API endpoint in a batch.
1. What is Postman Runner?
The Postman Runner is a feature in Postman that enables you to execute entire collections of requests in bulk. This tool runs your requests and executes the associated tests, providing detailed feedback in terms of response status, time, and results of predefined tests. It allows you to test various endpoints in one go, ensuring your API behaves as expected.
2. How to Use the Postman Collection Runner
To run a collection using Postman Runner, follow the steps below:
Steps to Run a Collection in Postman Runner
- Open the Postman application and navigate to the Collection you want to run.
- Click on the Runner button located at the top-right corner of the Postman interface.
- A new window will open, showing the collection you've selected.
- Select the Environment (optional) and Data File (if you're using one) for the run.
- Click Start Run to begin executing your requests.
Example: Running a Collection
In this example, you can run a collection of API requests to test a login system. The collection could contain requests for logging in, fetching user data, and checking if the user has the correct permissions.
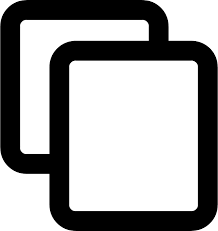
# Example Collection of Login System API Requests:
1. POST /login
2. GET /user-profile
3. GET /user-permissions
Once you click Start Run, Postman will execute all requests in this collection and display the results in the Runner window.
3. Using Data Files with Postman Runner
Postman Runner allows you to use external data files (CSV or JSON) to run the same collection with different sets of input data. This is especially useful when testing an API with multiple data sets or performing load testing on your API.
Steps to Use Data Files with Postman Runner
- Prepare a CSV or JSON file containing the input data for your requests.
- Click on the Runner button and select the collection to run.
- Click on Select File under the Data section in the Runner window.
- Choose the CSV or JSON file that you want to use for the test runs.
- Click Start Run to execute the collection with the input data from the file.
Example: Using a CSV Data File
Here’s a sample data file (CSV) that passes user credentials for the login API:
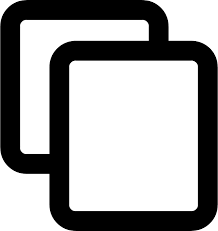
# Example CSV Data File (login_data.csv)
username, password
user1, password123
user2, password456
user3, password789
Each row in the CSV file represents a different set of user credentials that will be used to run the login request in the collection.
4. Monitoring Collection Run Results
Once the collection run is completed, the Postman Runner provides detailed feedback on the results:
- Request Status: The status of each request (e.g., 200 OK, 404 Not Found).
- Response Time: The time taken for each request to complete.
- Tests Results: The results of any predefined tests for each request.
Example: Viewing Results in Postman Runner
In the results window, you’ll see a table with all requests listed, their statuses, response times, and test results. If a request fails, you can click on it to view the response body and error details for further troubleshooting.
5. Exporting Results from Postman Runner
After running a collection, you can export the results as an HTML or JSON report for further analysis or sharing with your team. To export the results:
Steps to Export Results from Postman Runner
- After the run completes, click the Export button in the results window.
- Choose the format (e.g., HTML or JSON) for the report.
- Save the report file to your desired location.
Example: Exporting Results to HTML
Once exported, you can open the HTML report in a browser to view a detailed summary of the test run, including the status of each request, response time, and any failed assertions.
6. Benefits of Using Postman Runner
- Batch Testing: Run multiple requests at once in a predefined order, which is useful for testing workflows and validating multiple endpoints.
- Automated Data Testing: Use CSV and JSON data files to automate testing with different sets of data.
- Easy Debugging: View detailed results and error information to troubleshoot failing requests.
- Integration with CI/CD: Postman Runner can be integrated with continuous integration pipelines, allowing you to automate the testing of your API during every build or deployment.
7. Diagram: Postman Runner Workflow
The following diagram shows the flow of using Postman Runner to run collections with data files and monitor the results:

This diagram illustrates how data files and the Runner interface come together to create automated testing workflows in Postman.
Data-driven Testing Using CSV and JSON Files
Data-driven testing in Postman allows you to run the same set of requests multiple times with different input values. By using external data files, such as CSV or JSON, you can automate the testing of your API with various data sets, improving the efficiency and coverage of your tests. This approach is ideal when you need to test the same API with different parameters or inputs.
1. What is Data-driven Testing?
Data-driven testing is a method that involves running the same tests with different sets of input data to verify the behavior of an API under various scenarios. In Postman, you can use external data files (CSV or JSON) to supply different values for your requests, enabling you to test the same collection with multiple data sets.
2. Preparing Your Data Files
Before you can perform data-driven testing in Postman, you need to prepare a CSV or JSON file that contains the input data for your tests. Below are examples of both types of data files:
Example CSV Data File
In a CSV file, you structure the data in rows and columns. Each row represents a test case, and each column represents a parameter for the test:
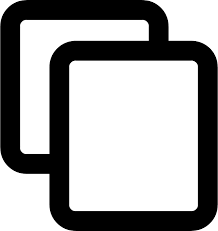
# Example CSV Data File (login_data.csv)
username, password
user1, password123
user2, password456
user3, password789
Example JSON Data File
In a JSON file, you structure the data as key-value pairs. Each object in the array represents a test case:
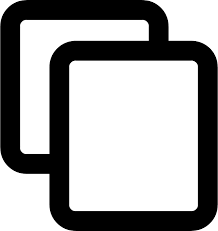
# Example JSON Data File (login_data.json)
[
{
"username": "user1",
"password": "password123"
},
{
"username": "user2",
"password": "password456"
},
{
"username": "user3",
"password": "password789"
}
]
3. Running Data-driven Tests in Postman
Once you've prepared your data files, you can use Postman Runner to execute the tests with different input values. Here's how to run a collection using data-driven testing:
Steps to Run Data-driven Tests in Postman
- Open Postman and select the Collection you want to run.
- Click on the Runner button at the top-right corner of the interface.
- In the Runner window, select the Data file you want to use (CSV or JSON).
- Choose your Environment (optional), which contains the variables you want to use in the requests.
- Click Start Run to begin executing your requests using the input data from your file.
Running with CSV Data
When you run a collection with a CSV file, Postman will iterate through each row of the file and use the values in the columns to populate the variables in the requests. For example, if your CSV file has columns for username
and password
, Postman will substitute these values into the request body or URL as needed.
Running with JSON Data
Similarly, when you use a JSON file, Postman will treat each object in the array as a separate test case and populate the request data with the corresponding values from the JSON keys.
4. Using Data from CSV/JSON in Requests
To use the data from the CSV or JSON file in your requests, you need to reference the column names (CSV) or keys (JSON) in your request body, URL, or parameters. Here's how to do it:
Using CSV Data in Requests
In your request, use the following syntax to reference a column in the CSV file:
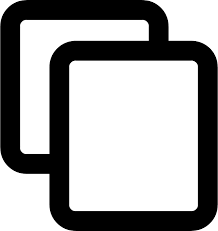
# Example POST request using CSV data
{
"username": "{{username}}",
"password": "{{password}}"
}
Using JSON Data in Requests
In your request, use the following syntax to reference a key in the JSON file:
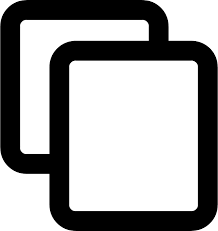
# Example POST request using JSON data
{
"username": "{{username}}",
"password": "{{password}}"
}
5. Monitoring Results of Data-driven Testing
After running data-driven tests, Postman Runner will show the results for each iteration. You can view the status and response for each test case, including whether the tests passed or failed. If a test case fails, you can click on the failed request to view the response body and error details.
Example: Viewing Results for Each Test Case
In the Runner window, you'll see a table with the results of each request, including:
- Request Status: The HTTP status code (e.g., 200 OK, 401 Unauthorized).
- Response Time: The time taken for the request to complete.
- Test Results: The outcome of the tests defined in the collection (e.g., assert statements).
6. Benefits of Data-driven Testing in Postman
- Test Coverage: Run the same test with different data sets to ensure comprehensive coverage of your API.
- Efficiency: Automate repetitive tests, saving time and effort during manual testing.
- Scalability: Easily scale your tests to cover hundreds or even thousands of data variations.
- Consistency: Ensure that your API behaves consistently across different input values.
7. Diagram: Data-driven Testing Flow
The following diagram illustrates the process of data-driven testing using CSV and JSON files:

The diagram shows how data from external files is fed into Postman requests, tested, and the results monitored.
Iterating Over Data Files in Tests
Iterating over data files in Postman tests allows you to automatically run a set of requests multiple times with different data inputs. This is particularly useful when you need to test multiple variations of an API with a single collection. By utilizing a data file (CSV or JSON), you can loop through each entry to test different parameters or payloads, streamlining your API testing process.
1. What Does Iteration Mean in Data-Driven Testing?
In data-driven testing, iteration refers to running a test multiple times, where each iteration uses a different set of input data. When you use a data file such as CSV or JSON, each row (CSV) or object (JSON) represents a different test case. During the run, Postman iterates over each item in the file and runs the requests accordingly.
2. Preparing Your Data Files for Iteration
Before you can iterate over data files, you need to prepare the data in CSV or JSON format. Postman will read the file and use the data in each row or object to execute the tests.
Example CSV Data File
A CSV file allows you to structure the data in rows and columns. Each row will represent a test case, and each column will represent a parameter:
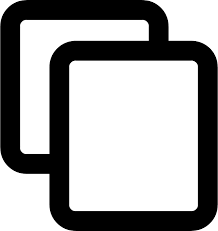
# Example CSV File (user_data.csv)
username,email,password
user1,user1@example.com,pass123
user2,user2@example.com,pass456
user3,user3@example.com,pass789
Example JSON Data File
A JSON file contains an array of objects, with each object representing a test case and containing key-value pairs for each parameter:
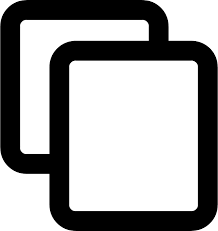
# Example JSON File (user_data.json)
[
{
"username": "user1",
"email": "user1@example.com",
"password": "pass123"
},
{
"username": "user2",
"email": "user2@example.com",
"password": "pass456"
},
{
"username": "user3",
"email": "user3@example.com",
"password": "pass789"
}
]
3. Iterating Over Data in Postman
Once you've prepared your data file, you can run your collection using the data file in Postman Runner. Postman will iterate over the data file row by row (CSV) or object by object (JSON) and use the values to execute requests.
Steps to Iterate Over Data in Postman
- Open Postman and select the Collection you want to run.
- Click on the Runner button at the top-right corner of the interface.
- In the Runner window, click on the Data button and select your CSV or JSON file.
- Select any Environment variables if needed.
- Click the Start Run button to begin the iteration process.
4. Using Data in Requests During Iteration
Postman will automatically replace variables in your requests with the values from each row (CSV) or object (JSON). You can reference these values in your request body, headers, URL, or parameters using the following syntax:
Using CSV Data in Requests
In the request, you can reference the column names from the CSV file by using the following format:
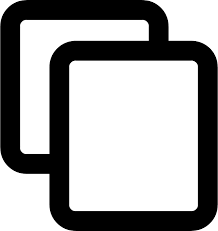
# Example POST request using CSV data
{
"username": "{{username}}",
"email": "{{email}}",
"password": "{{password}}"
}
Using JSON Data in Requests
In your request, you can reference the keys in the JSON file using the following format:
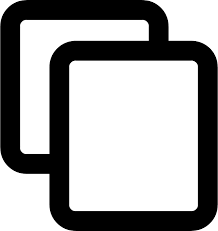
# Example POST request using JSON data
{
"username": "{{username}}",
"email": "{{email}}",
"password": "{{password}}"
}
5. Iteration with Pre-request Scripts and Tests
In addition to using the data in requests, you can also use the data in Pre-request scripts and tests to manipulate or validate the data before or after the requests are sent.
Example: Pre-request Script Using CSV Data
You can access the current iteration's data using pm.iterationData
in a pre-request script:
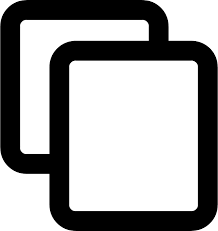
# Pre-request Script Example
pm.environment.set("username", pm.iterationData.get("username"));
pm.environment.set("email", pm.iterationData.get("email"));
pm.environment.set("password", pm.iterationData.get("password"));
Example: Test Using CSV Data
You can also use the iteration data in your test scripts to validate the responses for each iteration:
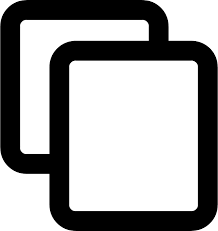
# Test Example
pm.test("Verify response for username: " + pm.iterationData.get("username"), function () {
pm.response.to.have.status(200);
pm.response.to.have.jsonBody("username", pm.iterationData.get("username"));
});
6. Benefits of Iterating Over Data Files
- Automation: Automatically test your API with multiple sets of data without manually modifying requests.
- Efficiency: Save time by testing different data variations in a single run.
- Scalability: Easily scale your tests to handle large data sets and test more scenarios.
- Consistency: Ensure that your API behaves consistently with different input data across multiple iterations.
7. Diagram: Iterating Over Data Files
The following diagram illustrates how Postman iterates over data files in the testing process:

This diagram shows the flow of data from the data file to the requests, tests, and response validation steps.
Creating Monitors for APIs
Postman monitors are a great way to automate and schedule the execution of your API tests. With monitors, you can run a collection of requests at specified intervals to check the health and functionality of your API endpoints. Monitors help you ensure that your API is working as expected, even outside of the development environment, by running tests regularly and sending alerts if something goes wrong.
1. What is a Postman Monitor?
A Postman monitor is a tool within Postman that allows you to run a collection of requests periodically, on a schedule. It executes the requests, checks the responses, and verifies that your API is functioning correctly. You can set the frequency of the runs, and the results are automatically logged for future analysis.
2. Benefits of Using Postman Monitors
- Automated Testing: Schedule your tests to run automatically at regular intervals, ensuring continuous monitoring without manual intervention.
- API Health Monitoring: Quickly detect and respond to issues with your API, such as broken endpoints, slow responses, or incorrect responses.
- Performance Tracking: Monitor the performance of your API over time, checking response times, latency, and uptime metrics.
- Alerting and Notifications: Receive alerts via email or integrations when your API tests fail, helping you address issues proactively.
- Historical Data: Access detailed logs and results of previous runs to track your API’s performance trends over time.
3. Creating a Monitor in Postman
To create a monitor in Postman, follow these steps:
- Open Postman and navigate to the Monitors tab on the left sidebar.
- Click the Create a Monitor button.
- Select the Collection that you want to monitor.
- Choose the environment that you want to associate with the monitor (optional).
- Set the frequency of the monitor by selecting an interval (e.g., every 5 minutes, hourly, daily, etc.).
- Set the email notifications for the monitor to notify you if the tests fail or succeed.
- Click Create Monitor to finalize your setup.
4. Configuring Monitor Settings
When setting up a monitor, there are several configuration options that you can customize:
Monitor Frequency
The frequency determines how often the monitor will run the selected collection. Options include:
- Every 5 minutes
- Hourly
- Daily
- Custom intervals (e.g., every 30 minutes)
Email Notifications
You can enable email notifications to receive alerts when a test fails. You can select who will receive notifications when the monitor runs.
Environment Selection
You can associate a specific environment with your monitor. This allows the monitor to run with the necessary variables (e.g., API keys, URLs) for the selected environment (e.g., production, development).
Monitor Timeout
Set a timeout value to control how long the monitor should wait for a response from the API before marking it as failed.
5. Running a Monitor
Once the monitor is created, it will automatically run at the specified frequency. You can track the results of each run, which will include:
- Test results (pass/fail)
- Response time
- Status codes
- Logs for each test
You can view the results of each monitor run in the Postman dashboard. If any tests fail, you will receive an alert via email, and you can investigate the issue by reviewing the logs and response details.
6. Viewing Monitor Results and Logs
After each monitor run, you can view detailed results that include:
- Status: Whether the test passed or failed.
- Response Time: The time it took for the API to respond to the request.
- Assertions: The assertions or checks that were made during the test (e.g., checking for a 200 status code).
- Logs: Detailed logs of each request and response, including headers, body, and status codes.
Example: Monitor Results
Here’s an example of the results you would see after running a monitor:
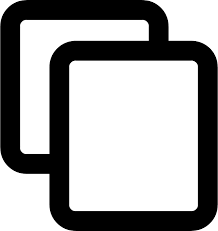
# Example Monitor Result
Test Run: Successful
- Test: "GET /users" - Passed
- Response Time: 250ms
- Status Code: 200 OK
- Test: "POST /users" - Failed
- Response Time: 300ms
- Status Code: 500 Internal Server Error
- Logs: "Error: API failed to create user"
7. Using Monitors for Performance Monitoring
Postman monitors can be used for performance monitoring by tracking the response times and status codes over time. You can set up monitors to run frequently and track performance metrics such as:
- Average response time
- Response time trends
- Uptime and availability of API endpoints
By regularly monitoring these metrics, you can identify potential performance bottlenecks or issues that may affect your API’s responsiveness.
8. Diagram: Postman Monitor Flow
The following diagram illustrates how a Postman monitor works:

This diagram shows the process from creating a monitor, running it at scheduled intervals, to viewing test results and logs for analysis.
Setting Up Alerts for Monitors
Postman allows you to configure alerts for monitors to notify you when something goes wrong with your API tests. These alerts ensure that you can quickly respond to issues, minimizing downtime and maintaining the reliability of your APIs.
1. What Are Monitor Alerts?
Monitor alerts notify you about the success or failure of your scheduled API tests. Notifications can be sent via email or through integrations with third-party tools like Slack, Microsoft Teams, or PagerDuty. These alerts help you stay informed about the health and performance of your APIs.
2. Benefits of Setting Up Alerts
- Real-time Notifications: Get instant updates when a test fails, allowing you to address issues quickly.
- Proactive Monitoring: Alerts help you stay ahead of potential problems by identifying issues before they impact users.
- Customizable Alert Settings: Configure alerts to notify specific team members or channels based on the nature of the issue.
- Integration with Tools: Integrate alerts with popular collaboration and incident management tools for streamlined communication.
3. Configuring Alerts for Monitors
Follow these steps to set up alerts for your Postman monitors:
- Navigate to the Monitors tab in Postman.
- Select the monitor for which you want to configure alerts.
- Click the Edit Monitor button.
- Scroll to the Notifications section.
- Enable the Email Notifications toggle.
- Add the email addresses of team members who should receive alerts.
- Optionally, configure integrations with third-party tools like Slack or PagerDuty.
- Click Save Changes to apply your settings.
4. Integrating with Third-party Tools
Postman supports integrations with various third-party tools to send alerts. Here’s how you can set up these integrations:
Slack Integration
- Go to the Integrations section in Postman.
- Search for Slack and click on it.
- Click Add Integration and authorize Postman to send messages to your Slack workspace.
- Select the channel where you want to receive notifications.
- Save the integration settings.
PagerDuty Integration
- In the Integrations section, search for PagerDuty.
- Click Add Integration and provide your PagerDuty API key.
- Map the monitor to a specific PagerDuty service.
- Save the integration settings.
5. Customizing Alert Triggers
Postman allows you to customize when alerts are triggered. You can configure alerts for the following scenarios:
- All Test Runs: Receive alerts for every test run, regardless of success or failure.
- Failed Test Runs Only: Receive alerts only when a test fails.
- Threshold-based Alerts: Configure alerts based on response times or other metrics exceeding specific thresholds.
6. Viewing Alert History
You can view the history of alerts in the Postman dashboard. This log provides insights into the frequency and nature of issues, helping you identify recurring problems. To access alert history:
- Open the Postman dashboard and navigate to the Monitors tab.
- Select the monitor you want to review.
- Click on the Runs tab to view detailed logs of previous runs.
- Check the Notifications section to see when alerts were sent and to whom.
7. Example: Email Alert
Here’s an example of an email alert you might receive when a monitor test fails:
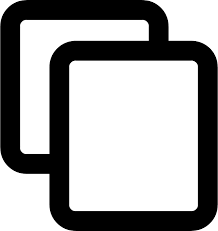
# Example Email Alert
Subject: Monitor Alert: "API Health Check" - Test Failed
Body:
Monitor Name: API Health Check
Collection: User Management API
Environment: Production
Test Run: Failed
Failed Tests: 2
- Test: "GET /users" - Status Code: 500
- Test: "POST /users" - Timeout Error
Timestamp: 2025-01-22 14:00:00 UTC
Please address these issues promptly.
8. Diagram: Alert Workflow
The following diagram illustrates the workflow of setting up and receiving alerts in Postman:

This diagram shows the process of configuring alerts, running monitors, and receiving notifications.
Generating API Documentation with Postman
Postman makes it easy to generate comprehensive and interactive API documentation. This documentation can be shared with team members, clients, or developers to ensure clarity and consistency in API usage.
1. What is API Documentation?
API documentation is a detailed manual that explains how to use an API, including endpoints, parameters, request/response examples, and error codes. Well-written documentation ensures developers can easily integrate and use the API effectively.
2. Benefits of Generating API Documentation in Postman
- Automatic Generation: Postman automatically generates documentation based on your collections and requests.
- Interactive UI: Users can interact with the API directly from the documentation.
- Real-time Updates: Changes in your collections are reflected in the documentation immediately.
- Ease of Sharing: Shareable URLs allow easy access to the documentation for team members or clients.
3. Steps to Generate API Documentation
Follow these steps to generate API documentation in Postman:
- Open the Postman app and navigate to the Collections tab.
- Select the collection for which you want to generate documentation.
- Click the View More Actions (⋮) button next to the collection name.
- Select View Documentation from the dropdown menu.
- Review and customize the auto-generated documentation. Add descriptions, example responses, or additional details.
- Click Publish to generate a shareable URL for your documentation.
4. Customizing API Documentation
You can enhance your API documentation by adding the following details:
- Request Descriptions: Provide a detailed description of each request, including the purpose and use case.
- Parameter Details: Document query parameters, headers, and body fields with clear explanations.
- Sample Responses: Include example responses for successful and failed requests.
- Error Codes: Provide a list of potential error codes and their meanings.
5. Sharing API Documentation
Once your documentation is ready, you can share it with others:
- Click the Publish button in the documentation view.
- Choose the visibility settings for your documentation (Public or Team).
- Copy the shareable URL and distribute it to your intended audience.
- Optionally, embed the documentation URL in your website or project wiki for easy access.
6. Example: Published API Documentation
Here’s an example of how published API documentation might look:
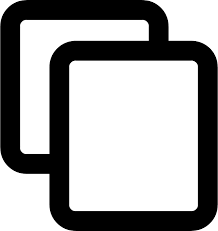
# Example API Documentation
Endpoint: GET /users
Description: Retrieve a list of all users.
Query Parameters:
- limit (optional): Number of users to return. Default is 10.
- sort (optional): Sort order (asc or desc). Default is asc.
Example Request:
GET https://api.example.com/users?limit=10&sort=asc
Example Response:
[
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
},
{
"id": 2,
"name": "Jane Smith",
"email": "jane.smith@example.com"
}
]
7. Diagram: API Documentation Workflow
The following diagram illustrates the steps involved in generating and sharing API documentation:

This diagram shows the process of creating, customizing, and publishing API documentation in Postman.
Publishing API Documentation
Publishing API documentation in Postman allows you to share your APIs with team members, clients, or the public. It creates a professional, interactive, and easily accessible resource for understanding and using your APIs.
1. Why Publish API Documentation?
Publishing API documentation provides several benefits:
- Easy Sharing: Generate a shareable URL for quick distribution.
- Interactive Interface: Users can test API endpoints directly within the documentation.
- Professional Presentation: Deliver a polished and consistent view of your API.
- Real-time Updates: Automatically synchronize changes to your documentation with your Postman collection.
2. Steps to Publish API Documentation
Follow these steps to publish your API documentation:
- In Postman, navigate to the Collections tab and select the collection you want to publish.
- Click the View More Actions (⋮) button next to the collection name and choose View Documentation.
- Customize the documentation by adding detailed descriptions, examples, and error codes.
- Click the Publish button at the top of the documentation view.
- Choose the visibility setting:
- Public: Anyone with the URL can view the documentation.
- Team: Documentation is restricted to your team members.
- Copy the generated URL and share it with the intended audience.
3. Customizing Published Documentation
Enhance your published API documentation by:
- Adding request and response examples for clarity.
- Including detailed descriptions for endpoints, parameters, and headers.
- Providing error code references to help users troubleshoot issues.
4. Managing Published Documentation
After publishing, you can manage your documentation as follows:
- Update the collection in Postman to automatically sync changes to the documentation.
- Use Postman’s dashboard to unpublish or modify the visibility of the documentation.
- Monitor usage statistics to understand how your documentation is being accessed.
5. Example: Published Documentation
Here’s an example of a published API documentation URL structure:
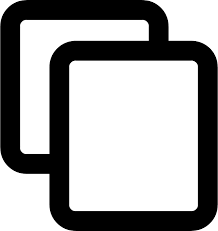
https://documenter.getpostman.com/view/12345678/ExampleAPI
6. Sharing Published Documentation
Share the documentation URL via email, embedded links in your project documentation, or within your team collaboration tools. This ensures easy access for your audience.
7. Diagram: Publishing Workflow
The following diagram illustrates the workflow of publishing API documentation in Postman:

The diagram shows the flow from creating collections to publishing and sharing the documentation.
Creating Mock Servers with Postman
Mock servers in Postman allow you to simulate API responses without needing an actual backend. This is useful for testing client-side applications, prototyping APIs, or demonstrating endpoints before the server is implemented.
1. What is a Mock Server?
A mock server replicates the behavior of a real API by responding to requests with predefined data. It helps developers test APIs and clients in isolation, ensuring that development can progress without dependencies on a live backend.
2. Benefits of Using Mock Servers
- Early Testing: Test APIs before they are fully implemented.
- Prototyping: Share a working prototype of your API with stakeholders.
- Independent Development: Work on the frontend and backend simultaneously.
- Consistent Responses: Ensure predictable and repeatable responses for testing.
3. Steps to Create a Mock Server in Postman
Follow these steps to create a mock server:
- Go to the Collections tab in Postman.
- Click the New button in the navigation bar and select Mock Server.
- Choose one of the following options:
- Create a New Collection: Set up a mock server for a brand-new collection.
- Use an Existing Collection: Enable mocking for an existing collection.
- Add examples for the requests in your collection. Examples define the mock server's response for specific requests.
- Click Create Mock Server and note the unique URL generated for your mock server.
- Use the mock server URL to send requests and receive responses based on the defined examples.
4. Adding Examples to Mock Servers
Examples are critical to defining the behavior of a mock server. To add examples:
- Select a request in your collection.
- Click on the Examples tab and then Add Example.
- Define the response body, headers, and status code for the example.
- Save the example to associate it with the request.
5. Managing Mock Servers
After creating a mock server, you can manage it as follows:
- View your mock server in the Mock Servers tab on Postman’s dashboard.
- Edit the mock server settings, such as name and visibility (public or private).
- Delete the mock server if it is no longer needed.
6. Example: Mock Server in Action
Here’s how to use a mock server:
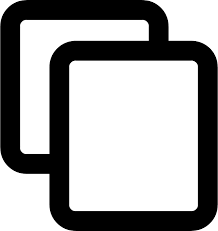
Request:
GET https://mockserver.example.com/users/123
Response Example:
{
"id": 123,
"name": "John Doe",
"email": "john.doe@example.com"
}
7. Diagram: Mock Server Workflow
The following diagram illustrates the workflow of creating and using a mock server:

The diagram shows how requests are sent to the mock server and how it responds with predefined data.
8. Tips for Using Mock Servers
- Organize your mock responses into collections for easier management.
- Use detailed examples to cover different scenarios, including error responses.
- Test your mock server thoroughly to ensure it behaves as expected.
Simulating Responses for Testing
Simulating responses is a crucial feature in Postman that allows developers to mimic the behavior of real APIs without needing an actual backend. This capability is particularly useful for testing, debugging, and prototyping client-side applications during the development process.
1. What is Response Simulation?
Response simulation involves creating predefined responses to API requests based on specific conditions or scenarios. This enables developers to test their applications under controlled environments, ensuring consistent and predictable outcomes.
2. Why Simulate API Responses?
- Early Testing: Test client-side code before the server-side implementation is complete.
- Error Handling: Validate how your application handles various responses, including errors.
- Consistency: Ensure that tests are repeatable and not dependent on the availability of a live backend.
- Prototyping: Demonstrate API functionality to stakeholders using realistic responses.
3. Steps to Simulate Responses in Postman
Follow these steps to simulate API responses:
- Create a Collection in Postman to organize your requests.
- Add a request to the collection that you wish to simulate.
- Click on the Examples tab below the request builder and select Add Example.
- Define the response for the example:
- Specify the response body (JSON, XML, etc.).
- Set headers, if required.
- Choose an appropriate status code (e.g., 200 OK, 404 Not Found).
- Save the example. The example will now be associated with your request.
- Enable a mock server for the collection or use an existing one.
- Send a request to the mock server URL, and it will return the simulated response.
4. Customizing Responses
You can customize responses to cover a wide range of scenarios:
- Success Responses: Simulate valid API responses with status code 200.
- Error Responses: Create examples with 400, 404, or 500 status codes to test error handling.
- Dynamic Responses: Include placeholders for dynamic data like timestamps or user-specific information.
5. Example: Simulated API Response
Here’s an example of a simulated response for a user profile request:
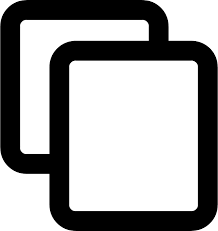
Request:
GET https://mockserver.example.com/api/users/1
Simulated Response:
{
"id": 1,
"name": "Jane Doe",
"email": "jane.doe@example.com",
"status": "active"
}
6. Tips for Simulating Responses
- Include Headers: Add headers like
Content-Type
to ensure client compatibility. - Use Multiple Examples: Create multiple examples for the same request to simulate different scenarios.
- Organize Examples: Group related examples into collections for easy access and management.
7. Diagram: Response Simulation Workflow
The diagram below illustrates the workflow of simulating API responses in Postman:

The diagram shows how requests are routed to a mock server, which returns predefined responses based on the associated examples.
8. Advantages of Response Simulation
- Reduces dependency on live backend systems during development.
- Enables offline testing and debugging.
- Supports iterative development and testing processes.
Using Mock Servers for Prototyping APIs
Mock servers in Postman allow developers to simulate APIs and their responses, enabling faster development and collaboration. By prototyping APIs with mock servers, you can test client applications, gather feedback, and iterate on API design without requiring a fully implemented backend.
1. What are Mock Servers?
Mock servers are virtual servers that replicate the behavior of real APIs. They return predefined responses based on the request, mimicking the actual API’s functionality. This enables development and testing to proceed independently of backend implementation.
2. Why Use Mock Servers for Prototyping?
- Faster Development: Start working on the frontend before the backend is ready.
- Improved Collaboration: Share mock endpoints with team members for testing and feedback.
- Iterative Design: Refine API structure based on client-side requirements without backend changes.
- Testing Scenarios: Test edge cases and error handling in a controlled environment.
3. Steps to Create a Mock Server in Postman
Follow these steps to set up and use a mock server:
- Navigate to the Collections tab in Postman and create a new collection or select an existing one.
- Add requests to the collection that you want to mock.
- For each request, define an example response by clicking on Examples under the request details and selecting Add Example.
- Go to the Postman workspace, click on Mock Server, and choose to create a mock server for an existing collection.
- Provide a name for the mock server and set visibility to either public or private.
- Copy the generated mock server URL and use it as the base URL for requests in your client application.
- Send requests to the mock server, and it will return the predefined responses.
4. Example: Setting Up a Mock Server
Here’s an example of setting up a mock server for a user management API:
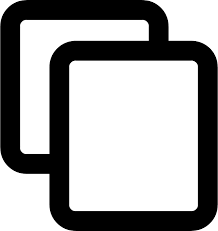
Mock Server URL:
https://mockserver.example.com
Request:
GET /api/users/1
Mock Response:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"role": "admin"
}
5. Customizing Mock Servers
You can customize mock servers to handle a variety of use cases:
- Multiple Examples: Attach multiple examples to a single request to simulate different scenarios.
- Dynamic Variables: Use Postman variables to dynamically generate parts of the response.
- Status Codes: Define responses with different HTTP status codes to test error handling.
6. Sharing Mock Servers
Mock servers can be shared with team members for collaborative development and testing:
- Share the mock server URL with developers, testers, and stakeholders.
- Use Postman’s shared workspace feature to collaborate on collections and mock servers.
- Provide API documentation alongside the mock server for better understanding.
7. Advantages of Using Mock Servers
- Independent Development: Frontend and backend teams can work simultaneously.
- Cost-Efficient: Eliminate the need for a live backend for testing and prototyping.
- Consistency: Ensure consistent API responses during development and testing.
8. Diagram: Workflow for Mock Servers
The following diagram illustrates how mock servers fit into the development workflow:

The diagram highlights how requests are sent to the mock server, which returns predefined responses, enabling seamless development and testing.
API Design and Development
API design and development is a critical part of software engineering, as it involves creating robust, scalable, and user-friendly APIs that power modern applications. Postman provides a comprehensive toolkit to streamline this process, from designing APIs to implementing and testing them.
1. What is API Design?
API design refers to the process of defining the structure, endpoints, and data formats of an API. A well-designed API ensures ease of use, maintainability, and compatibility with different client applications. Key considerations include:
- Consistency: Use consistent naming conventions and structures for endpoints.
- Clarity: Clearly define the purpose and functionality of each endpoint.
- Scalability: Design APIs to handle growing data and user demands.
- Security: Implement proper authentication, authorization, and validation mechanisms.
2. API Development Workflow in Postman
Postman simplifies the API development process with the following tools and features:
- API Definitions: Import or create API specifications using OpenAPI, Swagger, RAML, or GraphQL.
- Design: Define endpoints, request/response schemas, and example responses directly in Postman.
- Documentation: Automatically generate and publish API documentation for easy sharing and reference.
- Testing: Validate APIs with Postman’s testing tools, including pre-request scripts and assertions.
- Mock Servers: Use mock servers to simulate API behavior before backend implementation is complete.
- Versioning: Track changes and maintain versions of your API as it evolves over time.
3. Steps to Design an API in Postman
Follow these steps to design an API in Postman:
- Go to the APIs tab in Postman and click + New API.
- Provide a name, version, and description for your API.
- Select the API schema format (OpenAPI, GraphQL, etc.) and import an existing specification or create one from scratch.
- Define endpoints by specifying methods (GET, POST, etc.), paths, and example requests/responses.
- Save and organize your API schema for collaboration and further development.
4. Example: Creating an API Schema
Here’s an example of an API schema for a user management system:
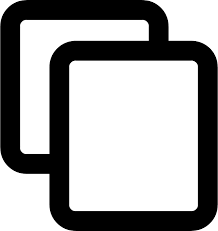
{
"openapi": "3.0.0",
"info": {
"title": "User Management API",
"version": "1.0.0",
"description": "An API for managing user data"
},
"paths": {
"/users": {
"get": {
"summary": "Get all users",
"responses": {
"200": {
"description": "A list of users",
"content": {
"application/json": {
"schema": {
"type": "array",
"items": {
"type": "object",
"properties": {
"id": { "type": "integer" },
"name": { "type": "string" },
"email": { "type": "string" }
}
}
}
}
}
}
}
}
}
}
}
5. Best Practices for API Design
- Use RESTful Principles: Stick to REST conventions for resource naming and HTTP methods.
- Provide Clear Documentation: Ensure that your API documentation is detailed and easy to understand.
- Include Error Handling: Provide meaningful error messages and HTTP status codes.
- Implement Security: Use secure authentication methods such as OAuth 2.0 and enforce SSL/TLS encryption.
- Enable Versioning: Use version numbers in API URLs or headers to maintain backward compatibility.
6. Diagram: API Design Workflow
The following diagram illustrates the API design and development workflow in Postman:

This diagram shows how different stages of API development, such as design, testing, and documentation, come together in Postman.
Importing and Editing API Specifications
Postman provides robust support for importing and editing API specifications, enabling teams to streamline their API design and development processes. You can import specifications in popular formats like OpenAPI, Swagger, RAML, and GraphQL, and make necessary edits directly within Postman’s intuitive interface.
1. Supported API Specification Formats
Postman supports the following API specification formats:
- OpenAPI: A widely used standard for designing RESTful APIs (versions 2.0 and 3.x).
- Swagger: A predecessor of OpenAPI, used for documenting APIs.
- RAML: A format for RESTful API modeling.
- GraphQL: A query language for APIs.
2. Importing API Specifications
Follow these steps to import an API specification into Postman:
- Go to the APIs tab in Postman and click + New API.
- Provide a name for your API and select the desired API specification format.
- Click Import and choose one of the following options:
- File: Upload a file from your local system.
- URL: Provide a publicly accessible URL.
- Paste Raw Text: Paste the API specification text directly.
- Click Import to load the API specification into Postman.
3. Editing API Specifications
After importing an API specification, you can edit it directly in Postman:
- Navigate to the APIs tab and select the API you want to edit.
- Click on the Edit button to open the specification editor.
- Make changes to the specification, such as adding or modifying endpoints, request/response schemas, and example responses.
- Use the Validate button to ensure your API schema adheres to the specification standard.
- Save your changes and share the updated specification with your team.
4. Example: Importing an OpenAPI Specification
Here’s an example of a simple OpenAPI specification that can be imported into Postman:
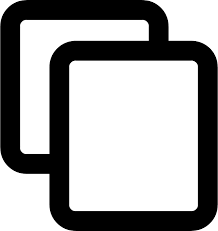
{
"openapi": "3.0.0",
"info": {
"title": "Sample API",
"version": "1.0.0",
"description": "A simple API example"
},
"paths": {
"/items": {
"get": {
"summary": "Retrieve a list of items",
"responses": {
"200": {
"description": "A JSON array of items",
"content": {
"application/json": {
"schema": {
"type": "array",
"items": {
"type": "object",
"properties": {
"id": { "type": "integer" },
"name": { "type": "string" }
}
}
}
}
}
}
}
}
}
}
}
5. Best Practices for Managing API Specifications
- Use Version Control: Keep track of changes to your API specifications using version control tools like Git.
- Validate Specifications: Regularly validate your API specifications to ensure compliance with standards.
- Collaborate with Teams: Share your API specifications with your team using Postman’s shared workspaces.
- Document Changes: Maintain detailed documentation for any updates or modifications to your API specifications.
6. Diagram: API Specification Import Workflow
The following diagram illustrates the workflow for importing and editing API specifications in Postman:

This diagram outlines the steps from importing an API specification to editing and validating it.
Versioning and Maintaining APIs
API versioning and maintenance are essential for ensuring the stability and evolution of your APIs over time. Postman provides tools to help you manage versions effectively, enabling developers to make changes while maintaining backward compatibility and minimizing disruptions for API consumers.
1. Importance of API Versioning
API versioning is critical for the following reasons:
- Backward Compatibility: Prevents breaking changes for existing users when new features or updates are introduced.
- Incremental Updates: Allows developers to add new functionalities without disrupting older versions.
- Clear Communication: Communicates changes and updates clearly to API consumers.
2. Common API Versioning Strategies
Here are some widely used strategies for versioning APIs:
- URI Versioning: Add the version number to the API endpoint URI (e.g.,
/v1/resource
). - Query Parameters: Specify the version as a query parameter (e.g.,
?version=1
). - Header Versioning: Include the version in the request header (e.g.,
API-Version: 1
). - Content Negotiation: Use the
Accept
header to specify the version (e.g.,application/vnd.api+json; version=1
).
3. Using Postman for API Versioning
Postman offers features to manage API versions seamlessly:
- Create Versions: Navigate to the APIs tab, select your API, and click Create Version to define a new version.
- Tag Versions: Use semantic versioning (e.g., 1.0.0, 2.0.0) to define major, minor, and patch updates.
- Maintain Multiple Versions: Postman allows you to manage multiple versions of an API in a single workspace.
- Link Collections: Associate specific collections with different API versions for better organization.
4. Best Practices for API Maintenance
Follow these best practices to effectively maintain your APIs:
- Deprecation Policy: Clearly communicate deprecations and provide a timeline for transitioning to newer versions.
- Monitoring and Testing: Regularly monitor API performance and test for issues using Postman’s monitors and test scripts.
- Documentation: Update API documentation to reflect changes and include examples for each version.
- Feedback Loop: Gather feedback from API consumers to identify and address issues proactively.
5. Example: URI Versioning
Here’s an example of how URI versioning works:
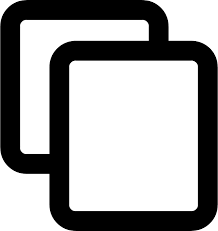
GET /v1/products
Response:
{
"version": "1.0",
"products": [
{ "id": 1, "name": "Product A" },
{ "id": 2, "name": "Product B" }
]
}
GET /v2/products
Response:
{
"version": "2.0",
"products": [
{ "id": 1, "name": "Product A", "price": 100 },
{ "id": 2, "name": "Product B", "price": 150 }
]
}
6. Diagram: API Versioning Workflow
The diagram below illustrates the typical workflow for versioning and maintaining APIs:

This workflow demonstrates how to create, manage, and transition between API versions effectively.
Integrating Postman with GitHub and CI/CD Tools
Integrating Postman with GitHub and CI/CD tools allows you to streamline your API testing and automate workflows for consistent and efficient development. This integration helps in automating the execution of Postman collections, synchronizing your testing environment, and ensuring that your API tests are part of the continuous integration and deployment pipeline.
1. Integrating Postman with GitHub
Postman can be integrated with GitHub to manage your Postman collections and environments in a version-controlled manner. This integration makes it easier for teams to collaborate and maintain their API tests, share collections, and track changes over time.
Steps to Integrate Postman with GitHub:
- Link GitHub to Postman: In Postman, go to your profile icon and select Settings. Under the Integrations tab, enable the GitHub integration and sign in to your GitHub account.
- Create a GitHub Repository: Create a repository on GitHub where you will store your Postman collections and environments.
- Push Collections to GitHub: Once linked, you can push your Postman collections directly to GitHub. You will be able to commit changes and manage versions using Git.
- Pull Collections from GitHub: You can also pull the collections and environments from GitHub into Postman, ensuring that all team members have access to the latest API test definitions.
2. Integrating Postman with CI/CD Tools
Integrating Postman with CI/CD tools like Jenkins, GitLab CI, and CircleCI ensures that your API tests are automatically executed as part of the build pipeline. This integration helps catch errors early by testing APIs with every code change.
Steps to Integrate Postman with CI/CD Tools:
- Install Newman: Newman is the Postman CLI tool that can be used to run Postman collections from the command line. Install Newman globally using the following command:
npm install -g newman
- Create a Postman Collection: Create a collection in Postman and export it as a JSON file.
- Configure CI/CD Pipeline: Configure your CI/CD tool (e.g., Jenkins, CircleCI, GitLab CI) to run your Postman collection using Newman. Add a step in your pipeline to execute the collection file:
newman run /path/to/your/postman_collection.json
- Automate Tests: Add further configuration to run your Postman tests as part of your build or deployment process. This ensures that API tests are validated before deploying code to production.
3. Example: Running Postman Tests in Jenkins
Here’s an example of how you can integrate Postman API tests into a Jenkins pipeline:
- Create a Jenkins job and add a build step to execute the Newman command:
- Set up the Jenkins job to trigger automatically on every push to your GitHub repository or on a scheduled basis.
- View the results in Jenkins, including a detailed test report and status of the API tests.
newman run /path/to/your/postman_collection.json --environment /path/to/your/environment.json
4. Benefits of Integration
The integration of Postman with GitHub and CI/CD tools offers several benefits:
- Automation: Automate your API testing process, ensuring consistent and repeatable tests at every stage of development.
- Collaboration: Share Postman collections and environments with your team through GitHub, making collaboration easier and improving version control.
- Faster Feedback: Quickly identify issues in your API by running tests automatically with every code push, ensuring faster feedback loops.
- Continuous Integration: Integrate API tests as part of the continuous integration pipeline, ensuring that your APIs are always tested before deployment.
5. Example: GitHub Actions for Postman API Tests
Here’s an example configuration for running Postman tests as part of a GitHub Actions pipeline:
name: Run Postman Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Install Newman
run: npm install -g newman
- name: Run Postman Collection
run: newman run /path/to/your/postman_collection.json --environment /path/to/your/environment.json
6. Diagram: CI/CD Pipeline with Postman Integration
The following diagram illustrates the workflow of integrating Postman with CI/CD tools like Jenkins or GitHub Actions:

This diagram shows how Postman tests are automatically triggered after code changes and integrated into the CI/CD pipeline.
Working with Jenkins, Newman, and Docker for CI/CD
Integrating Postman API tests into your CI/CD pipeline with Jenkins, Newman, and Docker enables automated testing of APIs as part of the build and deployment process. This combination provides a robust solution for running Postman collections in a controlled and repeatable environment.
1. Overview of Jenkins, Newman, and Docker
Jenkins is an open-source automation server often used to implement CI/CD workflows. Newman is the command-line companion for Postman that allows you to run Postman collections and tests in a CI/CD pipeline. Docker is a containerization platform that enables you to package and run applications in isolated environments, ensuring consistent results across different environments.
2. Setting Up Jenkins for Postman API Tests
Jenkins is used to automate the execution of Postman tests through Newman in the CI/CD pipeline. Follow these steps to set up Jenkins to run Postman tests:
- Install Jenkins: Install Jenkins on your server or use Jenkins in a cloud environment like AWS, Azure, or GitHub Actions.
- Install Newman on Jenkins: To run Postman collections, you need to install Newman on the Jenkins server. You can install Newman using the following command:
npm install -g newman
- Create a Jenkins Job: Create a Jenkins pipeline job that triggers on code changes in your repository or on a scheduled basis.
- Configure the Job to Run Postman Tests: Add a build step in Jenkins to run your Postman collection using Newman. Here's an example command to run your collection:
newman run /path/to/your/postman_collection.json --environment /path/to/your/environment.json
- View Test Results: Jenkins will execute your Postman tests and display the results in the console output or a report. You can also use Jenkins plugins like "Newman Plugin" to visualize the results better.
3. Using Docker for Isolated Postman Test Execution
Docker can be used to run Postman tests in a containerized environment, ensuring consistency across different machines. Using Docker allows you to package your Postman collections and Newman in a container, so it can be executed on any platform with Docker installed.
Steps to Set Up Docker for Postman Tests:
- Create a Dockerfile: Write a Dockerfile to define the image for running Postman tests. Below is an example Dockerfile:
FROM node:14-alpine WORKDIR /app COPY . /app RUN npm install -g newman CMD ["newman", "run", "postman_collection.json", "--environment", "environment.json"]
- Build the Docker Image: Build the Docker image with the following command:
docker build -t postman-tests .
- Run the Container: After building the Docker image, run the container to execute the Postman tests:
docker run postman-tests
- Automate with Jenkins: In Jenkins, you can set up a pipeline that builds and runs the Docker container to execute Postman tests. Use a Jenkinsfile to create pipeline steps for building the Docker image and running the tests in the container.
4. Example Jenkinsfile with Docker
Below is an example Jenkinsfile configuration that uses Docker to run Postman tests:
pipeline {
agent any
stages {
stage('Build Docker Image') {
steps {
script {
sh 'docker build -t postman-tests .'
}
}
}
stage('Run Postman Tests in Docker') {
steps {
script {
sh 'docker run postman-tests'
}
}
}
}
}
5. Benefits of Using Jenkins, Newman, and Docker for CI/CD
Using Jenkins, Newman, and Docker in combination provides several advantages for CI/CD pipelines:
- Automation: Automate the execution of Postman tests every time code is pushed to the repository, ensuring that API issues are caught early.
- Isolation: Docker ensures that Postman tests run in a clean, isolated environment, eliminating discrepancies across different machines.
- Consistency: By using Docker containers, you ensure that tests are run in the same environment every time, improving test reliability.
- Scalability: Jenkins can scale to handle multiple test executions simultaneously, speeding up the feedback loop and improving the developer experience.
- Reporting: Jenkins can generate detailed reports of the test results, allowing you to track the health of your API over time.
6. Diagram: Jenkins, Newman, and Docker Workflow
The following diagram illustrates how Jenkins, Newman, and Docker work together in a CI/CD pipeline to automate Postman API tests:

This diagram shows the process of building and running Postman tests in a Docker container as part of a Jenkins pipeline.
Collaboration Features in Postman (Comments, Sharing, Roles)
Postman provides a set of collaboration features that allow teams to efficiently work together on API development and testing. These features include comments, sharing collections, and assigning roles to users, enabling seamless communication and coordination among team members.
1. Comments in Postman
Postman allows you to add comments to API requests, collections, and environments. This feature helps you provide feedback, share insights, and discuss specific aspects of your API with other team members. You can comment on individual requests or entire collections, making it easy to track discussions and suggestions.
How to Add Comments:
- Click on the request or collection you want to comment on.
- In the right sidebar, you’ll find the “Comments” section.
- Click the “Add a comment” button, type your message, and hit Enter to post your comment.
Example:
You can use comments to suggest improvements or ask questions related to a specific request in your collection. This helps in resolving issues or discussing changes without leaving the Postman environment.
2. Sharing Collections
Postman makes it easy to share your collections with teammates, allowing them to view, modify, or run the same API tests. This is particularly useful when working on large teams or when collaborating with external partners. You can share collections via the Postman workspace, providing a centralized place to store and access API tests.
How to Share Collections:
- Open the collection you want to share.
- Click on the "Share" button in the top-right corner of the collection view.
- Choose whether to share the collection with specific team members, teams, or publicly via a link.
- If sharing with team members, select the specific workspace or team, and set their permissions (view, edit, or comment).
Example:
Sharing a collection with a team allows everyone to test and develop the API together, ensuring that all members have access to the latest version of the API endpoints and tests.
3. Roles and Permissions in Postman
Postman provides role-based access control (RBAC) that allows workspace admins to assign different roles to team members. These roles determine what actions users can perform on collections, environments, and other assets within a workspace. The different roles in Postman include:
- Admin: Can manage all aspects of the workspace, including adding/removing members and changing permissions.
- Editor: Can edit collections, environments, and other workspace assets but cannot modify workspace settings or member roles.
- Viewer: Can only view the collections, environments, and requests but cannot make any changes.
- Commenter: Can add comments to requests, collections, and other resources but cannot edit or view detailed data.
How to Assign Roles:
- Go to the "Team" tab in your Postman workspace settings.
- Click on the member you want to assign a role to.
- Select the desired role from the dropdown menu and save the changes.
Example:
Assigning different roles to team members ensures that only the right people can access or modify sensitive resources, such as authentication credentials or environment configurations.
4. Benefits of Postman Collaboration Features
Postman’s collaboration features provide several advantages for teams working on APIs:
- Improved Communication: Comments allow for direct feedback and discussion about specific requests, making it easier to resolve issues and share insights.
- Seamless Sharing: By sharing collections and environments, everyone on the team stays up-to-date with the latest changes, ensuring consistency and reducing versioning issues.
- Access Control: Role-based permissions ensure that sensitive data is only accessible by the right team members, reducing the risk of unauthorized changes.
- Increased Productivity: Collaboration tools streamline the workflow, enabling faster API development and testing by reducing the need for external communication tools.
5. Diagram: Postman Collaboration Workflow
The following diagram illustrates the collaboration workflow in Postman, showing how team members can comment, share collections, and assign roles within a workspace:

This diagram demonstrates how various collaboration features work together to enhance the development process in Postman.
Introduction to Newman (CLI for Postman)
Newman is the command-line interface (CLI) for Postman, allowing you to run Postman collections directly from the terminal or command line. It enables automation of API tests, integration into CI/CD pipelines, and running tests in a more flexible, scriptable environment. Newman acts as a bridge between Postman and other development tools, offering a simple yet powerful way to execute and manage API test collections outside of the Postman application.
Why Use Newman?
Newman provides several advantages for developers, testers, and DevOps teams looking to automate or integrate their API testing workflow:
- Automation: Run Postman collections programmatically in automation scripts, reducing manual intervention.
- CI/CD Integration: Seamlessly integrate API tests into your CI/CD pipelines, allowing for continuous testing and faster feedback loops.
- Command-Line Flexibility: Execute tests without needing the Postman app, offering greater flexibility for headless environments.
- Environment Support: Easily switch between environments and variables, making it suitable for multi-environment testing.
How to Install Newman
To get started with Newman, you need Node.js installed on your system. Newman is a Node.js package that can be installed globally using npm (Node Package Manager). Below are the installation steps:
- Ensure that Node.js and npm are installed on your system. You can check by running the following commands:
node -v npm -v
- Install Newman globally by running the following command:
npm install -g newman
- Once installed, verify the installation by running:
newman -v
Running a Postman Collection with Newman
After installing Newman, you can run your Postman collection from the command line. To do so, you’ll need to export your collection from Postman and use the collection file in the Newman command.
Steps to Run a Collection:
- Export the Postman collection you want to run. In Postman, go to the "Collections" tab, click on the three dots next to the collection, and select "Export".
- Save the exported collection as a JSON file on your computer.
- Open the terminal or command line and navigate to the directory where the collection file is located.
- Run the following command in the terminal to execute the collection:
newman run
.json
Example Command:
Here’s an example of how to run a collection using Newman:
newman run "My Collection.json"
Newman with Environment and Global Variables
Newman supports the use of environments and global variables, just like the Postman app. You can specify the environment or global variables to be used during the collection run by including them in the command.
Example Command with Environment:
newman run "My Collection.json" -e "My Environment.json"
Generating Reports with Newman
Newman allows you to generate detailed reports after running the collection. You can choose from multiple formats such as HTML, JSON, or CLI output.
Example Command to Generate HTML Report:
newman run "My Collection.json" -r html
This generates an HTML report that can be opened in any web browser for a detailed view of the test results.
Running Newman in CI/CD Pipelines
Newman can be easily integrated into CI/CD pipelines to ensure that your API tests are executed automatically each time changes are made to your code or API. This integration helps ensure that your API remains functional and any issues are caught early in the development process.
Example Integration in Jenkins Pipeline:
pipeline {
agent any
stages {
stage('Run API Tests') {
steps {
sh 'newman run "My Collection.json"'
}
}
}
}
Benefits of Using Newman
By using Newman, you can automate and streamline your API testing process, improving the speed and reliability of your testing efforts.
- Headless Testing: Run tests from the command line without needing the Postman application.
- CI/CD Automation: Integrate API tests into your CI/CD pipeline for continuous testing.
- Flexible Reporting: Generate detailed reports for test results in multiple formats (HTML, JSON, etc.).
- Environment and Global Variables: Easily switch between environments and variables for multi-environment testing.
Diagram: Running Postman Collections with Newman
The following diagram illustrates how you can automate API testing using Newman, highlighting the flow from running tests in the CLI to generating reports and integrating them into CI/CD pipelines:

This diagram visualizes how Newman fits into the broader testing and CI/CD workflow, helping automate and streamline API test execution.
Installing and Running Newman
Newman, the command-line interface (CLI) for Postman, allows you to automate and run Postman collections from the terminal. It's a powerful tool for API testing, CI/CD pipeline integration, and running collections outside the Postman app. This section will guide you through the process of installing and running Newman on your system.
Prerequisites
Before installing Newman, ensure that you have Node.js and npm (Node Package Manager) installed on your system. If you don’t have them installed, follow these steps:
- Visit the official Node.js website and download the installer for your operating system.
- Run the installer and follow the prompts to complete the installation process.
- To verify that Node.js and npm are installed correctly, open your terminal and run the following commands:
You should see the version numbers of Node.js and npm if the installation was successful.node -v npm -v
Installing Newman
Once Node.js and npm are set up, you can install Newman globally on your system using npm. To install Newman, run the following command in your terminal:
npm install -g newman
This command installs Newman globally, making it available from any directory in the terminal. Once the installation is complete, you can verify that Newman is installed by checking its version:
newman -v
If the installation was successful, you should see the version number of Newman displayed in the terminal.
Running a Postman Collection with Newman
After installing Newman, you can begin running Postman collections from the command line. Follow these steps:
- Export the Postman collection you want to run. In Postman, go to the "Collections" tab, click on the three dots next to the collection, and select "Export".
- Save the collection as a JSON file on your computer.
- Open the terminal or command prompt and navigate to the directory where the collection file is saved.
- Run the following command to execute the collection:
- Newman will run the collection and output the results to the terminal.
newman run .json
Replace
with the actual filename of your exported collection.
Example Command to Run a Collection
Here’s an example of how to run a collection using Newman:
newman run "My API Collection.json"
This will execute the collection named "My API Collection.json" and display the test results in the terminal.
Running Collections with Environment Files
If your collection uses environments or variables, you can specify an environment file to use with the collection run. You can export an environment from Postman in a similar way to exporting a collection.
Example Command with Environment:
newman run "My API Collection.json" -e "My Environment.json"
This command will run the "My API Collection.json" collection while using the "My Environment.json" environment file to replace variables within the collection.
Generating Reports with Newman
Newman can generate detailed reports after executing a collection, which can be useful for logging and analysis. You can specify the desired report format, such as HTML, JSON, or CLI output. To generate a report in HTML format, use the following command:
newman run "My API Collection.json" -r html
This will generate an HTML report and save it in the current directory. You can open this report in any web browser to view the test results in a visually friendly format.
Running Collections with Additional Options
Newman also supports various options to customize the test execution. Some of the useful options include:
- -r: Specify the report format (e.g., HTML, JSON, CLI).
- -e: Use a specific environment file.
- -d: Provide a data file (e.g., CSV or JSON) for data-driven testing.
For example, to run a collection with a report in HTML format and a specific environment, you would use:
newman run "My API Collection.json" -e "My Environment.json" -r html
Integrating Newman into CI/CD Pipelines
Newman can be integrated into your CI/CD pipelines to automate API testing and ensure that your API remains functional during development. For example, you can integrate Newman into Jenkins pipelines to run API tests as part of your continuous integration process.
Example of Running Newman in Jenkins Pipeline:
pipeline {
agent any
stages {
stage('Run API Tests') {
steps {
sh 'newman run "My API Collection.json"'
}
}
}
}
Benefits of Using Newman
Newman provides several benefits for API testing and automation:
- Automated Testing: Easily integrate API tests into automated workflows.
- CI/CD Integration: Seamlessly run tests in your CI/CD pipeline for continuous feedback.
- Customizable Reports: Generate detailed reports in multiple formats for analysis and monitoring.
- Environment Management: Use environments and variables to customize your test runs across different stages of development.
Diagram: Running Postman Collections with Newman
The following diagram shows the process of running a Postman collection with Newman, from installation to execution in a CI/CD pipeline:

This diagram illustrates the flow of how Newman integrates into the development and testing lifecycle, automating the execution of API tests and reporting results.
Running Postman Collections from CLI
Running Postman collections from the Command-Line Interface (CLI) allows you to automate API tests and execute collections outside the Postman app. This is particularly useful for integrating API testing into CI/CD pipelines, performing batch testing, or running tests on remote environments. In this section, we’ll cover how to run Postman collections from the command line using Newman, the CLI tool for Postman.
Prerequisites
Before running Postman collections from the CLI, make sure you have the following:
- Newman installed: Ensure that you have installed Newman on your system. Follow the instructions in the previous section, Installing and Running Newman.
- Exported Postman Collection: You’ll need to export the Postman collection you want to run. To export a collection from Postman, go to the "Collections" tab, click on the three dots next to the collection, and select "Export". Save the collection as a JSON file.
Running a Collection Using Newman
Once you have exported your Postman collection and installed Newman, you can run your collection from the CLI by following these steps:
- Open the terminal or command prompt.
- Navigate to the directory where your exported collection file is located.
- Use the following command to run the collection:
newman run .json
Replace
with the actual filename of your exported Postman collection.
Example Command
Here’s an example of how to run a collection from the CLI:
newman run "My API Collection.json"
This will execute the collection "My API Collection.json" and display the results in the terminal.
Running Collections with Environment Files
If your collection uses environment variables, you can specify an environment file when running the collection. This allows you to use different environments (e.g., development, staging, production) in different scenarios.
- Export the environment you want to use from Postman (similar to exporting a collection).
- Run the collection with the environment file using the following command:
newman run .json -e .json
Replace
with your collection file and
with your environment file.
Example with Environment File
newman run "My API Collection.json" -e "My Environment.json"
This command runs the collection "My API Collection.json" with the environment "My Environment.json".
Running Collections with Data Files
Newman also supports data-driven testing, allowing you to run a collection with different sets of input data from a CSV or JSON file. This is useful for running the same collection with multiple sets of data.
- Prepare a CSV or JSON file containing the data you want to use for testing.
- Run the collection with the data file using the following command:
newman run .json -d .json
Replace
with your CSV or JSON file containing test data.
Example with Data File
newman run "My API Collection.json" -d "test-data.json"
This will run the "My API Collection.json" collection with the input data from the "test-data.json" file.
Generating Reports with Newman
Newman can generate detailed reports in different formats after running a collection. To generate a report in HTML format, use the -r
option:
newman run .json -r html
This command will generate an HTML report and save it in the current directory. You can view this report in any web browser.
Other Report Formats
In addition to HTML, Newman supports other report formats such as JSON, CLI, and more. For example, to generate a JSON report:
newman run .json -r json
Running Collections in Parallel
For larger projects, it might be beneficial to run multiple collections in parallel. Newman allows you to execute several collections simultaneously using the --parallel
option:
newman run .json --parallel 2
newman run .json --parallel 2
This will execute two collections in parallel, improving the efficiency of your testing process.
Example of Running Multiple Collections
newman run "Collection1.json" & newman run "Collection2.json"
This command will run both "Collection1.json" and "Collection2.json" collections simultaneously.
Automating Collection Runs with Scripts
You can automate the execution of Postman collections using scripts. For example, you can create a batch file or shell script that runs the collections with the desired options. This is especially useful for automating tests in a CI/CD pipeline.
Example Shell Script
#!/bin/bash
newman run "My API Collection.json" -e "My Environment.json" -r html
newman run "Another Collection.json" -r json
This script will run two collections: one with an HTML report and another with a JSON report. You can schedule this script to run at specific times, or trigger it as part of an automated testing workflow.
Benefits of Running Postman Collections from CLI
Running Postman collections from the CLI offers several advantages:
- Automation: Easily automate API tests and integrate them into continuous integration/continuous deployment (CI/CD) pipelines.
- Batch Testing: Run tests on multiple collections simultaneously or with different sets of data.
- Customizable Reports: Generate detailed reports in multiple formats for further analysis and monitoring.
- Environment Flexibility: Customize your tests for different environments and configurations.
Diagram: Running Postman Collections from CLI
The following diagram illustrates the process of running Postman collections from the CLI, from exporting the collection to generating reports and integrating into automated workflows:

This diagram highlights how you can leverage the CLI for automated and batch testing of Postman collections across different environments and data sets.
Generating Test Reports with Newman
Generating test reports is an essential part of API testing. When running Postman collections via the command line using Newman, you can generate detailed test reports that provide insights into the status, performance, and details of your tests. In this section, we will explore how to generate various test reports and understand their output.
Prerequisites
Before generating test reports with Newman, make sure you have:
- Newman installed: Ensure that you have Newman installed on your system. Follow the instructions in the Installing and Running Newman section.
- Postman collection to run: You need to have a Postman collection exported as a JSON file that you will run to generate the test reports.
Generating Default CLI Test Report
When you run a Postman collection with Newman, the default output will be displayed in the terminal/command prompt. This output includes information about the collection run, such as:
- Total requests made.
- Number of tests passed and failed.
- Response times and any errors.
To run a collection and generate the default test report, use the following command:
newman run .json
For example:
newman run "My API Collection.json"
Generating HTML Reports
Newman allows you to generate test reports in multiple formats. One of the most common formats is HTML, which provides a user-friendly, detailed report of the collection run. To generate an HTML report, use the -r
option followed by html
:
newman run .json -r html
This command will generate an HTML report and display it in the terminal. You can also specify the file path where you want the report to be saved by using the --reporter-html-export
option:
newman run .json -r html --reporter-html-export /report.html
This will save the HTML report in the specified directory.
Example Command
newman run "My API Collection.json" -r html --reporter-html-export "C:/Reports/api-test-report.html"
This generates the test report in HTML format and saves it in the C:/Reports/api-test-report.html
location.
Generating JSON Reports
In addition to HTML, Newman can generate a JSON report, which is useful for programmatically processing the test results. To generate a JSON report, use the -r
option followed by json
:
newman run .json -r json
To save the JSON report to a specific location, use the --reporter-json-export
option:
newman run .json -r json --reporter-json-export /report.json
Example Command
newman run "My API Collection.json" -r json --reporter-json-export "C:/Reports/api-test-report.json"
This will generate a JSON report and save it in the specified directory.
Generating CLI Reports
Newman also generates a command-line (CLI) report that is displayed directly in the terminal. This default report includes the collection run details and test results in a compact format. You can view this output by running the following command:
newman run .json
CLI reports are useful when you just need a quick overview of the test run and the results without generating a full report.
Generating JUnit Reports
If you want to integrate your tests with CI/CD tools like Jenkins, generating JUnit-style XML reports is useful. Newman supports JUnit reports, which can be processed by CI/CD tools to track the test results.
To generate a JUnit report, use the -r
option followed by junit
:
newman run .json -r junit
To specify the location to save the report, use the --reporter-junit-export
option:
newman run .json -r junit --reporter-junit-export /report.xml
Example Command
newman run "My API Collection.json" -r junit --reporter-junit-export "C:/Reports/api-test-report.xml"
This will generate a JUnit report and save it in the specified location.
Generating Multiple Reports Simultaneously
Newman allows you to generate multiple reports at the same time in different formats. For example, you can generate both HTML and JSON reports simultaneously by running the following command:
newman run .json -r html,json
You can also specify different file paths for each report format:
newman run .json -r html --reporter-html-export "C:/Reports/api-test-report.html" -r json --reporter-json-export "C:/Reports/api-test-report.json"
Customizing Report Output
Newman’s reporting options allow you to customize the output format, file location, and other settings. For instance, you can control whether to include detailed logs, request/response data, or additional information in the reports. These customizations can help tailor the report to your specific needs.
Viewing and Analyzing Test Reports
Once the report is generated, you can open it in a browser (for HTML reports) or use a text editor (for JSON and JUnit reports) to review the test results. The reports contain detailed information on:
- Request and response data.
- Assertions and whether they passed or failed.
- Execution time and performance metrics.
- Error details and stack traces (if any tests failed).
Benefits of Generating Test Reports with Newman
Generating test reports with Newman offers several benefits:
- Automated Tracking: Automatically generate test reports after each collection run to monitor your API tests over time.
- Integration with CI/CD: Use JSON or JUnit reports to integrate with CI/CD tools, ensuring continuous testing and validation of APIs.
- Customizable Output: Customize the report format to meet the needs of different stakeholders (e.g., development teams, QA, management).
- Detailed Analysis: Get detailed insights into the performance, errors, and status of your API requests.
Diagram: Newman Report Generation Process
The following diagram illustrates the process of generating test reports with Newman, from running the collection to generating and saving reports in different formats:

This diagram highlights the key steps in generating test reports, from executing the collection to storing the results in your preferred format.
Organizing Collections and Environments
Effective organization of collections and environments is crucial for maintaining a well-structured and scalable API testing workflow in Postman. This section will guide you on how to organize your collections and environments efficiently to optimize your testing process and make it easier to manage different API scenarios and configurations.
What are Collections and Environments?
Before diving into organization strategies, let's define what collections and environments are:
- Collections: A collection is a group of API requests that can be executed together. Collections allow you to group related requests, tests, and scripts that belong to a particular API or project.
- Environments: Environments are sets of variables that can be used across requests. These variables can hold values like URLs, authentication tokens, or other configuration settings that vary based on the environment (e.g., development, testing, production).
Organizing Collections
Properly organizing your collections will help you maintain clarity, reduce confusion, and improve testing efficiency. Here are some tips for organizing your collections:
- Use Folders Within Collections: Postman allows you to create folders within collections to organize requests by functionality, endpoint, or feature. For example, you can create folders for authentication, user management, or product API requests.
- Naming Conventions: Use consistent and descriptive naming conventions for collections, folders, and individual requests. For example, name collections after the API or project they belong to, and name requests based on the action they perform (e.g., "Create User" or "Get Product Details").
- Tagging Requests: Postman allows you to add tags to requests for better categorization. Tags are useful for quickly identifying and filtering requests related to specific topics or functionalities.
- Separate Test and Production Collections: Maintain separate collections for testing and production environments. This ensures that test data and production data do not overlap and minimizes the risk of accidentally making changes to the production environment.
Organizing Environments
Environments allow you to switch between different sets of configurations for various stages of development. Properly organizing your environments will help you efficiently manage and switch between settings for different scenarios. Here's how you can organize your environments:
- Use Descriptive Environment Names: Name your environments based on the stage of development they represent, such as "Development," "Staging," "Production," or "QA." This makes it easier to identify the correct environment for each scenario.
- Separate API URLs: Use environment variables to store API base URLs for different environments. For example, in the "Development" environment, you may use the URL
http://localhost:3000
, while in the "Production" environment, you may usehttps://api.example.com
. - Environment-Specific Variables: Store other environment-specific values, such as authentication tokens or user credentials, in environment variables. This ensures that sensitive data is kept separate and secure for each environment.
- Versioning Environments: When working on different API versions, you can create multiple environments to test against each version. This will allow you to ensure backward compatibility and manage different API behaviors effectively.
Best Practices for Managing Collections and Environments
Here are some best practices for managing collections and environments in Postman:
- Use Collection and Environment Templates: If you frequently use similar collections or environments, create templates that can be reused across different projects. This can save time and ensure consistency in your testing setups.
- Version Control for Collections: Use Postman’s version control feature to track changes in your collections. This is especially useful in team environments, where multiple people may be working on the same collections.
- Leverage Workspaces for Collaboration: Postman workspaces allow teams to collaborate on collections and environments in a shared space. Organize your collections and environments within workspaces to keep them isolated and easily accessible for all team members.
- Regularly Clean and Update Collections and Environments: As your project evolves, regularly review and clean up your collections and environments. Remove outdated requests, variables, and configurations that are no longer relevant.
Using Variables in Collections and Environments
Variables are essential in Postman, and they can be used to dynamically configure your collections and environments. There are three types of variables you can use:
- Global Variables: These variables are available across all collections and environments. Use them for values that are consistent across all projects, such as your organization's API base URL or other global settings.
- Environment Variables: These variables are specific to a particular environment. Use them for environment-specific values like API URLs, authentication tokens, or other configuration settings.
- Collection Variables: These variables are available only within a specific collection. Use them to define values that are specific to that collection, such as request IDs or test-specific parameters.
By using variables effectively, you can simplify your testing workflows and make it easier to manage different configurations for different environments.
Example: Organizing Collections and Environments
Let’s consider an example of a project with separate collections for "User Management" and "Product API." Each collection will have separate folders for "Create," "Read," "Update," and "Delete" operations. The project will also have different environments for "Development," "Staging," and "Production," with separate API base URLs and authentication tokens for each.
Here’s how the setup would look:
- Collections:
- User Management (Folder: Create, Read, Update, Delete)
- Product API (Folder: Create, Read, Update, Delete)
- Environments:
- Development (Variables:
base_url=http://localhost:3000
,auth_token=dev_token
) - Staging (Variables:
base_url=https://staging.api.example.com
,auth_token=staging_token
) - Production (Variables:
base_url=https://api.example.com
,auth_token=prod_token
)
- Development (Variables:
Summary
Organizing collections and environments in Postman is essential for maintaining a smooth and efficient API testing process. By using naming conventions, folders, variables, and maintaining separate collections for different stages, you can easily manage and scale your API testing efforts. Additionally, leveraging workspaces and version control ensures seamless collaboration within teams.
Using Consistent Naming Conventions
Using consistent naming conventions is crucial for maintaining clarity, improving collaboration, and managing Postman collections and environments effectively. Naming conventions help standardize the way you name your requests, collections, variables, and environments, making it easier for team members to understand and work with the API testing setup. This section will outline best practices for naming in Postman and how consistency can enhance your workflow.
Why Naming Conventions Matter
Consistent naming conventions help to:
- Improve Readability: Consistently named collections, requests, and variables make it easier for anyone working on the project to understand the structure and purpose of each element.
- Enhance Collaboration: When working in a team, consistent naming ensures that all team members are on the same page and reduces confusion when sharing collections or environments.
- Maintain Scalability: As your project grows, a consistent naming strategy ensures that your testing setup remains organized and easy to navigate, even with hundreds of requests or environments.
- Reduce Errors: With predictable naming, it's easier to identify and avoid conflicts, making your testing setup more reliable and reducing the likelihood of mistakes.
Best Practices for Naming in Postman
Here are the best practices for naming collections, requests, variables, environments, and other elements in Postman:
1. Naming Collections
The collection name should be descriptive and reflect the API or project it belongs to. You can follow these guidelines:
- API or Project Name: Start the collection name with the API or project name (e.g., "User Management API" or "Product Service").
- Functionality or Service: If the collection is for a specific functionality, include that in the name (e.g., "Authentication API," "Order Service").
- Versioning: If you are managing multiple versions of an API, consider including the version number in the collection name (e.g., "User Management API v1" or "Product API v2").
2. Naming Requests
Each request name should clearly describe the action it performs. Here are some naming tips for requests:
- Use Action Verbs: Start the request name with an action verb that clearly describes what the request does (e.g., "Create User," "Get Product Details," "Update Order").
- Include Resource or Endpoint: Include the resource or endpoint being accessed in the request name (e.g., "Get User By ID," "Delete Product," "Update Payment Info").
- Versioning in Requests: If applicable, include version details or environment-specific information in the request name (e.g., "Create User v2" or "Get Product Details (Dev)").
3. Naming Folders within Collections
Folders help organize requests within a collection. Use folder names to categorize requests based on functionality or endpoint:
- Group by Functionality: Organize requests into folders based on different functionality or features (e.g., "Authentication," "User Management," "Order Processing").
- Group by HTTP Method: If it makes sense for your project, you can organize requests by HTTP method (e.g., "GET Requests," "POST Requests," "PUT Requests").
- Group by Endpoint: You can also organize by different API endpoints (e.g., "Products API," "Users API").
4. Naming Environment Variables
Environment variables are essential for configuring your requests in different environments. Use clear, descriptive names for environment variables:
- Use Clear Descriptions: Name environment variables based on their purpose (e.g.,
base_url
,auth_token
,api_key
). - Prefix Variables for Clarity: Use prefixes to group related variables (e.g.,
dev_base_url
,prod_base_url
,dev_api_key
). - Follow Consistent Naming Patterns: Consistently use lower-case letters with underscores for multi-word variables (e.g.,
api_token
,user_id
). This makes variable names easy to read and understand.
5. Naming Global Variables
Global variables can be accessed across all collections and environments. When naming them, follow these best practices:
- Use for Global Data: Global variables should hold values that are consistent across your entire project (e.g.,
api_base_url
,auth_token
,user_id
). - Avoid Overuse: Global variables should only be used when necessary. Excessive use of global variables can lead to conflicts and confusion.
6. Naming Test Scripts and Pre-request Scripts
When naming your test scripts and pre-request scripts, it’s important to be clear about their purpose:
- Pre-request Script Names: Name the script based on the action it performs before the request is sent (e.g., "Set Auth Token," "Generate Random User ID").
- Test Script Names: Name the script based on the test it is performing (e.g., "Check Status Code," "Validate Response Body").
Example of Consistent Naming Convention
Here’s an example of how you can organize your Postman collections and environment variables using consistent naming conventions:
- Collection Name: "User Management API v1"
- Folder Names: "Authentication," "Create User," "Update User," "Delete User"
- Request Names: "Create User," "Get User By ID," "Update User Info"
- Environment Variables:
dev_base_url
,prod_base_url
,auth_token
- Global Variables:
api_base_url
,user_id
Summary
Consistent naming conventions are vital for maintaining clarity and efficiency when working with Postman. By using descriptive names for collections, folders, requests, and environment variables, you ensure that everyone working on the project can easily navigate and understand the structure of your API testing setup. Following these best practices will help improve collaboration, reduce errors, and make your Postman workflow more manageable and scalable as your project grows.
Writing Effective Tests and Assertions
Writing tests and assertions is a critical part of API testing to ensure the reliability and correctness of your API. In Postman, tests are written using JavaScript, and assertions are used to validate the responses from your API requests. This section will guide you through the process of writing effective tests, using assertions, and making sure your API performs as expected.
What Are Tests and Assertions?
Tests in Postman are scripts that run after the API response is received. They are used to validate the correctness of the response, ensuring that the API behaves as expected. Assertions are specific conditions or checks that you define in your test scripts. If the condition is met, the test passes; if not, it fails.
Examples of Assertions
Here are some common types of assertions used in API testing:
- Status Code Assertions: Ensure the API returns the expected HTTP status code (e.g., 200 OK, 404 Not Found).
- Response Time Assertions: Check that the response time is below a defined threshold.
- Response Body Assertions: Verify that the response body contains expected data or matches a specific structure.
- Headers Assertions: Validate the presence and correctness of response headers.
Writing Basic Tests in Postman
In Postman, tests are written in the Tests tab of the request. The tests are written in JavaScript, and the Postman API provides various libraries to help with writing assertions. Below is a simple example of a test script:
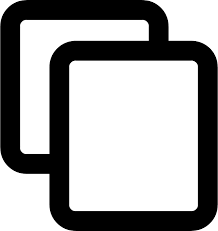
// Simple test to check the status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
Common Types of Assertions
Here are several types of assertions you can use in your Postman tests:
1. Status Code Assertions
You can check if the response returns the expected status code. For example:
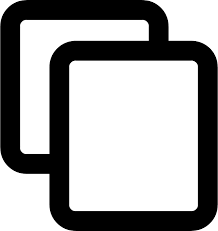
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
2. Response Body Assertions
Assertions can be made on the content of the response body. For example, you can check if a specific key exists or if a value matches:
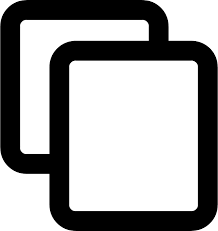
pm.test("Response body contains 'success'", function () {
pm.response.to.have.body('success');
});
3. JSON Response Assertions
For JSON responses, you can use Postman’s built-in helper methods to assert on specific fields in the JSON response:
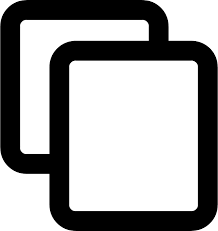
pm.test("Response has user_id", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.user_id).to.eql(123);
});
4. Response Time Assertions
It’s important to ensure that your API responds within a reasonable time. You can set an assertion on the response time:
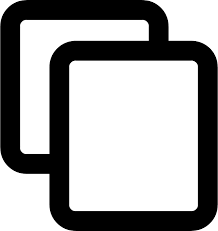
pm.test("Response time is less than 200ms", function () {
pm.expect(pm.response.responseTime).to.be.below(200);
});
5. Header Assertions
You can also assert on response headers to ensure they contain the necessary information. For example, checking the Content-Type header:
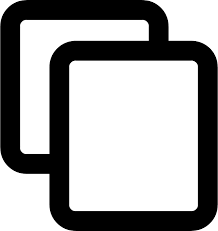
pm.test("Content-Type is JSON", function () {
pm.response.to.have.header("Content-Type", "application/json");
});
Using pm.expect for Custom Assertions
Postman’s pm.expect()
is a powerful assertion library that can be used to create complex assertions. For example:
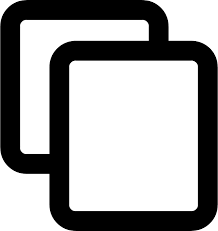
pm.test("Check if username is 'john_doe'", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.username).to.eql('john_doe');
});
Chaining Assertions
You can chain multiple assertions in a single test to check various conditions. For example:
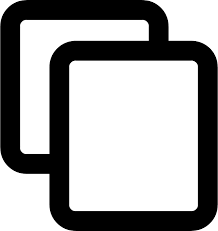
pm.test("Check status code and response body", function () {
pm.response.to.have.status(200);
pm.response.to.have.body('success');
});
Best Practices for Writing Tests
To write effective tests and assertions, follow these best practices:
- Keep Tests Simple: Focus on testing the most important aspects of the API, like status codes and key response fields.
- Write Readable Tests: Write clear and descriptive test names to improve readability for your team.
- Use Variables: Use environment variables, global variables, and data files to avoid hardcoding values in your tests.
- Test Edge Cases: Don't just test the happy path; include tests for invalid requests, error responses, and edge cases.
- Organize Tests: Group similar tests together and consider using folders in Postman to keep tests organized.
Summary
Writing effective tests and assertions in Postman ensures the reliability of your API by validating the response data, status codes, headers, and response times. By using the right assertions, following best practices, and organizing your tests well, you can create a robust API testing suite that helps prevent errors and ensures your API behaves as expected.
Version Control and Backup Strategies
Version control and backups are essential practices in software development and API management to ensure that your work is safe, traceable, and recoverable. Version control allows you to track changes to your collections and environments over time, while backup strategies ensure that your important data is securely stored and can be restored in case of data loss or corruption. This section discusses the best practices for version control and backup strategies within Postman.
Importance of Version Control
Version control allows you to keep track of changes in your Postman collections, environments, and other configurations. It ensures that you can revert to previous versions, collaborate efficiently with teams, and maintain a history of changes made over time. Version control is crucial when working in teams, as it helps prevent conflicts and allows seamless collaboration.
Benefits of Version Control
- Track Changes: Keep a history of all changes made to collections, environments, and scripts.
- Collaboration: Work with team members on the same collections and environments without overwriting each other's changes.
- Rollback: Roll back to a previous version of a collection or environment in case of issues or mistakes.
- Audit Trail: Maintain an audit trail of who changed what and when, which is essential for debugging and accountability.
Using Postman with Git for Version Control
Postman collections and environments can be exported as JSON files, which can then be version-controlled using Git. This allows you to use Git's version control features, such as commits, branches, and merges, to manage your Postman assets.
Setting Up Version Control with Git
Follow these steps to start using Git for version control with Postman:
- Export your Postman collection or environment as a JSON file.
- Initialize a Git repository in your project folder using the
git init
command. - Add the exported JSON files to the Git repository using
git add
and commit the changes usinggit commit
. - Push the repository to a remote service like GitHub, GitLab, or Bitbucket for collaboration and storage.
Example: Committing Postman Collection
Here's an example of how you might commit a Postman collection to Git:
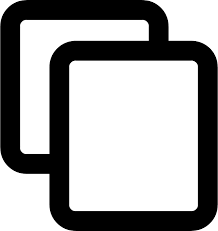
# Initialize Git repository
git init
# Add Postman collection JSON file
git add my-postman-collection.json
# Commit changes
git commit -m "Initial commit of Postman collection"
# Push to remote repository
git push origin main
Best Practices for Version Control
To manage your Postman assets efficiently, follow these version control best practices:
- Use Descriptive Commit Messages: Always use clear and descriptive commit messages that explain the reason for the changes.
- Branching: Use branches to experiment with different versions of your collections or environments without affecting the main version.
- Frequent Commits: Commit changes regularly to avoid losing progress and to keep the history organized.
- Review Pull Requests: When working with a team, use pull requests to review changes before merging them into the main branch.
Backup Strategies for Postman Data
Backups are critical to ensure that you don't lose important Postman collections, environments, or data. Regular backups ensure that you can recover your data in the event of system failures or mistakes. Postman provides several ways to back up your data, and these strategies will help you safeguard your work.
Manual Backup
Postman allows you to export collections and environments manually as JSON files. You can save these files to your local machine or a cloud storage service as backups.
Backup Process
To manually back up your collections and environments, follow these steps:
- Click on the collection or environment you want to back up.
- Click on the "..." (more options) button next to the collection or environment name.
- Select Export and choose the format you want (usually JSON).
- Save the exported file to a secure location on your computer or cloud storage service.
Automated Backup with Postman Sync
Postman provides a sync feature that automatically backs up your collections, environments, and other data to the cloud. To enable syncing:
- Sign in to Postman with your Postman account.
- Your collections, environments, and other data will be synced to the cloud automatically.
- You can access your data from any device by signing in with the same account.
