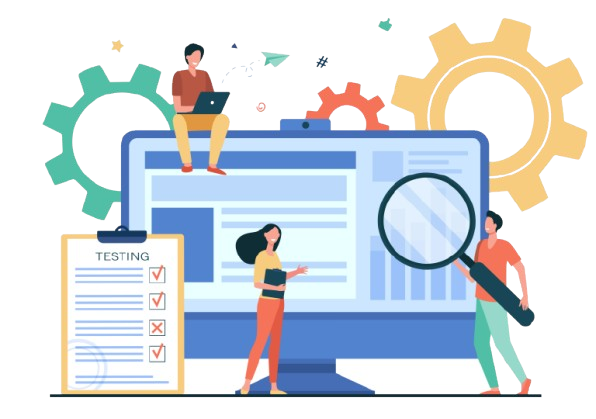
What is Software Testing?
Software testing is the process of evaluating and verifying that a software application or system works as intended. It involves executing a program to identify bugs or defects in the software and ensure that it meets the required standards and business objectives. Testing helps to maintain the quality of software and ensures that it functions correctly under various conditions.
Objectives of Software Testing
The main objectives of software testing include:
- Identifying software defects before they reach production.
- Ensuring that the software meets user requirements and expectations.
- Verifying the performance, security, and reliability of the software.
- Ensuring that the software is robust and can handle unexpected conditions.
Importance of Software Testing
Software testing is crucial for the following reasons:
- Quality Assurance: It helps ensure that the software is free from defects and functions as intended, leading to higher user satisfaction.
- Cost-effectiveness: Identifying and fixing issues early in the development cycle saves time and resources in the long run.
- Security: Testing helps identify vulnerabilities in the software, reducing the risk of potential cyber-attacks or data breaches.
- Compliance: It ensures that the software meets industry standards, legal, and regulatory requirements.
Types of Software Testing
Software testing can be broadly classified into two categories:
- Manual Testing: Testing is performed by human testers who execute the test cases without using any automation tools.
- Automated Testing: Testing is performed using software tools and scripts to automate the execution of test cases.
Testing Life Cycle
The software testing life cycle consists of several stages:
- Requirement Analysis: Understanding the software requirements and planning the testing process accordingly.
- Test Planning: Creating a test plan that outlines the scope, objectives, resources, and schedule for testing.
- Test Design: Designing detailed test cases based on the requirements and specifications.
- Test Execution: Executing the test cases and documenting the results.
- Defect Reporting: Reporting any issues or defects found during testing to the development team.
- Test Closure: Closing the testing phase and preparing test reports and documentation.
Code Example: Simple Test Case
Here’s an example of a simple test case for verifying that a login form on a website works as expected:
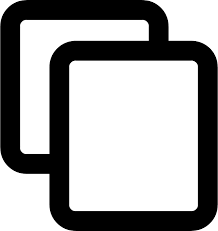
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: Software Testing Process
The following diagram outlines the typical process flow in software testing:

This diagram illustrates the stages from requirement analysis to test closure and how each step contributes to ensuring the software’s quality.
Why is Software Testing Important?
Software testing is a critical phase in the software development lifecycle as it helps ensure the quality, functionality, and reliability of a software product. Without proper testing, software may be released with bugs, performance issues, or security vulnerabilities that can negatively impact users and businesses.
Key Reasons for the Importance of Software Testing
Here are the main reasons why software testing is crucial:
- Ensures Product Quality: Software testing ensures that the product meets the specified requirements, functions properly, and satisfies user expectations.
- Identifies Bugs and Defects Early: Testing helps identify and fix bugs at an early stage, reducing the cost and time required for fixing issues later in the development cycle.
- Improves User Experience: By identifying usability issues and ensuring that the software works as expected, testing contributes to a better user experience and customer satisfaction.
- Reduces Risks: Through testing, potential risks related to security vulnerabilities, performance issues, or system crashes can be identified and mitigated before the software reaches the end users.
- Ensures Security: Security testing ensures that the software is protected against threats such as data breaches, hacking, or unauthorized access, safeguarding user data and business operations.
- Compliance and Standards: Testing ensures that the software meets industry standards, legal, and regulatory compliance requirements, which is particularly important for domains like healthcare, finance, and government.
- Cost Savings: Detecting defects early through testing can prevent costly fixes after deployment, saving businesses money in the long run.
Impact of Software Testing on Business
Software testing directly impacts a business by ensuring that the final product is reliable, secure, and free from defects. Here are some of the key ways software testing can benefit a business:
- Enhanced Customer Trust: A bug-free and high-quality product increases customer confidence, leading to better reviews, recommendations, and customer loyalty.
- Competitive Advantage: Software that functions smoothly and meets customer needs provides a competitive edge in the market, helping businesses stand out from competitors.
- Increased Revenue: A high-quality product leads to higher customer satisfaction, which translates into better sales, more customers, and increased revenue.
- Reduced Downtime: By identifying and addressing issues before release, testing helps to minimize system downtime, ensuring continuous business operations.
Types of Testing Contributing to Software Quality
Several types of testing contribute to software quality:
- Unit Testing: Ensures individual components of the software work as intended.
- Integration Testing: Verifies that different components or modules of the software work together as expected.
- System Testing: Tests the complete system for functionality, performance, and security.
- Acceptance Testing: Ensures the software meets business requirements and is ready for deployment.
Code Example: Simple Test Case for User Login
Here’s an example of a simple test case for ensuring that the login functionality works correctly:
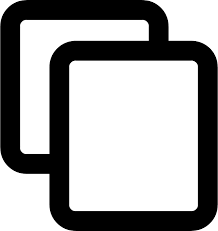
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: Impact of Testing on Software Quality
The diagram below illustrates the relationship between testing and the overall quality of the software product:

This diagram shows how various testing activities contribute to the software's functionality, performance, security, and user satisfaction.
Software Development Life Cycle (SDLC) vs. Software Testing Life Cycle (STLC)
The Software Development Life Cycle (SDLC) and Software Testing Life Cycle (STLC) are both crucial processes in the software development and testing industry. While SDLC focuses on the overall development of software, STLC focuses specifically on the testing phase to ensure quality and functionality. Both are integral to delivering high-quality software, but they serve different purposes and have distinct phases.
Software Development Life Cycle (SDLC)
SDLC is a structured process used by software development teams to design, develop, test, and deploy software applications. It provides a systematic approach to software development, ensuring that the final product meets the specified requirements and is delivered on time and within budget.
Phases of SDLC
- Requirement Gathering: The first step involves gathering the client’s requirements and understanding the needs for the software to be developed.
- System Design: Based on the requirements, system architecture and design are created, including technical specifications and a detailed plan for development.
- Implementation (Coding): The actual software is built by developers according to the design specifications.
- Testing: Once the development is complete, the software undergoes testing to identify and fix any bugs or issues.
- Deployment: After successful testing, the software is deployed to a production environment for end users.
- Maintenance: Post-deployment, the software is maintained by fixing any issues, releasing updates, and making improvements as needed.
Software Testing Life Cycle (STLC)
STLC is a part of the SDLC that focuses specifically on the testing phase. It involves a series of steps to ensure that the software is thoroughly tested and validated before deployment. The goal of STLC is to identify bugs and ensure the software meets the required quality standards.
Phases of STLC
- Requirement Analysis: Testers review the requirements to understand the testing needs and create a test plan.
- Test Planning: Test strategies, objectives, and resources are planned. Test cases and test data are created based on the requirements.
- Test Design: Detailed test cases and test scripts are designed, ensuring all functionalities are covered during testing.
- Test Execution: The actual execution of test cases is performed, and the results are documented.
- Defect Reporting: Any defects or issues identified during testing are reported, tracked, and managed.
- Test Closure: Once testing is complete, the results are evaluated, and the test artifacts are archived. A final report is generated to summarize the testing process.
Comparison Between SDLC and STLC
Though both SDLC and STLC play important roles in software development, they differ in scope and focus. Here is a comparison between the two:
Aspect | SDLC | STLC |
---|---|---|
Focus | Overall software development process, from requirement gathering to deployment and maintenance. | Focused on the testing phase, ensuring that software is thoroughly tested and validated. |
Phases | Requirement gathering, system design, implementation, testing, deployment, maintenance. | Requirement analysis, test planning, test design, test execution, defect reporting, test closure. |
Involvement | Involves developers, project managers, and stakeholders. | Involves testers, quality assurance teams, and test managers. |
Duration | Longer process, spanning the entire software development lifecycle. | Shorter process, focused on the testing phase within the SDLC. |
Code Example: Test Case Creation
Here’s an example of a simple test case to verify user login functionality in the STLC process:
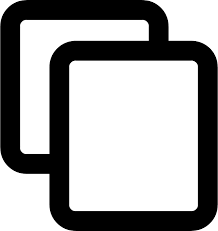
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: SDLC vs STLC
The following diagram compares the SDLC and STLC processes, highlighting their distinct phases and how they align:

This diagram provides a clear visual of the SDLC and STLC lifecycle phases, helping to understand their roles in the software development process.
Objectives of Software Testing
Software testing is a crucial phase in the software development process. It aims to ensure that the software is free of defects, functional, and meets the specified requirements. The primary objectives of software testing are to verify the software's functionality, performance, security, and usability. Let’s explore these objectives in more detail:
1. Verifying Functionality
The main objective of software testing is to verify that the software performs as expected and meets the specified requirements. It ensures that all features work correctly and that the system behaves as intended under various conditions.
- Confirm that all functionalities are implemented correctly.
- Test the software against functional requirements to ensure it meets user needs.
2. Identifying and Fixing Defects
Another key objective is to identify defects or bugs in the software early in the development process. Testing helps to detect issues that may affect the software’s performance, user experience, or security. By identifying and fixing defects early, the cost of fixing them is reduced.
- Detect and report defects that impact software behavior.
- Fix defects to ensure the software meets quality standards.
3. Ensuring Software Quality
Testing aims to ensure that the software meets quality standards and satisfies both functional and non-functional requirements. It helps to ensure that the software is reliable, efficient, and performs well under various conditions.
- Ensure that the software meets user expectations and quality criteria.
- Evaluate the software’s reliability and stability over time.
4. Validating Performance
Performance testing is another important objective of software testing. It ensures that the software performs well under heavy loads, stress, and high traffic. This includes testing the system for responsiveness, stability, and scalability to handle high volumes of users and data.
- Check how the software performs under varying load conditions.
- Ensure the system can handle peak loads and stress situations without breaking down.
5. Ensuring Security
Security testing is crucial to ensure that the software is secure from external threats and vulnerabilities. This includes checking for potential security risks such as data breaches, unauthorized access, and other security issues.
- Check for vulnerabilities that could lead to security breaches.
- Ensure the software complies with security standards and best practices.
6. Verifying Compatibility
Compatibility testing ensures that the software works correctly across different operating systems, browsers, devices, and configurations. This is critical for ensuring a seamless user experience for a wide range of users.
- Test the software across different operating systems, browsers, and devices.
- Ensure compatibility with various hardware and software configurations.
7. Improving User Experience
One of the objectives of testing is to ensure that the software provides a positive user experience. This includes testing for ease of use, navigation, and overall usability of the application. User feedback and usability testing are essential for identifying areas of improvement.
- Ensure the software is user-friendly and easy to navigate.
- Test the software to meet users’ expectations and improve the overall user experience.
8. Reducing Risk
By identifying potential issues early in the software development process, testing helps to reduce the risk of failure after deployment. Risk-based testing focuses on identifying critical areas of the application that could cause significant issues if not addressed, ensuring that high-risk areas are thoroughly tested.
- Identify and mitigate potential risks that may impact the success of the software.
- Prioritize testing of high-risk areas to reduce the chances of failure.
Code Example: Test Case for User Login
Below is an example of a simple test case that verifies the login functionality of a web application:
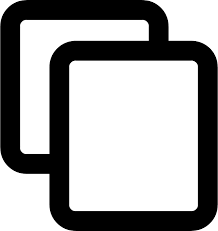
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: Software Testing Objectives
The diagram below illustrates the key objectives of software testing and how they contribute to the overall quality of the software:

This diagram helps visualize how different testing objectives align with the goal of delivering high-quality software.
Software Testing Principles
Software testing is governed by certain principles that guide testers in ensuring the quality and functionality of the software. These principles are widely accepted in the software testing community and are essential for effective testing. Below are the key principles of software testing:
1. Testing Shows the Presence of Defects
The primary goal of testing is to identify defects or bugs in the software. Testing can only prove that defects are present, not that the software is completely free of defects. Even after extensive testing, some defects may remain undiscovered.
- Testing can only confirm the existence of defects, not their absence.
- It is important to understand that no software is entirely bug-free, but rigorous testing helps minimize defects.
2. Exhaustive Testing is Impossible
It is impossible to test all possible inputs and configurations of the software. Given the vast number of possible combinations, exhaustive testing (testing every scenario) is often impractical and time-consuming. Instead, risk-based and focused testing should be done to cover the most critical areas.
- Testing every possible scenario is not feasible, so we test the most critical and high-risk areas.
- Prioritizing tests based on risk is more effective than exhaustive testing.
3. Early Testing
Testing should be performed as early as possible in the software development lifecycle. Early testing helps identify defects while the cost of fixing them is low. It is essential to adopt a shift-left testing approach, where testing starts as soon as the requirements are available.
- Begin testing early to detect defects early in the development process.
- Shift-left testing helps reduce the cost and complexity of finding defects later in the project.
4. Defect Clustering
Most defects tend to be concentrated in a few areas of the software. This is known as the 80/20 rule or Pareto principle, where 80% of the defects are found in 20% of the code. Identifying these "hot spots" can help focus testing efforts on the most defect-prone parts of the software.
- Focus testing efforts on areas with a high concentration of defects.
- Use defect clustering to prioritize testing efforts and improve efficiency.
5. Testing is Context Dependent
The testing process should be tailored to the context of the software and the project. The approach for testing a web application will differ from that of testing a mobile app or a desktop application. The testing environment and objectives must be considered to choose the appropriate testing techniques.
- Adapt the testing approach based on the specific context of the software being tested.
- Different types of applications (web, mobile, etc.) require different testing strategies.
6. Absence of Errors Fallacy
Testing cannot guarantee that the software is error-free, but it can ensure that the software meets the required quality standards. It is important to understand that having no defects in software does not mean that the software meets the business needs or user expectations. Even if the software is free from bugs, it may still fail to meet the user's goals.
- Just because there are no defects, it doesn't mean the software is perfect or meets user expectations.
- Focus on delivering software that solves the user's problem, not just defect-free software.
7. Early Defect Detection Saves Time and Costs
Identifying defects early in the development process can significantly reduce the cost and time required to fix them. The earlier defects are found, the easier and cheaper they are to fix. This principle supports the idea of shifting left in the software development lifecycle.
- Find and fix defects early to reduce the cost of fixing them later.
- Early defect detection helps improve the overall software quality and project timeline.
8. Continuous Improvement
Testing processes should be continuously improved based on feedback and lessons learned from previous projects. This includes refining test cases, improving automation, and keeping up with new testing tools and methodologies. Regularly reviewing and improving testing practices helps ensure more efficient and effective testing in future projects.
- Continuously improve testing processes based on insights from past testing experiences.
- Stay updated with new tools, technologies, and testing methodologies for better efficiency.
Code Example: Testing a Login Function with Assertions
Here's an example of a simple test case for testing the login functionality of a web application:
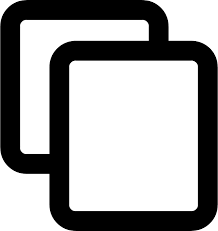
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: Software Testing Principles
The diagram below illustrates the key principles of software testing and how they guide the testing process:

This diagram helps visualize the core principles and how they impact the overall software testing strategy.
The Role of a Tester in a Project
Testers play a critical role in the software development lifecycle (SDLC) by ensuring that the software meets the required quality standards. They focus on identifying defects, validating requirements, and ensuring the software meets user expectations. Testers collaborate with various teams to ensure a product is free from critical issues and works as intended. Below are the key responsibilities and the importance of a tester in a project:
1. Understanding Project Requirements
Before starting the testing process, the tester needs to thoroughly understand the project requirements. This includes functional and non-functional requirements, business objectives, and user expectations. Understanding the requirements helps testers design effective test cases and ensures that the software meets the desired goals.
- Collaborate with stakeholders to gather and understand requirements.
- Identify key features that need to be tested based on requirements.
2. Test Planning
Test planning is one of the crucial roles of a tester. The tester creates a comprehensive test plan that outlines the scope, objectives, resources, schedule, and deliverables for testing. This plan serves as a roadmap for the testing phase and helps the team stay organized and focused throughout the process.
- Create a test plan that outlines testing scope, objectives, and deliverables.
- Determine test environments and resources needed for testing.
3. Designing Test Cases
Once the requirements are clear, testers design test cases that validate the functionality of the software. Test cases should cover various scenarios, including positive and negative test cases, edge cases, and boundary conditions. Clear and detailed test cases ensure that testing is thorough and effective.
- Design test cases based on the requirements and use cases.
- Include positive, negative, and edge cases in the test cases.
4. Test Execution
Testers execute the test cases to identify defects in the software. During execution, they track defects, report them to the development team, and ensure that any issues are addressed. Test execution is a continuous process where the tester checks if the software behaves as expected under different conditions.
- Execute the test cases and document the results.
- Track defects and issues identified during testing.
5. Defect Reporting and Communication
Testers are responsible for reporting defects and bugs discovered during testing. They document the defect’s nature, severity, and steps to reproduce it. Effective communication with developers and other team members is crucial for ensuring the defects are fixed in a timely manner.
- Report defects with detailed information, including severity and steps to reproduce.
- Communicate defects clearly to the development team for prompt resolution.
6. Re-Testing and Regression Testing
Once defects are fixed, testers perform re-testing to verify that the issues have been addressed. They also conduct regression testing to ensure that new changes do not negatively impact existing functionality. This step is crucial for maintaining the stability of the software throughout the development process.
- Verify that defects are fixed by re-testing the related functionality.
- Ensure that new changes do not break existing features through regression testing.
7. Test Automation
In modern software development, testers are often involved in automating repetitive and time-consuming test cases. Automation helps speed up the testing process, especially for regression testing, and ensures consistency in test execution. Testers may use tools like Selenium, Cypress, or Appium for automation.
- Automate repetitive test cases to improve efficiency and speed.
- Use automation tools to ensure consistent and reliable testing.
8. Collaboration with Development and QA Teams
Testers work closely with developers and other quality assurance (QA) team members to ensure that the product meets the required quality standards. They participate in code reviews, sprint meetings, and discussions to ensure the product meets both functional and non-functional requirements.
- Collaborate with developers during code reviews and sprint meetings.
- Participate in discussions to ensure alignment between development and testing activities.
9. Test Reporting and Documentation
Testers document the results of their testing activities and generate test reports that provide insights into the quality of the software. These reports often include the number of passed, failed, and blocked test cases, as well as a summary of defects and testing progress.
- Generate test reports that summarize testing activities and results.
- Document the overall quality of the software and any identified issues.
Code Example: Simple Test Case
Here's an example of a simple test case for testing the login functionality of a web application:
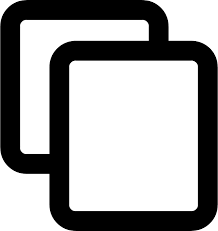
# Test case to verify login functionality
def test_login():
# Enter valid username and password
username = "testuser"
password = "password123"
# Submit the login form
result = login(username, password)
# Assert that the login is successful
assert result == "Login successful"
Diagram: Role of Tester in a Project
The following diagram illustrates the role of a tester in the software development lifecycle:

This diagram helps visualize how testers interact with different project teams and contribute to the overall quality of the software.
Overview of Manual Testing
Manual testing is the process of manually checking software for defects. It involves a tester executing test cases without the use of automation tools to ensure that the software behaves as expected. Manual testing is essential for identifying bugs and verifying the functionality of software from an end-user perspective. It helps ensure that the software meets the required quality standards before it is released to the public.
1. What is Manual Testing?
Manual testing involves human testers performing tests on software applications to detect defects, ensure that features work as intended, and validate the software’s overall functionality. Unlike automated testing, manual testing requires testers to manually perform test steps and evaluate results.
- Test cases are executed manually by the tester.
- Testers verify the functionality, usability, and performance of the software.
2. Types of Manual Testing
Manual testing can be classified into different types based on the testing objectives and areas being tested. Some common types include:
- Functional Testing: Verifying that the software performs the functions it is designed to do.
- Integration Testing: Ensuring that different modules or components of the software work together correctly.
- System Testing: Testing the complete system as a whole to ensure it meets the requirements.
- Acceptance Testing: Verifying the software against user requirements to ensure it is ready for deployment.
- Usability Testing: Assessing the user interface (UI) and overall user experience (UX) of the software.
- Exploratory Testing: Testers explore the application to find defects based on their experience and intuition.
3. Manual Testing Process
The process of manual testing generally involves the following steps:
- Requirement Analysis: Testers review the project requirements to understand what needs to be tested.
- Test Planning: A test plan is created that defines the scope, objectives, and resources needed for testing.
- Test Case Design: Test cases are created based on the requirements and use cases.
- Test Execution: Testers manually execute the test cases and document the results.
- Defect Reporting: Any defects found during testing are reported and communicated to the development team for resolution.
- Test Closure: Once testing is complete, test results are documented, and the testing process is concluded.
4. Advantages of Manual Testing
Manual testing has several advantages, especially in the early phases of development or when dealing with complex scenarios that require human judgment. Some key benefits include:
- Flexibility: Testers can adapt to changing requirements and make real-time decisions.
- Human Intuition: Manual testing allows testers to identify issues that automated tests might miss, especially in terms of user experience and usability.
- Better for Small Projects: For smaller applications or projects with limited resources, manual testing can be more effective and cost-efficient.
- No Need for Technical Skills: Manual testing does not require advanced programming or automation skills, making it accessible to many testers.
5. Challenges of Manual Testing
While manual testing is essential, it also comes with its own set of challenges:
- Time-Consuming: Manual testing can be time-intensive, especially for large applications with many test cases.
- Repetitive: Running the same tests repeatedly across different versions of the software can be monotonous and prone to human error.
- Limited Coverage: Manual testing might not cover all possible scenarios, especially when there is a vast number of combinations to test.
- Expensive: Since it requires human resources for every test run, manual testing can be more costly compared to automated testing in the long run.
6. When to Use Manual Testing
Manual testing is typically used in the following scenarios:
- When test cases are not repetitive and require human judgment (e.g., usability testing).
- In the early stages of development when automation may not be feasible or practical.
- When testing new features that have not yet been automated.
- When performing exploratory or ad-hoc testing to uncover unexpected issues.
7. Best Practices for Manual Testing
To ensure the effectiveness of manual testing, testers should follow best practices:
- Define clear and detailed test cases with expected results.
- Keep track of testing progress and document any defects found.
- Perform regression testing to ensure that new changes don’t introduce issues.
- Involve both technical and non-technical team members for diverse perspectives.
- Focus on high-risk areas first and ensure critical functionalities are thoroughly tested.
Code Example: Manual Test Case
Here’s an example of a manual test case for testing the login functionality of a web application:
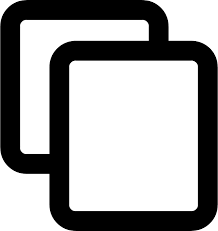
# Test Case: Login Functionality
Test ID: TC_Login_01
Test Description: Verify that users can successfully log in with valid credentials.
Preconditions:
- User is on the login page.
Test Steps:
1. Enter valid username in the "Username" field.
2. Enter valid password in the "Password" field.
3. Click the "Login" button.
Expected Result:
- User should be redirected to the dashboard page with a successful login message.
Actual Result:
- [Test result: Pass/Fail]
Diagram: Manual Testing Process
The following diagram illustrates the manual testing process from start to finish:

This diagram shows the flow of manual testing, from requirement analysis to test closure.
Exploratory Testing
Exploratory testing is an approach where testers actively explore the application, learn about its functionality, and simultaneously design and execute tests. Unlike scripted testing, which follows predefined test cases, exploratory testing emphasizes the tester’s creativity, intuition, and experience to identify defects that might be missed by automated or traditional testing methods.
1. What is Exploratory Testing?
Exploratory testing is an unscripted testing technique that relies on the tester’s knowledge, experience, and creativity to explore the software application. Testers investigate the application based on their understanding of the software’s purpose and behavior, attempting to identify unexpected issues or defects in real-time.
- The tester actively interacts with the application while simultaneously designing test cases.
- There is minimal documentation or predefined test steps involved.
- Testers rely heavily on their intuition, creativity, and experience to find issues.
2. Key Characteristics of Exploratory Testing
Exploratory testing has several key characteristics that distinguish it from other testing approaches:
- Simultaneous Learning, Test Design, and Execution: Testers learn about the system, design test cases, and execute them all in parallel.
- Minimal Documentation: There is often little to no documentation involved, and the test steps are created on the fly.
- Creativity and Intuition: Testers leverage their creativity and intuition to explore the application beyond predefined scripts.
- Adaptability: Testers can adapt their testing approach based on the findings and results during the exploration.
3. When to Use Exploratory Testing
Exploratory testing is particularly useful in the following scenarios:
- When Requirements Are Unclear: Exploratory testing can help find defects when the application’s requirements are incomplete or ambiguous.
- In Short Testing Cycles: When there is limited time for testing, exploratory testing allows testers to quickly discover issues without relying on detailed test scripts.
- To Discover Unforeseen Issues: Exploratory testing helps uncover hidden issues or defects that may not be covered by traditional test cases.
- During Early Stages of Development: It is useful during the early stages of development when features are still evolving and may not have formal test cases.
4. Advantages of Exploratory Testing
Exploratory testing offers several advantages, making it a valuable approach in software testing:
- Faster Testing: Testers can start testing immediately without waiting for detailed test cases to be written.
- More Realistic Testing: The tester’s experience allows for more realistic test scenarios that can better mimic real-world usage.
- Flexibility: Testers can shift focus to different areas of the application based on their findings, enabling a more adaptive approach.
- Helps Identify Hidden Defects: Exploratory testing is highly effective at finding defects that might be missed by scripted tests or automated testing.
5. Challenges of Exploratory Testing
Although exploratory testing has several benefits, it also comes with its challenges:
- Lack of Coverage: Since tests are unscripted, there is a risk of missing critical areas of the software.
- Inconsistent Results: Due to the tester’s subjective nature, exploratory tests may yield inconsistent results across different testers.
- Hard to Track Progress: Since there is no predefined test plan, it can be difficult to track the exact scope and progress of testing.
- Requires Skilled Testers: Exploratory testing depends on the tester’s skills and experience, making it less effective when less experienced testers are involved.
6. Best Practices for Exploratory Testing
To maximize the effectiveness of exploratory testing, the following best practices can be followed:
- Time-Boxing: Set a specific amount of time for each exploratory testing session to avoid aimless exploration and focus the tester’s efforts.
- Session-Based Test Management: Organize exploratory testing into structured sessions where testers focus on specific features or areas of the application.
- Keep Notes: Testers should document their observations, actions, and defects encountered during testing to ensure findings are captured for later analysis.
- Collaborate with Developers: Collaboration between testers and developers can ensure that testers understand the application’s objectives and areas to focus on.
- Follow a Charter: While the test may be unscripted, providing testers with a specific goal or objective (called a "charter") can help guide the testing session.
7. Code Example: Exploratory Testing Session
Here’s an example of an exploratory testing session for testing the login feature:
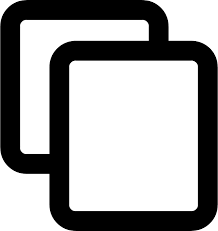
# Exploratory Testing Session: Login Functionality
Session Goal: Verify that the login functionality works across different browsers and with valid/invalid credentials.
Test Steps:
1. Open the application in Chrome, Firefox, and Edge.
2. Try logging in with valid credentials.
3. Try logging in with invalid credentials (incorrect username/password).
4. Test the "Forgot Password" feature.
5. Check if login is successful after multiple failed attempts (lockout mechanism).
6. Test the login with special characters or very long usernames and passwords.
Observations:
- [Tester notes and findings]
8. Diagram: Exploratory Testing Process
The following diagram illustrates the process of conducting exploratory testing:

This diagram shows how testers learn about the application, create test scenarios, and run tests simultaneously during an exploratory testing session.
Ad Hoc Testing
Ad hoc testing is a type of informal and unplanned testing where testers try to find defects without following any predefined test cases or test plans. It is a random and spontaneous approach, relying heavily on the tester's intuition and experience to explore the application and identify issues that may not have been covered in formal testing efforts.
1. What is Ad Hoc Testing?
Ad hoc testing is an informal testing technique in which the tester does not follow a structured test plan or documentation. Testers use their knowledge, experience, and creativity to explore the application in an attempt to uncover defects. It is often performed at the end of the testing cycle or when there is a need to explore certain parts of the software quickly.
- There is no formal test plan or design documentation.
- Testers use their intuition, knowledge, and experience to identify issues.
- It is often conducted when there is limited time or resources for structured testing.
- Usually performed after other testing activities like functional or regression testing.
2. Key Characteristics of Ad Hoc Testing
Ad hoc testing has several key characteristics that differentiate it from other testing methodologies:
- Unstructured: There are no predefined test cases or formal test plans. Testers explore the application freely without strict guidelines.
- Spontaneous: Ad hoc testing is often performed on the spur of the moment, based on the tester’s instinct or experience with the application.
- Exploratory: Testers use their knowledge of the application and the domain to guide their testing, focusing on areas they think may have defects.
- Minimal Documentation: Testers do not document detailed steps or results unless a defect is found.
3. When to Use Ad Hoc Testing
Ad hoc testing is useful in the following scenarios:
- When Time or Resources Are Limited: If there is insufficient time for extensive test planning or execution, ad hoc testing can quickly identify defects.
- After Formal Testing: It can be performed after structured testing (e.g., functional testing, regression testing) to find additional issues that may have been missed.
- To Focus on Specific Areas: If there is a specific feature or area of the application that needs extra attention, ad hoc testing can help uncover issues in that area.
- When There Is a Lack of Documentation: If the application lacks detailed specifications or requirements, ad hoc testing can be performed based on the tester's knowledge of the product.
4. Advantages of Ad Hoc Testing
Ad hoc testing has several benefits, making it a valuable testing approach:
- Quick and Flexible: Ad hoc testing can be performed quickly without the need for complex test plans or case designs, allowing testers to focus on immediate issues.
- Uncover Unexpected Defects: By not following predefined scripts, ad hoc testing can help identify defects that might not be covered by structured tests.
- Less Time-Consuming: Since there is no need for extensive test documentation or case preparation, ad hoc testing is less time-consuming.
- Encourages Creativity: Testers can be more creative and explore the application from different perspectives, increasing the chances of finding hidden defects.
5. Challenges of Ad Hoc Testing
While ad hoc testing has its advantages, it also presents some challenges:
- Lack of Test Coverage: Since there are no formal test plans, there is a risk of missing critical areas of the application, leading to incomplete testing.
- Inconsistent Results: The unstructured nature of ad hoc testing can lead to inconsistent results, as different testers might explore the application in different ways.
- Hard to Track Progress: Without a formal test plan, it can be difficult to track the progress of the testing and measure test coverage effectively.
- Relies on Tester’s Experience: The success of ad hoc testing depends heavily on the tester's knowledge and experience, which may vary between individuals.
6. Best Practices for Ad Hoc Testing
To make ad hoc testing more effective, the following best practices can be followed:
- Focus on Critical Areas: Focus on the most critical or complex areas of the application, as these are more likely to have defects.
- Document Defects: When defects are found, document them thoroughly with detailed steps, screenshots, and logs for further investigation.
- Work with Developers: Collaboration with developers can help identify areas of the application that may require additional testing or attention.
- Time-Boxing: Set a time limit for ad hoc testing sessions to avoid aimless testing and ensure focused exploration.
- Test Different Scenarios: Try different configurations, inputs, and conditions to explore how the application behaves under various circumstances.
7. Example: Ad Hoc Testing Session
Here’s an example of an ad hoc testing session for testing the login feature:
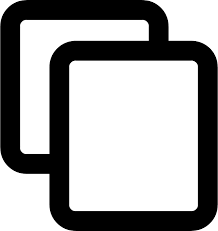
# Ad Hoc Testing Session: Login Functionality
Session Goal: Verify login functionality under different scenarios.
Test Steps:
1. Try logging in with valid credentials.
2. Try logging in with invalid credentials (incorrect username/password).
3. Test login with a special character in the password field.
4. Try logging in with a very long username or password.
5. Test the "Forgot Password" functionality.
6. Test login after multiple failed attempts (check for account lockout).
Observations:
- [Tester notes and findings]
8. Diagram: Ad Hoc Testing Process
The following diagram illustrates the ad hoc testing process:

This diagram shows how testers explore the application freely without predefined test cases, focusing on critical areas and uncovering defects through unstructured testing.
Boundary Value Testing
Boundary Value Testing (BVT) is a type of black-box testing technique where test cases are designed to check the boundary conditions of input values. The idea is to focus on the values at the edges of the input range, as errors are most likely to occur at these boundary points. This technique helps identify defects that may arise when the system handles values at the minimum, maximum, or just beyond valid ranges.
1. What is Boundary Value Testing?
Boundary Value Testing is based on the principle that defects are more likely to occur at the boundaries of input ranges rather than the middle. The technique involves creating test cases that focus on the values at the boundaries of input limits, as well as values just below and above these boundaries. These boundary conditions are crucial in ensuring that the system behaves correctly when handling edge cases.
- Test cases are designed to focus on the boundary values of input ranges.
- It helps uncover issues that may arise when the system is given extreme or edge-case inputs.
- Testers often test values just below, at, and just above the boundary limits.
2. Key Concepts of Boundary Value Testing
The primary concepts of Boundary Value Testing include the following:
- Lower Bound: The smallest acceptable value within the input range.
- Upper Bound: The largest acceptable value within the input range.
- Just Below Lower Bound: The value just below the lower bound to test how the system handles values outside the valid range.
- Just Above Upper Bound: The value just above the upper bound to test how the system handles values outside the valid range.
- Valid Boundary Values: The values that lie at the boundary and should be accepted by the system.
3. When to Use Boundary Value Testing
Boundary Value Testing is particularly useful in the following scenarios:
- Validating Input Ranges: When a system accepts input within a specific range, BVT ensures that it handles the boundary values correctly.
- Verifying Edge Cases: It helps verify the system's behavior when inputs are at the extreme ends of the acceptable range.
- Preventing Boundary-Related Defects: BVT can uncover defects caused by improper handling of boundary values.
- Testing Validation Logic: When a system has specific validation rules for input values, BVT ensures that the validation works as expected.
4. Boundary Value Testing Example
Consider a scenario where a system accepts input numbers between 1 and 100. The boundary values for testing would be:
- Lower Bound: 1 (the smallest valid number)
- Upper Bound: 100 (the largest valid number)
- Just Below Lower Bound: 0 (an invalid value that is too small)
- Just Above Upper Bound: 101 (an invalid value that is too large)
Test cases for this scenario would include:
- Test Case 1: Input = 1 (Lower Bound)
- Test Case 2: Input = 100 (Upper Bound)
- Test Case 3: Input = 0 (Just Below Lower Bound)
- Test Case 4: Input = 101 (Just Above Upper Bound)
5. Advantages of Boundary Value Testing
Boundary Value Testing offers several key benefits:
- Effective at Finding Defects: Since boundary errors are common, BVT is highly effective at identifying issues at the edges of input ranges.
- Improves Test Coverage: It helps ensure that edge cases are tested, improving the overall test coverage.
- Simple and Efficient: BVT is relatively simple to implement and can be applied quickly, making it cost-effective.
- Helps Prevent Overflow and Underflow Errors: It helps uncover issues related to data overflow and underflow that can occur at boundary conditions.
6. Challenges of Boundary Value Testing
While Boundary Value Testing is valuable, it also presents some challenges:
- Limited Scope: BVT focuses only on boundary conditions and may not catch defects in the middle of the input range.
- Requires Detailed Knowledge of Input Ranges: Testers need to have a clear understanding of the valid input ranges to create effective boundary test cases.
- May Miss Complex Scenarios: BVT may not be sufficient for complex applications where defects arise from combinations of inputs, not just boundaries.
7. Best Practices for Boundary Value Testing
To maximize the effectiveness of Boundary Value Testing, consider these best practices:
- Identify All Boundary Conditions: Ensure you identify all relevant boundary values, including both valid and invalid conditions.
- Test Beyond Boundaries: Always test values just below and just above the defined boundary limits.
- Combine with Other Testing Techniques: While BVT is effective for boundary-related issues, combine it with other techniques (e.g., equivalence partitioning) for comprehensive testing.
- Document Test Cases Clearly: Record test cases, including boundary values and expected results, for traceability and reporting.
8. Code Example: Boundary Value Testing in Code
Here’s an example of applying Boundary Value Testing in code for a function that validates numbers between 1 and 100:
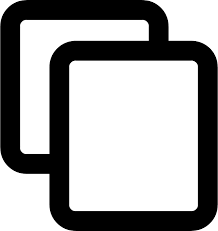
# Function to validate if a number is between 1 and 100
def validate_number(number):
if number < 1 or number > 100:
return "Invalid"
return "Valid"
# Test Cases
print(validate_number(1)) # Lower Bound: Valid
print(validate_number(100)) # Upper Bound: Valid
print(validate_number(0)) # Just Below Lower Bound: Invalid
print(validate_number(101)) # Just Above Upper Bound: Invalid
9. Diagram: Boundary Value Testing Process
The following diagram illustrates the Boundary Value Testing process:

This diagram shows the different boundary values (lower bound, upper bound, just below, and just above) and how they are tested to ensure proper system behavior.
Equivalence Partitioning
Equivalence Partitioning (EP) is a black-box testing technique that divides input data into partitions or classes. The idea is that if a system behaves correctly for one value in a given partition, it will behave correctly for all values in that partition. The goal is to reduce the number of test cases by selecting representative test cases from each partition, while still ensuring effective coverage and the likelihood of finding defects.
1. What is Equivalence Partitioning?
Equivalence Partitioning involves dividing input data into valid and invalid partitions. Test cases are then selected from each partition. This method assumes that all values within a partition will be treated the same by the system, and testing one value from each partition is sufficient to ensure the system behaves correctly for the entire partition.
- Test cases are designed to cover different partitions of input data.
- It reduces the number of test cases by selecting one test case per partition.
- The technique is used to identify equivalence classes where the system is expected to behave similarly.
2. Key Concepts of Equivalence Partitioning
There are two types of equivalence classes in Equivalence Partitioning:
- Valid Equivalence Class: This class includes all valid values that the system should accept as input. These values are within the acceptable range or meet the specified criteria.
- Invalid Equivalence Class: This class includes values that the system should reject. These values are outside the valid range or don't meet the required criteria.
Test cases are then selected from each equivalence class. A single test case is typically selected from each partition, as it is assumed that all values within the partition will be treated the same by the system.
3. When to Use Equivalence Partitioning
Equivalence Partitioning is useful in the following scenarios:
- When Input Range is Large: If a system accepts a large range of inputs, EP helps reduce the number of test cases needed by dividing the inputs into smaller, manageable partitions.
- When System Behavior is Expected to be Uniform: If the system processes inputs in a uniform way within each partition, EP allows testers to focus on representative values.
- For Systems with Defined Validation Rules: When there are specific validation rules for inputs, EP helps identify the boundaries of valid and invalid input ranges.
4. Equivalence Partitioning Example
Consider a scenario where a system accepts input values between 1 and 100. In this case, the equivalence classes would be:
- Valid Equivalence Class: All values between 1 and 100 (inclusive).
- Invalid Equivalence Class 1: Values less than 1 (e.g., 0, -5).
- Invalid Equivalence Class 2: Values greater than 100 (e.g., 101, 150).
Test cases could be selected from the following partitions:
- Test Case 1: Input = 50 (Valid class)
- Test Case 2: Input = 0 (Invalid class – below lower bound)
- Test Case 3: Input = 101 (Invalid class – above upper bound)
5. Advantages of Equivalence Partitioning
Equivalence Partitioning offers several key advantages:
- Reduces Test Cases: EP reduces the number of test cases needed by selecting representative values from each partition, making testing more efficient.
- Improves Coverage: It ensures that all possible input scenarios are covered by selecting test cases from both valid and invalid partitions.
- Focuses on Key Scenarios: By testing representative values, EP focuses on the most critical scenarios, helping ensure that the system handles typical and edge cases correctly.
- Saves Time and Resources: EP minimizes redundant testing and saves time and resources by testing fewer but more effective cases.
6. Challenges of Equivalence Partitioning
While Equivalence Partitioning is a powerful technique, it also has some challenges:
- Over-Simplification: EP assumes that all values in a partition will be treated the same, but some systems might behave differently for different values within the same partition.
- Requires Clear Input Criteria: Clear knowledge of the input criteria and boundaries is required to effectively apply EP.
- May Miss Complex Scenarios: EP focuses on individual partitions and may not catch defects arising from combinations of inputs or more complex interactions.
7. Best Practices for Equivalence Partitioning
To maximize the effectiveness of Equivalence Partitioning, consider the following best practices:
- Identify All Equivalence Classes: Thoroughly analyze the input data to identify all valid and invalid equivalence classes.
- Combine with Other Testing Techniques: Use EP alongside other techniques (e.g., Boundary Value Testing) for comprehensive coverage.
- Use Clear and Defined Criteria: Ensure that the input criteria and rules for partitioning are clearly defined and understood.
- Test with Representative Values: Select test cases that represent the entire partition, ensuring that the system behaves as expected for all possible values in that class.
8. Code Example: Equivalence Partitioning in Code
Here’s an example of applying Equivalence Partitioning in code for a function that validates numbers between 1 and 100:
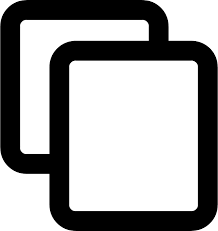
# Function to validate if a number is between 1 and 100
def validate_number(number):
if number < 1 or number > 100:
return "Invalid"
return "Valid"
# Test Cases
print(validate_number(50)) # Valid class: Valid
print(validate_number(0)) # Invalid class: Invalid (below lower bound)
print(validate_number(101)) # Invalid class: Invalid (above upper bound)
9. Diagram: Equivalence Partitioning Process
The following diagram illustrates the Equivalence Partitioning process:

This diagram shows how the input values are divided into valid and invalid partitions, with test cases selected from each partition for effective testing.
What is Automation Testing?
Automation Testing is the process of using specialized software tools and scripts to perform tests on a software application automatically, rather than performing the tests manually. It involves the use of tools, frameworks, and scripts to validate that the software behaves as expected under various conditions, and it can significantly improve the efficiency, effectiveness, and coverage of testing processes. Automation testing is particularly useful in repetitive and time-consuming tasks, as well as in scenarios requiring frequent test execution, such as continuous integration/continuous delivery (CI/CD) pipelines.
1. What is Automation Testing?
Automation Testing uses software to control the execution of tests, compare actual outcomes with expected results, and report the results. The goal is to reduce human intervention, speed up the testing process, and increase test coverage. It is commonly used for regression testing, performance testing, and repetitive tasks where the test script can be reused multiple times.
- Automated Test Script: A test script written using programming languages (e.g., Java, Python) or scripting languages (e.g., JavaScript, Ruby) that performs specific testing actions automatically.
- Test Automation Tools: Specialized software tools (e.g., Selenium, Appium, QTP) that allow testers to automate the execution of tests.
- Continuous Integration (CI) and Continuous Delivery (CD): Automation plays a key role in CI/CD pipelines by enabling automated testing as part of the build and deployment process.
2. Benefits of Automation Testing
Automation Testing brings a wide range of advantages to the software development process:
- Faster Execution: Automation allows tests to be executed faster than manual testing, especially for repetitive tasks and large test suites.
- Higher Test Coverage: Automated tests can cover more scenarios and variations, ensuring broader test coverage in less time.
- Consistency: Automated tests run the same way every time, eliminating the risk of human error and ensuring consistent execution.
- Reusability: Once automated test scripts are created, they can be reused across different versions of the application, reducing the effort required for repeated testing.
- Cost-Effective in the Long Run: While initial automation setup might be costly, over time, it reduces the cost of manual testing, especially for large and complex applications.
- Early Bug Detection: Automated tests can be run frequently, allowing for early detection of bugs and issues as part of the CI/CD pipeline.
3. Types of Automation Testing
Automation Testing can be applied to various testing types, and it is especially useful in the following areas:
- Regression Testing: Automation is ideal for regression testing, ensuring that new changes or features do not break existing functionality.
- Performance Testing: Automation tools such as JMeter and LoadRunner can simulate multiple users to test how the application performs under load.
- Smoke Testing: Automated smoke tests can quickly verify that the critical functionalities of an application are working after a new build or release.
- Functional Testing: Automation can be used to validate that the system functions as expected, especially for repetitive tasks.
- UI Testing: Tools like Selenium WebDriver help in automating user interface testing by simulating user interactions with the application.
4. When to Use Automation Testing
While automation testing offers many benefits, it is not suitable for every scenario. It is most beneficial in the following cases:
- Repetitive Test Cases: Automation is most effective when performing repetitive tests that need to be run frequently.
- Large Test Suites: Automation helps in running large sets of tests quickly, which would be time-consuming and error-prone if done manually.
- Regression Testing: Automation is ideal when testing large systems where changes are frequent, and a large number of tests need to be re-executed with each release.
- Performance Testing: Automation can simulate high loads and stress test the application by generating multiple virtual users at once.
- Continuous Integration Environments: Automated tests can be integrated into CI pipelines to run after every code commit, ensuring the application is always tested after changes.
5. Challenges of Automation Testing
While automation testing offers many advantages, there are some challenges that teams may face:
- High Initial Investment: Setting up automated testing tools and creating the initial test scripts can be expensive in terms of time and resources.
- Maintenance Overhead: Automated tests require regular updates and maintenance to keep up with changes in the application, which can lead to added overhead.
- Not Suitable for All Test Cases: Automation is not always effective for tests that require human judgment or tests with unpredictable results.
- Complexity of Test Script Development: Writing and maintaining complex automation scripts can be difficult, especially for non-technical testers.
6. Popular Automation Testing Tools
There are several widely used tools for automation testing. Some of the most popular ones include:
- Selenium: An open-source tool that automates web browsers. It supports various programming languages like Java, Python, and JavaScript.
- Appium: An open-source tool used for automating mobile applications on Android and iOS platforms.
- Jenkins: A widely used automation server for continuous integration and continuous delivery, often integrated with automated tests.
- JUnit: A widely used testing framework for Java applications that supports unit testing and automation.
- LoadRunner: A performance testing tool that simulates virtual users to test how the application performs under load.
7. Code Example: Automation Test Script (Selenium)
Here’s an example of an automation test script written in Java using Selenium WebDriver:
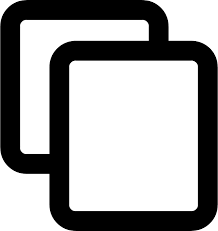
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AutomationTest {
public static void main(String[] args) {
// Set the path to the ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Open the URL
driver.get("https://www.example.com");
// Verify the title of the page
if (driver.getTitle().equals("Example Domain")) {
System.out.println("Test Passed!");
} else {
System.out.println("Test Failed!");
}
// Close the browser
driver.quit();
}
}
8. Diagram: Automation Testing Process
The following diagram illustrates the typical process of automation testing:

This diagram shows the various stages of the automation testing process, from test script development to execution and reporting.
Manual Testing vs. Automation Testing
Manual testing and automation testing are two fundamental approaches to testing software applications. Each approach has its own advantages, limitations, and use cases. Understanding the differences between manual and automation testing is crucial for selecting the appropriate testing method based on the requirements of the project.
1. What is Manual Testing?
Manual testing is the process of manually checking software for defects. It requires human testers to execute test cases, explore the application, and verify its functionality. Manual testing is typically used for small projects, exploratory testing, and when human judgment and intuition are needed. The tester performs tests step by step and compares the actual results with the expected results.
Advantages of Manual Testing
- Flexibility: Manual testing is useful for exploratory testing where human intuition and creativity are needed.
- No Initial Investment: It does not require the upfront cost of automation tools or writing scripts.
- Human Insight: Testers can use their judgment to identify issues that might not be captured by automated tests.
- Suitable for Small Projects: Manual testing is ideal for small-scale or short-term projects where automation may not be cost-effective.
Disadvantages of Manual Testing
- Time-Consuming: Manual testing can be slow, especially for large and complex applications with repetitive test cases.
- Prone to Human Error: Manual testers may miss defects or make mistakes due to fatigue or oversight.
- Limited Test Coverage: It is difficult to test a large number of scenarios or perform extensive regression testing manually.
- High Cost in the Long Run: Repeated manual testing can be resource-intensive and costly over time.
2. What is Automation Testing?
Automation testing is the process of using automated tools and scripts to perform tests on a software application. Unlike manual testing, where a human tester interacts with the software, automation testing uses scripts to execute predefined tests automatically. It is ideal for repetitive, time-consuming tasks, regression testing, and performance testing.
Advantages of Automation Testing
- Faster Execution: Automation testing allows for faster test execution, especially for repetitive tests or large test suites.
- Reusability: Once test scripts are created, they can be reused across different versions of the software, reducing redundant work.
- Higher Test Coverage: Automation allows testers to execute a larger number of test cases in a shorter period, increasing test coverage.
- Consistency: Automated tests run in the same way each time, eliminating the risk of human error.
- Cost-Effective in the Long Run: Although initial setup costs are high, automation can save costs in the long term due to faster execution and reusability.
Disadvantages of Automation Testing
- High Initial Investment: Setting up automated testing requires purchasing tools, setting up environments, and writing scripts, which can be costly.
- Maintenance Overhead: Automated tests require ongoing maintenance to keep up with changes in the application or test environment.
- Not Suitable for All Tests: Some tests, such as exploratory testing or tests requiring human judgment, cannot be automated effectively.
- Requires Technical Expertise: Automation testing requires developers or testers with programming knowledge to write and maintain test scripts.
3. Key Differences Between Manual and Automation Testing
Aspect | Manual Testing | Automation Testing |
---|---|---|
Execution | Test cases are executed manually by the tester. | Test cases are executed automatically using scripts and tools. |
Time | Time-consuming for large projects and repetitive tasks. | Faster execution, especially for repetitive and large test suites. |
Cost | Low initial cost but higher long-term costs due to manual effort. | High initial cost but lower long-term costs due to automation. |
Test Coverage | Limited test coverage due to time and resource constraints. | Higher test coverage due to the ability to run tests more frequently. |
Human Insight | Testers can apply their judgment and intuition to identify defects. | Automation tools execute tests based on predefined scripts without human insights. |
Flexibility | Highly flexible, useful for exploratory and ad-hoc testing. | Best suited for repetitive and regression testing scenarios. |
4. When to Use Manual Testing
Manual testing is best suited for the following scenarios:
- Small projects with limited resources.
- Exploratory testing where human intuition is required to identify potential issues.
- One-time tests or tests that do not need to be repeated.
- When you need to validate the user experience, UI/UX, or usability of the application.
5. When to Use Automation Testing
Automation testing is ideal for the following situations:
- Large projects with complex, repetitive test cases.
- Regression testing after software updates or changes.
- Performance testing for checking how the application behaves under load.
- Tests that need to be run frequently, such as in a continuous integration pipeline.
6. Conclusion
Both manual and automation testing have their place in the software development lifecycle. Manual testing is best suited for small-scale projects and scenarios requiring human judgment, while automation testing excels in large projects with repetitive tests, regression testing, and performance testing. The key is to choose the right approach based on the nature of the project, the complexity of the tests, and the available resources.
Benefits and Limitations of Automation Testing
Automation testing involves using software tools and scripts to automatically perform tests on an application. While automation offers several advantages, it also comes with certain limitations. Understanding both the benefits and drawbacks is essential for deciding whether automation is the right approach for your testing needs.
Benefits of Automation Testing
- Faster Execution: Automated tests can execute much faster than manual tests, especially for repetitive or large-scale test cases. This speed allows for quicker feedback and faster releases.
- Improved Test Accuracy: Automated tests eliminate the risk of human error that can occur in manual testing. Tests are executed in exactly the same way every time, ensuring consistent results.
- Reusability of Test Scripts: Once automation scripts are written, they can be reused across multiple versions of the software, saving time and effort in the long run. These scripts can also be used for different types of testing like regression, functional, and performance testing.
- Higher Test Coverage: Automated testing allows you to run a large number of test cases in a short amount of time, increasing the overall test coverage. This is particularly beneficial for performing regression testing on complex applications with numerous features.
- No Human Fatigue: Unlike manual testing, automated tests can run continuously without tiring or losing focus, making them ideal for long-running tests, such as performance and stress testing.
- Continuous Integration and Continuous Testing: Automation is an essential part of CI/CD pipelines, allowing for continuous testing as code changes are deployed. This helps identify issues early in the development cycle, improving overall code quality.
- Cost-Effective in the Long Run: While automation requires an initial investment, it can become cost-effective over time, especially for large, repetitive projects that require frequent execution of the same test cases.
Limitations of Automation Testing
- High Initial Investment: The upfront cost of automation can be significant. This includes the cost of purchasing automation tools, setting up test environments, and writing and maintaining test scripts. For small projects, these costs may outweigh the benefits.
- Not Suitable for All Tests: Some types of tests, such as exploratory testing, usability testing, and ad-hoc testing, require human intuition and judgment, which automation cannot replicate effectively.
- Requires Technical Expertise: Automation testing requires specialized knowledge in programming and test scripting. Testers need to have skills in writing and maintaining automation scripts, which may require additional training.
- Test Maintenance: Automated test scripts need to be updated and maintained as the application evolves. Changes in the application's user interface, functionality, or requirements may require changes to the test scripts, which can be time-consuming and resource-intensive.
- Limited by Tool Capabilities: Automation tools are limited in their ability to interact with complex or dynamic user interfaces. Some tools may not be able to test certain types of functionality or may require significant customization to do so.
- Not Ideal for Short-Term Projects: For small, one-time, or short-term projects, the effort and cost of setting up automated testing may not be justified, as the time and resources required for automation can exceed the benefits.
- False Sense of Security: Relying entirely on automated tests can give a false sense of security. Automation can only validate what is scripted, and may miss subtle defects that a manual tester might uncover, especially in terms of user experience.
When to Use Automation Testing
Automation testing is ideal in the following scenarios:
- When tests need to be executed frequently, such as in regression testing or continuous integration pipelines.
- When large volumes of data or complex test cases need to be tested.
- For repetitive tasks that would be time-consuming and error-prone if done manually.
- When fast feedback is required to ensure timely releases, such as in agile development environments.
- For performance, load, and stress testing where automated scripts can simulate multiple users or system loads.
When Not to Use Automation Testing
Automation may not be the best choice in the following cases:
- For small projects or one-time projects where the cost of automation outweighs the benefits.
- When the application is constantly changing, as automation scripts will require frequent maintenance.
- For testing areas where human judgment, intuition, or creativity is needed, such as usability testing.
- In early stages of development where the application is still being designed and functionality is highly fluid.
Conclusion
Automation testing offers several benefits, including faster execution, improved accuracy, and higher test coverage, making it an essential tool in large, complex, and repetitive projects. However, its limitations, such as high initial costs, maintenance overhead, and the inability to handle certain types of testing, must also be considered. The decision to use automation should be based on the project's size, complexity, and testing requirements, and it should complement manual testing rather than replace it entirely.
Popular Automation Tools (Selenium, Cypress, TestComplete, etc.)
Automation testing tools are essential for performing various types of testing, such as functional, regression, and performance testing. Below is an overview of some of the most popular automation testing tools available in the market.
Selenium
Selenium is one of the most widely used open-source testing frameworks for web applications. It supports multiple programming languages like Java, C#, Python, and Ruby, and can be used with browsers like Chrome, Firefox, and Safari.
- Key Features:
- Supports multiple browsers and operating systems.
- Can automate web applications across different platforms.
- Flexible and supports a wide range of programming languages.
- Integrates well with other tools like TestNG, JUnit, and Jenkins.
- Allows parallel test execution for faster results.
- Use Cases:
- Cross-browser testing for web applications.
- Regression and functional testing of web applications.
- Limitations:
- Requires programming skills to write scripts.
- Not suitable for desktop or mobile testing without additional tools (e.g., Appium).
Cypress
Cypress is a modern and fast JavaScript-based end-to-end testing framework for web applications. It is known for its simplicity and speed, and it provides an easy-to-use interface for writing and running tests.
- Key Features:
- Real-time browser interaction and debugging.
- Built-in test runner and assertions.
- Automatic waiting for elements before performing actions.
- Easy to set up and use with minimal configuration.
- Supports testing both the frontend and backend.
- Use Cases:
- End-to-end testing of web applications.
- Testing single-page applications (SPA).
- Limitations:
- Only supports JavaScript.
- Limited support for cross-browser testing (works best on Chrome-based browsers).
TestComplete
TestComplete is a commercial, comprehensive automated testing tool that supports functional, regression, and performance testing. It provides an easy-to-use interface for creating automated tests for desktop, mobile, and web applications.
- Key Features:
- Supports multiple scripting languages (JavaScript, Python, VBScript, etc.).
- Can automate tests for desktop, web, and mobile applications.
- Provides a powerful record-and-playback feature for non-technical users.
- Integrates with version control, CI/CD, and defect management tools.
- Supports data-driven testing with external data sources like Excel and databases.
- Use Cases:
- Automating functional and regression tests for web, desktop, and mobile apps.
- Cross-platform testing for multiple OS and browsers.
- Limitations:
- TestComplete is a paid tool and can be expensive for smaller teams or projects.
- May require some learning curve for advanced features.
Other Popular Automation Tools
- Appium: Open-source tool for mobile application testing (both Android and iOS). It supports multiple languages, including Java, JavaScript, and Python, and can automate both native and hybrid mobile apps.
- Katalon Studio: A free tool with an integrated IDE for test automation. It supports both web and mobile testing, and it comes with built-in features like test recording, real-time reporting, and integration with CI tools.
- Ranorex: A commercial test automation tool for desktop, web, and mobile applications. It offers a user-friendly interface with code-free test automation and integration with other tools like Jenkins and Jira.
- TestNG: A testing framework for Java-based applications that is often used alongside Selenium. It supports parallel test execution, data-driven testing, and integration with CI/CD tools like Jenkins.
- Jest: A JavaScript testing framework that is often used for testing React applications. It is known for its simplicity, fast test execution, and built-in mocking capabilities.
Conclusion
Each automation testing tool has its strengths and weaknesses. The choice of tool depends on various factors such as the type of application being tested, programming language preferences, team expertise, and budget. While Selenium and Cypress are great options for web applications, tools like TestComplete offer more comprehensive solutions for desktop and mobile testing. By understanding the features, use cases, and limitations of these tools, teams can choose the best tool for their testing needs.
Unit Testing
Unit testing is a software testing technique where individual units or components of a software are tested in isolation from the rest of the application. It focuses on verifying the correctness of small, specific pieces of code, typically functions or methods, to ensure they work as expected. Unit testing is typically done by developers during the development phase to catch defects early in the process.
What is Unit Testing?
Unit testing involves testing individual functions, methods, or classes in isolation to ensure they produce the expected output given certain inputs. The goal is to ensure that each unit of the software performs as expected without relying on other parts of the system. Unit tests are typically automated and are run frequently to catch bugs early in the development process.
Benefits of Unit Testing
- Early Bug Detection: Unit testing helps identify bugs early in the development process, reducing the cost of fixing them later.
- Improved Code Quality: Writing unit tests forces developers to think critically about the logic and design of their code, leading to better code quality.
- Faster Development: Unit tests can be executed automatically, allowing for quicker feedback and faster iterations.
- Refactoring Confidence: Unit tests provide a safety net when refactoring code, ensuring that changes don't break existing functionality.
- Documentation: Unit tests can serve as documentation, providing examples of how individual functions or methods are expected to behave.
Unit Testing Frameworks
There are several popular unit testing frameworks that make it easier to write and execute unit tests. These frameworks provide built-in assertions and utilities to streamline the testing process. Some popular frameworks include:
- JUnit: A widely used unit testing framework for Java applications. It provides annotations for defining test methods and assertions to verify expected outcomes.
- JUnit 5: The latest version of JUnit, which offers a more modular and flexible approach to testing, supporting features like parameterized tests and testing multiple assertions.
- Mocha: A JavaScript testing framework that works well with Node.js and browser-based applications. Mocha supports asynchronous testing and provides various assertion libraries like Chai.
- Jest: A JavaScript testing framework often used with React applications. Jest is known for its simplicity, built-in mocking capabilities, and fast test execution.
- pytest: A testing framework for Python that is simple to use and supports fixtures, parameterized tests, and detailed reporting.
- NUnit: A popular unit testing framework for .NET applications. It supports a wide range of assertion types and test attributes.
Best Practices for Unit Testing
- Test One Thing at a Time: Each unit test should focus on testing a single piece of functionality to ensure that the test is clear and manageable.
- Write Independent Tests: Unit tests should be independent of one another to avoid dependencies between tests, which can cause false positives or negatives.
- Use Descriptive Test Names: Test names should clearly describe the behavior being tested, making it easier to understand the test's purpose.
- Keep Tests Small and Focused: Unit tests should be small and fast to execute, ensuring that they can be run frequently during development.
- Mock Dependencies: If a unit depends on external systems (e.g., databases, APIs), mock those dependencies to isolate the unit under test.
Example of a Unit Test
Below is an example of a unit test using the Jest framework to test a simple function that adds two numbers:
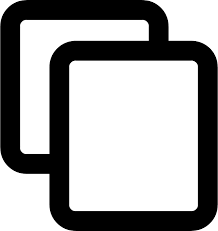
// Function to add two numbers
function add(a, b) {
return a + b;
}
// Jest test case
test('adds two numbers', () => {
expect(add(1, 2)).toBe(3);
});
Running Unit Tests
Unit tests are typically run using a test runner that is part of the testing framework. For example, in Jest, you can run tests using the following command:
npm test
Conclusion
Unit testing is an essential practice in modern software development that helps ensure the correctness and reliability of individual components. By catching defects early, improving code quality, and providing confidence during code changes, unit tests play a crucial role in maintaining high-quality software. Using unit testing frameworks like JUnit, Mocha, Jest, or pytest can help automate the testing process, making it easier and faster to identify issues in the code.
Integration Testing
Integration testing is a type of software testing where individual units of code are combined and tested as a group. The purpose of integration testing is to identify issues that may arise when different modules or components of the system interact with each other, ensuring that they work together as expected.
What is Integration Testing?
Integration testing focuses on verifying the communication and interaction between different modules or components of a software application. Unlike unit testing, which tests individual pieces of code in isolation, integration testing evaluates how well different parts of the application work together. It helps in detecting issues such as data flow problems, interface mismatches, and incorrect behavior when components are integrated.
Types of Integration Testing
There are several different approaches to performing integration testing:
- Big Bang Integration: In this approach, all the modules are integrated at once, and the system is tested as a whole. It can be challenging to pinpoint issues since the integration is done all at once.
- Incremental Integration: This approach involves integrating one module at a time with the system and testing it before adding another. This method is more methodical and allows for easier identification of problems. It can be further divided into:
- Top-Down Integration: Testing begins with the top-level modules and works downward to the lower-level modules.
- Bottom-Up Integration: Testing starts with the lower-level modules and progresses upwards to the higher-level modules.
- Sandwich Integration: This approach combines both top-down and bottom-up integration strategies, testing both higher and lower modules simultaneously.
Benefits of Integration Testing
- Early Detection of Issues: Integration testing helps identify issues that arise when different modules interact, allowing them to be addressed early in the development cycle.
- Improved System Reliability: By testing the integration of modules, it ensures that the system works as a whole and improves the reliability of the final product.
- Reduced Risk of Failure: Integration testing helps reduce the risk of integration failures that could potentially disrupt the system during later stages of development or after deployment.
- Increased Confidence in System Functionality: Integration testing helps ensure that different parts of the system work together smoothly, providing confidence in the overall functionality of the application.
Challenges of Integration Testing
- Complexity: As the number of modules or components increases, integration testing can become complex, requiring careful planning and coordination.
- Dependency Issues: If modules have external dependencies such as databases or third-party services, it can be difficult to simulate or test these integrations effectively.
- Resource Intensive: Depending on the scope of the system being tested, integration testing can require significant resources, such as time, infrastructure, and test environments.
- Test Data Management: Managing test data for integration testing can be challenging, especially when there are multiple modules interacting with each other and sharing common data.
Integration Testing Tools
Several tools are available to assist with integration testing, including:
- JUnit: A widely used testing framework for Java that can be used for both unit and integration testing. JUnit offers various annotations and assertions for testing integration points between modules.
- Postman: Primarily used for API testing, Postman can be used to test the integration of different services by sending requests between them and validating responses.
- Mockito: A mocking framework for Java that is often used in integration testing to simulate the behavior of dependencies and isolate the modules under test.
- FitNesse: A collaborative testing tool that can be used for both unit and integration testing. It allows developers, testers, and business users to define tests in a simple and readable format.
- SoapUI: A popular tool for testing SOAP and REST APIs, SoapUI can be used for integration testing of web services and APIs.
Best Practices for Integration Testing
- Test Interfaces: Pay special attention to the interfaces between modules to ensure data flows correctly and modules interact properly.
- Test with Realistic Data: Use realistic test data that closely mirrors the actual data the application will handle in production.
- Isolate Dependencies: Use mocking frameworks or stubbing to isolate external dependencies such as databases, third-party services, or APIs for more controlled and effective testing.
- Automate Integration Tests: Automate integration tests to ensure that they can be run frequently and consistently, especially in continuous integration (CI) and continuous deployment (CD) pipelines.
- Perform Regression Testing: As new modules are integrated, perform regression tests to ensure that no existing functionality is broken by the new changes.
Example of Integration Testing
Here is an example of integration testing in Java using JUnit. This test verifies the integration between two modules: a database service and a user authentication service:
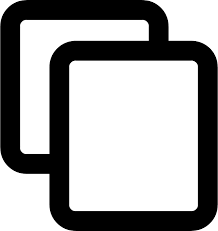
import org.junit.Test;
import static org.junit.Assert.*;
public class UserAuthenticationTest {
@Test
public void testUserLoginIntegration() {
// Simulating user login process
UserService userService = new UserService();
DatabaseService databaseService = new DatabaseService();
String username = "testuser";
String password = "password123";
// Database call and user authentication integration
boolean result = userService.authenticateUser(username, password, databaseService);
// Assert that the user is authenticated successfully
assertTrue(result);
}
}
Conclusion
Integration testing is a vital part of the software testing process, ensuring that various components of a system work together as expected. By identifying and resolving integration issues early, you can improve system reliability, reduce risks, and enhance the overall quality of the software. Using proper testing tools, following best practices, and addressing challenges effectively will help ensure successful integration testing.
System Testing
System testing is a type of software testing where the complete and integrated software is tested as a whole. The objective of system testing is to evaluate the system's compliance with its specified requirements, ensuring that all components of the software work together as expected in a fully integrated environment.
What is System Testing?
System testing is a high-level testing process that involves testing the entire application or software as a complete system. It verifies whether the system meets the functional and non-functional requirements specified in the software requirement specification (SRS) document. System testing takes place after integration testing and before acceptance testing.
Types of System Testing
System testing includes various types of tests to validate the entire system's behavior. Some of the common types of system testing are:
- Functional Testing: This type of testing verifies that the system behaves according to the functional requirements. It checks whether the system performs its intended functions as specified.
- Usability Testing: Usability testing evaluates how user-friendly and intuitive the system is. It ensures that the application is easy to use and provides a good user experience.
- Performance Testing: This type of testing focuses on evaluating the system's performance under normal and peak loads. It checks for scalability, responsiveness, and stability under various conditions.
- Security Testing: Security testing ensures that the system is secure and protected from vulnerabilities, unauthorized access, and data breaches.
- Compatibility Testing: Compatibility testing verifies that the system works across different environments, browsers, devices, and operating systems.
- Regression Testing: Regression testing ensures that new changes or additions to the system do not break existing functionality.
- Recovery Testing: Recovery testing evaluates the system's ability to recover from failures, such as crashes, network issues, or power loss.
- Installation Testing: Installation testing checks that the system installs and configures correctly on various platforms, ensuring a smooth installation process.
Benefits of System Testing
- Complete System Validation: System testing ensures that the entire system works as expected and meets the specified requirements, offering confidence in the system's functionality.
- Identification of Integration Issues: By testing the system as a whole, system testing can help uncover issues that may arise from interactions between different components or modules.
- Improved Quality and Reliability: System testing helps improve the overall quality and reliability of the software by identifying defects and ensuring that the system behaves as expected under various conditions.
- Reduced Risk of Post-Deployment Issues: Thorough system testing reduces the likelihood of issues arising after deployment by ensuring that the system has been fully tested and validated before release.
Challenges in System Testing
- Complexity: As system testing involves testing the entire application, it can be complex, especially when the system is large and composed of many components.
- Time-Consuming: System testing can be time-consuming due to the need to test multiple aspects of the system, including functional, non-functional, and user acceptance requirements.
- Dependency on Other Testing Phases: System testing is dependent on the successful completion of previous testing phases, such as unit testing and integration testing. Delays in these phases can impact the overall testing process.
- Resource Intensive: System testing requires significant resources, such as skilled testers, hardware, and test environments, which can increase costs and time.
System Testing Tools
There are several testing tools available to help perform system testing efficiently:
- LoadRunner: A performance testing tool that helps evaluate the system's behavior under heavy load and stress conditions. LoadRunner can simulate multiple users to test the system's scalability and performance.
- QTP (QuickTest Professional): A functional testing tool that automates user interactions with the application and checks whether the system meets the specified functional requirements.
- JMeter: A popular open-source tool for performance and load testing that can be used to test the system's performance under varying load conditions.
- Selenium: Selenium is a widely used tool for automating web applications for functional testing, especially for system testing in web-based applications.
- Appium: A tool for automating mobile applications, which can be used for system testing of mobile apps across various devices and operating systems.
- TestComplete: A comprehensive automated testing tool for system testing that supports functional, regression, and performance testing across different platforms.
Best Practices for System Testing
- Test Early and Often: Begin system testing early in the software development life cycle to identify and address issues promptly. Perform regular tests to catch issues as the system evolves.
- Understand Requirements Thoroughly: Ensure that you have a clear understanding of the system's functional and non-functional requirements, which will help in designing effective test cases.
- Automate Repetitive Tests: Automate repetitive tests, such as regression testing, to save time and ensure consistent results across different test iterations.
- Test in Realistic Environments: Perform system testing in environments that closely resemble production environments to uncover issues related to performance, security, and compatibility.
- Involve Stakeholders: Involve stakeholders, such as developers, business analysts, and end users, during system testing to ensure that all aspects of the system are validated and that it meets user expectations.
- Document Test Results: Keep detailed records of test results, including defects, issues, and feedback from stakeholders, to ensure effective communication and traceability.
System Testing Example
Here's an example of system testing for a login functionality of an online banking system:
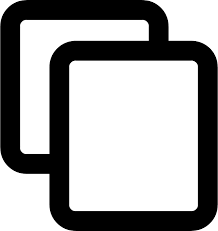
import org.junit.Test;
import static org.junit.Assert.*;
public class SystemTest {
@Test
public void testLoginFunctionality() {
// Simulating a user login attempt
LoginPage loginPage = new LoginPage();
HomePage homePage = loginPage.login("testuser", "password123");
// Assert that the user is redirected to the home page after successful login
assertNotNull(homePage);
assertTrue(homePage.isUserLoggedIn());
}
}
Conclusion
System testing is a crucial phase in the software development process, ensuring that the complete system functions as expected across various scenarios. By validating the system's behavior as a whole, system testing helps detect defects early, reduce risks, and improve the overall quality of the software before deployment.
User Acceptance Testing (UAT)
User Acceptance Testing (UAT) is the final phase of the software testing process, where the end users or clients test the software to ensure that it meets their requirements and is ready for production. UAT helps determine if the system is functional, reliable, and acceptable for deployment in the real-world environment.
What is User Acceptance Testing?
User Acceptance Testing (UAT) is conducted by the actual users of the system to verify if the software can perform the tasks it was intended to do in the real-world scenario. This testing phase ensures that the software meets the business requirements, works in the intended environment, and satisfies the user needs before it is released for production.
UAT Process
The UAT process typically follows these steps:
- Requirements Review: The stakeholders and end users review the software requirements to ensure that they align with business needs and user expectations.
- Test Plan Creation: A UAT test plan is developed that outlines the scope, objectives, and criteria for success. It also includes test scenarios based on real-world use cases.
- Test Case Development: Test cases are created based on the business requirements and end-user expectations. These test cases focus on validating functional and non-functional requirements from the user’s perspective.
- Test Execution: The end users test the software based on the defined test cases. They perform tasks and validate that the system works as expected and meets their needs.
- Defect Reporting: If any issues or defects are found during the testing process, they are reported to the development team for resolution.
- Test Results Evaluation: After completing the tests, the results are evaluated to determine if the system is ready for deployment based on the business acceptance criteria.
- Sign-Off: If the system passes the tests and meets the acceptance criteria, the end users give their approval or sign-off, allowing the system to be deployed to production.
Types of User Acceptance Testing
There are several types of User Acceptance Testing, each with different focuses:
- Alpha Testing: This is an early form of UAT, typically conducted by internal staff or a small group of end users before releasing the software to a broader audience. The goal is to identify bugs, inconsistencies, and usability issues.
- Beta Testing: Beta testing is the next phase of UAT, where the software is released to a larger group of end users. Feedback from this group helps identify any remaining issues before the final release.
- Contractual Acceptance Testing: This type of UAT is conducted to ensure that the software meets the contractual requirements agreed upon between the client and the vendor. It focuses on verifying that all terms and conditions are met.
- Regulatory Acceptance Testing: This is conducted to ensure that the system complies with regulatory standards and laws, such as industry-specific guidelines or security and privacy regulations.
Benefits of User Acceptance Testing
- Ensures Business Requirements are Met: UAT ensures that the software meets the specific business needs and objectives defined by the stakeholders.
- Validates Real-World Scenarios: By testing the software in real-world scenarios, UAT helps ensure that the system behaves as expected in the production environment.
- Reduces Risk of Post-Deployment Issues: UAT helps identify issues that might have been overlooked during earlier phases of testing, reducing the risk of defects in production.
- Improves User Satisfaction: Involving users in the testing process helps ensure that the software is user-friendly, intuitive, and aligned with their needs, leading to higher user satisfaction.
- Increases Stakeholder Confidence: Successful UAT results in stakeholders' confidence that the software is ready for release, increasing trust in the development process and final product.
Challenges in User Acceptance Testing
- Test Case Coverage: Designing comprehensive test cases that cover all real-world scenarios can be challenging, as it requires a deep understanding of user needs and workflows.
- End-User Availability: UAT requires active participation from end users, which can be challenging if they have limited availability or are not fully engaged in the process.
- Miscommunication: Misunderstandings between the development team and end users regarding acceptance criteria or test cases may lead to confusion and delays.
- Time Constraints: UAT is typically performed in the final stages of the project, and time constraints can lead to incomplete or rushed testing, potentially missing critical issues.
UAT Best Practices
- Involve Stakeholders Early: Engage stakeholders and end users early in the process to define clear acceptance criteria and ensure their needs are adequately addressed in the test cases.
- Define Clear Requirements: Make sure that the business requirements are clearly defined and understood by both the development team and the testers to avoid misunderstandings during testing.
- Provide Training to Users: Offer training to the end users to ensure they understand the testing process and the software features they need to validate during UAT.
- Communicate Effectively: Maintain open communication between the testers, developers, and business stakeholders throughout the UAT process to ensure issues are addressed promptly.
- Document Results: Properly document the results of UAT, including any defects or feedback from end users, to ensure that all issues are tracked and resolved before deployment.
Example of User Acceptance Testing
Here's an example of a UAT scenario for an online shopping platform:
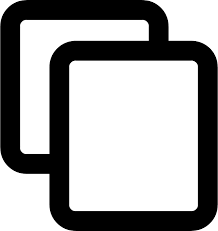
import org.junit.Test;
import static org.junit.Assert.*;
public class UAT {
@Test
public void testCheckoutProcess() {
// Simulating a user adding items to the cart and checking out
ShoppingCart cart = new ShoppingCart();
cart.addItem("Laptop", 1);
cart.addItem("Phone", 2);
CheckoutPage checkout = cart.proceedToCheckout();
// Verify the checkout process works as expected
assertTrue(checkout.isTotalAmountCorrect());
assertTrue(checkout.isPaymentGatewayAvailable());
assertTrue(checkout.isShippingAddressValid());
}
}
Conclusion
User Acceptance Testing (UAT) plays a crucial role in ensuring that the software meets business requirements and user expectations before it is deployed to production. By involving real users in the testing process, UAT helps uncover issues that may not have been identified during earlier testing phases, ensuring a smooth and successful product release.
Performance Testing
Performance Testing is a type of testing aimed at ensuring that software applications perform well under expected or peak load conditions. It involves assessing the speed, responsiveness, and stability of a system under varying levels of stress.
Types of Performance Testing
- Load Testing: Load testing is performed to evaluate the system's behavior under normal and peak load conditions. The goal is to ensure that the system can handle a specified number of users or transactions without performance degradation.
- Stress Testing: Stress testing involves testing the system beyond its specified limits to determine how it behaves under extreme conditions. It helps identify the breaking point of the system and how it recovers from failure.
- Spike Testing: Spike testing evaluates the system's response to sudden and extreme increases in load. It helps assess how the system handles sudden spikes in traffic or user activity.
- Endurance Testing: Endurance testing, also known as soak testing, is conducted to assess the system's performance over an extended period. This test checks for memory leaks, performance degradation, and other issues that may arise during prolonged usage.
Performance Testing Process
- Test Planning: Define performance requirements, goals, and metrics based on the business needs and expected system usage.
- Test Design: Create test scripts, scenarios, and data sets to simulate real-world user behavior and performance conditions.
- Test Execution: Run the performance tests under different conditions, such as varying load, stress, and endurance, using tools like JMeter, LoadRunner, or Gatling.
- Test Monitoring: Monitor system parameters such as CPU usage, memory usage, network bandwidth, and database performance during the test.
- Test Analysis: Analyze the results to identify bottlenecks, performance issues, and areas for improvement in the system.
- Reporting: Document the test results, including any performance issues identified, and provide recommendations for optimization.
Tools for Performance Testing
Some popular tools used for performance testing include:
- Apache JMeter: A widely used open-source tool for load testing and performance measurement of web applications.
- LoadRunner: A comprehensive performance testing tool from Micro Focus that simulates virtual users to test the performance of applications under load.
- Gatling: An open-source performance testing tool designed for testing web applications with a focus on high performance and scalability.
- NeoLoad: A performance testing tool for web and mobile applications that allows for load, stress, and performance testing.
Benefits of Performance Testing
- Improves User Experience: Ensures that the system provides a smooth and responsive user experience even during peak load conditions.
- Identifies Bottlenecks: Helps identify performance bottlenecks and areas of the system that need optimization.
- Ensures Scalability: Ensures that the system can scale effectively to accommodate an increasing number of users or transactions.
- Reduces Risk: Performance testing helps reduce the risk of performance-related failures after deployment, improving reliability and stability.
- Optimizes Resources: Helps optimize system resources, ensuring that they are used efficiently under varying load conditions.
Challenges in Performance Testing
- Accurate Simulation of Real-World Load: Creating realistic test scenarios that accurately simulate real-world usage patterns can be challenging.
- Environment Setup: Setting up a testing environment that mirrors the production environment can be difficult and time-consuming.
- Data Management: Managing test data, such as user profiles and transaction records, can become complex in large-scale performance tests.
- Interpretation of Results: Analyzing performance test results can be complex, requiring expertise to identify the root cause of performance issues.
Conclusion
Performance testing is essential for ensuring that an application can handle expected traffic and perform optimally under varying conditions. By identifying and addressing performance issues early in the development process, organizations can deliver high-quality, scalable, and user-friendly systems.
Load Testing Example
Here’s an example of a simple load testing scenario for an e-commerce website:
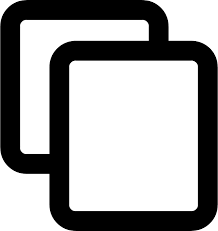
// Apache JMeter Example for Load Testing
// Create a Thread Group with 100 users
// Set ramp-up time to 10 seconds
ThreadGroup threadGroup = new ThreadGroup();
threadGroup.setNumThreads(100);
threadGroup.setRampUp(10);
// Add an HTTP Request sampler
HTTPRequest httpRequest = new HTTPRequest();
httpRequest.setMethod("GET");
httpRequest.setURL("https://www.example.com");
// Add HTTP Request to the Thread Group
threadGroup.addTestElement(httpRequest);
// Add the Thread Group to the Test Plan
TestPlan testPlan = new TestPlan("Load Testing Plan");
testPlan.addThreadGroup(threadGroup);
// Run the test and analyze the results
JMeter.run(testPlan);
Security Testing
Security Testing is a type of software testing that ensures the software application is protected from unauthorized access, data breaches, and vulnerabilities. It is important to safeguard applications from malicious attacks, ensuring data privacy and system integrity.
What is Security Testing?
Security testing is the process of identifying and addressing security vulnerabilities in software applications. It focuses on verifying that the system’s security mechanisms are working as intended and that unauthorized access, data leaks, and other malicious activities are prevented.
Goals of Security Testing
- Data Protection: Ensuring that sensitive data is protected from unauthorized access, theft, or manipulation.
- Authentication and Authorization: Verifying that only authorized users can access certain resources and perform specific actions.
- Preventing Data Breaches: Ensuring that the system is resistant to attacks that attempt to steal or tamper with data.
- System Integrity: Ensuring that the integrity of the system is maintained, and no unauthorized modifications or injections occur.
- Compliance: Ensuring the application meets regulatory and organizational security requirements, such as GDPR, HIPAA, and PCI-DSS.
Types of Security Testing
- Vulnerability Scanning: An automated process of scanning the system for known vulnerabilities and weaknesses that could be exploited by attackers.
- Penetration Testing: Ethical hacking performed by security professionals to identify vulnerabilities by simulating real-world attacks.
- Security Auditing: A comprehensive review of the system's security policies, controls, and configuration to ensure compliance with security standards.
- Risk Assessment: Identifying potential risks to the system and evaluating the severity of these risks to determine mitigation strategies.
- Posture Assessment: A comprehensive evaluation of the security posture of an organization, focusing on its ability to defend against cyberattacks.
- Fuzz Testing: A technique used to discover vulnerabilities by inputting random, unexpected, or invalid data into a program to see how it handles it.
Common Security Vulnerabilities
- SQL Injection: Attackers can inject malicious SQL code into an input field, potentially gaining unauthorized access to the database.
- Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by other users, allowing them to steal session cookies or credentials.
- Cross-Site Request Forgery (CSRF): Attackers trick users into performing actions on a web application without their knowledge or consent.
- Broken Authentication: Weak authentication mechanisms can allow attackers to bypass security measures and gain unauthorized access to the system.
- Insecure Deserialization: Attackers exploit insecure deserialization to execute malicious code and manipulate application behavior.
- Security Misconfigurations: Incorrectly configured security settings or the use of default passwords can expose the system to attacks.
Tools for Security Testing
There are several tools available for security testing to identify and address vulnerabilities in software applications:
- Burp Suite: A comprehensive platform for web application security testing, offering tools for scanning, crawling, and analyzing security issues.
- OWASP ZAP (Zed Attack Proxy): An open-source security testing tool used for finding vulnerabilities in web applications through automated and manual testing.
- Nessus: A vulnerability scanner that helps identify security flaws in a network, systems, and applications.
- Metasploit: A penetration testing tool that allows security professionals to simulate attacks and test system vulnerabilities.
- Wireshark: A network protocol analyzer that helps in capturing and analyzing network traffic to detect security issues.
Writing Secure Test Cases
When performing security testing, it's important to write test cases that cover various attack vectors and security risks:
- Input Validation: Test the application for input validation vulnerabilities, such as SQL injection or cross-site scripting (XSS), by providing unexpected or malicious input.
- Session Management: Ensure that the application uses secure session management techniques, such as session timeouts and encrypted session IDs.
- Access Control: Test the system to ensure that users can only access resources they are authorized for, and that there are no privilege escalation issues.
- Authentication Mechanisms: Verify that authentication mechanisms, such as login pages and password resets, are secure and resistant to brute-force or credential stuffing attacks.
- Data Encryption: Ensure that sensitive data is encrypted both at rest and in transit to prevent unauthorized access or interception.
Benefits of Security Testing
- Prevents Data Breaches: Helps prevent unauthorized access to sensitive data, reducing the risk of data breaches.
- Identifies Vulnerabilities: Identifies potential weaknesses in the system that could be exploited by attackers.
- Improves Trust: Ensures that users and stakeholders trust the security and integrity of the application.
- Regulatory Compliance: Helps ensure that the application meets industry standards and regulatory requirements for data security and privacy.
- Reduces Risk: By identifying and addressing security issues, security testing reduces the risk of successful cyberattacks.
Conclusion
Security testing is an essential part of the software development lifecycle. It helps ensure that applications are protected from unauthorized access, data leaks, and other security threats. By identifying vulnerabilities early in the development process, security testing helps to build more secure and trustworthy software systems.
Usability Testing
Usability Testing is a type of software testing that focuses on evaluating the user-friendliness, ease of use, and overall user experience of a software application. It ensures that the product is intuitive, efficient, and provides a positive experience for its intended users.
What is Usability Testing?
Usability testing involves assessing a software application by testing it with real users to gather feedback about its functionality, design, and usability. The goal is to identify any usability issues that may hinder the user's interaction with the product and improve the overall user experience.
Objectives of Usability Testing
- Evaluate User Interface (UI): Testing the interface of the software to ensure that it is easy to navigate, aesthetically pleasing, and intuitive.
- Identify User Pain Points: Identifying any obstacles or confusion users may encounter during interaction with the application.
- Improve User Satisfaction: Ensuring the user’s experience is positive, efficient, and satisfying, leading to higher user retention and engagement.
- Enhance Product Accessibility: Testing whether the application is accessible to all user groups, including those with disabilities, by following accessibility guidelines.
- Validate Design Choices: Verifying whether the design choices align with user expectations and enhance the overall user experience.
Types of Usability Testing
- Formative Testing: Conducted early in the development process to evaluate design concepts and gather feedback for improvements before the product is finalized.
- Summative Testing: Conducted after the product is developed to validate the final design and ensure it meets the users' needs and expectations.
- Remote Usability Testing: Users test the software from their own location, typically using screen recording and remote collaboration tools to gather feedback.
- Moderated Usability Testing: Involves a facilitator guiding users through tasks and asking questions in real-time to observe their behavior and gather insights.
- Unmoderated Usability Testing: Users perform tasks independently without a facilitator, often using automated tools that record their actions and responses for later analysis.
- Exploratory Usability Testing: Users explore the product freely, without specific tasks, to discover usability issues or unexpected behaviors.
Usability Testing Process
- Planning: Define the testing objectives, select the target audience, and create test scenarios that reflect real-world usage of the application.
- Recruiting Participants: Select participants who represent the target user group and ensure a diverse range of users are involved to gain valuable feedback.
- Test Execution: Conduct the usability testing session, observe user interactions, and collect qualitative and quantitative data on usability issues.
- Data Analysis: Analyze the feedback collected from users, identify trends and issues, and prioritize areas that need improvement.
- Reporting and Recommendations: Provide actionable recommendations based on the findings, including specific design changes, feature improvements, or usability adjustments.
Benefits of Usability Testing
- Improves User Experience: Helps identify issues that may negatively impact the user’s experience, leading to more intuitive and user-friendly designs.
- Increases User Satisfaction: Ensures the product meets user needs, preferences, and expectations, resulting in higher satisfaction and retention.
- Reduces Development Costs: Identifying usability issues early in the development process helps avoid costly redesigns and fixes later on.
- Enhances Product Adoption: A user-friendly product that meets the needs of its target audience is more likely to be adopted and widely used.
- Validates Design Decisions: Helps verify that design choices, such as layout, navigation, and features, align with user expectations and work effectively.
Common Usability Issues
- Poor Navigation: Difficult or confusing navigation can frustrate users and make it hard for them to find what they need.
- Unclear Instructions: Lack of clear instructions or guidance can lead to user confusion and mistakes.
- Inconsistent Design: Inconsistent UI elements, such as buttons, fonts, or color schemes, can create confusion and hinder usability.
- Slow Performance: Slow load times or delayed interactions can lead to a poor user experience and frustration.
- Accessibility Issues: Not adhering to accessibility guidelines can exclude users with disabilities and limit the product’s reach.
Usability Testing Tools
Several tools are available for conducting usability testing and gathering feedback from users:
- Hotjar: A tool that provides heatmaps, session recordings, and surveys to understand user behavior and identify usability issues.
- Lookback: A remote usability testing platform that allows you to conduct live sessions, record user interactions, and analyze feedback.
- UserTesting: A platform for gathering real-time feedback from users through video-based testing and user interviews.
- Crazy Egg: A tool that offers heatmaps and A/B testing features to assess user interaction and improve website usability.
- Optimal Workshop: A suite of usability testing tools, including card sorting, tree testing, and surveys, to enhance information architecture and navigation design.
Conclusion
Usability testing is essential for creating applications that are easy to use, intuitive, and meet the needs of users. By identifying usability issues and making necessary improvements, businesses can enhance user satisfaction, improve product adoption, and ultimately deliver a more successful product. Regular usability testing throughout the development lifecycle ensures that the final product aligns with user expectations and delivers a positive experience.
Compatibility Testing
Compatibility Testing is a type of software testing that checks whether a software application works as expected across different environments, platforms, devices, and browsers. It aims to ensure that the application functions properly on a variety of configurations, including different operating systems, hardware, and network environments.
What is Compatibility Testing?
Compatibility Testing involves testing an application in various environments to confirm that it works seamlessly and consistently across all supported platforms. This type of testing ensures that the software is compatible with different versions of operating systems, browsers, hardware configurations, and network settings.
Objectives of Compatibility Testing
- Ensure Cross-Browser Compatibility: Test the application on different browsers (e.g., Chrome, Firefox, Safari, Edge) to ensure consistent behavior and appearance.
- Verify Operating System Compatibility: Ensure the application functions correctly on multiple operating systems (e.g., Windows, macOS, Linux, Android, iOS).
- Test Hardware Compatibility: Verify that the application is compatible with various hardware configurations, including different screen sizes, resolutions, and devices (e.g., desktops, laptops, smartphones, tablets).
- Check Network Environment Compatibility: Ensure the software works optimally under various network conditions such as different bandwidths, firewalls, and proxies.
- Ensure Backward Compatibility: Verify that the application works with older versions of browsers, operating systems, and hardware components.
Types of Compatibility Testing
- Browser Compatibility Testing: Ensures that the application works consistently across a wide range of browsers, including all major and popular web browsers like Chrome, Firefox, Safari, and Internet Explorer.
- Operating System Compatibility Testing: Tests the software’s compatibility across different operating systems such as Windows, macOS, Linux, and mobile OS like Android and iOS.
- Device Compatibility Testing: Verifies that the application works correctly across various devices, including desktops, laptops, tablets, and smartphones, ensuring responsiveness and usability.
- Network Compatibility Testing: Assesses how the application behaves under different network conditions, including varying network speeds, bandwidths, and the impact of firewalls, VPNs, and proxies.
- Backward Compatibility Testing: Checks whether the software is compatible with older versions of browsers, operating systems, and other components while maintaining its functionality.
Compatibility Testing Process
- Identify Target Configurations: Define the platforms, devices, browsers, and OS versions the application must support based on the target audience.
- Set Up Test Environments: Prepare various test environments, including different operating systems, browsers, and devices, to simulate real-world conditions.
- Perform Functional Testing: Conduct functional testing on each environment to ensure the software behaves as expected without errors or issues.
- Test for User Interface (UI) Consistency: Verify that the UI elements, layout, and design are consistent across different environments, ensuring a seamless user experience.
- Analyze Results: After testing, analyze the results and identify any compatibility issues, such as layout problems, missing features, or performance degradation.
- Fix Issues and Retest: Resolve any compatibility issues found during testing and retest the application to verify that the issues are fixed and no new issues have been introduced.
Benefits of Compatibility Testing
- Increased User Satisfaction: Ensures that users have a consistent and seamless experience, regardless of the platform or device they are using.
- Wider Audience Reach: By ensuring compatibility with various platforms and devices, you increase the potential user base of the software.
- Improved Brand Reputation: A well-tested, compatible application enhances the brand's reputation by providing a stable and reliable user experience across multiple environments.
- Reduced Risk of Compatibility Issues: Identifying and fixing compatibility issues during testing reduces the risk of critical issues arising post-launch.
- Enhanced Product Quality: Compatibility testing helps improve the overall quality of the software, ensuring it works as expected in a variety of real-world scenarios.
Challenges of Compatibility Testing
- Large Number of Configurations: It can be difficult to test every possible combination of platforms, devices, and configurations, especially with constantly evolving technologies.
- Time and Resource Intensive: Compatibility testing can be resource-intensive, requiring significant time and effort to set up different test environments and manage testing across numerous configurations.
- Tools and Automation: While some tools can help automate compatibility testing, they may not cover every scenario, and manual testing may still be necessary.
- Frequent Updates: Keeping up with frequent updates to browsers, operating systems, and devices can make it challenging to maintain compatibility over time.
Common Tools for Compatibility Testing
- BrowserStack: A cloud-based tool that allows testing across a wide range of browsers, operating systems, and devices.
- CrossBrowserTesting: A tool that offers live testing and automated testing across various browsers and devices.
- Sauce Labs: Provides cross-browser testing on cloud-based virtual machines and real devices.
- LambdaTest: A cloud testing platform that allows cross-browser and cross-platform testing in real-time and automated modes.
- Appium: A popular tool for automating mobile app testing on both Android and iOS devices, ensuring compatibility across platforms.
Conclusion
Compatibility testing is essential for ensuring that your application functions correctly across different platforms, devices, browsers, and operating systems. It enhances the user experience, increases user satisfaction, and helps reach a broader audience. Although it can be challenging and resource-intensive, the benefits of ensuring software compatibility far outweigh the difficulties, leading to a higher-quality product and fewer post-launch issues.
Scalability Testing
Scalability Testing is a type of software testing that evaluates how well a software application can scale in response to an increase in load, whether it's more users, data, transactions, or other workloads. The goal is to determine the system’s ability to handle growth and ensure it can maintain performance as demand increases.
What is Scalability Testing?
Scalability Testing is designed to assess the ability of an application to accommodate growth. It involves testing the system to see how it behaves when subjected to increased loads, such as a growing number of concurrent users, larger datasets, or an increase in transactions. Scalability testing helps identify bottlenecks, limitations, and performance degradation as the system scales.
Objectives of Scalability Testing
- Evaluate System Performance Under Load: Test how the system performs when subjected to an increasing amount of traffic, data, or users.
- Identify Bottlenecks: Find performance bottlenecks that can prevent the system from scaling efficiently, such as CPU, memory, or database limitations.
- Measure System Response Time: Assess how the response time of the system changes as the load increases.
- Determine System Capacity: Establish the maximum load the system can handle while maintaining acceptable performance.
- Ensure Stability During Growth: Ensure that the system can maintain its stability and reliability as traffic or data volume increases.
Types of Scalability Testing
- Vertical Scalability Testing: Involves testing how the system behaves when more resources (such as CPU, memory, or storage) are added to a single machine or server.
- Horizontal Scalability Testing: Involves testing how the system performs when the load is distributed across multiple machines or servers, such as adding more nodes to a cloud infrastructure.
- Elastic Scalability Testing: Focuses on testing how well the system can scale dynamically, adjusting resources based on real-time load demands, often in cloud-based environments.
Scalability Testing Process
- Define Performance Metrics: Establish the key metrics to measure, such as response time, throughput, resource usage (CPU, memory), and system availability.
- Identify the Scaling Scenarios: Determine the different types of loads that will be tested, such as increasing the number of users, data, or transactions.
- Prepare the Test Environment: Set up the environment to simulate real-world loads and ensure the system is configured properly for the test.
- Conduct the Scalability Test: Gradually increase the load on the system while monitoring performance. Test vertical, horizontal, or elastic scaling depending on the goals.
- Analyze Results: Analyze the data collected during testing, focusing on system response times, resource utilization, and any performance degradation or bottlenecks.
- Optimize and Retest: Based on the results, make necessary optimizations to the system, then retest to ensure improvements are effective and scalability has been achieved.
Benefits of Scalability Testing
- Ensures Long-Term Viability: Scalability testing ensures that the system can handle growth and future demands without failing or encountering performance issues.
- Improves System Performance: Identifying bottlenecks and limitations allows for performance optimization, improving the user experience as the system scales.
- Enhances Stability and Reliability: Scalability testing helps ensure that the system remains stable and reliable as traffic or data volume increases.
- Reduces Risk of Downtime: By testing scalability in advance, the likelihood of unexpected system failures or downtime due to increased load is minimized.
- Optimizes Resource Usage: Scalability testing helps optimize the use of available resources, ensuring that the system can scale efficiently without over-provisioning or under-provisioning resources.
Challenges of Scalability Testing
- Setting Realistic Load Scenarios: It can be challenging to predict the exact load that the system will face in real-world conditions, making it difficult to design accurate scalability tests.
- Complexity of Distributed Systems: Testing scalability in distributed systems or cloud environments can be more complex due to the dynamic nature of resources and the involvement of multiple components.
- Resource Intensive: Scalability testing can be resource-intensive, requiring significant computational power and infrastructure to simulate large-scale loads and monitor system performance.
- Difficulty in Analyzing Results: Analyzing the results of scalability tests can be challenging, especially when dealing with large amounts of data and complex performance metrics.
Tools for Scalability Testing
- Apache JMeter: A popular open-source tool for performance and scalability testing that can simulate high loads and measure various performance metrics.
- LoadRunner: A comprehensive tool for load and scalability testing that helps simulate virtual users and analyze system performance under various conditions.
- Gatling: A powerful load testing tool designed for high-performance testing and measuring scalability across applications.
- BlazeMeter: A cloud-based performance testing tool that allows scalability testing in a variety of environments, including mobile, API, and web applications.
- NeoLoad: A load testing tool that is designed to help identify bottlenecks and ensure scalability by simulating user traffic and load on applications.
Conclusion
Scalability testing is crucial for ensuring that a system can handle increasing loads while maintaining optimal performance. By identifying bottlenecks, measuring system capacity, and optimizing resource usage, scalability testing helps prevent performance degradation as traffic, data, and user counts grow. Conducting effective scalability tests allows organizations to ensure their systems are prepared for future growth and can deliver a stable, reliable user experience even under heavy loads.
Accessibility Testing
Accessibility Testing ensures that software applications and websites are usable by people with disabilities. The objective is to verify that the application is accessible to all users, including those with visual, auditory, motor, and cognitive impairments. Accessibility testing helps ensure compliance with accessibility standards and improves the overall user experience for all individuals.
What is Accessibility Testing?
Accessibility Testing is a process used to ensure that applications are usable by people with disabilities. This testing aims to identify potential accessibility issues for users with visual, auditory, motor, and cognitive impairments. It involves evaluating user interfaces and workflows to ensure they meet accessibility guidelines, such as the Web Content Accessibility Guidelines (WCAG) or Section 508 compliance.
Objectives of Accessibility Testing
- Ensure Inclusivity: Make sure the application is usable by people with disabilities, ensuring that all users can access and interact with the system effectively.
- Comply with Legal Standards: Ensure the software complies with accessibility laws and regulations, such as WCAG, ADA, and Section 508.
- Improve User Experience: Enhance the overall user experience by addressing accessibility barriers and providing equal access to all users.
- Increase Reach: By making the application accessible, organizations can expand their reach to a broader audience, including people with disabilities.
- Identify Usability Issues: Accessibility testing helps identify usability issues that may affect not only users with disabilities but also users with temporary impairments or in challenging environments.
Types of Accessibility Testing
- Manual Testing: Involves human testers evaluating an application using assistive technologies (screen readers, magnifiers, etc.) to identify accessibility issues.
- Automated Testing: Uses tools or scripts to scan the application for common accessibility issues, such as missing alt text or improper heading structure.
- User Testing with Disabled Individuals: Conducting tests with real users who have disabilities to observe how they interact with the application and identify potential issues.
Accessibility Testing Process
- Define Accessibility Requirements: Identify the accessibility standards to be followed (e.g., WCAG 2.1, Section 508, ADA) and the types of disabilities to be considered.
- Prepare the Test Environment: Set up the application and testing tools, including assistive technologies such as screen readers, keyboard-only navigation, and voice recognition tools.
- Perform Manual and Automated Tests: Test the application using both manual and automated testing techniques to identify issues related to accessibility guidelines.
- Analyze Results: Review the results of the tests to identify areas where the application fails to meet accessibility standards or where usability improvements can be made.
- Fix Accessibility Issues: Based on the findings, work with the development team to resolve issues and improve the accessibility of the application.
- Retest and Validate: After fixes are implemented, retest the application to ensure that the accessibility issues have been resolved and the application is now accessible.
Benefits of Accessibility Testing
- Increased Audience Reach: Ensures that your application is accessible to people with disabilities, helping you reach a wider audience.
- Legal Compliance: Helps ensure compliance with accessibility laws and regulations, reducing the risk of legal action or penalties.
- Improved User Experience: Enhances the overall user experience by removing barriers for users with disabilities and improving usability for all users.
- Enhanced Brand Reputation: Demonstrates social responsibility and commitment to inclusivity, leading to a positive brand image.
- Better SEO: Some accessibility features, such as proper heading structure and alt text, also contribute to better search engine optimization (SEO).
Challenges of Accessibility Testing
- Complexity of Guidelines: Accessibility guidelines can be complex, and different guidelines may apply to different platforms, making it challenging to ensure full compliance.
- Limited Resources: Testing with real users with disabilities may require additional resources, such as recruiting testers, and may not always be feasible.
- Tool Limitations: Automated testing tools may not catch all accessibility issues, especially those related to complex user interactions or custom elements.
- Time-Consuming Process: Accessibility testing can be time-consuming, especially when testing for a wide range of disabilities and ensuring compliance with multiple guidelines.
Tools for Accessibility Testing
- WAVE (Web Accessibility Evaluation Tool): A browser extension that provides visual feedback about the accessibility of web content.
- Axe: An automated accessibility testing tool that integrates with browsers and development workflows to detect accessibility issues.
- JAWs (Job Access With Speech): A screen reader used by visually impaired users to access content and test the accessibility of websites.
- NVDA (NonVisual Desktop Access): A free screen reader for Windows that helps evaluate how accessible an application is for visually impaired users.
- Color Oracle: A tool that simulates color blindness and helps test how applications appear to users with different types of color blindness.
Conclusion
Accessibility Testing is crucial to ensure that software applications and websites are usable by people with disabilities. By performing comprehensive accessibility testing, organizations can ensure that their applications are inclusive, comply with legal standards, and provide an improved user experience for all users, regardless of their abilities. It helps create a more equitable digital environment and enhances the reputation of the organization as a responsible and socially conscious entity.
Regression Testing
Regression Testing is a type of software testing conducted after changes such as bug fixes, enhancements, or configuration changes are made to an application. The goal is to ensure that the new changes have not affected the existing functionality of the software. It helps identify defects that may have been introduced unintentionally, ensuring the stability of the application.
What is Regression Testing?
Regression Testing refers to the process of re-running previously completed test cases to verify that recent changes to the software have not affected existing functionality. It is performed whenever there is a need to update or modify the application, such as fixing bugs or adding new features. The primary objective of regression testing is to catch potential issues early and ensure that the software remains functional after the changes.
Objectives of Regression Testing
- Ensure Stability: To verify that recent changes or enhancements have not negatively impacted the existing features of the software.
- Catch Unintended Side Effects: To detect any unintended consequences or regressions introduced by new changes, bug fixes, or enhancements.
- Maintain Quality: To ensure that the software continues to meet the required quality standards after changes are made.
- Verify Fixes: To confirm that the previously identified bugs have been fixed without introducing new problems.
- Boost Confidence: To ensure that the system works as expected and to increase stakeholder confidence in the stability of the software.
When to Perform Regression Testing?
Regression testing should be performed in the following scenarios:
- Bug Fixes: After fixing a defect, regression testing ensures that the fix has not broken any other part of the application.
- Feature Enhancements: When new features are added to the software, regression testing ensures that the existing functionality remains unaffected.
- Configuration Changes: After making changes to the system configuration, regression testing verifies that the changes don't impact other parts of the system.
- Version Updates: After updating the application to a new version or releasing a new build, regression testing is essential to ensure that the changes have not introduced new problems.
- Infrastructure Changes: When there are updates or changes to the underlying infrastructure or environment, regression testing helps to confirm that the software continues to function correctly.
Regression Testing Types
- Complete Regression Testing: Involves testing the entire application to ensure that no area has been affected by the recent changes. It is a comprehensive test but can be time-consuming.
- Selective Regression Testing: Focuses on testing only the parts of the application affected by the recent changes. This type of regression testing is more efficient but may miss issues in other parts of the software.
- Progressive Regression Testing: Conducted when new features are added to the application. It tests both the new and existing functionality to ensure that the new features do not cause problems.
- Partial Regression Testing: Tests only the affected areas where changes have occurred, ensuring that the modifications have not caused issues in those specific areas.
Regression Testing Process
- Identify Changes: Determine the changes made to the application, such as bug fixes, enhancements, or new features.
- Select Test Cases: Choose the appropriate test cases to be executed based on the changes made, including test cases for affected areas and other critical parts of the system.
- Execute Test Cases: Run the selected test cases on the application, either manually or using automated testing tools.
- Analyze Results: Review the test results to identify any issues or regressions caused by the recent changes. Investigate any failed test cases.
- Report Findings: Document the findings and report any issues discovered during the regression testing process to the development team for resolution.
- Retest and Validate: After the issues are fixed, re-execute the regression test cases to ensure that the fixes have been applied and that no other issues have been introduced.
Benefits of Regression Testing
- Ensures Software Stability: Helps maintain the stability of the software as new changes are applied, ensuring that no unintended side effects occur.
- Reduces the Risk of Defects: Helps identify and resolve defects caused by recent changes, reducing the risk of critical issues in production.
- Improves Confidence: Provides confidence to stakeholders that the software remains functional and meets quality standards after changes.
- Faster Time to Market: Automated regression testing helps speed up the testing process, enabling faster releases and updates.
- Cost-Effective: Detecting issues early in the development cycle helps avoid costly fixes later, saving time and resources.
Challenges of Regression Testing
- Time-Consuming: Depending on the scope of the changes, regression testing can be time-consuming, especially when the application is large and complex.
- Test Maintenance: As the application evolves, maintaining the regression test suite can be difficult, especially when new features are added or existing functionality is modified.
- Test Redundancy: Some test cases may become redundant over time, requiring regular updates to the test suite to ensure that only relevant tests are executed.
- Difficulty in Identifying Affected Areas: Sometimes, it may be challenging to determine which areas of the application are impacted by recent changes, leading to incomplete regression testing.
Tools for Regression Testing
- Selenium: A popular open-source tool for automating web applications, widely used for regression testing in web-based applications.
- JUnit: A widely used framework for unit testing in Java, but it can also be used for regression testing in Java-based applications.
- TestComplete: A comprehensive automated testing tool that supports regression testing for desktop, web, and mobile applications.
- QTP/UFT (Unified Functional Testing): A functional testing tool by Micro Focus that supports regression testing and can automate both desktop and web applications.
- LoadRunner: A tool used for performance and load testing, which can also be used for regression testing under load conditions.
Conclusion
Regression Testing is an essential part of the software development lifecycle that helps ensure the stability and quality of an application after changes are made. By identifying and resolving issues caused by recent modifications, regression testing helps maintain software reliability and reduces the risk of defects. Properly implemented regression testing increases confidence in the software and accelerates the release process, making it a critical practice for high-quality software delivery.
Smoke Testing
Smoke Testing, also known as "Build Verification Testing," is a preliminary level of testing conducted to check whether the basic functionalities of a software build are working as expected. It is typically performed after a new build is received to ensure that the software is stable enough for further, more detailed testing.
What is Smoke Testing?
Smoke Testing is a quick and shallow testing process that helps identify whether the core functionalities of the application are functioning properly. It acts as a quick check to verify that the software build is stable enough to proceed with more in-depth testing. The term "smoke test" is borrowed from hardware testing, where a device is powered on for the first time to check if it "smokes" (i.e., works) or fails immediately.
Objectives of Smoke Testing
- Verify Critical Functionality: Ensure that the most important features of the software are working correctly before proceeding with further testing.
- Identify Showstopper Issues: Detect any critical issues early in the testing phase that would prevent further detailed testing or invalidate the build.
- Save Time and Resources: By performing smoke testing, teams can quickly decide whether a build is worth spending time on for additional testing.
- Ensure Basic Stability: Confirm that the basic functionality and features of the application are working before moving on to more complex testing.
When to Perform Smoke Testing?
Smoke Testing is typically performed under the following circumstances:
- After a New Build: Every time a new build is delivered, smoke testing is performed to verify that the core features are functioning as expected.
- Before Detailed Testing: To ensure that the application is stable enough to proceed with more extensive testing, such as functional or regression testing.
- After Bug Fixes: When critical bugs have been fixed, smoke testing ensures that the fixes have not caused any issues with the basic functionality.
- After Configuration Changes: When there are changes to the system or environment configurations, smoke testing checks if the changes have impacted core features.
Smoke Testing Process
- Identify Core Features: Determine the key functionalities of the application that must be tested during smoke testing. These are usually the most critical features that users rely on.
- Perform Basic Test Cases: Execute the test cases that verify the functionality of the core features. These tests should be quick and cover critical paths in the application.
- Analyze Results: Review the results to check if the basic functionalities work as expected. If there are major failures, the build is rejected, and further testing is halted.
- Report Findings: Document any issues found during the smoke testing process and report them to the development team for resolution.
- Repeat Testing: Once the issues are fixed, smoke testing is repeated to ensure that the build is stable before further testing is conducted.
Benefits of Smoke Testing
- Quick Feedback: Smoke testing provides immediate feedback on the stability of a build, allowing teams to identify critical issues early in the process.
- Reduced Testing Time: By identifying major defects early, smoke testing helps save time by preventing wasted effort on detailed testing when the build is not stable.
- Increases Efficiency: Helps testers focus on valid builds that are ready for further testing, improving the overall efficiency of the testing process.
- Prevents Wasted Resources: Ensures that resources are not wasted on testing builds that are not stable or ready for detailed testing.
- Early Problem Detection: Quickly identifies showstopper defects that would prevent further testing, allowing the development team to fix issues before they escalate.
Challenges of Smoke Testing
- Limited Coverage: Smoke testing focuses only on the critical features, so it may not catch all possible issues in the application. It does not replace comprehensive testing.
- Shallow Testing: The tests performed during smoke testing are usually shallow and do not go deep into the application's functionality, which means it may miss non-critical but important issues.
- Needs Continuous Maintenance: The core functionalities may change as the application evolves, requiring the smoke test suite to be maintained and updated accordingly.
- Cannot Detect Complex Issues: Since smoke testing only verifies basic functionality, it is not effective for detecting complex, business logic-related issues.
Tools for Smoke Testing
Smoke testing can be performed manually or with the help of automation tools. Some common tools used for smoke testing include:
- Selenium: Selenium can be used to automate smoke tests for web applications, allowing teams to quickly verify critical functionalities.
- JUnit: JUnit is a popular testing framework for Java-based applications that can be used to automate smoke testing of critical features.
- TestComplete: TestComplete is an automated testing tool that can be used to perform smoke testing for desktop, web, and mobile applications.
- Ranorex: Ranorex is an automation tool that can be used to run smoke tests for applications, especially in enterprise environments.
Conclusion
Smoke Testing is an essential practice that allows teams to verify the basic functionality of a software build before embarking on more extensive testing. While it does not replace detailed testing, it plays a crucial role in ensuring that only stable builds are tested further, saving time and resources in the development cycle. By performing smoke testing regularly, teams can quickly identify critical issues and maintain a smoother development process.
Sanity Testing
Sanity Testing is a type of software testing that is performed to verify that specific functionality or bug fixes are working as expected after a build or a change. It is a focused and narrow testing process conducted to ensure that the key functionalities are functioning correctly without going into deep testing.
What is Sanity Testing?
Sanity Testing is a subset of regression testing that is performed after receiving a new build or bug fix. The goal of sanity testing is to verify that the changes or new features introduced in the build are working as expected, and no major issues have been introduced in the application. It is typically performed when there are minor changes or fixes, and the testing scope is limited to those specific areas of the application.
Objectives of Sanity Testing
- Verify Bug Fixes: Ensure that the bug fixes or changes made to the application are working as expected.
- Check Core Functionality: Confirm that the specific functionality or feature impacted by the changes is functioning properly without any new issues.
- Ensure Stability: Verify that no major issues have been introduced into the software after the changes or bug fixes.
- Save Time: Quickly verify that the changes are correct without performing in-depth or broad regression testing.
When to Perform Sanity Testing?
Sanity Testing is typically performed under the following circumstances:
- After Bug Fixes: When developers fix a bug or issue, sanity testing ensures that the fix works and hasn't introduced new problems.
- After Minor Changes: When new features or changes are introduced that affect limited parts of the application, sanity testing checks if the changes are functioning correctly.
- Before Detailed Testing: Sanity testing is used to quickly determine whether the build is stable enough to proceed with detailed regression testing or more complex testing stages.
- During Early Stages of Testing: In the early stages of testing, sanity testing helps confirm that the application is stable enough to move forward with more thorough testing.
Sanity Testing Process
- Identify Changed Areas: Focus on the specific areas or functionality that has been modified or fixed in the build.
- Execute Test Cases: Execute test cases or perform manual tests that verify the correct functioning of the changes or new features.
- Verify Fixes: Confirm that the issues or bugs that prompted the changes have been resolved and that the system behaves as expected in those areas.
- Evaluate Results: If the sanity tests pass, the build is deemed stable for further testing. If they fail, the build is rejected for further investigation and fixes.
Benefits of Sanity Testing
- Quick Verification: Sanity testing allows teams to quickly verify that the changes or fixes made to the application are working as expected without performing extensive testing.
- Reduced Testing Time: Since sanity testing is focused on specific areas, it helps reduce the overall time spent on testing by focusing only on critical changes.
- Identifies Critical Issues Early: Sanity testing helps detect major issues early in the process, preventing unnecessary delays in the project.
- Helps Focus on Key Areas: It ensures that the most critical aspects of the application that were impacted by changes are functioning correctly before proceeding with more detailed testing.
Challenges of Sanity Testing
- Limited Scope: Sanity testing only focuses on specific changes or fixes, which means it cannot identify issues outside of those areas.
- Does Not Replace Other Testing Types: Sanity testing is not a substitute for detailed testing, such as regression or functional testing. It is only a quick check to verify basic functionality.
- Requires Expertise: Sanity testing requires experienced testers who can quickly identify the important areas to test and ensure that critical functionality is working correctly.
- Not Suitable for Major Changes: If the changes are widespread or affect the overall system significantly, a more comprehensive testing approach (like regression testing) is needed.
Tools for Sanity Testing
Sanity testing can be done manually or with the help of automated testing tools. Some common tools used for sanity testing include:
- Selenium: Selenium is a popular open-source tool for automating web application testing, which can be used for performing sanity testing on web applications.
- JUnit: JUnit is a widely used framework for Java applications that can be used to automate sanity testing of specific functionalities or features in the software.
- TestComplete: TestComplete is a powerful test automation tool that can be used to perform sanity testing for desktop, mobile, and web applications.
- Ranorex: Ranorex is an automation tool that allows users to perform sanity testing in an efficient manner, especially in enterprise-level applications.
Conclusion
Sanity Testing plays an essential role in the software testing process by quickly verifying that the changes made to the software work as expected and that no major issues have been introduced. While it is not a replacement for detailed testing, it helps save time and resources by identifying problems early and ensuring that builds are stable enough to proceed with further testing. By performing sanity testing regularly, teams can ensure that critical functionality is working properly before diving into more comprehensive testing stages.
Alpha Testing
Alpha Testing is an early phase of software testing that takes place in the development environment. It is performed by the internal development team or a specialized testing team to identify and fix bugs and issues before the software is released to a limited set of users in a beta phase.
What is Alpha Testing?
Alpha Testing is a type of acceptance testing that is conducted by the internal team of the organization (developers, QA engineers, etc.) before the software is made available to external users. The goal is to identify and fix issues in the software before releasing it to a larger audience. Alpha testing is typically performed in a controlled environment, usually in the development or staging environment, and is the first step in ensuring the software meets the required quality standards.
Objectives of Alpha Testing
- Identify Bugs Early: Alpha testing aims to detect critical bugs and issues in the software at an early stage of development, before releasing it to users.
- Ensure Quality: It helps ensure that the software meets the basic quality standards, functionality, and usability requirements.
- Test Stability: Alpha testing checks the stability of the software, ensuring that it functions as expected in different scenarios and configurations.
- Improve User Experience: By testing the software in a controlled environment, alpha testing helps improve the user experience by addressing usability issues early on.
When to Perform Alpha Testing?
Alpha Testing is typically performed under the following circumstances:
- Before Beta Testing: Alpha testing is performed before beta testing, as it helps identify and fix issues that could affect the software during beta testing.
- Before Product Release: It is carried out in the final stages of development to ensure that the product is stable and free from major issues before it is released to a larger audience.
- When New Features Are Added: Alpha testing is performed when new features or changes are introduced to verify their functionality and identify any issues that need to be addressed.
Alpha Testing Process
- Test Planning: The first step in alpha testing is to define the test plan, including the scope, test cases, and objectives to be achieved during the testing process.
- Test Execution: The internal testing team executes the planned test cases, focusing on core functionality, usability, and stability of the software.
- Defect Identification: During test execution, testers identify any defects, bugs, or issues and document them for the development team to fix.
- Issue Resolution: The development team addresses the identified issues, and the software is tested again to ensure the fixes are successful.
- Test Report: After the testing phase, a test report is created, summarizing the testing results, defects found, and the current status of the software.
Benefits of Alpha Testing
- Early Bug Detection: Alpha testing helps identify and fix bugs early in the development process, reducing the risk of defects in later stages.
- Improved Software Quality: It ensures that the software meets quality standards and that key functionality is working as expected.
- Cost Savings: By addressing issues early in the development phase, alpha testing helps reduce the cost of fixing defects later in the process.
- Enhances User Experience: Alpha testing helps improve the user experience by identifying usability issues early, which can be fixed before the software reaches beta testers.
Challenges of Alpha Testing
- Limited Feedback: Since alpha testing is performed by an internal team, the feedback may be limited and may not represent real-world user experiences.
- Resource-Intensive: Alpha testing requires significant time and resources to plan, execute, and address any issues found during the process.
- Potential Bias: Internal testers may have biases or assumptions about the software, which could affect the quality and accuracy of the testing.
- Not Comprehensive: Alpha testing focuses on identifying major issues and bugs, but it may not catch all the minor defects or edge cases that beta testing might uncover.
Tools for Alpha Testing
Alpha testing can be performed manually or with the help of automated tools. Some common tools used for alpha testing include:
- Selenium: Selenium is an open-source tool that can be used for automated testing of web applications, helping speed up the testing process during alpha testing.
- JUnit: JUnit is a popular testing framework for Java applications that can be used for unit and integration testing during the alpha phase.
- TestComplete: TestComplete is a powerful automated testing tool that can be used for functional, regression, and alpha testing of desktop, mobile, and web applications.
- Bug Tracking Tools: Tools such as Jira, Bugzilla, or Trello are used to track and manage the issues and defects identified during the alpha testing phase.
Conclusion
Alpha Testing plays a critical role in the software development process by ensuring that the software is free of major bugs and issues before it is released to a wider audience. It is performed by internal teams, usually in the later stages of development, to assess the software's stability, functionality, and overall quality. Alpha testing helps detect defects early, improve the user experience, and reduce the risk of costly issues in the beta and production phases. By performing effective alpha testing, teams can ensure that the software is ready for the next stage of testing and eventually for a successful release.
Beta Testing
Beta Testing is the second phase of software testing, performed after Alpha Testing. It involves releasing the software to a selected group of external users (beta testers) who test the product in a real-world environment. The goal of beta testing is to gather feedback from actual users and identify any remaining bugs or issues before the final release.
What is Beta Testing?
Beta Testing is a type of user acceptance testing that occurs after alpha testing. During this phase, the software is made available to a limited audience outside the development team, allowing them to use the software in real-world conditions. Beta testers report bugs, issues, and feedback that are then addressed by the development team before the product is released to the general public. This phase allows developers to understand how the software performs under normal user conditions and identify any issues that weren't discovered during alpha testing.
Objectives of Beta Testing
- Gather Feedback: Beta testing helps gather feedback from real users to ensure the software meets their needs and expectations.
- Identify Remaining Bugs: It helps identify any remaining bugs, issues, or defects that were not found during alpha testing.
- Test Usability: Beta testing evaluates the usability and user experience of the software to ensure it is intuitive and user-friendly.
- Validate Functionality: It validates whether the software performs as intended in a real-world environment with a diverse set of users.
When to Perform Beta Testing?
Beta Testing typically occurs after Alpha Testing, when the software is stable enough to be tested by external users. It is usually performed when:
- The software is feature-complete: Most of the core features have been implemented, and the software is stable enough for real-world testing.
- Critical bugs have been fixed: Major issues identified during alpha testing have been resolved, and the focus shifts to gathering user feedback.
- The software is ready for feedback: The product is ready to be tested by real users to gather insights about its performance and usability.
Beta Testing Process
- Planning: The first step in beta testing is to define the testing objectives, recruit beta testers, and create a testing plan that outlines the scope, feedback collection process, and tools to be used.
- Beta Tester Recruitment: Beta testers are recruited from various user groups, including customers, volunteers, or selected individuals who match the target audience for the software.
- Beta Test Execution: Testers begin using the software in real-world conditions, performing typical tasks and providing feedback on their experience.
- Issue Reporting: Beta testers report bugs, issues, and suggestions for improvements through bug tracking tools or feedback forms.
- Feedback Analysis: The development team reviews the feedback, prioritizes issues, and fixes bugs based on the severity and importance of the reported problems.
- Final Release: After the issues have been addressed, the software is finalized and released to the general public.
Benefits of Beta Testing
- Real-World Feedback: Beta testing provides valuable feedback from actual users, which helps improve the software's quality and usability.
- Identifying Bugs in Real-World Conditions: Beta testers can uncover issues that may not have been found during alpha testing or in a controlled testing environment.