Backup with External Cloud Services
You can also integrate Postman with external cloud services like Google Drive, Dropbox, or OneDrive for additional backup options. Simply export your Postman assets and upload them to your chosen cloud storage service for remote backup.
Best Practices for Backup Strategies
To ensure your data is backed up securely and consistently, follow these best practices:
- Regular Backups: Back up your data regularly, especially after making important changes to your collections or environments.
- Use Cloud Backup: Store backups in the cloud to ensure they're accessible from any device and protected from local system failures.
- Automate Backups: Use automated backup options like Postman Sync or integrations with cloud services to ensure your data is always backed up.
- Versioned Backups: Maintain multiple versions of your backups, so you can easily roll back to a previous state if needed.
Restoring Data from Backups
If you need to restore your data from a backup, simply import the JSON files you’ve saved from your manual or cloud backup into Postman. You can restore collections, environments, and other data with just a few clicks.
Summary
Version control and backup strategies are essential for managing Postman collections, environments, and other assets. By using Git for version control and implementing regular backup strategies, you can safeguard your work, collaborate efficiently with teams, and ensure that your data is recoverable in case of issues or data loss.
Debugging Requests in Postman
Debugging is a crucial part of the development process. When working with APIs, it’s common to encounter issues such as incorrect responses, failed requests, or misconfigured parameters. Postman provides several tools and features to help you debug requests and understand what's happening under the hood. This section will explore the different ways to debug API requests and troubleshoot issues efficiently using Postman.
Why Debugging is Important
Debugging helps you identify errors, find out why requests are failing, and correct issues with the API or request setup. By using Postman’s debugging features, you can quickly pinpoint problems with the request, the server, or the underlying code. Effective debugging improves the reliability of your API and helps speed up development time.
Postman Debugging Tools
Postman offers several built-in tools to assist with debugging, including the Console, Request and Response Logs, and detailed error messages. Here’s how you can use these tools:
1. Postman Console
The Postman Console is one of the most powerful debugging tools in Postman. It displays detailed logs of requests, responses, and scripts executed during a session. The console can help you identify issues such as incorrect headers, parameters, or request bodies, as well as errors thrown by pre-request scripts and tests.
How to Access the Postman Console
To open the Postman Console, click on the “Console” button at the bottom left of the Postman window or use the shortcut Ctrl + Alt + C (Windows/Linux)
or Cmd + Option + C (Mac)
.
What the Console Shows
- Request Logs: Details about the request, including the URL, headers, body, and method used.
- Response Logs: Information on the response from the server, including the status code, headers, and body.
- Pre-request and Test Logs: Logs from scripts, including any console logs added during testing or pre-request scripting.
- Error Messages: Any errors or warnings that occurred during the execution of the request or associated scripts.
2. View Detailed Request and Response Information
Postman provides detailed information about both the request and the response, which is useful when debugging. Below are some tips to help you debug:
Request Details
- Request URL: Make sure the URL is correct and properly formatted.
- Request Method: Ensure you're using the correct HTTP method (GET, POST, PUT, DELETE, etc.) for the intended action.
- Headers: Confirm that the headers are correctly set, especially for authorization, content type, and other API-specific headers.
- Request Body: Check the request body (if applicable) to ensure the data is correctly formatted (JSON, form-data, etc.).
Response Details
- Status Code: The status code (e.g., 200 OK, 400 Bad Request, 500 Internal Server Error) provides a quick indication of the request's success or failure.
- Response Body: Review the response body for error messages, missing data, or unexpected results. This can help identify the cause of issues.
- Headers: Check the response headers for useful information, such as content type, caching details, and custom headers defined by the API.
3. Common Debugging Scenarios
Here are some common scenarios you may encounter when debugging requests in Postman:
Incorrect Request Method
Using the wrong HTTP method (e.g., sending a POST request when a GET request is expected) can cause issues. Double-check the API documentation to ensure you’re using the correct method.
Authorization Failures
Many APIs require authentication (e.g., API keys, OAuth tokens). If you’re receiving a 401 Unauthorized response, make sure your authorization credentials are correct and included in the request headers.
Missing or Incorrect Parameters
If the API expects specific query parameters, headers, or request body data, missing or misconfigured parameters can lead to errors. Check the API documentation and ensure all required parameters are included.
Invalid JSON or Data Format
If your API expects data in JSON format and you're sending it as raw text or in another format, the request may fail. Use the raw body type and ensure the data is formatted correctly (e.g., valid JSON).
4. Using Console Logs for Scripts
If you’re using pre-request scripts or tests in Postman, you can log useful information to the Postman Console to track the execution flow. For example:
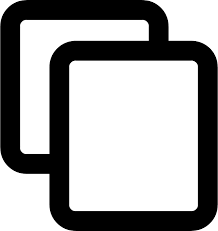
// Example of logging in a pre-request script
console.log('Request is about to be sent!');
console.log('Request URL:', pm.request.url.toString());
// Example of logging in a test script
pm.test("Response status is OK", function () {
pm.response.to.have.status(200);
console.log('Response Body:', pm.response.body);
});
5. Handling Timeout and Connection Errors
Sometimes requests may fail due to connection issues, such as timeouts. In Postman, you can adjust the timeout settings to allow more time for requests to complete:
- Go to Settings in Postman.
- Select the Request tab.
- Adjust the Request Timeout value to a higher number if you suspect that the server requires more time to respond.
Advanced Debugging with Postman Features
Postman also provides advanced features like mock servers, monitors, and environment variables to help with debugging complex API workflows. Here are a few ways to use these features for debugging:
Mock Servers
Mock servers allow you to simulate API responses. You can use mock servers to test your requests without hitting a live server or to simulate error conditions that are hard to replicate in real environments.
Monitors
Monitors help you schedule and run collections at regular intervals. These can be useful for detecting issues with your API over time or monitoring the performance of your API endpoints.
Environment Variables
Using environment variables can help you test the same API with different configurations (e.g., testing different environments such as staging and production) without changing the requests manually. This makes debugging easier when working across multiple environments.
Summary
Effective debugging in Postman involves using the Postman Console, reviewing request and response details, and identifying common issues such as incorrect methods, missing parameters, and authorization failures. By leveraging Postman’s debugging features and following best practices, you can troubleshoot API requests efficiently and improve the overall quality of your API interactions.
Common Errors and How to Fix Them
When working with APIs in Postman, it's common to encounter a variety of errors. These errors can arise from multiple sources, such as incorrect request configurations, server-side issues, or network problems. In this section, we’ll cover some of the most common errors you might face in Postman and provide troubleshooting steps to help you resolve them quickly.
1. 400 Bad Request
The 400 Bad Request error indicates that the server cannot process the request due to malformed syntax or missing parameters.
Possible Causes:
- Incorrect URL or endpoint.
- Missing required parameters in the request body, query, or headers.
- Malformed data in the body (e.g., invalid JSON or missing fields).
How to Fix:
- Double-check the URL and ensure it matches the API documentation.
- Verify that all required parameters are included in the request and that they are correctly formatted.
- If sending data in JSON format, ensure the JSON is valid. You can use a JSON validator to check the syntax.
2. 401 Unauthorized
The 401 Unauthorized error occurs when the server requires authentication, but the provided credentials are missing or invalid.
Possible Causes:
- Missing or incorrect API key, Bearer token, or other authentication credentials.
- Expired or revoked credentials.
How to Fix:
- Ensure that you have provided valid authorization credentials (API key, token, etc.) in the request headers.
- Check if the credentials have expired or been revoked. If so, renew them.
- If you're using Bearer tokens, verify that the token is correctly formatted and has the required scope.
3. 403 Forbidden
The 403 Forbidden error indicates that the server understands the request, but the client does not have permission to access the resource.
Possible Causes:
- Insufficient permissions for the authenticated user.
- IP restrictions or firewall settings blocking access to the API.
How to Fix:
- Check the permissions associated with your API key or credentials. Ensure that the user has access to the resource.
- Verify that IP restrictions or firewalls are not blocking your request.
4. 404 Not Found
The 404 Not Found error occurs when the requested endpoint does not exist on the server.
Possible Causes:
- The endpoint URL is incorrect or has a typo.
- The resource you're trying to access no longer exists or has been moved.
How to Fix:
- Verify that the URL is correct and matches the API documentation.
- Ensure that the endpoint exists and hasn’t been deprecated or moved to a different URL.
5. 500 Internal Server Error
The 500 Internal Server Error indicates that something went wrong on the server side. This error is usually not caused by the client but could be due to a server misconfiguration or overload.
Possible Causes:
- Server misconfiguration or bug in the backend code.
- Server overload or downtime.
How to Fix:
- Since this is a server-side error, there isn’t much you can do on the client side. However, check the server status or API status page for known issues or maintenance downtime.
- If you're the API provider, check server logs for any errors or misconfigurations.
6. Timeout Errors
A timeout error occurs when the server takes too long to respond, and the request times out before it can be completed.
Possible Causes:
- Server issues causing delays in processing the request.
- Network connectivity issues between Postman and the server.
- Large payload or complex operation causing server delay.
How to Fix:
- Check if the server is experiencing high traffic or performance issues. Consider retrying the request after some time.
- Increase the timeout setting in Postman if the server response time is expected to be longer.
- Ensure that your network connection is stable and reliable.
7. Invalid JSON or Data Format
When sending data in the request body, Postman may encounter issues if the data is not correctly formatted, such as an invalid JSON structure or incorrect Content-Type header.
Possible Causes:
- Improperly formatted JSON or XML data in the request body.
- Incorrect Content-Type header for the data being sent.
How to Fix:
- Ensure that the data you are sending is properly formatted. Use a JSON validator for JSON data or an XML validator for XML data.
- Set the correct Content-Type header (e.g.,
application/json
for JSON data) in the request.
8. 429 Too Many Requests
The 429 Too Many Requests error occurs when you exceed the rate limit imposed by the server or API provider.
Possible Causes:
- Sending too many requests in a short period, exceeding the server’s rate limit.
How to Fix:
- Check the API documentation for rate limit details and ensure that you're not exceeding the limit.
- Try to reduce the frequency of your requests or implement retry logic with exponential backoff.
- If you continue to hit the rate limit, consider reaching out to the API provider for increased limits.
Summary
Postman users may encounter a variety of errors, ranging from client-side issues like missing parameters or authentication failures to server-side errors like 500 Internal Server Error or 429 Too Many Requests. By understanding these common errors and following the troubleshooting steps provided, you can fix most issues quickly and continue testing your APIs with minimal disruption.
Interpreting HTTP Status Codes
HTTP status codes are responses from a server that indicate the result of a client’s request. Each status code is a three-digit number that belongs to a specific category, helping developers understand whether the request was successful, encountered an error, or requires additional action. Here’s a guide to the most common status codes and their meanings:
1. Informational Responses (1xx)
These status codes indicate that the server has received the request and is continuing to process it.
- 100 Continue: The server has received the request headers and the client can proceed to send the request body.
- 101 Switching Protocols: The server is switching to a different protocol as requested by the client.
2. Successful Responses (2xx)
These status codes signify that the request was successfully received, understood, and processed by the server.
- 200 OK: The request was successful, and the server returned the requested data.
- 201 Created: The request was successful, and a new resource was created as a result.
- 202 Accepted: The request has been received but has not yet been processed.
- 204 No Content: The server successfully processed the request, but there is no content to return.
3. Redirection Messages (3xx)
These codes indicate that further action is needed to complete the request, often involving a redirect to a different resource.
- 301 Moved Permanently: The requested resource has been permanently moved to a new URL.
- 302 Found: The requested resource resides temporarily under a different URL.
- 304 Not Modified: Indicates that the resource has not been modified since the last request, allowing the client to use a cached version.
4. Client Errors (4xx)
These status codes indicate that the request contains errors or is invalid from the client’s side.
- 400 Bad Request: The server cannot process the request due to malformed syntax or missing parameters.
- 401 Unauthorized: Authentication is required, and the provided credentials are missing or invalid.
- 403 Forbidden: The client does not have permission to access the resource.
- 404 Not Found: The requested resource could not be found on the server.
- 429 Too Many Requests: The client has sent too many requests in a given timeframe, exceeding the server’s rate limit.
5. Server Errors (5xx)
These codes indicate that the server encountered an error and could not process the request.
- 500 Internal Server Error: A generic error message indicating an unexpected server issue.
- 501 Not Implemented: The server does not support the functionality required to fulfill the request.
- 502 Bad Gateway: The server received an invalid response from an upstream server.
- 503 Service Unavailable: The server is temporarily unable to handle the request, often due to maintenance or overload.
- 504 Gateway Timeout: The server did not receive a timely response from an upstream server.
Tips for Handling HTTP Status Codes
- Monitor Server Responses: Use tools like Postman to check the status code of each request and ensure the API behaves as expected.
- Use Retry Logic: For temporary errors (e.g., 503 or 504), implement retry logic with exponential backoff to handle server unavailability gracefully.
- Validate Requests: Ensure that your requests include all required parameters and headers to avoid client-side errors (e.g., 400 or 401).
- Handle Redirects: For 3xx status codes, make sure your application can follow redirects if needed.
Summary
Understanding HTTP status codes is essential for effective API testing and debugging. By interpreting these codes correctly, developers can identify issues, improve request accuracy, and ensure a seamless integration between clients and servers.
Checking Response Time
Response time is a critical performance metric in API testing. It measures the time taken by the server to respond to a client’s request. Ensuring that APIs respond within acceptable time limits is essential for delivering a seamless user experience. Postman provides built-in tools to monitor and validate response times effectively.
Why Measure Response Time?
Measuring response time helps in:
- Performance Optimization: Identifying slow endpoints and optimizing server performance.
- User Experience: Ensuring that users do not experience delays in application interactions.
- SLA Compliance: Verifying that APIs meet agreed-upon Service Level Agreements (SLAs) for response times.
Checking Response Time in Postman
Postman displays the response time for every request in the interface. Follow these steps to check it:
- Send an API request using Postman.
- After the response is received, locate the Response Time value displayed in the Postman interface, near the status code and size.
Adding Response Time Validation in Tests
You can write test scripts in Postman to validate that the response time is within acceptable limits. Here’s how:
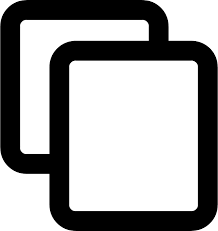
// Validate response time
pm.test("Response time is less than 200ms", function () {
pm.expect(pm.response.responseTime).to.be.below(200);
});
Explanation: The pm.response.responseTime
object retrieves the response time in milliseconds. The test ensures that the response time is below 200ms.
Best Practices for Response Time Validation
- Define Thresholds: Establish acceptable response time limits for each endpoint based on its purpose and user expectations.
- Run Load Tests: Use tools like Postman Runner or Newman to simulate multiple requests and observe response times under load.
- Monitor Trends: Regularly monitor response times to detect performance degradation or improvements.
- Include Tolerance: Allow a small margin for fluctuation in response times due to network conditions or server load.
Summary
Checking and validating response times is essential for ensuring optimal API performance and user satisfaction. Postman’s tools and scripting capabilities make it easy to measure, monitor, and enforce response time limits effectively.
Benchmarking API Performance with Postman
Benchmarking API performance is an essential practice to ensure that your APIs deliver consistent and reliable performance under varying conditions. Postman provides tools and techniques to test, measure, and analyze the performance of your API endpoints effectively.
What is API Performance Benchmarking?
API performance benchmarking involves measuring key metrics such as response time, throughput, and error rates under different conditions. The goal is to set performance standards and identify areas for optimization.
Steps to Benchmark API Performance in Postman
Follow these steps to benchmark your API performance:
- Create a Collection: Group API endpoints you want to benchmark into a Postman collection.
- Add Tests for Metrics: Write test scripts to validate response time, status codes, and other performance metrics.
- Use Postman Runner: Run the collection multiple times using Postman Runner to simulate load.
- Analyze Results: Evaluate response times, success rates, and error messages from the test runs.
Sample Test Script for Performance Metrics
Here’s an example of a test script to validate performance metrics:
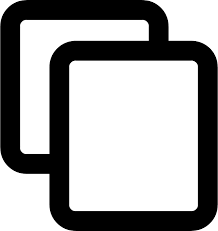
// Validate response time and status code
pm.test("Response time is within acceptable limits", function () {
pm.expect(pm.response.responseTime).to.be.below(300); // Adjust the threshold as needed
});
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
Using Newman for Load Testing
Newman, Postman’s CLI tool, allows you to run collections in a controlled environment and simulate concurrent requests. This is useful for load testing and benchmarking. Example command:
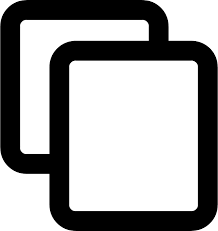
newman run MyCollection.json --iteration-count 100 --delay-request 100
Explanation: The command runs the collection 100 times with a delay of 100ms between requests.
Performance Metrics to Monitor
When benchmarking, consider the following key metrics:
- Response Time: Time taken by the server to respond to a request.
- Throughput: Number of requests handled per second.
- Error Rate: Percentage of requests that fail.
- Latency: Time taken for data to travel between the client and server.
Best Practices for API Benchmarking
- Set Baseline Metrics: Define acceptable performance benchmarks for your APIs.
- Test Under Load: Simulate concurrent users to measure how the API performs under stress.
- Monitor Trends: Regularly benchmark APIs to detect performance degradation over time.
- Optimize Bottlenecks: Use benchmarking results to identify and resolve performance bottlenecks.
Summary