- Improved User Experience: The feedback gathered during beta testing helps improve the user interface and overall experience, making the software more user-friendly.
- Increased Confidence Before Launch: Beta testing helps the development team feel more confident about releasing the software to a broader audience, knowing that it has been tested in diverse environments.
Challenges of Beta Testing
- Limited Control: Since beta testers are external users, there is limited control over how they use the software, which can lead to inconsistent feedback.
- Inconsistent Testing Environments: Beta testers may use different hardware, operating systems, or network conditions, which can affect the consistency of the test results.
- Unpredictable Bugs: Bugs that arise during beta testing may be difficult to replicate in a controlled environment, making it challenging to fix them.
- Feedback Overload: With a large number of testers, sorting through and prioritizing feedback can become overwhelming for the development team.
Tools for Beta Testing
There are several tools available to help manage and streamline beta testing, including:
- TestFlight: A popular tool for iOS beta testing that allows developers to distribute pre-release versions of their apps to testers and gather feedback.
- BetaTesting: A platform that connects companies with beta testers to conduct feedback-driven testing for web and mobile applications.
- Bugzilla: A widely used bug tracking tool that helps developers manage and track reported bugs from beta testers.
- Jira: A project management tool that can be used to track beta testing progress, manage feedback, and prioritize issues for resolution.
Conclusion
Beta Testing is an essential phase of the software development life cycle, as it allows the development team to gather valuable feedback from real users, identify remaining bugs, and improve the software before its public release. By testing the software in real-world conditions, beta testing helps ensure that the product is ready for general availability, providing a better experience for end users. While it comes with its own challenges, such as managing user feedback and dealing with unpredictable bugs, beta testing ultimately plays a crucial role in delivering a high-quality product to users.
White-Box Testing
White-Box Testing, also known as Clear-Box Testing, Structural Testing, or Glass-Box Testing, is a type of software testing where the tester has access to the internal structure, design, and implementation of the software. It focuses on testing the internal workings of an application rather than its functionality from the user's perspective. The goal is to ensure that the code is working as expected and to identify potential issues in the internal structure of the software.
What is White-Box Testing?
In White-Box Testing, the tester is aware of the internal architecture of the software, including the code, logic, and algorithms. This type of testing is performed from the developer's perspective, where the tester validates the flow of inputs through the software, checks the logic of the code, and ensures that the code behaves as expected under various scenarios. White-box testing is typically performed at the unit testing level but can also be applied to other testing phases, such as integration and system testing.
Objectives of White-Box Testing
- Ensure Code Quality: White-box testing helps ensure the quality of the code by detecting errors, bugs, and inefficiencies in the program’s internal logic.
- Improve Code Coverage: It aims to achieve high code coverage by testing all possible paths, branches, and conditions in the code.
- Identify Security Vulnerabilities: It helps identify potential security issues in the software’s code, such as data leaks, security loopholes, or code injection vulnerabilities.
- Optimize Code Performance: White-box testing can identify inefficient code segments that may negatively impact software performance.
Types of White-Box Testing
- Unit Testing: Focuses on testing individual units or components of the software. The goal is to verify that each unit of code functions as expected in isolation.
- Integration Testing: Tests the interaction between integrated units or modules to ensure they work together as expected.
- Code Coverage Analysis: A process of analyzing the code to ensure that all paths, branches, and conditions are tested at least once to maximize code coverage.
- Path Testing: Involves testing all the possible paths in the code to ensure that each path is executed and behaves as expected.
- Branch Testing: Focuses on testing each possible branch (decision points) in the code to ensure that all branches are covered.
White-Box Testing Techniques
- Statement Coverage: Ensures that each statement in the code is executed at least once during testing.
- Branch Coverage: Ensures that every possible branch (if-else conditions) in the program is tested.
- Path Coverage: Ensures that all possible paths through the program’s control flow are tested, including combinations of branches.
- Condition Coverage: Ensures that each condition in decision statements (such as if and while) is evaluated both to true and false.
- Loop Coverage: Ensures that loops in the code (such as for, while, or do-while loops) are tested for different numbers of iterations, including edge cases (0, 1, and maximum iterations).
Advantages of White-Box Testing
- Thorough Testing: White-box testing provides thorough testing of the internal logic of the software, which helps identify hidden errors and bugs that may not be detectable through black-box testing.
- Early Detection of Bugs: Since white-box testing is performed at the code level, bugs can be identified and fixed early in the development process, reducing the risk of defects in later stages.
- Improved Code Quality: By testing the internal workings of the software, white-box testing helps improve the overall quality of the code, making it more efficient, secure, and maintainable.
- Code Optimization: White-box testing can uncover areas of the code that are inefficient, enabling developers to optimize the code for better performance.
Disadvantages of White-Box Testing
- Requires Technical Expertise: White-box testing requires testers to have a deep understanding of the code, algorithms, and internal structure, which may not be feasible for all testers.
- Time-Consuming: Thorough white-box testing can be time-consuming, especially for complex applications with a large codebase, as all code paths need to be tested.
- Limited Focus on Functionality: White-box testing focuses on the code and internal structure, rather than the software’s functionality from the user’s perspective. This means it might miss functional defects.
Tools for White-Box Testing
There are several tools available to assist with white-box testing:
- JUnit: A widely used testing framework for Java, primarily used for unit testing of individual code units or methods.
- JUnit: Used for testing code in Java applications. It is also used for test-driven development (TDD) to verify that individual units of code function as expected.
- PyTest: A testing framework for Python that supports unit testing and integration testing and provides powerful features such as fixtures and assertions.
- SonarQube: A platform for continuous inspection of code quality that provides static code analysis to detect bugs, security vulnerabilities, and code smells.
- JaCoCo: A code coverage library for Java that helps measure the percentage of code covered by tests.
Conclusion
White-Box Testing is an essential testing technique for ensuring the internal quality of software. It provides a deep dive into the internal structure, logic, and performance of the software. By testing the code at a granular level, white-box testing helps identify hidden issues, optimize code, and improve software quality. While it requires technical expertise and can be time-consuming, it is a valuable tool for developers and testers to ensure robust and efficient software. It works best when combined with black-box testing to provide comprehensive test coverage from both internal and external perspectives.
Black-Box Testing
Black-Box Testing is a type of software testing where the internal structure, design, and implementation of the software being tested are not known to the tester. The tester focuses on testing the functionality of the software by providing inputs and checking for the expected outputs, without any knowledge of the internal workings of the software. It is also referred to as functional testing or behavioral testing.
What is Black-Box Testing?
In Black-Box Testing, the tester is only concerned with the inputs and expected outputs of the system. The tester does not have access to the internal code or logic, and the testing is done based on the software’s requirements and specifications. The primary goal of black-box testing is to verify that the software functions as expected and meets the user's needs, without getting involved with the internal code structure.
Objectives of Black-Box Testing
- Verify Functionality: The main objective is to verify whether the software functions as expected and meets the specified requirements.
- Identify Defects: Black-box testing helps identify defects related to incorrect functionality, missing features, and discrepancies between the expected and actual outputs.
- Ensure Usability: It ensures the system behaves properly from the user's perspective and is user-friendly.
- Validate Compliance: It validates whether the software complies with the defined specifications, regulations, and standards.
Types of Black-Box Testing
- Functional Testing: Focuses on verifying that the software performs the functions it is intended to perform, based on the requirements and specifications.
- Non-Functional Testing: Tests the non-functional aspects of the system, such as performance, security, and usability, to ensure the software meets quality standards.
- Regression Testing: Ensures that new changes or updates to the software do not negatively impact the existing functionality.
- Acceptance Testing: Verifies whether the software meets the business requirements and is ready for deployment or delivery to the client.
- Smoke Testing: A preliminary test to check whether the most critical functionalities of the software are working as expected.
- Sanity Testing: A quick check to ensure that the software is working correctly after a change or bug fix.
Black-Box Testing Techniques
- Equivalence Partitioning: Divides the input data into equivalent partitions to reduce the number of test cases, ensuring each partition is tested to represent a group of similar inputs.
- Boundary Value Analysis: Focuses on testing the values at the boundaries of input ranges to identify defects that may occur at the edge of valid input ranges.
- Decision Table Testing: Uses decision tables to represent the different combinations of inputs and outputs, ensuring that each combination is tested.
- State Transition Testing: Involves testing the system’s behavior based on different states of the application, including transitions between states triggered by events.
- Use Case Testing: Focuses on testing the functionality of the system based on use cases, which describe how users interact with the system to achieve a goal.
Advantages of Black-Box Testing
- Tester Independence: Since the tester does not need to know the internal workings of the software, black-box testing can be performed by testers who are not familiar with the codebase.
- Real-World Simulation: Black-box testing simulates the user's perspective and interaction with the software, ensuring that the software meets the user’s expectations.
- Effective for Complex Systems: It is effective for testing complex systems where the internal logic and design are too difficult to understand or not necessary for the tester to know.
- Comprehensive Coverage: Black-box testing can cover a wide range of test cases, including functional and non-functional aspects of the system.
Disadvantages of Black-Box Testing
- Limited Coverage of Internal Logic: Since the tester does not have access to the internal code, some defects related to the internal logic may go unnoticed.
- Lack of Code Optimization: Black-box testing does not focus on optimizing the internal code and may not identify inefficient or poorly written code.
- Redundant Test Cases: Without knowledge of the code, it is possible that redundant test cases may be created, leading to inefficiency in testing.
- Difficulty in Traceability: Since the tester does not have access to the code, it may be difficult to trace the cause of a defect to a specific part of the code.
Tools for Black-Box Testing
There are several tools available to assist with black-box testing:
- TestComplete: A popular automated testing tool that supports functional and regression testing for both desktop and web applications.
- Selenium: An open-source tool for automating web applications, which is widely used for testing web applications from a black-box perspective.
- JMeter: An open-source tool for load testing and performance testing, which is often used for black-box testing of web applications and services.
- LoadRunner: A performance testing tool used to simulate virtual users to test the performance and scalability of software applications.
- Postman: A popular tool for testing APIs, Postman allows testers to validate the functionality of APIs from a black-box perspective.
Conclusion
Black-Box Testing is an essential part of the software testing process, focusing on verifying that the software works as intended from the user's perspective. It allows testers to assess the functionality and performance of the system without delving into the internal code. Despite its limitations, such as not covering the internal logic and being prone to redundant tests, black-box testing is a valuable technique to ensure that the software meets the requirements and provides a positive user experience. It works best when combined with white-box testing to provide comprehensive test coverage for both internal and external aspects of the software.
Grey-Box Testing
Grey-Box Testing is a software testing methodology that combines elements of both Black-Box and White-Box Testing. It involves testing the application with some knowledge of the internal structure, design, and implementation, but not full access to the code. Grey-box testing is typically performed by testers who have partial knowledge of the internals of the system, such as an understanding of the architecture, databases, or network protocols, but do not have access to the full source code.
What is Grey-Box Testing?
In Grey-Box Testing, the tester has a limited understanding of the internal workings of the application, typically at the architectural level, without having access to the full codebase. The aim is to combine the advantages of both Black-Box and White-Box Testing to provide a more comprehensive testing approach. Grey-box testing bridges the gap between purely functional testing (Black-Box) and detailed code-level testing (White-Box), offering a balanced approach for effective testing.
Objectives of Grey-Box Testing
- Ensure Comprehensive Coverage: It aims to provide a balanced approach that ensures both the functionality and internal aspects of the system are tested effectively.
- Identify Integration Issues: By having partial access to the system’s internals, grey-box testing helps identify issues related to integration points, databases, or other internal components.
- Verify System Behavior: It ensures that the system behaves as expected under various scenarios, considering both functional and non-functional requirements.
- Improve Test Efficiency: With partial knowledge of the system’s internals, testers can focus on testing areas that are more likely to contain defects, making testing more efficient.
Types of Grey-Box Testing
- Authentication Testing: Involves testing the authentication and authorization mechanisms of the system with partial access to the application’s internal structure.
- Session Management Testing: Focuses on testing how sessions are handled within the application, ensuring that data is securely stored and managed.
- Interface Testing: Involves testing how different components or subsystems of the application interact with each other, ensuring that data flows correctly between them.
- Security Testing: While limited access to the internal code is given, testers can assess the security of the system, checking for vulnerabilities such as improper data handling, authentication issues, or security flaws.
- Data Flow Testing: Verifies that data flows through the system properly, identifying issues with data handling, transformations, or storage, while having partial access to the system's internal workings.
Advantages of Grey-Box Testing
- Balanced Approach: Combines the advantages of both Black-Box and White-Box Testing, providing a more comprehensive testing approach.
- Efficient Testing: With partial knowledge of the internal structure, testers can focus on critical parts of the application, making the testing process more efficient and reducing redundancy.
- Better Defect Detection: By understanding certain parts of the internal workings, testers can identify defects that might be missed in purely Black-Box Testing.
- Cost-Effective: As testers have partial access to the internal code, it may reduce the need for extensive code reviews, making it more cost-effective compared to White-Box Testing.
- Improved Security Testing: Grey-box testing allows testers to probe for security vulnerabilities using both external and internal perspectives, offering more comprehensive security testing.
Disadvantages of Grey-Box Testing
- Limited Knowledge: Since testers do not have full access to the internal code, they may miss defects that are hidden deep within the codebase.
- Requires Skilled Testers: Grey-box testing demands testers who have some knowledge of the system’s internal workings, which may require specialized skills and training.
- Partial Test Coverage: While it offers more comprehensive coverage than Black-Box Testing, it may still miss some areas of the system, especially those that require full access to the code.
- Increased Complexity: The combination of functional and internal testing can make it harder to manage and analyze test results, especially for large and complex systems.
Grey-Box Testing Techniques
- Access Control Testing: Ensures that only authorized users can access certain parts of the system, particularly those that involve sensitive or confidential information.
- Database Testing: Involves testing the database interactions of the application by understanding its structure and testing the flows of data between the application and the database.
- API Testing: Tests the interactions between various components of the application using APIs, ensuring that data is passed correctly between them.
- Boundary Value Analysis: Performs boundary value testing where the tester has partial access to understand how the system handles input values at the boundary of valid input ranges.
- State Transition Testing: Tests how the system transitions between different states based on internal logic and user actions, with partial knowledge of the system’s state management.
Tools for Grey-Box Testing
There are several tools that can be used for grey-box testing, depending on the aspect of the system being tested:
- Burp Suite: A powerful tool for security testing that is commonly used for grey-box testing to probe for vulnerabilities in web applications.
- Wireshark: A network protocol analyzer that helps testers examine data flow and network traffic within the system, useful for grey-box testing.
- Postman: A popular tool for API testing that can be used in grey-box testing to validate API interactions with partial knowledge of the internal structure.
- Selenium: An automation testing tool for web applications that can be used for grey-box testing by interacting with the application’s UI while having partial knowledge of its internal workings.
- JMeter: A tool for load and performance testing, which can be used for grey-box testing of web applications with partial knowledge of their architecture.
Conclusion
Grey-Box Testing is a useful testing approach that combines the benefits of Black-Box and White-Box Testing, offering a balanced and efficient way to test software. By having partial knowledge of the system’s internal workings, testers can focus on both functional and non-functional aspects of the system, ensuring comprehensive test coverage. While it has its limitations, such as partial test coverage and the need for skilled testers, grey-box testing is an effective way to identify defects and enhance the overall quality and security of a software application.
Mobile Testing
Mobile Testing refers to the process of testing mobile applications to ensure their functionality, usability, performance, and security across a wide variety of mobile devices, operating systems, and networks. Given the rapid evolution of mobile technologies and the diversity of devices in the market, mobile testing is crucial to deliver high-quality applications that offer seamless user experiences.
What is Mobile Testing?
Mobile Testing involves testing mobile applications (native, web, or hybrid) to verify that they work as expected across different mobile platforms and devices. Mobile applications are tested for functionality, usability, performance, security, and compatibility. Mobile testing ensures that the application delivers the intended user experience and meets the required quality standards.
Types of Mobile Testing
- Functional Testing: Verifies that the mobile application functions as intended, ensuring that all features work correctly.
- Usability Testing: Focuses on evaluating the user experience (UX) and the app’s ease of use on mobile devices.
- Performance Testing: Assesses the mobile app’s performance under various conditions, such as network speed, device memory, and CPU usage.
- Security Testing: Ensures that the mobile application is free from vulnerabilities and that sensitive data is protected.
- Compatibility Testing: Ensures that the app works across different devices, screen sizes, operating systems (iOS, Android), and network environments.
- Interruption Testing: Involves testing how the mobile application behaves when interrupted (e.g., incoming calls, message notifications, low battery, etc.).
- Localization Testing: Ensures that the mobile application’s content, language, and visuals are appropriately adapted to different regions and cultures.
Objectives of Mobile Testing
- Verify Functionality: Ensures that the mobile app performs all its intended functions accurately on different mobile devices.
- Ensure Usability: Confirms that the app is easy to use, with an intuitive interface and smooth navigation.
- Optimize Performance: Ensures the app performs well under different conditions, such as varying network speeds and device configurations.
- Enhance Compatibility: Ensures the app works across a wide range of mobile devices, screen sizes, OS versions, and network environments.
- Ensure Security: Confirms that the app is secure and does not expose sensitive user data to potential threats.
Mobile Testing Challenges
- Diverse Devices and OS Versions: The wide variety of mobile devices, operating system versions, and screen sizes makes it difficult to ensure compatibility across all configurations.
- Network Variability: Mobile apps often need to perform under varying network conditions, and testing under these circumstances can be challenging.
- Battery Consumption: Some mobile apps may consume excessive battery, which can impact user experience, requiring careful performance testing.
- Frequent OS and Hardware Updates: Mobile devices and operating systems are frequently updated, making it necessary to test the app with every new release.
- Security Concerns: Mobile apps often store sensitive data, and ensuring their security can be complex due to various attack vectors targeting mobile platforms.
Mobile Testing Approaches
- Manual Testing: Testing is done manually on real devices or simulators/emulators to check the app’s functionality, usability, and compatibility.
- Automated Testing: Uses automated tools to execute predefined test scripts, especially for regression testing, to ensure the app’s stability across releases.
Mobile Testing Tools
There are several tools available for mobile testing, each serving different testing needs:
- Appium: An open-source tool for automating mobile applications for both Android and iOS platforms.
- Selendroid: A test automation framework that supports Android devices for mobile app testing.
- Robot Framework: A generic test automation framework that is capable of testing mobile applications with Appium and other tools.
- Espresso: A popular testing framework for Android apps, focusing on UI testing.
- XCUITest: A testing framework for iOS applications, used to test the user interface of iOS apps.
- BrowserStack: A cloud-based testing platform that allows you to test mobile apps on real devices in different environments.
- TestComplete: A test automation tool that supports mobile app testing with both Android and iOS platforms.
- JMeter: Primarily used for performance testing, JMeter can also be used for mobile app performance testing under varying network conditions.
Best Practices for Mobile Testing
- Test on Real Devices: Always test the app on real devices in addition to simulators/emulators for more accurate testing results.
- Ensure Cross-Device Compatibility: Test the app across multiple devices with different screen sizes, OS versions, and hardware configurations.
- Focus on UX/UI: Prioritize usability testing to ensure the app is intuitive and provides a smooth user experience.
- Optimize App Performance: Ensure that the app performs well under different network conditions, with low battery consumption and minimal memory usage.
- Security Testing: Implement thorough security testing to prevent vulnerabilities, such as data leaks or unauthorized access.
- Perform Regression Testing: After each update or release, make sure that the new changes don’t break existing functionality by performing regression tests.
Mobile Testing Challenges
- Device Fragmentation: The varying screen sizes, hardware configurations, and operating systems make testing on mobile devices more complex.
- Network Conditions: Mobile apps must work efficiently under varying network conditions, from high-speed Wi-Fi to low-bandwidth mobile data connections.
- Security Risks: Mobile apps are often used to store sensitive user information, making them prime targets for attackers. Security testing is crucial.
Conclusion
Mobile testing is crucial to ensure that mobile applications work seamlessly across different devices, operating systems, and network conditions. By focusing on functionality, performance, security, and compatibility, mobile testing helps developers deliver high-quality applications that provide a great user experience. With the increasing variety of mobile devices and platforms, testing mobile applications has become more complex, requiring a combination of manual and automated testing approaches to ensure that the app performs well under different scenarios.
API Testing
API Testing involves testing the Application Programming Interfaces (APIs) to ensure that they function as expected, are secure, and interact properly with other systems and applications. APIs are crucial for enabling communication between different software components, and testing them ensures that data is correctly passed between systems and that the functionality of the API is correct.
What is API Testing?
API testing is a type of software testing that focuses on testing the APIs directly, rather than testing the user interface (UI). It involves sending requests to an API and verifying the response to ensure that the API behaves as expected. This includes checking for correct data responses, proper error handling, security, and performance under load.
Objectives of API Testing
- Verify API Functionality: Ensure that the API performs all the intended operations, such as creating, reading, updating, and deleting data (CRUD operations).
- Ensure Data Integrity: Check that the API returns the correct and expected data in response to requests.
- Check Security: Test the API for security vulnerabilities and ensure proper authentication and authorization mechanisms are in place.
- Verify Error Handling: Ensure that the API handles errors gracefully and returns proper error codes and messages in case of invalid requests.
- Validate Performance: Assess the performance of the API, including response times and load handling.
Types of API Testing
- Functional Testing: Ensures that the API performs the expected operations correctly and returns the correct responses.
- Performance Testing: Tests how well the API performs under different conditions, such as high traffic or load, and evaluates response times.
- Security Testing: Ensures that the API is secure from common vulnerabilities such as unauthorized access, data breaches, and input validation issues.
- Reliability Testing: Verifies that the API functions reliably over extended periods and under different conditions, such as high stress or low bandwidth.
- Load Testing: Assesses the ability of the API to handle a large number of requests or high volumes of data.
- Usability Testing: Ensures that the API is easy to use and well-documented for developers who will consume the API.
- Compatibility Testing: Ensures that the API works with different systems, platforms, and versions of the software.
API Testing Tools
There are several tools available for API testing, each serving different testing needs. Some popular API testing tools include:
- Postman: A popular tool used for testing APIs manually. Postman allows you to create and send API requests, analyze responses, and automate testing.
- SoapUI: A tool for testing SOAP and REST APIs. It allows for functional testing, security testing, and performance testing.
- RestAssured: A Java-based library that simplifies testing REST APIs. It supports both JSON and XML-based APIs.
- JMeter: A performance testing tool that can be used to test the load and stress capabilities of APIs.
- Newman: A command-line collection runner for Postman, allowing you to automate API tests in continuous integration pipelines.
- Swagger: An open-source framework for designing, building, and documenting APIs. It also offers testing capabilities using Swagger UI and Swagger Codegen.
- Karate: A framework that simplifies API testing by allowing you to test APIs with a simple syntax. It supports BDD (Behavior Driven Development).
- RestClient: A plugin for testing RESTful APIs within a browser, often used for quick and simple tests.
API Testing Best Practices
- Test All Endpoints: Ensure that all endpoints of the API are tested for functionality and performance. This includes checking for both valid and invalid requests.
- Use Automation: Automate repetitive tests to save time and ensure consistency across multiple test runs. Use tools like Postman, RestAssured, or Newman for this.
- Check for Correct Status Codes: Ensure that the API returns the correct HTTP status codes for different scenarios (e.g., 200 for success, 404 for not found, 500 for server errors).
- Validate Response Data: Verify that the response from the API matches the expected output and that data is returned in the correct format (JSON, XML, etc.).
- Security Considerations: Always perform security testing to validate that the API is protected from common attacks like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Performance Testing: Evaluate the API's performance under load and stress conditions to ensure it can handle high volumes of traffic and data.
- Version Control: Ensure that the API supports versioning to handle changes in functionality and backward compatibility.
Challenges in API Testing
- Complex API Workflows: APIs may involve complex workflows with multiple dependencies, making it challenging to test all possible scenarios.
- Authentication and Authorization: Many APIs require secure authentication mechanisms, such as OAuth, which can make testing more complicated.
- Dynamic Data Handling: APIs may rely on dynamic data such as timestamps or tokens, which can change with each request, making it more difficult to test consistently.
- Third-Party Integrations: Many APIs interact with third-party systems, which can introduce external dependencies and cause issues during testing.
- Error Handling: Ensuring that the API responds correctly to errors (e.g., bad requests, timeouts) and handles them gracefully can be a challenging aspect of testing.
Conclusion
API testing is an essential practice for ensuring that APIs function as expected, are secure, perform well, and provide the correct data to users or other systems. Effective API testing helps identify issues early in the development cycle, ensuring better quality, reliability, and security for the API and the application it supports. By using a combination of manual and automated testing approaches, testers can ensure that APIs are thoroughly tested and meet business and technical requirements.
Static Testing vs. Dynamic Testing
Software testing can be broadly classified into two types: Static Testing and Dynamic Testing. Both play an essential role in ensuring the quality and functionality of software, but they differ in approach, techniques, and timing. Let's explore the differences between these two types of testing.
What is Static Testing?
Static testing involves reviewing and inspecting software artifacts (such as code, documentation, and design) without executing the program. It is a form of verification that focuses on finding errors or issues in the early stages of development, before the software is run. Static testing typically involves code reviews, walkthroughs, inspections, and static analysis tools.
Types of Static Testing
- Code Reviews: Involves manually reviewing the code to find issues or potential improvements.
- Walkthroughs: A developer or tester walks through the code or design to identify issues or discuss implementation details.
- Inspections: A formal process where a group of peers evaluates the code or documentation to identify defects.
- Static Analysis Tools: Tools that analyze the code for potential errors, security vulnerabilities, or non-compliance with coding standards.
Advantages of Static Testing
- Early Detection of Errors: Since static testing occurs before execution, it helps detect errors early in the development process, reducing the cost of fixing defects.
- Improves Code Quality: Regular code reviews and inspections lead to cleaner, more maintainable code.
- Faster Feedback: Static testing can be done without having to wait for the system to be completely developed, providing quicker feedback to developers.
- No Need for Execution: Static testing does not require the system to be executed, making it useful for reviewing code early on or in case of incomplete functionality.
What is Dynamic Testing?
Dynamic testing involves executing the software to validate its functionality and behavior in real-time. It checks whether the software performs as expected under different conditions. Dynamic testing typically involves running test cases, functional testing, performance testing, and security testing, among others.
Types of Dynamic Testing
- Functional Testing: Ensures that the software behaves as expected according to the functional requirements.
- Performance Testing: Evaluates the system's behavior under load, including response times, stability, and scalability.
- Security Testing: Tests the application for vulnerabilities, data protection, and potential security risks.
- Integration Testing: Validates the interaction between different modules and components of the system.
- System Testing: Tests the complete system to ensure it meets the specified requirements.
Advantages of Dynamic Testing
- Real-Time Execution: Dynamic testing tests the actual functionality of the software by running it and simulating real-world conditions.
- Validation of Behavior: Unlike static testing, dynamic testing validates the software's behavior and ensures that it meets the user requirements.
- Uncovering Runtime Issues: Dynamic testing helps identify issues that cannot be detected through static testing, such as runtime errors, memory leaks, and performance bottlenecks.
- Improves End-User Experience: By testing software in real-time, dynamic testing helps ensure that the application functions smoothly and delivers a good user experience.
Key Differences Between Static and Dynamic Testing
Aspect | Static Testing | Dynamic Testing |
---|---|---|
Definition | Testing without executing the code or system (reviewing documentation, code, etc.). | Testing by executing the software and validating its behavior in real-time. |
Objective | Detecting defects early in the development process (e.g., code quality, design flaws, etc.). | Validating the software's functionality, performance, and behavior under real conditions. |
Execution | No execution of the software is required. | Execution of the software is required to test its behavior. |
Techniques | Code reviews, walkthroughs, inspections, static analysis tools. | Test cases, functional testing, performance testing, security testing, etc. |
Timing | Occurs early in the software development life cycle, before or during the development process. | Occurs later in the development cycle, after the software has been built and is ready for testing. |
Examples | Code reviews, walkthroughs, design inspections. | Unit testing, integration testing, system testing, user acceptance testing. |
Tools | Static analysis tools (e.g., SonarQube, Checkstyle). | Test automation tools (e.g., Selenium, JUnit, LoadRunner, JMeter). |
When to Use Static Testing vs. Dynamic Testing?
Both static and dynamic testing should be used together for comprehensive quality assurance. Static testing is best for early-stage defect detection, reviewing code quality, and ensuring adherence to coding standards. Dynamic testing, on the other hand, is essential for verifying the software's actual behavior, performance, and security during runtime.
Conclusion
In summary, static testing and dynamic testing are both critical components of the software testing process. Static testing allows for early defect detection and code quality improvements, while dynamic testing ensures that the software performs as expected under real-world conditions. By utilizing both testing methods, teams can ensure that the software is both well-written and fully functional, ultimately leading to better quality and user satisfaction.
Black-Box Testing Techniques
Black-box testing focuses on testing the system from the user's perspective, where testers do not need to understand the internal workings or code of the application. The goal is to validate the software's functionality based on the given inputs and expected outputs. Below are some popular black-box testing techniques that help in identifying defects related to functionality, user requirements, and system behavior.
1. Equivalence Partitioning
Equivalence Partitioning is a technique used in black-box testing where input data is divided into distinct partitions or classes. Each class represents a set of valid or invalid input values that are treated the same by the software. The goal is to minimize the number of test cases by selecting one test case from each partition, assuming that if one value in the partition works, the rest will work as well.
For example, if a system accepts ages from 18 to 60, the input values can be divided into three partitions:
- Valid Age: 18 to 60
- Invalid Age (Too Low): Below 18
- Invalid Age (Too High): Above 60
One test case from each partition is selected, reducing the number of test cases needed for thorough testing.
2. Boundary Value Analysis
Boundary Value Analysis (BVA) is based on the premise that errors often occur at the boundary of input ranges. This technique involves testing the boundaries between valid and invalid input values to identify potential defects. Testers check both the boundary values and values just inside and outside the boundaries to ensure the system handles them correctly.
For example, if a system accepts an input age between 18 and 60, boundary value tests would include:
- Minimum Boundary: 18 (valid)
- Just Below Minimum Boundary: 17 (invalid)
- Just Above Maximum Boundary: 61 (invalid)
- Maximum Boundary: 60 (valid)
By testing these boundary values, testers can uncover defects that might arise when the system processes extreme input values.
3. Decision Table Testing
Decision Table Testing is a technique used to test systems with complex business rules or logical conditions. It involves creating a table that lists all possible combinations of inputs and the corresponding expected outputs. This technique is particularly useful when there are multiple conditions that affect the output, and the relationships between conditions are complex.
The decision table typically has four sections:
- Conditions: The various input conditions or variables.
- Actions: The outputs or actions based on the conditions.
- Rules: The different combinations of conditions (rows in the table).
- Result: The expected outcome for each rule combination.
This technique helps ensure that all possible scenarios are considered, leading to more comprehensive testing. Below is a simple example of a decision table for a login system:
Conditions | Action 1: Login | Action 2: Show Error |
---|---|---|
Password Correct, Username Correct | Login Successful | None |
Password Incorrect, Username Correct | None | Show "Incorrect Password" Error |
Password Correct, Username Incorrect | None | Show "Username Not Found" Error |
Password Incorrect, Username Incorrect | None | Show "Incorrect Credentials" Error |
4. State Transition Testing
State Transition Testing is a technique used to test the behavior of a system based on different states and transitions between those states. It is particularly useful for systems with multiple states that change based on user actions or system events. Testers create a state diagram to map out the different states, events, and transitions, and then develop test cases to ensure that the system behaves correctly when transitioning between states.
For example, consider a simple order processing system with the following states:
- Order Placed
- Order Confirmed
- Order Shipped
- Order Delivered
- Order Cancelled
Test cases can be designed to verify that the system correctly transitions between these states based on specific actions, such as placing an order, confirming it, shipping it, or canceling it.
Comparison of Black-Box Testing Techniques
Testing Technique | Description | When to Use |
---|---|---|
Equivalence Partitioning | Divides input data into valid and invalid partitions to reduce the number of test cases. | When the input domain is large, and you need to identify representative test cases. |
Boundary Value Analysis | Focuses on testing boundary values (edges) to find defects at the boundaries of input ranges. | When there are clearly defined input ranges or boundaries. |
Decision Table Testing | Uses a table to represent business rules and conditions for complex logic. | When the software requires multiple inputs with complex rules and conditions. |
State Transition Testing | Tests state changes based on events, ensuring the system behaves correctly in all states. | When testing systems with multiple states and transitions between them. |
Conclusion
Black-box testing techniques like Equivalence Partitioning, Boundary Value Analysis, Decision Table Testing, and State Transition Testing provide structured approaches for testing software functionality without knowing the internal workings of the system. By using these techniques, testers can systematically identify defects and ensure that the software behaves as expected across different scenarios and input combinations.
White-Box Testing Techniques
White-box testing, also known as structural or clear-box testing, focuses on testing the internal structures or workings of an application. It requires knowledge of the code and internal logic of the software to design test cases. Below are some common white-box testing techniques used to ensure that the code works as expected.
1. Statement Coverage
Statement Coverage is a white-box testing technique that ensures every statement in the code is executed at least once during testing. The goal is to check that all lines of code are covered by test cases, which helps identify areas where the code may not be executed under certain conditions.
For example, consider the following simple code snippet:
if (x > 0) {
y = 10;
} else {
y = 5;
}
To achieve 100% statement coverage, test cases need to be designed such that both the `if` and `else` blocks are executed. One test case should have `x > 0`, and another should have `x <= 0`.
2. Branch Coverage
Branch Coverage, also known as decision coverage, ensures that each possible branch or decision point in the code is tested at least once. In other words, all possible outcomes of every decision (like `if`, `else`, `for`, `while`, etc.) should be covered by test cases. This helps ensure that all paths are checked for correctness.
For the same code snippet:
if (x > 0) {
y = 10;
} else {
y = 5;
}
To achieve 100% branch coverage, the test cases should cover both branches (`if` and `else`). For example:
- Test case 1: `x > 0`, which will execute the `if` branch.
- Test case 2: `x <= 0`, which will execute the `else` branch.
3. Path Coverage
Path Coverage is an advanced white-box testing technique that aims to test all possible execution paths in the code. It is based on the premise that different combinations of decisions can lead to different paths, and all possible paths should be tested. Path coverage ensures that every unique path through the code is exercised, which helps uncover defects related to specific path combinations.
Consider a more complex code with nested conditions:
if (x > 0) {
if (y > 5) {
z = x + y;
} else {
z = x - y;
}
} else {
z = x * y;
}
In this example, there are multiple paths based on the values of `x` and `y`. To achieve 100% path coverage, you would need to design test cases that cover all possible paths through the code:
- Test case 1: `x > 0`, `y > 5` (Path 1)
- Test case 2: `x > 0`, `y <= 5` (Path 2)
- Test case 3: `x <= 0`, any `y` (Path 3)
Comparison of White-Box Testing Techniques
Testing Technique | Description | When to Use |
---|---|---|
Statement Coverage | Ensures that every statement in the code is executed at least once. | When you need to ensure basic code coverage and identify untested statements. |
Branch Coverage | Ensures that each possible branch or decision point is tested at least once. | When you need to verify that all possible decision outcomes (e.g., `if`, `else`) are covered. |
Path Coverage | Ensures that every unique path through the code is tested to cover all possible execution flows. | When you need to test all combinations of decisions and ensure comprehensive path coverage. |
Conclusion
White-box testing techniques like Statement Coverage, Branch Coverage, and Path Coverage help ensure that the code is thoroughly tested and all logical conditions are evaluated. By applying these techniques, testers can identify potential defects related to code logic, decision points, and execution paths, leading to a more robust and reliable software application.
Exploratory Testing Techniques
Exploratory testing is a hands-on, unscripted approach to software testing where testers actively explore the application to identify defects. Unlike traditional testing, where test cases are pre-written, exploratory testing allows testers to use their knowledge, experience, and intuition to test different aspects of the software. Below are some common exploratory testing techniques to make this process more effective.
1. Session-Based Testing
Session-based testing is one of the most popular techniques used in exploratory testing. It involves testing the software in time-boxed sessions, typically lasting 60-90 minutes, where the tester explores the application and focuses on specific areas during the session. After each session, the tester documents the findings, observations, and any defects discovered.
The process typically involves the following steps:
- Define the session objective (e.g., testing a specific feature or workflow).
- Test the application within the time-boxed session.
- Record the test results, including bugs, issues, and observations.
- Review the results and plan the next session.
This technique is particularly useful for uncovering new types of defects and for scenarios where scripted testing might be inadequate.
2. Charter-Based Testing
Charter-based testing is a technique where the tester is given a charter, which is a high-level mission or goal for the testing session. The charter defines the scope and focus areas of the test but leaves the actual exploration up to the tester. It helps testers stay focused while providing the flexibility to explore different aspects of the software.
For example, a charter might be: "Test the login functionality for both valid and invalid credentials and explore error messages." The tester can then explore different login scenarios without predefined steps, allowing creativity and problem-solving to uncover defects.
3. Risk-Based Testing
Risk-based testing is a technique that focuses on testing the most critical and high-risk areas of the software. Testers use their knowledge of the system to prioritize testing based on the likelihood and impact of potential defects. It involves identifying risk factors such as complex business logic, high-traffic features, or security vulnerabilities, and allocating more time and effort to testing those areas.
Risk-based testing helps ensure that the most important areas of the software are tested thoroughly while allowing less critical areas to be explored as time permits.
4. Mind Mapping
Mind mapping is a technique that helps testers organize their thoughts and ideas while exploring the application. It involves creating a visual representation of the areas of the software being tested and how they relate to each other. Testers use mind maps to identify key areas to focus on, outline testing scenarios, and track the progression of exploratory testing sessions.
Mind maps can help testers stay organized, spot areas that require additional attention, and generate new ideas for testing. They are particularly useful in complex applications with numerous features and interactions.
5. Pair Testing
Pair testing is a technique where two testers work together to explore the application. One tester is typically the "driver," responsible for interacting with the application, while the other is the "navigator," providing guidance, generating ideas, and spotting potential issues. This collaborative approach leads to a more thorough exploration of the software and often results in the discovery of defects that might have been missed during individual testing.
Pair testing encourages knowledge sharing, brainstorming, and collaboration, which can improve the effectiveness of the testing process.
6. Exploratory Testing with Tools
Although exploratory testing is primarily unscripted, testers can use various tools to enhance the process. Tools like screen recorders, log analyzers, or test automation scripts can help document the testing session, track test results, and capture logs or screenshots of any defects discovered.
For example, testers can use a screen recording tool to capture their interactions with the application, and then review the video to identify any issues. This can be particularly useful when testing complex scenarios or when working in a team to review the testing results later.
Comparison of Exploratory Testing Techniques
Testing Technique | Description | When to Use |
---|---|---|
Session-Based Testing | Time-boxed sessions focused on specific objectives, followed by documentation of findings. | When you need structured testing sessions with a clear objective but flexibility to explore. |
Charter-Based Testing | Testers are given a high-level objective (charter) and explore freely within it. | When you need focus on specific areas but want the tester to have creative freedom. |
Risk-Based Testing | Prioritizes testing based on the likelihood and impact of defects. | When you need to focus on high-risk or business-critical areas of the software. |
Mind Mapping | Visual representation of testing areas, helping testers organize and track progress. | When you need to explore complex applications and keep track of testing areas. |
Pair Testing | Two testers collaborate to explore the application, with one driving and the other navigating. | When you need collaboration and knowledge sharing between testers to improve effectiveness. |
Exploratory Testing with Tools | Using tools like screen recorders or log analyzers to enhance the exploratory testing process. | When you want to capture logs, screenshots, or videos of the testing process for later analysis. |
Conclusion
Exploratory testing techniques are highly effective in discovering defects that may be missed by traditional scripted testing. By using techniques like session-based testing, charter-based testing, and risk-based testing, testers can explore the software freely while focusing on critical areas. These techniques help uncover new issues, promote collaboration, and provide flexibility to testers, ensuring that software is thoroughly tested from different perspectives.
Writing Test Cases: Best Practices
Writing effective test cases is a crucial skill for any software tester. A well-written test case ensures that the software is thoroughly tested, and defects are identified early in the development process. Below are some best practices to follow when writing test cases:
1. Understand the Requirements
Before writing test cases, it is essential to have a clear understanding of the software requirements. This includes understanding the functionality, features, and user scenarios that the software is expected to support. Test cases should directly align with the requirements and cover all possible use cases.
Key points to consider:
- Review requirement documentation, user stories, or use cases.
- Clarify any ambiguities with stakeholders or developers.
- Ensure that your test cases cover both functional and non-functional requirements.
2. Be Clear and Concise
Test cases should be clear and easy to understand. Use simple and precise language to describe the test case steps and expected results. Avoid ambiguous terms that could cause confusion or misinterpretation.
Best practices include:
- Write test case descriptions in a straightforward manner.
- Avoid complex sentences or jargon.
- Ensure that the test steps are actionable and easy to follow.
3. Use Descriptive Test Case Titles
Each test case should have a descriptive title that clearly indicates its purpose and the functionality being tested. A good title helps testers and stakeholders quickly understand the focus of the test case without needing to read the entire case.
Test case titles should:
- Clearly represent the functionality being tested.
- Be brief but informative (e.g., "Verify login functionality with valid credentials").
- Use consistent naming conventions across all test cases.
4. Define Preconditions and Postconditions
Preconditions and postconditions are essential components of a test case. Preconditions define the setup or state that must be in place before the test case can be executed, while postconditions describe the expected state after test execution.
Follow these guidelines:
- Preconditions should specify any setup steps, data requirements, or environmental conditions.
- Postconditions should define the expected result, such as system state or data changes after test execution.
5. Define Expected Results Clearly
The expected result section of a test case should clearly describe the outcome that the tester anticipates. This helps determine whether the software behaves as expected. Clearly defined expected results make it easier to identify defects.
For example:
- Expected Result: "User is successfully logged in and redirected to the dashboard."
- Expected Result: "Error message 'Invalid credentials' is displayed when entering incorrect login details."
6. Keep Test Cases Reusable and Modular
Test cases should be reusable and modular, meaning that they should focus on testing one specific functionality at a time. This way, test cases can be reused in different scenarios, reducing redundancy and improving test suite maintainability.
To make test cases reusable:
- Write test cases that are independent and test a single feature.
- Avoid hardcoding values; use variables where possible.
- Focus on testing one condition or scenario per test case.
7. Organize and Categorize Test Cases
Organizing test cases into logical categories makes it easier to manage large test suites. Group test cases based on functionality, modules, or features to keep them well-structured and easy to navigate.
Test case categories can be based on:
- Functionality or feature (e.g., login, payment, registration).
- Type of testing (e.g., positive tests, negative tests, boundary tests).
- Priority (e.g., high priority, low priority).
8. Prioritize Test Cases
Not all test cases are equally important. Prioritize test cases based on factors such as critical functionality, user impact, and risk. High-priority test cases should be executed first to ensure that the most important aspects of the application are working correctly.
Test case prioritization can be based on:
- Risk and impact on the user experience.
- Frequency of use or criticality of the feature.
- Complexity or likelihood of defects in the specific functionality.
9. Maintain Test Case Version Control
Test cases may change over time due to changes in requirements, features, or bug fixes. Keeping track of test case versions ensures that you always have the latest version and that you can refer to or revert to previous versions if needed.
Follow these best practices:
- Use version numbers or revision history in test case documentation.
- Track changes to test cases with a clear log (e.g., "Test case updated to reflect the new UI design").
- Store test cases in a central repository or test management tool for easy access and version control.
10. Review and Update Test Cases Regularly
Test cases should be reviewed regularly to ensure they are up-to-date and relevant. Regular reviews help identify redundant, obsolete, or incomplete test cases and ensure that new test scenarios are added as the software evolves.
Consider conducting periodic reviews and updates based on:
- Changes in the application (e.g., new features or bug fixes).
- Feedback from testers and stakeholders.
- Lessons learned from previous testing cycles.
Conclusion
Writing effective test cases is a vital aspect of the software testing process. By following these best practices, testers can create robust, reliable, and reusable test cases that ensure comprehensive testing of the software. Clear, well-structured, and organized test cases lead to improved test coverage, better defect identification, and overall higher-quality software.
Test Plan and Test Strategy
A test plan and test strategy are two essential documents in the software testing process. They help define the scope, approach, resources, and schedule for testing activities. While both documents serve the same purpose of guiding the testing effort, they have different focuses and levels of detail.
1. What is a Test Plan?
A test plan is a detailed document that outlines the objectives, approach, resources, schedule, and scope of testing. It serves as a blueprint for the entire testing process, ensuring that the testing activities are organized, systematic, and aligned with the project goals.
Key components of a test plan include:
- Test Plan Identifier: A unique identifier for the test plan document.
- Test Objectives: The goals of the testing process, such as verifying that the system meets the specified requirements.
- Test Scope: The boundaries of the testing process, including which features and functionalities will be tested and which will not.
- Test Approach: The testing strategy and techniques that will be used (e.g., manual testing, automation, functional testing).
- Resource Requirements: The human, software, and hardware resources needed to conduct the tests.
- Test Environment: The hardware, software, network configuration, and tools required for testing.
- Test Schedule: The timeline for testing activities, including milestones and deadlines.
- Test Deliverables: The documents and artifacts that will be produced during the testing phase (e.g., test cases, test reports, bug reports).
- Risk and Mitigation: Potential risks that could impact the testing process, and the strategies to mitigate them.
- Exit Criteria: The conditions that must be met to consider the testing phase complete (e.g., all critical defects have been resolved).
2. What is a Test Strategy?
A test strategy is a high-level document that outlines the overall approach and vision for testing. It focuses on the testing goals, scope, techniques, and methods to be used but does not go into the level of detail that a test plan does. The test strategy is typically created by senior management or the test lead and provides a broad overview of the testing process.
Key components of a test strategy include:
- Test Objectives: The primary goals of testing, such as ensuring software quality and meeting customer requirements.
- Test Scope: The features and functionalities that will be tested and those that will be excluded.
- Test Levels: The different levels of testing to be performed (e.g., unit testing, integration testing, system testing, user acceptance testing).
- Testing Types: The types of testing that will be used (e.g., functional testing, non-functional testing, regression testing, security testing).
- Testing Techniques: The techniques that will be employed (e.g., black-box testing, white-box testing, exploratory testing).
- Test Tools: The tools that will be used for automation, test management, and defect tracking (e.g., Selenium, Jira, Jenkins).
- Test Environment: The hardware, software, and network environment where testing will take place.
- Risk Management: The risks associated with testing and strategies for addressing them.
- Test Metrics: The metrics that will be used to measure the effectiveness of testing (e.g., test coverage, defect density, pass/fail rate).
3. Key Differences Between Test Plan and Test Strategy
While both the test plan and test strategy are used to define the testing process, they differ in scope, level of detail, and focus. Here's a comparison:
Aspect | Test Plan | Test Strategy |
---|---|---|
Level of Detail | Detailed and specific to the project | High-level and general for the organization |
Focus | Focuses on the specific project and its testing activities | Focuses on the overall approach for testing across projects |
Audience | Testers, developers, project managers, and stakeholders | Senior management, test leads, and stakeholders |
Content | Defines the test objectives, scope, schedule, resources, and deliverables | Outlines the overall testing approach, techniques, and tools |
Time of Creation | Created at the beginning of the testing phase | Created at the start of the project or testing phase |
4. Importance of Test Plan and Test Strategy
Both the test plan and test strategy play a crucial role in ensuring the success of the testing process:
- Test Plan: Provides detailed guidance on the testing process, resources, and schedule, ensuring that testing is organized and systematic.
- Test Strategy: Provides a high-level overview of the testing approach, ensuring that testing aligns with organizational goals and standards.
By having both documents in place, teams can ensure that testing efforts are well-coordinated, efficient, and effective in identifying defects and improving software quality.
Creating Test Scenarios and Test Data
Creating effective test scenarios and test data is crucial for ensuring thorough test coverage and detecting defects in software. Test scenarios are high-level descriptions of what needs to be tested, and test data refers to the inputs used during testing. Together, they form the foundation of a well-structured testing process.
1. What is a Test Scenario?
A test scenario is a high-level description of a specific functionality or behavior that needs to be tested within a system. It helps testers understand what needs to be validated, ensuring that all important aspects of the application are covered.
Test scenarios are typically derived from the requirements, use cases, and user stories. They represent the various ways in which the system could be used, and they help ensure that the application works as expected in different situations.
2. Importance of Test Scenarios
- Ensures Test Coverage: Test scenarios help identify the key functionalities to be tested, ensuring that critical paths are covered.
- Reduces Redundancy: By focusing on different use cases, test scenarios help reduce redundant test cases.
- Improves Test Efficiency: With clearly defined test scenarios, testers can avoid unnecessary tests and focus on what matters.
- Helps in Traceability: Test scenarios provide a clear link between requirements and testing activities, ensuring traceability throughout the testing lifecycle.
3. How to Create Test Scenarios?
Creating test scenarios involves the following steps:
- Understand Requirements: Gather information about system requirements, user stories, and use cases to understand the scope of testing.
- Identify Key Functionality: Identify the primary functionalities and features that need testing based on the requirements.
- Define Test Conditions: Determine the conditions under which the system should be tested. These include valid and invalid inputs, boundary conditions, etc.
- Write Test Scenarios: Write concise and clear test scenarios that describe the functionality to be tested. Each scenario should focus on a specific aspect of the application.
- Prioritize Scenarios: Prioritize the test scenarios based on criticality, risk, and usage frequency to ensure high-impact scenarios are tested first.
4. What is Test Data?
Test data refers to the set of inputs that are used during testing to validate the functionality of an application. The right test data ensures that the system behaves correctly in different situations and helps identify defects that could otherwise go unnoticed.
Test data can include:
- Valid Data: Data that meets the expected input criteria and helps verify that the system works as intended.
- Invalid Data: Data that is incorrect or doesn't meet the required format, used to test error handling and validation.
- Boundary Data: Data that tests the limits of the system (e.g., maximum values, minimum values).
- Null or Empty Data: Data that tests how the system handles missing or empty fields.
5. Importance of Test Data
- Validates System Functionality: Test data ensures that the system behaves as expected with correct inputs.
- Helps in Error Detection: Invalid data helps identify bugs related to input validation, error handling, and boundary conditions.
- Ensures Data Integrity: Boundary and edge-case test data helps validate that the system handles extreme values and edge cases properly.
- Improves Coverage: Using a variety of test data types ensures comprehensive test coverage, including valid, invalid, and boundary cases.
6. How to Create Test Data?
Creating test data involves the following steps:
- Understand Test Requirements: Based on test scenarios, identify the data required for each test case. This may include valid, invalid, boundary, and edge-case data.
- Identify Data Sources: Determine where the test data will come from (e.g., databases, spreadsheets, or generated manually).
- Generate Test Data: Create the test data based on the identified criteria. This can involve generating random data or using automated tools to create large sets of data.
- Ensure Realism: Ensure that the test data closely resembles real-world data to simulate realistic scenarios and uncover potential issues.
- Maintain Consistency: Make sure that the test data is consistent across different test cases and environments. This ensures that the results are reliable and reproducible.
7. Best Practices for Creating Test Scenarios and Test Data
- Clarity and Simplicity: Write clear and simple test scenarios to avoid confusion and ensure that they are easy to follow.
- Comprehensive Coverage: Ensure that the test scenarios cover all aspects of the system, including positive, negative, and edge cases.
- Reusability: Create test scenarios and test data that can be reused across different test cases and environments to save time and effort.
- Maintain Data Security: When using real-world data, ensure that it complies with data protection and privacy regulations (e.g., GDPR, HIPAA).
- Automate Test Data Generation: Use tools and scripts to automate the generation of test data, especially for large datasets or complex test cases.
8. Example: Test Scenario and Test Data
Here is an example of a test scenario and the corresponding test data:
Test Scenario
Scenario: Verify that the login functionality works with valid credentials.
Test Data
Field | Value |
---|---|
Username | testuser |
Password | Test@1234 |
Expected Result
The system should log in the user successfully and redirect them to the dashboard.
Test Metrics and Measurements
Test metrics and measurements are essential tools for tracking the progress, quality, and effectiveness of the testing process. They help ensure that testing is aligned with project goals and that the product meets its quality standards. By collecting and analyzing relevant data, teams can identify bottlenecks, areas for improvement, and potential risks early in the development cycle.
1. What are Test Metrics?
Test metrics are quantitative measures that provide insights into the efficiency, effectiveness, and quality of the testing process. They help teams make data-driven decisions and assess whether the testing efforts are on track. Test metrics can be applied at various stages of the software development lifecycle (SDLC) and testing phases.
2. Importance of Test Metrics
- Improves Test Efficiency: By tracking key metrics, teams can identify inefficiencies in the testing process and make improvements.
- Monitors Testing Progress: Test metrics help monitor the progress of testing activities and ensure timely completion of testing tasks.
- Measures Test Effectiveness: Metrics help evaluate the effectiveness of test cases and identify gaps in test coverage.
- Helps in Decision Making: Test metrics provide the necessary data for making informed decisions about the testing process and product quality.
- Ensures Quality Control: Metrics help ensure that the product meets the quality standards set by the project and stakeholders.
3. Common Test Metrics
There are several test metrics that are commonly used to measure the effectiveness and efficiency of the testing process. These metrics can be divided into different categories:
Test Coverage Metrics
Test coverage metrics help assess how much of the application has been tested and whether all functional areas have been covered.
- Code Coverage: Percentage of the code that is executed during testing. It includes metrics like statement coverage, branch coverage, and path coverage.
- Requirement Coverage: Measures the percentage of requirements that have been tested. It ensures that all requirements are validated against test cases.
- Test Case Coverage: Percentage of test cases executed during the testing phase. It ensures that all test cases are run and any defects are identified.
Defect Metrics
Defect metrics help track the number and severity of defects found during testing, providing insights into the quality of the software product.
- Defect Density: Number of defects per unit of size (e.g., per KLOC or per function point). It helps measure the quality of the code and identify problematic areas.
- Defect Severity: Measures the severity of defects based on their impact on the system. It helps prioritize defect resolution.
- Defect Discovery Rate: Rate at which defects are discovered during the testing cycle. It helps assess the effectiveness of the testing process.
- Defect Resolution Rate: Rate at which defects are fixed during the testing cycle. It helps assess how quickly the development team is addressing defects.
Test Execution Metrics
Test execution metrics track the progress of test execution and the completion of testing tasks.
- Test Case Pass Rate: Percentage of test cases that pass during testing. A high pass rate indicates that the software works as expected.
- Test Case Fail Rate: Percentage of test cases that fail during testing. A high fail rate suggests that the software has significant issues.
- Test Execution Time: Time taken to execute a test case or a set of test cases. It helps track the efficiency of the testing process.
- Tests Executed per Day: The number of test cases executed per day. It helps measure the testing throughput and productivity.
Test Efficiency Metrics
Test efficiency metrics focus on how effectively testing resources are used and whether testing activities are yielding valuable results.
- Test Efficiency: Ratio of defects found in testing to the total number of test cases executed. It helps measure how effective the testing process is in identifying defects.
- Test Return on Investment (ROI): Measures the value of the testing efforts in relation to the cost and resources spent on testing.
- Test Cost per Defect: Measures the cost of testing efforts to identify and resolve each defect. It helps assess the cost-effectiveness of the testing process.
4. Key Performance Indicators (KPIs) for Software Testing
Key Performance Indicators (KPIs) are important for tracking the performance of the software testing process. Some common KPIs for software testing include:
- Test Coverage: Percentage of the application or functionality covered by test cases.
- Defect Leakage: The number of defects that escape into production despite testing efforts.
- Test Execution Time: Time taken to execute a test case or a set of test cases.
- Defect Resolution Time: Time taken to resolve defects found during testing.
- Customer Satisfaction: The level of satisfaction of the end users with the quality and performance of the product.
5. How to Use Test Metrics?
Test metrics should be used to monitor and improve the testing process. Here are some ways to effectively use test metrics:
- Track Progress: Use metrics to track the progress of testing and ensure that it is on schedule.
- Assess Quality: Analyze defect metrics to evaluate the quality of the software and identify areas for improvement.
- Prioritize Testing: Use coverage metrics to prioritize testing activities, ensuring that critical features and requirements are tested first.
- Make Informed Decisions: Use test metrics to make data-driven decisions about test execution, defect resolution, and resource allocation.
- Report Status: Use metrics to report the status of testing to stakeholders and provide transparency into the testing process.
6. Challenges in Test Metrics
While test metrics are valuable, there are challenges associated with their implementation:
- Data Overload: Gathering too many metrics can overwhelm the team and make it difficult to focus on the most important data.
- Misinterpretation: Incorrect interpretation of metrics can lead to wrong conclusions and decision-making.
- Quality vs. Quantity: Focusing too much on quantitative metrics like test execution rate may overlook the quality of testing.
- Inconsistent Data: If the metrics are not tracked consistently, they may lead to inaccurate or unreliable insights.
7. Example: Defect Density Metric
Here's an example of how defect density is calculated:
Metric | Value |
---|---|
Number of defects | 50 defects |
Code size (KLOC) | 10 KLOC |
Defect Density | 50 defects / 10 KLOC = 5 defects per KLOC |
Defect Life Cycle: Identifying and Reporting Bugs
The Defect Life Cycle (also known as Bug Life Cycle) is the journey of a defect from its identification to its resolution and closure. It involves several stages, including reporting, fixing, verifying, and closing the defect. Properly managing defects helps ensure software quality and timely releases by addressing issues early in the development process.
1. What is the Defect Life Cycle?
The Defect Life Cycle is a systematic process that tracks the flow of a defect as it moves through different stages of identification, reporting, fixing, and closure. It helps teams efficiently manage defects and ensures that all defects are addressed before the software is released to the end user.
2. Stages of the Defect Life Cycle
The Defect Life Cycle consists of several stages, from defect identification to its resolution. Below are the common stages:
1. New
A defect is reported for the first time and is not yet reviewed by the development team. It is in the initial state where the defect is logged in the defect tracking system. The defect may be a new issue or could be the result of a missed test case.
2. Assigned
Once the defect is reviewed and acknowledged by the development team, it is assigned to a developer or relevant team member for investigation. The developer will assess the severity and root cause of the defect.
3. Open
In this stage, the developer starts working on the defect and attempts to fix it. The defect is actively being worked on and has not yet been resolved. The developer may analyze the code, isolate the issue, and identify possible solutions.
4. Fixed
Once the defect is resolved by the developer, the status is updated to "Fixed." At this stage, the fix is implemented, and the code changes have been applied to the product. The defect is considered to be fixed from the development side, but further verification is required.
5. Retested
After the defect fix is implemented, the tester retests the application to ensure that the defect has been fixed and that no new issues have been introduced. The tester verifies the functionality of the affected area to confirm that the defect is truly resolved.
6. Closed
If the defect is successfully fixed and verified, it is moved to the "Closed" status. This means that the defect has been resolved, verified, and is no longer an issue. The bug is considered fixed, and no further action is required.
7. Reopened
If the defect is retested but the issue persists or the fix does not resolve the defect, the defect status is updated to "Reopened." The defect then goes back to the development team for further investigation and fixing. This could happen when the defect was not properly fixed or was misunderstood.
3. Types of Defects
Defects can vary based on their severity, nature, and impact on the software. Below are the common types of defects:
- Functional Defects: Issues related to the functionality of the software. The system does not perform as expected or specified.
- Performance Defects: Issues that cause the application to perform poorly under normal or heavy load conditions, such as slow response times, high CPU usage, or memory leaks.
- Security Defects: Vulnerabilities or weaknesses that can be exploited by unauthorized users, leading to data breaches, security threats, or system compromise.
- Usability Defects: Problems with the software’s user interface or experience, which can hinder the user’s ability to interact with the system effectively.
- Compatibility Defects: Issues where the software does not work as intended across different operating systems, browsers, or devices.
4. Identifying and Reporting Defects
Effective defect identification and reporting are key to the success of the testing process. Defects should be reported in a clear and structured manner to ensure that they are understood by the development team and can be addressed efficiently. The following information should be included when reporting a defect:
- Defect ID: A unique identifier for the defect.
- Summary: A brief description of the defect.
- Severity: The impact level of the defect (e.g., critical, major, minor).
- Priority: The urgency of addressing the defect (e.g., high, medium, low).
- Steps to Reproduce: A detailed description of the steps needed to reproduce the defect.
- Expected vs. Actual Results: A clear comparison of what was expected to happen versus what actually occurred.
- Environment: Details about the testing environment, such as the operating system, browser, and hardware configuration.
- Attachments: Any relevant screenshots, logs, or error messages to help clarify the defect.
5. Defect Reporting Tools
There are several defect tracking tools available to help teams manage and track defects effectively. These tools offer features for reporting, assigning, and resolving defects, as well as generating reports and analytics. Some popular defect reporting tools include:
- Jira: A widely used project management and issue tracking tool that integrates with various testing tools.
- Bugzilla: An open-source defect tracking tool that provides comprehensive defect management features.
- Redmine: A flexible project management tool that includes defect tracking features.
- TestRail: A test management tool that also supports defect tracking and reporting.
- Asana: A project management tool with defect tracking capabilities to help teams collaborate on fixing issues.
6. Best Practices for Defect Management
- Clear and Detailed Reporting: Ensure that defects are reported with enough detail to enable the development team to reproduce and fix the issue.
- Regular Defect Review: Hold regular meetings to review and prioritize defects, ensuring that high-priority issues are addressed first.
- Defect Tracking Tools: Use defect tracking tools to centralize defect management and ensure that defects are easily monitored and resolved.
- Effective Communication: Maintain open communication between the testing and development teams to ensure that defects are resolved in a timely manner.
- Root Cause Analysis: Perform root cause analysis for critical defects to prevent recurrence and improve the quality of the product.
7. Example: Defect Reporting
Here’s an example of how a defect may be reported:
Defect ID | Summary | Severity | Priority | Steps to Reproduce | Expected Result | Actual Result | Environment |
---|---|---|---|---|---|---|---|
DF001 | Login button not working on the homepage | Major | High | 1. Open homepage. 2. Click on the login button. | The login page should open. | The login page does not open. | Windows 10, Chrome 92.0 |
Using Traceability Matrix for Requirements Mapping
A Traceability Matrix is a document that helps ensure that the requirements of a project are being met by the test cases. It is a key tool in software testing to verify that all requirements have been tested and validated during the testing phase. The matrix provides a clear mapping between requirements and test cases, ensuring that each requirement is verified through adequate testing.
1. What is a Traceability Matrix?
The Traceability Matrix is a table that links requirements to test cases. It helps in tracking the progress of test case execution and ensures that each requirement has corresponding test coverage. The matrix acts as a bridge between the development and testing teams, offering transparency and alignment between the requirements and the test cases.
2. Types of Traceability Matrices
There are different types of Traceability Matrices, depending on the focus of the traceability. Below are the common types:
- Forward Traceability: Ensures that each requirement has been implemented and tested by verifying that the corresponding test cases are executed.
- Backward Traceability: Ensures that all implemented features align with the original requirements, preventing unnecessary functionality from being built.
- Bi-Directional Traceability: Ensures that all requirements are covered by test cases and that all test cases are mapped back to specific requirements, offering a comprehensive approach.
3. Benefits of Using a Traceability Matrix
The Traceability Matrix provides several key benefits to the software testing process, such as:
- Ensures Requirement Coverage: Ensures that all requirements are covered by test cases, reducing the chances of missed requirements.
- Improves Test Planning: Helps in the creation of comprehensive test cases that cover all aspects of the requirements.
- Tracks Test Execution: Enables tracking of test case execution for each requirement, ensuring that testing is complete and on schedule.
- Facilitates Change Management: When changes to requirements occur, the Traceability Matrix helps identify which test cases need to be updated to ensure continued alignment with the updated requirements.
- Improves Communication: Helps communicate the testing coverage and progress clearly between stakeholders, including developers, testers, and managers.
4. Components of a Traceability Matrix
Each Traceability Matrix typically includes the following components:
- Requirement ID: A unique identifier assigned to each requirement.
- Requirement Description: A brief description of the requirement being tested.
- Test Case ID: A unique identifier assigned to each test case.
- Test Case Description: A brief description of the test case that corresponds to the requirement.
- Test Status: The current status of the test case (e.g., Pass, Fail, In Progress).
- Test Execution Date: The date when the test case was executed.
- Remarks: Any additional notes or comments related to the test case or requirement.
5. How to Create a Traceability Matrix
To create a Traceability Matrix, follow these steps:
- List all requirements: Start by listing all the functional and non-functional requirements of the project.
- Identify test cases: For each requirement, write test cases that will verify whether the requirement has been met.
- Map requirements to test cases: In the matrix, map each requirement to its corresponding test cases.
- Track test execution: As the testing phase progresses, track the execution status of each test case and update the matrix accordingly.
- Maintain the matrix: Keep the Traceability Matrix up to date throughout the project lifecycle, ensuring that it reflects any changes in requirements or test cases.
6. Example of a Traceability Matrix
Below is an example of a simple Traceability Matrix:
Requirement ID | Requirement Description | Test Case ID | Test Case Description | Test Status |
---|---|---|---|---|
REQ001 | Login functionality should be secure | TC001 | Test login with valid credentials | Passed |
REQ002 | System should handle invalid login attempts | TC002 | Test login with invalid credentials | Failed |
REQ003 | User profile should be editable | TC003 | Test editing user profile | Passed |
7. Tools for Traceability Matrix Management
There are various tools available to help manage and generate Traceability Matrices. Some popular tools include:
- Jira: A widely used project management tool that integrates with test management plugins to generate Traceability Matrices.
- TestRail: A test management tool that offers built-in features for creating Traceability Matrices.
- Quality Center (ALM): A test management tool that helps in managing requirements and test cases, generating Traceability Matrices.
- Microsoft Excel: A commonly used tool for creating simple Traceability Matrices manually.
8. Best Practices for Using Traceability Matrix
- Ensure Complete Requirement Coverage: Always ensure that every requirement is linked to at least one test case.
- Update the Matrix Regularly: Keep the Traceability Matrix up to date to reflect any changes in requirements or test cases.
- Ensure Clear Mapping: Maintain clear and consistent mapping between requirements and test cases to avoid confusion.
- Use Automation Tools: Leverage automated tools to help manage and generate Traceability Matrices efficiently, especially for larger projects.
Test Environment Setup
A Test Environment is a critical setup for executing tests effectively. It is a controlled environment where software applications are tested under different configurations to ensure their functionality, performance, security, and compatibility. Setting up the right test environment helps in identifying bugs, improving the quality of the software, and ensuring it behaves as expected in real-world scenarios.
1. What is a Test Environment?
A test environment is a set of hardware, software, network configurations, and tools used to run test cases. This environment is designed to mimic the production system as closely as possible to simulate realistic conditions during testing. It includes servers, databases, operating systems, network configurations, browsers, and any other tools required for testing the application.
2. Key Components of a Test Environment
The following components are essential to set up a test environment:
- Hardware: The physical machines, servers, or virtual machines on which the testing will be executed. This includes CPU, memory, storage, and other hardware resources.
- Software: The operating systems, database systems, web servers, and other software tools required for testing.
- Network Configuration: The network setup, such as firewalls, routers, load balancers, and connectivity configurations, must mimic the production environment.
- Test Data: A set of data used to run tests. This data should represent real-world scenarios to ensure the application is thoroughly tested.
- Test Tools: The tools required to automate tests, track bugs, or simulate real-user behavior during testing (e.g., Selenium, JMeter, LoadRunner).
- Security Configuration: Ensuring that the environment has the necessary security measures in place, including firewalls, antivirus, encryption, and user access controls.
3. Types of Test Environments
There are different types of test environments used for various testing purposes:
- Development Environment: A setup for development purposes, where developers write and test their code. It is not typically used for testing by QA teams.
- Test/QA Environment: This environment is specifically designed for quality assurance testing. It is where all functional, integration, and system tests are executed.
- Staging Environment: A pre-production environment that mimics the production environment as closely as possible. It is used for final testing before deployment.
- Production Environment: The live environment where the application is deployed and accessed by end-users. It is not generally used for testing, but performance or security testing can be done on production-level data.
- Continuous Integration (CI) Environment: Used in CI/CD pipelines for automated testing and integration of new code changes.
4. Steps to Set Up a Test Environment
Follow these steps to set up an effective test environment:
- Understand the Requirements: Gather all the requirements, including the hardware, software, network configurations, and tools needed for the testing process.
- Define Test Environment Configuration: Define the exact configuration of the environment to match the production setup. This should include the operating system, software versions, and dependencies.
- Provision Resources: Set up the required hardware (physical or virtual machines) and provision cloud or on-premise resources as needed.
- Install Necessary Software: Install required software components like operating systems, databases, web servers, and any other software or dependencies for the test environment.
- Configure Network and Security: Set up network configurations, firewalls, routers, load balancers, and ensure that the environment has the proper security settings for testing.
- Prepare Test Data: Set up the test data that will be used during the testing process. This should cover different scenarios and edge cases to ensure thorough testing.
- Install Test Tools: Install any tools needed for automation, performance testing, or bug tracking, such as Selenium, JMeter, or Jira.
- Verify Environment Setup: Verify the environment setup by running a few initial tests to ensure everything is functioning as expected.
5. Best Practices for Test Environment Setup
- Consistency with Production: Ensure that the test environment closely mirrors the production environment to get accurate results.
- Automate Environment Setup: Use automation tools to set up environments quickly and consistently, especially in continuous integration workflows.
- Document the Environment: Properly document the environment setup, configurations, and any dependencies to ensure that it can be reproduced in the future.
- Monitor and Maintain the Environment: Regularly monitor and maintain the test environment to ensure it is up-to-date and free of issues.
- Isolate the Test Environment: Keep the test environment isolated from the production environment to avoid interference with live data or users.
6. Tools for Test Environment Management
There are several tools available to help manage and set up test environments:
- Docker: A containerization tool that allows developers and testers to set up isolated environments and replicate environments across different machines.
- Vagrant: A tool for managing virtualized test environments. It simplifies the setup of reproducible test environments.
- Terraform: A tool for infrastructure as code (IaC) that helps in automating the setup and provisioning of test environments in cloud platforms.
- Jenkins: A popular continuous integration tool that can be configured to automatically set up and deploy test environments for automated testing.
- VirtualBox: A free and open-source virtualization tool that helps create and manage virtual machines for testing purposes.
7. Challenges in Test Environment Setup
Setting up a test environment can come with several challenges:
- Resource Constraints: Limited hardware or cloud resources may hinder the ability to set up an ideal test environment.
- Complex Configuration: The complexity of replicating the production environment, especially in large-scale systems, can make environment setup difficult.
- Environment Drift: Over time, the test environment may drift from the production environment due to updates and patches, leading to inconsistent test results.
- Data Privacy and Security: Ensuring that the test data does not violate privacy regulations or compromise sensitive data is critical during environment setup.
Overview of Popular Testing Tools
In software testing, using the right set of tools is crucial for improving efficiency, accuracy, and coverage. These tools help automate repetitive tasks, ensure thorough testing, and improve the overall quality of the software. Below is an overview of some of the most popular testing tools used in the industry, categorized by their functionality.
1. Test Automation Tools
Test automation tools allow testers to write scripts that automatically execute test cases on the application, saving time and effort in repeated manual testing.
- Selenium: A widely-used open-source tool for automating web browsers. It supports multiple programming languages, including Java, Python, C#, and Ruby. Selenium is ideal for functional testing of web applications.
- Cypress: An end-to-end testing framework for web applications. Unlike Selenium, Cypress runs directly inside the browser, offering faster execution and a better debugging experience.
- TestComplete: A commercial test automation tool that supports automated testing for desktop, mobile, and web applications. It offers scripting options, keyword-driven testing, and visual testing.
2. Performance Testing Tools
Performance testing tools help measure the speed, responsiveness, and stability of a software application under different conditions. These tools are essential for ensuring that the application can handle high loads and perform efficiently.
- JMeter: An open-source tool for performance and load testing. It is widely used to simulate heavy loads on web servers, databases, and other services to test performance under stress.
- LoadRunner: A comprehensive performance testing tool from Micro Focus. It is used to test applications and infrastructure by simulating thousands of virtual users to analyze the performance and scalability of the system.
- Gatling: A highly scalable performance testing tool designed for ease of use. It is particularly effective for simulating and analyzing HTTP-based traffic in web applications.
3. API Testing Tools
API testing tools are used to test the functionality, security, and reliability of APIs. These tools help ensure that the APIs perform as expected and handle edge cases effectively.
- Postman: A popular tool for testing and interacting with REST APIs. It provides an intuitive interface for creating and sending requests, as well as features like collections, environments, and automated test scripts.
- SoapUI: A tool for testing SOAP and REST APIs. It includes features for functional testing, security testing, and load testing APIs, making it a comprehensive solution for API testing.
- RestAssured: A Java library used for testing REST APIs. It simplifies testing by providing a readable and concise syntax for writing tests and assertions.
4. Mobile Testing Tools
Mobile testing tools are specialized for testing mobile applications on different platforms, including Android and iOS. These tools help ensure that mobile applications function properly across a variety of devices and operating systems.
- Appium: An open-source tool for automating mobile applications. It supports both Android and iOS and allows for cross-platform testing using a single script.
- Espresso: A Google-developed tool for automating tests for Android applications. It is designed for writing reliable and fast UI tests for native Android apps.
- XCUITest: A testing framework provided by Apple for iOS applications. It is used to write unit and UI tests for iOS apps, offering deep integration with the Xcode IDE.
5. Continuous Integration (CI) Tools
CI tools are used to automate the build, testing, and deployment processes. They help ensure that new code is integrated into the main branch frequently and tested automatically, reducing the risk of bugs in production.
- Jenkins: A widely-used open-source automation server for continuous integration and continuous delivery. Jenkins integrates with testing tools and allows for automated testing, building, and deployment of applications.
- GitLab CI/CD: A tool integrated with GitLab that offers continuous integration and delivery features. It automates the testing, building, and deployment process within the GitLab ecosystem.
- CircleCI: A cloud-based CI/CD tool that supports building, testing, and deploying applications. CircleCI integrates with popular version control systems like GitHub and Bitbucket.
6. Test Management Tools
Test management tools help organize and manage the testing process, including test case creation, execution, and tracking of defects. These tools ensure that all aspects of testing are documented and tracked efficiently.
- Jira: A popular project management tool that includes test management features. Jira integrates with other testing tools like Selenium and Jenkins for a seamless testing process.
- TestRail: A comprehensive test management tool that helps organize test cases, track test execution, and generate reports. It integrates with various bug tracking and CI tools.
- Quality Center (QC): A test management tool by Micro Focus, which supports test planning, test case management, and defect tracking in large projects.
7. Bug Tracking and Issue Tracking Tools
Bug tracking tools are essential for logging, managing, and tracking the progress of defects found during the testing process. These tools help teams collaborate and prioritize issues for resolution.
- Bugzilla: An open-source bug tracking tool that allows teams to log, track, and manage defects throughout the software development lifecycle.
- Redmine: A flexible, open-source project management tool that includes features for issue tracking, project planning, and collaboration.
- Asana: A work management tool that can be used for bug tracking and project management. Asana integrates with various test management and development tools.
8. Code Quality and Static Analysis Tools
Static analysis tools scan the source code for potential issues and vulnerabilities without executing it. They help identify security flaws, code smells, and other problems early in the development process.
- SonarQube: A tool for continuous inspection of code quality that supports multiple languages. It provides insights into bugs, security vulnerabilities, and code smells.
- PMD: A source code analyzer that identifies potential issues in Java code, such as unused variables, empty catch blocks, and more.
- Checkmarx: A security-focused static analysis tool that scans code for vulnerabilities, helping teams identify and address security flaws early in the development cycle.
9. Code Coverage Tools
Code coverage tools measure the percentage of code that is executed during testing. These tools help ensure that the test suite provides sufficient coverage of the application's codebase.
- Jacoco: A code coverage tool for Java applications that generates reports on which parts of the code are covered during testing.
- Coveralls: A code coverage service that integrates with CI tools and provides coverage reports for various programming languages.
- Clover: A Java-based code coverage tool that helps developers analyze test coverage and identify areas of the code that need additional tests.
Conclusion
These are just a few of the most popular testing tools used in the software industry. The right tool selection depends on the type of testing required, the project needs, and the technologies being used. Utilizing the appropriate tools can significantly enhance the effectiveness and efficiency of the testing process, leading to higher-quality software.
Test Automation Tools: Selenium, Cypress, Katalon Studio
Test automation tools help automate repetitive tasks, improve efficiency, and ensure the accuracy of testing. Below is an overview of three popular test automation tools: Selenium, Cypress, and Katalon Studio. These tools are widely used in the industry for automating web applications and improving software quality.
1. Selenium
Selenium is one of the most widely used open-source test automation tools for web applications. It supports multiple programming languages, including Java, Python, C#, and Ruby, and can be used for automated functional testing of web applications across different browsers and platforms.
Key Features of Selenium:
- Cross-browser Compatibility: Selenium supports all major browsers, including Chrome, Firefox, Safari, and Internet Explorer, ensuring tests can be executed across different environments.
- Multiple Language Support: It supports various programming languages such as Java, Python, C#, Ruby, and JavaScript, providing flexibility to testers based on their preferred language.
- Integration with CI/CD Tools: Selenium integrates seamlessly with continuous integration (CI) tools like Jenkins, allowing automated tests to be run as part of the CI pipeline.
- WebDriver: Selenium WebDriver is a key component that allows interaction with web elements in a browser, providing accurate and reliable results.
Disadvantages of Selenium:
- Steep Learning Curve: Selenium requires knowledge of programming and scripting, which can be challenging for beginners.
- Limited Support for Desktop Applications: Selenium is mainly used for web application testing and doesn’t offer robust support for desktop or mobile app testing.
- Maintenance Overhead: As the application under test evolves, Selenium scripts may require frequent maintenance due to changes in the web elements.
2. Cypress
Cypress is a next-generation, open-source test automation tool designed for modern web applications. It is particularly known for its fast execution and developer-friendly features, making it a popular choice for frontend testing.
Key Features of Cypress:
- Real-Time Test Execution: Cypress runs tests directly inside the browser, providing real-time feedback and allowing easy debugging and inspection of test results.
- Easy to Setup: Unlike Selenium, Cypress does not require separate drivers or browser configurations, making it quick and easy to set up and start testing.
- Automatic Waiting: Cypress automatically waits for elements to be available before performing actions, eliminating the need for adding manual waits in the test scripts.
- Powerful Debugging: Cypress allows debugging tests directly in the browser with powerful debugging tools and logs, making it easier for testers to identify issues.
Disadvantages of Cypress:
- Limited Browser Support: Cypress currently supports only Chrome, Chromium-based browsers, and Electron, which limits its cross-browser compatibility compared to Selenium.
- Limited Support for Mobile Testing: Cypress is primarily focused on web testing and does not offer direct support for mobile applications.
- Integration with CI/CD Tools: While Cypress can be integrated with CI/CD tools, the setup process may require additional configuration compared to Selenium.
3. Katalon Studio
Katalon Studio is a comprehensive test automation tool that provides an integrated environment for automated testing of web, mobile, and API applications. It is designed to be user-friendly and suitable for both technical and non-technical users.
Key Features of Katalon Studio:
- Intuitive Interface: Katalon Studio offers a user-friendly interface with a no-code/low-code approach for creating test scripts, making it accessible for both developers and non-technical testers.
- Cross-platform Support: Katalon Studio supports web, mobile, and API testing, making it an all-in-one solution for test automation across different platforms.
- Built-in Keywords and Functions: Katalon comes with a wide range of built-in functions and pre-configured keywords that simplify test script creation and execution.
- Integration with CI/CD Tools: Katalon integrates with popular CI/CD tools like Jenkins, Git, and JIRA, enabling automated testing in continuous delivery pipelines.
Disadvantages of Katalon Studio:
- Limited Customization: While Katalon Studio offers built-in functions, it may lack the flexibility for customizations that advanced users might need for complex test cases.
- Resource Intensive: Katalon Studio can be resource-heavy, particularly when running tests in parallel, which may impact system performance on lower-end hardware.
- Free Version Limitations: The free version of Katalon Studio has certain limitations, such as limited access to advanced features and support.
Conclusion
Each of these test automation tools—Selenium, Cypress, and Katalon Studio—has its unique strengths and is suited for different testing needs. Selenium is highly flexible and supports multiple languages, making it ideal for large and complex projects. Cypress is fast, developer-friendly, and best suited for modern web applications, while Katalon Studio offers a comprehensive and easy-to-use platform for testing across web, mobile, and APIs. Choosing the right tool depends on your project’s requirements, team expertise, and testing goals.
Performance Testing Tools: JMeter, LoadRunner
Performance testing tools are essential for evaluating the speed, scalability, and stability of an application under various load conditions. Two of the most popular performance testing tools are **JMeter** and **LoadRunner**. These tools help ensure that applications perform well under expected user load and identify bottlenecks before going live.
1. JMeter
Apache JMeter is an open-source performance testing tool designed for load testing and performance measurement of web applications. It is one of the most widely used tools for testing the performance of web applications, databases, and other services.
Key Features of JMeter:
- Open-Source: JMeter is free to use and has a large community of users and contributors that provide ongoing support and updates.
- Support for Multiple Protocols: JMeter supports various protocols, including HTTP, HTTPS, FTP, JDBC, SOAP, REST, and more, making it suitable for testing different types of applications.
- User-Friendly Interface: JMeter offers an intuitive graphical user interface (GUI) for creating and managing performance test plans, making it easy for testers to design and execute performance tests.
- Distributed Testing: JMeter supports distributed testing, allowing you to run tests from multiple machines to simulate a large number of users and measure system performance at scale.
- Extensive Reporting: JMeter provides detailed performance reports and analysis, including response times, throughput, and error rates, which help identify performance bottlenecks.
Disadvantages of JMeter:
- Limited Support for Complex Applications: While JMeter is suitable for testing web applications, it may struggle with complex applications or services that require high levels of integration.
- Memory Consumption: JMeter can consume a significant amount of memory when running tests with a large number of virtual users, which may affect its performance on low-end systems.
- Steep Learning Curve for Advanced Features: While JMeter offers an easy-to-use GUI for basic testing, advanced features like scripting and distributed testing may require additional learning and expertise.
2. LoadRunner
LoadRunner, developed by Micro Focus, is a comprehensive performance testing tool that is widely used in large enterprises for testing the performance of web and mobile applications. It supports a wide range of protocols and provides extensive reporting and analysis capabilities.
Key Features of LoadRunner:
- Comprehensive Protocol Support: LoadRunner supports a broad range of protocols, including web (HTTP/HTTPS), database, SAP, Citrix, and more, making it suitable for testing various enterprise applications.
- Virtual User Generator (VuGen): LoadRunner includes VuGen, which records user interactions with the application and generates scripts for load testing. This allows for simulating real user behavior and testing application performance under load.
- Advanced Analysis and Reporting: LoadRunner offers advanced performance monitoring and analysis tools, enabling users to measure response times, system resource utilization, and more, with detailed graphical reports and insights.
- Integration with Other Tools: LoadRunner integrates with a variety of other testing and monitoring tools, including APM (Application Performance Monitoring) tools like Dynatrace and AppDynamics.
- Scalability: LoadRunner is capable of simulating a large number of virtual users (up to hundreds of thousands) and can be scaled to test applications at enterprise-level loads.
Disadvantages of LoadRunner:
- Expensive: LoadRunner is a commercial tool with a high licensing cost, which might not be suitable for smaller teams or organizations with limited budgets.
- Complex Setup: Setting up LoadRunner and creating performance tests can be more complex compared to other tools, especially for new users who are unfamiliar with the tool.
- Heavy Resource Usage: LoadRunner requires significant system resources for large-scale tests, which can affect the performance of the testing environment and increase hardware requirements.
Comparison: JMeter vs. LoadRunner
Feature | JMeter | LoadRunner |
---|---|---|
License | Open-source (Free) | Commercial (Paid) |
Protocol Support | Web, Database, FTP, SOAP, REST, etc. | Comprehensive (Web, SAP, Citrix, Database, etc.) |
Ease of Use | Relatively easy for beginners | More complex setup and configuration |
Scalability | Supports distributed testing, but limited by memory | Highly scalable for large enterprise applications |
Cost | Free | Expensive (requires licensing) |
Conclusion
Both JMeter and LoadRunner are powerful performance testing tools, each with its strengths and limitations. JMeter is an open-source tool that is well-suited for smaller projects and teams with limited budgets, offering support for a variety of protocols and being easy to use. LoadRunner, on the other hand, is a commercial tool that offers advanced features and excellent scalability, making it ideal for large enterprise applications and high-load testing scenarios. The choice between JMeter and LoadRunner depends on your project requirements, budget, and scale of testing.
API Testing Tools: Postman, REST Assured
API testing is critical for verifying the functionality, reliability, performance, and security of application programming interfaces (APIs). Two of the most popular API testing tools are **Postman** and **REST Assured**. These tools help developers and testers ensure that APIs work as expected, return the correct responses, and perform well under various conditions.
1. Postman
Postman is an open-source API testing tool that provides a user-friendly interface for creating, testing, and managing APIs. It is widely used for testing RESTful APIs and offers a wide range of features that enable efficient API development and testing.
Key Features of Postman:
- User-Friendly Interface: Postman has an intuitive graphical interface that allows users to easily send HTTP requests, view responses, and manage multiple API tests.
- Support for Various HTTP Methods: Postman supports a wide range of HTTP methods such as GET, POST, PUT, DELETE, PATCH, etc., allowing users to test various API endpoints and operations.
- Automated Testing with Collections: Users can create test collections in Postman, which allow for grouping multiple API requests and running them sequentially to automate testing processes.
- Environment Management: Postman allows for environment management, which means that you can define different sets of variables (e.g., URLs, authentication tokens) for different testing environments (e.g., development, staging, production).
- Test Scripts and Assertions: Postman enables users to write test scripts using JavaScript to assert API responses, check the status codes, validate response times, and more.
- Collaboration and Sharing: Postman provides collaboration features, allowing teams to share API requests, test collections, and environments, making it easier for teams to collaborate on API testing.
Disadvantages of Postman:
- Limited Support for Complex Assertions: While Postman provides basic testing capabilities, more complex assertions and validations require custom scripting, which can be difficult for non-developers.
- Not Ideal for Large-Scale Tests: Postman might not be the best choice for large-scale API tests or those requiring high concurrency, as it is more suited for functional testing and small-scale scenarios.
- Limited Reporting Features: Postman’s reporting features are basic and not as comprehensive as other tools. For detailed performance metrics and logs, users may need to rely on external tools or integrations.
2. REST Assured
REST Assured is a Java-based library for testing REST APIs. It provides a simple, domain-specific language (DSL) for writing tests for RESTful web services, making it a popular choice for developers working with Java.
Key Features of REST Assured:
- Support for RESTful APIs: REST Assured provides a powerful and easy-to-use DSL for testing REST APIs and allows users to send HTTP requests, validate responses, and perform various assertions.
- Extensive Assertion Capabilities: REST Assured supports a wide range of assertions, including status code checks, response time validation, header validation, and body content validation, which makes it suitable for complex testing scenarios.
- Seamless Integration with Testing Frameworks: REST Assured integrates easily with popular testing frameworks such as JUnit, TestNG, and Cucumber, allowing you to create comprehensive and automated test suites.
- Supports JSON and XML: REST Assured supports both JSON and XML formats, enabling users to test APIs that respond with either type of data.
- Authentication Support: REST Assured provides built-in support for various authentication mechanisms, including basic authentication, OAuth, and API keys, which simplifies testing secured APIs.
Disadvantages of REST Assured:
- Requires Java Knowledge: As REST Assured is a Java-based tool, it requires users to have knowledge of Java programming to write and execute API tests.
- Setup Complexity: Setting up REST Assured might be more complex compared to tools like Postman, especially for teams unfamiliar with Java or Maven.
- Not Ideal for Non-Technical Users: Since REST Assured is a code-based tool, it may not be the best choice for testers who do not have a programming background.
Comparison: Postman vs. REST Assured
Feature | Postman | REST Assured |
---|---|---|
Type | GUI-based API Testing Tool | Code-based Java Library for API Testing |
Ease of Use | Easy to use with a user-friendly interface | Requires Java knowledge and coding skills |
Test Automation | Supports automated testing with collections | Requires integration with Java testing frameworks like JUnit or TestNG |
Protocol Support | Supports REST, SOAP, GraphQL, and other protocols | Primarily for testing REST APIs |
Advanced Features | Good for basic functional testing, lacks advanced reporting | Great for complex assertions and integration with existing test frameworks |
Ideal User | Testers, developers, and non-technical users | Java developers and technical users |
Conclusion
Both Postman and REST Assured are powerful tools for API testing, but they serve different use cases. Postman is ideal for manual API testing, especially for those who prefer a GUI interface and do not have programming experience. It is great for quick functional testing and exploring APIs. On the other hand, REST Assured is more suited for automated testing in a development environment, offering advanced capabilities for validating API responses. It is particularly useful for Java developers who want to integrate API testing into their existing test suites.
Test Management Tools: TestRail, Zephyr, Jira
Test management tools are essential for organizing, planning, and tracking the progress of software testing activities. They help teams manage test cases, track defects, and ensure the effective execution of tests throughout the software development lifecycle. Popular test management tools include **TestRail**, **Zephyr**, and **Jira**, each offering unique features for managing testing projects efficiently.
1. TestRail
TestRail is a comprehensive test management tool used by teams to manage test cases, track testing progress, and organize test runs. It is a web-based application that provides features for planning, executing, and reporting on testing activities.
Key Features of TestRail:
- Test Case Management: TestRail allows for the creation, organization, and maintenance of test cases. You can categorize test cases by suites, milestones, and plans.
- Test Plan and Test Run Management: Users can create detailed test plans, organize test runs, and assign them to specific testers. It provides the ability to execute tests, log results, and track overall test progress.
- Customizable Reporting: TestRail offers built-in reporting features to track test progress, defect counts, and test execution metrics. It also allows users to create custom reports for better insights.
- Integration with Other Tools: TestRail integrates with various issue tracking and version control tools such as Jira, GitHub, and Bugzilla to streamline testing workflows.
- Collaboration Features: TestRail provides team collaboration features, including test case sharing, comment threads, and email notifications to keep all team members updated.
Disadvantages of TestRail:
- Cost: TestRail is a premium tool, and its pricing might be a barrier for smaller teams or organizations with limited budgets.
- Complex Setup: TestRail requires some configuration for initial setup, which might take time for new users.
2. Zephyr
Zephyr is a widely used test management tool that helps teams manage test cases, track test execution, and monitor defect statuses. It integrates seamlessly with Jira, making it a popular choice for teams already using Jira for project management.
Key Features of Zephyr:
- Test Case Creation and Management: Zephyr provides a user-friendly interface for creating and managing test cases, assigning them to testers, and tracking their execution status.
- Test Execution and Defect Tracking: Zephyr allows teams to execute test cases, log test results, and associate defects with the corresponding test cases for better tracking.
- Integration with Jira: Zephyr integrates with Jira to provide a seamless experience for managing test cases and defects within the same platform. You can create Jira issues directly from Zephyr.
- Real-Time Reporting: Zephyr offers real-time reporting for test execution, defect tracking, and test coverage, allowing teams to keep track of progress and make data-driven decisions.
- Cloud and On-Premise Deployment Options: Zephyr provides both cloud-based and on-premise deployment options, allowing organizations to choose based on their needs.
Disadvantages of Zephyr:
- Learning Curve: Zephyr's user interface might take some time to get used to, especially for teams new to test management tools.
- Pricing: Zephyr is a premium tool, and its pricing structure can be expensive for small teams.
3. Jira
Jira, developed by Atlassian, is primarily a project management and issue tracking tool. However, it is widely used in agile environments for managing test cases and defects, especially when integrated with other test management tools like Zephyr or Xray.
Key Features of Jira:
- Issue Tracking: Jira allows teams to track issues and bugs, create user stories, assign tasks, and monitor progress throughout the software development lifecycle.
- Agile Project Management: Jira supports agile methodologies, including Scrum and Kanban, with features like sprint planning, burndown charts, and backlog management.
- Customizable Workflows: Jira allows teams to create customizable workflows for managing tasks, including test case management workflows, defect resolution processes, and more.
- Integration with Test Management Tools: Jira can be integrated with test management tools like TestRail, Zephyr, and Xray to manage testing activities within the same platform.
- Real-Time Reporting and Dashboards: Jira offers built-in reporting and customizable dashboards to track project progress, test execution, defect resolution, and more.
Disadvantages of Jira:
- Complex Setup for Testing: While Jira excels in issue tracking and project management, it requires third-party tools like Zephyr or Xray for comprehensive test management, which can add complexity to setup and maintenance.
- Expensive for Small Teams: Jira's pricing can be high, especially for small teams or organizations with limited budgets.
Comparison: TestRail vs. Zephyr vs. Jira
Feature | TestRail | Zephyr | Jira |
---|---|---|---|
Test Case Management | Comprehensive test case management with strong reporting features | Strong test case management, integrates well with Jira | Can manage test cases with third-party integrations |
Defect Tracking | Built-in defect tracking and integration with issue tracking tools | Defect tracking integration with Jira | Powerful defect tracking as a core feature |
Integration with Jira | Integrates with Jira | Native integration with Jira | Native tool for project management and issue tracking |
Cloud vs On-Premise | Cloud-based tool with on-premise options | Cloud and on-premise options | Cloud-based and on-premise options |
Pricing | Premium pricing | Premium pricing | Subscription-based pricing |
Learning Curve | Moderate learning curve | Moderate learning curve | Low to moderate learning curve |
Conclusion
TestRail, Zephyr, and Jira are all powerful test management tools, each offering different strengths. **TestRail** is ideal for teams looking for a comprehensive test management solution with advanced reporting features. **Zephyr** is perfect for teams already using Jira, offering seamless integration for test case management and defect tracking. **Jira** excels at project management and issue tracking but requires third-party tools for full test management functionality. Choosing the right tool depends on your team's needs, existing workflows, and budget considerations.
Bug Tracking Tools: Bugzilla, Mantis, Jira
Bug tracking tools are critical for managing and tracking defects or issues in a software project. They enable teams to report bugs, assign them to the right developers, track progress, and ensure that issues are addressed before software release. Popular bug tracking tools include **Bugzilla**, **Mantis**, and **Jira**, each offering a set of features tailored to different team needs.
1. Bugzilla
Bugzilla is an open-source, web-based bug tracking system developed by Mozilla. It is widely used in various industries for managing defects, tracking changes, and organizing bug reports. Bugzilla is known for its flexibility, scalability, and ability to handle large numbers of issues efficiently.
Key Features of Bugzilla:
- Customizable Bug Reports: Bugzilla allows users to create customizable bug reports, making it easy to capture detailed information about defects, including severity, status, and priority.
- Advanced Search Capabilities: Bugzilla has a powerful search engine that enables users to query bugs based on various filters such as status, assignee, and creation date.
- Bug Lifecycle Management: Bugzilla provides detailed workflows for managing the lifecycle of a bug, including stages like New, Assigned, Resolved, and Verified.
- Integration with Version Control Systems: Bugzilla can be integrated with popular version control systems (e.g., Git, SVN), allowing developers to link code commits directly to bug reports.
- Custom Fields and Workflows: Bugzilla allows users to define custom fields and create custom workflows to suit specific project needs.
Disadvantages of Bugzilla:
- User Interface: Bugzilla's user interface is often considered outdated and not as intuitive as some other bug tracking tools.
- Complex Configuration: Setting up and configuring Bugzilla can be complex, especially for teams that lack technical expertise.
2. Mantis
Mantis is another open-source bug tracking tool that offers a simple yet powerful platform for managing defects. It is user-friendly and flexible, with features that cater to both small teams and large enterprises. Mantis also offers cloud hosting options for those who prefer a hosted solution.
Key Features of Mantis:
- Simple and Intuitive Interface: Mantis has a clean and easy-to-use interface, making it accessible to both technical and non-technical users.
- Customizable Workflow: Mantis allows users to define custom workflows, including custom statuses, priorities, and categories for defect management.
- Email Notifications: Mantis automatically sends email notifications to stakeholders when bug statuses change or new issues are assigned.
- Integration with Version Control Systems: Like Bugzilla, Mantis integrates with version control systems to track code commits and relate them to specific bugs.
- Reporting and Statistics: Mantis offers built-in reports and statistics to monitor bug resolution progress, including charts and graphs that visualize key metrics.
Disadvantages of Mantis:
- Limited Customization: While Mantis allows some customization, it is not as flexible as other tools like Bugzilla when it comes to creating custom fields and workflows.
- Lack of Advanced Features: Mantis lacks some of the more advanced features offered by other bug tracking tools, such as detailed project management capabilities or extensive reporting features.
3. Jira
Jira, developed by Atlassian, is one of the most popular issue and project tracking tools. Although it is often used for project management and agile workflows, it also provides powerful bug tracking capabilities. Jira is highly customizable and integrates with a variety of other tools, making it suitable for teams of all sizes and industries.
Key Features of Jira:
- Comprehensive Issue Tracking: Jira is designed to track not just bugs, but also user stories, tasks, and other project issues. It provides a comprehensive way to manage all aspects of a project.
- Customizable Workflows: Jira offers highly customizable workflows for bug tracking, allowing teams to define their own bug lifecycle and stages.
- Agile Project Management: Jira includes built-in support for Scrum and Kanban methodologies, which makes it suitable for agile development teams.
- Real-Time Reporting and Dashboards: Jira provides a wide range of reporting and dashboard features that allow teams to track bug resolution progress, sprint velocity, and other key metrics.
- Integration with Other Tools: Jira integrates seamlessly with other Atlassian products (e.g., Confluence, Bitbucket), as well as third-party tools like Git, Jenkins, and Slack.
Disadvantages of Jira:
- Complex Setup for Beginners: Jira’s many features and customization options can make the setup process complex, especially for teams that are new to the tool.
- Pricing: Jira’s pricing can be high for small teams, and its cost increases as the number of users grows.
Comparison: Bugzilla vs. Mantis vs. Jira
Feature | Bugzilla | Mantis | Jira |
---|---|---|---|
Ease of Use | Moderate, requires technical expertise | Simple and intuitive interface | Moderate, complex for beginners |
Customization | Highly customizable with custom fields and workflows | Moderate customization options | Highly customizable workflows and features |
Integration with Version Control | Integrates with popular version control systems | Integrates with version control systems | Integrates with version control and other Atlassian tools |
Advanced Features | Advanced search and reporting features | Lacks advanced features compared to Bugzilla and Jira | Comprehensive reporting, dashboards, and agile support |
Pricing | Free (Open Source) | Free (Open Source) | Subscription-based, can be expensive for small teams |
Support | Community support | Community support | Excellent support, especially for Jira users within the Atlassian ecosystem |
Conclusion
Choosing the right bug tracking tool depends on your team’s requirements and budget. **Bugzilla** is perfect for teams looking for a highly customizable, open-source solution. **Mantis** offers simplicity and ease of use, making it ideal for smaller teams. **Jira**, on the other hand, provides powerful bug tracking along with project management features, though it may be more suitable for larger teams due to its cost and complexity. Each tool has its strengths and weaknesses, and the right choice depends on your specific needs.
Types of Automation Frameworks
Automation frameworks are structured approaches used to automate the process of testing software applications. These frameworks provide guidelines, best practices, and tools to create efficient and maintainable automation tests. There are several types of automation frameworks, each designed to address specific challenges in software testing. The most common types include the Data-Driven Framework, Keyword-Driven Framework, Hybrid Framework, and Behavior-Driven Development (BDD) Framework.
1. Data-Driven Framework
The Data-Driven Framework is designed to separate test scripts from test data. This framework enables the same test script to be executed with multiple sets of input data, allowing for extensive testing with minimal code duplication. It is ideal for testing scenarios where the same set of actions needs to be performed using different data inputs.
Key Features of Data-Driven Framework:
- Separation of Data and Script: Test data is stored externally (in files like CSV, Excel, or databases), and test scripts are designed to be reusable, making the framework easy to maintain.
- Reusability of Test Scripts: The same test script can be used for different sets of data, improving test coverage and reducing the need for writing repetitive scripts.
- Easy to Maintain: Changes in test data do not require changes in the test scripts. This simplifies the maintenance of test cases.
Disadvantages of Data-Driven Framework:
- Complexity in Data Management: Managing large datasets can become cumbersome, especially when dealing with complex test scenarios.
- Limited Flexibility: This approach is best suited for simple tests and can be less effective for complex applications requiring multiple dependencies.
2. Keyword-Driven Framework
The Keyword-Driven Framework is a high-level approach that separates the test logic from the test implementation. It uses keywords to represent actions that the automation script will perform. Test scripts are written in such a way that keywords can be mapped to specific functions in the code.
Key Features of Keyword-Driven Framework:
- Separation of Logic and Test Data: The framework separates test logic from the test data, making the tests more understandable and easier to maintain.
- Non-Technical Users: Testers or business analysts who may not have coding experience can write the test scripts by simply using appropriate keywords, making this framework user-friendly.
- Flexibility: The framework allows for flexibility in terms of adding new keywords or modifying the existing ones.
Disadvantages of Keyword-Driven Framework:
- High Overhead in Keyword Maintenance: As the number of keywords grows, managing them and maintaining consistency across the scripts becomes challenging.
- Complexity: Writing and managing tests with keywords can be complex if there are too many keywords or if they are not well-documented.
3. Hybrid Framework
The Hybrid Framework combines features from multiple types of automation frameworks, such as data-driven, keyword-driven, and sometimes even modular frameworks. This combination provides flexibility and allows for a more comprehensive approach to automation testing.
Key Features of Hybrid Framework:
- Combination of Approaches: The hybrid framework is a blend of different frameworks, allowing the user to leverage the strengths of each framework while mitigating their weaknesses.
- Scalability: It is scalable and can adapt to the changing needs of the project. It is ideal for larger projects with complex requirements.
- Best of Both Worlds: By integrating the best features of data-driven, keyword-driven, and other frameworks, the hybrid framework provides an efficient solution for a wide range of scenarios.
Disadvantages of Hybrid Framework:
- Complex Setup: Setting up a hybrid framework can be challenging and time-consuming, especially for smaller projects that do not require such complexity.
- Higher Maintenance: Since it combines multiple frameworks, maintaining and updating the framework can become difficult as the project grows.
4. Behavior-Driven Development (BDD) Framework (Cucumber, SpecFlow)
The Behavior-Driven Development (BDD) framework is an extension of Test-Driven Development (TDD). It encourages collaboration between developers, testers, and non-technical stakeholders. The framework uses natural language (like Gherkin) to write test scenarios in a way that is easy for everyone to understand. Popular BDD tools include Cucumber and SpecFlow.
Key Features of BDD Framework:
- Natural Language Syntax: BDD tests are written in plain language (using Gherkin syntax), making it accessible to non-technical team members like business analysts and product owners.
- Collaboration: BDD fosters collaboration between developers, testers, and other stakeholders, making it easier to understand requirements and expectations.
- Readable and Understandable Tests: BDD tests are written in a way that anyone in the team can understand, reducing the communication gap between technical and non-technical stakeholders.
- Reusable Step Definitions: BDD frameworks allow for reusable step definitions, meaning that common steps can be written once and reused across multiple test scenarios.
Disadvantages of BDD Framework:
- Learning Curve: While BDD promotes collaboration, there is a learning curve for non-technical stakeholders to understand how to write and interpret the tests properly.
- Initial Setup: Setting up a BDD framework like Cucumber or SpecFlow may require additional configuration and setup compared to traditional frameworks.
Conclusion
Different types of automation frameworks are suited for different testing needs. The Data-Driven Framework is ideal when tests need to be run with different data sets, while the Keyword-Driven Framework is helpful for business analysts and non-technical testers who need to define test logic using keywords. The Hybrid Framework provides a flexible solution by combining the best features of multiple frameworks, and the Behavior-Driven Development (BDD) Framework is great for fostering collaboration and writing tests in plain language for better communication between all stakeholders.
Building a Custom Test Automation Framework
Building a custom test automation framework allows organizations to tailor the automation process to their specific needs, ensuring greater flexibility, reusability, and maintainability. A custom framework can integrate with various tools and technologies while addressing unique testing requirements. In this section, we will walk you through the process of building a robust test automation framework from scratch.
1. Defining the Objectives of the Framework
The first step in building a custom test automation framework is to clearly define the objectives of the framework. This includes understanding the type of applications being tested, the technologies involved, and the goals of automation. Some key questions to consider:
- What types of tests will be automated (functional, regression, performance)?
- What tools will be used for test execution and reporting?
- How will the framework integrate with CI/CD pipelines?
- Will the framework support multiple platforms (Web, Mobile, API)?
- What is the expected level of reusability and maintainability?
2. Choosing the Right Tools and Technologies
Once the objectives are clear, the next step is selecting the tools and technologies for the framework. Depending on the project’s requirements, you may choose from various tools for test automation, reporting, and version control. Some popular tools include:
- Test Automation Tools: Selenium, Cypress, Appium, TestComplete, etc.
- Test Reporting Tools: Allure, Extent Reports, TestNG, JUnit, etc.
- Version Control: Git, Bitbucket, GitHub, etc.
- Build Tools: Jenkins, Bamboo, Travis CI, etc.
- Continuous Integration Tools: Jenkins, GitLab CI, CircleCI, etc.
3. Designing the Framework Architecture
The architecture of the framework should be designed to ensure scalability, maintainability, and reusability. A modular approach to architecture allows for easier updates and management of tests. Some key components to include in your framework architecture:
- Test Data Management: Create a separate layer for handling test data. This allows the test scripts to be independent of the test data, making it easier to maintain and reuse test cases.
- Test Execution: Organize the test execution process into reusable modules, such as setting up test environments, running tests, and generating reports.
- Logging and Reporting: Integrate a robust logging and reporting mechanism to track test execution status and generate detailed reports for analysis.
- Configurations: Externalize configuration settings (e.g., URLs, credentials, environment variables) so they can be easily modified without changing the core codebase.
4. Writing Test Cases and Test Scripts
Once the framework architecture is designed, you can start writing test cases and automation scripts. Keep the following best practices in mind when writing your test scripts:
- Modularization: Write modular test cases that can be reused across different scenarios. This reduces redundancy and increases maintainability.
- Maintainability: Ensure that test scripts are easy to maintain by following coding standards and clear naming conventions.
- Data-Driven Testing: Use data-driven testing techniques to allow the same script to run with different sets of test data.
- Exception Handling: Implement robust exception handling to ensure that tests continue running even if one step fails.
- Assertions: Use assertions to verify that the expected results match the actual outputs.
5. Integrating with Continuous Integration/Continuous Delivery (CI/CD) Pipeline
Integrating the test automation framework with a CI/CD pipeline enables automated execution of tests as part of the build process. This ensures that tests are run continuously and any issues are detected early. Common CI/CD integration steps include:
- Version Control Integration: Link your test automation repository with version control systems like GitHub or Bitbucket.
- Build Automation Tools: Configure build tools like Jenkins, GitLab CI, or CircleCI to trigger test execution during each build.
- Test Execution Automation: Set up test execution for each code commit or pull request to ensure that new changes do not break existing functionality.
- Test Reporting: Configure the pipeline to generate and send test reports after each build to track test results.
6. Test Reporting and Analysis
Effective reporting and analysis of test results are essential for monitoring the health of the application. The framework should include an automated reporting mechanism that provides detailed insights into test execution, such as:
- Pass/Fail Status: Include clear information about which tests passed and which failed.
- Error Logs: Include error logs for failed tests to help developers quickly identify and resolve issues.
- Test Coverage: Track test coverage to ensure that all areas of the application are being tested adequately.
- Historical Data: Maintain historical test data to identify trends and patterns in test results over time.
7. Continuous Maintenance of the Framework
A test automation framework requires ongoing maintenance to keep it up to date with changes in the application. Some key activities for continuous maintenance include:
- Test Script Updates: Regularly update test scripts to reflect new features, bug fixes, or changes to the application.
- Framework Optimization: Periodically review and optimize the framework to improve performance and scalability.
- Tool Upgrades: Keep the tools and technologies used in the framework up to date to take advantage of new features and improvements.
8. Conclusion
Building a custom test automation framework allows teams to tailor the testing process to their specific needs, enabling flexibility and maintaining control over the automation process. By following best practices for design, architecture, and integration, organizations can create a robust framework that supports efficient and scalable test automation. Continuous maintenance and updates to the framework ensure that it evolves alongside the application to deliver reliable and effective test results.
Best Practices for Automation Framework Design
Designing a robust and efficient test automation framework is crucial for maximizing the effectiveness of automated testing. A well-designed framework ensures maintainability, scalability, and reusability, which are essential for long-term success. In this section, we will explore the best practices for building a high-quality automation framework.
1. Keep the Framework Modular and Reusable
A modular framework ensures that components are loosely coupled and can be reused across different tests. This reduces redundancy and allows easy updates to individual modules without affecting the entire framework. Modularization enables:
- Code Reusability: Reuse common functions, test data, and utilities across multiple test cases.
- Easy Maintenance: Independent modules can be updated without affecting the rest of the framework.
- Efficient Scaling: As the system grows, it’s easier to extend and scale a modular framework.
2. Implement Data-Driven Testing
Data-driven testing allows you to run the same test case with different sets of input data. This approach improves test coverage and ensures that a wide variety of conditions are tested. Benefits of a data-driven approach include:
- Separation of Test Logic and Test Data: Test logic remains the same while input data can be modified separately, leading to more maintainable tests.
- Improved Test Coverage: Running tests with multiple data sets ensures that the system is validated under various conditions.
- Less Redundancy: Instead of writing multiple test cases, a single data-driven test case can cover multiple scenarios.
3. Use Page Object Model (POM) for UI Testing
The Page Object Model (POM) is a design pattern used in UI test automation to enhance maintainability and reusability. In this pattern, each web page or component of the application is represented as a separate class. Benefits of using POM include:
- Separation of Concerns: The test scripts only focus on the logic, while the page objects handle interactions with the UI, improving readability and maintainability.
- Easy Maintenance: Changes to the UI are localized within the page object classes, reducing the impact on test scripts.
- Reusability: Page objects can be reused across multiple tests, reducing code duplication.
4. Maintain Clear and Consistent Naming Conventions
Clear and consistent naming conventions make the framework easier to understand and navigate. Establishing naming conventions for test cases, functions, variables, and classes ensures:
- Readability: A well-named function or test case clearly communicates its purpose.
- Consistency: Consistent naming conventions allow team members to quickly understand the framework’s structure, regardless of who wrote the code.
- Better Collaboration: When the naming conventions are standardized, team members can collaborate more effectively and reduce misunderstandings.
5. Modularize Test Data Management
Test data management is a crucial aspect of test automation. Keep test data externalized from the test scripts so that it can be easily modified or updated without changing the test logic. Best practices for test data management include:
- External Data Sources: Use external data sources such as CSV files, databases, or APIs to store test data, ensuring that it can be easily modified.
- Use Config Files: Store configuration data, such as URLs or credentials, in separate configuration files to simplify updates.
- Keep Data Independent: Test data should be independent of the test scripts so that tests can be executed with different data sets without any changes to the code.
6. Implement Effective Logging and Reporting
Effective logging and reporting provide valuable insights into test execution. The framework should generate detailed logs for each test case, including pass/fail status, error messages, and stack traces. Best practices for logging and reporting include:
- Clear and Concise Logs: Logs should include useful information, such as the test name, status, and any error messages, for troubleshooting.
- Automated Test Reports: Configure the framework to automatically generate test reports in formats like HTML or XML that summarize test execution.
- Easy-to-Read Reports: Reports should be structured in a way that stakeholders can easily interpret the results and take necessary actions.
7. Integrate with CI/CD Pipelines
Integrating the automation framework with a CI/CD pipeline allows automated tests to run with every code change, ensuring continuous validation of the application. Benefits of CI/CD integration include:
- Faster Feedback: Automated tests can run as part of the build process, providing immediate feedback to developers.
- Early Detection of Bugs: Automated tests in CI/CD pipelines help detect issues at an early stage, reducing the time and cost of fixing bugs later in the development cycle.
- Improved Test Coverage: As tests are run continuously, the coverage improves, ensuring that the application is tested regularly and thoroughly.
8. Use Version Control for Test Scripts
Version control systems (VCS) like Git are essential for maintaining the history of changes made to the test automation scripts. Key benefits of using version control include:
- Collaboration: Version control allows multiple team members to work on the framework simultaneously without conflicts.
- History Tracking: You can track changes over time and revert to previous versions if needed.
- Efficient Maintenance: Version control makes it easier to manage test scripts as the framework evolves, allowing teams to coordinate changes efficiently.
9. Keep the Framework Scalable
As the application grows, so should your test automation framework. Design the framework with scalability in mind to accommodate increased test cases, new testing requirements, and added features. Consider the following practices:
- Modular Architecture: Use a modular design that makes it easy to add new components or modify existing ones without affecting the entire framework.
- Extensibility: Ensure that new test cases, tools, or features can be added to the framework without significant changes to the core codebase.
- Flexible Configurations: Allow easy customization of the framework’s configuration to adapt to different environments or testing needs.
10. Keep the Framework Simple and Maintainable
Simplicity is key to a successful automation framework. Avoid over-complicating the design or adding unnecessary features that can increase maintenance efforts. Best practices for keeping the framework simple and maintainable include:
- Clear Structure: Organize the framework logically, with well-defined directories for test scripts, data, reports, and utilities.
- Minimize Dependencies: Keep external dependencies to a minimum to reduce complexity and ensure easy maintenance.
- Keep Test Scripts Simple: Write simple and clean test scripts that focus on testing, rather than trying to include all possible features in one script.
11. Conclusion
By following these best practices for automation framework design, you can build a robust and scalable test automation framework that is easy to maintain and extend. A well-designed framework improves the efficiency of testing, reduces the cost of maintenance, and ensures that automated tests provide valuable insights into the quality of the software.
What is Performance Testing?
Performance testing is a type of software testing that focuses on evaluating how well a system performs under different conditions. It measures the responsiveness, stability, scalability, and overall behavior of a system under normal and extreme load conditions. The primary goal of performance testing is to identify performance bottlenecks and ensure that the application can handle the expected number of users or transactions without issues.
Types of Performance Testing
Performance testing encompasses several key types, each focusing on a different aspect of the system's performance. These types include:
- Load Testing: Tests how the application performs under expected user load to ensure it can handle the required traffic.
- Stress Testing: Evaluates the system’s behavior under extreme conditions, such as an excessive number of users or transactions, to understand how it handles stress and failure.
- Spike Testing: Involves testing the system with sudden, sharp increases in load to see how it handles sudden spikes in traffic.
- Endurance Testing: Tests the system’s ability to handle a continuous load over an extended period, ensuring it does not degrade over time.
- Scalability Testing: Focuses on the application’s ability to scale up or scale out when additional resources are added.
Importance of Performance Testing
Performance testing is critical to ensure that applications meet the following requirements:
- Reliability: Performance testing ensures the system performs consistently under different conditions, providing a reliable experience for users.
- Scalability: It helps in understanding how the system behaves under varying loads and whether it can handle increasing traffic or transactions.
- Efficiency: It ensures that the system uses resources effectively, reducing overhead, and optimizing performance.
- End User Experience: It helps ensure that the system responds quickly to user requests, providing a seamless user experience.
Key Metrics in Performance Testing
Performance testing involves measuring various metrics to evaluate the system's behavior. The key performance metrics include:
- Response Time: The time it takes for the application to respond to a user request. It should be as low as possible to provide a fast user experience.
- Throughput: The number of requests or transactions the system can handle within a given time frame.
- Latency: The delay before a transfer of data begins after a request is made.
- Resource Utilization: Measures how much CPU, memory, disk, and network resources the system uses during operation.
- Error Rate: The percentage of failed requests or transactions during the test.
Performance Testing Tools
There are several performance testing tools that help simulate different user loads and measure various performance metrics. Some popular tools include:
- Apache JMeter: An open-source performance testing tool that allows for load, stress, and other types of performance testing.
- LoadRunner: A performance testing tool by Micro Focus that supports a wide variety of protocols and provides detailed reporting.
- Gatling: An open-source load testing tool that focuses on high-performance testing with features like real-time metrics and a clear reporting interface.
- NeoLoad: A performance testing tool designed for testing web and mobile applications, focusing on load and stress testing.
Best Practices for Performance Testing
To get meaningful results from performance testing, it's important to follow best practices:
- Define Clear Objectives: Set performance goals for the system based on expected user behavior and business requirements.
- Test Early and Often: Conduct performance testing as early as possible in the software development life cycle (SDLC) and repeat the tests throughout the development process.
- Simulate Real-World Usage: Test the system under conditions that closely mimic real-world usage, including load, stress, and spike testing.
- Monitor Resource Utilization: Track system resource usage (CPU, memory, network) during tests to identify potential bottlenecks.
- Analyze and Tune: After identifying performance issues, analyze the results and optimize the system for better performance.
Conclusion
Performance testing is essential for ensuring that an application can meet the demands of users and handle varying levels of traffic without crashing or degrading. By evaluating the system’s scalability, responsiveness, and stability under different conditions, performance testing helps deliver high-quality applications that provide a smooth and efficient user experience.
Goals of Performance Testing
Performance testing is essential to ensure that a software application performs well under expected and peak load conditions. The main goal of performance testing is to identify and fix any performance-related issues that could affect the user experience or system stability. Below are the key goals of performance testing:
1. Ensure the Application Can Handle Expected Load
The primary goal of performance testing is to verify that the application can handle the expected number of users and transactions without any performance degradation. This includes measuring the system’s response time, throughput, and resource utilization under normal conditions.
2. Identify Bottlenecks and Performance Issues
Performance testing helps to identify bottlenecks, such as slow database queries, inefficient code, or inadequate server resources, that could hinder the performance of the application. By running stress and load tests, testers can pinpoint where the performance degradation occurs.
3. Ensure Scalability
Scalability refers to the ability of the system to handle increased load when required. Performance testing helps to ensure that the application can scale effectively to accommodate increased traffic, such as adding more users or transactions, without compromising performance.
4. Measure Response Times and Throughput
Performance testing evaluates the response time of the application under various conditions. Response time is crucial for user satisfaction, and throughputs measure the system’s ability to process requests efficiently. The goal is to ensure that the response times meet the service-level agreements (SLAs) and that the system can handle the expected number of transactions per second (TPS).
5. Evaluate Resource Utilization
It’s important to assess how efficiently the application utilizes system resources, including CPU, memory, disk space, and network bandwidth. Performance testing helps detect resource leaks or inefficient usage that could lead to system crashes or slowdowns under heavy load.
6. Test for Stability and Reliability
Performance testing also aims to ensure the stability and reliability of the system over time. Through endurance testing, testers can evaluate how the system behaves under continuous load and for prolonged periods. This helps identify any issues that may arise due to prolonged use, such as memory leaks or resource exhaustion.
7. Evaluate the Impact of Configuration and Infrastructure Changes
When there are changes to the application infrastructure or configuration settings, it’s crucial to assess how these changes affect performance. Performance testing helps evaluate the impact of new features, database optimizations, or configuration updates on the overall system performance.
8. Ensure Consistent User Experience
Ultimately, the goal of performance testing is to ensure that the end users experience consistent, fast, and reliable application performance. This includes testing under various conditions, such as peak traffic, network latency, and different device configurations, to guarantee a smooth user experience across different environments.
9. Validate Compliance with SLAs
Performance testing ensures that the application meets predefined Service Level Agreements (SLAs) regarding performance metrics. These SLAs may include response times, transaction rates, uptime, and availability. Validating compliance with SLAs ensures that the system meets the expectations of both internal stakeholders and customers.
10. Provide Insights for Optimization
Performance testing helps identify areas where the application can be optimized for better performance. This could involve optimizing database queries, enhancing server capacity, improving code efficiency, or scaling infrastructure. Insights gained from performance tests guide the development team in making improvements for better performance.
Conclusion
The goal of performance testing is to ensure that an application can handle the expected load, perform efficiently, scale effectively, and provide a seamless experience for users. Identifying and resolving performance issues early in the development cycle can help avoid potential failures in production and ensure that the application meets user expectations and business requirements.
Load Testing vs. Stress Testing
Load testing and stress testing are two essential types of performance testing used to evaluate how an application performs under various conditions. While both aim to assess the behavior of a system under load, they have distinct objectives and approaches. Below is a comparison between load testing and stress testing:
1. Load Testing
Load testing is the process of evaluating an application’s performance under normal and expected load conditions. The goal is to measure the system’s response time, throughput, and resource utilization under typical user traffic and usage patterns.
Key Objectives of Load Testing:
- To determine how the system performs under normal and peak usage conditions.
- To measure the response time, throughput, and resource consumption under typical load.
- To validate if the system meets the required performance criteria and service-level agreements (SLAs).
Common Load Testing Scenarios:
- Simulating the expected number of users accessing the application at the same time.
- Testing the system's performance under typical traffic during peak hours.
- Evaluating the system's response times and throughput when multiple users interact with the application simultaneously.
Tools for Load Testing:
- Apache JMeter
- LoadRunner
- Gatling
2. Stress Testing
Stress testing, on the other hand, focuses on determining the application's behavior under extreme and beyond-normal load conditions. The objective of stress testing is to identify the system’s breaking point and evaluate how it recovers when resources are exhausted or when the system exceeds its capacity.
Key Objectives of Stress Testing:
- To evaluate the system’s performance when subjected to extreme load conditions, such as a high number of users or transactions.
- To assess how the system behaves when it exceeds its maximum capacity (e.g., crashes, slowdowns, or failures).
- To test the system's recovery capabilities after failure, including how it handles data loss or corruption.
Common Stress Testing Scenarios:
- Simulating a large number of users beyond the system's expected load to determine how it reacts under stress.
- Introducing unexpected traffic spikes to evaluate how the application manages sudden changes in load.
- Simulating resource exhaustion (e.g., CPU, memory, or network bandwidth) to see how the application handles extreme conditions.
Tools for Stress Testing:
- Apache JMeter
- StressTester
- LoadRunner
Key Differences Between Load Testing and Stress Testing
Aspect | Load Testing | Stress Testing |
---|---|---|
Objective | To evaluate system performance under normal and peak load conditions. | To identify the system's breaking point by testing its behavior under extreme load conditions. |
Focus | Normal usage and expected traffic patterns. | Beyond normal usage and unexpected, high traffic spikes or load. |
Test Conditions | Simulate realistic, expected traffic with a steady number of users. | Simulate extreme conditions, often pushing the system beyond its limits. |
Performance Metrics | Response time, throughput, resource utilization under normal conditions. | System failure points, recovery time, resource exhaustion, and system stability. |
Purpose | To ensure the system can handle expected traffic without issues. | To determine how the system reacts to extreme conditions and how it recovers from failure. |
Conclusion
Both load testing and stress testing are essential for evaluating an application's performance, but they serve different purposes. Load testing ensures that the system can handle expected usage patterns, while stress testing identifies the system's limits and ensures it can recover from extreme conditions. By conducting both tests, organizations can ensure that their applications will perform reliably under both normal and stressful conditions.