Benchmarking API performance with Postman ensures that your APIs meet performance standards and provide a smooth user experience. By leveraging Postman’s tools and techniques, you can identify performance issues early and optimize your APIs effectively.
Sending GraphQL Queries and Mutations
Postman makes it easy to work with GraphQL APIs by allowing you to send both queries and mutations. With its user-friendly interface, you can structure, test, and debug your GraphQL requests effectively.
What is GraphQL?
GraphQL is a query language for APIs that allows clients to request only the data they need. Unlike REST, which has multiple endpoints, GraphQL typically exposes a single endpoint, making it flexible and efficient for modern applications.
Steps to Send GraphQL Queries and Mutations in Postman
- Set the Request Type: Open Postman and set the request type to
POST
. - Enter the Endpoint: Add your GraphQL server’s endpoint (e.g.,
https://api.example.com/graphql
). - Switch to GraphQL Mode: Under the "Body" tab, select the "GraphQL" option.
- Write Your Query or Mutation: Enter your GraphQL query or mutation in the provided editor.
- Send the Request: Click the "Send" button to execute your request and view the response.
Example: GraphQL Query
The following example demonstrates a GraphQL query to fetch a list of users:
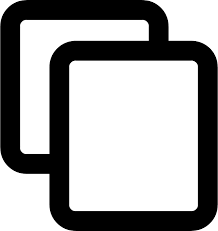
{
users {
id
name
email
}
}
Response Example:
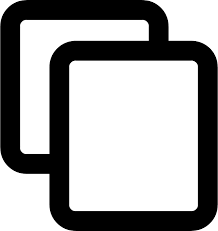
{
"data": {
"users": [
{
"id": "1",
"name": "John Doe",
"email": "john.doe@example.com"
},
{
"id": "2",
"name": "Jane Smith",
"email": "jane.smith@example.com"
}
]
}
}
Example: GraphQL Mutation
The following example demonstrates a GraphQL mutation to create a new user:
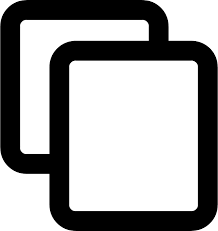
mutation {
createUser(input: {
name: "Alice Johnson",
email: "alice.johnson@example.com"
}) {
id
name
email
}
}
Response Example:
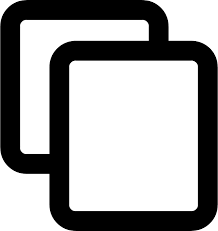
{
"data": {
"createUser": {
"id": "3",
"name": "Alice Johnson",
"email": "alice.johnson@example.com"
}
}
}
Tips for Working with GraphQL in Postman
- Use Variables: Define and reuse variables in your GraphQL requests for better maintainability.
- Enable Introspection: If your GraphQL server supports introspection, use it to explore available queries and mutations.
- Validate Responses: Use Postman tests to validate the structure and content of GraphQL responses.
- Organize Queries and Mutations: Group related GraphQL requests in Postman collections for better organization.
Summary
Postman simplifies working with GraphQL APIs, enabling you to send queries and mutations with ease. By leveraging Postman's features like variables, tests, and collections, you can efficiently develop and debug your GraphQL-based applications.
Adding Variables to GraphQL Queries
GraphQL queries can be made more dynamic and reusable by using variables. Postman supports adding variables to GraphQL queries, which simplifies the process of passing dynamic values to your API requests.
Why Use Variables in GraphQL?
Variables in GraphQL allow you to separate the query structure from the dynamic data being passed. This makes queries cleaner, reduces redundancy, and improves maintainability. With variables, you can reuse the same query with different inputs.
Steps to Add Variables to GraphQL Queries in Postman
- Write a Query with Variables: Define your GraphQL query and use placeholders for the variables. Variables are prefixed with a
$
sign. - Define Variables in JSON: Provide the values for the placeholders in the "GraphQL Variables" section in Postman, formatted as JSON.
- Send the Request: Postman will replace the placeholders with the provided values before sending the query to the server.
Example: GraphQL Query with Variables
The following example demonstrates a query with variables to fetch a user by their ID:
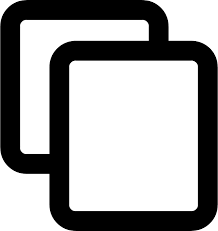
query GetUser($userId: ID!) {
user(id: $userId) {
id
name
email
}
}
Defining Variables
In the "GraphQL Variables" section of Postman, provide the variable values in JSON format:
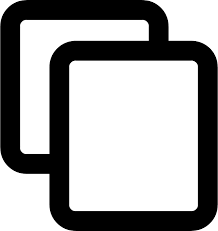
{
"userId": "123"
}
Response Example
Here’s an example of a response for the query:
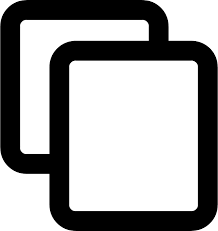
{
"data": {
"user": {
"id": "123",
"name": "Alice Johnson",
"email": "alice.johnson@example.com"
}
}
}
Example: Mutation with Variables
The following example demonstrates a mutation with variables to update a user’s email:
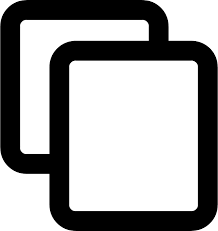
mutation UpdateUser($userId: ID!, $newEmail: String!) {
updateUser(id: $userId, email: $newEmail) {
id
name
email
}
}
Defining Mutation Variables
Provide the values for the mutation variables in JSON format:
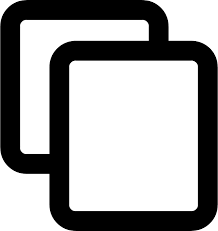
{
"userId": "123",
"newEmail": "updated.email@example.com"
}
Best Practices for Using Variables
- Use Descriptive Variable Names: Ensure variable names clearly indicate their purpose.
- Validate Variable Values: Ensure the values provided in the "GraphQL Variables" section match the expected types in the query or mutation.
- Reuse Queries: Store queries with variables in Postman collections for easy reuse.
- Test with Different Values: Use Postman environments or external files for data-driven testing with GraphQL variables.
Summary
Adding variables to GraphQL queries in Postman enhances flexibility and reusability. By separating the query structure from the dynamic data, you can streamline your API testing and development workflow.
Testing for Vulnerabilities (Injection, Authentication Flaws)
Security testing is a crucial aspect of API development and testing. Postman provides tools and features to help identify vulnerabilities such as injection attacks, authentication flaws, and other security issues in your APIs.
1. Testing for Injection Vulnerabilities
Injection vulnerabilities occur when an application processes untrusted data as part of a command or query. Common types of injection attacks include SQL injection, command injection, and script injection.
Steps to Test for Injection Vulnerabilities:
- Send Malicious Input: Modify request parameters, headers, or body to include special characters or scripts (e.g.,
' OR '1'='1
for SQL injection). - Analyze the Response: Check for unexpected behavior, such as revealing sensitive data, executing unintended commands, or application crashes.
- Use Automation: Utilize Postman scripts to automate the testing of multiple payloads against your API endpoints.
Example of SQL Injection Test:
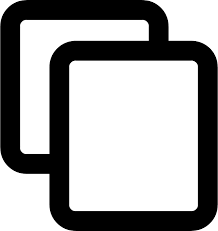
GET /api/users?id=' OR '1'='1 HTTP/1.1
Host: example.com
Authorization: Bearer
Expected Result: The API should return an error or sanitized response instead of executing the query.
2. Testing for Authentication Flaws
Authentication flaws occur when APIs fail to properly enforce authentication mechanisms, allowing unauthorized access to sensitive data or functionality.
Steps to Test for Authentication Flaws:
- Test with Invalid Tokens: Use expired, tampered, or invalid tokens to see if the API still grants access.
- Bypass Authentication: Attempt to access endpoints without authentication headers or credentials.
- Check Role-Based Access Control (RBAC): Ensure that users with different roles only access the endpoints they are authorized for.
Example of Authentication Bypass Test:
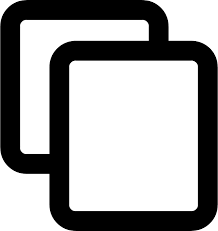
GET /api/admin/settings HTTP/1.1
Host: example.com
Authorization: Bearer
Expected Result: The API should return a 401 Unauthorized or 403 Forbidden response.
3. Tools and Techniques
- Custom Scripts in Postman: Write pre-request and test scripts to inject malicious payloads and validate responses.
- Postman Environments: Use environments to store and test multiple token scenarios (valid, invalid, expired).
- Security Testing Tools: Combine Postman with tools like OWASP ZAP or Burp Suite for comprehensive testing.
4. Best Practices for Securing APIs
- Validate Input: Implement server-side validation to sanitize and filter incoming data.
- Use Parameterized Queries: Prevent SQL injection by using parameterized queries or ORM frameworks.
- Implement Strong Authentication: Use secure methods like OAuth 2.0 and enforce token expiration policies.
- Monitor and Log: Set up monitoring and logging to detect unauthorized access attempts and suspicious behavior.
Summary
Testing for vulnerabilities such as injection and authentication flaws is essential to ensure API security. Using Postman’s features alongside dedicated security tools helps identify and mitigate these risks effectively.
Rate Limiting and Error Handling
Properly implementing rate limiting and error handling is critical to maintaining the performance and reliability of APIs. Postman allows you to test these features effectively to ensure your API behaves as expected under various conditions.
1. Understanding Rate Limiting
Rate limiting is a mechanism to control the number of API requests a client can make within a specific time frame. It helps protect APIs from overloading and ensures fair usage for all users.
Key Concepts of Rate Limiting:
- Requests Per Second (RPS): The number of requests a client can send in a second.
- Quota Limits: The total number of requests allowed within a specific period (e.g., hourly or daily).
- HTTP 429 Status Code: Indicates that the client has hit the rate limit and must wait before making more requests.
Testing Rate Limiting with Postman:
- Create a collection with multiple requests targeting the API endpoint.
- Use the Postman Runner to send requests in rapid succession or at defined intervals.
- Monitor responses for HTTP 429 errors and observe the API's behavior when the limit is exceeded.
Example of Rate Limiting Response:
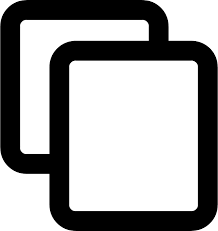
HTTP/1.1 429 Too Many Requests
Content-Type: application/json
Retry-After: 30
{
"error": "Too many requests",
"message": "You have exceeded the request limit. Try again after 30 seconds."
}
2. Error Handling in APIs
Error handling ensures that APIs return meaningful and consistent error messages when something goes wrong. Proper error handling improves the developer experience and aids in debugging.
Common HTTP Error Codes:
Status Code | Description |
---|---|
400 Bad Request | The request was malformed or invalid. |
401 Unauthorized | The client needs to authenticate to access the resource. |
403 Forbidden | The client does not have permission to access the resource. |
404 Not Found | The requested resource could not be found. |
500 Internal Server Error | The server encountered an unexpected error. |
Testing Error Handling with Postman:
- Send Invalid Inputs: Provide incorrect or incomplete parameters in the request body or query string.
- Test Authentication and Authorization: Omit or use invalid tokens to access protected endpoints.
- Simulate Server Errors: Test how the API handles server-side exceptions or unexpected conditions.
Example of Error Response:
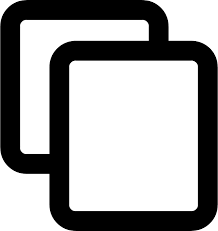
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"error": "Invalid request",
"message": "The 'email' field is required.",
"status": 400
}
3. Best Practices for Rate Limiting and Error Handling
- Implement Retry Headers: Use headers like
Retry-After
to inform clients when they can retry after hitting rate limits. - Return Consistent Errors: Ensure error responses follow a standard structure, such as using JSON for all errors.
- Provide Clear Error Messages: Include detailed but non-sensitive information in error messages to help developers debug quickly.
- Monitor API Usage: Set up monitoring to track request patterns and identify potential abuse or misconfigurations.
Summary
Rate limiting and error handling are vital for building reliable and user-friendly APIs. Postman provides the tools to test these features effectively, ensuring your API meets performance and usability standards while maintaining security and fairness.
Building a Mock API with Postman
Mock APIs are crucial for testing and prototyping without the need for a fully developed backend. Postman provides a simple and efficient way to create mock servers, allowing teams to collaborate and test API functionality early in the development process.
1. What is a Mock API?
A mock API simulates the behavior of a real API. It allows you to define endpoints and responses without requiring an actual backend implementation. This helps developers and testers work independently of backend development progress.
2. Why Use Mock APIs?
- Early Testing: Test frontend applications before the backend is ready.
- Collaboration: Share mock APIs with team members to align on API specifications.
- Prototyping: Quickly iterate on API designs and get feedback from stakeholders.
- Simulate Edge Cases: Test how your application handles specific scenarios like errors or delays.
3. Creating a Mock API in Postman
Follow these steps to create a mock API:
- Create a Collection:
- Open Postman and click on Collections.
- Click the + New Collection button.
- Give your collection a name, such as Mock API Demo, and save it.
- Add Requests to the Collection:
- Create a new request and specify the HTTP method (GET, POST, etc.) and endpoint path (e.g.,
/users
). - Define the request body, headers, or query parameters as needed.
- Save the request to your collection.
- Create a new request and specify the HTTP method (GET, POST, etc.) and endpoint path (e.g.,
- Enable Mock Server:
- Click the three-dot menu next to your collection and select Mock Collection.
- Choose whether to log requests made to the mock server.
- Click Create Mock Server and copy the mock server URL provided.
Example Mock API Response:
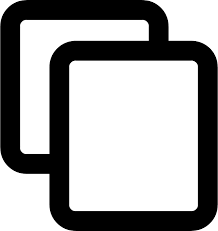
{
"status": "success",
"data": {
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
}
4. Testing Your Mock API
Once the mock server is set up, you can send requests to the mock server URL using Postman or any HTTP client. This allows you to test your frontend or third-party integrations without relying on a live backend.
Steps to Test:
- Copy the mock server URL.
- Use the URL to send requests via Postman or integrate it into your application.
- Verify the responses match the defined mock data.
5. Sharing Mock APIs
Postman makes it easy to share your mock API with others:
- Share the mock server URL with your team or clients.
- Export the collection and share it via email or version control systems like GitHub.
- Use Postman’s collaborative workspaces to share the mock API directly.
6. Best Practices for Mock APIs
- Document Responses: Include detailed examples for each endpoint in your mock API.
- Simulate Real-world Scenarios: Add different responses for success, errors, and edge cases.
- Version Your APIs: Use separate collections or mock servers to maintain multiple versions of your API.
- Monitor Usage: Keep track of who uses your mock API and how often.
Summary
Mock APIs in Postman simplify the development and testing process by decoupling frontend and backend workflows. By creating a mock server, you can prototype, test, and iterate on API designs efficiently, ensuring smoother team collaboration and faster project delivery.
Automating an E-commerce API Workflow
Automating API workflows in an e-commerce application can significantly streamline operations such as order processing, inventory management, and customer communication. Postman provides tools like collections, pre-request scripts, and tests to automate and manage these workflows efficiently.
1. What is an API Workflow?
An API workflow involves a sequence of API calls that perform related operations. In an e-commerce context, this could include:
- Authenticating the user or system.
- Fetching product details or inventory levels.
- Placing an order.
- Notifying customers about their order status.
2. Setting Up the Workflow in Postman
Follow these steps to set up an automated e-commerce API workflow:
- Create a Collection:
- Click + New Collection in Postman.
- Name the collection E-commerce API Workflow and save it.
- Add Requests to the Collection:
- Include requests for login, fetching products, placing orders, and sending notifications.
- Save each request with descriptive names like Login, Get Products, Place Order, etc.
- Set Up Environment Variables:
- Create variables for API base URL, authentication tokens, product IDs, etc.
- Use these variables in your requests for flexibility and reusability.
- Use Pre-request Scripts:
- Write scripts to generate dynamic data, such as timestamps or session tokens.
- Example: Automatically refresh an authentication token before making requests.
- Write Tests:
- Validate responses for each request to ensure the workflow is functioning correctly.
- Example: Check if the order ID is returned after placing an order.
3. Example Workflow
Below is a typical e-commerce API workflow:
- Step 1: Login
Authenticate using a POST request to the login endpoint and store the access token.
{ "username": "user@example.com", "password": "securepassword" }
- Step 2: Fetch Products
Use a GET request to retrieve product details from the catalog.
{ "products": [ { "id": 101, "name": "Laptop", "price": 1500 }, { "id": 102, "name": "Smartphone", "price": 800 } ] }
- Step 3: Place an Order
Send a POST request to the order endpoint with the product ID and quantity.
{ "productId": 101, "quantity": 1, "orderStatus": "success", "orderId": "ORD12345" }
- Step 4: Notify the Customer
Trigger a notification by sending a POST request to the notification endpoint.
4. Running the Workflow
Once all the requests are set up, you can run the workflow using Postman Runner:
- Select the E-commerce API Workflow collection.
- Configure the environment and data file, if applicable.
- Click Run to execute the workflow.
5. Best Practices
- Use Variables: Define reusable variables for dynamic data like order IDs and tokens.
- Validate Responses: Include tests to ensure each step of the workflow executes correctly.
- Handle Errors: Add error-handling logic in pre-request scripts or tests.
- Document the Workflow: Provide clear documentation for each request and its purpose.
Summary
Automating e-commerce API workflows in Postman helps streamline operations, reduces manual effort, and ensures consistent performance. By leveraging Postman’s powerful tools like collections, scripts, and the Runner, you can efficiently design, test, and optimize your API workflows.
Creating a Postman Collection for User Authentication
Postman makes it simple to design, test, and manage API requests for user authentication workflows. By creating a dedicated collection, you can organize authentication-related requests, streamline testing, and ensure consistency across your API calls.
1. Why Use a Collection for User Authentication?
Grouping authentication-related API calls into a Postman collection offers several benefits:
- Centralized management of login, registration, and token refresh workflows.
- Reusable variables for dynamic data such as access tokens and user credentials.
- Facilitates testing of authentication flows for multiple environments (e.g., development, staging, production).
2. Steps to Create a Collection for User Authentication
Follow these steps to create and configure a Postman collection for user authentication:
- Create a New Collection:
- Open Postman and click + New Collection.
- Name the collection User Authentication and save it.
- Add Requests:
- Include essential requests such as:
- Register: API call for user signup.
- Login: API call to authenticate a user and retrieve a token.
- Token Refresh: Request to refresh expired access tokens.
- Logout: Endpoint to revoke tokens or end sessions.
- Save each request with descriptive names for easy identification.
- Include essential requests such as:
- Set Up Environment Variables:
- Define variables for dynamic data such as
baseUrl
,username
,password
, andaccessToken
. - Example:
{ "baseUrl": "https://api.example.com", "username": "testuser@example.com", "password": "securepassword", "accessToken": "" }
- Define variables for dynamic data such as
- Write Pre-request Scripts:
- Use pre-request scripts to dynamically update headers or tokens before sending requests.
- Example: Add a token to the Authorization header:
pm.request.headers.add({ key: "Authorization", value: `Bearer ${pm.environment.get("accessToken")}` });
- Add Tests for Validation:
- Write tests to validate responses and store dynamic data.
- Example: Extract and save a token from the login response:
const response = pm.response.json(); if (response.accessToken) { pm.environment.set("accessToken", response.accessToken); }
3. Example Requests
Here’s an example of commonly used authentication requests:
- Register: POST request to create a new user account.
{ "username": "newuser@example.com", "password": "securepassword", "email": "newuser@example.com" }
- Login: POST request to authenticate a user.
{ "username": "testuser@example.com", "password": "securepassword" }
- Token Refresh: POST request to get a new access token.
{ "refreshToken": "abcd1234refreshToken" }
4. Running and Testing the Collection
- Use Postman Runner to execute the authentication flow sequentially.
- Verify that each request executes successfully and that tokens or other data are stored correctly.
- Test error scenarios such as invalid credentials or expired tokens.
5. Best Practices
- Secure Sensitive Data: Avoid hardcoding sensitive information like passwords or tokens.
- Use Environment Variables: Parameterize requests to make them reusable across environments.
- Validate Responses: Add assertions to ensure API responses are as expected.
- Organize Requests: Group related requests into folders for better structure.
Summary
Creating a Postman collection for user authentication helps you efficiently manage and test the authentication process. By organizing requests, leveraging variables, and automating workflows, you can streamline your API development and ensure secure, reliable authentication flows.