Performance Testing Metrics
Performance testing metrics are key indicators used to evaluate the performance and efficiency of a system during performance testing. These metrics help to assess how well the system performs under various conditions, such as load, stress, and scalability. The following are common performance testing metrics:
1. Response Time
Response time is the time taken by the system to respond to a user request. It is measured from the moment a request is sent to the system until the response is received by the user. Lower response time is crucial for providing a positive user experience.
Key Points:
- Response time is typically measured in milliseconds (ms).
- It is crucial for web applications and APIs to have low response times, especially for high traffic volumes.
- High response time can lead to user dissatisfaction and can negatively impact business outcomes.
2. Throughput
Throughput refers to the amount of data the system can process in a given time frame. It is typically measured in requests per second (RPS) or transactions per second (TPS). Throughput is an important indicator of the system’s capacity to handle concurrent users or requests.
Key Points:
- Higher throughput indicates better performance and the ability to handle more users or transactions.
- It is essential to measure throughput during load testing to ensure the system can handle expected traffic volumes.
3. Latency
Latency is the delay between sending a request and receiving a response. It is often referred to as the "lag time." Latency can affect the overall user experience, especially in real-time applications like video streaming, gaming, or online trading platforms.
Key Points:
- Low latency is crucial for real-time applications, where delays can cause significant issues.
- Latency can be affected by network conditions, server performance, and application architecture.
4. Error Rate
Error rate is the percentage of requests that result in errors compared to the total number of requests. It helps in identifying issues like system crashes, failed transactions, or broken APIs. A high error rate indicates that the system is not functioning as expected and may require optimization or bug fixes.
Key Points:
- Error rate should ideally be as low as possible, ideally 0%. However, some systems may tolerate minor errors during high load conditions.
- It is important to monitor error rates continuously during performance testing and production to detect issues early.
5. CPU Usage
CPU usage is the amount of processing power consumed by the system's processor during testing. High CPU usage can indicate inefficiencies or bottlenecks in the application’s code or infrastructure. It is important to monitor CPU usage to ensure that the system is not overloading the server’s processing capacity.
Key Points:
- Excessive CPU usage can lead to slower response times and degraded system performance.
- Optimization may be required if CPU usage consistently remains high during load testing.
6. Memory Usage
Memory usage refers to the amount of system memory (RAM) consumed by the application during performance testing. High memory usage can indicate issues like memory leaks or inefficient resource management, which can lead to crashes or slowdowns under heavy load.
Key Points:
- Memory usage should be monitored to detect potential memory leaks that may lead to system instability.
- Memory optimization is critical for systems that are expected to handle a large number of concurrent users or complex operations.
7. Disk I/O
Disk I/O refers to the rate at which the system reads from or writes to the disk. Poor disk I/O performance can lead to slower response times and system performance, especially in database-intensive applications. High disk I/O can be caused by inefficient database queries, excessive logging, or large file operations.
Key Points:
- Monitoring disk I/O is important for systems that interact with large data sets or perform frequent database operations.
- High disk I/O can be mitigated by optimizing database queries, reducing unnecessary file operations, and implementing caching strategies.
8. Network Throughput
Network throughput refers to the amount of data successfully transferred over the network in a given time period. It is an important metric for assessing the performance of web applications, APIs, and cloud-based systems that rely on network communication.
Key Points:
- Network throughput can be affected by factors like bandwidth, latency, and packet loss.
- Optimizing network throughput is important for high-traffic applications or systems that rely on cloud infrastructure.
9. Scalability
Scalability refers to the ability of the system to handle increasing load by adding resources, such as CPU, memory, or storage. Performance testing can help determine how well a system scales when subjected to increased traffic or data volume.
Key Points:
- Scalability testing measures how efficiently the system can scale up or down based on demand.
- It ensures that the system can handle growth without performance degradation or failures.
10. Concurrent Users
Concurrent users refer to the number of users accessing the system simultaneously. Performance testing tracks how well the system handles a large number of concurrent users, ensuring the system can maintain responsiveness and stability during peak traffic periods.
Key Points:
- The system should be able to handle a specified number of concurrent users without performance degradation.
- Monitoring concurrent users is crucial for high-traffic websites, applications, and services.
Conclusion
Performance testing metrics help measure the efficiency, stability, and scalability of a system under various conditions. By tracking these metrics, organizations can identify bottlenecks, optimize performance, and ensure their systems can handle the required user load and traffic volumes without issues. Regular monitoring of performance metrics is essential for maintaining a high-quality user experience and system reliability.
Using Tools like JMeter and LoadRunner
Performance testing tools like JMeter and LoadRunner play a crucial role in assessing the performance, scalability, and reliability of a system under different load conditions. These tools help simulate real-world usage scenarios to evaluate how the application performs under stress, load, and peak conditions.
1. JMeter Overview
Apache JMeter is an open-source, widely-used performance testing tool designed to load test functional behavior and measure performance. It is primarily used for testing web applications, databases, and other services like FTP, JMS, and more.
Key Features of JMeter:
- Open Source: JMeter is free to use and open-source, making it a popular choice among developers and testers.
- Support for Multiple Protocols: JMeter supports testing various types of protocols such as HTTP, HTTPS, SOAP, REST, FTP, JDBC, and more.
- Real-Time Monitoring: JMeter provides real-time monitoring of the system’s performance, including metrics like response time, throughput, and error rate.
- Distributed Testing: JMeter allows the distribution of test execution across multiple machines, allowing the creation of complex load testing scenarios.
- Easy-to-Use Interface: JMeter has a user-friendly graphical interface for test creation, simulation, and reporting.
When to Use JMeter:
- For load testing of web applications and APIs.
- For stress testing, where the system is put under extreme conditions to see how it reacts.
- For performance benchmarking, to evaluate the performance of different versions of the application or system.
- For distributed testing scenarios where multiple machines simulate traffic to test a high-performance system.
2. LoadRunner Overview
LoadRunner is a comprehensive performance testing tool from Micro Focus used for analyzing and measuring the performance of a system under load. It is widely used by enterprises for load testing applications, websites, and services to ensure they can handle the required user traffic.
Key Features of LoadRunner:
- Comprehensive Load Testing: LoadRunner supports testing of web, mobile, enterprise, and cloud applications, providing detailed performance insights.
- Extensive Protocol Support: LoadRunner supports a wide range of protocols such as HTTP, HTTPS, Web Services, Citrix, SAP, and more.
- Integrated Performance Monitoring: LoadRunner provides built-in tools for monitoring the performance of servers, databases, and other system components during testing.
- Advanced Scripting Capabilities: LoadRunner offers advanced scripting features, allowing testers to create custom load test scenarios using the VuGen scripting tool.
- Scalability: LoadRunner can simulate millions of virtual users, making it suitable for large-scale enterprise-level performance testing.
When to Use LoadRunner:
- For large-scale load and stress testing of complex systems, particularly in large enterprises.
- For end-to-end performance testing across various client-server applications.
- For simulating thousands or even millions of virtual users to measure system scalability and performance.
- For detailed analysis of bottlenecks using server-side and client-side metrics.
3. Comparison: JMeter vs LoadRunner
Both JMeter and LoadRunner are powerful performance testing tools, but they have different strengths and use cases. Below is a comparison of the two:
Feature | JMeter | LoadRunner |
---|---|---|
Cost | Free and open-source | Paid, with licensing costs |
Ease of Use | User-friendly, with a GUI-based interface | More complex, with advanced scripting capabilities |
Protocol Support | Supports HTTP, FTP, JMS, and more | Supports a wide range of protocols, including SAP, Citrix, Web Services, and more |
Scalability | Good for moderate load testing, supports distributed testing | Ideal for large-scale testing, can simulate millions of virtual users |
Integration | Integrates easily with CI/CD tools | Advanced integration with enterprise tools like ALM and SiteScope |
Support | Community support | Dedicated support from Micro Focus |
4. Conclusion
Both JMeter and LoadRunner are excellent tools for performance testing, with each offering unique advantages. JMeter is a cost-effective, open-source solution suitable for small-to-medium-sized testing projects, while LoadRunner is better suited for large enterprises that require more advanced features and scalability. The choice between the two depends on your specific testing requirements, budget, and the scale of the system you're testing.
Real-World Performance Testing Scenarios
Performance testing is vital to ensure that applications and systems meet the required performance standards under expected and peak loads. Below are some real-world performance testing scenarios that demonstrate how performance testing is applied in different industries and use cases.
1. E-Commerce Website Load Testing
E-commerce websites experience varying levels of traffic, especially during sales events, product launches, or festive seasons. It is crucial to ensure the website can handle high traffic volumes without crashing or slowing down.
Key Testing Areas:
- Simulating Heavy Traffic: Simulating thousands of users browsing products, adding items to the cart, and completing transactions.
- Database Load: Testing how the website handles concurrent database queries, especially during checkout and payment processes.
- Scalability: Ensuring the system can scale horizontally (adding more servers) or vertically (increasing server resources) to meet demand.
- Response Time: Measuring the time it takes for pages to load, particularly the homepage, product pages, and checkout process.
Tools:
- JMeter
- LoadRunner
- Gatling
2. Online Banking Application Performance Testing
Online banking applications require rigorous performance testing to ensure they can handle heavy traffic and maintain security and data integrity while providing users with a seamless experience.
Key Testing Areas:
- High Concurrent Users: Simulating a large number of users performing different actions like transferring money, checking balances, and paying bills.
- Transaction Load: Testing the system's ability to process multiple transactions simultaneously without delays or failures.
- Data Security: Ensuring that performance does not compromise data security, especially under high load.
- API Testing: Verifying the performance of backend APIs used for balance checking, payments, and other banking functions.
Tools:
- JMeter
- LoadRunner
- NeoLoad
3. Mobile Application Performance Testing
With the increasing use of mobile apps, it is essential to test how mobile applications perform under different conditions, including varying network speeds, device types, and usage patterns.
Key Testing Areas:
- Network Conditions: Testing app performance on different network conditions such as 3G, 4G, and Wi-Fi.
- Device Performance: Evaluating the app’s performance across different devices with varying processing power, memory, and screen resolutions.
- Battery and Resource Usage: Monitoring the app’s impact on battery life, CPU, and memory usage during intensive tasks.
- App Responsiveness: Measuring the app’s response time during various actions like loading, scrolling, and user interaction.
Tools:
- Appium
- MonkeyTalk
- LoadRunner Mobile
4. Video Streaming Service Performance Testing
Video streaming services such as Netflix, Hulu, and YouTube require performance testing to ensure they can handle thousands or millions of users streaming content simultaneously without buffering or quality degradation.
Key Testing Areas:
- Concurrent Stream Testing: Simulating thousands of users streaming videos simultaneously on different devices and network conditions.
- Video Quality: Ensuring the video streams at the correct resolution and quality, especially during peak hours.
- Latency and Buffering: Measuring latency and buffering times when users start streaming videos, and ensuring they are minimal.
- Server Load: Testing the backend infrastructure to handle the massive load of concurrent streams and requests for content.
Tools:
- JMeter
- Gatling
- LoadRunner
5. SaaS Application Performance Testing
Software-as-a-Service (SaaS) applications are used by organizations and individuals around the globe. It is crucial to ensure that these applications can scale and perform well under high user loads to meet business needs.
Key Testing Areas:
- Multi-Tenant Load Testing: Ensuring the SaaS application can handle multiple customers (tenants) without affecting performance or security.
- Scalability Testing: Testing the ability to scale the application horizontally or vertically to support increasing user traffic.
- API Performance: Testing the performance of APIs used by the application for data retrieval, user authentication, and third-party integrations.
- Database Performance: Ensuring the database can handle large queries, concurrent user updates, and complex calculations efficiently.
Tools:
- JMeter
- LoadRunner
- NeoLoad
6. Cloud Application Performance Testing
Cloud applications are often deployed across multiple servers or regions, requiring performance testing to ensure they remain responsive and scalable under high loads.
Key Testing Areas:
- Cloud Scalability: Testing how the application scales across various cloud environments (AWS, Azure, Google Cloud) under varying workloads.
- Server Response: Testing server response time and the ability to handle spikes in traffic efficiently.
- Load Balancing: Ensuring that the load balancing mechanisms work correctly to distribute traffic evenly across servers.
- Fault Tolerance: Testing how the system behaves under failure conditions, such as server crashes or network disconnections.
Tools:
- JMeter
- LoadRunner
- Cloud Testing Tools (e.g., BlazeMeter)
Conclusion
Performance testing is critical across various industries to ensure applications and systems meet user expectations and perform well under different conditions. By simulating real-world usage scenarios with tools like JMeter and LoadRunner, performance testers can identify bottlenecks, optimize resources, and improve the overall user experience.
Introduction to API Testing
API (Application Programming Interface) testing is a type of software testing that focuses on verifying the functionality, reliability, performance, and security of APIs. APIs serve as a bridge between different software applications, allowing them to communicate and exchange data. Since APIs are essential for application interaction, ensuring they function as expected is crucial for the overall success of an application.
What is API Testing?
API testing involves testing the interfaces between different software systems to ensure that data exchange happens correctly. It is an essential process for ensuring the integration of applications and services, especially in systems where multiple services interact with each other via APIs.
API testing does not involve the UI or graphical interface but rather focuses on ensuring that the API endpoints behave as expected under various conditions, including incorrect or malicious inputs.
Importance of API Testing
API testing is important for several reasons:
- Ensures Functional Integrity: Verifies that the API performs as intended and meets the requirements set forth in the API documentation.
- Validates Data Accuracy: Ensures that the API returns the correct data and handles various input scenarios (e.g., valid, invalid, or edge cases).
- Improves Security: Helps identify vulnerabilities or weaknesses in the API that could be exploited by attackers.
- Reduces System Downtime: Detecting issues before the API is used in production reduces the chances of failures and downtimes.
- Faster Release Cycle: By automating API tests, organizations can achieve faster release cycles without compromising quality.
Types of API Tests
API testing encompasses various types of tests, including:
- Functional Testing: Ensures that the API functions according to the requirements. It verifies whether the API returns the expected results for different inputs.
- Security Testing: Ensures that the API is secure and cannot be exploited by unauthorized users. It involves testing for authentication, authorization, encryption, and other security measures.
- Performance Testing: Measures the performance of the API, such as response time, load handling, and scalability under varying traffic levels.
- Reliability Testing: Ensures that the API is stable and functions properly under different conditions, including during high traffic or after long periods of use.
- Validation Testing: Verifies that the API returns the correct data and handles edge cases properly.
- Error Handling Testing: Ensures that the API handles erroneous inputs and returns appropriate error codes and messages.
API Testing Process
The following steps are typically followed in API testing:
- Define the API Requirements: Understand the requirements and functionality of the API. This includes reviewing API documentation, endpoints, parameters, and expected responses.
- Choose API Testing Tools: Select tools that can automate API tests, such as Postman, SoapUI, or REST Assured.
- Create Test Cases: Develop test cases based on the API endpoints, methods, and expected responses. Test cases should include a variety of scenarios, including valid, invalid, and boundary cases.
- Execute Tests: Run the API tests and check for successful execution, correct response codes, and expected data returned.
- Analyze Results: Review the test results to identify any discrepancies or issues with the API.
- Report Findings: Document the issues found during testing and report them to the development team for resolution.
Common API Testing Tools
There are several tools available for performing API testing, some of the most popular being:
- Postman: A popular tool for manual API testing that allows users to send HTTP requests, define test scripts, and view responses in various formats.
- SoapUI: A powerful testing tool for SOAP and REST APIs that supports functional, security, and load testing.
- REST Assured: A Java-based library for testing RESTful APIs, focusing on ease of use and automatic verification of responses.
- JMeter: Primarily a performance testing tool, JMeter also supports API testing, especially for load and stress testing APIs.
- Postman Newman's CLI: Allows users to run Postman collections from the command line, facilitating automated API testing.
Best Practices for API Testing
To ensure comprehensive and effective API testing, consider the following best practices:
- Understand API Documentation: Thoroughly read the API documentation to understand the available endpoints, methods, parameters, and expected responses.
- Automate API Tests: Automate repetitive API tests to ensure consistent coverage and quicker feedback during the development lifecycle.
- Test Positive and Negative Scenarios: Ensure the API works as expected for valid inputs (positive tests) and gracefully handles invalid inputs (negative tests).
- Test for Security Vulnerabilities: Always test for common security vulnerabilities such as SQL injections, authentication, and authorization flaws.
- Use Data-Driven Testing: Use data-driven testing to verify how the API behaves with different sets of inputs, ensuring it can handle various scenarios.
- Test Performance: Assess how the API performs under load and how it scales when handling multiple simultaneous requests.
Conclusion
API testing is a crucial part of the software development lifecycle, ensuring the reliability, performance, security, and functionality of APIs. By using the right tools and techniques, API testing can help identify issues early, improve the quality of software, and ensure smooth communication between different systems.
Understanding REST and SOAP APIs
APIs (Application Programming Interfaces) are essential components in modern software systems, enabling communication between different applications and services. Two of the most widely used types of APIs are REST (Representational State Transfer) and SOAP (Simple Object Access Protocol). Each has its own unique characteristics, advantages, and use cases. This section will help you understand the differences between REST and SOAP APIs, their features, and when to use them.
What is REST?
REST is an architectural style for designing networked applications. It uses a stateless communication model and relies on standard HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations. REST APIs are lightweight, flexible, and scalable, making them a popular choice for web services.
Key Characteristics of REST
- Stateless: Each REST request from the client to the server must contain all the information needed to understand and process the request. The server does not store any session information about the client between requests.
- Uses HTTP Methods: REST uses standard HTTP methods to perform operations on resources (e.g., GET for retrieving data, POST for creating data, PUT for updating data, DELETE for removing data).
- Resource-Based: In REST, every entity (such as a user, product, or order) is treated as a resource, and each resource is identified by a unique URL.
- Lightweight: REST APIs usually return data in lightweight formats, such as JSON or XML, making them efficient in terms of bandwidth and speed.
- Scalability: REST is highly scalable, as it is stateless and can handle large volumes of traffic efficiently.
When to Use REST APIs
REST APIs are ideal for the following situations:
- Web Applications: REST is commonly used for building web applications and services that require quick data exchanges and high performance.
- Mobile Applications: REST APIs are widely used in mobile application development because of their lightweight nature and efficient use of resources.
- Public APIs: REST is commonly used for public APIs where different clients (web, mobile, desktop) need to access the same resources.
- Microservices: REST is often used to build microservices-based architectures where different services interact with each other through lightweight, stateless communication.
What is SOAP?
SOAP is a protocol for exchanging structured information in the implementation of web services. Unlike REST, SOAP is a formalized and rigid specification that uses XML as its message format. SOAP APIs require more overhead than REST APIs but provide more robust security, reliability, and transaction support.
Key Characteristics of SOAP
- Protocol-Based: SOAP is a protocol, not an architectural style like REST. It defines a strict set of rules for structuring requests and responses.
- Uses XML: SOAP messages are formatted in XML, which makes them more complex and heavier than the JSON format used in REST.
- Stateful: Unlike REST, SOAP can be stateful, meaning it can maintain session information between requests. This is useful in scenarios that require multiple operations to be performed in sequence.
- Built-in Error Handling: SOAP has built-in error handling in the form of standard fault elements, which provide detailed error messages in case something goes wrong.
- Security Features: SOAP supports WS-Security, which provides advanced security features, such as encryption, authentication, and digital signatures, making it suitable for applications that require high security.
- Supports Transactions: SOAP supports ACID (Atomicity, Consistency, Isolation, Durability) transactions, making it suitable for applications that require complex business transactions, such as banking systems.
When to Use SOAP APIs
SOAP APIs are ideal for the following scenarios:
- Enterprise Applications: SOAP is often used in large enterprise applications where security, reliability, and transaction management are critical.
- Financial Services: SOAP is commonly used in the financial sector, where complex transactions and high security are required.
- Legacy Systems: SOAP is often used to integrate with legacy systems that require a formal protocol for communication.
- Multi-Platform Applications: SOAP is suitable for applications that need to support communication across different platforms and technologies.
Comparison of REST and SOAP
The table below summarizes the main differences between REST and SOAP APIs:
Feature | REST | SOAP |
---|---|---|
Protocol | Architectural style | Protocol |
Message Format | JSON, XML | XML |
State | Stateless | Stateful or Stateless |
Security | Depends on transport layer (SSL/TLS) | WS-Security (built-in security features) |
Performance | Faster due to lightweight message format (JSON) | Slower due to XML and overhead |
Standardization | Less rigid, flexible | Highly standardized |
Error Handling | Custom error messages | Built-in error handling (SOAP Faults) |
Transaction Support | No built-in transaction support | Supports ACID transactions |
Conclusion
In summary, both REST and SOAP have their advantages and are suited to different use cases. REST is lightweight, faster, and easier to implement, making it ideal for most modern web applications, mobile apps, and microservices architectures. SOAP, on the other hand, offers more robust features like security, transaction management, and reliability, making it better suited for enterprise applications, financial systems, and services that require a high level of security and formal transactions.
Tools for API Testing (Postman, REST Assured)
API testing is essential for ensuring the reliability, performance, and functionality of APIs. Tools like Postman and REST Assured are widely used to perform API testing, each offering unique features and advantages. This section will introduce these two popular API testing tools, their key features, and how they can be effectively used for API testing.
What is Postman?
Postman is one of the most popular API testing tools that allows developers and testers to easily test, debug, and automate API requests. It provides an intuitive user interface and a set of powerful features for testing REST, SOAP, and GraphQL APIs. Postman is widely used for functional testing, exploring API endpoints, and automating API tests.
Key Features of Postman
- API Request Creation: Postman allows you to create and send HTTP requests (GET, POST, PUT, DELETE, etc.) to test APIs. You can customize headers, parameters, body data, and authentication methods to simulate real-world scenarios.
- Collections and Environments: Postman allows you to organize your API tests into collections, which can be grouped and shared with team members. You can also set up environments for different stages (e.g., development, staging, production) to make testing more efficient.
- Automation: Postman supports automated testing with its built-in scripting feature. You can write test scripts using JavaScript to validate API responses, check status codes, and handle various testing scenarios.
- Mock Servers: Postman allows you to create mock servers to simulate API responses. This is useful when the actual API is unavailable or under development.
- Integration with CI/CD: Postman integrates with continuous integration and delivery (CI/CD) tools like Jenkins, GitHub, and GitLab, enabling automated testing and continuous monitoring of APIs.
- Visualizations: Postman allows you to visualize API response data in various formats like charts, tables, and graphs, which can help in better analysis of the results.
When to Use Postman
Postman is ideal for the following scenarios:
- Manual API Testing: Postman is perfect for manual testing of APIs where you can quickly create requests and analyze the responses without writing code.
- API Documentation: Postman allows you to document your API endpoints, request/response formats, and authentication mechanisms, making it easier for other developers to integrate with your API.
- Integration Testing: Postman is commonly used for integration testing to ensure that APIs communicate correctly with other services, databases, or components in the system.
- Automation of API Tests: Postman can be used for automating API test cases in a CI/CD pipeline, ensuring that new changes don’t break existing functionality.
What is REST Assured?
REST Assured is a Java-based library for testing REST APIs. It is commonly used for automating functional API tests, especially in Java projects. REST Assured provides an easy-to-use, fluent API for sending HTTP requests, validating responses, and handling common API testing tasks. It is popular for its integration with Java-based test frameworks like JUnit and TestNG.
Key Features of REST Assured
- Fluent Interface: REST Assured provides a fluent API, allowing you to chain method calls in a natural, readable way for writing tests efficiently.
- Easy to Set Up: REST Assured is simple to integrate with Java projects. It doesn’t require complex setup and can be added as a dependency in Maven or Gradle.
- Supports Various HTTP Methods: REST Assured supports all standard HTTP methods (GET, POST, PUT, DELETE, etc.) and allows you to customize headers, request bodies, and parameters.
- Response Validation: With REST Assured, you can easily validate API responses for status codes, headers, content types, and body content using built-in methods for assertions.
- JSON and XML Support: REST Assured provides convenient methods for parsing and validating JSON and XML responses, which are commonly used in RESTful APIs.
- Authentication Support: REST Assured supports multiple types of authentication, including basic authentication, OAuth, and form-based authentication, making it versatile for different API security scenarios.
- Integration with Test Frameworks: REST Assured integrates seamlessly with popular test frameworks like JUnit and TestNG, allowing you to write and run automated API tests.
When to Use REST Assured
REST Assured is ideal for the following scenarios:
- Automated API Testing: REST Assured is perfect for automating functional and regression tests of REST APIs. It is designed for developers and testers who are familiar with Java.
- Integration with Java Projects: If your project is Java-based and you need to automate API testing, REST Assured fits naturally into the development and testing workflow.
- Complex API Tests: REST Assured is a great choice for complex API testing scenarios that require advanced features like custom authentication, parameterization, and detailed response validation.
- Integration Testing: REST Assured can be used to validate how your APIs interact with other components or services within a system.
Postman vs. REST Assured
The table below summarizes the main differences between Postman and REST Assured:
Feature | Postman | REST Assured |
---|---|---|
Type | GUI tool | Java-based library |
Ease of Use | Beginner-friendly, user interface for manual testing | Requires knowledge of Java, suitable for automated testing |
Automation | Supports automation through collection runners and CI/CD integrations | Primarily used for automated testing with Java frameworks |
Integration | Integrates with various CI/CD tools like Jenkins | Integrates with Java testing frameworks like JUnit and TestNG |
Response Validation | Validates responses using built-in assertions and scripting | Validates responses with assertions and fluent API |
Support for Formats | Supports JSON, XML, and GraphQL | Supports JSON and XML |
Conclusion
Both Postman and REST Assured are powerful tools for API testing, but they serve different purposes and suit different workflows. Postman is ideal for quick, manual testing and automation, especially for users who prefer a graphical interface. REST Assured, on the other hand, is designed for developers who prefer to write Java-based automated tests and integrate them into their existing test frameworks. Depending on your testing needs and expertise, you can choose the tool that best fits your project requirements.
Writing API Test Cases
Writing effective API test cases is essential for ensuring that your APIs function as intended and meet the specified requirements. Well-written API test cases help in validating the correctness, performance, security, and reliability of the API. This section will cover the key components of writing API test cases, important considerations, and best practices.
What is an API Test Case?
An API test case is a set of conditions or actions used to verify if the API behaves as expected under certain conditions. It involves sending requests to the API and validating the responses to ensure that the API performs its intended functions correctly. API test cases can test various aspects, such as functional behavior, error handling, performance, and security.
Key Components of an API Test Case
To write comprehensive and effective API test cases, the following components should be included:
- Test Case ID: A unique identifier for the test case to distinguish it from others.
- API Endpoint: The URL of the API method being tested, including any query parameters or path variables.
- Request Method: The HTTP method used for the API request (e.g., GET, POST, PUT, DELETE).
- Request Headers: Any necessary headers required for the API request, such as authentication tokens, content type, or user-agent.
- Request Body: The data sent in the request, if applicable (for methods like POST, PUT, PATCH). This should include the required fields and any valid/invalid data.
- Expected Response: The anticipated response from the API, including status code, headers, and body content. This section specifies what should be returned when the request is successful or when it fails.
- Actual Response: The response actually received from the API. The test case will compare this with the expected response to validate the behavior of the API.
- Test Data: A set of input data used for testing the API, such as valid, invalid, or edge-case data.
- Preconditions: Any conditions that must be met before running the test, such as authentication, setup of test data, or specific environment configurations.
- Postconditions: Any conditions that should be true after the test has been executed, such as data cleanup or rollback actions.
- Pass/Fail Criteria: The conditions that determine if the test case passes or fails, based on the comparison of expected vs. actual responses.
Types of API Test Cases
API test cases can be categorized into different types depending on the aspect of the API being tested. Some common types of API test cases include:
- Functional Test Cases: These test cases validate the functionality of the API by verifying whether it produces the expected output for valid inputs and behaves correctly under different scenarios.
- Negative Test Cases: These test cases check how the API handles invalid or incorrect inputs, such as missing required fields, incorrect data types, or invalid authentication tokens.
- Boundary Test Cases: These test cases validate how the API handles input values at the boundaries of acceptable ranges, such as maximum and minimum lengths, sizes, or numbers.
- Performance Test Cases: These test cases measure the performance of the API under various conditions, such as high traffic, large requests, or heavy data processing.
- Security Test Cases: These test cases focus on testing the security aspects of the API, such as authentication, authorization, data encryption, and protection against attacks (e.g., SQL injection, XSS, CSRF).
- Compliance Test Cases: These test cases verify that the API adheres to relevant standards, regulations, and compliance requirements, such as GDPR or HIPAA.
Best Practices for Writing API Test Cases
Writing effective API test cases requires following best practices to ensure that the tests are comprehensive, maintainable, and easy to understand. Here are some best practices to keep in mind:
- Start with Clear Requirements: Ensure you have a clear understanding of the API’s functionality, business requirements, and any specific behavior to be tested. Collaborate with developers, product owners, and other stakeholders to clarify the requirements.
- Use Descriptive Test Case Names: Use meaningful names for your test cases that clearly describe the purpose of the test. This will make it easier to identify and maintain test cases over time.
- Test with Valid and Invalid Data: Always test with both valid and invalid data to check how the API responds to different inputs. Include edge cases, null values, and unexpected inputs.
- Include Error Scenarios: Ensure that your test cases cover all possible error scenarios, such as failed authentication, missing parameters, or server errors. This will help ensure robustness and error handling.
- Be Specific with Expected Results: Clearly define the expected results for each test case, including status codes, response times, and specific content in the response body. This helps in comparing the actual response with the expected one.
- Automate Where Possible: If you are writing repetitive or complex test cases, consider automating them using API testing tools like Postman, REST Assured, or JUnit. Automated tests can be run frequently, saving time and effort.
- Ensure Reusability: Create reusable test data and test case templates where possible. This ensures that you don’t need to rewrite test cases for every minor change and helps maintain consistency.
- Use Version Control: Store your test cases and test data in a version control system (e.g., Git) to track changes, collaborate with team members, and maintain historical versions of your tests.
Example of an API Test Case
Here is an example of how an API test case can be written for a simple login API:
Test Case ID: TC_API_001
API Endpoint: /api/login
Request Method: POST
Request Headers:
Content-Type: application/json
Request Body:
{
"username": "testuser",
"password": "password123"
}
Expected Response:
Status Code: 200
Response Body:
{
"status": "success",
"message": "Login successful",
"token": "abcd1234"
}
Actual Response:
Status Code: 200
Response Body:
{
"status": "success",
"message": "Login successful",
"token": "abcd1234"
}
Test Data:
Valid credentials (username: testuser, password: password123)
Preconditions:
User exists in the database with the given username and password
Postconditions:
User is logged in and an authentication token is issued
Pass/Fail Criteria:
The test case passes if the actual response matches the expected response
Conclusion
Writing effective API test cases is crucial for verifying that an API functions correctly and reliably. By including all relevant components, following best practices, and ensuring comprehensive test coverage, you can ensure that your API meets the requirements and behaves as expected in various scenarios. Automated testing can also help in speeding up the testing process and ensuring continuous integration and delivery of high-quality APIs.
Automating API Testing with Scripts
Automating API testing with scripts helps streamline the testing process, increase test coverage, and ensure that the APIs perform as expected across different environments and scenarios. Automation also enables continuous testing as part of the CI/CD pipeline, providing quick feedback on changes. In this section, we’ll discuss how to automate API testing using scripts, the benefits, tools, and best practices.
Why Automate API Testing?
Automating API testing offers numerous advantages, including:
- Faster Execution: Automated tests can run much faster than manual tests, helping you test APIs more frequently and efficiently.
- Consistency: Automated tests ensure that the same test steps are followed every time, reducing human error and ensuring consistency in test execution.
- Scalability: Automation allows you to easily scale your tests to include a large number of test cases, making it easier to test APIs under different conditions and with varying data inputs.
- Quick Feedback: Automated tests provide quick feedback on the health of your APIs, helping to identify issues early in the development cycle.
- Reusability: Once written, automated tests can be reused across different test environments and can be easily maintained and updated as the API changes.
Tools for Automating API Testing
Several tools can be used to automate API testing with scripts. Some popular ones include:
- Postman: Postman is a widely used tool for testing APIs. It allows you to write test scripts in JavaScript, which can be executed to verify API responses. Postman also integrates with CI/CD tools for automated testing.
- REST Assured: REST Assured is a Java-based library for testing RESTful APIs. It provides a fluent API for making HTTP requests and validating responses. It integrates with testing frameworks like JUnit and TestNG for automation.
- SoapUI: SoapUI is an API testing tool that supports both REST and SOAP APIs. It allows you to create and run automated functional, security, and load tests.
- JMeter: Apache JMeter is a popular open-source tool for performance and load testing APIs. It supports creating automated scripts for API testing and can simulate different load conditions.
- Karate: Karate is a testing framework for API testing that combines API testing and performance testing in a single framework. It uses Gherkin syntax for behavior-driven development (BDD) and provides easy-to-use scripts for API automation.
Writing API Test Scripts
When automating API tests, the script needs to validate the API’s behavior, check the response status, and ensure that the response content is correct. Below are the steps for writing an API test script:
- Define the API Endpoint: Identify the API endpoint that you want to test and the type of request (GET, POST, PUT, DELETE, etc.).
- Prepare Request Data: Define the required input data for the API request, such as parameters, headers, and body content. This could include authentication details, content type, and request payload.
- Send Request: Use the appropriate method in the automation tool to send the API request. For example, in Postman, you can use the built-in HTTP methods to make requests.
- Validate Response: Check the response status code, headers, and body content. Verify that the status code matches the expected value (e.g., 200 OK for a successful request) and that the response body contains the expected data.
- Handle Assertions: Use assertions to verify that the actual response matches the expected response. For example, you can assert that the response body contains a specific field, or that the response time is within an acceptable limit.
- Log Results: Log the results of each test case, including passed or failed status and any error messages. This helps with debugging and reporting test outcomes.
Example of API Test Automation Script
Here’s an example of an API test automation script using Postman’s scripting feature, written in JavaScript:
// Set up the test URL and request details
const apiUrl = "https://api.example.com/login";
const requestBody = {
username: "testuser",
password: "password123"
};
// Send the POST request
pm.sendRequest({
url: apiUrl,
method: "POST",
header: {
"Content-Type": "application/json"
},
body: {
mode: "raw",
raw: JSON.stringify(requestBody)
}
}, function (err, res) {
// Assert the status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Assert the response body
pm.test("Response body contains success message", function () {
pm.response.to.have.jsonBody('status', 'success');
pm.response.to.have.jsonBody('message', 'Login successful');
});
// Log the response time
pm.test("Response time is less than 200ms", function () {
pm.response.to.have.responseTime.lessThan(200);
});
});
Best Practices for Automating API Testing with Scripts
Here are some best practices to follow while automating API tests:
- Keep Test Cases Simple: Write simple and clear test cases that focus on specific functionality. Avoid making tests too complex or too broad.
- Reusability: Organize your test scripts in a modular way so they can be reused across different test cases. This can help reduce redundancy and improve maintainability.
- Data-Driven Testing: Use data-driven testing to run the same test case with different sets of input data. This helps ensure that the API behaves as expected under various conditions.
- Handle Test Data: Use mock data or test-specific data to avoid affecting production data. Ensure that your test data is isolated from real user data.
- Integrate with CI/CD: Integrate automated API tests into the Continuous Integration/Continuous Delivery (CI/CD) pipeline to run tests automatically whenever new code is deployed or changes are made.
- Use Assertions Effectively: Use assertions to check that the response data is correct. Assert on response status, headers, body, and performance metrics.
- Focus on Error Handling: Ensure that your scripts handle error conditions properly, such as network failures or server errors, and include logic to retry requests when necessary.
Conclusion
Automating API testing with scripts helps increase the efficiency and accuracy of your testing process. It allows you to quickly verify the behavior of your APIs, catch issues early, and integrate testing into your CI/CD pipeline. By following best practices and using the right tools, you can build reliable and maintainable automated API tests that ensure the quality and performance of your APIs.
Validating API Responses and Error Codes
Validating API responses and error codes is a critical part of API testing. It ensures that the API behaves as expected and returns the appropriate responses for different requests. Proper validation helps identify issues early, ensuring that the API provides correct and consistent data to users or other systems. In this section, we’ll explore how to validate API responses and error codes effectively using various tools and methods.
Why Validate API Responses?
Validating API responses ensures that the API is working correctly and meets the expected requirements. Some key reasons to validate API responses include:
- Correctness: Ensure that the API returns the correct data, including status codes, response body, and headers.
- Consistency: Ensure that the API behaves consistently across different scenarios, environments, and inputs.
- Performance: Check that the API performs within acceptable limits, including response time and resource utilization.
- Security: Validate that the API does not expose sensitive data and that proper authentication and authorization mechanisms are in place.
Validating API Responses
API responses consist of several elements that need to be validated, including:
- Status Code: The HTTP status code indicates the result of the API request. Common status codes include 200 (OK), 201 (Created), 400 (Bad Request), 404 (Not Found), and 500 (Internal Server Error).
- Response Body: The response body contains the data returned by the API. It’s important to validate that the data is correct, complete, and in the expected format (e.g., JSON, XML).
- Headers: API response headers contain metadata about the response, such as content type, date, and caching information. These headers should be validated to ensure they match the expected values.
- Response Time: Ensure that the API responds within an acceptable time frame. High latency can affect user experience and performance.
Validating Error Codes
Error codes are returned by the API when something goes wrong with the request. Validating error codes is crucial for ensuring that the API handles failures properly and provides useful feedback to users or other systems. Common error codes include:
- 400 – Bad Request: The request is malformed or contains invalid parameters.
- 401 – Unauthorized: The request lacks valid authentication credentials.
- 403 – Forbidden: The request is valid, but the server refuses to authorize it.
- 404 – Not Found: The requested resource could not be found on the server.
- 500 – Internal Server Error: A generic error occurred on the server while processing the request.
Best Practices for Validating API Responses and Error Codes
Here are some best practices to follow while validating API responses and error codes:
- Check Status Codes: Always validate the HTTP status code to ensure that the API request was processed correctly. For example, a successful GET request should return a 200 status code, while a POST request should return a 201 if the resource was created.
- Validate Response Body: Ensure that the response body contains the expected data. For example, if you are testing a user creation endpoint, verify that the response body contains the newly created user’s ID and other relevant details.
- Test Error Responses: For each API endpoint, test different invalid inputs and scenarios to ensure that the API returns the correct error code and message. This includes testing for missing parameters, incorrect data types, and unauthorized access.
- Use Assertions: Use assertions to check that the response matches the expected values. For example, assert that the response body contains a specific field, or that the status code is 200 for a successful request.
- Verify Headers: Make sure that the response headers contain the correct values, such as the correct content type (e.g., application/json) and caching policies.
- Test Boundary Cases: Test the API with edge cases, such as empty fields, large data inputs, and invalid formats, to ensure that the API handles them gracefully.
Example of Validating API Responses
Here’s an example of how you can validate API responses using Postman’s script feature:
// Send the GET request
pm.sendRequest({
url: "https://api.example.com/users/1",
method: "GET"
}, function (err, res) {
// Validate status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Validate response body
pm.test("Response body contains user data", function () {
pm.response.to.have.jsonBody('id', 1);
pm.response.to.have.jsonBody('name', 'John Doe');
});
// Validate response headers
pm.test("Response contains correct Content-Type header", function () {
pm.response.to.have.header("Content-Type", "application/json; charset=utf-8");
});
// Validate error response for invalid user ID
pm.sendRequest({
url: "https://api.example.com/users/9999",
method: "GET"
}, function (err, res) {
pm.test("Status code is 404 for invalid user", function () {
pm.response.to.have.status(404);
});
});
});
Conclusion
Validating API responses and error codes is a fundamental part of API testing. Ensuring that the API returns the correct status codes, data, and headers, and that it handles errors appropriately, helps ensure that the API functions as expected and provides a smooth user experience. By following best practices and using appropriate tools, you can effectively validate API responses and error codes and improve the quality of your APIs.
Introduction to Mobile Testing
Mobile testing involves evaluating the functionality, usability, and performance of mobile applications across different devices, operating systems, and network conditions. As mobile devices have become integral to everyday life, it’s crucial for mobile apps to provide a seamless and reliable experience for users. Mobile testing is essential to ensure that applications function well across various screen sizes, resolutions, and mobile platforms such as Android and iOS.
Why Mobile Testing is Important?
Mobile testing is crucial for the following reasons:
- Device Fragmentation: With numerous mobile devices available in the market, testing ensures that the application is compatible with different screen sizes, resolutions, and hardware configurations.
- Operating System Variations: Mobile apps need to function properly on different operating systems like Android and iOS, as well as various versions within each OS. Testing ensures compatibility with multiple OS versions.
- Network Conditions: Mobile apps are often used in varying network conditions, such as 3G, 4G, Wi-Fi, and even offline. Testing helps ensure that the app performs well under different network speeds and connectivity scenarios.
- User Expectations: Users have high expectations for mobile apps, and any issues, such as performance lags, crashes, or poor usability, can lead to negative reviews and uninstallations. Proper testing ensures that the app meets user expectations.
Types of Mobile Testing
Mobile testing can be categorized into different types based on the areas of focus:
- Functional Testing: Verifies that the app functions as expected, including features like login, registration, navigation, and interactions with UI elements.
- Usability Testing: Evaluates the user experience, ensuring that the app is intuitive, easy to navigate, and visually appealing on different devices.
- Performance Testing: Assesses the app’s performance under different conditions, such as varying network speeds, battery consumption, CPU usage, and responsiveness.
- Compatibility Testing: Ensures that the app works correctly across different devices, screen sizes, resolutions, and operating system versions.
- Security Testing: Validates that the app is secure from vulnerabilities like data breaches, insecure communications, and unauthorized access.
- Regression Testing: Ensures that new updates or changes to the app do not break existing functionality.
- Device Testing: Focuses on ensuring that the app works correctly on different physical devices, testing for hardware compatibility and device-specific features.
Challenges in Mobile Testing
Mobile testing comes with its own set of challenges, including:
- Device Fragmentation: The variety of mobile devices, screen sizes, resolutions, and configurations makes it challenging to test on every possible device.
- Operating System Differences: Different versions of Android and iOS may exhibit different behaviors, making cross-platform testing necessary.
- Performance on Low-End Devices: Testing how the app performs on low-end devices with limited processing power and memory is crucial for ensuring a smooth user experience across devices.
- Connectivity Issues: Mobile apps often rely on internet connectivity, and testing how the app behaves under varying network conditions (3G, 4G, Wi-Fi, offline) is essential to simulate real-world usage.
- App Updates: Ensuring that updates do not introduce new bugs or break existing functionality requires thorough testing after each release.
Best Practices for Mobile Testing
To conduct effective mobile testing, consider the following best practices:
- Test on Real Devices: While emulators and simulators can be useful for early-stage testing, it’s important to test on real devices to ensure the app performs accurately in real-world conditions.
- Test Across Multiple Devices: Test the app on various devices, including different brands, screen sizes, resolutions, and operating system versions, to ensure compatibility.
- Consider Network Variability: Simulate different network conditions, including low bandwidth, high latency, or no network at all, to assess how the app performs in different scenarios.
- Automate Testing Where Possible: Automate repetitive testing tasks, such as regression testing, to save time and improve efficiency.
- Test Battery and Resource Consumption: Ensure that the app doesn’t drain the device’s battery excessively and that it doesn’t consume too many resources (CPU, memory).
- Perform Security Testing: Check for potential security risks, such as data leakage, vulnerabilities in authentication mechanisms, and encrypted data storage.
Mobile Testing Tools
Various tools can help in mobile testing, such as:
- Appium: An open-source tool for automating mobile applications on Android and iOS devices.
- Selendroid: A test automation framework for Android applications, supporting hybrid and native apps.
- Robot Framework: A generic test automation framework that can be used for mobile apps using Appium or Selenium libraries.
- Ranorex: A comprehensive test automation tool for mobile, desktop, and web applications.
- Calabash: An open-source mobile testing framework for Android and iOS apps, primarily used for acceptance testing.
Conclusion
Mobile testing is a critical component of ensuring a high-quality mobile application that delivers a seamless experience for users. With the rapid growth of mobile devices and applications, it’s essential to ensure that apps are functional, user-friendly, and perform well under various conditions. By following best practices, leveraging the right tools, and testing across a range of devices and scenarios, you can ensure that your mobile apps meet user expectations and perform as intended across platforms.
Differences Between Mobile and Web Testing
Mobile testing and web testing both involve the process of evaluating the functionality and performance of applications, but they differ in several aspects due to the unique characteristics of mobile devices and web environments. Below, we outline the key differences between mobile testing and web testing:
1. Device & Platform Variety
Mobile Testing: Mobile apps must be tested on various physical devices, including smartphones and tablets, with different operating systems (Android, iOS) and versions. Device fragmentation is a major challenge in mobile testing.
Web Testing: Web applications are typically tested on different browsers and operating systems (Windows, macOS, Linux) rather than specific devices. Web testing often focuses on browser compatibility and responsiveness.
2. User Interface (UI) Design
Mobile Testing: Mobile apps must be designed for small screens with touch-based interactions. Testing involves evaluating the responsiveness of touch gestures, screen size adaptability, and ensuring the app functions well across different screen resolutions and orientations.
Web Testing: Web apps are designed for larger screens and are typically interacted with using a mouse and keyboard. Testing focuses on ensuring that elements such as buttons, links, and forms are functional and that the layout adapts to different screen sizes through responsive design.
3. Network Conditions
Mobile Testing: Mobile apps often operate under varying network conditions, including 3G, 4G, Wi-Fi, and offline scenarios. Mobile testing needs to evaluate how the app behaves under different connectivity conditions and how it handles interruptions like signal loss.
Web Testing: While web apps can also be affected by network conditions, they generally depend on a stable internet connection. Testing web applications often focuses on ensuring performance under varying internet speeds.
4. Performance Testing
Mobile Testing: Mobile app performance testing includes evaluating battery consumption, CPU, memory usage, and app responsiveness on different devices. It also involves testing how the app performs on low-end devices.
Web Testing: Web performance testing focuses on how fast the web app loads across different browsers and network conditions, as well as its ability to handle a high number of concurrent users.
5. Security Testing
Mobile Testing: Mobile security testing includes assessing app security for sensitive data storage, communication encryption, device-specific security features like fingerprint authentication, and protection against unauthorized access.
Web Testing: Web security testing focuses on protecting against vulnerabilities such as cross-site scripting (XSS), SQL injection, and ensuring secure data transmission (HTTPS). It also includes testing user authentication and authorization mechanisms.
6. Testing Devices
Mobile Testing: Mobile testing requires testing on a variety of real devices or emulators/simulators to account for various screen sizes, hardware configurations, and operating system versions. Real-device testing ensures more accurate results, though it can be resource-intensive.
Web Testing: Web testing typically involves testing on different browsers (Chrome, Firefox, Safari, etc.) and browser versions. Web apps are generally tested on desktops or laptops, though compatibility with mobile browsers is also important for responsive designs.
7. Interruption Handling
Mobile Testing: Mobile apps must be tested for interruptions, such as incoming calls, messages, notifications, and app switching. This ensures that the app handles interruptions gracefully without losing data or crashing.
Web Testing: While web apps may also face interruptions like browser crashes or network failures, they don’t experience the same set of interruptions common to mobile devices. Web apps are tested for session timeouts, browser crashes, and unexpected refreshes.
8. Updates and Maintenance
Mobile Testing: Mobile apps often require frequent updates to adapt to new OS versions, device capabilities, and user expectations. Testing must ensure that updates don’t break existing features and that the app remains compatible with new devices and OS versions.
Web Testing: Web applications are typically updated more regularly through server-side changes, and testing focuses on ensuring that new updates don’t negatively affect the functionality across different browsers and devices.
9. Automation
Mobile Testing: Automation in mobile testing can be more challenging due to device fragmentation, variable network conditions, and the need for real device testing. Tools like Appium, Espresso, and XCUITest are commonly used for automating mobile tests.
Web Testing: Web testing automation is relatively easier, especially with the availability of tools like Selenium, Cypress, and TestCafe. Automation focuses on browser compatibility, UI testing, and performance testing.
10. Tools for Testing
Mobile Testing: Popular mobile testing tools include Appium, Espresso, XCUITest, Robot Framework, and Calabash, which help automate mobile app testing across multiple devices.
Web Testing: Web testing tools include Selenium, Cypress, WebDriverIO, and TestCafe, which help automate browser testing for web applications.
Conclusion
While mobile and web testing share some common principles, they differ significantly in the way they are carried out due to the unique characteristics of mobile devices and web platforms. Mobile testing requires careful attention to device variations, network conditions, and performance on different screen sizes and hardware configurations, while web testing focuses more on browser compatibility, responsiveness, and server-side performance. Both types of testing are essential for ensuring the quality and reliability of applications across platforms.
Testing Mobile App Functionality (Native, Hybrid, Web Apps)
Mobile app functionality testing ensures that the app works as expected across different mobile platforms. The type of mobile app (Native, Hybrid, or Web) determines the testing strategy and tools used. Each type of app has unique features that require different testing approaches.
1. Native Mobile Apps
Native Apps are developed specifically for one platform (e.g., iOS or Android) using platform-specific programming languages like Swift (for iOS) or Java/Kotlin (for Android). Native apps are installed directly on the device and have full access to device features like the camera, GPS, and sensors.
Testing Native Mobile App Functionality
Testing native apps focuses on ensuring that the app performs correctly on the intended platform, handling device-specific features and functionality. Key testing areas include:
- UI/UX Testing: Ensuring that the app follows platform-specific design guidelines, offers a user-friendly interface, and operates intuitively.
- Performance Testing: Measuring the app’s performance, including speed, response time, memory usage, and CPU consumption.
- Device Feature Testing: Verifying that the app interacts correctly with hardware features like the camera, GPS, sensors, microphone, and more.
- Security Testing: Ensuring that sensitive data is protected, and the app is secure against unauthorized access.
2. Hybrid Mobile Apps
Hybrid Apps combine elements of both native and web apps. They are developed using web technologies (HTML, CSS, JavaScript) and wrapped in a native container (e.g., using frameworks like Apache Cordova or Ionic) to be deployed on multiple platforms.
Testing Hybrid Mobile App Functionality
Testing hybrid apps involves ensuring that the app functions properly across different platforms and performs as expected on both web and native components. Key testing areas include:
- Cross-Platform Testing: Verifying that the app works correctly on multiple platforms (iOS, Android, etc.) and devices with different screen sizes.
- Integration Testing: Ensuring that the hybrid components (native and web) work seamlessly together, without breaking functionality.
- Performance Testing: Testing the app's performance, including loading time, responsiveness, and resource usage across various devices.
- Compatibility Testing: Ensuring the app functions properly on various OS versions and devices.
3. Web Mobile Apps
Web Apps are mobile-optimized websites designed to function on a mobile browser. They are not installed on the device and do not have access to device features like native apps. Web apps are accessed via URLs and rely on an internet connection to work.
Testing Web Mobile App Functionality
Testing web apps focuses on their functionality in mobile browsers and their ability to adapt to different screen sizes and resolutions. Key testing areas include:
- Responsiveness Testing: Ensuring that the web app adapts to different screen sizes and resolutions on mobile devices.
- Cross-Browser Testing: Verifying that the web app works correctly across different mobile browsers (e.g., Safari, Chrome, Firefox).
- Network Performance Testing: Checking how the app performs under various network conditions (e.g., 3G, 4G, Wi-Fi).
- Security Testing: Ensuring secure communication (e.g., HTTPS) and protecting sensitive data in transit.
4. Common Testing Areas for All Types of Mobile Apps
Regardless of whether the app is native, hybrid, or web-based, there are common areas of functionality that need to be tested:
- Authentication and Authorization: Testing the login and registration processes, ensuring that user credentials and permissions are properly handled.
- Data Synchronization: Ensuring that data is correctly synced between the mobile app and the backend server, particularly for apps that require offline functionality.
- Error Handling and Validation: Verifying that the app handles errors gracefully, providing users with clear error messages when necessary.
- Push Notifications: Ensuring that push notifications are correctly received and displayed on the mobile device.
- App Launch and Shutdown: Testing the app’s behavior during startup, shutdown, and transitions between states (e.g., background to foreground).
Conclusion
Mobile app functionality testing is essential to ensure that the app delivers a seamless user experience, works as expected on different devices and platforms, and performs optimally under various conditions. Native, hybrid, and web apps each require specialized testing techniques to ensure their success. By thoroughly testing each type of app, developers can identify and fix issues early in the development process, leading to higher-quality mobile applications.
Simulators vs. Real Devices
In mobile app testing, one of the key debates is whether to test on simulators/emulators or real devices. Both have their pros and cons, and the decision largely depends on the specific needs of the test, the type of app being tested, and the available resources. This section explores the differences between simulators/emulators and real devices, the advantages and disadvantages of each, and when to use them for testing.
What are Simulators and Emulators?
Simulators are software-based tools that mimic the behavior of a mobile device’s operating system and hardware, but they do not replicate the exact physical characteristics of the device. They simulate the environment of a specific OS (e.g., iOS) but do not account for hardware features like CPU performance, touch response, or real-world device interaction.
Emulators are similar to simulators but often replicate both the software environment (OS) and the hardware of a device. They are commonly used for Android testing, where tools like Android Studio’s emulator can mimic different Android devices, OS versions, and configurations.
Advantages of Simulators and Emulators
- Cost-Effective: Simulators and emulators are usually free or come at a low cost compared to real devices. This makes them an affordable option for testing.
- Easy to Set Up: These tools can be quickly installed and configured, allowing testers to start testing without needing to physically manage multiple devices.
- Access to Multiple Device Configurations: Simulators and emulators can simulate a wide range of devices, OS versions, and screen sizes, enabling testers to quickly test different configurations.
- Automated Testing: Since simulators and emulators are software-based, they are ideal for automated testing where multiple tests can be run on various device configurations simultaneously.
Disadvantages of Simulators and Emulators
- Inaccurate Representation: Simulators and emulators cannot perfectly replicate the behavior of real devices, especially in terms of performance, touchscreen sensitivity, battery usage, and hardware interactions.
- No Real-World Environment: They do not capture real-world factors such as network speed, device temperature, or real-time background activities that can affect app performance.
- Limited Hardware Testing: Features like GPS, camera, or accelerometer may not function accurately on simulators or emulators, which can lead to gaps in testing.
- Performance Differences: The performance of an app on a simulator/emulator may not reflect how the app will perform on a physical device, especially in terms of memory usage and load times.
Advantages of Real Devices
- Accurate Testing: Real devices provide a true representation of how an app will behave in the hands of users. They can test hardware features like the camera, GPS, accelerometer, and more, which simulators and emulators may not fully replicate.
- Real-World Performance: Testing on real devices provides a true measure of the app’s performance, including factors like CPU usage, battery life, and memory consumption.
- Better User Experience Simulation: Real devices allow testers to experience the app as end users would, with real touch interactions, screen responsiveness, and device behavior.
- Network and Hardware Testing: Real devices can simulate real-world network conditions, such as 4G, 3G, Wi-Fi, and low bandwidth scenarios, helping testers observe how the app behaves under various network conditions.
Disadvantages of Real Devices
- Costly: Real devices are more expensive to acquire and maintain, especially when testing across multiple device models and OS versions.
- Limited Availability: It may not be feasible to have access to every device and OS version, particularly with the wide variety of mobile devices in the market.
- Time-Consuming Setup: Setting up real devices for testing can be time-consuming, as it may involve installing apps, updating OS versions, and configuring network settings manually.
- Manual Testing Overhead: Real devices are more suited to manual testing. Testers need to physically interact with the devices, which can slow down the testing process compared to automated tests on simulators/emulators.
When to Use Simulators/Emulators vs. Real Devices
Both simulators/emulators and real devices have their places in mobile app testing. Here’s when you might choose one over the other:
- Simulators/Emulators:
- When you need to run quick tests on multiple devices and OS versions.
- When testing app functionality without focusing on performance or real-world conditions.
- When you have a limited budget and resources and need a cost-effective solution.
- When automating tests that don’t require real-world interactions or hardware features.
- Real Devices:
- When you need to test the performance of your app on real hardware, such as memory usage, CPU load, or battery life.
- When testing hardware features like GPS, camera, microphone, etc., that simulators/emulators can't replicate accurately.
- When you want to simulate real-world user behavior and ensure the best user experience.
- When testing app behavior under various network conditions like 4G, Wi-Fi, or weak signals.
Conclusion
While simulators and emulators provide a cost-effective and convenient way to test mobile apps, they cannot fully replicate the behavior of real devices. Real devices are essential for ensuring accurate performance, hardware interaction, and real-world usability. The best approach to mobile app testing often involves a combination of both simulators/emulators and real devices, depending on the type of test being conducted, the stage of development, and available resources.
What is Security Testing?
Security testing is a type of software testing that focuses on identifying vulnerabilities, threats, risks, and potential security breaches in an application or system. The primary goal of security testing is to ensure that the software is protected against unauthorized access, data breaches, and any other security-related issues that could compromise the system’s integrity, confidentiality, and availability.
Importance of Security Testing
With the increasing number of cyberattacks, data breaches, and vulnerabilities, security testing has become a critical aspect of software development. Security flaws can lead to severe consequences, such as loss of sensitive data, damage to brand reputation, legal implications, and financial losses. By performing security testing, you can ensure that your application is resilient to common security threats and provides a safe environment for users and data.
Objectives of Security Testing
- Identify Vulnerabilities: Security testing helps identify vulnerabilities in an application that could be exploited by attackers.
- Ensure Data Protection: It verifies that the application securely handles sensitive data, such as passwords, personal information, and payment details.
- Verify Authentication and Authorization: It ensures that proper authentication and authorization mechanisms are in place to prevent unauthorized access.
- Compliance with Security Standards: Security testing ensures that the application complies with relevant security regulations and standards, such as GDPR, HIPAA, and PCI-DSS.
- Prevent Security Breaches: The ultimate goal is to prevent security breaches, hacking attempts, and data leaks that could negatively impact the system and its users.
Types of Security Testing
- Vulnerability Scanning: This is the process of identifying known vulnerabilities in the system using automated tools. It helps in identifying weaknesses in the system’s security configuration.
- Penetration Testing (Pen Testing): Penetration testing simulates real-world attacks to identify security weaknesses. Testers attempt to exploit vulnerabilities and assess the security of the system under attack.
- Security Audits: A security audit involves reviewing the entire application or system’s security posture to identify areas that need improvement. This includes reviewing code, configurations, and security policies.
- Risk Assessment: This process involves evaluating potential security risks and determining the impact of these risks on the system. It helps in prioritizing security efforts based on risk severity.
- Authentication Testing: Authentication testing ensures that the application verifies the identity of users correctly using techniques such as password policies, multi-factor authentication, and secure login mechanisms.
- Authorization Testing: Authorization testing ensures that authenticated users have access only to the resources they are authorized to access. It tests role-based access control (RBAC) and permissions.
Common Security Vulnerabilities
Security testing aims to uncover common security vulnerabilities that could put the application at risk. Some of the most common vulnerabilities include:
- SQL Injection: Attackers inject malicious SQL code into a query, potentially gaining unauthorized access to the database and altering or stealing data.
- Cross-Site Scripting (XSS): XSS vulnerabilities allow attackers to inject malicious scripts into web pages, which can be executed by unsuspecting users' browsers.
- Cross-Site Request Forgery (CSRF): This type of attack tricks users into making unintended requests, potentially compromising their account or data.
- Insecure Direct Object References (IDOR): Attackers manipulate input to access unauthorized resources, such as files or databases, that they are not authorized to view.
- Broken Authentication: Weak or poorly implemented authentication mechanisms can allow attackers to bypass security measures and gain unauthorized access.
- Data Exposure: Insufficient encryption or improper handling of sensitive data can expose information such as passwords, credit card details, or personal information.
Security Testing Process
The security testing process typically follows these steps:
- Planning and Scoping: Define the security requirements, objectives, and scope of testing. This includes identifying the assets to be tested, such as the application, server, network, or database.
- Test Case Design: Create test cases that cover different security aspects, such as authentication, authorization, data protection, and vulnerability identification.
- Test Execution: Conduct the security tests, such as penetration tests, vulnerability scans, and security audits, on the system to identify potential weaknesses.
- Reporting and Remediation: Document the findings, including identified vulnerabilities, risks, and their potential impact. Provide recommendations for remediation and security improvements.
- Re-Testing: After the remediation steps are implemented, perform re-testing to ensure that the vulnerabilities have been fixed and no new issues have been introduced.
Tools for Security Testing
Several tools are available to assist with security testing, helping testers identify vulnerabilities and perform penetration tests. Some popular security testing tools include:
- OWASP ZAP: An open-source web application security scanner that helps identify security vulnerabilities in web applications.
- Burp Suite: A popular suite of tools for performing security testing of web applications, including vulnerability scanning and penetration testing.
- Nessus: A vulnerability scanner that helps identify security flaws, misconfigurations, and other vulnerabilities in systems and networks.
- Wireshark: A network protocol analyzer that helps capture and analyze network traffic, identifying potential security issues.
- Metasploit: A penetration testing tool that helps security professionals identify, exploit, and validate vulnerabilities in systems and applications.
Conclusion
Security testing is a crucial part of the software development lifecycle, ensuring that applications are secure from potential threats and vulnerabilities. By performing thorough security testing, you can safeguard your application, protect user data, and maintain the integrity and trustworthiness of your system. Regular security testing helps identify vulnerabilities early, reducing the risk of security breaches and protecting both users and businesses from the consequences of cyberattacks.
Common Vulnerabilities (OWASP Top 10)
The OWASP Top 10 is a list of the ten most critical web application security risks, compiled by the Open Web Application Security Project (OWASP). These vulnerabilities are common in many web applications, and understanding them is essential for both developers and testers to ensure secure software. Below are the OWASP Top 10 vulnerabilities:
1. Injection
Injection attacks occur when an attacker sends untrusted data into a program, causing it to execute unintended commands. The most common types of injection attacks are SQL injection, command injection, and XML injection. These attacks can lead to unauthorized access to databases, servers, or sensitive data.
- Example: SQL injection allows attackers to execute arbitrary SQL code on a database to retrieve, modify, or delete data.
2. Broken Authentication
Broken authentication occurs when an application does not properly verify the identity of a user or fails to protect session management mechanisms. This vulnerability allows attackers to impersonate legitimate users, hijack user sessions, or gain unauthorized access to an application.
- Example: Weak password policies or improper session management can allow attackers to gain access to user accounts.
3. Sensitive Data Exposure
Sensitive data exposure occurs when sensitive data, such as passwords, credit card information, or personal details, is exposed due to weak encryption or improper handling. This vulnerability can lead to data breaches, identity theft, and financial losses.
- Example: Storing passwords in plaintext or failing to encrypt sensitive data during transmission can expose it to attackers.
4. XML External Entities (XXE)
XML External Entities (XXE) attacks occur when an XML parser processes malicious input containing external entities. These attacks can allow attackers to view sensitive files on the system, perform server-side request forgery (SSRF), or cause denial of service (DoS) attacks.
- Example: A vulnerable XML parser can be tricked into fetching external resources, leading to unauthorized information disclosure.
5. Broken Access Control
Broken access control occurs when an application does not properly enforce restrictions on what authenticated users are allowed to do. This can lead to unauthorized access to sensitive data or functionality based on the user’s role or permissions.
- Example: A user with normal privileges accessing admin pages due to improper role-based access control.
6. Security Misconfiguration
Security misconfiguration occurs when an application or its environment is not securely configured, leaving it vulnerable to attacks. This includes improper settings in web servers, databases, and application frameworks, as well as unused features or services that are left enabled.
- Example: Leaving default credentials enabled, misconfigured security headers, or exposing unnecessary services in the production environment.
7. Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) occurs when an attacker injects malicious scripts into web pages that are later executed in the browser of unsuspecting users. XSS can lead to stolen user data, session hijacking, or malware installation.
- Example: A script injected into a website’s comment section could steal the user’s cookies and send them to the attacker.
8. Insecure Deserialization
Insecure deserialization occurs when untrusted data is deserialized and executed by the application. Attackers can exploit insecure deserialization to execute arbitrary code, bypass authentication, or manipulate data.
- Example: Deserializing untrusted data from a user input can lead to remote code execution (RCE) vulnerabilities.
9. Using Components with Known Vulnerabilities
Using components with known vulnerabilities occurs when an application uses outdated or unpatched software components, such as libraries or frameworks, which contain known security vulnerabilities.
- Example: Using an outdated version of a library that has known security flaws, which can be exploited by attackers.
10. Insufficient Logging and Monitoring
Insufficient logging and monitoring occurs when an application does not properly log security events or monitor for suspicious activity. Without adequate logging, it becomes difficult to detect and respond to security incidents in a timely manner.
- Example: Failure to log failed login attempts or unusual API requests, making it harder to detect and mitigate attacks.
Conclusion
Understanding the OWASP Top 10 vulnerabilities is crucial for developers and security testers to build secure applications and prevent common threats. By addressing these security risks and implementing best practices, organizations can protect their users, data, and systems from potential attacks and breaches.
Penetration Testing Basics
Penetration Testing (often referred to as "pen testing" or "ethical hacking") is a simulated cyber attack conducted by security professionals to identify vulnerabilities in an application, network, or system. The goal is to uncover weaknesses before malicious attackers can exploit them. Penetration testing involves assessing the security of a system by testing its defenses against various attack techniques.
What is Penetration Testing?
Penetration testing is a proactive approach to discovering vulnerabilities in a system by attempting to exploit them, simulating the actions of potential cybercriminals. Pen testers mimic various attack strategies to assess the security posture of systems, applications, and networks. This helps in identifying areas of weakness and suggests improvements to enhance security.
Key Phases of Penetration Testing
Penetration testing generally follows a systematic process. The main phases of penetration testing include:
- Planning & Information Gathering: In this phase, the scope of the test is defined, and information about the target system is collected through methods like footprinting, scanning, and enumeration.
- Vulnerability Assessment: Once the information is gathered, penetration testers analyze the data to identify potential vulnerabilities in the system, such as outdated software or misconfigurations.
- Exploitation: In this phase, testers attempt to exploit the identified vulnerabilities to gain unauthorized access to the system or data. The goal is to determine the extent to which an attacker could compromise the system.
- Post-Exploitation: After gaining access, testers perform further analysis to understand the impact of the compromise, such as access to sensitive data or escalating privileges.
- Reporting: The final phase involves documenting the findings, including the vulnerabilities discovered, exploitation techniques used, and recommendations for fixing the issues.
Types of Penetration Testing
Penetration testing can be performed using different approaches, depending on the level of knowledge the tester has about the target system:
- Black-Box Testing: In black-box testing, the tester has no prior knowledge of the system. The goal is to simulate an external attacker who has no access to internal information.
- White-Box Testing: White-box testing involves complete knowledge of the system, including source code and architecture. This type of test simulates an internal attacker or someone who already has access to the network.
- Gray-Box Testing: Gray-box testing is a combination of both black-box and white-box testing. The tester has partial knowledge of the system, often limited to specific areas, simulating a user with privileged access.
Penetration Testing Tools
Penetration testers use various tools to assist in identifying vulnerabilities and exploiting them. Some popular penetration testing tools include:
- Metasploit: A powerful framework for developing and executing exploit code against a remote target machine. It helps automate attacks and simulate real-world cyber threats.
- Nmap: A network scanning tool used to discover devices, services, and vulnerabilities on a network. It helps identify open ports and services that may be exploited.
- Burp Suite: A web vulnerability scanner that helps identify security flaws in web applications. It provides a set of tools for scanning, intercepting, and manipulating HTTP requests and responses.
- Wireshark: A network protocol analyzer that captures and inspects network traffic. It helps identify sensitive data transmitted over the network or unusual patterns of communication.
Benefits of Penetration Testing
Penetration testing provides several benefits, including:
- Identifying Vulnerabilities: Penetration testing helps uncover security weaknesses that could otherwise be exploited by attackers.
- Preventing Security Breaches: By identifying and addressing vulnerabilities, organizations can reduce the risk of data breaches and unauthorized access.
- Improving Security Posture: Penetration testing helps organizations understand their security gaps and provides actionable recommendations to strengthen defenses.
- Compliance: Many regulatory standards and frameworks (e.g., PCI DSS, HIPAA) require regular penetration testing to ensure the security of sensitive data.
Challenges in Penetration Testing
While penetration testing is an effective method for identifying vulnerabilities, there are several challenges to consider:
- Scope Limitations: Sometimes penetration testing is limited to specific areas or systems, which can result in missed vulnerabilities in other parts of the network.
- Time Constraints: Pen testers are often given limited time to perform tests, which may affect the depth of the testing conducted.
- False Positives: Some tools may report false positives, requiring testers to manually verify and confirm vulnerabilities.
Conclusion
Penetration testing is a crucial part of any organization's cybersecurity strategy. It helps identify weaknesses before malicious attackers can exploit them, ensuring that systems and applications are secure. By performing regular penetration tests and addressing vulnerabilities, organizations can reduce the risk of security breaches and protect sensitive data from cyber threats.
Tools for Security Testing (Burp Suite, ZAP)
Security Testing is an essential part of the software development lifecycle, ensuring that applications and systems are protected from potential security threats. Security testing tools help identify vulnerabilities in applications, networks, and systems. Two popular tools for security testing are Burp Suite and OWASP ZAP, both of which are widely used for web application security testing.
What is Burp Suite?
Burp Suite is a popular security testing tool used for web application vulnerability scanning and penetration testing. It is used to identify security weaknesses and vulnerabilities in web applications and networks, helping security professionals simulate attacks and assess the application's security posture.
Key Features of Burp Suite:
- Intercepting Proxy: Burp Suite acts as an intercepting proxy between the browser and the web application, allowing testers to inspect and modify HTTP requests and responses in real-time.
- Scanner: Burp Suite includes an automated vulnerability scanner that scans for common security vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Intruder: The Intruder tool is used for automated attacks such as brute force and fuzz testing to discover vulnerabilities in the system.
- Repeater: The Repeater tool helps testers modify and resend HTTP requests to the server for manual testing and vulnerability analysis.
- Extensibility: Burp Suite supports a variety of extensions and plugins that allow security professionals to extend its functionality and tailor it to specific testing needs.
Advantages of Burp Suite:
- Comprehensive set of tools for web application security testing
- Powerful automated scanning capabilities for common vulnerabilities
- Real-time interception and modification of HTTP traffic
- Extensive community support and frequent updates
Disadvantages of Burp Suite:
- The free version is limited in functionality, and advanced features are only available in the paid version.
- Can be resource-intensive, requiring significant processing power for large-scale scans.
What is OWASP ZAP?
OWASP ZAP (Zed Attack Proxy) is an open-source security testing tool designed for finding vulnerabilities in web applications. ZAP is part of the OWASP (Open Web Application Security Project) initiative and is widely used for both manual and automated web application security testing.
Key Features of OWASP ZAP:
- Intercepting Proxy: Similar to Burp Suite, ZAP acts as an intercepting proxy, allowing testers to monitor and modify HTTP requests and responses.
- Automated Scanner: ZAP includes an automated scanner that identifies common vulnerabilities, such as SQL injection, XSS, and directory traversal.
- Active and Passive Scanning: ZAP provides both active and passive scanning options. Active scanning sends requests to the target to actively probe for vulnerabilities, while passive scanning analyzes the traffic without affecting the target system.
- Spidering: ZAP includes a spider tool that helps automatically discover all the pages and endpoints in a web application, which can then be tested for vulnerabilities.
- Fuzzer: ZAP includes a fuzzer that allows testers to send a wide range of data inputs to a target application in an attempt to uncover vulnerabilities.
Advantages of OWASP ZAP:
- Free and open-source, making it an accessible option for security testing.
- Active community support and frequent updates from OWASP.
- Rich set of tools for both manual and automated security testing.
- Easy to use with an intuitive interface and extensive documentation.
Disadvantages of OWASP ZAP:
- May not be as feature-rich as Burp Suite in terms of advanced features and customization.
- Performance can be slower compared to other commercial tools for large applications.
Comparing Burp Suite and OWASP ZAP
Both Burp Suite and OWASP ZAP are excellent tools for security testing, but they have some key differences:
Feature | Burp Suite | OWASP ZAP |
---|---|---|
Cost | Free (limited) / Paid | Free |
Automated Scanning | Yes | Yes |
Extensibility | Highly Extensible | Moderately Extensible |
Community Support | Strong | Strong (OWASP community) |
Performance | Good for large-scale scans | Can be slower on large apps |
Conclusion
Both Burp Suite and OWASP ZAP are powerful tools for security testing, offering comprehensive features for identifying and mitigating vulnerabilities in web applications. While Burp Suite is often preferred by professionals for its advanced features and commercial support, OWASP ZAP is a great open-source alternative for those looking for a cost-effective solution. The choice between the two depends on the specific needs of the security testing process and budget constraints.
Writing Secure Test Cases
Writing secure test cases is an essential part of the software testing process. Secure test cases focus on identifying potential security vulnerabilities within the system, ensuring that sensitive data is protected, and testing the system's resistance to unauthorized access, attacks, and malicious actions. Security testing is crucial to prevent security breaches and data leaks in applications.
Why Secure Test Cases Are Important
Security testing helps detect flaws that could be exploited by attackers. A secure application should be resilient to risks such as unauthorized access, data manipulation, or information leakage. Writing secure test cases ensures that security flaws are identified early in the development process and mitigated before deployment.
Best Practices for Writing Secure Test Cases
- Understand Security Requirements: Before writing test cases, ensure that you thoroughly understand the security requirements and threat models of the application. This includes knowing sensitive data, access control mechanisms, and authentication methods.
- Test Authentication Mechanisms: Test cases should cover different authentication scenarios, including strong password policies, multi-factor authentication, session management, and token expiration. Ensure that unauthorized users cannot gain access to restricted areas.
- Test Input Validation: Test cases should verify input validation on all inputs, including form fields, URL parameters, cookies, and headers. Check for SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), and other common vulnerabilities.
- Test Access Control: Ensure that users can only access the data and functionality they are authorized to. Test cases should verify that roles and permissions are properly enforced across the application. Check for privilege escalation and ensure that unauthorized users cannot access or modify sensitive information.
- Ensure Data Encryption: Test cases should verify that sensitive data such as passwords, personal information, and credit card details are encrypted both at rest and in transit. Ensure the use of strong encryption algorithms like AES and SSL/TLS for secure communication.
- Session Management Testing: Test cases should cover sessions' security, including session expiration, session hijacking, and session fixation attacks. Validate that session cookies are secure and properly configured with flags like HttpOnly and Secure.
- Test Error Handling: Ensure that error messages do not expose sensitive information like database details, stack traces, or authentication details. Test cases should verify that error messages are generic and do not reveal system vulnerabilities.
- Test for Common Vulnerabilities: Test cases should cover the OWASP Top 10 vulnerabilities, including SQL injection, XSS, CSRF, and broken authentication. Use tools to automate security tests and ensure that these vulnerabilities are mitigated.
- Test Logging and Auditing: Test cases should verify that appropriate logging and auditing mechanisms are in place. Ensure that security events such as failed login attempts, access to sensitive data, and system errors are logged securely.
Writing Secure Test Cases: Example
Here’s an example of a secure test case for testing the login functionality of an application:
Test Case: Verify Login Functionality with Invalid Password
Test Steps:
1. Navigate to the login page.
2. Enter a valid username (e.g., 'user123').
3. Enter an invalid password (e.g., 'wrongpassword').
4. Click the login button.
5. Verify that the system does not grant access to the dashboard.
6. Ensure that the error message is generic, such as "Invalid credentials", and does not reveal whether the username or password is incorrect.
7. Verify that the login attempt is logged for security auditing purposes.
Expected Result:
- User should not be granted access with an incorrect password.
- Error message should not provide clues about the type of invalid input.
- Login attempt should be logged with relevant details, except for sensitive information like the password.
Common Security Testing Tools for Writing Secure Test Cases
- Burp Suite: A powerful tool for web application security testing, providing features like a web vulnerability scanner and the ability to intercept and modify HTTP requests.
- OWASP ZAP: An open-source security testing tool that helps identify security flaws in web applications through automated and manual testing techniques.
- OWASP Dependency-Check: A tool that identifies publicly known vulnerabilities in project dependencies, ensuring that third-party libraries do not introduce security risks.
- SonarQube: A static analysis tool that helps identify security issues in source code during the development process, including vulnerabilities related to authentication, authorization, and data encryption.
- Postman: A tool for testing APIs, including security-specific tests like authentication, authorization, and input validation for web services.
Conclusion
Writing secure test cases is a critical part of ensuring the integrity and confidentiality of an application. By following best practices and using the right tools, testers can identify and mitigate security vulnerabilities before they are exploited. Security testing should be integrated into the development lifecycle from the beginning to ensure a secure and robust application.
Agile Testing: Overview and Best Practices
Agile testing is an integral part of the agile development methodology, which emphasizes iterative development, flexibility, and collaboration. Agile testing focuses on testing early and often, ensuring that the product is continuously improved based on feedback. Testers work closely with developers, business stakeholders, and product owners to ensure that quality is maintained throughout the development cycle. This approach allows teams to respond to changes quickly and deliver software that meets user needs.
What is Agile Testing?
Agile testing is a software testing practice that follows the principles of agile software development. Unlike traditional testing methods, agile testing is done continuously throughout the development process, with testers and developers collaborating to ensure that software meets both functional and non-functional requirements. It involves testing from the beginning of the development cycle and encourages frequent iterations and feedback loops.
Key Principles of Agile Testing
- Continuous Testing: Testing is done frequently and continuously throughout the development lifecycle, rather than just at the end of a project.
- Collaboration: Testers work closely with developers, product owners, and business stakeholders to ensure that the software meets user expectations and quality standards.
- Customer-Centric: Agile testing is focused on delivering value to the customer by ensuring that the features developed align with the business requirements and user stories.
- Early Testing: Testing starts early in the development process, often with test-driven development (TDD) or behavior-driven development (BDD), to ensure that potential issues are identified and addressed early.
- Adaptability: Agile testing embraces change and is flexible enough to accommodate evolving requirements throughout the project lifecycle.
Agile Testing Life Cycle
The agile testing lifecycle is iterative, where testing activities are performed in small cycles, each corresponding to a sprint in the agile development process. The key steps involved in this lifecycle include:
- Test Planning: In an agile project, planning is done at the start of every sprint. Testers collaborate with developers to define the scope of testing based on user stories, features, and requirements.
- Test Design: Based on the sprint backlog, test cases and scenarios are designed, and acceptance criteria are defined to ensure that the feature meets the requirements.
- Test Execution: Tests are executed during the sprint, and feedback is provided on the quality of the features. This includes manual and automated testing activities.
- Defect Reporting: Any defects found during testing are reported and tracked. These defects are prioritized based on their severity and impact on the user experience.
- Retesting: Defects are fixed, and the software is retested during subsequent sprints to ensure that the fixes work and no new issues have been introduced.
Best Practices for Agile Testing
- Start Testing Early: Testing should begin as soon as possible in the development process. Agile testers often use test-driven development (TDD) or behavior-driven development (BDD) practices to ensure that testing is integrated into the development process from the outset.
- Collaboration is Key: Successful agile testing requires close collaboration between testers, developers, product owners, and business stakeholders. This ensures that the test cases are aligned with business requirements and that feedback can be incorporated quickly.
- Automate Where Possible: Automation plays a crucial role in agile testing, especially for repetitive tasks. Automated tests should be integrated into the continuous integration pipeline to ensure that tests are executed frequently and efficiently.
- Frequent Feedback: In agile testing, feedback is provided frequently. Testers should work closely with developers to discuss defects and share insights early in the development cycle, enabling faster fixes and improvements.
- Test the Entire System: Agile testing should not be limited to functional testing. Non-functional aspects such as performance, security, and usability should also be tested to ensure that the product meets all quality standards.
- Prioritize Testing Based on Risk: Not all features need the same level of testing. Agile testers should prioritize testing based on the risk of failure and the impact of defects on the user experience.
- Use Exploratory Testing: Exploratory testing is a valuable technique in agile testing, allowing testers to investigate areas of the application that may not be fully covered by automated tests or predefined test cases.
- Maintain a Strong Test Suite: As the software evolves, the test suite should evolve as well. Test cases should be continuously refined and updated to ensure that they remain relevant and effective.
Agile Testing Methods and Techniques
- Test-Driven Development (TDD): A development approach where tests are written before the code, ensuring that the code meets the specified requirements from the start.
- Behavior-Driven Development (BDD): A collaborative approach where developers, testers, and business stakeholders define the system’s behavior in the form of user stories and use cases, which are then translated into automated tests.
- Pair Testing: Two testers (or a developer and a tester) work together to test the software, sharing knowledge and insights to find defects more effectively.
- Exploratory Testing: Testers explore the application without predefined test cases, using their intuition and experience to identify potential issues.
- Continuous Integration: Agile testing involves frequent integration of code into a shared repository, with automated tests run to ensure that changes do not break the software.
Agile Testing Tools
- Selenium: An open-source tool for automating web applications. It is commonly used in agile environments to automate functional testing.
- Jenkins: A popular tool for continuous integration that allows teams to automatically run unit tests, integration tests, and UI tests as part of the build process.
- JIRA: A project management tool commonly used in agile teams for tracking user stories, defects, and overall project progress.
- Cucumber: A tool for BDD that allows teams to write tests in natural language, making it easier for non-technical stakeholders to collaborate on test scenarios.
- TestComplete: A test automation tool that supports functional, regression, and performance testing for web, desktop, and mobile applications in agile environments.
Conclusion
Agile testing is a vital aspect of the agile software development process, where collaboration, flexibility, and continuous improvement are key. By starting testing early, automating where possible, and prioritizing test coverage based on business needs, agile teams can ensure that software is delivered with high quality. Following best practices in agile testing enables teams to adapt to changing requirements and deliver software that meets user expectations while being secure, performant, and reliable.
Role of Testers in Agile Teams
In agile teams, testers play a critical role in ensuring the quality of the software throughout the development process. Unlike traditional software development, where testing is often a distinct phase that occurs after development, agile testing involves continuous collaboration, feedback, and iterative testing throughout the entire development lifecycle. Testers in agile teams are not just responsible for finding defects; they also work closely with developers, product owners, and business stakeholders to ensure that the product meets user needs and business requirements.
Key Responsibilities of Testers in Agile Teams
- Collaborating with Developers: Testers work closely with developers from the start of the project. They help in writing user stories, defining acceptance criteria, and ensuring that the requirements are clear and testable. This collaboration ensures that testing is integrated into the development process from the very beginning.
- Defining and Designing Test Cases: Testers are responsible for designing test cases based on the user stories and acceptance criteria. This includes both functional and non-functional test cases that cover all aspects of the application, such as performance, security, and usability.
- Test Automation: In agile teams, automation plays a major role in accelerating the testing process. Testers design and implement automated tests for regression, functional, and integration testing. This ensures that testing is fast, repeatable, and scalable, especially in iterative agile environments.
- Exploratory Testing: Testers engage in exploratory testing, where they use their experience and intuition to identify potential issues that may not be covered by automated tests or predefined test cases. This is particularly important in agile projects where requirements may evolve rapidly.
- Continuous Integration and Continuous Testing: Testers are responsible for ensuring that automated tests are integrated into the continuous integration (CI) pipeline. They ensure that tests are executed regularly, providing constant feedback to the development team about the software’s quality and functionality.
- Defect Identification and Reporting: Testers play a vital role in identifying defects and reporting them to the development team. They provide detailed information on the defect’s impact, help prioritize fixes, and verify whether defects are resolved during subsequent sprints.
- Acceptance Testing: Testers validate user stories and features against the acceptance criteria defined by the product owner. They ensure that the developed software meets the business requirements and is ready for release.
- Providing Feedback: Agile testers provide continuous feedback during the sprint. This feedback is valuable for improving both the product and the testing process. Testers are encouraged to give insights into the usability, functionality, and potential risks early in the development cycle.
- Maintaining Test Suites: As agile projects are iterative, the test suite evolves over time. Testers ensure that test cases are updated to reflect new features, changes in requirements, and defect fixes. They also maintain the test environment to ensure that it is stable and reliable.
Agile Testing Skills for Testers
- Collaboration and Communication: Testers need strong communication skills to work effectively with developers, business analysts, product owners, and other stakeholders. They must be able to clearly articulate testing requirements and results to ensure that everyone is aligned on quality expectations.
- Test Automation Skills: Testers should have knowledge of test automation tools and frameworks such as Selenium, Cypress, and JUnit. They should be able to design, write, and maintain automated tests to support continuous testing and integration.
- Knowledge of Agile Methodologies: Testers must be familiar with agile practices such as Scrum, Kanban, and Lean, as well as agile testing methodologies like Test-Driven Development (TDD) and Behavior-Driven Development (BDD).
- Exploratory Testing Expertise: Testers must be skilled in exploratory testing, which requires creative thinking and problem-solving to uncover issues that automated tests may miss.
- Strong Analytical Skills: Testers must be able to understand complex requirements and identify potential areas of risk in the software. They need to design effective test cases that ensure comprehensive test coverage.
- Adaptability: Agile testers need to be flexible and adaptable to changing requirements, priorities, and timelines. They must be able to adjust their testing approach based on the project’s needs and the feedback they receive during the development process.
- Attention to Detail: Testers need to be detail-oriented to identify even the smallest defects or inconsistencies in the application. Their focus on quality helps ensure that the software is reliable and meets user expectations.
Role of Testers in Different Agile Phases
1. During the Planning Phase
Testers participate in sprint planning meetings to understand the user stories and requirements. They help define the acceptance criteria, ensuring that testable requirements are captured. Testers also estimate the effort required for testing and help prioritize the tasks for the upcoming sprint.
2. During the Development Phase
Testers collaborate closely with developers during the development phase. They provide feedback on the implementation of features and ensure that the code meets the acceptance criteria. Testers may also write automated tests and contribute to the design of the system architecture to ensure that testing is integrated throughout the development process.
3. During the Testing Phase
Testers are actively involved in executing manual and automated tests. They conduct functional testing, regression testing, and exploratory testing to ensure that the software meets the defined requirements. They also validate bug fixes and ensure that defects are resolved.
4. During the Review Phase
Testers participate in sprint reviews, presenting the results of testing and discussing any outstanding issues or defects. They provide feedback on the quality of the product and collaborate with the development team to determine the next steps for improvement.
5. During the Retrospective Phase
Testers participate in agile retrospectives to reflect on the testing process. They share feedback on what went well, what can be improved, and how the testing process can be made more efficient in future sprints. Continuous improvement is key to ensuring that testing keeps pace with the rest of the development process.
Conclusion
In agile teams, testers are not just responsible for finding defects; they are essential members of the development process, collaborating with developers, product owners, and business stakeholders to ensure that the software meets high-quality standards. Testers contribute throughout the entire agile lifecycle, from planning and development to testing and review, ensuring that the product is functional, usable, and aligned with business goals. Their role is crucial in delivering software that meets user expectations and is free from defects, making them key players in agile success.
Continuous Integration/Continuous Delivery (CI/CD)
Continuous Integration (CI) and Continuous Delivery (CD) are two key practices in modern software development that focus on automating the process of software integration, testing, and deployment. By implementing CI/CD pipelines, teams can achieve faster release cycles, higher-quality software, and a more efficient development process.
What is Continuous Integration (CI)?
Continuous Integration is the practice of automatically integrating code changes into a shared repository multiple times a day. Developers frequently commit their code to the repository, and the integration system automatically runs tests to validate that the new code does not introduce issues or conflicts. CI aims to detect bugs early in the development cycle, thus improving the overall quality of the software.
Benefits of Continuous Integration
- Early Bug Detection: With frequent integrations, errors are detected early, making them easier and faster to fix.
- Faster Development Process: Frequent and automated testing reduces the time spent in manual testing, allowing for faster development and fewer integration issues.
- Improved Code Quality: CI encourages writing clean, maintainable code, as developers know their code will be frequently tested for integration issues.
- Increased Collaboration: Developers collaborate more effectively since they commit code to a shared repository, ensuring that everyone is working with the latest version of the code.
What is Continuous Delivery (CD)?
Continuous Delivery is the practice of automatically deploying code changes to a staging or production environment after they pass the automated tests. CD ensures that the software can be reliably and consistently released at any time, with minimal manual intervention. Unlike CI, which focuses on code integration, CD extends this process by automating the deployment pipeline.
Benefits of Continuous Delivery
- Faster Time-to-Market: By automating the deployment process, teams can release new features and bug fixes more quickly, enhancing the overall speed of the development cycle.
- Reduced Risk of Deployment Failures: Since the deployment pipeline is automated and thoroughly tested, the risk of introducing defects during deployment is minimized.
- Reliable Releases: CD ensures that the software is always in a deployable state, making it easier to release updates and roll back changes if needed.
- Improved Customer Satisfaction: Faster releases, with improved software quality, result in higher customer satisfaction as bugs are resolved and features are delivered more quickly.
CI/CD Pipeline: Overview
A CI/CD pipeline is a series of automated steps that allow developers to continuously integrate, test, and deploy code. The pipeline typically consists of the following stages:
- Code Commit: Developers commit their code to the version control system (e.g., Git). This triggers the CI process.
- Build: The CI system automatically builds the application to ensure that the code is compiled and packaged correctly.
- Test: Automated tests (unit tests, integration tests, etc.) are executed to validate the functionality and quality of the code.
- Deploy: If the tests pass, the code is automatically deployed to a staging environment. In the case of Continuous Delivery, this step can also trigger deployment to production.
- Monitor: After deployment, monitoring tools track the performance, usage, and potential issues in production. This data is used to improve the next cycle of development.
CI/CD Tools
There are several tools available to implement and manage CI/CD pipelines. Some popular tools include:
- Jenkins: One of the most widely used CI/CD tools, Jenkins helps automate the integration, testing, and deployment process.
- GitLab CI/CD: GitLab's built-in CI/CD functionality allows developers to automate the entire DevOps pipeline, from integration to delivery.
- Travis CI: A cloud-based CI/CD tool that integrates with GitHub to automate testing and deployment.
- CircleCI: A tool that automates the CI/CD pipeline for faster, more reliable software development.
- Azure DevOps: Microsoft's suite of development tools includes CI/CD services that support building, testing, and deploying applications.
- GitHub Actions: GitHub's CI/CD service that automates workflows and integrates easily with GitHub repositories.
Best Practices for CI/CD
- Automate Everything: Automate the entire process, from code integration and testing to deployment. This minimizes the risk of human error and speeds up the development cycle.
- Test Early and Often: Run automated tests on every change to ensure that the code remains stable and functional. This helps catch defects early in the process.
- Keep the Pipeline Fast: Ensure that the CI/CD pipeline runs quickly by optimizing test cases and automation scripts. A slow pipeline can hinder productivity.
- Maintain a Deployable State: Always keep the code in a state that can be deployed to production. This reduces the risks associated with release failures.
- Monitor and Analyze: Continuously monitor the software performance and gather feedback to improve future iterations. Use monitoring tools to track the health of the application in production.
Challenges of CI/CD
- Complexity of Setup: Setting up and maintaining a CI/CD pipeline can be complex, especially in large organizations with legacy systems.
- High Initial Investment: Implementing CI/CD may require an initial investment in tools, infrastructure, and training.
- Integration with Legacy Systems: Integrating CI/CD with existing systems, especially legacy applications, can be challenging and time-consuming.
- Managing Dependencies: Managing dependencies across various environments and stages in the pipeline can be difficult, especially in complex projects.
Conclusion
CI/CD is a powerful approach to software development that helps teams deliver high-quality software faster and more reliably. By automating the integration, testing, and deployment processes, CI/CD ensures that developers can focus on writing code and innovating, while the pipeline handles the tedious and error-prone tasks. Although it requires initial investment and effort, the benefits of faster releases, improved quality, and reduced risks make CI/CD an essential practice for modern software development teams.
Testing in DevOps: Shift Left Approach
DevOps is a set of practices aimed at integrating and automating the work of software development (Dev) and IT operations (Ops) as a means to shorten the systems development life cycle and provide continuous delivery with high software quality. One of the key principles in DevOps is the "Shift Left" approach to testing, which emphasizes testing earlier in the software development life cycle (SDLC) to identify and fix defects sooner. This approach helps teams deliver high-quality software at a faster pace.
What is the Shift Left Approach?
The "Shift Left" approach refers to moving testing activities earlier in the development process, as opposed to waiting until the later stages of development (traditionally done in "Shift Right"). The goal is to detect bugs and vulnerabilities as early as possible, ideally during the coding and integration phases, rather than during post-development or pre-release testing. The earlier defects are identified, the cheaper and easier they are to fix.
Why is the Shift Left Approach Important?
Shifting testing left in the development process helps organizations reap several benefits:
- Faster Feedback: By testing earlier, developers get immediate feedback on the quality of their code, helping them address issues before they become larger problems.
- Reduced Costs: Catching bugs early reduces the costs associated with fixing defects later in the development cycle, especially after the code has been deployed to production.
- Higher Quality Software: Continuous testing ensures that the software is always in a testable and deployable state, leading to fewer defects and a more reliable product.
- Faster Releases: Shifting left allows for more frequent releases, as testing and feedback are integrated earlier in the process, accelerating the delivery cycle.
- Improved Collaboration: Developers and testers work together closely from the beginning, helping to align the development process with testing efforts and ensuring that quality is maintained throughout the pipeline.
Testing in DevOps: Key Elements
In a DevOps environment, testing becomes an integral part of the entire development and deployment pipeline. Some key testing practices in DevOps include:
- Automated Testing: Automation is a critical part of DevOps, enabling teams to run tests frequently, continuously, and in parallel with development activities. Automated tests such as unit tests, integration tests, and UI tests provide fast feedback.
- Continuous Integration (CI): CI involves continuously integrating code changes into a shared repository, where automated tests are run to validate the changes. This ensures that the latest code changes do not break the software.
- Continuous Testing: Continuous testing is the practice of executing automated tests throughout the development pipeline. It helps to validate code at every stage and ensure that quality is maintained at all times.
- Test-Driven Development (TDD): TDD is a practice where tests are written before the code itself. This ensures that code is always written with testability in mind and that defects are caught early.
- Shift Left Security (DevSecOps): In addition to functional testing, security testing is integrated earlier in the development process, ensuring that vulnerabilities are detected and fixed as part of the development lifecycle.
Benefits of the Shift Left Approach
- Reduced Time to Market: By identifying defects and issues early, the development team spends less time fixing problems in later stages, which speeds up the overall time to market for software products.
- Improved Collaboration and Communication: The shift left approach encourages collaboration between developers, testers, and operations teams from the beginning, fostering a shared responsibility for quality across the organization.
- Early Problem Detection: With testing occurring earlier, defects are discovered and addressed promptly, minimizing the risk of critical issues arising at the end of the development cycle or post-production.
- Improved Customer Satisfaction: A faster, more efficient testing and delivery process enables organizations to deliver high-quality software more frequently, which improves customer satisfaction and trust.
- Increased Efficiency: By automating tests and integrating them early in the development process, teams can reduce manual testing efforts, focus on high-priority tasks, and improve overall productivity.
Challenges of the Shift Left Approach
- Initial Setup Effort: Implementing shift left testing requires investment in tools, automation, and skilled resources to create a robust testing pipeline, which can be time-consuming and costly in the beginning.
- Skillset and Training: Teams may require specialized training in new testing practices and tools, especially in areas like test automation and continuous integration, to ensure the success of the shift left approach.
- Test Maintenance: As code changes frequently, automated tests must be updated regularly to ensure they remain effective. Test scripts can become stale if not maintained properly, requiring additional overhead.
- Cultural Shift: Shifting left involves a cultural shift in the organization, as developers, testers, and operations teams need to work more collaboratively. This may require a change in mindset and workflow.
Tools for Testing in DevOps
Several tools can be used to support the shift left approach in DevOps, enabling teams to automate testing and integrate it early in the development lifecycle. Some popular tools include:
- Jenkins: Jenkins is a widely used tool for automating the build and deployment process, supporting continuous integration and continuous testing.
- GitLab CI/CD: GitLab offers a full suite of DevOps tools, including CI/CD and automated testing capabilities, that facilitate the shift left approach.
- SonarQube: SonarQube provides static code analysis to detect vulnerabilities, bugs, and code smells early in the development process, supporting quality control in DevOps.
- Selenium: Selenium is a popular tool for automating web application testing, enabling teams to test functionality early and continuously integrate tests into the development pipeline.
- JUnit: JUnit is a widely used framework for writing and running unit tests, commonly integrated into CI/CD pipelines to ensure code quality.
- Appium: Appium is an open-source tool for automating mobile app testing, allowing teams to test mobile applications as part of the shift left strategy.
Conclusion
The shift left approach in DevOps emphasizes early testing and continuous quality assurance throughout the software development life cycle. By integrating testing earlier, teams can catch defects sooner, reduce costs, improve collaboration, and deliver high-quality software faster. Although implementing shift left testing requires an investment in tools, automation, and training, the benefits of faster releases, increased quality, and improved customer satisfaction make it an essential practice in modern software development and DevOps environments.
Automating Testing in CI/CD Pipelines
Continuous Integration (CI) and Continuous Delivery (CD) are practices that enable teams to deliver code changes frequently and reliably. In modern software development, testing is crucial to ensure that new code is both functional and does not introduce defects. Automating testing in CI/CD pipelines ensures that tests are executed continuously as part of the development process, enabling fast feedback and high-quality software delivery.
What is CI/CD?
CI/CD refers to the practices of Continuous Integration and Continuous Delivery. These practices emphasize automating the software development lifecycle, from code integration to deployment. The goal is to make code changes available to end-users quickly and reliably, with minimal manual intervention.
- Continuous Integration (CI): CI involves continuously integrating new code changes into a shared repository. Automated tests are run on each integration to ensure that the new code does not break the existing functionality.
- Continuous Delivery (CD): CD extends CI by automating the deployment process, allowing for frequent releases to production. With CD, once code is integrated and passes testing, it can be automatically deployed to production or staging environments.
Importance of Automated Testing in CI/CD
Automating testing in CI/CD pipelines is crucial to ensure that defects are detected early, and that the software is always in a deployable state. Some key benefits include:
- Faster Feedback: Automated tests can quickly detect defects in code changes, giving developers immediate feedback so they can make necessary adjustments.
- Reduced Risk of Bugs: By running automated tests frequently as part of the CI/CD pipeline, teams can detect bugs early in the development cycle, reducing the risk of defects reaching production.
- Consistent Testing: Automated tests ensure that the same tests are run every time a code change is made, providing consistent and reliable results.
- Faster Releases: Automated testing reduces the time required for manual testing, allowing for faster releases and more frequent deployments.
Types of Tests to Automate in CI/CD Pipelines
In CI/CD pipelines, different types of tests can be automated to ensure the quality of the software:
- Unit Testing: Unit tests validate the smallest units of code, such as functions or methods. These tests are typically run first in the CI/CD pipeline, as they are fast and help identify issues early in the development process.
- Integration Testing: Integration tests verify that different components of the system work together as expected. These tests are run after unit tests to ensure that the integrated code functions as intended.
- UI/Functional Testing: Automated UI tests validate the functionality of user interfaces. These tests are typically run after integration tests and simulate real user interactions to ensure that the application behaves as expected.
- Performance Testing: Performance testing evaluates how well the application performs under different conditions. These tests can be automated to run in the CI/CD pipeline, helping to ensure that new code does not degrade the system’s performance.
- Security Testing: Security tests automate the detection of vulnerabilities such as code injections, authentication issues, and other security risks. These tests help ensure that the software remains secure throughout development and deployment.
Steps to Automate Testing in CI/CD Pipelines
To successfully automate testing in CI/CD pipelines, follow these key steps:
- Choose the Right Testing Tools: Select appropriate testing tools for each type of test. Popular tools include Selenium for UI testing, JUnit for unit testing, Postman for API testing, and JMeter for performance testing.
- Integrate Testing with Version Control: Set up automated tests to run every time code is pushed to the version control system (e.g., Git). This ensures that each code change is validated before it is merged into the main branch.
- Configure CI/CD Pipeline: Set up a CI/CD pipeline using tools such as Jenkins, GitLab CI/CD, or CircleCI. The pipeline should automatically trigger tests whenever code changes are pushed, and deploy the application to staging or production if the tests pass.
- Run Tests in Parallel: To speed up the testing process, consider running tests in parallel. Many CI/CD tools support parallel test execution, allowing multiple tests to run simultaneously, reducing overall test execution time.
- Monitor Test Results: Set up proper monitoring and reporting for test results. Tools like TestRail or Jenkins can provide detailed reports that highlight failed tests, allowing developers to quickly identify issues and take corrective actions.
Popular Tools for Automating Testing in CI/CD Pipelines
There are various tools available to help automate testing in CI/CD pipelines. Some popular ones include:
- Jenkins: Jenkins is one of the most popular CI/CD tools for automating builds and tests. It supports a wide range of plugins for different types of testing, including unit, integration, and performance testing.
- GitLab CI/CD: GitLab CI/CD allows teams to automate the testing and deployment of code directly from the GitLab repository. It integrates well with version control and offers built-in support for testing and deployment automation.
- CircleCI: CircleCI is a cloud-based CI/CD tool that automates testing, integration, and deployment. It integrates with popular version control systems and provides powerful features for automating testing workflows.
- Travis CI: Travis CI is a cloud-based service that automatically runs tests and deploys code when changes are pushed to a GitHub repository. It integrates well with various testing frameworks and CI/CD tools.
- Selenium: Selenium is a widely used tool for automating web application testing. It is often used in CI/CD pipelines to automate functional and UI testing for web applications.
- JUnit: JUnit is a popular testing framework for Java applications. It is commonly used in CI/CD pipelines to automate unit and integration tests for Java-based applications.
Challenges of Automating Testing in CI/CD Pipelines
- Test Flakiness: Automated tests can sometimes become flaky, meaning they pass or fail inconsistently. This can cause instability in the CI/CD pipeline and make it difficult to trust test results.
- High Initial Setup Effort: Setting up a comprehensive automated testing framework for a CI/CD pipeline requires significant effort in terms of choosing the right tools, writing test cases, and configuring the pipeline.
- Test Maintenance: Automated tests require ongoing maintenance to keep up with changes in the codebase. Tests may need to be updated when there are changes to the user interface or business logic, which can be time-consuming.
- Complexity: In large applications, automating all the necessary tests for CI/CD pipelines can be complex. Teams must ensure that the pipeline remains efficient and does not become bottlenecked by excessive testing.
Conclusion
Automating testing in CI/CD pipelines is essential for ensuring that code changes are validated quickly and efficiently, allowing teams to deliver high-quality software with faster release cycles. By selecting the right tools, configuring a robust pipeline, and continuously monitoring test results, organizations can achieve seamless integration and delivery while maintaining a high level of software quality. However, it is important to overcome challenges such as test flakiness, maintenance, and setup complexity to ensure the automation process remains effective over time.
Tools for CI/CD Testing (Jenkins, GitHub Actions)
Continuous Integration and Continuous Delivery (CI/CD) are essential practices in modern software development. CI/CD tools automate the testing, integration, and deployment processes to help deliver high-quality software quickly and reliably. Two of the most popular CI/CD tools used for testing are Jenkins and GitHub Actions.
What is CI/CD Testing?
CI/CD testing involves automating the process of running tests as part of the integration and delivery pipeline. As code is checked into a version control system, it triggers automated tests to ensure the new code does not introduce bugs and that the system is functioning as expected. The goal of CI/CD testing is to provide fast feedback, improve code quality, and enable frequent and smooth releases.
Jenkins: Overview
Jenkins is an open-source automation server that is widely used for CI/CD pipeline automation. It helps automate parts of software development related to building, testing, and deploying, making it a popular tool for integrating and testing code continuously.
Key Features of Jenkins
- Flexible Configuration: Jenkins supports a wide range of plugins that allow it to integrate with many different tools, including version control systems (e.g., Git), testing frameworks (e.g., JUnit, Selenium), and deployment platforms (e.g., Docker, Kubernetes).
- Pipeline as Code: Jenkins supports the creation of CI/CD pipelines as code, using the Jenkinsfile configuration, which enables versioning and better management of complex workflows.
- Integration with Popular Testing Tools: Jenkins can integrate with testing tools like Selenium, JUnit, and NUnit, allowing teams to automate functional, unit, and integration tests.
- Scalability: Jenkins can handle projects of all sizes, from small applications to large-scale systems, and can be scaled horizontally to handle high-demand testing environments.
How Jenkins Works in CI/CD Testing
In Jenkins, CI/CD testing is configured through pipelines that specify the steps for building, testing, and deploying the application. These pipelines are triggered automatically when code changes are pushed to the repository, and Jenkins runs the necessary tests to validate the changes. Jenkins provides detailed feedback on test results, allowing developers to identify and fix issues early in the development process.
GitHub Actions: Overview
GitHub Actions is an automation tool integrated within GitHub, enabling developers to create CI/CD workflows directly in their GitHub repositories. It is ideal for automating the testing, building, and deployment processes for projects hosted on GitHub.
Key Features of GitHub Actions
- Seamless GitHub Integration: GitHub Actions is built directly into GitHub, making it easy for developers to create workflows without needing to configure external CI/CD tools.
- Custom Workflows: With GitHub Actions, developers can define custom workflows using YAML configuration files, specifying actions for testing, building, and deploying code based on events like pull requests or commits.
- Matrix Builds: GitHub Actions supports matrix builds, allowing developers to test code on multiple environments and configurations simultaneously, speeding up the testing process.
- Free for Public Repositories: GitHub Actions is free for public repositories, making it an attractive choice for open-source projects. It also offers generous free tiers for private repositories, depending on usage.
How GitHub Actions Works in CI/CD Testing
GitHub Actions enables teams to define a series of steps in YAML files to automate testing workflows. These steps can include running unit tests, integration tests, and other checks. Each workflow is triggered by GitHub events (such as a commit, pull request, or release), and tests are executed as part of the process. GitHub Actions integrates well with various testing tools like Jest, Mocha, and Selenium, allowing developers to automate their testing pipelines directly within GitHub.
Comparison: Jenkins vs. GitHub Actions for CI/CD Testing
Both Jenkins and GitHub Actions are excellent tools for automating CI/CD pipelines, but they offer different features and integration options. Here's a comparison:
Feature | Jenkins | GitHub Actions |
---|---|---|
Integration | Integrates with many third-party tools through plugins | Integrated within GitHub, no setup needed for GitHub repositories |
Ease of Setup | Requires installation and configuration | Easy to set up directly in GitHub repositories |
Scalability | Highly scalable with distributed agents | Scales based on GitHub's infrastructure, with limited concurrency |
Cost | Free, but requires infrastructure for hosting | Free for public repositories, generous free tiers for private repositories |
Customization | Highly customizable with plugins | Customizable through YAML configuration files |
Popular Use Cases for CI/CD Testing Tools
Both Jenkins and GitHub Actions are widely used for various types of testing in CI/CD pipelines:
- Unit Testing: Running unit tests automatically on each code change ensures that individual components of the application work as expected.
- Integration Testing: Both tools can automate integration testing to validate that different parts of the application interact correctly.
- UI/Functional Testing: Jenkins and GitHub Actions can integrate with tools like Selenium or Cypress to automatically run end-to-end tests on web applications.
- Performance Testing: Performance tests can be automated using tools like JMeter or LoadRunner, which can be integrated into CI/CD pipelines through Jenkins or GitHub Actions.
- Security Testing: Automated security tests can be run to ensure that code changes do not introduce vulnerabilities, using tools like OWASP ZAP or Burp Suite.
Conclusion
Automating testing in CI/CD pipelines is essential for ensuring that code changes are validated quickly and efficiently. Tools like Jenkins and GitHub Actions make this process seamless by integrating testing into the development workflow. While Jenkins is highly customizable and scalable, GitHub Actions provides easy-to-use automation directly within GitHub repositories. Both tools support a wide range of testing frameworks and can be used to implement effective CI/CD testing pipelines that improve software quality and speed up the release cycle.
Selenium Tutorial
Selenium is a powerful and widely-used open-source tool for automating web browsers. It provides a framework for automating web applications, performing functional and regression testing, and simulating real user interactions with web pages. Selenium supports multiple programming languages (Java, Python, C#, etc.), making it a versatile tool for testers and developers.
What is Selenium?
Selenium is a suite of tools used for automating web browsers. It allows you to simulate user interactions such as clicking buttons, entering text in input fields, and navigating through web pages. Selenium can be used for both functional and regression testing of web applications, as well as for automating repetitive web tasks.
Components of Selenium
Selenium consists of several components that cater to different needs in web automation:
- Selenium WebDriver: The core component that allows direct interaction with web browsers. It provides APIs for controlling browser behavior, including actions like clicking, typing, and navigating.
- Selenium IDE: A browser extension that allows recording and playback of tests. It is useful for beginners and for quick test generation but is limited in terms of automation capabilities.
- Selenium Grid: A tool for running tests on multiple machines and different browsers in parallel, improving test execution speed and scalability.
- Selenium RC (Remote Control): An older tool that allows you to control a web browser remotely, but it has been largely replaced by WebDriver.
Setting Up Selenium
To get started with Selenium, you need to set up the necessary tools and dependencies. Below is a simple guide to setting up Selenium WebDriver in Java and Python:
Setting Up Selenium with Java
- Install Java: Ensure that Java is installed on your system. You can download the latest version of Java from the official Oracle website.
- Set Up IDE: Use an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse for Java development.
- Download Selenium WebDriver: Download the appropriate version of Selenium WebDriver from the official Selenium website (https://www.selenium.dev/downloads/).
- Add WebDriver to Project: Include the Selenium WebDriver JAR files in your project dependencies.
- Download Browser Driver: Download the appropriate browser driver (e.g., ChromeDriver, GeckoDriver for Firefox) from the official browser driver sites, and ensure they are in your system's PATH.
Setting Up Selenium with Python
- Install Python: Ensure Python is installed on your system. You can download Python from the official Python website.
- Install Selenium: Use pip to install Selenium by running the command:
pip install selenium
in your terminal. - Download Browser Driver: Download the appropriate browser driver (e.g., ChromeDriver, GeckoDriver for Firefox) from their respective websites.
Writing Your First Selenium Test
Once you have set up Selenium, you can start writing your first automation test. Below is an example of a simple Selenium WebDriver test using Java and Python:
Java Example: Open Google and Search
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class SeleniumTest {
public static void main(String[] args) {
// Set path to the ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize the Chrome WebDriver
WebDriver driver = new ChromeDriver();
// Open Google
driver.get("https://www.google.com");
// Find the search box and enter a query
driver.findElement(By.name("q")).sendKeys("Selenium Tutorial");
// Submit the search form
driver.findElement(By.name("q")).submit();
// Close the browser
driver.quit();
}
}
Python Example: Open Google and Search
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Initialize the Chrome WebDriver
driver = webdriver.Chrome(executable_path="path/to/chromedriver")
# Open Google
driver.get("https://www.google.com")
# Find the search box and enter a query
search_box = driver.find_element_by_name("q")
search_box.send_keys("Selenium Tutorial")
search_box.send_keys(Keys.RETURN)
# Close the browser
driver.quit()
Common Selenium Commands
Below are some commonly used Selenium WebDriver commands:
- driver.get(url): Opens the specified URL in the browser.
- driver.findElement(By.xpath(...)): Locates an element on the web page using XPath or other locators (e.g., ID, name, class, etc.).
- element.click(): Simulates a click action on the web element.
- element.sendKeys(text): Types the specified text into a text field or input element.
- driver.quit(): Closes the browser window and ends the WebDriver session.
Selenium WebDriver Locators
Selenium WebDriver allows you to locate elements on a web page using several methods:
- ID: Locates an element by its unique ID.
- Name: Locates an element by its name attribute.
- Class Name: Locates an element by its class name.
- XPath: Locates an element using an XPath expression.
- CSS Selector: Locates an element using a CSS selector.
- Link Text: Locates an anchor (link) element by its exact text.
- Partial Link Text: Locates an anchor (link) element by a partial match of its text.
Advanced Selenium Features
Selenium WebDriver also provides advanced features such as:
- Handling Alerts: Selenium can interact with pop-up alerts, prompting users with a message (e.g., accepting or dismissing alerts).
- Handling Multiple Windows: WebDriver can switch between multiple browser windows or tabs during test execution.
- Executing JavaScript: Selenium can execute JavaScript code in the context of the browser for more complex interactions.
- Taking Screenshots: Selenium can capture screenshots during test execution to provide visual evidence of the test results.
Selenium Grid
Selenium Grid is an extension of Selenium that allows you to run your tests in parallel on multiple machines and browsers. It is useful for running large test suites and speeding up test execution by distributing tests across different environments. Selenium Grid consists of two main components:
- Hub: The central server that manages the distribution of tests to the available nodes.
- Node: The machines that run the tests, which can be configured to run specific browser instances.
Conclusion
Selenium is a versatile tool that provides developers and testers with the ability to automate web browser interactions. Whether you are testing functionality, performing regression testing, or automating repetitive tasks, Selenium WebDriver provides a reliable solution. With its wide support for programming languages, advanced features, and integration with other tools, Selenium is a powerful choice for web automation and testing.
Cypress Tutorial
Cypress is a modern, fast, and reliable testing framework designed for testing web applications. It provides an easy-to-use interface for writing and executing tests. Cypress runs directly inside the browser, allowing it to offer real-time feedback during test execution, making it a popular choice for web developers and testers alike.
What is Cypress?
Cypress is an open-source testing framework that primarily focuses on end-to-end (E2E) testing for web applications. Unlike other testing frameworks, Cypress runs directly inside the browser, providing a unique testing experience. It offers features such as automatic waiting, time-travel debugging, and the ability to control the behavior of your application during tests.
Advantages of Cypress
- Fast Execution: Tests run directly in the browser, making Cypress much faster than traditional tools.
- Real-Time Browser Interaction: Cypress allows you to see your tests run in real-time inside the browser.
- Automatic Waiting: Cypress waits for elements to appear, eliminating the need for artificial waits and retries.
- Easy Setup: Cypress requires minimal setup and provides an easy-to-use test runner interface.
- Great Debugging Tools: Cypress provides time-travel and error snapshots, making debugging easier.
Setting Up Cypress
To get started with Cypress, you need to follow these steps:
Step 1: Install Cypress
- Ensure that Node.js and npm (Node Package Manager) are installed on your system.
- In your project directory, run the following command to install Cypress via npm:
npm install cypress --save-dev
Step 2: Open Cypress
- After installation, you can open Cypress using the following command:
npx cypress open
- This will open the Cypress Test Runner, where you can write and run tests.
Step 3: Write Your First Test
Cypress automatically creates a folder called cypress
in your project directory. Inside this folder, you'll find a folder called integration
, where you can write your test scripts.
Here’s a simple test example:
Example: Visit a Page and Assert Title
describe('My First Test', () => {
it('Visits the Cypress Documentation page', () => {
cy.visit('https://www.cypress.io');
cy.title().should('include', 'Cypress');
});
});
This test does the following:
- cy.visit(url): Opens the specified URL in the browser.
- cy.title(): Gets the title of the page.
- should('include', 'Cypress'): Asserts that the page title includes the word "Cypress".
Cypress Commands
Cypress provides a wide range of commands to interact with elements, perform assertions, and control the flow of tests. Some common commands include:
- cy.get(selector): Selects an element by its CSS selector.
- cy.contains(text): Selects an element that contains the specified text.
- cy.click(): Simulates a click action on the selected element.
- cy.type(text): Types the specified text into a text input field.
- cy.should(condition): Asserts that the selected element meets the specified condition (e.g., visibility, presence, value).
Handling Different Elements
Cypress allows you to interact with various web elements easily. Here are some examples:
Clicking a Button
cy.get('button').click();
Typing in a Text Field
cy.get('input[type="text"]').type('Cypress is awesome!');
Assertions
Assertions are used to verify the state of elements. Below are some common assertions in Cypress:
- cy.should('be.visible'): Asserts that the selected element is visible.
- cy.should('have.text', 'Hello World'): Asserts that the selected element has the specified text.
- cy.should('be.checked'): Asserts that the checkbox is checked.
- cy.should('have.class', 'active'): Asserts that the element has the specified class.
Running Tests in Cypress
Cypress provides two ways to run your tests:
- Interactive Mode: Opens the Cypress Test Runner where you can run tests interactively and see the results in real-time.
- Headless Mode: Runs the tests in the background without opening the browser UI, useful for CI/CD pipelines. You can run tests in headless mode with:
npx cypress run
Best Practices in Cypress Testing
Here are some best practices when writing tests with Cypress:
- Keep Tests Independent: Each test should be independent and isolated from others to avoid interdependencies.
- Use Custom Commands: Create reusable custom commands to improve test readability and reduce duplication.
- Use Fixtures for Test Data: Store test data in fixtures and load them in your tests to keep your code clean and maintainable.
- Use Cypress Selectors: Prefer using data attributes (e.g.,
data-cy
) as selectors to avoid brittle tests due to changes in the UI.
Advanced Cypress Features
Cypress offers several advanced features that enhance testing capabilities:
- Time Travel: Cypress allows you to see the state of the application at every step of the test, making it easier to debug and analyze failures.
- Network Stubbing: Cypress allows you to stub network requests and responses to simulate various scenarios without needing a real server.
- Screenshots and Videos: Cypress automatically takes screenshots on test failure and can capture videos of the entire test run for debugging purposes.
Integrating Cypress with CI/CD
Cypress can be easily integrated into Continuous Integration (CI) and Continuous Delivery (CD) pipelines. Popular CI/CD tools such as Jenkins, GitHub Actions, and GitLab CI can run Cypress tests automatically whenever new code is pushed, ensuring that your web application is always tested and ready for deployment.
Conclusion
Cypress is an excellent choice for testing modern web applications. It provides fast, reliable, and easy-to-use tools for writing end-to-end tests. With its rich set of features, real-time feedback, and excellent debugging capabilities, Cypress is quickly becoming one of the most popular testing frameworks for front-end developers and testers.
Appium for Mobile Testing
Appium is an open-source test automation framework designed for testing mobile applications. It supports both Android and iOS platforms, allowing testers to write tests in various programming languages like Java, JavaScript, Python, and Ruby. Appium is highly flexible, supporting native, hybrid, and mobile web application testing.
What is Appium?
Appium is a cross-platform testing framework that allows you to write tests for mobile applications. It supports Android and iOS and can be used for testing native apps, hybrid apps, and mobile web apps. Appium uses WebDriver APIs to interact with mobile apps, making it a popular choice for mobile testing.
Advantages of Appium
- Cross-Platform Support: Appium supports both Android and iOS, allowing you to write tests that can be executed on both platforms.
- Supports Multiple Languages: Appium supports several programming languages, including Java, JavaScript, Python, Ruby, and C#, enabling developers to write tests in the language they are most comfortable with.
- Open Source: Appium is free and open-source, making it easily accessible for developers and testers.
- Flexible Testing: Appium allows you to test native, hybrid, and mobile web applications, providing a wide range of testing possibilities.
- Integration with Other Tools: Appium can be easily integrated with other tools like Selenium, Jenkins, and TestNG for reporting and continuous integration.
Setting Up Appium
To get started with Appium, follow these steps:
Step 1: Install Node.js and npm
- Appium requires Node.js and npm (Node Package Manager). Download and install Node.js from the official website: https://nodejs.org.
Step 2: Install Appium
- Once Node.js and npm are installed, open a command prompt or terminal and run the following command to install Appium:
npm install -g appium
Step 3: Install Appium Doctor
- Appium Doctor is a tool that checks whether all the dependencies for Appium are correctly installed. Install it by running:
npm install -g appium-doctor
- Run
appium-doctor
to verify your environment is set up correctly.
Step 4: Start Appium Server
- Once everything is set up, you can start the Appium server by running the following command:
appium
Writing Your First Test with Appium
Here’s how to write a simple Appium test in Java using TestNG:
Example: Test for Opening a Mobile App
import io.appium.java_client.MobileElement;
import io.appium.java_client.android.AndroidDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
public class AppiumTest {
private AndroidDriver driver;
@BeforeClass
public void setUp() {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("platformName", "Android");
caps.setCapability("deviceName", "emulator-5554");
caps.setCapability("appPackage", "com.android.chrome");
caps.setCapability("appActivity", "com.google.android.apps.chrome.Main");
driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), caps);
}
@Test
public void testAppLaunch() {
System.out.println("App is launched successfully");
// Add more actions, like clicking or typing, here.
}
}
This test does the following:
- Sets the desired capabilities for the test (platform, device, app package, and activity).
- Initializes the driver and launches the Chrome app on an Android emulator.
- Logs a message when the app is launched successfully.
Appium Commands
Appium provides several commands to interact with mobile applications. Here are some common commands:
- driver.findElementById(id): Finds an element by its ID in the mobile app.
- driver.findElementByName(name): Finds an element by its name in the mobile app.
- driver.tap(x, y): Taps on a specified position on the screen (useful for custom gestures).
- driver.sendKeys(text): Types text into an input field.
- driver.getText(): Retrieves the text from an element.
Running Appium Tests
Appium tests can be run using your preferred test framework. For example, you can run the above test using TestNG or JUnit. Appium tests can also be integrated into a Continuous Integration (CI) pipeline using Jenkins or other CI tools.
Advanced Appium Features
- Mobile Gestures: Appium allows you to simulate advanced gestures like swipe, pinch, zoom, and drag and drop.
- Appium Inspector: A tool for inspecting mobile app elements and generating code for interactions with those elements.
- Cloud Testing: Appium supports cloud-based mobile testing platforms like Sauce Labs, BrowserStack, and AWS Device Farm for running tests on real devices in the cloud.
Best Practices for Appium Testing
- Use DesiredCapabilities: Always specify the desired capabilities for your tests, including platform name, device name, app package, and activity.
- Write Reusable Methods: Create reusable methods for interacting with elements to make your tests more maintainable.
- Use Explicit Waits: Use explicit waits to handle synchronization issues, ensuring that elements are ready before interacting with them.
- Test on Real Devices: While simulators/emulators are useful, testing on real devices provides a more accurate representation of app behavior.
- Use Logging: Always log key actions and results to help with debugging and understanding test failures.
Integrating Appium with CI/CD
Appium can be integrated into Continuous Integration (CI) and Continuous Delivery (CD) pipelines to automate mobile app testing. Popular tools like Jenkins, Travis CI, and CircleCI can be configured to run Appium tests automatically, ensuring that your app is always tested before deployment.
Conclusion
Appium is an excellent choice for automating mobile application testing across both Android and iOS platforms. With its support for multiple programming languages, cloud testing, and advanced mobile gestures, Appium provides the flexibility and power to handle various mobile testing scenarios efficiently. Whether you're testing native, hybrid, or mobile web apps, Appium is a versatile solution for your mobile testing needs.
JMeter for Load Testing
Apache JMeter is an open-source tool designed for performance testing, especially load testing, for web applications. It can simulate heavy loads on a server, group of servers, network, or object to test its strength and analyze overall performance under different load types. JMeter is widely used for load testing, stress testing, and performance testing, providing detailed insights into the system's behavior under different conditions.
What is JMeter?
JMeter is a Java-based desktop application designed to load test functional behavior and measure the performance of web applications. While originally designed for web application testing, JMeter has evolved to support a variety of protocols such as HTTP, FTP, database testing, and more. Its user-friendly interface allows testers to set up, execute, and analyze load tests with ease.
Why Use JMeter for Load Testing?
- Open Source: JMeter is free to use and open-source, making it an accessible tool for testers.
- Protocol Support: JMeter supports multiple protocols, such as HTTP, HTTPS, FTP, JDBC, and more.
- Scalability: It can simulate a large number of users, making it suitable for testing applications under varying loads.
- Powerful Reporting: JMeter generates detailed reports, graphs, and logs, which help in analyzing the performance and behavior of the application under load.
- Extensibility: JMeter allows custom plugins and scripts for more advanced testing needs.
Setting Up JMeter for Load Testing
Follow these steps to set up JMeter for your load testing needs:
Step 1: Install JMeter
- Download the latest version of JMeter from the official website: https://jmeter.apache.org.
- Extract the downloaded ZIP file to a directory of your choice.
- Ensure you have Java installed on your system, as JMeter is a Java-based application. To verify, run
java -version
in the command prompt or terminal. - Navigate to the bin folder of the extracted JMeter directory and run
jmeter.bat
(for Windows) orjmeter
(for Linux/macOS) to launch the JMeter GUI.
Step 2: Create a Test Plan
After launching JMeter, the first step is to create a Test Plan. The Test Plan contains all the configurations for the load test.
- Right-click on the Test Plan node and select Add → Threads (Users) → Thread Group.
- The Thread Group allows you to simulate a number of virtual users and configure their behavior.
Step 3: Add Samplers
Samplers are used in JMeter to send requests to the server. For web applications, you typically use the HTTP Request sampler.
- Right-click on the Thread Group and select Add → Sampler → HTTP Request.
- Configure the HTTP Request sampler by specifying the server name, port, and path to the resource being tested.
Step 4: Add Listeners for Results
Listeners in JMeter are used to capture the results of your test execution. There are several types of listeners, such as Summary Report, Graph Results, and View Results Tree.
- Right-click on the Thread Group and select Add → Listener → View Results in Table.
- Listeners allow you to view the response times, throughput, and other performance metrics as the test runs.
Step 5: Run the Test
Once the test plan is set up, you can start the test by clicking the green Start button in the toolbar.
JMeter will simulate the load as per the configuration, and you can view the results in real-time via the listeners.
Analyzing Results
After running the test, JMeter provides various reports and graphs that help you analyze the performance of your application:
- Response Time: Measures the time it takes for the application to respond to requests.
- Throughput: Shows the number of requests that the server can handle per unit of time.
- Error Rate: Displays the percentage of requests that failed during the test.
- CPU and Memory Usage: Helps you monitor the resource utilization of the server during the test.
JMeter Command-Line Mode
JMeter can also be run in non-GUI mode, which is useful for running performance tests in a CI/CD pipeline or on a remote server. To run JMeter in command-line mode, use the following command:
jmeter -n -t test-plan.jmx -l result.jtl -e -o /path/to/report
This command runs a test plan file test-plan.jmx
and saves the results to result.jtl
, generating an HTML report at the specified path.
JMeter Load Testing Best Practices
- Start Small: Begin with a small number of users and gradually increase the load to avoid overwhelming the system.
- Use Realistic Test Data: Ensure that the test data mimics real-world scenarios to get accurate results.
- Monitor Server Resources: Track CPU, memory, and network usage on the server to identify potential bottlenecks.
- Run Multiple Tests: Conduct tests at different times of day, under different network conditions, and using varying loads to get comprehensive data.
- Analyze Results Thoroughly: Focus on key performance metrics such as response times, error rates, and throughput to determine application bottlenecks.
Advanced JMeter Features
- Distributed Testing: JMeter allows you to run tests from multiple machines to simulate a larger load and test scalability.
- Custom Plugins: JMeter supports custom plugins for advanced testing needs, such as WebSocket, JMS, and database testing.
- Assertions: Assertions in JMeter allow you to verify the correctness of responses during the load test, ensuring that the application behaves as expected under load.
Conclusion
JMeter is a powerful, flexible tool for load testing and performance testing of web applications. Its user-friendly interface, scalability, and extensive reporting capabilities make it an ideal choice for testers looking to evaluate the performance of their applications under varying loads. By following best practices and leveraging JMeter’s advanced features, you can effectively test your application’s performance and ensure it meets the required standards before going live.
Postman for API Testing
Postman is a popular, powerful tool used for testing APIs by sending requests and analyzing responses. It provides an intuitive, easy-to-use interface that helps developers and testers create, test, and debug APIs in various formats such as REST, SOAP, and GraphQL. Postman simplifies the process of API testing by allowing for automation, collaboration, and integration with CI/CD pipelines.
What is Postman?
Postman is an API development and testing tool that facilitates the creation, execution, and automation of API requests. It offers a comprehensive suite of features, including request building, response viewing, automated testing, and integration with other testing tools and services. Postman is widely used for both manual and automated API testing, offering support for a variety of protocols such as HTTP, HTTPS, and WebSocket.
Why Use Postman for API Testing?
- Ease of Use: Postman’s graphical interface is simple to use, making it easy to send requests and analyze responses.
- Comprehensive API Testing: Postman supports various types of API testing such as functional, regression, and performance testing.
- Automation Support: Postman provides support for scripting and automation, making it easier to run tests in continuous integration pipelines.
- Collaboration: Postman allows teams to collaborate on API development and testing by sharing collections and environments.
- Environment Variables: Postman allows the use of variables in the request, making it easy to switch between different environments, such as development, staging, and production.
Setting Up Postman for API Testing
Follow these steps to get started with Postman for API testing:
Step 1: Download and Install Postman
- Visit the official Postman website: https://www.postman.com.
- Download the version suitable for your operating system (Windows, macOS, or Linux).
- Follow the installation instructions to set up Postman on your machine.
Step 2: Create a New Request
Once Postman is installed, you can start creating API requests:
- Click on the New button in the upper-left corner of the Postman window.
- Select Request from the list of options.
- Provide a name for your request and add it to a collection for better organization.
- In the request editor, enter the API endpoint URL, choose the HTTP method (GET, POST, PUT, DELETE), and set any necessary headers, parameters, or authentication.
Step 3: Send the Request
Once you’ve configured the request, click the Send button to execute the request. Postman will display the response in the lower section of the screen, which will include details such as:
- Status Code: HTTP status code (e.g., 200 OK, 404 Not Found).
- Response Body: The content returned by the API (e.g., JSON, XML).
- Response Time: Time taken by the server to respond to the request.
Step 4: Automate API Testing
Postman allows you to automate API tests using the built-in scripting feature. To add tests to your API requests:
- Click on the Tests tab in the request editor.
- Use JavaScript to write test scripts for validating responses. For example:
// Check for a successful status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
Step 5: Run Automated Tests Using Collection Runner
Postman’s Collection Runner enables you to run a series of tests (requests) in bulk:
- Click on the Runner button located at the top of the Postman window.
- Select a collection that contains your tests and configure options such as iterations and environment variables.
- Click Start Test to run the tests and view the results in real-time.
Postman Features for API Testing
- Collections: Organize API requests into collections for better management and reuse.
- Environments: Set up different environments (e.g., development, staging, production) and use environment variables to manage different sets of data.
- Pre-request Scripts: Postman allows you to run JavaScript code before a request is sent to set up conditions (e.g., authentication tokens).
- Mock Servers: Test your APIs without needing an actual backend server by creating mock servers that simulate API responses.
- Monitors: Automatically run your API tests at regular intervals and monitor API health with Postman monitors.
Integration with CI/CD
Postman can be integrated with continuous integration/continuous delivery (CI/CD) pipelines to automate API testing as part of the software delivery process:
- Newman: Postman’s command-line tool, Newman, allows you to run Postman collections from the command line, making it suitable for integration with CI/CD tools like Jenkins, GitLab CI, and more.
- Postman Collection Runner: Use the Collection Runner to run tests during a build process and gather results that can be integrated into a CI/CD pipeline.
Best Practices for API Testing with Postman
- Organize Requests: Use collections to group related requests and make your tests more manageable.
- Use Environment Variables: Leverage environment variables to easily switch between different environments (e.g., dev, staging, prod).
- Automate Tests: Write test scripts for each request to validate API responses and ensure functionality is as expected.
- Monitor API Health: Use Postman Monitors to run your tests at regular intervals and receive notifications on test results.
- Document API: Document your API tests and requests within Postman to improve collaboration with team members.
Conclusion
Postman is a versatile and powerful tool for API testing that streamlines the process of creating, sending, and automating API requests. With features such as collections, environments, and automated testing, Postman simplifies the process of API testing for developers and testers. By following best practices and integrating Postman into your CI/CD pipeline, you can ensure that your APIs are thoroughly tested and maintained, leading to higher-quality software and better user experiences.
Waterfall Model and Testing
The Waterfall Model is a traditional software development life cycle (SDLC) method where each phase of the process is completed sequentially. It is one of the earliest SDLC models and is characterized by a linear and structured approach to software development. In this model, the testing phase typically occurs after the development phase is complete, which means there is minimal overlap between development and testing efforts. While this approach works well for simpler projects with well-defined requirements, it can be less flexible in handling changes and iterations during development.
Overview of the Waterfall Model
The Waterfall Model is divided into distinct phases that must be completed before moving on to the next. The key phases are:
- Requirement Analysis: Gathering and documenting all the requirements for the software project.
- System Design: Creating the software architecture and design based on the requirements.
- Implementation: Writing the code based on the design and system specifications.
- Integration and Testing: Conducting system integration and performing thorough testing of the software.
- Deployment: Deploying the software to the production environment.
- Maintenance: Managing and resolving any issues or bugs after deployment.
Waterfall Model and Testing
Testing in the Waterfall Model occurs after the development phase, making it a "big bang" testing approach. The testing team begins testing only once the development team has completed all the coding and the system is integrated. Since the testing phase is separate and follows the development phase, it can lead to delays if issues are found late in the process.
Key Characteristics of Testing in the Waterfall Model
- Sequential Process: Testing occurs after development, meaning that testers do not have access to the product until the development phase is complete.
- Late Testing: Since testing starts only after the development phase, any bugs or issues discovered during testing can lead to delays in the project timeline.
- Defect Fixing: Once testing is completed, any defects or issues identified must be sent back for correction in the development phase, which can be time-consuming.
- Fixed Requirements: As requirements are typically gathered at the beginning and are not expected to change, the testing focuses only on the predefined functionality of the system.
Challenges with Testing in the Waterfall Model
- Limited Flexibility: If requirements change after the development process has started, it can be difficult to accommodate these changes, as testing has to wait until after development is complete.
- Late Detection of Issues: Since testing begins after development, defects or issues discovered can be costly and time-consuming to fix, leading to delays.
- Overlapping Phases: The lack of overlap between development and testing means that the testing team may not have enough time to fully understand the software before beginning testing, which can affect the quality of test cases and results.
- Risk of Miscommunication: As the testing team only gets involved after development, there is a higher risk of miscommunication or misunderstanding between developers and testers, leading to gaps in testing coverage.
Benefits of Testing in the Waterfall Model
- Clear Structure: The Waterfall Model’s sequential nature offers a clear structure, where each phase is well-defined and easy to manage. Testing is conducted only once all development work is finished.
- Documentation: The Waterfall approach relies heavily on documentation, making it easier to track the progress of the project, including testing activities and defect reports.
- Predictable Timeline: Since each phase is completed before the next begins, it is easier to estimate the overall project timeline, allowing for better planning and resource allocation.
Best Practices for Testing in the Waterfall Model
- Thorough Requirement Analysis: Before starting the development phase, ensure that all requirements are clearly defined and documented. This minimizes the risk of scope changes and helps testers focus on the correct functionality.
- Early Test Planning: Even though testing happens after development, test planning should start early. The test team should create detailed test plans, test cases, and test scripts based on the requirements analysis.
- Effective Communication: Foster collaboration between developers and testers to ensure that the testing phase starts smoothly, and testers fully understand the system before they begin testing.
- Comprehensive Documentation: Maintain accurate records of test results, defects, and issues discovered during testing to ensure that they are properly addressed and can be tracked through the defect life cycle.
- Early Detection of Issues: While testing occurs after development, it’s crucial to conduct thorough reviews and inspections during the development phase to catch potential issues early and reduce the risk of defects in the later stages.
Waterfall Model in Modern Testing Approaches
While the Waterfall Model has been largely replaced by more iterative and flexible models like Agile, it is still used in certain scenarios, especially in projects with well-defined requirements and limited scope for changes. In modern testing, some organizations still use aspects of the Waterfall Model, such as having distinct phases for testing, while incorporating more flexible techniques like Agile testing or DevOps practices to improve the overall efficiency and responsiveness of the testing process.
Conclusion
The Waterfall Model is a traditional SDLC approach that places testing after the development phase. While this approach offers a clear structure and predictability, it can introduce challenges such as late detection of issues, limited flexibility, and the risk of rework. However, following best practices, such as early test planning, thorough requirement analysis, and effective communication, can help mitigate these challenges. The Waterfall Model may still be suitable for projects with stable requirements, but more modern, iterative approaches like Agile and DevOps are preferred for projects that require flexibility and quick feedback loops.
V-Model (Verification and Validation Model)
The V-Model is an extension of the Waterfall Model and is a software development life cycle (SDLC) model that emphasizes the relationship between each phase of development and its corresponding phase of testing. The V-Model is also known as the Verification and Validation model because it focuses on verification during the development phase and validation during the testing phase. The V-Model is structured in a way that for every development activity, there is a corresponding testing activity. This model is widely used for projects where requirements are well-defined and unlikely to change.
Overview of the V-Model
The V-Model is often represented in a V-shape, where the left side represents the stages of development, and the right side represents the corresponding stages of testing. The key stages of the V-Model are:
- Requirement Analysis: Gathering and documenting the system’s requirements.
- System Design: Creating the architecture and design based on the requirements.
- High-Level Design: Creating a high-level design of the system or software.
- Low-Level Design: Detailed design of individual modules or components.
- Implementation: Writing the code based on the design specifications.
- Unit Testing: Testing individual modules or components developed during the implementation phase.
- Integration Testing: Testing the interactions between integrated modules to ensure that they work together as expected.
- System Testing: Testing the entire system to validate that it meets the specified requirements.
- Acceptance Testing: Conducting final validation to ensure the product meets the customer’s expectations and requirements.
Verification and Validation in the V-Model
The V-Model gets its name from the two key activities: Verification and Validation. These activities occur at different phases of the model:
- Verification: This occurs during the development phases (on the left side of the V) and ensures that the system is being built correctly. The activities involved in verification include requirement analysis, design, and coding. The primary goal of verification is to ensure that the system is being developed according to the requirements and specifications.
- Validation: This occurs during the testing phases (on the right side of the V) and ensures that the system is built as per the user’s needs and expectations. The validation activities include unit testing, integration testing, system testing, and acceptance testing. The goal of validation is to ensure the system meets the real-world use case and works as expected.
V-Model Testing Phases
Each phase of the V-Model corresponds to a specific type of testing. These are aligned as follows:
- Requirement Analysis (Verification): The first step is to gather and analyze the requirements. The verification activity involves reviewing requirements to ensure that they are complete, clear, and feasible.
- System Design (Verification): This phase focuses on the high-level design of the system. The verification activity includes testing the design to ensure it aligns with the requirements.
- High-Level Design (Verification): The high-level design phase involves breaking down the system into modules. Verification checks the design to confirm that the system architecture is robust and meets the system’s needs.
- Low-Level Design (Verification): This phase involves detailing the modules. During verification, reviews and audits are conducted to ensure the modules are designed correctly.
- Implementation (Verification): The implementation phase is when the coding happens. Verification includes code reviews, static analysis, and unit testing to ensure that the code is correct and adheres to the design.
- Unit Testing (Validation): After the individual modules are developed, unit testing is done to validate the functionality of each module. It checks if the module behaves as expected according to the design specifications.
- Integration Testing (Validation): When the modules are integrated, integration testing validates that the interaction between modules works correctly.
- System Testing (Validation): This phase validates the entire system, ensuring that it meets the functional and non-functional requirements specified in the requirement analysis phase.
- Acceptance Testing (Validation): Acceptance testing ensures that the system is ready for deployment and that it meets the client’s expectations and business requirements. It is usually done by the customer or end users.
Advantages of the V-Model
- Clear Structure: The V-Model provides a clear structure for both development and testing, with each phase having corresponding verification and validation activities.
- Early Detection of Defects: Because verification is done at each phase of development, defects can be detected early, which reduces the cost and time of fixing them.
- Emphasis on Testing: Testing is given importance throughout the development process, ensuring that the final product is of high quality.
- Traceability: The V-Model ensures traceability from the requirements phase through to the acceptance testing phase, making it easy to verify that the system meets the requirements.
Disadvantages of the V-Model
- Rigid Structure: The V-Model follows a strict sequence of phases, and it is difficult to make changes after the project has begun. This makes it less flexible than models like Agile.
- Late Testing: While testing begins early in the V-Model, the actual validation of the system comes late in the process. If defects are found during validation, it may require significant rework.
- High Cost for Changes: Since the V-Model does not accommodate changes easily, making changes to the product after development has begun can be costly and time-consuming.
When to Use the V-Model
The V-Model is well-suited for projects that have well-defined requirements and are unlikely to change during development. It works best for systems where the requirements are stable, and there is little need for frequent iterations or changes throughout the development process. The V-Model is commonly used in industries such as manufacturing, healthcare, and embedded systems, where stability and clear requirements are essential.
Conclusion
The V-Model (Verification and Validation Model) is a software development life cycle model that emphasizes a structured and sequential approach to development and testing. By ensuring that testing is integrated into each phase of development, the V-Model helps detect defects early, ensures that the product meets the requirements, and provides traceability throughout the process. While the V-Model has advantages, such as early defect detection and a clear structure, it may not be suitable for projects that require flexibility and frequent changes. It is best used in scenarios where requirements are stable and well-understood.
Agile Model and Testing Approach
The Agile Model is an iterative and incremental approach to software development where both development and testing activities are carried out simultaneously in short cycles known as iterations or sprints. This model emphasizes flexibility, collaboration, and customer feedback, allowing teams to adapt to changing requirements and deliver working software at the end of each iteration.
Overview of the Agile Model
In the Agile Model, development is broken down into small, manageable units, and each unit is developed and tested within a short time frame called a sprint. The Agile process involves regular feedback from stakeholders and continuous collaboration between the development and testing teams. Agile promotes adaptive planning, continuous improvement, and rapid delivery of high-quality software.
Key Features of Agile Model
- Iterative Development: Software is developed in small, incremental units called iterations or sprints, typically lasting 1-4 weeks.
- Collaboration: Continuous collaboration between developers, testers, and stakeholders ensures that the product meets user needs.
- Customer Feedback: Frequent customer feedback is integrated into each iteration to ensure that the software aligns with user expectations.
- Flexibility: The Agile Model allows teams to adapt to changing requirements and priorities throughout the development process.
- Continuous Delivery: Working software is delivered at the end of each sprint, allowing teams to release frequently and iteratively.
Agile Testing Approach
In Agile, testing is integrated into the development process and occurs continuously throughout each iteration. The Agile testing approach emphasizes early and frequent testing, with testers participating in all phases of development. Agile testing focuses on collaboration, quick feedback, and the ability to adapt to changing requirements quickly.
Testing in Agile Phases
Agile testing is conducted in parallel with development tasks and involves frequent feedback between the developers and testers. The testing process in Agile follows these phases:
- Test Planning: In the early stages of a sprint, the testing team collaborates with developers to understand the user stories, acceptance criteria, and overall goals of the sprint. Test plans and strategies are created based on these discussions.
- Test Design: Testers design test cases based on user stories and acceptance criteria. These test cases focus on both functional and non-functional aspects of the product.
- Test Execution: Testing is performed continuously during each iteration. Testers execute test cases as code is developed, often using automated testing to speed up the process.
- Defect Reporting: If defects are found, they are reported quickly, and developers work collaboratively with testers to address them. Agile encourages immediate fixes to defects within the same sprint.
- Test Closure: At the end of each sprint, testing results are evaluated, and the team discusses what went well and what could be improved for the next sprint.
Agile Testing Principles
Agile testing follows key principles that help ensure the success of the testing process:
- Testing is done continuously: Testing is not a separate phase but is performed throughout the development cycle. This ensures that defects are identified early.
- Collaboration between testers and developers: Testers and developers work closely together to ensure that testing is integrated into the development process.
- Automated testing: Automated tests are often used in Agile to speed up regression testing, particularly for repetitive tasks, and to ensure that changes do not break existing functionality.
- Customer involvement: Feedback from customers or stakeholders is incorporated throughout the process to ensure that the product meets user needs.
- Acceptance criteria: Clear acceptance criteria are defined for each feature or user story to guide testing and ensure that the software meets the required functionality.
Benefits of Agile Testing
- Early detection of defects: Testing occurs early and frequently, which allows defects to be identified and fixed faster.
- Flexibility: Agile testing can easily accommodate changes in requirements or priorities, allowing teams to adapt quickly and efficiently.
- Increased collaboration: Continuous collaboration between developers, testers, and stakeholders leads to better-quality software and more effective problem-solving.
- Faster delivery: Agile testing contributes to faster delivery of working software, as frequent iterations allow teams to release smaller, incremental updates regularly.
- Customer satisfaction: Regular feedback from customers ensures that the software continuously meets their needs, improving customer satisfaction.
Challenges of Agile Testing
- Requires a collaborative mindset: Agile testing relies heavily on communication and collaboration, which can be challenging for teams that are not accustomed to working closely together.
- Test automation challenges: While automation is encouraged in Agile testing, it can be difficult to automate certain types of tests, such as exploratory or usability tests.
- Frequent changes in scope: Agile projects often experience changes in requirements, which can complicate testing efforts and require adjustments to test cases and plans.
- High pressure to deliver quickly: The fast pace of Agile development can put pressure on testers to keep up with frequent releases and manage quick turnaround times for testing.
Tools for Agile Testing
Several tools are commonly used in Agile testing to help manage test cases, automate testing, and ensure continuous integration. Some of the popular tools include:
- Jira: A widely used project management tool for tracking and managing user stories, tasks, and defects in Agile projects.
- TestRail: A test case management tool that helps teams organize and track manual and automated test cases.
- Jenkins: An automation server used for continuous integration and testing of the software in Agile projects.
- Selenium: A popular tool for automating functional testing in Agile projects, particularly for web applications.
- Appium: A cross-platform mobile testing tool used in Agile projects for automating mobile application testing.
Conclusion
The Agile Model is a flexible and adaptive approach to software development that emphasizes collaboration, customer feedback, and iterative delivery of working software. Testing in Agile is an integral part of the development process, with testers collaborating closely with developers and stakeholders to ensure high-quality software. Agile testing enables teams to identify and resolve defects early, deliver working software frequently, and respond to changing requirements efficiently. By following Agile testing principles, teams can improve software quality, customer satisfaction, and overall project success.
Spiral Model and Risk-Based Testing
Overview of the Spiral Model
The Spiral Model is a software development life cycle (SDLC) model that combines iterative development with systematic risk management. It is particularly useful for large, complex, and high-risk projects where requirements are likely to change over time. The Spiral Model emphasizes continuous refinement and improvement through repeated iterations or "spirals," each of which involves a cycle of planning, design, development, and testing.
Key Features of the Spiral Model
- Risk-driven: The Spiral Model focuses on identifying and mitigating risks throughout the project lifecycle.
- Iterative process: The project is divided into smaller, manageable phases (spirals) that are repeated, with each iteration refining the product.
- Prototyping: Prototypes are often built and tested during the early stages of the project, enabling stakeholders to provide feedback.
- Flexible and adaptive: The model allows for changes in the project’s scope, design, and requirements during each iteration.
- Continuous testing and evaluation: Testing is performed in each iteration, ensuring that the product meets quality standards at every stage of development.
Phases of the Spiral Model
The Spiral Model is divided into four main phases, which are repeated iteratively in each spiral:
- Planning: This phase involves defining objectives, identifying risks, and determining the scope of the project. A risk analysis is conducted to identify potential issues and mitigation strategies.
- Risk Analysis: The team evaluates risks in the project, such as technological risks, financial risks, and resource risks. Based on the analysis, decisions are made about the project’s course of action.
- Engineering and Development: In this phase, the actual development and design of the product take place. Prototypes may be built, and development continues based on feedback from earlier phases.
- Testing and Evaluation: At this stage, testing is performed to ensure that the product meets the requirements. Any issues discovered during testing are addressed before moving to the next spiral.
Risk-Based Testing
Risk-Based Testing (RBT) is an approach to software testing where testing efforts are prioritized based on the risks associated with different parts of the application. The goal of RBT is to focus testing resources on the most critical areas of the system to reduce the likelihood of failure and deliver high-quality software. This approach is especially useful in projects following the Spiral Model, as it aligns with the risk-driven nature of the model.
Key Concepts of Risk-Based Testing
- Risk Identification: The first step in RBT is to identify potential risks that might affect the product. These risks can be related to functionality, performance, security, or any other aspect of the system.
- Risk Assessment: Once risks are identified, they are assessed in terms of their likelihood of occurrence and the impact they would have on the project. This helps prioritize testing efforts for the most critical risks.
- Risk Mitigation: Testing is focused on high-risk areas to ensure that critical issues are identified and resolved early in the development process.
- Continuous Monitoring: Risks are continuously monitored throughout the project, and testing efforts are adjusted accordingly to address any new or evolving risks.
Benefits of Risk-Based Testing
- Efficient resource allocation: By focusing on high-risk areas, testing efforts and resources are used more effectively, ensuring that critical defects are caught early.
- Improved test coverage: RBT ensures that the most important areas of the product are thoroughly tested, providing better test coverage for high-risk features.
- Better risk management: By identifying and addressing risks early, RBT helps in managing the overall project risks, ensuring a higher-quality product.
- Early detection of defects: By focusing on critical areas, RBT increases the chances of detecting defects earlier in the development process.
Steps to Implement Risk-Based Testing
- Identify potential risks: Work with stakeholders to identify areas of the system that pose the highest risk. This can include technical risks, business risks, and environmental risks.
- Assess risks: Evaluate the likelihood of each identified risk and the potential impact it could have on the project. This helps prioritize testing efforts.
- Prioritize testing areas: Use the risk assessment to focus testing on the areas that are most critical or have the highest probability of failure.
- Design test cases: Create test cases that specifically address the identified risks. Ensure that these tests are thorough and focus on high-risk areas.
- Execute tests: Perform testing based on the prioritized risk areas. Use appropriate testing techniques, such as boundary value analysis or equivalence partitioning, to ensure comprehensive coverage.
- Track and mitigate risks: Continuously monitor and assess risks throughout the development cycle. Adjust testing efforts as necessary based on new risks or changes in priorities.
Combining Spiral Model and Risk-Based Testing
The Spiral Model and Risk-Based Testing go hand in hand, as both approaches emphasize the importance of managing and mitigating risks throughout the development process. The Spiral Model's iterative nature and focus on risk management make it well-suited for projects that require flexibility and continuous improvement. By integrating Risk-Based Testing into the Spiral Model, teams can ensure that testing efforts are aligned with the project's most critical risks and that defects are caught early in the development cycle.
Benefits of Combining Spiral Model and Risk-Based Testing
- Proactive risk management: The combination allows teams to proactively manage and mitigate risks, ensuring that critical issues are addressed early in the development process.
- Efficient use of resources: Testing efforts are focused on the highest-risk areas, leading to more efficient resource allocation and better test coverage.
- Continuous improvement: Both approaches emphasize iterative development and testing, enabling teams to continuously improve the product with each spiral.
- Higher-quality software: By focusing on high-risk areas and addressing defects early, teams can deliver a higher-quality product that meets user needs and expectations.
Conclusion
The Spiral Model is a risk-driven, iterative approach to software development that allows for flexibility and continuous improvement. When combined with Risk-Based Testing, it provides a comprehensive framework for identifying, assessing, and mitigating risks throughout the development cycle. By focusing testing efforts on high-risk areas, teams can ensure that critical defects are detected early, leading to higher-quality software and more efficient resource usage. The combination of the Spiral Model and Risk-Based Testing is particularly effective for complex projects where managing risks is essential to success.
Incremental Model Testing
Overview of the Incremental Model
The Incremental Model is a software development methodology where the system is designed, developed, and tested in small, manageable parts or increments. These increments are built one after another and are progressively integrated into the system until the full application is complete. Each increment is typically a functional component of the system, and as each part is developed, it is tested before moving on to the next part. This model emphasizes continuous delivery and iterative development, allowing for faster delivery of functional software.
Key Features of the Incremental Model
- Modular Development: The system is divided into smaller, functional parts or modules. Each module is developed and tested separately before being integrated with the rest of the system.
- Continuous Integration: As each increment is developed, it is integrated into the existing system, ensuring that the software grows progressively while maintaining functionality.
- Early Testing: Testing is done early, as each increment is tested independently, which allows for the early identification of issues and defects in specific parts of the software.
- Flexibility: Since each increment is developed separately, there is flexibility for changes to be made during later stages of development.
- Faster Delivery: The Incremental Model allows for early delivery of functional software. Each increment can be released as a working part of the system, providing tangible progress for stakeholders.
Phases of the Incremental Model
The Incremental Model typically follows a straightforward set of phases, repeated iteratively for each increment:
- Requirement Analysis: The system's requirements are identified and prioritized. The most critical or high-priority features are selected for the first increment.
- System Design: A high-level design is created for the system, and the design for the first increment is developed. This design is refined as each subsequent increment is added.
- Implementation: The code for the first increment is written and implemented. As each subsequent increment is planned, it is developed, implemented, and tested independently.
- Testing: Each increment is tested in isolation. The testing focuses on the individual functionality and integration of that increment within the larger system.
- Integration: Once the increment is developed and tested, it is integrated into the overall system. This process continues for each subsequent increment until the system is complete.
Testing in the Incremental Model
Testing plays a crucial role in the Incremental Model. Each module or increment is tested thoroughly to ensure that it functions correctly and integrates well with the other parts of the system. The testing process in this model focuses on verifying the functionality of each increment and ensuring that it meets the defined requirements.
Types of Testing in Incremental Model
- Unit Testing: Each increment undergoes unit testing to validate its individual functionality. This ensures that the module or component works independently before being integrated into the system.
- Integration Testing: After each increment is added to the system, integration testing is performed to check how well it interacts with other parts of the system. It ensures that the increments work together smoothly.
- System Testing: As the system grows incrementally, system testing is performed on the complete system to ensure that all components function together as intended.
- Regression Testing: Each new increment may affect previously developed parts of the system. Regression testing is conducted to ensure that new changes do not break existing functionality.
- Acceptance Testing: Each increment is tested for user acceptance to verify that it meets business or user requirements. This ensures that each part of the system meets the necessary standards before moving forward.
Advantages of Incremental Model Testing
- Early Testing: Since each increment is tested individually as it is developed, defects can be identified and addressed earlier in the development process.
- Faster Feedback: Stakeholders can see working software at the end of each increment, which provides faster feedback on the development progress and functionality.
- Risk Mitigation: Testing smaller portions of the software reduces the risk of major issues affecting the entire system. Problems can be addressed in isolation before they affect the overall project.
- Improved Quality: With each increment being thoroughly tested, the quality of the final product is higher, as individual defects are caught and resolved early.
- Flexibility for Changes: The Incremental Model allows for flexibility in responding to changes or new requirements, as each increment is developed and tested independently.
Challenges of Incremental Model Testing
- Complex Integration: As the system grows, the integration of each new increment with the existing system may become more complex, requiring careful management of dependencies between modules.
- Testing Dependencies: Some increments may have dependencies on others, and testing may be more challenging if these dependencies are not clearly defined or managed.
- Increased Overhead: Each increment requires testing, which can increase the overhead in terms of time and resources, especially if there are many increments to be developed and tested.
- Incomplete Features: Since the system is developed incrementally, stakeholders may have to wait for multiple increments to be completed before they see the full functionality, potentially delaying feedback on some features.
Best Practices for Incremental Model Testing
- Define Clear Requirements: Ensure that each increment is based on well-defined requirements, prioritizing the most important features for the first increment.
- Focus on Test Automation: Automating tests for each increment can reduce testing time and effort, especially when performing repetitive tests like regression or integration testing.
- Prioritize Critical Functions: Focus on testing the most critical functions in the early increments to ensure that they are thoroughly tested and validated before moving on to less critical features.
- Maintain Continuous Integration: Ensure that increments are integrated into the system as soon as they are developed, which helps identify integration issues early and facilitates continuous testing.
- Use Version Control Systems: Implement version control to manage the different versions of each increment, ensuring that changes are tracked and testing is performed on the correct versions of the system.
Conclusion
The Incremental Model is a software development approach that allows for the gradual development and testing of systems through small, manageable increments. Testing in this model is critical, as each increment is tested individually and integrated into the overall system. By conducting thorough testing at each stage, teams can ensure that defects are caught early, the system functions as expected, and the project stays on track. While the Incremental Model offers many benefits, such as early testing and faster feedback, it also presents challenges, particularly around integration and dependencies. However, with careful planning and a focus on best practices, these challenges can be managed effectively.
AI and Machine Learning in Testing
Overview of AI and Machine Learning in Testing
Artificial Intelligence (AI) and Machine Learning (ML) are transforming the software testing landscape by enhancing test automation, improving defect detection, and optimizing testing processes. AI in testing refers to the integration of intelligent algorithms that mimic human decision-making to automate and improve testing processes. Machine learning, a subset of AI, involves training algorithms to learn from historical test data and adapt to new testing scenarios, improving testing effectiveness over time. By leveraging AI and ML, testing becomes more efficient, accurate, and scalable, with the ability to detect complex issues that traditional methods may miss.
Key Benefits of AI and Machine Learning in Testing
- Enhanced Test Automation: AI and ML can automate complex and repetitive testing tasks, allowing testers to focus on high-level test design. These technologies help in automating test case generation, execution, and maintenance, reducing manual effort and errors.
- Faster Test Execution: AI can optimize test execution and prioritize test cases based on risk, impact, and historical data, improving the overall speed of testing. ML algorithms can predict which parts of the software are most likely to fail, enabling testers to focus their efforts on critical areas.
- Smarter Test Case Generation: AI-driven tools can generate new test cases based on requirements, previous test results, and code changes, ensuring comprehensive test coverage without manual intervention.
- Reduced Testing Time: ML models can analyze test execution data and suggest the most effective testing strategies, ensuring that only the necessary tests are run, thus reducing testing time and resource consumption.
- Improved Defect Detection: AI can analyze historical defect data to identify patterns and suggest areas of the application that are more prone to errors, enabling more focused and precise defect detection.
- Continuous Learning and Adaptation: Machine learning models continuously learn from historical test data and adapt to new testing scenarios. This results in more accurate predictions for test outcomes, which improves the overall effectiveness of the testing process.
Applications of AI and Machine Learning in Testing
- Test Automation: AI can automate test case creation, execution, and maintenance. For example, AI-based tools can automatically generate test scripts by analyzing application code and identifying potential test scenarios.
- Predictive Analytics: Machine learning models can predict defects based on historical data, prioritizing testing efforts in areas that are most likely to experience issues. This predictive analysis can help testing teams focus on high-risk areas of the application.
- Visual Testing: AI-powered visual testing tools can automatically detect UI bugs by comparing the current application UI with the expected UI. This technique helps detect visual regressions, which may be difficult to spot manually.
- Test Optimization: AI can optimize the test suite by identifying redundant or obsolete tests, reducing the overall number of tests while maintaining adequate coverage. This helps save time and resources during the testing process.
- Self-Healing Tests: AI-powered tools can automatically detect and repair broken test scripts due to changes in the application’s UI or functionality, minimizing the maintenance effort required for test automation.
- Intelligent Defect Detection: AI can be used to analyze logs, errors, and user behavior to detect patterns that indicate potential defects. This enables more proactive detection of issues even before they manifest in the user experience.
How AI and Machine Learning Improve Test Automation
AI and machine learning can take traditional test automation to the next level by making the testing process more intelligent and adaptable. Here are several ways AI and ML enhance test automation:
- Test Script Generation: ML algorithms analyze the application and automatically generate test scripts based on the code changes, requirements, and previous test executions. This eliminates the need for manual test script writing and ensures better test coverage.
- Self-Healing Automation: With AI, test scripts can automatically identify and adjust to changes in the application, such as UI updates or changes in business logic. This reduces the manual effort required to update test scripts and ensures that tests remain relevant and executable without constant maintenance.
- Test Prioritization: ML models use historical test data to prioritize tests based on their risk, impact, and likelihood of failure. This ensures that the most critical tests are executed first, leading to a faster feedback loop and quicker detection of defects.
- Intelligent Regression Testing: AI can analyze previous test runs and determine which tests should be run during regression testing. It can identify which parts of the application are most likely to be impacted by recent code changes, optimizing the regression suite and reducing unnecessary tests.
- Automated Bug Detection: AI can analyze logs, errors, and application data to identify potential defects faster. By applying machine learning models, it can detect patterns that would be difficult for a human tester to catch, improving the accuracy of defect detection.
AI and Machine Learning Tools for Testing
Several AI and ML-powered tools are available for enhancing various aspects of the testing process:
- Testim: Testim uses AI and machine learning to automate test creation, execution, and maintenance. The tool is capable of self-healing tests, making it easier to manage automated tests over time.
- Applitools: Applitools leverages AI for visual testing and can automatically detect visual bugs by comparing expected and actual UI states. Its machine learning algorithms can also adapt to different resolutions and screen sizes.
- Eggplant: Eggplant uses AI for intelligent test automation, including visual testing, performance testing, and functional testing. It uses machine learning to predict potential defects and optimize test execution strategies.
- Functionize: Functionize is an AI-based test automation platform that analyzes applications and automatically generates test scripts. It also uses machine learning to optimize test execution and maintain automation scripts.
- Test.ai: Test.ai uses AI to automate test case generation, execution, and bug detection. It also uses machine learning to improve test accuracy and efficiency over time.
Challenges in AI and Machine Learning in Testing
- Data Quality: AI and ML models rely on large amounts of high-quality data to learn and make accurate predictions. Poor-quality or biased data may lead to inaccurate results and ineffective test automation.
- Complexity: Implementing AI and ML in testing requires specialized knowledge and expertise. The setup and maintenance of AI-powered testing tools may require more effort than traditional test automation.
- Initial Investment: AI and ML tools often require significant initial investment in terms of time, resources, and training. This may be a barrier for smaller teams or organizations with limited budgets.
- Adaptability: While AI can adapt to changes in the application, it may still require manual intervention when there are significant changes or complex scenarios. The learning curve may also be steep for teams unfamiliar with AI-driven testing tools.
Conclusion
AI and Machine Learning are revolutionizing the testing industry by making test automation smarter, faster, and more efficient. These technologies enhance test case generation, defect detection, and predictive analysis, ultimately improving the quality and speed of software development. Though there are challenges in adopting AI and ML in testing, their benefits far outweigh the obstacles, particularly as AI continues to evolve and integrate more seamlessly into testing workflows. As AI and ML evolve, they will undoubtedly play an increasingly vital role in driving innovation and improving the efficiency of software testing.
Testing Blockchain Applications
Introduction to Blockchain Testing
Blockchain applications are decentralized, secure, and immutable systems that require rigorous testing to ensure their functionality, security, and performance. Testing these applications involves validating the blockchain network, consensus algorithms, smart contracts, and overall system integration. Blockchain testing ensures the system operates as intended, handles diverse scenarios, and maintains data integrity across distributed nodes.
Key Areas of Blockchain Application Testing
- Functional Testing: Verifies that the blockchain application functions according to the requirements. It includes testing transactions, blocks, and smart contract execution.
- Performance Testing: Evaluates the application's ability to handle high transaction loads, network latency, and scalability under varying conditions.
- Security Testing: Identifies vulnerabilities in the blockchain application, such as susceptibility to hacking, double-spending, or unauthorized access.
- Integration Testing: Ensures smooth interaction between different components of the blockchain application, including APIs, databases, and third-party systems.
- Smart Contract Testing: Validates the logic, execution, and security of smart contracts deployed on the blockchain.
- Node Testing: Ensures that individual blockchain nodes perform as expected, including synchronization, data propagation, and resilience.
- Data Integrity Testing: Verifies that data on the blockchain remains immutable and consistent across all participating nodes.
Challenges in Blockchain Application Testing
- Decentralization: Testing a distributed system with multiple nodes requires simulating real-world scenarios involving different nodes communicating across a network.
- Complexity: Blockchain systems involve intricate algorithms, cryptographic protocols, and consensus mechanisms, making testing a highly specialized task.
- Immutability: The irreversible nature of blockchain transactions makes it challenging to test and debug without impacting the integrity of the chain.
- Smart Contract Bugs: Bugs in smart contracts can lead to financial losses or security breaches, emphasizing the need for thorough testing.
- Scalability: Testing the scalability of blockchain applications is complex due to their reliance on distributed networks and consensus protocols.
Testing Strategies for Blockchain Applications
- Test on Private Blockchain: Use a private blockchain environment for initial testing to simulate real-world scenarios without impacting the main network.
- Automated Testing: Automate repetitive tasks such as functional and regression testing to improve efficiency and consistency.
- Smart Contract Audits: Perform thorough code reviews and audits to identify vulnerabilities and ensure the correctness of smart contracts.
- Stress Testing: Simulate high transaction volumes and node failures to evaluate the system's performance and resilience.
- Penetration Testing: Conduct security testing to identify potential vulnerabilities and safeguard against unauthorized access or attacks.
Tools for Blockchain Application Testing
- Ganache: A personal blockchain for testing Ethereum-based applications. It allows developers to deploy, test, and debug smart contracts locally.
- Truffle Suite: A framework for developing and testing blockchain applications, including smart contract deployment and automated testing.
- Hardhat: A development environment for Ethereum that supports debugging, deployment, and testing of smart contracts.
- Hyperledger Caliper: A performance testing tool for blockchain applications, compatible with various blockchain platforms like Hyperledger Fabric and Ethereum.
- Postman: Used for testing APIs in blockchain applications to ensure smooth communication between components.
- MythX: A security analysis tool for smart contracts to identify vulnerabilities and provide actionable insights.
Best Practices for Blockchain Testing
- Understand the Blockchain: Familiarize yourself with the blockchain platform, consensus mechanism, and smart contract language before testing.
- Design Comprehensive Test Cases: Cover all functional, non-functional, and edge-case scenarios to ensure thorough testing.
- Focus on Security: Prioritize security testing to protect against common blockchain vulnerabilities like 51% attacks, Sybil attacks, and double-spending.
- Test Multiple Scenarios: Simulate various network conditions, such as high latency or node failures, to evaluate system resilience.
- Perform Regular Audits: Continuously audit smart contracts and the blockchain system to ensure security and compliance.
- Leverage Testing Tools: Use specialized tools like Ganache, Truffle, and Hyperledger Caliper to streamline testing processes.
Conclusion
Testing blockchain applications is critical to ensure their security, functionality, and performance. With the unique challenges posed by decentralized systems, testing strategies must be adapted to address issues like smart contract vulnerabilities, network scalability, and data integrity. By leveraging the right tools and best practices, teams can deliver robust blockchain applications that meet user expectations and maintain the integrity of the blockchain ecosystem.
Cloud-Based Testing Platforms
Introduction to Cloud-Based Testing
Cloud-based testing platforms provide scalable, on-demand environments for testing applications over the internet. These platforms enable teams to perform various types of testing, such as functional, performance, security, and compatibility testing, without the need to manage and maintain physical infrastructure. Cloud testing is cost-effective, flexible, and ideal for distributed teams.
Key Features of Cloud-Based Testing Platforms
- Scalability: Easily scale resources up or down based on testing requirements, supporting high-demand scenarios like performance and load testing.
- Cost-Effectiveness: Pay-as-you-go pricing models reduce the cost of maintaining dedicated infrastructure for testing.
- Global Access: Teams from different locations can access the testing platform seamlessly, enabling collaboration in real time.
- Cross-Browser and Device Testing: Test applications across various browsers, operating systems, and devices to ensure compatibility.
- Integration Capabilities: Easily integrate with CI/CD pipelines, test management tools, and bug-tracking systems for streamlined workflows.
Advantages of Cloud-Based Testing
- Faster Time to Market: On-demand testing environments speed up the development and testing cycles.
- Flexibility: Test from anywhere with internet access, allowing remote and distributed teams to collaborate effectively.
- Reduced Maintenance: The platform provider manages updates, patches, and infrastructure, freeing up teams to focus on testing.
- Enhanced Test Coverage: Access to a wide range of devices, browsers, and operating systems ensures comprehensive compatibility testing.
- Improved Collaboration: Cloud platforms facilitate communication and transparency among team members by providing centralized access to testing results.
Challenges of Cloud-Based Testing
- Data Security: Ensuring the security and privacy of sensitive test data can be a concern for organizations using shared cloud environments.
- Latency: Network connectivity issues can affect the performance of testing activities in the cloud.
- Vendor Lock-In: Dependency on a specific cloud vendor may limit flexibility and increase switching costs.
- Compliance: Ensuring compliance with data protection regulations, such as GDPR or HIPAA, when using cloud platforms.
Popular Cloud-Based Testing Platforms
- BrowserStack: A popular platform for cross-browser and mobile testing, providing access to real devices and browsers.
- Sauce Labs: Offers cloud-based automated testing for web and mobile applications across multiple devices and operating systems.
- Lambdatest: A scalable platform for browser and app testing with real-time debugging capabilities.
- BlazeMeter: Designed for performance testing, BlazeMeter allows users to test APIs and applications at scale.
- AWS Device Farm: A cloud service that allows testing of mobile and web applications on a wide range of devices.
- Microsoft Azure DevTest Labs: Provides pre-configured testing environments to reduce setup time and enable testing on-demand.
- Google Cloud Test Lab (Firebase Test Lab): A platform for testing Android and iOS apps on real devices hosted by Google.
Best Practices for Cloud-Based Testing
- Define Clear Objectives: Identify the specific testing goals, such as functionality, performance, or compatibility testing, before starting.
- Secure Test Data: Use encryption and anonymization techniques to safeguard sensitive data during testing.
- Optimize Resource Usage: Monitor resource consumption and scale environments appropriately to optimize costs.
- Leverage Automation: Automate repetitive tests to improve efficiency and reduce manual effort.
- Integrate with DevOps: Incorporate cloud testing into CI/CD pipelines to enable continuous testing and faster releases.
- Evaluate Platform Capabilities: Regularly assess the features and limitations of the chosen cloud testing platform to ensure alignment with project needs.
Conclusion
Cloud-based testing platforms are transforming the way applications are tested, offering flexibility, scalability, and cost-efficiency. By leveraging these platforms, organizations can enhance their testing processes, improve collaboration, and deliver high-quality software at a faster pace. Choosing the right platform and adopting best practices are critical for maximizing the benefits of cloud-based testing.
IoT Testing: Strategies and Challenges
Introduction to IoT Testing
Internet of Things (IoT) testing involves verifying the functionality, performance, security, and reliability of IoT devices and their connected systems. As IoT ecosystems include a combination of hardware, software, sensors, and network communication, testing these systems requires specialized strategies to ensure seamless operation.
Key Objectives of IoT Testing
- Functionality: Ensuring that the IoT device and its associated applications perform as expected.
- Interoperability: Testing the compatibility of IoT devices with various platforms, protocols, and devices.
- Security: Identifying and mitigating vulnerabilities to protect sensitive data and prevent unauthorized access.
- Performance: Verifying that the system can handle real-time data processing under various conditions.
- Reliability: Ensuring consistent performance in diverse environments and usage scenarios.
Strategies for IoT Testing
- Device Testing: Test the hardware components, sensors, and embedded software of IoT devices to verify their functionality and durability.
- Protocol Testing: Ensure that the IoT devices communicate effectively using standard protocols like MQTT, HTTP, CoAP, or Bluetooth.
- Network Testing: Validate the performance of IoT systems under different network conditions, such as latency, bandwidth limitations, and connectivity interruptions.
- Security Testing: Perform penetration testing, data encryption validation, and authentication testing to identify vulnerabilities in the IoT ecosystem.
- Performance and Scalability Testing: Assess the system's ability to handle multiple devices, high data loads, and concurrent connections.
- Interoperability Testing: Verify that IoT devices operate seamlessly with various hardware, software, and third-party integrations.
- Usability Testing: Evaluate the user experience of IoT applications to ensure they are intuitive and easy to use.
- End-to-End Testing: Test the entire IoT ecosystem, including devices, gateways, cloud services, and applications, to ensure seamless integration and operation.
Challenges in IoT Testing
- Complex Ecosystems: Testing IoT systems involves multiple components, including hardware, software, networks, and cloud platforms, making it challenging to ensure complete coverage.
- Diverse Protocols: The use of various communication protocols requires testers to have expertise in handling protocol-specific testing.
- Security Concerns: IoT devices are often vulnerable to cyberattacks, requiring rigorous security testing to address potential risks.
- Scalability Issues: Testing the system's ability to handle a large number of devices and data streams is complex and resource-intensive.
- Real-World Environment Simulation: Replicating real-world conditions, such as network disruptions and environmental factors, is challenging but necessary for reliable testing.
- Hardware Constraints: Limited processing power, memory, and storage in IoT devices can restrict the scope of testing.
- Rapid Technology Evolution: The fast-paced advancements in IoT technologies require testers to constantly update their knowledge and tools.
Best Practices for IoT Testing
- Early Testing: Begin testing during the development phase to identify and address issues early.
- Automated Testing: Use automation tools for repetitive and large-scale testing tasks to save time and improve accuracy.
- Simulators and Emulators: Leverage simulators and emulators to create real-world scenarios and test devices in controlled environments.
- Collaboration: Work closely with developers, network engineers, and security teams to ensure comprehensive testing coverage.
- Focus on Security: Prioritize robust security testing to safeguard IoT systems from cyber threats.
- Continuous Monitoring: Implement continuous testing and monitoring to identify and resolve issues in production environments.
Conclusion
IoT testing is a critical aspect of ensuring the reliability, security, and performance of interconnected devices and systems. By adopting effective testing strategies and addressing the unique challenges of IoT ecosystems, organizations can deliver high-quality IoT solutions that meet user expectations and industry standards.
RPA (Robotic Process Automation) in Testing
Introduction to RPA in Testing
Robotic Process Automation (RPA) is the use of software robots or "bots" to automate repetitive, rule-based tasks. In the context of testing, RPA enhances efficiency by automating tasks such as data entry, test execution, and report generation. Unlike traditional test automation, which focuses on functional and regression testing, RPA can automate end-to-end processes across multiple systems without the need for deep integration.
Key Benefits of RPA in Testing
- Increased Efficiency: RPA bots can execute repetitive tasks faster and with fewer errors compared to manual efforts.
- Cost-Effectiveness: Reduces the need for manual intervention, leading to significant cost savings in the long term.
- Improved Accuracy: Eliminates human errors in processes such as data validation and test result comparison.
- Cross-System Integration: RPA can work across different applications and systems without requiring APIs or complex integrations.
- 24/7 Operations: Bots can run continuously, enabling round-the-clock testing and monitoring.
- Scalability: Organizations can scale RPA operations by adding more bots as needed.
Applications of RPA in Testing
- Regression Testing: Automate repetitive regression test cases to validate new builds and updates.
- Data-Driven Testing: Use RPA to populate test data in applications and verify results.
- API Testing: Automate API calls and validate responses for consistency and accuracy.
- GUI Testing: Simulate user interactions with web and desktop applications to test the UI and workflows.
- Test Data Management: Automate the creation, cleanup, and manipulation of test data across environments.
- Monitoring and Reporting: Generate test execution reports and monitor application performance in real-time.
Challenges of Using RPA in Testing
- Complex Scenarios: RPA is best suited for rule-based tasks and may struggle with dynamic or highly complex scenarios.
- Initial Setup: Developing and configuring RPA bots require an upfront investment in time and resources.
- Maintenance: Bots need to be updated whenever application workflows or user interfaces change.
- Lack of Decision-Making: RPA bots cannot handle tasks that require judgment or decision-making without explicit instructions.
Popular RPA Tools for Testing
- UiPath: A widely used RPA tool with features for test automation, workflow automation, and analytics.
- Automation Anywhere: Offers robust capabilities for automating business processes and testing workflows.
- Blue Prism: Known for its scalable and secure RPA platform, suitable for enterprise-level automation.
- WorkFusion: Combines RPA with AI to handle both structured and unstructured data in testing scenarios.
- Microsoft Power Automate: Simplifies automation of repetitive tasks and integrates well with other Microsoft products.
Best Practices for Implementing RPA in Testing
- Identify Suitable Use Cases: Focus on repetitive, rule-based tasks that are time-consuming and prone to human error.
- Start Small: Begin with a pilot project to understand the potential benefits and challenges of RPA in your testing process.
- Collaboration: Work closely with testers, developers, and business stakeholders to design effective RPA workflows.
- Regular Maintenance: Continuously update and optimize bots to keep up with changes in applications and environments.
- Monitor Performance: Track the performance of RPA bots and refine their workflows for better efficiency and accuracy.
Conclusion
RPA in testing is a game-changer for organizations aiming to improve efficiency and reduce manual effort. By automating repetitive tasks and enabling seamless integration across systems, RPA enhances the overall testing process. With the right tools, strategies, and best practices, organizations can maximize the benefits of RPA and deliver high-quality software faster.
ISTQB Foundation and Advanced Levels
Introduction to ISTQB Certification
The International Software Testing Qualifications Board (ISTQB) offers globally recognized certifications for software testing professionals. These certifications help validate a tester's knowledge and skills in software testing practices and methodologies. ISTQB is structured into different levels to cater to professionals at various stages of their careers.
ISTQB Foundation Level
The ISTQB Foundation Level certification is designed for individuals new to software testing or those looking to formalize their basic knowledge. It provides an understanding of fundamental testing concepts, techniques, and best practices.
Key Objectives
- Understand basic software testing principles and terminology.
- Learn the software testing process, including planning, designing, and execution.
- Familiarize with different testing techniques such as black-box and white-box testing.
- Comprehend the role of testing within the software development lifecycle.
- Gain knowledge of test management tools and defect reporting.
Who Should Take This Certification?
- Individuals starting their career in software testing.
- Developers looking to understand the testing process.
- Project managers aiming to strengthen their testing knowledge.
ISTQB Advanced Level
The ISTQB Advanced Level certification is aimed at experienced professionals who wish to deepen their knowledge and specialize in specific areas of testing. It offers advanced insights into testing practices and prepares individuals for leadership roles in testing.
Key Modules
- Test Manager: Focuses on test management, planning, monitoring, and control.
- Test Analyst: Covers advanced testing techniques, requirements analysis, and risk-based testing.
- Technical Test Analyst: Focuses on technical aspects such as white-box testing, performance testing, and security testing.
Key Objectives
- Develop expertise in advanced testing techniques and methodologies.
- Learn how to manage complex testing projects and teams effectively.
- Specialize in technical testing, test analysis, or test management.
- Master the use of testing tools for automation and performance testing.
- Understand risk management and test strategy development.
Who Should Take This Certification?
- Experienced testers looking to advance their careers.
- Test leads and managers.
- Technical testers aiming to specialize in specific domains.
Benefits of ISTQB Certification
- Global Recognition: ISTQB certifications are recognized worldwide, enhancing career opportunities.
- Skill Development: Improves knowledge of testing principles and methodologies.
- Career Growth: Helps testers specialize and move into leadership roles.
- Standardized Knowledge: Promotes a standardized understanding of testing practices.
- Networking Opportunities: Connect with a global community of certified professionals.
Preparing for ISTQB Certification
Here are some tips to prepare for the ISTQB Foundation and Advanced Level certifications:
- Review the official ISTQB syllabus for the chosen level and module.
- Use ISTQB-recommended study materials and sample papers.
- Enroll in training courses offered by accredited providers.
- Practice with mock exams to familiarize yourself with the question format.
- Join online forums and communities to discuss topics and share knowledge.
Conclusion
ISTQB certifications are a great way to validate your skills and knowledge in software testing. Whether you're a beginner or an experienced tester, these certifications can help you enhance your career and stay competitive in the software testing industry. By choosing the right level and preparing systematically, you can achieve this globally recognized credential and advance your professional journey.
Certified Software Tester (CSTE)
Introduction to CSTE Certification
The Certified Software Tester (CSTE) certification, offered by the Quality Assurance Institute (QAI), is a globally recognized credential for software testing professionals. It validates an individual’s understanding of fundamental testing principles, processes, and practices, demonstrating their competence in the field of software testing.
Objectives of CSTE Certification
- To establish a standard for assessing the competency of software testing professionals.
- To validate the knowledge, skills, and expertise required for effective software testing.
- To enhance the professional credibility of software testers.
- To promote a comprehensive understanding of the software testing process and best practices.
Eligibility Criteria
To apply for the CSTE certification, candidates must meet one of the following criteria:
- A 4-year degree from an accredited college or university, plus 2 years of experience in the software testing field.
- A 3-year degree from an accredited college or university, plus 3 years of experience in the software testing field.
- A 2-year degree from an accredited college or university, plus 4 years of experience in the software testing field.
- Six years of experience in the software testing field (if no degree is held).
Key Areas Covered in CSTE Certification
The CSTE certification exam focuses on various aspects of software testing, including:
- Software Testing Principles: Fundamental concepts and goals of testing.
- Test Planning and Design: Creating effective test plans and cases.
- Test Execution and Reporting: Performing tests and documenting results.
- Defect Management: Identifying, reporting, and managing software defects.
- Metrics and Measurement: Using metrics to evaluate testing processes and results.
- Tools and Techniques: Leveraging testing tools and methodologies.
Benefits of CSTE Certification
- Global Recognition: CSTE is recognized worldwide, enhancing career prospects.
- Skill Enhancement: Develops a deeper understanding of software testing practices.
- Professional Credibility: Establishes you as a certified expert in testing.
- Career Advancement: Opens doors to leadership roles and better opportunities.
- Networking Opportunities: Connects you with a global community of certified professionals.
Exam Structure
The CSTE certification exam is designed to assess a candidate’s knowledge and skills in software testing. It consists of:
- Multiple-choice questions to test theoretical knowledge.
- Scenario-based questions to evaluate practical application skills.
- Open-ended questions requiring detailed written responses.
Preparing for the CSTE Certification
Effective preparation is essential for success in the CSTE exam. Here are some tips:
- Review the official CSTE body of knowledge and study guide.
- Participate in training programs offered by QAI or accredited providers.
- Use sample papers and practice exams to familiarize yourself with the format.
- Join forums and communities to discuss topics with other aspirants.
- Focus on both theoretical knowledge and practical application.
Conclusion
The CSTE certification is an excellent way to validate your expertise in software testing and advance your career. It equips professionals with the knowledge and skills needed to excel in the field, while also providing global recognition and credibility. By preparing effectively and leveraging this certification, you can position yourself as a leader in the software testing domain.
Certified Test Automation Engineer (CTAE)
Introduction to CTAE Certification
The Certified Test Automation Engineer (CTAE) certification is a professional credential designed to validate the expertise of individuals in designing, implementing, and managing test automation frameworks. It equips software testers and automation engineers with advanced skills in automating testing processes, improving efficiency, and ensuring high-quality software delivery.
Objectives of CTAE Certification
- To validate the knowledge and skills required for successful test automation implementation.
- To enhance understanding of advanced automation techniques and tools.
- To establish industry standards for test automation engineering.
- To promote best practices in building and maintaining robust automation frameworks.
Eligibility Criteria
Candidates applying for CTAE certification typically need:
- Experience in software testing or development (2+ years recommended).
- Basic understanding of programming languages such as Java, Python, or JavaScript.
- Familiarity with manual testing processes and methodologies.
- Prior exposure to test automation tools like Selenium, Cypress, or Appium (preferred).
Key Areas Covered in CTAE Certification
The CTAE certification program focuses on various aspects of test automation, including:
- Automation Fundamentals: Introduction to test automation principles and advantages.
- Framework Design: Designing reusable and scalable automation frameworks.
- Automation Tools: Hands-on experience with tools like Selenium, Appium, and Cypress.
- Scripting Techniques: Writing efficient, maintainable automation scripts.
- CI/CD Integration: Automating tests in Continuous Integration/Continuous Delivery pipelines.
- Test Data Management: Creating and managing data for automated testing.
- Reporting and Metrics: Generating automation reports and analyzing results.
- Debugging and Maintenance: Identifying and fixing issues in test scripts.
Benefits of CTAE Certification
- Professional Recognition: Demonstrates expertise in test automation engineering.
- Career Advancement: Opens doors to senior roles and specialized positions.
- Skill Enhancement: Develops advanced automation and framework design skills.
- Industry Demand: Aligns with the growing need for skilled automation engineers.
- Efficiency Gains: Learn techniques to optimize test cycles and reduce manual effort.
Exam Structure
The CTAE certification exam is designed to assess both theoretical knowledge and practical application. It typically includes:
- Multiple-choice questions: Testing foundational knowledge of automation concepts.
- Practical scenarios: Evaluating the ability to design and implement automation solutions.
- Hands-on tasks: Requiring candidates to write scripts or debug existing automation code.
Preparation Tips for CTAE Certification
To prepare for the CTAE certification exam, candidates should:
- Review the official syllabus and study guide provided by the certification body.
- Gain hands-on experience with popular automation tools.
- Practice designing and implementing test automation frameworks.
- Participate in workshops or training sessions focused on test automation.
- Join forums or groups to exchange knowledge with other automation professionals.
Conclusion
The Certified Test Automation Engineer (CTAE) certification is an excellent way to showcase expertise in the rapidly growing field of test automation. By obtaining this certification, professionals can enhance their career prospects, contribute to efficient testing processes, and stay ahead in the competitive software industry.
Agile Testing Certifications (ICP-TST)
Introduction to ICP-TST
The ICAgile Certified Professional in Agile Testing (ICP-TST) is a globally recognized certification that focuses on equipping testers with the skills and mindset required to thrive in Agile environments. This certification emphasizes collaboration, continuous feedback, and quality-driven approaches aligned with Agile principles.
Objectives of ICP-TST Certification
- To introduce Agile testing principles and methodologies.
- To enable testers to collaborate effectively with Agile teams.
- To promote a shift-left testing mindset for early defect detection.
- To cultivate skills for exploratory, automated, and continuous testing.
Eligibility Criteria
The ICP-TST certification is suitable for:
- Manual testers, automation testers, and QA professionals transitioning to Agile projects.
- Team members involved in Agile software development and delivery.
- Individuals seeking to enhance their testing skills in Agile environments.
Key Areas Covered in ICP-TST Certification
The ICP-TST program offers in-depth learning on the following topics:
- Agile Fundamentals: Agile Manifesto, principles, and methodologies such as Scrum and Kanban.
- Testing in Agile: The role of testing in Agile development and delivery cycles.
- Collaboration and Communication: Working effectively with developers, product owners, and stakeholders.
- Exploratory Testing: Techniques for identifying defects through unscripted testing.
- Test Automation in Agile: Building and integrating automation into Agile workflows.
- Continuous Testing: Ensuring quality throughout the development lifecycle.
- Test Metrics: Measuring and improving testing effectiveness in Agile projects.
Benefits of ICP-TST Certification
- Global Recognition: Acknowledged worldwide as a mark of Agile testing expertise.
- Career Advancement: Opens opportunities for roles in Agile teams and organizations.
- Enhanced Skills: Provides practical knowledge of Agile testing methodologies and tools.
- Team Collaboration: Strengthens the ability to work cohesively with cross-functional teams.
- Adaptability: Prepares testers to thrive in dynamic, fast-paced Agile environments.
Exam Structure and Certification Process
The ICP-TST certification is typically awarded after completing a training course delivered by an ICAgile-accredited training provider. Key details include:
- Training Duration: 2–3 days of instructor-led sessions.
- Evaluation: Based on participation, group activities, and real-world case studies.
- Certification: No formal exam; certification is granted upon successful course completion.
Preparation Tips for ICP-TST
To make the most of the ICP-TST certification, candidates should:
- Familiarize themselves with Agile principles and practices.
- Gain hands-on experience with Agile testing approaches and tools.
- Participate actively in team discussions and collaborative exercises during training.
- Explore case studies and real-world Agile testing scenarios.
- Engage with Agile communities to exchange knowledge and best practices.
Conclusion
The ICAgile Certified Professional in Agile Testing (ICP-TST) certification is an excellent way to demonstrate proficiency in Agile testing methodologies. It empowers testers to contribute effectively to Agile projects, fosters a quality-first mindset, and ensures continuous improvement in software delivery.
Career Path of a Tester
Introduction
A career in software testing offers diverse opportunities for growth and specialization. Starting as a manual tester, individuals can advance to automation testing, specialize in niche areas, or take on leadership roles. The testing career path provides avenues for continuous learning and skill enhancement.
Entry-Level Roles
The journey of a tester often begins with foundational roles that focus on understanding testing processes and methodologies:
- Manual Tester: Focuses on executing test cases manually to identify bugs and ensure application functionality.
- QA Analyst: Works on analyzing requirements, creating test cases, and executing tests to validate software quality.
Mid-Level Roles
With experience and expertise, testers can transition into more specialized roles:
- Automation Tester: Develops and maintains automated test scripts using tools like Selenium, Cypress, or Appium.
- Performance Tester: Focuses on testing application performance under various load conditions using tools like JMeter or LoadRunner.
- API Tester: Validates APIs for functionality, security, and performance using tools like Postman or REST Assured.
Advanced Roles
Testers with significant experience can pursue leadership or technical specialization roles:
- Test Lead/Test Manager: Manages testing teams, plans testing activities, and ensures project quality goals are met.
- Quality Assurance Manager: Oversees the entire QA process, including strategy, tools, and team management.
- Test Architect: Designs testing strategies and frameworks, ensuring scalability and efficiency in testing processes.
Specialized Testing Roles
Testers can also branch into niche domains based on interest and industry demand:
- Security Tester: Focuses on identifying vulnerabilities and ensuring software security using tools like Burp Suite or ZAP.
- Mobile Tester: Specializes in testing mobile applications for functionality, usability, and compatibility across devices.
- Blockchain Tester: Tests blockchain-based applications for security, performance, and scalability.
- IoT Tester: Validates the functionality and performance of IoT devices and their integrations.
Leadership Roles
For those aspiring to take on managerial responsibilities, leadership roles offer opportunities to guide testing teams and influence organizational quality goals:
- QA Director: Defines the organization's quality strategy, aligns testing processes with business goals, and manages large testing teams.
- VP of Quality Assurance: Oversees quality initiatives across the organization, focusing on innovation and process excellence.
Non-Traditional Career Paths
Testers can also explore alternative career paths leveraging their testing expertise:
- DevOps Engineer: Integrates testing into CI/CD pipelines and ensures seamless deployments.
- Business Analyst: Uses domain knowledge and testing experience to bridge the gap between business and technical teams.
- Product Owner: Takes ownership of product quality and works closely with development and testing teams to deliver high-quality software.
Skills for Career Growth
To advance in a testing career, testers should focus on the following skills:
- Strong understanding of testing methodologies and frameworks.
- Proficiency in programming languages for test automation (e.g., Java, Python, JavaScript).
- Knowledge of tools and technologies for automation, performance, and security testing.
- Effective communication and collaboration skills.
- Continuous learning and adaptation to emerging trends like AI, IoT, and blockchain testing.
Conclusion
The career path of a tester is dynamic and offers numerous opportunities for growth and specialization. By developing technical expertise, adapting to new technologies, and embracing leadership roles, testers can build fulfilling and rewarding careers in the ever-evolving field of software testing.
Skills Required for a Software Tester
Introduction
Software testing is a critical component of the software development life cycle, and testers play a vital role in ensuring software quality. To excel in this role, software testers need a blend of technical, analytical, and interpersonal skills. Below is an overview of the essential skills required for a successful software tester.
Technical Skills
Proficiency in technical skills is crucial for identifying defects, automating tests, and working with testing tools and frameworks:
- Programming Knowledge: Understanding of programming languages like Java, Python, or JavaScript for writing automated test scripts.
- Test Automation Tools: Familiarity with tools like Selenium, Cypress, Appium, and JUnit for creating and managing automated tests.
- API Testing: Skills in testing APIs using tools like Postman, REST Assured, or SoapUI.
- Database Knowledge: Ability to write and execute SQL queries to validate backend data.
- Performance Testing Tools: Experience with tools like JMeter or LoadRunner to evaluate system performance under load.
- Version Control: Knowledge of version control systems like Git for managing test scripts and documentation.
Analytical and Logical Thinking
A software tester needs strong analytical and logical thinking abilities to understand complex systems and identify potential defects:
- Analyzing requirements to create effective test cases and scenarios.
- Breaking down complex systems into smaller components for detailed testing.
- Identifying edge cases and boundary conditions for thorough validation.
Attention to Detail
Meticulous attention to detail is essential for spotting even the smallest defects that could impact the software's functionality or user experience.
Communication Skills
Effective communication is critical for collaborating with developers, stakeholders, and team members:
- Documenting test cases, defect reports, and testing strategies clearly and concisely.
- Communicating issues and test results effectively to technical and non-technical audiences.
- Collaborating with cross-functional teams to ensure quality at every stage of development.
Problem-Solving Skills
Testers must have strong problem-solving skills to identify the root cause of defects and suggest potential solutions:
- Diagnosing issues by analyzing logs, system behavior, and test results.
- Collaborating with developers to resolve defects efficiently.
Domain Knowledge
Familiarity with the domain or industry the application serves (e.g., healthcare, finance, e-commerce) helps testers understand business requirements and user expectations better.
Adaptability and Continuous Learning
The field of software testing evolves rapidly, requiring testers to stay updated with the latest tools, technologies, and methodologies:
- Learning new tools and frameworks as required by project demands.
- Adapting to agile and DevOps workflows for faster releases.
Time Management
Testers often work under tight deadlines and need excellent time management skills to prioritize tasks and ensure timely delivery:
- Balancing manual and automated testing activities.
- Ensuring thorough testing within limited timeframes.
Teamwork and Collaboration
Software testing is a collaborative effort, and testers need to work closely with developers, product managers, and other stakeholders:
- Contributing to team discussions and planning sessions.
- Supporting team members in achieving project goals.
Conclusion
A successful software tester is a combination of a technical expert, an analytical thinker, and a collaborative team player. By developing these skills and staying updated with industry trends, testers can contribute significantly to software quality and build a rewarding career in the field.
Manual Testing vs. Automation Testing Career Opportunities
Introduction
The world of software testing offers various career paths for professionals, and one of the key decisions testers face is whether to pursue a career in manual testing or automation testing. Both fields provide distinct career opportunities, skill sets, and growth prospects. In this section, we will explore the career opportunities in both manual and automation testing, helping you understand the unique benefits of each and how to choose the right path based on your preferences and goals.
Manual Testing Career Opportunities
Manual testing involves testing software applications manually without the use of automation tools. Testers in this field focus on executing test cases, identifying defects, and ensuring the software works as expected from a user’s perspective. The career opportunities in manual testing often revolve around the following roles:
- Manual Tester: Entry-level positions where testers perform manual testing by executing predefined test cases, reporting defects, and verifying bug fixes.
- Test Lead: A senior role responsible for leading the manual testing team, creating test plans, and ensuring the successful execution of test cases.
- QA Analyst: Focuses on analyzing requirements, defining test cases, and verifying that the software meets quality standards.
- Test Manager: Manages the testing team, oversees the entire testing process, and ensures the quality of the product meets organizational standards.
Manual testing remains relevant in many industries, especially in situations where automation isn't feasible, such as with small projects, complex UIs, or exploratory testing. Manual testers are often needed for:
- Exploratory and ad-hoc testing
- Usability and user experience (UX) testing
- Testing in smaller teams or with limited resources
Automation Testing Career Opportunities
Automation testing involves using automated test scripts and tools to perform tests on software applications. This type of testing is essential for speeding up the testing process, especially for repetitive tasks like regression testing. Career opportunities in automation testing typically require knowledge of programming and specialized testing tools. Some key roles include:
- Automation Test Engineer: Develops automated test scripts using tools like Selenium, Cypress, or JUnit. They are responsible for automating test cases and maintaining automation frameworks.
- Test Automation Architect: Designs and builds the overall automation strategy and framework. Works closely with developers and other stakeholders to ensure that automated testing aligns with business needs.
- Automation Tester: Focuses on writing, executing, and maintaining automated tests to ensure software quality, often using scripting languages like Java, Python, or JavaScript.
- Senior Automation Engineer: A highly skilled professional responsible for leading the automation testing process, mentoring junior testers, and ensuring the success of automation initiatives in large projects.
Automation testing is in high demand due to the growing need for faster release cycles, particularly in agile and DevOps environments. Automation testers are often required for:
- Regression testing
- Load and performance testing
- Continuous integration and delivery (CI/CD) pipelines
- Enterprise-level software applications with complex functionality
Which Career Path is Right for You?
The choice between manual and automation testing depends on various factors, such as your interest in programming, career goals, and the type of projects you want to work on. Here are some key considerations:
- Manual Testing: Ideal for those who prefer to focus on testing from a user experience perspective, with an emphasis on exploratory and usability testing. Manual testing can also be a good starting point for beginners to understand the fundamentals of quality assurance.
- Automation Testing: Best suited for individuals who enjoy working with code, solving complex technical challenges, and automating repetitive tasks. Automation testers often have higher earning potential due to the demand for specialized skills and the rapid growth of testing automation in modern development processes.
Conclusion
Both manual and automation testing offer rewarding career opportunities. Manual testing provides a solid foundation in quality assurance and offers roles for those interested in user-focused testing. Automation testing, on the other hand, offers more technical challenges and career growth potential, especially in agile and DevOps environments. The key is to evaluate your interests, skillset, and long-term career goals to choose the path that aligns best with your aspirations.
Preparing for a Testing Interview
Introduction
Preparing for a software testing interview requires a combination of technical knowledge, practical skills, and the ability to demonstrate your problem-solving capabilities. A successful interview can set you on the path to a rewarding career in software testing, whether you are applying for a manual testing position, an automation testing role, or a specialized position like performance or security testing. In this section, we’ll guide you through the key steps to prepare effectively for a testing interview.
1. Understand the Fundamentals of Software Testing
Before heading into any testing interview, it’s crucial to have a solid understanding of the core concepts of software testing. These include:
- Software Development Life Cycle (SDLC): Be familiar with the various phases such as requirement gathering, design, development, testing, and deployment.
- Types of Testing: Understand different types of testing, including manual testing, automation testing, unit testing, integration testing, system testing, and user acceptance testing (UAT).
- Testing Techniques: Know the different testing techniques like black-box testing, white-box testing, boundary value analysis, and equivalence partitioning.
- Bug Reporting: Be able to explain how to identify, document, and track defects, and understand the defect life cycle.
2. Brush Up on Tools and Technologies
If the job requires expertise with specific testing tools, make sure you are familiar with them. Some common tools include:
- Manual Testing Tools: Tools like Jira, Bugzilla, and TestRail for bug tracking and test management.
- Automation Testing Tools: Brush up on tools such as Selenium, Cypress, or Appium if the position is focused on automation testing.
- Performance Testing Tools: If applicable, be prepared to discuss tools like JMeter or LoadRunner.
- API Testing Tools: For API testing roles, familiarize yourself with tools like Postman and REST Assured.
3. Prepare for Technical Questions
Expect to face technical questions that test your knowledge of the various types of testing and your problem-solving abilities. Some common questions include:
- How do you write test cases? Can you provide an example?
- What is the difference between functional and non-functional testing?
- What is regression testing, and when do you perform it?
- Explain the difference between a bug, defect, and failure.
- How do you prioritize which tests to automate in an automation testing project?
- Can you explain how to test an API? What are common methods for testing REST APIs?
4. Work on Practical Skills and Problem-Solving
In addition to theoretical knowledge, you may be asked to solve practical problems during the interview. This could involve:
- Writing test cases for a given scenario (e.g., testing a login page, shopping cart, or specific feature).
- Debugging code or identifying issues in a test script.
- Solving puzzles or logical problems that demonstrate your analytical thinking.
- Demonstrating your knowledge of automation tools by writing a simple script or explaining how to set up an automation framework.
5. Study Agile and DevOps Methodologies (If Applicable)
Many modern software development projects follow agile or DevOps methodologies. It’s essential to understand how testing fits into these processes. Expect questions related to:
- Agile Testing: How do you conduct testing in an agile environment? What are the challenges of testing in Scrum or Kanban?
- DevOps and CI/CD: How do you integrate testing into continuous integration/continuous delivery pipelines? What tools do you use for automated testing in CI/CD?
6. Behavioral Interview Questions
In addition to technical questions, most interviews will also include behavioral questions to assess your soft skills, such as teamwork, communication, and problem-solving abilities. Some common behavioral questions for testers include:
- Tell me about a time you found a critical bug. How did you handle it?
- Describe a situation where you had to meet tight deadlines. How did you manage your testing tasks?
- How do you deal with disagreements or conflicts within a testing team?
7. Mock Interviews and Practice
To feel more confident and well-prepared, try conducting mock interviews with a friend or mentor. Practice answering both technical and behavioral questions aloud. You can also take part in online mock interview platforms to simulate real interview scenarios.
8. Keep up with Industry Trends
Stay informed about the latest trends and technologies in software testing. Follow blogs, attend webinars, or take online courses to learn about emerging tools, frameworks, and methodologies. This will not only help you during the interview but also show your passion for continuous learning.
Conclusion
Preparing for a testing interview is about more than just knowing the answers to technical questions. It involves a thorough understanding of software testing principles, hands-on experience with tools, and the ability to demonstrate your problem-solving and communication skills. By following these tips and thoroughly preparing, you can increase your chances of landing the job and advancing your career as a software tester.
Creating a Strong Testing Portfolio
Introduction
A well-crafted testing portfolio is an essential tool for showcasing your skills, experience, and achievements as a software tester. It serves as a personal marketing tool that can set you apart from other candidates in job interviews and can be a valuable asset to demonstrate your expertise. A strong portfolio allows potential employers to see your hands-on abilities, your approach to problem-solving, and your knowledge of various testing tools and techniques.
1. What to Include in Your Testing Portfolio
Your testing portfolio should highlight your skills, experience, and successful projects. Here’s a breakdown of what should be included:
- Introduction and Bio: Start with a brief introduction that highlights your background, testing experience, and areas of expertise. Include your career objectives, certifications, and any relevant personal achievements.
- Skills and Tools: List the testing tools, frameworks, and technologies you are proficient in, such as Selenium, JMeter, Postman, Jenkins, etc. Make sure to mention your experience with manual and automation testing and any relevant programming or scripting languages.
- Certifications: Include any professional certifications you have earned, such as ISTQB, CSTE, or CTAL. These certifications validate your skills and commitment to professional development in the testing field.
- Test Projects: Showcase your hands-on experience by including detailed descriptions of the testing projects you have worked on. For each project, mention:
- The type of testing performed (manual, automation, performance, etc.)
- The tools and technologies used
- Challenges faced and how you overcame them
- The impact of your testing on the overall project
- Test Cases and Scripts: Include some of the test cases you’ve written, as well as any automation scripts you’ve developed. This demonstrates your ability to document tests, think critically, and create effective test strategies.
- Bug Reports and Defects Tracked: Share examples of how you’ve tracked and reported bugs, including the types of defects, the tools used for bug tracking (e.g., Jira, Bugzilla), and how your efforts contributed to the resolution of the issues.
2. Showcasing Your Technical Abilities
In addition to describing your work experience, you should highlight your technical abilities in your portfolio. Here are some strategies to effectively demonstrate your skills:
- Automated Testing Projects: Show your proficiency with automation tools such as Selenium, Appium, or Cypress by including sample automation scripts and explaining how they were used to test different applications.
- Performance Testing: Include examples of load or stress testing, using tools like JMeter or LoadRunner, to demonstrate how you analyze system performance under different conditions.
- API Testing: Share examples of API testing using tools like Postman or REST Assured, including test cases and scripts, highlighting your ability to test APIs in various scenarios.
- Continuous Integration (CI): If you have experience with CI/CD pipelines, include examples of how you’ve integrated automated tests into a CI/CD pipeline using Jenkins, GitHub Actions, or GitLab CI.
3. Organizing Your Portfolio
A well-structured portfolio is crucial for making a good impression. Here’s how you can organize your portfolio:
- Clean and Professional Design: Choose a clean, professional design for your portfolio. If possible, consider creating a personal website or GitHub page to showcase your work. Ensure the portfolio is easy to navigate and visually appealing.
- Project Sections: Organize your portfolio into sections based on the types of projects you’ve worked on (e.g., manual testing, automation, performance testing). This makes it easier for employers to find relevant examples.
- Consistent Formatting: Use consistent formatting throughout your portfolio to keep it organized. Provide a clear title, description, and screenshots (if applicable) for each project or test case.
- Details for Each Project: For each project, provide a brief overview that includes:
- The project’s objectives and scope
- The testing types involved (functional, non-functional, etc.)
- The tools and technologies used
- Challenges faced and how you resolved them
4. Keeping Your Portfolio Up-to-Date
A strong testing portfolio is a living document that should evolve as you gain new skills, work on more projects, and learn new tools. Make sure to update your portfolio regularly with:
- New projects or testing experiences
- Certifications or courses completed
- New tools or technologies learned
- Any new test cases, scripts, or automation frameworks you’ve developed
5. Additional Tips for Your Portfolio
- Include Testimonials or References: If possible, add testimonials from previous employers, colleagues, or clients to vouch for your skills and work ethic.
- Showcase Collaboration: Demonstrate your ability to work in a team, whether it’s with developers, other testers, or project managers. Mention any collaborative tools you’ve used, such as Jira or Confluence.
- Highlight Continuous Learning: Emphasize your commitment to learning by mentioning any online courses, webinars, or certifications you’ve taken to stay updated on the latest trends in software testing.
Conclusion
Building a strong testing portfolio is an essential step in advancing your career as a software tester. By showcasing your skills, knowledge, and practical experience, you’ll be able to demonstrate your expertise to potential employers and stand out in job interviews. A strong portfolio not only highlights your technical abilities but also reflects your passion for software testing and continuous learning. Keep it up-to-date, professional, and comprehensive to make the best impression possible.
Examples of Software Testing Failures
Introduction
Software testing failures can happen due to various reasons, such as incomplete test coverage, poor test case design, or lack of proper communication between development and testing teams. These failures can lead to undetected bugs, performance issues, or even system crashes, which can negatively impact the user experience and the business. In this section, we’ll explore some notable examples of software testing failures that had significant consequences.
1. The Therac-25 Radiation Overdose Incident
One of the most infamous software testing failures occurred in the 1980s with the Therac-25, a medical radiation therapy machine. The software, which controlled the radiation dose administered to patients, contained a race condition that allowed the machine to deliver excessive radiation doses in certain situations. This led to several patients receiving lethal overdoses of radiation. The failure occurred because the software wasn't thoroughly tested under all conditions, and there were no proper safety mechanisms in place to catch errors. The incident highlights the importance of rigorous testing, especially in life-critical systems.
2. The Knight Capital Group Trading Glitch
In 2012, Knight Capital Group, a financial services firm, experienced a major testing failure that resulted in a loss of $440 million within 45 minutes. The issue was caused by a bug in their trading software, which had not been fully tested after a new version was deployed. The bug caused the software to execute erroneous trades, leading to massive financial losses. The failure was attributed to insufficient regression testing and a lack of proper checks before deploying the new code in the live trading environment.
3. The Ariane 5 Rocket Launch Failure
In 1996, the European Space Agency’s Ariane 5 rocket exploded 37 seconds after liftoff, resulting in the loss of the rocket and its payload, valued at $370 million. The failure was traced back to a software error in the inertial guidance system, which was not properly tested for the new rocket’s specifications. The issue arose because the software was reused from the Ariane 4 rocket without sufficient testing in the new context. The Ariane 5 disaster serves as a stark reminder of the importance of thorough software testing when reusing code and integrating new systems.
4. The Healthcare.gov Website Launch
The launch of the U.S. government’s Healthcare.gov website in 2013 was marred by severe technical issues, including long loading times, crashes, and errors during user registration. The site had not undergone adequate load testing to handle the surge of visitors on launch day. Additionally, the testing failed to uncover key issues related to integration with other systems. The failure of Healthcare.gov’s testing process led to delays, a damaged public image, and the need for extensive fixes and remediation efforts in the months following the launch.
5. The Volkswagen Emissions Scandal
In 2015, Volkswagen was caught in a major scandal when it was revealed that the company had installed software in its cars to cheat emissions tests. The software was designed to detect when the car was undergoing an emissions test and adjust the engine performance to meet the required standards. When the cars were on the road, however, the software would allow emissions to exceed legal limits. The failure here was not in the testing process itself but in the ethical breach and the lack of proper validation and verification of the software. This incident demonstrates the critical importance of ethical testing and the repercussions of cutting corners.
6. The Apple Maps Disaster
When Apple launched its own mapping service in 2012, it quickly became infamous for providing inaccurate and incomplete maps, leading to a poor user experience. The software was not properly tested to ensure the quality of the maps, and there were significant problems with directions, landmarks, and missing locations. The failure was a result of inadequate testing, including a lack of real-world testing and failure to address issues in beta testing. Apple had to apologize publicly and encourage users to switch to other mapping services until the issues were resolved.
7. The Windows Vista Launch
Microsoft’s Windows Vista operating system, launched in 2007, was plagued with performance issues and compatibility problems, which led to a lackluster reception. Many users reported system slowdowns, driver incompatibility, and software crashes. One of the key reasons for the failure was insufficient testing of the system’s performance on real-world hardware. Additionally, hardware vendors had not been adequately involved in the testing process, leading to compatibility issues. While the OS was technically advanced, its failure to meet user expectations was a direct result of poor testing and planning.
8. The Boeing 737 MAX Crisis
The Boeing 737 MAX crisis, which involved two fatal crashes of the 737 MAX aircraft, was partly caused by a software failure in the aircraft's Maneuvering Characteristics Augmentation System (MCAS). The system was designed to prevent the plane from stalling, but due to a software flaw, it caused the plane to dive unexpectedly. The software was not adequately tested, and there were insufficient checks to ensure its safety. The disaster underlined the importance of thorough software testing, especially for safety-critical systems, and the need for robust validation and verification processes.
Lessons Learned from Software Testing Failures
These high-profile software testing failures highlight several key lessons:
- Comprehensive Testing is Crucial: Inadequate test coverage or skipping important testing phases can lead to catastrophic failures. It’s vital to include all aspects of testing: functional, non-functional, and integration testing.
- Real-World Scenarios Matter: Test environments should closely mimic real-world usage. For example, load testing should simulate actual user traffic, and compatibility testing should cover a wide range of devices, browsers, and operating systems.
- Regression Testing is Essential: When new features or code are added, regression testing helps identify any negative impact on existing functionality.
- Collaboration Between Teams: Close communication between developers, testers, and business stakeholders is essential to ensure that the software meets both functional and non-functional requirements.
- Testing Must be Ethical: Ethical considerations should always be a part of the testing process. Software that is intended to deceive or manipulate users can have severe consequences.
Conclusion
Software testing failures can have significant consequences, both financially and reputationally. By learning from past failures, testing teams can improve their processes, ensure better quality, and avoid making similar mistakes in the future. Thorough testing, clear communication, and a focus on real-world scenarios are all crucial in mitigating the risk of failure in software development.
Lessons Learned from Industry Scenarios
Introduction
In the software development and testing world, real-world industry scenarios provide valuable insights into what can go wrong during the development and testing processes. These scenarios highlight the importance of effective communication, thorough testing, and strong project management practices. By analyzing these lessons, organizations can avoid common pitfalls and ensure the successful delivery of high-quality software. This section explores key lessons learned from various industry scenarios and how they can shape better practices for the future.
1. The Importance of Comprehensive Testing
One of the most common lessons learned from industry scenarios is the importance of comprehensive testing. In several high-profile cases, such as the failure of the Healthcare.gov website and the Therac-25 radiation overdose incident, inadequate testing led to significant issues. Comprehensive testing should cover functional, non-functional, integration, and regression testing to ensure all aspects of the system work as expected under different conditions.
Takeaway: Ensure that testing efforts are not limited to just functional verification but also include performance, security, usability, and other critical factors.
2. Real-World Testing is Essential
Testing software in controlled environments can often miss issues that only arise under real-world conditions. For example, the Apple Maps disaster occurred because the mapping software was not thoroughly tested with real users and real-world data, leading to inaccurate directions and missing locations. Similarly, in the case of Knight Capital, the failure to test the trading software under live conditions caused a massive financial loss.
Takeaway: Simulate real-world usage and test with real data wherever possible to identify potential issues that may not appear in a controlled testing environment.
3. Regression Testing is a Must
In several industry failures, such as the Windows Vista launch, issues arose due to poorly executed regression testing. New software updates or features should always be tested to ensure they do not negatively affect existing functionality. This is particularly true when dealing with complex software systems with multiple dependencies.
Takeaway: Always conduct rigorous regression testing to ensure that new changes do not introduce bugs or break existing functionality.
4. The Need for Early and Continuous Testing
In agile and DevOps environments, continuous testing is emphasized as a key practice for detecting and resolving issues early in the development cycle. The Boeing 737 MAX crisis, for example, was partly due to a lack of thorough testing during the development stage of the flight control software. Issues could have been identified earlier if more rigorous and continuous testing had been in place.
Takeaway: Integrate testing early in the software development lifecycle and perform it continuously through automation to catch issues as soon as they arise.
5. Communication and Collaboration Between Teams
Effective communication between developers, testers, project managers, and other stakeholders is crucial to preventing software failures. Many failures, such as the Healthcare.gov website launch, were caused by poor collaboration and a lack of understanding of requirements between teams. Clear communication can help ensure that everyone is aligned on the goals and requirements of the project.
Takeaway: Foster a collaborative environment where all teams communicate effectively and work together to identify and solve potential issues early on.
6. Emphasizing Security in Testing
Security testing is often overlooked, as seen in the case of the Volkswagen emissions scandal. The software was intentionally designed to cheat emissions tests, highlighting the importance of ethical considerations during testing. Failure to conduct thorough security testing can expose software to vulnerabilities that may be exploited by malicious actors.
Takeaway: Incorporate security testing into your testing process, and always verify that software complies with ethical standards and regulations.
7. Risk-Based Testing Helps Prioritize Resources
In the case of the Ariane 5 rocket failure, poor risk assessment led to a catastrophic software bug that resulted in the loss of the rocket and payload. A better understanding of the risks involved and more targeted testing could have helped prevent the disaster. Risk-based testing helps prioritize the most critical areas of the software, ensuring that resources are focused on the most high-risk components.
Takeaway: Use risk-based testing to focus efforts on the most critical and high-risk areas of the software, minimizing the potential for catastrophic failures.
8. The Need for Ethical Testing Practices
Ethical considerations should always be at the forefront of software testing. The Volkswagen scandal exemplifies how neglecting ethical principles in testing can lead to severe consequences. In this case, the software was intentionally designed to cheat emissions tests, which was both illegal and unethical.
Takeaway: Ensure that testing practices adhere to ethical standards and do not compromise user safety, security, or trust.
9. Testing in Different Environments and Platforms
Compatibility testing, as seen in the Windows Vista launch, is essential to ensure that the software works across various environments, platforms, and devices. Windows Vista's issues arose in part because the operating system had not been tested adequately across all hardware configurations, leading to performance issues and crashes.
Takeaway: Test software on various hardware, operating systems, browsers, and devices to ensure compatibility and a seamless user experience.
10. Learning From Past Mistakes
Every software failure provides valuable lessons that can be used to improve future testing practices. By analyzing past mistakes, teams can identify weak points in their testing processes and make improvements. Continuous learning and adapting to new challenges are essential for growth.
Takeaway: Learn from previous failures and continuously improve testing practices to prevent similar mistakes in the future.
Conclusion
Industry scenarios provide valuable lessons that can guide future software testing efforts. By learning from past mistakes and continuously improving testing practices, teams can avoid common pitfalls and deliver high-quality software that meets user expectations. The key to success lies in comprehensive testing, effective communication, continuous integration, and ethical considerations. Software testing is a dynamic and evolving field, and the lessons learned from industry scenarios are essential for driving improvements and innovation in the software development lifecycle.
Successful Testing Practices in Large Projects
Introduction
Large-scale software projects often come with significant challenges, such as complex requirements, vast teams, and tight deadlines. Successful testing practices are crucial to ensuring that software is reliable, efficient, and meets both functional and non-functional requirements. In such projects, testing must be systematic, comprehensive, and adaptable to handle the scale and complexity of the development process. This section explores key testing practices that have proven successful in large projects, helping organizations deliver high-quality software while managing risks.
1. Early Involvement of Testing Teams
In large projects, it is crucial to involve the testing team early in the development process. Early involvement ensures that testers understand the requirements, design, and potential challenges. By participating in discussions and planning from the beginning, testers can provide valuable insights and contribute to the creation of test cases and test scenarios based on the project’s needs.
Takeaway: Bring testers on board as early as possible to create test plans, identify requirements, and ensure alignment with development goals.
2. Clear Requirements and Test Case Documentation
Large projects often involve multiple teams, which can lead to miscommunication or misunderstandings. To prevent this, it is essential to have clear, well-documented requirements and test cases. Detailed test case documentation ensures that all team members are aligned and testing is consistent across different modules. It also provides a reference for future testing phases, such as regression testing.
Takeaway: Create comprehensive, clear, and detailed test cases and maintain proper documentation for future reference and alignment among teams.
3. Automating Repetitive Tests
Automation is one of the key strategies for managing large projects effectively. Repetitive tests, such as regression tests, can be automated to save time and resources. Automation also helps in increasing test coverage, providing faster feedback, and ensuring that existing functionality is not broken as new features are added. Tools like Selenium, Appium, and JUnit can significantly enhance the efficiency and effectiveness of testing in large projects.
Takeaway: Implement test automation for repetitive tasks and regression testing to improve efficiency, reduce human error, and speed up the testing process.
4. Parallel Testing to Expedite Execution
In large projects, testing a system with multiple components and configurations can take a lot of time. Parallel testing is an effective strategy to shorten the testing cycle. By running multiple test cases simultaneously, you can test different modules or environments concurrently, speeding up the overall testing process without compromising quality.
Takeaway: Leverage parallel testing to execute multiple test cases concurrently and reduce the overall testing time in large projects.
5. Risk-Based Testing
Large projects often have tight deadlines and limited resources, making it essential to focus testing efforts on the areas with the highest risk. Risk-based testing prioritizes testing based on the criticality of features, likelihood of failure, and potential impact. By focusing on the most critical components, testing teams can ensure that the highest-priority issues are identified and addressed first.
Takeaway: Apply risk-based testing to prioritize high-risk areas and allocate resources efficiently, especially when working under time constraints.
6. Continuous Testing in the CI/CD Pipeline
In large projects, where software is constantly evolving, continuous testing ensures that every change is verified and integrated into the software seamlessly. Continuous integration (CI) and continuous delivery (CD) practices allow teams to test code continuously and automatically. By integrating testing into the CI/CD pipeline, you can ensure that code changes are verified in real-time, reducing the risk of defects in production.
Takeaway: Incorporate continuous testing within the CI/CD pipeline to ensure that code changes are tested as part of the development process, leading to early defect detection.
7. Scalable Test Environments
Large projects often require multiple test environments to simulate different configurations, operating systems, browsers, or devices. It is essential to design scalable and flexible test environments that can be quickly replicated and managed. Cloud-based testing platforms, such as BrowserStack and Sauce Labs, allow teams to run tests across a variety of environments without the need for maintaining physical hardware.
Takeaway: Implement scalable and flexible test environments to ensure that tests can be run on multiple configurations and platforms without manual intervention.
8. Collaboration Between Teams
Collaboration between development, testing, and operations teams is crucial for the success of large projects. In a large project, cross-functional communication can often become fragmented, leading to gaps in testing and missed issues. Regular meetings, shared tools, and clear communication channels help ensure that everyone is aligned and working towards the same objectives.
Takeaway: Foster strong collaboration and communication between all stakeholders to ensure smooth execution and early identification of issues.
9. Use of Test Metrics and Reporting
In large projects, tracking the progress and success of testing efforts is essential. Test metrics, such as test coverage, defect density, pass/fail ratio, and defect discovery rate, provide valuable insights into the effectiveness of testing. Regular reporting helps stakeholders understand the testing status and make informed decisions about the next steps.
Takeaway: Use test metrics and reporting to track the progress of testing, identify areas for improvement, and keep all stakeholders informed.
10. Post-Project Review and Continuous Improvement
After the completion of a large project, it is essential to conduct a post-project review to analyze what went well and what could be improved. This review helps identify bottlenecks in the testing process, challenges faced during execution, and areas where testing practices could be improved for future projects. Continuous improvement ensures that the testing process evolves and becomes more efficient over time.
Takeaway: Conduct post-project reviews to capture lessons learned and continuously improve the testing processes for future projects.
Conclusion
Testing in large projects requires a well-thought-out strategy to address complexity, scale, and time constraints. By following best practices such as early involvement of testers, clear documentation, automation, risk-based testing, and continuous testing, teams can overcome the challenges posed by large projects. Effective collaboration, scalable test environments, and the use of metrics ensure that testing remains efficient and comprehensive. Lastly, a focus on continuous improvement helps teams refine their testing processes for future projects, ultimately leading to the successful delivery of high-quality software.
Automating Login for an E-Commerce Site
Introduction
Automating the login functionality for an e-commerce site is a crucial step in ensuring that the site's authentication process works reliably across different environments and use cases. Login is one of the most frequently tested features in e-commerce platforms, as it directly impacts user experience and security. Automation of login testing can help verify the functionality and efficiency of the login process, ensuring a smooth customer journey and preventing downtime during critical periods, such as sales events.
Why Automate Login Testing?
Automating the login process in an e-commerce platform offers several benefits:
- Efficiency: Automated tests can run quickly and repeatedly, ensuring that login functionality is consistently verified without manual intervention.
- Consistency: Automation ensures that the login process is tested under the same conditions every time, eliminating human error.
- Scalability: With automated testing, you can test multiple login scenarios simultaneously, across different browsers and devices.
- Regression Testing: Automated login tests are essential for regression testing, ensuring that new updates or features do not break the authentication process.
Steps to Automate Login for an E-Commerce Site
1. Setting Up Test Environment
Before automating login, it is essential to set up a stable test environment. This includes:
- Access to a staging or test environment of the e-commerce site.
- Test accounts with valid and invalid credentials.
- Access to automation tools like Selenium, Cypress, or Playwright.
- A test strategy that defines the scope, such as testing valid login, invalid login, password reset, and session management.
2. Choosing the Right Automation Tool
There are several tools available for automating login tests. Some popular choices include:
- Selenium: A widely used tool that supports multiple browsers, allowing you to test login functionality across different environments.
- Cypress: A modern testing framework suitable for testing web applications, with fast execution and support for real-time debugging.
- Playwright: A newer tool that supports multi-browser testing and provides better handling of dynamic content, which is common in e-commerce sites.
3. Writing the Test Script
To automate the login process, you need to write test scripts to simulate user actions on the login page. Here’s an example using Selenium WebDriver with Python:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
# Set up the WebDriver
driver = webdriver.Chrome()
# Navigate to the e-commerce site
driver.get("https://www.example-ecommerce-site.com/login")
# Find the username and password fields
username_field = driver.find_element(By.ID, "username")
password_field = driver.find_element(By.ID, "password")
# Enter login credentials
username_field.send_keys("valid_username")
password_field.send_keys("valid_password")
# Submit the login form
password_field.send_keys(Keys.RETURN)
# Verify successful login
assert "Welcome" in driver.page_source
# Close the browser
driver.quit()
In this example, the script opens the login page, enters the username and password, and submits the form. After that, it verifies if the login was successful by checking if the "Welcome" message is present on the page.
4. Testing Different Scenarios
It is essential to test multiple login scenarios to ensure robustness:
- Valid Login: Ensure that the correct credentials allow the user to log in successfully.
- Invalid Login: Test with incorrect credentials to verify that the system displays an appropriate error message.
- Empty Fields: Test the behavior when the username or password fields are left empty.
- Password Reset: Test the password reset functionality by triggering a password reset request.
- Session Management: Test that users can log out and that the session expires correctly after a period of inactivity.
5. Handling Dynamic Elements
E-commerce sites often use dynamic content, such as CAPTCHA, pop-ups, or animations, which may interfere with automated tests. To handle these scenarios, consider:
- Explicit Waits: Use explicit waits in Selenium to wait for elements to load before interacting with them, which helps avoid timing issues.
- Handling CAPTCHAs: For testing purposes, you can disable CAPTCHA for the test environment or use CAPTCHA-solving services.
- Pop-up Handling: Use automation scripts to handle pop-ups by accepting or dismissing them as needed.
6. Continuous Integration and Reporting
To ensure the continuous functionality of the login system, integrate the login automation tests into a CI/CD pipeline. Tools like Jenkins, GitHub Actions, or GitLab CI can automatically run the tests whenever new code is pushed. After the tests run, generate detailed reports to track the results and identify any failures immediately.
Best Practices for Automating Login Testing
- Maintain Test Data: Ensure that test data, such as user credentials, are up-to-date and stored securely.
- Keep Tests Isolated: Each test should be independent, so failures can be isolated and fixed without affecting other tests.
- Simulate Real User Behavior: Automate the login process in a way that mimics real user interactions, such as typing the username and password instead of using direct field assignments.
- Use a Dedicated Test Environment: Always test on a staging environment that mirrors the production environment to avoid disrupting real users.
- Implement Robust Error Handling: Add error handling to manage failed test cases, capture screenshots, and log detailed information for debugging.
Conclusion
Automating the login functionality for an e-commerce site is essential for ensuring a seamless user experience and efficient testing. By using automated testing tools like Selenium, Cypress, or Playwright, you can run consistent and repeatable tests across various scenarios. The key is to maintain best practices such as handling dynamic elements, testing for various login scenarios, and incorporating the tests into a CI/CD pipeline for continuous integration. Automated login tests are a valuable asset in delivering high-quality e-commerce applications with minimal downtime and maximum reliability.
Testing a REST API for a Weather App
Introduction
A weather app typically relies on a REST API to fetch weather data for different locations. Testing a REST API for a weather app is crucial to ensure that the API responses are accurate, efficient, and consistent across various scenarios. As a tester, you need to verify that the API correctly handles requests, returns the expected data, and performs well under different conditions. The goal of API testing is to ensure the reliability, security, and performance of the weather data that the app provides to its users.
Key Aspects of Testing a REST API
- Correctness: Ensure that the API returns the correct weather data for a given location.
- Performance: Verify that the API responds quickly and can handle a large number of requests efficiently.
- Security: Test the API for vulnerabilities such as unauthorized access or data leaks.
- Edge Cases: Ensure that the API handles edge cases like invalid locations, incorrect parameters, or missing data gracefully.
- Consistency: Test that the API responses are consistent over time and across different versions of the API.
Steps to Test a REST API for a Weather App
1. Understand the API Endpoints
To properly test the weather app's API, you need to understand the different endpoints and their expected behavior. For instance:
- GET /weather: Fetches current weather data for a specific location (e.g., city name or latitude/longitude).
- GET /forecast: Fetches weather forecast data for a specific location.
- GET /locations: Provides a list of supported locations or cities for weather data.
2. Setting Up the Test Environment
Before starting the testing process, ensure the following:
- Access to the API: Make sure you have API keys, access tokens, or any other authentication credentials required to interact with the API.
- API Documentation: Review the API documentation to understand the request formats, parameters, and expected responses.
- Test Data: Prepare test data such as valid and invalid city names, coordinates, and API keys.
3. Writing API Test Cases
It’s important to create test cases that cover different aspects of the API. Here are some examples:
- Valid Request: Test the API with valid data (e.g., valid city name, valid API key) and verify the correctness of the response.
- Invalid Request: Test with invalid data (e.g., non-existent city, incorrect API key) and verify that the API returns appropriate error messages.
- Boundary Testing: Test with edge cases, such as empty parameters or extreme values for coordinates.
- Rate Limiting: Test the API’s behavior under high request volumes and check if it correctly applies rate limits.
- Missing Data: Test how the API handles situations where the weather data is missing or incomplete.
4. Using Postman for Testing
Postman is a widely used API testing tool that allows you to manually send requests to the API and inspect the responses. To test a weather app API using Postman:
- Open Postman and create a new request.
- Choose the appropriate HTTP method (e.g., GET) and enter the API endpoint URL (e.g.,
https://api.weatherapp.com/weather?city=London&apikey=YOUR_API_KEY
). - Add any necessary parameters (e.g., city name, API key) and click "Send."
- Inspect the response body to check if the weather data is returned correctly.
- Validate the HTTP status code (e.g., 200 for success, 401 for unauthorized).
5. Writing Automated API Tests with REST Assured
For automated testing, you can use REST Assured, a Java library for testing REST APIs. Here’s an example of how to write an automated test for the weather app's API:
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.junit.Assert;
import org.junit.Test;
public class WeatherApiTest {
@Test
public void testWeatherAPI() {
// Send GET request to the weather API
Response response = RestAssured.given()
.queryParam("city", "London")
.queryParam("apikey", "YOUR_API_KEY")
.get("https://api.weatherapp.com/weather");
// Validate the status code is 200 (OK)
Assert.assertEquals(200, response.getStatusCode());
// Validate the response body contains weather data
Assert.assertTrue(response.getBody().asString().contains("temperature"));
Assert.assertTrue(response.getBody().asString().contains("humidity"));
}
}
This simple test sends a GET request to the weather API, checks if the status code is 200, and verifies that the response contains temperature and humidity data.
6. Validating API Responses
It is essential to validate the API responses to ensure that the weather data is accurate and complete. Some key validations include:
- HTTP Status Codes: Ensure that the API returns the correct status codes for different scenarios (e.g., 200 for success, 400 for bad request).
- Response Body: Verify that the response body contains the expected fields, such as temperature, humidity, and weather conditions.
- Data Accuracy: Check that the weather data corresponds to the expected values for the given location.
- Response Time: Measure the response time to ensure the API performs within acceptable limits.
7. Handling Errors and Edge Cases
Testing how the API handles errors and edge cases is crucial:
- Invalid API Key: Ensure that the API returns an error (e.g., 401 Unauthorized) when an invalid API key is provided.
- Non-Existent City: Test the API with a city that does not exist and verify that it returns an appropriate error message.
- Empty Parameters: Test the API with missing required parameters and verify that it handles the missing data appropriately.
8. Performance Testing
Performance testing ensures that the weather API can handle a large number of concurrent requests and return weather data in a timely manner. Tools like JMeter or LoadRunner can be used to simulate a high volume of requests to the API and measure its response time, throughput, and stability under load.
Conclusion
Testing a REST API for a weather app involves ensuring that the API handles valid and invalid requests, provides accurate weather data, performs well under load, and handles edge cases appropriately. By using tools like Postman for manual testing and REST Assured for automated tests, you can efficiently test the functionality of the weather API. Additionally, validating the API responses and testing for performance, security, and error handling will ensure that the weather app provides a seamless user experience.
Load Testing for a Ticket Booking Website
Introduction
Load testing is a type of performance testing that focuses on testing how a website or web application performs under a heavy load of users. For a ticket booking website, load testing is crucial to ensure that the website can handle a large number of concurrent users, especially during peak times such as ticket sales for popular events. The goal is to ensure that the website remains responsive, stable, and functional despite high traffic volumes.
Why Load Testing is Important for a Ticket Booking Website
- Simulating High Traffic: Ticket booking websites often experience spikes in traffic during ticket releases for popular events. Load testing simulates this high traffic to ensure the website can handle it.
- Ensuring Performance: Load testing helps identify performance bottlenecks that could degrade user experience, such as slow page load times, server crashes, or unresponsiveness.
- Optimizing Resource Usage: Load testing helps determine if the website’s infrastructure is appropriately scaled and if resource utilization (CPU, memory, bandwidth) is optimized.
- Preparing for Scalability: Load testing ensures that the website can scale seamlessly to meet the demands of a growing user base or large traffic spikes.
Steps to Perform Load Testing for a Ticket Booking Website
1. Define Testing Objectives
Before starting load testing, it’s essential to define the objectives, such as:
- Determine the maximum number of concurrent users the website can handle.
- Measure the response time of key pages (e.g., homepage, ticket selection, checkout page).
- Identify potential performance bottlenecks.
- Test the website’s stability under expected peak traffic loads.
2. Identify Critical User Journeys
A ticket booking website typically has several key user journeys. Identifying these critical workflows will help you focus your load testing on the most important user actions. Some of the most common critical user journeys include:
- Homepage Loading: The page that loads when a user visits the website.
- Ticket Search: The process of searching for available tickets by event, date, or location.
- Ticket Selection: The process of selecting a ticket, choosing seat options, and adding it to the cart.
- Checkout Process: The process of entering payment details and completing the purchase.
- Confirmation Page: The page displaying the booking confirmation or error message if the purchase fails.
3. Choose the Right Load Testing Tool
To conduct load testing, you need to select a tool that can simulate multiple concurrent users and generate load on the website. Some popular load testing tools include:
- Apache JMeter: A powerful tool for simulating traffic and measuring response times for web applications.
- LoadRunner: A comprehensive load testing tool that supports a wide range of protocols and technologies.
- Gatling: A highly scalable open-source tool for load testing designed to simulate high numbers of users.
- BlazeMeter: A cloud-based platform for running load tests at scale, based on Apache JMeter.
4. Set Up the Load Test Plan
A well-defined load test plan includes:
- Test Scenarios: Define the specific user journeys to simulate (e.g., user loading the homepage, selecting a ticket, and completing the checkout process).
- Load Profiles: Create profiles for expected traffic patterns (e.g., normal load, peak load, stress load).
- Performance Metrics: Identify key performance metrics to track during the test, such as response times, error rates, throughput, and server resource utilization (CPU, memory, bandwidth).
- Success Criteria: Define what constitutes a successful test, such as response times under a certain threshold, no server crashes, and no critical errors.
5. Execute the Load Test
Once the test plan is in place, execute the load test by simulating multiple virtual users interacting with the website. The load testing tool will mimic the traffic pattern you defined in your test plan and simulate concurrent user actions on the website.
6. Monitor the Performance During the Test
While the test is running, monitor the performance of the website, including:
- Server Load: Monitor server CPU, memory, and disk usage to check for resource exhaustion.
- Response Time: Track how long it takes for the website to respond to user actions (e.g., loading the homepage, selecting a ticket).
- Throughput: Measure the number of transactions (e.g., ticket bookings) processed per unit of time.
- Errors: Look for any errors, such as timeouts, 500 internal server errors, or page failures.
7. Analyze the Results
After the load test is complete, analyze the results to identify performance issues:
- Response Time Analysis: Compare the actual response times against the defined success criteria. Long response times could indicate bottlenecks.
- Error Analysis: Review any errors that occurred during the test, such as 500 errors or failed requests, and investigate their causes.
- Resource Utilization: Check if the server infrastructure was sufficient to handle the load or if resource limits were reached, causing performance degradation.
8. Optimize Based on Results
Based on the results, identify areas for optimization:
- Database Optimization: Slow database queries can be a bottleneck. Consider optimizing database queries or implementing caching mechanisms.
- Server Scaling: If the server struggles to handle the load, consider scaling horizontally (adding more servers) or vertically (upgrading server resources).
- Code Optimization: If the response times are slow, review the backend code for inefficiencies or issues that can be improved.
- Content Delivery Network (CDN): Use a CDN to cache static resources like images and CSS files, improving page load times.
Conclusion
Load testing is essential for a ticket booking website to ensure that it can handle high traffic volumes during peak times without performance degradation or failure. By simulating real-world traffic patterns and monitoring key performance metrics, you can identify bottlenecks, optimize server resources, and ensure a smooth user experience even during high demand. Tools like JMeter, LoadRunner, and Gatling are essential for conducting robust load tests and ensuring your ticket booking website is ready for any traffic surge.
Automating Mobile App Testing Using Appium
Introduction
Mobile app testing is an essential part of ensuring a seamless user experience. With the increasing use of mobile apps across various platforms, it becomes crucial to automate mobile app testing to ensure that apps perform well and maintain quality. Appium is a widely used open-source tool for automating mobile applications across both Android and iOS platforms. It supports a wide range of programming languages such as Java, Python, Ruby, and JavaScript, making it versatile for different teams and projects.
Why Choose Appium for Mobile App Testing?
- Cross-Platform Testing: Appium supports both Android and iOS platforms, allowing you to write tests once and run them on both platforms, saving time and effort.
- Language Agnostic: Appium allows you to write tests in any programming language, such as Java, Python, Ruby, JavaScript, and more, making it flexible for teams with varying technical expertise.
- Native, Hybrid, and Web App Support: Appium can automate testing for native apps, hybrid apps, and mobile web apps, making it versatile for different types of mobile applications.
- Open Source: Appium is free to use and has a large community for support and continuous improvements.
- Real Device and Emulator/Simulator Support: Appium supports testing on real devices as well as emulators and simulators, which ensures that the app performs well across different environments.
Setting Up Appium for Mobile App Testing
To get started with Appium for mobile app testing, follow these steps:
1. Install Appium
To install Appium, you can use the Node Package Manager (NPM). Run the following command:
npm install -g appium
2. Install Dependencies
Depending on whether you're testing on Android or iOS, you need to install additional dependencies:
- Android: Install Android SDK and set up the Android environment variables (e.g., ANDROID_HOME).
- iOS: Install Xcode and set up the necessary iOS simulators and devices for testing.
3. Set Up Your Development Environment
Appium supports various programming languages like Java, Python, Ruby, and JavaScript. Here’s how to set up your development environment for Java (example):
- Install Java Development Kit (JDK).
- Set up a testing framework such as TestNG or JUnit for Java-based testing.
- Use Maven or Gradle for dependency management in your project.
Writing Your First Appium Test
Once you’ve set up your environment, you can start writing your first test. Here’s an example of how to write a basic Appium test in Java:
Example Test Code (Java)
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.MobileElement;
import io.appium.java_client.android.AndroidDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import java.net.URL;
public class AppiumTest {
private AppiumDriver driver;
@BeforeClass
public void setUp() throws Exception {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability("platformName", "Android");
caps.setCapability("deviceName", "emulator-5554");
caps.setCapability("app", "/path/to/your/app.apk");
// Initialize Appium driver
driver = new AndroidDriver<>(new URL("http://127.0.0.1:4723/wd/hub"), caps);
}
@Test
public void testApp() {
// Locate an element and perform an action
MobileElement element = driver.findElementById("com.example:id/button");
element.click();
}
@AfterClass
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
Running Appium Tests
To run your Appium tests, simply start the Appium server by running:
appiumAfter the server is running, execute your test script as you would with any other test automation framework (e.g., using Maven or a test runner like TestNG).
Appium Mobile Testing Strategies
- Parallel Testing: Use Appium to run tests in parallel on multiple devices or emulators to reduce testing time and cover more scenarios.
- Cross-Platform Testing: Write test scripts once and execute them on both Android and iOS devices to ensure consistent behavior across platforms.
- Real Device Testing: Conduct tests on real mobile devices to ensure that the app performs as expected in a real-world environment.
- Regression Testing: Automate regression tests to ensure that new updates or features do not break existing functionality.
Challenges in Mobile App Automation with Appium
- Device Fragmentation: Testing on multiple devices with different screen sizes, OS versions, and hardware specifications can be challenging.
- Appium Setup Complexity: Setting up Appium with the correct environment and dependencies can be complex for new users, especially when working with iOS devices.
- Performance Overhead: Running tests on real devices can have performance overhead, especially when testing resource-intensive apps.
Best Practices for Mobile App Testing with Appium
- Use Page Object Model: Organize your test code using the Page Object Model (POM) pattern to separate logic and UI elements, making tests more maintainable.
- Test on Real Devices: While simulators are useful, testing on real devices ensures that your app performs well in real-world conditions.
- Keep Tests Small and Focused: Break down your tests into smaller units to ensure that they are fast, reliable, and easy to debug.
- Use Appium Desktop: Appium Desktop provides an easy-to-use interface for inspecting mobile apps and elements, which helps in writing more accurate test scripts.
Conclusion
Appium is a powerful and versatile tool for automating mobile app testing. By supporting both Android and iOS, real devices, and emulators, Appium allows you to write tests once and run them on multiple platforms. With its support for multiple programming languages and testing frameworks, Appium fits into a wide variety of development and testing environments. By following best practices, using the right strategies, and addressing common challenges, you can effectively use Appium to automate and streamline your mobile app testing process.
Security Testing for a Web Application
Introduction
In today’s digital landscape, securing web applications is essential to protect sensitive data and maintain user trust. Security testing helps identify vulnerabilities and weaknesses in web applications before attackers can exploit them. Web applications are often targeted by hackers, making security a critical focus during the software development lifecycle. Security testing involves various techniques to ensure that a web application is resistant to common security threats such as SQL injection, cross-site scripting (XSS), and unauthorized access.
Importance of Security Testing for Web Applications
- Data Protection: Ensures that sensitive user and business data are protected from unauthorized access, theft, or modification.
- Regulatory Compliance: Helps web applications meet industry-specific regulations such as GDPR, HIPAA, and PCI DSS, which require robust security measures.
- Prevention of Cyberattacks: Helps identify and fix vulnerabilities that could be exploited by malicious hackers for cyberattacks.
- Maintaining Reputation: Securing your web application helps maintain customer trust and prevents reputational damage caused by data breaches or security incidents.
Common Security Threats in Web Applications
- SQL Injection: Attackers inject malicious SQL code into input fields to manipulate the backend database.
- Cross-Site Scripting (XSS): Malicious scripts are injected into web pages viewed by other users, leading to data theft or session hijacking.
- Cross-Site Request Forgery (CSRF): Attackers trick a user into unknowingly executing actions on a web application without their consent.
- Session Hijacking: Attackers steal or guess session tokens to impersonate a legitimate user.
- Insecure Direct Object References (IDOR): Attackers exploit references to internal objects, such as files or database records, to gain unauthorized access.
- Broken Authentication: Weak authentication mechanisms allow attackers to bypass login systems and gain unauthorized access to web applications.
- Sensitive Data Exposure: Improper handling of sensitive data (e.g., passwords, credit card numbers) can lead to leaks or breaches.
Techniques for Security Testing of Web Applications
1. Static Application Security Testing (SAST)
SAST is a white-box testing technique that analyzes the source code, binaries, or bytecode of the application to find vulnerabilities before execution. It helps identify coding flaws and security weaknesses early in the development process. Tools such as Checkmarx and Fortify are commonly used for SAST.
2. Dynamic Application Security Testing (DAST)
DAST is a black-box testing technique that tests web applications in their running state. It simulates real-world attacks by interacting with the application through the user interface (UI). DAST tools like OWASP ZAP and Burp Suite help detect vulnerabilities such as XSS, CSRF, and SQL injection.
3. Penetration Testing
Penetration testing (pen testing) involves ethical hackers attempting to exploit vulnerabilities in the web application. This simulated attack identifies weaknesses that could be exploited in a real attack scenario. Pen testers use a combination of manual testing and automated tools to assess the application’s security posture.
4. Security Code Review
A security code review is a manual process where developers or security experts examine the application’s source code to identify security flaws. This technique focuses on finding issues such as hardcoded credentials, improper input validation, and insecure data handling.
5. Vulnerability Scanning
Vulnerability scanning tools are used to automate the identification of known vulnerabilities in a web application. These tools compare the web application against a database of known security issues and provide a report on potential risks. Popular tools include Qualys and Acunetix.
6. Security Audits
Security audits involve a comprehensive review of the web application’s architecture, design, and implementation. Auditors assess compliance with security best practices, identify potential threats, and recommend security improvements. Regular security audits help ensure ongoing security for the web application.
Tools for Security Testing
1. OWASP ZAP (Zed Attack Proxy)
OWASP ZAP is a free, open-source security testing tool used for finding security vulnerabilities in web applications. It is highly customizable and provides automated scanners and various tools for manual testing. ZAP is ideal for DAST and is widely used in the security testing community.
2. Burp Suite
Burp Suite is an integrated platform for performing security testing of web applications. It provides tools for crawling, scanning, and testing web applications for vulnerabilities. The free version offers essential features, while the professional version includes advanced scanning tools for more in-depth testing.
3. Nikto
Nikto is an open-source web server scanner that identifies vulnerabilities such as outdated software versions, configuration issues, and potential security risks. It is a useful tool for assessing web servers and applications for common security flaws.
4. Acunetix
Acunetix is a web vulnerability scanner that identifies and reports common security issues such as XSS, SQL injection, and more. It offers automated scanning and vulnerability management to help ensure that your web application is secure.
Best Practices for Security Testing in Web Applications
- Implement Secure Coding Standards: Ensure that developers follow secure coding best practices to prevent vulnerabilities such as SQL injection and XSS during the development process.
- Use Strong Authentication Mechanisms: Implement multi-factor authentication (MFA), strong password policies, and secure session management to protect user accounts.
- Regularly Update Software: Keep all software, libraries, and plugins up to date to avoid security vulnerabilities from outdated versions.
- Perform Regular Security Testing: Conduct regular security testing using a combination of SAST, DAST, and penetration testing to identify new vulnerabilities as the application evolves.
- Encrypt Sensitive Data: Use strong encryption techniques to protect sensitive data such as passwords, payment details, and personal information both in transit and at rest.
- Monitor and Respond to Security Incidents: Set up logging and monitoring systems to detect suspicious activities and respond to security incidents promptly.
Challenges in Security Testing
- Complexity of Modern Web Applications: The complexity of modern web applications, including third-party integrations and microservices, makes it challenging to identify and address all security vulnerabilities.
- Limited Resources: Security testing often requires specialized skills and tools, which may not be available in all organizations, leading to insufficient testing coverage.
- Evolving Threat Landscape: Attackers continuously evolve their tactics, making it challenging to stay ahead of new threats and vulnerabilities.
Conclusion
Security testing is an essential aspect of web application development. Identifying and mitigating vulnerabilities helps protect sensitive data, ensures compliance with regulations, and prevents potential cyberattacks. By leveraging a combination of security testing techniques such as SAST, DAST, penetration testing, and vulnerability scanning, organizations can secure their web applications and deliver a safe user experience. Regularly performing security testing and staying up to date with the latest security trends will help ensure that web applications remain secure as threats evolve.